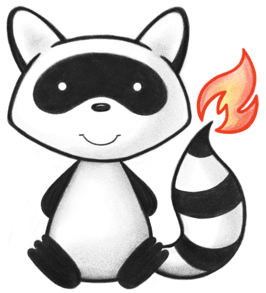
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045/** 046 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 047 */ 048@ResourceDef(name="Endpoint", profile="http://hl7.org/fhir/Profile/Endpoint") 049public class Endpoint extends DomainResource { 050 051 public enum EndpointStatus { 052 /** 053 * This endpoint is expected to be active and can be used 054 */ 055 ACTIVE, 056 /** 057 * This endpoint is temporarily unavailable 058 */ 059 SUSPENDED, 060 /** 061 * This endpoint has exceeded connectivity thresholds and is considered in an error state and should no longer be attempted to connect to until corrective action is taken 062 */ 063 ERROR, 064 /** 065 * This endpoint is no longer to be used 066 */ 067 OFF, 068 /** 069 * This instance should not have been part of this patient's medical record. 070 */ 071 ENTEREDINERROR, 072 /** 073 * This endpoint is not intended for production usage. 074 */ 075 TEST, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 public static EndpointStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("suspended".equals(codeString)) 086 return SUSPENDED; 087 if ("error".equals(codeString)) 088 return ERROR; 089 if ("off".equals(codeString)) 090 return OFF; 091 if ("entered-in-error".equals(codeString)) 092 return ENTEREDINERROR; 093 if ("test".equals(codeString)) 094 return TEST; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown EndpointStatus code '"+codeString+"'"); 099 } 100 public String toCode() { 101 switch (this) { 102 case ACTIVE: return "active"; 103 case SUSPENDED: return "suspended"; 104 case ERROR: return "error"; 105 case OFF: return "off"; 106 case ENTEREDINERROR: return "entered-in-error"; 107 case TEST: return "test"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getSystem() { 113 switch (this) { 114 case ACTIVE: return "http://hl7.org/fhir/endpoint-status"; 115 case SUSPENDED: return "http://hl7.org/fhir/endpoint-status"; 116 case ERROR: return "http://hl7.org/fhir/endpoint-status"; 117 case OFF: return "http://hl7.org/fhir/endpoint-status"; 118 case ENTEREDINERROR: return "http://hl7.org/fhir/endpoint-status"; 119 case TEST: return "http://hl7.org/fhir/endpoint-status"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getDefinition() { 125 switch (this) { 126 case ACTIVE: return "This endpoint is expected to be active and can be used"; 127 case SUSPENDED: return "This endpoint is temporarily unavailable"; 128 case ERROR: return "This endpoint has exceeded connectivity thresholds and is considered in an error state and should no longer be attempted to connect to until corrective action is taken"; 129 case OFF: return "This endpoint is no longer to be used"; 130 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 131 case TEST: return "This endpoint is not intended for production usage."; 132 case NULL: return null; 133 default: return "?"; 134 } 135 } 136 public String getDisplay() { 137 switch (this) { 138 case ACTIVE: return "Active"; 139 case SUSPENDED: return "Suspended"; 140 case ERROR: return "Error"; 141 case OFF: return "Off"; 142 case ENTEREDINERROR: return "Entered in error"; 143 case TEST: return "Test"; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 } 149 150 public static class EndpointStatusEnumFactory implements EnumFactory<EndpointStatus> { 151 public EndpointStatus fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("active".equals(codeString)) 156 return EndpointStatus.ACTIVE; 157 if ("suspended".equals(codeString)) 158 return EndpointStatus.SUSPENDED; 159 if ("error".equals(codeString)) 160 return EndpointStatus.ERROR; 161 if ("off".equals(codeString)) 162 return EndpointStatus.OFF; 163 if ("entered-in-error".equals(codeString)) 164 return EndpointStatus.ENTEREDINERROR; 165 if ("test".equals(codeString)) 166 return EndpointStatus.TEST; 167 throw new IllegalArgumentException("Unknown EndpointStatus code '"+codeString+"'"); 168 } 169 public Enumeration<EndpointStatus> fromType(PrimitiveType<?> code) throws FHIRException { 170 if (code == null) 171 return null; 172 if (code.isEmpty()) 173 return new Enumeration<EndpointStatus>(this); 174 String codeString = code.asStringValue(); 175 if (codeString == null || "".equals(codeString)) 176 return null; 177 if ("active".equals(codeString)) 178 return new Enumeration<EndpointStatus>(this, EndpointStatus.ACTIVE); 179 if ("suspended".equals(codeString)) 180 return new Enumeration<EndpointStatus>(this, EndpointStatus.SUSPENDED); 181 if ("error".equals(codeString)) 182 return new Enumeration<EndpointStatus>(this, EndpointStatus.ERROR); 183 if ("off".equals(codeString)) 184 return new Enumeration<EndpointStatus>(this, EndpointStatus.OFF); 185 if ("entered-in-error".equals(codeString)) 186 return new Enumeration<EndpointStatus>(this, EndpointStatus.ENTEREDINERROR); 187 if ("test".equals(codeString)) 188 return new Enumeration<EndpointStatus>(this, EndpointStatus.TEST); 189 throw new FHIRException("Unknown EndpointStatus code '"+codeString+"'"); 190 } 191 public String toCode(EndpointStatus code) { 192 if (code == EndpointStatus.ACTIVE) 193 return "active"; 194 if (code == EndpointStatus.SUSPENDED) 195 return "suspended"; 196 if (code == EndpointStatus.ERROR) 197 return "error"; 198 if (code == EndpointStatus.OFF) 199 return "off"; 200 if (code == EndpointStatus.ENTEREDINERROR) 201 return "entered-in-error"; 202 if (code == EndpointStatus.TEST) 203 return "test"; 204 return "?"; 205 } 206 public String toSystem(EndpointStatus code) { 207 return code.getSystem(); 208 } 209 } 210 211 /** 212 * Identifier for the organization that is used to identify the endpoint across multiple disparate systems. 213 */ 214 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 215 @Description(shortDefinition="Identifies this endpoint across multiple systems", formalDefinition="Identifier for the organization that is used to identify the endpoint across multiple disparate systems." ) 216 protected List<Identifier> identifier; 217 218 /** 219 * active | suspended | error | off | test. 220 */ 221 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 222 @Description(shortDefinition="active | suspended | error | off | entered-in-error | test", formalDefinition="active | suspended | error | off | test." ) 223 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-status") 224 protected Enumeration<EndpointStatus> status; 225 226 /** 227 * A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook). 228 */ 229 @Child(name = "connectionType", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=true) 230 @Description(shortDefinition="Protocol/Profile/Standard to be used with this endpoint connection", formalDefinition="A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook)." ) 231 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-connection-type") 232 protected Coding connectionType; 233 234 /** 235 * A friendly name that this endpoint can be referred to with. 236 */ 237 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 238 @Description(shortDefinition="A name that this endpoint can be identified by", formalDefinition="A friendly name that this endpoint can be referred to with." ) 239 protected StringType name; 240 241 /** 242 * The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data). 243 */ 244 @Child(name = "managingOrganization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 245 @Description(shortDefinition="Organization that manages this endpoint (may not be the organization that exposes the endpoint)", formalDefinition="The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data)." ) 246 protected Reference managingOrganization; 247 248 /** 249 * The actual object that is the target of the reference (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 250 */ 251 protected Organization managingOrganizationTarget; 252 253 /** 254 * Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting. 255 */ 256 @Child(name = "contact", type = {ContactPoint.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 257 @Description(shortDefinition="Contact details for source (e.g. troubleshooting)", formalDefinition="Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting." ) 258 protected List<ContactPoint> contact; 259 260 /** 261 * The interval during which the endpoint is expected to be operational. 262 */ 263 @Child(name = "period", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 264 @Description(shortDefinition="Interval the endpoint is expected to be operational", formalDefinition="The interval during which the endpoint is expected to be operational." ) 265 protected Period period; 266 267 /** 268 * The payload type describes the acceptable content that can be communicated on the endpoint. 269 */ 270 @Child(name = "payloadType", type = {CodeableConcept.class}, order=7, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 271 @Description(shortDefinition="The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)", formalDefinition="The payload type describes the acceptable content that can be communicated on the endpoint." ) 272 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-payload-type") 273 protected List<CodeableConcept> payloadType; 274 275 /** 276 * The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType). 277 */ 278 @Child(name = "payloadMimeType", type = {CodeType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 279 @Description(shortDefinition="Mimetype to send. If not specified, the content could be anything (including no payload, if the connectionType defined this)", formalDefinition="The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType)." ) 280 protected List<CodeType> payloadMimeType; 281 282 /** 283 * The uri that describes the actual end-point to connect to. 284 */ 285 @Child(name = "address", type = {UriType.class}, order=9, min=1, max=1, modifier=false, summary=true) 286 @Description(shortDefinition="The technical base address for connecting to this endpoint", formalDefinition="The uri that describes the actual end-point to connect to." ) 287 protected UriType address; 288 289 /** 290 * Additional headers / information to send as part of the notification. 291 */ 292 @Child(name = "header", type = {StringType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 293 @Description(shortDefinition="Usage depends on the channel type", formalDefinition="Additional headers / information to send as part of the notification." ) 294 protected List<StringType> header; 295 296 private static final long serialVersionUID = 694168955L; 297 298 /** 299 * Constructor 300 */ 301 public Endpoint() { 302 super(); 303 } 304 305 /** 306 * Constructor 307 */ 308 public Endpoint(Enumeration<EndpointStatus> status, Coding connectionType, UriType address) { 309 super(); 310 this.status = status; 311 this.connectionType = connectionType; 312 this.address = address; 313 } 314 315 /** 316 * @return {@link #identifier} (Identifier for the organization that is used to identify the endpoint across multiple disparate systems.) 317 */ 318 public List<Identifier> getIdentifier() { 319 if (this.identifier == null) 320 this.identifier = new ArrayList<Identifier>(); 321 return this.identifier; 322 } 323 324 /** 325 * @return Returns a reference to <code>this</code> for easy method chaining 326 */ 327 public Endpoint setIdentifier(List<Identifier> theIdentifier) { 328 this.identifier = theIdentifier; 329 return this; 330 } 331 332 public boolean hasIdentifier() { 333 if (this.identifier == null) 334 return false; 335 for (Identifier item : this.identifier) 336 if (!item.isEmpty()) 337 return true; 338 return false; 339 } 340 341 public Identifier addIdentifier() { //3 342 Identifier t = new Identifier(); 343 if (this.identifier == null) 344 this.identifier = new ArrayList<Identifier>(); 345 this.identifier.add(t); 346 return t; 347 } 348 349 public Endpoint addIdentifier(Identifier t) { //3 350 if (t == null) 351 return this; 352 if (this.identifier == null) 353 this.identifier = new ArrayList<Identifier>(); 354 this.identifier.add(t); 355 return this; 356 } 357 358 /** 359 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 360 */ 361 public Identifier getIdentifierFirstRep() { 362 if (getIdentifier().isEmpty()) { 363 addIdentifier(); 364 } 365 return getIdentifier().get(0); 366 } 367 368 /** 369 * @return {@link #status} (active | suspended | error | off | test.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 370 */ 371 public Enumeration<EndpointStatus> getStatusElement() { 372 if (this.status == null) 373 if (Configuration.errorOnAutoCreate()) 374 throw new Error("Attempt to auto-create Endpoint.status"); 375 else if (Configuration.doAutoCreate()) 376 this.status = new Enumeration<EndpointStatus>(new EndpointStatusEnumFactory()); // bb 377 return this.status; 378 } 379 380 public boolean hasStatusElement() { 381 return this.status != null && !this.status.isEmpty(); 382 } 383 384 public boolean hasStatus() { 385 return this.status != null && !this.status.isEmpty(); 386 } 387 388 /** 389 * @param value {@link #status} (active | suspended | error | off | test.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 390 */ 391 public Endpoint setStatusElement(Enumeration<EndpointStatus> value) { 392 this.status = value; 393 return this; 394 } 395 396 /** 397 * @return active | suspended | error | off | test. 398 */ 399 public EndpointStatus getStatus() { 400 return this.status == null ? null : this.status.getValue(); 401 } 402 403 /** 404 * @param value active | suspended | error | off | test. 405 */ 406 public Endpoint setStatus(EndpointStatus value) { 407 if (this.status == null) 408 this.status = new Enumeration<EndpointStatus>(new EndpointStatusEnumFactory()); 409 this.status.setValue(value); 410 return this; 411 } 412 413 /** 414 * @return {@link #connectionType} (A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).) 415 */ 416 public Coding getConnectionType() { 417 if (this.connectionType == null) 418 if (Configuration.errorOnAutoCreate()) 419 throw new Error("Attempt to auto-create Endpoint.connectionType"); 420 else if (Configuration.doAutoCreate()) 421 this.connectionType = new Coding(); // cc 422 return this.connectionType; 423 } 424 425 public boolean hasConnectionType() { 426 return this.connectionType != null && !this.connectionType.isEmpty(); 427 } 428 429 /** 430 * @param value {@link #connectionType} (A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).) 431 */ 432 public Endpoint setConnectionType(Coding value) { 433 this.connectionType = value; 434 return this; 435 } 436 437 /** 438 * @return {@link #name} (A friendly name that this endpoint can be referred to with.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 439 */ 440 public StringType getNameElement() { 441 if (this.name == null) 442 if (Configuration.errorOnAutoCreate()) 443 throw new Error("Attempt to auto-create Endpoint.name"); 444 else if (Configuration.doAutoCreate()) 445 this.name = new StringType(); // bb 446 return this.name; 447 } 448 449 public boolean hasNameElement() { 450 return this.name != null && !this.name.isEmpty(); 451 } 452 453 public boolean hasName() { 454 return this.name != null && !this.name.isEmpty(); 455 } 456 457 /** 458 * @param value {@link #name} (A friendly name that this endpoint can be referred to with.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 459 */ 460 public Endpoint setNameElement(StringType value) { 461 this.name = value; 462 return this; 463 } 464 465 /** 466 * @return A friendly name that this endpoint can be referred to with. 467 */ 468 public String getName() { 469 return this.name == null ? null : this.name.getValue(); 470 } 471 472 /** 473 * @param value A friendly name that this endpoint can be referred to with. 474 */ 475 public Endpoint setName(String value) { 476 if (Utilities.noString(value)) 477 this.name = null; 478 else { 479 if (this.name == null) 480 this.name = new StringType(); 481 this.name.setValue(value); 482 } 483 return this; 484 } 485 486 /** 487 * @return {@link #managingOrganization} (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 488 */ 489 public Reference getManagingOrganization() { 490 if (this.managingOrganization == null) 491 if (Configuration.errorOnAutoCreate()) 492 throw new Error("Attempt to auto-create Endpoint.managingOrganization"); 493 else if (Configuration.doAutoCreate()) 494 this.managingOrganization = new Reference(); // cc 495 return this.managingOrganization; 496 } 497 498 public boolean hasManagingOrganization() { 499 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 500 } 501 502 /** 503 * @param value {@link #managingOrganization} (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 504 */ 505 public Endpoint setManagingOrganization(Reference value) { 506 this.managingOrganization = value; 507 return this; 508 } 509 510 /** 511 * @return {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 512 */ 513 public Organization getManagingOrganizationTarget() { 514 if (this.managingOrganizationTarget == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create Endpoint.managingOrganization"); 517 else if (Configuration.doAutoCreate()) 518 this.managingOrganizationTarget = new Organization(); // aa 519 return this.managingOrganizationTarget; 520 } 521 522 /** 523 * @param value {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 524 */ 525 public Endpoint setManagingOrganizationTarget(Organization value) { 526 this.managingOrganizationTarget = value; 527 return this; 528 } 529 530 /** 531 * @return {@link #contact} (Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.) 532 */ 533 public List<ContactPoint> getContact() { 534 if (this.contact == null) 535 this.contact = new ArrayList<ContactPoint>(); 536 return this.contact; 537 } 538 539 /** 540 * @return Returns a reference to <code>this</code> for easy method chaining 541 */ 542 public Endpoint setContact(List<ContactPoint> theContact) { 543 this.contact = theContact; 544 return this; 545 } 546 547 public boolean hasContact() { 548 if (this.contact == null) 549 return false; 550 for (ContactPoint item : this.contact) 551 if (!item.isEmpty()) 552 return true; 553 return false; 554 } 555 556 public ContactPoint addContact() { //3 557 ContactPoint t = new ContactPoint(); 558 if (this.contact == null) 559 this.contact = new ArrayList<ContactPoint>(); 560 this.contact.add(t); 561 return t; 562 } 563 564 public Endpoint addContact(ContactPoint t) { //3 565 if (t == null) 566 return this; 567 if (this.contact == null) 568 this.contact = new ArrayList<ContactPoint>(); 569 this.contact.add(t); 570 return this; 571 } 572 573 /** 574 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 575 */ 576 public ContactPoint getContactFirstRep() { 577 if (getContact().isEmpty()) { 578 addContact(); 579 } 580 return getContact().get(0); 581 } 582 583 /** 584 * @return {@link #period} (The interval during which the endpoint is expected to be operational.) 585 */ 586 public Period getPeriod() { 587 if (this.period == null) 588 if (Configuration.errorOnAutoCreate()) 589 throw new Error("Attempt to auto-create Endpoint.period"); 590 else if (Configuration.doAutoCreate()) 591 this.period = new Period(); // cc 592 return this.period; 593 } 594 595 public boolean hasPeriod() { 596 return this.period != null && !this.period.isEmpty(); 597 } 598 599 /** 600 * @param value {@link #period} (The interval during which the endpoint is expected to be operational.) 601 */ 602 public Endpoint setPeriod(Period value) { 603 this.period = value; 604 return this; 605 } 606 607 /** 608 * @return {@link #payloadType} (The payload type describes the acceptable content that can be communicated on the endpoint.) 609 */ 610 public List<CodeableConcept> getPayloadType() { 611 if (this.payloadType == null) 612 this.payloadType = new ArrayList<CodeableConcept>(); 613 return this.payloadType; 614 } 615 616 /** 617 * @return Returns a reference to <code>this</code> for easy method chaining 618 */ 619 public Endpoint setPayloadType(List<CodeableConcept> thePayloadType) { 620 this.payloadType = thePayloadType; 621 return this; 622 } 623 624 public boolean hasPayloadType() { 625 if (this.payloadType == null) 626 return false; 627 for (CodeableConcept item : this.payloadType) 628 if (!item.isEmpty()) 629 return true; 630 return false; 631 } 632 633 public CodeableConcept addPayloadType() { //3 634 CodeableConcept t = new CodeableConcept(); 635 if (this.payloadType == null) 636 this.payloadType = new ArrayList<CodeableConcept>(); 637 this.payloadType.add(t); 638 return t; 639 } 640 641 public Endpoint addPayloadType(CodeableConcept t) { //3 642 if (t == null) 643 return this; 644 if (this.payloadType == null) 645 this.payloadType = new ArrayList<CodeableConcept>(); 646 this.payloadType.add(t); 647 return this; 648 } 649 650 /** 651 * @return The first repetition of repeating field {@link #payloadType}, creating it if it does not already exist 652 */ 653 public CodeableConcept getPayloadTypeFirstRep() { 654 if (getPayloadType().isEmpty()) { 655 addPayloadType(); 656 } 657 return getPayloadType().get(0); 658 } 659 660 /** 661 * @return {@link #payloadMimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 662 */ 663 public List<CodeType> getPayloadMimeType() { 664 if (this.payloadMimeType == null) 665 this.payloadMimeType = new ArrayList<CodeType>(); 666 return this.payloadMimeType; 667 } 668 669 /** 670 * @return Returns a reference to <code>this</code> for easy method chaining 671 */ 672 public Endpoint setPayloadMimeType(List<CodeType> thePayloadMimeType) { 673 this.payloadMimeType = thePayloadMimeType; 674 return this; 675 } 676 677 public boolean hasPayloadMimeType() { 678 if (this.payloadMimeType == null) 679 return false; 680 for (CodeType item : this.payloadMimeType) 681 if (!item.isEmpty()) 682 return true; 683 return false; 684 } 685 686 /** 687 * @return {@link #payloadMimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 688 */ 689 public CodeType addPayloadMimeTypeElement() {//2 690 CodeType t = new CodeType(); 691 if (this.payloadMimeType == null) 692 this.payloadMimeType = new ArrayList<CodeType>(); 693 this.payloadMimeType.add(t); 694 return t; 695 } 696 697 /** 698 * @param value {@link #payloadMimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 699 */ 700 public Endpoint addPayloadMimeType(String value) { //1 701 CodeType t = new CodeType(); 702 t.setValue(value); 703 if (this.payloadMimeType == null) 704 this.payloadMimeType = new ArrayList<CodeType>(); 705 this.payloadMimeType.add(t); 706 return this; 707 } 708 709 /** 710 * @param value {@link #payloadMimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 711 */ 712 public boolean hasPayloadMimeType(String value) { 713 if (this.payloadMimeType == null) 714 return false; 715 for (CodeType v : this.payloadMimeType) 716 if (v.getValue().equals(value)) // code 717 return true; 718 return false; 719 } 720 721 /** 722 * @return {@link #address} (The uri that describes the actual end-point to connect to.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 723 */ 724 public UriType getAddressElement() { 725 if (this.address == null) 726 if (Configuration.errorOnAutoCreate()) 727 throw new Error("Attempt to auto-create Endpoint.address"); 728 else if (Configuration.doAutoCreate()) 729 this.address = new UriType(); // bb 730 return this.address; 731 } 732 733 public boolean hasAddressElement() { 734 return this.address != null && !this.address.isEmpty(); 735 } 736 737 public boolean hasAddress() { 738 return this.address != null && !this.address.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #address} (The uri that describes the actual end-point to connect to.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 743 */ 744 public Endpoint setAddressElement(UriType value) { 745 this.address = value; 746 return this; 747 } 748 749 /** 750 * @return The uri that describes the actual end-point to connect to. 751 */ 752 public String getAddress() { 753 return this.address == null ? null : this.address.getValue(); 754 } 755 756 /** 757 * @param value The uri that describes the actual end-point to connect to. 758 */ 759 public Endpoint setAddress(String value) { 760 if (this.address == null) 761 this.address = new UriType(); 762 this.address.setValue(value); 763 return this; 764 } 765 766 /** 767 * @return {@link #header} (Additional headers / information to send as part of the notification.) 768 */ 769 public List<StringType> getHeader() { 770 if (this.header == null) 771 this.header = new ArrayList<StringType>(); 772 return this.header; 773 } 774 775 /** 776 * @return Returns a reference to <code>this</code> for easy method chaining 777 */ 778 public Endpoint setHeader(List<StringType> theHeader) { 779 this.header = theHeader; 780 return this; 781 } 782 783 public boolean hasHeader() { 784 if (this.header == null) 785 return false; 786 for (StringType item : this.header) 787 if (!item.isEmpty()) 788 return true; 789 return false; 790 } 791 792 /** 793 * @return {@link #header} (Additional headers / information to send as part of the notification.) 794 */ 795 public StringType addHeaderElement() {//2 796 StringType t = new StringType(); 797 if (this.header == null) 798 this.header = new ArrayList<StringType>(); 799 this.header.add(t); 800 return t; 801 } 802 803 /** 804 * @param value {@link #header} (Additional headers / information to send as part of the notification.) 805 */ 806 public Endpoint addHeader(String value) { //1 807 StringType t = new StringType(); 808 t.setValue(value); 809 if (this.header == null) 810 this.header = new ArrayList<StringType>(); 811 this.header.add(t); 812 return this; 813 } 814 815 /** 816 * @param value {@link #header} (Additional headers / information to send as part of the notification.) 817 */ 818 public boolean hasHeader(String value) { 819 if (this.header == null) 820 return false; 821 for (StringType v : this.header) 822 if (v.getValue().equals(value)) // string 823 return true; 824 return false; 825 } 826 827 protected void listChildren(List<Property> children) { 828 super.listChildren(children); 829 children.add(new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 830 children.add(new Property("status", "code", "active | suspended | error | off | test.", 0, 1, status)); 831 children.add(new Property("connectionType", "Coding", "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).", 0, 1, connectionType)); 832 children.add(new Property("name", "string", "A friendly name that this endpoint can be referred to with.", 0, 1, name)); 833 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).", 0, 1, managingOrganization)); 834 children.add(new Property("contact", "ContactPoint", "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact)); 835 children.add(new Property("period", "Period", "The interval during which the endpoint is expected to be operational.", 0, 1, period)); 836 children.add(new Property("payloadType", "CodeableConcept", "The payload type describes the acceptable content that can be communicated on the endpoint.", 0, java.lang.Integer.MAX_VALUE, payloadType)); 837 children.add(new Property("payloadMimeType", "code", "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).", 0, java.lang.Integer.MAX_VALUE, payloadMimeType)); 838 children.add(new Property("address", "uri", "The uri that describes the actual end-point to connect to.", 0, 1, address)); 839 children.add(new Property("header", "string", "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, header)); 840 } 841 842 @Override 843 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 844 switch (_hash) { 845 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 846 case -892481550: /*status*/ return new Property("status", "code", "active | suspended | error | off | test.", 0, 1, status); 847 case 1270211384: /*connectionType*/ return new Property("connectionType", "Coding", "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).", 0, 1, connectionType); 848 case 3373707: /*name*/ return new Property("name", "string", "A friendly name that this endpoint can be referred to with.", 0, 1, name); 849 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).", 0, 1, managingOrganization); 850 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact); 851 case -991726143: /*period*/ return new Property("period", "Period", "The interval during which the endpoint is expected to be operational.", 0, 1, period); 852 case 909929960: /*payloadType*/ return new Property("payloadType", "CodeableConcept", "The payload type describes the acceptable content that can be communicated on the endpoint.", 0, java.lang.Integer.MAX_VALUE, payloadType); 853 case -1702836932: /*payloadMimeType*/ return new Property("payloadMimeType", "code", "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).", 0, java.lang.Integer.MAX_VALUE, payloadMimeType); 854 case -1147692044: /*address*/ return new Property("address", "uri", "The uri that describes the actual end-point to connect to.", 0, 1, address); 855 case -1221270899: /*header*/ return new Property("header", "string", "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, header); 856 default: return super.getNamedProperty(_hash, _name, _checkValid); 857 } 858 859 } 860 861 @Override 862 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 863 switch (hash) { 864 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 865 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EndpointStatus> 866 case 1270211384: /*connectionType*/ return this.connectionType == null ? new Base[0] : new Base[] {this.connectionType}; // Coding 867 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 868 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 869 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 870 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 871 case 909929960: /*payloadType*/ return this.payloadType == null ? new Base[0] : this.payloadType.toArray(new Base[this.payloadType.size()]); // CodeableConcept 872 case -1702836932: /*payloadMimeType*/ return this.payloadMimeType == null ? new Base[0] : this.payloadMimeType.toArray(new Base[this.payloadMimeType.size()]); // CodeType 873 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // UriType 874 case -1221270899: /*header*/ return this.header == null ? new Base[0] : this.header.toArray(new Base[this.header.size()]); // StringType 875 default: return super.getProperty(hash, name, checkValid); 876 } 877 878 } 879 880 @Override 881 public Base setProperty(int hash, String name, Base value) throws FHIRException { 882 switch (hash) { 883 case -1618432855: // identifier 884 this.getIdentifier().add(castToIdentifier(value)); // Identifier 885 return value; 886 case -892481550: // status 887 value = new EndpointStatusEnumFactory().fromType(castToCode(value)); 888 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 889 return value; 890 case 1270211384: // connectionType 891 this.connectionType = castToCoding(value); // Coding 892 return value; 893 case 3373707: // name 894 this.name = castToString(value); // StringType 895 return value; 896 case -2058947787: // managingOrganization 897 this.managingOrganization = castToReference(value); // Reference 898 return value; 899 case 951526432: // contact 900 this.getContact().add(castToContactPoint(value)); // ContactPoint 901 return value; 902 case -991726143: // period 903 this.period = castToPeriod(value); // Period 904 return value; 905 case 909929960: // payloadType 906 this.getPayloadType().add(castToCodeableConcept(value)); // CodeableConcept 907 return value; 908 case -1702836932: // payloadMimeType 909 this.getPayloadMimeType().add(castToCode(value)); // CodeType 910 return value; 911 case -1147692044: // address 912 this.address = castToUri(value); // UriType 913 return value; 914 case -1221270899: // header 915 this.getHeader().add(castToString(value)); // StringType 916 return value; 917 default: return super.setProperty(hash, name, value); 918 } 919 920 } 921 922 @Override 923 public Base setProperty(String name, Base value) throws FHIRException { 924 if (name.equals("identifier")) { 925 this.getIdentifier().add(castToIdentifier(value)); 926 } else if (name.equals("status")) { 927 value = new EndpointStatusEnumFactory().fromType(castToCode(value)); 928 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 929 } else if (name.equals("connectionType")) { 930 this.connectionType = castToCoding(value); // Coding 931 } else if (name.equals("name")) { 932 this.name = castToString(value); // StringType 933 } else if (name.equals("managingOrganization")) { 934 this.managingOrganization = castToReference(value); // Reference 935 } else if (name.equals("contact")) { 936 this.getContact().add(castToContactPoint(value)); 937 } else if (name.equals("period")) { 938 this.period = castToPeriod(value); // Period 939 } else if (name.equals("payloadType")) { 940 this.getPayloadType().add(castToCodeableConcept(value)); 941 } else if (name.equals("payloadMimeType")) { 942 this.getPayloadMimeType().add(castToCode(value)); 943 } else if (name.equals("address")) { 944 this.address = castToUri(value); // UriType 945 } else if (name.equals("header")) { 946 this.getHeader().add(castToString(value)); 947 } else 948 return super.setProperty(name, value); 949 return value; 950 } 951 952 @Override 953 public Base makeProperty(int hash, String name) throws FHIRException { 954 switch (hash) { 955 case -1618432855: return addIdentifier(); 956 case -892481550: return getStatusElement(); 957 case 1270211384: return getConnectionType(); 958 case 3373707: return getNameElement(); 959 case -2058947787: return getManagingOrganization(); 960 case 951526432: return addContact(); 961 case -991726143: return getPeriod(); 962 case 909929960: return addPayloadType(); 963 case -1702836932: return addPayloadMimeTypeElement(); 964 case -1147692044: return getAddressElement(); 965 case -1221270899: return addHeaderElement(); 966 default: return super.makeProperty(hash, name); 967 } 968 969 } 970 971 @Override 972 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 973 switch (hash) { 974 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 975 case -892481550: /*status*/ return new String[] {"code"}; 976 case 1270211384: /*connectionType*/ return new String[] {"Coding"}; 977 case 3373707: /*name*/ return new String[] {"string"}; 978 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 979 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 980 case -991726143: /*period*/ return new String[] {"Period"}; 981 case 909929960: /*payloadType*/ return new String[] {"CodeableConcept"}; 982 case -1702836932: /*payloadMimeType*/ return new String[] {"code"}; 983 case -1147692044: /*address*/ return new String[] {"uri"}; 984 case -1221270899: /*header*/ return new String[] {"string"}; 985 default: return super.getTypesForProperty(hash, name); 986 } 987 988 } 989 990 @Override 991 public Base addChild(String name) throws FHIRException { 992 if (name.equals("identifier")) { 993 return addIdentifier(); 994 } 995 else if (name.equals("status")) { 996 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.status"); 997 } 998 else if (name.equals("connectionType")) { 999 this.connectionType = new Coding(); 1000 return this.connectionType; 1001 } 1002 else if (name.equals("name")) { 1003 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.name"); 1004 } 1005 else if (name.equals("managingOrganization")) { 1006 this.managingOrganization = new Reference(); 1007 return this.managingOrganization; 1008 } 1009 else if (name.equals("contact")) { 1010 return addContact(); 1011 } 1012 else if (name.equals("period")) { 1013 this.period = new Period(); 1014 return this.period; 1015 } 1016 else if (name.equals("payloadType")) { 1017 return addPayloadType(); 1018 } 1019 else if (name.equals("payloadMimeType")) { 1020 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.payloadMimeType"); 1021 } 1022 else if (name.equals("address")) { 1023 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.address"); 1024 } 1025 else if (name.equals("header")) { 1026 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.header"); 1027 } 1028 else 1029 return super.addChild(name); 1030 } 1031 1032 public String fhirType() { 1033 return "Endpoint"; 1034 1035 } 1036 1037 public Endpoint copy() { 1038 Endpoint dst = new Endpoint(); 1039 copyValues(dst); 1040 if (identifier != null) { 1041 dst.identifier = new ArrayList<Identifier>(); 1042 for (Identifier i : identifier) 1043 dst.identifier.add(i.copy()); 1044 }; 1045 dst.status = status == null ? null : status.copy(); 1046 dst.connectionType = connectionType == null ? null : connectionType.copy(); 1047 dst.name = name == null ? null : name.copy(); 1048 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1049 if (contact != null) { 1050 dst.contact = new ArrayList<ContactPoint>(); 1051 for (ContactPoint i : contact) 1052 dst.contact.add(i.copy()); 1053 }; 1054 dst.period = period == null ? null : period.copy(); 1055 if (payloadType != null) { 1056 dst.payloadType = new ArrayList<CodeableConcept>(); 1057 for (CodeableConcept i : payloadType) 1058 dst.payloadType.add(i.copy()); 1059 }; 1060 if (payloadMimeType != null) { 1061 dst.payloadMimeType = new ArrayList<CodeType>(); 1062 for (CodeType i : payloadMimeType) 1063 dst.payloadMimeType.add(i.copy()); 1064 }; 1065 dst.address = address == null ? null : address.copy(); 1066 if (header != null) { 1067 dst.header = new ArrayList<StringType>(); 1068 for (StringType i : header) 1069 dst.header.add(i.copy()); 1070 }; 1071 return dst; 1072 } 1073 1074 protected Endpoint typedCopy() { 1075 return copy(); 1076 } 1077 1078 @Override 1079 public boolean equalsDeep(Base other_) { 1080 if (!super.equalsDeep(other_)) 1081 return false; 1082 if (!(other_ instanceof Endpoint)) 1083 return false; 1084 Endpoint o = (Endpoint) other_; 1085 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(connectionType, o.connectionType, true) 1086 && compareDeep(name, o.name, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1087 && compareDeep(contact, o.contact, true) && compareDeep(period, o.period, true) && compareDeep(payloadType, o.payloadType, true) 1088 && compareDeep(payloadMimeType, o.payloadMimeType, true) && compareDeep(address, o.address, true) 1089 && compareDeep(header, o.header, true); 1090 } 1091 1092 @Override 1093 public boolean equalsShallow(Base other_) { 1094 if (!super.equalsShallow(other_)) 1095 return false; 1096 if (!(other_ instanceof Endpoint)) 1097 return false; 1098 Endpoint o = (Endpoint) other_; 1099 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(payloadMimeType, o.payloadMimeType, true) 1100 && compareValues(address, o.address, true) && compareValues(header, o.header, true); 1101 } 1102 1103 public boolean isEmpty() { 1104 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, connectionType 1105 , name, managingOrganization, contact, period, payloadType, payloadMimeType, address 1106 , header); 1107 } 1108 1109 @Override 1110 public ResourceType getResourceType() { 1111 return ResourceType.Endpoint; 1112 } 1113 1114 /** 1115 * Search parameter: <b>payload-type</b> 1116 * <p> 1117 * Description: <b>The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)</b><br> 1118 * Type: <b>token</b><br> 1119 * Path: <b>Endpoint.payloadType</b><br> 1120 * </p> 1121 */ 1122 @SearchParamDefinition(name="payload-type", path="Endpoint.payloadType", description="The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)", type="token" ) 1123 public static final String SP_PAYLOAD_TYPE = "payload-type"; 1124 /** 1125 * <b>Fluent Client</b> search parameter constant for <b>payload-type</b> 1126 * <p> 1127 * Description: <b>The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)</b><br> 1128 * Type: <b>token</b><br> 1129 * Path: <b>Endpoint.payloadType</b><br> 1130 * </p> 1131 */ 1132 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYLOAD_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PAYLOAD_TYPE); 1133 1134 /** 1135 * Search parameter: <b>identifier</b> 1136 * <p> 1137 * Description: <b>Identifies this endpoint across multiple systems</b><br> 1138 * Type: <b>token</b><br> 1139 * Path: <b>Endpoint.identifier</b><br> 1140 * </p> 1141 */ 1142 @SearchParamDefinition(name="identifier", path="Endpoint.identifier", description="Identifies this endpoint across multiple systems", type="token" ) 1143 public static final String SP_IDENTIFIER = "identifier"; 1144 /** 1145 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1146 * <p> 1147 * Description: <b>Identifies this endpoint across multiple systems</b><br> 1148 * Type: <b>token</b><br> 1149 * Path: <b>Endpoint.identifier</b><br> 1150 * </p> 1151 */ 1152 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1153 1154 /** 1155 * Search parameter: <b>organization</b> 1156 * <p> 1157 * Description: <b>The organization that is managing the endpoint</b><br> 1158 * Type: <b>reference</b><br> 1159 * Path: <b>Endpoint.managingOrganization</b><br> 1160 * </p> 1161 */ 1162 @SearchParamDefinition(name="organization", path="Endpoint.managingOrganization", description="The organization that is managing the endpoint", type="reference", target={Organization.class } ) 1163 public static final String SP_ORGANIZATION = "organization"; 1164 /** 1165 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1166 * <p> 1167 * Description: <b>The organization that is managing the endpoint</b><br> 1168 * Type: <b>reference</b><br> 1169 * Path: <b>Endpoint.managingOrganization</b><br> 1170 * </p> 1171 */ 1172 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1173 1174/** 1175 * Constant for fluent queries to be used to add include statements. Specifies 1176 * the path value of "<b>Endpoint:organization</b>". 1177 */ 1178 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Endpoint:organization").toLocked(); 1179 1180 /** 1181 * Search parameter: <b>connection-type</b> 1182 * <p> 1183 * Description: <b>Protocol/Profile/Standard to be used with this endpoint connection</b><br> 1184 * Type: <b>token</b><br> 1185 * Path: <b>Endpoint.connectionType</b><br> 1186 * </p> 1187 */ 1188 @SearchParamDefinition(name="connection-type", path="Endpoint.connectionType", description="Protocol/Profile/Standard to be used with this endpoint connection", type="token" ) 1189 public static final String SP_CONNECTION_TYPE = "connection-type"; 1190 /** 1191 * <b>Fluent Client</b> search parameter constant for <b>connection-type</b> 1192 * <p> 1193 * Description: <b>Protocol/Profile/Standard to be used with this endpoint connection</b><br> 1194 * Type: <b>token</b><br> 1195 * Path: <b>Endpoint.connectionType</b><br> 1196 * </p> 1197 */ 1198 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONNECTION_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONNECTION_TYPE); 1199 1200 /** 1201 * Search parameter: <b>name</b> 1202 * <p> 1203 * Description: <b>A name that this endpoint can be identified by</b><br> 1204 * Type: <b>string</b><br> 1205 * Path: <b>Endpoint.name</b><br> 1206 * </p> 1207 */ 1208 @SearchParamDefinition(name="name", path="Endpoint.name", description="A name that this endpoint can be identified by", type="string" ) 1209 public static final String SP_NAME = "name"; 1210 /** 1211 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1212 * <p> 1213 * Description: <b>A name that this endpoint can be identified by</b><br> 1214 * Type: <b>string</b><br> 1215 * Path: <b>Endpoint.name</b><br> 1216 * </p> 1217 */ 1218 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1219 1220 /** 1221 * Search parameter: <b>status</b> 1222 * <p> 1223 * Description: <b>The current status of the Endpoint (usually expected to be active)</b><br> 1224 * Type: <b>token</b><br> 1225 * Path: <b>Endpoint.status</b><br> 1226 * </p> 1227 */ 1228 @SearchParamDefinition(name="status", path="Endpoint.status", description="The current status of the Endpoint (usually expected to be active)", type="token" ) 1229 public static final String SP_STATUS = "status"; 1230 /** 1231 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1232 * <p> 1233 * Description: <b>The current status of the Endpoint (usually expected to be active)</b><br> 1234 * Type: <b>token</b><br> 1235 * Path: <b>Endpoint.status</b><br> 1236 * </p> 1237 */ 1238 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1239 1240 1241}