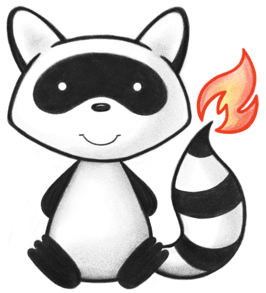
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient. 048 */ 049@ResourceDef(name="Encounter", profile="http://hl7.org/fhir/Profile/Encounter") 050public class Encounter extends DomainResource { 051 052 public enum EncounterStatus { 053 /** 054 * The Encounter has not yet started. 055 */ 056 PLANNED, 057 /** 058 * The Patient is present for the encounter, however is not currently meeting with a practitioner. 059 */ 060 ARRIVED, 061 /** 062 * The patient has been assessed for the priority of their treatment based on the severity of their condition. 063 */ 064 TRIAGED, 065 /** 066 * The Encounter has begun and the patient is present / the practitioner and the patient are meeting. 067 */ 068 INPROGRESS, 069 /** 070 * The Encounter has begun, but the patient is temporarily on leave. 071 */ 072 ONLEAVE, 073 /** 074 * The Encounter has ended. 075 */ 076 FINISHED, 077 /** 078 * The Encounter has ended before it has begun. 079 */ 080 CANCELLED, 081 /** 082 * This instance should not have been part of this patient's medical record. 083 */ 084 ENTEREDINERROR, 085 /** 086 * The encounter status is unknown. Note that "unknown" is a value of last resort and every attempt should be made to provide a meaningful value other than "unknown". 087 */ 088 UNKNOWN, 089 /** 090 * added to help the parsers with the generic types 091 */ 092 NULL; 093 public static EncounterStatus fromCode(String codeString) throws FHIRException { 094 if (codeString == null || "".equals(codeString)) 095 return null; 096 if ("planned".equals(codeString)) 097 return PLANNED; 098 if ("arrived".equals(codeString)) 099 return ARRIVED; 100 if ("triaged".equals(codeString)) 101 return TRIAGED; 102 if ("in-progress".equals(codeString)) 103 return INPROGRESS; 104 if ("onleave".equals(codeString)) 105 return ONLEAVE; 106 if ("finished".equals(codeString)) 107 return FINISHED; 108 if ("cancelled".equals(codeString)) 109 return CANCELLED; 110 if ("entered-in-error".equals(codeString)) 111 return ENTEREDINERROR; 112 if ("unknown".equals(codeString)) 113 return UNKNOWN; 114 if (Configuration.isAcceptInvalidEnums()) 115 return null; 116 else 117 throw new FHIRException("Unknown EncounterStatus code '"+codeString+"'"); 118 } 119 public String toCode() { 120 switch (this) { 121 case PLANNED: return "planned"; 122 case ARRIVED: return "arrived"; 123 case TRIAGED: return "triaged"; 124 case INPROGRESS: return "in-progress"; 125 case ONLEAVE: return "onleave"; 126 case FINISHED: return "finished"; 127 case CANCELLED: return "cancelled"; 128 case ENTEREDINERROR: return "entered-in-error"; 129 case UNKNOWN: return "unknown"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 public String getSystem() { 135 switch (this) { 136 case PLANNED: return "http://hl7.org/fhir/encounter-status"; 137 case ARRIVED: return "http://hl7.org/fhir/encounter-status"; 138 case TRIAGED: return "http://hl7.org/fhir/encounter-status"; 139 case INPROGRESS: return "http://hl7.org/fhir/encounter-status"; 140 case ONLEAVE: return "http://hl7.org/fhir/encounter-status"; 141 case FINISHED: return "http://hl7.org/fhir/encounter-status"; 142 case CANCELLED: return "http://hl7.org/fhir/encounter-status"; 143 case ENTEREDINERROR: return "http://hl7.org/fhir/encounter-status"; 144 case UNKNOWN: return "http://hl7.org/fhir/encounter-status"; 145 case NULL: return null; 146 default: return "?"; 147 } 148 } 149 public String getDefinition() { 150 switch (this) { 151 case PLANNED: return "The Encounter has not yet started."; 152 case ARRIVED: return "The Patient is present for the encounter, however is not currently meeting with a practitioner."; 153 case TRIAGED: return "The patient has been assessed for the priority of their treatment based on the severity of their condition."; 154 case INPROGRESS: return "The Encounter has begun and the patient is present / the practitioner and the patient are meeting."; 155 case ONLEAVE: return "The Encounter has begun, but the patient is temporarily on leave."; 156 case FINISHED: return "The Encounter has ended."; 157 case CANCELLED: return "The Encounter has ended before it has begun."; 158 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 159 case UNKNOWN: return "The encounter status is unknown. Note that \"unknown\" is a value of last resort and every attempt should be made to provide a meaningful value other than \"unknown\"."; 160 case NULL: return null; 161 default: return "?"; 162 } 163 } 164 public String getDisplay() { 165 switch (this) { 166 case PLANNED: return "Planned"; 167 case ARRIVED: return "Arrived"; 168 case TRIAGED: return "Triaged"; 169 case INPROGRESS: return "In Progress"; 170 case ONLEAVE: return "On Leave"; 171 case FINISHED: return "Finished"; 172 case CANCELLED: return "Cancelled"; 173 case ENTEREDINERROR: return "Entered in Error"; 174 case UNKNOWN: return "Unknown"; 175 case NULL: return null; 176 default: return "?"; 177 } 178 } 179 } 180 181 public static class EncounterStatusEnumFactory implements EnumFactory<EncounterStatus> { 182 public EncounterStatus fromCode(String codeString) throws IllegalArgumentException { 183 if (codeString == null || "".equals(codeString)) 184 if (codeString == null || "".equals(codeString)) 185 return null; 186 if ("planned".equals(codeString)) 187 return EncounterStatus.PLANNED; 188 if ("arrived".equals(codeString)) 189 return EncounterStatus.ARRIVED; 190 if ("triaged".equals(codeString)) 191 return EncounterStatus.TRIAGED; 192 if ("in-progress".equals(codeString)) 193 return EncounterStatus.INPROGRESS; 194 if ("onleave".equals(codeString)) 195 return EncounterStatus.ONLEAVE; 196 if ("finished".equals(codeString)) 197 return EncounterStatus.FINISHED; 198 if ("cancelled".equals(codeString)) 199 return EncounterStatus.CANCELLED; 200 if ("entered-in-error".equals(codeString)) 201 return EncounterStatus.ENTEREDINERROR; 202 if ("unknown".equals(codeString)) 203 return EncounterStatus.UNKNOWN; 204 throw new IllegalArgumentException("Unknown EncounterStatus code '"+codeString+"'"); 205 } 206 public Enumeration<EncounterStatus> fromType(PrimitiveType<?> code) throws FHIRException { 207 if (code == null) 208 return null; 209 if (code.isEmpty()) 210 return new Enumeration<EncounterStatus>(this); 211 String codeString = code.asStringValue(); 212 if (codeString == null || "".equals(codeString)) 213 return null; 214 if ("planned".equals(codeString)) 215 return new Enumeration<EncounterStatus>(this, EncounterStatus.PLANNED); 216 if ("arrived".equals(codeString)) 217 return new Enumeration<EncounterStatus>(this, EncounterStatus.ARRIVED); 218 if ("triaged".equals(codeString)) 219 return new Enumeration<EncounterStatus>(this, EncounterStatus.TRIAGED); 220 if ("in-progress".equals(codeString)) 221 return new Enumeration<EncounterStatus>(this, EncounterStatus.INPROGRESS); 222 if ("onleave".equals(codeString)) 223 return new Enumeration<EncounterStatus>(this, EncounterStatus.ONLEAVE); 224 if ("finished".equals(codeString)) 225 return new Enumeration<EncounterStatus>(this, EncounterStatus.FINISHED); 226 if ("cancelled".equals(codeString)) 227 return new Enumeration<EncounterStatus>(this, EncounterStatus.CANCELLED); 228 if ("entered-in-error".equals(codeString)) 229 return new Enumeration<EncounterStatus>(this, EncounterStatus.ENTEREDINERROR); 230 if ("unknown".equals(codeString)) 231 return new Enumeration<EncounterStatus>(this, EncounterStatus.UNKNOWN); 232 throw new FHIRException("Unknown EncounterStatus code '"+codeString+"'"); 233 } 234 public String toCode(EncounterStatus code) { 235 if (code == EncounterStatus.NULL) 236 return null; 237 if (code == EncounterStatus.PLANNED) 238 return "planned"; 239 if (code == EncounterStatus.ARRIVED) 240 return "arrived"; 241 if (code == EncounterStatus.TRIAGED) 242 return "triaged"; 243 if (code == EncounterStatus.INPROGRESS) 244 return "in-progress"; 245 if (code == EncounterStatus.ONLEAVE) 246 return "onleave"; 247 if (code == EncounterStatus.FINISHED) 248 return "finished"; 249 if (code == EncounterStatus.CANCELLED) 250 return "cancelled"; 251 if (code == EncounterStatus.ENTEREDINERROR) 252 return "entered-in-error"; 253 if (code == EncounterStatus.UNKNOWN) 254 return "unknown"; 255 return "?"; 256 } 257 public String toSystem(EncounterStatus code) { 258 return code.getSystem(); 259 } 260 } 261 262 public enum EncounterLocationStatus { 263 /** 264 * The patient is planned to be moved to this location at some point in the future. 265 */ 266 PLANNED, 267 /** 268 * The patient is currently at this location, or was between the period specified. 269 270A system may update these records when the patient leaves the location to either reserved, or completed 271 */ 272 ACTIVE, 273 /** 274 * This location is held empty for this patient. 275 */ 276 RESERVED, 277 /** 278 * The patient was at this location during the period specified. 279 280Not to be used when the patient is currently at the location 281 */ 282 COMPLETED, 283 /** 284 * added to help the parsers with the generic types 285 */ 286 NULL; 287 public static EncounterLocationStatus fromCode(String codeString) throws FHIRException { 288 if (codeString == null || "".equals(codeString)) 289 return null; 290 if ("planned".equals(codeString)) 291 return PLANNED; 292 if ("active".equals(codeString)) 293 return ACTIVE; 294 if ("reserved".equals(codeString)) 295 return RESERVED; 296 if ("completed".equals(codeString)) 297 return COMPLETED; 298 if (Configuration.isAcceptInvalidEnums()) 299 return null; 300 else 301 throw new FHIRException("Unknown EncounterLocationStatus code '"+codeString+"'"); 302 } 303 public String toCode() { 304 switch (this) { 305 case PLANNED: return "planned"; 306 case ACTIVE: return "active"; 307 case RESERVED: return "reserved"; 308 case COMPLETED: return "completed"; 309 case NULL: return null; 310 default: return "?"; 311 } 312 } 313 public String getSystem() { 314 switch (this) { 315 case PLANNED: return "http://hl7.org/fhir/encounter-location-status"; 316 case ACTIVE: return "http://hl7.org/fhir/encounter-location-status"; 317 case RESERVED: return "http://hl7.org/fhir/encounter-location-status"; 318 case COMPLETED: return "http://hl7.org/fhir/encounter-location-status"; 319 case NULL: return null; 320 default: return "?"; 321 } 322 } 323 public String getDefinition() { 324 switch (this) { 325 case PLANNED: return "The patient is planned to be moved to this location at some point in the future."; 326 case ACTIVE: return "The patient is currently at this location, or was between the period specified.\r\rA system may update these records when the patient leaves the location to either reserved, or completed"; 327 case RESERVED: return "This location is held empty for this patient."; 328 case COMPLETED: return "The patient was at this location during the period specified.\r\rNot to be used when the patient is currently at the location"; 329 case NULL: return null; 330 default: return "?"; 331 } 332 } 333 public String getDisplay() { 334 switch (this) { 335 case PLANNED: return "Planned"; 336 case ACTIVE: return "Active"; 337 case RESERVED: return "Reserved"; 338 case COMPLETED: return "Completed"; 339 case NULL: return null; 340 default: return "?"; 341 } 342 } 343 } 344 345 public static class EncounterLocationStatusEnumFactory implements EnumFactory<EncounterLocationStatus> { 346 public EncounterLocationStatus fromCode(String codeString) throws IllegalArgumentException { 347 if (codeString == null || "".equals(codeString)) 348 if (codeString == null || "".equals(codeString)) 349 return null; 350 if ("planned".equals(codeString)) 351 return EncounterLocationStatus.PLANNED; 352 if ("active".equals(codeString)) 353 return EncounterLocationStatus.ACTIVE; 354 if ("reserved".equals(codeString)) 355 return EncounterLocationStatus.RESERVED; 356 if ("completed".equals(codeString)) 357 return EncounterLocationStatus.COMPLETED; 358 throw new IllegalArgumentException("Unknown EncounterLocationStatus code '"+codeString+"'"); 359 } 360 public Enumeration<EncounterLocationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 361 if (code == null) 362 return null; 363 if (code.isEmpty()) 364 return new Enumeration<EncounterLocationStatus>(this); 365 String codeString = code.asStringValue(); 366 if (codeString == null || "".equals(codeString)) 367 return null; 368 if ("planned".equals(codeString)) 369 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.PLANNED); 370 if ("active".equals(codeString)) 371 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.ACTIVE); 372 if ("reserved".equals(codeString)) 373 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.RESERVED); 374 if ("completed".equals(codeString)) 375 return new Enumeration<EncounterLocationStatus>(this, EncounterLocationStatus.COMPLETED); 376 throw new FHIRException("Unknown EncounterLocationStatus code '"+codeString+"'"); 377 } 378 public String toCode(EncounterLocationStatus code) { 379 if (code == EncounterLocationStatus.NULL) 380 return null; 381 if (code == EncounterLocationStatus.PLANNED) 382 return "planned"; 383 if (code == EncounterLocationStatus.ACTIVE) 384 return "active"; 385 if (code == EncounterLocationStatus.RESERVED) 386 return "reserved"; 387 if (code == EncounterLocationStatus.COMPLETED) 388 return "completed"; 389 return "?"; 390 } 391 public String toSystem(EncounterLocationStatus code) { 392 return code.getSystem(); 393 } 394 } 395 396 @Block() 397 public static class StatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 398 /** 399 * planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 400 */ 401 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 402 @Description(shortDefinition="planned | arrived | triaged | in-progress | onleave | finished | cancelled +", formalDefinition="planned | arrived | triaged | in-progress | onleave | finished | cancelled +." ) 403 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-status") 404 protected Enumeration<EncounterStatus> status; 405 406 /** 407 * The time that the episode was in the specified status. 408 */ 409 @Child(name = "period", type = {Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 410 @Description(shortDefinition="The time that the episode was in the specified status", formalDefinition="The time that the episode was in the specified status." ) 411 protected Period period; 412 413 private static final long serialVersionUID = -1893906736L; 414 415 /** 416 * Constructor 417 */ 418 public StatusHistoryComponent() { 419 super(); 420 } 421 422 /** 423 * Constructor 424 */ 425 public StatusHistoryComponent(Enumeration<EncounterStatus> status, Period period) { 426 super(); 427 this.status = status; 428 this.period = period; 429 } 430 431 /** 432 * @return {@link #status} (planned | arrived | triaged | in-progress | onleave | finished | cancelled +.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 433 */ 434 public Enumeration<EncounterStatus> getStatusElement() { 435 if (this.status == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create StatusHistoryComponent.status"); 438 else if (Configuration.doAutoCreate()) 439 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); // bb 440 return this.status; 441 } 442 443 public boolean hasStatusElement() { 444 return this.status != null && !this.status.isEmpty(); 445 } 446 447 public boolean hasStatus() { 448 return this.status != null && !this.status.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #status} (planned | arrived | triaged | in-progress | onleave | finished | cancelled +.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 453 */ 454 public StatusHistoryComponent setStatusElement(Enumeration<EncounterStatus> value) { 455 this.status = value; 456 return this; 457 } 458 459 /** 460 * @return planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 461 */ 462 public EncounterStatus getStatus() { 463 return this.status == null ? null : this.status.getValue(); 464 } 465 466 /** 467 * @param value planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 468 */ 469 public StatusHistoryComponent setStatus(EncounterStatus value) { 470 if (this.status == null) 471 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); 472 this.status.setValue(value); 473 return this; 474 } 475 476 /** 477 * @return {@link #period} (The time that the episode was in the specified status.) 478 */ 479 public Period getPeriod() { 480 if (this.period == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create StatusHistoryComponent.period"); 483 else if (Configuration.doAutoCreate()) 484 this.period = new Period(); // cc 485 return this.period; 486 } 487 488 public boolean hasPeriod() { 489 return this.period != null && !this.period.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #period} (The time that the episode was in the specified status.) 494 */ 495 public StatusHistoryComponent setPeriod(Period value) { 496 this.period = value; 497 return this; 498 } 499 500 protected void listChildren(List<Property> children) { 501 super.listChildren(children); 502 children.add(new Property("status", "code", "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status)); 503 children.add(new Property("period", "Period", "The time that the episode was in the specified status.", 0, 1, period)); 504 } 505 506 @Override 507 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 508 switch (_hash) { 509 case -892481550: /*status*/ return new Property("status", "code", "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status); 510 case -991726143: /*period*/ return new Property("period", "Period", "The time that the episode was in the specified status.", 0, 1, period); 511 default: return super.getNamedProperty(_hash, _name, _checkValid); 512 } 513 514 } 515 516 @Override 517 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 518 switch (hash) { 519 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EncounterStatus> 520 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 521 default: return super.getProperty(hash, name, checkValid); 522 } 523 524 } 525 526 @Override 527 public Base setProperty(int hash, String name, Base value) throws FHIRException { 528 switch (hash) { 529 case -892481550: // status 530 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 531 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 532 return value; 533 case -991726143: // period 534 this.period = castToPeriod(value); // Period 535 return value; 536 default: return super.setProperty(hash, name, value); 537 } 538 539 } 540 541 @Override 542 public Base setProperty(String name, Base value) throws FHIRException { 543 if (name.equals("status")) { 544 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 545 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 546 } else if (name.equals("period")) { 547 this.period = castToPeriod(value); // Period 548 } else 549 return super.setProperty(name, value); 550 return value; 551 } 552 553 @Override 554 public Base makeProperty(int hash, String name) throws FHIRException { 555 switch (hash) { 556 case -892481550: return getStatusElement(); 557 case -991726143: return getPeriod(); 558 default: return super.makeProperty(hash, name); 559 } 560 561 } 562 563 @Override 564 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 565 switch (hash) { 566 case -892481550: /*status*/ return new String[] {"code"}; 567 case -991726143: /*period*/ return new String[] {"Period"}; 568 default: return super.getTypesForProperty(hash, name); 569 } 570 571 } 572 573 @Override 574 public Base addChild(String name) throws FHIRException { 575 if (name.equals("status")) { 576 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 577 } 578 else if (name.equals("period")) { 579 this.period = new Period(); 580 return this.period; 581 } 582 else 583 return super.addChild(name); 584 } 585 586 public StatusHistoryComponent copy() { 587 StatusHistoryComponent dst = new StatusHistoryComponent(); 588 copyValues(dst); 589 dst.status = status == null ? null : status.copy(); 590 dst.period = period == null ? null : period.copy(); 591 return dst; 592 } 593 594 @Override 595 public boolean equalsDeep(Base other_) { 596 if (!super.equalsDeep(other_)) 597 return false; 598 if (!(other_ instanceof StatusHistoryComponent)) 599 return false; 600 StatusHistoryComponent o = (StatusHistoryComponent) other_; 601 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 602 } 603 604 @Override 605 public boolean equalsShallow(Base other_) { 606 if (!super.equalsShallow(other_)) 607 return false; 608 if (!(other_ instanceof StatusHistoryComponent)) 609 return false; 610 StatusHistoryComponent o = (StatusHistoryComponent) other_; 611 return compareValues(status, o.status, true); 612 } 613 614 public boolean isEmpty() { 615 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, period); 616 } 617 618 public String fhirType() { 619 return "Encounter.statusHistory"; 620 621 } 622 623 } 624 625 @Block() 626 public static class ClassHistoryComponent extends BackboneElement implements IBaseBackboneElement { 627 /** 628 * inpatient | outpatient | ambulatory | emergency +. 629 */ 630 @Child(name = "class", type = {Coding.class}, order=1, min=1, max=1, modifier=false, summary=false) 631 @Description(shortDefinition="inpatient | outpatient | ambulatory | emergency +", formalDefinition="inpatient | outpatient | ambulatory | emergency +." ) 632 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActEncounterCode") 633 protected Coding class_; 634 635 /** 636 * The time that the episode was in the specified class. 637 */ 638 @Child(name = "period", type = {Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 639 @Description(shortDefinition="The time that the episode was in the specified class", formalDefinition="The time that the episode was in the specified class." ) 640 protected Period period; 641 642 private static final long serialVersionUID = 1331020311L; 643 644 /** 645 * Constructor 646 */ 647 public ClassHistoryComponent() { 648 super(); 649 } 650 651 /** 652 * Constructor 653 */ 654 public ClassHistoryComponent(Coding class_, Period period) { 655 super(); 656 this.class_ = class_; 657 this.period = period; 658 } 659 660 /** 661 * @return {@link #class_} (inpatient | outpatient | ambulatory | emergency +.) 662 */ 663 public Coding getClass_() { 664 if (this.class_ == null) 665 if (Configuration.errorOnAutoCreate()) 666 throw new Error("Attempt to auto-create ClassHistoryComponent.class_"); 667 else if (Configuration.doAutoCreate()) 668 this.class_ = new Coding(); // cc 669 return this.class_; 670 } 671 672 public boolean hasClass_() { 673 return this.class_ != null && !this.class_.isEmpty(); 674 } 675 676 /** 677 * @param value {@link #class_} (inpatient | outpatient | ambulatory | emergency +.) 678 */ 679 public ClassHistoryComponent setClass_(Coding value) { 680 this.class_ = value; 681 return this; 682 } 683 684 /** 685 * @return {@link #period} (The time that the episode was in the specified class.) 686 */ 687 public Period getPeriod() { 688 if (this.period == null) 689 if (Configuration.errorOnAutoCreate()) 690 throw new Error("Attempt to auto-create ClassHistoryComponent.period"); 691 else if (Configuration.doAutoCreate()) 692 this.period = new Period(); // cc 693 return this.period; 694 } 695 696 public boolean hasPeriod() { 697 return this.period != null && !this.period.isEmpty(); 698 } 699 700 /** 701 * @param value {@link #period} (The time that the episode was in the specified class.) 702 */ 703 public ClassHistoryComponent setPeriod(Period value) { 704 this.period = value; 705 return this; 706 } 707 708 protected void listChildren(List<Property> children) { 709 super.listChildren(children); 710 children.add(new Property("class", "Coding", "inpatient | outpatient | ambulatory | emergency +.", 0, 1, class_)); 711 children.add(new Property("period", "Period", "The time that the episode was in the specified class.", 0, 1, period)); 712 } 713 714 @Override 715 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 716 switch (_hash) { 717 case 94742904: /*class*/ return new Property("class", "Coding", "inpatient | outpatient | ambulatory | emergency +.", 0, 1, class_); 718 case -991726143: /*period*/ return new Property("period", "Period", "The time that the episode was in the specified class.", 0, 1, period); 719 default: return super.getNamedProperty(_hash, _name, _checkValid); 720 } 721 722 } 723 724 @Override 725 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 726 switch (hash) { 727 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // Coding 728 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 729 default: return super.getProperty(hash, name, checkValid); 730 } 731 732 } 733 734 @Override 735 public Base setProperty(int hash, String name, Base value) throws FHIRException { 736 switch (hash) { 737 case 94742904: // class 738 this.class_ = castToCoding(value); // Coding 739 return value; 740 case -991726143: // period 741 this.period = castToPeriod(value); // Period 742 return value; 743 default: return super.setProperty(hash, name, value); 744 } 745 746 } 747 748 @Override 749 public Base setProperty(String name, Base value) throws FHIRException { 750 if (name.equals("class")) { 751 this.class_ = castToCoding(value); // Coding 752 } else if (name.equals("period")) { 753 this.period = castToPeriod(value); // Period 754 } else 755 return super.setProperty(name, value); 756 return value; 757 } 758 759 @Override 760 public Base makeProperty(int hash, String name) throws FHIRException { 761 switch (hash) { 762 case 94742904: return getClass_(); 763 case -991726143: return getPeriod(); 764 default: return super.makeProperty(hash, name); 765 } 766 767 } 768 769 @Override 770 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 771 switch (hash) { 772 case 94742904: /*class*/ return new String[] {"Coding"}; 773 case -991726143: /*period*/ return new String[] {"Period"}; 774 default: return super.getTypesForProperty(hash, name); 775 } 776 777 } 778 779 @Override 780 public Base addChild(String name) throws FHIRException { 781 if (name.equals("class")) { 782 this.class_ = new Coding(); 783 return this.class_; 784 } 785 else if (name.equals("period")) { 786 this.period = new Period(); 787 return this.period; 788 } 789 else 790 return super.addChild(name); 791 } 792 793 public ClassHistoryComponent copy() { 794 ClassHistoryComponent dst = new ClassHistoryComponent(); 795 copyValues(dst); 796 dst.class_ = class_ == null ? null : class_.copy(); 797 dst.period = period == null ? null : period.copy(); 798 return dst; 799 } 800 801 @Override 802 public boolean equalsDeep(Base other_) { 803 if (!super.equalsDeep(other_)) 804 return false; 805 if (!(other_ instanceof ClassHistoryComponent)) 806 return false; 807 ClassHistoryComponent o = (ClassHistoryComponent) other_; 808 return compareDeep(class_, o.class_, true) && compareDeep(period, o.period, true); 809 } 810 811 @Override 812 public boolean equalsShallow(Base other_) { 813 if (!super.equalsShallow(other_)) 814 return false; 815 if (!(other_ instanceof ClassHistoryComponent)) 816 return false; 817 ClassHistoryComponent o = (ClassHistoryComponent) other_; 818 return true; 819 } 820 821 public boolean isEmpty() { 822 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(class_, period); 823 } 824 825 public String fhirType() { 826 return "Encounter.classHistory"; 827 828 } 829 830 } 831 832 @Block() 833 public static class EncounterParticipantComponent extends BackboneElement implements IBaseBackboneElement { 834 /** 835 * Role of participant in encounter. 836 */ 837 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 838 @Description(shortDefinition="Role of participant in encounter", formalDefinition="Role of participant in encounter." ) 839 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-participant-type") 840 protected List<CodeableConcept> type; 841 842 /** 843 * The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period. 844 */ 845 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 846 @Description(shortDefinition="Period of time during the encounter that the participant participated", formalDefinition="The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period." ) 847 protected Period period; 848 849 /** 850 * Persons involved in the encounter other than the patient. 851 */ 852 @Child(name = "individual", type = {Practitioner.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=true) 853 @Description(shortDefinition="Persons involved in the encounter other than the patient", formalDefinition="Persons involved in the encounter other than the patient." ) 854 protected Reference individual; 855 856 /** 857 * The actual object that is the target of the reference (Persons involved in the encounter other than the patient.) 858 */ 859 protected Resource individualTarget; 860 861 private static final long serialVersionUID = 317095765L; 862 863 /** 864 * Constructor 865 */ 866 public EncounterParticipantComponent() { 867 super(); 868 } 869 870 /** 871 * @return {@link #type} (Role of participant in encounter.) 872 */ 873 public List<CodeableConcept> getType() { 874 if (this.type == null) 875 this.type = new ArrayList<CodeableConcept>(); 876 return this.type; 877 } 878 879 /** 880 * @return Returns a reference to <code>this</code> for easy method chaining 881 */ 882 public EncounterParticipantComponent setType(List<CodeableConcept> theType) { 883 this.type = theType; 884 return this; 885 } 886 887 public boolean hasType() { 888 if (this.type == null) 889 return false; 890 for (CodeableConcept item : this.type) 891 if (!item.isEmpty()) 892 return true; 893 return false; 894 } 895 896 public CodeableConcept addType() { //3 897 CodeableConcept t = new CodeableConcept(); 898 if (this.type == null) 899 this.type = new ArrayList<CodeableConcept>(); 900 this.type.add(t); 901 return t; 902 } 903 904 public EncounterParticipantComponent addType(CodeableConcept t) { //3 905 if (t == null) 906 return this; 907 if (this.type == null) 908 this.type = new ArrayList<CodeableConcept>(); 909 this.type.add(t); 910 return this; 911 } 912 913 /** 914 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 915 */ 916 public CodeableConcept getTypeFirstRep() { 917 if (getType().isEmpty()) { 918 addType(); 919 } 920 return getType().get(0); 921 } 922 923 /** 924 * @return {@link #period} (The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.) 925 */ 926 public Period getPeriod() { 927 if (this.period == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create EncounterParticipantComponent.period"); 930 else if (Configuration.doAutoCreate()) 931 this.period = new Period(); // cc 932 return this.period; 933 } 934 935 public boolean hasPeriod() { 936 return this.period != null && !this.period.isEmpty(); 937 } 938 939 /** 940 * @param value {@link #period} (The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.) 941 */ 942 public EncounterParticipantComponent setPeriod(Period value) { 943 this.period = value; 944 return this; 945 } 946 947 /** 948 * @return {@link #individual} (Persons involved in the encounter other than the patient.) 949 */ 950 public Reference getIndividual() { 951 if (this.individual == null) 952 if (Configuration.errorOnAutoCreate()) 953 throw new Error("Attempt to auto-create EncounterParticipantComponent.individual"); 954 else if (Configuration.doAutoCreate()) 955 this.individual = new Reference(); // cc 956 return this.individual; 957 } 958 959 public boolean hasIndividual() { 960 return this.individual != null && !this.individual.isEmpty(); 961 } 962 963 /** 964 * @param value {@link #individual} (Persons involved in the encounter other than the patient.) 965 */ 966 public EncounterParticipantComponent setIndividual(Reference value) { 967 this.individual = value; 968 return this; 969 } 970 971 /** 972 * @return {@link #individual} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Persons involved in the encounter other than the patient.) 973 */ 974 public Resource getIndividualTarget() { 975 return this.individualTarget; 976 } 977 978 /** 979 * @param value {@link #individual} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Persons involved in the encounter other than the patient.) 980 */ 981 public EncounterParticipantComponent setIndividualTarget(Resource value) { 982 this.individualTarget = value; 983 return this; 984 } 985 986 protected void listChildren(List<Property> children) { 987 super.listChildren(children); 988 children.add(new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, java.lang.Integer.MAX_VALUE, type)); 989 children.add(new Property("period", "Period", "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.", 0, 1, period)); 990 children.add(new Property("individual", "Reference(Practitioner|RelatedPerson)", "Persons involved in the encounter other than the patient.", 0, 1, individual)); 991 } 992 993 @Override 994 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 995 switch (_hash) { 996 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Role of participant in encounter.", 0, java.lang.Integer.MAX_VALUE, type); 997 case -991726143: /*period*/ return new Property("period", "Period", "The period of time that the specified participant participated in the encounter. These can overlap or be sub-sets of the overall encounter's period.", 0, 1, period); 998 case -46292327: /*individual*/ return new Property("individual", "Reference(Practitioner|RelatedPerson)", "Persons involved in the encounter other than the patient.", 0, 1, individual); 999 default: return super.getNamedProperty(_hash, _name, _checkValid); 1000 } 1001 1002 } 1003 1004 @Override 1005 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1006 switch (hash) { 1007 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1008 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1009 case -46292327: /*individual*/ return this.individual == null ? new Base[0] : new Base[] {this.individual}; // Reference 1010 default: return super.getProperty(hash, name, checkValid); 1011 } 1012 1013 } 1014 1015 @Override 1016 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1017 switch (hash) { 1018 case 3575610: // type 1019 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1020 return value; 1021 case -991726143: // period 1022 this.period = castToPeriod(value); // Period 1023 return value; 1024 case -46292327: // individual 1025 this.individual = castToReference(value); // Reference 1026 return value; 1027 default: return super.setProperty(hash, name, value); 1028 } 1029 1030 } 1031 1032 @Override 1033 public Base setProperty(String name, Base value) throws FHIRException { 1034 if (name.equals("type")) { 1035 this.getType().add(castToCodeableConcept(value)); 1036 } else if (name.equals("period")) { 1037 this.period = castToPeriod(value); // Period 1038 } else if (name.equals("individual")) { 1039 this.individual = castToReference(value); // Reference 1040 } else 1041 return super.setProperty(name, value); 1042 return value; 1043 } 1044 1045 @Override 1046 public Base makeProperty(int hash, String name) throws FHIRException { 1047 switch (hash) { 1048 case 3575610: return addType(); 1049 case -991726143: return getPeriod(); 1050 case -46292327: return getIndividual(); 1051 default: return super.makeProperty(hash, name); 1052 } 1053 1054 } 1055 1056 @Override 1057 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1058 switch (hash) { 1059 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1060 case -991726143: /*period*/ return new String[] {"Period"}; 1061 case -46292327: /*individual*/ return new String[] {"Reference"}; 1062 default: return super.getTypesForProperty(hash, name); 1063 } 1064 1065 } 1066 1067 @Override 1068 public Base addChild(String name) throws FHIRException { 1069 if (name.equals("type")) { 1070 return addType(); 1071 } 1072 else if (name.equals("period")) { 1073 this.period = new Period(); 1074 return this.period; 1075 } 1076 else if (name.equals("individual")) { 1077 this.individual = new Reference(); 1078 return this.individual; 1079 } 1080 else 1081 return super.addChild(name); 1082 } 1083 1084 public EncounterParticipantComponent copy() { 1085 EncounterParticipantComponent dst = new EncounterParticipantComponent(); 1086 copyValues(dst); 1087 if (type != null) { 1088 dst.type = new ArrayList<CodeableConcept>(); 1089 for (CodeableConcept i : type) 1090 dst.type.add(i.copy()); 1091 }; 1092 dst.period = period == null ? null : period.copy(); 1093 dst.individual = individual == null ? null : individual.copy(); 1094 return dst; 1095 } 1096 1097 @Override 1098 public boolean equalsDeep(Base other_) { 1099 if (!super.equalsDeep(other_)) 1100 return false; 1101 if (!(other_ instanceof EncounterParticipantComponent)) 1102 return false; 1103 EncounterParticipantComponent o = (EncounterParticipantComponent) other_; 1104 return compareDeep(type, o.type, true) && compareDeep(period, o.period, true) && compareDeep(individual, o.individual, true) 1105 ; 1106 } 1107 1108 @Override 1109 public boolean equalsShallow(Base other_) { 1110 if (!super.equalsShallow(other_)) 1111 return false; 1112 if (!(other_ instanceof EncounterParticipantComponent)) 1113 return false; 1114 EncounterParticipantComponent o = (EncounterParticipantComponent) other_; 1115 return true; 1116 } 1117 1118 public boolean isEmpty() { 1119 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, period, individual 1120 ); 1121 } 1122 1123 public String fhirType() { 1124 return "Encounter.participant"; 1125 1126 } 1127 1128 } 1129 1130 @Block() 1131 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1132 /** 1133 * Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure. 1134 */ 1135 @Child(name = "condition", type = {Condition.class, Procedure.class}, order=1, min=1, max=1, modifier=false, summary=false) 1136 @Description(shortDefinition="Reason the encounter takes place (resource)", formalDefinition="Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure." ) 1137 protected Reference condition; 1138 1139 /** 1140 * The actual object that is the target of the reference (Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.) 1141 */ 1142 protected Resource conditionTarget; 1143 1144 /** 1145 * Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?). 1146 */ 1147 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1148 @Description(shortDefinition="Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?)", formalDefinition="Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?)." ) 1149 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnosis-role") 1150 protected CodeableConcept role; 1151 1152 /** 1153 * Ranking of the diagnosis (for each role type). 1154 */ 1155 @Child(name = "rank", type = {PositiveIntType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1156 @Description(shortDefinition="Ranking of the diagnosis (for each role type)", formalDefinition="Ranking of the diagnosis (for each role type)." ) 1157 protected PositiveIntType rank; 1158 1159 private static final long serialVersionUID = 1005913665L; 1160 1161 /** 1162 * Constructor 1163 */ 1164 public DiagnosisComponent() { 1165 super(); 1166 } 1167 1168 /** 1169 * Constructor 1170 */ 1171 public DiagnosisComponent(Reference condition) { 1172 super(); 1173 this.condition = condition; 1174 } 1175 1176 /** 1177 * @return {@link #condition} (Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.) 1178 */ 1179 public Reference getCondition() { 1180 if (this.condition == null) 1181 if (Configuration.errorOnAutoCreate()) 1182 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 1183 else if (Configuration.doAutoCreate()) 1184 this.condition = new Reference(); // cc 1185 return this.condition; 1186 } 1187 1188 public boolean hasCondition() { 1189 return this.condition != null && !this.condition.isEmpty(); 1190 } 1191 1192 /** 1193 * @param value {@link #condition} (Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.) 1194 */ 1195 public DiagnosisComponent setCondition(Reference value) { 1196 this.condition = value; 1197 return this; 1198 } 1199 1200 /** 1201 * @return {@link #condition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.) 1202 */ 1203 public Resource getConditionTarget() { 1204 return this.conditionTarget; 1205 } 1206 1207 /** 1208 * @param value {@link #condition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.) 1209 */ 1210 public DiagnosisComponent setConditionTarget(Resource value) { 1211 this.conditionTarget = value; 1212 return this; 1213 } 1214 1215 /** 1216 * @return {@link #role} (Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).) 1217 */ 1218 public CodeableConcept getRole() { 1219 if (this.role == null) 1220 if (Configuration.errorOnAutoCreate()) 1221 throw new Error("Attempt to auto-create DiagnosisComponent.role"); 1222 else if (Configuration.doAutoCreate()) 1223 this.role = new CodeableConcept(); // cc 1224 return this.role; 1225 } 1226 1227 public boolean hasRole() { 1228 return this.role != null && !this.role.isEmpty(); 1229 } 1230 1231 /** 1232 * @param value {@link #role} (Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).) 1233 */ 1234 public DiagnosisComponent setRole(CodeableConcept value) { 1235 this.role = value; 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #rank} (Ranking of the diagnosis (for each role type).). This is the underlying object with id, value and extensions. The accessor "getRank" gives direct access to the value 1241 */ 1242 public PositiveIntType getRankElement() { 1243 if (this.rank == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create DiagnosisComponent.rank"); 1246 else if (Configuration.doAutoCreate()) 1247 this.rank = new PositiveIntType(); // bb 1248 return this.rank; 1249 } 1250 1251 public boolean hasRankElement() { 1252 return this.rank != null && !this.rank.isEmpty(); 1253 } 1254 1255 public boolean hasRank() { 1256 return this.rank != null && !this.rank.isEmpty(); 1257 } 1258 1259 /** 1260 * @param value {@link #rank} (Ranking of the diagnosis (for each role type).). This is the underlying object with id, value and extensions. The accessor "getRank" gives direct access to the value 1261 */ 1262 public DiagnosisComponent setRankElement(PositiveIntType value) { 1263 this.rank = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return Ranking of the diagnosis (for each role type). 1269 */ 1270 public int getRank() { 1271 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 1272 } 1273 1274 /** 1275 * @param value Ranking of the diagnosis (for each role type). 1276 */ 1277 public DiagnosisComponent setRank(int value) { 1278 if (this.rank == null) 1279 this.rank = new PositiveIntType(); 1280 this.rank.setValue(value); 1281 return this; 1282 } 1283 1284 protected void listChildren(List<Property> children) { 1285 super.listChildren(children); 1286 children.add(new Property("condition", "Reference(Condition|Procedure)", "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 0, 1, condition)); 1287 children.add(new Property("role", "CodeableConcept", "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).", 0, 1, role)); 1288 children.add(new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, rank)); 1289 } 1290 1291 @Override 1292 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1293 switch (_hash) { 1294 case -861311717: /*condition*/ return new Property("condition", "Reference(Condition|Procedure)", "Reason the encounter takes place, as specified using information from another resource. For admissions, this is the admission diagnosis. The indication will typically be a Condition (with other resources referenced in the evidence.detail), or a Procedure.", 0, 1, condition); 1295 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Role that this diagnosis has within the encounter (e.g. admission, billing, discharge ?).", 0, 1, role); 1296 case 3492908: /*rank*/ return new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, rank); 1297 default: return super.getNamedProperty(_hash, _name, _checkValid); 1298 } 1299 1300 } 1301 1302 @Override 1303 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1304 switch (hash) { 1305 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // Reference 1306 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1307 case 3492908: /*rank*/ return this.rank == null ? new Base[0] : new Base[] {this.rank}; // PositiveIntType 1308 default: return super.getProperty(hash, name, checkValid); 1309 } 1310 1311 } 1312 1313 @Override 1314 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1315 switch (hash) { 1316 case -861311717: // condition 1317 this.condition = castToReference(value); // Reference 1318 return value; 1319 case 3506294: // role 1320 this.role = castToCodeableConcept(value); // CodeableConcept 1321 return value; 1322 case 3492908: // rank 1323 this.rank = castToPositiveInt(value); // PositiveIntType 1324 return value; 1325 default: return super.setProperty(hash, name, value); 1326 } 1327 1328 } 1329 1330 @Override 1331 public Base setProperty(String name, Base value) throws FHIRException { 1332 if (name.equals("condition")) { 1333 this.condition = castToReference(value); // Reference 1334 } else if (name.equals("role")) { 1335 this.role = castToCodeableConcept(value); // CodeableConcept 1336 } else if (name.equals("rank")) { 1337 this.rank = castToPositiveInt(value); // PositiveIntType 1338 } else 1339 return super.setProperty(name, value); 1340 return value; 1341 } 1342 1343 @Override 1344 public Base makeProperty(int hash, String name) throws FHIRException { 1345 switch (hash) { 1346 case -861311717: return getCondition(); 1347 case 3506294: return getRole(); 1348 case 3492908: return getRankElement(); 1349 default: return super.makeProperty(hash, name); 1350 } 1351 1352 } 1353 1354 @Override 1355 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1356 switch (hash) { 1357 case -861311717: /*condition*/ return new String[] {"Reference"}; 1358 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1359 case 3492908: /*rank*/ return new String[] {"positiveInt"}; 1360 default: return super.getTypesForProperty(hash, name); 1361 } 1362 1363 } 1364 1365 @Override 1366 public Base addChild(String name) throws FHIRException { 1367 if (name.equals("condition")) { 1368 this.condition = new Reference(); 1369 return this.condition; 1370 } 1371 else if (name.equals("role")) { 1372 this.role = new CodeableConcept(); 1373 return this.role; 1374 } 1375 else if (name.equals("rank")) { 1376 throw new FHIRException("Cannot call addChild on a singleton property Encounter.rank"); 1377 } 1378 else 1379 return super.addChild(name); 1380 } 1381 1382 public DiagnosisComponent copy() { 1383 DiagnosisComponent dst = new DiagnosisComponent(); 1384 copyValues(dst); 1385 dst.condition = condition == null ? null : condition.copy(); 1386 dst.role = role == null ? null : role.copy(); 1387 dst.rank = rank == null ? null : rank.copy(); 1388 return dst; 1389 } 1390 1391 @Override 1392 public boolean equalsDeep(Base other_) { 1393 if (!super.equalsDeep(other_)) 1394 return false; 1395 if (!(other_ instanceof DiagnosisComponent)) 1396 return false; 1397 DiagnosisComponent o = (DiagnosisComponent) other_; 1398 return compareDeep(condition, o.condition, true) && compareDeep(role, o.role, true) && compareDeep(rank, o.rank, true) 1399 ; 1400 } 1401 1402 @Override 1403 public boolean equalsShallow(Base other_) { 1404 if (!super.equalsShallow(other_)) 1405 return false; 1406 if (!(other_ instanceof DiagnosisComponent)) 1407 return false; 1408 DiagnosisComponent o = (DiagnosisComponent) other_; 1409 return compareValues(rank, o.rank, true); 1410 } 1411 1412 public boolean isEmpty() { 1413 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, role, rank); 1414 } 1415 1416 public String fhirType() { 1417 return "Encounter.diagnosis"; 1418 1419 } 1420 1421 } 1422 1423 @Block() 1424 public static class EncounterHospitalizationComponent extends BackboneElement implements IBaseBackboneElement { 1425 /** 1426 * Pre-admission identifier. 1427 */ 1428 @Child(name = "preAdmissionIdentifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=false) 1429 @Description(shortDefinition="Pre-admission identifier", formalDefinition="Pre-admission identifier." ) 1430 protected Identifier preAdmissionIdentifier; 1431 1432 /** 1433 * The location from which the patient came before admission. 1434 */ 1435 @Child(name = "origin", type = {Location.class}, order=2, min=0, max=1, modifier=false, summary=false) 1436 @Description(shortDefinition="The location from which the patient came before admission", formalDefinition="The location from which the patient came before admission." ) 1437 protected Reference origin; 1438 1439 /** 1440 * The actual object that is the target of the reference (The location from which the patient came before admission.) 1441 */ 1442 protected Location originTarget; 1443 1444 /** 1445 * From where patient was admitted (physician referral, transfer). 1446 */ 1447 @Child(name = "admitSource", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 1448 @Description(shortDefinition="From where patient was admitted (physician referral, transfer)", formalDefinition="From where patient was admitted (physician referral, transfer)." ) 1449 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-admit-source") 1450 protected CodeableConcept admitSource; 1451 1452 /** 1453 * Whether this hospitalization is a readmission and why if known. 1454 */ 1455 @Child(name = "reAdmission", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1456 @Description(shortDefinition="The type of hospital re-admission that has occurred (if any). If the value is absent, then this is not identified as a readmission", formalDefinition="Whether this hospitalization is a readmission and why if known." ) 1457 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v2-0092") 1458 protected CodeableConcept reAdmission; 1459 1460 /** 1461 * Diet preferences reported by the patient. 1462 */ 1463 @Child(name = "dietPreference", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1464 @Description(shortDefinition="Diet preferences reported by the patient", formalDefinition="Diet preferences reported by the patient." ) 1465 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-diet") 1466 protected List<CodeableConcept> dietPreference; 1467 1468 /** 1469 * Special courtesies (VIP, board member). 1470 */ 1471 @Child(name = "specialCourtesy", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1472 @Description(shortDefinition="Special courtesies (VIP, board member)", formalDefinition="Special courtesies (VIP, board member)." ) 1473 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-special-courtesy") 1474 protected List<CodeableConcept> specialCourtesy; 1475 1476 /** 1477 * Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things. 1478 */ 1479 @Child(name = "specialArrangement", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1480 @Description(shortDefinition="Wheelchair, translator, stretcher, etc.", formalDefinition="Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things." ) 1481 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-special-arrangements") 1482 protected List<CodeableConcept> specialArrangement; 1483 1484 /** 1485 * Location to which the patient is discharged. 1486 */ 1487 @Child(name = "destination", type = {Location.class}, order=8, min=0, max=1, modifier=false, summary=false) 1488 @Description(shortDefinition="Location to which the patient is discharged", formalDefinition="Location to which the patient is discharged." ) 1489 protected Reference destination; 1490 1491 /** 1492 * The actual object that is the target of the reference (Location to which the patient is discharged.) 1493 */ 1494 protected Location destinationTarget; 1495 1496 /** 1497 * Category or kind of location after discharge. 1498 */ 1499 @Child(name = "dischargeDisposition", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 1500 @Description(shortDefinition="Category or kind of location after discharge", formalDefinition="Category or kind of location after discharge." ) 1501 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-discharge-disposition") 1502 protected CodeableConcept dischargeDisposition; 1503 1504 private static final long serialVersionUID = -1165804076L; 1505 1506 /** 1507 * Constructor 1508 */ 1509 public EncounterHospitalizationComponent() { 1510 super(); 1511 } 1512 1513 /** 1514 * @return {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1515 */ 1516 public Identifier getPreAdmissionIdentifier() { 1517 if (this.preAdmissionIdentifier == null) 1518 if (Configuration.errorOnAutoCreate()) 1519 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.preAdmissionIdentifier"); 1520 else if (Configuration.doAutoCreate()) 1521 this.preAdmissionIdentifier = new Identifier(); // cc 1522 return this.preAdmissionIdentifier; 1523 } 1524 1525 public boolean hasPreAdmissionIdentifier() { 1526 return this.preAdmissionIdentifier != null && !this.preAdmissionIdentifier.isEmpty(); 1527 } 1528 1529 /** 1530 * @param value {@link #preAdmissionIdentifier} (Pre-admission identifier.) 1531 */ 1532 public EncounterHospitalizationComponent setPreAdmissionIdentifier(Identifier value) { 1533 this.preAdmissionIdentifier = value; 1534 return this; 1535 } 1536 1537 /** 1538 * @return {@link #origin} (The location from which the patient came before admission.) 1539 */ 1540 public Reference getOrigin() { 1541 if (this.origin == null) 1542 if (Configuration.errorOnAutoCreate()) 1543 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.origin"); 1544 else if (Configuration.doAutoCreate()) 1545 this.origin = new Reference(); // cc 1546 return this.origin; 1547 } 1548 1549 public boolean hasOrigin() { 1550 return this.origin != null && !this.origin.isEmpty(); 1551 } 1552 1553 /** 1554 * @param value {@link #origin} (The location from which the patient came before admission.) 1555 */ 1556 public EncounterHospitalizationComponent setOrigin(Reference value) { 1557 this.origin = value; 1558 return this; 1559 } 1560 1561 /** 1562 * @return {@link #origin} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The location from which the patient came before admission.) 1563 */ 1564 public Location getOriginTarget() { 1565 if (this.originTarget == null) 1566 if (Configuration.errorOnAutoCreate()) 1567 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.origin"); 1568 else if (Configuration.doAutoCreate()) 1569 this.originTarget = new Location(); // aa 1570 return this.originTarget; 1571 } 1572 1573 /** 1574 * @param value {@link #origin} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The location from which the patient came before admission.) 1575 */ 1576 public EncounterHospitalizationComponent setOriginTarget(Location value) { 1577 this.originTarget = value; 1578 return this; 1579 } 1580 1581 /** 1582 * @return {@link #admitSource} (From where patient was admitted (physician referral, transfer).) 1583 */ 1584 public CodeableConcept getAdmitSource() { 1585 if (this.admitSource == null) 1586 if (Configuration.errorOnAutoCreate()) 1587 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.admitSource"); 1588 else if (Configuration.doAutoCreate()) 1589 this.admitSource = new CodeableConcept(); // cc 1590 return this.admitSource; 1591 } 1592 1593 public boolean hasAdmitSource() { 1594 return this.admitSource != null && !this.admitSource.isEmpty(); 1595 } 1596 1597 /** 1598 * @param value {@link #admitSource} (From where patient was admitted (physician referral, transfer).) 1599 */ 1600 public EncounterHospitalizationComponent setAdmitSource(CodeableConcept value) { 1601 this.admitSource = value; 1602 return this; 1603 } 1604 1605 /** 1606 * @return {@link #reAdmission} (Whether this hospitalization is a readmission and why if known.) 1607 */ 1608 public CodeableConcept getReAdmission() { 1609 if (this.reAdmission == null) 1610 if (Configuration.errorOnAutoCreate()) 1611 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.reAdmission"); 1612 else if (Configuration.doAutoCreate()) 1613 this.reAdmission = new CodeableConcept(); // cc 1614 return this.reAdmission; 1615 } 1616 1617 public boolean hasReAdmission() { 1618 return this.reAdmission != null && !this.reAdmission.isEmpty(); 1619 } 1620 1621 /** 1622 * @param value {@link #reAdmission} (Whether this hospitalization is a readmission and why if known.) 1623 */ 1624 public EncounterHospitalizationComponent setReAdmission(CodeableConcept value) { 1625 this.reAdmission = value; 1626 return this; 1627 } 1628 1629 /** 1630 * @return {@link #dietPreference} (Diet preferences reported by the patient.) 1631 */ 1632 public List<CodeableConcept> getDietPreference() { 1633 if (this.dietPreference == null) 1634 this.dietPreference = new ArrayList<CodeableConcept>(); 1635 return this.dietPreference; 1636 } 1637 1638 /** 1639 * @return Returns a reference to <code>this</code> for easy method chaining 1640 */ 1641 public EncounterHospitalizationComponent setDietPreference(List<CodeableConcept> theDietPreference) { 1642 this.dietPreference = theDietPreference; 1643 return this; 1644 } 1645 1646 public boolean hasDietPreference() { 1647 if (this.dietPreference == null) 1648 return false; 1649 for (CodeableConcept item : this.dietPreference) 1650 if (!item.isEmpty()) 1651 return true; 1652 return false; 1653 } 1654 1655 public CodeableConcept addDietPreference() { //3 1656 CodeableConcept t = new CodeableConcept(); 1657 if (this.dietPreference == null) 1658 this.dietPreference = new ArrayList<CodeableConcept>(); 1659 this.dietPreference.add(t); 1660 return t; 1661 } 1662 1663 public EncounterHospitalizationComponent addDietPreference(CodeableConcept t) { //3 1664 if (t == null) 1665 return this; 1666 if (this.dietPreference == null) 1667 this.dietPreference = new ArrayList<CodeableConcept>(); 1668 this.dietPreference.add(t); 1669 return this; 1670 } 1671 1672 /** 1673 * @return The first repetition of repeating field {@link #dietPreference}, creating it if it does not already exist 1674 */ 1675 public CodeableConcept getDietPreferenceFirstRep() { 1676 if (getDietPreference().isEmpty()) { 1677 addDietPreference(); 1678 } 1679 return getDietPreference().get(0); 1680 } 1681 1682 /** 1683 * @return {@link #specialCourtesy} (Special courtesies (VIP, board member).) 1684 */ 1685 public List<CodeableConcept> getSpecialCourtesy() { 1686 if (this.specialCourtesy == null) 1687 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1688 return this.specialCourtesy; 1689 } 1690 1691 /** 1692 * @return Returns a reference to <code>this</code> for easy method chaining 1693 */ 1694 public EncounterHospitalizationComponent setSpecialCourtesy(List<CodeableConcept> theSpecialCourtesy) { 1695 this.specialCourtesy = theSpecialCourtesy; 1696 return this; 1697 } 1698 1699 public boolean hasSpecialCourtesy() { 1700 if (this.specialCourtesy == null) 1701 return false; 1702 for (CodeableConcept item : this.specialCourtesy) 1703 if (!item.isEmpty()) 1704 return true; 1705 return false; 1706 } 1707 1708 public CodeableConcept addSpecialCourtesy() { //3 1709 CodeableConcept t = new CodeableConcept(); 1710 if (this.specialCourtesy == null) 1711 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1712 this.specialCourtesy.add(t); 1713 return t; 1714 } 1715 1716 public EncounterHospitalizationComponent addSpecialCourtesy(CodeableConcept t) { //3 1717 if (t == null) 1718 return this; 1719 if (this.specialCourtesy == null) 1720 this.specialCourtesy = new ArrayList<CodeableConcept>(); 1721 this.specialCourtesy.add(t); 1722 return this; 1723 } 1724 1725 /** 1726 * @return The first repetition of repeating field {@link #specialCourtesy}, creating it if it does not already exist 1727 */ 1728 public CodeableConcept getSpecialCourtesyFirstRep() { 1729 if (getSpecialCourtesy().isEmpty()) { 1730 addSpecialCourtesy(); 1731 } 1732 return getSpecialCourtesy().get(0); 1733 } 1734 1735 /** 1736 * @return {@link #specialArrangement} (Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.) 1737 */ 1738 public List<CodeableConcept> getSpecialArrangement() { 1739 if (this.specialArrangement == null) 1740 this.specialArrangement = new ArrayList<CodeableConcept>(); 1741 return this.specialArrangement; 1742 } 1743 1744 /** 1745 * @return Returns a reference to <code>this</code> for easy method chaining 1746 */ 1747 public EncounterHospitalizationComponent setSpecialArrangement(List<CodeableConcept> theSpecialArrangement) { 1748 this.specialArrangement = theSpecialArrangement; 1749 return this; 1750 } 1751 1752 public boolean hasSpecialArrangement() { 1753 if (this.specialArrangement == null) 1754 return false; 1755 for (CodeableConcept item : this.specialArrangement) 1756 if (!item.isEmpty()) 1757 return true; 1758 return false; 1759 } 1760 1761 public CodeableConcept addSpecialArrangement() { //3 1762 CodeableConcept t = new CodeableConcept(); 1763 if (this.specialArrangement == null) 1764 this.specialArrangement = new ArrayList<CodeableConcept>(); 1765 this.specialArrangement.add(t); 1766 return t; 1767 } 1768 1769 public EncounterHospitalizationComponent addSpecialArrangement(CodeableConcept t) { //3 1770 if (t == null) 1771 return this; 1772 if (this.specialArrangement == null) 1773 this.specialArrangement = new ArrayList<CodeableConcept>(); 1774 this.specialArrangement.add(t); 1775 return this; 1776 } 1777 1778 /** 1779 * @return The first repetition of repeating field {@link #specialArrangement}, creating it if it does not already exist 1780 */ 1781 public CodeableConcept getSpecialArrangementFirstRep() { 1782 if (getSpecialArrangement().isEmpty()) { 1783 addSpecialArrangement(); 1784 } 1785 return getSpecialArrangement().get(0); 1786 } 1787 1788 /** 1789 * @return {@link #destination} (Location to which the patient is discharged.) 1790 */ 1791 public Reference getDestination() { 1792 if (this.destination == null) 1793 if (Configuration.errorOnAutoCreate()) 1794 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.destination"); 1795 else if (Configuration.doAutoCreate()) 1796 this.destination = new Reference(); // cc 1797 return this.destination; 1798 } 1799 1800 public boolean hasDestination() { 1801 return this.destination != null && !this.destination.isEmpty(); 1802 } 1803 1804 /** 1805 * @param value {@link #destination} (Location to which the patient is discharged.) 1806 */ 1807 public EncounterHospitalizationComponent setDestination(Reference value) { 1808 this.destination = value; 1809 return this; 1810 } 1811 1812 /** 1813 * @return {@link #destination} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Location to which the patient is discharged.) 1814 */ 1815 public Location getDestinationTarget() { 1816 if (this.destinationTarget == null) 1817 if (Configuration.errorOnAutoCreate()) 1818 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.destination"); 1819 else if (Configuration.doAutoCreate()) 1820 this.destinationTarget = new Location(); // aa 1821 return this.destinationTarget; 1822 } 1823 1824 /** 1825 * @param value {@link #destination} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Location to which the patient is discharged.) 1826 */ 1827 public EncounterHospitalizationComponent setDestinationTarget(Location value) { 1828 this.destinationTarget = value; 1829 return this; 1830 } 1831 1832 /** 1833 * @return {@link #dischargeDisposition} (Category or kind of location after discharge.) 1834 */ 1835 public CodeableConcept getDischargeDisposition() { 1836 if (this.dischargeDisposition == null) 1837 if (Configuration.errorOnAutoCreate()) 1838 throw new Error("Attempt to auto-create EncounterHospitalizationComponent.dischargeDisposition"); 1839 else if (Configuration.doAutoCreate()) 1840 this.dischargeDisposition = new CodeableConcept(); // cc 1841 return this.dischargeDisposition; 1842 } 1843 1844 public boolean hasDischargeDisposition() { 1845 return this.dischargeDisposition != null && !this.dischargeDisposition.isEmpty(); 1846 } 1847 1848 /** 1849 * @param value {@link #dischargeDisposition} (Category or kind of location after discharge.) 1850 */ 1851 public EncounterHospitalizationComponent setDischargeDisposition(CodeableConcept value) { 1852 this.dischargeDisposition = value; 1853 return this; 1854 } 1855 1856 protected void listChildren(List<Property> children) { 1857 super.listChildren(children); 1858 children.add(new Property("preAdmissionIdentifier", "Identifier", "Pre-admission identifier.", 0, 1, preAdmissionIdentifier)); 1859 children.add(new Property("origin", "Reference(Location)", "The location from which the patient came before admission.", 0, 1, origin)); 1860 children.add(new Property("admitSource", "CodeableConcept", "From where patient was admitted (physician referral, transfer).", 0, 1, admitSource)); 1861 children.add(new Property("reAdmission", "CodeableConcept", "Whether this hospitalization is a readmission and why if known.", 0, 1, reAdmission)); 1862 children.add(new Property("dietPreference", "CodeableConcept", "Diet preferences reported by the patient.", 0, java.lang.Integer.MAX_VALUE, dietPreference)); 1863 children.add(new Property("specialCourtesy", "CodeableConcept", "Special courtesies (VIP, board member).", 0, java.lang.Integer.MAX_VALUE, specialCourtesy)); 1864 children.add(new Property("specialArrangement", "CodeableConcept", "Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.", 0, java.lang.Integer.MAX_VALUE, specialArrangement)); 1865 children.add(new Property("destination", "Reference(Location)", "Location to which the patient is discharged.", 0, 1, destination)); 1866 children.add(new Property("dischargeDisposition", "CodeableConcept", "Category or kind of location after discharge.", 0, 1, dischargeDisposition)); 1867 } 1868 1869 @Override 1870 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1871 switch (_hash) { 1872 case -965394961: /*preAdmissionIdentifier*/ return new Property("preAdmissionIdentifier", "Identifier", "Pre-admission identifier.", 0, 1, preAdmissionIdentifier); 1873 case -1008619738: /*origin*/ return new Property("origin", "Reference(Location)", "The location from which the patient came before admission.", 0, 1, origin); 1874 case 538887120: /*admitSource*/ return new Property("admitSource", "CodeableConcept", "From where patient was admitted (physician referral, transfer).", 0, 1, admitSource); 1875 case 669348630: /*reAdmission*/ return new Property("reAdmission", "CodeableConcept", "Whether this hospitalization is a readmission and why if known.", 0, 1, reAdmission); 1876 case -1360641041: /*dietPreference*/ return new Property("dietPreference", "CodeableConcept", "Diet preferences reported by the patient.", 0, java.lang.Integer.MAX_VALUE, dietPreference); 1877 case 1583588345: /*specialCourtesy*/ return new Property("specialCourtesy", "CodeableConcept", "Special courtesies (VIP, board member).", 0, java.lang.Integer.MAX_VALUE, specialCourtesy); 1878 case 47410321: /*specialArrangement*/ return new Property("specialArrangement", "CodeableConcept", "Any special requests that have been made for this hospitalization encounter, such as the provision of specific equipment or other things.", 0, java.lang.Integer.MAX_VALUE, specialArrangement); 1879 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location)", "Location to which the patient is discharged.", 0, 1, destination); 1880 case 528065941: /*dischargeDisposition*/ return new Property("dischargeDisposition", "CodeableConcept", "Category or kind of location after discharge.", 0, 1, dischargeDisposition); 1881 default: return super.getNamedProperty(_hash, _name, _checkValid); 1882 } 1883 1884 } 1885 1886 @Override 1887 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1888 switch (hash) { 1889 case -965394961: /*preAdmissionIdentifier*/ return this.preAdmissionIdentifier == null ? new Base[0] : new Base[] {this.preAdmissionIdentifier}; // Identifier 1890 case -1008619738: /*origin*/ return this.origin == null ? new Base[0] : new Base[] {this.origin}; // Reference 1891 case 538887120: /*admitSource*/ return this.admitSource == null ? new Base[0] : new Base[] {this.admitSource}; // CodeableConcept 1892 case 669348630: /*reAdmission*/ return this.reAdmission == null ? new Base[0] : new Base[] {this.reAdmission}; // CodeableConcept 1893 case -1360641041: /*dietPreference*/ return this.dietPreference == null ? new Base[0] : this.dietPreference.toArray(new Base[this.dietPreference.size()]); // CodeableConcept 1894 case 1583588345: /*specialCourtesy*/ return this.specialCourtesy == null ? new Base[0] : this.specialCourtesy.toArray(new Base[this.specialCourtesy.size()]); // CodeableConcept 1895 case 47410321: /*specialArrangement*/ return this.specialArrangement == null ? new Base[0] : this.specialArrangement.toArray(new Base[this.specialArrangement.size()]); // CodeableConcept 1896 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 1897 case 528065941: /*dischargeDisposition*/ return this.dischargeDisposition == null ? new Base[0] : new Base[] {this.dischargeDisposition}; // CodeableConcept 1898 default: return super.getProperty(hash, name, checkValid); 1899 } 1900 1901 } 1902 1903 @Override 1904 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1905 switch (hash) { 1906 case -965394961: // preAdmissionIdentifier 1907 this.preAdmissionIdentifier = castToIdentifier(value); // Identifier 1908 return value; 1909 case -1008619738: // origin 1910 this.origin = castToReference(value); // Reference 1911 return value; 1912 case 538887120: // admitSource 1913 this.admitSource = castToCodeableConcept(value); // CodeableConcept 1914 return value; 1915 case 669348630: // reAdmission 1916 this.reAdmission = castToCodeableConcept(value); // CodeableConcept 1917 return value; 1918 case -1360641041: // dietPreference 1919 this.getDietPreference().add(castToCodeableConcept(value)); // CodeableConcept 1920 return value; 1921 case 1583588345: // specialCourtesy 1922 this.getSpecialCourtesy().add(castToCodeableConcept(value)); // CodeableConcept 1923 return value; 1924 case 47410321: // specialArrangement 1925 this.getSpecialArrangement().add(castToCodeableConcept(value)); // CodeableConcept 1926 return value; 1927 case -1429847026: // destination 1928 this.destination = castToReference(value); // Reference 1929 return value; 1930 case 528065941: // dischargeDisposition 1931 this.dischargeDisposition = castToCodeableConcept(value); // CodeableConcept 1932 return value; 1933 default: return super.setProperty(hash, name, value); 1934 } 1935 1936 } 1937 1938 @Override 1939 public Base setProperty(String name, Base value) throws FHIRException { 1940 if (name.equals("preAdmissionIdentifier")) { 1941 this.preAdmissionIdentifier = castToIdentifier(value); // Identifier 1942 } else if (name.equals("origin")) { 1943 this.origin = castToReference(value); // Reference 1944 } else if (name.equals("admitSource")) { 1945 this.admitSource = castToCodeableConcept(value); // CodeableConcept 1946 } else if (name.equals("reAdmission")) { 1947 this.reAdmission = castToCodeableConcept(value); // CodeableConcept 1948 } else if (name.equals("dietPreference")) { 1949 this.getDietPreference().add(castToCodeableConcept(value)); 1950 } else if (name.equals("specialCourtesy")) { 1951 this.getSpecialCourtesy().add(castToCodeableConcept(value)); 1952 } else if (name.equals("specialArrangement")) { 1953 this.getSpecialArrangement().add(castToCodeableConcept(value)); 1954 } else if (name.equals("destination")) { 1955 this.destination = castToReference(value); // Reference 1956 } else if (name.equals("dischargeDisposition")) { 1957 this.dischargeDisposition = castToCodeableConcept(value); // CodeableConcept 1958 } else 1959 return super.setProperty(name, value); 1960 return value; 1961 } 1962 1963 @Override 1964 public Base makeProperty(int hash, String name) throws FHIRException { 1965 switch (hash) { 1966 case -965394961: return getPreAdmissionIdentifier(); 1967 case -1008619738: return getOrigin(); 1968 case 538887120: return getAdmitSource(); 1969 case 669348630: return getReAdmission(); 1970 case -1360641041: return addDietPreference(); 1971 case 1583588345: return addSpecialCourtesy(); 1972 case 47410321: return addSpecialArrangement(); 1973 case -1429847026: return getDestination(); 1974 case 528065941: return getDischargeDisposition(); 1975 default: return super.makeProperty(hash, name); 1976 } 1977 1978 } 1979 1980 @Override 1981 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1982 switch (hash) { 1983 case -965394961: /*preAdmissionIdentifier*/ return new String[] {"Identifier"}; 1984 case -1008619738: /*origin*/ return new String[] {"Reference"}; 1985 case 538887120: /*admitSource*/ return new String[] {"CodeableConcept"}; 1986 case 669348630: /*reAdmission*/ return new String[] {"CodeableConcept"}; 1987 case -1360641041: /*dietPreference*/ return new String[] {"CodeableConcept"}; 1988 case 1583588345: /*specialCourtesy*/ return new String[] {"CodeableConcept"}; 1989 case 47410321: /*specialArrangement*/ return new String[] {"CodeableConcept"}; 1990 case -1429847026: /*destination*/ return new String[] {"Reference"}; 1991 case 528065941: /*dischargeDisposition*/ return new String[] {"CodeableConcept"}; 1992 default: return super.getTypesForProperty(hash, name); 1993 } 1994 1995 } 1996 1997 @Override 1998 public Base addChild(String name) throws FHIRException { 1999 if (name.equals("preAdmissionIdentifier")) { 2000 this.preAdmissionIdentifier = new Identifier(); 2001 return this.preAdmissionIdentifier; 2002 } 2003 else if (name.equals("origin")) { 2004 this.origin = new Reference(); 2005 return this.origin; 2006 } 2007 else if (name.equals("admitSource")) { 2008 this.admitSource = new CodeableConcept(); 2009 return this.admitSource; 2010 } 2011 else if (name.equals("reAdmission")) { 2012 this.reAdmission = new CodeableConcept(); 2013 return this.reAdmission; 2014 } 2015 else if (name.equals("dietPreference")) { 2016 return addDietPreference(); 2017 } 2018 else if (name.equals("specialCourtesy")) { 2019 return addSpecialCourtesy(); 2020 } 2021 else if (name.equals("specialArrangement")) { 2022 return addSpecialArrangement(); 2023 } 2024 else if (name.equals("destination")) { 2025 this.destination = new Reference(); 2026 return this.destination; 2027 } 2028 else if (name.equals("dischargeDisposition")) { 2029 this.dischargeDisposition = new CodeableConcept(); 2030 return this.dischargeDisposition; 2031 } 2032 else 2033 return super.addChild(name); 2034 } 2035 2036 public EncounterHospitalizationComponent copy() { 2037 EncounterHospitalizationComponent dst = new EncounterHospitalizationComponent(); 2038 copyValues(dst); 2039 dst.preAdmissionIdentifier = preAdmissionIdentifier == null ? null : preAdmissionIdentifier.copy(); 2040 dst.origin = origin == null ? null : origin.copy(); 2041 dst.admitSource = admitSource == null ? null : admitSource.copy(); 2042 dst.reAdmission = reAdmission == null ? null : reAdmission.copy(); 2043 if (dietPreference != null) { 2044 dst.dietPreference = new ArrayList<CodeableConcept>(); 2045 for (CodeableConcept i : dietPreference) 2046 dst.dietPreference.add(i.copy()); 2047 }; 2048 if (specialCourtesy != null) { 2049 dst.specialCourtesy = new ArrayList<CodeableConcept>(); 2050 for (CodeableConcept i : specialCourtesy) 2051 dst.specialCourtesy.add(i.copy()); 2052 }; 2053 if (specialArrangement != null) { 2054 dst.specialArrangement = new ArrayList<CodeableConcept>(); 2055 for (CodeableConcept i : specialArrangement) 2056 dst.specialArrangement.add(i.copy()); 2057 }; 2058 dst.destination = destination == null ? null : destination.copy(); 2059 dst.dischargeDisposition = dischargeDisposition == null ? null : dischargeDisposition.copy(); 2060 return dst; 2061 } 2062 2063 @Override 2064 public boolean equalsDeep(Base other_) { 2065 if (!super.equalsDeep(other_)) 2066 return false; 2067 if (!(other_ instanceof EncounterHospitalizationComponent)) 2068 return false; 2069 EncounterHospitalizationComponent o = (EncounterHospitalizationComponent) other_; 2070 return compareDeep(preAdmissionIdentifier, o.preAdmissionIdentifier, true) && compareDeep(origin, o.origin, true) 2071 && compareDeep(admitSource, o.admitSource, true) && compareDeep(reAdmission, o.reAdmission, true) 2072 && compareDeep(dietPreference, o.dietPreference, true) && compareDeep(specialCourtesy, o.specialCourtesy, true) 2073 && compareDeep(specialArrangement, o.specialArrangement, true) && compareDeep(destination, o.destination, true) 2074 && compareDeep(dischargeDisposition, o.dischargeDisposition, true); 2075 } 2076 2077 @Override 2078 public boolean equalsShallow(Base other_) { 2079 if (!super.equalsShallow(other_)) 2080 return false; 2081 if (!(other_ instanceof EncounterHospitalizationComponent)) 2082 return false; 2083 EncounterHospitalizationComponent o = (EncounterHospitalizationComponent) other_; 2084 return true; 2085 } 2086 2087 public boolean isEmpty() { 2088 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(preAdmissionIdentifier, origin 2089 , admitSource, reAdmission, dietPreference, specialCourtesy, specialArrangement, destination 2090 , dischargeDisposition); 2091 } 2092 2093 public String fhirType() { 2094 return "Encounter.hospitalization"; 2095 2096 } 2097 2098 } 2099 2100 @Block() 2101 public static class EncounterLocationComponent extends BackboneElement implements IBaseBackboneElement { 2102 /** 2103 * The location where the encounter takes place. 2104 */ 2105 @Child(name = "location", type = {Location.class}, order=1, min=1, max=1, modifier=false, summary=false) 2106 @Description(shortDefinition="Location the encounter takes place", formalDefinition="The location where the encounter takes place." ) 2107 protected Reference location; 2108 2109 /** 2110 * The actual object that is the target of the reference (The location where the encounter takes place.) 2111 */ 2112 protected Location locationTarget; 2113 2114 /** 2115 * The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time. 2116 */ 2117 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2118 @Description(shortDefinition="planned | active | reserved | completed", formalDefinition="The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time." ) 2119 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-location-status") 2120 protected Enumeration<EncounterLocationStatus> status; 2121 2122 /** 2123 * Time period during which the patient was present at the location. 2124 */ 2125 @Child(name = "period", type = {Period.class}, order=3, min=0, max=1, modifier=false, summary=false) 2126 @Description(shortDefinition="Time period during which the patient was present at the location", formalDefinition="Time period during which the patient was present at the location." ) 2127 protected Period period; 2128 2129 private static final long serialVersionUID = -322984880L; 2130 2131 /** 2132 * Constructor 2133 */ 2134 public EncounterLocationComponent() { 2135 super(); 2136 } 2137 2138 /** 2139 * Constructor 2140 */ 2141 public EncounterLocationComponent(Reference location) { 2142 super(); 2143 this.location = location; 2144 } 2145 2146 /** 2147 * @return {@link #location} (The location where the encounter takes place.) 2148 */ 2149 public Reference getLocation() { 2150 if (this.location == null) 2151 if (Configuration.errorOnAutoCreate()) 2152 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 2153 else if (Configuration.doAutoCreate()) 2154 this.location = new Reference(); // cc 2155 return this.location; 2156 } 2157 2158 public boolean hasLocation() { 2159 return this.location != null && !this.location.isEmpty(); 2160 } 2161 2162 /** 2163 * @param value {@link #location} (The location where the encounter takes place.) 2164 */ 2165 public EncounterLocationComponent setLocation(Reference value) { 2166 this.location = value; 2167 return this; 2168 } 2169 2170 /** 2171 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The location where the encounter takes place.) 2172 */ 2173 public Location getLocationTarget() { 2174 if (this.locationTarget == null) 2175 if (Configuration.errorOnAutoCreate()) 2176 throw new Error("Attempt to auto-create EncounterLocationComponent.location"); 2177 else if (Configuration.doAutoCreate()) 2178 this.locationTarget = new Location(); // aa 2179 return this.locationTarget; 2180 } 2181 2182 /** 2183 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The location where the encounter takes place.) 2184 */ 2185 public EncounterLocationComponent setLocationTarget(Location value) { 2186 this.locationTarget = value; 2187 return this; 2188 } 2189 2190 /** 2191 * @return {@link #status} (The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2192 */ 2193 public Enumeration<EncounterLocationStatus> getStatusElement() { 2194 if (this.status == null) 2195 if (Configuration.errorOnAutoCreate()) 2196 throw new Error("Attempt to auto-create EncounterLocationComponent.status"); 2197 else if (Configuration.doAutoCreate()) 2198 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); // bb 2199 return this.status; 2200 } 2201 2202 public boolean hasStatusElement() { 2203 return this.status != null && !this.status.isEmpty(); 2204 } 2205 2206 public boolean hasStatus() { 2207 return this.status != null && !this.status.isEmpty(); 2208 } 2209 2210 /** 2211 * @param value {@link #status} (The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2212 */ 2213 public EncounterLocationComponent setStatusElement(Enumeration<EncounterLocationStatus> value) { 2214 this.status = value; 2215 return this; 2216 } 2217 2218 /** 2219 * @return The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time. 2220 */ 2221 public EncounterLocationStatus getStatus() { 2222 return this.status == null ? null : this.status.getValue(); 2223 } 2224 2225 /** 2226 * @param value The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time. 2227 */ 2228 public EncounterLocationComponent setStatus(EncounterLocationStatus value) { 2229 if (value == null) 2230 this.status = null; 2231 else { 2232 if (this.status == null) 2233 this.status = new Enumeration<EncounterLocationStatus>(new EncounterLocationStatusEnumFactory()); 2234 this.status.setValue(value); 2235 } 2236 return this; 2237 } 2238 2239 /** 2240 * @return {@link #period} (Time period during which the patient was present at the location.) 2241 */ 2242 public Period getPeriod() { 2243 if (this.period == null) 2244 if (Configuration.errorOnAutoCreate()) 2245 throw new Error("Attempt to auto-create EncounterLocationComponent.period"); 2246 else if (Configuration.doAutoCreate()) 2247 this.period = new Period(); // cc 2248 return this.period; 2249 } 2250 2251 public boolean hasPeriod() { 2252 return this.period != null && !this.period.isEmpty(); 2253 } 2254 2255 /** 2256 * @param value {@link #period} (Time period during which the patient was present at the location.) 2257 */ 2258 public EncounterLocationComponent setPeriod(Period value) { 2259 this.period = value; 2260 return this; 2261 } 2262 2263 protected void listChildren(List<Property> children) { 2264 super.listChildren(children); 2265 children.add(new Property("location", "Reference(Location)", "The location where the encounter takes place.", 0, 1, location)); 2266 children.add(new Property("status", "code", "The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time.", 0, 1, status)); 2267 children.add(new Property("period", "Period", "Time period during which the patient was present at the location.", 0, 1, period)); 2268 } 2269 2270 @Override 2271 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2272 switch (_hash) { 2273 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location where the encounter takes place.", 0, 1, location); 2274 case -892481550: /*status*/ return new Property("status", "code", "The status of the participants' presence at the specified location during the period specified. If the participant is is no longer at the location, then the period will have an end date/time.", 0, 1, status); 2275 case -991726143: /*period*/ return new Property("period", "Period", "Time period during which the patient was present at the location.", 0, 1, period); 2276 default: return super.getNamedProperty(_hash, _name, _checkValid); 2277 } 2278 2279 } 2280 2281 @Override 2282 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2283 switch (hash) { 2284 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2285 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EncounterLocationStatus> 2286 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 2287 default: return super.getProperty(hash, name, checkValid); 2288 } 2289 2290 } 2291 2292 @Override 2293 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2294 switch (hash) { 2295 case 1901043637: // location 2296 this.location = castToReference(value); // Reference 2297 return value; 2298 case -892481550: // status 2299 value = new EncounterLocationStatusEnumFactory().fromType(castToCode(value)); 2300 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 2301 return value; 2302 case -991726143: // period 2303 this.period = castToPeriod(value); // Period 2304 return value; 2305 default: return super.setProperty(hash, name, value); 2306 } 2307 2308 } 2309 2310 @Override 2311 public Base setProperty(String name, Base value) throws FHIRException { 2312 if (name.equals("location")) { 2313 this.location = castToReference(value); // Reference 2314 } else if (name.equals("status")) { 2315 value = new EncounterLocationStatusEnumFactory().fromType(castToCode(value)); 2316 this.status = (Enumeration) value; // Enumeration<EncounterLocationStatus> 2317 } else if (name.equals("period")) { 2318 this.period = castToPeriod(value); // Period 2319 } else 2320 return super.setProperty(name, value); 2321 return value; 2322 } 2323 2324 @Override 2325 public Base makeProperty(int hash, String name) throws FHIRException { 2326 switch (hash) { 2327 case 1901043637: return getLocation(); 2328 case -892481550: return getStatusElement(); 2329 case -991726143: return getPeriod(); 2330 default: return super.makeProperty(hash, name); 2331 } 2332 2333 } 2334 2335 @Override 2336 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2337 switch (hash) { 2338 case 1901043637: /*location*/ return new String[] {"Reference"}; 2339 case -892481550: /*status*/ return new String[] {"code"}; 2340 case -991726143: /*period*/ return new String[] {"Period"}; 2341 default: return super.getTypesForProperty(hash, name); 2342 } 2343 2344 } 2345 2346 @Override 2347 public Base addChild(String name) throws FHIRException { 2348 if (name.equals("location")) { 2349 this.location = new Reference(); 2350 return this.location; 2351 } 2352 else if (name.equals("status")) { 2353 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 2354 } 2355 else if (name.equals("period")) { 2356 this.period = new Period(); 2357 return this.period; 2358 } 2359 else 2360 return super.addChild(name); 2361 } 2362 2363 public EncounterLocationComponent copy() { 2364 EncounterLocationComponent dst = new EncounterLocationComponent(); 2365 copyValues(dst); 2366 dst.location = location == null ? null : location.copy(); 2367 dst.status = status == null ? null : status.copy(); 2368 dst.period = period == null ? null : period.copy(); 2369 return dst; 2370 } 2371 2372 @Override 2373 public boolean equalsDeep(Base other_) { 2374 if (!super.equalsDeep(other_)) 2375 return false; 2376 if (!(other_ instanceof EncounterLocationComponent)) 2377 return false; 2378 EncounterLocationComponent o = (EncounterLocationComponent) other_; 2379 return compareDeep(location, o.location, true) && compareDeep(status, o.status, true) && compareDeep(period, o.period, true) 2380 ; 2381 } 2382 2383 @Override 2384 public boolean equalsShallow(Base other_) { 2385 if (!super.equalsShallow(other_)) 2386 return false; 2387 if (!(other_ instanceof EncounterLocationComponent)) 2388 return false; 2389 EncounterLocationComponent o = (EncounterLocationComponent) other_; 2390 return compareValues(status, o.status, true); 2391 } 2392 2393 public boolean isEmpty() { 2394 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(location, status, period 2395 ); 2396 } 2397 2398 public String fhirType() { 2399 return "Encounter.location"; 2400 2401 } 2402 2403 } 2404 2405 /** 2406 * Identifier(s) by which this encounter is known. 2407 */ 2408 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2409 @Description(shortDefinition="Identifier(s) by which this encounter is known", formalDefinition="Identifier(s) by which this encounter is known." ) 2410 protected List<Identifier> identifier; 2411 2412 /** 2413 * planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 2414 */ 2415 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 2416 @Description(shortDefinition="planned | arrived | triaged | in-progress | onleave | finished | cancelled +", formalDefinition="planned | arrived | triaged | in-progress | onleave | finished | cancelled +." ) 2417 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-status") 2418 protected Enumeration<EncounterStatus> status; 2419 2420 /** 2421 * The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them. 2422 */ 2423 @Child(name = "statusHistory", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2424 @Description(shortDefinition="List of past encounter statuses", formalDefinition="The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them." ) 2425 protected List<StatusHistoryComponent> statusHistory; 2426 2427 /** 2428 * inpatient | outpatient | ambulatory | emergency +. 2429 */ 2430 @Child(name = "class", type = {Coding.class}, order=3, min=0, max=1, modifier=false, summary=true) 2431 @Description(shortDefinition="inpatient | outpatient | ambulatory | emergency +", formalDefinition="inpatient | outpatient | ambulatory | emergency +." ) 2432 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActEncounterCode") 2433 protected Coding class_; 2434 2435 /** 2436 * The class history permits the tracking of the encounters transitions without needing to go through the resource history. 2437 2438This would be used for a case where an admission starts of as an emergency encounter, then transisions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kindof discharge from emergency to inpatient. 2439 */ 2440 @Child(name = "classHistory", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2441 @Description(shortDefinition="List of past encounter classes", formalDefinition="The class history permits the tracking of the encounters transitions without needing to go through the resource history.\n\nThis would be used for a case where an admission starts of as an emergency encounter, then transisions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kindof discharge from emergency to inpatient." ) 2442 protected List<ClassHistoryComponent> classHistory; 2443 2444 /** 2445 * Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation). 2446 */ 2447 @Child(name = "type", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2448 @Description(shortDefinition="Specific type of encounter", formalDefinition="Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation)." ) 2449 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-type") 2450 protected List<CodeableConcept> type; 2451 2452 /** 2453 * Indicates the urgency of the encounter. 2454 */ 2455 @Child(name = "priority", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 2456 @Description(shortDefinition="Indicates the urgency of the encounter", formalDefinition="Indicates the urgency of the encounter." ) 2457 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActPriority") 2458 protected CodeableConcept priority; 2459 2460 /** 2461 * The patient ro group present at the encounter. 2462 */ 2463 @Child(name = "subject", type = {Patient.class, Group.class}, order=7, min=0, max=1, modifier=false, summary=true) 2464 @Description(shortDefinition="The patient ro group present at the encounter", formalDefinition="The patient ro group present at the encounter." ) 2465 protected Reference subject; 2466 2467 /** 2468 * The actual object that is the target of the reference (The patient ro group present at the encounter.) 2469 */ 2470 protected Resource subjectTarget; 2471 2472 /** 2473 * Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care, and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years). 2474 */ 2475 @Child(name = "episodeOfCare", type = {EpisodeOfCare.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2476 @Description(shortDefinition="Episode(s) of care that this encounter should be recorded against", formalDefinition="Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care, and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years)." ) 2477 protected List<Reference> episodeOfCare; 2478 /** 2479 * The actual objects that are the target of the reference (Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care, and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).) 2480 */ 2481 protected List<EpisodeOfCare> episodeOfCareTarget; 2482 2483 2484 /** 2485 * The referral request this encounter satisfies (incoming referral). 2486 */ 2487 @Child(name = "incomingReferral", type = {ReferralRequest.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2488 @Description(shortDefinition="The ReferralRequest that initiated this encounter", formalDefinition="The referral request this encounter satisfies (incoming referral)." ) 2489 protected List<Reference> incomingReferral; 2490 /** 2491 * The actual objects that are the target of the reference (The referral request this encounter satisfies (incoming referral).) 2492 */ 2493 protected List<ReferralRequest> incomingReferralTarget; 2494 2495 2496 /** 2497 * The list of people responsible for providing the service. 2498 */ 2499 @Child(name = "participant", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2500 @Description(shortDefinition="List of participants involved in the encounter", formalDefinition="The list of people responsible for providing the service." ) 2501 protected List<EncounterParticipantComponent> participant; 2502 2503 /** 2504 * The appointment that scheduled this encounter. 2505 */ 2506 @Child(name = "appointment", type = {Appointment.class}, order=11, min=0, max=1, modifier=false, summary=true) 2507 @Description(shortDefinition="The appointment that scheduled this encounter", formalDefinition="The appointment that scheduled this encounter." ) 2508 protected Reference appointment; 2509 2510 /** 2511 * The actual object that is the target of the reference (The appointment that scheduled this encounter.) 2512 */ 2513 protected Appointment appointmentTarget; 2514 2515 /** 2516 * The start and end time of the encounter. 2517 */ 2518 @Child(name = "period", type = {Period.class}, order=12, min=0, max=1, modifier=false, summary=false) 2519 @Description(shortDefinition="The start and end time of the encounter", formalDefinition="The start and end time of the encounter." ) 2520 protected Period period; 2521 2522 /** 2523 * Quantity of time the encounter lasted. This excludes the time during leaves of absence. 2524 */ 2525 @Child(name = "length", type = {Duration.class}, order=13, min=0, max=1, modifier=false, summary=false) 2526 @Description(shortDefinition="Quantity of time the encounter lasted (less time absent)", formalDefinition="Quantity of time the encounter lasted. This excludes the time during leaves of absence." ) 2527 protected Duration length; 2528 2529 /** 2530 * Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis. 2531 */ 2532 @Child(name = "reason", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2533 @Description(shortDefinition="Reason the encounter takes place (code)", formalDefinition="Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis." ) 2534 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/encounter-reason") 2535 protected List<CodeableConcept> reason; 2536 2537 /** 2538 * The list of diagnosis relevant to this encounter. 2539 */ 2540 @Child(name = "diagnosis", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2541 @Description(shortDefinition="The list of diagnosis relevant to this encounter", formalDefinition="The list of diagnosis relevant to this encounter." ) 2542 protected List<DiagnosisComponent> diagnosis; 2543 2544 /** 2545 * The set of accounts that may be used for billing for this Encounter. 2546 */ 2547 @Child(name = "account", type = {Account.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2548 @Description(shortDefinition="The set of accounts that may be used for billing for this Encounter", formalDefinition="The set of accounts that may be used for billing for this Encounter." ) 2549 protected List<Reference> account; 2550 /** 2551 * The actual objects that are the target of the reference (The set of accounts that may be used for billing for this Encounter.) 2552 */ 2553 protected List<Account> accountTarget; 2554 2555 2556 /** 2557 * Details about the admission to a healthcare service. 2558 */ 2559 @Child(name = "hospitalization", type = {}, order=17, min=0, max=1, modifier=false, summary=false) 2560 @Description(shortDefinition="Details about the admission to a healthcare service", formalDefinition="Details about the admission to a healthcare service." ) 2561 protected EncounterHospitalizationComponent hospitalization; 2562 2563 /** 2564 * List of locations where the patient has been during this encounter. 2565 */ 2566 @Child(name = "location", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2567 @Description(shortDefinition="List of locations where the patient has been", formalDefinition="List of locations where the patient has been during this encounter." ) 2568 protected List<EncounterLocationComponent> location; 2569 2570 /** 2571 * An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization. 2572 */ 2573 @Child(name = "serviceProvider", type = {Organization.class}, order=19, min=0, max=1, modifier=false, summary=false) 2574 @Description(shortDefinition="The custodian organization of this Encounter record", formalDefinition="An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization." ) 2575 protected Reference serviceProvider; 2576 2577 /** 2578 * The actual object that is the target of the reference (An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.) 2579 */ 2580 protected Organization serviceProviderTarget; 2581 2582 /** 2583 * Another Encounter of which this encounter is a part of (administratively or in time). 2584 */ 2585 @Child(name = "partOf", type = {Encounter.class}, order=20, min=0, max=1, modifier=false, summary=false) 2586 @Description(shortDefinition="Another Encounter this encounter is part of", formalDefinition="Another Encounter of which this encounter is a part of (administratively or in time)." ) 2587 protected Reference partOf; 2588 2589 /** 2590 * The actual object that is the target of the reference (Another Encounter of which this encounter is a part of (administratively or in time).) 2591 */ 2592 protected Encounter partOfTarget; 2593 2594 private static final long serialVersionUID = 2124102382L; 2595 2596 /** 2597 * Constructor 2598 */ 2599 public Encounter() { 2600 super(); 2601 } 2602 2603 /** 2604 * Constructor 2605 */ 2606 public Encounter(Enumeration<EncounterStatus> status) { 2607 super(); 2608 this.status = status; 2609 } 2610 2611 /** 2612 * @return {@link #identifier} (Identifier(s) by which this encounter is known.) 2613 */ 2614 public List<Identifier> getIdentifier() { 2615 if (this.identifier == null) 2616 this.identifier = new ArrayList<Identifier>(); 2617 return this.identifier; 2618 } 2619 2620 /** 2621 * @return Returns a reference to <code>this</code> for easy method chaining 2622 */ 2623 public Encounter setIdentifier(List<Identifier> theIdentifier) { 2624 this.identifier = theIdentifier; 2625 return this; 2626 } 2627 2628 public boolean hasIdentifier() { 2629 if (this.identifier == null) 2630 return false; 2631 for (Identifier item : this.identifier) 2632 if (!item.isEmpty()) 2633 return true; 2634 return false; 2635 } 2636 2637 public Identifier addIdentifier() { //3 2638 Identifier t = new Identifier(); 2639 if (this.identifier == null) 2640 this.identifier = new ArrayList<Identifier>(); 2641 this.identifier.add(t); 2642 return t; 2643 } 2644 2645 public Encounter addIdentifier(Identifier t) { //3 2646 if (t == null) 2647 return this; 2648 if (this.identifier == null) 2649 this.identifier = new ArrayList<Identifier>(); 2650 this.identifier.add(t); 2651 return this; 2652 } 2653 2654 /** 2655 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 2656 */ 2657 public Identifier getIdentifierFirstRep() { 2658 if (getIdentifier().isEmpty()) { 2659 addIdentifier(); 2660 } 2661 return getIdentifier().get(0); 2662 } 2663 2664 /** 2665 * @return {@link #status} (planned | arrived | triaged | in-progress | onleave | finished | cancelled +.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2666 */ 2667 public Enumeration<EncounterStatus> getStatusElement() { 2668 if (this.status == null) 2669 if (Configuration.errorOnAutoCreate()) 2670 throw new Error("Attempt to auto-create Encounter.status"); 2671 else if (Configuration.doAutoCreate()) 2672 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); // bb 2673 return this.status; 2674 } 2675 2676 public boolean hasStatusElement() { 2677 return this.status != null && !this.status.isEmpty(); 2678 } 2679 2680 public boolean hasStatus() { 2681 return this.status != null && !this.status.isEmpty(); 2682 } 2683 2684 /** 2685 * @param value {@link #status} (planned | arrived | triaged | in-progress | onleave | finished | cancelled +.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2686 */ 2687 public Encounter setStatusElement(Enumeration<EncounterStatus> value) { 2688 this.status = value; 2689 return this; 2690 } 2691 2692 /** 2693 * @return planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 2694 */ 2695 public EncounterStatus getStatus() { 2696 return this.status == null ? null : this.status.getValue(); 2697 } 2698 2699 /** 2700 * @param value planned | arrived | triaged | in-progress | onleave | finished | cancelled +. 2701 */ 2702 public Encounter setStatus(EncounterStatus value) { 2703 if (this.status == null) 2704 this.status = new Enumeration<EncounterStatus>(new EncounterStatusEnumFactory()); 2705 this.status.setValue(value); 2706 return this; 2707 } 2708 2709 /** 2710 * @return {@link #statusHistory} (The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.) 2711 */ 2712 public List<StatusHistoryComponent> getStatusHistory() { 2713 if (this.statusHistory == null) 2714 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 2715 return this.statusHistory; 2716 } 2717 2718 /** 2719 * @return Returns a reference to <code>this</code> for easy method chaining 2720 */ 2721 public Encounter setStatusHistory(List<StatusHistoryComponent> theStatusHistory) { 2722 this.statusHistory = theStatusHistory; 2723 return this; 2724 } 2725 2726 public boolean hasStatusHistory() { 2727 if (this.statusHistory == null) 2728 return false; 2729 for (StatusHistoryComponent item : this.statusHistory) 2730 if (!item.isEmpty()) 2731 return true; 2732 return false; 2733 } 2734 2735 public StatusHistoryComponent addStatusHistory() { //3 2736 StatusHistoryComponent t = new StatusHistoryComponent(); 2737 if (this.statusHistory == null) 2738 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 2739 this.statusHistory.add(t); 2740 return t; 2741 } 2742 2743 public Encounter addStatusHistory(StatusHistoryComponent t) { //3 2744 if (t == null) 2745 return this; 2746 if (this.statusHistory == null) 2747 this.statusHistory = new ArrayList<StatusHistoryComponent>(); 2748 this.statusHistory.add(t); 2749 return this; 2750 } 2751 2752 /** 2753 * @return The first repetition of repeating field {@link #statusHistory}, creating it if it does not already exist 2754 */ 2755 public StatusHistoryComponent getStatusHistoryFirstRep() { 2756 if (getStatusHistory().isEmpty()) { 2757 addStatusHistory(); 2758 } 2759 return getStatusHistory().get(0); 2760 } 2761 2762 /** 2763 * @return {@link #class_} (inpatient | outpatient | ambulatory | emergency +.) 2764 */ 2765 public Coding getClass_() { 2766 if (this.class_ == null) 2767 if (Configuration.errorOnAutoCreate()) 2768 throw new Error("Attempt to auto-create Encounter.class_"); 2769 else if (Configuration.doAutoCreate()) 2770 this.class_ = new Coding(); // cc 2771 return this.class_; 2772 } 2773 2774 public boolean hasClass_() { 2775 return this.class_ != null && !this.class_.isEmpty(); 2776 } 2777 2778 /** 2779 * @param value {@link #class_} (inpatient | outpatient | ambulatory | emergency +.) 2780 */ 2781 public Encounter setClass_(Coding value) { 2782 this.class_ = value; 2783 return this; 2784 } 2785 2786 /** 2787 * @return {@link #classHistory} (The class history permits the tracking of the encounters transitions without needing to go through the resource history. 2788 2789This would be used for a case where an admission starts of as an emergency encounter, then transisions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kindof discharge from emergency to inpatient.) 2790 */ 2791 public List<ClassHistoryComponent> getClassHistory() { 2792 if (this.classHistory == null) 2793 this.classHistory = new ArrayList<ClassHistoryComponent>(); 2794 return this.classHistory; 2795 } 2796 2797 /** 2798 * @return Returns a reference to <code>this</code> for easy method chaining 2799 */ 2800 public Encounter setClassHistory(List<ClassHistoryComponent> theClassHistory) { 2801 this.classHistory = theClassHistory; 2802 return this; 2803 } 2804 2805 public boolean hasClassHistory() { 2806 if (this.classHistory == null) 2807 return false; 2808 for (ClassHistoryComponent item : this.classHistory) 2809 if (!item.isEmpty()) 2810 return true; 2811 return false; 2812 } 2813 2814 public ClassHistoryComponent addClassHistory() { //3 2815 ClassHistoryComponent t = new ClassHistoryComponent(); 2816 if (this.classHistory == null) 2817 this.classHistory = new ArrayList<ClassHistoryComponent>(); 2818 this.classHistory.add(t); 2819 return t; 2820 } 2821 2822 public Encounter addClassHistory(ClassHistoryComponent t) { //3 2823 if (t == null) 2824 return this; 2825 if (this.classHistory == null) 2826 this.classHistory = new ArrayList<ClassHistoryComponent>(); 2827 this.classHistory.add(t); 2828 return this; 2829 } 2830 2831 /** 2832 * @return The first repetition of repeating field {@link #classHistory}, creating it if it does not already exist 2833 */ 2834 public ClassHistoryComponent getClassHistoryFirstRep() { 2835 if (getClassHistory().isEmpty()) { 2836 addClassHistory(); 2837 } 2838 return getClassHistory().get(0); 2839 } 2840 2841 /** 2842 * @return {@link #type} (Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).) 2843 */ 2844 public List<CodeableConcept> getType() { 2845 if (this.type == null) 2846 this.type = new ArrayList<CodeableConcept>(); 2847 return this.type; 2848 } 2849 2850 /** 2851 * @return Returns a reference to <code>this</code> for easy method chaining 2852 */ 2853 public Encounter setType(List<CodeableConcept> theType) { 2854 this.type = theType; 2855 return this; 2856 } 2857 2858 public boolean hasType() { 2859 if (this.type == null) 2860 return false; 2861 for (CodeableConcept item : this.type) 2862 if (!item.isEmpty()) 2863 return true; 2864 return false; 2865 } 2866 2867 public CodeableConcept addType() { //3 2868 CodeableConcept t = new CodeableConcept(); 2869 if (this.type == null) 2870 this.type = new ArrayList<CodeableConcept>(); 2871 this.type.add(t); 2872 return t; 2873 } 2874 2875 public Encounter addType(CodeableConcept t) { //3 2876 if (t == null) 2877 return this; 2878 if (this.type == null) 2879 this.type = new ArrayList<CodeableConcept>(); 2880 this.type.add(t); 2881 return this; 2882 } 2883 2884 /** 2885 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 2886 */ 2887 public CodeableConcept getTypeFirstRep() { 2888 if (getType().isEmpty()) { 2889 addType(); 2890 } 2891 return getType().get(0); 2892 } 2893 2894 /** 2895 * @return {@link #priority} (Indicates the urgency of the encounter.) 2896 */ 2897 public CodeableConcept getPriority() { 2898 if (this.priority == null) 2899 if (Configuration.errorOnAutoCreate()) 2900 throw new Error("Attempt to auto-create Encounter.priority"); 2901 else if (Configuration.doAutoCreate()) 2902 this.priority = new CodeableConcept(); // cc 2903 return this.priority; 2904 } 2905 2906 public boolean hasPriority() { 2907 return this.priority != null && !this.priority.isEmpty(); 2908 } 2909 2910 /** 2911 * @param value {@link #priority} (Indicates the urgency of the encounter.) 2912 */ 2913 public Encounter setPriority(CodeableConcept value) { 2914 this.priority = value; 2915 return this; 2916 } 2917 2918 /** 2919 * @return {@link #subject} (The patient ro group present at the encounter.) 2920 */ 2921 public Reference getSubject() { 2922 if (this.subject == null) 2923 if (Configuration.errorOnAutoCreate()) 2924 throw new Error("Attempt to auto-create Encounter.subject"); 2925 else if (Configuration.doAutoCreate()) 2926 this.subject = new Reference(); // cc 2927 return this.subject; 2928 } 2929 2930 public boolean hasSubject() { 2931 return this.subject != null && !this.subject.isEmpty(); 2932 } 2933 2934 /** 2935 * @param value {@link #subject} (The patient ro group present at the encounter.) 2936 */ 2937 public Encounter setSubject(Reference value) { 2938 this.subject = value; 2939 return this; 2940 } 2941 2942 /** 2943 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient ro group present at the encounter.) 2944 */ 2945 public Resource getSubjectTarget() { 2946 return this.subjectTarget; 2947 } 2948 2949 /** 2950 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient ro group present at the encounter.) 2951 */ 2952 public Encounter setSubjectTarget(Resource value) { 2953 this.subjectTarget = value; 2954 return this; 2955 } 2956 2957 /** 2958 * @return {@link #episodeOfCare} (Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care, and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).) 2959 */ 2960 public List<Reference> getEpisodeOfCare() { 2961 if (this.episodeOfCare == null) 2962 this.episodeOfCare = new ArrayList<Reference>(); 2963 return this.episodeOfCare; 2964 } 2965 2966 /** 2967 * @return Returns a reference to <code>this</code> for easy method chaining 2968 */ 2969 public Encounter setEpisodeOfCare(List<Reference> theEpisodeOfCare) { 2970 this.episodeOfCare = theEpisodeOfCare; 2971 return this; 2972 } 2973 2974 public boolean hasEpisodeOfCare() { 2975 if (this.episodeOfCare == null) 2976 return false; 2977 for (Reference item : this.episodeOfCare) 2978 if (!item.isEmpty()) 2979 return true; 2980 return false; 2981 } 2982 2983 public Reference addEpisodeOfCare() { //3 2984 Reference t = new Reference(); 2985 if (this.episodeOfCare == null) 2986 this.episodeOfCare = new ArrayList<Reference>(); 2987 this.episodeOfCare.add(t); 2988 return t; 2989 } 2990 2991 public Encounter addEpisodeOfCare(Reference t) { //3 2992 if (t == null) 2993 return this; 2994 if (this.episodeOfCare == null) 2995 this.episodeOfCare = new ArrayList<Reference>(); 2996 this.episodeOfCare.add(t); 2997 return this; 2998 } 2999 3000 /** 3001 * @return The first repetition of repeating field {@link #episodeOfCare}, creating it if it does not already exist 3002 */ 3003 public Reference getEpisodeOfCareFirstRep() { 3004 if (getEpisodeOfCare().isEmpty()) { 3005 addEpisodeOfCare(); 3006 } 3007 return getEpisodeOfCare().get(0); 3008 } 3009 3010 /** 3011 * @deprecated Use Reference#setResource(IBaseResource) instead 3012 */ 3013 @Deprecated 3014 public List<EpisodeOfCare> getEpisodeOfCareTarget() { 3015 if (this.episodeOfCareTarget == null) 3016 this.episodeOfCareTarget = new ArrayList<EpisodeOfCare>(); 3017 return this.episodeOfCareTarget; 3018 } 3019 3020 /** 3021 * @deprecated Use Reference#setResource(IBaseResource) instead 3022 */ 3023 @Deprecated 3024 public EpisodeOfCare addEpisodeOfCareTarget() { 3025 EpisodeOfCare r = new EpisodeOfCare(); 3026 if (this.episodeOfCareTarget == null) 3027 this.episodeOfCareTarget = new ArrayList<EpisodeOfCare>(); 3028 this.episodeOfCareTarget.add(r); 3029 return r; 3030 } 3031 3032 /** 3033 * @return {@link #incomingReferral} (The referral request this encounter satisfies (incoming referral).) 3034 */ 3035 public List<Reference> getIncomingReferral() { 3036 if (this.incomingReferral == null) 3037 this.incomingReferral = new ArrayList<Reference>(); 3038 return this.incomingReferral; 3039 } 3040 3041 /** 3042 * @return Returns a reference to <code>this</code> for easy method chaining 3043 */ 3044 public Encounter setIncomingReferral(List<Reference> theIncomingReferral) { 3045 this.incomingReferral = theIncomingReferral; 3046 return this; 3047 } 3048 3049 public boolean hasIncomingReferral() { 3050 if (this.incomingReferral == null) 3051 return false; 3052 for (Reference item : this.incomingReferral) 3053 if (!item.isEmpty()) 3054 return true; 3055 return false; 3056 } 3057 3058 public Reference addIncomingReferral() { //3 3059 Reference t = new Reference(); 3060 if (this.incomingReferral == null) 3061 this.incomingReferral = new ArrayList<Reference>(); 3062 this.incomingReferral.add(t); 3063 return t; 3064 } 3065 3066 public Encounter addIncomingReferral(Reference t) { //3 3067 if (t == null) 3068 return this; 3069 if (this.incomingReferral == null) 3070 this.incomingReferral = new ArrayList<Reference>(); 3071 this.incomingReferral.add(t); 3072 return this; 3073 } 3074 3075 /** 3076 * @return The first repetition of repeating field {@link #incomingReferral}, creating it if it does not already exist 3077 */ 3078 public Reference getIncomingReferralFirstRep() { 3079 if (getIncomingReferral().isEmpty()) { 3080 addIncomingReferral(); 3081 } 3082 return getIncomingReferral().get(0); 3083 } 3084 3085 /** 3086 * @deprecated Use Reference#setResource(IBaseResource) instead 3087 */ 3088 @Deprecated 3089 public List<ReferralRequest> getIncomingReferralTarget() { 3090 if (this.incomingReferralTarget == null) 3091 this.incomingReferralTarget = new ArrayList<ReferralRequest>(); 3092 return this.incomingReferralTarget; 3093 } 3094 3095 /** 3096 * @deprecated Use Reference#setResource(IBaseResource) instead 3097 */ 3098 @Deprecated 3099 public ReferralRequest addIncomingReferralTarget() { 3100 ReferralRequest r = new ReferralRequest(); 3101 if (this.incomingReferralTarget == null) 3102 this.incomingReferralTarget = new ArrayList<ReferralRequest>(); 3103 this.incomingReferralTarget.add(r); 3104 return r; 3105 } 3106 3107 /** 3108 * @return {@link #participant} (The list of people responsible for providing the service.) 3109 */ 3110 public List<EncounterParticipantComponent> getParticipant() { 3111 if (this.participant == null) 3112 this.participant = new ArrayList<EncounterParticipantComponent>(); 3113 return this.participant; 3114 } 3115 3116 /** 3117 * @return Returns a reference to <code>this</code> for easy method chaining 3118 */ 3119 public Encounter setParticipant(List<EncounterParticipantComponent> theParticipant) { 3120 this.participant = theParticipant; 3121 return this; 3122 } 3123 3124 public boolean hasParticipant() { 3125 if (this.participant == null) 3126 return false; 3127 for (EncounterParticipantComponent item : this.participant) 3128 if (!item.isEmpty()) 3129 return true; 3130 return false; 3131 } 3132 3133 public EncounterParticipantComponent addParticipant() { //3 3134 EncounterParticipantComponent t = new EncounterParticipantComponent(); 3135 if (this.participant == null) 3136 this.participant = new ArrayList<EncounterParticipantComponent>(); 3137 this.participant.add(t); 3138 return t; 3139 } 3140 3141 public Encounter addParticipant(EncounterParticipantComponent t) { //3 3142 if (t == null) 3143 return this; 3144 if (this.participant == null) 3145 this.participant = new ArrayList<EncounterParticipantComponent>(); 3146 this.participant.add(t); 3147 return this; 3148 } 3149 3150 /** 3151 * @return The first repetition of repeating field {@link #participant}, creating it if it does not already exist 3152 */ 3153 public EncounterParticipantComponent getParticipantFirstRep() { 3154 if (getParticipant().isEmpty()) { 3155 addParticipant(); 3156 } 3157 return getParticipant().get(0); 3158 } 3159 3160 /** 3161 * @return {@link #appointment} (The appointment that scheduled this encounter.) 3162 */ 3163 public Reference getAppointment() { 3164 if (this.appointment == null) 3165 if (Configuration.errorOnAutoCreate()) 3166 throw new Error("Attempt to auto-create Encounter.appointment"); 3167 else if (Configuration.doAutoCreate()) 3168 this.appointment = new Reference(); // cc 3169 return this.appointment; 3170 } 3171 3172 public boolean hasAppointment() { 3173 return this.appointment != null && !this.appointment.isEmpty(); 3174 } 3175 3176 /** 3177 * @param value {@link #appointment} (The appointment that scheduled this encounter.) 3178 */ 3179 public Encounter setAppointment(Reference value) { 3180 this.appointment = value; 3181 return this; 3182 } 3183 3184 /** 3185 * @return {@link #appointment} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The appointment that scheduled this encounter.) 3186 */ 3187 public Appointment getAppointmentTarget() { 3188 if (this.appointmentTarget == null) 3189 if (Configuration.errorOnAutoCreate()) 3190 throw new Error("Attempt to auto-create Encounter.appointment"); 3191 else if (Configuration.doAutoCreate()) 3192 this.appointmentTarget = new Appointment(); // aa 3193 return this.appointmentTarget; 3194 } 3195 3196 /** 3197 * @param value {@link #appointment} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The appointment that scheduled this encounter.) 3198 */ 3199 public Encounter setAppointmentTarget(Appointment value) { 3200 this.appointmentTarget = value; 3201 return this; 3202 } 3203 3204 /** 3205 * @return {@link #period} (The start and end time of the encounter.) 3206 */ 3207 public Period getPeriod() { 3208 if (this.period == null) 3209 if (Configuration.errorOnAutoCreate()) 3210 throw new Error("Attempt to auto-create Encounter.period"); 3211 else if (Configuration.doAutoCreate()) 3212 this.period = new Period(); // cc 3213 return this.period; 3214 } 3215 3216 public boolean hasPeriod() { 3217 return this.period != null && !this.period.isEmpty(); 3218 } 3219 3220 /** 3221 * @param value {@link #period} (The start and end time of the encounter.) 3222 */ 3223 public Encounter setPeriod(Period value) { 3224 this.period = value; 3225 return this; 3226 } 3227 3228 /** 3229 * @return {@link #length} (Quantity of time the encounter lasted. This excludes the time during leaves of absence.) 3230 */ 3231 public Duration getLength() { 3232 if (this.length == null) 3233 if (Configuration.errorOnAutoCreate()) 3234 throw new Error("Attempt to auto-create Encounter.length"); 3235 else if (Configuration.doAutoCreate()) 3236 this.length = new Duration(); // cc 3237 return this.length; 3238 } 3239 3240 public boolean hasLength() { 3241 return this.length != null && !this.length.isEmpty(); 3242 } 3243 3244 /** 3245 * @param value {@link #length} (Quantity of time the encounter lasted. This excludes the time during leaves of absence.) 3246 */ 3247 public Encounter setLength(Duration value) { 3248 this.length = value; 3249 return this; 3250 } 3251 3252 /** 3253 * @return {@link #reason} (Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.) 3254 */ 3255 public List<CodeableConcept> getReason() { 3256 if (this.reason == null) 3257 this.reason = new ArrayList<CodeableConcept>(); 3258 return this.reason; 3259 } 3260 3261 /** 3262 * @return Returns a reference to <code>this</code> for easy method chaining 3263 */ 3264 public Encounter setReason(List<CodeableConcept> theReason) { 3265 this.reason = theReason; 3266 return this; 3267 } 3268 3269 public boolean hasReason() { 3270 if (this.reason == null) 3271 return false; 3272 for (CodeableConcept item : this.reason) 3273 if (!item.isEmpty()) 3274 return true; 3275 return false; 3276 } 3277 3278 public CodeableConcept addReason() { //3 3279 CodeableConcept t = new CodeableConcept(); 3280 if (this.reason == null) 3281 this.reason = new ArrayList<CodeableConcept>(); 3282 this.reason.add(t); 3283 return t; 3284 } 3285 3286 public Encounter addReason(CodeableConcept t) { //3 3287 if (t == null) 3288 return this; 3289 if (this.reason == null) 3290 this.reason = new ArrayList<CodeableConcept>(); 3291 this.reason.add(t); 3292 return this; 3293 } 3294 3295 /** 3296 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist 3297 */ 3298 public CodeableConcept getReasonFirstRep() { 3299 if (getReason().isEmpty()) { 3300 addReason(); 3301 } 3302 return getReason().get(0); 3303 } 3304 3305 /** 3306 * @return {@link #diagnosis} (The list of diagnosis relevant to this encounter.) 3307 */ 3308 public List<DiagnosisComponent> getDiagnosis() { 3309 if (this.diagnosis == null) 3310 this.diagnosis = new ArrayList<DiagnosisComponent>(); 3311 return this.diagnosis; 3312 } 3313 3314 /** 3315 * @return Returns a reference to <code>this</code> for easy method chaining 3316 */ 3317 public Encounter setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 3318 this.diagnosis = theDiagnosis; 3319 return this; 3320 } 3321 3322 public boolean hasDiagnosis() { 3323 if (this.diagnosis == null) 3324 return false; 3325 for (DiagnosisComponent item : this.diagnosis) 3326 if (!item.isEmpty()) 3327 return true; 3328 return false; 3329 } 3330 3331 public DiagnosisComponent addDiagnosis() { //3 3332 DiagnosisComponent t = new DiagnosisComponent(); 3333 if (this.diagnosis == null) 3334 this.diagnosis = new ArrayList<DiagnosisComponent>(); 3335 this.diagnosis.add(t); 3336 return t; 3337 } 3338 3339 public Encounter addDiagnosis(DiagnosisComponent t) { //3 3340 if (t == null) 3341 return this; 3342 if (this.diagnosis == null) 3343 this.diagnosis = new ArrayList<DiagnosisComponent>(); 3344 this.diagnosis.add(t); 3345 return this; 3346 } 3347 3348 /** 3349 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist 3350 */ 3351 public DiagnosisComponent getDiagnosisFirstRep() { 3352 if (getDiagnosis().isEmpty()) { 3353 addDiagnosis(); 3354 } 3355 return getDiagnosis().get(0); 3356 } 3357 3358 /** 3359 * @return {@link #account} (The set of accounts that may be used for billing for this Encounter.) 3360 */ 3361 public List<Reference> getAccount() { 3362 if (this.account == null) 3363 this.account = new ArrayList<Reference>(); 3364 return this.account; 3365 } 3366 3367 /** 3368 * @return Returns a reference to <code>this</code> for easy method chaining 3369 */ 3370 public Encounter setAccount(List<Reference> theAccount) { 3371 this.account = theAccount; 3372 return this; 3373 } 3374 3375 public boolean hasAccount() { 3376 if (this.account == null) 3377 return false; 3378 for (Reference item : this.account) 3379 if (!item.isEmpty()) 3380 return true; 3381 return false; 3382 } 3383 3384 public Reference addAccount() { //3 3385 Reference t = new Reference(); 3386 if (this.account == null) 3387 this.account = new ArrayList<Reference>(); 3388 this.account.add(t); 3389 return t; 3390 } 3391 3392 public Encounter addAccount(Reference t) { //3 3393 if (t == null) 3394 return this; 3395 if (this.account == null) 3396 this.account = new ArrayList<Reference>(); 3397 this.account.add(t); 3398 return this; 3399 } 3400 3401 /** 3402 * @return The first repetition of repeating field {@link #account}, creating it if it does not already exist 3403 */ 3404 public Reference getAccountFirstRep() { 3405 if (getAccount().isEmpty()) { 3406 addAccount(); 3407 } 3408 return getAccount().get(0); 3409 } 3410 3411 /** 3412 * @deprecated Use Reference#setResource(IBaseResource) instead 3413 */ 3414 @Deprecated 3415 public List<Account> getAccountTarget() { 3416 if (this.accountTarget == null) 3417 this.accountTarget = new ArrayList<Account>(); 3418 return this.accountTarget; 3419 } 3420 3421 /** 3422 * @deprecated Use Reference#setResource(IBaseResource) instead 3423 */ 3424 @Deprecated 3425 public Account addAccountTarget() { 3426 Account r = new Account(); 3427 if (this.accountTarget == null) 3428 this.accountTarget = new ArrayList<Account>(); 3429 this.accountTarget.add(r); 3430 return r; 3431 } 3432 3433 /** 3434 * @return {@link #hospitalization} (Details about the admission to a healthcare service.) 3435 */ 3436 public EncounterHospitalizationComponent getHospitalization() { 3437 if (this.hospitalization == null) 3438 if (Configuration.errorOnAutoCreate()) 3439 throw new Error("Attempt to auto-create Encounter.hospitalization"); 3440 else if (Configuration.doAutoCreate()) 3441 this.hospitalization = new EncounterHospitalizationComponent(); // cc 3442 return this.hospitalization; 3443 } 3444 3445 public boolean hasHospitalization() { 3446 return this.hospitalization != null && !this.hospitalization.isEmpty(); 3447 } 3448 3449 /** 3450 * @param value {@link #hospitalization} (Details about the admission to a healthcare service.) 3451 */ 3452 public Encounter setHospitalization(EncounterHospitalizationComponent value) { 3453 this.hospitalization = value; 3454 return this; 3455 } 3456 3457 /** 3458 * @return {@link #location} (List of locations where the patient has been during this encounter.) 3459 */ 3460 public List<EncounterLocationComponent> getLocation() { 3461 if (this.location == null) 3462 this.location = new ArrayList<EncounterLocationComponent>(); 3463 return this.location; 3464 } 3465 3466 /** 3467 * @return Returns a reference to <code>this</code> for easy method chaining 3468 */ 3469 public Encounter setLocation(List<EncounterLocationComponent> theLocation) { 3470 this.location = theLocation; 3471 return this; 3472 } 3473 3474 public boolean hasLocation() { 3475 if (this.location == null) 3476 return false; 3477 for (EncounterLocationComponent item : this.location) 3478 if (!item.isEmpty()) 3479 return true; 3480 return false; 3481 } 3482 3483 public EncounterLocationComponent addLocation() { //3 3484 EncounterLocationComponent t = new EncounterLocationComponent(); 3485 if (this.location == null) 3486 this.location = new ArrayList<EncounterLocationComponent>(); 3487 this.location.add(t); 3488 return t; 3489 } 3490 3491 public Encounter addLocation(EncounterLocationComponent t) { //3 3492 if (t == null) 3493 return this; 3494 if (this.location == null) 3495 this.location = new ArrayList<EncounterLocationComponent>(); 3496 this.location.add(t); 3497 return this; 3498 } 3499 3500 /** 3501 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist 3502 */ 3503 public EncounterLocationComponent getLocationFirstRep() { 3504 if (getLocation().isEmpty()) { 3505 addLocation(); 3506 } 3507 return getLocation().get(0); 3508 } 3509 3510 /** 3511 * @return {@link #serviceProvider} (An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.) 3512 */ 3513 public Reference getServiceProvider() { 3514 if (this.serviceProvider == null) 3515 if (Configuration.errorOnAutoCreate()) 3516 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 3517 else if (Configuration.doAutoCreate()) 3518 this.serviceProvider = new Reference(); // cc 3519 return this.serviceProvider; 3520 } 3521 3522 public boolean hasServiceProvider() { 3523 return this.serviceProvider != null && !this.serviceProvider.isEmpty(); 3524 } 3525 3526 /** 3527 * @param value {@link #serviceProvider} (An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.) 3528 */ 3529 public Encounter setServiceProvider(Reference value) { 3530 this.serviceProvider = value; 3531 return this; 3532 } 3533 3534 /** 3535 * @return {@link #serviceProvider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.) 3536 */ 3537 public Organization getServiceProviderTarget() { 3538 if (this.serviceProviderTarget == null) 3539 if (Configuration.errorOnAutoCreate()) 3540 throw new Error("Attempt to auto-create Encounter.serviceProvider"); 3541 else if (Configuration.doAutoCreate()) 3542 this.serviceProviderTarget = new Organization(); // aa 3543 return this.serviceProviderTarget; 3544 } 3545 3546 /** 3547 * @param value {@link #serviceProvider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.) 3548 */ 3549 public Encounter setServiceProviderTarget(Organization value) { 3550 this.serviceProviderTarget = value; 3551 return this; 3552 } 3553 3554 /** 3555 * @return {@link #partOf} (Another Encounter of which this encounter is a part of (administratively or in time).) 3556 */ 3557 public Reference getPartOf() { 3558 if (this.partOf == null) 3559 if (Configuration.errorOnAutoCreate()) 3560 throw new Error("Attempt to auto-create Encounter.partOf"); 3561 else if (Configuration.doAutoCreate()) 3562 this.partOf = new Reference(); // cc 3563 return this.partOf; 3564 } 3565 3566 public boolean hasPartOf() { 3567 return this.partOf != null && !this.partOf.isEmpty(); 3568 } 3569 3570 /** 3571 * @param value {@link #partOf} (Another Encounter of which this encounter is a part of (administratively or in time).) 3572 */ 3573 public Encounter setPartOf(Reference value) { 3574 this.partOf = value; 3575 return this; 3576 } 3577 3578 /** 3579 * @return {@link #partOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Another Encounter of which this encounter is a part of (administratively or in time).) 3580 */ 3581 public Encounter getPartOfTarget() { 3582 if (this.partOfTarget == null) 3583 if (Configuration.errorOnAutoCreate()) 3584 throw new Error("Attempt to auto-create Encounter.partOf"); 3585 else if (Configuration.doAutoCreate()) 3586 this.partOfTarget = new Encounter(); // aa 3587 return this.partOfTarget; 3588 } 3589 3590 /** 3591 * @param value {@link #partOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Another Encounter of which this encounter is a part of (administratively or in time).) 3592 */ 3593 public Encounter setPartOfTarget(Encounter value) { 3594 this.partOfTarget = value; 3595 return this; 3596 } 3597 3598 protected void listChildren(List<Property> children) { 3599 super.listChildren(children); 3600 children.add(new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3601 children.add(new Property("status", "code", "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status)); 3602 children.add(new Property("statusHistory", "", "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.", 0, java.lang.Integer.MAX_VALUE, statusHistory)); 3603 children.add(new Property("class", "Coding", "inpatient | outpatient | ambulatory | emergency +.", 0, 1, class_)); 3604 children.add(new Property("classHistory", "", "The class history permits the tracking of the encounters transitions without needing to go through the resource history.\n\nThis would be used for a case where an admission starts of as an emergency encounter, then transisions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kindof discharge from emergency to inpatient.", 0, java.lang.Integer.MAX_VALUE, classHistory)); 3605 children.add(new Property("type", "CodeableConcept", "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, java.lang.Integer.MAX_VALUE, type)); 3606 children.add(new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 1, priority)); 3607 children.add(new Property("subject", "Reference(Patient|Group)", "The patient ro group present at the encounter.", 0, 1, subject)); 3608 children.add(new Property("episodeOfCare", "Reference(EpisodeOfCare)", "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care, and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 0, java.lang.Integer.MAX_VALUE, episodeOfCare)); 3609 children.add(new Property("incomingReferral", "Reference(ReferralRequest)", "The referral request this encounter satisfies (incoming referral).", 0, java.lang.Integer.MAX_VALUE, incomingReferral)); 3610 children.add(new Property("participant", "", "The list of people responsible for providing the service.", 0, java.lang.Integer.MAX_VALUE, participant)); 3611 children.add(new Property("appointment", "Reference(Appointment)", "The appointment that scheduled this encounter.", 0, 1, appointment)); 3612 children.add(new Property("period", "Period", "The start and end time of the encounter.", 0, 1, period)); 3613 children.add(new Property("length", "Duration", "Quantity of time the encounter lasted. This excludes the time during leaves of absence.", 0, 1, length)); 3614 children.add(new Property("reason", "CodeableConcept", "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 0, java.lang.Integer.MAX_VALUE, reason)); 3615 children.add(new Property("diagnosis", "", "The list of diagnosis relevant to this encounter.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 3616 children.add(new Property("account", "Reference(Account)", "The set of accounts that may be used for billing for this Encounter.", 0, java.lang.Integer.MAX_VALUE, account)); 3617 children.add(new Property("hospitalization", "", "Details about the admission to a healthcare service.", 0, 1, hospitalization)); 3618 children.add(new Property("location", "", "List of locations where the patient has been during this encounter.", 0, java.lang.Integer.MAX_VALUE, location)); 3619 children.add(new Property("serviceProvider", "Reference(Organization)", "An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.", 0, 1, serviceProvider)); 3620 children.add(new Property("partOf", "Reference(Encounter)", "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 1, partOf)); 3621 } 3622 3623 @Override 3624 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3625 switch (_hash) { 3626 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier(s) by which this encounter is known.", 0, java.lang.Integer.MAX_VALUE, identifier); 3627 case -892481550: /*status*/ return new Property("status", "code", "planned | arrived | triaged | in-progress | onleave | finished | cancelled +.", 0, 1, status); 3628 case -986695614: /*statusHistory*/ return new Property("statusHistory", "", "The status history permits the encounter resource to contain the status history without needing to read through the historical versions of the resource, or even have the server store them.", 0, java.lang.Integer.MAX_VALUE, statusHistory); 3629 case 94742904: /*class*/ return new Property("class", "Coding", "inpatient | outpatient | ambulatory | emergency +.", 0, 1, class_); 3630 case 962575356: /*classHistory*/ return new Property("classHistory", "", "The class history permits the tracking of the encounters transitions without needing to go through the resource history.\n\nThis would be used for a case where an admission starts of as an emergency encounter, then transisions into an inpatient scenario. Doing this and not restarting a new encounter ensures that any lab/diagnostic results can more easily follow the patient and not require re-processing and not get lost or cancelled during a kindof discharge from emergency to inpatient.", 0, java.lang.Integer.MAX_VALUE, classHistory); 3631 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Specific type of encounter (e.g. e-mail consultation, surgical day-care, skilled nursing, rehabilitation).", 0, java.lang.Integer.MAX_VALUE, type); 3632 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Indicates the urgency of the encounter.", 0, 1, priority); 3633 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient ro group present at the encounter.", 0, 1, subject); 3634 case -1892140189: /*episodeOfCare*/ return new Property("episodeOfCare", "Reference(EpisodeOfCare)", "Where a specific encounter should be classified as a part of a specific episode(s) of care this field should be used. This association can facilitate grouping of related encounters together for a specific purpose, such as government reporting, issue tracking, association via a common problem. The association is recorded on the encounter as these are typically created after the episode of care, and grouped on entry rather than editing the episode of care to append another encounter to it (the episode of care could span years).", 0, java.lang.Integer.MAX_VALUE, episodeOfCare); 3635 case -1258204701: /*incomingReferral*/ return new Property("incomingReferral", "Reference(ReferralRequest)", "The referral request this encounter satisfies (incoming referral).", 0, java.lang.Integer.MAX_VALUE, incomingReferral); 3636 case 767422259: /*participant*/ return new Property("participant", "", "The list of people responsible for providing the service.", 0, java.lang.Integer.MAX_VALUE, participant); 3637 case -1474995297: /*appointment*/ return new Property("appointment", "Reference(Appointment)", "The appointment that scheduled this encounter.", 0, 1, appointment); 3638 case -991726143: /*period*/ return new Property("period", "Period", "The start and end time of the encounter.", 0, 1, period); 3639 case -1106363674: /*length*/ return new Property("length", "Duration", "Quantity of time the encounter lasted. This excludes the time during leaves of absence.", 0, 1, length); 3640 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Reason the encounter takes place, expressed as a code. For admissions, this can be used for a coded admission diagnosis.", 0, java.lang.Integer.MAX_VALUE, reason); 3641 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "The list of diagnosis relevant to this encounter.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 3642 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "The set of accounts that may be used for billing for this Encounter.", 0, java.lang.Integer.MAX_VALUE, account); 3643 case 1057894634: /*hospitalization*/ return new Property("hospitalization", "", "Details about the admission to a healthcare service.", 0, 1, hospitalization); 3644 case 1901043637: /*location*/ return new Property("location", "", "List of locations where the patient has been during this encounter.", 0, java.lang.Integer.MAX_VALUE, location); 3645 case 243182534: /*serviceProvider*/ return new Property("serviceProvider", "Reference(Organization)", "An organization that is in charge of maintaining the information of this Encounter (e.g. who maintains the report or the master service catalog item, etc.). This MAY be the same as the organization on the Patient record, however it could be different. This MAY not be not the Service Delivery Location's Organization.", 0, 1, serviceProvider); 3646 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Encounter)", "Another Encounter of which this encounter is a part of (administratively or in time).", 0, 1, partOf); 3647 default: return super.getNamedProperty(_hash, _name, _checkValid); 3648 } 3649 3650 } 3651 3652 @Override 3653 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3654 switch (hash) { 3655 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3656 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EncounterStatus> 3657 case -986695614: /*statusHistory*/ return this.statusHistory == null ? new Base[0] : this.statusHistory.toArray(new Base[this.statusHistory.size()]); // StatusHistoryComponent 3658 case 94742904: /*class*/ return this.class_ == null ? new Base[0] : new Base[] {this.class_}; // Coding 3659 case 962575356: /*classHistory*/ return this.classHistory == null ? new Base[0] : this.classHistory.toArray(new Base[this.classHistory.size()]); // ClassHistoryComponent 3660 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3661 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 3662 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 3663 case -1892140189: /*episodeOfCare*/ return this.episodeOfCare == null ? new Base[0] : this.episodeOfCare.toArray(new Base[this.episodeOfCare.size()]); // Reference 3664 case -1258204701: /*incomingReferral*/ return this.incomingReferral == null ? new Base[0] : this.incomingReferral.toArray(new Base[this.incomingReferral.size()]); // Reference 3665 case 767422259: /*participant*/ return this.participant == null ? new Base[0] : this.participant.toArray(new Base[this.participant.size()]); // EncounterParticipantComponent 3666 case -1474995297: /*appointment*/ return this.appointment == null ? new Base[0] : new Base[] {this.appointment}; // Reference 3667 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 3668 case -1106363674: /*length*/ return this.length == null ? new Base[0] : new Base[] {this.length}; // Duration 3669 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 3670 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 3671 case -1177318867: /*account*/ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 3672 case 1057894634: /*hospitalization*/ return this.hospitalization == null ? new Base[0] : new Base[] {this.hospitalization}; // EncounterHospitalizationComponent 3673 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // EncounterLocationComponent 3674 case 243182534: /*serviceProvider*/ return this.serviceProvider == null ? new Base[0] : new Base[] {this.serviceProvider}; // Reference 3675 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : new Base[] {this.partOf}; // Reference 3676 default: return super.getProperty(hash, name, checkValid); 3677 } 3678 3679 } 3680 3681 @Override 3682 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3683 switch (hash) { 3684 case -1618432855: // identifier 3685 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3686 return value; 3687 case -892481550: // status 3688 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 3689 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 3690 return value; 3691 case -986695614: // statusHistory 3692 this.getStatusHistory().add((StatusHistoryComponent) value); // StatusHistoryComponent 3693 return value; 3694 case 94742904: // class 3695 this.class_ = castToCoding(value); // Coding 3696 return value; 3697 case 962575356: // classHistory 3698 this.getClassHistory().add((ClassHistoryComponent) value); // ClassHistoryComponent 3699 return value; 3700 case 3575610: // type 3701 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 3702 return value; 3703 case -1165461084: // priority 3704 this.priority = castToCodeableConcept(value); // CodeableConcept 3705 return value; 3706 case -1867885268: // subject 3707 this.subject = castToReference(value); // Reference 3708 return value; 3709 case -1892140189: // episodeOfCare 3710 this.getEpisodeOfCare().add(castToReference(value)); // Reference 3711 return value; 3712 case -1258204701: // incomingReferral 3713 this.getIncomingReferral().add(castToReference(value)); // Reference 3714 return value; 3715 case 767422259: // participant 3716 this.getParticipant().add((EncounterParticipantComponent) value); // EncounterParticipantComponent 3717 return value; 3718 case -1474995297: // appointment 3719 this.appointment = castToReference(value); // Reference 3720 return value; 3721 case -991726143: // period 3722 this.period = castToPeriod(value); // Period 3723 return value; 3724 case -1106363674: // length 3725 this.length = castToDuration(value); // Duration 3726 return value; 3727 case -934964668: // reason 3728 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 3729 return value; 3730 case 1196993265: // diagnosis 3731 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 3732 return value; 3733 case -1177318867: // account 3734 this.getAccount().add(castToReference(value)); // Reference 3735 return value; 3736 case 1057894634: // hospitalization 3737 this.hospitalization = (EncounterHospitalizationComponent) value; // EncounterHospitalizationComponent 3738 return value; 3739 case 1901043637: // location 3740 this.getLocation().add((EncounterLocationComponent) value); // EncounterLocationComponent 3741 return value; 3742 case 243182534: // serviceProvider 3743 this.serviceProvider = castToReference(value); // Reference 3744 return value; 3745 case -995410646: // partOf 3746 this.partOf = castToReference(value); // Reference 3747 return value; 3748 default: return super.setProperty(hash, name, value); 3749 } 3750 3751 } 3752 3753 @Override 3754 public Base setProperty(String name, Base value) throws FHIRException { 3755 if (name.equals("identifier")) { 3756 this.getIdentifier().add(castToIdentifier(value)); 3757 } else if (name.equals("status")) { 3758 value = new EncounterStatusEnumFactory().fromType(castToCode(value)); 3759 this.status = (Enumeration) value; // Enumeration<EncounterStatus> 3760 } else if (name.equals("statusHistory")) { 3761 this.getStatusHistory().add((StatusHistoryComponent) value); 3762 } else if (name.equals("class")) { 3763 this.class_ = castToCoding(value); // Coding 3764 } else if (name.equals("classHistory")) { 3765 this.getClassHistory().add((ClassHistoryComponent) value); 3766 } else if (name.equals("type")) { 3767 this.getType().add(castToCodeableConcept(value)); 3768 } else if (name.equals("priority")) { 3769 this.priority = castToCodeableConcept(value); // CodeableConcept 3770 } else if (name.equals("subject")) { 3771 this.subject = castToReference(value); // Reference 3772 } else if (name.equals("episodeOfCare")) { 3773 this.getEpisodeOfCare().add(castToReference(value)); 3774 } else if (name.equals("incomingReferral")) { 3775 this.getIncomingReferral().add(castToReference(value)); 3776 } else if (name.equals("participant")) { 3777 this.getParticipant().add((EncounterParticipantComponent) value); 3778 } else if (name.equals("appointment")) { 3779 this.appointment = castToReference(value); // Reference 3780 } else if (name.equals("period")) { 3781 this.period = castToPeriod(value); // Period 3782 } else if (name.equals("length")) { 3783 this.length = castToDuration(value); // Duration 3784 } else if (name.equals("reason")) { 3785 this.getReason().add(castToCodeableConcept(value)); 3786 } else if (name.equals("diagnosis")) { 3787 this.getDiagnosis().add((DiagnosisComponent) value); 3788 } else if (name.equals("account")) { 3789 this.getAccount().add(castToReference(value)); 3790 } else if (name.equals("hospitalization")) { 3791 this.hospitalization = (EncounterHospitalizationComponent) value; // EncounterHospitalizationComponent 3792 } else if (name.equals("location")) { 3793 this.getLocation().add((EncounterLocationComponent) value); 3794 } else if (name.equals("serviceProvider")) { 3795 this.serviceProvider = castToReference(value); // Reference 3796 } else if (name.equals("partOf")) { 3797 this.partOf = castToReference(value); // Reference 3798 } else 3799 return super.setProperty(name, value); 3800 return value; 3801 } 3802 3803 @Override 3804 public Base makeProperty(int hash, String name) throws FHIRException { 3805 switch (hash) { 3806 case -1618432855: return addIdentifier(); 3807 case -892481550: return getStatusElement(); 3808 case -986695614: return addStatusHistory(); 3809 case 94742904: return getClass_(); 3810 case 962575356: return addClassHistory(); 3811 case 3575610: return addType(); 3812 case -1165461084: return getPriority(); 3813 case -1867885268: return getSubject(); 3814 case -1892140189: return addEpisodeOfCare(); 3815 case -1258204701: return addIncomingReferral(); 3816 case 767422259: return addParticipant(); 3817 case -1474995297: return getAppointment(); 3818 case -991726143: return getPeriod(); 3819 case -1106363674: return getLength(); 3820 case -934964668: return addReason(); 3821 case 1196993265: return addDiagnosis(); 3822 case -1177318867: return addAccount(); 3823 case 1057894634: return getHospitalization(); 3824 case 1901043637: return addLocation(); 3825 case 243182534: return getServiceProvider(); 3826 case -995410646: return getPartOf(); 3827 default: return super.makeProperty(hash, name); 3828 } 3829 3830 } 3831 3832 @Override 3833 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3834 switch (hash) { 3835 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3836 case -892481550: /*status*/ return new String[] {"code"}; 3837 case -986695614: /*statusHistory*/ return new String[] {}; 3838 case 94742904: /*class*/ return new String[] {"Coding"}; 3839 case 962575356: /*classHistory*/ return new String[] {}; 3840 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 3841 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 3842 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3843 case -1892140189: /*episodeOfCare*/ return new String[] {"Reference"}; 3844 case -1258204701: /*incomingReferral*/ return new String[] {"Reference"}; 3845 case 767422259: /*participant*/ return new String[] {}; 3846 case -1474995297: /*appointment*/ return new String[] {"Reference"}; 3847 case -991726143: /*period*/ return new String[] {"Period"}; 3848 case -1106363674: /*length*/ return new String[] {"Duration"}; 3849 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 3850 case 1196993265: /*diagnosis*/ return new String[] {}; 3851 case -1177318867: /*account*/ return new String[] {"Reference"}; 3852 case 1057894634: /*hospitalization*/ return new String[] {}; 3853 case 1901043637: /*location*/ return new String[] {}; 3854 case 243182534: /*serviceProvider*/ return new String[] {"Reference"}; 3855 case -995410646: /*partOf*/ return new String[] {"Reference"}; 3856 default: return super.getTypesForProperty(hash, name); 3857 } 3858 3859 } 3860 3861 @Override 3862 public Base addChild(String name) throws FHIRException { 3863 if (name.equals("identifier")) { 3864 return addIdentifier(); 3865 } 3866 else if (name.equals("status")) { 3867 throw new FHIRException("Cannot call addChild on a singleton property Encounter.status"); 3868 } 3869 else if (name.equals("statusHistory")) { 3870 return addStatusHistory(); 3871 } 3872 else if (name.equals("class")) { 3873 this.class_ = new Coding(); 3874 return this.class_; 3875 } 3876 else if (name.equals("classHistory")) { 3877 return addClassHistory(); 3878 } 3879 else if (name.equals("type")) { 3880 return addType(); 3881 } 3882 else if (name.equals("priority")) { 3883 this.priority = new CodeableConcept(); 3884 return this.priority; 3885 } 3886 else if (name.equals("subject")) { 3887 this.subject = new Reference(); 3888 return this.subject; 3889 } 3890 else if (name.equals("episodeOfCare")) { 3891 return addEpisodeOfCare(); 3892 } 3893 else if (name.equals("incomingReferral")) { 3894 return addIncomingReferral(); 3895 } 3896 else if (name.equals("participant")) { 3897 return addParticipant(); 3898 } 3899 else if (name.equals("appointment")) { 3900 this.appointment = new Reference(); 3901 return this.appointment; 3902 } 3903 else if (name.equals("period")) { 3904 this.period = new Period(); 3905 return this.period; 3906 } 3907 else if (name.equals("length")) { 3908 this.length = new Duration(); 3909 return this.length; 3910 } 3911 else if (name.equals("reason")) { 3912 return addReason(); 3913 } 3914 else if (name.equals("diagnosis")) { 3915 return addDiagnosis(); 3916 } 3917 else if (name.equals("account")) { 3918 return addAccount(); 3919 } 3920 else if (name.equals("hospitalization")) { 3921 this.hospitalization = new EncounterHospitalizationComponent(); 3922 return this.hospitalization; 3923 } 3924 else if (name.equals("location")) { 3925 return addLocation(); 3926 } 3927 else if (name.equals("serviceProvider")) { 3928 this.serviceProvider = new Reference(); 3929 return this.serviceProvider; 3930 } 3931 else if (name.equals("partOf")) { 3932 this.partOf = new Reference(); 3933 return this.partOf; 3934 } 3935 else 3936 return super.addChild(name); 3937 } 3938 3939 public String fhirType() { 3940 return "Encounter"; 3941 3942 } 3943 3944 public Encounter copy() { 3945 Encounter dst = new Encounter(); 3946 copyValues(dst); 3947 if (identifier != null) { 3948 dst.identifier = new ArrayList<Identifier>(); 3949 for (Identifier i : identifier) 3950 dst.identifier.add(i.copy()); 3951 }; 3952 dst.status = status == null ? null : status.copy(); 3953 if (statusHistory != null) { 3954 dst.statusHistory = new ArrayList<StatusHistoryComponent>(); 3955 for (StatusHistoryComponent i : statusHistory) 3956 dst.statusHistory.add(i.copy()); 3957 }; 3958 dst.class_ = class_ == null ? null : class_.copy(); 3959 if (classHistory != null) { 3960 dst.classHistory = new ArrayList<ClassHistoryComponent>(); 3961 for (ClassHistoryComponent i : classHistory) 3962 dst.classHistory.add(i.copy()); 3963 }; 3964 if (type != null) { 3965 dst.type = new ArrayList<CodeableConcept>(); 3966 for (CodeableConcept i : type) 3967 dst.type.add(i.copy()); 3968 }; 3969 dst.priority = priority == null ? null : priority.copy(); 3970 dst.subject = subject == null ? null : subject.copy(); 3971 if (episodeOfCare != null) { 3972 dst.episodeOfCare = new ArrayList<Reference>(); 3973 for (Reference i : episodeOfCare) 3974 dst.episodeOfCare.add(i.copy()); 3975 }; 3976 if (incomingReferral != null) { 3977 dst.incomingReferral = new ArrayList<Reference>(); 3978 for (Reference i : incomingReferral) 3979 dst.incomingReferral.add(i.copy()); 3980 }; 3981 if (participant != null) { 3982 dst.participant = new ArrayList<EncounterParticipantComponent>(); 3983 for (EncounterParticipantComponent i : participant) 3984 dst.participant.add(i.copy()); 3985 }; 3986 dst.appointment = appointment == null ? null : appointment.copy(); 3987 dst.period = period == null ? null : period.copy(); 3988 dst.length = length == null ? null : length.copy(); 3989 if (reason != null) { 3990 dst.reason = new ArrayList<CodeableConcept>(); 3991 for (CodeableConcept i : reason) 3992 dst.reason.add(i.copy()); 3993 }; 3994 if (diagnosis != null) { 3995 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 3996 for (DiagnosisComponent i : diagnosis) 3997 dst.diagnosis.add(i.copy()); 3998 }; 3999 if (account != null) { 4000 dst.account = new ArrayList<Reference>(); 4001 for (Reference i : account) 4002 dst.account.add(i.copy()); 4003 }; 4004 dst.hospitalization = hospitalization == null ? null : hospitalization.copy(); 4005 if (location != null) { 4006 dst.location = new ArrayList<EncounterLocationComponent>(); 4007 for (EncounterLocationComponent i : location) 4008 dst.location.add(i.copy()); 4009 }; 4010 dst.serviceProvider = serviceProvider == null ? null : serviceProvider.copy(); 4011 dst.partOf = partOf == null ? null : partOf.copy(); 4012 return dst; 4013 } 4014 4015 protected Encounter typedCopy() { 4016 return copy(); 4017 } 4018 4019 @Override 4020 public boolean equalsDeep(Base other_) { 4021 if (!super.equalsDeep(other_)) 4022 return false; 4023 if (!(other_ instanceof Encounter)) 4024 return false; 4025 Encounter o = (Encounter) other_; 4026 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(statusHistory, o.statusHistory, true) 4027 && compareDeep(class_, o.class_, true) && compareDeep(classHistory, o.classHistory, true) && compareDeep(type, o.type, true) 4028 && compareDeep(priority, o.priority, true) && compareDeep(subject, o.subject, true) && compareDeep(episodeOfCare, o.episodeOfCare, true) 4029 && compareDeep(incomingReferral, o.incomingReferral, true) && compareDeep(participant, o.participant, true) 4030 && compareDeep(appointment, o.appointment, true) && compareDeep(period, o.period, true) && compareDeep(length, o.length, true) 4031 && compareDeep(reason, o.reason, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(account, o.account, true) 4032 && compareDeep(hospitalization, o.hospitalization, true) && compareDeep(location, o.location, true) 4033 && compareDeep(serviceProvider, o.serviceProvider, true) && compareDeep(partOf, o.partOf, true) 4034 ; 4035 } 4036 4037 @Override 4038 public boolean equalsShallow(Base other_) { 4039 if (!super.equalsShallow(other_)) 4040 return false; 4041 if (!(other_ instanceof Encounter)) 4042 return false; 4043 Encounter o = (Encounter) other_; 4044 return compareValues(status, o.status, true); 4045 } 4046 4047 public boolean isEmpty() { 4048 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusHistory 4049 , class_, classHistory, type, priority, subject, episodeOfCare, incomingReferral 4050 , participant, appointment, period, length, reason, diagnosis, account, hospitalization 4051 , location, serviceProvider, partOf); 4052 } 4053 4054 @Override 4055 public ResourceType getResourceType() { 4056 return ResourceType.Encounter; 4057 } 4058 4059 /** 4060 * Search parameter: <b>date</b> 4061 * <p> 4062 * Description: <b>A date within the period the Encounter lasted</b><br> 4063 * Type: <b>date</b><br> 4064 * Path: <b>Encounter.period</b><br> 4065 * </p> 4066 */ 4067 @SearchParamDefinition(name="date", path="Encounter.period", description="A date within the period the Encounter lasted", type="date" ) 4068 public static final String SP_DATE = "date"; 4069 /** 4070 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4071 * <p> 4072 * Description: <b>A date within the period the Encounter lasted</b><br> 4073 * Type: <b>date</b><br> 4074 * Path: <b>Encounter.period</b><br> 4075 * </p> 4076 */ 4077 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4078 4079 /** 4080 * Search parameter: <b>identifier</b> 4081 * <p> 4082 * Description: <b>Identifier(s) by which this encounter is known</b><br> 4083 * Type: <b>token</b><br> 4084 * Path: <b>Encounter.identifier</b><br> 4085 * </p> 4086 */ 4087 @SearchParamDefinition(name="identifier", path="Encounter.identifier", description="Identifier(s) by which this encounter is known", type="token" ) 4088 public static final String SP_IDENTIFIER = "identifier"; 4089 /** 4090 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4091 * <p> 4092 * Description: <b>Identifier(s) by which this encounter is known</b><br> 4093 * Type: <b>token</b><br> 4094 * Path: <b>Encounter.identifier</b><br> 4095 * </p> 4096 */ 4097 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4098 4099 /** 4100 * Search parameter: <b>reason</b> 4101 * <p> 4102 * Description: <b>Reason the encounter takes place (code)</b><br> 4103 * Type: <b>token</b><br> 4104 * Path: <b>Encounter.reason</b><br> 4105 * </p> 4106 */ 4107 @SearchParamDefinition(name="reason", path="Encounter.reason", description="Reason the encounter takes place (code)", type="token" ) 4108 public static final String SP_REASON = "reason"; 4109 /** 4110 * <b>Fluent Client</b> search parameter constant for <b>reason</b> 4111 * <p> 4112 * Description: <b>Reason the encounter takes place (code)</b><br> 4113 * Type: <b>token</b><br> 4114 * Path: <b>Encounter.reason</b><br> 4115 * </p> 4116 */ 4117 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON); 4118 4119 /** 4120 * Search parameter: <b>episodeofcare</b> 4121 * <p> 4122 * Description: <b>Episode(s) of care that this encounter should be recorded against</b><br> 4123 * Type: <b>reference</b><br> 4124 * Path: <b>Encounter.episodeOfCare</b><br> 4125 * </p> 4126 */ 4127 @SearchParamDefinition(name="episodeofcare", path="Encounter.episodeOfCare", description="Episode(s) of care that this encounter should be recorded against", type="reference", target={EpisodeOfCare.class } ) 4128 public static final String SP_EPISODEOFCARE = "episodeofcare"; 4129 /** 4130 * <b>Fluent Client</b> search parameter constant for <b>episodeofcare</b> 4131 * <p> 4132 * Description: <b>Episode(s) of care that this encounter should be recorded against</b><br> 4133 * Type: <b>reference</b><br> 4134 * Path: <b>Encounter.episodeOfCare</b><br> 4135 * </p> 4136 */ 4137 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam EPISODEOFCARE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_EPISODEOFCARE); 4138 4139/** 4140 * Constant for fluent queries to be used to add include statements. Specifies 4141 * the path value of "<b>Encounter:episodeofcare</b>". 4142 */ 4143 public static final ca.uhn.fhir.model.api.Include INCLUDE_EPISODEOFCARE = new ca.uhn.fhir.model.api.Include("Encounter:episodeofcare").toLocked(); 4144 4145 /** 4146 * Search parameter: <b>participant-type</b> 4147 * <p> 4148 * Description: <b>Role of participant in encounter</b><br> 4149 * Type: <b>token</b><br> 4150 * Path: <b>Encounter.participant.type</b><br> 4151 * </p> 4152 */ 4153 @SearchParamDefinition(name="participant-type", path="Encounter.participant.type", description="Role of participant in encounter", type="token" ) 4154 public static final String SP_PARTICIPANT_TYPE = "participant-type"; 4155 /** 4156 * <b>Fluent Client</b> search parameter constant for <b>participant-type</b> 4157 * <p> 4158 * Description: <b>Role of participant in encounter</b><br> 4159 * Type: <b>token</b><br> 4160 * Path: <b>Encounter.participant.type</b><br> 4161 * </p> 4162 */ 4163 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PARTICIPANT_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PARTICIPANT_TYPE); 4164 4165 /** 4166 * Search parameter: <b>incomingreferral</b> 4167 * <p> 4168 * Description: <b>The ReferralRequest that initiated this encounter</b><br> 4169 * Type: <b>reference</b><br> 4170 * Path: <b>Encounter.incomingReferral</b><br> 4171 * </p> 4172 */ 4173 @SearchParamDefinition(name="incomingreferral", path="Encounter.incomingReferral", description="The ReferralRequest that initiated this encounter", type="reference", target={ReferralRequest.class } ) 4174 public static final String SP_INCOMINGREFERRAL = "incomingreferral"; 4175 /** 4176 * <b>Fluent Client</b> search parameter constant for <b>incomingreferral</b> 4177 * <p> 4178 * Description: <b>The ReferralRequest that initiated this encounter</b><br> 4179 * Type: <b>reference</b><br> 4180 * Path: <b>Encounter.incomingReferral</b><br> 4181 * </p> 4182 */ 4183 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INCOMINGREFERRAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INCOMINGREFERRAL); 4184 4185/** 4186 * Constant for fluent queries to be used to add include statements. Specifies 4187 * the path value of "<b>Encounter:incomingreferral</b>". 4188 */ 4189 public static final ca.uhn.fhir.model.api.Include INCLUDE_INCOMINGREFERRAL = new ca.uhn.fhir.model.api.Include("Encounter:incomingreferral").toLocked(); 4190 4191 /** 4192 * Search parameter: <b>practitioner</b> 4193 * <p> 4194 * Description: <b>Persons involved in the encounter other than the patient</b><br> 4195 * Type: <b>reference</b><br> 4196 * Path: <b>Encounter.participant.individual</b><br> 4197 * </p> 4198 */ 4199 @SearchParamDefinition(name="practitioner", path="Encounter.participant.individual", description="Persons involved in the encounter other than the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 4200 public static final String SP_PRACTITIONER = "practitioner"; 4201 /** 4202 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 4203 * <p> 4204 * Description: <b>Persons involved in the encounter other than the patient</b><br> 4205 * Type: <b>reference</b><br> 4206 * Path: <b>Encounter.participant.individual</b><br> 4207 * </p> 4208 */ 4209 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 4210 4211/** 4212 * Constant for fluent queries to be used to add include statements. Specifies 4213 * the path value of "<b>Encounter:practitioner</b>". 4214 */ 4215 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Encounter:practitioner").toLocked(); 4216 4217 /** 4218 * Search parameter: <b>subject</b> 4219 * <p> 4220 * Description: <b>The patient ro group present at the encounter</b><br> 4221 * Type: <b>reference</b><br> 4222 * Path: <b>Encounter.subject</b><br> 4223 * </p> 4224 */ 4225 @SearchParamDefinition(name="subject", path="Encounter.subject", description="The patient ro group present at the encounter", type="reference", target={Group.class, Patient.class } ) 4226 public static final String SP_SUBJECT = "subject"; 4227 /** 4228 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 4229 * <p> 4230 * Description: <b>The patient ro group present at the encounter</b><br> 4231 * Type: <b>reference</b><br> 4232 * Path: <b>Encounter.subject</b><br> 4233 * </p> 4234 */ 4235 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 4236 4237/** 4238 * Constant for fluent queries to be used to add include statements. Specifies 4239 * the path value of "<b>Encounter:subject</b>". 4240 */ 4241 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Encounter:subject").toLocked(); 4242 4243 /** 4244 * Search parameter: <b>length</b> 4245 * <p> 4246 * Description: <b>Length of encounter in days</b><br> 4247 * Type: <b>number</b><br> 4248 * Path: <b>Encounter.length</b><br> 4249 * </p> 4250 */ 4251 @SearchParamDefinition(name="length", path="Encounter.length", description="Length of encounter in days", type="number" ) 4252 public static final String SP_LENGTH = "length"; 4253 /** 4254 * <b>Fluent Client</b> search parameter constant for <b>length</b> 4255 * <p> 4256 * Description: <b>Length of encounter in days</b><br> 4257 * Type: <b>number</b><br> 4258 * Path: <b>Encounter.length</b><br> 4259 * </p> 4260 */ 4261 public static final ca.uhn.fhir.rest.gclient.NumberClientParam LENGTH = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_LENGTH); 4262 4263 /** 4264 * Search parameter: <b>diagnosis</b> 4265 * <p> 4266 * Description: <b>Reason the encounter takes place (resource)</b><br> 4267 * Type: <b>reference</b><br> 4268 * Path: <b>Encounter.diagnosis.condition</b><br> 4269 * </p> 4270 */ 4271 @SearchParamDefinition(name="diagnosis", path="Encounter.diagnosis.condition", description="Reason the encounter takes place (resource)", type="reference", target={Condition.class, Procedure.class } ) 4272 public static final String SP_DIAGNOSIS = "diagnosis"; 4273 /** 4274 * <b>Fluent Client</b> search parameter constant for <b>diagnosis</b> 4275 * <p> 4276 * Description: <b>Reason the encounter takes place (resource)</b><br> 4277 * Type: <b>reference</b><br> 4278 * Path: <b>Encounter.diagnosis.condition</b><br> 4279 * </p> 4280 */ 4281 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DIAGNOSIS = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DIAGNOSIS); 4282 4283/** 4284 * Constant for fluent queries to be used to add include statements. Specifies 4285 * the path value of "<b>Encounter:diagnosis</b>". 4286 */ 4287 public static final ca.uhn.fhir.model.api.Include INCLUDE_DIAGNOSIS = new ca.uhn.fhir.model.api.Include("Encounter:diagnosis").toLocked(); 4288 4289 /** 4290 * Search parameter: <b>appointment</b> 4291 * <p> 4292 * Description: <b>The appointment that scheduled this encounter</b><br> 4293 * Type: <b>reference</b><br> 4294 * Path: <b>Encounter.appointment</b><br> 4295 * </p> 4296 */ 4297 @SearchParamDefinition(name="appointment", path="Encounter.appointment", description="The appointment that scheduled this encounter", type="reference", target={Appointment.class } ) 4298 public static final String SP_APPOINTMENT = "appointment"; 4299 /** 4300 * <b>Fluent Client</b> search parameter constant for <b>appointment</b> 4301 * <p> 4302 * Description: <b>The appointment that scheduled this encounter</b><br> 4303 * Type: <b>reference</b><br> 4304 * Path: <b>Encounter.appointment</b><br> 4305 * </p> 4306 */ 4307 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam APPOINTMENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_APPOINTMENT); 4308 4309/** 4310 * Constant for fluent queries to be used to add include statements. Specifies 4311 * the path value of "<b>Encounter:appointment</b>". 4312 */ 4313 public static final ca.uhn.fhir.model.api.Include INCLUDE_APPOINTMENT = new ca.uhn.fhir.model.api.Include("Encounter:appointment").toLocked(); 4314 4315 /** 4316 * Search parameter: <b>part-of</b> 4317 * <p> 4318 * Description: <b>Another Encounter this encounter is part of</b><br> 4319 * Type: <b>reference</b><br> 4320 * Path: <b>Encounter.partOf</b><br> 4321 * </p> 4322 */ 4323 @SearchParamDefinition(name="part-of", path="Encounter.partOf", description="Another Encounter this encounter is part of", type="reference", target={Encounter.class } ) 4324 public static final String SP_PART_OF = "part-of"; 4325 /** 4326 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 4327 * <p> 4328 * Description: <b>Another Encounter this encounter is part of</b><br> 4329 * Type: <b>reference</b><br> 4330 * Path: <b>Encounter.partOf</b><br> 4331 * </p> 4332 */ 4333 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 4334 4335/** 4336 * Constant for fluent queries to be used to add include statements. Specifies 4337 * the path value of "<b>Encounter:part-of</b>". 4338 */ 4339 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("Encounter:part-of").toLocked(); 4340 4341 /** 4342 * Search parameter: <b>type</b> 4343 * <p> 4344 * Description: <b>Specific type of encounter</b><br> 4345 * Type: <b>token</b><br> 4346 * Path: <b>Encounter.type</b><br> 4347 * </p> 4348 */ 4349 @SearchParamDefinition(name="type", path="Encounter.type", description="Specific type of encounter", type="token" ) 4350 public static final String SP_TYPE = "type"; 4351 /** 4352 * <b>Fluent Client</b> search parameter constant for <b>type</b> 4353 * <p> 4354 * Description: <b>Specific type of encounter</b><br> 4355 * Type: <b>token</b><br> 4356 * Path: <b>Encounter.type</b><br> 4357 * </p> 4358 */ 4359 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 4360 4361 /** 4362 * Search parameter: <b>participant</b> 4363 * <p> 4364 * Description: <b>Persons involved in the encounter other than the patient</b><br> 4365 * Type: <b>reference</b><br> 4366 * Path: <b>Encounter.participant.individual</b><br> 4367 * </p> 4368 */ 4369 @SearchParamDefinition(name="participant", path="Encounter.participant.individual", description="Persons involved in the encounter other than the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Practitioner.class, RelatedPerson.class } ) 4370 public static final String SP_PARTICIPANT = "participant"; 4371 /** 4372 * <b>Fluent Client</b> search parameter constant for <b>participant</b> 4373 * <p> 4374 * Description: <b>Persons involved in the encounter other than the patient</b><br> 4375 * Type: <b>reference</b><br> 4376 * Path: <b>Encounter.participant.individual</b><br> 4377 * </p> 4378 */ 4379 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTICIPANT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTICIPANT); 4380 4381/** 4382 * Constant for fluent queries to be used to add include statements. Specifies 4383 * the path value of "<b>Encounter:participant</b>". 4384 */ 4385 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTICIPANT = new ca.uhn.fhir.model.api.Include("Encounter:participant").toLocked(); 4386 4387 /** 4388 * Search parameter: <b>patient</b> 4389 * <p> 4390 * Description: <b>The patient ro group present at the encounter</b><br> 4391 * Type: <b>reference</b><br> 4392 * Path: <b>Encounter.subject</b><br> 4393 * </p> 4394 */ 4395 @SearchParamDefinition(name="patient", path="Encounter.subject", description="The patient ro group present at the encounter", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 4396 public static final String SP_PATIENT = "patient"; 4397 /** 4398 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 4399 * <p> 4400 * Description: <b>The patient ro group present at the encounter</b><br> 4401 * Type: <b>reference</b><br> 4402 * Path: <b>Encounter.subject</b><br> 4403 * </p> 4404 */ 4405 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 4406 4407/** 4408 * Constant for fluent queries to be used to add include statements. Specifies 4409 * the path value of "<b>Encounter:patient</b>". 4410 */ 4411 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Encounter:patient").toLocked(); 4412 4413 /** 4414 * Search parameter: <b>location-period</b> 4415 * <p> 4416 * Description: <b>Time period during which the patient was present at the location</b><br> 4417 * Type: <b>date</b><br> 4418 * Path: <b>Encounter.location.period</b><br> 4419 * </p> 4420 */ 4421 @SearchParamDefinition(name="location-period", path="Encounter.location.period", description="Time period during which the patient was present at the location", type="date" ) 4422 public static final String SP_LOCATION_PERIOD = "location-period"; 4423 /** 4424 * <b>Fluent Client</b> search parameter constant for <b>location-period</b> 4425 * <p> 4426 * Description: <b>Time period during which the patient was present at the location</b><br> 4427 * Type: <b>date</b><br> 4428 * Path: <b>Encounter.location.period</b><br> 4429 * </p> 4430 */ 4431 public static final ca.uhn.fhir.rest.gclient.DateClientParam LOCATION_PERIOD = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_LOCATION_PERIOD); 4432 4433 /** 4434 * Search parameter: <b>location</b> 4435 * <p> 4436 * Description: <b>Location the encounter takes place</b><br> 4437 * Type: <b>reference</b><br> 4438 * Path: <b>Encounter.location.location</b><br> 4439 * </p> 4440 */ 4441 @SearchParamDefinition(name="location", path="Encounter.location.location", description="Location the encounter takes place", type="reference", target={Location.class } ) 4442 public static final String SP_LOCATION = "location"; 4443 /** 4444 * <b>Fluent Client</b> search parameter constant for <b>location</b> 4445 * <p> 4446 * Description: <b>Location the encounter takes place</b><br> 4447 * Type: <b>reference</b><br> 4448 * Path: <b>Encounter.location.location</b><br> 4449 * </p> 4450 */ 4451 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 4452 4453/** 4454 * Constant for fluent queries to be used to add include statements. Specifies 4455 * the path value of "<b>Encounter:location</b>". 4456 */ 4457 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Encounter:location").toLocked(); 4458 4459 /** 4460 * Search parameter: <b>service-provider</b> 4461 * <p> 4462 * Description: <b>The custodian organization of this Encounter record</b><br> 4463 * Type: <b>reference</b><br> 4464 * Path: <b>Encounter.serviceProvider</b><br> 4465 * </p> 4466 */ 4467 @SearchParamDefinition(name="service-provider", path="Encounter.serviceProvider", description="The custodian organization of this Encounter record", type="reference", target={Organization.class } ) 4468 public static final String SP_SERVICE_PROVIDER = "service-provider"; 4469 /** 4470 * <b>Fluent Client</b> search parameter constant for <b>service-provider</b> 4471 * <p> 4472 * Description: <b>The custodian organization of this Encounter record</b><br> 4473 * Type: <b>reference</b><br> 4474 * Path: <b>Encounter.serviceProvider</b><br> 4475 * </p> 4476 */ 4477 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SERVICE_PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SERVICE_PROVIDER); 4478 4479/** 4480 * Constant for fluent queries to be used to add include statements. Specifies 4481 * the path value of "<b>Encounter:service-provider</b>". 4482 */ 4483 public static final ca.uhn.fhir.model.api.Include INCLUDE_SERVICE_PROVIDER = new ca.uhn.fhir.model.api.Include("Encounter:service-provider").toLocked(); 4484 4485 /** 4486 * Search parameter: <b>special-arrangement</b> 4487 * <p> 4488 * Description: <b>Wheelchair, translator, stretcher, etc.</b><br> 4489 * Type: <b>token</b><br> 4490 * Path: <b>Encounter.hospitalization.specialArrangement</b><br> 4491 * </p> 4492 */ 4493 @SearchParamDefinition(name="special-arrangement", path="Encounter.hospitalization.specialArrangement", description="Wheelchair, translator, stretcher, etc.", type="token" ) 4494 public static final String SP_SPECIAL_ARRANGEMENT = "special-arrangement"; 4495 /** 4496 * <b>Fluent Client</b> search parameter constant for <b>special-arrangement</b> 4497 * <p> 4498 * Description: <b>Wheelchair, translator, stretcher, etc.</b><br> 4499 * Type: <b>token</b><br> 4500 * Path: <b>Encounter.hospitalization.specialArrangement</b><br> 4501 * </p> 4502 */ 4503 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SPECIAL_ARRANGEMENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SPECIAL_ARRANGEMENT); 4504 4505 /** 4506 * Search parameter: <b>class</b> 4507 * <p> 4508 * Description: <b>inpatient | outpatient | ambulatory | emergency +</b><br> 4509 * Type: <b>token</b><br> 4510 * Path: <b>Encounter.class</b><br> 4511 * </p> 4512 */ 4513 @SearchParamDefinition(name="class", path="Encounter.class", description="inpatient | outpatient | ambulatory | emergency +", type="token" ) 4514 public static final String SP_CLASS = "class"; 4515 /** 4516 * <b>Fluent Client</b> search parameter constant for <b>class</b> 4517 * <p> 4518 * Description: <b>inpatient | outpatient | ambulatory | emergency +</b><br> 4519 * Type: <b>token</b><br> 4520 * Path: <b>Encounter.class</b><br> 4521 * </p> 4522 */ 4523 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CLASS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CLASS); 4524 4525 /** 4526 * Search parameter: <b>status</b> 4527 * <p> 4528 * Description: <b>planned | arrived | triaged | in-progress | onleave | finished | cancelled +</b><br> 4529 * Type: <b>token</b><br> 4530 * Path: <b>Encounter.status</b><br> 4531 * </p> 4532 */ 4533 @SearchParamDefinition(name="status", path="Encounter.status", description="planned | arrived | triaged | in-progress | onleave | finished | cancelled +", type="token" ) 4534 public static final String SP_STATUS = "status"; 4535 /** 4536 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4537 * <p> 4538 * Description: <b>planned | arrived | triaged | in-progress | onleave | finished | cancelled +</b><br> 4539 * Type: <b>token</b><br> 4540 * Path: <b>Encounter.status</b><br> 4541 * </p> 4542 */ 4543 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4544 4545 4546}