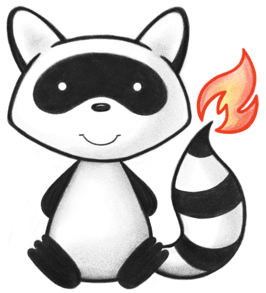
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.exceptions.FHIRFormatError; 040import org.hl7.fhir.instance.model.api.ICompositeType; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046/** 047 * Indicates how the medication is/was taken or should be taken by the patient. 048 */ 049@DatatypeDef(name="Dosage") 050public class Dosage extends Type implements ICompositeType { 051 052 /** 053 * Indicates the order in which the dosage instructions should be applied or interpreted. 054 */ 055 @Child(name = "sequence", type = {IntegerType.class}, order=0, min=0, max=1, modifier=false, summary=true) 056 @Description(shortDefinition="The order of the dosage instructions", formalDefinition="Indicates the order in which the dosage instructions should be applied or interpreted." ) 057 protected IntegerType sequence; 058 059 /** 060 * Free text dosage instructions e.g. SIG. 061 */ 062 @Child(name = "text", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 063 @Description(shortDefinition="Free text dosage instructions e.g. SIG", formalDefinition="Free text dosage instructions e.g. SIG." ) 064 protected StringType text; 065 066 /** 067 * Supplemental instruction - e.g. "with meals". 068 */ 069 @Child(name = "additionalInstruction", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 070 @Description(shortDefinition="Supplemental instruction - e.g. \"with meals\"", formalDefinition="Supplemental instruction - e.g. \"with meals\"." ) 071 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/additional-instruction-codes") 072 protected List<CodeableConcept> additionalInstruction; 073 074 /** 075 * Instructions in terms that are understood by the patient or consumer. 076 */ 077 @Child(name = "patientInstruction", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 078 @Description(shortDefinition="Patient or consumer oriented instructions", formalDefinition="Instructions in terms that are understood by the patient or consumer." ) 079 protected StringType patientInstruction; 080 081 /** 082 * When medication should be administered. 083 */ 084 @Child(name = "timing", type = {Timing.class}, order=4, min=0, max=1, modifier=false, summary=true) 085 @Description(shortDefinition="When medication should be administered", formalDefinition="When medication should be administered." ) 086 protected Timing timing; 087 088 /** 089 * Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept). 090 */ 091 @Child(name = "asNeeded", type = {BooleanType.class, CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 092 @Description(shortDefinition="Take \"as needed\" (for x)", formalDefinition="Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept)." ) 093 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-as-needed-reason") 094 protected Type asNeeded; 095 096 /** 097 * Body site to administer to. 098 */ 099 @Child(name = "site", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 100 @Description(shortDefinition="Body site to administer to", formalDefinition="Body site to administer to." ) 101 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/approach-site-codes") 102 protected CodeableConcept site; 103 104 /** 105 * How drug should enter body. 106 */ 107 @Child(name = "route", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 108 @Description(shortDefinition="How drug should enter body", formalDefinition="How drug should enter body." ) 109 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 110 protected CodeableConcept route; 111 112 /** 113 * Technique for administering medication. 114 */ 115 @Child(name = "method", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=true) 116 @Description(shortDefinition="Technique for administering medication", formalDefinition="Technique for administering medication." ) 117 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administration-method-codes") 118 protected CodeableConcept method; 119 120 /** 121 * Amount of medication per dose. 122 */ 123 @Child(name = "dose", type = {Range.class, SimpleQuantity.class}, order=9, min=0, max=1, modifier=false, summary=true) 124 @Description(shortDefinition="Amount of medication per dose", formalDefinition="Amount of medication per dose." ) 125 protected Type dose; 126 127 /** 128 * Upper limit on medication per unit of time. 129 */ 130 @Child(name = "maxDosePerPeriod", type = {Ratio.class}, order=10, min=0, max=1, modifier=false, summary=true) 131 @Description(shortDefinition="Upper limit on medication per unit of time", formalDefinition="Upper limit on medication per unit of time." ) 132 protected Ratio maxDosePerPeriod; 133 134 /** 135 * Upper limit on medication per administration. 136 */ 137 @Child(name = "maxDosePerAdministration", type = {SimpleQuantity.class}, order=11, min=0, max=1, modifier=false, summary=true) 138 @Description(shortDefinition="Upper limit on medication per administration", formalDefinition="Upper limit on medication per administration." ) 139 protected SimpleQuantity maxDosePerAdministration; 140 141 /** 142 * Upper limit on medication per lifetime of the patient. 143 */ 144 @Child(name = "maxDosePerLifetime", type = {SimpleQuantity.class}, order=12, min=0, max=1, modifier=false, summary=true) 145 @Description(shortDefinition="Upper limit on medication per lifetime of the patient", formalDefinition="Upper limit on medication per lifetime of the patient." ) 146 protected SimpleQuantity maxDosePerLifetime; 147 148 /** 149 * Amount of medication per unit of time. 150 */ 151 @Child(name = "rate", type = {Ratio.class, Range.class, SimpleQuantity.class}, order=13, min=0, max=1, modifier=false, summary=true) 152 @Description(shortDefinition="Amount of medication per unit of time", formalDefinition="Amount of medication per unit of time." ) 153 protected Type rate; 154 155 private static final long serialVersionUID = 70626458L; 156 157 /** 158 * Constructor 159 */ 160 public Dosage() { 161 super(); 162 } 163 164 /** 165 * @return {@link #sequence} (Indicates the order in which the dosage instructions should be applied or interpreted.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 166 */ 167 public IntegerType getSequenceElement() { 168 if (this.sequence == null) 169 if (Configuration.errorOnAutoCreate()) 170 throw new Error("Attempt to auto-create Dosage.sequence"); 171 else if (Configuration.doAutoCreate()) 172 this.sequence = new IntegerType(); // bb 173 return this.sequence; 174 } 175 176 public boolean hasSequenceElement() { 177 return this.sequence != null && !this.sequence.isEmpty(); 178 } 179 180 public boolean hasSequence() { 181 return this.sequence != null && !this.sequence.isEmpty(); 182 } 183 184 /** 185 * @param value {@link #sequence} (Indicates the order in which the dosage instructions should be applied or interpreted.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 186 */ 187 public Dosage setSequenceElement(IntegerType value) { 188 this.sequence = value; 189 return this; 190 } 191 192 /** 193 * @return Indicates the order in which the dosage instructions should be applied or interpreted. 194 */ 195 public int getSequence() { 196 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 197 } 198 199 /** 200 * @param value Indicates the order in which the dosage instructions should be applied or interpreted. 201 */ 202 public Dosage setSequence(int value) { 203 if (this.sequence == null) 204 this.sequence = new IntegerType(); 205 this.sequence.setValue(value); 206 return this; 207 } 208 209 /** 210 * @return {@link #text} (Free text dosage instructions e.g. SIG.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 211 */ 212 public StringType getTextElement() { 213 if (this.text == null) 214 if (Configuration.errorOnAutoCreate()) 215 throw new Error("Attempt to auto-create Dosage.text"); 216 else if (Configuration.doAutoCreate()) 217 this.text = new StringType(); // bb 218 return this.text; 219 } 220 221 public boolean hasTextElement() { 222 return this.text != null && !this.text.isEmpty(); 223 } 224 225 public boolean hasText() { 226 return this.text != null && !this.text.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #text} (Free text dosage instructions e.g. SIG.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 231 */ 232 public Dosage setTextElement(StringType value) { 233 this.text = value; 234 return this; 235 } 236 237 /** 238 * @return Free text dosage instructions e.g. SIG. 239 */ 240 public String getText() { 241 return this.text == null ? null : this.text.getValue(); 242 } 243 244 /** 245 * @param value Free text dosage instructions e.g. SIG. 246 */ 247 public Dosage setText(String value) { 248 if (Utilities.noString(value)) 249 this.text = null; 250 else { 251 if (this.text == null) 252 this.text = new StringType(); 253 this.text.setValue(value); 254 } 255 return this; 256 } 257 258 /** 259 * @return {@link #additionalInstruction} (Supplemental instruction - e.g. "with meals".) 260 */ 261 public List<CodeableConcept> getAdditionalInstruction() { 262 if (this.additionalInstruction == null) 263 this.additionalInstruction = new ArrayList<CodeableConcept>(); 264 return this.additionalInstruction; 265 } 266 267 /** 268 * @return Returns a reference to <code>this</code> for easy method chaining 269 */ 270 public Dosage setAdditionalInstruction(List<CodeableConcept> theAdditionalInstruction) { 271 this.additionalInstruction = theAdditionalInstruction; 272 return this; 273 } 274 275 public boolean hasAdditionalInstruction() { 276 if (this.additionalInstruction == null) 277 return false; 278 for (CodeableConcept item : this.additionalInstruction) 279 if (!item.isEmpty()) 280 return true; 281 return false; 282 } 283 284 public CodeableConcept addAdditionalInstruction() { //3 285 CodeableConcept t = new CodeableConcept(); 286 if (this.additionalInstruction == null) 287 this.additionalInstruction = new ArrayList<CodeableConcept>(); 288 this.additionalInstruction.add(t); 289 return t; 290 } 291 292 public Dosage addAdditionalInstruction(CodeableConcept t) { //3 293 if (t == null) 294 return this; 295 if (this.additionalInstruction == null) 296 this.additionalInstruction = new ArrayList<CodeableConcept>(); 297 this.additionalInstruction.add(t); 298 return this; 299 } 300 301 /** 302 * @return The first repetition of repeating field {@link #additionalInstruction}, creating it if it does not already exist 303 */ 304 public CodeableConcept getAdditionalInstructionFirstRep() { 305 if (getAdditionalInstruction().isEmpty()) { 306 addAdditionalInstruction(); 307 } 308 return getAdditionalInstruction().get(0); 309 } 310 311 /** 312 * @return {@link #patientInstruction} (Instructions in terms that are understood by the patient or consumer.). This is the underlying object with id, value and extensions. The accessor "getPatientInstruction" gives direct access to the value 313 */ 314 public StringType getPatientInstructionElement() { 315 if (this.patientInstruction == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create Dosage.patientInstruction"); 318 else if (Configuration.doAutoCreate()) 319 this.patientInstruction = new StringType(); // bb 320 return this.patientInstruction; 321 } 322 323 public boolean hasPatientInstructionElement() { 324 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 325 } 326 327 public boolean hasPatientInstruction() { 328 return this.patientInstruction != null && !this.patientInstruction.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #patientInstruction} (Instructions in terms that are understood by the patient or consumer.). This is the underlying object with id, value and extensions. The accessor "getPatientInstruction" gives direct access to the value 333 */ 334 public Dosage setPatientInstructionElement(StringType value) { 335 this.patientInstruction = value; 336 return this; 337 } 338 339 /** 340 * @return Instructions in terms that are understood by the patient or consumer. 341 */ 342 public String getPatientInstruction() { 343 return this.patientInstruction == null ? null : this.patientInstruction.getValue(); 344 } 345 346 /** 347 * @param value Instructions in terms that are understood by the patient or consumer. 348 */ 349 public Dosage setPatientInstruction(String value) { 350 if (Utilities.noString(value)) 351 this.patientInstruction = null; 352 else { 353 if (this.patientInstruction == null) 354 this.patientInstruction = new StringType(); 355 this.patientInstruction.setValue(value); 356 } 357 return this; 358 } 359 360 /** 361 * @return {@link #timing} (When medication should be administered.) 362 */ 363 public Timing getTiming() { 364 if (this.timing == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create Dosage.timing"); 367 else if (Configuration.doAutoCreate()) 368 this.timing = new Timing(); // cc 369 return this.timing; 370 } 371 372 public boolean hasTiming() { 373 return this.timing != null && !this.timing.isEmpty(); 374 } 375 376 /** 377 * @param value {@link #timing} (When medication should be administered.) 378 */ 379 public Dosage setTiming(Timing value) { 380 this.timing = value; 381 return this; 382 } 383 384 /** 385 * @return {@link #asNeeded} (Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).) 386 */ 387 public Type getAsNeeded() { 388 return this.asNeeded; 389 } 390 391 /** 392 * @return {@link #asNeeded} (Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).) 393 */ 394 public BooleanType getAsNeededBooleanType() throws FHIRException { 395 if (this.asNeeded == null) 396 return null; 397 if (!(this.asNeeded instanceof BooleanType)) 398 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 399 return (BooleanType) this.asNeeded; 400 } 401 402 public boolean hasAsNeededBooleanType() { 403 return this != null && this.asNeeded instanceof BooleanType; 404 } 405 406 /** 407 * @return {@link #asNeeded} (Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).) 408 */ 409 public CodeableConcept getAsNeededCodeableConcept() throws FHIRException { 410 if (this.asNeeded == null) 411 return null; 412 if (!(this.asNeeded instanceof CodeableConcept)) 413 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.asNeeded.getClass().getName()+" was encountered"); 414 return (CodeableConcept) this.asNeeded; 415 } 416 417 public boolean hasAsNeededCodeableConcept() { 418 return this != null && this.asNeeded instanceof CodeableConcept; 419 } 420 421 public boolean hasAsNeeded() { 422 return this.asNeeded != null && !this.asNeeded.isEmpty(); 423 } 424 425 /** 426 * @param value {@link #asNeeded} (Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).) 427 */ 428 public Dosage setAsNeeded(Type value) throws FHIRFormatError { 429 if (value != null && !(value instanceof BooleanType || value instanceof CodeableConcept)) 430 throw new FHIRFormatError("Not the right type for Dosage.asNeeded[x]: "+value.fhirType()); 431 this.asNeeded = value; 432 return this; 433 } 434 435 /** 436 * @return {@link #site} (Body site to administer to.) 437 */ 438 public CodeableConcept getSite() { 439 if (this.site == null) 440 if (Configuration.errorOnAutoCreate()) 441 throw new Error("Attempt to auto-create Dosage.site"); 442 else if (Configuration.doAutoCreate()) 443 this.site = new CodeableConcept(); // cc 444 return this.site; 445 } 446 447 public boolean hasSite() { 448 return this.site != null && !this.site.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #site} (Body site to administer to.) 453 */ 454 public Dosage setSite(CodeableConcept value) { 455 this.site = value; 456 return this; 457 } 458 459 /** 460 * @return {@link #route} (How drug should enter body.) 461 */ 462 public CodeableConcept getRoute() { 463 if (this.route == null) 464 if (Configuration.errorOnAutoCreate()) 465 throw new Error("Attempt to auto-create Dosage.route"); 466 else if (Configuration.doAutoCreate()) 467 this.route = new CodeableConcept(); // cc 468 return this.route; 469 } 470 471 public boolean hasRoute() { 472 return this.route != null && !this.route.isEmpty(); 473 } 474 475 /** 476 * @param value {@link #route} (How drug should enter body.) 477 */ 478 public Dosage setRoute(CodeableConcept value) { 479 this.route = value; 480 return this; 481 } 482 483 /** 484 * @return {@link #method} (Technique for administering medication.) 485 */ 486 public CodeableConcept getMethod() { 487 if (this.method == null) 488 if (Configuration.errorOnAutoCreate()) 489 throw new Error("Attempt to auto-create Dosage.method"); 490 else if (Configuration.doAutoCreate()) 491 this.method = new CodeableConcept(); // cc 492 return this.method; 493 } 494 495 public boolean hasMethod() { 496 return this.method != null && !this.method.isEmpty(); 497 } 498 499 /** 500 * @param value {@link #method} (Technique for administering medication.) 501 */ 502 public Dosage setMethod(CodeableConcept value) { 503 this.method = value; 504 return this; 505 } 506 507 /** 508 * @return {@link #dose} (Amount of medication per dose.) 509 */ 510 public Type getDose() { 511 return this.dose; 512 } 513 514 /** 515 * @return {@link #dose} (Amount of medication per dose.) 516 */ 517 public Range getDoseRange() throws FHIRException { 518 if (this.dose == null) 519 return null; 520 if (!(this.dose instanceof Range)) 521 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.dose.getClass().getName()+" was encountered"); 522 return (Range) this.dose; 523 } 524 525 public boolean hasDoseRange() { 526 return this != null && this.dose instanceof Range; 527 } 528 529 /** 530 * @return {@link #dose} (Amount of medication per dose.) 531 */ 532 public SimpleQuantity getDoseSimpleQuantity() throws FHIRException { 533 if (this.dose == null) 534 return null; 535 if (!(this.dose instanceof SimpleQuantity)) 536 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but "+this.dose.getClass().getName()+" was encountered"); 537 return (SimpleQuantity) this.dose; 538 } 539 540 public boolean hasDoseSimpleQuantity() { 541 return this != null && this.dose instanceof SimpleQuantity; 542 } 543 544 public boolean hasDose() { 545 return this.dose != null && !this.dose.isEmpty(); 546 } 547 548 /** 549 * @param value {@link #dose} (Amount of medication per dose.) 550 */ 551 public Dosage setDose(Type value) throws FHIRFormatError { 552 if (value != null && !(value instanceof Range || value instanceof Quantity)) 553 throw new FHIRFormatError("Not the right type for Dosage.dose[x]: "+value.fhirType()); 554 this.dose = value; 555 return this; 556 } 557 558 /** 559 * @return {@link #maxDosePerPeriod} (Upper limit on medication per unit of time.) 560 */ 561 public Ratio getMaxDosePerPeriod() { 562 if (this.maxDosePerPeriod == null) 563 if (Configuration.errorOnAutoCreate()) 564 throw new Error("Attempt to auto-create Dosage.maxDosePerPeriod"); 565 else if (Configuration.doAutoCreate()) 566 this.maxDosePerPeriod = new Ratio(); // cc 567 return this.maxDosePerPeriod; 568 } 569 570 public boolean hasMaxDosePerPeriod() { 571 return this.maxDosePerPeriod != null && !this.maxDosePerPeriod.isEmpty(); 572 } 573 574 /** 575 * @param value {@link #maxDosePerPeriod} (Upper limit on medication per unit of time.) 576 */ 577 public Dosage setMaxDosePerPeriod(Ratio value) { 578 this.maxDosePerPeriod = value; 579 return this; 580 } 581 582 /** 583 * @return {@link #maxDosePerAdministration} (Upper limit on medication per administration.) 584 */ 585 public SimpleQuantity getMaxDosePerAdministration() { 586 if (this.maxDosePerAdministration == null) 587 if (Configuration.errorOnAutoCreate()) 588 throw new Error("Attempt to auto-create Dosage.maxDosePerAdministration"); 589 else if (Configuration.doAutoCreate()) 590 this.maxDosePerAdministration = new SimpleQuantity(); // cc 591 return this.maxDosePerAdministration; 592 } 593 594 public boolean hasMaxDosePerAdministration() { 595 return this.maxDosePerAdministration != null && !this.maxDosePerAdministration.isEmpty(); 596 } 597 598 /** 599 * @param value {@link #maxDosePerAdministration} (Upper limit on medication per administration.) 600 */ 601 public Dosage setMaxDosePerAdministration(SimpleQuantity value) { 602 this.maxDosePerAdministration = value; 603 return this; 604 } 605 606 /** 607 * @return {@link #maxDosePerLifetime} (Upper limit on medication per lifetime of the patient.) 608 */ 609 public SimpleQuantity getMaxDosePerLifetime() { 610 if (this.maxDosePerLifetime == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create Dosage.maxDosePerLifetime"); 613 else if (Configuration.doAutoCreate()) 614 this.maxDosePerLifetime = new SimpleQuantity(); // cc 615 return this.maxDosePerLifetime; 616 } 617 618 public boolean hasMaxDosePerLifetime() { 619 return this.maxDosePerLifetime != null && !this.maxDosePerLifetime.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #maxDosePerLifetime} (Upper limit on medication per lifetime of the patient.) 624 */ 625 public Dosage setMaxDosePerLifetime(SimpleQuantity value) { 626 this.maxDosePerLifetime = value; 627 return this; 628 } 629 630 /** 631 * @return {@link #rate} (Amount of medication per unit of time.) 632 */ 633 public Type getRate() { 634 return this.rate; 635 } 636 637 /** 638 * @return {@link #rate} (Amount of medication per unit of time.) 639 */ 640 public Ratio getRateRatio() throws FHIRException { 641 if (this.rate == null) 642 return null; 643 if (!(this.rate instanceof Ratio)) 644 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.rate.getClass().getName()+" was encountered"); 645 return (Ratio) this.rate; 646 } 647 648 public boolean hasRateRatio() { 649 return this != null && this.rate instanceof Ratio; 650 } 651 652 /** 653 * @return {@link #rate} (Amount of medication per unit of time.) 654 */ 655 public Range getRateRange() throws FHIRException { 656 if (this.rate == null) 657 return null; 658 if (!(this.rate instanceof Range)) 659 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.rate.getClass().getName()+" was encountered"); 660 return (Range) this.rate; 661 } 662 663 public boolean hasRateRange() { 664 return this != null && this.rate instanceof Range; 665 } 666 667 /** 668 * @return {@link #rate} (Amount of medication per unit of time.) 669 */ 670 public SimpleQuantity getRateSimpleQuantity() throws FHIRException { 671 if (this.rate == null) 672 return null; 673 if (!(this.rate instanceof SimpleQuantity)) 674 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but "+this.rate.getClass().getName()+" was encountered"); 675 return (SimpleQuantity) this.rate; 676 } 677 678 public boolean hasRateSimpleQuantity() { 679 return this != null && this.rate instanceof SimpleQuantity; 680 } 681 682 public boolean hasRate() { 683 return this.rate != null && !this.rate.isEmpty(); 684 } 685 686 /** 687 * @param value {@link #rate} (Amount of medication per unit of time.) 688 */ 689 public Dosage setRate(Type value) throws FHIRFormatError { 690 if (value != null && !(value instanceof Ratio || value instanceof Range || value instanceof Quantity)) 691 throw new FHIRFormatError("Not the right type for Dosage.rate[x]: "+value.fhirType()); 692 this.rate = value; 693 return this; 694 } 695 696 protected void listChildren(List<Property> children) { 697 super.listChildren(children); 698 children.add(new Property("sequence", "integer", "Indicates the order in which the dosage instructions should be applied or interpreted.", 0, 1, sequence)); 699 children.add(new Property("text", "string", "Free text dosage instructions e.g. SIG.", 0, 1, text)); 700 children.add(new Property("additionalInstruction", "CodeableConcept", "Supplemental instruction - e.g. \"with meals\".", 0, java.lang.Integer.MAX_VALUE, additionalInstruction)); 701 children.add(new Property("patientInstruction", "string", "Instructions in terms that are understood by the patient or consumer.", 0, 1, patientInstruction)); 702 children.add(new Property("timing", "Timing", "When medication should be administered.", 0, 1, timing)); 703 children.add(new Property("asNeeded[x]", "boolean|CodeableConcept", "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 0, 1, asNeeded)); 704 children.add(new Property("site", "CodeableConcept", "Body site to administer to.", 0, 1, site)); 705 children.add(new Property("route", "CodeableConcept", "How drug should enter body.", 0, 1, route)); 706 children.add(new Property("method", "CodeableConcept", "Technique for administering medication.", 0, 1, method)); 707 children.add(new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, dose)); 708 children.add(new Property("maxDosePerPeriod", "Ratio", "Upper limit on medication per unit of time.", 0, 1, maxDosePerPeriod)); 709 children.add(new Property("maxDosePerAdministration", "SimpleQuantity", "Upper limit on medication per administration.", 0, 1, maxDosePerAdministration)); 710 children.add(new Property("maxDosePerLifetime", "SimpleQuantity", "Upper limit on medication per lifetime of the patient.", 0, 1, maxDosePerLifetime)); 711 children.add(new Property("rate[x]", "Ratio|Range|SimpleQuantity", "Amount of medication per unit of time.", 0, 1, rate)); 712 } 713 714 @Override 715 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 716 switch (_hash) { 717 case 1349547969: /*sequence*/ return new Property("sequence", "integer", "Indicates the order in which the dosage instructions should be applied or interpreted.", 0, 1, sequence); 718 case 3556653: /*text*/ return new Property("text", "string", "Free text dosage instructions e.g. SIG.", 0, 1, text); 719 case 1623641575: /*additionalInstruction*/ return new Property("additionalInstruction", "CodeableConcept", "Supplemental instruction - e.g. \"with meals\".", 0, java.lang.Integer.MAX_VALUE, additionalInstruction); 720 case 737543241: /*patientInstruction*/ return new Property("patientInstruction", "string", "Instructions in terms that are understood by the patient or consumer.", 0, 1, patientInstruction); 721 case -873664438: /*timing*/ return new Property("timing", "Timing", "When medication should be administered.", 0, 1, timing); 722 case -544329575: /*asNeeded[x]*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 0, 1, asNeeded); 723 case -1432923513: /*asNeeded*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 0, 1, asNeeded); 724 case -591717471: /*asNeededBoolean*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 0, 1, asNeeded); 725 case 1556420122: /*asNeededCodeableConcept*/ return new Property("asNeeded[x]", "boolean|CodeableConcept", "Indicates whether the Medication is only taken when needed within a specific dosing schedule (Boolean option), or it indicates the precondition for taking the Medication (CodeableConcept).", 0, 1, asNeeded); 726 case 3530567: /*site*/ return new Property("site", "CodeableConcept", "Body site to administer to.", 0, 1, site); 727 case 108704329: /*route*/ return new Property("route", "CodeableConcept", "How drug should enter body.", 0, 1, route); 728 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "Technique for administering medication.", 0, 1, method); 729 case 1843195715: /*dose[x]*/ return new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, dose); 730 case 3089437: /*dose*/ return new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, dose); 731 case 1775578912: /*doseRange*/ return new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, dose); 732 case 1230053850: /*doseSimpleQuantity*/ return new Property("dose[x]", "Range|SimpleQuantity", "Amount of medication per dose.", 0, 1, dose); 733 case 1506263709: /*maxDosePerPeriod*/ return new Property("maxDosePerPeriod", "Ratio", "Upper limit on medication per unit of time.", 0, 1, maxDosePerPeriod); 734 case 2004889914: /*maxDosePerAdministration*/ return new Property("maxDosePerAdministration", "SimpleQuantity", "Upper limit on medication per administration.", 0, 1, maxDosePerAdministration); 735 case 642099621: /*maxDosePerLifetime*/ return new Property("maxDosePerLifetime", "SimpleQuantity", "Upper limit on medication per lifetime of the patient.", 0, 1, maxDosePerLifetime); 736 case 983460768: /*rate[x]*/ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", "Amount of medication per unit of time.", 0, 1, rate); 737 case 3493088: /*rate*/ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", "Amount of medication per unit of time.", 0, 1, rate); 738 case 204021515: /*rateRatio*/ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", "Amount of medication per unit of time.", 0, 1, rate); 739 case 204015677: /*rateRange*/ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", "Amount of medication per unit of time.", 0, 1, rate); 740 case -2121057955: /*rateSimpleQuantity*/ return new Property("rate[x]", "Ratio|Range|SimpleQuantity", "Amount of medication per unit of time.", 0, 1, rate); 741 default: return super.getNamedProperty(_hash, _name, _checkValid); 742 } 743 744 } 745 746 @Override 747 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 748 switch (hash) { 749 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // IntegerType 750 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 751 case 1623641575: /*additionalInstruction*/ return this.additionalInstruction == null ? new Base[0] : this.additionalInstruction.toArray(new Base[this.additionalInstruction.size()]); // CodeableConcept 752 case 737543241: /*patientInstruction*/ return this.patientInstruction == null ? new Base[0] : new Base[] {this.patientInstruction}; // StringType 753 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Timing 754 case -1432923513: /*asNeeded*/ return this.asNeeded == null ? new Base[0] : new Base[] {this.asNeeded}; // Type 755 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // CodeableConcept 756 case 108704329: /*route*/ return this.route == null ? new Base[0] : new Base[] {this.route}; // CodeableConcept 757 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 758 case 3089437: /*dose*/ return this.dose == null ? new Base[0] : new Base[] {this.dose}; // Type 759 case 1506263709: /*maxDosePerPeriod*/ return this.maxDosePerPeriod == null ? new Base[0] : new Base[] {this.maxDosePerPeriod}; // Ratio 760 case 2004889914: /*maxDosePerAdministration*/ return this.maxDosePerAdministration == null ? new Base[0] : new Base[] {this.maxDosePerAdministration}; // SimpleQuantity 761 case 642099621: /*maxDosePerLifetime*/ return this.maxDosePerLifetime == null ? new Base[0] : new Base[] {this.maxDosePerLifetime}; // SimpleQuantity 762 case 3493088: /*rate*/ return this.rate == null ? new Base[0] : new Base[] {this.rate}; // Type 763 default: return super.getProperty(hash, name, checkValid); 764 } 765 766 } 767 768 @Override 769 public Base setProperty(int hash, String name, Base value) throws FHIRException { 770 switch (hash) { 771 case 1349547969: // sequence 772 this.sequence = castToInteger(value); // IntegerType 773 return value; 774 case 3556653: // text 775 this.text = castToString(value); // StringType 776 return value; 777 case 1623641575: // additionalInstruction 778 this.getAdditionalInstruction().add(castToCodeableConcept(value)); // CodeableConcept 779 return value; 780 case 737543241: // patientInstruction 781 this.patientInstruction = castToString(value); // StringType 782 return value; 783 case -873664438: // timing 784 this.timing = castToTiming(value); // Timing 785 return value; 786 case -1432923513: // asNeeded 787 this.asNeeded = castToType(value); // Type 788 return value; 789 case 3530567: // site 790 this.site = castToCodeableConcept(value); // CodeableConcept 791 return value; 792 case 108704329: // route 793 this.route = castToCodeableConcept(value); // CodeableConcept 794 return value; 795 case -1077554975: // method 796 this.method = castToCodeableConcept(value); // CodeableConcept 797 return value; 798 case 3089437: // dose 799 this.dose = castToType(value); // Type 800 return value; 801 case 1506263709: // maxDosePerPeriod 802 this.maxDosePerPeriod = castToRatio(value); // Ratio 803 return value; 804 case 2004889914: // maxDosePerAdministration 805 this.maxDosePerAdministration = castToSimpleQuantity(value); // SimpleQuantity 806 return value; 807 case 642099621: // maxDosePerLifetime 808 this.maxDosePerLifetime = castToSimpleQuantity(value); // SimpleQuantity 809 return value; 810 case 3493088: // rate 811 this.rate = castToType(value); // Type 812 return value; 813 default: return super.setProperty(hash, name, value); 814 } 815 816 } 817 818 @Override 819 public Base setProperty(String name, Base value) throws FHIRException { 820 if (name.equals("sequence")) { 821 this.sequence = castToInteger(value); // IntegerType 822 } else if (name.equals("text")) { 823 this.text = castToString(value); // StringType 824 } else if (name.equals("additionalInstruction")) { 825 this.getAdditionalInstruction().add(castToCodeableConcept(value)); 826 } else if (name.equals("patientInstruction")) { 827 this.patientInstruction = castToString(value); // StringType 828 } else if (name.equals("timing")) { 829 this.timing = castToTiming(value); // Timing 830 } else if (name.equals("asNeeded[x]")) { 831 this.asNeeded = castToType(value); // Type 832 } else if (name.equals("site")) { 833 this.site = castToCodeableConcept(value); // CodeableConcept 834 } else if (name.equals("route")) { 835 this.route = castToCodeableConcept(value); // CodeableConcept 836 } else if (name.equals("method")) { 837 this.method = castToCodeableConcept(value); // CodeableConcept 838 } else if (name.equals("dose[x]")) { 839 this.dose = castToType(value); // Type 840 } else if (name.equals("maxDosePerPeriod")) { 841 this.maxDosePerPeriod = castToRatio(value); // Ratio 842 } else if (name.equals("maxDosePerAdministration")) { 843 this.maxDosePerAdministration = castToSimpleQuantity(value); // SimpleQuantity 844 } else if (name.equals("maxDosePerLifetime")) { 845 this.maxDosePerLifetime = castToSimpleQuantity(value); // SimpleQuantity 846 } else if (name.equals("rate[x]")) { 847 this.rate = castToType(value); // Type 848 } else 849 return super.setProperty(name, value); 850 return value; 851 } 852 853 @Override 854 public Base makeProperty(int hash, String name) throws FHIRException { 855 switch (hash) { 856 case 1349547969: return getSequenceElement(); 857 case 3556653: return getTextElement(); 858 case 1623641575: return addAdditionalInstruction(); 859 case 737543241: return getPatientInstructionElement(); 860 case -873664438: return getTiming(); 861 case -544329575: return getAsNeeded(); 862 case -1432923513: return getAsNeeded(); 863 case 3530567: return getSite(); 864 case 108704329: return getRoute(); 865 case -1077554975: return getMethod(); 866 case 1843195715: return getDose(); 867 case 3089437: return getDose(); 868 case 1506263709: return getMaxDosePerPeriod(); 869 case 2004889914: return getMaxDosePerAdministration(); 870 case 642099621: return getMaxDosePerLifetime(); 871 case 983460768: return getRate(); 872 case 3493088: return getRate(); 873 default: return super.makeProperty(hash, name); 874 } 875 876 } 877 878 @Override 879 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 880 switch (hash) { 881 case 1349547969: /*sequence*/ return new String[] {"integer"}; 882 case 3556653: /*text*/ return new String[] {"string"}; 883 case 1623641575: /*additionalInstruction*/ return new String[] {"CodeableConcept"}; 884 case 737543241: /*patientInstruction*/ return new String[] {"string"}; 885 case -873664438: /*timing*/ return new String[] {"Timing"}; 886 case -1432923513: /*asNeeded*/ return new String[] {"boolean", "CodeableConcept"}; 887 case 3530567: /*site*/ return new String[] {"CodeableConcept"}; 888 case 108704329: /*route*/ return new String[] {"CodeableConcept"}; 889 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 890 case 3089437: /*dose*/ return new String[] {"Range", "SimpleQuantity"}; 891 case 1506263709: /*maxDosePerPeriod*/ return new String[] {"Ratio"}; 892 case 2004889914: /*maxDosePerAdministration*/ return new String[] {"SimpleQuantity"}; 893 case 642099621: /*maxDosePerLifetime*/ return new String[] {"SimpleQuantity"}; 894 case 3493088: /*rate*/ return new String[] {"Ratio", "Range", "SimpleQuantity"}; 895 default: return super.getTypesForProperty(hash, name); 896 } 897 898 } 899 900 @Override 901 public Base addChild(String name) throws FHIRException { 902 if (name.equals("sequence")) { 903 throw new FHIRException("Cannot call addChild on a singleton property Dosage.sequence"); 904 } 905 else if (name.equals("text")) { 906 throw new FHIRException("Cannot call addChild on a singleton property Dosage.text"); 907 } 908 else if (name.equals("additionalInstruction")) { 909 return addAdditionalInstruction(); 910 } 911 else if (name.equals("patientInstruction")) { 912 throw new FHIRException("Cannot call addChild on a singleton property Dosage.patientInstruction"); 913 } 914 else if (name.equals("timing")) { 915 this.timing = new Timing(); 916 return this.timing; 917 } 918 else if (name.equals("asNeededBoolean")) { 919 this.asNeeded = new BooleanType(); 920 return this.asNeeded; 921 } 922 else if (name.equals("asNeededCodeableConcept")) { 923 this.asNeeded = new CodeableConcept(); 924 return this.asNeeded; 925 } 926 else if (name.equals("site")) { 927 this.site = new CodeableConcept(); 928 return this.site; 929 } 930 else if (name.equals("route")) { 931 this.route = new CodeableConcept(); 932 return this.route; 933 } 934 else if (name.equals("method")) { 935 this.method = new CodeableConcept(); 936 return this.method; 937 } 938 else if (name.equals("doseRange")) { 939 this.dose = new Range(); 940 return this.dose; 941 } 942 else if (name.equals("doseSimpleQuantity")) { 943 this.dose = new SimpleQuantity(); 944 return this.dose; 945 } 946 else if (name.equals("maxDosePerPeriod")) { 947 this.maxDosePerPeriod = new Ratio(); 948 return this.maxDosePerPeriod; 949 } 950 else if (name.equals("maxDosePerAdministration")) { 951 this.maxDosePerAdministration = new SimpleQuantity(); 952 return this.maxDosePerAdministration; 953 } 954 else if (name.equals("maxDosePerLifetime")) { 955 this.maxDosePerLifetime = new SimpleQuantity(); 956 return this.maxDosePerLifetime; 957 } 958 else if (name.equals("rateRatio")) { 959 this.rate = new Ratio(); 960 return this.rate; 961 } 962 else if (name.equals("rateRange")) { 963 this.rate = new Range(); 964 return this.rate; 965 } 966 else if (name.equals("rateSimpleQuantity")) { 967 this.rate = new SimpleQuantity(); 968 return this.rate; 969 } 970 else 971 return super.addChild(name); 972 } 973 974 public String fhirType() { 975 return "Dosage"; 976 977 } 978 979 public Dosage copy() { 980 Dosage dst = new Dosage(); 981 copyValues(dst); 982 dst.sequence = sequence == null ? null : sequence.copy(); 983 dst.text = text == null ? null : text.copy(); 984 if (additionalInstruction != null) { 985 dst.additionalInstruction = new ArrayList<CodeableConcept>(); 986 for (CodeableConcept i : additionalInstruction) 987 dst.additionalInstruction.add(i.copy()); 988 }; 989 dst.patientInstruction = patientInstruction == null ? null : patientInstruction.copy(); 990 dst.timing = timing == null ? null : timing.copy(); 991 dst.asNeeded = asNeeded == null ? null : asNeeded.copy(); 992 dst.site = site == null ? null : site.copy(); 993 dst.route = route == null ? null : route.copy(); 994 dst.method = method == null ? null : method.copy(); 995 dst.dose = dose == null ? null : dose.copy(); 996 dst.maxDosePerPeriod = maxDosePerPeriod == null ? null : maxDosePerPeriod.copy(); 997 dst.maxDosePerAdministration = maxDosePerAdministration == null ? null : maxDosePerAdministration.copy(); 998 dst.maxDosePerLifetime = maxDosePerLifetime == null ? null : maxDosePerLifetime.copy(); 999 dst.rate = rate == null ? null : rate.copy(); 1000 return dst; 1001 } 1002 1003 protected Dosage typedCopy() { 1004 return copy(); 1005 } 1006 1007 @Override 1008 public boolean equalsDeep(Base other_) { 1009 if (!super.equalsDeep(other_)) 1010 return false; 1011 if (!(other_ instanceof Dosage)) 1012 return false; 1013 Dosage o = (Dosage) other_; 1014 return compareDeep(sequence, o.sequence, true) && compareDeep(text, o.text, true) && compareDeep(additionalInstruction, o.additionalInstruction, true) 1015 && compareDeep(patientInstruction, o.patientInstruction, true) && compareDeep(timing, o.timing, true) 1016 && compareDeep(asNeeded, o.asNeeded, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 1017 && compareDeep(method, o.method, true) && compareDeep(dose, o.dose, true) && compareDeep(maxDosePerPeriod, o.maxDosePerPeriod, true) 1018 && compareDeep(maxDosePerAdministration, o.maxDosePerAdministration, true) && compareDeep(maxDosePerLifetime, o.maxDosePerLifetime, true) 1019 && compareDeep(rate, o.rate, true); 1020 } 1021 1022 @Override 1023 public boolean equalsShallow(Base other_) { 1024 if (!super.equalsShallow(other_)) 1025 return false; 1026 if (!(other_ instanceof Dosage)) 1027 return false; 1028 Dosage o = (Dosage) other_; 1029 return compareValues(sequence, o.sequence, true) && compareValues(text, o.text, true) && compareValues(patientInstruction, o.patientInstruction, true) 1030 ; 1031 } 1032 1033 public boolean isEmpty() { 1034 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, text, additionalInstruction 1035 , patientInstruction, timing, asNeeded, site, route, method, dose, maxDosePerPeriod 1036 , maxDosePerAdministration, maxDosePerLifetime, rate); 1037 } 1038 1039 1040}