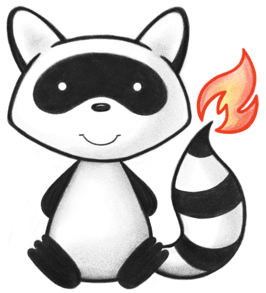
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 049 */ 050@ResourceDef(name="EligibilityRequest", profile="http://hl7.org/fhir/Profile/EligibilityRequest") 051public class EligibilityRequest extends DomainResource { 052 053 public enum EligibilityRequestStatus { 054 /** 055 * The instance is currently in-force. 056 */ 057 ACTIVE, 058 /** 059 * The instance is withdrawn, rescinded or reversed. 060 */ 061 CANCELLED, 062 /** 063 * A new instance the contents of which is not complete. 064 */ 065 DRAFT, 066 /** 067 * The instance was entered in error. 068 */ 069 ENTEREDINERROR, 070 /** 071 * added to help the parsers with the generic types 072 */ 073 NULL; 074 public static EligibilityRequestStatus fromCode(String codeString) throws FHIRException { 075 if (codeString == null || "".equals(codeString)) 076 return null; 077 if ("active".equals(codeString)) 078 return ACTIVE; 079 if ("cancelled".equals(codeString)) 080 return CANCELLED; 081 if ("draft".equals(codeString)) 082 return DRAFT; 083 if ("entered-in-error".equals(codeString)) 084 return ENTEREDINERROR; 085 if (Configuration.isAcceptInvalidEnums()) 086 return null; 087 else 088 throw new FHIRException("Unknown EligibilityRequestStatus code '"+codeString+"'"); 089 } 090 public String toCode() { 091 switch (this) { 092 case ACTIVE: return "active"; 093 case CANCELLED: return "cancelled"; 094 case DRAFT: return "draft"; 095 case ENTEREDINERROR: return "entered-in-error"; 096 case NULL: return null; 097 default: return "?"; 098 } 099 } 100 public String getSystem() { 101 switch (this) { 102 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 103 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 104 case DRAFT: return "http://hl7.org/fhir/fm-status"; 105 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getDefinition() { 111 switch (this) { 112 case ACTIVE: return "The instance is currently in-force."; 113 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 114 case DRAFT: return "A new instance the contents of which is not complete."; 115 case ENTEREDINERROR: return "The instance was entered in error."; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getDisplay() { 121 switch (this) { 122 case ACTIVE: return "Active"; 123 case CANCELLED: return "Cancelled"; 124 case DRAFT: return "Draft"; 125 case ENTEREDINERROR: return "Entered in Error"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 } 131 132 public static class EligibilityRequestStatusEnumFactory implements EnumFactory<EligibilityRequestStatus> { 133 public EligibilityRequestStatus fromCode(String codeString) throws IllegalArgumentException { 134 if (codeString == null || "".equals(codeString)) 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("active".equals(codeString)) 138 return EligibilityRequestStatus.ACTIVE; 139 if ("cancelled".equals(codeString)) 140 return EligibilityRequestStatus.CANCELLED; 141 if ("draft".equals(codeString)) 142 return EligibilityRequestStatus.DRAFT; 143 if ("entered-in-error".equals(codeString)) 144 return EligibilityRequestStatus.ENTEREDINERROR; 145 throw new IllegalArgumentException("Unknown EligibilityRequestStatus code '"+codeString+"'"); 146 } 147 public Enumeration<EligibilityRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 148 if (code == null) 149 return null; 150 if (code.isEmpty()) 151 return new Enumeration<EligibilityRequestStatus>(this); 152 String codeString = code.asStringValue(); 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("active".equals(codeString)) 156 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.ACTIVE); 157 if ("cancelled".equals(codeString)) 158 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.CANCELLED); 159 if ("draft".equals(codeString)) 160 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.DRAFT); 161 if ("entered-in-error".equals(codeString)) 162 return new Enumeration<EligibilityRequestStatus>(this, EligibilityRequestStatus.ENTEREDINERROR); 163 throw new FHIRException("Unknown EligibilityRequestStatus code '"+codeString+"'"); 164 } 165 public String toCode(EligibilityRequestStatus code) { 166 if (code == EligibilityRequestStatus.NULL) 167 return null; 168 if (code == EligibilityRequestStatus.ACTIVE) 169 return "active"; 170 if (code == EligibilityRequestStatus.CANCELLED) 171 return "cancelled"; 172 if (code == EligibilityRequestStatus.DRAFT) 173 return "draft"; 174 if (code == EligibilityRequestStatus.ENTEREDINERROR) 175 return "entered-in-error"; 176 return "?"; 177 } 178 public String toSystem(EligibilityRequestStatus code) { 179 return code.getSystem(); 180 } 181 } 182 183 /** 184 * The Response business identifier. 185 */ 186 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 187 @Description(shortDefinition="Business Identifier", formalDefinition="The Response business identifier." ) 188 protected List<Identifier> identifier; 189 190 /** 191 * The status of the resource instance. 192 */ 193 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 194 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 195 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 196 protected Enumeration<EligibilityRequestStatus> status; 197 198 /** 199 * Immediate (STAT), best effort (NORMAL), deferred (DEFER). 200 */ 201 @Child(name = "priority", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 202 @Description(shortDefinition="Desired processing priority", formalDefinition="Immediate (STAT), best effort (NORMAL), deferred (DEFER)." ) 203 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/process-priority") 204 protected CodeableConcept priority; 205 206 /** 207 * Patient Resource. 208 */ 209 @Child(name = "patient", type = {Patient.class}, order=3, min=0, max=1, modifier=false, summary=false) 210 @Description(shortDefinition="The subject of the Products and Services", formalDefinition="Patient Resource." ) 211 protected Reference patient; 212 213 /** 214 * The actual object that is the target of the reference (Patient Resource.) 215 */ 216 protected Patient patientTarget; 217 218 /** 219 * The date or dates when the enclosed suite of services were performed or completed. 220 */ 221 @Child(name = "serviced", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 222 @Description(shortDefinition="Estimated date or dates of Service", formalDefinition="The date or dates when the enclosed suite of services were performed or completed." ) 223 protected Type serviced; 224 225 /** 226 * The date when this resource was created. 227 */ 228 @Child(name = "created", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 229 @Description(shortDefinition="Creation date", formalDefinition="The date when this resource was created." ) 230 protected DateTimeType created; 231 232 /** 233 * Person who created the invoice/claim/pre-determination or pre-authorization. 234 */ 235 @Child(name = "enterer", type = {Practitioner.class}, order=6, min=0, max=1, modifier=false, summary=false) 236 @Description(shortDefinition="Author", formalDefinition="Person who created the invoice/claim/pre-determination or pre-authorization." ) 237 protected Reference enterer; 238 239 /** 240 * The actual object that is the target of the reference (Person who created the invoice/claim/pre-determination or pre-authorization.) 241 */ 242 protected Practitioner entererTarget; 243 244 /** 245 * The practitioner who is responsible for the services rendered to the patient. 246 */ 247 @Child(name = "provider", type = {Practitioner.class}, order=7, min=0, max=1, modifier=false, summary=false) 248 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 249 protected Reference provider; 250 251 /** 252 * The actual object that is the target of the reference (The practitioner who is responsible for the services rendered to the patient.) 253 */ 254 protected Practitioner providerTarget; 255 256 /** 257 * The organization which is responsible for the services rendered to the patient. 258 */ 259 @Child(name = "organization", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 260 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the services rendered to the patient." ) 261 protected Reference organization; 262 263 /** 264 * The actual object that is the target of the reference (The organization which is responsible for the services rendered to the patient.) 265 */ 266 protected Organization organizationTarget; 267 268 /** 269 * The Insurer who is target of the request. 270 */ 271 @Child(name = "insurer", type = {Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 272 @Description(shortDefinition="Target", formalDefinition="The Insurer who is target of the request." ) 273 protected Reference insurer; 274 275 /** 276 * The actual object that is the target of the reference (The Insurer who is target of the request.) 277 */ 278 protected Organization insurerTarget; 279 280 /** 281 * Facility where the services were provided. 282 */ 283 @Child(name = "facility", type = {Location.class}, order=10, min=0, max=1, modifier=false, summary=false) 284 @Description(shortDefinition="Servicing Facility", formalDefinition="Facility where the services were provided." ) 285 protected Reference facility; 286 287 /** 288 * The actual object that is the target of the reference (Facility where the services were provided.) 289 */ 290 protected Location facilityTarget; 291 292 /** 293 * Financial instrument by which payment information for health care. 294 */ 295 @Child(name = "coverage", type = {Coverage.class}, order=11, min=0, max=1, modifier=false, summary=false) 296 @Description(shortDefinition="Insurance or medical plan", formalDefinition="Financial instrument by which payment information for health care." ) 297 protected Reference coverage; 298 299 /** 300 * The actual object that is the target of the reference (Financial instrument by which payment information for health care.) 301 */ 302 protected Coverage coverageTarget; 303 304 /** 305 * The contract number of a business agreement which describes the terms and conditions. 306 */ 307 @Child(name = "businessArrangement", type = {StringType.class}, order=12, min=0, max=1, modifier=false, summary=false) 308 @Description(shortDefinition="Business agreement", formalDefinition="The contract number of a business agreement which describes the terms and conditions." ) 309 protected StringType businessArrangement; 310 311 /** 312 * Dental, Vision, Medical, Pharmacy, Rehab etc. 313 */ 314 @Child(name = "benefitCategory", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 315 @Description(shortDefinition="Type of services covered", formalDefinition="Dental, Vision, Medical, Pharmacy, Rehab etc." ) 316 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-category") 317 protected CodeableConcept benefitCategory; 318 319 /** 320 * Dental: basic, major, ortho; Vision exam, glasses, contacts; etc. 321 */ 322 @Child(name = "benefitSubCategory", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 323 @Description(shortDefinition="Detailed services covered within the type", formalDefinition="Dental: basic, major, ortho; Vision exam, glasses, contacts; etc." ) 324 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 325 protected CodeableConcept benefitSubCategory; 326 327 private static final long serialVersionUID = 899259023L; 328 329 /** 330 * Constructor 331 */ 332 public EligibilityRequest() { 333 super(); 334 } 335 336 /** 337 * @return {@link #identifier} (The Response business identifier.) 338 */ 339 public List<Identifier> getIdentifier() { 340 if (this.identifier == null) 341 this.identifier = new ArrayList<Identifier>(); 342 return this.identifier; 343 } 344 345 /** 346 * @return Returns a reference to <code>this</code> for easy method chaining 347 */ 348 public EligibilityRequest setIdentifier(List<Identifier> theIdentifier) { 349 this.identifier = theIdentifier; 350 return this; 351 } 352 353 public boolean hasIdentifier() { 354 if (this.identifier == null) 355 return false; 356 for (Identifier item : this.identifier) 357 if (!item.isEmpty()) 358 return true; 359 return false; 360 } 361 362 public Identifier addIdentifier() { //3 363 Identifier t = new Identifier(); 364 if (this.identifier == null) 365 this.identifier = new ArrayList<Identifier>(); 366 this.identifier.add(t); 367 return t; 368 } 369 370 public EligibilityRequest addIdentifier(Identifier t) { //3 371 if (t == null) 372 return this; 373 if (this.identifier == null) 374 this.identifier = new ArrayList<Identifier>(); 375 this.identifier.add(t); 376 return this; 377 } 378 379 /** 380 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 381 */ 382 public Identifier getIdentifierFirstRep() { 383 if (getIdentifier().isEmpty()) { 384 addIdentifier(); 385 } 386 return getIdentifier().get(0); 387 } 388 389 /** 390 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 391 */ 392 public Enumeration<EligibilityRequestStatus> getStatusElement() { 393 if (this.status == null) 394 if (Configuration.errorOnAutoCreate()) 395 throw new Error("Attempt to auto-create EligibilityRequest.status"); 396 else if (Configuration.doAutoCreate()) 397 this.status = new Enumeration<EligibilityRequestStatus>(new EligibilityRequestStatusEnumFactory()); // bb 398 return this.status; 399 } 400 401 public boolean hasStatusElement() { 402 return this.status != null && !this.status.isEmpty(); 403 } 404 405 public boolean hasStatus() { 406 return this.status != null && !this.status.isEmpty(); 407 } 408 409 /** 410 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 411 */ 412 public EligibilityRequest setStatusElement(Enumeration<EligibilityRequestStatus> value) { 413 this.status = value; 414 return this; 415 } 416 417 /** 418 * @return The status of the resource instance. 419 */ 420 public EligibilityRequestStatus getStatus() { 421 return this.status == null ? null : this.status.getValue(); 422 } 423 424 /** 425 * @param value The status of the resource instance. 426 */ 427 public EligibilityRequest setStatus(EligibilityRequestStatus value) { 428 if (value == null) 429 this.status = null; 430 else { 431 if (this.status == null) 432 this.status = new Enumeration<EligibilityRequestStatus>(new EligibilityRequestStatusEnumFactory()); 433 this.status.setValue(value); 434 } 435 return this; 436 } 437 438 /** 439 * @return {@link #priority} (Immediate (STAT), best effort (NORMAL), deferred (DEFER).) 440 */ 441 public CodeableConcept getPriority() { 442 if (this.priority == null) 443 if (Configuration.errorOnAutoCreate()) 444 throw new Error("Attempt to auto-create EligibilityRequest.priority"); 445 else if (Configuration.doAutoCreate()) 446 this.priority = new CodeableConcept(); // cc 447 return this.priority; 448 } 449 450 public boolean hasPriority() { 451 return this.priority != null && !this.priority.isEmpty(); 452 } 453 454 /** 455 * @param value {@link #priority} (Immediate (STAT), best effort (NORMAL), deferred (DEFER).) 456 */ 457 public EligibilityRequest setPriority(CodeableConcept value) { 458 this.priority = value; 459 return this; 460 } 461 462 /** 463 * @return {@link #patient} (Patient Resource.) 464 */ 465 public Reference getPatient() { 466 if (this.patient == null) 467 if (Configuration.errorOnAutoCreate()) 468 throw new Error("Attempt to auto-create EligibilityRequest.patient"); 469 else if (Configuration.doAutoCreate()) 470 this.patient = new Reference(); // cc 471 return this.patient; 472 } 473 474 public boolean hasPatient() { 475 return this.patient != null && !this.patient.isEmpty(); 476 } 477 478 /** 479 * @param value {@link #patient} (Patient Resource.) 480 */ 481 public EligibilityRequest setPatient(Reference value) { 482 this.patient = value; 483 return this; 484 } 485 486 /** 487 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient Resource.) 488 */ 489 public Patient getPatientTarget() { 490 if (this.patientTarget == null) 491 if (Configuration.errorOnAutoCreate()) 492 throw new Error("Attempt to auto-create EligibilityRequest.patient"); 493 else if (Configuration.doAutoCreate()) 494 this.patientTarget = new Patient(); // aa 495 return this.patientTarget; 496 } 497 498 /** 499 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient Resource.) 500 */ 501 public EligibilityRequest setPatientTarget(Patient value) { 502 this.patientTarget = value; 503 return this; 504 } 505 506 /** 507 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 508 */ 509 public Type getServiced() { 510 return this.serviced; 511 } 512 513 /** 514 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 515 */ 516 public DateType getServicedDateType() throws FHIRException { 517 if (this.serviced == null) 518 return null; 519 if (!(this.serviced instanceof DateType)) 520 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 521 return (DateType) this.serviced; 522 } 523 524 public boolean hasServicedDateType() { 525 return this.serviced instanceof DateType; 526 } 527 528 /** 529 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 530 */ 531 public Period getServicedPeriod() throws FHIRException { 532 if (this.serviced == null) 533 return null; 534 if (!(this.serviced instanceof Period)) 535 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 536 return (Period) this.serviced; 537 } 538 539 public boolean hasServicedPeriod() { 540 return this.serviced instanceof Period; 541 } 542 543 public boolean hasServiced() { 544 return this.serviced != null && !this.serviced.isEmpty(); 545 } 546 547 /** 548 * @param value {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 549 */ 550 public EligibilityRequest setServiced(Type value) throws FHIRFormatError { 551 if (value != null && !(value instanceof DateType || value instanceof Period)) 552 throw new FHIRFormatError("Not the right type for EligibilityRequest.serviced[x]: "+value.fhirType()); 553 this.serviced = value; 554 return this; 555 } 556 557 /** 558 * @return {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 559 */ 560 public DateTimeType getCreatedElement() { 561 if (this.created == null) 562 if (Configuration.errorOnAutoCreate()) 563 throw new Error("Attempt to auto-create EligibilityRequest.created"); 564 else if (Configuration.doAutoCreate()) 565 this.created = new DateTimeType(); // bb 566 return this.created; 567 } 568 569 public boolean hasCreatedElement() { 570 return this.created != null && !this.created.isEmpty(); 571 } 572 573 public boolean hasCreated() { 574 return this.created != null && !this.created.isEmpty(); 575 } 576 577 /** 578 * @param value {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 579 */ 580 public EligibilityRequest setCreatedElement(DateTimeType value) { 581 this.created = value; 582 return this; 583 } 584 585 /** 586 * @return The date when this resource was created. 587 */ 588 public Date getCreated() { 589 return this.created == null ? null : this.created.getValue(); 590 } 591 592 /** 593 * @param value The date when this resource was created. 594 */ 595 public EligibilityRequest setCreated(Date value) { 596 if (value == null) 597 this.created = null; 598 else { 599 if (this.created == null) 600 this.created = new DateTimeType(); 601 this.created.setValue(value); 602 } 603 return this; 604 } 605 606 /** 607 * @return {@link #enterer} (Person who created the invoice/claim/pre-determination or pre-authorization.) 608 */ 609 public Reference getEnterer() { 610 if (this.enterer == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create EligibilityRequest.enterer"); 613 else if (Configuration.doAutoCreate()) 614 this.enterer = new Reference(); // cc 615 return this.enterer; 616 } 617 618 public boolean hasEnterer() { 619 return this.enterer != null && !this.enterer.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #enterer} (Person who created the invoice/claim/pre-determination or pre-authorization.) 624 */ 625 public EligibilityRequest setEnterer(Reference value) { 626 this.enterer = value; 627 return this; 628 } 629 630 /** 631 * @return {@link #enterer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Person who created the invoice/claim/pre-determination or pre-authorization.) 632 */ 633 public Practitioner getEntererTarget() { 634 if (this.entererTarget == null) 635 if (Configuration.errorOnAutoCreate()) 636 throw new Error("Attempt to auto-create EligibilityRequest.enterer"); 637 else if (Configuration.doAutoCreate()) 638 this.entererTarget = new Practitioner(); // aa 639 return this.entererTarget; 640 } 641 642 /** 643 * @param value {@link #enterer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Person who created the invoice/claim/pre-determination or pre-authorization.) 644 */ 645 public EligibilityRequest setEntererTarget(Practitioner value) { 646 this.entererTarget = value; 647 return this; 648 } 649 650 /** 651 * @return {@link #provider} (The practitioner who is responsible for the services rendered to the patient.) 652 */ 653 public Reference getProvider() { 654 if (this.provider == null) 655 if (Configuration.errorOnAutoCreate()) 656 throw new Error("Attempt to auto-create EligibilityRequest.provider"); 657 else if (Configuration.doAutoCreate()) 658 this.provider = new Reference(); // cc 659 return this.provider; 660 } 661 662 public boolean hasProvider() { 663 return this.provider != null && !this.provider.isEmpty(); 664 } 665 666 /** 667 * @param value {@link #provider} (The practitioner who is responsible for the services rendered to the patient.) 668 */ 669 public EligibilityRequest setProvider(Reference value) { 670 this.provider = value; 671 return this; 672 } 673 674 /** 675 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 676 */ 677 public Practitioner getProviderTarget() { 678 if (this.providerTarget == null) 679 if (Configuration.errorOnAutoCreate()) 680 throw new Error("Attempt to auto-create EligibilityRequest.provider"); 681 else if (Configuration.doAutoCreate()) 682 this.providerTarget = new Practitioner(); // aa 683 return this.providerTarget; 684 } 685 686 /** 687 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 688 */ 689 public EligibilityRequest setProviderTarget(Practitioner value) { 690 this.providerTarget = value; 691 return this; 692 } 693 694 /** 695 * @return {@link #organization} (The organization which is responsible for the services rendered to the patient.) 696 */ 697 public Reference getOrganization() { 698 if (this.organization == null) 699 if (Configuration.errorOnAutoCreate()) 700 throw new Error("Attempt to auto-create EligibilityRequest.organization"); 701 else if (Configuration.doAutoCreate()) 702 this.organization = new Reference(); // cc 703 return this.organization; 704 } 705 706 public boolean hasOrganization() { 707 return this.organization != null && !this.organization.isEmpty(); 708 } 709 710 /** 711 * @param value {@link #organization} (The organization which is responsible for the services rendered to the patient.) 712 */ 713 public EligibilityRequest setOrganization(Reference value) { 714 this.organization = value; 715 return this; 716 } 717 718 /** 719 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 720 */ 721 public Organization getOrganizationTarget() { 722 if (this.organizationTarget == null) 723 if (Configuration.errorOnAutoCreate()) 724 throw new Error("Attempt to auto-create EligibilityRequest.organization"); 725 else if (Configuration.doAutoCreate()) 726 this.organizationTarget = new Organization(); // aa 727 return this.organizationTarget; 728 } 729 730 /** 731 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 732 */ 733 public EligibilityRequest setOrganizationTarget(Organization value) { 734 this.organizationTarget = value; 735 return this; 736 } 737 738 /** 739 * @return {@link #insurer} (The Insurer who is target of the request.) 740 */ 741 public Reference getInsurer() { 742 if (this.insurer == null) 743 if (Configuration.errorOnAutoCreate()) 744 throw new Error("Attempt to auto-create EligibilityRequest.insurer"); 745 else if (Configuration.doAutoCreate()) 746 this.insurer = new Reference(); // cc 747 return this.insurer; 748 } 749 750 public boolean hasInsurer() { 751 return this.insurer != null && !this.insurer.isEmpty(); 752 } 753 754 /** 755 * @param value {@link #insurer} (The Insurer who is target of the request.) 756 */ 757 public EligibilityRequest setInsurer(Reference value) { 758 this.insurer = value; 759 return this; 760 } 761 762 /** 763 * @return {@link #insurer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 764 */ 765 public Organization getInsurerTarget() { 766 if (this.insurerTarget == null) 767 if (Configuration.errorOnAutoCreate()) 768 throw new Error("Attempt to auto-create EligibilityRequest.insurer"); 769 else if (Configuration.doAutoCreate()) 770 this.insurerTarget = new Organization(); // aa 771 return this.insurerTarget; 772 } 773 774 /** 775 * @param value {@link #insurer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 776 */ 777 public EligibilityRequest setInsurerTarget(Organization value) { 778 this.insurerTarget = value; 779 return this; 780 } 781 782 /** 783 * @return {@link #facility} (Facility where the services were provided.) 784 */ 785 public Reference getFacility() { 786 if (this.facility == null) 787 if (Configuration.errorOnAutoCreate()) 788 throw new Error("Attempt to auto-create EligibilityRequest.facility"); 789 else if (Configuration.doAutoCreate()) 790 this.facility = new Reference(); // cc 791 return this.facility; 792 } 793 794 public boolean hasFacility() { 795 return this.facility != null && !this.facility.isEmpty(); 796 } 797 798 /** 799 * @param value {@link #facility} (Facility where the services were provided.) 800 */ 801 public EligibilityRequest setFacility(Reference value) { 802 this.facility = value; 803 return this; 804 } 805 806 /** 807 * @return {@link #facility} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 808 */ 809 public Location getFacilityTarget() { 810 if (this.facilityTarget == null) 811 if (Configuration.errorOnAutoCreate()) 812 throw new Error("Attempt to auto-create EligibilityRequest.facility"); 813 else if (Configuration.doAutoCreate()) 814 this.facilityTarget = new Location(); // aa 815 return this.facilityTarget; 816 } 817 818 /** 819 * @param value {@link #facility} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 820 */ 821 public EligibilityRequest setFacilityTarget(Location value) { 822 this.facilityTarget = value; 823 return this; 824 } 825 826 /** 827 * @return {@link #coverage} (Financial instrument by which payment information for health care.) 828 */ 829 public Reference getCoverage() { 830 if (this.coverage == null) 831 if (Configuration.errorOnAutoCreate()) 832 throw new Error("Attempt to auto-create EligibilityRequest.coverage"); 833 else if (Configuration.doAutoCreate()) 834 this.coverage = new Reference(); // cc 835 return this.coverage; 836 } 837 838 public boolean hasCoverage() { 839 return this.coverage != null && !this.coverage.isEmpty(); 840 } 841 842 /** 843 * @param value {@link #coverage} (Financial instrument by which payment information for health care.) 844 */ 845 public EligibilityRequest setCoverage(Reference value) { 846 this.coverage = value; 847 return this; 848 } 849 850 /** 851 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Financial instrument by which payment information for health care.) 852 */ 853 public Coverage getCoverageTarget() { 854 if (this.coverageTarget == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create EligibilityRequest.coverage"); 857 else if (Configuration.doAutoCreate()) 858 this.coverageTarget = new Coverage(); // aa 859 return this.coverageTarget; 860 } 861 862 /** 863 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Financial instrument by which payment information for health care.) 864 */ 865 public EligibilityRequest setCoverageTarget(Coverage value) { 866 this.coverageTarget = value; 867 return this; 868 } 869 870 /** 871 * @return {@link #businessArrangement} (The contract number of a business agreement which describes the terms and conditions.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 872 */ 873 public StringType getBusinessArrangementElement() { 874 if (this.businessArrangement == null) 875 if (Configuration.errorOnAutoCreate()) 876 throw new Error("Attempt to auto-create EligibilityRequest.businessArrangement"); 877 else if (Configuration.doAutoCreate()) 878 this.businessArrangement = new StringType(); // bb 879 return this.businessArrangement; 880 } 881 882 public boolean hasBusinessArrangementElement() { 883 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 884 } 885 886 public boolean hasBusinessArrangement() { 887 return this.businessArrangement != null && !this.businessArrangement.isEmpty(); 888 } 889 890 /** 891 * @param value {@link #businessArrangement} (The contract number of a business agreement which describes the terms and conditions.). This is the underlying object with id, value and extensions. The accessor "getBusinessArrangement" gives direct access to the value 892 */ 893 public EligibilityRequest setBusinessArrangementElement(StringType value) { 894 this.businessArrangement = value; 895 return this; 896 } 897 898 /** 899 * @return The contract number of a business agreement which describes the terms and conditions. 900 */ 901 public String getBusinessArrangement() { 902 return this.businessArrangement == null ? null : this.businessArrangement.getValue(); 903 } 904 905 /** 906 * @param value The contract number of a business agreement which describes the terms and conditions. 907 */ 908 public EligibilityRequest setBusinessArrangement(String value) { 909 if (Utilities.noString(value)) 910 this.businessArrangement = null; 911 else { 912 if (this.businessArrangement == null) 913 this.businessArrangement = new StringType(); 914 this.businessArrangement.setValue(value); 915 } 916 return this; 917 } 918 919 /** 920 * @return {@link #benefitCategory} (Dental, Vision, Medical, Pharmacy, Rehab etc.) 921 */ 922 public CodeableConcept getBenefitCategory() { 923 if (this.benefitCategory == null) 924 if (Configuration.errorOnAutoCreate()) 925 throw new Error("Attempt to auto-create EligibilityRequest.benefitCategory"); 926 else if (Configuration.doAutoCreate()) 927 this.benefitCategory = new CodeableConcept(); // cc 928 return this.benefitCategory; 929 } 930 931 public boolean hasBenefitCategory() { 932 return this.benefitCategory != null && !this.benefitCategory.isEmpty(); 933 } 934 935 /** 936 * @param value {@link #benefitCategory} (Dental, Vision, Medical, Pharmacy, Rehab etc.) 937 */ 938 public EligibilityRequest setBenefitCategory(CodeableConcept value) { 939 this.benefitCategory = value; 940 return this; 941 } 942 943 /** 944 * @return {@link #benefitSubCategory} (Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.) 945 */ 946 public CodeableConcept getBenefitSubCategory() { 947 if (this.benefitSubCategory == null) 948 if (Configuration.errorOnAutoCreate()) 949 throw new Error("Attempt to auto-create EligibilityRequest.benefitSubCategory"); 950 else if (Configuration.doAutoCreate()) 951 this.benefitSubCategory = new CodeableConcept(); // cc 952 return this.benefitSubCategory; 953 } 954 955 public boolean hasBenefitSubCategory() { 956 return this.benefitSubCategory != null && !this.benefitSubCategory.isEmpty(); 957 } 958 959 /** 960 * @param value {@link #benefitSubCategory} (Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.) 961 */ 962 public EligibilityRequest setBenefitSubCategory(CodeableConcept value) { 963 this.benefitSubCategory = value; 964 return this; 965 } 966 967 protected void listChildren(List<Property> children) { 968 super.listChildren(children); 969 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 970 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 971 children.add(new Property("priority", "CodeableConcept", "Immediate (STAT), best effort (NORMAL), deferred (DEFER).", 0, 1, priority)); 972 children.add(new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient)); 973 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 974 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 975 children.add(new Property("enterer", "Reference(Practitioner)", "Person who created the invoice/claim/pre-determination or pre-authorization.", 0, 1, enterer)); 976 children.add(new Property("provider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider)); 977 children.add(new Property("organization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, organization)); 978 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 979 children.add(new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility)); 980 children.add(new Property("coverage", "Reference(Coverage)", "Financial instrument by which payment information for health care.", 0, 1, coverage)); 981 children.add(new Property("businessArrangement", "string", "The contract number of a business agreement which describes the terms and conditions.", 0, 1, businessArrangement)); 982 children.add(new Property("benefitCategory", "CodeableConcept", "Dental, Vision, Medical, Pharmacy, Rehab etc.", 0, 1, benefitCategory)); 983 children.add(new Property("benefitSubCategory", "CodeableConcept", "Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.", 0, 1, benefitSubCategory)); 984 } 985 986 @Override 987 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 988 switch (_hash) { 989 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 990 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 991 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Immediate (STAT), best effort (NORMAL), deferred (DEFER).", 0, 1, priority); 992 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient); 993 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 994 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 995 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 996 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 997 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created); 998 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner)", "Person who created the invoice/claim/pre-determination or pre-authorization.", 0, 1, enterer); 999 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider); 1000 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, organization); 1001 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer); 1002 case 501116579: /*facility*/ return new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility); 1003 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Financial instrument by which payment information for health care.", 0, 1, coverage); 1004 case 259920682: /*businessArrangement*/ return new Property("businessArrangement", "string", "The contract number of a business agreement which describes the terms and conditions.", 0, 1, businessArrangement); 1005 case -1023390027: /*benefitCategory*/ return new Property("benefitCategory", "CodeableConcept", "Dental, Vision, Medical, Pharmacy, Rehab etc.", 0, 1, benefitCategory); 1006 case 1987878471: /*benefitSubCategory*/ return new Property("benefitSubCategory", "CodeableConcept", "Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.", 0, 1, benefitSubCategory); 1007 default: return super.getNamedProperty(_hash, _name, _checkValid); 1008 } 1009 1010 } 1011 1012 @Override 1013 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1014 switch (hash) { 1015 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1016 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EligibilityRequestStatus> 1017 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 1018 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1019 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // Type 1020 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 1021 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 1022 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1023 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 1024 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 1025 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 1026 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 1027 case 259920682: /*businessArrangement*/ return this.businessArrangement == null ? new Base[0] : new Base[] {this.businessArrangement}; // StringType 1028 case -1023390027: /*benefitCategory*/ return this.benefitCategory == null ? new Base[0] : new Base[] {this.benefitCategory}; // CodeableConcept 1029 case 1987878471: /*benefitSubCategory*/ return this.benefitSubCategory == null ? new Base[0] : new Base[] {this.benefitSubCategory}; // CodeableConcept 1030 default: return super.getProperty(hash, name, checkValid); 1031 } 1032 1033 } 1034 1035 @Override 1036 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1037 switch (hash) { 1038 case -1618432855: // identifier 1039 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1040 return value; 1041 case -892481550: // status 1042 value = new EligibilityRequestStatusEnumFactory().fromType(castToCode(value)); 1043 this.status = (Enumeration) value; // Enumeration<EligibilityRequestStatus> 1044 return value; 1045 case -1165461084: // priority 1046 this.priority = castToCodeableConcept(value); // CodeableConcept 1047 return value; 1048 case -791418107: // patient 1049 this.patient = castToReference(value); // Reference 1050 return value; 1051 case 1379209295: // serviced 1052 this.serviced = castToType(value); // Type 1053 return value; 1054 case 1028554472: // created 1055 this.created = castToDateTime(value); // DateTimeType 1056 return value; 1057 case -1591951995: // enterer 1058 this.enterer = castToReference(value); // Reference 1059 return value; 1060 case -987494927: // provider 1061 this.provider = castToReference(value); // Reference 1062 return value; 1063 case 1178922291: // organization 1064 this.organization = castToReference(value); // Reference 1065 return value; 1066 case 1957615864: // insurer 1067 this.insurer = castToReference(value); // Reference 1068 return value; 1069 case 501116579: // facility 1070 this.facility = castToReference(value); // Reference 1071 return value; 1072 case -351767064: // coverage 1073 this.coverage = castToReference(value); // Reference 1074 return value; 1075 case 259920682: // businessArrangement 1076 this.businessArrangement = castToString(value); // StringType 1077 return value; 1078 case -1023390027: // benefitCategory 1079 this.benefitCategory = castToCodeableConcept(value); // CodeableConcept 1080 return value; 1081 case 1987878471: // benefitSubCategory 1082 this.benefitSubCategory = castToCodeableConcept(value); // CodeableConcept 1083 return value; 1084 default: return super.setProperty(hash, name, value); 1085 } 1086 1087 } 1088 1089 @Override 1090 public Base setProperty(String name, Base value) throws FHIRException { 1091 if (name.equals("identifier")) { 1092 this.getIdentifier().add(castToIdentifier(value)); 1093 } else if (name.equals("status")) { 1094 value = new EligibilityRequestStatusEnumFactory().fromType(castToCode(value)); 1095 this.status = (Enumeration) value; // Enumeration<EligibilityRequestStatus> 1096 } else if (name.equals("priority")) { 1097 this.priority = castToCodeableConcept(value); // CodeableConcept 1098 } else if (name.equals("patient")) { 1099 this.patient = castToReference(value); // Reference 1100 } else if (name.equals("serviced[x]")) { 1101 this.serviced = castToType(value); // Type 1102 } else if (name.equals("created")) { 1103 this.created = castToDateTime(value); // DateTimeType 1104 } else if (name.equals("enterer")) { 1105 this.enterer = castToReference(value); // Reference 1106 } else if (name.equals("provider")) { 1107 this.provider = castToReference(value); // Reference 1108 } else if (name.equals("organization")) { 1109 this.organization = castToReference(value); // Reference 1110 } else if (name.equals("insurer")) { 1111 this.insurer = castToReference(value); // Reference 1112 } else if (name.equals("facility")) { 1113 this.facility = castToReference(value); // Reference 1114 } else if (name.equals("coverage")) { 1115 this.coverage = castToReference(value); // Reference 1116 } else if (name.equals("businessArrangement")) { 1117 this.businessArrangement = castToString(value); // StringType 1118 } else if (name.equals("benefitCategory")) { 1119 this.benefitCategory = castToCodeableConcept(value); // CodeableConcept 1120 } else if (name.equals("benefitSubCategory")) { 1121 this.benefitSubCategory = castToCodeableConcept(value); // CodeableConcept 1122 } else 1123 return super.setProperty(name, value); 1124 return value; 1125 } 1126 1127 @Override 1128 public Base makeProperty(int hash, String name) throws FHIRException { 1129 switch (hash) { 1130 case -1618432855: return addIdentifier(); 1131 case -892481550: return getStatusElement(); 1132 case -1165461084: return getPriority(); 1133 case -791418107: return getPatient(); 1134 case -1927922223: return getServiced(); 1135 case 1379209295: return getServiced(); 1136 case 1028554472: return getCreatedElement(); 1137 case -1591951995: return getEnterer(); 1138 case -987494927: return getProvider(); 1139 case 1178922291: return getOrganization(); 1140 case 1957615864: return getInsurer(); 1141 case 501116579: return getFacility(); 1142 case -351767064: return getCoverage(); 1143 case 259920682: return getBusinessArrangementElement(); 1144 case -1023390027: return getBenefitCategory(); 1145 case 1987878471: return getBenefitSubCategory(); 1146 default: return super.makeProperty(hash, name); 1147 } 1148 1149 } 1150 1151 @Override 1152 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1153 switch (hash) { 1154 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1155 case -892481550: /*status*/ return new String[] {"code"}; 1156 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 1157 case -791418107: /*patient*/ return new String[] {"Reference"}; 1158 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 1159 case 1028554472: /*created*/ return new String[] {"dateTime"}; 1160 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 1161 case -987494927: /*provider*/ return new String[] {"Reference"}; 1162 case 1178922291: /*organization*/ return new String[] {"Reference"}; 1163 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 1164 case 501116579: /*facility*/ return new String[] {"Reference"}; 1165 case -351767064: /*coverage*/ return new String[] {"Reference"}; 1166 case 259920682: /*businessArrangement*/ return new String[] {"string"}; 1167 case -1023390027: /*benefitCategory*/ return new String[] {"CodeableConcept"}; 1168 case 1987878471: /*benefitSubCategory*/ return new String[] {"CodeableConcept"}; 1169 default: return super.getTypesForProperty(hash, name); 1170 } 1171 1172 } 1173 1174 @Override 1175 public Base addChild(String name) throws FHIRException { 1176 if (name.equals("identifier")) { 1177 return addIdentifier(); 1178 } 1179 else if (name.equals("status")) { 1180 throw new FHIRException("Cannot call addChild on a singleton property EligibilityRequest.status"); 1181 } 1182 else if (name.equals("priority")) { 1183 this.priority = new CodeableConcept(); 1184 return this.priority; 1185 } 1186 else if (name.equals("patient")) { 1187 this.patient = new Reference(); 1188 return this.patient; 1189 } 1190 else if (name.equals("servicedDate")) { 1191 this.serviced = new DateType(); 1192 return this.serviced; 1193 } 1194 else if (name.equals("servicedPeriod")) { 1195 this.serviced = new Period(); 1196 return this.serviced; 1197 } 1198 else if (name.equals("created")) { 1199 throw new FHIRException("Cannot call addChild on a singleton property EligibilityRequest.created"); 1200 } 1201 else if (name.equals("enterer")) { 1202 this.enterer = new Reference(); 1203 return this.enterer; 1204 } 1205 else if (name.equals("provider")) { 1206 this.provider = new Reference(); 1207 return this.provider; 1208 } 1209 else if (name.equals("organization")) { 1210 this.organization = new Reference(); 1211 return this.organization; 1212 } 1213 else if (name.equals("insurer")) { 1214 this.insurer = new Reference(); 1215 return this.insurer; 1216 } 1217 else if (name.equals("facility")) { 1218 this.facility = new Reference(); 1219 return this.facility; 1220 } 1221 else if (name.equals("coverage")) { 1222 this.coverage = new Reference(); 1223 return this.coverage; 1224 } 1225 else if (name.equals("businessArrangement")) { 1226 throw new FHIRException("Cannot call addChild on a singleton property EligibilityRequest.businessArrangement"); 1227 } 1228 else if (name.equals("benefitCategory")) { 1229 this.benefitCategory = new CodeableConcept(); 1230 return this.benefitCategory; 1231 } 1232 else if (name.equals("benefitSubCategory")) { 1233 this.benefitSubCategory = new CodeableConcept(); 1234 return this.benefitSubCategory; 1235 } 1236 else 1237 return super.addChild(name); 1238 } 1239 1240 public String fhirType() { 1241 return "EligibilityRequest"; 1242 1243 } 1244 1245 public EligibilityRequest copy() { 1246 EligibilityRequest dst = new EligibilityRequest(); 1247 copyValues(dst); 1248 if (identifier != null) { 1249 dst.identifier = new ArrayList<Identifier>(); 1250 for (Identifier i : identifier) 1251 dst.identifier.add(i.copy()); 1252 }; 1253 dst.status = status == null ? null : status.copy(); 1254 dst.priority = priority == null ? null : priority.copy(); 1255 dst.patient = patient == null ? null : patient.copy(); 1256 dst.serviced = serviced == null ? null : serviced.copy(); 1257 dst.created = created == null ? null : created.copy(); 1258 dst.enterer = enterer == null ? null : enterer.copy(); 1259 dst.provider = provider == null ? null : provider.copy(); 1260 dst.organization = organization == null ? null : organization.copy(); 1261 dst.insurer = insurer == null ? null : insurer.copy(); 1262 dst.facility = facility == null ? null : facility.copy(); 1263 dst.coverage = coverage == null ? null : coverage.copy(); 1264 dst.businessArrangement = businessArrangement == null ? null : businessArrangement.copy(); 1265 dst.benefitCategory = benefitCategory == null ? null : benefitCategory.copy(); 1266 dst.benefitSubCategory = benefitSubCategory == null ? null : benefitSubCategory.copy(); 1267 return dst; 1268 } 1269 1270 protected EligibilityRequest typedCopy() { 1271 return copy(); 1272 } 1273 1274 @Override 1275 public boolean equalsDeep(Base other_) { 1276 if (!super.equalsDeep(other_)) 1277 return false; 1278 if (!(other_ instanceof EligibilityRequest)) 1279 return false; 1280 EligibilityRequest o = (EligibilityRequest) other_; 1281 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(priority, o.priority, true) 1282 && compareDeep(patient, o.patient, true) && compareDeep(serviced, o.serviced, true) && compareDeep(created, o.created, true) 1283 && compareDeep(enterer, o.enterer, true) && compareDeep(provider, o.provider, true) && compareDeep(organization, o.organization, true) 1284 && compareDeep(insurer, o.insurer, true) && compareDeep(facility, o.facility, true) && compareDeep(coverage, o.coverage, true) 1285 && compareDeep(businessArrangement, o.businessArrangement, true) && compareDeep(benefitCategory, o.benefitCategory, true) 1286 && compareDeep(benefitSubCategory, o.benefitSubCategory, true); 1287 } 1288 1289 @Override 1290 public boolean equalsShallow(Base other_) { 1291 if (!super.equalsShallow(other_)) 1292 return false; 1293 if (!(other_ instanceof EligibilityRequest)) 1294 return false; 1295 EligibilityRequest o = (EligibilityRequest) other_; 1296 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(businessArrangement, o.businessArrangement, true) 1297 ; 1298 } 1299 1300 public boolean isEmpty() { 1301 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, priority 1302 , patient, serviced, created, enterer, provider, organization, insurer, facility 1303 , coverage, businessArrangement, benefitCategory, benefitSubCategory); 1304 } 1305 1306 @Override 1307 public ResourceType getResourceType() { 1308 return ResourceType.EligibilityRequest; 1309 } 1310 1311 /** 1312 * Search parameter: <b>identifier</b> 1313 * <p> 1314 * Description: <b>The business identifier of the Eligibility</b><br> 1315 * Type: <b>token</b><br> 1316 * Path: <b>EligibilityRequest.identifier</b><br> 1317 * </p> 1318 */ 1319 @SearchParamDefinition(name="identifier", path="EligibilityRequest.identifier", description="The business identifier of the Eligibility", type="token" ) 1320 public static final String SP_IDENTIFIER = "identifier"; 1321 /** 1322 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1323 * <p> 1324 * Description: <b>The business identifier of the Eligibility</b><br> 1325 * Type: <b>token</b><br> 1326 * Path: <b>EligibilityRequest.identifier</b><br> 1327 * </p> 1328 */ 1329 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1330 1331 /** 1332 * Search parameter: <b>provider</b> 1333 * <p> 1334 * Description: <b>The reference to the provider</b><br> 1335 * Type: <b>reference</b><br> 1336 * Path: <b>EligibilityRequest.provider</b><br> 1337 * </p> 1338 */ 1339 @SearchParamDefinition(name="provider", path="EligibilityRequest.provider", description="The reference to the provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 1340 public static final String SP_PROVIDER = "provider"; 1341 /** 1342 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 1343 * <p> 1344 * Description: <b>The reference to the provider</b><br> 1345 * Type: <b>reference</b><br> 1346 * Path: <b>EligibilityRequest.provider</b><br> 1347 * </p> 1348 */ 1349 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 1350 1351/** 1352 * Constant for fluent queries to be used to add include statements. Specifies 1353 * the path value of "<b>EligibilityRequest:provider</b>". 1354 */ 1355 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("EligibilityRequest:provider").toLocked(); 1356 1357 /** 1358 * Search parameter: <b>patient</b> 1359 * <p> 1360 * Description: <b>The reference to the patient</b><br> 1361 * Type: <b>reference</b><br> 1362 * Path: <b>EligibilityRequest.patient</b><br> 1363 * </p> 1364 */ 1365 @SearchParamDefinition(name="patient", path="EligibilityRequest.patient", description="The reference to the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1366 public static final String SP_PATIENT = "patient"; 1367 /** 1368 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1369 * <p> 1370 * Description: <b>The reference to the patient</b><br> 1371 * Type: <b>reference</b><br> 1372 * Path: <b>EligibilityRequest.patient</b><br> 1373 * </p> 1374 */ 1375 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1376 1377/** 1378 * Constant for fluent queries to be used to add include statements. Specifies 1379 * the path value of "<b>EligibilityRequest:patient</b>". 1380 */ 1381 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("EligibilityRequest:patient").toLocked(); 1382 1383 /** 1384 * Search parameter: <b>created</b> 1385 * <p> 1386 * Description: <b>The creation date for the EOB</b><br> 1387 * Type: <b>date</b><br> 1388 * Path: <b>EligibilityRequest.created</b><br> 1389 * </p> 1390 */ 1391 @SearchParamDefinition(name="created", path="EligibilityRequest.created", description="The creation date for the EOB", type="date" ) 1392 public static final String SP_CREATED = "created"; 1393 /** 1394 * <b>Fluent Client</b> search parameter constant for <b>created</b> 1395 * <p> 1396 * Description: <b>The creation date for the EOB</b><br> 1397 * Type: <b>date</b><br> 1398 * Path: <b>EligibilityRequest.created</b><br> 1399 * </p> 1400 */ 1401 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 1402 1403 /** 1404 * Search parameter: <b>organization</b> 1405 * <p> 1406 * Description: <b>The reference to the providing organization</b><br> 1407 * Type: <b>reference</b><br> 1408 * Path: <b>EligibilityRequest.organization</b><br> 1409 * </p> 1410 */ 1411 @SearchParamDefinition(name="organization", path="EligibilityRequest.organization", description="The reference to the providing organization", type="reference", target={Organization.class } ) 1412 public static final String SP_ORGANIZATION = "organization"; 1413 /** 1414 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1415 * <p> 1416 * Description: <b>The reference to the providing organization</b><br> 1417 * Type: <b>reference</b><br> 1418 * Path: <b>EligibilityRequest.organization</b><br> 1419 * </p> 1420 */ 1421 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1422 1423/** 1424 * Constant for fluent queries to be used to add include statements. Specifies 1425 * the path value of "<b>EligibilityRequest:organization</b>". 1426 */ 1427 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("EligibilityRequest:organization").toLocked(); 1428 1429 /** 1430 * Search parameter: <b>enterer</b> 1431 * <p> 1432 * Description: <b>The party who is responsible for the request</b><br> 1433 * Type: <b>reference</b><br> 1434 * Path: <b>EligibilityRequest.enterer</b><br> 1435 * </p> 1436 */ 1437 @SearchParamDefinition(name="enterer", path="EligibilityRequest.enterer", description="The party who is responsible for the request", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 1438 public static final String SP_ENTERER = "enterer"; 1439 /** 1440 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 1441 * <p> 1442 * Description: <b>The party who is responsible for the request</b><br> 1443 * Type: <b>reference</b><br> 1444 * Path: <b>EligibilityRequest.enterer</b><br> 1445 * </p> 1446 */ 1447 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 1448 1449/** 1450 * Constant for fluent queries to be used to add include statements. Specifies 1451 * the path value of "<b>EligibilityRequest:enterer</b>". 1452 */ 1453 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("EligibilityRequest:enterer").toLocked(); 1454 1455 /** 1456 * Search parameter: <b>facility</b> 1457 * <p> 1458 * Description: <b>Facility responsible for the goods and services</b><br> 1459 * Type: <b>reference</b><br> 1460 * Path: <b>EligibilityRequest.facility</b><br> 1461 * </p> 1462 */ 1463 @SearchParamDefinition(name="facility", path="EligibilityRequest.facility", description="Facility responsible for the goods and services", type="reference", target={Location.class } ) 1464 public static final String SP_FACILITY = "facility"; 1465 /** 1466 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 1467 * <p> 1468 * Description: <b>Facility responsible for the goods and services</b><br> 1469 * Type: <b>reference</b><br> 1470 * Path: <b>EligibilityRequest.facility</b><br> 1471 * </p> 1472 */ 1473 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 1474 1475/** 1476 * Constant for fluent queries to be used to add include statements. Specifies 1477 * the path value of "<b>EligibilityRequest:facility</b>". 1478 */ 1479 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("EligibilityRequest:facility").toLocked(); 1480 1481 1482}