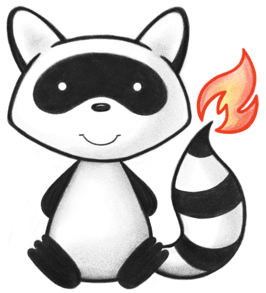
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040 041import ca.uhn.fhir.model.api.annotation.DatatypeDef; 042/** 043 * A length of time. 044 */ 045@DatatypeDef(name="Duration") 046public class Duration extends Quantity implements ICompositeType { 047 048 private static final long serialVersionUID = 0L; 049 050 /** 051 * Constructor 052 */ 053 public Duration() { 054 super(); 055 } 056 057 protected void listChildren(List<Property> children) { 058 super.listChildren(children); 059 } 060 061 @Override 062 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 063 switch (_hash) { 064 default: return super.getNamedProperty(_hash, _name, _checkValid); 065 } 066 067 } 068 069 @Override 070 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 071 switch (hash) { 072 default: return super.getProperty(hash, name, checkValid); 073 } 074 075 } 076 077 @Override 078 public Base setProperty(int hash, String name, Base value) throws FHIRException { 079 switch (hash) { 080 default: return super.setProperty(hash, name, value); 081 } 082 083 } 084 085 @Override 086 public Base setProperty(String name, Base value) throws FHIRException { 087 return super.setProperty(name, value); 088 } 089 090 @Override 091 public Base makeProperty(int hash, String name) throws FHIRException { 092 switch (hash) { 093 default: return super.makeProperty(hash, name); 094 } 095 096 } 097 098 @Override 099 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 100 switch (hash) { 101 default: return super.getTypesForProperty(hash, name); 102 } 103 104 } 105 106 @Override 107 public Base addChild(String name) throws FHIRException { 108 return super.addChild(name); 109 } 110 111 public String fhirType() { 112 return "Duration"; 113 114 } 115 116 public Duration copy() { 117 Duration dst = new Duration(); 118 copyValues(dst); 119 return dst; 120 } 121 122 protected Duration typedCopy() { 123 return copy(); 124 } 125 126 @Override 127 public boolean equalsDeep(Base other_) { 128 if (!super.equalsDeep(other_)) 129 return false; 130 if (!(other_ instanceof Duration)) 131 return false; 132 Duration o = (Duration) other_; 133 return true; 134 } 135 136 @Override 137 public boolean equalsShallow(Base other_) { 138 if (!super.equalsShallow(other_)) 139 return false; 140 if (!(other_ instanceof Duration)) 141 return false; 142 Duration o = (Duration) other_; 143 return true; 144 } 145 146 public boolean isEmpty() { 147 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(); 148 } 149 150 151}