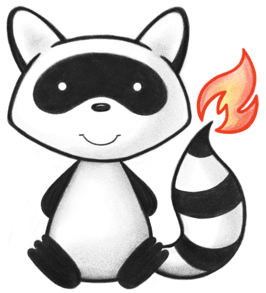
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032 */ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseElement; 040import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * Base definition for all elements in a resource. 047 */ 048public abstract class Element extends Base implements IBaseHasExtensions, IBaseElement { 049 050 /** 051 * unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces. 052 */ 053 @Child(name = "id", type = {StringType.class}, order=0, min=0, max=1, modifier=false, summary=false) 054 @Description(shortDefinition="xml:id (or equivalent in JSON)", formalDefinition="unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces." ) 055 protected StringType id; 056 057 /** 058 * May be used to represent additional information that is not part of the basic definition of the element. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension. 059 */ 060 @Child(name = "extension", type = {Extension.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 061 @Description(shortDefinition="Additional Content defined by implementations", formalDefinition="May be used to represent additional information that is not part of the basic definition of the element. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension." ) 062 protected List<Extension> extension; 063 064 private static final long serialVersionUID = -1452745816L; 065 066 /** 067 * Constructor 068 */ 069 public Element() { 070 super(); 071 } 072 073 /** 074 * @return {@link #id} (unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 075 */ 076 public StringType getIdElement() { 077 if (this.id == null) 078 if (Configuration.errorOnAutoCreate()) 079 throw new Error("Attempt to auto-create Element.id"); 080 else if (Configuration.doAutoCreate()) 081 this.id = new StringType(); // bb 082 return this.id; 083 } 084 085 public boolean hasIdElement() { 086 return this.id != null && !this.id.isEmpty(); 087 } 088 089 public boolean hasId() { 090 return this.id != null && !this.id.isEmpty(); 091 } 092 093 /** 094 * @param value {@link #id} (unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.). This is the underlying object with id, value and extensions. The accessor "getId" gives direct access to the value 095 */ 096 public Element setIdElement(StringType value) { 097 this.id = value; 098 return this; 099 } 100 101 /** 102 * @return unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces. 103 */ 104 public String getId() { 105 return this.id == null ? null : this.id.getValue(); 106 } 107 108 /** 109 * @param value unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces. 110 */ 111 public Element setId(String value) { 112 if (Utilities.noString(value)) 113 this.id = null; 114 else { 115 if (this.id == null) 116 this.id = new StringType(); 117 this.id.setValue(value); 118 } 119 return this; 120 } 121 122 /** 123 * @return {@link #extension} (May be used to represent additional information that is not part of the basic definition of the element. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.) 124 */ 125 public List<Extension> getExtension() { 126 if (this.extension == null) 127 this.extension = new ArrayList<Extension>(); 128 return this.extension; 129 } 130 131 /** 132 * @return Returns a reference to <code>this</code> for easy method chaining 133 */ 134 public Element setExtension(List<Extension> theExtension) { 135 this.extension = theExtension; 136 return this; 137 } 138 139 public boolean hasExtension() { 140 if (this.extension == null) 141 return false; 142 for (Extension item : this.extension) 143 if (!item.isEmpty()) 144 return true; 145 return false; 146 } 147 148 public Extension addExtension() { //3 149 Extension t = new Extension(); 150 if (this.extension == null) 151 this.extension = new ArrayList<Extension>(); 152 this.extension.add(t); 153 return t; 154 } 155 156 public Element addExtension(Extension t) { //3 157 if (t == null) 158 return this; 159 if (this.extension == null) 160 this.extension = new ArrayList<Extension>(); 161 this.extension.add(t); 162 return this; 163 } 164 165 /** 166 * @return The first repetition of repeating field {@link #extension}, creating it if it does not already exist 167 */ 168 public Extension getExtensionFirstRep() { 169 if (getExtension().isEmpty()) { 170 addExtension(); 171 } 172 return getExtension().get(0); 173 } 174 175 176 public Extension getExtensionByUrl(String theUrl) { 177 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 178 ArrayList<Extension> retVal = new ArrayList<Extension>(); 179 for (Extension next : getExtension()) { 180 if (theUrl.equals(next.getUrl())) { 181 retVal.add(next); 182 } 183 } 184 if (retVal.size() == 0) 185 return null; 186 else { 187 org.apache.commons.lang3.Validate.isTrue(retVal.size() == 1, "Url "+theUrl+" must have only one match"); 188 return retVal.get(0); 189 } 190 } 191 192 /** 193 * Returns an unmodifiable list containing all extensions on this element which 194 * match the given URL. 195 * 196 * @param theUrl The URL. Must not be blank or null. 197 * @return an unmodifiable list containing all extensions on this element which 198 * match the given URL 199 */ 200 public List<Extension> getExtensionsByUrl(String theUrl) { 201 org.apache.commons.lang3.Validate.notBlank(theUrl, "theUrl must not be blank or null"); 202 ArrayList<Extension> retVal = new ArrayList<Extension>(); 203 for (Extension next : getExtension()) { 204 if (theUrl.equals(next.getUrl())) { 205 retVal.add(next); 206 } 207 } 208 return java.util.Collections.unmodifiableList(retVal); 209 } 210 public boolean hasExtension(String theUrl) { 211 return !getExtensionsByUrl(theUrl).isEmpty(); 212 } 213 214 public String getExtensionString(String theUrl) throws FHIRException { 215 List<Extension> ext = getExtensionsByUrl(theUrl); 216 if (ext.isEmpty()) 217 return null; 218 if (ext.size() > 1) 219 throw new FHIRException("Multiple matching extensions found"); 220 if (!ext.get(0).getValue().isPrimitive()) 221 throw new FHIRException("Extension could not be converted to a string"); 222 return ext.get(0).getValue().primitiveValue(); 223 } 224 225 protected void listChildren(List<Property> children) { 226 children.add(new Property("id", "string", "unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.", 0, 1, id)); 227 children.add(new Property("extension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 0, java.lang.Integer.MAX_VALUE, extension)); 228 } 229 230 @Override 231 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 232 switch (_hash) { 233 case 3355: /*id*/ return new Property("id", "string", "unique id for the element within a resource (for internal references). This may be any string value that does not contain spaces.", 0, 1, id); 234 case -612557761: /*extension*/ return new Property("extension", "Extension", "May be used to represent additional information that is not part of the basic definition of the element. In order to make the use of extensions safe and manageable, there is a strict set of governance applied to the definition and use of extensions. Though any implementer is allowed to define an extension, there is a set of requirements that SHALL be met as part of the definition of the extension.", 0, java.lang.Integer.MAX_VALUE, extension); 235 default: return super.getNamedProperty(_hash, _name, _checkValid); 236 } 237 238 } 239 240 @Override 241 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 242 switch (hash) { 243 case 3355: /*id*/ return this.id == null ? new Base[0] : new Base[] {this.id}; // StringType 244 case -612557761: /*extension*/ return this.extension == null ? new Base[0] : this.extension.toArray(new Base[this.extension.size()]); // Extension 245 default: return super.getProperty(hash, name, checkValid); 246 } 247 248 } 249 250 @Override 251 public Base setProperty(int hash, String name, Base value) throws FHIRException { 252 switch (hash) { 253 case 3355: // id 254 this.id = castToString(value); // StringType 255 return value; 256 case -612557761: // extension 257 this.getExtension().add(castToExtension(value)); // Extension 258 return value; 259 default: return super.setProperty(hash, name, value); 260 } 261 262 } 263 264 @Override 265 public Base setProperty(String name, Base value) throws FHIRException { 266 if (name.equals("id")) { 267 this.id = castToString(value); // StringType 268 } else if (name.equals("extension")) { 269 this.getExtension().add(castToExtension(value)); 270 } else 271 return super.setProperty(name, value); 272 return value; 273 } 274 275 @Override 276 public Base makeProperty(int hash, String name) throws FHIRException { 277 switch (hash) { 278 case 3355: return getIdElement(); 279 case -612557761: return addExtension(); 280 default: return super.makeProperty(hash, name); 281 } 282 283 } 284 285 @Override 286 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 287 switch (hash) { 288 case 3355: /*id*/ return new String[] {"string"}; 289 case -612557761: /*extension*/ return new String[] {"Extension"}; 290 default: return super.getTypesForProperty(hash, name); 291 } 292 293 } 294 295 @Override 296 public Base addChild(String name) throws FHIRException { 297 if (name.equals("id")) { 298 throw new FHIRException("Cannot call addChild on a singleton property Element.id"); 299 } 300 else if (name.equals("extension")) { 301 return addExtension(); 302 } 303 else 304 return super.addChild(name); 305 } 306 307 public String fhirType() { 308 return "Element"; 309 310 } 311 312 public abstract Element copy(); 313 314 public void copyValues(Element dst) { 315 dst.id = id == null ? null : id.copy(); 316 if (extension != null) { 317 dst.extension = new ArrayList<Extension>(); 318 for (Extension i : extension) 319 dst.extension.add(i.copy()); 320 }; 321 } 322 323 @Override 324 public boolean equalsDeep(Base other_) { 325 if (!super.equalsDeep(other_)) 326 return false; 327 if (!(other_ instanceof Element)) 328 return false; 329 Element o = (Element) other_; 330 return compareDeep(id, o.id, true) && compareDeep(extension, o.extension, true); 331 } 332 333 @Override 334 public boolean equalsShallow(Base other_) { 335 if (!super.equalsShallow(other_)) 336 return false; 337 if (!(other_ instanceof Element)) 338 return false; 339 Element o = (Element) other_; 340 return compareValues(id, o.id, true); 341 } 342 343 public boolean isEmpty() { 344 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(id, extension); 345 } 346 347 @Override 348 public String getIdBase() { 349 return getId(); 350 } 351 352 @Override 353 public void setIdBase(String value) { 354 setId(value); 355 } 356 // added from java-adornments.txt: 357 public void addExtension(String url, Type value) { 358 Extension ex = new Extension(); 359 ex.setUrl(url); 360 ex.setValue(value); 361 getExtension().add(ex); 362 } 363 364 365 // end addition 366 367}