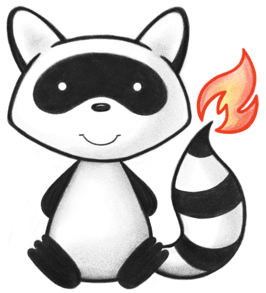
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.utilities.Utilities; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * This resource provides enrollment and plan details from the processing of an Enrollment resource. 048 */ 049@ResourceDef(name="EnrollmentResponse", profile="http://hl7.org/fhir/Profile/EnrollmentResponse") 050public class EnrollmentResponse extends DomainResource { 051 052 public enum EnrollmentResponseStatus { 053 /** 054 * The instance is currently in-force. 055 */ 056 ACTIVE, 057 /** 058 * The instance is withdrawn, rescinded or reversed. 059 */ 060 CANCELLED, 061 /** 062 * A new instance the contents of which is not complete. 063 */ 064 DRAFT, 065 /** 066 * The instance was entered in error. 067 */ 068 ENTEREDINERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static EnrollmentResponseStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("cancelled".equals(codeString)) 079 return CANCELLED; 080 if ("draft".equals(codeString)) 081 return DRAFT; 082 if ("entered-in-error".equals(codeString)) 083 return ENTEREDINERROR; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown EnrollmentResponseStatus code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case ACTIVE: return "active"; 092 case CANCELLED: return "cancelled"; 093 case DRAFT: return "draft"; 094 case ENTEREDINERROR: return "entered-in-error"; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 public String getSystem() { 100 switch (this) { 101 case ACTIVE: return "http://hl7.org/fhir/fm-status"; 102 case CANCELLED: return "http://hl7.org/fhir/fm-status"; 103 case DRAFT: return "http://hl7.org/fhir/fm-status"; 104 case ENTEREDINERROR: return "http://hl7.org/fhir/fm-status"; 105 case NULL: return null; 106 default: return "?"; 107 } 108 } 109 public String getDefinition() { 110 switch (this) { 111 case ACTIVE: return "The instance is currently in-force."; 112 case CANCELLED: return "The instance is withdrawn, rescinded or reversed."; 113 case DRAFT: return "A new instance the contents of which is not complete."; 114 case ENTEREDINERROR: return "The instance was entered in error."; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 public String getDisplay() { 120 switch (this) { 121 case ACTIVE: return "Active"; 122 case CANCELLED: return "Cancelled"; 123 case DRAFT: return "Draft"; 124 case ENTEREDINERROR: return "Entered in Error"; 125 case NULL: return null; 126 default: return "?"; 127 } 128 } 129 } 130 131 public static class EnrollmentResponseStatusEnumFactory implements EnumFactory<EnrollmentResponseStatus> { 132 public EnrollmentResponseStatus fromCode(String codeString) throws IllegalArgumentException { 133 if (codeString == null || "".equals(codeString)) 134 if (codeString == null || "".equals(codeString)) 135 return null; 136 if ("active".equals(codeString)) 137 return EnrollmentResponseStatus.ACTIVE; 138 if ("cancelled".equals(codeString)) 139 return EnrollmentResponseStatus.CANCELLED; 140 if ("draft".equals(codeString)) 141 return EnrollmentResponseStatus.DRAFT; 142 if ("entered-in-error".equals(codeString)) 143 return EnrollmentResponseStatus.ENTEREDINERROR; 144 throw new IllegalArgumentException("Unknown EnrollmentResponseStatus code '"+codeString+"'"); 145 } 146 public Enumeration<EnrollmentResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 147 if (code == null) 148 return null; 149 if (code.isEmpty()) 150 return new Enumeration<EnrollmentResponseStatus>(this); 151 String codeString = code.asStringValue(); 152 if (codeString == null || "".equals(codeString)) 153 return null; 154 if ("active".equals(codeString)) 155 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.ACTIVE); 156 if ("cancelled".equals(codeString)) 157 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.CANCELLED); 158 if ("draft".equals(codeString)) 159 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.DRAFT); 160 if ("entered-in-error".equals(codeString)) 161 return new Enumeration<EnrollmentResponseStatus>(this, EnrollmentResponseStatus.ENTEREDINERROR); 162 throw new FHIRException("Unknown EnrollmentResponseStatus code '"+codeString+"'"); 163 } 164 public String toCode(EnrollmentResponseStatus code) { 165 if (code == EnrollmentResponseStatus.NULL) 166 return null; 167 if (code == EnrollmentResponseStatus.ACTIVE) 168 return "active"; 169 if (code == EnrollmentResponseStatus.CANCELLED) 170 return "cancelled"; 171 if (code == EnrollmentResponseStatus.DRAFT) 172 return "draft"; 173 if (code == EnrollmentResponseStatus.ENTEREDINERROR) 174 return "entered-in-error"; 175 return "?"; 176 } 177 public String toSystem(EnrollmentResponseStatus code) { 178 return code.getSystem(); 179 } 180 } 181 182 /** 183 * The Response business identifier. 184 */ 185 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 186 @Description(shortDefinition="Business Identifier", formalDefinition="The Response business identifier." ) 187 protected List<Identifier> identifier; 188 189 /** 190 * The status of the resource instance. 191 */ 192 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 193 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 194 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/fm-status") 195 protected Enumeration<EnrollmentResponseStatus> status; 196 197 /** 198 * Original request resource reference. 199 */ 200 @Child(name = "request", type = {EnrollmentRequest.class}, order=2, min=0, max=1, modifier=false, summary=false) 201 @Description(shortDefinition="Claim reference", formalDefinition="Original request resource reference." ) 202 protected Reference request; 203 204 /** 205 * The actual object that is the target of the reference (Original request resource reference.) 206 */ 207 protected EnrollmentRequest requestTarget; 208 209 /** 210 * Processing status: error, complete. 211 */ 212 @Child(name = "outcome", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 213 @Description(shortDefinition="complete | error | partial", formalDefinition="Processing status: error, complete." ) 214 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/remittance-outcome") 215 protected CodeableConcept outcome; 216 217 /** 218 * A description of the status of the adjudication. 219 */ 220 @Child(name = "disposition", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 221 @Description(shortDefinition="Disposition Message", formalDefinition="A description of the status of the adjudication." ) 222 protected StringType disposition; 223 224 /** 225 * The date when the enclosed suite of services were performed or completed. 226 */ 227 @Child(name = "created", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=false) 228 @Description(shortDefinition="Creation date", formalDefinition="The date when the enclosed suite of services were performed or completed." ) 229 protected DateTimeType created; 230 231 /** 232 * The Insurer who produced this adjudicated response. 233 */ 234 @Child(name = "organization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=false) 235 @Description(shortDefinition="Insurer", formalDefinition="The Insurer who produced this adjudicated response." ) 236 protected Reference organization; 237 238 /** 239 * The actual object that is the target of the reference (The Insurer who produced this adjudicated response.) 240 */ 241 protected Organization organizationTarget; 242 243 /** 244 * The practitioner who is responsible for the services rendered to the patient. 245 */ 246 @Child(name = "requestProvider", type = {Practitioner.class}, order=7, min=0, max=1, modifier=false, summary=false) 247 @Description(shortDefinition="Responsible practitioner", formalDefinition="The practitioner who is responsible for the services rendered to the patient." ) 248 protected Reference requestProvider; 249 250 /** 251 * The actual object that is the target of the reference (The practitioner who is responsible for the services rendered to the patient.) 252 */ 253 protected Practitioner requestProviderTarget; 254 255 /** 256 * The organization which is responsible for the services rendered to the patient. 257 */ 258 @Child(name = "requestOrganization", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 259 @Description(shortDefinition="Responsible organization", formalDefinition="The organization which is responsible for the services rendered to the patient." ) 260 protected Reference requestOrganization; 261 262 /** 263 * The actual object that is the target of the reference (The organization which is responsible for the services rendered to the patient.) 264 */ 265 protected Organization requestOrganizationTarget; 266 267 private static final long serialVersionUID = -386781115L; 268 269 /** 270 * Constructor 271 */ 272 public EnrollmentResponse() { 273 super(); 274 } 275 276 /** 277 * @return {@link #identifier} (The Response business identifier.) 278 */ 279 public List<Identifier> getIdentifier() { 280 if (this.identifier == null) 281 this.identifier = new ArrayList<Identifier>(); 282 return this.identifier; 283 } 284 285 /** 286 * @return Returns a reference to <code>this</code> for easy method chaining 287 */ 288 public EnrollmentResponse setIdentifier(List<Identifier> theIdentifier) { 289 this.identifier = theIdentifier; 290 return this; 291 } 292 293 public boolean hasIdentifier() { 294 if (this.identifier == null) 295 return false; 296 for (Identifier item : this.identifier) 297 if (!item.isEmpty()) 298 return true; 299 return false; 300 } 301 302 public Identifier addIdentifier() { //3 303 Identifier t = new Identifier(); 304 if (this.identifier == null) 305 this.identifier = new ArrayList<Identifier>(); 306 this.identifier.add(t); 307 return t; 308 } 309 310 public EnrollmentResponse addIdentifier(Identifier t) { //3 311 if (t == null) 312 return this; 313 if (this.identifier == null) 314 this.identifier = new ArrayList<Identifier>(); 315 this.identifier.add(t); 316 return this; 317 } 318 319 /** 320 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 321 */ 322 public Identifier getIdentifierFirstRep() { 323 if (getIdentifier().isEmpty()) { 324 addIdentifier(); 325 } 326 return getIdentifier().get(0); 327 } 328 329 /** 330 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 331 */ 332 public Enumeration<EnrollmentResponseStatus> getStatusElement() { 333 if (this.status == null) 334 if (Configuration.errorOnAutoCreate()) 335 throw new Error("Attempt to auto-create EnrollmentResponse.status"); 336 else if (Configuration.doAutoCreate()) 337 this.status = new Enumeration<EnrollmentResponseStatus>(new EnrollmentResponseStatusEnumFactory()); // bb 338 return this.status; 339 } 340 341 public boolean hasStatusElement() { 342 return this.status != null && !this.status.isEmpty(); 343 } 344 345 public boolean hasStatus() { 346 return this.status != null && !this.status.isEmpty(); 347 } 348 349 /** 350 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 351 */ 352 public EnrollmentResponse setStatusElement(Enumeration<EnrollmentResponseStatus> value) { 353 this.status = value; 354 return this; 355 } 356 357 /** 358 * @return The status of the resource instance. 359 */ 360 public EnrollmentResponseStatus getStatus() { 361 return this.status == null ? null : this.status.getValue(); 362 } 363 364 /** 365 * @param value The status of the resource instance. 366 */ 367 public EnrollmentResponse setStatus(EnrollmentResponseStatus value) { 368 if (value == null) 369 this.status = null; 370 else { 371 if (this.status == null) 372 this.status = new Enumeration<EnrollmentResponseStatus>(new EnrollmentResponseStatusEnumFactory()); 373 this.status.setValue(value); 374 } 375 return this; 376 } 377 378 /** 379 * @return {@link #request} (Original request resource reference.) 380 */ 381 public Reference getRequest() { 382 if (this.request == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create EnrollmentResponse.request"); 385 else if (Configuration.doAutoCreate()) 386 this.request = new Reference(); // cc 387 return this.request; 388 } 389 390 public boolean hasRequest() { 391 return this.request != null && !this.request.isEmpty(); 392 } 393 394 /** 395 * @param value {@link #request} (Original request resource reference.) 396 */ 397 public EnrollmentResponse setRequest(Reference value) { 398 this.request = value; 399 return this; 400 } 401 402 /** 403 * @return {@link #request} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original request resource reference.) 404 */ 405 public EnrollmentRequest getRequestTarget() { 406 if (this.requestTarget == null) 407 if (Configuration.errorOnAutoCreate()) 408 throw new Error("Attempt to auto-create EnrollmentResponse.request"); 409 else if (Configuration.doAutoCreate()) 410 this.requestTarget = new EnrollmentRequest(); // aa 411 return this.requestTarget; 412 } 413 414 /** 415 * @param value {@link #request} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original request resource reference.) 416 */ 417 public EnrollmentResponse setRequestTarget(EnrollmentRequest value) { 418 this.requestTarget = value; 419 return this; 420 } 421 422 /** 423 * @return {@link #outcome} (Processing status: error, complete.) 424 */ 425 public CodeableConcept getOutcome() { 426 if (this.outcome == null) 427 if (Configuration.errorOnAutoCreate()) 428 throw new Error("Attempt to auto-create EnrollmentResponse.outcome"); 429 else if (Configuration.doAutoCreate()) 430 this.outcome = new CodeableConcept(); // cc 431 return this.outcome; 432 } 433 434 public boolean hasOutcome() { 435 return this.outcome != null && !this.outcome.isEmpty(); 436 } 437 438 /** 439 * @param value {@link #outcome} (Processing status: error, complete.) 440 */ 441 public EnrollmentResponse setOutcome(CodeableConcept value) { 442 this.outcome = value; 443 return this; 444 } 445 446 /** 447 * @return {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 448 */ 449 public StringType getDispositionElement() { 450 if (this.disposition == null) 451 if (Configuration.errorOnAutoCreate()) 452 throw new Error("Attempt to auto-create EnrollmentResponse.disposition"); 453 else if (Configuration.doAutoCreate()) 454 this.disposition = new StringType(); // bb 455 return this.disposition; 456 } 457 458 public boolean hasDispositionElement() { 459 return this.disposition != null && !this.disposition.isEmpty(); 460 } 461 462 public boolean hasDisposition() { 463 return this.disposition != null && !this.disposition.isEmpty(); 464 } 465 466 /** 467 * @param value {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 468 */ 469 public EnrollmentResponse setDispositionElement(StringType value) { 470 this.disposition = value; 471 return this; 472 } 473 474 /** 475 * @return A description of the status of the adjudication. 476 */ 477 public String getDisposition() { 478 return this.disposition == null ? null : this.disposition.getValue(); 479 } 480 481 /** 482 * @param value A description of the status of the adjudication. 483 */ 484 public EnrollmentResponse setDisposition(String value) { 485 if (Utilities.noString(value)) 486 this.disposition = null; 487 else { 488 if (this.disposition == null) 489 this.disposition = new StringType(); 490 this.disposition.setValue(value); 491 } 492 return this; 493 } 494 495 /** 496 * @return {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 497 */ 498 public DateTimeType getCreatedElement() { 499 if (this.created == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create EnrollmentResponse.created"); 502 else if (Configuration.doAutoCreate()) 503 this.created = new DateTimeType(); // bb 504 return this.created; 505 } 506 507 public boolean hasCreatedElement() { 508 return this.created != null && !this.created.isEmpty(); 509 } 510 511 public boolean hasCreated() { 512 return this.created != null && !this.created.isEmpty(); 513 } 514 515 /** 516 * @param value {@link #created} (The date when the enclosed suite of services were performed or completed.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 517 */ 518 public EnrollmentResponse setCreatedElement(DateTimeType value) { 519 this.created = value; 520 return this; 521 } 522 523 /** 524 * @return The date when the enclosed suite of services were performed or completed. 525 */ 526 public Date getCreated() { 527 return this.created == null ? null : this.created.getValue(); 528 } 529 530 /** 531 * @param value The date when the enclosed suite of services were performed or completed. 532 */ 533 public EnrollmentResponse setCreated(Date value) { 534 if (value == null) 535 this.created = null; 536 else { 537 if (this.created == null) 538 this.created = new DateTimeType(); 539 this.created.setValue(value); 540 } 541 return this; 542 } 543 544 /** 545 * @return {@link #organization} (The Insurer who produced this adjudicated response.) 546 */ 547 public Reference getOrganization() { 548 if (this.organization == null) 549 if (Configuration.errorOnAutoCreate()) 550 throw new Error("Attempt to auto-create EnrollmentResponse.organization"); 551 else if (Configuration.doAutoCreate()) 552 this.organization = new Reference(); // cc 553 return this.organization; 554 } 555 556 public boolean hasOrganization() { 557 return this.organization != null && !this.organization.isEmpty(); 558 } 559 560 /** 561 * @param value {@link #organization} (The Insurer who produced this adjudicated response.) 562 */ 563 public EnrollmentResponse setOrganization(Reference value) { 564 this.organization = value; 565 return this; 566 } 567 568 /** 569 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The Insurer who produced this adjudicated response.) 570 */ 571 public Organization getOrganizationTarget() { 572 if (this.organizationTarget == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create EnrollmentResponse.organization"); 575 else if (Configuration.doAutoCreate()) 576 this.organizationTarget = new Organization(); // aa 577 return this.organizationTarget; 578 } 579 580 /** 581 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The Insurer who produced this adjudicated response.) 582 */ 583 public EnrollmentResponse setOrganizationTarget(Organization value) { 584 this.organizationTarget = value; 585 return this; 586 } 587 588 /** 589 * @return {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 590 */ 591 public Reference getRequestProvider() { 592 if (this.requestProvider == null) 593 if (Configuration.errorOnAutoCreate()) 594 throw new Error("Attempt to auto-create EnrollmentResponse.requestProvider"); 595 else if (Configuration.doAutoCreate()) 596 this.requestProvider = new Reference(); // cc 597 return this.requestProvider; 598 } 599 600 public boolean hasRequestProvider() { 601 return this.requestProvider != null && !this.requestProvider.isEmpty(); 602 } 603 604 /** 605 * @param value {@link #requestProvider} (The practitioner who is responsible for the services rendered to the patient.) 606 */ 607 public EnrollmentResponse setRequestProvider(Reference value) { 608 this.requestProvider = value; 609 return this; 610 } 611 612 /** 613 * @return {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 614 */ 615 public Practitioner getRequestProviderTarget() { 616 if (this.requestProviderTarget == null) 617 if (Configuration.errorOnAutoCreate()) 618 throw new Error("Attempt to auto-create EnrollmentResponse.requestProvider"); 619 else if (Configuration.doAutoCreate()) 620 this.requestProviderTarget = new Practitioner(); // aa 621 return this.requestProviderTarget; 622 } 623 624 /** 625 * @param value {@link #requestProvider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner who is responsible for the services rendered to the patient.) 626 */ 627 public EnrollmentResponse setRequestProviderTarget(Practitioner value) { 628 this.requestProviderTarget = value; 629 return this; 630 } 631 632 /** 633 * @return {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 634 */ 635 public Reference getRequestOrganization() { 636 if (this.requestOrganization == null) 637 if (Configuration.errorOnAutoCreate()) 638 throw new Error("Attempt to auto-create EnrollmentResponse.requestOrganization"); 639 else if (Configuration.doAutoCreate()) 640 this.requestOrganization = new Reference(); // cc 641 return this.requestOrganization; 642 } 643 644 public boolean hasRequestOrganization() { 645 return this.requestOrganization != null && !this.requestOrganization.isEmpty(); 646 } 647 648 /** 649 * @param value {@link #requestOrganization} (The organization which is responsible for the services rendered to the patient.) 650 */ 651 public EnrollmentResponse setRequestOrganization(Reference value) { 652 this.requestOrganization = value; 653 return this; 654 } 655 656 /** 657 * @return {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 658 */ 659 public Organization getRequestOrganizationTarget() { 660 if (this.requestOrganizationTarget == null) 661 if (Configuration.errorOnAutoCreate()) 662 throw new Error("Attempt to auto-create EnrollmentResponse.requestOrganization"); 663 else if (Configuration.doAutoCreate()) 664 this.requestOrganizationTarget = new Organization(); // aa 665 return this.requestOrganizationTarget; 666 } 667 668 /** 669 * @param value {@link #requestOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization which is responsible for the services rendered to the patient.) 670 */ 671 public EnrollmentResponse setRequestOrganizationTarget(Organization value) { 672 this.requestOrganizationTarget = value; 673 return this; 674 } 675 676 protected void listChildren(List<Property> children) { 677 super.listChildren(children); 678 children.add(new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 679 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 680 children.add(new Property("request", "Reference(EnrollmentRequest)", "Original request resource reference.", 0, 1, request)); 681 children.add(new Property("outcome", "CodeableConcept", "Processing status: error, complete.", 0, 1, outcome)); 682 children.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition)); 683 children.add(new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created)); 684 children.add(new Property("organization", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, organization)); 685 children.add(new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider)); 686 children.add(new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization)); 687 } 688 689 @Override 690 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 691 switch (_hash) { 692 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The Response business identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 693 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 694 case 1095692943: /*request*/ return new Property("request", "Reference(EnrollmentRequest)", "Original request resource reference.", 0, 1, request); 695 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Processing status: error, complete.", 0, 1, outcome); 696 case 583380919: /*disposition*/ return new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition); 697 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the enclosed suite of services were performed or completed.", 0, 1, created); 698 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The Insurer who produced this adjudicated response.", 0, 1, organization); 699 case 1601527200: /*requestProvider*/ return new Property("requestProvider", "Reference(Practitioner)", "The practitioner who is responsible for the services rendered to the patient.", 0, 1, requestProvider); 700 case 599053666: /*requestOrganization*/ return new Property("requestOrganization", "Reference(Organization)", "The organization which is responsible for the services rendered to the patient.", 0, 1, requestOrganization); 701 default: return super.getNamedProperty(_hash, _name, _checkValid); 702 } 703 704 } 705 706 @Override 707 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 708 switch (hash) { 709 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 710 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EnrollmentResponseStatus> 711 case 1095692943: /*request*/ return this.request == null ? new Base[0] : new Base[] {this.request}; // Reference 712 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 713 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 714 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 715 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 716 case 1601527200: /*requestProvider*/ return this.requestProvider == null ? new Base[0] : new Base[] {this.requestProvider}; // Reference 717 case 599053666: /*requestOrganization*/ return this.requestOrganization == null ? new Base[0] : new Base[] {this.requestOrganization}; // Reference 718 default: return super.getProperty(hash, name, checkValid); 719 } 720 721 } 722 723 @Override 724 public Base setProperty(int hash, String name, Base value) throws FHIRException { 725 switch (hash) { 726 case -1618432855: // identifier 727 this.getIdentifier().add(castToIdentifier(value)); // Identifier 728 return value; 729 case -892481550: // status 730 value = new EnrollmentResponseStatusEnumFactory().fromType(castToCode(value)); 731 this.status = (Enumeration) value; // Enumeration<EnrollmentResponseStatus> 732 return value; 733 case 1095692943: // request 734 this.request = castToReference(value); // Reference 735 return value; 736 case -1106507950: // outcome 737 this.outcome = castToCodeableConcept(value); // CodeableConcept 738 return value; 739 case 583380919: // disposition 740 this.disposition = castToString(value); // StringType 741 return value; 742 case 1028554472: // created 743 this.created = castToDateTime(value); // DateTimeType 744 return value; 745 case 1178922291: // organization 746 this.organization = castToReference(value); // Reference 747 return value; 748 case 1601527200: // requestProvider 749 this.requestProvider = castToReference(value); // Reference 750 return value; 751 case 599053666: // requestOrganization 752 this.requestOrganization = castToReference(value); // Reference 753 return value; 754 default: return super.setProperty(hash, name, value); 755 } 756 757 } 758 759 @Override 760 public Base setProperty(String name, Base value) throws FHIRException { 761 if (name.equals("identifier")) { 762 this.getIdentifier().add(castToIdentifier(value)); 763 } else if (name.equals("status")) { 764 value = new EnrollmentResponseStatusEnumFactory().fromType(castToCode(value)); 765 this.status = (Enumeration) value; // Enumeration<EnrollmentResponseStatus> 766 } else if (name.equals("request")) { 767 this.request = castToReference(value); // Reference 768 } else if (name.equals("outcome")) { 769 this.outcome = castToCodeableConcept(value); // CodeableConcept 770 } else if (name.equals("disposition")) { 771 this.disposition = castToString(value); // StringType 772 } else if (name.equals("created")) { 773 this.created = castToDateTime(value); // DateTimeType 774 } else if (name.equals("organization")) { 775 this.organization = castToReference(value); // Reference 776 } else if (name.equals("requestProvider")) { 777 this.requestProvider = castToReference(value); // Reference 778 } else if (name.equals("requestOrganization")) { 779 this.requestOrganization = castToReference(value); // Reference 780 } else 781 return super.setProperty(name, value); 782 return value; 783 } 784 785 @Override 786 public Base makeProperty(int hash, String name) throws FHIRException { 787 switch (hash) { 788 case -1618432855: return addIdentifier(); 789 case -892481550: return getStatusElement(); 790 case 1095692943: return getRequest(); 791 case -1106507950: return getOutcome(); 792 case 583380919: return getDispositionElement(); 793 case 1028554472: return getCreatedElement(); 794 case 1178922291: return getOrganization(); 795 case 1601527200: return getRequestProvider(); 796 case 599053666: return getRequestOrganization(); 797 default: return super.makeProperty(hash, name); 798 } 799 800 } 801 802 @Override 803 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 804 switch (hash) { 805 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 806 case -892481550: /*status*/ return new String[] {"code"}; 807 case 1095692943: /*request*/ return new String[] {"Reference"}; 808 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 809 case 583380919: /*disposition*/ return new String[] {"string"}; 810 case 1028554472: /*created*/ return new String[] {"dateTime"}; 811 case 1178922291: /*organization*/ return new String[] {"Reference"}; 812 case 1601527200: /*requestProvider*/ return new String[] {"Reference"}; 813 case 599053666: /*requestOrganization*/ return new String[] {"Reference"}; 814 default: return super.getTypesForProperty(hash, name); 815 } 816 817 } 818 819 @Override 820 public Base addChild(String name) throws FHIRException { 821 if (name.equals("identifier")) { 822 return addIdentifier(); 823 } 824 else if (name.equals("status")) { 825 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.status"); 826 } 827 else if (name.equals("request")) { 828 this.request = new Reference(); 829 return this.request; 830 } 831 else if (name.equals("outcome")) { 832 this.outcome = new CodeableConcept(); 833 return this.outcome; 834 } 835 else if (name.equals("disposition")) { 836 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.disposition"); 837 } 838 else if (name.equals("created")) { 839 throw new FHIRException("Cannot call addChild on a singleton property EnrollmentResponse.created"); 840 } 841 else if (name.equals("organization")) { 842 this.organization = new Reference(); 843 return this.organization; 844 } 845 else if (name.equals("requestProvider")) { 846 this.requestProvider = new Reference(); 847 return this.requestProvider; 848 } 849 else if (name.equals("requestOrganization")) { 850 this.requestOrganization = new Reference(); 851 return this.requestOrganization; 852 } 853 else 854 return super.addChild(name); 855 } 856 857 public String fhirType() { 858 return "EnrollmentResponse"; 859 860 } 861 862 public EnrollmentResponse copy() { 863 EnrollmentResponse dst = new EnrollmentResponse(); 864 copyValues(dst); 865 if (identifier != null) { 866 dst.identifier = new ArrayList<Identifier>(); 867 for (Identifier i : identifier) 868 dst.identifier.add(i.copy()); 869 }; 870 dst.status = status == null ? null : status.copy(); 871 dst.request = request == null ? null : request.copy(); 872 dst.outcome = outcome == null ? null : outcome.copy(); 873 dst.disposition = disposition == null ? null : disposition.copy(); 874 dst.created = created == null ? null : created.copy(); 875 dst.organization = organization == null ? null : organization.copy(); 876 dst.requestProvider = requestProvider == null ? null : requestProvider.copy(); 877 dst.requestOrganization = requestOrganization == null ? null : requestOrganization.copy(); 878 return dst; 879 } 880 881 protected EnrollmentResponse typedCopy() { 882 return copy(); 883 } 884 885 @Override 886 public boolean equalsDeep(Base other_) { 887 if (!super.equalsDeep(other_)) 888 return false; 889 if (!(other_ instanceof EnrollmentResponse)) 890 return false; 891 EnrollmentResponse o = (EnrollmentResponse) other_; 892 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(request, o.request, true) 893 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) && compareDeep(created, o.created, true) 894 && compareDeep(organization, o.organization, true) && compareDeep(requestProvider, o.requestProvider, true) 895 && compareDeep(requestOrganization, o.requestOrganization, true); 896 } 897 898 @Override 899 public boolean equalsShallow(Base other_) { 900 if (!super.equalsShallow(other_)) 901 return false; 902 if (!(other_ instanceof EnrollmentResponse)) 903 return false; 904 EnrollmentResponse o = (EnrollmentResponse) other_; 905 return compareValues(status, o.status, true) && compareValues(disposition, o.disposition, true) && compareValues(created, o.created, true) 906 ; 907 } 908 909 public boolean isEmpty() { 910 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, request 911 , outcome, disposition, created, organization, requestProvider, requestOrganization 912 ); 913 } 914 915 @Override 916 public ResourceType getResourceType() { 917 return ResourceType.EnrollmentResponse; 918 } 919 920 /** 921 * Search parameter: <b>identifier</b> 922 * <p> 923 * Description: <b>The business identifier of the EnrollmentResponse</b><br> 924 * Type: <b>token</b><br> 925 * Path: <b>EnrollmentResponse.identifier</b><br> 926 * </p> 927 */ 928 @SearchParamDefinition(name="identifier", path="EnrollmentResponse.identifier", description="The business identifier of the EnrollmentResponse", type="token" ) 929 public static final String SP_IDENTIFIER = "identifier"; 930 /** 931 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 932 * <p> 933 * Description: <b>The business identifier of the EnrollmentResponse</b><br> 934 * Type: <b>token</b><br> 935 * Path: <b>EnrollmentResponse.identifier</b><br> 936 * </p> 937 */ 938 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 939 940 /** 941 * Search parameter: <b>request</b> 942 * <p> 943 * Description: <b>The reference to the claim</b><br> 944 * Type: <b>reference</b><br> 945 * Path: <b>EnrollmentResponse.request</b><br> 946 * </p> 947 */ 948 @SearchParamDefinition(name="request", path="EnrollmentResponse.request", description="The reference to the claim", type="reference", target={EnrollmentRequest.class } ) 949 public static final String SP_REQUEST = "request"; 950 /** 951 * <b>Fluent Client</b> search parameter constant for <b>request</b> 952 * <p> 953 * Description: <b>The reference to the claim</b><br> 954 * Type: <b>reference</b><br> 955 * Path: <b>EnrollmentResponse.request</b><br> 956 * </p> 957 */ 958 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUEST = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUEST); 959 960/** 961 * Constant for fluent queries to be used to add include statements. Specifies 962 * the path value of "<b>EnrollmentResponse:request</b>". 963 */ 964 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUEST = new ca.uhn.fhir.model.api.Include("EnrollmentResponse:request").toLocked(); 965 966 /** 967 * Search parameter: <b>organization</b> 968 * <p> 969 * Description: <b>The organization who generated this resource</b><br> 970 * Type: <b>reference</b><br> 971 * Path: <b>EnrollmentResponse.organization</b><br> 972 * </p> 973 */ 974 @SearchParamDefinition(name="organization", path="EnrollmentResponse.organization", description="The organization who generated this resource", type="reference", target={Organization.class } ) 975 public static final String SP_ORGANIZATION = "organization"; 976 /** 977 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 978 * <p> 979 * Description: <b>The organization who generated this resource</b><br> 980 * Type: <b>reference</b><br> 981 * Path: <b>EnrollmentResponse.organization</b><br> 982 * </p> 983 */ 984 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 985 986/** 987 * Constant for fluent queries to be used to add include statements. Specifies 988 * the path value of "<b>EnrollmentResponse:organization</b>". 989 */ 990 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("EnrollmentResponse:organization").toLocked(); 991 992 993}