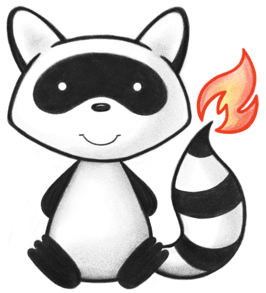
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * This resource provides eligibility and plan details from the processing of an Eligibility resource. 051 */ 052@ResourceDef(name="EligibilityResponse", profile="http://hl7.org/fhir/Profile/EligibilityResponse") 053public class EligibilityResponse extends DomainResource { 054 055 public enum EligibilityResponseStatus { 056 /** 057 * The instance is currently in-force. 058 */ 059 ACTIVE, 060 /** 061 * The instance is withdrawn, rescinded or reversed. 062 */ 063 CANCELLED, 064 /** 065 * A new instance the contents of which is not complete. 066 */ 067 DRAFT, 068 /** 069 * The instance was entered in error. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static EligibilityResponseStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("cancelled".equals(codeString)) 082 return CANCELLED; 083 if ("draft".equals(codeString)) 084 return DRAFT; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown EligibilityResponseStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: return "active"; 095 case CANCELLED: return "cancelled"; 096 case DRAFT: return "draft"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 105 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 106 case DRAFT: return "http://hl7.org/fhir/fm-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case ACTIVE: return "The instance is currently in-force."; 115 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 116 case DRAFT: return "A new instance the contents of which is not complete."; 117 case ENTEREDINERROR: return "The instance was entered in error."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case ACTIVE: return "Active"; 125 case CANCELLED: return "Cancelled"; 126 case DRAFT: return "Draft"; 127 case ENTEREDINERROR: return "Entered in Error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class EligibilityResponseStatusEnumFactory implements EnumFactory<EligibilityResponseStatus> { 135 public EligibilityResponseStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("active".equals(codeString)) 140 return EligibilityResponseStatus.ACTIVE; 141 if ("cancelled".equals(codeString)) 142 return EligibilityResponseStatus.CANCELLED; 143 if ("draft".equals(codeString)) 144 return EligibilityResponseStatus.DRAFT; 145 if ("entered-in-error".equals(codeString)) 146 return EligibilityResponseStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown EligibilityResponseStatus code '"+codeString+"'"); 148 } 149 public Enumeration<EligibilityResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<EligibilityResponseStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("active".equals(codeString)) 158 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.ACTIVE); 159 if ("cancelled".equals(codeString)) 160 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.CANCELLED); 161 if ("draft".equals(codeString)) 162 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.DRAFT); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<EligibilityResponseStatus>(this, EligibilityResponseStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown EligibilityResponseStatus code '"+codeString+"'"); 166 } 167 public String toCode(EligibilityResponseStatus code) { 168 if (code == EligibilityResponseStatus.NULL) 169 return null; 170 if (code == EligibilityResponseStatus.ACTIVE) 171 return "active"; 172 if (code == EligibilityResponseStatus.CANCELLED) 173 return "cancelled"; 174 if (code == EligibilityResponseStatus.DRAFT) 175 return "draft"; 176 if (code == EligibilityResponseStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(EligibilityResponseStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 @Block() 186 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 187 /** 188 * A suite of updated or additional Coverages from the Insurer. 189 */ 190 @Child(name = "coverage", type = {Coverage.class}, order=1, min=0, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="Updated Coverage details", formalDefinition="A suite of updated or additional Coverages from the Insurer." ) 192 protected Reference coverage; 193 194 /** 195 * The actual object that is the target of the reference (A suite of updated or additional Coverages from the Insurer.) 196 */ 197 protected Coverage coverageTarget; 198 199 /** 200 * The contract resource which may provide more detailed information. 201 */ 202 @Child(name = "contract", type = {Contract.class}, order=2, min=0, max=1, modifier=false, summary=false) 203 @Description(shortDefinition="Contract details", formalDefinition="The contract resource which may provide more detailed information." ) 204 protected Reference contract; 205 206 /** 207 * The actual object that is the target of the reference (The contract resource which may provide more detailed information.) 208 */ 209 protected Contract contractTarget; 210 211 /** 212 * Benefits and optionally current balances by Category. 213 */ 214 @Child(name = "benefitBalance", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 215 @Description(shortDefinition="Benefits by Category", formalDefinition="Benefits and optionally current balances by Category." ) 216 protected List<BenefitsComponent> benefitBalance; 217 218 private static final long serialVersionUID = 821384102L; 219 220 /** 221 * Constructor 222 */ 223 public InsuranceComponent() { 224 super(); 225 } 226 227 /** 228 * @return {@link #coverage} (A suite of updated or additional Coverages from the Insurer.) 229 */ 230 public Reference getCoverage() { 231 if (this.coverage == null) 232 if (Configuration.errorOnAutoCreate()) 233 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 234 else if (Configuration.doAutoCreate()) 235 this.coverage = new Reference(); // cc 236 return this.coverage; 237 } 238 239 public boolean hasCoverage() { 240 return this.coverage != null && !this.coverage.isEmpty(); 241 } 242 243 /** 244 * @param value {@link #coverage} (A suite of updated or additional Coverages from the Insurer.) 245 */ 246 public InsuranceComponent setCoverage(Reference value) { 247 this.coverage = value; 248 return this; 249 } 250 251 /** 252 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A suite of updated or additional Coverages from the Insurer.) 253 */ 254 public Coverage getCoverageTarget() { 255 if (this.coverageTarget == null) 256 if (Configuration.errorOnAutoCreate()) 257 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 258 else if (Configuration.doAutoCreate()) 259 this.coverageTarget = new Coverage(); // aa 260 return this.coverageTarget; 261 } 262 263 /** 264 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A suite of updated or additional Coverages from the Insurer.) 265 */ 266 public InsuranceComponent setCoverageTarget(Coverage value) { 267 this.coverageTarget = value; 268 return this; 269 } 270 271 /** 272 * @return {@link #contract} (The contract resource which may provide more detailed information.) 273 */ 274 public Reference getContract() { 275 if (this.contract == null) 276 if (Configuration.errorOnAutoCreate()) 277 throw new Error("Attempt to auto-create InsuranceComponent.contract"); 278 else if (Configuration.doAutoCreate()) 279 this.contract = new Reference(); // cc 280 return this.contract; 281 } 282 283 public boolean hasContract() { 284 return this.contract != null && !this.contract.isEmpty(); 285 } 286 287 /** 288 * @param value {@link #contract} (The contract resource which may provide more detailed information.) 289 */ 290 public InsuranceComponent setContract(Reference value) { 291 this.contract = value; 292 return this; 293 } 294 295 /** 296 * @return {@link #contract} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The contract resource which may provide more detailed information.) 297 */ 298 public Contract getContractTarget() { 299 if (this.contractTarget == null) 300 if (Configuration.errorOnAutoCreate()) 301 throw new Error("Attempt to auto-create InsuranceComponent.contract"); 302 else if (Configuration.doAutoCreate()) 303 this.contractTarget = new Contract(); // aa 304 return this.contractTarget; 305 } 306 307 /** 308 * @param value {@link #contract} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The contract resource which may provide more detailed information.) 309 */ 310 public InsuranceComponent setContractTarget(Contract value) { 311 this.contractTarget = value; 312 return this; 313 } 314 315 /** 316 * @return {@link #benefitBalance} (Benefits and optionally current balances by Category.) 317 */ 318 public List<BenefitsComponent> getBenefitBalance() { 319 if (this.benefitBalance == null) 320 this.benefitBalance = new ArrayList<BenefitsComponent>(); 321 return this.benefitBalance; 322 } 323 324 /** 325 * @return Returns a reference to <code>this</code> for easy method chaining 326 */ 327 public InsuranceComponent setBenefitBalance(List<BenefitsComponent> theBenefitBalance) { 328 this.benefitBalance = theBenefitBalance; 329 return this; 330 } 331 332 public boolean hasBenefitBalance() { 333 if (this.benefitBalance == null) 334 return false; 335 for (BenefitsComponent item : this.benefitBalance) 336 if (!item.isEmpty()) 337 return true; 338 return false; 339 } 340 341 public BenefitsComponent addBenefitBalance() { //3 342 BenefitsComponent t = new BenefitsComponent(); 343 if (this.benefitBalance == null) 344 this.benefitBalance = new ArrayList<BenefitsComponent>(); 345 this.benefitBalance.add(t); 346 return t; 347 } 348 349 public InsuranceComponent addBenefitBalance(BenefitsComponent t) { //3 350 if (t == null) 351 return this; 352 if (this.benefitBalance == null) 353 this.benefitBalance = new ArrayList<BenefitsComponent>(); 354 this.benefitBalance.add(t); 355 return this; 356 } 357 358 /** 359 * @return The first repetition of repeating field {@link #benefitBalance}, creating it if it does not already exist 360 */ 361 public BenefitsComponent getBenefitBalanceFirstRep() { 362 if (getBenefitBalance().isEmpty()) { 363 addBenefitBalance(); 364 } 365 return getBenefitBalance().get(0); 366 } 367 368 protected void listChildren(List<Property> children) { 369 super.listChildren(children); 370 children.add(new Property("coverage", "Reference(Coverage)", "A suite of updated or additional Coverages from the Insurer.", 0, 1, coverage)); 371 children.add(new Property("contract", "Reference(Contract)", "The contract resource which may provide more detailed information.", 0, 1, contract)); 372 children.add(new Property("benefitBalance", "", "Benefits and optionally current balances by Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance)); 373 } 374 375 @Override 376 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 377 switch (_hash) { 378 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "A suite of updated or additional Coverages from the Insurer.", 0, 1, coverage); 379 case -566947566: /*contract*/ return new Property("contract", "Reference(Contract)", "The contract resource which may provide more detailed information.", 0, 1, contract); 380 case 596003397: /*benefitBalance*/ return new Property("benefitBalance", "", "Benefits and optionally current balances by Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance); 381 default: return super.getNamedProperty(_hash, _name, _checkValid); 382 } 383 384 } 385 386 @Override 387 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 388 switch (hash) { 389 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 390 case -566947566: /*contract*/ return this.contract == null ? new Base[0] : new Base[] {this.contract}; // Reference 391 case 596003397: /*benefitBalance*/ return this.benefitBalance == null ? new Base[0] : this.benefitBalance.toArray(new Base[this.benefitBalance.size()]); // BenefitsComponent 392 default: return super.getProperty(hash, name, checkValid); 393 } 394 395 } 396 397 @Override 398 public Base setProperty(int hash, String name, Base value) throws FHIRException { 399 switch (hash) { 400 case -351767064: // coverage 401 this.coverage = castToReference(value); // Reference 402 return value; 403 case -566947566: // contract 404 this.contract = castToReference(value); // Reference 405 return value; 406 case 596003397: // benefitBalance 407 this.getBenefitBalance().add((BenefitsComponent) value); // BenefitsComponent 408 return value; 409 default: return super.setProperty(hash, name, value); 410 } 411 412 } 413 414 @Override 415 public Base setProperty(String name, Base value) throws FHIRException { 416 if (name.equals("coverage")) { 417 this.coverage = castToReference(value); // Reference 418 } else if (name.equals("contract")) { 419 this.contract = castToReference(value); // Reference 420 } else if (name.equals("benefitBalance")) { 421 this.getBenefitBalance().add((BenefitsComponent) value); 422 } else 423 return super.setProperty(name, value); 424 return value; 425 } 426 427 @Override 428 public Base makeProperty(int hash, String name) throws FHIRException { 429 switch (hash) { 430 case -351767064: return getCoverage(); 431 case -566947566: return getContract(); 432 case 596003397: return addBenefitBalance(); 433 default: return super.makeProperty(hash, name); 434 } 435 436 } 437 438 @Override 439 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 440 switch (hash) { 441 case -351767064: /*coverage*/ return new String[] {"Reference"}; 442 case -566947566: /*contract*/ return new String[] {"Reference"}; 443 case 596003397: /*benefitBalance*/ return new String[] {}; 444 default: return super.getTypesForProperty(hash, name); 445 } 446 447 } 448 449 @Override 450 public Base addChild(String name) throws FHIRException { 451 if (name.equals("coverage")) { 452 this.coverage = new Reference(); 453 return this.coverage; 454 } 455 else if (name.equals("contract")) { 456 this.contract = new Reference(); 457 return this.contract; 458 } 459 else if (name.equals("benefitBalance")) { 460 return addBenefitBalance(); 461 } 462 else 463 return super.addChild(name); 464 } 465 466 public InsuranceComponent copy() { 467 InsuranceComponent dst = new InsuranceComponent(); 468 copyValues(dst); 469 dst.coverage = coverage == null ? null : coverage.copy(); 470 dst.contract = contract == null ? null : contract.copy(); 471 if (benefitBalance != null) { 472 dst.benefitBalance = new ArrayList<BenefitsComponent>(); 473 for (BenefitsComponent i : benefitBalance) 474 dst.benefitBalance.add(i.copy()); 475 }; 476 return dst; 477 } 478 479 @Override 480 public boolean equalsDeep(Base other_) { 481 if (!super.equalsDeep(other_)) 482 return false; 483 if (!(other_ instanceof InsuranceComponent)) 484 return false; 485 InsuranceComponent o = (InsuranceComponent) other_; 486 return compareDeep(coverage, o.coverage, true) && compareDeep(contract, o.contract, true) && compareDeep(benefitBalance, o.benefitBalance, true) 487 ; 488 } 489 490 @Override 491 public boolean equalsShallow(Base other_) { 492 if (!super.equalsShallow(other_)) 493 return false; 494 if (!(other_ instanceof InsuranceComponent)) 495 return false; 496 InsuranceComponent o = (InsuranceComponent) other_; 497 return true; 498 } 499 500 public boolean isEmpty() { 501 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, contract, benefitBalance 502 ); 503 } 504 505 public String fhirType() { 506 return "EligibilityResponse.insurance"; 507 508 } 509 510 } 511 512 @Block() 513 public static class BenefitsComponent extends BackboneElement implements IBaseBackboneElement { 514 /** 515 * Dental, Vision, Medical, Pharmacy, Rehab etc. 516 */ 517 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 518 @Description(shortDefinition="Type of services covered", formalDefinition="Dental, Vision, Medical, Pharmacy, Rehab etc." ) 519 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-category") 520 protected CodeableConcept category; 521 522 /** 523 * Dental: basic, major, ortho; Vision exam, glasses, contacts; etc. 524 */ 525 @Child(name = "subCategory", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 526 @Description(shortDefinition="Detailed services covered within the type", formalDefinition="Dental: basic, major, ortho; Vision exam, glasses, contacts; etc." ) 527 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 528 protected CodeableConcept subCategory; 529 530 /** 531 * True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 532 */ 533 @Child(name = "excluded", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 534 @Description(shortDefinition="Excluded from the plan", formalDefinition="True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage." ) 535 protected BooleanType excluded; 536 537 /** 538 * A short name or tag for the benefit, for example MED01, or DENT2. 539 */ 540 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 541 @Description(shortDefinition="Short name for the benefit", formalDefinition="A short name or tag for the benefit, for example MED01, or DENT2." ) 542 protected StringType name; 543 544 /** 545 * A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 546 */ 547 @Child(name = "description", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 548 @Description(shortDefinition="Description of the benefit or services covered", formalDefinition="A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'." ) 549 protected StringType description; 550 551 /** 552 * Network designation. 553 */ 554 @Child(name = "network", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 555 @Description(shortDefinition="In or out of network", formalDefinition="Network designation." ) 556 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-network") 557 protected CodeableConcept network; 558 559 /** 560 * Unit designation: individual or family. 561 */ 562 @Child(name = "unit", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 563 @Description(shortDefinition="Individual or family", formalDefinition="Unit designation: individual or family." ) 564 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-unit") 565 protected CodeableConcept unit; 566 567 /** 568 * The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'. 569 */ 570 @Child(name = "term", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 571 @Description(shortDefinition="Annual or lifetime", formalDefinition="The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'." ) 572 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-term") 573 protected CodeableConcept term; 574 575 /** 576 * Benefits Used to date. 577 */ 578 @Child(name = "financial", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 579 @Description(shortDefinition="Benefit Summary", formalDefinition="Benefits Used to date." ) 580 protected List<BenefitComponent> financial; 581 582 private static final long serialVersionUID = 833826021L; 583 584 /** 585 * Constructor 586 */ 587 public BenefitsComponent() { 588 super(); 589 } 590 591 /** 592 * Constructor 593 */ 594 public BenefitsComponent(CodeableConcept category) { 595 super(); 596 this.category = category; 597 } 598 599 /** 600 * @return {@link #category} (Dental, Vision, Medical, Pharmacy, Rehab etc.) 601 */ 602 public CodeableConcept getCategory() { 603 if (this.category == null) 604 if (Configuration.errorOnAutoCreate()) 605 throw new Error("Attempt to auto-create BenefitsComponent.category"); 606 else if (Configuration.doAutoCreate()) 607 this.category = new CodeableConcept(); // cc 608 return this.category; 609 } 610 611 public boolean hasCategory() { 612 return this.category != null && !this.category.isEmpty(); 613 } 614 615 /** 616 * @param value {@link #category} (Dental, Vision, Medical, Pharmacy, Rehab etc.) 617 */ 618 public BenefitsComponent setCategory(CodeableConcept value) { 619 this.category = value; 620 return this; 621 } 622 623 /** 624 * @return {@link #subCategory} (Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.) 625 */ 626 public CodeableConcept getSubCategory() { 627 if (this.subCategory == null) 628 if (Configuration.errorOnAutoCreate()) 629 throw new Error("Attempt to auto-create BenefitsComponent.subCategory"); 630 else if (Configuration.doAutoCreate()) 631 this.subCategory = new CodeableConcept(); // cc 632 return this.subCategory; 633 } 634 635 public boolean hasSubCategory() { 636 return this.subCategory != null && !this.subCategory.isEmpty(); 637 } 638 639 /** 640 * @param value {@link #subCategory} (Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.) 641 */ 642 public BenefitsComponent setSubCategory(CodeableConcept value) { 643 this.subCategory = value; 644 return this; 645 } 646 647 /** 648 * @return {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 649 */ 650 public BooleanType getExcludedElement() { 651 if (this.excluded == null) 652 if (Configuration.errorOnAutoCreate()) 653 throw new Error("Attempt to auto-create BenefitsComponent.excluded"); 654 else if (Configuration.doAutoCreate()) 655 this.excluded = new BooleanType(); // bb 656 return this.excluded; 657 } 658 659 public boolean hasExcludedElement() { 660 return this.excluded != null && !this.excluded.isEmpty(); 661 } 662 663 public boolean hasExcluded() { 664 return this.excluded != null && !this.excluded.isEmpty(); 665 } 666 667 /** 668 * @param value {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 669 */ 670 public BenefitsComponent setExcludedElement(BooleanType value) { 671 this.excluded = value; 672 return this; 673 } 674 675 /** 676 * @return True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 677 */ 678 public boolean getExcluded() { 679 return this.excluded == null || this.excluded.isEmpty() ? false : this.excluded.getValue(); 680 } 681 682 /** 683 * @param value True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 684 */ 685 public BenefitsComponent setExcluded(boolean value) { 686 if (this.excluded == null) 687 this.excluded = new BooleanType(); 688 this.excluded.setValue(value); 689 return this; 690 } 691 692 /** 693 * @return {@link #name} (A short name or tag for the benefit, for example MED01, or DENT2.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 694 */ 695 public StringType getNameElement() { 696 if (this.name == null) 697 if (Configuration.errorOnAutoCreate()) 698 throw new Error("Attempt to auto-create BenefitsComponent.name"); 699 else if (Configuration.doAutoCreate()) 700 this.name = new StringType(); // bb 701 return this.name; 702 } 703 704 public boolean hasNameElement() { 705 return this.name != null && !this.name.isEmpty(); 706 } 707 708 public boolean hasName() { 709 return this.name != null && !this.name.isEmpty(); 710 } 711 712 /** 713 * @param value {@link #name} (A short name or tag for the benefit, for example MED01, or DENT2.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 714 */ 715 public BenefitsComponent setNameElement(StringType value) { 716 this.name = value; 717 return this; 718 } 719 720 /** 721 * @return A short name or tag for the benefit, for example MED01, or DENT2. 722 */ 723 public String getName() { 724 return this.name == null ? null : this.name.getValue(); 725 } 726 727 /** 728 * @param value A short name or tag for the benefit, for example MED01, or DENT2. 729 */ 730 public BenefitsComponent setName(String value) { 731 if (Utilities.noString(value)) 732 this.name = null; 733 else { 734 if (this.name == null) 735 this.name = new StringType(); 736 this.name.setValue(value); 737 } 738 return this; 739 } 740 741 /** 742 * @return {@link #description} (A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 743 */ 744 public StringType getDescriptionElement() { 745 if (this.description == null) 746 if (Configuration.errorOnAutoCreate()) 747 throw new Error("Attempt to auto-create BenefitsComponent.description"); 748 else if (Configuration.doAutoCreate()) 749 this.description = new StringType(); // bb 750 return this.description; 751 } 752 753 public boolean hasDescriptionElement() { 754 return this.description != null && !this.description.isEmpty(); 755 } 756 757 public boolean hasDescription() { 758 return this.description != null && !this.description.isEmpty(); 759 } 760 761 /** 762 * @param value {@link #description} (A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 763 */ 764 public BenefitsComponent setDescriptionElement(StringType value) { 765 this.description = value; 766 return this; 767 } 768 769 /** 770 * @return A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 771 */ 772 public String getDescription() { 773 return this.description == null ? null : this.description.getValue(); 774 } 775 776 /** 777 * @param value A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 778 */ 779 public BenefitsComponent setDescription(String value) { 780 if (Utilities.noString(value)) 781 this.description = null; 782 else { 783 if (this.description == null) 784 this.description = new StringType(); 785 this.description.setValue(value); 786 } 787 return this; 788 } 789 790 /** 791 * @return {@link #network} (Network designation.) 792 */ 793 public CodeableConcept getNetwork() { 794 if (this.network == null) 795 if (Configuration.errorOnAutoCreate()) 796 throw new Error("Attempt to auto-create BenefitsComponent.network"); 797 else if (Configuration.doAutoCreate()) 798 this.network = new CodeableConcept(); // cc 799 return this.network; 800 } 801 802 public boolean hasNetwork() { 803 return this.network != null && !this.network.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #network} (Network designation.) 808 */ 809 public BenefitsComponent setNetwork(CodeableConcept value) { 810 this.network = value; 811 return this; 812 } 813 814 /** 815 * @return {@link #unit} (Unit designation: individual or family.) 816 */ 817 public CodeableConcept getUnit() { 818 if (this.unit == null) 819 if (Configuration.errorOnAutoCreate()) 820 throw new Error("Attempt to auto-create BenefitsComponent.unit"); 821 else if (Configuration.doAutoCreate()) 822 this.unit = new CodeableConcept(); // cc 823 return this.unit; 824 } 825 826 public boolean hasUnit() { 827 return this.unit != null && !this.unit.isEmpty(); 828 } 829 830 /** 831 * @param value {@link #unit} (Unit designation: individual or family.) 832 */ 833 public BenefitsComponent setUnit(CodeableConcept value) { 834 this.unit = value; 835 return this; 836 } 837 838 /** 839 * @return {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.) 840 */ 841 public CodeableConcept getTerm() { 842 if (this.term == null) 843 if (Configuration.errorOnAutoCreate()) 844 throw new Error("Attempt to auto-create BenefitsComponent.term"); 845 else if (Configuration.doAutoCreate()) 846 this.term = new CodeableConcept(); // cc 847 return this.term; 848 } 849 850 public boolean hasTerm() { 851 return this.term != null && !this.term.isEmpty(); 852 } 853 854 /** 855 * @param value {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.) 856 */ 857 public BenefitsComponent setTerm(CodeableConcept value) { 858 this.term = value; 859 return this; 860 } 861 862 /** 863 * @return {@link #financial} (Benefits Used to date.) 864 */ 865 public List<BenefitComponent> getFinancial() { 866 if (this.financial == null) 867 this.financial = new ArrayList<BenefitComponent>(); 868 return this.financial; 869 } 870 871 /** 872 * @return Returns a reference to <code>this</code> for easy method chaining 873 */ 874 public BenefitsComponent setFinancial(List<BenefitComponent> theFinancial) { 875 this.financial = theFinancial; 876 return this; 877 } 878 879 public boolean hasFinancial() { 880 if (this.financial == null) 881 return false; 882 for (BenefitComponent item : this.financial) 883 if (!item.isEmpty()) 884 return true; 885 return false; 886 } 887 888 public BenefitComponent addFinancial() { //3 889 BenefitComponent t = new BenefitComponent(); 890 if (this.financial == null) 891 this.financial = new ArrayList<BenefitComponent>(); 892 this.financial.add(t); 893 return t; 894 } 895 896 public BenefitsComponent addFinancial(BenefitComponent t) { //3 897 if (t == null) 898 return this; 899 if (this.financial == null) 900 this.financial = new ArrayList<BenefitComponent>(); 901 this.financial.add(t); 902 return this; 903 } 904 905 /** 906 * @return The first repetition of repeating field {@link #financial}, creating it if it does not already exist 907 */ 908 public BenefitComponent getFinancialFirstRep() { 909 if (getFinancial().isEmpty()) { 910 addFinancial(); 911 } 912 return getFinancial().get(0); 913 } 914 915 protected void listChildren(List<Property> children) { 916 super.listChildren(children); 917 children.add(new Property("category", "CodeableConcept", "Dental, Vision, Medical, Pharmacy, Rehab etc.", 0, 1, category)); 918 children.add(new Property("subCategory", "CodeableConcept", "Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.", 0, 1, subCategory)); 919 children.add(new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.", 0, 1, excluded)); 920 children.add(new Property("name", "string", "A short name or tag for the benefit, for example MED01, or DENT2.", 0, 1, name)); 921 children.add(new Property("description", "string", "A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.", 0, 1, description)); 922 children.add(new Property("network", "CodeableConcept", "Network designation.", 0, 1, network)); 923 children.add(new Property("unit", "CodeableConcept", "Unit designation: individual or family.", 0, 1, unit)); 924 children.add(new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.", 0, 1, term)); 925 children.add(new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial)); 926 } 927 928 @Override 929 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 930 switch (_hash) { 931 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Dental, Vision, Medical, Pharmacy, Rehab etc.", 0, 1, category); 932 case 1365024606: /*subCategory*/ return new Property("subCategory", "CodeableConcept", "Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.", 0, 1, subCategory); 933 case 1994055114: /*excluded*/ return new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.", 0, 1, excluded); 934 case 3373707: /*name*/ return new Property("name", "string", "A short name or tag for the benefit, for example MED01, or DENT2.", 0, 1, name); 935 case -1724546052: /*description*/ return new Property("description", "string", "A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.", 0, 1, description); 936 case 1843485230: /*network*/ return new Property("network", "CodeableConcept", "Network designation.", 0, 1, network); 937 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Unit designation: individual or family.", 0, 1, unit); 938 case 3556460: /*term*/ return new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.", 0, 1, term); 939 case 357555337: /*financial*/ return new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial); 940 default: return super.getNamedProperty(_hash, _name, _checkValid); 941 } 942 943 } 944 945 @Override 946 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 947 switch (hash) { 948 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 949 case 1365024606: /*subCategory*/ return this.subCategory == null ? new Base[0] : new Base[] {this.subCategory}; // CodeableConcept 950 case 1994055114: /*excluded*/ return this.excluded == null ? new Base[0] : new Base[] {this.excluded}; // BooleanType 951 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 952 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 953 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // CodeableConcept 954 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 955 case 3556460: /*term*/ return this.term == null ? new Base[0] : new Base[] {this.term}; // CodeableConcept 956 case 357555337: /*financial*/ return this.financial == null ? new Base[0] : this.financial.toArray(new Base[this.financial.size()]); // BenefitComponent 957 default: return super.getProperty(hash, name, checkValid); 958 } 959 960 } 961 962 @Override 963 public Base setProperty(int hash, String name, Base value) throws FHIRException { 964 switch (hash) { 965 case 50511102: // category 966 this.category = castToCodeableConcept(value); // CodeableConcept 967 return value; 968 case 1365024606: // subCategory 969 this.subCategory = castToCodeableConcept(value); // CodeableConcept 970 return value; 971 case 1994055114: // excluded 972 this.excluded = castToBoolean(value); // BooleanType 973 return value; 974 case 3373707: // name 975 this.name = castToString(value); // StringType 976 return value; 977 case -1724546052: // description 978 this.description = castToString(value); // StringType 979 return value; 980 case 1843485230: // network 981 this.network = castToCodeableConcept(value); // CodeableConcept 982 return value; 983 case 3594628: // unit 984 this.unit = castToCodeableConcept(value); // CodeableConcept 985 return value; 986 case 3556460: // term 987 this.term = castToCodeableConcept(value); // CodeableConcept 988 return value; 989 case 357555337: // financial 990 this.getFinancial().add((BenefitComponent) value); // BenefitComponent 991 return value; 992 default: return super.setProperty(hash, name, value); 993 } 994 995 } 996 997 @Override 998 public Base setProperty(String name, Base value) throws FHIRException { 999 if (name.equals("category")) { 1000 this.category = castToCodeableConcept(value); // CodeableConcept 1001 } else if (name.equals("subCategory")) { 1002 this.subCategory = castToCodeableConcept(value); // CodeableConcept 1003 } else if (name.equals("excluded")) { 1004 this.excluded = castToBoolean(value); // BooleanType 1005 } else if (name.equals("name")) { 1006 this.name = castToString(value); // StringType 1007 } else if (name.equals("description")) { 1008 this.description = castToString(value); // StringType 1009 } else if (name.equals("network")) { 1010 this.network = castToCodeableConcept(value); // CodeableConcept 1011 } else if (name.equals("unit")) { 1012 this.unit = castToCodeableConcept(value); // CodeableConcept 1013 } else if (name.equals("term")) { 1014 this.term = castToCodeableConcept(value); // CodeableConcept 1015 } else if (name.equals("financial")) { 1016 this.getFinancial().add((BenefitComponent) value); 1017 } else 1018 return super.setProperty(name, value); 1019 return value; 1020 } 1021 1022 @Override 1023 public Base makeProperty(int hash, String name) throws FHIRException { 1024 switch (hash) { 1025 case 50511102: return getCategory(); 1026 case 1365024606: return getSubCategory(); 1027 case 1994055114: return getExcludedElement(); 1028 case 3373707: return getNameElement(); 1029 case -1724546052: return getDescriptionElement(); 1030 case 1843485230: return getNetwork(); 1031 case 3594628: return getUnit(); 1032 case 3556460: return getTerm(); 1033 case 357555337: return addFinancial(); 1034 default: return super.makeProperty(hash, name); 1035 } 1036 1037 } 1038 1039 @Override 1040 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1041 switch (hash) { 1042 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1043 case 1365024606: /*subCategory*/ return new String[] {"CodeableConcept"}; 1044 case 1994055114: /*excluded*/ return new String[] {"boolean"}; 1045 case 3373707: /*name*/ return new String[] {"string"}; 1046 case -1724546052: /*description*/ return new String[] {"string"}; 1047 case 1843485230: /*network*/ return new String[] {"CodeableConcept"}; 1048 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 1049 case 3556460: /*term*/ return new String[] {"CodeableConcept"}; 1050 case 357555337: /*financial*/ return new String[] {}; 1051 default: return super.getTypesForProperty(hash, name); 1052 } 1053 1054 } 1055 1056 @Override 1057 public Base addChild(String name) throws FHIRException { 1058 if (name.equals("category")) { 1059 this.category = new CodeableConcept(); 1060 return this.category; 1061 } 1062 else if (name.equals("subCategory")) { 1063 this.subCategory = new CodeableConcept(); 1064 return this.subCategory; 1065 } 1066 else if (name.equals("excluded")) { 1067 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.excluded"); 1068 } 1069 else if (name.equals("name")) { 1070 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.name"); 1071 } 1072 else if (name.equals("description")) { 1073 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.description"); 1074 } 1075 else if (name.equals("network")) { 1076 this.network = new CodeableConcept(); 1077 return this.network; 1078 } 1079 else if (name.equals("unit")) { 1080 this.unit = new CodeableConcept(); 1081 return this.unit; 1082 } 1083 else if (name.equals("term")) { 1084 this.term = new CodeableConcept(); 1085 return this.term; 1086 } 1087 else if (name.equals("financial")) { 1088 return addFinancial(); 1089 } 1090 else 1091 return super.addChild(name); 1092 } 1093 1094 public BenefitsComponent copy() { 1095 BenefitsComponent dst = new BenefitsComponent(); 1096 copyValues(dst); 1097 dst.category = category == null ? null : category.copy(); 1098 dst.subCategory = subCategory == null ? null : subCategory.copy(); 1099 dst.excluded = excluded == null ? null : excluded.copy(); 1100 dst.name = name == null ? null : name.copy(); 1101 dst.description = description == null ? null : description.copy(); 1102 dst.network = network == null ? null : network.copy(); 1103 dst.unit = unit == null ? null : unit.copy(); 1104 dst.term = term == null ? null : term.copy(); 1105 if (financial != null) { 1106 dst.financial = new ArrayList<BenefitComponent>(); 1107 for (BenefitComponent i : financial) 1108 dst.financial.add(i.copy()); 1109 }; 1110 return dst; 1111 } 1112 1113 @Override 1114 public boolean equalsDeep(Base other_) { 1115 if (!super.equalsDeep(other_)) 1116 return false; 1117 if (!(other_ instanceof BenefitsComponent)) 1118 return false; 1119 BenefitsComponent o = (BenefitsComponent) other_; 1120 return compareDeep(category, o.category, true) && compareDeep(subCategory, o.subCategory, true) 1121 && compareDeep(excluded, o.excluded, true) && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 1122 && compareDeep(network, o.network, true) && compareDeep(unit, o.unit, true) && compareDeep(term, o.term, true) 1123 && compareDeep(financial, o.financial, true); 1124 } 1125 1126 @Override 1127 public boolean equalsShallow(Base other_) { 1128 if (!super.equalsShallow(other_)) 1129 return false; 1130 if (!(other_ instanceof BenefitsComponent)) 1131 return false; 1132 BenefitsComponent o = (BenefitsComponent) other_; 1133 return compareValues(excluded, o.excluded, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 1134 ; 1135 } 1136 1137 public boolean isEmpty() { 1138 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, subCategory, excluded 1139 , name, description, network, unit, term, financial); 1140 } 1141 1142 public String fhirType() { 1143 return "EligibilityResponse.insurance.benefitBalance"; 1144 1145 } 1146 1147 } 1148 1149 @Block() 1150 public static class BenefitComponent extends BackboneElement implements IBaseBackboneElement { 1151 /** 1152 * Deductable, visits, benefit amount. 1153 */ 1154 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1155 @Description(shortDefinition="Deductable, visits, benefit amount", formalDefinition="Deductable, visits, benefit amount." ) 1156 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-type") 1157 protected CodeableConcept type; 1158 1159 /** 1160 * Benefits allowed. 1161 */ 1162 @Child(name = "allowed", type = {UnsignedIntType.class, StringType.class, Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 1163 @Description(shortDefinition="Benefits allowed", formalDefinition="Benefits allowed." ) 1164 protected Type allowed; 1165 1166 /** 1167 * Benefits used. 1168 */ 1169 @Child(name = "used", type = {UnsignedIntType.class, Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 1170 @Description(shortDefinition="Benefits used", formalDefinition="Benefits used." ) 1171 protected Type used; 1172 1173 private static final long serialVersionUID = -1506285314L; 1174 1175 /** 1176 * Constructor 1177 */ 1178 public BenefitComponent() { 1179 super(); 1180 } 1181 1182 /** 1183 * Constructor 1184 */ 1185 public BenefitComponent(CodeableConcept type) { 1186 super(); 1187 this.type = type; 1188 } 1189 1190 /** 1191 * @return {@link #type} (Deductable, visits, benefit amount.) 1192 */ 1193 public CodeableConcept getType() { 1194 if (this.type == null) 1195 if (Configuration.errorOnAutoCreate()) 1196 throw new Error("Attempt to auto-create BenefitComponent.type"); 1197 else if (Configuration.doAutoCreate()) 1198 this.type = new CodeableConcept(); // cc 1199 return this.type; 1200 } 1201 1202 public boolean hasType() { 1203 return this.type != null && !this.type.isEmpty(); 1204 } 1205 1206 /** 1207 * @param value {@link #type} (Deductable, visits, benefit amount.) 1208 */ 1209 public BenefitComponent setType(CodeableConcept value) { 1210 this.type = value; 1211 return this; 1212 } 1213 1214 /** 1215 * @return {@link #allowed} (Benefits allowed.) 1216 */ 1217 public Type getAllowed() { 1218 return this.allowed; 1219 } 1220 1221 /** 1222 * @return {@link #allowed} (Benefits allowed.) 1223 */ 1224 public UnsignedIntType getAllowedUnsignedIntType() throws FHIRException { 1225 if (this.allowed == null) 1226 return null; 1227 if (!(this.allowed instanceof UnsignedIntType)) 1228 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 1229 return (UnsignedIntType) this.allowed; 1230 } 1231 1232 public boolean hasAllowedUnsignedIntType() { 1233 return this != null && this.allowed instanceof UnsignedIntType; 1234 } 1235 1236 /** 1237 * @return {@link #allowed} (Benefits allowed.) 1238 */ 1239 public StringType getAllowedStringType() throws FHIRException { 1240 if (this.allowed == null) 1241 return null; 1242 if (!(this.allowed instanceof StringType)) 1243 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 1244 return (StringType) this.allowed; 1245 } 1246 1247 public boolean hasAllowedStringType() { 1248 return this != null && this.allowed instanceof StringType; 1249 } 1250 1251 /** 1252 * @return {@link #allowed} (Benefits allowed.) 1253 */ 1254 public Money getAllowedMoney() throws FHIRException { 1255 if (this.allowed == null) 1256 return null; 1257 if (!(this.allowed instanceof Money)) 1258 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.allowed.getClass().getName()+" was encountered"); 1259 return (Money) this.allowed; 1260 } 1261 1262 public boolean hasAllowedMoney() { 1263 return this != null && this.allowed instanceof Money; 1264 } 1265 1266 public boolean hasAllowed() { 1267 return this.allowed != null && !this.allowed.isEmpty(); 1268 } 1269 1270 /** 1271 * @param value {@link #allowed} (Benefits allowed.) 1272 */ 1273 public BenefitComponent setAllowed(Type value) throws FHIRFormatError { 1274 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 1275 throw new FHIRFormatError("Not the right type for EligibilityResponse.insurance.benefitBalance.financial.allowed[x]: "+value.fhirType()); 1276 this.allowed = value; 1277 return this; 1278 } 1279 1280 /** 1281 * @return {@link #used} (Benefits used.) 1282 */ 1283 public Type getUsed() { 1284 return this.used; 1285 } 1286 1287 /** 1288 * @return {@link #used} (Benefits used.) 1289 */ 1290 public UnsignedIntType getUsedUnsignedIntType() throws FHIRException { 1291 if (this.used == null) 1292 return null; 1293 if (!(this.used instanceof UnsignedIntType)) 1294 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.used.getClass().getName()+" was encountered"); 1295 return (UnsignedIntType) this.used; 1296 } 1297 1298 public boolean hasUsedUnsignedIntType() { 1299 return this != null && this.used instanceof UnsignedIntType; 1300 } 1301 1302 /** 1303 * @return {@link #used} (Benefits used.) 1304 */ 1305 public Money getUsedMoney() throws FHIRException { 1306 if (this.used == null) 1307 return null; 1308 if (!(this.used instanceof Money)) 1309 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.used.getClass().getName()+" was encountered"); 1310 return (Money) this.used; 1311 } 1312 1313 public boolean hasUsedMoney() { 1314 return this != null && this.used instanceof Money; 1315 } 1316 1317 public boolean hasUsed() { 1318 return this.used != null && !this.used.isEmpty(); 1319 } 1320 1321 /** 1322 * @param value {@link #used} (Benefits used.) 1323 */ 1324 public BenefitComponent setUsed(Type value) throws FHIRFormatError { 1325 if (value != null && !(value instanceof UnsignedIntType || value instanceof Money)) 1326 throw new FHIRFormatError("Not the right type for EligibilityResponse.insurance.benefitBalance.financial.used[x]: "+value.fhirType()); 1327 this.used = value; 1328 return this; 1329 } 1330 1331 protected void listChildren(List<Property> children) { 1332 super.listChildren(children); 1333 children.add(new Property("type", "CodeableConcept", "Deductable, visits, benefit amount.", 0, 1, type)); 1334 children.add(new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed)); 1335 children.add(new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used)); 1336 } 1337 1338 @Override 1339 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1340 switch (_hash) { 1341 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Deductable, visits, benefit amount.", 0, 1, type); 1342 case -1336663592: /*allowed[x]*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 1343 case -911343192: /*allowed*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 1344 case 1668802034: /*allowedUnsignedInt*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 1345 case -2135265319: /*allowedString*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 1346 case -351668232: /*allowedMoney*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 1347 case -147553373: /*used[x]*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 1348 case 3599293: /*used*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 1349 case 1252740285: /*usedUnsignedInt*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 1350 case -78048509: /*usedMoney*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 1351 default: return super.getNamedProperty(_hash, _name, _checkValid); 1352 } 1353 1354 } 1355 1356 @Override 1357 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1358 switch (hash) { 1359 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1360 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // Type 1361 case 3599293: /*used*/ return this.used == null ? new Base[0] : new Base[] {this.used}; // Type 1362 default: return super.getProperty(hash, name, checkValid); 1363 } 1364 1365 } 1366 1367 @Override 1368 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1369 switch (hash) { 1370 case 3575610: // type 1371 this.type = castToCodeableConcept(value); // CodeableConcept 1372 return value; 1373 case -911343192: // allowed 1374 this.allowed = castToType(value); // Type 1375 return value; 1376 case 3599293: // used 1377 this.used = castToType(value); // Type 1378 return value; 1379 default: return super.setProperty(hash, name, value); 1380 } 1381 1382 } 1383 1384 @Override 1385 public Base setProperty(String name, Base value) throws FHIRException { 1386 if (name.equals("type")) { 1387 this.type = castToCodeableConcept(value); // CodeableConcept 1388 } else if (name.equals("allowed[x]")) { 1389 this.allowed = castToType(value); // Type 1390 } else if (name.equals("used[x]")) { 1391 this.used = castToType(value); // Type 1392 } else 1393 return super.setProperty(name, value); 1394 return value; 1395 } 1396 1397 @Override 1398 public Base makeProperty(int hash, String name) throws FHIRException { 1399 switch (hash) { 1400 case 3575610: return getType(); 1401 case -1336663592: return getAllowed(); 1402 case -911343192: return getAllowed(); 1403 case -147553373: return getUsed(); 1404 case 3599293: return getUsed(); 1405 default: return super.makeProperty(hash, name); 1406 } 1407 1408 } 1409 1410 @Override 1411 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1412 switch (hash) { 1413 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1414 case -911343192: /*allowed*/ return new String[] {"unsignedInt", "string", "Money"}; 1415 case 3599293: /*used*/ return new String[] {"unsignedInt", "Money"}; 1416 default: return super.getTypesForProperty(hash, name); 1417 } 1418 1419 } 1420 1421 @Override 1422 public Base addChild(String name) throws FHIRException { 1423 if (name.equals("type")) { 1424 this.type = new CodeableConcept(); 1425 return this.type; 1426 } 1427 else if (name.equals("allowedUnsignedInt")) { 1428 this.allowed = new UnsignedIntType(); 1429 return this.allowed; 1430 } 1431 else if (name.equals("allowedString")) { 1432 this.allowed = new StringType(); 1433 return this.allowed; 1434 } 1435 else if (name.equals("allowedMoney")) { 1436 this.allowed = new Money(); 1437 return this.allowed; 1438 } 1439 else if (name.equals("usedUnsignedInt")) { 1440 this.used = new UnsignedIntType(); 1441 return this.used; 1442 } 1443 else if (name.equals("usedMoney")) { 1444 this.used = new Money(); 1445 return this.used; 1446 } 1447 else 1448 return super.addChild(name); 1449 } 1450 1451 public BenefitComponent copy() { 1452 BenefitComponent dst = new BenefitComponent(); 1453 copyValues(dst); 1454 dst.type = type == null ? null : type.copy(); 1455 dst.allowed = allowed == null ? null : allowed.copy(); 1456 dst.used = used == null ? null : used.copy(); 1457 return dst; 1458 } 1459 1460 @Override 1461 public boolean equalsDeep(Base other_) { 1462 if (!super.equalsDeep(other_)) 1463 return false; 1464 if (!(other_ instanceof BenefitComponent)) 1465 return false; 1466 BenefitComponent o = (BenefitComponent) other_; 1467 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true) && compareDeep(used, o.used, true) 1468 ; 1469 } 1470 1471 @Override 1472 public boolean equalsShallow(Base other_) { 1473 if (!super.equalsShallow(other_)) 1474 return false; 1475 if (!(other_ instanceof BenefitComponent)) 1476 return false; 1477 BenefitComponent o = (BenefitComponent) other_; 1478 return true; 1479 } 1480 1481 public boolean isEmpty() { 1482 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed, used); 1483 } 1484 1485 public String fhirType() { 1486 return "EligibilityResponse.insurance.benefitBalance.financial"; 1487 1488 } 1489 1490 } 1491 1492 @Block() 1493 public static class ErrorsComponent extends BackboneElement implements IBaseBackboneElement { 1494 /** 1495 * An error code,from a specified code system, which details why the eligibility check could not be performed. 1496 */ 1497 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 1498 @Description(shortDefinition="Error code detailing processing issues", formalDefinition="An error code,from a specified code system, which details why the eligibility check could not be performed." ) 1499 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-error") 1500 protected CodeableConcept code; 1501 1502 private static final long serialVersionUID = -1048343046L; 1503 1504 /** 1505 * Constructor 1506 */ 1507 public ErrorsComponent() { 1508 super(); 1509 } 1510 1511 /** 1512 * Constructor 1513 */ 1514 public ErrorsComponent(CodeableConcept code) { 1515 super(); 1516 this.code = code; 1517 } 1518 1519 /** 1520 * @return {@link #code} (An error code,from a specified code system, which details why the eligibility check could not be performed.) 1521 */ 1522 public CodeableConcept getCode() { 1523 if (this.code == null) 1524 if (Configuration.errorOnAutoCreate()) 1525 throw new Error("Attempt to auto-create ErrorsComponent.code"); 1526 else if (Configuration.doAutoCreate()) 1527 this.code = new CodeableConcept(); // cc 1528 return this.code; 1529 } 1530 1531 public boolean hasCode() { 1532 return this.code != null && !this.code.isEmpty(); 1533 } 1534 1535 /** 1536 * @param value {@link #code} (An error code,from a specified code system, which details why the eligibility check could not be performed.) 1537 */ 1538 public ErrorsComponent setCode(CodeableConcept value) { 1539 this.code = value; 1540 return this; 1541 } 1542 1543 protected void listChildren(List<Property> children) { 1544 super.listChildren(children); 1545 children.add(new Property("code", "CodeableConcept", "An error code,from a specified code system, which details why the eligibility check could not be performed.", 0, 1, code)); 1546 } 1547 1548 @Override 1549 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1550 switch (_hash) { 1551 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "An error code,from a specified code system, which details why the eligibility check could not be performed.", 0, 1, code); 1552 default: return super.getNamedProperty(_hash, _name, _checkValid); 1553 } 1554 1555 } 1556 1557 @Override 1558 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1559 switch (hash) { 1560 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1561 default: return super.getProperty(hash, name, checkValid); 1562 } 1563 1564 } 1565 1566 @Override 1567 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1568 switch (hash) { 1569 case 3059181: // code 1570 this.code = castToCodeableConcept(value); // CodeableConcept 1571 return value; 1572 default: return super.setProperty(hash, name, value); 1573 } 1574 1575 } 1576 1577 @Override 1578 public Base setProperty(String name, Base value) throws FHIRException { 1579 if (name.equals("code")) { 1580 this.code = castToCodeableConcept(value); // CodeableConcept 1581 } else 1582 return super.setProperty(name, value); 1583 return value; 1584 } 1585 1586 @Override 1587 public Base makeProperty(int hash, String name) throws FHIRException { 1588 switch (hash) { 1589 case 3059181: return getCode(); 1590 default: return super.makeProperty(hash, name); 1591 } 1592 1593 } 1594 1595 @Override 1596 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1597 switch (hash) { 1598 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1599 default: return super.getTypesForProperty(hash, name); 1600 } 1601 1602 } 1603 1604 @Override 1605 public Base addChild(String name) throws FHIRException { 1606 if (name.equals("code")) { 1607 this.code = new CodeableConcept(); 1608 return this.code; 1609 } 1610 else 1611 return super.addChild(name); 1612 } 1613 1614 public ErrorsComponent copy() { 1615 ErrorsComponent dst = new ErrorsComponent(); 1616 copyValues(dst); 1617 dst.code = code == null ? null : code.copy(); 1618 return dst; 1619 } 1620 1621 @Override 1622 public boolean equalsDeep(Base other_) { 1623 if (!super.equalsDeep(other_)) 1624 return false; 1625 if (!(other_ instanceof ErrorsComponent)) 1626 return false; 1627 ErrorsComponent o = (ErrorsComponent) other_; 1628 return compareDeep(code, o.code, true); 1629 } 1630 1631 @Override 1632 public boolean equalsShallow(Base other_) { 1633 if (!super.equalsShallow(other_)) 1634 return false; 1635 if (!(other_ instanceof ErrorsComponent)) 1636 return false; 1637 ErrorsComponent o = (ErrorsComponent) other_; 1638 return true; 1639 } 1640 1641 public boolean isEmpty() { 1642 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code); 1643 } 1644 1645 public String fhirType() { 1646 return "EligibilityResponse.error"; 1647 1648 } 1649 1650 } 1651 1652 /** 1653 * The Response business identifier. 1654 */ 1655 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1656 @Description(shortDefinition="Business Identifier", formalDefinition="The Response business identifier." ) 1657 protected List<Identifier> identifier; 1658 1659 /** 1660 * The status of the resource instance. 1661 */ 1662 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 1663 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 1664 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 1665 protected Enumeration<EligibilityResponseStatus> status; 1666 1667 /** 1668 * The date when the enclosed suite of services were performed or completed. 1669 */ 1670 @Child(name = "created", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1671 @Description(shortDefinition="Creation date", formalDefinition="The date when the enclosed suite of services were performed or completed." ) 1672 protected DateTimeType created; 1673 1674 /** 1675 * The practitioner who is responsible for the services rendered to the patient. 1676 */ 1677 @Child(name = "requestProvider", type = {Practitioner.class}, order=3, min=0, max=1, modifier=false, summary=false) 1678 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 1679 protected Reference requestProvider; 1680 1681 /** 1682 * The actual object that is the target of the reference (The practitioner who is responsible for the services rendered to the patient.) 1683 */ 1684 protected Practitioner requestProviderTarget; 1685 1686 /** 1687 * The organization which is responsible for the services rendered to the patient. 1688 */ 1689 @Child(name = "requestOrganization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=false) 1690 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the services rendered to the patient." ) 1691 protected Reference requestOrganization; 1692 1693 /** 1694 * The actual object that is the target of the reference (The organization which is responsible for the services rendered to the patient.) 1695 */ 1696 protected Organization requestOrganizationTarget; 1697 1698 /** 1699 * Original request resource reference. 1700 */ 1701 @Child(name = "request", type = {EligibilityRequest.class}, order=5, min=0, max=1, modifier=false, summary=false) 1702 @Description(shortDefinition="Eligibility reference", formalDefinition="Original request resource reference." ) 1703 protected Reference request; 1704 1705 /** 1706 * The actual object that is the target of the reference (Original request resource reference.) 1707 */ 1708 protected EligibilityRequest requestTarget; 1709 1710 /** 1711 * Transaction status: error, complete. 1712 */ 1713 @Child(name = "outcome", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1714 @Description(shortDefinition="complete | error | partial", formalDefinition="Transaction status: error, complete." ) 1715 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/remittance-outcome") 1716 protected CodeableConcept outcome; 1717 1718 /** 1719 * A description of the status of the adjudication. 1720 */ 1721 @Child(name = "disposition", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=false) 1722 @Description(shortDefinition="Disposition Message", formalDefinition="A description of the status of the adjudication." ) 1723 protected StringType disposition; 1724 1725 /** 1726 * The Insurer who produced this adjudicated response. 1727 */ 1728 @Child(name = "insurer", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 1729 @Description(shortDefinition="Insurer issuing the coverage", formalDefinition="The Insurer who produced this adjudicated response." ) 1730 protected Reference insurer; 1731 1732 /** 1733 * The actual object that is the target of the reference (The Insurer who produced this adjudicated response.) 1734 */ 1735 protected Organization insurerTarget; 1736 1737 /** 1738 * Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates. 1739 */ 1740 @Child(name = "inforce", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 1741 @Description(shortDefinition="Coverage inforce indicator", formalDefinition="Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates." ) 1742 protected BooleanType inforce; 1743 1744 /** 1745 * The insurer may provide both the details for the requested coverage as well as details for additional coverages known to the insurer. 1746 */ 1747 @Child(name = "insurance", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1748 @Description(shortDefinition="Details by insurance coverage", formalDefinition="The insurer may provide both the details for the requested coverage as well as details for additional coverages known to the insurer." ) 1749 protected List<InsuranceComponent> insurance; 1750 1751 /** 1752 * The form to be used for printing the content. 1753 */ 1754 @Child(name = "form", type = {CodeableConcept.class}, order=11, min=0, max=1, modifier=false, summary=false) 1755 @Description(shortDefinition="Printed Form Identifier", formalDefinition="The form to be used for printing the content." ) 1756 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 1757 protected CodeableConcept form; 1758 1759 /** 1760 * Mutually exclusive with Services Provided (Item). 1761 */ 1762 @Child(name = "error", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1763 @Description(shortDefinition="Processing errors", formalDefinition="Mutually exclusive with Services Provided (Item)." ) 1764 protected List<ErrorsComponent> error; 1765 1766 private static final long serialVersionUID = 954270539L; 1767 1768 /** 1769 * Constructor 1770 */ 1771 public EligibilityResponse() { 1772 super(); 1773 } 1774 1775 /** 1776 * @return {@link #identifier} (The Response business identifier.) 1777 */ 1778 public List<Identifier> getIdentifier() { 1779 if (this.identifier == null) 1780 this.identifier = new ArrayList<Identifier>(); 1781 return this.identifier; 1782 } 1783 1784 /** 1785 * @return Returns a reference to <code>this</code> for easy method chaining 1786 */ 1787 public EligibilityResponse setIdentifier(List<Identifier> theIdentifier) { 1788 this.identifier = theIdentifier; 1789 return this; 1790 } 1791 1792 public boolean hasIdentifier() { 1793 if (this.identifier == null) 1794 return false; 1795 for (Identifier item : this.identifier) 1796 if (!item.isEmpty()) 1797 return true; 1798 return false; 1799 } 1800 1801 public Identifier addIdentifier() { //3 1802 Identifier t = new Identifier(); 1803 if (this.identifier == null) 1804 this.identifier = new ArrayList<Identifier>(); 1805 this.identifier.add(t); 1806 return t; 1807 } 1808 1809 public EligibilityResponse addIdentifier(Identifier t) { //3 1810 if (t == null) 1811 return this; 1812 if (this.identifier == null) 1813 this.identifier = new ArrayList<Identifier>(); 1814 this.identifier.add(t); 1815 return this; 1816 } 1817 1818 /** 1819 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1820 */ 1821 public Identifier getIdentifierFirstRep() { 1822 if (getIdentifier().isEmpty()) { 1823 addIdentifier(); 1824 } 1825 return getIdentifier().get(0); 1826 } 1827 1828 /** 1829 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1830 */ 1831 public Enumeration<EligibilityResponseStatus> getStatusElement() { 1832 if (this.status == null) 1833 if (Configuration.errorOnAutoCreate()) 1834 throw new Error("Attempt to auto-create EligibilityResponse.status"); 1835 else if (Configuration.doAutoCreate()) 1836 this.status = new Enumeration<EligibilityResponseStatus>(new EligibilityResponseStatusEnumFactory()); // bb 1837 return this.status; 1838 } 1839 1840 public boolean hasStatusElement() { 1841 return this.status != null && !this.status.isEmpty(); 1842 } 1843 1844 public boolean hasStatus() { 1845 return this.status != null && !this.status.isEmpty(); 1846 } 1847 1848 /** 1849 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1850 */ 1851 public EligibilityResponse setStatusElement(Enumeration<EligibilityResponseStatus> value) { 1852 this.status = value; 1853 return this; 1854 } 1855 1856 /** 1857 * @return The status of the resource instance. 1858 */ 1859 public EligibilityResponseStatus getStatus() { 1860 return this.status == null ? null : this.status.getValue(); 1861 } 1862 1863 /** 1864 * @param value The status of the resource instance. 1865 */ 1866 public EligibilityResponse setStatus(EligibilityResponseStatus value) { 1867 if (value == null) 1868 this.status = null; 1869 else { 1870 if (this.status == null) 1871 this.status = new Enumeration<EligibilityResponseStatus>(new EligibilityResponseStatusEnumFactory()); 1872 this.status.setValue(value); 1873 } 1874 return this; 1875 } 1876 1877 /** 1878 * @return {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1879 */ 1880 public DateTimeType getCreatedElement() { 1881 if (this.created == null) 1882 if (Configuration.errorOnAutoCreate()) 1883 throw new Error("Attempt to auto-create EligibilityResponse.created"); 1884 else if (Configuration.doAutoCreate()) 1885 this.created = new DateTimeType(); // bb 1886 return this.created; 1887 } 1888 1889 public boolean hasCreatedElement() { 1890 return this.created != null && !this.created.isEmpty(); 1891 } 1892 1893 public boolean hasCreated() { 1894 return this.created != null && !this.created.isEmpty(); 1895 } 1896 1897 /** 1898 * @param value {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 1899 */ 1900 public EligibilityResponse setCreatedElement(DateTimeType value) { 1901 this.created = value; 1902 return this; 1903 } 1904 1905 /** 1906 * @return The date when the enclosed suite of services were performed or completed. 1907 */ 1908 public Date getCreated() { 1909 return this.created == null ? null : this.created.getValue(); 1910 } 1911 1912 /** 1913 * @param value The date when the enclosed suite of services were performed or completed. 1914 */ 1915 public EligibilityResponse setCreated(Date value) { 1916 if (value == null) 1917 this.created = null; 1918 else { 1919 if (this.created == null) 1920 this.created = new DateTimeType(); 1921 this.created.setValue(value); 1922 } 1923 return this; 1924 } 1925 1926 /** 1927 * @return {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 1928 */ 1929 public Reference getRequestProvider() { 1930 if (this.requestProvider == null) 1931 if (Configuration.errorOnAutoCreate()) 1932 throw new Error("Attempt to auto-create EligibilityResponse.requestProvider"); 1933 else if (Configuration.doAutoCreate()) 1934 this.requestProvider = new Reference(); // cc 1935 return this.requestProvider; 1936 } 1937 1938 public boolean hasRequestProvider() { 1939 return this.requestProvider != null && !this.requestProvider.isEmpty(); 1940 } 1941 1942 /** 1943 * @param value {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 1944 */ 1945 public EligibilityResponse setRequestProvider(Reference value) { 1946 this.requestProvider = value; 1947 return this; 1948 } 1949 1950 /** 1951 * @return {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 1952 */ 1953 public Practitioner getRequestProviderTarget() { 1954 if (this.requestProviderTarget == null) 1955 if (Configuration.errorOnAutoCreate()) 1956 throw new Error("Attempt to auto-create EligibilityResponse.requestProvider"); 1957 else if (Configuration.doAutoCreate()) 1958 this.requestProviderTarget = new Practitioner(); // aa 1959 return this.requestProviderTarget; 1960 } 1961 1962 /** 1963 * @param value {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 1964 */ 1965 public EligibilityResponse setRequestProviderTarget(Practitioner value) { 1966 this.requestProviderTarget = value; 1967 return this; 1968 } 1969 1970 /** 1971 * @return {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 1972 */ 1973 public Reference getRequestOrganization() { 1974 if (this.requestOrganization == null) 1975 if (Configuration.errorOnAutoCreate()) 1976 throw new Error("Attempt to auto-create EligibilityResponse.requestOrganization"); 1977 else if (Configuration.doAutoCreate()) 1978 this.requestOrganization = new Reference(); // cc 1979 return this.requestOrganization; 1980 } 1981 1982 public boolean hasRequestOrganization() { 1983 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 1984 } 1985 1986 /** 1987 * @param value {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 1988 */ 1989 public EligibilityResponse setRequestOrganization(Reference value) { 1990 this.requestOrganization = value; 1991 return this; 1992 } 1993 1994 /** 1995 * @return {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 1996 */ 1997 public Organization getRequestOrganizationTarget() { 1998 if (this.requestOrganizationTarget == null) 1999 if (Configuration.errorOnAutoCreate()) 2000 throw new Error("Attempt to auto-create EligibilityResponse.requestOrganization"); 2001 else if (Configuration.doAutoCreate()) 2002 this.requestOrganizationTarget = new Organization(); // aa 2003 return this.requestOrganizationTarget; 2004 } 2005 2006 /** 2007 * @param value {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 2008 */ 2009 public EligibilityResponse setRequestOrganizationTarget(Organization value) { 2010 this.requestOrganizationTarget = value; 2011 return this; 2012 } 2013 2014 /** 2015 * @return {@link #request} (Original request resource reference.) 2016 */ 2017 public Reference getRequest() { 2018 if (this.request == null) 2019 if (Configuration.errorOnAutoCreate()) 2020 throw new Error("Attempt to auto-create EligibilityResponse.request"); 2021 else if (Configuration.doAutoCreate()) 2022 this.request = new Reference(); // cc 2023 return this.request; 2024 } 2025 2026 public boolean hasRequest() { 2027 return this.request != null && !this.request.isEmpty(); 2028 } 2029 2030 /** 2031 * @param value {@link #request} (Original request resource reference.) 2032 */ 2033 public EligibilityResponse setRequest(Reference value) { 2034 this.request = value; 2035 return this; 2036 } 2037 2038 /** 2039 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original request resource reference.) 2040 */ 2041 public EligibilityRequest getRequestTarget() { 2042 if (this.requestTarget == null) 2043 if (Configuration.errorOnAutoCreate()) 2044 throw new Error("Attempt to auto-create EligibilityResponse.request"); 2045 else if (Configuration.doAutoCreate()) 2046 this.requestTarget = new EligibilityRequest(); // aa 2047 return this.requestTarget; 2048 } 2049 2050 /** 2051 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original request resource reference.) 2052 */ 2053 public EligibilityResponse setRequestTarget(EligibilityRequest value) { 2054 this.requestTarget = value; 2055 return this; 2056 } 2057 2058 /** 2059 * @return {@link #outcome} (Transaction status: error, complete.) 2060 */ 2061 public CodeableConcept getOutcome() { 2062 if (this.outcome == null) 2063 if (Configuration.errorOnAutoCreate()) 2064 throw new Error("Attempt to auto-create EligibilityResponse.outcome"); 2065 else if (Configuration.doAutoCreate()) 2066 this.outcome = new CodeableConcept(); // cc 2067 return this.outcome; 2068 } 2069 2070 public boolean hasOutcome() { 2071 return this.outcome != null && !this.outcome.isEmpty(); 2072 } 2073 2074 /** 2075 * @param value {@link #outcome} (Transaction status: error, complete.) 2076 */ 2077 public EligibilityResponse setOutcome(CodeableConcept value) { 2078 this.outcome = value; 2079 return this; 2080 } 2081 2082 /** 2083 * @return {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 2084 */ 2085 public StringType getDispositionElement() { 2086 if (this.disposition == null) 2087 if (Configuration.errorOnAutoCreate()) 2088 throw new Error("Attempt to auto-create EligibilityResponse.disposition"); 2089 else if (Configuration.doAutoCreate()) 2090 this.disposition = new StringType(); // bb 2091 return this.disposition; 2092 } 2093 2094 public boolean hasDispositionElement() { 2095 return this.disposition != null && !this.disposition.isEmpty(); 2096 } 2097 2098 public boolean hasDisposition() { 2099 return this.disposition != null && !this.disposition.isEmpty(); 2100 } 2101 2102 /** 2103 * @param value {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 2104 */ 2105 public EligibilityResponse setDispositionElement(StringType value) { 2106 this.disposition = value; 2107 return this; 2108 } 2109 2110 /** 2111 * @return A description of the status of the adjudication. 2112 */ 2113 public String getDisposition() { 2114 return this.disposition == null ? null : this.disposition.getValue(); 2115 } 2116 2117 /** 2118 * @param value A description of the status of the adjudication. 2119 */ 2120 public EligibilityResponse setDisposition(String value) { 2121 if (Utilities.noString(value)) 2122 this.disposition = null; 2123 else { 2124 if (this.disposition == null) 2125 this.disposition = new StringType(); 2126 this.disposition.setValue(value); 2127 } 2128 return this; 2129 } 2130 2131 /** 2132 * @return {@link #insurer} (The Insurer who produced this adjudicated response.) 2133 */ 2134 public Reference getInsurer() { 2135 if (this.insurer == null) 2136 if (Configuration.errorOnAutoCreate()) 2137 throw new Error("Attempt to auto-create EligibilityResponse.insurer"); 2138 else if (Configuration.doAutoCreate()) 2139 this.insurer = new Reference(); // cc 2140 return this.insurer; 2141 } 2142 2143 public boolean hasInsurer() { 2144 return this.insurer != null && !this.insurer.isEmpty(); 2145 } 2146 2147 /** 2148 * @param value {@link #insurer} (The Insurer who produced this adjudicated response.) 2149 */ 2150 public EligibilityResponse setInsurer(Reference value) { 2151 this.insurer = value; 2152 return this; 2153 } 2154 2155 /** 2156 * @return {@link #insurer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who produced this adjudicated response.) 2157 */ 2158 public Organization getInsurerTarget() { 2159 if (this.insurerTarget == null) 2160 if (Configuration.errorOnAutoCreate()) 2161 throw new Error("Attempt to auto-create EligibilityResponse.insurer"); 2162 else if (Configuration.doAutoCreate()) 2163 this.insurerTarget = new Organization(); // aa 2164 return this.insurerTarget; 2165 } 2166 2167 /** 2168 * @param value {@link #insurer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who produced this adjudicated response.) 2169 */ 2170 public EligibilityResponse setInsurerTarget(Organization value) { 2171 this.insurerTarget = value; 2172 return this; 2173 } 2174 2175 /** 2176 * @return {@link #inforce} (Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.). This is the underlying object with id, value and extensions. The accessor "getInforce" gives direct access to the value 2177 */ 2178 public BooleanType getInforceElement() { 2179 if (this.inforce == null) 2180 if (Configuration.errorOnAutoCreate()) 2181 throw new Error("Attempt to auto-create EligibilityResponse.inforce"); 2182 else if (Configuration.doAutoCreate()) 2183 this.inforce = new BooleanType(); // bb 2184 return this.inforce; 2185 } 2186 2187 public boolean hasInforceElement() { 2188 return this.inforce != null && !this.inforce.isEmpty(); 2189 } 2190 2191 public boolean hasInforce() { 2192 return this.inforce != null && !this.inforce.isEmpty(); 2193 } 2194 2195 /** 2196 * @param value {@link #inforce} (Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.). This is the underlying object with id, value and extensions. The accessor "getInforce" gives direct access to the value 2197 */ 2198 public EligibilityResponse setInforceElement(BooleanType value) { 2199 this.inforce = value; 2200 return this; 2201 } 2202 2203 /** 2204 * @return Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates. 2205 */ 2206 public boolean getInforce() { 2207 return this.inforce == null || this.inforce.isEmpty() ? false : this.inforce.getValue(); 2208 } 2209 2210 /** 2211 * @param value Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates. 2212 */ 2213 public EligibilityResponse setInforce(boolean value) { 2214 if (this.inforce == null) 2215 this.inforce = new BooleanType(); 2216 this.inforce.setValue(value); 2217 return this; 2218 } 2219 2220 /** 2221 * @return {@link #insurance} (The insurer may provide both the details for the requested coverage as well as details for additional coverages known to the insurer.) 2222 */ 2223 public List<InsuranceComponent> getInsurance() { 2224 if (this.insurance == null) 2225 this.insurance = new ArrayList<InsuranceComponent>(); 2226 return this.insurance; 2227 } 2228 2229 /** 2230 * @return Returns a reference to <code>this</code> for easy method chaining 2231 */ 2232 public EligibilityResponse setInsurance(List<InsuranceComponent> theInsurance) { 2233 this.insurance = theInsurance; 2234 return this; 2235 } 2236 2237 public boolean hasInsurance() { 2238 if (this.insurance == null) 2239 return false; 2240 for (InsuranceComponent item : this.insurance) 2241 if (!item.isEmpty()) 2242 return true; 2243 return false; 2244 } 2245 2246 public InsuranceComponent addInsurance() { //3 2247 InsuranceComponent t = new InsuranceComponent(); 2248 if (this.insurance == null) 2249 this.insurance = new ArrayList<InsuranceComponent>(); 2250 this.insurance.add(t); 2251 return t; 2252 } 2253 2254 public EligibilityResponse addInsurance(InsuranceComponent t) { //3 2255 if (t == null) 2256 return this; 2257 if (this.insurance == null) 2258 this.insurance = new ArrayList<InsuranceComponent>(); 2259 this.insurance.add(t); 2260 return this; 2261 } 2262 2263 /** 2264 * @return The first repetition of repeating field {@link #insurance}, creating it if it does not already exist 2265 */ 2266 public InsuranceComponent getInsuranceFirstRep() { 2267 if (getInsurance().isEmpty()) { 2268 addInsurance(); 2269 } 2270 return getInsurance().get(0); 2271 } 2272 2273 /** 2274 * @return {@link #form} (The form to be used for printing the content.) 2275 */ 2276 public CodeableConcept getForm() { 2277 if (this.form == null) 2278 if (Configuration.errorOnAutoCreate()) 2279 throw new Error("Attempt to auto-create EligibilityResponse.form"); 2280 else if (Configuration.doAutoCreate()) 2281 this.form = new CodeableConcept(); // cc 2282 return this.form; 2283 } 2284 2285 public boolean hasForm() { 2286 return this.form != null && !this.form.isEmpty(); 2287 } 2288 2289 /** 2290 * @param value {@link #form} (The form to be used for printing the content.) 2291 */ 2292 public EligibilityResponse setForm(CodeableConcept value) { 2293 this.form = value; 2294 return this; 2295 } 2296 2297 /** 2298 * @return {@link #error} (Mutually exclusive with Services Provided (Item).) 2299 */ 2300 public List<ErrorsComponent> getError() { 2301 if (this.error == null) 2302 this.error = new ArrayList<ErrorsComponent>(); 2303 return this.error; 2304 } 2305 2306 /** 2307 * @return Returns a reference to <code>this</code> for easy method chaining 2308 */ 2309 public EligibilityResponse setError(List<ErrorsComponent> theError) { 2310 this.error = theError; 2311 return this; 2312 } 2313 2314 public boolean hasError() { 2315 if (this.error == null) 2316 return false; 2317 for (ErrorsComponent item : this.error) 2318 if (!item.isEmpty()) 2319 return true; 2320 return false; 2321 } 2322 2323 public ErrorsComponent addError() { //3 2324 ErrorsComponent t = new ErrorsComponent(); 2325 if (this.error == null) 2326 this.error = new ArrayList<ErrorsComponent>(); 2327 this.error.add(t); 2328 return t; 2329 } 2330 2331 public EligibilityResponse addError(ErrorsComponent t) { //3 2332 if (t == null) 2333 return this; 2334 if (this.error == null) 2335 this.error = new ArrayList<ErrorsComponent>(); 2336 this.error.add(t); 2337 return this; 2338 } 2339 2340 /** 2341 * @return The first repetition of repeating field {@link #error}, creating it if it does not already exist 2342 */ 2343 public ErrorsComponent getErrorFirstRep() { 2344 if (getError().isEmpty()) { 2345 addError(); 2346 } 2347 return getError().get(0); 2348 } 2349 2350 protected void listChildren(List<Property> children) { 2351 super.listChildren(children); 2352 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2353 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 2354 children.add(new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 2355 children.add(new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider)); 2356 children.add(new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization)); 2357 children.add(new Property("request", "Reference(EligibilityRequest)", "Original request resource reference.", 0, 1, request)); 2358 children.add(new Property("outcome", "CodeableConcept", "Transaction status: error, complete.", 0, 1, outcome)); 2359 children.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition)); 2360 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, insurer)); 2361 children.add(new Property("inforce", "boolean", "Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.", 0, 1, inforce)); 2362 children.add(new Property("insurance", "", "The insurer may provide both the details for the requested coverage as well as details for additional coverages known to the insurer.", 0, java.lang.Integer.MAX_VALUE, insurance)); 2363 children.add(new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form)); 2364 children.add(new Property("error", "", "Mutually exclusive with Services Provided (Item).", 0, java.lang.Integer.MAX_VALUE, error)); 2365 } 2366 2367 @Override 2368 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2369 switch (_hash) { 2370 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 2371 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 2372 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 2373 case 1601527200: /*requestProvider*/ return new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider); 2374 case 599053666: /*requestOrganization*/ return new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization); 2375 case 1095692943: /*request*/ return new Property("request", "Reference(EligibilityRequest)", "Original request resource reference.", 0, 1, request); 2376 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Transaction status: error, complete.", 0, 1, outcome); 2377 case 583380919: /*disposition*/ return new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition); 2378 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, insurer); 2379 case 1945431270: /*inforce*/ return new Property("inforce", "boolean", "Flag indicating if the coverage provided is inforce currently if no service date(s) specified or for the whole duration of the service dates.", 0, 1, inforce); 2380 case 73049818: /*insurance*/ return new Property("insurance", "", "The insurer may provide both the details for the requested coverage as well as details for additional coverages known to the insurer.", 0, java.lang.Integer.MAX_VALUE, insurance); 2381 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form); 2382 case 96784904: /*error*/ return new Property("error", "", "Mutually exclusive with Services Provided (Item).", 0, java.lang.Integer.MAX_VALUE, error); 2383 default: return super.getNamedProperty(_hash, _name, _checkValid); 2384 } 2385 2386 } 2387 2388 @Override 2389 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2390 switch (hash) { 2391 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2392 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EligibilityResponseStatus> 2393 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 2394 case 1601527200: /*requestProvider*/ return this.requestProvider == null ? new Base[0] : new Base[] {this.requestProvider}; // Reference 2395 case 599053666: /*requestOrganization*/ return this.requestOrganization == null ? new Base[0] : new Base[] {this.requestOrganization}; // Reference 2396 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 2397 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 2398 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 2399 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 2400 case 1945431270: /*inforce*/ return this.inforce == null ? new Base[0] : new Base[] {this.inforce}; // BooleanType 2401 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : this.insurance.toArray(new Base[this.insurance.size()]); // InsuranceComponent 2402 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 2403 case 96784904: /*error*/ return this.error == null ? new Base[0] : this.error.toArray(new Base[this.error.size()]); // ErrorsComponent 2404 default: return super.getProperty(hash, name, checkValid); 2405 } 2406 2407 } 2408 2409 @Override 2410 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2411 switch (hash) { 2412 case -1618432855: // identifier 2413 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2414 return value; 2415 case -892481550: // status 2416 value = new EligibilityResponseStatusEnumFactory().fromType(castToCode(value)); 2417 this.status = (Enumeration) value; // Enumeration<EligibilityResponseStatus> 2418 return value; 2419 case 1028554472: // created 2420 this.created = castToDateTime(value); // DateTimeType 2421 return value; 2422 case 1601527200: // requestProvider 2423 this.requestProvider = castToReference(value); // Reference 2424 return value; 2425 case 599053666: // requestOrganization 2426 this.requestOrganization = castToReference(value); // Reference 2427 return value; 2428 case 1095692943: // request 2429 this.request = castToReference(value); // Reference 2430 return value; 2431 case -1106507950: // outcome 2432 this.outcome = castToCodeableConcept(value); // CodeableConcept 2433 return value; 2434 case 583380919: // disposition 2435 this.disposition = castToString(value); // StringType 2436 return value; 2437 case 1957615864: // insurer 2438 this.insurer = castToReference(value); // Reference 2439 return value; 2440 case 1945431270: // inforce 2441 this.inforce = castToBoolean(value); // BooleanType 2442 return value; 2443 case 73049818: // insurance 2444 this.getInsurance().add((InsuranceComponent) value); // InsuranceComponent 2445 return value; 2446 case 3148996: // form 2447 this.form = castToCodeableConcept(value); // CodeableConcept 2448 return value; 2449 case 96784904: // error 2450 this.getError().add((ErrorsComponent) value); // ErrorsComponent 2451 return value; 2452 default: return super.setProperty(hash, name, value); 2453 } 2454 2455 } 2456 2457 @Override 2458 public Base setProperty(String name, Base value) throws FHIRException { 2459 if (name.equals("identifier")) { 2460 this.getIdentifier().add(castToIdentifier(value)); 2461 } else if (name.equals("status")) { 2462 value = new EligibilityResponseStatusEnumFactory().fromType(castToCode(value)); 2463 this.status = (Enumeration) value; // Enumeration<EligibilityResponseStatus> 2464 } else if (name.equals("created")) { 2465 this.created = castToDateTime(value); // DateTimeType 2466 } else if (name.equals("requestProvider")) { 2467 this.requestProvider = castToReference(value); // Reference 2468 } else if (name.equals("requestOrganization")) { 2469 this.requestOrganization = castToReference(value); // Reference 2470 } else if (name.equals("request")) { 2471 this.request = castToReference(value); // Reference 2472 } else if (name.equals("outcome")) { 2473 this.outcome = castToCodeableConcept(value); // CodeableConcept 2474 } else if (name.equals("disposition")) { 2475 this.disposition = castToString(value); // StringType 2476 } else if (name.equals("insurer")) { 2477 this.insurer = castToReference(value); // Reference 2478 } else if (name.equals("inforce")) { 2479 this.inforce = castToBoolean(value); // BooleanType 2480 } else if (name.equals("insurance")) { 2481 this.getInsurance().add((InsuranceComponent) value); 2482 } else if (name.equals("form")) { 2483 this.form = castToCodeableConcept(value); // CodeableConcept 2484 } else if (name.equals("error")) { 2485 this.getError().add((ErrorsComponent) value); 2486 } else 2487 return super.setProperty(name, value); 2488 return value; 2489 } 2490 2491 @Override 2492 public Base makeProperty(int hash, String name) throws FHIRException { 2493 switch (hash) { 2494 case -1618432855: return addIdentifier(); 2495 case -892481550: return getStatusElement(); 2496 case 1028554472: return getCreatedElement(); 2497 case 1601527200: return getRequestProvider(); 2498 case 599053666: return getRequestOrganization(); 2499 case 1095692943: return getRequest(); 2500 case -1106507950: return getOutcome(); 2501 case 583380919: return getDispositionElement(); 2502 case 1957615864: return getInsurer(); 2503 case 1945431270: return getInforceElement(); 2504 case 73049818: return addInsurance(); 2505 case 3148996: return getForm(); 2506 case 96784904: return addError(); 2507 default: return super.makeProperty(hash, name); 2508 } 2509 2510 } 2511 2512 @Override 2513 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2514 switch (hash) { 2515 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2516 case -892481550: /*status*/ return new String[] {"code"}; 2517 case 1028554472: /*created*/ return new String[] {"dateTime"}; 2518 case 1601527200: /*requestProvider*/ return new String[] {"Reference"}; 2519 case 599053666: /*requestOrganization*/ return new String[] {"Reference"}; 2520 case 1095692943: /*request*/ return new String[] {"Reference"}; 2521 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 2522 case 583380919: /*disposition*/ return new String[] {"string"}; 2523 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 2524 case 1945431270: /*inforce*/ return new String[] {"boolean"}; 2525 case 73049818: /*insurance*/ return new String[] {}; 2526 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 2527 case 96784904: /*error*/ return new String[] {}; 2528 default: return super.getTypesForProperty(hash, name); 2529 } 2530 2531 } 2532 2533 @Override 2534 public Base addChild(String name) throws FHIRException { 2535 if (name.equals("identifier")) { 2536 return addIdentifier(); 2537 } 2538 else if (name.equals("status")) { 2539 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.status"); 2540 } 2541 else if (name.equals("created")) { 2542 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.created"); 2543 } 2544 else if (name.equals("requestProvider")) { 2545 this.requestProvider = new Reference(); 2546 return this.requestProvider; 2547 } 2548 else if (name.equals("requestOrganization")) { 2549 this.requestOrganization = new Reference(); 2550 return this.requestOrganization; 2551 } 2552 else if (name.equals("request")) { 2553 this.request = new Reference(); 2554 return this.request; 2555 } 2556 else if (name.equals("outcome")) { 2557 this.outcome = new CodeableConcept(); 2558 return this.outcome; 2559 } 2560 else if (name.equals("disposition")) { 2561 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.disposition"); 2562 } 2563 else if (name.equals("insurer")) { 2564 this.insurer = new Reference(); 2565 return this.insurer; 2566 } 2567 else if (name.equals("inforce")) { 2568 throw new FHIRException("Cannot call addChild on a singleton property EligibilityResponse.inforce"); 2569 } 2570 else if (name.equals("insurance")) { 2571 return addInsurance(); 2572 } 2573 else if (name.equals("form")) { 2574 this.form = new CodeableConcept(); 2575 return this.form; 2576 } 2577 else if (name.equals("error")) { 2578 return addError(); 2579 } 2580 else 2581 return super.addChild(name); 2582 } 2583 2584 public String fhirType() { 2585 return "EligibilityResponse"; 2586 2587 } 2588 2589 public EligibilityResponse copy() { 2590 EligibilityResponse dst = new EligibilityResponse(); 2591 copyValues(dst); 2592 if (identifier != null) { 2593 dst.identifier = new ArrayList<Identifier>(); 2594 for (Identifier i : identifier) 2595 dst.identifier.add(i.copy()); 2596 }; 2597 dst.status = status == null ? null : status.copy(); 2598 dst.created = created == null ? null : created.copy(); 2599 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 2600 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 2601 dst.request = request == null ? null : request.copy(); 2602 dst.outcome = outcome == null ? null : outcome.copy(); 2603 dst.disposition = disposition == null ? null : disposition.copy(); 2604 dst.insurer = insurer == null ? null : insurer.copy(); 2605 dst.inforce = inforce == null ? null : inforce.copy(); 2606 if (insurance != null) { 2607 dst.insurance = new ArrayList<InsuranceComponent>(); 2608 for (InsuranceComponent i : insurance) 2609 dst.insurance.add(i.copy()); 2610 }; 2611 dst.form = form == null ? null : form.copy(); 2612 if (error != null) { 2613 dst.error = new ArrayList<ErrorsComponent>(); 2614 for (ErrorsComponent i : error) 2615 dst.error.add(i.copy()); 2616 }; 2617 return dst; 2618 } 2619 2620 protected EligibilityResponse typedCopy() { 2621 return copy(); 2622 } 2623 2624 @Override 2625 public boolean equalsDeep(Base other_) { 2626 if (!super.equalsDeep(other_)) 2627 return false; 2628 if (!(other_ instanceof EligibilityResponse)) 2629 return false; 2630 EligibilityResponse o = (EligibilityResponse) other_; 2631 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(created, o.created, true) 2632 && compareDeep(requestProvider, o.requestProvider, true) && compareDeep(requestOrganization, o.requestOrganization, true) 2633 && compareDeep(request, o.request, true) && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) 2634 && compareDeep(insurer, o.insurer, true) && compareDeep(inforce, o.inforce, true) && compareDeep(insurance, o.insurance, true) 2635 && compareDeep(form, o.form, true) && compareDeep(error, o.error, true); 2636 } 2637 2638 @Override 2639 public boolean equalsShallow(Base other_) { 2640 if (!super.equalsShallow(other_)) 2641 return false; 2642 if (!(other_ instanceof EligibilityResponse)) 2643 return false; 2644 EligibilityResponse o = (EligibilityResponse) other_; 2645 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(disposition, o.disposition, true) 2646 && compareValues(inforce, o.inforce, true); 2647 } 2648 2649 public boolean isEmpty() { 2650 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, created 2651 , requestProvider, requestOrganization, request, outcome, disposition, insurer, inforce 2652 , insurance, form, error); 2653 } 2654 2655 @Override 2656 public ResourceType getResourceType() { 2657 return ResourceType.EligibilityResponse; 2658 } 2659 2660 /** 2661 * Search parameter: <b>identifier</b> 2662 * <p> 2663 * Description: <b>The business identifier</b><br> 2664 * Type: <b>token</b><br> 2665 * Path: <b>EligibilityResponse.identifier</b><br> 2666 * </p> 2667 */ 2668 @SearchParamDefinition(name="identifier", path="EligibilityResponse.identifier", description="The business identifier", type="token" ) 2669 public static final String SP_IDENTIFIER = "identifier"; 2670 /** 2671 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2672 * <p> 2673 * Description: <b>The business identifier</b><br> 2674 * Type: <b>token</b><br> 2675 * Path: <b>EligibilityResponse.identifier</b><br> 2676 * </p> 2677 */ 2678 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2679 2680 /** 2681 * Search parameter: <b>request</b> 2682 * <p> 2683 * Description: <b>The EligibilityRequest reference</b><br> 2684 * Type: <b>reference</b><br> 2685 * Path: <b>EligibilityResponse.request</b><br> 2686 * </p> 2687 */ 2688 @SearchParamDefinition(name="request", path="EligibilityResponse.request", description="The EligibilityRequest reference", type="reference", target={EligibilityRequest.class } ) 2689 public static final String SP_REQUEST = "request"; 2690 /** 2691 * <b>Fluent Client</b> search parameter constant for <b>request</b> 2692 * <p> 2693 * Description: <b>The EligibilityRequest reference</b><br> 2694 * Type: <b>reference</b><br> 2695 * Path: <b>EligibilityResponse.request</b><br> 2696 * </p> 2697 */ 2698 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 2699 2700/** 2701 * Constant for fluent queries to be used to add include statements. Specifies 2702 * the path value of "<b>EligibilityResponse:request</b>". 2703 */ 2704 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("EligibilityResponse:request").toLocked(); 2705 2706 /** 2707 * Search parameter: <b>disposition</b> 2708 * <p> 2709 * Description: <b>The contents of the disposition message</b><br> 2710 * Type: <b>string</b><br> 2711 * Path: <b>EligibilityResponse.disposition</b><br> 2712 * </p> 2713 */ 2714 @SearchParamDefinition(name="disposition", path="EligibilityResponse.disposition", description="The contents of the disposition message", type="string" ) 2715 public static final String SP_DISPOSITION = "disposition"; 2716 /** 2717 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 2718 * <p> 2719 * Description: <b>The contents of the disposition message</b><br> 2720 * Type: <b>string</b><br> 2721 * Path: <b>EligibilityResponse.disposition</b><br> 2722 * </p> 2723 */ 2724 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 2725 2726 /** 2727 * Search parameter: <b>insurer</b> 2728 * <p> 2729 * Description: <b>The organization which generated this resource</b><br> 2730 * Type: <b>reference</b><br> 2731 * Path: <b>EligibilityResponse.insurer</b><br> 2732 * </p> 2733 */ 2734 @SearchParamDefinition(name="insurer", path="EligibilityResponse.insurer", description="The organization which generated this resource", type="reference", target={Organization.class } ) 2735 public static final String SP_INSURER = "insurer"; 2736 /** 2737 * <b>Fluent Client</b> search parameter constant for <b>insurer</b> 2738 * <p> 2739 * Description: <b>The organization which generated this resource</b><br> 2740 * Type: <b>reference</b><br> 2741 * Path: <b>EligibilityResponse.insurer</b><br> 2742 * </p> 2743 */ 2744 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INSURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INSURER); 2745 2746/** 2747 * Constant for fluent queries to be used to add include statements. Specifies 2748 * the path value of "<b>EligibilityResponse:insurer</b>". 2749 */ 2750 public static final ca.uhn.fhir.model.api.Include INCLUDE_INSURER = new ca.uhn.fhir.model.api.Include("EligibilityResponse:insurer").toLocked(); 2751 2752 /** 2753 * Search parameter: <b>created</b> 2754 * <p> 2755 * Description: <b>The creation date</b><br> 2756 * Type: <b>date</b><br> 2757 * Path: <b>EligibilityResponse.created</b><br> 2758 * </p> 2759 */ 2760 @SearchParamDefinition(name="created", path="EligibilityResponse.created", description="The creation date", type="date" ) 2761 public static final String SP_CREATED = "created"; 2762 /** 2763 * <b>Fluent Client</b> search parameter constant for <b>created</b> 2764 * <p> 2765 * Description: <b>The creation date</b><br> 2766 * Type: <b>date</b><br> 2767 * Path: <b>EligibilityResponse.created</b><br> 2768 * </p> 2769 */ 2770 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 2771 2772 /** 2773 * Search parameter: <b>request-organization</b> 2774 * <p> 2775 * Description: <b>The EligibilityRequest organization</b><br> 2776 * Type: <b>reference</b><br> 2777 * Path: <b>EligibilityResponse.requestOrganization</b><br> 2778 * </p> 2779 */ 2780 @SearchParamDefinition(name="request-organization", path="EligibilityResponse.requestOrganization", description="The EligibilityRequest organization", type="reference", target={Organization.class } ) 2781 public static final String SP_REQUEST_ORGANIZATION = "request-organization"; 2782 /** 2783 * <b>Fluent Client</b> search parameter constant for <b>request-organization</b> 2784 * <p> 2785 * Description: <b>The EligibilityRequest organization</b><br> 2786 * Type: <b>reference</b><br> 2787 * Path: <b>EligibilityResponse.requestOrganization</b><br> 2788 * </p> 2789 */ 2790 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST_ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST_ORGANIZATION); 2791 2792/** 2793 * Constant for fluent queries to be used to add include statements. Specifies 2794 * the path value of "<b>EligibilityResponse:request-organization</b>". 2795 */ 2796 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST_ORGANIZATION = new ca.uhn.fhir.model.api.Include("EligibilityResponse:request-organization").toLocked(); 2797 2798 /** 2799 * Search parameter: <b>request-provider</b> 2800 * <p> 2801 * Description: <b>The EligibilityRequest provider</b><br> 2802 * Type: <b>reference</b><br> 2803 * Path: <b>EligibilityResponse.requestProvider</b><br> 2804 * </p> 2805 */ 2806 @SearchParamDefinition(name="request-provider", path="EligibilityResponse.requestProvider", description="The EligibilityRequest provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2807 public static final String SP_REQUEST_PROVIDER = "request-provider"; 2808 /** 2809 * <b>Fluent Client</b> search parameter constant for <b>request-provider</b> 2810 * <p> 2811 * Description: <b>The EligibilityRequest provider</b><br> 2812 * Type: <b>reference</b><br> 2813 * Path: <b>EligibilityResponse.requestProvider</b><br> 2814 * </p> 2815 */ 2816 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST_PROVIDER); 2817 2818/** 2819 * Constant for fluent queries to be used to add include statements. Specifies 2820 * the path value of "<b>EligibilityResponse:request-provider</b>". 2821 */ 2822 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST_PROVIDER = new ca.uhn.fhir.model.api.Include("EligibilityResponse:request-provider").toLocked(); 2823 2824 /** 2825 * Search parameter: <b>outcome</b> 2826 * <p> 2827 * Description: <b>The processing outcome</b><br> 2828 * Type: <b>token</b><br> 2829 * Path: <b>EligibilityResponse.outcome</b><br> 2830 * </p> 2831 */ 2832 @SearchParamDefinition(name="outcome", path="EligibilityResponse.outcome", description="The processing outcome", type="token" ) 2833 public static final String SP_OUTCOME = "outcome"; 2834 /** 2835 * <b>Fluent Client</b> search parameter constant for <b>outcome</b> 2836 * <p> 2837 * Description: <b>The processing outcome</b><br> 2838 * Type: <b>token</b><br> 2839 * Path: <b>EligibilityResponse.outcome</b><br> 2840 * </p> 2841 */ 2842 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OUTCOME = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OUTCOME); 2843 2844 2845}