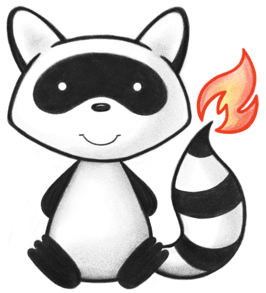
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.dstu3.model.Enumerations.BindingStrength; 039import org.hl7.fhir.dstu3.model.Enumerations.BindingStrengthEnumFactory; 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseDatatypeElement; 043import org.hl7.fhir.instance.model.api.ICompositeType; 044import org.hl7.fhir.utilities.CommaSeparatedStringBuilder; 045import org.hl7.fhir.utilities.Utilities; 046 047import ca.uhn.fhir.model.api.annotation.Block; 048import ca.uhn.fhir.model.api.annotation.Child; 049import ca.uhn.fhir.model.api.annotation.DatatypeDef; 050import ca.uhn.fhir.model.api.annotation.Description; 051/** 052 * Captures constraints on each element within the resource, profile, or extension. 053 */ 054@DatatypeDef(name="ElementDefinition") 055public class ElementDefinition extends Type implements ICompositeType { 056 057 public enum PropertyRepresentation { 058 /** 059 * In XML, this property is represented as an attribute not an element. 060 */ 061 XMLATTR, 062 /** 063 * This element is represented using the XML text attribute (primitives only) 064 */ 065 XMLTEXT, 066 /** 067 * The type of this element is indicated using xsi:type 068 */ 069 TYPEATTR, 070 /** 071 * Use CDA narrative instead of XHTML 072 */ 073 CDATEXT, 074 /** 075 * The property is represented using XHTML 076 */ 077 XHTML, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 public static PropertyRepresentation fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("xmlAttr".equals(codeString)) 086 return XMLATTR; 087 if ("xmlText".equals(codeString)) 088 return XMLTEXT; 089 if ("typeAttr".equals(codeString)) 090 return TYPEATTR; 091 if ("cdaText".equals(codeString)) 092 return CDATEXT; 093 if ("xhtml".equals(codeString)) 094 return XHTML; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown PropertyRepresentation code '"+codeString+"'"); 099 } 100 public String toCode() { 101 switch (this) { 102 case XMLATTR: return "xmlAttr"; 103 case XMLTEXT: return "xmlText"; 104 case TYPEATTR: return "typeAttr"; 105 case CDATEXT: return "cdaText"; 106 case XHTML: return "xhtml"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getSystem() { 112 switch (this) { 113 case XMLATTR: return "http://hl7.org/fhir/property-representation"; 114 case XMLTEXT: return "http://hl7.org/fhir/property-representation"; 115 case TYPEATTR: return "http://hl7.org/fhir/property-representation"; 116 case CDATEXT: return "http://hl7.org/fhir/property-representation"; 117 case XHTML: return "http://hl7.org/fhir/property-representation"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDefinition() { 123 switch (this) { 124 case XMLATTR: return "In XML, this property is represented as an attribute not an element."; 125 case XMLTEXT: return "This element is represented using the XML text attribute (primitives only)"; 126 case TYPEATTR: return "The type of this element is indicated using xsi:type"; 127 case CDATEXT: return "Use CDA narrative instead of XHTML"; 128 case XHTML: return "The property is represented using XHTML"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 public String getDisplay() { 134 switch (this) { 135 case XMLATTR: return "XML Attribute"; 136 case XMLTEXT: return "XML Text"; 137 case TYPEATTR: return "Type Attribute"; 138 case CDATEXT: return "CDA Text Format"; 139 case XHTML: return "XHTML"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 } 145 146 public static class PropertyRepresentationEnumFactory implements EnumFactory<PropertyRepresentation> { 147 public PropertyRepresentation fromCode(String codeString) throws IllegalArgumentException { 148 if (codeString == null || "".equals(codeString)) 149 if (codeString == null || "".equals(codeString)) 150 return null; 151 if ("xmlAttr".equals(codeString)) 152 return PropertyRepresentation.XMLATTR; 153 if ("xmlText".equals(codeString)) 154 return PropertyRepresentation.XMLTEXT; 155 if ("typeAttr".equals(codeString)) 156 return PropertyRepresentation.TYPEATTR; 157 if ("cdaText".equals(codeString)) 158 return PropertyRepresentation.CDATEXT; 159 if ("xhtml".equals(codeString)) 160 return PropertyRepresentation.XHTML; 161 throw new IllegalArgumentException("Unknown PropertyRepresentation code '"+codeString+"'"); 162 } 163 public Enumeration<PropertyRepresentation> fromType(PrimitiveType<?> code) throws FHIRException { 164 if (code == null) 165 return null; 166 if (code.isEmpty()) 167 return new Enumeration<PropertyRepresentation>(this); 168 String codeString = code.asStringValue(); 169 if (codeString == null || "".equals(codeString)) 170 return null; 171 if ("xmlAttr".equals(codeString)) 172 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLATTR); 173 if ("xmlText".equals(codeString)) 174 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XMLTEXT); 175 if ("typeAttr".equals(codeString)) 176 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.TYPEATTR); 177 if ("cdaText".equals(codeString)) 178 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.CDATEXT); 179 if ("xhtml".equals(codeString)) 180 return new Enumeration<PropertyRepresentation>(this, PropertyRepresentation.XHTML); 181 throw new FHIRException("Unknown PropertyRepresentation code '"+codeString+"'"); 182 } 183 public String toCode(PropertyRepresentation code) { 184 if (code == PropertyRepresentation.NULL) 185 return null; 186 if (code == PropertyRepresentation.XMLATTR) 187 return "xmlAttr"; 188 if (code == PropertyRepresentation.XMLTEXT) 189 return "xmlText"; 190 if (code == PropertyRepresentation.TYPEATTR) 191 return "typeAttr"; 192 if (code == PropertyRepresentation.CDATEXT) 193 return "cdaText"; 194 if (code == PropertyRepresentation.XHTML) 195 return "xhtml"; 196 return "?"; 197 } 198 public String toSystem(PropertyRepresentation code) { 199 return code.getSystem(); 200 } 201 } 202 203 public enum DiscriminatorType { 204 /** 205 * The slices have different values in the nominated element 206 */ 207 VALUE, 208 /** 209 * The slices are differentiated by the presence or absence of the nominated element 210 */ 211 EXISTS, 212 /** 213 * The slices have different values in the nominated element, as determined by testing them against the applicable ElementDefinition.pattern[x] 214 */ 215 PATTERN, 216 /** 217 * The slices are differentiated by type of the nominated element to a specifed profile 218 */ 219 TYPE, 220 /** 221 * The slices are differentiated by conformance of the nominated element to a specifed profile 222 */ 223 PROFILE, 224 /** 225 * added to help the parsers with the generic types 226 */ 227 NULL; 228 public static DiscriminatorType fromCode(String codeString) throws FHIRException { 229 if (codeString == null || "".equals(codeString)) 230 return null; 231 if ("value".equals(codeString)) 232 return VALUE; 233 if ("exists".equals(codeString)) 234 return EXISTS; 235 if ("pattern".equals(codeString)) 236 return PATTERN; 237 if ("type".equals(codeString)) 238 return TYPE; 239 if ("profile".equals(codeString)) 240 return PROFILE; 241 if (Configuration.isAcceptInvalidEnums()) 242 return null; 243 else 244 throw new FHIRException("Unknown DiscriminatorType code '"+codeString+"'"); 245 } 246 public String toCode() { 247 switch (this) { 248 case VALUE: return "value"; 249 case EXISTS: return "exists"; 250 case PATTERN: return "pattern"; 251 case TYPE: return "type"; 252 case PROFILE: return "profile"; 253 case NULL: return null; 254 default: return "?"; 255 } 256 } 257 public String getSystem() { 258 switch (this) { 259 case VALUE: return "http://hl7.org/fhir/discriminator-type"; 260 case EXISTS: return "http://hl7.org/fhir/discriminator-type"; 261 case PATTERN: return "http://hl7.org/fhir/discriminator-type"; 262 case TYPE: return "http://hl7.org/fhir/discriminator-type"; 263 case PROFILE: return "http://hl7.org/fhir/discriminator-type"; 264 case NULL: return null; 265 default: return "?"; 266 } 267 } 268 public String getDefinition() { 269 switch (this) { 270 case VALUE: return "The slices have different values in the nominated element"; 271 case EXISTS: return "The slices are differentiated by the presence or absence of the nominated element"; 272 case PATTERN: return "The slices have different values in the nominated element, as determined by testing them against the applicable ElementDefinition.pattern[x]"; 273 case TYPE: return "The slices are differentiated by type of the nominated element to a specifed profile"; 274 case PROFILE: return "The slices are differentiated by conformance of the nominated element to a specifed profile"; 275 case NULL: return null; 276 default: return "?"; 277 } 278 } 279 public String getDisplay() { 280 switch (this) { 281 case VALUE: return "Value"; 282 case EXISTS: return "Exists"; 283 case PATTERN: return "Pattern"; 284 case TYPE: return "Type"; 285 case PROFILE: return "Profile"; 286 case NULL: return null; 287 default: return "?"; 288 } 289 } 290 } 291 292 public static class DiscriminatorTypeEnumFactory implements EnumFactory<DiscriminatorType> { 293 public DiscriminatorType fromCode(String codeString) throws IllegalArgumentException { 294 if (codeString == null || "".equals(codeString)) 295 if (codeString == null || "".equals(codeString)) 296 return null; 297 if ("value".equals(codeString)) 298 return DiscriminatorType.VALUE; 299 if ("exists".equals(codeString)) 300 return DiscriminatorType.EXISTS; 301 if ("pattern".equals(codeString)) 302 return DiscriminatorType.PATTERN; 303 if ("type".equals(codeString)) 304 return DiscriminatorType.TYPE; 305 if ("profile".equals(codeString)) 306 return DiscriminatorType.PROFILE; 307 throw new IllegalArgumentException("Unknown DiscriminatorType code '"+codeString+"'"); 308 } 309 public Enumeration<DiscriminatorType> fromType(PrimitiveType<?> code) throws FHIRException { 310 if (code == null) 311 return null; 312 if (code.isEmpty()) 313 return new Enumeration<DiscriminatorType>(this); 314 String codeString = code.asStringValue(); 315 if (codeString == null || "".equals(codeString)) 316 return null; 317 if ("value".equals(codeString)) 318 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.VALUE); 319 if ("exists".equals(codeString)) 320 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.EXISTS); 321 if ("pattern".equals(codeString)) 322 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PATTERN); 323 if ("type".equals(codeString)) 324 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.TYPE); 325 if ("profile".equals(codeString)) 326 return new Enumeration<DiscriminatorType>(this, DiscriminatorType.PROFILE); 327 throw new FHIRException("Unknown DiscriminatorType code '"+codeString+"'"); 328 } 329 public String toCode(DiscriminatorType code) { 330 if (code == DiscriminatorType.NULL) 331 return null; 332 if (code == DiscriminatorType.VALUE) 333 return "value"; 334 if (code == DiscriminatorType.EXISTS) 335 return "exists"; 336 if (code == DiscriminatorType.PATTERN) 337 return "pattern"; 338 if (code == DiscriminatorType.TYPE) 339 return "type"; 340 if (code == DiscriminatorType.PROFILE) 341 return "profile"; 342 return "?"; 343 } 344 public String toSystem(DiscriminatorType code) { 345 return code.getSystem(); 346 } 347 } 348 349 public enum SlicingRules { 350 /** 351 * No additional content is allowed other than that described by the slices in this profile. 352 */ 353 CLOSED, 354 /** 355 * Additional content is allowed anywhere in the list. 356 */ 357 OPEN, 358 /** 359 * Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required. 360 */ 361 OPENATEND, 362 /** 363 * added to help the parsers with the generic types 364 */ 365 NULL; 366 public static SlicingRules fromCode(String codeString) throws FHIRException { 367 if (codeString == null || "".equals(codeString)) 368 return null; 369 if ("closed".equals(codeString)) 370 return CLOSED; 371 if ("open".equals(codeString)) 372 return OPEN; 373 if ("openAtEnd".equals(codeString)) 374 return OPENATEND; 375 if (Configuration.isAcceptInvalidEnums()) 376 return null; 377 else 378 throw new FHIRException("Unknown SlicingRules code '"+codeString+"'"); 379 } 380 public String toCode() { 381 switch (this) { 382 case CLOSED: return "closed"; 383 case OPEN: return "open"; 384 case OPENATEND: return "openAtEnd"; 385 case NULL: return null; 386 default: return "?"; 387 } 388 } 389 public String getSystem() { 390 switch (this) { 391 case CLOSED: return "http://hl7.org/fhir/resource-slicing-rules"; 392 case OPEN: return "http://hl7.org/fhir/resource-slicing-rules"; 393 case OPENATEND: return "http://hl7.org/fhir/resource-slicing-rules"; 394 case NULL: return null; 395 default: return "?"; 396 } 397 } 398 public String getDefinition() { 399 switch (this) { 400 case CLOSED: return "No additional content is allowed other than that described by the slices in this profile."; 401 case OPEN: return "Additional content is allowed anywhere in the list."; 402 case OPENATEND: return "Additional content is allowed, but only at the end of the list. Note that using this requires that the slices be ordered, which makes it hard to share uses. This should only be done where absolutely required."; 403 case NULL: return null; 404 default: return "?"; 405 } 406 } 407 public String getDisplay() { 408 switch (this) { 409 case CLOSED: return "Closed"; 410 case OPEN: return "Open"; 411 case OPENATEND: return "Open at End"; 412 case NULL: return null; 413 default: return "?"; 414 } 415 } 416 } 417 418 public static class SlicingRulesEnumFactory implements EnumFactory<SlicingRules> { 419 public SlicingRules fromCode(String codeString) throws IllegalArgumentException { 420 if (codeString == null || "".equals(codeString)) 421 if (codeString == null || "".equals(codeString)) 422 return null; 423 if ("closed".equals(codeString)) 424 return SlicingRules.CLOSED; 425 if ("open".equals(codeString)) 426 return SlicingRules.OPEN; 427 if ("openAtEnd".equals(codeString)) 428 return SlicingRules.OPENATEND; 429 throw new IllegalArgumentException("Unknown SlicingRules code '"+codeString+"'"); 430 } 431 public Enumeration<SlicingRules> fromType(PrimitiveType<?> code) throws FHIRException { 432 if (code == null) 433 return null; 434 if (code.isEmpty()) 435 return new Enumeration<SlicingRules>(this); 436 String codeString = code.asStringValue(); 437 if (codeString == null || "".equals(codeString)) 438 return null; 439 if ("closed".equals(codeString)) 440 return new Enumeration<SlicingRules>(this, SlicingRules.CLOSED); 441 if ("open".equals(codeString)) 442 return new Enumeration<SlicingRules>(this, SlicingRules.OPEN); 443 if ("openAtEnd".equals(codeString)) 444 return new Enumeration<SlicingRules>(this, SlicingRules.OPENATEND); 445 throw new FHIRException("Unknown SlicingRules code '"+codeString+"'"); 446 } 447 public String toCode(SlicingRules code) { 448 if (code == SlicingRules.NULL) 449 return null; 450 if (code == SlicingRules.CLOSED) 451 return "closed"; 452 if (code == SlicingRules.OPEN) 453 return "open"; 454 if (code == SlicingRules.OPENATEND) 455 return "openAtEnd"; 456 return "?"; 457 } 458 public String toSystem(SlicingRules code) { 459 return code.getSystem(); 460 } 461 } 462 463 public enum AggregationMode { 464 /** 465 * The reference is a local reference to a contained resource. 466 */ 467 CONTAINED, 468 /** 469 * The reference to a resource that has to be resolved externally to the resource that includes the reference. 470 */ 471 REFERENCED, 472 /** 473 * The resource the reference points to will be found in the same bundle as the resource that includes the reference. 474 */ 475 BUNDLED, 476 /** 477 * added to help the parsers with the generic types 478 */ 479 NULL; 480 public static AggregationMode fromCode(String codeString) throws FHIRException { 481 if (codeString == null || "".equals(codeString)) 482 return null; 483 if ("contained".equals(codeString)) 484 return CONTAINED; 485 if ("referenced".equals(codeString)) 486 return REFERENCED; 487 if ("bundled".equals(codeString)) 488 return BUNDLED; 489 if (Configuration.isAcceptInvalidEnums()) 490 return null; 491 else 492 throw new FHIRException("Unknown AggregationMode code '"+codeString+"'"); 493 } 494 public String toCode() { 495 switch (this) { 496 case CONTAINED: return "contained"; 497 case REFERENCED: return "referenced"; 498 case BUNDLED: return "bundled"; 499 case NULL: return null; 500 default: return "?"; 501 } 502 } 503 public String getSystem() { 504 switch (this) { 505 case CONTAINED: return "http://hl7.org/fhir/resource-aggregation-mode"; 506 case REFERENCED: return "http://hl7.org/fhir/resource-aggregation-mode"; 507 case BUNDLED: return "http://hl7.org/fhir/resource-aggregation-mode"; 508 case NULL: return null; 509 default: return "?"; 510 } 511 } 512 public String getDefinition() { 513 switch (this) { 514 case CONTAINED: return "The reference is a local reference to a contained resource."; 515 case REFERENCED: return "The reference to a resource that has to be resolved externally to the resource that includes the reference."; 516 case BUNDLED: return "The resource the reference points to will be found in the same bundle as the resource that includes the reference."; 517 case NULL: return null; 518 default: return "?"; 519 } 520 } 521 public String getDisplay() { 522 switch (this) { 523 case CONTAINED: return "Contained"; 524 case REFERENCED: return "Referenced"; 525 case BUNDLED: return "Bundled"; 526 case NULL: return null; 527 default: return "?"; 528 } 529 } 530 } 531 532 public static class AggregationModeEnumFactory implements EnumFactory<AggregationMode> { 533 public AggregationMode fromCode(String codeString) throws IllegalArgumentException { 534 if (codeString == null || "".equals(codeString)) 535 if (codeString == null || "".equals(codeString)) 536 return null; 537 if ("contained".equals(codeString)) 538 return AggregationMode.CONTAINED; 539 if ("referenced".equals(codeString)) 540 return AggregationMode.REFERENCED; 541 if ("bundled".equals(codeString)) 542 return AggregationMode.BUNDLED; 543 throw new IllegalArgumentException("Unknown AggregationMode code '"+codeString+"'"); 544 } 545 public Enumeration<AggregationMode> fromType(PrimitiveType<?> code) throws FHIRException { 546 if (code == null) 547 return null; 548 if (code.isEmpty()) 549 return new Enumeration<AggregationMode>(this); 550 String codeString = code.asStringValue(); 551 if (codeString == null || "".equals(codeString)) 552 return null; 553 if ("contained".equals(codeString)) 554 return new Enumeration<AggregationMode>(this, AggregationMode.CONTAINED); 555 if ("referenced".equals(codeString)) 556 return new Enumeration<AggregationMode>(this, AggregationMode.REFERENCED); 557 if ("bundled".equals(codeString)) 558 return new Enumeration<AggregationMode>(this, AggregationMode.BUNDLED); 559 throw new FHIRException("Unknown AggregationMode code '"+codeString+"'"); 560 } 561 public String toCode(AggregationMode code) { 562 if (code == AggregationMode.NULL) 563 return null; 564 if (code == AggregationMode.CONTAINED) 565 return "contained"; 566 if (code == AggregationMode.REFERENCED) 567 return "referenced"; 568 if (code == AggregationMode.BUNDLED) 569 return "bundled"; 570 return "?"; 571 } 572 public String toSystem(AggregationMode code) { 573 return code.getSystem(); 574 } 575 } 576 577 public enum ReferenceVersionRules { 578 /** 579 * The reference may be either version independent or version specific 580 */ 581 EITHER, 582 /** 583 * The reference must be version independent 584 */ 585 INDEPENDENT, 586 /** 587 * The reference must be version specific 588 */ 589 SPECIFIC, 590 /** 591 * added to help the parsers with the generic types 592 */ 593 NULL; 594 public static ReferenceVersionRules fromCode(String codeString) throws FHIRException { 595 if (codeString == null || "".equals(codeString)) 596 return null; 597 if ("either".equals(codeString)) 598 return EITHER; 599 if ("independent".equals(codeString)) 600 return INDEPENDENT; 601 if ("specific".equals(codeString)) 602 return SPECIFIC; 603 if (Configuration.isAcceptInvalidEnums()) 604 return null; 605 else 606 throw new FHIRException("Unknown ReferenceVersionRules code '"+codeString+"'"); 607 } 608 public String toCode() { 609 switch (this) { 610 case EITHER: return "either"; 611 case INDEPENDENT: return "independent"; 612 case SPECIFIC: return "specific"; 613 case NULL: return null; 614 default: return "?"; 615 } 616 } 617 public String getSystem() { 618 switch (this) { 619 case EITHER: return "http://hl7.org/fhir/reference-version-rules"; 620 case INDEPENDENT: return "http://hl7.org/fhir/reference-version-rules"; 621 case SPECIFIC: return "http://hl7.org/fhir/reference-version-rules"; 622 case NULL: return null; 623 default: return "?"; 624 } 625 } 626 public String getDefinition() { 627 switch (this) { 628 case EITHER: return "The reference may be either version independent or version specific"; 629 case INDEPENDENT: return "The reference must be version independent"; 630 case SPECIFIC: return "The reference must be version specific"; 631 case NULL: return null; 632 default: return "?"; 633 } 634 } 635 public String getDisplay() { 636 switch (this) { 637 case EITHER: return "Either Specific or independent"; 638 case INDEPENDENT: return "Version independent"; 639 case SPECIFIC: return "Version Specific"; 640 case NULL: return null; 641 default: return "?"; 642 } 643 } 644 } 645 646 public static class ReferenceVersionRulesEnumFactory implements EnumFactory<ReferenceVersionRules> { 647 public ReferenceVersionRules fromCode(String codeString) throws IllegalArgumentException { 648 if (codeString == null || "".equals(codeString)) 649 if (codeString == null || "".equals(codeString)) 650 return null; 651 if ("either".equals(codeString)) 652 return ReferenceVersionRules.EITHER; 653 if ("independent".equals(codeString)) 654 return ReferenceVersionRules.INDEPENDENT; 655 if ("specific".equals(codeString)) 656 return ReferenceVersionRules.SPECIFIC; 657 throw new IllegalArgumentException("Unknown ReferenceVersionRules code '"+codeString+"'"); 658 } 659 public Enumeration<ReferenceVersionRules> fromType(PrimitiveType<?> code) throws FHIRException { 660 if (code == null) 661 return null; 662 if (code.isEmpty()) 663 return new Enumeration<ReferenceVersionRules>(this); 664 String codeString = code.asStringValue(); 665 if (codeString == null || "".equals(codeString)) 666 return null; 667 if ("either".equals(codeString)) 668 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.EITHER); 669 if ("independent".equals(codeString)) 670 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.INDEPENDENT); 671 if ("specific".equals(codeString)) 672 return new Enumeration<ReferenceVersionRules>(this, ReferenceVersionRules.SPECIFIC); 673 throw new FHIRException("Unknown ReferenceVersionRules code '"+codeString+"'"); 674 } 675 public String toCode(ReferenceVersionRules code) { 676 if (code == ReferenceVersionRules.NULL) 677 return null; 678 if (code == ReferenceVersionRules.EITHER) 679 return "either"; 680 if (code == ReferenceVersionRules.INDEPENDENT) 681 return "independent"; 682 if (code == ReferenceVersionRules.SPECIFIC) 683 return "specific"; 684 return "?"; 685 } 686 public String toSystem(ReferenceVersionRules code) { 687 return code.getSystem(); 688 } 689 } 690 691 public enum ConstraintSeverity { 692 /** 693 * If the constraint is violated, the resource is not conformant. 694 */ 695 ERROR, 696 /** 697 * If the constraint is violated, the resource is conformant, but it is not necessarily following best practice. 698 */ 699 WARNING, 700 /** 701 * added to help the parsers with the generic types 702 */ 703 NULL; 704 public static ConstraintSeverity fromCode(String codeString) throws FHIRException { 705 if (codeString == null || "".equals(codeString)) 706 return null; 707 if ("error".equals(codeString)) 708 return ERROR; 709 if ("warning".equals(codeString)) 710 return WARNING; 711 if (Configuration.isAcceptInvalidEnums()) 712 return null; 713 else 714 throw new FHIRException("Unknown ConstraintSeverity code '"+codeString+"'"); 715 } 716 public String toCode() { 717 switch (this) { 718 case ERROR: return "error"; 719 case WARNING: return "warning"; 720 case NULL: return null; 721 default: return "?"; 722 } 723 } 724 public String getSystem() { 725 switch (this) { 726 case ERROR: return "http://hl7.org/fhir/constraint-severity"; 727 case WARNING: return "http://hl7.org/fhir/constraint-severity"; 728 case NULL: return null; 729 default: return "?"; 730 } 731 } 732 public String getDefinition() { 733 switch (this) { 734 case ERROR: return "If the constraint is violated, the resource is not conformant."; 735 case WARNING: return "If the constraint is violated, the resource is conformant, but it is not necessarily following best practice."; 736 case NULL: return null; 737 default: return "?"; 738 } 739 } 740 public String getDisplay() { 741 switch (this) { 742 case ERROR: return "Error"; 743 case WARNING: return "Warning"; 744 case NULL: return null; 745 default: return "?"; 746 } 747 } 748 } 749 750 public static class ConstraintSeverityEnumFactory implements EnumFactory<ConstraintSeverity> { 751 public ConstraintSeverity fromCode(String codeString) throws IllegalArgumentException { 752 if (codeString == null || "".equals(codeString)) 753 if (codeString == null || "".equals(codeString)) 754 return null; 755 if ("error".equals(codeString)) 756 return ConstraintSeverity.ERROR; 757 if ("warning".equals(codeString)) 758 return ConstraintSeverity.WARNING; 759 throw new IllegalArgumentException("Unknown ConstraintSeverity code '"+codeString+"'"); 760 } 761 public Enumeration<ConstraintSeverity> fromType(PrimitiveType<?> code) throws FHIRException { 762 if (code == null) 763 return null; 764 if (code.isEmpty()) 765 return new Enumeration<ConstraintSeverity>(this); 766 String codeString = code.asStringValue(); 767 if (codeString == null || "".equals(codeString)) 768 return null; 769 if ("error".equals(codeString)) 770 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.ERROR); 771 if ("warning".equals(codeString)) 772 return new Enumeration<ConstraintSeverity>(this, ConstraintSeverity.WARNING); 773 throw new FHIRException("Unknown ConstraintSeverity code '"+codeString+"'"); 774 } 775 public String toCode(ConstraintSeverity code) { 776 if (code == ConstraintSeverity.NULL) 777 return null; 778 if (code == ConstraintSeverity.ERROR) 779 return "error"; 780 if (code == ConstraintSeverity.WARNING) 781 return "warning"; 782 return "?"; 783 } 784 public String toSystem(ConstraintSeverity code) { 785 return code.getSystem(); 786 } 787 } 788 789 @Block() 790 public static class ElementDefinitionSlicingComponent extends Element implements IBaseDatatypeElement { 791 /** 792 * Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices. 793 */ 794 @Child(name = "discriminator", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 795 @Description(shortDefinition="Element values that are used to distinguish the slices", formalDefinition="Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices." ) 796 protected List<ElementDefinitionSlicingDiscriminatorComponent> discriminator; 797 798 /** 799 * A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 800 */ 801 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 802 @Description(shortDefinition="Text description of how slicing works (or not)", formalDefinition="A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated." ) 803 protected StringType description; 804 805 /** 806 * If the matching elements have to occur in the same order as defined in the profile. 807 */ 808 @Child(name = "ordered", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 809 @Description(shortDefinition="If elements must be in same order as slices", formalDefinition="If the matching elements have to occur in the same order as defined in the profile." ) 810 protected BooleanType ordered; 811 812 /** 813 * Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 814 */ 815 @Child(name = "rules", type = {CodeType.class}, order=4, min=1, max=1, modifier=false, summary=true) 816 @Description(shortDefinition="closed | open | openAtEnd", formalDefinition="Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end." ) 817 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-slicing-rules") 818 protected Enumeration<SlicingRules> rules; 819 820 private static final long serialVersionUID = -311635839L; 821 822 /** 823 * Constructor 824 */ 825 public ElementDefinitionSlicingComponent() { 826 super(); 827 } 828 829 /** 830 * Constructor 831 */ 832 public ElementDefinitionSlicingComponent(Enumeration<SlicingRules> rules) { 833 super(); 834 this.rules = rules; 835 } 836 837 /** 838 * @return {@link #discriminator} (Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.) 839 */ 840 public List<ElementDefinitionSlicingDiscriminatorComponent> getDiscriminator() { 841 if (this.discriminator == null) 842 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 843 return this.discriminator; 844 } 845 846 /** 847 * @return Returns a reference to <code>this</code> for easy method chaining 848 */ 849 public ElementDefinitionSlicingComponent setDiscriminator(List<ElementDefinitionSlicingDiscriminatorComponent> theDiscriminator) { 850 this.discriminator = theDiscriminator; 851 return this; 852 } 853 854 public boolean hasDiscriminator() { 855 if (this.discriminator == null) 856 return false; 857 for (ElementDefinitionSlicingDiscriminatorComponent item : this.discriminator) 858 if (!item.isEmpty()) 859 return true; 860 return false; 861 } 862 863 public ElementDefinitionSlicingDiscriminatorComponent addDiscriminator() { //3 864 ElementDefinitionSlicingDiscriminatorComponent t = new ElementDefinitionSlicingDiscriminatorComponent(); 865 if (this.discriminator == null) 866 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 867 this.discriminator.add(t); 868 return t; 869 } 870 871 public ElementDefinitionSlicingComponent addDiscriminator(ElementDefinitionSlicingDiscriminatorComponent t) { //3 872 if (t == null) 873 return this; 874 if (this.discriminator == null) 875 this.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 876 this.discriminator.add(t); 877 return this; 878 } 879 880 /** 881 * @return The first repetition of repeating field {@link #discriminator}, creating it if it does not already exist 882 */ 883 public ElementDefinitionSlicingDiscriminatorComponent getDiscriminatorFirstRep() { 884 if (getDiscriminator().isEmpty()) { 885 addDiscriminator(); 886 } 887 return getDiscriminator().get(0); 888 } 889 890 /** 891 * @return {@link #description} (A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 892 */ 893 public StringType getDescriptionElement() { 894 if (this.description == null) 895 if (Configuration.errorOnAutoCreate()) 896 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.description"); 897 else if (Configuration.doAutoCreate()) 898 this.description = new StringType(); // bb 899 return this.description; 900 } 901 902 public boolean hasDescriptionElement() { 903 return this.description != null && !this.description.isEmpty(); 904 } 905 906 public boolean hasDescription() { 907 return this.description != null && !this.description.isEmpty(); 908 } 909 910 /** 911 * @param value {@link #description} (A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 912 */ 913 public ElementDefinitionSlicingComponent setDescriptionElement(StringType value) { 914 this.description = value; 915 return this; 916 } 917 918 /** 919 * @return A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 920 */ 921 public String getDescription() { 922 return this.description == null ? null : this.description.getValue(); 923 } 924 925 /** 926 * @param value A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated. 927 */ 928 public ElementDefinitionSlicingComponent setDescription(String value) { 929 if (Utilities.noString(value)) 930 this.description = null; 931 else { 932 if (this.description == null) 933 this.description = new StringType(); 934 this.description.setValue(value); 935 } 936 return this; 937 } 938 939 /** 940 * @return {@link #ordered} (If the matching elements have to occur in the same order as defined in the profile.). This is the underlying object with id, value and extensions. The accessor "getOrdered" gives direct access to the value 941 */ 942 public BooleanType getOrderedElement() { 943 if (this.ordered == null) 944 if (Configuration.errorOnAutoCreate()) 945 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.ordered"); 946 else if (Configuration.doAutoCreate()) 947 this.ordered = new BooleanType(); // bb 948 return this.ordered; 949 } 950 951 public boolean hasOrderedElement() { 952 return this.ordered != null && !this.ordered.isEmpty(); 953 } 954 955 public boolean hasOrdered() { 956 return this.ordered != null && !this.ordered.isEmpty(); 957 } 958 959 /** 960 * @param value {@link #ordered} (If the matching elements have to occur in the same order as defined in the profile.). This is the underlying object with id, value and extensions. The accessor "getOrdered" gives direct access to the value 961 */ 962 public ElementDefinitionSlicingComponent setOrderedElement(BooleanType value) { 963 this.ordered = value; 964 return this; 965 } 966 967 /** 968 * @return If the matching elements have to occur in the same order as defined in the profile. 969 */ 970 public boolean getOrdered() { 971 return this.ordered == null || this.ordered.isEmpty() ? false : this.ordered.getValue(); 972 } 973 974 /** 975 * @param value If the matching elements have to occur in the same order as defined in the profile. 976 */ 977 public ElementDefinitionSlicingComponent setOrdered(boolean value) { 978 if (this.ordered == null) 979 this.ordered = new BooleanType(); 980 this.ordered.setValue(value); 981 return this; 982 } 983 984 /** 985 * @return {@link #rules} (Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.). This is the underlying object with id, value and extensions. The accessor "getRules" gives direct access to the value 986 */ 987 public Enumeration<SlicingRules> getRulesElement() { 988 if (this.rules == null) 989 if (Configuration.errorOnAutoCreate()) 990 throw new Error("Attempt to auto-create ElementDefinitionSlicingComponent.rules"); 991 else if (Configuration.doAutoCreate()) 992 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); // bb 993 return this.rules; 994 } 995 996 public boolean hasRulesElement() { 997 return this.rules != null && !this.rules.isEmpty(); 998 } 999 1000 public boolean hasRules() { 1001 return this.rules != null && !this.rules.isEmpty(); 1002 } 1003 1004 /** 1005 * @param value {@link #rules} (Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.). This is the underlying object with id, value and extensions. The accessor "getRules" gives direct access to the value 1006 */ 1007 public ElementDefinitionSlicingComponent setRulesElement(Enumeration<SlicingRules> value) { 1008 this.rules = value; 1009 return this; 1010 } 1011 1012 /** 1013 * @return Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 1014 */ 1015 public SlicingRules getRules() { 1016 return this.rules == null ? null : this.rules.getValue(); 1017 } 1018 1019 /** 1020 * @param value Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end. 1021 */ 1022 public ElementDefinitionSlicingComponent setRules(SlicingRules value) { 1023 if (this.rules == null) 1024 this.rules = new Enumeration<SlicingRules>(new SlicingRulesEnumFactory()); 1025 this.rules.setValue(value); 1026 return this; 1027 } 1028 1029 protected void listChildren(List<Property> children) { 1030 super.listChildren(children); 1031 children.add(new Property("discriminator", "", "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 0, java.lang.Integer.MAX_VALUE, discriminator)); 1032 children.add(new Property("description", "string", "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 0, 1, description)); 1033 children.add(new Property("ordered", "boolean", "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered)); 1034 children.add(new Property("rules", "code", "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 0, 1, rules)); 1035 } 1036 1037 @Override 1038 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1039 switch (_hash) { 1040 case -1888270692: /*discriminator*/ return new Property("discriminator", "", "Designates which child elements are used to discriminate between the slices when processing an instance. If one or more discriminators are provided, the value of the child elements in the instance data SHALL completely distinguish which slice the element in the resource matches based on the allowed values for those elements in each of the slices.", 0, java.lang.Integer.MAX_VALUE, discriminator); 1041 case -1724546052: /*description*/ return new Property("description", "string", "A human-readable text description of how the slicing works. If there is no discriminator, this is required to be present to provide whatever information is possible about how the slices can be differentiated.", 0, 1, description); 1042 case -1207109523: /*ordered*/ return new Property("ordered", "boolean", "If the matching elements have to occur in the same order as defined in the profile.", 0, 1, ordered); 1043 case 108873975: /*rules*/ return new Property("rules", "code", "Whether additional slices are allowed or not. When the slices are ordered, profile authors can also say that additional slices are only allowed at the end.", 0, 1, rules); 1044 default: return super.getNamedProperty(_hash, _name, _checkValid); 1045 } 1046 1047 } 1048 1049 @Override 1050 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1051 switch (hash) { 1052 case -1888270692: /*discriminator*/ return this.discriminator == null ? new Base[0] : this.discriminator.toArray(new Base[this.discriminator.size()]); // ElementDefinitionSlicingDiscriminatorComponent 1053 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1054 case -1207109523: /*ordered*/ return this.ordered == null ? new Base[0] : new Base[] {this.ordered}; // BooleanType 1055 case 108873975: /*rules*/ return this.rules == null ? new Base[0] : new Base[] {this.rules}; // Enumeration<SlicingRules> 1056 default: return super.getProperty(hash, name, checkValid); 1057 } 1058 1059 } 1060 1061 @Override 1062 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1063 switch (hash) { 1064 case -1888270692: // discriminator 1065 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); // ElementDefinitionSlicingDiscriminatorComponent 1066 return value; 1067 case -1724546052: // description 1068 this.description = castToString(value); // StringType 1069 return value; 1070 case -1207109523: // ordered 1071 this.ordered = castToBoolean(value); // BooleanType 1072 return value; 1073 case 108873975: // rules 1074 value = new SlicingRulesEnumFactory().fromType(castToCode(value)); 1075 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1076 return value; 1077 default: return super.setProperty(hash, name, value); 1078 } 1079 1080 } 1081 1082 @Override 1083 public Base setProperty(String name, Base value) throws FHIRException { 1084 if (name.equals("discriminator")) { 1085 this.getDiscriminator().add((ElementDefinitionSlicingDiscriminatorComponent) value); 1086 } else if (name.equals("description")) { 1087 this.description = castToString(value); // StringType 1088 } else if (name.equals("ordered")) { 1089 this.ordered = castToBoolean(value); // BooleanType 1090 } else if (name.equals("rules")) { 1091 value = new SlicingRulesEnumFactory().fromType(castToCode(value)); 1092 this.rules = (Enumeration) value; // Enumeration<SlicingRules> 1093 } else 1094 return super.setProperty(name, value); 1095 return value; 1096 } 1097 1098 @Override 1099 public Base makeProperty(int hash, String name) throws FHIRException { 1100 switch (hash) { 1101 case -1888270692: return addDiscriminator(); 1102 case -1724546052: return getDescriptionElement(); 1103 case -1207109523: return getOrderedElement(); 1104 case 108873975: return getRulesElement(); 1105 default: return super.makeProperty(hash, name); 1106 } 1107 1108 } 1109 1110 @Override 1111 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1112 switch (hash) { 1113 case -1888270692: /*discriminator*/ return new String[] {}; 1114 case -1724546052: /*description*/ return new String[] {"string"}; 1115 case -1207109523: /*ordered*/ return new String[] {"boolean"}; 1116 case 108873975: /*rules*/ return new String[] {"code"}; 1117 default: return super.getTypesForProperty(hash, name); 1118 } 1119 1120 } 1121 1122 @Override 1123 public Base addChild(String name) throws FHIRException { 1124 if (name.equals("discriminator")) { 1125 return addDiscriminator(); 1126 } 1127 else if (name.equals("description")) { 1128 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.description"); 1129 } 1130 else if (name.equals("ordered")) { 1131 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.ordered"); 1132 } 1133 else if (name.equals("rules")) { 1134 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.rules"); 1135 } 1136 else 1137 return super.addChild(name); 1138 } 1139 1140 public ElementDefinitionSlicingComponent copy() { 1141 ElementDefinitionSlicingComponent dst = new ElementDefinitionSlicingComponent(); 1142 copyValues(dst); 1143 if (discriminator != null) { 1144 dst.discriminator = new ArrayList<ElementDefinitionSlicingDiscriminatorComponent>(); 1145 for (ElementDefinitionSlicingDiscriminatorComponent i : discriminator) 1146 dst.discriminator.add(i.copy()); 1147 }; 1148 dst.description = description == null ? null : description.copy(); 1149 dst.ordered = ordered == null ? null : ordered.copy(); 1150 dst.rules = rules == null ? null : rules.copy(); 1151 return dst; 1152 } 1153 1154 @Override 1155 public boolean equalsDeep(Base other_) { 1156 if (!super.equalsDeep(other_)) 1157 return false; 1158 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1159 return false; 1160 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1161 return compareDeep(discriminator, o.discriminator, true) && compareDeep(description, o.description, true) 1162 && compareDeep(ordered, o.ordered, true) && compareDeep(rules, o.rules, true); 1163 } 1164 1165 @Override 1166 public boolean equalsShallow(Base other_) { 1167 if (!super.equalsShallow(other_)) 1168 return false; 1169 if (!(other_ instanceof ElementDefinitionSlicingComponent)) 1170 return false; 1171 ElementDefinitionSlicingComponent o = (ElementDefinitionSlicingComponent) other_; 1172 return compareValues(description, o.description, true) && compareValues(ordered, o.ordered, true) && compareValues(rules, o.rules, true) 1173 ; 1174 } 1175 1176 public boolean isEmpty() { 1177 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(discriminator, description 1178 , ordered, rules); 1179 } 1180 1181 public String fhirType() { 1182 return "ElementDefinition.slicing"; 1183 1184 } 1185 1186 } 1187 1188 @Block() 1189 public static class ElementDefinitionSlicingDiscriminatorComponent extends Element implements IBaseDatatypeElement { 1190 /** 1191 * How the element value is interpreted when discrimination is evaluated. 1192 */ 1193 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1194 @Description(shortDefinition="value | exists | pattern | type | profile", formalDefinition="How the element value is interpreted when discrimination is evaluated." ) 1195 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/discriminator-type") 1196 protected Enumeration<DiscriminatorType> type; 1197 1198 /** 1199 * A FHIRPath expression, using a restricted subset of FHIRPath, that is used to identify the element on which discrimination is based. 1200 */ 1201 @Child(name = "path", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1202 @Description(shortDefinition="Path to element value", formalDefinition="A FHIRPath expression, using a restricted subset of FHIRPath, that is used to identify the element on which discrimination is based." ) 1203 protected StringType path; 1204 1205 private static final long serialVersionUID = 1151159293L; 1206 1207 /** 1208 * Constructor 1209 */ 1210 public ElementDefinitionSlicingDiscriminatorComponent() { 1211 super(); 1212 } 1213 1214 /** 1215 * Constructor 1216 */ 1217 public ElementDefinitionSlicingDiscriminatorComponent(Enumeration<DiscriminatorType> type, StringType path) { 1218 super(); 1219 this.type = type; 1220 this.path = path; 1221 } 1222 1223 /** 1224 * @return {@link #type} (How the element value is interpreted when discrimination is evaluated.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1225 */ 1226 public Enumeration<DiscriminatorType> getTypeElement() { 1227 if (this.type == null) 1228 if (Configuration.errorOnAutoCreate()) 1229 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.type"); 1230 else if (Configuration.doAutoCreate()) 1231 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); // bb 1232 return this.type; 1233 } 1234 1235 public boolean hasTypeElement() { 1236 return this.type != null && !this.type.isEmpty(); 1237 } 1238 1239 public boolean hasType() { 1240 return this.type != null && !this.type.isEmpty(); 1241 } 1242 1243 /** 1244 * @param value {@link #type} (How the element value is interpreted when discrimination is evaluated.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1245 */ 1246 public ElementDefinitionSlicingDiscriminatorComponent setTypeElement(Enumeration<DiscriminatorType> value) { 1247 this.type = value; 1248 return this; 1249 } 1250 1251 /** 1252 * @return How the element value is interpreted when discrimination is evaluated. 1253 */ 1254 public DiscriminatorType getType() { 1255 return this.type == null ? null : this.type.getValue(); 1256 } 1257 1258 /** 1259 * @param value How the element value is interpreted when discrimination is evaluated. 1260 */ 1261 public ElementDefinitionSlicingDiscriminatorComponent setType(DiscriminatorType value) { 1262 if (this.type == null) 1263 this.type = new Enumeration<DiscriminatorType>(new DiscriminatorTypeEnumFactory()); 1264 this.type.setValue(value); 1265 return this; 1266 } 1267 1268 /** 1269 * @return {@link #path} (A FHIRPath expression, using a restricted subset of FHIRPath, that is used to identify the element on which discrimination is based.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1270 */ 1271 public StringType getPathElement() { 1272 if (this.path == null) 1273 if (Configuration.errorOnAutoCreate()) 1274 throw new Error("Attempt to auto-create ElementDefinitionSlicingDiscriminatorComponent.path"); 1275 else if (Configuration.doAutoCreate()) 1276 this.path = new StringType(); // bb 1277 return this.path; 1278 } 1279 1280 public boolean hasPathElement() { 1281 return this.path != null && !this.path.isEmpty(); 1282 } 1283 1284 public boolean hasPath() { 1285 return this.path != null && !this.path.isEmpty(); 1286 } 1287 1288 /** 1289 * @param value {@link #path} (A FHIRPath expression, using a restricted subset of FHIRPath, that is used to identify the element on which discrimination is based.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1290 */ 1291 public ElementDefinitionSlicingDiscriminatorComponent setPathElement(StringType value) { 1292 this.path = value; 1293 return this; 1294 } 1295 1296 /** 1297 * @return A FHIRPath expression, using a restricted subset of FHIRPath, that is used to identify the element on which discrimination is based. 1298 */ 1299 public String getPath() { 1300 return this.path == null ? null : this.path.getValue(); 1301 } 1302 1303 /** 1304 * @param value A FHIRPath expression, using a restricted subset of FHIRPath, that is used to identify the element on which discrimination is based. 1305 */ 1306 public ElementDefinitionSlicingDiscriminatorComponent setPath(String value) { 1307 if (this.path == null) 1308 this.path = new StringType(); 1309 this.path.setValue(value); 1310 return this; 1311 } 1312 1313 protected void listChildren(List<Property> children) { 1314 super.listChildren(children); 1315 children.add(new Property("type", "code", "How the element value is interpreted when discrimination is evaluated.", 0, 1, type)); 1316 children.add(new Property("path", "string", "A FHIRPath expression, using a restricted subset of FHIRPath, that is used to identify the element on which discrimination is based.", 0, 1, path)); 1317 } 1318 1319 @Override 1320 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1321 switch (_hash) { 1322 case 3575610: /*type*/ return new Property("type", "code", "How the element value is interpreted when discrimination is evaluated.", 0, 1, type); 1323 case 3433509: /*path*/ return new Property("path", "string", "A FHIRPath expression, using a restricted subset of FHIRPath, that is used to identify the element on which discrimination is based.", 0, 1, path); 1324 default: return super.getNamedProperty(_hash, _name, _checkValid); 1325 } 1326 1327 } 1328 1329 @Override 1330 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1331 switch (hash) { 1332 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DiscriminatorType> 1333 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1334 default: return super.getProperty(hash, name, checkValid); 1335 } 1336 1337 } 1338 1339 @Override 1340 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1341 switch (hash) { 1342 case 3575610: // type 1343 value = new DiscriminatorTypeEnumFactory().fromType(castToCode(value)); 1344 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1345 return value; 1346 case 3433509: // path 1347 this.path = castToString(value); // StringType 1348 return value; 1349 default: return super.setProperty(hash, name, value); 1350 } 1351 1352 } 1353 1354 @Override 1355 public Base setProperty(String name, Base value) throws FHIRException { 1356 if (name.equals("type")) { 1357 value = new DiscriminatorTypeEnumFactory().fromType(castToCode(value)); 1358 this.type = (Enumeration) value; // Enumeration<DiscriminatorType> 1359 } else if (name.equals("path")) { 1360 this.path = castToString(value); // StringType 1361 } else 1362 return super.setProperty(name, value); 1363 return value; 1364 } 1365 1366 @Override 1367 public Base makeProperty(int hash, String name) throws FHIRException { 1368 switch (hash) { 1369 case 3575610: return getTypeElement(); 1370 case 3433509: return getPathElement(); 1371 default: return super.makeProperty(hash, name); 1372 } 1373 1374 } 1375 1376 @Override 1377 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1378 switch (hash) { 1379 case 3575610: /*type*/ return new String[] {"code"}; 1380 case 3433509: /*path*/ return new String[] {"string"}; 1381 default: return super.getTypesForProperty(hash, name); 1382 } 1383 1384 } 1385 1386 @Override 1387 public Base addChild(String name) throws FHIRException { 1388 if (name.equals("type")) { 1389 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.type"); 1390 } 1391 else if (name.equals("path")) { 1392 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 1393 } 1394 else 1395 return super.addChild(name); 1396 } 1397 1398 public ElementDefinitionSlicingDiscriminatorComponent copy() { 1399 ElementDefinitionSlicingDiscriminatorComponent dst = new ElementDefinitionSlicingDiscriminatorComponent(); 1400 copyValues(dst); 1401 dst.type = type == null ? null : type.copy(); 1402 dst.path = path == null ? null : path.copy(); 1403 return dst; 1404 } 1405 1406 @Override 1407 public boolean equalsDeep(Base other_) { 1408 if (!super.equalsDeep(other_)) 1409 return false; 1410 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1411 return false; 1412 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1413 return compareDeep(type, o.type, true) && compareDeep(path, o.path, true); 1414 } 1415 1416 @Override 1417 public boolean equalsShallow(Base other_) { 1418 if (!super.equalsShallow(other_)) 1419 return false; 1420 if (!(other_ instanceof ElementDefinitionSlicingDiscriminatorComponent)) 1421 return false; 1422 ElementDefinitionSlicingDiscriminatorComponent o = (ElementDefinitionSlicingDiscriminatorComponent) other_; 1423 return compareValues(type, o.type, true) && compareValues(path, o.path, true); 1424 } 1425 1426 public boolean isEmpty() { 1427 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, path); 1428 } 1429 1430 public String fhirType() { 1431 return "ElementDefinition.slicing.discriminator"; 1432 1433 } 1434 1435 } 1436 1437 @Block() 1438 public static class ElementDefinitionBaseComponent extends Element implements IBaseDatatypeElement { 1439 /** 1440 * The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base. 1441 */ 1442 @Child(name = "path", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1443 @Description(shortDefinition="Path that identifies the base element", formalDefinition="The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base." ) 1444 protected StringType path; 1445 1446 /** 1447 * Minimum cardinality of the base element identified by the path. 1448 */ 1449 @Child(name = "min", type = {UnsignedIntType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1450 @Description(shortDefinition="Min cardinality of the base element", formalDefinition="Minimum cardinality of the base element identified by the path." ) 1451 protected UnsignedIntType min; 1452 1453 /** 1454 * Maximum cardinality of the base element identified by the path. 1455 */ 1456 @Child(name = "max", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1457 @Description(shortDefinition="Max cardinality of the base element", formalDefinition="Maximum cardinality of the base element identified by the path." ) 1458 protected StringType max; 1459 1460 private static final long serialVersionUID = -1412704221L; 1461 1462 /** 1463 * Constructor 1464 */ 1465 public ElementDefinitionBaseComponent() { 1466 super(); 1467 } 1468 1469 /** 1470 * Constructor 1471 */ 1472 public ElementDefinitionBaseComponent(StringType path, UnsignedIntType min, StringType max) { 1473 super(); 1474 this.path = path; 1475 this.min = min; 1476 this.max = max; 1477 } 1478 1479 /** 1480 * @return {@link #path} (The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1481 */ 1482 public StringType getPathElement() { 1483 if (this.path == null) 1484 if (Configuration.errorOnAutoCreate()) 1485 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.path"); 1486 else if (Configuration.doAutoCreate()) 1487 this.path = new StringType(); // bb 1488 return this.path; 1489 } 1490 1491 public boolean hasPathElement() { 1492 return this.path != null && !this.path.isEmpty(); 1493 } 1494 1495 public boolean hasPath() { 1496 return this.path != null && !this.path.isEmpty(); 1497 } 1498 1499 /** 1500 * @param value {@link #path} (The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1501 */ 1502 public ElementDefinitionBaseComponent setPathElement(StringType value) { 1503 this.path = value; 1504 return this; 1505 } 1506 1507 /** 1508 * @return The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base. 1509 */ 1510 public String getPath() { 1511 return this.path == null ? null : this.path.getValue(); 1512 } 1513 1514 /** 1515 * @param value The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base. 1516 */ 1517 public ElementDefinitionBaseComponent setPath(String value) { 1518 if (this.path == null) 1519 this.path = new StringType(); 1520 this.path.setValue(value); 1521 return this; 1522 } 1523 1524 /** 1525 * @return {@link #min} (Minimum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 1526 */ 1527 public UnsignedIntType getMinElement() { 1528 if (this.min == null) 1529 if (Configuration.errorOnAutoCreate()) 1530 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.min"); 1531 else if (Configuration.doAutoCreate()) 1532 this.min = new UnsignedIntType(); // bb 1533 return this.min; 1534 } 1535 1536 public boolean hasMinElement() { 1537 return this.min != null && !this.min.isEmpty(); 1538 } 1539 1540 public boolean hasMin() { 1541 return this.min != null && !this.min.isEmpty(); 1542 } 1543 1544 /** 1545 * @param value {@link #min} (Minimum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 1546 */ 1547 public ElementDefinitionBaseComponent setMinElement(UnsignedIntType value) { 1548 this.min = value; 1549 return this; 1550 } 1551 1552 /** 1553 * @return Minimum cardinality of the base element identified by the path. 1554 */ 1555 public int getMin() { 1556 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 1557 } 1558 1559 /** 1560 * @param value Minimum cardinality of the base element identified by the path. 1561 */ 1562 public ElementDefinitionBaseComponent setMin(int value) { 1563 if (this.min == null) 1564 this.min = new UnsignedIntType(); 1565 this.min.setValue(value); 1566 return this; 1567 } 1568 1569 /** 1570 * @return {@link #max} (Maximum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 1571 */ 1572 public StringType getMaxElement() { 1573 if (this.max == null) 1574 if (Configuration.errorOnAutoCreate()) 1575 throw new Error("Attempt to auto-create ElementDefinitionBaseComponent.max"); 1576 else if (Configuration.doAutoCreate()) 1577 this.max = new StringType(); // bb 1578 return this.max; 1579 } 1580 1581 public boolean hasMaxElement() { 1582 return this.max != null && !this.max.isEmpty(); 1583 } 1584 1585 public boolean hasMax() { 1586 return this.max != null && !this.max.isEmpty(); 1587 } 1588 1589 /** 1590 * @param value {@link #max} (Maximum cardinality of the base element identified by the path.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 1591 */ 1592 public ElementDefinitionBaseComponent setMaxElement(StringType value) { 1593 this.max = value; 1594 return this; 1595 } 1596 1597 /** 1598 * @return Maximum cardinality of the base element identified by the path. 1599 */ 1600 public String getMax() { 1601 return this.max == null ? null : this.max.getValue(); 1602 } 1603 1604 /** 1605 * @param value Maximum cardinality of the base element identified by the path. 1606 */ 1607 public ElementDefinitionBaseComponent setMax(String value) { 1608 if (this.max == null) 1609 this.max = new StringType(); 1610 this.max.setValue(value); 1611 return this; 1612 } 1613 1614 protected void listChildren(List<Property> children) { 1615 super.listChildren(children); 1616 children.add(new Property("path", "string", "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.", 0, 1, path)); 1617 children.add(new Property("min", "unsignedInt", "Minimum cardinality of the base element identified by the path.", 0, 1, min)); 1618 children.add(new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 0, 1, max)); 1619 } 1620 1621 @Override 1622 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1623 switch (_hash) { 1624 case 3433509: /*path*/ return new Property("path", "string", "The Path that identifies the base element - this matches the ElementDefinition.path for that element. Across FHIR, there is only one base definition of any element - that is, an element definition on a [[[StructureDefinition]]] without a StructureDefinition.base.", 0, 1, path); 1625 case 108114: /*min*/ return new Property("min", "unsignedInt", "Minimum cardinality of the base element identified by the path.", 0, 1, min); 1626 case 107876: /*max*/ return new Property("max", "string", "Maximum cardinality of the base element identified by the path.", 0, 1, max); 1627 default: return super.getNamedProperty(_hash, _name, _checkValid); 1628 } 1629 1630 } 1631 1632 @Override 1633 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1634 switch (hash) { 1635 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1636 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 1637 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 1638 default: return super.getProperty(hash, name, checkValid); 1639 } 1640 1641 } 1642 1643 @Override 1644 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1645 switch (hash) { 1646 case 3433509: // path 1647 this.path = castToString(value); // StringType 1648 return value; 1649 case 108114: // min 1650 this.min = castToUnsignedInt(value); // UnsignedIntType 1651 return value; 1652 case 107876: // max 1653 this.max = castToString(value); // StringType 1654 return value; 1655 default: return super.setProperty(hash, name, value); 1656 } 1657 1658 } 1659 1660 @Override 1661 public Base setProperty(String name, Base value) throws FHIRException { 1662 if (name.equals("path")) { 1663 this.path = castToString(value); // StringType 1664 } else if (name.equals("min")) { 1665 this.min = castToUnsignedInt(value); // UnsignedIntType 1666 } else if (name.equals("max")) { 1667 this.max = castToString(value); // StringType 1668 } else 1669 return super.setProperty(name, value); 1670 return value; 1671 } 1672 1673 @Override 1674 public Base makeProperty(int hash, String name) throws FHIRException { 1675 switch (hash) { 1676 case 3433509: return getPathElement(); 1677 case 108114: return getMinElement(); 1678 case 107876: return getMaxElement(); 1679 default: return super.makeProperty(hash, name); 1680 } 1681 1682 } 1683 1684 @Override 1685 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1686 switch (hash) { 1687 case 3433509: /*path*/ return new String[] {"string"}; 1688 case 108114: /*min*/ return new String[] {"unsignedInt"}; 1689 case 107876: /*max*/ return new String[] {"string"}; 1690 default: return super.getTypesForProperty(hash, name); 1691 } 1692 1693 } 1694 1695 @Override 1696 public Base addChild(String name) throws FHIRException { 1697 if (name.equals("path")) { 1698 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 1699 } 1700 else if (name.equals("min")) { 1701 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 1702 } 1703 else if (name.equals("max")) { 1704 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 1705 } 1706 else 1707 return super.addChild(name); 1708 } 1709 1710 public ElementDefinitionBaseComponent copy() { 1711 ElementDefinitionBaseComponent dst = new ElementDefinitionBaseComponent(); 1712 copyValues(dst); 1713 dst.path = path == null ? null : path.copy(); 1714 dst.min = min == null ? null : min.copy(); 1715 dst.max = max == null ? null : max.copy(); 1716 return dst; 1717 } 1718 1719 @Override 1720 public boolean equalsDeep(Base other_) { 1721 if (!super.equalsDeep(other_)) 1722 return false; 1723 if (!(other_ instanceof ElementDefinitionBaseComponent)) 1724 return false; 1725 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 1726 return compareDeep(path, o.path, true) && compareDeep(min, o.min, true) && compareDeep(max, o.max, true) 1727 ; 1728 } 1729 1730 @Override 1731 public boolean equalsShallow(Base other_) { 1732 if (!super.equalsShallow(other_)) 1733 return false; 1734 if (!(other_ instanceof ElementDefinitionBaseComponent)) 1735 return false; 1736 ElementDefinitionBaseComponent o = (ElementDefinitionBaseComponent) other_; 1737 return compareValues(path, o.path, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 1738 ; 1739 } 1740 1741 public boolean isEmpty() { 1742 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, min, max); 1743 } 1744 1745 public String fhirType() { 1746 return "ElementDefinition.base"; 1747 1748 } 1749 1750 } 1751 1752 @Block() 1753 public static class TypeRefComponent extends Element implements IBaseDatatypeElement { 1754 /** 1755 * URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 1756 */ 1757 @Child(name = "code", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1758 @Description(shortDefinition="Data type or Resource (reference to definition)", formalDefinition="URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models." ) 1759 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/defined-types") 1760 protected UriType code; 1761 1762 /** 1763 * Identifies a profile structure or implementation Guide that SHALL hold for the datatype this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide. 1764 */ 1765 @Child(name = "profile", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1766 @Description(shortDefinition="Profile (StructureDefinition) to apply (or IG)", formalDefinition="Identifies a profile structure or implementation Guide that SHALL hold for the datatype this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide." ) 1767 protected UriType profile; 1768 1769 /** 1770 * Identifies a profile structure or implementation Guide that SHALL hold for the target of the reference this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide. 1771 */ 1772 @Child(name = "targetProfile", type = {UriType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1773 @Description(shortDefinition="Profile (StructureDefinition) to apply to reference target (or IG)", formalDefinition="Identifies a profile structure or implementation Guide that SHALL hold for the target of the reference this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide." ) 1774 protected UriType targetProfile; 1775 1776 /** 1777 * If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle. 1778 */ 1779 @Child(name = "aggregation", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1780 @Description(shortDefinition="contained | referenced | bundled - how aggregated", formalDefinition="If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle." ) 1781 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-aggregation-mode") 1782 protected List<Enumeration<AggregationMode>> aggregation; 1783 1784 /** 1785 * Whether this reference needs to be version specific or version independent, or whether either can be used. 1786 */ 1787 @Child(name = "versioning", type = {CodeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1788 @Description(shortDefinition="either | independent | specific", formalDefinition="Whether this reference needs to be version specific or version independent, or whether either can be used." ) 1789 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reference-version-rules") 1790 protected Enumeration<ReferenceVersionRules> versioning; 1791 1792 private static final long serialVersionUID = -560921355L; 1793 1794 /** 1795 * Constructor 1796 */ 1797 public TypeRefComponent() { 1798 super(); 1799 } 1800 1801 /** 1802 * Constructor 1803 */ 1804 public TypeRefComponent(UriType code) { 1805 super(); 1806 this.code = code; 1807 } 1808 1809 /** 1810 * @return {@link #code} (URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1811 */ 1812 public UriType getCodeElement() { 1813 if (this.code == null) 1814 if (Configuration.errorOnAutoCreate()) 1815 throw new Error("Attempt to auto-create TypeRefComponent.code"); 1816 else if (Configuration.doAutoCreate()) 1817 this.code = new UriType(); // bb 1818 return this.code; 1819 } 1820 1821 public boolean hasCodeElement() { 1822 return this.code != null && !this.code.isEmpty(); 1823 } 1824 1825 public boolean hasCode() { 1826 return this.code != null && !this.code.isEmpty(); 1827 } 1828 1829 /** 1830 * @param value {@link #code} (URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1831 */ 1832 public TypeRefComponent setCodeElement(UriType value) { 1833 this.code = value; 1834 return this; 1835 } 1836 1837 /** 1838 * @return URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 1839 */ 1840 public String getCode() { 1841 return this.code == null ? null : this.code.getValue(); 1842 } 1843 1844 /** 1845 * @param value URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. "string" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models. 1846 */ 1847 public TypeRefComponent setCode(String value) { 1848 if (this.code == null) 1849 this.code = new UriType(); 1850 this.code.setValue(value); 1851 return this; 1852 } 1853 1854 /** 1855 * @return {@link #profile} (Identifies a profile structure or implementation Guide that SHALL hold for the datatype this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 1856 */ 1857 public UriType getProfileElement() { 1858 if (this.profile == null) 1859 if (Configuration.errorOnAutoCreate()) 1860 throw new Error("Attempt to auto-create TypeRefComponent.profile"); 1861 else if (Configuration.doAutoCreate()) 1862 this.profile = new UriType(); // bb 1863 return this.profile; 1864 } 1865 1866 public boolean hasProfileElement() { 1867 return this.profile != null && !this.profile.isEmpty(); 1868 } 1869 1870 public boolean hasProfile() { 1871 return this.profile != null && !this.profile.isEmpty(); 1872 } 1873 1874 /** 1875 * @param value {@link #profile} (Identifies a profile structure or implementation Guide that SHALL hold for the datatype this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 1876 */ 1877 public TypeRefComponent setProfileElement(UriType value) { 1878 this.profile = value; 1879 return this; 1880 } 1881 1882 /** 1883 * @return Identifies a profile structure or implementation Guide that SHALL hold for the datatype this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide. 1884 */ 1885 public String getProfile() { 1886 return this.profile == null ? null : this.profile.getValue(); 1887 } 1888 1889 /** 1890 * @param value Identifies a profile structure or implementation Guide that SHALL hold for the datatype this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide. 1891 */ 1892 public TypeRefComponent setProfile(String value) { 1893 if (Utilities.noString(value)) 1894 this.profile = null; 1895 else { 1896 if (this.profile == null) 1897 this.profile = new UriType(); 1898 this.profile.setValue(value); 1899 } 1900 return this; 1901 } 1902 1903 /** 1904 * @return {@link #targetProfile} (Identifies a profile structure or implementation Guide that SHALL hold for the target of the reference this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getTargetProfile" gives direct access to the value 1905 */ 1906 public UriType getTargetProfileElement() { 1907 if (this.targetProfile == null) 1908 if (Configuration.errorOnAutoCreate()) 1909 throw new Error("Attempt to auto-create TypeRefComponent.targetProfile"); 1910 else if (Configuration.doAutoCreate()) 1911 this.targetProfile = new UriType(); // bb 1912 return this.targetProfile; 1913 } 1914 1915 public boolean hasTargetProfileElement() { 1916 return this.targetProfile != null && !this.targetProfile.isEmpty(); 1917 } 1918 1919 public boolean hasTargetProfile() { 1920 return this.targetProfile != null && !this.targetProfile.isEmpty(); 1921 } 1922 1923 /** 1924 * @param value {@link #targetProfile} (Identifies a profile structure or implementation Guide that SHALL hold for the target of the reference this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getTargetProfile" gives direct access to the value 1925 */ 1926 public TypeRefComponent setTargetProfileElement(UriType value) { 1927 this.targetProfile = value; 1928 return this; 1929 } 1930 1931 /** 1932 * @return Identifies a profile structure or implementation Guide that SHALL hold for the target of the reference this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide. 1933 */ 1934 public String getTargetProfile() { 1935 return this.targetProfile == null ? null : this.targetProfile.getValue(); 1936 } 1937 1938 /** 1939 * @param value Identifies a profile structure or implementation Guide that SHALL hold for the target of the reference this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide. 1940 */ 1941 public TypeRefComponent setTargetProfile(String value) { 1942 if (Utilities.noString(value)) 1943 this.targetProfile = null; 1944 else { 1945 if (this.targetProfile == null) 1946 this.targetProfile = new UriType(); 1947 this.targetProfile.setValue(value); 1948 } 1949 return this; 1950 } 1951 1952 /** 1953 * @return {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 1954 */ 1955 public List<Enumeration<AggregationMode>> getAggregation() { 1956 if (this.aggregation == null) 1957 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 1958 return this.aggregation; 1959 } 1960 1961 /** 1962 * @return Returns a reference to <code>this</code> for easy method chaining 1963 */ 1964 public TypeRefComponent setAggregation(List<Enumeration<AggregationMode>> theAggregation) { 1965 this.aggregation = theAggregation; 1966 return this; 1967 } 1968 1969 public boolean hasAggregation() { 1970 if (this.aggregation == null) 1971 return false; 1972 for (Enumeration<AggregationMode> item : this.aggregation) 1973 if (!item.isEmpty()) 1974 return true; 1975 return false; 1976 } 1977 1978 /** 1979 * @return {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 1980 */ 1981 public Enumeration<AggregationMode> addAggregationElement() {//2 1982 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 1983 if (this.aggregation == null) 1984 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 1985 this.aggregation.add(t); 1986 return t; 1987 } 1988 1989 /** 1990 * @param value {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 1991 */ 1992 public TypeRefComponent addAggregation(AggregationMode value) { //1 1993 Enumeration<AggregationMode> t = new Enumeration<AggregationMode>(new AggregationModeEnumFactory()); 1994 t.setValue(value); 1995 if (this.aggregation == null) 1996 this.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 1997 this.aggregation.add(t); 1998 return this; 1999 } 2000 2001 /** 2002 * @param value {@link #aggregation} (If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.) 2003 */ 2004 public boolean hasAggregation(AggregationMode value) { 2005 if (this.aggregation == null) 2006 return false; 2007 for (Enumeration<AggregationMode> v : this.aggregation) 2008 if (v.getValue().equals(value)) // code 2009 return true; 2010 return false; 2011 } 2012 2013 /** 2014 * @return {@link #versioning} (Whether this reference needs to be version specific or version independent, or whether either can be used.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 2015 */ 2016 public Enumeration<ReferenceVersionRules> getVersioningElement() { 2017 if (this.versioning == null) 2018 if (Configuration.errorOnAutoCreate()) 2019 throw new Error("Attempt to auto-create TypeRefComponent.versioning"); 2020 else if (Configuration.doAutoCreate()) 2021 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); // bb 2022 return this.versioning; 2023 } 2024 2025 public boolean hasVersioningElement() { 2026 return this.versioning != null && !this.versioning.isEmpty(); 2027 } 2028 2029 public boolean hasVersioning() { 2030 return this.versioning != null && !this.versioning.isEmpty(); 2031 } 2032 2033 /** 2034 * @param value {@link #versioning} (Whether this reference needs to be version specific or version independent, or whether either can be used.). This is the underlying object with id, value and extensions. The accessor "getVersioning" gives direct access to the value 2035 */ 2036 public TypeRefComponent setVersioningElement(Enumeration<ReferenceVersionRules> value) { 2037 this.versioning = value; 2038 return this; 2039 } 2040 2041 /** 2042 * @return Whether this reference needs to be version specific or version independent, or whether either can be used. 2043 */ 2044 public ReferenceVersionRules getVersioning() { 2045 return this.versioning == null ? null : this.versioning.getValue(); 2046 } 2047 2048 /** 2049 * @param value Whether this reference needs to be version specific or version independent, or whether either can be used. 2050 */ 2051 public TypeRefComponent setVersioning(ReferenceVersionRules value) { 2052 if (value == null) 2053 this.versioning = null; 2054 else { 2055 if (this.versioning == null) 2056 this.versioning = new Enumeration<ReferenceVersionRules>(new ReferenceVersionRulesEnumFactory()); 2057 this.versioning.setValue(value); 2058 } 2059 return this; 2060 } 2061 2062 protected void listChildren(List<Property> children) { 2063 super.listChildren(children); 2064 children.add(new Property("code", "uri", "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 0, 1, code)); 2065 children.add(new Property("profile", "uri", "Identifies a profile structure or implementation Guide that SHALL hold for the datatype this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.", 0, 1, profile)); 2066 children.add(new Property("targetProfile", "uri", "Identifies a profile structure or implementation Guide that SHALL hold for the target of the reference this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.", 0, 1, targetProfile)); 2067 children.add(new Property("aggregation", "code", "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 0, java.lang.Integer.MAX_VALUE, aggregation)); 2068 children.add(new Property("versioning", "code", "Whether this reference needs to be version specific or version independent, or whether either can be used.", 0, 1, versioning)); 2069 } 2070 2071 @Override 2072 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2073 switch (_hash) { 2074 case 3059181: /*code*/ return new Property("code", "uri", "URL of Data type or Resource that is a(or the) type used for this element. References are URLs that are relative to http://hl7.org/fhir/StructureDefinition e.g. \"string\" is a reference to http://hl7.org/fhir/StructureDefinition/string. Absolute URLs are only allowed in logical models.", 0, 1, code); 2075 case -309425751: /*profile*/ return new Property("profile", "uri", "Identifies a profile structure or implementation Guide that SHALL hold for the datatype this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.", 0, 1, profile); 2076 case 1994521304: /*targetProfile*/ return new Property("targetProfile", "uri", "Identifies a profile structure or implementation Guide that SHALL hold for the target of the reference this element refers to. Can be a local reference - to a contained StructureDefinition, or a reference to another StructureDefinition or Implementation Guide by a canonical URL. When an implementation guide is specified, the resource SHALL conform to at least one profile defined in the implementation guide.", 0, 1, targetProfile); 2077 case 841524962: /*aggregation*/ return new Property("aggregation", "code", "If the type is a reference to another resource, how the resource is or can be aggregated - is it a contained resource, or a reference, and if the context is a bundle, is it included in the bundle.", 0, java.lang.Integer.MAX_VALUE, aggregation); 2078 case -670487542: /*versioning*/ return new Property("versioning", "code", "Whether this reference needs to be version specific or version independent, or whether either can be used.", 0, 1, versioning); 2079 default: return super.getNamedProperty(_hash, _name, _checkValid); 2080 } 2081 2082 } 2083 2084 @Override 2085 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2086 switch (hash) { 2087 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // UriType 2088 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // UriType 2089 case 1994521304: /*targetProfile*/ return this.targetProfile == null ? new Base[0] : new Base[] {this.targetProfile}; // UriType 2090 case 841524962: /*aggregation*/ return this.aggregation == null ? new Base[0] : this.aggregation.toArray(new Base[this.aggregation.size()]); // Enumeration<AggregationMode> 2091 case -670487542: /*versioning*/ return this.versioning == null ? new Base[0] : new Base[] {this.versioning}; // Enumeration<ReferenceVersionRules> 2092 default: return super.getProperty(hash, name, checkValid); 2093 } 2094 2095 } 2096 2097 @Override 2098 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2099 switch (hash) { 2100 case 3059181: // code 2101 this.code = castToUri(value); // UriType 2102 return value; 2103 case -309425751: // profile 2104 this.profile = castToUri(value); // UriType 2105 return value; 2106 case 1994521304: // targetProfile 2107 this.targetProfile = castToUri(value); // UriType 2108 return value; 2109 case 841524962: // aggregation 2110 value = new AggregationModeEnumFactory().fromType(castToCode(value)); 2111 this.getAggregation().add((Enumeration) value); // Enumeration<AggregationMode> 2112 return value; 2113 case -670487542: // versioning 2114 value = new ReferenceVersionRulesEnumFactory().fromType(castToCode(value)); 2115 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2116 return value; 2117 default: return super.setProperty(hash, name, value); 2118 } 2119 2120 } 2121 2122 @Override 2123 public Base setProperty(String name, Base value) throws FHIRException { 2124 if (name.equals("code")) { 2125 this.code = castToUri(value); // UriType 2126 } else if (name.equals("profile")) { 2127 this.profile = castToUri(value); // UriType 2128 } else if (name.equals("targetProfile")) { 2129 this.targetProfile = castToUri(value); // UriType 2130 } else if (name.equals("aggregation")) { 2131 value = new AggregationModeEnumFactory().fromType(castToCode(value)); 2132 this.getAggregation().add((Enumeration) value); 2133 } else if (name.equals("versioning")) { 2134 value = new ReferenceVersionRulesEnumFactory().fromType(castToCode(value)); 2135 this.versioning = (Enumeration) value; // Enumeration<ReferenceVersionRules> 2136 } else 2137 return super.setProperty(name, value); 2138 return value; 2139 } 2140 2141 @Override 2142 public Base makeProperty(int hash, String name) throws FHIRException { 2143 switch (hash) { 2144 case 3059181: return getCodeElement(); 2145 case -309425751: return getProfileElement(); 2146 case 1994521304: return getTargetProfileElement(); 2147 case 841524962: return addAggregationElement(); 2148 case -670487542: return getVersioningElement(); 2149 default: return super.makeProperty(hash, name); 2150 } 2151 2152 } 2153 2154 @Override 2155 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2156 switch (hash) { 2157 case 3059181: /*code*/ return new String[] {"uri"}; 2158 case -309425751: /*profile*/ return new String[] {"uri"}; 2159 case 1994521304: /*targetProfile*/ return new String[] {"uri"}; 2160 case 841524962: /*aggregation*/ return new String[] {"code"}; 2161 case -670487542: /*versioning*/ return new String[] {"code"}; 2162 default: return super.getTypesForProperty(hash, name); 2163 } 2164 2165 } 2166 2167 @Override 2168 public Base addChild(String name) throws FHIRException { 2169 if (name.equals("code")) { 2170 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.code"); 2171 } 2172 else if (name.equals("profile")) { 2173 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.profile"); 2174 } 2175 else if (name.equals("targetProfile")) { 2176 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.targetProfile"); 2177 } 2178 else if (name.equals("aggregation")) { 2179 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.aggregation"); 2180 } 2181 else if (name.equals("versioning")) { 2182 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.versioning"); 2183 } 2184 else 2185 return super.addChild(name); 2186 } 2187 2188 public TypeRefComponent copy() { 2189 TypeRefComponent dst = new TypeRefComponent(); 2190 copyValues(dst); 2191 dst.code = code == null ? null : code.copy(); 2192 dst.profile = profile == null ? null : profile.copy(); 2193 dst.targetProfile = targetProfile == null ? null : targetProfile.copy(); 2194 if (aggregation != null) { 2195 dst.aggregation = new ArrayList<Enumeration<AggregationMode>>(); 2196 for (Enumeration<AggregationMode> i : aggregation) 2197 dst.aggregation.add(i.copy()); 2198 }; 2199 dst.versioning = versioning == null ? null : versioning.copy(); 2200 return dst; 2201 } 2202 2203 @Override 2204 public boolean equalsDeep(Base other_) { 2205 if (!super.equalsDeep(other_)) 2206 return false; 2207 if (!(other_ instanceof TypeRefComponent)) 2208 return false; 2209 TypeRefComponent o = (TypeRefComponent) other_; 2210 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) && compareDeep(targetProfile, o.targetProfile, true) 2211 && compareDeep(aggregation, o.aggregation, true) && compareDeep(versioning, o.versioning, true) 2212 ; 2213 } 2214 2215 @Override 2216 public boolean equalsShallow(Base other_) { 2217 if (!super.equalsShallow(other_)) 2218 return false; 2219 if (!(other_ instanceof TypeRefComponent)) 2220 return false; 2221 TypeRefComponent o = (TypeRefComponent) other_; 2222 return compareValues(code, o.code, true) && compareValues(profile, o.profile, true) && compareValues(targetProfile, o.targetProfile, true) 2223 && compareValues(aggregation, o.aggregation, true) && compareValues(versioning, o.versioning, true) 2224 ; 2225 } 2226 2227 public boolean isEmpty() { 2228 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, targetProfile 2229 , aggregation, versioning); 2230 } 2231 2232 public String fhirType() { 2233 return "ElementDefinition.type"; 2234 2235 } 2236 2237 public boolean hasTarget() { 2238 return Utilities.existsInList(getCode(), "Reference"); 2239 2240 } 2241 2242 } 2243 2244 @Block() 2245 public static class ElementDefinitionExampleComponent extends Element implements IBaseDatatypeElement { 2246 /** 2247 * Describes the purpose of this example amoung the set of examples. 2248 */ 2249 @Child(name = "label", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2250 @Description(shortDefinition="Describes the purpose of this example", formalDefinition="Describes the purpose of this example amoung the set of examples." ) 2251 protected StringType label; 2252 2253 /** 2254 * The actual value for the element, which must be one of the types allowed for this element. 2255 */ 2256 @Child(name = "value", type = {}, order=2, min=1, max=1, modifier=false, summary=true) 2257 @Description(shortDefinition="Value of Example (one of allowed types)", formalDefinition="The actual value for the element, which must be one of the types allowed for this element." ) 2258 protected org.hl7.fhir.dstu3.model.Type value; 2259 2260 private static final long serialVersionUID = 1346348024L; 2261 2262 /** 2263 * Constructor 2264 */ 2265 public ElementDefinitionExampleComponent() { 2266 super(); 2267 } 2268 2269 /** 2270 * Constructor 2271 */ 2272 public ElementDefinitionExampleComponent(StringType label, org.hl7.fhir.dstu3.model.Type value) { 2273 super(); 2274 this.label = label; 2275 this.value = value; 2276 } 2277 2278 /** 2279 * @return {@link #label} (Describes the purpose of this example amoung the set of examples.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2280 */ 2281 public StringType getLabelElement() { 2282 if (this.label == null) 2283 if (Configuration.errorOnAutoCreate()) 2284 throw new Error("Attempt to auto-create ElementDefinitionExampleComponent.label"); 2285 else if (Configuration.doAutoCreate()) 2286 this.label = new StringType(); // bb 2287 return this.label; 2288 } 2289 2290 public boolean hasLabelElement() { 2291 return this.label != null && !this.label.isEmpty(); 2292 } 2293 2294 public boolean hasLabel() { 2295 return this.label != null && !this.label.isEmpty(); 2296 } 2297 2298 /** 2299 * @param value {@link #label} (Describes the purpose of this example amoung the set of examples.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 2300 */ 2301 public ElementDefinitionExampleComponent setLabelElement(StringType value) { 2302 this.label = value; 2303 return this; 2304 } 2305 2306 /** 2307 * @return Describes the purpose of this example amoung the set of examples. 2308 */ 2309 public String getLabel() { 2310 return this.label == null ? null : this.label.getValue(); 2311 } 2312 2313 /** 2314 * @param value Describes the purpose of this example amoung the set of examples. 2315 */ 2316 public ElementDefinitionExampleComponent setLabel(String value) { 2317 if (this.label == null) 2318 this.label = new StringType(); 2319 this.label.setValue(value); 2320 return this; 2321 } 2322 2323 /** 2324 * @return {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2325 */ 2326 public org.hl7.fhir.dstu3.model.Type getValue() { 2327 return this.value; 2328 } 2329 2330 public boolean hasValue() { 2331 return this.value != null && !this.value.isEmpty(); 2332 } 2333 2334 /** 2335 * @param value {@link #value} (The actual value for the element, which must be one of the types allowed for this element.) 2336 */ 2337 public ElementDefinitionExampleComponent setValue(org.hl7.fhir.dstu3.model.Type value) { 2338 this.value = value; 2339 return this; 2340 } 2341 2342 protected void listChildren(List<Property> children) { 2343 super.listChildren(children); 2344 children.add(new Property("label", "string", "Describes the purpose of this example amoung the set of examples.", 0, 1, label)); 2345 children.add(new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value)); 2346 } 2347 2348 @Override 2349 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2350 switch (_hash) { 2351 case 102727412: /*label*/ return new Property("label", "string", "Describes the purpose of this example amoung the set of examples.", 0, 1, label); 2352 case -1410166417: /*value[x]*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2353 case 111972721: /*value*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2354 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2355 case 733421943: /*valueBoolean*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2356 case -786218365: /*valueCanonical*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2357 case -766209282: /*valueCode*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2358 case -766192449: /*valueDate*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2359 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2360 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2361 case 231604844: /*valueId*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2362 case -1668687056: /*valueInstant*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2363 case -1668204915: /*valueInteger*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2364 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2365 case -1410178407: /*valueOid*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2366 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2367 case -1424603934: /*valueString*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2368 case -765708322: /*valueTime*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2369 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2370 case -1410172357: /*valueUri*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2371 case -1410172354: /*valueUrl*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2372 case -765667124: /*valueUuid*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2373 case -478981821: /*valueAddress*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2374 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2375 case -475566732: /*valueAttachment*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2376 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2377 case -1887705029: /*valueCoding*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2378 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2379 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2380 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2381 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2382 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2383 case 2030761548: /*valueRange*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2384 case 2030767386: /*valueRatio*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2385 case 1755241690: /*valueReference*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2386 case -962229101: /*valueSampledData*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2387 case -540985785: /*valueSignature*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2388 case -1406282469: /*valueTiming*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2389 case -1858636920: /*valueDosage*/ return new Property("value[x]", "*", "The actual value for the element, which must be one of the types allowed for this element.", 0, 1, value); 2390 default: return super.getNamedProperty(_hash, _name, _checkValid); 2391 } 2392 2393 } 2394 2395 @Override 2396 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2397 switch (hash) { 2398 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 2399 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // org.hl7.fhir.dstu3.model.Type 2400 default: return super.getProperty(hash, name, checkValid); 2401 } 2402 2403 } 2404 2405 @Override 2406 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2407 switch (hash) { 2408 case 102727412: // label 2409 this.label = castToString(value); // StringType 2410 return value; 2411 case 111972721: // value 2412 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 2413 return value; 2414 default: return super.setProperty(hash, name, value); 2415 } 2416 2417 } 2418 2419 @Override 2420 public Base setProperty(String name, Base value) throws FHIRException { 2421 if (name.equals("label")) { 2422 this.label = castToString(value); // StringType 2423 } else if (name.equals("value[x]")) { 2424 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 2425 } else 2426 return super.setProperty(name, value); 2427 return value; 2428 } 2429 2430 @Override 2431 public Base makeProperty(int hash, String name) throws FHIRException { 2432 switch (hash) { 2433 case 102727412: return getLabelElement(); 2434 case -1410166417: return getValue(); 2435 case 111972721: return getValue(); 2436 default: return super.makeProperty(hash, name); 2437 } 2438 2439 } 2440 2441 @Override 2442 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2443 switch (hash) { 2444 case 102727412: /*label*/ return new String[] {"string"}; 2445 case 111972721: /*value*/ return new String[] {"*"}; 2446 default: return super.getTypesForProperty(hash, name); 2447 } 2448 2449 } 2450 2451 @Override 2452 public Base addChild(String name) throws FHIRException { 2453 if (name.equals("label")) { 2454 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 2455 } 2456 else if (name.equals("valueBoolean")) { 2457 this.value = new BooleanType(); 2458 return this.value; 2459 } 2460 else if (name.equals("valueInteger")) { 2461 this.value = new IntegerType(); 2462 return this.value; 2463 } 2464 else if (name.equals("valueDecimal")) { 2465 this.value = new DecimalType(); 2466 return this.value; 2467 } 2468 else if (name.equals("valueBase64Binary")) { 2469 this.value = new Base64BinaryType(); 2470 return this.value; 2471 } 2472 else if (name.equals("valueInstant")) { 2473 this.value = new InstantType(); 2474 return this.value; 2475 } 2476 else if (name.equals("valueString")) { 2477 this.value = new StringType(); 2478 return this.value; 2479 } 2480 else if (name.equals("valueUri")) { 2481 this.value = new UriType(); 2482 return this.value; 2483 } 2484 else if (name.equals("valueDate")) { 2485 this.value = new DateType(); 2486 return this.value; 2487 } 2488 else if (name.equals("valueDateTime")) { 2489 this.value = new DateTimeType(); 2490 return this.value; 2491 } 2492 else if (name.equals("valueTime")) { 2493 this.value = new TimeType(); 2494 return this.value; 2495 } 2496 else if (name.equals("valueCode")) { 2497 this.value = new CodeType(); 2498 return this.value; 2499 } 2500 else if (name.equals("valueOid")) { 2501 this.value = new OidType(); 2502 return this.value; 2503 } 2504 else if (name.equals("valueId")) { 2505 this.value = new IdType(); 2506 return this.value; 2507 } 2508 else if (name.equals("valueUnsignedInt")) { 2509 this.value = new UnsignedIntType(); 2510 return this.value; 2511 } 2512 else if (name.equals("valuePositiveInt")) { 2513 this.value = new PositiveIntType(); 2514 return this.value; 2515 } 2516 else if (name.equals("valueMarkdown")) { 2517 this.value = new MarkdownType(); 2518 return this.value; 2519 } 2520 else if (name.equals("valueAnnotation")) { 2521 this.value = new Annotation(); 2522 return this.value; 2523 } 2524 else if (name.equals("valueAttachment")) { 2525 this.value = new Attachment(); 2526 return this.value; 2527 } 2528 else if (name.equals("valueIdentifier")) { 2529 this.value = new Identifier(); 2530 return this.value; 2531 } 2532 else if (name.equals("valueCodeableConcept")) { 2533 this.value = new CodeableConcept(); 2534 return this.value; 2535 } 2536 else if (name.equals("valueCoding")) { 2537 this.value = new Coding(); 2538 return this.value; 2539 } 2540 else if (name.equals("valueQuantity")) { 2541 this.value = new Quantity(); 2542 return this.value; 2543 } 2544 else if (name.equals("valueRange")) { 2545 this.value = new Range(); 2546 return this.value; 2547 } 2548 else if (name.equals("valuePeriod")) { 2549 this.value = new Period(); 2550 return this.value; 2551 } 2552 else if (name.equals("valueRatio")) { 2553 this.value = new Ratio(); 2554 return this.value; 2555 } 2556 else if (name.equals("valueSampledData")) { 2557 this.value = new SampledData(); 2558 return this.value; 2559 } 2560 else if (name.equals("valueSignature")) { 2561 this.value = new Signature(); 2562 return this.value; 2563 } 2564 else if (name.equals("valueHumanName")) { 2565 this.value = new HumanName(); 2566 return this.value; 2567 } 2568 else if (name.equals("valueAddress")) { 2569 this.value = new Address(); 2570 return this.value; 2571 } 2572 else if (name.equals("valueContactPoint")) { 2573 this.value = new ContactPoint(); 2574 return this.value; 2575 } 2576 else if (name.equals("valueTiming")) { 2577 this.value = new Timing(); 2578 return this.value; 2579 } 2580 else if (name.equals("valueReference")) { 2581 this.value = new Reference(); 2582 return this.value; 2583 } 2584 else if (name.equals("valueMeta")) { 2585 this.value = new Meta(); 2586 return this.value; 2587 } 2588 else 2589 return super.addChild(name); 2590 } 2591 2592 public ElementDefinitionExampleComponent copy() { 2593 ElementDefinitionExampleComponent dst = new ElementDefinitionExampleComponent(); 2594 copyValues(dst); 2595 dst.label = label == null ? null : label.copy(); 2596 dst.value = value == null ? null : value.copy(); 2597 return dst; 2598 } 2599 2600 @Override 2601 public boolean equalsDeep(Base other_) { 2602 if (!super.equalsDeep(other_)) 2603 return false; 2604 if (!(other_ instanceof ElementDefinitionExampleComponent)) 2605 return false; 2606 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 2607 return compareDeep(label, o.label, true) && compareDeep(value, o.value, true); 2608 } 2609 2610 @Override 2611 public boolean equalsShallow(Base other_) { 2612 if (!super.equalsShallow(other_)) 2613 return false; 2614 if (!(other_ instanceof ElementDefinitionExampleComponent)) 2615 return false; 2616 ElementDefinitionExampleComponent o = (ElementDefinitionExampleComponent) other_; 2617 return compareValues(label, o.label, true); 2618 } 2619 2620 public boolean isEmpty() { 2621 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(label, value); 2622 } 2623 2624 public String fhirType() { 2625 return "ElementDefinition.example"; 2626 2627 } 2628 2629 } 2630 2631 @Block() 2632 public static class ElementDefinitionConstraintComponent extends Element implements IBaseDatatypeElement { 2633 /** 2634 * Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 2635 */ 2636 @Child(name = "key", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 2637 @Description(shortDefinition="Target of 'condition' reference above", formalDefinition="Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality." ) 2638 protected IdType key; 2639 2640 /** 2641 * Description of why this constraint is necessary or appropriate. 2642 */ 2643 @Child(name = "requirements", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 2644 @Description(shortDefinition="Why this constraint is necessary or appropriate", formalDefinition="Description of why this constraint is necessary or appropriate." ) 2645 protected StringType requirements; 2646 2647 /** 2648 * Identifies the impact constraint violation has on the conformance of the instance. 2649 */ 2650 @Child(name = "severity", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 2651 @Description(shortDefinition="error | warning", formalDefinition="Identifies the impact constraint violation has on the conformance of the instance." ) 2652 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/constraint-severity") 2653 protected Enumeration<ConstraintSeverity> severity; 2654 2655 /** 2656 * Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 2657 */ 2658 @Child(name = "human", type = {StringType.class}, order=4, min=1, max=1, modifier=false, summary=true) 2659 @Description(shortDefinition="Human description of constraint", formalDefinition="Text that can be used to describe the constraint in messages identifying that the constraint has been violated." ) 2660 protected StringType human; 2661 2662 /** 2663 * A [FHIRPath](http://hl7.org/fluentpath) expression of constraint that can be executed to see if this constraint is met. 2664 */ 2665 @Child(name = "expression", type = {StringType.class}, order=5, min=1, max=1, modifier=false, summary=true) 2666 @Description(shortDefinition="FHIRPath expression of constraint", formalDefinition="A [FHIRPath](http://hl7.org/fluentpath) expression of constraint that can be executed to see if this constraint is met." ) 2667 protected StringType expression; 2668 2669 /** 2670 * An XPath expression of constraint that can be executed to see if this constraint is met. 2671 */ 2672 @Child(name = "xpath", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 2673 @Description(shortDefinition="XPath expression of constraint", formalDefinition="An XPath expression of constraint that can be executed to see if this constraint is met." ) 2674 protected StringType xpath; 2675 2676 /** 2677 * A reference to the original source of the constraint, for traceability purposes. 2678 */ 2679 @Child(name = "source", type = {UriType.class}, order=7, min=0, max=1, modifier=false, summary=true) 2680 @Description(shortDefinition="Reference to original source of constraint", formalDefinition="A reference to the original source of the constraint, for traceability purposes." ) 2681 protected UriType source; 2682 2683 private static final long serialVersionUID = 1860862205L; 2684 2685 /** 2686 * Constructor 2687 */ 2688 public ElementDefinitionConstraintComponent() { 2689 super(); 2690 } 2691 2692 /** 2693 * Constructor 2694 */ 2695 public ElementDefinitionConstraintComponent(IdType key, Enumeration<ConstraintSeverity> severity, StringType human, StringType expression) { 2696 super(); 2697 this.key = key; 2698 this.severity = severity; 2699 this.human = human; 2700 this.expression = expression; 2701 } 2702 2703 /** 2704 * @return {@link #key} (Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 2705 */ 2706 public IdType getKeyElement() { 2707 if (this.key == null) 2708 if (Configuration.errorOnAutoCreate()) 2709 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.key"); 2710 else if (Configuration.doAutoCreate()) 2711 this.key = new IdType(); // bb 2712 return this.key; 2713 } 2714 2715 public boolean hasKeyElement() { 2716 return this.key != null && !this.key.isEmpty(); 2717 } 2718 2719 public boolean hasKey() { 2720 return this.key != null && !this.key.isEmpty(); 2721 } 2722 2723 /** 2724 * @param value {@link #key} (Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.). This is the underlying object with id, value and extensions. The accessor "getKey" gives direct access to the value 2725 */ 2726 public ElementDefinitionConstraintComponent setKeyElement(IdType value) { 2727 this.key = value; 2728 return this; 2729 } 2730 2731 /** 2732 * @return Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 2733 */ 2734 public String getKey() { 2735 return this.key == null ? null : this.key.getValue(); 2736 } 2737 2738 /** 2739 * @param value Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality. 2740 */ 2741 public ElementDefinitionConstraintComponent setKey(String value) { 2742 if (this.key == null) 2743 this.key = new IdType(); 2744 this.key.setValue(value); 2745 return this; 2746 } 2747 2748 /** 2749 * @return {@link #requirements} (Description of why this constraint is necessary or appropriate.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 2750 */ 2751 public StringType getRequirementsElement() { 2752 if (this.requirements == null) 2753 if (Configuration.errorOnAutoCreate()) 2754 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.requirements"); 2755 else if (Configuration.doAutoCreate()) 2756 this.requirements = new StringType(); // bb 2757 return this.requirements; 2758 } 2759 2760 public boolean hasRequirementsElement() { 2761 return this.requirements != null && !this.requirements.isEmpty(); 2762 } 2763 2764 public boolean hasRequirements() { 2765 return this.requirements != null && !this.requirements.isEmpty(); 2766 } 2767 2768 /** 2769 * @param value {@link #requirements} (Description of why this constraint is necessary or appropriate.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 2770 */ 2771 public ElementDefinitionConstraintComponent setRequirementsElement(StringType value) { 2772 this.requirements = value; 2773 return this; 2774 } 2775 2776 /** 2777 * @return Description of why this constraint is necessary or appropriate. 2778 */ 2779 public String getRequirements() { 2780 return this.requirements == null ? null : this.requirements.getValue(); 2781 } 2782 2783 /** 2784 * @param value Description of why this constraint is necessary or appropriate. 2785 */ 2786 public ElementDefinitionConstraintComponent setRequirements(String value) { 2787 if (Utilities.noString(value)) 2788 this.requirements = null; 2789 else { 2790 if (this.requirements == null) 2791 this.requirements = new StringType(); 2792 this.requirements.setValue(value); 2793 } 2794 return this; 2795 } 2796 2797 /** 2798 * @return {@link #severity} (Identifies the impact constraint violation has on the conformance of the instance.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 2799 */ 2800 public Enumeration<ConstraintSeverity> getSeverityElement() { 2801 if (this.severity == null) 2802 if (Configuration.errorOnAutoCreate()) 2803 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.severity"); 2804 else if (Configuration.doAutoCreate()) 2805 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); // bb 2806 return this.severity; 2807 } 2808 2809 public boolean hasSeverityElement() { 2810 return this.severity != null && !this.severity.isEmpty(); 2811 } 2812 2813 public boolean hasSeverity() { 2814 return this.severity != null && !this.severity.isEmpty(); 2815 } 2816 2817 /** 2818 * @param value {@link #severity} (Identifies the impact constraint violation has on the conformance of the instance.). This is the underlying object with id, value and extensions. The accessor "getSeverity" gives direct access to the value 2819 */ 2820 public ElementDefinitionConstraintComponent setSeverityElement(Enumeration<ConstraintSeverity> value) { 2821 this.severity = value; 2822 return this; 2823 } 2824 2825 /** 2826 * @return Identifies the impact constraint violation has on the conformance of the instance. 2827 */ 2828 public ConstraintSeverity getSeverity() { 2829 return this.severity == null ? null : this.severity.getValue(); 2830 } 2831 2832 /** 2833 * @param value Identifies the impact constraint violation has on the conformance of the instance. 2834 */ 2835 public ElementDefinitionConstraintComponent setSeverity(ConstraintSeverity value) { 2836 if (this.severity == null) 2837 this.severity = new Enumeration<ConstraintSeverity>(new ConstraintSeverityEnumFactory()); 2838 this.severity.setValue(value); 2839 return this; 2840 } 2841 2842 /** 2843 * @return {@link #human} (Text that can be used to describe the constraint in messages identifying that the constraint has been violated.). This is the underlying object with id, value and extensions. The accessor "getHuman" gives direct access to the value 2844 */ 2845 public StringType getHumanElement() { 2846 if (this.human == null) 2847 if (Configuration.errorOnAutoCreate()) 2848 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.human"); 2849 else if (Configuration.doAutoCreate()) 2850 this.human = new StringType(); // bb 2851 return this.human; 2852 } 2853 2854 public boolean hasHumanElement() { 2855 return this.human != null && !this.human.isEmpty(); 2856 } 2857 2858 public boolean hasHuman() { 2859 return this.human != null && !this.human.isEmpty(); 2860 } 2861 2862 /** 2863 * @param value {@link #human} (Text that can be used to describe the constraint in messages identifying that the constraint has been violated.). This is the underlying object with id, value and extensions. The accessor "getHuman" gives direct access to the value 2864 */ 2865 public ElementDefinitionConstraintComponent setHumanElement(StringType value) { 2866 this.human = value; 2867 return this; 2868 } 2869 2870 /** 2871 * @return Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 2872 */ 2873 public String getHuman() { 2874 return this.human == null ? null : this.human.getValue(); 2875 } 2876 2877 /** 2878 * @param value Text that can be used to describe the constraint in messages identifying that the constraint has been violated. 2879 */ 2880 public ElementDefinitionConstraintComponent setHuman(String value) { 2881 if (this.human == null) 2882 this.human = new StringType(); 2883 this.human.setValue(value); 2884 return this; 2885 } 2886 2887 /** 2888 * @return {@link #expression} (A [FHIRPath](http://hl7.org/fluentpath) expression of constraint that can be executed to see if this constraint is met.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 2889 */ 2890 public StringType getExpressionElement() { 2891 if (this.expression == null) 2892 if (Configuration.errorOnAutoCreate()) 2893 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.expression"); 2894 else if (Configuration.doAutoCreate()) 2895 this.expression = new StringType(); // bb 2896 return this.expression; 2897 } 2898 2899 public boolean hasExpressionElement() { 2900 return this.expression != null && !this.expression.isEmpty(); 2901 } 2902 2903 public boolean hasExpression() { 2904 return this.expression != null && !this.expression.isEmpty(); 2905 } 2906 2907 /** 2908 * @param value {@link #expression} (A [FHIRPath](http://hl7.org/fluentpath) expression of constraint that can be executed to see if this constraint is met.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 2909 */ 2910 public ElementDefinitionConstraintComponent setExpressionElement(StringType value) { 2911 this.expression = value; 2912 return this; 2913 } 2914 2915 /** 2916 * @return A [FHIRPath](http://hl7.org/fluentpath) expression of constraint that can be executed to see if this constraint is met. 2917 */ 2918 public String getExpression() { 2919 return this.expression == null ? null : this.expression.getValue(); 2920 } 2921 2922 /** 2923 * @param value A [FHIRPath](http://hl7.org/fluentpath) expression of constraint that can be executed to see if this constraint is met. 2924 */ 2925 public ElementDefinitionConstraintComponent setExpression(String value) { 2926 if (this.expression == null) 2927 this.expression = new StringType(); 2928 this.expression.setValue(value); 2929 return this; 2930 } 2931 2932 /** 2933 * @return {@link #xpath} (An XPath expression of constraint that can be executed to see if this constraint is met.). This is the underlying object with id, value and extensions. The accessor "getXpath" gives direct access to the value 2934 */ 2935 public StringType getXpathElement() { 2936 if (this.xpath == null) 2937 if (Configuration.errorOnAutoCreate()) 2938 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.xpath"); 2939 else if (Configuration.doAutoCreate()) 2940 this.xpath = new StringType(); // bb 2941 return this.xpath; 2942 } 2943 2944 public boolean hasXpathElement() { 2945 return this.xpath != null && !this.xpath.isEmpty(); 2946 } 2947 2948 public boolean hasXpath() { 2949 return this.xpath != null && !this.xpath.isEmpty(); 2950 } 2951 2952 /** 2953 * @param value {@link #xpath} (An XPath expression of constraint that can be executed to see if this constraint is met.). This is the underlying object with id, value and extensions. The accessor "getXpath" gives direct access to the value 2954 */ 2955 public ElementDefinitionConstraintComponent setXpathElement(StringType value) { 2956 this.xpath = value; 2957 return this; 2958 } 2959 2960 /** 2961 * @return An XPath expression of constraint that can be executed to see if this constraint is met. 2962 */ 2963 public String getXpath() { 2964 return this.xpath == null ? null : this.xpath.getValue(); 2965 } 2966 2967 /** 2968 * @param value An XPath expression of constraint that can be executed to see if this constraint is met. 2969 */ 2970 public ElementDefinitionConstraintComponent setXpath(String value) { 2971 if (Utilities.noString(value)) 2972 this.xpath = null; 2973 else { 2974 if (this.xpath == null) 2975 this.xpath = new StringType(); 2976 this.xpath.setValue(value); 2977 } 2978 return this; 2979 } 2980 2981 /** 2982 * @return {@link #source} (A reference to the original source of the constraint, for traceability purposes.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 2983 */ 2984 public UriType getSourceElement() { 2985 if (this.source == null) 2986 if (Configuration.errorOnAutoCreate()) 2987 throw new Error("Attempt to auto-create ElementDefinitionConstraintComponent.source"); 2988 else if (Configuration.doAutoCreate()) 2989 this.source = new UriType(); // bb 2990 return this.source; 2991 } 2992 2993 public boolean hasSourceElement() { 2994 return this.source != null && !this.source.isEmpty(); 2995 } 2996 2997 public boolean hasSource() { 2998 return this.source != null && !this.source.isEmpty(); 2999 } 3000 3001 /** 3002 * @param value {@link #source} (A reference to the original source of the constraint, for traceability purposes.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 3003 */ 3004 public ElementDefinitionConstraintComponent setSourceElement(UriType value) { 3005 this.source = value; 3006 return this; 3007 } 3008 3009 /** 3010 * @return A reference to the original source of the constraint, for traceability purposes. 3011 */ 3012 public String getSource() { 3013 return this.source == null ? null : this.source.getValue(); 3014 } 3015 3016 /** 3017 * @param value A reference to the original source of the constraint, for traceability purposes. 3018 */ 3019 public ElementDefinitionConstraintComponent setSource(String value) { 3020 if (Utilities.noString(value)) 3021 this.source = null; 3022 else { 3023 if (this.source == null) 3024 this.source = new UriType(); 3025 this.source.setValue(value); 3026 } 3027 return this; 3028 } 3029 3030 protected void listChildren(List<Property> children) { 3031 super.listChildren(children); 3032 children.add(new Property("key", "id", "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 0, 1, key)); 3033 children.add(new Property("requirements", "string", "Description of why this constraint is necessary or appropriate.", 0, 1, requirements)); 3034 children.add(new Property("severity", "code", "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity)); 3035 children.add(new Property("human", "string", "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 0, 1, human)); 3036 children.add(new Property("expression", "string", "A [FHIRPath](http://hl7.org/fluentpath) expression of constraint that can be executed to see if this constraint is met.", 0, 1, expression)); 3037 children.add(new Property("xpath", "string", "An XPath expression of constraint that can be executed to see if this constraint is met.", 0, 1, xpath)); 3038 children.add(new Property("source", "uri", "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source)); 3039 } 3040 3041 @Override 3042 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3043 switch (_hash) { 3044 case 106079: /*key*/ return new Property("key", "id", "Allows identification of which elements have their cardinalities impacted by the constraint. Will not be referenced for constraints that do not affect cardinality.", 0, 1, key); 3045 case -1619874672: /*requirements*/ return new Property("requirements", "string", "Description of why this constraint is necessary or appropriate.", 0, 1, requirements); 3046 case 1478300413: /*severity*/ return new Property("severity", "code", "Identifies the impact constraint violation has on the conformance of the instance.", 0, 1, severity); 3047 case 99639597: /*human*/ return new Property("human", "string", "Text that can be used to describe the constraint in messages identifying that the constraint has been violated.", 0, 1, human); 3048 case -1795452264: /*expression*/ return new Property("expression", "string", "A [FHIRPath](http://hl7.org/fluentpath) expression of constraint that can be executed to see if this constraint is met.", 0, 1, expression); 3049 case 114256029: /*xpath*/ return new Property("xpath", "string", "An XPath expression of constraint that can be executed to see if this constraint is met.", 0, 1, xpath); 3050 case -896505829: /*source*/ return new Property("source", "uri", "A reference to the original source of the constraint, for traceability purposes.", 0, 1, source); 3051 default: return super.getNamedProperty(_hash, _name, _checkValid); 3052 } 3053 3054 } 3055 3056 @Override 3057 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3058 switch (hash) { 3059 case 106079: /*key*/ return this.key == null ? new Base[0] : new Base[] {this.key}; // IdType 3060 case -1619874672: /*requirements*/ return this.requirements == null ? new Base[0] : new Base[] {this.requirements}; // StringType 3061 case 1478300413: /*severity*/ return this.severity == null ? new Base[0] : new Base[] {this.severity}; // Enumeration<ConstraintSeverity> 3062 case 99639597: /*human*/ return this.human == null ? new Base[0] : new Base[] {this.human}; // StringType 3063 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 3064 case 114256029: /*xpath*/ return this.xpath == null ? new Base[0] : new Base[] {this.xpath}; // StringType 3065 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // UriType 3066 default: return super.getProperty(hash, name, checkValid); 3067 } 3068 3069 } 3070 3071 @Override 3072 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3073 switch (hash) { 3074 case 106079: // key 3075 this.key = castToId(value); // IdType 3076 return value; 3077 case -1619874672: // requirements 3078 this.requirements = castToString(value); // StringType 3079 return value; 3080 case 1478300413: // severity 3081 value = new ConstraintSeverityEnumFactory().fromType(castToCode(value)); 3082 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 3083 return value; 3084 case 99639597: // human 3085 this.human = castToString(value); // StringType 3086 return value; 3087 case -1795452264: // expression 3088 this.expression = castToString(value); // StringType 3089 return value; 3090 case 114256029: // xpath 3091 this.xpath = castToString(value); // StringType 3092 return value; 3093 case -896505829: // source 3094 this.source = castToUri(value); // UriType 3095 return value; 3096 default: return super.setProperty(hash, name, value); 3097 } 3098 3099 } 3100 3101 @Override 3102 public Base setProperty(String name, Base value) throws FHIRException { 3103 if (name.equals("key")) { 3104 this.key = castToId(value); // IdType 3105 } else if (name.equals("requirements")) { 3106 this.requirements = castToString(value); // StringType 3107 } else if (name.equals("severity")) { 3108 value = new ConstraintSeverityEnumFactory().fromType(castToCode(value)); 3109 this.severity = (Enumeration) value; // Enumeration<ConstraintSeverity> 3110 } else if (name.equals("human")) { 3111 this.human = castToString(value); // StringType 3112 } else if (name.equals("expression")) { 3113 this.expression = castToString(value); // StringType 3114 } else if (name.equals("xpath")) { 3115 this.xpath = castToString(value); // StringType 3116 } else if (name.equals("source")) { 3117 this.source = castToUri(value); // UriType 3118 } else 3119 return super.setProperty(name, value); 3120 return value; 3121 } 3122 3123 @Override 3124 public Base makeProperty(int hash, String name) throws FHIRException { 3125 switch (hash) { 3126 case 106079: return getKeyElement(); 3127 case -1619874672: return getRequirementsElement(); 3128 case 1478300413: return getSeverityElement(); 3129 case 99639597: return getHumanElement(); 3130 case -1795452264: return getExpressionElement(); 3131 case 114256029: return getXpathElement(); 3132 case -896505829: return getSourceElement(); 3133 default: return super.makeProperty(hash, name); 3134 } 3135 3136 } 3137 3138 @Override 3139 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3140 switch (hash) { 3141 case 106079: /*key*/ return new String[] {"id"}; 3142 case -1619874672: /*requirements*/ return new String[] {"string"}; 3143 case 1478300413: /*severity*/ return new String[] {"code"}; 3144 case 99639597: /*human*/ return new String[] {"string"}; 3145 case -1795452264: /*expression*/ return new String[] {"string"}; 3146 case 114256029: /*xpath*/ return new String[] {"string"}; 3147 case -896505829: /*source*/ return new String[] {"uri"}; 3148 default: return super.getTypesForProperty(hash, name); 3149 } 3150 3151 } 3152 3153 @Override 3154 public Base addChild(String name) throws FHIRException { 3155 if (name.equals("key")) { 3156 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.key"); 3157 } 3158 else if (name.equals("requirements")) { 3159 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 3160 } 3161 else if (name.equals("severity")) { 3162 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.severity"); 3163 } 3164 else if (name.equals("human")) { 3165 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.human"); 3166 } 3167 else if (name.equals("expression")) { 3168 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.expression"); 3169 } 3170 else if (name.equals("xpath")) { 3171 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.xpath"); 3172 } 3173 else if (name.equals("source")) { 3174 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.source"); 3175 } 3176 else 3177 return super.addChild(name); 3178 } 3179 3180 public ElementDefinitionConstraintComponent copy() { 3181 ElementDefinitionConstraintComponent dst = new ElementDefinitionConstraintComponent(); 3182 copyValues(dst); 3183 dst.key = key == null ? null : key.copy(); 3184 dst.requirements = requirements == null ? null : requirements.copy(); 3185 dst.severity = severity == null ? null : severity.copy(); 3186 dst.human = human == null ? null : human.copy(); 3187 dst.expression = expression == null ? null : expression.copy(); 3188 dst.xpath = xpath == null ? null : xpath.copy(); 3189 dst.source = source == null ? null : source.copy(); 3190 return dst; 3191 } 3192 3193 @Override 3194 public boolean equalsDeep(Base other_) { 3195 if (!super.equalsDeep(other_)) 3196 return false; 3197 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 3198 return false; 3199 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 3200 return compareDeep(key, o.key, true) && compareDeep(requirements, o.requirements, true) && compareDeep(severity, o.severity, true) 3201 && compareDeep(human, o.human, true) && compareDeep(expression, o.expression, true) && compareDeep(xpath, o.xpath, true) 3202 && compareDeep(source, o.source, true); 3203 } 3204 3205 @Override 3206 public boolean equalsShallow(Base other_) { 3207 if (!super.equalsShallow(other_)) 3208 return false; 3209 if (!(other_ instanceof ElementDefinitionConstraintComponent)) 3210 return false; 3211 ElementDefinitionConstraintComponent o = (ElementDefinitionConstraintComponent) other_; 3212 return compareValues(key, o.key, true) && compareValues(requirements, o.requirements, true) && compareValues(severity, o.severity, true) 3213 && compareValues(human, o.human, true) && compareValues(expression, o.expression, true) && compareValues(xpath, o.xpath, true) 3214 && compareValues(source, o.source, true); 3215 } 3216 3217 public boolean isEmpty() { 3218 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(key, requirements, severity 3219 , human, expression, xpath, source); 3220 } 3221 3222 public String fhirType() { 3223 return "ElementDefinition.constraint"; 3224 3225 } 3226 3227 } 3228 3229 @Block() 3230 public static class ElementDefinitionBindingComponent extends Element implements IBaseDatatypeElement { 3231 /** 3232 * Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 3233 */ 3234 @Child(name = "strength", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3235 @Description(shortDefinition="required | extensible | preferred | example", formalDefinition="Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances." ) 3236 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/binding-strength") 3237 protected Enumeration<BindingStrength> strength; 3238 3239 /** 3240 * Describes the intended use of this particular set of codes. 3241 */ 3242 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3243 @Description(shortDefinition="Human explanation of the value set", formalDefinition="Describes the intended use of this particular set of codes." ) 3244 protected StringType description; 3245 3246 /** 3247 * Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri. 3248 */ 3249 @Child(name = "valueSet", type = {UriType.class, ValueSet.class}, order=3, min=0, max=1, modifier=false, summary=true) 3250 @Description(shortDefinition="Source of value set", formalDefinition="Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri." ) 3251 protected Type valueSet; 3252 3253 private static final long serialVersionUID = 1355538460L; 3254 3255 /** 3256 * Constructor 3257 */ 3258 public ElementDefinitionBindingComponent() { 3259 super(); 3260 } 3261 3262 /** 3263 * Constructor 3264 */ 3265 public ElementDefinitionBindingComponent(Enumeration<BindingStrength> strength) { 3266 super(); 3267 this.strength = strength; 3268 } 3269 3270 /** 3271 * @return {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 3272 */ 3273 public Enumeration<BindingStrength> getStrengthElement() { 3274 if (this.strength == null) 3275 if (Configuration.errorOnAutoCreate()) 3276 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.strength"); 3277 else if (Configuration.doAutoCreate()) 3278 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); // bb 3279 return this.strength; 3280 } 3281 3282 public boolean hasStrengthElement() { 3283 return this.strength != null && !this.strength.isEmpty(); 3284 } 3285 3286 public boolean hasStrength() { 3287 return this.strength != null && !this.strength.isEmpty(); 3288 } 3289 3290 /** 3291 * @param value {@link #strength} (Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.). This is the underlying object with id, value and extensions. The accessor "getStrength" gives direct access to the value 3292 */ 3293 public ElementDefinitionBindingComponent setStrengthElement(Enumeration<BindingStrength> value) { 3294 this.strength = value; 3295 return this; 3296 } 3297 3298 /** 3299 * @return Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 3300 */ 3301 public BindingStrength getStrength() { 3302 return this.strength == null ? null : this.strength.getValue(); 3303 } 3304 3305 /** 3306 * @param value Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances. 3307 */ 3308 public ElementDefinitionBindingComponent setStrength(BindingStrength value) { 3309 if (this.strength == null) 3310 this.strength = new Enumeration<BindingStrength>(new BindingStrengthEnumFactory()); 3311 this.strength.setValue(value); 3312 return this; 3313 } 3314 3315 /** 3316 * @return {@link #description} (Describes the intended use of this particular set of codes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3317 */ 3318 public StringType getDescriptionElement() { 3319 if (this.description == null) 3320 if (Configuration.errorOnAutoCreate()) 3321 throw new Error("Attempt to auto-create ElementDefinitionBindingComponent.description"); 3322 else if (Configuration.doAutoCreate()) 3323 this.description = new StringType(); // bb 3324 return this.description; 3325 } 3326 3327 public boolean hasDescriptionElement() { 3328 return this.description != null && !this.description.isEmpty(); 3329 } 3330 3331 public boolean hasDescription() { 3332 return this.description != null && !this.description.isEmpty(); 3333 } 3334 3335 /** 3336 * @param value {@link #description} (Describes the intended use of this particular set of codes.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 3337 */ 3338 public ElementDefinitionBindingComponent setDescriptionElement(StringType value) { 3339 this.description = value; 3340 return this; 3341 } 3342 3343 /** 3344 * @return Describes the intended use of this particular set of codes. 3345 */ 3346 public String getDescription() { 3347 return this.description == null ? null : this.description.getValue(); 3348 } 3349 3350 /** 3351 * @param value Describes the intended use of this particular set of codes. 3352 */ 3353 public ElementDefinitionBindingComponent setDescription(String value) { 3354 if (Utilities.noString(value)) 3355 this.description = null; 3356 else { 3357 if (this.description == null) 3358 this.description = new StringType(); 3359 this.description.setValue(value); 3360 } 3361 return this; 3362 } 3363 3364 /** 3365 * @return {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.) 3366 */ 3367 public Type getValueSet() { 3368 return this.valueSet; 3369 } 3370 3371 /** 3372 * @return {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.) 3373 */ 3374 public UriType getValueSetUriType() throws FHIRException { 3375 if (this.valueSet == null) 3376 return null; 3377 if (!(this.valueSet instanceof UriType)) 3378 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.valueSet.getClass().getName()+" was encountered"); 3379 return (UriType) this.valueSet; 3380 } 3381 3382 public boolean hasValueSetUriType() { 3383 return this != null && this.valueSet instanceof UriType; 3384 } 3385 3386 /** 3387 * @return {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.) 3388 */ 3389 public Reference getValueSetReference() throws FHIRException { 3390 if (this.valueSet == null) 3391 return null; 3392 if (!(this.valueSet instanceof Reference)) 3393 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.valueSet.getClass().getName()+" was encountered"); 3394 return (Reference) this.valueSet; 3395 } 3396 3397 public boolean hasValueSetReference() { 3398 return this != null && this.valueSet instanceof Reference; 3399 } 3400 3401 public boolean hasValueSet() { 3402 return this.valueSet != null && !this.valueSet.isEmpty(); 3403 } 3404 3405 /** 3406 * @param value {@link #valueSet} (Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.) 3407 */ 3408 public ElementDefinitionBindingComponent setValueSet(Type value) throws FHIRFormatError { 3409 if (value != null && !(value instanceof UriType || value instanceof Reference)) 3410 throw new FHIRFormatError("Not the right type for ElementDefinition.binding.valueSet[x]: "+value.fhirType()); 3411 this.valueSet = value; 3412 return this; 3413 } 3414 3415 protected void listChildren(List<Property> children) { 3416 super.listChildren(children); 3417 children.add(new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength)); 3418 children.add(new Property("description", "string", "Describes the intended use of this particular set of codes.", 0, 1, description)); 3419 children.add(new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.", 0, 1, valueSet)); 3420 } 3421 3422 @Override 3423 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3424 switch (_hash) { 3425 case 1791316033: /*strength*/ return new Property("strength", "code", "Indicates the degree of conformance expectations associated with this binding - that is, the degree to which the provided value set must be adhered to in the instances.", 0, 1, strength); 3426 case -1724546052: /*description*/ return new Property("description", "string", "Describes the intended use of this particular set of codes.", 0, 1, description); 3427 case -1438410321: /*valueSet[x]*/ return new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.", 0, 1, valueSet); 3428 case -1410174671: /*valueSet*/ return new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.", 0, 1, valueSet); 3429 case -1438416261: /*valueSetUri*/ return new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.", 0, 1, valueSet); 3430 case 295220506: /*valueSetReference*/ return new Property("valueSet[x]", "uri|Reference(ValueSet)", "Points to the value set or external definition (e.g. implicit value set) that identifies the set of codes to be used. If the binding refers to an explicit value set - the normal case - then use a Reference(ValueSet) preferably containing the canonical URL for the value set. If the reference is to an implicit value set - usually, an IETF RFC that defines a grammar, such as mime types - then use a uri.", 0, 1, valueSet); 3431 default: return super.getNamedProperty(_hash, _name, _checkValid); 3432 } 3433 3434 } 3435 3436 @Override 3437 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3438 switch (hash) { 3439 case 1791316033: /*strength*/ return this.strength == null ? new Base[0] : new Base[] {this.strength}; // Enumeration<BindingStrength> 3440 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 3441 case -1410174671: /*valueSet*/ return this.valueSet == null ? new Base[0] : new Base[] {this.valueSet}; // Type 3442 default: return super.getProperty(hash, name, checkValid); 3443 } 3444 3445 } 3446 3447 @Override 3448 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3449 switch (hash) { 3450 case 1791316033: // strength 3451 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 3452 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 3453 return value; 3454 case -1724546052: // description 3455 this.description = castToString(value); // StringType 3456 return value; 3457 case -1410174671: // valueSet 3458 this.valueSet = castToType(value); // Type 3459 return value; 3460 default: return super.setProperty(hash, name, value); 3461 } 3462 3463 } 3464 3465 @Override 3466 public Base setProperty(String name, Base value) throws FHIRException { 3467 if (name.equals("strength")) { 3468 value = new BindingStrengthEnumFactory().fromType(castToCode(value)); 3469 this.strength = (Enumeration) value; // Enumeration<BindingStrength> 3470 } else if (name.equals("description")) { 3471 this.description = castToString(value); // StringType 3472 } else if (name.equals("valueSet[x]")) { 3473 this.valueSet = castToType(value); // Type 3474 } else 3475 return super.setProperty(name, value); 3476 return value; 3477 } 3478 3479 @Override 3480 public Base makeProperty(int hash, String name) throws FHIRException { 3481 switch (hash) { 3482 case 1791316033: return getStrengthElement(); 3483 case -1724546052: return getDescriptionElement(); 3484 case -1438410321: return getValueSet(); 3485 case -1410174671: return getValueSet(); 3486 default: return super.makeProperty(hash, name); 3487 } 3488 3489 } 3490 3491 @Override 3492 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3493 switch (hash) { 3494 case 1791316033: /*strength*/ return new String[] {"code"}; 3495 case -1724546052: /*description*/ return new String[] {"string"}; 3496 case -1410174671: /*valueSet*/ return new String[] {"uri", "Reference"}; 3497 default: return super.getTypesForProperty(hash, name); 3498 } 3499 3500 } 3501 3502 @Override 3503 public Base addChild(String name) throws FHIRException { 3504 if (name.equals("strength")) { 3505 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.strength"); 3506 } 3507 else if (name.equals("description")) { 3508 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.description"); 3509 } 3510 else if (name.equals("valueSetUri")) { 3511 this.valueSet = new UriType(); 3512 return this.valueSet; 3513 } 3514 else if (name.equals("valueSetReference")) { 3515 this.valueSet = new Reference(); 3516 return this.valueSet; 3517 } 3518 else 3519 return super.addChild(name); 3520 } 3521 3522 public ElementDefinitionBindingComponent copy() { 3523 ElementDefinitionBindingComponent dst = new ElementDefinitionBindingComponent(); 3524 copyValues(dst); 3525 dst.strength = strength == null ? null : strength.copy(); 3526 dst.description = description == null ? null : description.copy(); 3527 dst.valueSet = valueSet == null ? null : valueSet.copy(); 3528 return dst; 3529 } 3530 3531 @Override 3532 public boolean equalsDeep(Base other_) { 3533 if (!super.equalsDeep(other_)) 3534 return false; 3535 if (!(other_ instanceof ElementDefinitionBindingComponent)) 3536 return false; 3537 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 3538 return compareDeep(strength, o.strength, true) && compareDeep(description, o.description, true) 3539 && compareDeep(valueSet, o.valueSet, true); 3540 } 3541 3542 @Override 3543 public boolean equalsShallow(Base other_) { 3544 if (!super.equalsShallow(other_)) 3545 return false; 3546 if (!(other_ instanceof ElementDefinitionBindingComponent)) 3547 return false; 3548 ElementDefinitionBindingComponent o = (ElementDefinitionBindingComponent) other_; 3549 return compareValues(strength, o.strength, true) && compareValues(description, o.description, true) 3550 ; 3551 } 3552 3553 public boolean isEmpty() { 3554 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(strength, description, valueSet 3555 ); 3556 } 3557 3558 public String fhirType() { 3559 return "ElementDefinition.binding"; 3560 3561 } 3562 3563 } 3564 3565 @Block() 3566 public static class ElementDefinitionMappingComponent extends Element implements IBaseDatatypeElement { 3567 /** 3568 * An internal reference to the definition of a mapping. 3569 */ 3570 @Child(name = "identity", type = {IdType.class}, order=1, min=1, max=1, modifier=false, summary=true) 3571 @Description(shortDefinition="Reference to mapping declaration", formalDefinition="An internal reference to the definition of a mapping." ) 3572 protected IdType identity; 3573 3574 /** 3575 * Identifies the computable language in which mapping.map is expressed. 3576 */ 3577 @Child(name = "language", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3578 @Description(shortDefinition="Computable language of mapping", formalDefinition="Identifies the computable language in which mapping.map is expressed." ) 3579 protected CodeType language; 3580 3581 /** 3582 * Expresses what part of the target specification corresponds to this element. 3583 */ 3584 @Child(name = "map", type = {StringType.class}, order=3, min=1, max=1, modifier=false, summary=true) 3585 @Description(shortDefinition="Details of the mapping", formalDefinition="Expresses what part of the target specification corresponds to this element." ) 3586 protected StringType map; 3587 3588 /** 3589 * Comments that provide information about the mapping or its use. 3590 */ 3591 @Child(name = "comment", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 3592 @Description(shortDefinition="Comments about the mapping or its use", formalDefinition="Comments that provide information about the mapping or its use." ) 3593 protected StringType comment; 3594 3595 private static final long serialVersionUID = 1386816887L; 3596 3597 /** 3598 * Constructor 3599 */ 3600 public ElementDefinitionMappingComponent() { 3601 super(); 3602 } 3603 3604 /** 3605 * Constructor 3606 */ 3607 public ElementDefinitionMappingComponent(IdType identity, StringType map) { 3608 super(); 3609 this.identity = identity; 3610 this.map = map; 3611 } 3612 3613 /** 3614 * @return {@link #identity} (An internal reference to the definition of a mapping.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 3615 */ 3616 public IdType getIdentityElement() { 3617 if (this.identity == null) 3618 if (Configuration.errorOnAutoCreate()) 3619 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.identity"); 3620 else if (Configuration.doAutoCreate()) 3621 this.identity = new IdType(); // bb 3622 return this.identity; 3623 } 3624 3625 public boolean hasIdentityElement() { 3626 return this.identity != null && !this.identity.isEmpty(); 3627 } 3628 3629 public boolean hasIdentity() { 3630 return this.identity != null && !this.identity.isEmpty(); 3631 } 3632 3633 /** 3634 * @param value {@link #identity} (An internal reference to the definition of a mapping.). This is the underlying object with id, value and extensions. The accessor "getIdentity" gives direct access to the value 3635 */ 3636 public ElementDefinitionMappingComponent setIdentityElement(IdType value) { 3637 this.identity = value; 3638 return this; 3639 } 3640 3641 /** 3642 * @return An internal reference to the definition of a mapping. 3643 */ 3644 public String getIdentity() { 3645 return this.identity == null ? null : this.identity.getValue(); 3646 } 3647 3648 /** 3649 * @param value An internal reference to the definition of a mapping. 3650 */ 3651 public ElementDefinitionMappingComponent setIdentity(String value) { 3652 if (this.identity == null) 3653 this.identity = new IdType(); 3654 this.identity.setValue(value); 3655 return this; 3656 } 3657 3658 /** 3659 * @return {@link #language} (Identifies the computable language in which mapping.map is expressed.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 3660 */ 3661 public CodeType getLanguageElement() { 3662 if (this.language == null) 3663 if (Configuration.errorOnAutoCreate()) 3664 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.language"); 3665 else if (Configuration.doAutoCreate()) 3666 this.language = new CodeType(); // bb 3667 return this.language; 3668 } 3669 3670 public boolean hasLanguageElement() { 3671 return this.language != null && !this.language.isEmpty(); 3672 } 3673 3674 public boolean hasLanguage() { 3675 return this.language != null && !this.language.isEmpty(); 3676 } 3677 3678 /** 3679 * @param value {@link #language} (Identifies the computable language in which mapping.map is expressed.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 3680 */ 3681 public ElementDefinitionMappingComponent setLanguageElement(CodeType value) { 3682 this.language = value; 3683 return this; 3684 } 3685 3686 /** 3687 * @return Identifies the computable language in which mapping.map is expressed. 3688 */ 3689 public String getLanguage() { 3690 return this.language == null ? null : this.language.getValue(); 3691 } 3692 3693 /** 3694 * @param value Identifies the computable language in which mapping.map is expressed. 3695 */ 3696 public ElementDefinitionMappingComponent setLanguage(String value) { 3697 if (Utilities.noString(value)) 3698 this.language = null; 3699 else { 3700 if (this.language == null) 3701 this.language = new CodeType(); 3702 this.language.setValue(value); 3703 } 3704 return this; 3705 } 3706 3707 /** 3708 * @return {@link #map} (Expresses what part of the target specification corresponds to this element.). This is the underlying object with id, value and extensions. The accessor "getMap" gives direct access to the value 3709 */ 3710 public StringType getMapElement() { 3711 if (this.map == null) 3712 if (Configuration.errorOnAutoCreate()) 3713 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.map"); 3714 else if (Configuration.doAutoCreate()) 3715 this.map = new StringType(); // bb 3716 return this.map; 3717 } 3718 3719 public boolean hasMapElement() { 3720 return this.map != null && !this.map.isEmpty(); 3721 } 3722 3723 public boolean hasMap() { 3724 return this.map != null && !this.map.isEmpty(); 3725 } 3726 3727 /** 3728 * @param value {@link #map} (Expresses what part of the target specification corresponds to this element.). This is the underlying object with id, value and extensions. The accessor "getMap" gives direct access to the value 3729 */ 3730 public ElementDefinitionMappingComponent setMapElement(StringType value) { 3731 this.map = value; 3732 return this; 3733 } 3734 3735 /** 3736 * @return Expresses what part of the target specification corresponds to this element. 3737 */ 3738 public String getMap() { 3739 return this.map == null ? null : this.map.getValue(); 3740 } 3741 3742 /** 3743 * @param value Expresses what part of the target specification corresponds to this element. 3744 */ 3745 public ElementDefinitionMappingComponent setMap(String value) { 3746 if (this.map == null) 3747 this.map = new StringType(); 3748 this.map.setValue(value); 3749 return this; 3750 } 3751 3752 /** 3753 * @return {@link #comment} (Comments that provide information about the mapping or its use.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 3754 */ 3755 public StringType getCommentElement() { 3756 if (this.comment == null) 3757 if (Configuration.errorOnAutoCreate()) 3758 throw new Error("Attempt to auto-create ElementDefinitionMappingComponent.comment"); 3759 else if (Configuration.doAutoCreate()) 3760 this.comment = new StringType(); // bb 3761 return this.comment; 3762 } 3763 3764 public boolean hasCommentElement() { 3765 return this.comment != null && !this.comment.isEmpty(); 3766 } 3767 3768 public boolean hasComment() { 3769 return this.comment != null && !this.comment.isEmpty(); 3770 } 3771 3772 /** 3773 * @param value {@link #comment} (Comments that provide information about the mapping or its use.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 3774 */ 3775 public ElementDefinitionMappingComponent setCommentElement(StringType value) { 3776 this.comment = value; 3777 return this; 3778 } 3779 3780 /** 3781 * @return Comments that provide information about the mapping or its use. 3782 */ 3783 public String getComment() { 3784 return this.comment == null ? null : this.comment.getValue(); 3785 } 3786 3787 /** 3788 * @param value Comments that provide information about the mapping or its use. 3789 */ 3790 public ElementDefinitionMappingComponent setComment(String value) { 3791 if (Utilities.noString(value)) 3792 this.comment = null; 3793 else { 3794 if (this.comment == null) 3795 this.comment = new StringType(); 3796 this.comment.setValue(value); 3797 } 3798 return this; 3799 } 3800 3801 protected void listChildren(List<Property> children) { 3802 super.listChildren(children); 3803 children.add(new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 1, identity)); 3804 children.add(new Property("language", "code", "Identifies the computable language in which mapping.map is expressed.", 0, 1, language)); 3805 children.add(new Property("map", "string", "Expresses what part of the target specification corresponds to this element.", 0, 1, map)); 3806 children.add(new Property("comment", "string", "Comments that provide information about the mapping or its use.", 0, 1, comment)); 3807 } 3808 3809 @Override 3810 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3811 switch (_hash) { 3812 case -135761730: /*identity*/ return new Property("identity", "id", "An internal reference to the definition of a mapping.", 0, 1, identity); 3813 case -1613589672: /*language*/ return new Property("language", "code", "Identifies the computable language in which mapping.map is expressed.", 0, 1, language); 3814 case 107868: /*map*/ return new Property("map", "string", "Expresses what part of the target specification corresponds to this element.", 0, 1, map); 3815 case 950398559: /*comment*/ return new Property("comment", "string", "Comments that provide information about the mapping or its use.", 0, 1, comment); 3816 default: return super.getNamedProperty(_hash, _name, _checkValid); 3817 } 3818 3819 } 3820 3821 @Override 3822 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3823 switch (hash) { 3824 case -135761730: /*identity*/ return this.identity == null ? new Base[0] : new Base[] {this.identity}; // IdType 3825 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 3826 case 107868: /*map*/ return this.map == null ? new Base[0] : new Base[] {this.map}; // StringType 3827 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 3828 default: return super.getProperty(hash, name, checkValid); 3829 } 3830 3831 } 3832 3833 @Override 3834 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3835 switch (hash) { 3836 case -135761730: // identity 3837 this.identity = castToId(value); // IdType 3838 return value; 3839 case -1613589672: // language 3840 this.language = castToCode(value); // CodeType 3841 return value; 3842 case 107868: // map 3843 this.map = castToString(value); // StringType 3844 return value; 3845 case 950398559: // comment 3846 this.comment = castToString(value); // StringType 3847 return value; 3848 default: return super.setProperty(hash, name, value); 3849 } 3850 3851 } 3852 3853 @Override 3854 public Base setProperty(String name, Base value) throws FHIRException { 3855 if (name.equals("identity")) { 3856 this.identity = castToId(value); // IdType 3857 } else if (name.equals("language")) { 3858 this.language = castToCode(value); // CodeType 3859 } else if (name.equals("map")) { 3860 this.map = castToString(value); // StringType 3861 } else if (name.equals("comment")) { 3862 this.comment = castToString(value); // StringType 3863 } else 3864 return super.setProperty(name, value); 3865 return value; 3866 } 3867 3868 @Override 3869 public Base makeProperty(int hash, String name) throws FHIRException { 3870 switch (hash) { 3871 case -135761730: return getIdentityElement(); 3872 case -1613589672: return getLanguageElement(); 3873 case 107868: return getMapElement(); 3874 case 950398559: return getCommentElement(); 3875 default: return super.makeProperty(hash, name); 3876 } 3877 3878 } 3879 3880 @Override 3881 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3882 switch (hash) { 3883 case -135761730: /*identity*/ return new String[] {"id"}; 3884 case -1613589672: /*language*/ return new String[] {"code"}; 3885 case 107868: /*map*/ return new String[] {"string"}; 3886 case 950398559: /*comment*/ return new String[] {"string"}; 3887 default: return super.getTypesForProperty(hash, name); 3888 } 3889 3890 } 3891 3892 @Override 3893 public Base addChild(String name) throws FHIRException { 3894 if (name.equals("identity")) { 3895 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.identity"); 3896 } 3897 else if (name.equals("language")) { 3898 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.language"); 3899 } 3900 else if (name.equals("map")) { 3901 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.map"); 3902 } 3903 else if (name.equals("comment")) { 3904 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comment"); 3905 } 3906 else 3907 return super.addChild(name); 3908 } 3909 3910 public ElementDefinitionMappingComponent copy() { 3911 ElementDefinitionMappingComponent dst = new ElementDefinitionMappingComponent(); 3912 copyValues(dst); 3913 dst.identity = identity == null ? null : identity.copy(); 3914 dst.language = language == null ? null : language.copy(); 3915 dst.map = map == null ? null : map.copy(); 3916 dst.comment = comment == null ? null : comment.copy(); 3917 return dst; 3918 } 3919 3920 @Override 3921 public boolean equalsDeep(Base other_) { 3922 if (!super.equalsDeep(other_)) 3923 return false; 3924 if (!(other_ instanceof ElementDefinitionMappingComponent)) 3925 return false; 3926 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 3927 return compareDeep(identity, o.identity, true) && compareDeep(language, o.language, true) && compareDeep(map, o.map, true) 3928 && compareDeep(comment, o.comment, true); 3929 } 3930 3931 @Override 3932 public boolean equalsShallow(Base other_) { 3933 if (!super.equalsShallow(other_)) 3934 return false; 3935 if (!(other_ instanceof ElementDefinitionMappingComponent)) 3936 return false; 3937 ElementDefinitionMappingComponent o = (ElementDefinitionMappingComponent) other_; 3938 return compareValues(identity, o.identity, true) && compareValues(language, o.language, true) && compareValues(map, o.map, true) 3939 && compareValues(comment, o.comment, true); 3940 } 3941 3942 public boolean isEmpty() { 3943 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identity, language, map 3944 , comment); 3945 } 3946 3947 public String fhirType() { 3948 return "ElementDefinition.mapping"; 3949 3950 } 3951 3952 } 3953 3954 /** 3955 * The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 3956 */ 3957 @Child(name = "path", type = {StringType.class}, order=0, min=1, max=1, modifier=false, summary=true) 3958 @Description(shortDefinition="Path of the element in the hierarchy of elements", formalDefinition="The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension." ) 3959 protected StringType path; 3960 3961 /** 3962 * Codes that define how this element is represented in instances, when the deviation varies from the normal case. 3963 */ 3964 @Child(name = "representation", type = {CodeType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3965 @Description(shortDefinition="xmlAttr | xmlText | typeAttr | cdaText | xhtml", formalDefinition="Codes that define how this element is represented in instances, when the deviation varies from the normal case." ) 3966 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/property-representation") 3967 protected List<Enumeration<PropertyRepresentation>> representation; 3968 3969 /** 3970 * The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 3971 */ 3972 @Child(name = "sliceName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 3973 @Description(shortDefinition="Name for this particular element (in a set of slices)", formalDefinition="The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element." ) 3974 protected StringType sliceName; 3975 3976 /** 3977 * A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 3978 */ 3979 @Child(name = "label", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 3980 @Description(shortDefinition="Name for element to display with or prompt for element", formalDefinition="A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form." ) 3981 protected StringType label; 3982 3983 /** 3984 * A code that has the same meaning as the element in a particular terminology. 3985 */ 3986 @Child(name = "code", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 3987 @Description(shortDefinition="Corresponding codes in terminologies", formalDefinition="A code that has the same meaning as the element in a particular terminology." ) 3988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 3989 protected List<Coding> code; 3990 3991 /** 3992 * Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set). 3993 */ 3994 @Child(name = "slicing", type = {}, order=5, min=0, max=1, modifier=false, summary=true) 3995 @Description(shortDefinition="This element is sliced - slices follow", formalDefinition="Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set)." ) 3996 protected ElementDefinitionSlicingComponent slicing; 3997 3998 /** 3999 * A concise description of what this element means (e.g. for use in autogenerated summaries). 4000 */ 4001 @Child(name = "short", type = {StringType.class}, order=6, min=0, max=1, modifier=false, summary=true) 4002 @Description(shortDefinition="Concise definition for space-constrained presentation", formalDefinition="A concise description of what this element means (e.g. for use in autogenerated summaries)." ) 4003 protected StringType short_; 4004 4005 /** 4006 * Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. 4007 */ 4008 @Child(name = "definition", type = {MarkdownType.class}, order=7, min=0, max=1, modifier=false, summary=true) 4009 @Description(shortDefinition="Full formal definition as narrative text", formalDefinition="Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource." ) 4010 protected MarkdownType definition; 4011 4012 /** 4013 * Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. 4014 */ 4015 @Child(name = "comment", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=true) 4016 @Description(shortDefinition="Comments about the use of this element", formalDefinition="Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc." ) 4017 protected MarkdownType comment; 4018 4019 /** 4020 * This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 4021 */ 4022 @Child(name = "requirements", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=true) 4023 @Description(shortDefinition="Why this resource has been created", formalDefinition="This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element." ) 4024 protected MarkdownType requirements; 4025 4026 /** 4027 * Identifies additional names by which this element might also be known. 4028 */ 4029 @Child(name = "alias", type = {StringType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4030 @Description(shortDefinition="Other names", formalDefinition="Identifies additional names by which this element might also be known." ) 4031 protected List<StringType> alias; 4032 4033 /** 4034 * The minimum number of times this element SHALL appear in the instance. 4035 */ 4036 @Child(name = "min", type = {UnsignedIntType.class}, order=11, min=0, max=1, modifier=false, summary=true) 4037 @Description(shortDefinition="Minimum Cardinality", formalDefinition="The minimum number of times this element SHALL appear in the instance." ) 4038 protected UnsignedIntType min; 4039 4040 /** 4041 * The maximum number of times this element is permitted to appear in the instance. 4042 */ 4043 @Child(name = "max", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=true) 4044 @Description(shortDefinition="Maximum Cardinality (a number or *)", formalDefinition="The maximum number of times this element is permitted to appear in the instance." ) 4045 protected StringType max; 4046 4047 /** 4048 * Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. This information is provided when the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot. 4049 */ 4050 @Child(name = "base", type = {}, order=13, min=0, max=1, modifier=false, summary=true) 4051 @Description(shortDefinition="Base definition information for tools", formalDefinition="Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. This information is provided when the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot." ) 4052 protected ElementDefinitionBaseComponent base; 4053 4054 /** 4055 * Identifies the identity of an element defined elsewhere in the profile whose content rules should be applied to the current element. 4056 */ 4057 @Child(name = "contentReference", type = {UriType.class}, order=14, min=0, max=1, modifier=false, summary=true) 4058 @Description(shortDefinition="Reference to definition of content for the element", formalDefinition="Identifies the identity of an element defined elsewhere in the profile whose content rules should be applied to the current element." ) 4059 protected UriType contentReference; 4060 4061 /** 4062 * The data type or resource that the value of this element is permitted to be. 4063 */ 4064 @Child(name = "type", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4065 @Description(shortDefinition="Data type and Profile for this element", formalDefinition="The data type or resource that the value of this element is permitted to be." ) 4066 protected List<TypeRefComponent> type; 4067 4068 /** 4069 * The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false'). 4070 */ 4071 @Child(name = "defaultValue", type = {}, order=16, min=0, max=1, modifier=false, summary=true) 4072 @Description(shortDefinition="Specified value if missing from instance", formalDefinition="The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false')." ) 4073 protected org.hl7.fhir.dstu3.model.Type defaultValue; 4074 4075 /** 4076 * The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'. 4077 */ 4078 @Child(name = "meaningWhenMissing", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=true) 4079 @Description(shortDefinition="Implicit meaning when this element is missing", formalDefinition="The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'." ) 4080 protected MarkdownType meaningWhenMissing; 4081 4082 /** 4083 * If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 4084 */ 4085 @Child(name = "orderMeaning", type = {StringType.class}, order=18, min=0, max=1, modifier=false, summary=true) 4086 @Description(shortDefinition="What the order of the elements means", formalDefinition="If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning." ) 4087 protected StringType orderMeaning; 4088 4089 /** 4090 * Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing. 4091 */ 4092 @Child(name = "fixed", type = {}, order=19, min=0, max=1, modifier=false, summary=true) 4093 @Description(shortDefinition="Value must be exactly this", formalDefinition="Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing." ) 4094 protected org.hl7.fhir.dstu3.model.Type fixed; 4095 4096 /** 4097 * Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.). 4098 */ 4099 @Child(name = "pattern", type = {}, order=20, min=0, max=1, modifier=false, summary=true) 4100 @Description(shortDefinition="Value must have at least these property values", formalDefinition="Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.)." ) 4101 protected org.hl7.fhir.dstu3.model.Type pattern; 4102 4103 /** 4104 * A sample value for this element demonstrating the type of information that would typically be found in the element. 4105 */ 4106 @Child(name = "example", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4107 @Description(shortDefinition="Example value (as defined for type)", formalDefinition="A sample value for this element demonstrating the type of information that would typically be found in the element." ) 4108 protected List<ElementDefinitionExampleComponent> example; 4109 4110 /** 4111 * The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity. 4112 */ 4113 @Child(name = "minValue", type = {DateType.class, DateTimeType.class, InstantType.class, TimeType.class, DecimalType.class, IntegerType.class, PositiveIntType.class, UnsignedIntType.class, Quantity.class}, order=22, min=0, max=1, modifier=false, summary=true) 4114 @Description(shortDefinition="Minimum Allowed Value (for some types)", formalDefinition="The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity." ) 4115 protected Type minValue; 4116 4117 /** 4118 * The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity. 4119 */ 4120 @Child(name = "maxValue", type = {DateType.class, DateTimeType.class, InstantType.class, TimeType.class, DecimalType.class, IntegerType.class, PositiveIntType.class, UnsignedIntType.class, Quantity.class}, order=23, min=0, max=1, modifier=false, summary=true) 4121 @Description(shortDefinition="Maximum Allowed Value (for some types)", formalDefinition="The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity." ) 4122 protected Type maxValue; 4123 4124 /** 4125 * Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. 4126 */ 4127 @Child(name = "maxLength", type = {IntegerType.class}, order=24, min=0, max=1, modifier=false, summary=true) 4128 @Description(shortDefinition="Max length for strings", formalDefinition="Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element." ) 4129 protected IntegerType maxLength; 4130 4131 /** 4132 * A reference to an invariant that may make additional statements about the cardinality or value in the instance. 4133 */ 4134 @Child(name = "condition", type = {IdType.class}, order=25, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4135 @Description(shortDefinition="Reference to invariant about presence", formalDefinition="A reference to an invariant that may make additional statements about the cardinality or value in the instance." ) 4136 protected List<IdType> condition; 4137 4138 /** 4139 * Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance. 4140 */ 4141 @Child(name = "constraint", type = {}, order=26, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4142 @Description(shortDefinition="Condition that must evaluate to true", formalDefinition="Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance." ) 4143 protected List<ElementDefinitionConstraintComponent> constraint; 4144 4145 /** 4146 * If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. If false, the element may be ignored and not supported. 4147 */ 4148 @Child(name = "mustSupport", type = {BooleanType.class}, order=27, min=0, max=1, modifier=false, summary=true) 4149 @Description(shortDefinition="If the element must supported", formalDefinition="If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported." ) 4150 protected BooleanType mustSupport; 4151 4152 /** 4153 * If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. 4154 */ 4155 @Child(name = "isModifier", type = {BooleanType.class}, order=28, min=0, max=1, modifier=false, summary=true) 4156 @Description(shortDefinition="If this modifies the meaning of other elements", formalDefinition="If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system." ) 4157 protected BooleanType isModifier; 4158 4159 /** 4160 * Whether the element should be included if a client requests a search with the parameter _summary=true. 4161 */ 4162 @Child(name = "isSummary", type = {BooleanType.class}, order=29, min=0, max=1, modifier=false, summary=true) 4163 @Description(shortDefinition="Include when _summary = true?", formalDefinition="Whether the element should be included if a client requests a search with the parameter _summary=true." ) 4164 protected BooleanType isSummary; 4165 4166 /** 4167 * Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri). 4168 */ 4169 @Child(name = "binding", type = {}, order=30, min=0, max=1, modifier=false, summary=true) 4170 @Description(shortDefinition="ValueSet details if this is coded", formalDefinition="Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri)." ) 4171 protected ElementDefinitionBindingComponent binding; 4172 4173 /** 4174 * Identifies a concept from an external specification that roughly corresponds to this element. 4175 */ 4176 @Child(name = "mapping", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 4177 @Description(shortDefinition="Map element to another set of definitions", formalDefinition="Identifies a concept from an external specification that roughly corresponds to this element." ) 4178 protected List<ElementDefinitionMappingComponent> mapping; 4179 4180 private static final long serialVersionUID = -278262340L; 4181 4182 /** 4183 * Constructor 4184 */ 4185 public ElementDefinition() { 4186 super(); 4187 } 4188 4189 /** 4190 * Constructor 4191 */ 4192 public ElementDefinition(StringType path) { 4193 super(); 4194 this.path = path; 4195 } 4196 4197 /** 4198 * @return {@link #path} (The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 4199 */ 4200 public StringType getPathElement() { 4201 if (this.path == null) 4202 if (Configuration.errorOnAutoCreate()) 4203 throw new Error("Attempt to auto-create ElementDefinition.path"); 4204 else if (Configuration.doAutoCreate()) 4205 this.path = new StringType(); // bb 4206 return this.path; 4207 } 4208 4209 public boolean hasPathElement() { 4210 return this.path != null && !this.path.isEmpty(); 4211 } 4212 4213 public boolean hasPath() { 4214 return this.path != null && !this.path.isEmpty(); 4215 } 4216 4217 /** 4218 * @param value {@link #path} (The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 4219 */ 4220 public ElementDefinition setPathElement(StringType value) { 4221 this.path = value; 4222 return this; 4223 } 4224 4225 /** 4226 * @return The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 4227 */ 4228 public String getPath() { 4229 return this.path == null ? null : this.path.getValue(); 4230 } 4231 4232 /** 4233 * @param value The path identifies the element and is expressed as a "."-separated list of ancestor elements, beginning with the name of the resource or extension. 4234 */ 4235 public ElementDefinition setPath(String value) { 4236 if (this.path == null) 4237 this.path = new StringType(); 4238 this.path.setValue(value); 4239 return this; 4240 } 4241 4242 /** 4243 * @return {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case.) 4244 */ 4245 public List<Enumeration<PropertyRepresentation>> getRepresentation() { 4246 if (this.representation == null) 4247 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 4248 return this.representation; 4249 } 4250 4251 /** 4252 * @return Returns a reference to <code>this</code> for easy method chaining 4253 */ 4254 public ElementDefinition setRepresentation(List<Enumeration<PropertyRepresentation>> theRepresentation) { 4255 this.representation = theRepresentation; 4256 return this; 4257 } 4258 4259 public boolean hasRepresentation() { 4260 if (this.representation == null) 4261 return false; 4262 for (Enumeration<PropertyRepresentation> item : this.representation) 4263 if (!item.isEmpty()) 4264 return true; 4265 return false; 4266 } 4267 4268 /** 4269 * @return {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case.) 4270 */ 4271 public Enumeration<PropertyRepresentation> addRepresentationElement() {//2 4272 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>(new PropertyRepresentationEnumFactory()); 4273 if (this.representation == null) 4274 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 4275 this.representation.add(t); 4276 return t; 4277 } 4278 4279 /** 4280 * @param value {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case.) 4281 */ 4282 public ElementDefinition addRepresentation(PropertyRepresentation value) { //1 4283 Enumeration<PropertyRepresentation> t = new Enumeration<PropertyRepresentation>(new PropertyRepresentationEnumFactory()); 4284 t.setValue(value); 4285 if (this.representation == null) 4286 this.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 4287 this.representation.add(t); 4288 return this; 4289 } 4290 4291 /** 4292 * @param value {@link #representation} (Codes that define how this element is represented in instances, when the deviation varies from the normal case.) 4293 */ 4294 public boolean hasRepresentation(PropertyRepresentation value) { 4295 if (this.representation == null) 4296 return false; 4297 for (Enumeration<PropertyRepresentation> v : this.representation) 4298 if (v.getValue().equals(value)) // code 4299 return true; 4300 return false; 4301 } 4302 4303 /** 4304 * @return {@link #sliceName} (The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 4305 */ 4306 public StringType getSliceNameElement() { 4307 if (this.sliceName == null) 4308 if (Configuration.errorOnAutoCreate()) 4309 throw new Error("Attempt to auto-create ElementDefinition.sliceName"); 4310 else if (Configuration.doAutoCreate()) 4311 this.sliceName = new StringType(); // bb 4312 return this.sliceName; 4313 } 4314 4315 public boolean hasSliceNameElement() { 4316 return this.sliceName != null && !this.sliceName.isEmpty(); 4317 } 4318 4319 public boolean hasSliceName() { 4320 return this.sliceName != null && !this.sliceName.isEmpty(); 4321 } 4322 4323 /** 4324 * @param value {@link #sliceName} (The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 4325 */ 4326 public ElementDefinition setSliceNameElement(StringType value) { 4327 this.sliceName = value; 4328 return this; 4329 } 4330 4331 /** 4332 * @return The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 4333 */ 4334 public String getSliceName() { 4335 return this.sliceName == null ? null : this.sliceName.getValue(); 4336 } 4337 4338 /** 4339 * @param value The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element. 4340 */ 4341 public ElementDefinition setSliceName(String value) { 4342 if (Utilities.noString(value)) 4343 this.sliceName = null; 4344 else { 4345 if (this.sliceName == null) 4346 this.sliceName = new StringType(); 4347 this.sliceName.setValue(value); 4348 } 4349 return this; 4350 } 4351 4352 /** 4353 * @return {@link #label} (A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 4354 */ 4355 public StringType getLabelElement() { 4356 if (this.label == null) 4357 if (Configuration.errorOnAutoCreate()) 4358 throw new Error("Attempt to auto-create ElementDefinition.label"); 4359 else if (Configuration.doAutoCreate()) 4360 this.label = new StringType(); // bb 4361 return this.label; 4362 } 4363 4364 public boolean hasLabelElement() { 4365 return this.label != null && !this.label.isEmpty(); 4366 } 4367 4368 public boolean hasLabel() { 4369 return this.label != null && !this.label.isEmpty(); 4370 } 4371 4372 /** 4373 * @param value {@link #label} (A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.). This is the underlying object with id, value and extensions. The accessor "getLabel" gives direct access to the value 4374 */ 4375 public ElementDefinition setLabelElement(StringType value) { 4376 this.label = value; 4377 return this; 4378 } 4379 4380 /** 4381 * @return A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 4382 */ 4383 public String getLabel() { 4384 return this.label == null ? null : this.label.getValue(); 4385 } 4386 4387 /** 4388 * @param value A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form. 4389 */ 4390 public ElementDefinition setLabel(String value) { 4391 if (Utilities.noString(value)) 4392 this.label = null; 4393 else { 4394 if (this.label == null) 4395 this.label = new StringType(); 4396 this.label.setValue(value); 4397 } 4398 return this; 4399 } 4400 4401 /** 4402 * @return {@link #code} (A code that has the same meaning as the element in a particular terminology.) 4403 */ 4404 public List<Coding> getCode() { 4405 if (this.code == null) 4406 this.code = new ArrayList<Coding>(); 4407 return this.code; 4408 } 4409 4410 /** 4411 * @return Returns a reference to <code>this</code> for easy method chaining 4412 */ 4413 public ElementDefinition setCode(List<Coding> theCode) { 4414 this.code = theCode; 4415 return this; 4416 } 4417 4418 public boolean hasCode() { 4419 if (this.code == null) 4420 return false; 4421 for (Coding item : this.code) 4422 if (!item.isEmpty()) 4423 return true; 4424 return false; 4425 } 4426 4427 public Coding addCode() { //3 4428 Coding t = new Coding(); 4429 if (this.code == null) 4430 this.code = new ArrayList<Coding>(); 4431 this.code.add(t); 4432 return t; 4433 } 4434 4435 public ElementDefinition addCode(Coding t) { //3 4436 if (t == null) 4437 return this; 4438 if (this.code == null) 4439 this.code = new ArrayList<Coding>(); 4440 this.code.add(t); 4441 return this; 4442 } 4443 4444 /** 4445 * @return The first repetition of repeating field {@link #code}, creating it if it does not already exist 4446 */ 4447 public Coding getCodeFirstRep() { 4448 if (getCode().isEmpty()) { 4449 addCode(); 4450 } 4451 return getCode().get(0); 4452 } 4453 4454 /** 4455 * @return {@link #slicing} (Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).) 4456 */ 4457 public ElementDefinitionSlicingComponent getSlicing() { 4458 if (this.slicing == null) 4459 if (Configuration.errorOnAutoCreate()) 4460 throw new Error("Attempt to auto-create ElementDefinition.slicing"); 4461 else if (Configuration.doAutoCreate()) 4462 this.slicing = new ElementDefinitionSlicingComponent(); // cc 4463 return this.slicing; 4464 } 4465 4466 public boolean hasSlicing() { 4467 return this.slicing != null && !this.slicing.isEmpty(); 4468 } 4469 4470 /** 4471 * @param value {@link #slicing} (Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).) 4472 */ 4473 public ElementDefinition setSlicing(ElementDefinitionSlicingComponent value) { 4474 this.slicing = value; 4475 return this; 4476 } 4477 4478 /** 4479 * @return {@link #short_} (A concise description of what this element means (e.g. for use in autogenerated summaries).). This is the underlying object with id, value and extensions. The accessor "getShort" gives direct access to the value 4480 */ 4481 public StringType getShortElement() { 4482 if (this.short_ == null) 4483 if (Configuration.errorOnAutoCreate()) 4484 throw new Error("Attempt to auto-create ElementDefinition.short_"); 4485 else if (Configuration.doAutoCreate()) 4486 this.short_ = new StringType(); // bb 4487 return this.short_; 4488 } 4489 4490 public boolean hasShortElement() { 4491 return this.short_ != null && !this.short_.isEmpty(); 4492 } 4493 4494 public boolean hasShort() { 4495 return this.short_ != null && !this.short_.isEmpty(); 4496 } 4497 4498 /** 4499 * @param value {@link #short_} (A concise description of what this element means (e.g. for use in autogenerated summaries).). This is the underlying object with id, value and extensions. The accessor "getShort" gives direct access to the value 4500 */ 4501 public ElementDefinition setShortElement(StringType value) { 4502 this.short_ = value; 4503 return this; 4504 } 4505 4506 /** 4507 * @return A concise description of what this element means (e.g. for use in autogenerated summaries). 4508 */ 4509 public String getShort() { 4510 return this.short_ == null ? null : this.short_.getValue(); 4511 } 4512 4513 /** 4514 * @param value A concise description of what this element means (e.g. for use in autogenerated summaries). 4515 */ 4516 public ElementDefinition setShort(String value) { 4517 if (Utilities.noString(value)) 4518 this.short_ = null; 4519 else { 4520 if (this.short_ == null) 4521 this.short_ = new StringType(); 4522 this.short_.setValue(value); 4523 } 4524 return this; 4525 } 4526 4527 /** 4528 * @return {@link #definition} (Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 4529 */ 4530 public MarkdownType getDefinitionElement() { 4531 if (this.definition == null) 4532 if (Configuration.errorOnAutoCreate()) 4533 throw new Error("Attempt to auto-create ElementDefinition.definition"); 4534 else if (Configuration.doAutoCreate()) 4535 this.definition = new MarkdownType(); // bb 4536 return this.definition; 4537 } 4538 4539 public boolean hasDefinitionElement() { 4540 return this.definition != null && !this.definition.isEmpty(); 4541 } 4542 4543 public boolean hasDefinition() { 4544 return this.definition != null && !this.definition.isEmpty(); 4545 } 4546 4547 /** 4548 * @param value {@link #definition} (Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource.). This is the underlying object with id, value and extensions. The accessor "getDefinition" gives direct access to the value 4549 */ 4550 public ElementDefinition setDefinitionElement(MarkdownType value) { 4551 this.definition = value; 4552 return this; 4553 } 4554 4555 /** 4556 * @return Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. 4557 */ 4558 public String getDefinition() { 4559 return this.definition == null ? null : this.definition.getValue(); 4560 } 4561 4562 /** 4563 * @param value Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource. 4564 */ 4565 public ElementDefinition setDefinition(String value) { 4566 if (value == null) 4567 this.definition = null; 4568 else { 4569 if (this.definition == null) 4570 this.definition = new MarkdownType(); 4571 this.definition.setValue(value); 4572 } 4573 return this; 4574 } 4575 4576 /** 4577 * @return {@link #comment} (Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 4578 */ 4579 public MarkdownType getCommentElement() { 4580 if (this.comment == null) 4581 if (Configuration.errorOnAutoCreate()) 4582 throw new Error("Attempt to auto-create ElementDefinition.comment"); 4583 else if (Configuration.doAutoCreate()) 4584 this.comment = new MarkdownType(); // bb 4585 return this.comment; 4586 } 4587 4588 public boolean hasCommentElement() { 4589 return this.comment != null && !this.comment.isEmpty(); 4590 } 4591 4592 public boolean hasComment() { 4593 return this.comment != null && !this.comment.isEmpty(); 4594 } 4595 4596 /** 4597 * @param value {@link #comment} (Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 4598 */ 4599 public ElementDefinition setCommentElement(MarkdownType value) { 4600 this.comment = value; 4601 return this; 4602 } 4603 4604 /** 4605 * @return Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. 4606 */ 4607 public String getComment() { 4608 return this.comment == null ? null : this.comment.getValue(); 4609 } 4610 4611 /** 4612 * @param value Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc. 4613 */ 4614 public ElementDefinition setComment(String value) { 4615 if (value == null) 4616 this.comment = null; 4617 else { 4618 if (this.comment == null) 4619 this.comment = new MarkdownType(); 4620 this.comment.setValue(value); 4621 } 4622 return this; 4623 } 4624 4625 /** 4626 * @return {@link #requirements} (This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 4627 */ 4628 public MarkdownType getRequirementsElement() { 4629 if (this.requirements == null) 4630 if (Configuration.errorOnAutoCreate()) 4631 throw new Error("Attempt to auto-create ElementDefinition.requirements"); 4632 else if (Configuration.doAutoCreate()) 4633 this.requirements = new MarkdownType(); // bb 4634 return this.requirements; 4635 } 4636 4637 public boolean hasRequirementsElement() { 4638 return this.requirements != null && !this.requirements.isEmpty(); 4639 } 4640 4641 public boolean hasRequirements() { 4642 return this.requirements != null && !this.requirements.isEmpty(); 4643 } 4644 4645 /** 4646 * @param value {@link #requirements} (This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.). This is the underlying object with id, value and extensions. The accessor "getRequirements" gives direct access to the value 4647 */ 4648 public ElementDefinition setRequirementsElement(MarkdownType value) { 4649 this.requirements = value; 4650 return this; 4651 } 4652 4653 /** 4654 * @return This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 4655 */ 4656 public String getRequirements() { 4657 return this.requirements == null ? null : this.requirements.getValue(); 4658 } 4659 4660 /** 4661 * @param value This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element. 4662 */ 4663 public ElementDefinition setRequirements(String value) { 4664 if (value == null) 4665 this.requirements = null; 4666 else { 4667 if (this.requirements == null) 4668 this.requirements = new MarkdownType(); 4669 this.requirements.setValue(value); 4670 } 4671 return this; 4672 } 4673 4674 /** 4675 * @return {@link #alias} (Identifies additional names by which this element might also be known.) 4676 */ 4677 public List<StringType> getAlias() { 4678 if (this.alias == null) 4679 this.alias = new ArrayList<StringType>(); 4680 return this.alias; 4681 } 4682 4683 /** 4684 * @return Returns a reference to <code>this</code> for easy method chaining 4685 */ 4686 public ElementDefinition setAlias(List<StringType> theAlias) { 4687 this.alias = theAlias; 4688 return this; 4689 } 4690 4691 public boolean hasAlias() { 4692 if (this.alias == null) 4693 return false; 4694 for (StringType item : this.alias) 4695 if (!item.isEmpty()) 4696 return true; 4697 return false; 4698 } 4699 4700 /** 4701 * @return {@link #alias} (Identifies additional names by which this element might also be known.) 4702 */ 4703 public StringType addAliasElement() {//2 4704 StringType t = new StringType(); 4705 if (this.alias == null) 4706 this.alias = new ArrayList<StringType>(); 4707 this.alias.add(t); 4708 return t; 4709 } 4710 4711 /** 4712 * @param value {@link #alias} (Identifies additional names by which this element might also be known.) 4713 */ 4714 public ElementDefinition addAlias(String value) { //1 4715 StringType t = new StringType(); 4716 t.setValue(value); 4717 if (this.alias == null) 4718 this.alias = new ArrayList<StringType>(); 4719 this.alias.add(t); 4720 return this; 4721 } 4722 4723 /** 4724 * @param value {@link #alias} (Identifies additional names by which this element might also be known.) 4725 */ 4726 public boolean hasAlias(String value) { 4727 if (this.alias == null) 4728 return false; 4729 for (StringType v : this.alias) 4730 if (v.getValue().equals(value)) // string 4731 return true; 4732 return false; 4733 } 4734 4735 /** 4736 * @return {@link #min} (The minimum number of times this element SHALL appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 4737 */ 4738 public UnsignedIntType getMinElement() { 4739 if (this.min == null) 4740 if (Configuration.errorOnAutoCreate()) 4741 throw new Error("Attempt to auto-create ElementDefinition.min"); 4742 else if (Configuration.doAutoCreate()) 4743 this.min = new UnsignedIntType(); // bb 4744 return this.min; 4745 } 4746 4747 public boolean hasMinElement() { 4748 return this.min != null && !this.min.isEmpty(); 4749 } 4750 4751 public boolean hasMin() { 4752 return this.min != null && !this.min.isEmpty(); 4753 } 4754 4755 /** 4756 * @param value {@link #min} (The minimum number of times this element SHALL appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 4757 */ 4758 public ElementDefinition setMinElement(UnsignedIntType value) { 4759 this.min = value; 4760 return this; 4761 } 4762 4763 /** 4764 * @return The minimum number of times this element SHALL appear in the instance. 4765 */ 4766 public int getMin() { 4767 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 4768 } 4769 4770 /** 4771 * @param value The minimum number of times this element SHALL appear in the instance. 4772 */ 4773 public ElementDefinition setMin(int value) { 4774 if (this.min == null) 4775 this.min = new UnsignedIntType(); 4776 this.min.setValue(value); 4777 return this; 4778 } 4779 4780 /** 4781 * @return {@link #max} (The maximum number of times this element is permitted to appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 4782 */ 4783 public StringType getMaxElement() { 4784 if (this.max == null) 4785 if (Configuration.errorOnAutoCreate()) 4786 throw new Error("Attempt to auto-create ElementDefinition.max"); 4787 else if (Configuration.doAutoCreate()) 4788 this.max = new StringType(); // bb 4789 return this.max; 4790 } 4791 4792 public boolean hasMaxElement() { 4793 return this.max != null && !this.max.isEmpty(); 4794 } 4795 4796 public boolean hasMax() { 4797 return this.max != null && !this.max.isEmpty(); 4798 } 4799 4800 /** 4801 * @param value {@link #max} (The maximum number of times this element is permitted to appear in the instance.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 4802 */ 4803 public ElementDefinition setMaxElement(StringType value) { 4804 this.max = value; 4805 return this; 4806 } 4807 4808 /** 4809 * @return The maximum number of times this element is permitted to appear in the instance. 4810 */ 4811 public String getMax() { 4812 return this.max == null ? null : this.max.getValue(); 4813 } 4814 4815 /** 4816 * @param value The maximum number of times this element is permitted to appear in the instance. 4817 */ 4818 public ElementDefinition setMax(String value) { 4819 if (Utilities.noString(value)) 4820 this.max = null; 4821 else { 4822 if (this.max == null) 4823 this.max = new StringType(); 4824 this.max.setValue(value); 4825 } 4826 return this; 4827 } 4828 4829 /** 4830 * @return {@link #base} (Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. This information is provided when the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot.) 4831 */ 4832 public ElementDefinitionBaseComponent getBase() { 4833 if (this.base == null) 4834 if (Configuration.errorOnAutoCreate()) 4835 throw new Error("Attempt to auto-create ElementDefinition.base"); 4836 else if (Configuration.doAutoCreate()) 4837 this.base = new ElementDefinitionBaseComponent(); // cc 4838 return this.base; 4839 } 4840 4841 public boolean hasBase() { 4842 return this.base != null && !this.base.isEmpty(); 4843 } 4844 4845 /** 4846 * @param value {@link #base} (Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. This information is provided when the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot.) 4847 */ 4848 public ElementDefinition setBase(ElementDefinitionBaseComponent value) { 4849 this.base = value; 4850 return this; 4851 } 4852 4853 /** 4854 * @return {@link #contentReference} (Identifies the identity of an element defined elsewhere in the profile whose content rules should be applied to the current element.). This is the underlying object with id, value and extensions. The accessor "getContentReference" gives direct access to the value 4855 */ 4856 public UriType getContentReferenceElement() { 4857 if (this.contentReference == null) 4858 if (Configuration.errorOnAutoCreate()) 4859 throw new Error("Attempt to auto-create ElementDefinition.contentReference"); 4860 else if (Configuration.doAutoCreate()) 4861 this.contentReference = new UriType(); // bb 4862 return this.contentReference; 4863 } 4864 4865 public boolean hasContentReferenceElement() { 4866 return this.contentReference != null && !this.contentReference.isEmpty(); 4867 } 4868 4869 public boolean hasContentReference() { 4870 return this.contentReference != null && !this.contentReference.isEmpty(); 4871 } 4872 4873 /** 4874 * @param value {@link #contentReference} (Identifies the identity of an element defined elsewhere in the profile whose content rules should be applied to the current element.). This is the underlying object with id, value and extensions. The accessor "getContentReference" gives direct access to the value 4875 */ 4876 public ElementDefinition setContentReferenceElement(UriType value) { 4877 this.contentReference = value; 4878 return this; 4879 } 4880 4881 /** 4882 * @return Identifies the identity of an element defined elsewhere in the profile whose content rules should be applied to the current element. 4883 */ 4884 public String getContentReference() { 4885 return this.contentReference == null ? null : this.contentReference.getValue(); 4886 } 4887 4888 /** 4889 * @param value Identifies the identity of an element defined elsewhere in the profile whose content rules should be applied to the current element. 4890 */ 4891 public ElementDefinition setContentReference(String value) { 4892 if (Utilities.noString(value)) 4893 this.contentReference = null; 4894 else { 4895 if (this.contentReference == null) 4896 this.contentReference = new UriType(); 4897 this.contentReference.setValue(value); 4898 } 4899 return this; 4900 } 4901 4902 /** 4903 * @return {@link #type} (The data type or resource that the value of this element is permitted to be.) 4904 */ 4905 public List<TypeRefComponent> getType() { 4906 if (this.type == null) 4907 this.type = new ArrayList<TypeRefComponent>(); 4908 return this.type; 4909 } 4910 4911 /** 4912 * @return Returns a reference to <code>this</code> for easy method chaining 4913 */ 4914 public ElementDefinition setType(List<TypeRefComponent> theType) { 4915 this.type = theType; 4916 return this; 4917 } 4918 4919 public boolean hasType() { 4920 if (this.type == null) 4921 return false; 4922 for (TypeRefComponent item : this.type) 4923 if (!item.isEmpty()) 4924 return true; 4925 return false; 4926 } 4927 4928 public TypeRefComponent addType() { //3 4929 TypeRefComponent t = new TypeRefComponent(); 4930 if (this.type == null) 4931 this.type = new ArrayList<TypeRefComponent>(); 4932 this.type.add(t); 4933 return t; 4934 } 4935 4936 public ElementDefinition addType(TypeRefComponent t) { //3 4937 if (t == null) 4938 return this; 4939 if (this.type == null) 4940 this.type = new ArrayList<TypeRefComponent>(); 4941 this.type.add(t); 4942 return this; 4943 } 4944 4945 /** 4946 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 4947 */ 4948 public TypeRefComponent getTypeFirstRep() { 4949 if (getType().isEmpty()) { 4950 addType(); 4951 } 4952 return getType().get(0); 4953 } 4954 4955 /** 4956 * @return {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 4957 */ 4958 public org.hl7.fhir.dstu3.model.Type getDefaultValue() { 4959 return this.defaultValue; 4960 } 4961 4962 public boolean hasDefaultValue() { 4963 return this.defaultValue != null && !this.defaultValue.isEmpty(); 4964 } 4965 4966 /** 4967 * @param value {@link #defaultValue} (The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').) 4968 */ 4969 public ElementDefinition setDefaultValue(org.hl7.fhir.dstu3.model.Type value) { 4970 this.defaultValue = value; 4971 return this; 4972 } 4973 4974 /** 4975 * @return {@link #meaningWhenMissing} (The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'.). This is the underlying object with id, value and extensions. The accessor "getMeaningWhenMissing" gives direct access to the value 4976 */ 4977 public MarkdownType getMeaningWhenMissingElement() { 4978 if (this.meaningWhenMissing == null) 4979 if (Configuration.errorOnAutoCreate()) 4980 throw new Error("Attempt to auto-create ElementDefinition.meaningWhenMissing"); 4981 else if (Configuration.doAutoCreate()) 4982 this.meaningWhenMissing = new MarkdownType(); // bb 4983 return this.meaningWhenMissing; 4984 } 4985 4986 public boolean hasMeaningWhenMissingElement() { 4987 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 4988 } 4989 4990 public boolean hasMeaningWhenMissing() { 4991 return this.meaningWhenMissing != null && !this.meaningWhenMissing.isEmpty(); 4992 } 4993 4994 /** 4995 * @param value {@link #meaningWhenMissing} (The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'.). This is the underlying object with id, value and extensions. The accessor "getMeaningWhenMissing" gives direct access to the value 4996 */ 4997 public ElementDefinition setMeaningWhenMissingElement(MarkdownType value) { 4998 this.meaningWhenMissing = value; 4999 return this; 5000 } 5001 5002 /** 5003 * @return The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'. 5004 */ 5005 public String getMeaningWhenMissing() { 5006 return this.meaningWhenMissing == null ? null : this.meaningWhenMissing.getValue(); 5007 } 5008 5009 /** 5010 * @param value The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'. 5011 */ 5012 public ElementDefinition setMeaningWhenMissing(String value) { 5013 if (value == null) 5014 this.meaningWhenMissing = null; 5015 else { 5016 if (this.meaningWhenMissing == null) 5017 this.meaningWhenMissing = new MarkdownType(); 5018 this.meaningWhenMissing.setValue(value); 5019 } 5020 return this; 5021 } 5022 5023 /** 5024 * @return {@link #orderMeaning} (If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.). This is the underlying object with id, value and extensions. The accessor "getOrderMeaning" gives direct access to the value 5025 */ 5026 public StringType getOrderMeaningElement() { 5027 if (this.orderMeaning == null) 5028 if (Configuration.errorOnAutoCreate()) 5029 throw new Error("Attempt to auto-create ElementDefinition.orderMeaning"); 5030 else if (Configuration.doAutoCreate()) 5031 this.orderMeaning = new StringType(); // bb 5032 return this.orderMeaning; 5033 } 5034 5035 public boolean hasOrderMeaningElement() { 5036 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 5037 } 5038 5039 public boolean hasOrderMeaning() { 5040 return this.orderMeaning != null && !this.orderMeaning.isEmpty(); 5041 } 5042 5043 /** 5044 * @param value {@link #orderMeaning} (If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.). This is the underlying object with id, value and extensions. The accessor "getOrderMeaning" gives direct access to the value 5045 */ 5046 public ElementDefinition setOrderMeaningElement(StringType value) { 5047 this.orderMeaning = value; 5048 return this; 5049 } 5050 5051 /** 5052 * @return If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 5053 */ 5054 public String getOrderMeaning() { 5055 return this.orderMeaning == null ? null : this.orderMeaning.getValue(); 5056 } 5057 5058 /** 5059 * @param value If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning. 5060 */ 5061 public ElementDefinition setOrderMeaning(String value) { 5062 if (Utilities.noString(value)) 5063 this.orderMeaning = null; 5064 else { 5065 if (this.orderMeaning == null) 5066 this.orderMeaning = new StringType(); 5067 this.orderMeaning.setValue(value); 5068 } 5069 return this; 5070 } 5071 5072 /** 5073 * @return {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 5074 */ 5075 public org.hl7.fhir.dstu3.model.Type getFixed() { 5076 return this.fixed; 5077 } 5078 5079 public boolean hasFixed() { 5080 return this.fixed != null && !this.fixed.isEmpty(); 5081 } 5082 5083 /** 5084 * @param value {@link #fixed} (Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.) 5085 */ 5086 public ElementDefinition setFixed(org.hl7.fhir.dstu3.model.Type value) { 5087 this.fixed = value; 5088 return this; 5089 } 5090 5091 /** 5092 * @return {@link #pattern} (Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).) 5093 */ 5094 public org.hl7.fhir.dstu3.model.Type getPattern() { 5095 return this.pattern; 5096 } 5097 5098 public boolean hasPattern() { 5099 return this.pattern != null && !this.pattern.isEmpty(); 5100 } 5101 5102 /** 5103 * @param value {@link #pattern} (Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).) 5104 */ 5105 public ElementDefinition setPattern(org.hl7.fhir.dstu3.model.Type value) { 5106 this.pattern = value; 5107 return this; 5108 } 5109 5110 /** 5111 * @return {@link #example} (A sample value for this element demonstrating the type of information that would typically be found in the element.) 5112 */ 5113 public List<ElementDefinitionExampleComponent> getExample() { 5114 if (this.example == null) 5115 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 5116 return this.example; 5117 } 5118 5119 /** 5120 * @return Returns a reference to <code>this</code> for easy method chaining 5121 */ 5122 public ElementDefinition setExample(List<ElementDefinitionExampleComponent> theExample) { 5123 this.example = theExample; 5124 return this; 5125 } 5126 5127 public boolean hasExample() { 5128 if (this.example == null) 5129 return false; 5130 for (ElementDefinitionExampleComponent item : this.example) 5131 if (!item.isEmpty()) 5132 return true; 5133 return false; 5134 } 5135 5136 public ElementDefinitionExampleComponent addExample() { //3 5137 ElementDefinitionExampleComponent t = new ElementDefinitionExampleComponent(); 5138 if (this.example == null) 5139 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 5140 this.example.add(t); 5141 return t; 5142 } 5143 5144 public ElementDefinition addExample(ElementDefinitionExampleComponent t) { //3 5145 if (t == null) 5146 return this; 5147 if (this.example == null) 5148 this.example = new ArrayList<ElementDefinitionExampleComponent>(); 5149 this.example.add(t); 5150 return this; 5151 } 5152 5153 /** 5154 * @return The first repetition of repeating field {@link #example}, creating it if it does not already exist 5155 */ 5156 public ElementDefinitionExampleComponent getExampleFirstRep() { 5157 if (getExample().isEmpty()) { 5158 addExample(); 5159 } 5160 return getExample().get(0); 5161 } 5162 5163 /** 5164 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5165 */ 5166 public Type getMinValue() { 5167 return this.minValue; 5168 } 5169 5170 /** 5171 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5172 */ 5173 public DateType getMinValueDateType() throws FHIRException { 5174 if (this.minValue == null) 5175 return null; 5176 if (!(this.minValue instanceof DateType)) 5177 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5178 return (DateType) this.minValue; 5179 } 5180 5181 public boolean hasMinValueDateType() { 5182 return this != null && this.minValue instanceof DateType; 5183 } 5184 5185 /** 5186 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5187 */ 5188 public DateTimeType getMinValueDateTimeType() throws FHIRException { 5189 if (this.minValue == null) 5190 return null; 5191 if (!(this.minValue instanceof DateTimeType)) 5192 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5193 return (DateTimeType) this.minValue; 5194 } 5195 5196 public boolean hasMinValueDateTimeType() { 5197 return this != null && this.minValue instanceof DateTimeType; 5198 } 5199 5200 /** 5201 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5202 */ 5203 public InstantType getMinValueInstantType() throws FHIRException { 5204 if (this.minValue == null) 5205 return null; 5206 if (!(this.minValue instanceof InstantType)) 5207 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5208 return (InstantType) this.minValue; 5209 } 5210 5211 public boolean hasMinValueInstantType() { 5212 return this != null && this.minValue instanceof InstantType; 5213 } 5214 5215 /** 5216 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5217 */ 5218 public TimeType getMinValueTimeType() throws FHIRException { 5219 if (this.minValue == null) 5220 return null; 5221 if (!(this.minValue instanceof TimeType)) 5222 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5223 return (TimeType) this.minValue; 5224 } 5225 5226 public boolean hasMinValueTimeType() { 5227 return this != null && this.minValue instanceof TimeType; 5228 } 5229 5230 /** 5231 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5232 */ 5233 public DecimalType getMinValueDecimalType() throws FHIRException { 5234 if (this.minValue == null) 5235 return null; 5236 if (!(this.minValue instanceof DecimalType)) 5237 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5238 return (DecimalType) this.minValue; 5239 } 5240 5241 public boolean hasMinValueDecimalType() { 5242 return this != null && this.minValue instanceof DecimalType; 5243 } 5244 5245 /** 5246 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5247 */ 5248 public IntegerType getMinValueIntegerType() throws FHIRException { 5249 if (this.minValue == null) 5250 return null; 5251 if (!(this.minValue instanceof IntegerType)) 5252 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5253 return (IntegerType) this.minValue; 5254 } 5255 5256 public boolean hasMinValueIntegerType() { 5257 return this != null && this.minValue instanceof IntegerType; 5258 } 5259 5260 /** 5261 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5262 */ 5263 public PositiveIntType getMinValuePositiveIntType() throws FHIRException { 5264 if (this.minValue == null) 5265 return null; 5266 if (!(this.minValue instanceof PositiveIntType)) 5267 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5268 return (PositiveIntType) this.minValue; 5269 } 5270 5271 public boolean hasMinValuePositiveIntType() { 5272 return this != null && this.minValue instanceof PositiveIntType; 5273 } 5274 5275 /** 5276 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5277 */ 5278 public UnsignedIntType getMinValueUnsignedIntType() throws FHIRException { 5279 if (this.minValue == null) 5280 return null; 5281 if (!(this.minValue instanceof UnsignedIntType)) 5282 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5283 return (UnsignedIntType) this.minValue; 5284 } 5285 5286 public boolean hasMinValueUnsignedIntType() { 5287 return this != null && this.minValue instanceof UnsignedIntType; 5288 } 5289 5290 /** 5291 * @return {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5292 */ 5293 public Quantity getMinValueQuantity() throws FHIRException { 5294 if (this.minValue == null) 5295 return null; 5296 if (!(this.minValue instanceof Quantity)) 5297 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.minValue.getClass().getName()+" was encountered"); 5298 return (Quantity) this.minValue; 5299 } 5300 5301 public boolean hasMinValueQuantity() { 5302 return this != null && this.minValue instanceof Quantity; 5303 } 5304 5305 public boolean hasMinValue() { 5306 return this.minValue != null && !this.minValue.isEmpty(); 5307 } 5308 5309 /** 5310 * @param value {@link #minValue} (The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5311 */ 5312 public ElementDefinition setMinValue(Type value) throws FHIRFormatError { 5313 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 5314 throw new FHIRFormatError("Not the right type for ElementDefinition.minValue[x]: "+value.fhirType()); 5315 this.minValue = value; 5316 return this; 5317 } 5318 5319 /** 5320 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5321 */ 5322 public Type getMaxValue() { 5323 return this.maxValue; 5324 } 5325 5326 /** 5327 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5328 */ 5329 public DateType getMaxValueDateType() throws FHIRException { 5330 if (this.maxValue == null) 5331 return null; 5332 if (!(this.maxValue instanceof DateType)) 5333 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5334 return (DateType) this.maxValue; 5335 } 5336 5337 public boolean hasMaxValueDateType() { 5338 return this != null && this.maxValue instanceof DateType; 5339 } 5340 5341 /** 5342 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5343 */ 5344 public DateTimeType getMaxValueDateTimeType() throws FHIRException { 5345 if (this.maxValue == null) 5346 return null; 5347 if (!(this.maxValue instanceof DateTimeType)) 5348 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5349 return (DateTimeType) this.maxValue; 5350 } 5351 5352 public boolean hasMaxValueDateTimeType() { 5353 return this != null && this.maxValue instanceof DateTimeType; 5354 } 5355 5356 /** 5357 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5358 */ 5359 public InstantType getMaxValueInstantType() throws FHIRException { 5360 if (this.maxValue == null) 5361 return null; 5362 if (!(this.maxValue instanceof InstantType)) 5363 throw new FHIRException("Type mismatch: the type InstantType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5364 return (InstantType) this.maxValue; 5365 } 5366 5367 public boolean hasMaxValueInstantType() { 5368 return this != null && this.maxValue instanceof InstantType; 5369 } 5370 5371 /** 5372 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5373 */ 5374 public TimeType getMaxValueTimeType() throws FHIRException { 5375 if (this.maxValue == null) 5376 return null; 5377 if (!(this.maxValue instanceof TimeType)) 5378 throw new FHIRException("Type mismatch: the type TimeType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5379 return (TimeType) this.maxValue; 5380 } 5381 5382 public boolean hasMaxValueTimeType() { 5383 return this != null && this.maxValue instanceof TimeType; 5384 } 5385 5386 /** 5387 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5388 */ 5389 public DecimalType getMaxValueDecimalType() throws FHIRException { 5390 if (this.maxValue == null) 5391 return null; 5392 if (!(this.maxValue instanceof DecimalType)) 5393 throw new FHIRException("Type mismatch: the type DecimalType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5394 return (DecimalType) this.maxValue; 5395 } 5396 5397 public boolean hasMaxValueDecimalType() { 5398 return this != null && this.maxValue instanceof DecimalType; 5399 } 5400 5401 /** 5402 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5403 */ 5404 public IntegerType getMaxValueIntegerType() throws FHIRException { 5405 if (this.maxValue == null) 5406 return null; 5407 if (!(this.maxValue instanceof IntegerType)) 5408 throw new FHIRException("Type mismatch: the type IntegerType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5409 return (IntegerType) this.maxValue; 5410 } 5411 5412 public boolean hasMaxValueIntegerType() { 5413 return this != null && this.maxValue instanceof IntegerType; 5414 } 5415 5416 /** 5417 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5418 */ 5419 public PositiveIntType getMaxValuePositiveIntType() throws FHIRException { 5420 if (this.maxValue == null) 5421 return null; 5422 if (!(this.maxValue instanceof PositiveIntType)) 5423 throw new FHIRException("Type mismatch: the type PositiveIntType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5424 return (PositiveIntType) this.maxValue; 5425 } 5426 5427 public boolean hasMaxValuePositiveIntType() { 5428 return this != null && this.maxValue instanceof PositiveIntType; 5429 } 5430 5431 /** 5432 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5433 */ 5434 public UnsignedIntType getMaxValueUnsignedIntType() throws FHIRException { 5435 if (this.maxValue == null) 5436 return null; 5437 if (!(this.maxValue instanceof UnsignedIntType)) 5438 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5439 return (UnsignedIntType) this.maxValue; 5440 } 5441 5442 public boolean hasMaxValueUnsignedIntType() { 5443 return this != null && this.maxValue instanceof UnsignedIntType; 5444 } 5445 5446 /** 5447 * @return {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5448 */ 5449 public Quantity getMaxValueQuantity() throws FHIRException { 5450 if (this.maxValue == null) 5451 return null; 5452 if (!(this.maxValue instanceof Quantity)) 5453 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.maxValue.getClass().getName()+" was encountered"); 5454 return (Quantity) this.maxValue; 5455 } 5456 5457 public boolean hasMaxValueQuantity() { 5458 return this != null && this.maxValue instanceof Quantity; 5459 } 5460 5461 public boolean hasMaxValue() { 5462 return this.maxValue != null && !this.maxValue.isEmpty(); 5463 } 5464 5465 /** 5466 * @param value {@link #maxValue} (The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.) 5467 */ 5468 public ElementDefinition setMaxValue(Type value) throws FHIRFormatError { 5469 if (value != null && !(value instanceof DateType || value instanceof DateTimeType || value instanceof InstantType || value instanceof TimeType || value instanceof DecimalType || value instanceof IntegerType || value instanceof PositiveIntType || value instanceof UnsignedIntType || value instanceof Quantity)) 5470 throw new FHIRFormatError("Not the right type for ElementDefinition.maxValue[x]: "+value.fhirType()); 5471 this.maxValue = value; 5472 return this; 5473 } 5474 5475 /** 5476 * @return {@link #maxLength} (Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 5477 */ 5478 public IntegerType getMaxLengthElement() { 5479 if (this.maxLength == null) 5480 if (Configuration.errorOnAutoCreate()) 5481 throw new Error("Attempt to auto-create ElementDefinition.maxLength"); 5482 else if (Configuration.doAutoCreate()) 5483 this.maxLength = new IntegerType(); // bb 5484 return this.maxLength; 5485 } 5486 5487 public boolean hasMaxLengthElement() { 5488 return this.maxLength != null && !this.maxLength.isEmpty(); 5489 } 5490 5491 public boolean hasMaxLength() { 5492 return this.maxLength != null && !this.maxLength.isEmpty(); 5493 } 5494 5495 /** 5496 * @param value {@link #maxLength} (Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.). This is the underlying object with id, value and extensions. The accessor "getMaxLength" gives direct access to the value 5497 */ 5498 public ElementDefinition setMaxLengthElement(IntegerType value) { 5499 this.maxLength = value; 5500 return this; 5501 } 5502 5503 /** 5504 * @return Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. 5505 */ 5506 public int getMaxLength() { 5507 return this.maxLength == null || this.maxLength.isEmpty() ? 0 : this.maxLength.getValue(); 5508 } 5509 5510 /** 5511 * @param value Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element. 5512 */ 5513 public ElementDefinition setMaxLength(int value) { 5514 if (this.maxLength == null) 5515 this.maxLength = new IntegerType(); 5516 this.maxLength.setValue(value); 5517 return this; 5518 } 5519 5520 /** 5521 * @return {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 5522 */ 5523 public List<IdType> getCondition() { 5524 if (this.condition == null) 5525 this.condition = new ArrayList<IdType>(); 5526 return this.condition; 5527 } 5528 5529 /** 5530 * @return Returns a reference to <code>this</code> for easy method chaining 5531 */ 5532 public ElementDefinition setCondition(List<IdType> theCondition) { 5533 this.condition = theCondition; 5534 return this; 5535 } 5536 5537 public boolean hasCondition() { 5538 if (this.condition == null) 5539 return false; 5540 for (IdType item : this.condition) 5541 if (!item.isEmpty()) 5542 return true; 5543 return false; 5544 } 5545 5546 /** 5547 * @return {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 5548 */ 5549 public IdType addConditionElement() {//2 5550 IdType t = new IdType(); 5551 if (this.condition == null) 5552 this.condition = new ArrayList<IdType>(); 5553 this.condition.add(t); 5554 return t; 5555 } 5556 5557 /** 5558 * @param value {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 5559 */ 5560 public ElementDefinition addCondition(String value) { //1 5561 IdType t = new IdType(); 5562 t.setValue(value); 5563 if (this.condition == null) 5564 this.condition = new ArrayList<IdType>(); 5565 this.condition.add(t); 5566 return this; 5567 } 5568 5569 /** 5570 * @param value {@link #condition} (A reference to an invariant that may make additional statements about the cardinality or value in the instance.) 5571 */ 5572 public boolean hasCondition(String value) { 5573 if (this.condition == null) 5574 return false; 5575 for (IdType v : this.condition) 5576 if (v.getValue().equals(value)) // id 5577 return true; 5578 return false; 5579 } 5580 5581 /** 5582 * @return {@link #constraint} (Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.) 5583 */ 5584 public List<ElementDefinitionConstraintComponent> getConstraint() { 5585 if (this.constraint == null) 5586 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 5587 return this.constraint; 5588 } 5589 5590 /** 5591 * @return Returns a reference to <code>this</code> for easy method chaining 5592 */ 5593 public ElementDefinition setConstraint(List<ElementDefinitionConstraintComponent> theConstraint) { 5594 this.constraint = theConstraint; 5595 return this; 5596 } 5597 5598 public boolean hasConstraint() { 5599 if (this.constraint == null) 5600 return false; 5601 for (ElementDefinitionConstraintComponent item : this.constraint) 5602 if (!item.isEmpty()) 5603 return true; 5604 return false; 5605 } 5606 5607 public ElementDefinitionConstraintComponent addConstraint() { //3 5608 ElementDefinitionConstraintComponent t = new ElementDefinitionConstraintComponent(); 5609 if (this.constraint == null) 5610 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 5611 this.constraint.add(t); 5612 return t; 5613 } 5614 5615 public ElementDefinition addConstraint(ElementDefinitionConstraintComponent t) { //3 5616 if (t == null) 5617 return this; 5618 if (this.constraint == null) 5619 this.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 5620 this.constraint.add(t); 5621 return this; 5622 } 5623 5624 /** 5625 * @return The first repetition of repeating field {@link #constraint}, creating it if it does not already exist 5626 */ 5627 public ElementDefinitionConstraintComponent getConstraintFirstRep() { 5628 if (getConstraint().isEmpty()) { 5629 addConstraint(); 5630 } 5631 return getConstraint().get(0); 5632 } 5633 5634 /** 5635 * @return {@link #mustSupport} (If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. If false, the element may be ignored and not supported.). This is the underlying object with id, value and extensions. The accessor "getMustSupport" gives direct access to the value 5636 */ 5637 public BooleanType getMustSupportElement() { 5638 if (this.mustSupport == null) 5639 if (Configuration.errorOnAutoCreate()) 5640 throw new Error("Attempt to auto-create ElementDefinition.mustSupport"); 5641 else if (Configuration.doAutoCreate()) 5642 this.mustSupport = new BooleanType(); // bb 5643 return this.mustSupport; 5644 } 5645 5646 public boolean hasMustSupportElement() { 5647 return this.mustSupport != null && !this.mustSupport.isEmpty(); 5648 } 5649 5650 public boolean hasMustSupport() { 5651 return this.mustSupport != null && !this.mustSupport.isEmpty(); 5652 } 5653 5654 /** 5655 * @param value {@link #mustSupport} (If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. If false, the element may be ignored and not supported.). This is the underlying object with id, value and extensions. The accessor "getMustSupport" gives direct access to the value 5656 */ 5657 public ElementDefinition setMustSupportElement(BooleanType value) { 5658 this.mustSupport = value; 5659 return this; 5660 } 5661 5662 /** 5663 * @return If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. If false, the element may be ignored and not supported. 5664 */ 5665 public boolean getMustSupport() { 5666 return this.mustSupport == null || this.mustSupport.isEmpty() ? false : this.mustSupport.getValue(); 5667 } 5668 5669 /** 5670 * @param value If true, implementations that produce or consume resources SHALL provide "support" for the element in some meaningful way. If false, the element may be ignored and not supported. 5671 */ 5672 public ElementDefinition setMustSupport(boolean value) { 5673 if (this.mustSupport == null) 5674 this.mustSupport = new BooleanType(); 5675 this.mustSupport.setValue(value); 5676 return this; 5677 } 5678 5679 /** 5680 * @return {@link #isModifier} (If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.). This is the underlying object with id, value and extensions. The accessor "getIsModifier" gives direct access to the value 5681 */ 5682 public BooleanType getIsModifierElement() { 5683 if (this.isModifier == null) 5684 if (Configuration.errorOnAutoCreate()) 5685 throw new Error("Attempt to auto-create ElementDefinition.isModifier"); 5686 else if (Configuration.doAutoCreate()) 5687 this.isModifier = new BooleanType(); // bb 5688 return this.isModifier; 5689 } 5690 5691 public boolean hasIsModifierElement() { 5692 return this.isModifier != null && !this.isModifier.isEmpty(); 5693 } 5694 5695 public boolean hasIsModifier() { 5696 return this.isModifier != null && !this.isModifier.isEmpty(); 5697 } 5698 5699 /** 5700 * @param value {@link #isModifier} (If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.). This is the underlying object with id, value and extensions. The accessor "getIsModifier" gives direct access to the value 5701 */ 5702 public ElementDefinition setIsModifierElement(BooleanType value) { 5703 this.isModifier = value; 5704 return this; 5705 } 5706 5707 /** 5708 * @return If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. 5709 */ 5710 public boolean getIsModifier() { 5711 return this.isModifier == null || this.isModifier.isEmpty() ? false : this.isModifier.getValue(); 5712 } 5713 5714 /** 5715 * @param value If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system. 5716 */ 5717 public ElementDefinition setIsModifier(boolean value) { 5718 if (this.isModifier == null) 5719 this.isModifier = new BooleanType(); 5720 this.isModifier.setValue(value); 5721 return this; 5722 } 5723 5724 /** 5725 * @return {@link #isSummary} (Whether the element should be included if a client requests a search with the parameter _summary=true.). This is the underlying object with id, value and extensions. The accessor "getIsSummary" gives direct access to the value 5726 */ 5727 public BooleanType getIsSummaryElement() { 5728 if (this.isSummary == null) 5729 if (Configuration.errorOnAutoCreate()) 5730 throw new Error("Attempt to auto-create ElementDefinition.isSummary"); 5731 else if (Configuration.doAutoCreate()) 5732 this.isSummary = new BooleanType(); // bb 5733 return this.isSummary; 5734 } 5735 5736 public boolean hasIsSummaryElement() { 5737 return this.isSummary != null && !this.isSummary.isEmpty(); 5738 } 5739 5740 public boolean hasIsSummary() { 5741 return this.isSummary != null && !this.isSummary.isEmpty(); 5742 } 5743 5744 /** 5745 * @param value {@link #isSummary} (Whether the element should be included if a client requests a search with the parameter _summary=true.). This is the underlying object with id, value and extensions. The accessor "getIsSummary" gives direct access to the value 5746 */ 5747 public ElementDefinition setIsSummaryElement(BooleanType value) { 5748 this.isSummary = value; 5749 return this; 5750 } 5751 5752 /** 5753 * @return Whether the element should be included if a client requests a search with the parameter _summary=true. 5754 */ 5755 public boolean getIsSummary() { 5756 return this.isSummary == null || this.isSummary.isEmpty() ? false : this.isSummary.getValue(); 5757 } 5758 5759 /** 5760 * @param value Whether the element should be included if a client requests a search with the parameter _summary=true. 5761 */ 5762 public ElementDefinition setIsSummary(boolean value) { 5763 if (this.isSummary == null) 5764 this.isSummary = new BooleanType(); 5765 this.isSummary.setValue(value); 5766 return this; 5767 } 5768 5769 /** 5770 * @return {@link #binding} (Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).) 5771 */ 5772 public ElementDefinitionBindingComponent getBinding() { 5773 if (this.binding == null) 5774 if (Configuration.errorOnAutoCreate()) 5775 throw new Error("Attempt to auto-create ElementDefinition.binding"); 5776 else if (Configuration.doAutoCreate()) 5777 this.binding = new ElementDefinitionBindingComponent(); // cc 5778 return this.binding; 5779 } 5780 5781 public boolean hasBinding() { 5782 return this.binding != null && !this.binding.isEmpty(); 5783 } 5784 5785 /** 5786 * @param value {@link #binding} (Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).) 5787 */ 5788 public ElementDefinition setBinding(ElementDefinitionBindingComponent value) { 5789 this.binding = value; 5790 return this; 5791 } 5792 5793 /** 5794 * @return {@link #mapping} (Identifies a concept from an external specification that roughly corresponds to this element.) 5795 */ 5796 public List<ElementDefinitionMappingComponent> getMapping() { 5797 if (this.mapping == null) 5798 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 5799 return this.mapping; 5800 } 5801 5802 /** 5803 * @return Returns a reference to <code>this</code> for easy method chaining 5804 */ 5805 public ElementDefinition setMapping(List<ElementDefinitionMappingComponent> theMapping) { 5806 this.mapping = theMapping; 5807 return this; 5808 } 5809 5810 public boolean hasMapping() { 5811 if (this.mapping == null) 5812 return false; 5813 for (ElementDefinitionMappingComponent item : this.mapping) 5814 if (!item.isEmpty()) 5815 return true; 5816 return false; 5817 } 5818 5819 public ElementDefinitionMappingComponent addMapping() { //3 5820 ElementDefinitionMappingComponent t = new ElementDefinitionMappingComponent(); 5821 if (this.mapping == null) 5822 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 5823 this.mapping.add(t); 5824 return t; 5825 } 5826 5827 public ElementDefinition addMapping(ElementDefinitionMappingComponent t) { //3 5828 if (t == null) 5829 return this; 5830 if (this.mapping == null) 5831 this.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 5832 this.mapping.add(t); 5833 return this; 5834 } 5835 5836 /** 5837 * @return The first repetition of repeating field {@link #mapping}, creating it if it does not already exist 5838 */ 5839 public ElementDefinitionMappingComponent getMappingFirstRep() { 5840 if (getMapping().isEmpty()) { 5841 addMapping(); 5842 } 5843 return getMapping().get(0); 5844 } 5845 5846 protected void listChildren(List<Property> children) { 5847 super.listChildren(children); 5848 children.add(new Property("path", "string", "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 0, 1, path)); 5849 children.add(new Property("representation", "code", "Codes that define how this element is represented in instances, when the deviation varies from the normal case.", 0, java.lang.Integer.MAX_VALUE, representation)); 5850 children.add(new Property("sliceName", "string", "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 0, 1, sliceName)); 5851 children.add(new Property("label", "string", "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 0, 1, label)); 5852 children.add(new Property("code", "Coding", "A code that has the same meaning as the element in a particular terminology.", 0, java.lang.Integer.MAX_VALUE, code)); 5853 children.add(new Property("slicing", "", "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 0, 1, slicing)); 5854 children.add(new Property("short", "string", "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_)); 5855 children.add(new Property("definition", "markdown", "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource.", 0, 1, definition)); 5856 children.add(new Property("comment", "markdown", "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc.", 0, 1, comment)); 5857 children.add(new Property("requirements", "markdown", "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 0, 1, requirements)); 5858 children.add(new Property("alias", "string", "Identifies additional names by which this element might also be known.", 0, java.lang.Integer.MAX_VALUE, alias)); 5859 children.add(new Property("min", "unsignedInt", "The minimum number of times this element SHALL appear in the instance.", 0, 1, min)); 5860 children.add(new Property("max", "string", "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max)); 5861 children.add(new Property("base", "", "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. This information is provided when the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot.", 0, 1, base)); 5862 children.add(new Property("contentReference", "uri", "Identifies the identity of an element defined elsewhere in the profile whose content rules should be applied to the current element.", 0, 1, contentReference)); 5863 children.add(new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, java.lang.Integer.MAX_VALUE, type)); 5864 children.add(new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue)); 5865 children.add(new Property("meaningWhenMissing", "markdown", "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'.", 0, 1, meaningWhenMissing)); 5866 children.add(new Property("orderMeaning", "string", "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 0, 1, orderMeaning)); 5867 children.add(new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed)); 5868 children.add(new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern)); 5869 children.add(new Property("example", "", "A sample value for this element demonstrating the type of information that would typically be found in the element.", 0, java.lang.Integer.MAX_VALUE, example)); 5870 children.add(new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue)); 5871 children.add(new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue)); 5872 children.add(new Property("maxLength", "integer", "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.", 0, 1, maxLength)); 5873 children.add(new Property("condition", "id", "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 0, java.lang.Integer.MAX_VALUE, condition)); 5874 children.add(new Property("constraint", "", "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 0, java.lang.Integer.MAX_VALUE, constraint)); 5875 children.add(new Property("mustSupport", "boolean", "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported.", 0, 1, mustSupport)); 5876 children.add(new Property("isModifier", "boolean", "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.", 0, 1, isModifier)); 5877 children.add(new Property("isSummary", "boolean", "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 1, isSummary)); 5878 children.add(new Property("binding", "", "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 0, 1, binding)); 5879 children.add(new Property("mapping", "", "Identifies a concept from an external specification that roughly corresponds to this element.", 0, java.lang.Integer.MAX_VALUE, mapping)); 5880 } 5881 5882 @Override 5883 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5884 switch (_hash) { 5885 case 3433509: /*path*/ return new Property("path", "string", "The path identifies the element and is expressed as a \".\"-separated list of ancestor elements, beginning with the name of the resource or extension.", 0, 1, path); 5886 case -671065907: /*representation*/ return new Property("representation", "code", "Codes that define how this element is represented in instances, when the deviation varies from the normal case.", 0, java.lang.Integer.MAX_VALUE, representation); 5887 case -825289923: /*sliceName*/ return new Property("sliceName", "string", "The name of this element definition slice, when slicing is working. The name must be a token with no dots or spaces. This is a unique name referring to a specific set of constraints applied to this element, used to provide a name to different slices of the same element.", 0, 1, sliceName); 5888 case 102727412: /*label*/ return new Property("label", "string", "A single preferred label which is the text to display beside the element indicating its meaning or to use to prompt for the element in a user display or form.", 0, 1, label); 5889 case 3059181: /*code*/ return new Property("code", "Coding", "A code that has the same meaning as the element in a particular terminology.", 0, java.lang.Integer.MAX_VALUE, code); 5890 case -2119287345: /*slicing*/ return new Property("slicing", "", "Indicates that the element is sliced into a set of alternative definitions (i.e. in a structure definition, there are multiple different constraints on a single element in the base resource). Slicing can be used in any resource that has cardinality ..* on the base resource, or any resource with a choice of types. The set of slices is any elements that come after this in the element sequence that have the same path, until a shorter path occurs (the shorter path terminates the set).", 0, 1, slicing); 5891 case 109413500: /*short*/ return new Property("short", "string", "A concise description of what this element means (e.g. for use in autogenerated summaries).", 0, 1, short_); 5892 case -1014418093: /*definition*/ return new Property("definition", "markdown", "Provides a complete explanation of the meaning of the data element for human readability. For the case of elements derived from existing elements (e.g. constraints), the definition SHALL be consistent with the base definition, but convey the meaning of the element in the particular context of use of the resource.", 0, 1, definition); 5893 case 950398559: /*comment*/ return new Property("comment", "markdown", "Explanatory notes and implementation guidance about the data element, including notes about how to use the data properly, exceptions to proper use, etc.", 0, 1, comment); 5894 case -1619874672: /*requirements*/ return new Property("requirements", "markdown", "This element is for traceability of why the element was created and why the constraints exist as they do. This may be used to point to source materials or specifications that drove the structure of this element.", 0, 1, requirements); 5895 case 92902992: /*alias*/ return new Property("alias", "string", "Identifies additional names by which this element might also be known.", 0, java.lang.Integer.MAX_VALUE, alias); 5896 case 108114: /*min*/ return new Property("min", "unsignedInt", "The minimum number of times this element SHALL appear in the instance.", 0, 1, min); 5897 case 107876: /*max*/ return new Property("max", "string", "The maximum number of times this element is permitted to appear in the instance.", 0, 1, max); 5898 case 3016401: /*base*/ return new Property("base", "", "Information about the base definition of the element, provided to make it unnecessary for tools to trace the deviation of the element through the derived and related profiles. This information is provided when the element definition is not the original definition of an element - i.g. either in a constraint on another type, or for elements from a super type in a snap shot.", 0, 1, base); 5899 case 1193747154: /*contentReference*/ return new Property("contentReference", "uri", "Identifies the identity of an element defined elsewhere in the profile whose content rules should be applied to the current element.", 0, 1, contentReference); 5900 case 3575610: /*type*/ return new Property("type", "", "The data type or resource that the value of this element is permitted to be.", 0, java.lang.Integer.MAX_VALUE, type); 5901 case 587922128: /*defaultValue[x]*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5902 case -659125328: /*defaultValue*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5903 case 1470297600: /*defaultValueBase64Binary*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5904 case 600437336: /*defaultValueBoolean*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5905 case 264593188: /*defaultValueCanonical*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5906 case 1044993469: /*defaultValueCode*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5907 case 1045010302: /*defaultValueDate*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5908 case 1220374379: /*defaultValueDateTime*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5909 case 2077989249: /*defaultValueDecimal*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5910 case -2059245333: /*defaultValueId*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5911 case -1801671663: /*defaultValueInstant*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5912 case -1801189522: /*defaultValueInteger*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5913 case -325436225: /*defaultValueMarkdown*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5914 case 587910138: /*defaultValueOid*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5915 case -737344154: /*defaultValuePositiveInt*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5916 case -320515103: /*defaultValueString*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5917 case 1045494429: /*defaultValueTime*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5918 case 539117290: /*defaultValueUnsignedInt*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5919 case 587916188: /*defaultValueUri*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5920 case 587916191: /*defaultValueUrl*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5921 case 1045535627: /*defaultValueUuid*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5922 case -611966428: /*defaultValueAddress*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5923 case -1851689217: /*defaultValueAnnotation*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5924 case 2034820339: /*defaultValueAttachment*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5925 case -410434095: /*defaultValueCodeableConcept*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5926 case -783616198: /*defaultValueCoding*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5927 case -344740576: /*defaultValueContactPoint*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5928 case -975393912: /*defaultValueHumanName*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5929 case -1915078535: /*defaultValueIdentifier*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5930 case -420255343: /*defaultValuePeriod*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5931 case -1857379237: /*defaultValueQuantity*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5932 case -1951495315: /*defaultValueRange*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5933 case -1951489477: /*defaultValueRatio*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5934 case -1488914053: /*defaultValueReference*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5935 case -449641228: /*defaultValueSampledData*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5936 case 509825768: /*defaultValueSignature*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5937 case -302193638: /*defaultValueTiming*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5938 case -754548089: /*defaultValueDosage*/ return new Property("defaultValue[x]", "*", "The value that should be used if there is no value stated in the instance (e.g. 'if not otherwise specified, the abstract is false').", 0, 1, defaultValue); 5939 case 1857257103: /*meaningWhenMissing*/ return new Property("meaningWhenMissing", "markdown", "The Implicit meaning that is to be understood when this element is missing (e.g. 'when this element is missing, the period is ongoing'.", 0, 1, meaningWhenMissing); 5940 case 1828196047: /*orderMeaning*/ return new Property("orderMeaning", "string", "If present, indicates that the order of the repeating element has meaning and describes what that meaning is. If absent, it means that the order of the element has no meaning.", 0, 1, orderMeaning); 5941 case -391522164: /*fixed[x]*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5942 case 97445748: /*fixed*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5943 case -799290428: /*fixedBase64Binary*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5944 case 520851988: /*fixedBoolean*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5945 case 1092485088: /*fixedCanonical*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5946 case 746991489: /*fixedCode*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5947 case 747008322: /*fixedDate*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5948 case -1246771409: /*fixedDateTime*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5949 case 1998403901: /*fixedDecimal*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5950 case -843914321: /*fixedId*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5951 case -1881257011: /*fixedInstant*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5952 case -1880774870: /*fixedInteger*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5953 case 1502385283: /*fixedMarkdown*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5954 case -391534154: /*fixedOid*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5955 case 297821986: /*fixedPositiveInt*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5956 case 1062390949: /*fixedString*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5957 case 747492449: /*fixedTime*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5958 case 1574283430: /*fixedUnsignedInt*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5959 case -391528104: /*fixedUri*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5960 case -391528101: /*fixedUrl*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5961 case 747533647: /*fixedUuid*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5962 case -691551776: /*fixedAddress*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5963 case -1956844093: /*fixedAnnotation*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5964 case 1929665463: /*fixedAttachment*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5965 case 1962764685: /*fixedCodeableConcept*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5966 case 599289854: /*fixedCoding*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5967 case 1680638692: /*fixedContactPoint*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5968 case -147502012: /*fixedHumanName*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5969 case -2020233411: /*fixedIdentifier*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5970 case 962650709: /*fixedPeriod*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5971 case -29557729: /*fixedQuantity*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5972 case 1695345193: /*fixedRange*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5973 case 1695351031: /*fixedRatio*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5974 case -661022153: /*fixedReference*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5975 case 585524912: /*fixedSampledData*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5976 case 1337717668: /*fixedSignature*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5977 case 1080712414: /*fixedTiming*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5978 case 628357963: /*fixedDosage*/ return new Property("fixed[x]", "*", "Specifies a value that SHALL be exactly the value for this element in the instance. For purposes of comparison, non-significant whitespace is ignored, and all values must be an exact match (case and accent sensitive). Missing elements/attributes must also be missing.", 0, 1, fixed); 5979 case -885125392: /*pattern[x]*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5980 case -791090288: /*pattern*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5981 case 2127857120: /*patternBase64Binary*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5982 case -1776945544: /*patternBoolean*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5983 case 522246980: /*patternCanonical*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5984 case -1669806691: /*patternCode*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5985 case -1669789858: /*patternDate*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5986 case 535949131: /*patternDateTime*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5987 case -299393631: /*patternDecimal*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5988 case -28553013: /*patternId*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5989 case 115912753: /*patternInstant*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5990 case 116394894: /*patternInteger*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5991 case -1009861473: /*patternMarkdown*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5992 case -885137382: /*patternOid*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5993 case 2054814086: /*patternPositiveInt*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5994 case 2096647105: /*patternString*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5995 case -1669305731: /*patternTime*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5996 case -963691766: /*patternUnsignedInt*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5997 case -885131332: /*patternUri*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5998 case -885131329: /*patternUrl*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 5999 case -1669264533: /*patternUuid*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6000 case 1305617988: /*patternAddress*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6001 case 1840611039: /*patternAnnotation*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6002 case 1432153299: /*patternAttachment*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6003 case -400610831: /*patternCodeableConcept*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6004 case 1633546010: /*patternCoding*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6005 case 312818944: /*patternContactPoint*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6006 case -717740120: /*patternHumanName*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6007 case 1777221721: /*patternIdentifier*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6008 case 1996906865: /*patternPeriod*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6009 case 1753162811: /*patternQuantity*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6010 case -210954355: /*patternRange*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6011 case -210948517: /*patternRatio*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6012 case -1231260261: /*patternReference*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6013 case -1952450284: /*patternSampledData*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6014 case 767479560: /*patternSignature*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6015 case 2114968570: /*patternTiming*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6016 case 1662614119: /*patternDosage*/ return new Property("pattern[x]", "*", "Specifies a value that the value in the instance SHALL follow - that is, any value in the pattern must be found in the instance. Other additional values may be found too. This is effectively constraint by example. The values of elements present in the pattern must match exactly (case-sensitive, accent-sensitive, etc.).", 0, 1, pattern); 6017 case -1322970774: /*example*/ return new Property("example", "", "A sample value for this element demonstrating the type of information that would typically be found in the element.", 0, java.lang.Integer.MAX_VALUE, example); 6018 case -55301663: /*minValue[x]*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6019 case -1376969153: /*minValue*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6020 case -1715058035: /*minValueDate*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6021 case 1635517178: /*minValueDateTime*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6022 case 151382690: /*minValueInstant*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6023 case -1714573908: /*minValueTime*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6024 case -263923694: /*minValueDecimal*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6025 case 151864831: /*minValueInteger*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6026 case 1570935671: /*minValuePositiveInt*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6027 case -1447570181: /*minValueUnsignedInt*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6028 case -1442236438: /*minValueQuantity*/ return new Property("minValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The minimum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, minValue); 6029 case 622130931: /*maxValue[x]*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6030 case 399227501: /*maxValue*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6031 case 2105483195: /*maxValueDate*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6032 case 1699385640: /*maxValueDateTime*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6033 case 1261821620: /*maxValueInstant*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6034 case 2105967322: /*maxValueTime*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6035 case 846515236: /*maxValueDecimal*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6036 case 1262303761: /*maxValueInteger*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6037 case 1605774985: /*maxValuePositiveInt*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6038 case -1412730867: /*maxValueUnsignedInt*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6039 case -1378367976: /*maxValueQuantity*/ return new Property("maxValue[x]", "date|dateTime|instant|time|decimal|integer|positiveInt|unsignedInt|Quantity", "The maximum allowed value for the element. The value is inclusive. This is allowed for the types date, dateTime, instant, time, decimal, integer, and Quantity.", 0, 1, maxValue); 6040 case -791400086: /*maxLength*/ return new Property("maxLength", "integer", "Indicates the maximum length in characters that is permitted to be present in conformant instances and which is expected to be supported by conformant consumers that support the element.", 0, 1, maxLength); 6041 case -861311717: /*condition*/ return new Property("condition", "id", "A reference to an invariant that may make additional statements about the cardinality or value in the instance.", 0, java.lang.Integer.MAX_VALUE, condition); 6042 case -190376483: /*constraint*/ return new Property("constraint", "", "Formal constraints such as co-occurrence and other constraints that can be computationally evaluated within the context of the instance.", 0, java.lang.Integer.MAX_VALUE, constraint); 6043 case -1402857082: /*mustSupport*/ return new Property("mustSupport", "boolean", "If true, implementations that produce or consume resources SHALL provide \"support\" for the element in some meaningful way. If false, the element may be ignored and not supported.", 0, 1, mustSupport); 6044 case -1408783839: /*isModifier*/ return new Property("isModifier", "boolean", "If true, the value of this element affects the interpretation of the element or resource that contains it, and the value of the element cannot be ignored. Typically, this is used for status, negation and qualification codes. The effect of this is that the element cannot be ignored by systems: they SHALL either recognize the element and process it, and/or a pre-determination has been made that it is not relevant to their particular system.", 0, 1, isModifier); 6045 case 1857548060: /*isSummary*/ return new Property("isSummary", "boolean", "Whether the element should be included if a client requests a search with the parameter _summary=true.", 0, 1, isSummary); 6046 case -108220795: /*binding*/ return new Property("binding", "", "Binds to a value set if this element is coded (code, Coding, CodeableConcept, Quantity), or the data types (string, uri).", 0, 1, binding); 6047 case 837556430: /*mapping*/ return new Property("mapping", "", "Identifies a concept from an external specification that roughly corresponds to this element.", 0, java.lang.Integer.MAX_VALUE, mapping); 6048 default: return super.getNamedProperty(_hash, _name, _checkValid); 6049 } 6050 6051 } 6052 6053 @Override 6054 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6055 switch (hash) { 6056 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 6057 case -671065907: /*representation*/ return this.representation == null ? new Base[0] : this.representation.toArray(new Base[this.representation.size()]); // Enumeration<PropertyRepresentation> 6058 case -825289923: /*sliceName*/ return this.sliceName == null ? new Base[0] : new Base[] {this.sliceName}; // StringType 6059 case 102727412: /*label*/ return this.label == null ? new Base[0] : new Base[] {this.label}; // StringType 6060 case 3059181: /*code*/ return this.code == null ? new Base[0] : this.code.toArray(new Base[this.code.size()]); // Coding 6061 case -2119287345: /*slicing*/ return this.slicing == null ? new Base[0] : new Base[] {this.slicing}; // ElementDefinitionSlicingComponent 6062 case 109413500: /*short*/ return this.short_ == null ? new Base[0] : new Base[] {this.short_}; // StringType 6063 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : new Base[] {this.definition}; // MarkdownType 6064 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // MarkdownType 6065 case -1619874672: /*requirements*/ return this.requirements == null ? new Base[0] : new Base[] {this.requirements}; // MarkdownType 6066 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 6067 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 6068 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 6069 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // ElementDefinitionBaseComponent 6070 case 1193747154: /*contentReference*/ return this.contentReference == null ? new Base[0] : new Base[] {this.contentReference}; // UriType 6071 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // TypeRefComponent 6072 case -659125328: /*defaultValue*/ return this.defaultValue == null ? new Base[0] : new Base[] {this.defaultValue}; // org.hl7.fhir.dstu3.model.Type 6073 case 1857257103: /*meaningWhenMissing*/ return this.meaningWhenMissing == null ? new Base[0] : new Base[] {this.meaningWhenMissing}; // MarkdownType 6074 case 1828196047: /*orderMeaning*/ return this.orderMeaning == null ? new Base[0] : new Base[] {this.orderMeaning}; // StringType 6075 case 97445748: /*fixed*/ return this.fixed == null ? new Base[0] : new Base[] {this.fixed}; // org.hl7.fhir.dstu3.model.Type 6076 case -791090288: /*pattern*/ return this.pattern == null ? new Base[0] : new Base[] {this.pattern}; // org.hl7.fhir.dstu3.model.Type 6077 case -1322970774: /*example*/ return this.example == null ? new Base[0] : this.example.toArray(new Base[this.example.size()]); // ElementDefinitionExampleComponent 6078 case -1376969153: /*minValue*/ return this.minValue == null ? new Base[0] : new Base[] {this.minValue}; // Type 6079 case 399227501: /*maxValue*/ return this.maxValue == null ? new Base[0] : new Base[] {this.maxValue}; // Type 6080 case -791400086: /*maxLength*/ return this.maxLength == null ? new Base[0] : new Base[] {this.maxLength}; // IntegerType 6081 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // IdType 6082 case -190376483: /*constraint*/ return this.constraint == null ? new Base[0] : this.constraint.toArray(new Base[this.constraint.size()]); // ElementDefinitionConstraintComponent 6083 case -1402857082: /*mustSupport*/ return this.mustSupport == null ? new Base[0] : new Base[] {this.mustSupport}; // BooleanType 6084 case -1408783839: /*isModifier*/ return this.isModifier == null ? new Base[0] : new Base[] {this.isModifier}; // BooleanType 6085 case 1857548060: /*isSummary*/ return this.isSummary == null ? new Base[0] : new Base[] {this.isSummary}; // BooleanType 6086 case -108220795: /*binding*/ return this.binding == null ? new Base[0] : new Base[] {this.binding}; // ElementDefinitionBindingComponent 6087 case 837556430: /*mapping*/ return this.mapping == null ? new Base[0] : this.mapping.toArray(new Base[this.mapping.size()]); // ElementDefinitionMappingComponent 6088 default: return super.getProperty(hash, name, checkValid); 6089 } 6090 6091 } 6092 6093 @Override 6094 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6095 switch (hash) { 6096 case 3433509: // path 6097 this.path = castToString(value); // StringType 6098 return value; 6099 case -671065907: // representation 6100 value = new PropertyRepresentationEnumFactory().fromType(castToCode(value)); 6101 this.getRepresentation().add((Enumeration) value); // Enumeration<PropertyRepresentation> 6102 return value; 6103 case -825289923: // sliceName 6104 this.sliceName = castToString(value); // StringType 6105 return value; 6106 case 102727412: // label 6107 this.label = castToString(value); // StringType 6108 return value; 6109 case 3059181: // code 6110 this.getCode().add(castToCoding(value)); // Coding 6111 return value; 6112 case -2119287345: // slicing 6113 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 6114 return value; 6115 case 109413500: // short 6116 this.short_ = castToString(value); // StringType 6117 return value; 6118 case -1014418093: // definition 6119 this.definition = castToMarkdown(value); // MarkdownType 6120 return value; 6121 case 950398559: // comment 6122 this.comment = castToMarkdown(value); // MarkdownType 6123 return value; 6124 case -1619874672: // requirements 6125 this.requirements = castToMarkdown(value); // MarkdownType 6126 return value; 6127 case 92902992: // alias 6128 this.getAlias().add(castToString(value)); // StringType 6129 return value; 6130 case 108114: // min 6131 this.min = castToUnsignedInt(value); // UnsignedIntType 6132 return value; 6133 case 107876: // max 6134 this.max = castToString(value); // StringType 6135 return value; 6136 case 3016401: // base 6137 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 6138 return value; 6139 case 1193747154: // contentReference 6140 this.contentReference = castToUri(value); // UriType 6141 return value; 6142 case 3575610: // type 6143 this.getType().add((TypeRefComponent) value); // TypeRefComponent 6144 return value; 6145 case -659125328: // defaultValue 6146 this.defaultValue = castToType(value); // org.hl7.fhir.dstu3.model.Type 6147 return value; 6148 case 1857257103: // meaningWhenMissing 6149 this.meaningWhenMissing = castToMarkdown(value); // MarkdownType 6150 return value; 6151 case 1828196047: // orderMeaning 6152 this.orderMeaning = castToString(value); // StringType 6153 return value; 6154 case 97445748: // fixed 6155 this.fixed = castToType(value); // org.hl7.fhir.dstu3.model.Type 6156 return value; 6157 case -791090288: // pattern 6158 this.pattern = castToType(value); // org.hl7.fhir.dstu3.model.Type 6159 return value; 6160 case -1322970774: // example 6161 this.getExample().add((ElementDefinitionExampleComponent) value); // ElementDefinitionExampleComponent 6162 return value; 6163 case -1376969153: // minValue 6164 this.minValue = castToType(value); // Type 6165 return value; 6166 case 399227501: // maxValue 6167 this.maxValue = castToType(value); // Type 6168 return value; 6169 case -791400086: // maxLength 6170 this.maxLength = castToInteger(value); // IntegerType 6171 return value; 6172 case -861311717: // condition 6173 this.getCondition().add(castToId(value)); // IdType 6174 return value; 6175 case -190376483: // constraint 6176 this.getConstraint().add((ElementDefinitionConstraintComponent) value); // ElementDefinitionConstraintComponent 6177 return value; 6178 case -1402857082: // mustSupport 6179 this.mustSupport = castToBoolean(value); // BooleanType 6180 return value; 6181 case -1408783839: // isModifier 6182 this.isModifier = castToBoolean(value); // BooleanType 6183 return value; 6184 case 1857548060: // isSummary 6185 this.isSummary = castToBoolean(value); // BooleanType 6186 return value; 6187 case -108220795: // binding 6188 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 6189 return value; 6190 case 837556430: // mapping 6191 this.getMapping().add((ElementDefinitionMappingComponent) value); // ElementDefinitionMappingComponent 6192 return value; 6193 default: return super.setProperty(hash, name, value); 6194 } 6195 6196 } 6197 6198 @Override 6199 public Base setProperty(String name, Base value) throws FHIRException { 6200 if (name.equals("path")) { 6201 this.path = castToString(value); // StringType 6202 } else if (name.equals("representation")) { 6203 value = new PropertyRepresentationEnumFactory().fromType(castToCode(value)); 6204 this.getRepresentation().add((Enumeration) value); 6205 } else if (name.equals("sliceName")) { 6206 this.sliceName = castToString(value); // StringType 6207 } else if (name.equals("label")) { 6208 this.label = castToString(value); // StringType 6209 } else if (name.equals("code")) { 6210 this.getCode().add(castToCoding(value)); 6211 } else if (name.equals("slicing")) { 6212 this.slicing = (ElementDefinitionSlicingComponent) value; // ElementDefinitionSlicingComponent 6213 } else if (name.equals("short")) { 6214 this.short_ = castToString(value); // StringType 6215 } else if (name.equals("definition")) { 6216 this.definition = castToMarkdown(value); // MarkdownType 6217 } else if (name.equals("comment")) { 6218 this.comment = castToMarkdown(value); // MarkdownType 6219 } else if (name.equals("requirements")) { 6220 this.requirements = castToMarkdown(value); // MarkdownType 6221 } else if (name.equals("alias")) { 6222 this.getAlias().add(castToString(value)); 6223 } else if (name.equals("min")) { 6224 this.min = castToUnsignedInt(value); // UnsignedIntType 6225 } else if (name.equals("max")) { 6226 this.max = castToString(value); // StringType 6227 } else if (name.equals("base")) { 6228 this.base = (ElementDefinitionBaseComponent) value; // ElementDefinitionBaseComponent 6229 } else if (name.equals("contentReference")) { 6230 this.contentReference = castToUri(value); // UriType 6231 } else if (name.equals("type")) { 6232 this.getType().add((TypeRefComponent) value); 6233 } else if (name.equals("defaultValue[x]")) { 6234 this.defaultValue = castToType(value); // org.hl7.fhir.dstu3.model.Type 6235 } else if (name.equals("meaningWhenMissing")) { 6236 this.meaningWhenMissing = castToMarkdown(value); // MarkdownType 6237 } else if (name.equals("orderMeaning")) { 6238 this.orderMeaning = castToString(value); // StringType 6239 } else if (name.equals("fixed[x]")) { 6240 this.fixed = castToType(value); // org.hl7.fhir.dstu3.model.Type 6241 } else if (name.equals("pattern[x]")) { 6242 this.pattern = castToType(value); // org.hl7.fhir.dstu3.model.Type 6243 } else if (name.equals("example")) { 6244 this.getExample().add((ElementDefinitionExampleComponent) value); 6245 } else if (name.equals("minValue[x]")) { 6246 this.minValue = castToType(value); // Type 6247 } else if (name.equals("maxValue[x]")) { 6248 this.maxValue = castToType(value); // Type 6249 } else if (name.equals("maxLength")) { 6250 this.maxLength = castToInteger(value); // IntegerType 6251 } else if (name.equals("condition")) { 6252 this.getCondition().add(castToId(value)); 6253 } else if (name.equals("constraint")) { 6254 this.getConstraint().add((ElementDefinitionConstraintComponent) value); 6255 } else if (name.equals("mustSupport")) { 6256 this.mustSupport = castToBoolean(value); // BooleanType 6257 } else if (name.equals("isModifier")) { 6258 this.isModifier = castToBoolean(value); // BooleanType 6259 } else if (name.equals("isSummary")) { 6260 this.isSummary = castToBoolean(value); // BooleanType 6261 } else if (name.equals("binding")) { 6262 this.binding = (ElementDefinitionBindingComponent) value; // ElementDefinitionBindingComponent 6263 } else if (name.equals("mapping")) { 6264 this.getMapping().add((ElementDefinitionMappingComponent) value); 6265 } else 6266 return super.setProperty(name, value); 6267 return value; 6268 } 6269 6270 @Override 6271 public Base makeProperty(int hash, String name) throws FHIRException { 6272 switch (hash) { 6273 case 3433509: return getPathElement(); 6274 case -671065907: return addRepresentationElement(); 6275 case -825289923: return getSliceNameElement(); 6276 case 102727412: return getLabelElement(); 6277 case 3059181: return addCode(); 6278 case -2119287345: return getSlicing(); 6279 case 109413500: return getShortElement(); 6280 case -1014418093: return getDefinitionElement(); 6281 case 950398559: return getCommentElement(); 6282 case -1619874672: return getRequirementsElement(); 6283 case 92902992: return addAliasElement(); 6284 case 108114: return getMinElement(); 6285 case 107876: return getMaxElement(); 6286 case 3016401: return getBase(); 6287 case 1193747154: return getContentReferenceElement(); 6288 case 3575610: return addType(); 6289 case 587922128: return getDefaultValue(); 6290 case -659125328: return getDefaultValue(); 6291 case 1857257103: return getMeaningWhenMissingElement(); 6292 case 1828196047: return getOrderMeaningElement(); 6293 case -391522164: return getFixed(); 6294 case 97445748: return getFixed(); 6295 case -885125392: return getPattern(); 6296 case -791090288: return getPattern(); 6297 case -1322970774: return addExample(); 6298 case -55301663: return getMinValue(); 6299 case -1376969153: return getMinValue(); 6300 case 622130931: return getMaxValue(); 6301 case 399227501: return getMaxValue(); 6302 case -791400086: return getMaxLengthElement(); 6303 case -861311717: return addConditionElement(); 6304 case -190376483: return addConstraint(); 6305 case -1402857082: return getMustSupportElement(); 6306 case -1408783839: return getIsModifierElement(); 6307 case 1857548060: return getIsSummaryElement(); 6308 case -108220795: return getBinding(); 6309 case 837556430: return addMapping(); 6310 default: return super.makeProperty(hash, name); 6311 } 6312 6313 } 6314 6315 @Override 6316 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6317 switch (hash) { 6318 case 3433509: /*path*/ return new String[] {"string"}; 6319 case -671065907: /*representation*/ return new String[] {"code"}; 6320 case -825289923: /*sliceName*/ return new String[] {"string"}; 6321 case 102727412: /*label*/ return new String[] {"string"}; 6322 case 3059181: /*code*/ return new String[] {"Coding"}; 6323 case -2119287345: /*slicing*/ return new String[] {}; 6324 case 109413500: /*short*/ return new String[] {"string"}; 6325 case -1014418093: /*definition*/ return new String[] {"markdown"}; 6326 case 950398559: /*comment*/ return new String[] {"markdown"}; 6327 case -1619874672: /*requirements*/ return new String[] {"markdown"}; 6328 case 92902992: /*alias*/ return new String[] {"string"}; 6329 case 108114: /*min*/ return new String[] {"unsignedInt"}; 6330 case 107876: /*max*/ return new String[] {"string"}; 6331 case 3016401: /*base*/ return new String[] {}; 6332 case 1193747154: /*contentReference*/ return new String[] {"uri"}; 6333 case 3575610: /*type*/ return new String[] {}; 6334 case -659125328: /*defaultValue*/ return new String[] {"*"}; 6335 case 1857257103: /*meaningWhenMissing*/ return new String[] {"markdown"}; 6336 case 1828196047: /*orderMeaning*/ return new String[] {"string"}; 6337 case 97445748: /*fixed*/ return new String[] {"*"}; 6338 case -791090288: /*pattern*/ return new String[] {"*"}; 6339 case -1322970774: /*example*/ return new String[] {}; 6340 case -1376969153: /*minValue*/ return new String[] {"date", "dateTime", "instant", "time", "decimal", "integer", "positiveInt", "unsignedInt", "Quantity"}; 6341 case 399227501: /*maxValue*/ return new String[] {"date", "dateTime", "instant", "time", "decimal", "integer", "positiveInt", "unsignedInt", "Quantity"}; 6342 case -791400086: /*maxLength*/ return new String[] {"integer"}; 6343 case -861311717: /*condition*/ return new String[] {"id"}; 6344 case -190376483: /*constraint*/ return new String[] {}; 6345 case -1402857082: /*mustSupport*/ return new String[] {"boolean"}; 6346 case -1408783839: /*isModifier*/ return new String[] {"boolean"}; 6347 case 1857548060: /*isSummary*/ return new String[] {"boolean"}; 6348 case -108220795: /*binding*/ return new String[] {}; 6349 case 837556430: /*mapping*/ return new String[] {}; 6350 default: return super.getTypesForProperty(hash, name); 6351 } 6352 6353 } 6354 6355 @Override 6356 public Base addChild(String name) throws FHIRException { 6357 if (name.equals("path")) { 6358 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.path"); 6359 } 6360 else if (name.equals("representation")) { 6361 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.representation"); 6362 } 6363 else if (name.equals("sliceName")) { 6364 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.sliceName"); 6365 } 6366 else if (name.equals("label")) { 6367 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.label"); 6368 } 6369 else if (name.equals("code")) { 6370 return addCode(); 6371 } 6372 else if (name.equals("slicing")) { 6373 this.slicing = new ElementDefinitionSlicingComponent(); 6374 return this.slicing; 6375 } 6376 else if (name.equals("short")) { 6377 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.short"); 6378 } 6379 else if (name.equals("definition")) { 6380 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.definition"); 6381 } 6382 else if (name.equals("comment")) { 6383 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.comment"); 6384 } 6385 else if (name.equals("requirements")) { 6386 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.requirements"); 6387 } 6388 else if (name.equals("alias")) { 6389 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.alias"); 6390 } 6391 else if (name.equals("min")) { 6392 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.min"); 6393 } 6394 else if (name.equals("max")) { 6395 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.max"); 6396 } 6397 else if (name.equals("base")) { 6398 this.base = new ElementDefinitionBaseComponent(); 6399 return this.base; 6400 } 6401 else if (name.equals("contentReference")) { 6402 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.contentReference"); 6403 } 6404 else if (name.equals("type")) { 6405 return addType(); 6406 } 6407 else if (name.equals("defaultValueBoolean")) { 6408 this.defaultValue = new BooleanType(); 6409 return this.defaultValue; 6410 } 6411 else if (name.equals("defaultValueInteger")) { 6412 this.defaultValue = new IntegerType(); 6413 return this.defaultValue; 6414 } 6415 else if (name.equals("defaultValueDecimal")) { 6416 this.defaultValue = new DecimalType(); 6417 return this.defaultValue; 6418 } 6419 else if (name.equals("defaultValueBase64Binary")) { 6420 this.defaultValue = new Base64BinaryType(); 6421 return this.defaultValue; 6422 } 6423 else if (name.equals("defaultValueInstant")) { 6424 this.defaultValue = new InstantType(); 6425 return this.defaultValue; 6426 } 6427 else if (name.equals("defaultValueString")) { 6428 this.defaultValue = new StringType(); 6429 return this.defaultValue; 6430 } 6431 else if (name.equals("defaultValueUri")) { 6432 this.defaultValue = new UriType(); 6433 return this.defaultValue; 6434 } 6435 else if (name.equals("defaultValueDate")) { 6436 this.defaultValue = new DateType(); 6437 return this.defaultValue; 6438 } 6439 else if (name.equals("defaultValueDateTime")) { 6440 this.defaultValue = new DateTimeType(); 6441 return this.defaultValue; 6442 } 6443 else if (name.equals("defaultValueTime")) { 6444 this.defaultValue = new TimeType(); 6445 return this.defaultValue; 6446 } 6447 else if (name.equals("defaultValueCode")) { 6448 this.defaultValue = new CodeType(); 6449 return this.defaultValue; 6450 } 6451 else if (name.equals("defaultValueOid")) { 6452 this.defaultValue = new OidType(); 6453 return this.defaultValue; 6454 } 6455 else if (name.equals("defaultValueId")) { 6456 this.defaultValue = new IdType(); 6457 return this.defaultValue; 6458 } 6459 else if (name.equals("defaultValueUnsignedInt")) { 6460 this.defaultValue = new UnsignedIntType(); 6461 return this.defaultValue; 6462 } 6463 else if (name.equals("defaultValuePositiveInt")) { 6464 this.defaultValue = new PositiveIntType(); 6465 return this.defaultValue; 6466 } 6467 else if (name.equals("defaultValueMarkdown")) { 6468 this.defaultValue = new MarkdownType(); 6469 return this.defaultValue; 6470 } 6471 else if (name.equals("defaultValueAnnotation")) { 6472 this.defaultValue = new Annotation(); 6473 return this.defaultValue; 6474 } 6475 else if (name.equals("defaultValueAttachment")) { 6476 this.defaultValue = new Attachment(); 6477 return this.defaultValue; 6478 } 6479 else if (name.equals("defaultValueIdentifier")) { 6480 this.defaultValue = new Identifier(); 6481 return this.defaultValue; 6482 } 6483 else if (name.equals("defaultValueCodeableConcept")) { 6484 this.defaultValue = new CodeableConcept(); 6485 return this.defaultValue; 6486 } 6487 else if (name.equals("defaultValueCoding")) { 6488 this.defaultValue = new Coding(); 6489 return this.defaultValue; 6490 } 6491 else if (name.equals("defaultValueQuantity")) { 6492 this.defaultValue = new Quantity(); 6493 return this.defaultValue; 6494 } 6495 else if (name.equals("defaultValueRange")) { 6496 this.defaultValue = new Range(); 6497 return this.defaultValue; 6498 } 6499 else if (name.equals("defaultValuePeriod")) { 6500 this.defaultValue = new Period(); 6501 return this.defaultValue; 6502 } 6503 else if (name.equals("defaultValueRatio")) { 6504 this.defaultValue = new Ratio(); 6505 return this.defaultValue; 6506 } 6507 else if (name.equals("defaultValueSampledData")) { 6508 this.defaultValue = new SampledData(); 6509 return this.defaultValue; 6510 } 6511 else if (name.equals("defaultValueSignature")) { 6512 this.defaultValue = new Signature(); 6513 return this.defaultValue; 6514 } 6515 else if (name.equals("defaultValueHumanName")) { 6516 this.defaultValue = new HumanName(); 6517 return this.defaultValue; 6518 } 6519 else if (name.equals("defaultValueAddress")) { 6520 this.defaultValue = new Address(); 6521 return this.defaultValue; 6522 } 6523 else if (name.equals("defaultValueContactPoint")) { 6524 this.defaultValue = new ContactPoint(); 6525 return this.defaultValue; 6526 } 6527 else if (name.equals("defaultValueTiming")) { 6528 this.defaultValue = new Timing(); 6529 return this.defaultValue; 6530 } 6531 else if (name.equals("defaultValueReference")) { 6532 this.defaultValue = new Reference(); 6533 return this.defaultValue; 6534 } 6535 else if (name.equals("defaultValueMeta")) { 6536 this.defaultValue = new Meta(); 6537 return this.defaultValue; 6538 } 6539 else if (name.equals("meaningWhenMissing")) { 6540 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.meaningWhenMissing"); 6541 } 6542 else if (name.equals("orderMeaning")) { 6543 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.orderMeaning"); 6544 } 6545 else if (name.equals("fixedBoolean")) { 6546 this.fixed = new BooleanType(); 6547 return this.fixed; 6548 } 6549 else if (name.equals("fixedInteger")) { 6550 this.fixed = new IntegerType(); 6551 return this.fixed; 6552 } 6553 else if (name.equals("fixedDecimal")) { 6554 this.fixed = new DecimalType(); 6555 return this.fixed; 6556 } 6557 else if (name.equals("fixedBase64Binary")) { 6558 this.fixed = new Base64BinaryType(); 6559 return this.fixed; 6560 } 6561 else if (name.equals("fixedInstant")) { 6562 this.fixed = new InstantType(); 6563 return this.fixed; 6564 } 6565 else if (name.equals("fixedString")) { 6566 this.fixed = new StringType(); 6567 return this.fixed; 6568 } 6569 else if (name.equals("fixedUri")) { 6570 this.fixed = new UriType(); 6571 return this.fixed; 6572 } 6573 else if (name.equals("fixedDate")) { 6574 this.fixed = new DateType(); 6575 return this.fixed; 6576 } 6577 else if (name.equals("fixedDateTime")) { 6578 this.fixed = new DateTimeType(); 6579 return this.fixed; 6580 } 6581 else if (name.equals("fixedTime")) { 6582 this.fixed = new TimeType(); 6583 return this.fixed; 6584 } 6585 else if (name.equals("fixedCode")) { 6586 this.fixed = new CodeType(); 6587 return this.fixed; 6588 } 6589 else if (name.equals("fixedOid")) { 6590 this.fixed = new OidType(); 6591 return this.fixed; 6592 } 6593 else if (name.equals("fixedId")) { 6594 this.fixed = new IdType(); 6595 return this.fixed; 6596 } 6597 else if (name.equals("fixedUnsignedInt")) { 6598 this.fixed = new UnsignedIntType(); 6599 return this.fixed; 6600 } 6601 else if (name.equals("fixedPositiveInt")) { 6602 this.fixed = new PositiveIntType(); 6603 return this.fixed; 6604 } 6605 else if (name.equals("fixedMarkdown")) { 6606 this.fixed = new MarkdownType(); 6607 return this.fixed; 6608 } 6609 else if (name.equals("fixedAnnotation")) { 6610 this.fixed = new Annotation(); 6611 return this.fixed; 6612 } 6613 else if (name.equals("fixedAttachment")) { 6614 this.fixed = new Attachment(); 6615 return this.fixed; 6616 } 6617 else if (name.equals("fixedIdentifier")) { 6618 this.fixed = new Identifier(); 6619 return this.fixed; 6620 } 6621 else if (name.equals("fixedCodeableConcept")) { 6622 this.fixed = new CodeableConcept(); 6623 return this.fixed; 6624 } 6625 else if (name.equals("fixedCoding")) { 6626 this.fixed = new Coding(); 6627 return this.fixed; 6628 } 6629 else if (name.equals("fixedQuantity")) { 6630 this.fixed = new Quantity(); 6631 return this.fixed; 6632 } 6633 else if (name.equals("fixedRange")) { 6634 this.fixed = new Range(); 6635 return this.fixed; 6636 } 6637 else if (name.equals("fixedPeriod")) { 6638 this.fixed = new Period(); 6639 return this.fixed; 6640 } 6641 else if (name.equals("fixedRatio")) { 6642 this.fixed = new Ratio(); 6643 return this.fixed; 6644 } 6645 else if (name.equals("fixedSampledData")) { 6646 this.fixed = new SampledData(); 6647 return this.fixed; 6648 } 6649 else if (name.equals("fixedSignature")) { 6650 this.fixed = new Signature(); 6651 return this.fixed; 6652 } 6653 else if (name.equals("fixedHumanName")) { 6654 this.fixed = new HumanName(); 6655 return this.fixed; 6656 } 6657 else if (name.equals("fixedAddress")) { 6658 this.fixed = new Address(); 6659 return this.fixed; 6660 } 6661 else if (name.equals("fixedContactPoint")) { 6662 this.fixed = new ContactPoint(); 6663 return this.fixed; 6664 } 6665 else if (name.equals("fixedTiming")) { 6666 this.fixed = new Timing(); 6667 return this.fixed; 6668 } 6669 else if (name.equals("fixedReference")) { 6670 this.fixed = new Reference(); 6671 return this.fixed; 6672 } 6673 else if (name.equals("fixedMeta")) { 6674 this.fixed = new Meta(); 6675 return this.fixed; 6676 } 6677 else if (name.equals("patternBoolean")) { 6678 this.pattern = new BooleanType(); 6679 return this.pattern; 6680 } 6681 else if (name.equals("patternInteger")) { 6682 this.pattern = new IntegerType(); 6683 return this.pattern; 6684 } 6685 else if (name.equals("patternDecimal")) { 6686 this.pattern = new DecimalType(); 6687 return this.pattern; 6688 } 6689 else if (name.equals("patternBase64Binary")) { 6690 this.pattern = new Base64BinaryType(); 6691 return this.pattern; 6692 } 6693 else if (name.equals("patternInstant")) { 6694 this.pattern = new InstantType(); 6695 return this.pattern; 6696 } 6697 else if (name.equals("patternString")) { 6698 this.pattern = new StringType(); 6699 return this.pattern; 6700 } 6701 else if (name.equals("patternUri")) { 6702 this.pattern = new UriType(); 6703 return this.pattern; 6704 } 6705 else if (name.equals("patternDate")) { 6706 this.pattern = new DateType(); 6707 return this.pattern; 6708 } 6709 else if (name.equals("patternDateTime")) { 6710 this.pattern = new DateTimeType(); 6711 return this.pattern; 6712 } 6713 else if (name.equals("patternTime")) { 6714 this.pattern = new TimeType(); 6715 return this.pattern; 6716 } 6717 else if (name.equals("patternCode")) { 6718 this.pattern = new CodeType(); 6719 return this.pattern; 6720 } 6721 else if (name.equals("patternOid")) { 6722 this.pattern = new OidType(); 6723 return this.pattern; 6724 } 6725 else if (name.equals("patternId")) { 6726 this.pattern = new IdType(); 6727 return this.pattern; 6728 } 6729 else if (name.equals("patternUnsignedInt")) { 6730 this.pattern = new UnsignedIntType(); 6731 return this.pattern; 6732 } 6733 else if (name.equals("patternPositiveInt")) { 6734 this.pattern = new PositiveIntType(); 6735 return this.pattern; 6736 } 6737 else if (name.equals("patternMarkdown")) { 6738 this.pattern = new MarkdownType(); 6739 return this.pattern; 6740 } 6741 else if (name.equals("patternAnnotation")) { 6742 this.pattern = new Annotation(); 6743 return this.pattern; 6744 } 6745 else if (name.equals("patternAttachment")) { 6746 this.pattern = new Attachment(); 6747 return this.pattern; 6748 } 6749 else if (name.equals("patternIdentifier")) { 6750 this.pattern = new Identifier(); 6751 return this.pattern; 6752 } 6753 else if (name.equals("patternCodeableConcept")) { 6754 this.pattern = new CodeableConcept(); 6755 return this.pattern; 6756 } 6757 else if (name.equals("patternCoding")) { 6758 this.pattern = new Coding(); 6759 return this.pattern; 6760 } 6761 else if (name.equals("patternQuantity")) { 6762 this.pattern = new Quantity(); 6763 return this.pattern; 6764 } 6765 else if (name.equals("patternRange")) { 6766 this.pattern = new Range(); 6767 return this.pattern; 6768 } 6769 else if (name.equals("patternPeriod")) { 6770 this.pattern = new Period(); 6771 return this.pattern; 6772 } 6773 else if (name.equals("patternRatio")) { 6774 this.pattern = new Ratio(); 6775 return this.pattern; 6776 } 6777 else if (name.equals("patternSampledData")) { 6778 this.pattern = new SampledData(); 6779 return this.pattern; 6780 } 6781 else if (name.equals("patternSignature")) { 6782 this.pattern = new Signature(); 6783 return this.pattern; 6784 } 6785 else if (name.equals("patternHumanName")) { 6786 this.pattern = new HumanName(); 6787 return this.pattern; 6788 } 6789 else if (name.equals("patternAddress")) { 6790 this.pattern = new Address(); 6791 return this.pattern; 6792 } 6793 else if (name.equals("patternContactPoint")) { 6794 this.pattern = new ContactPoint(); 6795 return this.pattern; 6796 } 6797 else if (name.equals("patternTiming")) { 6798 this.pattern = new Timing(); 6799 return this.pattern; 6800 } 6801 else if (name.equals("patternReference")) { 6802 this.pattern = new Reference(); 6803 return this.pattern; 6804 } 6805 else if (name.equals("patternMeta")) { 6806 this.pattern = new Meta(); 6807 return this.pattern; 6808 } 6809 else if (name.equals("example")) { 6810 return addExample(); 6811 } 6812 else if (name.equals("minValueDate")) { 6813 this.minValue = new DateType(); 6814 return this.minValue; 6815 } 6816 else if (name.equals("minValueDateTime")) { 6817 this.minValue = new DateTimeType(); 6818 return this.minValue; 6819 } 6820 else if (name.equals("minValueInstant")) { 6821 this.minValue = new InstantType(); 6822 return this.minValue; 6823 } 6824 else if (name.equals("minValueTime")) { 6825 this.minValue = new TimeType(); 6826 return this.minValue; 6827 } 6828 else if (name.equals("minValueDecimal")) { 6829 this.minValue = new DecimalType(); 6830 return this.minValue; 6831 } 6832 else if (name.equals("minValueInteger")) { 6833 this.minValue = new IntegerType(); 6834 return this.minValue; 6835 } 6836 else if (name.equals("minValuePositiveInt")) { 6837 this.minValue = new PositiveIntType(); 6838 return this.minValue; 6839 } 6840 else if (name.equals("minValueUnsignedInt")) { 6841 this.minValue = new UnsignedIntType(); 6842 return this.minValue; 6843 } 6844 else if (name.equals("minValueQuantity")) { 6845 this.minValue = new Quantity(); 6846 return this.minValue; 6847 } 6848 else if (name.equals("maxValueDate")) { 6849 this.maxValue = new DateType(); 6850 return this.maxValue; 6851 } 6852 else if (name.equals("maxValueDateTime")) { 6853 this.maxValue = new DateTimeType(); 6854 return this.maxValue; 6855 } 6856 else if (name.equals("maxValueInstant")) { 6857 this.maxValue = new InstantType(); 6858 return this.maxValue; 6859 } 6860 else if (name.equals("maxValueTime")) { 6861 this.maxValue = new TimeType(); 6862 return this.maxValue; 6863 } 6864 else if (name.equals("maxValueDecimal")) { 6865 this.maxValue = new DecimalType(); 6866 return this.maxValue; 6867 } 6868 else if (name.equals("maxValueInteger")) { 6869 this.maxValue = new IntegerType(); 6870 return this.maxValue; 6871 } 6872 else if (name.equals("maxValuePositiveInt")) { 6873 this.maxValue = new PositiveIntType(); 6874 return this.maxValue; 6875 } 6876 else if (name.equals("maxValueUnsignedInt")) { 6877 this.maxValue = new UnsignedIntType(); 6878 return this.maxValue; 6879 } 6880 else if (name.equals("maxValueQuantity")) { 6881 this.maxValue = new Quantity(); 6882 return this.maxValue; 6883 } 6884 else if (name.equals("maxLength")) { 6885 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.maxLength"); 6886 } 6887 else if (name.equals("condition")) { 6888 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.condition"); 6889 } 6890 else if (name.equals("constraint")) { 6891 return addConstraint(); 6892 } 6893 else if (name.equals("mustSupport")) { 6894 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.mustSupport"); 6895 } 6896 else if (name.equals("isModifier")) { 6897 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isModifier"); 6898 } 6899 else if (name.equals("isSummary")) { 6900 throw new FHIRException("Cannot call addChild on a singleton property ElementDefinition.isSummary"); 6901 } 6902 else if (name.equals("binding")) { 6903 this.binding = new ElementDefinitionBindingComponent(); 6904 return this.binding; 6905 } 6906 else if (name.equals("mapping")) { 6907 return addMapping(); 6908 } 6909 else 6910 return super.addChild(name); 6911 } 6912 6913 public String fhirType() { 6914 return "ElementDefinition"; 6915 6916 } 6917 6918 public ElementDefinition copy() { 6919 ElementDefinition dst = new ElementDefinition(); 6920 copyValues(dst); 6921 dst.path = path == null ? null : path.copy(); 6922 if (representation != null) { 6923 dst.representation = new ArrayList<Enumeration<PropertyRepresentation>>(); 6924 for (Enumeration<PropertyRepresentation> i : representation) 6925 dst.representation.add(i.copy()); 6926 }; 6927 dst.sliceName = sliceName == null ? null : sliceName.copy(); 6928 dst.label = label == null ? null : label.copy(); 6929 if (code != null) { 6930 dst.code = new ArrayList<Coding>(); 6931 for (Coding i : code) 6932 dst.code.add(i.copy()); 6933 }; 6934 dst.slicing = slicing == null ? null : slicing.copy(); 6935 dst.short_ = short_ == null ? null : short_.copy(); 6936 dst.definition = definition == null ? null : definition.copy(); 6937 dst.comment = comment == null ? null : comment.copy(); 6938 dst.requirements = requirements == null ? null : requirements.copy(); 6939 if (alias != null) { 6940 dst.alias = new ArrayList<StringType>(); 6941 for (StringType i : alias) 6942 dst.alias.add(i.copy()); 6943 }; 6944 dst.min = min == null ? null : min.copy(); 6945 dst.max = max == null ? null : max.copy(); 6946 dst.base = base == null ? null : base.copy(); 6947 dst.contentReference = contentReference == null ? null : contentReference.copy(); 6948 if (type != null) { 6949 dst.type = new ArrayList<TypeRefComponent>(); 6950 for (TypeRefComponent i : type) 6951 dst.type.add(i.copy()); 6952 }; 6953 dst.defaultValue = defaultValue == null ? null : defaultValue.copy(); 6954 dst.meaningWhenMissing = meaningWhenMissing == null ? null : meaningWhenMissing.copy(); 6955 dst.orderMeaning = orderMeaning == null ? null : orderMeaning.copy(); 6956 dst.fixed = fixed == null ? null : fixed.copy(); 6957 dst.pattern = pattern == null ? null : pattern.copy(); 6958 if (example != null) { 6959 dst.example = new ArrayList<ElementDefinitionExampleComponent>(); 6960 for (ElementDefinitionExampleComponent i : example) 6961 dst.example.add(i.copy()); 6962 }; 6963 dst.minValue = minValue == null ? null : minValue.copy(); 6964 dst.maxValue = maxValue == null ? null : maxValue.copy(); 6965 dst.maxLength = maxLength == null ? null : maxLength.copy(); 6966 if (condition != null) { 6967 dst.condition = new ArrayList<IdType>(); 6968 for (IdType i : condition) 6969 dst.condition.add(i.copy()); 6970 }; 6971 if (constraint != null) { 6972 dst.constraint = new ArrayList<ElementDefinitionConstraintComponent>(); 6973 for (ElementDefinitionConstraintComponent i : constraint) 6974 dst.constraint.add(i.copy()); 6975 }; 6976 dst.mustSupport = mustSupport == null ? null : mustSupport.copy(); 6977 dst.isModifier = isModifier == null ? null : isModifier.copy(); 6978 dst.isSummary = isSummary == null ? null : isSummary.copy(); 6979 dst.binding = binding == null ? null : binding.copy(); 6980 if (mapping != null) { 6981 dst.mapping = new ArrayList<ElementDefinitionMappingComponent>(); 6982 for (ElementDefinitionMappingComponent i : mapping) 6983 dst.mapping.add(i.copy()); 6984 }; 6985 return dst; 6986 } 6987 6988 protected ElementDefinition typedCopy() { 6989 return copy(); 6990 } 6991 6992 @Override 6993 public boolean equalsDeep(Base other_) { 6994 if (!super.equalsDeep(other_)) 6995 return false; 6996 if (!(other_ instanceof ElementDefinition)) 6997 return false; 6998 ElementDefinition o = (ElementDefinition) other_; 6999 return compareDeep(path, o.path, true) && compareDeep(representation, o.representation, true) && compareDeep(sliceName, o.sliceName, true) 7000 && compareDeep(label, o.label, true) && compareDeep(code, o.code, true) && compareDeep(slicing, o.slicing, true) 7001 && compareDeep(short_, o.short_, true) && compareDeep(definition, o.definition, true) && compareDeep(comment, o.comment, true) 7002 && compareDeep(requirements, o.requirements, true) && compareDeep(alias, o.alias, true) && compareDeep(min, o.min, true) 7003 && compareDeep(max, o.max, true) && compareDeep(base, o.base, true) && compareDeep(contentReference, o.contentReference, true) 7004 && compareDeep(type, o.type, true) && compareDeep(defaultValue, o.defaultValue, true) && compareDeep(meaningWhenMissing, o.meaningWhenMissing, true) 7005 && compareDeep(orderMeaning, o.orderMeaning, true) && compareDeep(fixed, o.fixed, true) && compareDeep(pattern, o.pattern, true) 7006 && compareDeep(example, o.example, true) && compareDeep(minValue, o.minValue, true) && compareDeep(maxValue, o.maxValue, true) 7007 && compareDeep(maxLength, o.maxLength, true) && compareDeep(condition, o.condition, true) && compareDeep(constraint, o.constraint, true) 7008 && compareDeep(mustSupport, o.mustSupport, true) && compareDeep(isModifier, o.isModifier, true) 7009 && compareDeep(isSummary, o.isSummary, true) && compareDeep(binding, o.binding, true) && compareDeep(mapping, o.mapping, true) 7010 ; 7011 } 7012 7013 @Override 7014 public boolean equalsShallow(Base other_) { 7015 if (!super.equalsShallow(other_)) 7016 return false; 7017 if (!(other_ instanceof ElementDefinition)) 7018 return false; 7019 ElementDefinition o = (ElementDefinition) other_; 7020 return compareValues(path, o.path, true) && compareValues(representation, o.representation, true) && compareValues(sliceName, o.sliceName, true) 7021 && compareValues(label, o.label, true) && compareValues(short_, o.short_, true) && compareValues(definition, o.definition, true) 7022 && compareValues(comment, o.comment, true) && compareValues(requirements, o.requirements, true) && compareValues(alias, o.alias, true) 7023 && compareValues(min, o.min, true) && compareValues(max, o.max, true) && compareValues(contentReference, o.contentReference, true) 7024 && compareValues(meaningWhenMissing, o.meaningWhenMissing, true) && compareValues(orderMeaning, o.orderMeaning, true) 7025 && compareValues(maxLength, o.maxLength, true) && compareValues(condition, o.condition, true) && compareValues(mustSupport, o.mustSupport, true) 7026 && compareValues(isModifier, o.isModifier, true) && compareValues(isSummary, o.isSummary, true); 7027 } 7028 7029 public boolean isEmpty() { 7030 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, representation, sliceName 7031 , label, code, slicing, short_, definition, comment, requirements, alias, min 7032 , max, base, contentReference, type, defaultValue, meaningWhenMissing, orderMeaning 7033 , fixed, pattern, example, minValue, maxValue, maxLength, condition, constraint 7034 , mustSupport, isModifier, isSummary, binding, mapping); 7035 } 7036 7037// added from java-adornments.txt: 7038 7039 public String toString() { 7040 if (hasId()) 7041 return getId(); 7042 if (hasSliceName()) 7043 return getPath()+":"+getSliceName(); 7044 else 7045 return getPath(); 7046 } 7047 7048 public void makeBase(String path, int min, String max) { 7049 ElementDefinitionBaseComponent self = getBase(); 7050 self.setPath(path); 7051 self.setMin(min); 7052 self.setMax(max); 7053 } 7054 7055 public String typeSummary() { 7056 CommaSeparatedStringBuilder b = new CommaSeparatedStringBuilder(); 7057 for (TypeRefComponent tr : type) { 7058 if (tr.hasCode()) 7059 b.append(tr.getCode()); 7060 } 7061 return b.toString(); 7062 } 7063 7064 7065// end addition 7066 7067}