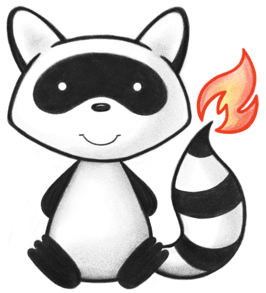
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.Description; 043import ca.uhn.fhir.model.api.annotation.ResourceDef; 044import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 045/** 046 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 047 */ 048@ResourceDef(name="EnrollmentRequest", profile="http://hl7.org/fhir/Profile/EnrollmentRequest") 049public class EnrollmentRequest extends DomainResource { 050 051 public enum EnrollmentRequestStatus { 052 /** 053 * The instance is currently in-force. 054 */ 055 ACTIVE, 056 /** 057 * The instance is withdrawn, rescinded or reversed. 058 */ 059 CANCELLED, 060 /** 061 * A new instance the contents of which is not complete. 062 */ 063 DRAFT, 064 /** 065 * The instance was entered in error. 066 */ 067 ENTEREDINERROR, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 public static EnrollmentRequestStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("active".equals(codeString)) 076 return ACTIVE; 077 if ("cancelled".equals(codeString)) 078 return CANCELLED; 079 if ("draft".equals(codeString)) 080 return DRAFT; 081 if ("entered-in-error".equals(codeString)) 082 return ENTEREDINERROR; 083 if (Configuration.isAcceptInvalidEnums()) 084 return null; 085 else 086 throw new FHIRException("Unknown EnrollmentRequestStatus code '"+codeString+"'"); 087 } 088 public String toCode() { 089 switch (this) { 090 case ACTIVE: return "active"; 091 case CANCELLED: return "cancelled"; 092 case DRAFT: return "draft"; 093 case ENTEREDINERROR: return "entered-in-error"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 101 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 102 case DRAFT: return "http://hl7.org/fhir/fm-status"; 103 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getDefinition() { 109 switch (this) { 110 case ACTIVE: return "The instance is currently in-force."; 111 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 112 case DRAFT: return "A new instance the contents of which is not complete."; 113 case ENTEREDINERROR: return "The instance was entered in error."; 114 case NULL: return null; 115 default: return "?"; 116 } 117 } 118 public String getDisplay() { 119 switch (this) { 120 case ACTIVE: return "Active"; 121 case CANCELLED: return "Cancelled"; 122 case DRAFT: return "Draft"; 123 case ENTEREDINERROR: return "Entered in Error"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 } 129 130 public static class EnrollmentRequestStatusEnumFactory implements EnumFactory<EnrollmentRequestStatus> { 131 public EnrollmentRequestStatus fromCode(String codeString) throws IllegalArgumentException { 132 if (codeString == null || "".equals(codeString)) 133 if (codeString == null || "".equals(codeString)) 134 return null; 135 if ("active".equals(codeString)) 136 return EnrollmentRequestStatus.ACTIVE; 137 if ("cancelled".equals(codeString)) 138 return EnrollmentRequestStatus.CANCELLED; 139 if ("draft".equals(codeString)) 140 return EnrollmentRequestStatus.DRAFT; 141 if ("entered-in-error".equals(codeString)) 142 return EnrollmentRequestStatus.ENTEREDINERROR; 143 throw new IllegalArgumentException("Unknown EnrollmentRequestStatus code '"+codeString+"'"); 144 } 145 public Enumeration<EnrollmentRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 146 if (code == null) 147 return null; 148 if (code.isEmpty()) 149 return new Enumeration<EnrollmentRequestStatus>(this); 150 String codeString = code.asStringValue(); 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("active".equals(codeString)) 154 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.ACTIVE); 155 if ("cancelled".equals(codeString)) 156 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.CANCELLED); 157 if ("draft".equals(codeString)) 158 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.DRAFT); 159 if ("entered-in-error".equals(codeString)) 160 return new Enumeration<EnrollmentRequestStatus>(this, EnrollmentRequestStatus.ENTEREDINERROR); 161 throw new FHIRException("Unknown EnrollmentRequestStatus code '"+codeString+"'"); 162 } 163 public String toCode(EnrollmentRequestStatus code) { 164 if (code == EnrollmentRequestStatus.NULL) 165 return null; 166 if (code == EnrollmentRequestStatus.ACTIVE) 167 return "active"; 168 if (code == EnrollmentRequestStatus.CANCELLED) 169 return "cancelled"; 170 if (code == EnrollmentRequestStatus.DRAFT) 171 return "draft"; 172 if (code == EnrollmentRequestStatus.ENTEREDINERROR) 173 return "entered-in-error"; 174 return "?"; 175 } 176 public String toSystem(EnrollmentRequestStatus code) { 177 return code.getSystem(); 178 } 179 } 180 181 /** 182 * The Response business identifier. 183 */ 184 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 185 @Description(shortDefinition="Business Identifier", formalDefinition="The Response business identifier." ) 186 protected List<Identifier> identifier; 187 188 /** 189 * The status of the resource instance. 190 */ 191 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 192 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 194 protected Enumeration<EnrollmentRequestStatus> status; 195 196 /** 197 * The date when this resource was created. 198 */ 199 @Child(name = "created", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 200 @Description(shortDefinition="Creation date", formalDefinition="The date when this resource was created." ) 201 protected DateTimeType created; 202 203 /** 204 * The Insurer who is target of the request. 205 */ 206 @Child(name = "insurer", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 207 @Description(shortDefinition="Target", formalDefinition="The Insurer who is target of the request." ) 208 protected Reference insurer; 209 210 /** 211 * The actual object that is the target of the reference (The Insurer who is target of the request.) 212 */ 213 protected Organization insurerTarget; 214 215 /** 216 * The practitioner who is responsible for the services rendered to the patient. 217 */ 218 @Child(name = "provider", type = {Practitioner.class}, order=4, min=0, max=1, modifier=false, summary=false) 219 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 220 protected Reference provider; 221 222 /** 223 * The actual object that is the target of the reference (The practitioner who is responsible for the services rendered to the patient.) 224 */ 225 protected Practitioner providerTarget; 226 227 /** 228 * The organization which is responsible for the services rendered to the patient. 229 */ 230 @Child(name = "organization", type = {Organization.class}, order=5, min=0, max=1, modifier=false, summary=false) 231 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the services rendered to the patient." ) 232 protected Reference organization; 233 234 /** 235 * The actual object that is the target of the reference (The organization which is responsible for the services rendered to the patient.) 236 */ 237 protected Organization organizationTarget; 238 239 /** 240 * Patient Resource. 241 */ 242 @Child(name = "subject", type = {Patient.class}, order=6, min=0, max=1, modifier=false, summary=false) 243 @Description(shortDefinition="The subject of the Products and Services", formalDefinition="Patient Resource." ) 244 protected Reference subject; 245 246 /** 247 * The actual object that is the target of the reference (Patient Resource.) 248 */ 249 protected Patient subjectTarget; 250 251 /** 252 * Reference to the program or plan identification, underwriter or payor. 253 */ 254 @Child(name = "coverage", type = {Coverage.class}, order=7, min=0, max=1, modifier=false, summary=false) 255 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the program or plan identification, underwriter or payor." ) 256 protected Reference coverage; 257 258 /** 259 * The actual object that is the target of the reference (Reference to the program or plan identification, underwriter or payor.) 260 */ 261 protected Coverage coverageTarget; 262 263 private static final long serialVersionUID = -214445454L; 264 265 /** 266 * Constructor 267 */ 268 public EnrollmentRequest() { 269 super(); 270 } 271 272 /** 273 * @return {@link #identifier} (The Response business identifier.) 274 */ 275 public List<Identifier> getIdentifier() { 276 if (this.identifier == null) 277 this.identifier = new ArrayList<Identifier>(); 278 return this.identifier; 279 } 280 281 /** 282 * @return Returns a reference to <code>this</code> for easy method chaining 283 */ 284 public EnrollmentRequest setIdentifier(List<Identifier> theIdentifier) { 285 this.identifier = theIdentifier; 286 return this; 287 } 288 289 public boolean hasIdentifier() { 290 if (this.identifier == null) 291 return false; 292 for (Identifier item : this.identifier) 293 if (!item.isEmpty()) 294 return true; 295 return false; 296 } 297 298 public Identifier addIdentifier() { //3 299 Identifier t = new Identifier(); 300 if (this.identifier == null) 301 this.identifier = new ArrayList<Identifier>(); 302 this.identifier.add(t); 303 return t; 304 } 305 306 public EnrollmentRequest addIdentifier(Identifier t) { //3 307 if (t == null) 308 return this; 309 if (this.identifier == null) 310 this.identifier = new ArrayList<Identifier>(); 311 this.identifier.add(t); 312 return this; 313 } 314 315 /** 316 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 317 */ 318 public Identifier getIdentifierFirstRep() { 319 if (getIdentifier().isEmpty()) { 320 addIdentifier(); 321 } 322 return getIdentifier().get(0); 323 } 324 325 /** 326 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 327 */ 328 public Enumeration<EnrollmentRequestStatus> getStatusElement() { 329 if (this.status == null) 330 if (Configuration.errorOnAutoCreate()) 331 throw new Error("Attempt to auto-create EnrollmentRequest.status"); 332 else if (Configuration.doAutoCreate()) 333 this.status = new Enumeration<EnrollmentRequestStatus>(new EnrollmentRequestStatusEnumFactory()); // bb 334 return this.status; 335 } 336 337 public boolean hasStatusElement() { 338 return this.status != null && !this.status.isEmpty(); 339 } 340 341 public boolean hasStatus() { 342 return this.status != null && !this.status.isEmpty(); 343 } 344 345 /** 346 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 347 */ 348 public EnrollmentRequest setStatusElement(Enumeration<EnrollmentRequestStatus> value) { 349 this.status = value; 350 return this; 351 } 352 353 /** 354 * @return The status of the resource instance. 355 */ 356 public EnrollmentRequestStatus getStatus() { 357 return this.status == null ? null : this.status.getValue(); 358 } 359 360 /** 361 * @param value The status of the resource instance. 362 */ 363 public EnrollmentRequest setStatus(EnrollmentRequestStatus value) { 364 if (value == null) 365 this.status = null; 366 else { 367 if (this.status == null) 368 this.status = new Enumeration<EnrollmentRequestStatus>(new EnrollmentRequestStatusEnumFactory()); 369 this.status.setValue(value); 370 } 371 return this; 372 } 373 374 /** 375 * @return {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 376 */ 377 public DateTimeType getCreatedElement() { 378 if (this.created == null) 379 if (Configuration.errorOnAutoCreate()) 380 throw new Error("Attempt to auto-create EnrollmentRequest.created"); 381 else if (Configuration.doAutoCreate()) 382 this.created = new DateTimeType(); // bb 383 return this.created; 384 } 385 386 public boolean hasCreatedElement() { 387 return this.created != null && !this.created.isEmpty(); 388 } 389 390 public boolean hasCreated() { 391 return this.created != null && !this.created.isEmpty(); 392 } 393 394 /** 395 * @param value {@link #created} (The date when this resource was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 396 */ 397 public EnrollmentRequest setCreatedElement(DateTimeType value) { 398 this.created = value; 399 return this; 400 } 401 402 /** 403 * @return The date when this resource was created. 404 */ 405 public Date getCreated() { 406 return this.created == null ? null : this.created.getValue(); 407 } 408 409 /** 410 * @param value The date when this resource was created. 411 */ 412 public EnrollmentRequest setCreated(Date value) { 413 if (value == null) 414 this.created = null; 415 else { 416 if (this.created == null) 417 this.created = new DateTimeType(); 418 this.created.setValue(value); 419 } 420 return this; 421 } 422 423 /** 424 * @return {@link #insurer} (The Insurer who is target of the request.) 425 */ 426 public Reference getInsurer() { 427 if (this.insurer == null) 428 if (Configuration.errorOnAutoCreate()) 429 throw new Error("Attempt to auto-create EnrollmentRequest.insurer"); 430 else if (Configuration.doAutoCreate()) 431 this.insurer = new Reference(); // cc 432 return this.insurer; 433 } 434 435 public boolean hasInsurer() { 436 return this.insurer != null && !this.insurer.isEmpty(); 437 } 438 439 /** 440 * @param value {@link #insurer} (The Insurer who is target of the request.) 441 */ 442 public EnrollmentRequest setInsurer(Reference value) { 443 this.insurer = value; 444 return this; 445 } 446 447 /** 448 * @return {@link #insurer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 449 */ 450 public Organization getInsurerTarget() { 451 if (this.insurerTarget == null) 452 if (Configuration.errorOnAutoCreate()) 453 throw new Error("Attempt to auto-create EnrollmentRequest.insurer"); 454 else if (Configuration.doAutoCreate()) 455 this.insurerTarget = new Organization(); // aa 456 return this.insurerTarget; 457 } 458 459 /** 460 * @param value {@link #insurer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who is target of the request.) 461 */ 462 public EnrollmentRequest setInsurerTarget(Organization value) { 463 this.insurerTarget = value; 464 return this; 465 } 466 467 /** 468 * @return {@link #provider} (The practitioner who is responsible for the services rendered to the patient.) 469 */ 470 public Reference getProvider() { 471 if (this.provider == null) 472 if (Configuration.errorOnAutoCreate()) 473 throw new Error("Attempt to auto-create EnrollmentRequest.provider"); 474 else if (Configuration.doAutoCreate()) 475 this.provider = new Reference(); // cc 476 return this.provider; 477 } 478 479 public boolean hasProvider() { 480 return this.provider != null && !this.provider.isEmpty(); 481 } 482 483 /** 484 * @param value {@link #provider} (The practitioner who is responsible for the services rendered to the patient.) 485 */ 486 public EnrollmentRequest setProvider(Reference value) { 487 this.provider = value; 488 return this; 489 } 490 491 /** 492 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 493 */ 494 public Practitioner getProviderTarget() { 495 if (this.providerTarget == null) 496 if (Configuration.errorOnAutoCreate()) 497 throw new Error("Attempt to auto-create EnrollmentRequest.provider"); 498 else if (Configuration.doAutoCreate()) 499 this.providerTarget = new Practitioner(); // aa 500 return this.providerTarget; 501 } 502 503 /** 504 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 505 */ 506 public EnrollmentRequest setProviderTarget(Practitioner value) { 507 this.providerTarget = value; 508 return this; 509 } 510 511 /** 512 * @return {@link #organization} (The organization which is responsible for the services rendered to the patient.) 513 */ 514 public Reference getOrganization() { 515 if (this.organization == null) 516 if (Configuration.errorOnAutoCreate()) 517 throw new Error("Attempt to auto-create EnrollmentRequest.organization"); 518 else if (Configuration.doAutoCreate()) 519 this.organization = new Reference(); // cc 520 return this.organization; 521 } 522 523 public boolean hasOrganization() { 524 return this.organization != null && !this.organization.isEmpty(); 525 } 526 527 /** 528 * @param value {@link #organization} (The organization which is responsible for the services rendered to the patient.) 529 */ 530 public EnrollmentRequest setOrganization(Reference value) { 531 this.organization = value; 532 return this; 533 } 534 535 /** 536 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 537 */ 538 public Organization getOrganizationTarget() { 539 if (this.organizationTarget == null) 540 if (Configuration.errorOnAutoCreate()) 541 throw new Error("Attempt to auto-create EnrollmentRequest.organization"); 542 else if (Configuration.doAutoCreate()) 543 this.organizationTarget = new Organization(); // aa 544 return this.organizationTarget; 545 } 546 547 /** 548 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 549 */ 550 public EnrollmentRequest setOrganizationTarget(Organization value) { 551 this.organizationTarget = value; 552 return this; 553 } 554 555 /** 556 * @return {@link #subject} (Patient Resource.) 557 */ 558 public Reference getSubject() { 559 if (this.subject == null) 560 if (Configuration.errorOnAutoCreate()) 561 throw new Error("Attempt to auto-create EnrollmentRequest.subject"); 562 else if (Configuration.doAutoCreate()) 563 this.subject = new Reference(); // cc 564 return this.subject; 565 } 566 567 public boolean hasSubject() { 568 return this.subject != null && !this.subject.isEmpty(); 569 } 570 571 /** 572 * @param value {@link #subject} (Patient Resource.) 573 */ 574 public EnrollmentRequest setSubject(Reference value) { 575 this.subject = value; 576 return this; 577 } 578 579 /** 580 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient Resource.) 581 */ 582 public Patient getSubjectTarget() { 583 if (this.subjectTarget == null) 584 if (Configuration.errorOnAutoCreate()) 585 throw new Error("Attempt to auto-create EnrollmentRequest.subject"); 586 else if (Configuration.doAutoCreate()) 587 this.subjectTarget = new Patient(); // aa 588 return this.subjectTarget; 589 } 590 591 /** 592 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient Resource.) 593 */ 594 public EnrollmentRequest setSubjectTarget(Patient value) { 595 this.subjectTarget = value; 596 return this; 597 } 598 599 /** 600 * @return {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 601 */ 602 public Reference getCoverage() { 603 if (this.coverage == null) 604 if (Configuration.errorOnAutoCreate()) 605 throw new Error("Attempt to auto-create EnrollmentRequest.coverage"); 606 else if (Configuration.doAutoCreate()) 607 this.coverage = new Reference(); // cc 608 return this.coverage; 609 } 610 611 public boolean hasCoverage() { 612 return this.coverage != null && !this.coverage.isEmpty(); 613 } 614 615 /** 616 * @param value {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 617 */ 618 public EnrollmentRequest setCoverage(Reference value) { 619 this.coverage = value; 620 return this; 621 } 622 623 /** 624 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 625 */ 626 public Coverage getCoverageTarget() { 627 if (this.coverageTarget == null) 628 if (Configuration.errorOnAutoCreate()) 629 throw new Error("Attempt to auto-create EnrollmentRequest.coverage"); 630 else if (Configuration.doAutoCreate()) 631 this.coverageTarget = new Coverage(); // aa 632 return this.coverageTarget; 633 } 634 635 /** 636 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 637 */ 638 public EnrollmentRequest setCoverageTarget(Coverage value) { 639 this.coverageTarget = value; 640 return this; 641 } 642 643 protected void listChildren(List<Property> children) { 644 super.listChildren(children); 645 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 646 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 647 children.add(new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created)); 648 children.add(new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer)); 649 children.add(new Property("provider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider)); 650 children.add(new Property("organization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, organization)); 651 children.add(new Property("subject", "Reference(Patient)", "Patient Resource.", 0, 1, subject)); 652 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage)); 653 } 654 655 @Override 656 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 657 switch (_hash) { 658 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 659 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 660 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when this resource was created.", 0, 1, created); 661 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The Insurer who is target of the request.", 0, 1, insurer); 662 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, provider); 663 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, organization); 664 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient)", "Patient Resource.", 0, 1, subject); 665 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage); 666 default: return super.getNamedProperty(_hash, _name, _checkValid); 667 } 668 669 } 670 671 @Override 672 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 673 switch (hash) { 674 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 675 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EnrollmentRequestStatus> 676 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 677 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 678 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 679 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 680 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 681 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 682 default: return super.getProperty(hash, name, checkValid); 683 } 684 685 } 686 687 @Override 688 public Base setProperty(int hash, String name, Base value) throws FHIRException { 689 switch (hash) { 690 case -1618432855: // identifier 691 this.getIdentifier().add(castToIdentifier(value)); // Identifier 692 return value; 693 case -892481550: // status 694 value = new EnrollmentRequestStatusEnumFactory().fromType(castToCode(value)); 695 this.status = (Enumeration) value; // Enumeration<EnrollmentRequestStatus> 696 return value; 697 case 1028554472: // created 698 this.created = castToDateTime(value); // DateTimeType 699 return value; 700 case 1957615864: // insurer 701 this.insurer = castToReference(value); // Reference 702 return value; 703 case -987494927: // provider 704 this.provider = castToReference(value); // Reference 705 return value; 706 case 1178922291: // organization 707 this.organization = castToReference(value); // Reference 708 return value; 709 case -1867885268: // subject 710 this.subject = castToReference(value); // Reference 711 return value; 712 case -351767064: // coverage 713 this.coverage = castToReference(value); // Reference 714 return value; 715 default: return super.setProperty(hash, name, value); 716 } 717 718 } 719 720 @Override 721 public Base setProperty(String name, Base value) throws FHIRException { 722 if (name.equals("identifier")) { 723 this.getIdentifier().add(castToIdentifier(value)); 724 } else if (name.equals("status")) { 725 value = new EnrollmentRequestStatusEnumFactory().fromType(castToCode(value)); 726 this.status = (Enumeration) value; // Enumeration<EnrollmentRequestStatus> 727 } else if (name.equals("created")) { 728 this.created = castToDateTime(value); // DateTimeType 729 } else if (name.equals("insurer")) { 730 this.insurer = castToReference(value); // Reference 731 } else if (name.equals("provider")) { 732 this.provider = castToReference(value); // Reference 733 } else if (name.equals("organization")) { 734 this.organization = castToReference(value); // Reference 735 } else if (name.equals("subject")) { 736 this.subject = castToReference(value); // Reference 737 } else if (name.equals("coverage")) { 738 this.coverage = castToReference(value); // Reference 739 } else 740 return super.setProperty(name, value); 741 return value; 742 } 743 744 @Override 745 public Base makeProperty(int hash, String name) throws FHIRException { 746 switch (hash) { 747 case -1618432855: return addIdentifier(); 748 case -892481550: return getStatusElement(); 749 case 1028554472: return getCreatedElement(); 750 case 1957615864: return getInsurer(); 751 case -987494927: return getProvider(); 752 case 1178922291: return getOrganization(); 753 case -1867885268: return getSubject(); 754 case -351767064: return getCoverage(); 755 default: return super.makeProperty(hash, name); 756 } 757 758 } 759 760 @Override 761 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 762 switch (hash) { 763 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 764 case -892481550: /*status*/ return new String[] {"code"}; 765 case 1028554472: /*created*/ return new String[] {"dateTime"}; 766 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 767 case -987494927: /*provider*/ return new String[] {"Reference"}; 768 case 1178922291: /*organization*/ return new String[] {"Reference"}; 769 case -1867885268: /*subject*/ return new String[] {"Reference"}; 770 case -351767064: /*coverage*/ return new String[] {"Reference"}; 771 default: return super.getTypesForProperty(hash, name); 772 } 773 774 } 775 776 @Override 777 public Base addChild(String name) throws FHIRException { 778 if (name.equals("identifier")) { 779 return addIdentifier(); 780 } 781 else if (name.equals("status")) { 782 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentRequest.status"); 783 } 784 else if (name.equals("created")) { 785 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentRequest.created"); 786 } 787 else if (name.equals("insurer")) { 788 this.insurer = new Reference(); 789 return this.insurer; 790 } 791 else if (name.equals("provider")) { 792 this.provider = new Reference(); 793 return this.provider; 794 } 795 else if (name.equals("organization")) { 796 this.organization = new Reference(); 797 return this.organization; 798 } 799 else if (name.equals("subject")) { 800 this.subject = new Reference(); 801 return this.subject; 802 } 803 else if (name.equals("coverage")) { 804 this.coverage = new Reference(); 805 return this.coverage; 806 } 807 else 808 return super.addChild(name); 809 } 810 811 public String fhirType() { 812 return "EnrollmentRequest"; 813 814 } 815 816 public EnrollmentRequest copy() { 817 EnrollmentRequest dst = new EnrollmentRequest(); 818 copyValues(dst); 819 if (identifier != null) { 820 dst.identifier = new ArrayList<Identifier>(); 821 for (Identifier i : identifier) 822 dst.identifier.add(i.copy()); 823 }; 824 dst.status = status == null ? null : status.copy(); 825 dst.created = created == null ? null : created.copy(); 826 dst.insurer = insurer == null ? null : insurer.copy(); 827 dst.provider = provider == null ? null : provider.copy(); 828 dst.organization = organization == null ? null : organization.copy(); 829 dst.subject = subject == null ? null : subject.copy(); 830 dst.coverage = coverage == null ? null : coverage.copy(); 831 return dst; 832 } 833 834 protected EnrollmentRequest typedCopy() { 835 return copy(); 836 } 837 838 @Override 839 public boolean equalsDeep(Base other_) { 840 if (!super.equalsDeep(other_)) 841 return false; 842 if (!(other_ instanceof EnrollmentRequest)) 843 return false; 844 EnrollmentRequest o = (EnrollmentRequest) other_; 845 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(created, o.created, true) 846 && compareDeep(insurer, o.insurer, true) && compareDeep(provider, o.provider, true) && compareDeep(organization, o.organization, true) 847 && compareDeep(subject, o.subject, true) && compareDeep(coverage, o.coverage, true); 848 } 849 850 @Override 851 public boolean equalsShallow(Base other_) { 852 if (!super.equalsShallow(other_)) 853 return false; 854 if (!(other_ instanceof EnrollmentRequest)) 855 return false; 856 EnrollmentRequest o = (EnrollmentRequest) other_; 857 return compareValues(status, o.status, true) && compareValues(created, o.created, true); 858 } 859 860 public boolean isEmpty() { 861 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, created 862 , insurer, provider, organization, subject, coverage); 863 } 864 865 @Override 866 public ResourceType getResourceType() { 867 return ResourceType.EnrollmentRequest; 868 } 869 870 /** 871 * Search parameter: <b>identifier</b> 872 * <p> 873 * Description: <b>The business identifier of the Enrollment</b><br> 874 * Type: <b>token</b><br> 875 * Path: <b>EnrollmentRequest.identifier</b><br> 876 * </p> 877 */ 878 @SearchParamDefinition(name="identifier", path="EnrollmentRequest.identifier", description="The business identifier of the Enrollment", type="token" ) 879 public static final String SP_IDENTIFIER = "identifier"; 880 /** 881 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 882 * <p> 883 * Description: <b>The business identifier of the Enrollment</b><br> 884 * Type: <b>token</b><br> 885 * Path: <b>EnrollmentRequest.identifier</b><br> 886 * </p> 887 */ 888 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 889 890 /** 891 * Search parameter: <b>subject</b> 892 * <p> 893 * Description: <b>The party to be enrolled</b><br> 894 * Type: <b>reference</b><br> 895 * Path: <b>EnrollmentRequest.subject</b><br> 896 * </p> 897 */ 898 @SearchParamDefinition(name="subject", path="EnrollmentRequest.subject", description="The party to be enrolled", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 899 public static final String SP_SUBJECT = "subject"; 900 /** 901 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 902 * <p> 903 * Description: <b>The party to be enrolled</b><br> 904 * Type: <b>reference</b><br> 905 * Path: <b>EnrollmentRequest.subject</b><br> 906 * </p> 907 */ 908 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 909 910/** 911 * Constant for fluent queries to be used to add include statements. Specifies 912 * the path value of "<b>EnrollmentRequest:subject</b>". 913 */ 914 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("EnrollmentRequest:subject").toLocked(); 915 916 /** 917 * Search parameter: <b>patient</b> 918 * <p> 919 * Description: <b>The party to be enrolled</b><br> 920 * Type: <b>reference</b><br> 921 * Path: <b>EnrollmentRequest.subject</b><br> 922 * </p> 923 */ 924 @SearchParamDefinition(name="patient", path="EnrollmentRequest.subject", description="The party to be enrolled", type="reference", target={Patient.class } ) 925 public static final String SP_PATIENT = "patient"; 926 /** 927 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 928 * <p> 929 * Description: <b>The party to be enrolled</b><br> 930 * Type: <b>reference</b><br> 931 * Path: <b>EnrollmentRequest.subject</b><br> 932 * </p> 933 */ 934 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 935 936/** 937 * Constant for fluent queries to be used to add include statements. Specifies 938 * the path value of "<b>EnrollmentRequest:patient</b>". 939 */ 940 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("EnrollmentRequest:patient").toLocked(); 941 942 /** 943 * Search parameter: <b>organization</b> 944 * <p> 945 * Description: <b>The organization who generated this resource</b><br> 946 * Type: <b>reference</b><br> 947 * Path: <b>EnrollmentRequest.organization</b><br> 948 * </p> 949 */ 950 @SearchParamDefinition(name="organization", path="EnrollmentRequest.organization", description="The organization who generated this resource", type="reference", target={Organization.class } ) 951 public static final String SP_ORGANIZATION = "organization"; 952 /** 953 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 954 * <p> 955 * Description: <b>The organization who generated this resource</b><br> 956 * Type: <b>reference</b><br> 957 * Path: <b>EnrollmentRequest.organization</b><br> 958 * </p> 959 */ 960 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 961 962/** 963 * Constant for fluent queries to be used to add include statements. Specifies 964 * the path value of "<b>EnrollmentRequest:organization</b>". 965 */ 966 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("EnrollmentRequest:organization").toLocked(); 967 968 969}