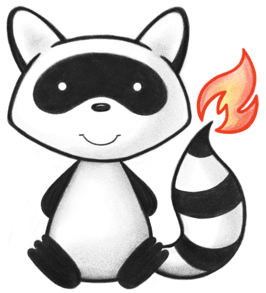
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045/** 046 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 047 */ 048@ResourceDef(name="Endpoint", profile="http://hl7.org/fhir/Profile/Endpoint") 049public class Endpoint extends DomainResource { 050 051 public enum EndpointStatus { 052 /** 053 * This endpoint is expected to be active and can be used 054 */ 055 ACTIVE, 056 /** 057 * This endpoint is temporarily unavailable 058 */ 059 SUSPENDED, 060 /** 061 * This endpoint has exceeded connectivity thresholds and is considered in an error state and should no longer be attempted to connect to until corrective action is taken 062 */ 063 ERROR, 064 /** 065 * This endpoint is no longer to be used 066 */ 067 OFF, 068 /** 069 * This instance should not have been part of this patient's medical record. 070 */ 071 ENTEREDINERROR, 072 /** 073 * This endpoint is not intended for production usage. 074 */ 075 TEST, 076 /** 077 * added to help the parsers with the generic types 078 */ 079 NULL; 080 public static EndpointStatus fromCode(String codeString) throws FHIRException { 081 if (codeString == null || "".equals(codeString)) 082 return null; 083 if ("active".equals(codeString)) 084 return ACTIVE; 085 if ("suspended".equals(codeString)) 086 return SUSPENDED; 087 if ("error".equals(codeString)) 088 return ERROR; 089 if ("off".equals(codeString)) 090 return OFF; 091 if ("entered-in-error".equals(codeString)) 092 return ENTEREDINERROR; 093 if ("test".equals(codeString)) 094 return TEST; 095 if (Configuration.isAcceptInvalidEnums()) 096 return null; 097 else 098 throw new FHIRException("Unknown EndpointStatus code '"+codeString+"'"); 099 } 100 public String toCode() { 101 switch (this) { 102 case ACTIVE: return "active"; 103 case SUSPENDED: return "suspended"; 104 case ERROR: return "error"; 105 case OFF: return "off"; 106 case ENTEREDINERROR: return "entered-in-error"; 107 case TEST: return "test"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getSystem() { 113 switch (this) { 114 case ACTIVE: return "http://hl7.org/fhir/endpoint-status"; 115 case SUSPENDED: return "http://hl7.org/fhir/endpoint-status"; 116 case ERROR: return "http://hl7.org/fhir/endpoint-status"; 117 case OFF: return "http://hl7.org/fhir/endpoint-status"; 118 case ENTEREDINERROR: return "http://hl7.org/fhir/endpoint-status"; 119 case TEST: return "http://hl7.org/fhir/endpoint-status"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getDefinition() { 125 switch (this) { 126 case ACTIVE: return "This endpoint is expected to be active and can be used"; 127 case SUSPENDED: return "This endpoint is temporarily unavailable"; 128 case ERROR: return "This endpoint has exceeded connectivity thresholds and is considered in an error state and should no longer be attempted to connect to until corrective action is taken"; 129 case OFF: return "This endpoint is no longer to be used"; 130 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 131 case TEST: return "This endpoint is not intended for production usage."; 132 case NULL: return null; 133 default: return "?"; 134 } 135 } 136 public String getDisplay() { 137 switch (this) { 138 case ACTIVE: return "Active"; 139 case SUSPENDED: return "Suspended"; 140 case ERROR: return "Error"; 141 case OFF: return "Off"; 142 case ENTEREDINERROR: return "Entered in error"; 143 case TEST: return "Test"; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 } 149 150 public static class EndpointStatusEnumFactory implements EnumFactory<EndpointStatus> { 151 public EndpointStatus fromCode(String codeString) throws IllegalArgumentException { 152 if (codeString == null || "".equals(codeString)) 153 if (codeString == null || "".equals(codeString)) 154 return null; 155 if ("active".equals(codeString)) 156 return EndpointStatus.ACTIVE; 157 if ("suspended".equals(codeString)) 158 return EndpointStatus.SUSPENDED; 159 if ("error".equals(codeString)) 160 return EndpointStatus.ERROR; 161 if ("off".equals(codeString)) 162 return EndpointStatus.OFF; 163 if ("entered-in-error".equals(codeString)) 164 return EndpointStatus.ENTEREDINERROR; 165 if ("test".equals(codeString)) 166 return EndpointStatus.TEST; 167 throw new IllegalArgumentException("Unknown EndpointStatus code '"+codeString+"'"); 168 } 169 public Enumeration<EndpointStatus> fromType(PrimitiveType<?> code) throws FHIRException { 170 if (code == null) 171 return null; 172 if (code.isEmpty()) 173 return new Enumeration<EndpointStatus>(this); 174 String codeString = code.asStringValue(); 175 if (codeString == null || "".equals(codeString)) 176 return null; 177 if ("active".equals(codeString)) 178 return new Enumeration<EndpointStatus>(this, EndpointStatus.ACTIVE); 179 if ("suspended".equals(codeString)) 180 return new Enumeration<EndpointStatus>(this, EndpointStatus.SUSPENDED); 181 if ("error".equals(codeString)) 182 return new Enumeration<EndpointStatus>(this, EndpointStatus.ERROR); 183 if ("off".equals(codeString)) 184 return new Enumeration<EndpointStatus>(this, EndpointStatus.OFF); 185 if ("entered-in-error".equals(codeString)) 186 return new Enumeration<EndpointStatus>(this, EndpointStatus.ENTEREDINERROR); 187 if ("test".equals(codeString)) 188 return new Enumeration<EndpointStatus>(this, EndpointStatus.TEST); 189 throw new FHIRException("Unknown EndpointStatus code '"+codeString+"'"); 190 } 191 public String toCode(EndpointStatus code) { 192 if (code == EndpointStatus.NULL) 193 return null; 194 if (code == EndpointStatus.ACTIVE) 195 return "active"; 196 if (code == EndpointStatus.SUSPENDED) 197 return "suspended"; 198 if (code == EndpointStatus.ERROR) 199 return "error"; 200 if (code == EndpointStatus.OFF) 201 return "off"; 202 if (code == EndpointStatus.ENTEREDINERROR) 203 return "entered-in-error"; 204 if (code == EndpointStatus.TEST) 205 return "test"; 206 return "?"; 207 } 208 public String toSystem(EndpointStatus code) { 209 return code.getSystem(); 210 } 211 } 212 213 /** 214 * Identifier for the organization that is used to identify the endpoint across multiple disparate systems. 215 */ 216 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 217 @Description(shortDefinition="Identifies this endpoint across multiple systems", formalDefinition="Identifier for the organization that is used to identify the endpoint across multiple disparate systems." ) 218 protected List<Identifier> identifier; 219 220 /** 221 * active | suspended | error | off | test. 222 */ 223 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 224 @Description(shortDefinition="active | suspended | error | off | entered-in-error | test", formalDefinition="active | suspended | error | off | test." ) 225 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-status") 226 protected Enumeration<EndpointStatus> status; 227 228 /** 229 * A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook). 230 */ 231 @Child(name = "connectionType", type = {Coding.class}, order=2, min=1, max=1, modifier=false, summary=true) 232 @Description(shortDefinition="Protocol/Profile/Standard to be used with this endpoint connection", formalDefinition="A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook)." ) 233 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-connection-type") 234 protected Coding connectionType; 235 236 /** 237 * A friendly name that this endpoint can be referred to with. 238 */ 239 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 240 @Description(shortDefinition="A name that this endpoint can be identified by", formalDefinition="A friendly name that this endpoint can be referred to with." ) 241 protected StringType name; 242 243 /** 244 * The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data). 245 */ 246 @Child(name = "managingOrganization", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 247 @Description(shortDefinition="Organization that manages this endpoint (may not be the organization that exposes the endpoint)", formalDefinition="The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data)." ) 248 protected Reference managingOrganization; 249 250 /** 251 * The actual object that is the target of the reference (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 252 */ 253 protected Organization managingOrganizationTarget; 254 255 /** 256 * Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting. 257 */ 258 @Child(name = "contact", type = {ContactPoint.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 259 @Description(shortDefinition="Contact details for source (e.g. troubleshooting)", formalDefinition="Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting." ) 260 protected List<ContactPoint> contact; 261 262 /** 263 * The interval during which the endpoint is expected to be operational. 264 */ 265 @Child(name = "period", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 266 @Description(shortDefinition="Interval the endpoint is expected to be operational", formalDefinition="The interval during which the endpoint is expected to be operational." ) 267 protected Period period; 268 269 /** 270 * The payload type describes the acceptable content that can be communicated on the endpoint. 271 */ 272 @Child(name = "payloadType", type = {CodeableConcept.class}, order=7, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 273 @Description(shortDefinition="The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)", formalDefinition="The payload type describes the acceptable content that can be communicated on the endpoint." ) 274 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/endpoint-payload-type") 275 protected List<CodeableConcept> payloadType; 276 277 /** 278 * The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType). 279 */ 280 @Child(name = "payloadMimeType", type = {CodeType.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 281 @Description(shortDefinition="Mimetype to send. If not specified, the content could be anything (including no payload, if the connectionType defined this)", formalDefinition="The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType)." ) 282 protected List<CodeType> payloadMimeType; 283 284 /** 285 * The uri that describes the actual end-point to connect to. 286 */ 287 @Child(name = "address", type = {UriType.class}, order=9, min=1, max=1, modifier=false, summary=true) 288 @Description(shortDefinition="The technical base address for connecting to this endpoint", formalDefinition="The uri that describes the actual end-point to connect to." ) 289 protected UriType address; 290 291 /** 292 * Additional headers / information to send as part of the notification. 293 */ 294 @Child(name = "header", type = {StringType.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 295 @Description(shortDefinition="Usage depends on the channel type", formalDefinition="Additional headers / information to send as part of the notification." ) 296 protected List<StringType> header; 297 298 private static final long serialVersionUID = 694168955L; 299 300 /** 301 * Constructor 302 */ 303 public Endpoint() { 304 super(); 305 } 306 307 /** 308 * Constructor 309 */ 310 public Endpoint(Enumeration<EndpointStatus> status, Coding connectionType, UriType address) { 311 super(); 312 this.status = status; 313 this.connectionType = connectionType; 314 this.address = address; 315 } 316 317 /** 318 * @return {@link #identifier} (Identifier for the organization that is used to identify the endpoint across multiple disparate systems.) 319 */ 320 public List<Identifier> getIdentifier() { 321 if (this.identifier == null) 322 this.identifier = new ArrayList<Identifier>(); 323 return this.identifier; 324 } 325 326 /** 327 * @return Returns a reference to <code>this</code> for easy method chaining 328 */ 329 public Endpoint setIdentifier(List<Identifier> theIdentifier) { 330 this.identifier = theIdentifier; 331 return this; 332 } 333 334 public boolean hasIdentifier() { 335 if (this.identifier == null) 336 return false; 337 for (Identifier item : this.identifier) 338 if (!item.isEmpty()) 339 return true; 340 return false; 341 } 342 343 public Identifier addIdentifier() { //3 344 Identifier t = new Identifier(); 345 if (this.identifier == null) 346 this.identifier = new ArrayList<Identifier>(); 347 this.identifier.add(t); 348 return t; 349 } 350 351 public Endpoint addIdentifier(Identifier t) { //3 352 if (t == null) 353 return this; 354 if (this.identifier == null) 355 this.identifier = new ArrayList<Identifier>(); 356 this.identifier.add(t); 357 return this; 358 } 359 360 /** 361 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 362 */ 363 public Identifier getIdentifierFirstRep() { 364 if (getIdentifier().isEmpty()) { 365 addIdentifier(); 366 } 367 return getIdentifier().get(0); 368 } 369 370 /** 371 * @return {@link #status} (active | suspended | error | off | test.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 372 */ 373 public Enumeration<EndpointStatus> getStatusElement() { 374 if (this.status == null) 375 if (Configuration.errorOnAutoCreate()) 376 throw new Error("Attempt to auto-create Endpoint.status"); 377 else if (Configuration.doAutoCreate()) 378 this.status = new Enumeration<EndpointStatus>(new EndpointStatusEnumFactory()); // bb 379 return this.status; 380 } 381 382 public boolean hasStatusElement() { 383 return this.status != null && !this.status.isEmpty(); 384 } 385 386 public boolean hasStatus() { 387 return this.status != null && !this.status.isEmpty(); 388 } 389 390 /** 391 * @param value {@link #status} (active | suspended | error | off | test.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 392 */ 393 public Endpoint setStatusElement(Enumeration<EndpointStatus> value) { 394 this.status = value; 395 return this; 396 } 397 398 /** 399 * @return active | suspended | error | off | test. 400 */ 401 public EndpointStatus getStatus() { 402 return this.status == null ? null : this.status.getValue(); 403 } 404 405 /** 406 * @param value active | suspended | error | off | test. 407 */ 408 public Endpoint setStatus(EndpointStatus value) { 409 if (this.status == null) 410 this.status = new Enumeration<EndpointStatus>(new EndpointStatusEnumFactory()); 411 this.status.setValue(value); 412 return this; 413 } 414 415 /** 416 * @return {@link #connectionType} (A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).) 417 */ 418 public Coding getConnectionType() { 419 if (this.connectionType == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create Endpoint.connectionType"); 422 else if (Configuration.doAutoCreate()) 423 this.connectionType = new Coding(); // cc 424 return this.connectionType; 425 } 426 427 public boolean hasConnectionType() { 428 return this.connectionType != null && !this.connectionType.isEmpty(); 429 } 430 431 /** 432 * @param value {@link #connectionType} (A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).) 433 */ 434 public Endpoint setConnectionType(Coding value) { 435 this.connectionType = value; 436 return this; 437 } 438 439 /** 440 * @return {@link #name} (A friendly name that this endpoint can be referred to with.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 441 */ 442 public StringType getNameElement() { 443 if (this.name == null) 444 if (Configuration.errorOnAutoCreate()) 445 throw new Error("Attempt to auto-create Endpoint.name"); 446 else if (Configuration.doAutoCreate()) 447 this.name = new StringType(); // bb 448 return this.name; 449 } 450 451 public boolean hasNameElement() { 452 return this.name != null && !this.name.isEmpty(); 453 } 454 455 public boolean hasName() { 456 return this.name != null && !this.name.isEmpty(); 457 } 458 459 /** 460 * @param value {@link #name} (A friendly name that this endpoint can be referred to with.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 461 */ 462 public Endpoint setNameElement(StringType value) { 463 this.name = value; 464 return this; 465 } 466 467 /** 468 * @return A friendly name that this endpoint can be referred to with. 469 */ 470 public String getName() { 471 return this.name == null ? null : this.name.getValue(); 472 } 473 474 /** 475 * @param value A friendly name that this endpoint can be referred to with. 476 */ 477 public Endpoint setName(String value) { 478 if (Utilities.noString(value)) 479 this.name = null; 480 else { 481 if (this.name == null) 482 this.name = new StringType(); 483 this.name.setValue(value); 484 } 485 return this; 486 } 487 488 /** 489 * @return {@link #managingOrganization} (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 490 */ 491 public Reference getManagingOrganization() { 492 if (this.managingOrganization == null) 493 if (Configuration.errorOnAutoCreate()) 494 throw new Error("Attempt to auto-create Endpoint.managingOrganization"); 495 else if (Configuration.doAutoCreate()) 496 this.managingOrganization = new Reference(); // cc 497 return this.managingOrganization; 498 } 499 500 public boolean hasManagingOrganization() { 501 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #managingOrganization} (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 506 */ 507 public Endpoint setManagingOrganization(Reference value) { 508 this.managingOrganization = value; 509 return this; 510 } 511 512 /** 513 * @return {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 514 */ 515 public Organization getManagingOrganizationTarget() { 516 if (this.managingOrganizationTarget == null) 517 if (Configuration.errorOnAutoCreate()) 518 throw new Error("Attempt to auto-create Endpoint.managingOrganization"); 519 else if (Configuration.doAutoCreate()) 520 this.managingOrganizationTarget = new Organization(); // aa 521 return this.managingOrganizationTarget; 522 } 523 524 /** 525 * @param value {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).) 526 */ 527 public Endpoint setManagingOrganizationTarget(Organization value) { 528 this.managingOrganizationTarget = value; 529 return this; 530 } 531 532 /** 533 * @return {@link #contact} (Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.) 534 */ 535 public List<ContactPoint> getContact() { 536 if (this.contact == null) 537 this.contact = new ArrayList<ContactPoint>(); 538 return this.contact; 539 } 540 541 /** 542 * @return Returns a reference to <code>this</code> for easy method chaining 543 */ 544 public Endpoint setContact(List<ContactPoint> theContact) { 545 this.contact = theContact; 546 return this; 547 } 548 549 public boolean hasContact() { 550 if (this.contact == null) 551 return false; 552 for (ContactPoint item : this.contact) 553 if (!item.isEmpty()) 554 return true; 555 return false; 556 } 557 558 public ContactPoint addContact() { //3 559 ContactPoint t = new ContactPoint(); 560 if (this.contact == null) 561 this.contact = new ArrayList<ContactPoint>(); 562 this.contact.add(t); 563 return t; 564 } 565 566 public Endpoint addContact(ContactPoint t) { //3 567 if (t == null) 568 return this; 569 if (this.contact == null) 570 this.contact = new ArrayList<ContactPoint>(); 571 this.contact.add(t); 572 return this; 573 } 574 575 /** 576 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 577 */ 578 public ContactPoint getContactFirstRep() { 579 if (getContact().isEmpty()) { 580 addContact(); 581 } 582 return getContact().get(0); 583 } 584 585 /** 586 * @return {@link #period} (The interval during which the endpoint is expected to be operational.) 587 */ 588 public Period getPeriod() { 589 if (this.period == null) 590 if (Configuration.errorOnAutoCreate()) 591 throw new Error("Attempt to auto-create Endpoint.period"); 592 else if (Configuration.doAutoCreate()) 593 this.period = new Period(); // cc 594 return this.period; 595 } 596 597 public boolean hasPeriod() { 598 return this.period != null && !this.period.isEmpty(); 599 } 600 601 /** 602 * @param value {@link #period} (The interval during which the endpoint is expected to be operational.) 603 */ 604 public Endpoint setPeriod(Period value) { 605 this.period = value; 606 return this; 607 } 608 609 /** 610 * @return {@link #payloadType} (The payload type describes the acceptable content that can be communicated on the endpoint.) 611 */ 612 public List<CodeableConcept> getPayloadType() { 613 if (this.payloadType == null) 614 this.payloadType = new ArrayList<CodeableConcept>(); 615 return this.payloadType; 616 } 617 618 /** 619 * @return Returns a reference to <code>this</code> for easy method chaining 620 */ 621 public Endpoint setPayloadType(List<CodeableConcept> thePayloadType) { 622 this.payloadType = thePayloadType; 623 return this; 624 } 625 626 public boolean hasPayloadType() { 627 if (this.payloadType == null) 628 return false; 629 for (CodeableConcept item : this.payloadType) 630 if (!item.isEmpty()) 631 return true; 632 return false; 633 } 634 635 public CodeableConcept addPayloadType() { //3 636 CodeableConcept t = new CodeableConcept(); 637 if (this.payloadType == null) 638 this.payloadType = new ArrayList<CodeableConcept>(); 639 this.payloadType.add(t); 640 return t; 641 } 642 643 public Endpoint addPayloadType(CodeableConcept t) { //3 644 if (t == null) 645 return this; 646 if (this.payloadType == null) 647 this.payloadType = new ArrayList<CodeableConcept>(); 648 this.payloadType.add(t); 649 return this; 650 } 651 652 /** 653 * @return The first repetition of repeating field {@link #payloadType}, creating it if it does not already exist 654 */ 655 public CodeableConcept getPayloadTypeFirstRep() { 656 if (getPayloadType().isEmpty()) { 657 addPayloadType(); 658 } 659 return getPayloadType().get(0); 660 } 661 662 /** 663 * @return {@link #payloadMimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 664 */ 665 public List<CodeType> getPayloadMimeType() { 666 if (this.payloadMimeType == null) 667 this.payloadMimeType = new ArrayList<CodeType>(); 668 return this.payloadMimeType; 669 } 670 671 /** 672 * @return Returns a reference to <code>this</code> for easy method chaining 673 */ 674 public Endpoint setPayloadMimeType(List<CodeType> thePayloadMimeType) { 675 this.payloadMimeType = thePayloadMimeType; 676 return this; 677 } 678 679 public boolean hasPayloadMimeType() { 680 if (this.payloadMimeType == null) 681 return false; 682 for (CodeType item : this.payloadMimeType) 683 if (!item.isEmpty()) 684 return true; 685 return false; 686 } 687 688 /** 689 * @return {@link #payloadMimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 690 */ 691 public CodeType addPayloadMimeTypeElement() {//2 692 CodeType t = new CodeType(); 693 if (this.payloadMimeType == null) 694 this.payloadMimeType = new ArrayList<CodeType>(); 695 this.payloadMimeType.add(t); 696 return t; 697 } 698 699 /** 700 * @param value {@link #payloadMimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 701 */ 702 public Endpoint addPayloadMimeType(String value) { //1 703 CodeType t = new CodeType(); 704 t.setValue(value); 705 if (this.payloadMimeType == null) 706 this.payloadMimeType = new ArrayList<CodeType>(); 707 this.payloadMimeType.add(t); 708 return this; 709 } 710 711 /** 712 * @param value {@link #payloadMimeType} (The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).) 713 */ 714 public boolean hasPayloadMimeType(String value) { 715 if (this.payloadMimeType == null) 716 return false; 717 for (CodeType v : this.payloadMimeType) 718 if (v.getValue().equals(value)) // code 719 return true; 720 return false; 721 } 722 723 /** 724 * @return {@link #address} (The uri that describes the actual end-point to connect to.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 725 */ 726 public UriType getAddressElement() { 727 if (this.address == null) 728 if (Configuration.errorOnAutoCreate()) 729 throw new Error("Attempt to auto-create Endpoint.address"); 730 else if (Configuration.doAutoCreate()) 731 this.address = new UriType(); // bb 732 return this.address; 733 } 734 735 public boolean hasAddressElement() { 736 return this.address != null && !this.address.isEmpty(); 737 } 738 739 public boolean hasAddress() { 740 return this.address != null && !this.address.isEmpty(); 741 } 742 743 /** 744 * @param value {@link #address} (The uri that describes the actual end-point to connect to.). This is the underlying object with id, value and extensions. The accessor "getAddress" gives direct access to the value 745 */ 746 public Endpoint setAddressElement(UriType value) { 747 this.address = value; 748 return this; 749 } 750 751 /** 752 * @return The uri that describes the actual end-point to connect to. 753 */ 754 public String getAddress() { 755 return this.address == null ? null : this.address.getValue(); 756 } 757 758 /** 759 * @param value The uri that describes the actual end-point to connect to. 760 */ 761 public Endpoint setAddress(String value) { 762 if (this.address == null) 763 this.address = new UriType(); 764 this.address.setValue(value); 765 return this; 766 } 767 768 /** 769 * @return {@link #header} (Additional headers / information to send as part of the notification.) 770 */ 771 public List<StringType> getHeader() { 772 if (this.header == null) 773 this.header = new ArrayList<StringType>(); 774 return this.header; 775 } 776 777 /** 778 * @return Returns a reference to <code>this</code> for easy method chaining 779 */ 780 public Endpoint setHeader(List<StringType> theHeader) { 781 this.header = theHeader; 782 return this; 783 } 784 785 public boolean hasHeader() { 786 if (this.header == null) 787 return false; 788 for (StringType item : this.header) 789 if (!item.isEmpty()) 790 return true; 791 return false; 792 } 793 794 /** 795 * @return {@link #header} (Additional headers / information to send as part of the notification.) 796 */ 797 public StringType addHeaderElement() {//2 798 StringType t = new StringType(); 799 if (this.header == null) 800 this.header = new ArrayList<StringType>(); 801 this.header.add(t); 802 return t; 803 } 804 805 /** 806 * @param value {@link #header} (Additional headers / information to send as part of the notification.) 807 */ 808 public Endpoint addHeader(String value) { //1 809 StringType t = new StringType(); 810 t.setValue(value); 811 if (this.header == null) 812 this.header = new ArrayList<StringType>(); 813 this.header.add(t); 814 return this; 815 } 816 817 /** 818 * @param value {@link #header} (Additional headers / information to send as part of the notification.) 819 */ 820 public boolean hasHeader(String value) { 821 if (this.header == null) 822 return false; 823 for (StringType v : this.header) 824 if (v.getValue().equals(value)) // string 825 return true; 826 return false; 827 } 828 829 protected void listChildren(List<Property> children) { 830 super.listChildren(children); 831 children.add(new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier)); 832 children.add(new Property("status", "code", "active | suspended | error | off | test.", 0, 1, status)); 833 children.add(new Property("connectionType", "Coding", "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).", 0, 1, connectionType)); 834 children.add(new Property("name", "string", "A friendly name that this endpoint can be referred to with.", 0, 1, name)); 835 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).", 0, 1, managingOrganization)); 836 children.add(new Property("contact", "ContactPoint", "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact)); 837 children.add(new Property("period", "Period", "The interval during which the endpoint is expected to be operational.", 0, 1, period)); 838 children.add(new Property("payloadType", "CodeableConcept", "The payload type describes the acceptable content that can be communicated on the endpoint.", 0, java.lang.Integer.MAX_VALUE, payloadType)); 839 children.add(new Property("payloadMimeType", "code", "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).", 0, java.lang.Integer.MAX_VALUE, payloadMimeType)); 840 children.add(new Property("address", "uri", "The uri that describes the actual end-point to connect to.", 0, 1, address)); 841 children.add(new Property("header", "string", "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, header)); 842 } 843 844 @Override 845 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 846 switch (_hash) { 847 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the organization that is used to identify the endpoint across multiple disparate systems.", 0, java.lang.Integer.MAX_VALUE, identifier); 848 case -892481550: /*status*/ return new Property("status", "code", "active | suspended | error | off | test.", 0, 1, status); 849 case 1270211384: /*connectionType*/ return new Property("connectionType", "Coding", "A coded value that represents the technical details of the usage of this endpoint, such as what WSDLs should be used in what way. (e.g. XDS.b/DICOM/cds-hook).", 0, 1, connectionType); 850 case 3373707: /*name*/ return new Property("name", "string", "A friendly name that this endpoint can be referred to with.", 0, 1, name); 851 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization that manages this endpoint (even if technically another organisation is hosting this in the cloud, it is the organisation associated with the data).", 0, 1, managingOrganization); 852 case 951526432: /*contact*/ return new Property("contact", "ContactPoint", "Contact details for a human to contact about the subscription. The primary use of this for system administrator troubleshooting.", 0, java.lang.Integer.MAX_VALUE, contact); 853 case -991726143: /*period*/ return new Property("period", "Period", "The interval during which the endpoint is expected to be operational.", 0, 1, period); 854 case 909929960: /*payloadType*/ return new Property("payloadType", "CodeableConcept", "The payload type describes the acceptable content that can be communicated on the endpoint.", 0, java.lang.Integer.MAX_VALUE, payloadType); 855 case -1702836932: /*payloadMimeType*/ return new Property("payloadMimeType", "code", "The mime type to send the payload in - e.g. application/fhir+xml, application/fhir+json. If the mime type is not specified, then the sender could send any content (including no content depending on the connectionType).", 0, java.lang.Integer.MAX_VALUE, payloadMimeType); 856 case -1147692044: /*address*/ return new Property("address", "uri", "The uri that describes the actual end-point to connect to.", 0, 1, address); 857 case -1221270899: /*header*/ return new Property("header", "string", "Additional headers / information to send as part of the notification.", 0, java.lang.Integer.MAX_VALUE, header); 858 default: return super.getNamedProperty(_hash, _name, _checkValid); 859 } 860 861 } 862 863 @Override 864 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 865 switch (hash) { 866 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 867 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EndpointStatus> 868 case 1270211384: /*connectionType*/ return this.connectionType == null ? new Base[0] : new Base[] {this.connectionType}; // Coding 869 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 870 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 871 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactPoint 872 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 873 case 909929960: /*payloadType*/ return this.payloadType == null ? new Base[0] : this.payloadType.toArray(new Base[this.payloadType.size()]); // CodeableConcept 874 case -1702836932: /*payloadMimeType*/ return this.payloadMimeType == null ? new Base[0] : this.payloadMimeType.toArray(new Base[this.payloadMimeType.size()]); // CodeType 875 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // UriType 876 case -1221270899: /*header*/ return this.header == null ? new Base[0] : this.header.toArray(new Base[this.header.size()]); // StringType 877 default: return super.getProperty(hash, name, checkValid); 878 } 879 880 } 881 882 @Override 883 public Base setProperty(int hash, String name, Base value) throws FHIRException { 884 switch (hash) { 885 case -1618432855: // identifier 886 this.getIdentifier().add(castToIdentifier(value)); // Identifier 887 return value; 888 case -892481550: // status 889 value = new EndpointStatusEnumFactory().fromType(castToCode(value)); 890 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 891 return value; 892 case 1270211384: // connectionType 893 this.connectionType = castToCoding(value); // Coding 894 return value; 895 case 3373707: // name 896 this.name = castToString(value); // StringType 897 return value; 898 case -2058947787: // managingOrganization 899 this.managingOrganization = castToReference(value); // Reference 900 return value; 901 case 951526432: // contact 902 this.getContact().add(castToContactPoint(value)); // ContactPoint 903 return value; 904 case -991726143: // period 905 this.period = castToPeriod(value); // Period 906 return value; 907 case 909929960: // payloadType 908 this.getPayloadType().add(castToCodeableConcept(value)); // CodeableConcept 909 return value; 910 case -1702836932: // payloadMimeType 911 this.getPayloadMimeType().add(castToCode(value)); // CodeType 912 return value; 913 case -1147692044: // address 914 this.address = castToUri(value); // UriType 915 return value; 916 case -1221270899: // header 917 this.getHeader().add(castToString(value)); // StringType 918 return value; 919 default: return super.setProperty(hash, name, value); 920 } 921 922 } 923 924 @Override 925 public Base setProperty(String name, Base value) throws FHIRException { 926 if (name.equals("identifier")) { 927 this.getIdentifier().add(castToIdentifier(value)); 928 } else if (name.equals("status")) { 929 value = new EndpointStatusEnumFactory().fromType(castToCode(value)); 930 this.status = (Enumeration) value; // Enumeration<EndpointStatus> 931 } else if (name.equals("connectionType")) { 932 this.connectionType = castToCoding(value); // Coding 933 } else if (name.equals("name")) { 934 this.name = castToString(value); // StringType 935 } else if (name.equals("managingOrganization")) { 936 this.managingOrganization = castToReference(value); // Reference 937 } else if (name.equals("contact")) { 938 this.getContact().add(castToContactPoint(value)); 939 } else if (name.equals("period")) { 940 this.period = castToPeriod(value); // Period 941 } else if (name.equals("payloadType")) { 942 this.getPayloadType().add(castToCodeableConcept(value)); 943 } else if (name.equals("payloadMimeType")) { 944 this.getPayloadMimeType().add(castToCode(value)); 945 } else if (name.equals("address")) { 946 this.address = castToUri(value); // UriType 947 } else if (name.equals("header")) { 948 this.getHeader().add(castToString(value)); 949 } else 950 return super.setProperty(name, value); 951 return value; 952 } 953 954 @Override 955 public Base makeProperty(int hash, String name) throws FHIRException { 956 switch (hash) { 957 case -1618432855: return addIdentifier(); 958 case -892481550: return getStatusElement(); 959 case 1270211384: return getConnectionType(); 960 case 3373707: return getNameElement(); 961 case -2058947787: return getManagingOrganization(); 962 case 951526432: return addContact(); 963 case -991726143: return getPeriod(); 964 case 909929960: return addPayloadType(); 965 case -1702836932: return addPayloadMimeTypeElement(); 966 case -1147692044: return getAddressElement(); 967 case -1221270899: return addHeaderElement(); 968 default: return super.makeProperty(hash, name); 969 } 970 971 } 972 973 @Override 974 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 975 switch (hash) { 976 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 977 case -892481550: /*status*/ return new String[] {"code"}; 978 case 1270211384: /*connectionType*/ return new String[] {"Coding"}; 979 case 3373707: /*name*/ return new String[] {"string"}; 980 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 981 case 951526432: /*contact*/ return new String[] {"ContactPoint"}; 982 case -991726143: /*period*/ return new String[] {"Period"}; 983 case 909929960: /*payloadType*/ return new String[] {"CodeableConcept"}; 984 case -1702836932: /*payloadMimeType*/ return new String[] {"code"}; 985 case -1147692044: /*address*/ return new String[] {"uri"}; 986 case -1221270899: /*header*/ return new String[] {"string"}; 987 default: return super.getTypesForProperty(hash, name); 988 } 989 990 } 991 992 @Override 993 public Base addChild(String name) throws FHIRException { 994 if (name.equals("identifier")) { 995 return addIdentifier(); 996 } 997 else if (name.equals("status")) { 998 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.status"); 999 } 1000 else if (name.equals("connectionType")) { 1001 this.connectionType = new Coding(); 1002 return this.connectionType; 1003 } 1004 else if (name.equals("name")) { 1005 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.name"); 1006 } 1007 else if (name.equals("managingOrganization")) { 1008 this.managingOrganization = new Reference(); 1009 return this.managingOrganization; 1010 } 1011 else if (name.equals("contact")) { 1012 return addContact(); 1013 } 1014 else if (name.equals("period")) { 1015 this.period = new Period(); 1016 return this.period; 1017 } 1018 else if (name.equals("payloadType")) { 1019 return addPayloadType(); 1020 } 1021 else if (name.equals("payloadMimeType")) { 1022 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.payloadMimeType"); 1023 } 1024 else if (name.equals("address")) { 1025 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.address"); 1026 } 1027 else if (name.equals("header")) { 1028 throw new FHIRException("Cannot call addChild on a singleton property Endpoint.header"); 1029 } 1030 else 1031 return super.addChild(name); 1032 } 1033 1034 public String fhirType() { 1035 return "Endpoint"; 1036 1037 } 1038 1039 public Endpoint copy() { 1040 Endpoint dst = new Endpoint(); 1041 copyValues(dst); 1042 if (identifier != null) { 1043 dst.identifier = new ArrayList<Identifier>(); 1044 for (Identifier i : identifier) 1045 dst.identifier.add(i.copy()); 1046 }; 1047 dst.status = status == null ? null : status.copy(); 1048 dst.connectionType = connectionType == null ? null : connectionType.copy(); 1049 dst.name = name == null ? null : name.copy(); 1050 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1051 if (contact != null) { 1052 dst.contact = new ArrayList<ContactPoint>(); 1053 for (ContactPoint i : contact) 1054 dst.contact.add(i.copy()); 1055 }; 1056 dst.period = period == null ? null : period.copy(); 1057 if (payloadType != null) { 1058 dst.payloadType = new ArrayList<CodeableConcept>(); 1059 for (CodeableConcept i : payloadType) 1060 dst.payloadType.add(i.copy()); 1061 }; 1062 if (payloadMimeType != null) { 1063 dst.payloadMimeType = new ArrayList<CodeType>(); 1064 for (CodeType i : payloadMimeType) 1065 dst.payloadMimeType.add(i.copy()); 1066 }; 1067 dst.address = address == null ? null : address.copy(); 1068 if (header != null) { 1069 dst.header = new ArrayList<StringType>(); 1070 for (StringType i : header) 1071 dst.header.add(i.copy()); 1072 }; 1073 return dst; 1074 } 1075 1076 protected Endpoint typedCopy() { 1077 return copy(); 1078 } 1079 1080 @Override 1081 public boolean equalsDeep(Base other_) { 1082 if (!super.equalsDeep(other_)) 1083 return false; 1084 if (!(other_ instanceof Endpoint)) 1085 return false; 1086 Endpoint o = (Endpoint) other_; 1087 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(connectionType, o.connectionType, true) 1088 && compareDeep(name, o.name, true) && compareDeep(managingOrganization, o.managingOrganization, true) 1089 && compareDeep(contact, o.contact, true) && compareDeep(period, o.period, true) && compareDeep(payloadType, o.payloadType, true) 1090 && compareDeep(payloadMimeType, o.payloadMimeType, true) && compareDeep(address, o.address, true) 1091 && compareDeep(header, o.header, true); 1092 } 1093 1094 @Override 1095 public boolean equalsShallow(Base other_) { 1096 if (!super.equalsShallow(other_)) 1097 return false; 1098 if (!(other_ instanceof Endpoint)) 1099 return false; 1100 Endpoint o = (Endpoint) other_; 1101 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(payloadMimeType, o.payloadMimeType, true) 1102 && compareValues(address, o.address, true) && compareValues(header, o.header, true); 1103 } 1104 1105 public boolean isEmpty() { 1106 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, connectionType 1107 , name, managingOrganization, contact, period, payloadType, payloadMimeType, address 1108 , header); 1109 } 1110 1111 @Override 1112 public ResourceType getResourceType() { 1113 return ResourceType.Endpoint; 1114 } 1115 1116 /** 1117 * Search parameter: <b>payload-type</b> 1118 * <p> 1119 * Description: <b>The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)</b><br> 1120 * Type: <b>token</b><br> 1121 * Path: <b>Endpoint.payloadType</b><br> 1122 * </p> 1123 */ 1124 @SearchParamDefinition(name="payload-type", path="Endpoint.payloadType", description="The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)", type="token" ) 1125 public static final String SP_PAYLOAD_TYPE = "payload-type"; 1126 /** 1127 * <b>Fluent Client</b> search parameter constant for <b>payload-type</b> 1128 * <p> 1129 * Description: <b>The type of content that may be used at this endpoint (e.g. XDS Discharge summaries)</b><br> 1130 * Type: <b>token</b><br> 1131 * Path: <b>Endpoint.payloadType</b><br> 1132 * </p> 1133 */ 1134 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PAYLOAD_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PAYLOAD_TYPE); 1135 1136 /** 1137 * Search parameter: <b>identifier</b> 1138 * <p> 1139 * Description: <b>Identifies this endpoint across multiple systems</b><br> 1140 * Type: <b>token</b><br> 1141 * Path: <b>Endpoint.identifier</b><br> 1142 * </p> 1143 */ 1144 @SearchParamDefinition(name="identifier", path="Endpoint.identifier", description="Identifies this endpoint across multiple systems", type="token" ) 1145 public static final String SP_IDENTIFIER = "identifier"; 1146 /** 1147 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1148 * <p> 1149 * Description: <b>Identifies this endpoint across multiple systems</b><br> 1150 * Type: <b>token</b><br> 1151 * Path: <b>Endpoint.identifier</b><br> 1152 * </p> 1153 */ 1154 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1155 1156 /** 1157 * Search parameter: <b>organization</b> 1158 * <p> 1159 * Description: <b>The organization that is managing the endpoint</b><br> 1160 * Type: <b>reference</b><br> 1161 * Path: <b>Endpoint.managingOrganization</b><br> 1162 * </p> 1163 */ 1164 @SearchParamDefinition(name="organization", path="Endpoint.managingOrganization", description="The organization that is managing the endpoint", type="reference", target={Organization.class } ) 1165 public static final String SP_ORGANIZATION = "organization"; 1166 /** 1167 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1168 * <p> 1169 * Description: <b>The organization that is managing the endpoint</b><br> 1170 * Type: <b>reference</b><br> 1171 * Path: <b>Endpoint.managingOrganization</b><br> 1172 * </p> 1173 */ 1174 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1175 1176/** 1177 * Constant for fluent queries to be used to add include statements. Specifies 1178 * the path value of "<b>Endpoint:organization</b>". 1179 */ 1180 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Endpoint:organization").toLocked(); 1181 1182 /** 1183 * Search parameter: <b>connection-type</b> 1184 * <p> 1185 * Description: <b>Protocol/Profile/Standard to be used with this endpoint connection</b><br> 1186 * Type: <b>token</b><br> 1187 * Path: <b>Endpoint.connectionType</b><br> 1188 * </p> 1189 */ 1190 @SearchParamDefinition(name="connection-type", path="Endpoint.connectionType", description="Protocol/Profile/Standard to be used with this endpoint connection", type="token" ) 1191 public static final String SP_CONNECTION_TYPE = "connection-type"; 1192 /** 1193 * <b>Fluent Client</b> search parameter constant for <b>connection-type</b> 1194 * <p> 1195 * Description: <b>Protocol/Profile/Standard to be used with this endpoint connection</b><br> 1196 * Type: <b>token</b><br> 1197 * Path: <b>Endpoint.connectionType</b><br> 1198 * </p> 1199 */ 1200 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONNECTION_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONNECTION_TYPE); 1201 1202 /** 1203 * Search parameter: <b>name</b> 1204 * <p> 1205 * Description: <b>A name that this endpoint can be identified by</b><br> 1206 * Type: <b>string</b><br> 1207 * Path: <b>Endpoint.name</b><br> 1208 * </p> 1209 */ 1210 @SearchParamDefinition(name="name", path="Endpoint.name", description="A name that this endpoint can be identified by", type="string" ) 1211 public static final String SP_NAME = "name"; 1212 /** 1213 * <b>Fluent Client</b> search parameter constant for <b>name</b> 1214 * <p> 1215 * Description: <b>A name that this endpoint can be identified by</b><br> 1216 * Type: <b>string</b><br> 1217 * Path: <b>Endpoint.name</b><br> 1218 * </p> 1219 */ 1220 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 1221 1222 /** 1223 * Search parameter: <b>status</b> 1224 * <p> 1225 * Description: <b>The current status of the Endpoint (usually expected to be active)</b><br> 1226 * Type: <b>token</b><br> 1227 * Path: <b>Endpoint.status</b><br> 1228 * </p> 1229 */ 1230 @SearchParamDefinition(name="status", path="Endpoint.status", description="The current status of the Endpoint (usually expected to be active)", type="token" ) 1231 public static final String SP_STATUS = "status"; 1232 /** 1233 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1234 * <p> 1235 * Description: <b>The current status of the Endpoint (usually expected to be active)</b><br> 1236 * Type: <b>token</b><br> 1237 * Path: <b>Endpoint.status</b><br> 1238 * </p> 1239 */ 1240 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1241 1242 1243}