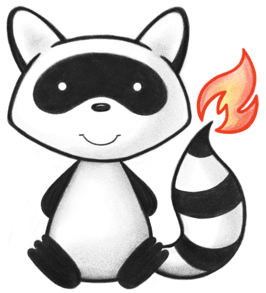
001package org.hl7.fhir.dstu3.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033import org.hl7.fhir.exceptions.FHIRException; 034 035public class Enumerations { 036 037// In here: 038// AbstractType: A type defined by FHIR that is an abstract type 039// AdministrativeGender: The gender of a person used for administrative purposes. 040// AgeUnits: A valueSet of UCUM codes for representing age value units. 041// BindingStrength: Indication of the degree of conformance expectations associated with a binding. 042// ConceptMapEquivalence: The degree of equivalence between concepts. 043// DataAbsentReason: Used to specify why the normally expected content of the data element is missing. 044// DataType: The type of an element - one of the FHIR data types. 045// DocumentReferenceStatus: The status of the document reference. 046// FHIRAllTypes: Either an abstract type, a resource or a data type. 047// FHIRDefinedType: Either a resource or a data type. 048// MessageEvent: One of the message events defined as part of FHIR. 049// NoteType: The presentation types of notes. 050// PublicationStatus: The lifecycle status of a Value Set or Concept Map. 051// RemittanceOutcome: The outcome of the processing. 052// ResourceType: One of the resource types defined as part of FHIR. 053// SearchParamType: Data types allowed to be used for search parameters. 054// SpecialValues: A set of generally useful codes defined so they can be included in value sets. 055 056 057 public enum AbstractType { 058 /** 059 * A place holder that means any kind of data type 060 */ 061 TYPE, 062 /** 063 * A place holder that means any kind of resource 064 */ 065 ANY, 066 /** 067 * added to help the parsers 068 */ 069 NULL; 070 public static AbstractType fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("Type".equals(codeString)) 074 return TYPE; 075 if ("Any".equals(codeString)) 076 return ANY; 077 throw new FHIRException("Unknown AbstractType code '"+codeString+"'"); 078 } 079 public String toCode() { 080 switch (this) { 081 case TYPE: return "Type"; 082 case ANY: return "Any"; 083 case NULL: return null; 084 default: return "?"; 085 } 086 } 087 public String getSystem() { 088 switch (this) { 089 case TYPE: return "http://hl7.org/fhir/abstract-types"; 090 case ANY: return "http://hl7.org/fhir/abstract-types"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getDefinition() { 096 switch (this) { 097 case TYPE: return "A place holder that means any kind of data type"; 098 case ANY: return "A place holder that means any kind of resource"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDisplay() { 104 switch (this) { 105 case TYPE: return "Type"; 106 case ANY: return "Any"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 } 112 113 public static class AbstractTypeEnumFactory implements EnumFactory<AbstractType> { 114 public AbstractType fromCode(String codeString) throws IllegalArgumentException { 115 if (codeString == null || "".equals(codeString)) 116 if (codeString == null || "".equals(codeString)) 117 return null; 118 if ("Type".equals(codeString)) 119 return AbstractType.TYPE; 120 if ("Any".equals(codeString)) 121 return AbstractType.ANY; 122 throw new IllegalArgumentException("Unknown AbstractType code '"+codeString+"'"); 123 } 124 public Enumeration<AbstractType> fromType(PrimitiveType<?> code) throws FHIRException { 125 if (code == null) 126 return null; 127 if (code.isEmpty()) 128 return new Enumeration<AbstractType>(this); 129 String codeString = code.asStringValue(); 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("Type".equals(codeString)) 133 return new Enumeration<AbstractType>(this, AbstractType.TYPE); 134 if ("Any".equals(codeString)) 135 return new Enumeration<AbstractType>(this, AbstractType.ANY); 136 throw new FHIRException("Unknown AbstractType code '"+codeString+"'"); 137 } 138 public String toCode(AbstractType code) { 139 if (code == AbstractType.NULL) 140 return null; 141 if (code == AbstractType.TYPE) 142 return "Type"; 143 if (code == AbstractType.ANY) 144 return "Any"; 145 return "?"; 146 } 147 public String toSystem(AbstractType code) { 148 return code.getSystem(); 149 } 150 } 151 152 public enum AdministrativeGender { 153 /** 154 * Male 155 */ 156 MALE, 157 /** 158 * Female 159 */ 160 FEMALE, 161 /** 162 * Other 163 */ 164 OTHER, 165 /** 166 * Unknown 167 */ 168 UNKNOWN, 169 /** 170 * added to help the parsers 171 */ 172 NULL; 173 public static AdministrativeGender fromCode(String codeString) throws FHIRException { 174 if (codeString == null || "".equals(codeString)) 175 return null; 176 if ("male".equals(codeString)) 177 return MALE; 178 if ("female".equals(codeString)) 179 return FEMALE; 180 if ("other".equals(codeString)) 181 return OTHER; 182 if ("unknown".equals(codeString)) 183 return UNKNOWN; 184 throw new FHIRException("Unknown AdministrativeGender code '"+codeString+"'"); 185 } 186 public String toCode() { 187 switch (this) { 188 case MALE: return "male"; 189 case FEMALE: return "female"; 190 case OTHER: return "other"; 191 case UNKNOWN: return "unknown"; 192 case NULL: return null; 193 default: return "?"; 194 } 195 } 196 public String getSystem() { 197 switch (this) { 198 case MALE: return "http://hl7.org/fhir/administrative-gender"; 199 case FEMALE: return "http://hl7.org/fhir/administrative-gender"; 200 case OTHER: return "http://hl7.org/fhir/administrative-gender"; 201 case UNKNOWN: return "http://hl7.org/fhir/administrative-gender"; 202 case NULL: return null; 203 default: return "?"; 204 } 205 } 206 public String getDefinition() { 207 switch (this) { 208 case MALE: return "Male"; 209 case FEMALE: return "Female"; 210 case OTHER: return "Other"; 211 case UNKNOWN: return "Unknown"; 212 case NULL: return null; 213 default: return "?"; 214 } 215 } 216 public String getDisplay() { 217 switch (this) { 218 case MALE: return "Male"; 219 case FEMALE: return "Female"; 220 case OTHER: return "Other"; 221 case UNKNOWN: return "Unknown"; 222 case NULL: return null; 223 default: return "?"; 224 } 225 } 226 } 227 228 public static class AdministrativeGenderEnumFactory implements EnumFactory<AdministrativeGender> { 229 public AdministrativeGender fromCode(String codeString) throws IllegalArgumentException { 230 if (codeString == null || "".equals(codeString)) 231 if (codeString == null || "".equals(codeString)) 232 return null; 233 if ("male".equals(codeString)) 234 return AdministrativeGender.MALE; 235 if ("female".equals(codeString)) 236 return AdministrativeGender.FEMALE; 237 if ("other".equals(codeString)) 238 return AdministrativeGender.OTHER; 239 if ("unknown".equals(codeString)) 240 return AdministrativeGender.UNKNOWN; 241 throw new IllegalArgumentException("Unknown AdministrativeGender code '"+codeString+"'"); 242 } 243 public Enumeration<AdministrativeGender> fromType(PrimitiveType<?> code) throws FHIRException { 244 if (code == null) 245 return null; 246 if (code.isEmpty()) 247 return new Enumeration<AdministrativeGender>(this); 248 String codeString = code.asStringValue(); 249 if (codeString == null || "".equals(codeString)) 250 return null; 251 if ("male".equals(codeString)) 252 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.MALE); 253 if ("female".equals(codeString)) 254 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.FEMALE); 255 if ("other".equals(codeString)) 256 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.OTHER); 257 if ("unknown".equals(codeString)) 258 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.UNKNOWN); 259 throw new FHIRException("Unknown AdministrativeGender code '"+codeString+"'"); 260 } 261 public String toCode(AdministrativeGender code) { 262 if (code == AdministrativeGender.NULL) 263 return null; 264 if (code == AdministrativeGender.MALE) 265 return "male"; 266 if (code == AdministrativeGender.FEMALE) 267 return "female"; 268 if (code == AdministrativeGender.OTHER) 269 return "other"; 270 if (code == AdministrativeGender.UNKNOWN) 271 return "unknown"; 272 return "?"; 273 } 274 public String toSystem(AdministrativeGender code) { 275 return code.getSystem(); 276 } 277 } 278 279 public enum AgeUnits { 280 /** 281 * null 282 */ 283 MIN, 284 /** 285 * null 286 */ 287 H, 288 /** 289 * null 290 */ 291 D, 292 /** 293 * null 294 */ 295 WK, 296 /** 297 * null 298 */ 299 MO, 300 /** 301 * null 302 */ 303 A, 304 /** 305 * added to help the parsers 306 */ 307 NULL; 308 public static AgeUnits fromCode(String codeString) throws FHIRException { 309 if (codeString == null || "".equals(codeString)) 310 return null; 311 if ("min".equals(codeString)) 312 return MIN; 313 if ("h".equals(codeString)) 314 return H; 315 if ("d".equals(codeString)) 316 return D; 317 if ("wk".equals(codeString)) 318 return WK; 319 if ("mo".equals(codeString)) 320 return MO; 321 if ("a".equals(codeString)) 322 return A; 323 throw new FHIRException("Unknown AgeUnits code '"+codeString+"'"); 324 } 325 public String toCode() { 326 switch (this) { 327 case MIN: return "min"; 328 case H: return "h"; 329 case D: return "d"; 330 case WK: return "wk"; 331 case MO: return "mo"; 332 case A: return "a"; 333 case NULL: return null; 334 default: return "?"; 335 } 336 } 337 public String getSystem() { 338 switch (this) { 339 case MIN: return "http://unitsofmeasure.org"; 340 case H: return "http://unitsofmeasure.org"; 341 case D: return "http://unitsofmeasure.org"; 342 case WK: return "http://unitsofmeasure.org"; 343 case MO: return "http://unitsofmeasure.org"; 344 case A: return "http://unitsofmeasure.org"; 345 case NULL: return null; 346 default: return "?"; 347 } 348 } 349 public String getDefinition() { 350 switch (this) { 351 case MIN: return ""; 352 case H: return ""; 353 case D: return ""; 354 case WK: return ""; 355 case MO: return ""; 356 case A: return ""; 357 case NULL: return null; 358 default: return "?"; 359 } 360 } 361 public String getDisplay() { 362 switch (this) { 363 case MIN: return "Minute"; 364 case H: return "Hour"; 365 case D: return "Day"; 366 case WK: return "Week"; 367 case MO: return "Month"; 368 case A: return "Year"; 369 case NULL: return null; 370 default: return "?"; 371 } 372 } 373 } 374 375 public static class AgeUnitsEnumFactory implements EnumFactory<AgeUnits> { 376 public AgeUnits fromCode(String codeString) throws IllegalArgumentException { 377 if (codeString == null || "".equals(codeString)) 378 if (codeString == null || "".equals(codeString)) 379 return null; 380 if ("min".equals(codeString)) 381 return AgeUnits.MIN; 382 if ("h".equals(codeString)) 383 return AgeUnits.H; 384 if ("d".equals(codeString)) 385 return AgeUnits.D; 386 if ("wk".equals(codeString)) 387 return AgeUnits.WK; 388 if ("mo".equals(codeString)) 389 return AgeUnits.MO; 390 if ("a".equals(codeString)) 391 return AgeUnits.A; 392 throw new IllegalArgumentException("Unknown AgeUnits code '"+codeString+"'"); 393 } 394 public Enumeration<AgeUnits> fromType(PrimitiveType<?> code) throws FHIRException { 395 if (code == null) 396 return null; 397 if (code.isEmpty()) 398 return new Enumeration<AgeUnits>(this); 399 String codeString = code.asStringValue(); 400 if (codeString == null || "".equals(codeString)) 401 return null; 402 if ("min".equals(codeString)) 403 return new Enumeration<AgeUnits>(this, AgeUnits.MIN); 404 if ("h".equals(codeString)) 405 return new Enumeration<AgeUnits>(this, AgeUnits.H); 406 if ("d".equals(codeString)) 407 return new Enumeration<AgeUnits>(this, AgeUnits.D); 408 if ("wk".equals(codeString)) 409 return new Enumeration<AgeUnits>(this, AgeUnits.WK); 410 if ("mo".equals(codeString)) 411 return new Enumeration<AgeUnits>(this, AgeUnits.MO); 412 if ("a".equals(codeString)) 413 return new Enumeration<AgeUnits>(this, AgeUnits.A); 414 throw new FHIRException("Unknown AgeUnits code '"+codeString+"'"); 415 } 416 public String toCode(AgeUnits code) { 417 if (code == AgeUnits.NULL) 418 return null; 419 if (code == AgeUnits.MIN) 420 return "min"; 421 if (code == AgeUnits.H) 422 return "h"; 423 if (code == AgeUnits.D) 424 return "d"; 425 if (code == AgeUnits.WK) 426 return "wk"; 427 if (code == AgeUnits.MO) 428 return "mo"; 429 if (code == AgeUnits.A) 430 return "a"; 431 return "?"; 432 } 433 public String toSystem(AgeUnits code) { 434 return code.getSystem(); 435 } 436 } 437 438 public enum BindingStrength { 439 /** 440 * To be conformant, the concept in this element SHALL be from the specified value set 441 */ 442 REQUIRED, 443 /** 444 * To be conformant, the concept in this element SHALL be from the specified value set if any of the codes within the value set can apply to the concept being communicated. If the value set does not cover the concept (based on human review), alternate codings (or, data type allowing, text) may be included instead. 445 */ 446 EXTENSIBLE, 447 /** 448 * Instances are encouraged to draw from the specified codes for interoperability purposes but are not required to do so to be considered conformant. 449 */ 450 PREFERRED, 451 /** 452 * Instances are not expected or even encouraged to draw from the specified value set. The value set merely provides examples of the types of concepts intended to be included. 453 */ 454 EXAMPLE, 455 /** 456 * added to help the parsers 457 */ 458 NULL; 459 public static BindingStrength fromCode(String codeString) throws FHIRException { 460 if (codeString == null || "".equals(codeString)) 461 return null; 462 if ("required".equals(codeString)) 463 return REQUIRED; 464 if ("extensible".equals(codeString)) 465 return EXTENSIBLE; 466 if ("preferred".equals(codeString)) 467 return PREFERRED; 468 if ("example".equals(codeString)) 469 return EXAMPLE; 470 throw new FHIRException("Unknown BindingStrength code '"+codeString+"'"); 471 } 472 public String toCode() { 473 switch (this) { 474 case REQUIRED: return "required"; 475 case EXTENSIBLE: return "extensible"; 476 case PREFERRED: return "preferred"; 477 case EXAMPLE: return "example"; 478 case NULL: return null; 479 default: return "?"; 480 } 481 } 482 public String getSystem() { 483 switch (this) { 484 case REQUIRED: return "http://hl7.org/fhir/binding-strength"; 485 case EXTENSIBLE: return "http://hl7.org/fhir/binding-strength"; 486 case PREFERRED: return "http://hl7.org/fhir/binding-strength"; 487 case EXAMPLE: return "http://hl7.org/fhir/binding-strength"; 488 case NULL: return null; 489 default: return "?"; 490 } 491 } 492 public String getDefinition() { 493 switch (this) { 494 case REQUIRED: return "To be conformant, the concept in this element SHALL be from the specified value set"; 495 case EXTENSIBLE: return "To be conformant, the concept in this element SHALL be from the specified value set if any of the codes within the value set can apply to the concept being communicated. If the value set does not cover the concept (based on human review), alternate codings (or, data type allowing, text) may be included instead."; 496 case PREFERRED: return "Instances are encouraged to draw from the specified codes for interoperability purposes but are not required to do so to be considered conformant."; 497 case EXAMPLE: return "Instances are not expected or even encouraged to draw from the specified value set. The value set merely provides examples of the types of concepts intended to be included."; 498 case NULL: return null; 499 default: return "?"; 500 } 501 } 502 public String getDisplay() { 503 switch (this) { 504 case REQUIRED: return "Required"; 505 case EXTENSIBLE: return "Extensible"; 506 case PREFERRED: return "Preferred"; 507 case EXAMPLE: return "Example"; 508 case NULL: return null; 509 default: return "?"; 510 } 511 } 512 } 513 514 public static class BindingStrengthEnumFactory implements EnumFactory<BindingStrength> { 515 public BindingStrength fromCode(String codeString) throws IllegalArgumentException { 516 if (codeString == null || "".equals(codeString)) 517 if (codeString == null || "".equals(codeString)) 518 return null; 519 if ("required".equals(codeString)) 520 return BindingStrength.REQUIRED; 521 if ("extensible".equals(codeString)) 522 return BindingStrength.EXTENSIBLE; 523 if ("preferred".equals(codeString)) 524 return BindingStrength.PREFERRED; 525 if ("example".equals(codeString)) 526 return BindingStrength.EXAMPLE; 527 throw new IllegalArgumentException("Unknown BindingStrength code '"+codeString+"'"); 528 } 529 public Enumeration<BindingStrength> fromType(PrimitiveType<?> code) throws FHIRException { 530 if (code == null) 531 return null; 532 if (code.isEmpty()) 533 return new Enumeration<BindingStrength>(this); 534 String codeString = code.asStringValue(); 535 if (codeString == null || "".equals(codeString)) 536 return null; 537 if ("required".equals(codeString)) 538 return new Enumeration<BindingStrength>(this, BindingStrength.REQUIRED); 539 if ("extensible".equals(codeString)) 540 return new Enumeration<BindingStrength>(this, BindingStrength.EXTENSIBLE); 541 if ("preferred".equals(codeString)) 542 return new Enumeration<BindingStrength>(this, BindingStrength.PREFERRED); 543 if ("example".equals(codeString)) 544 return new Enumeration<BindingStrength>(this, BindingStrength.EXAMPLE); 545 throw new FHIRException("Unknown BindingStrength code '"+codeString+"'"); 546 } 547 public String toCode(BindingStrength code) { 548 if (code == BindingStrength.NULL) 549 return null; 550 if (code == BindingStrength.REQUIRED) 551 return "required"; 552 if (code == BindingStrength.EXTENSIBLE) 553 return "extensible"; 554 if (code == BindingStrength.PREFERRED) 555 return "preferred"; 556 if (code == BindingStrength.EXAMPLE) 557 return "example"; 558 return "?"; 559 } 560 public String toSystem(BindingStrength code) { 561 return code.getSystem(); 562 } 563 } 564 565 public enum ConceptMapEquivalence { 566 /** 567 * The concepts are related to each other, and have at least some overlap in meaning, but the exact relationship is not known 568 */ 569 RELATEDTO, 570 /** 571 * The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical). 572 */ 573 EQUIVALENT, 574 /** 575 * The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical). 576 */ 577 EQUAL, 578 /** 579 * The target mapping is wider in meaning than the source concept. 580 */ 581 WIDER, 582 /** 583 * The target mapping subsumes the meaning of the source concept (e.g. the source is-a target). 584 */ 585 SUBSUMES, 586 /** 587 * The target mapping is narrower in meaning than the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally. 588 */ 589 NARROWER, 590 /** 591 * The target mapping specializes the meaning of the source concept (e.g. the target is-a source). 592 */ 593 SPECIALIZES, 594 /** 595 * The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally. 596 */ 597 INEXACT, 598 /** 599 * There is no match for this concept in the destination concept system. 600 */ 601 UNMATCHED, 602 /** 603 * This is an explicit assertion that there is no mapping between the source and target concept. 604 */ 605 DISJOINT, 606 /** 607 * added to help the parsers 608 */ 609 NULL; 610 public static ConceptMapEquivalence fromCode(String codeString) throws FHIRException { 611 if (codeString == null || "".equals(codeString)) 612 return null; 613 if ("relatedto".equals(codeString)) 614 return RELATEDTO; 615 if ("equivalent".equals(codeString)) 616 return EQUIVALENT; 617 if ("equal".equals(codeString)) 618 return EQUAL; 619 if ("wider".equals(codeString)) 620 return WIDER; 621 if ("subsumes".equals(codeString)) 622 return SUBSUMES; 623 if ("narrower".equals(codeString)) 624 return NARROWER; 625 if ("specializes".equals(codeString)) 626 return SPECIALIZES; 627 if ("inexact".equals(codeString)) 628 return INEXACT; 629 if ("unmatched".equals(codeString)) 630 return UNMATCHED; 631 if ("disjoint".equals(codeString)) 632 return DISJOINT; 633 throw new FHIRException("Unknown ConceptMapEquivalence code '"+codeString+"'"); 634 } 635 public String toCode() { 636 switch (this) { 637 case RELATEDTO: return "relatedto"; 638 case EQUIVALENT: return "equivalent"; 639 case EQUAL: return "equal"; 640 case WIDER: return "wider"; 641 case SUBSUMES: return "subsumes"; 642 case NARROWER: return "narrower"; 643 case SPECIALIZES: return "specializes"; 644 case INEXACT: return "inexact"; 645 case UNMATCHED: return "unmatched"; 646 case DISJOINT: return "disjoint"; 647 case NULL: return null; 648 default: return "?"; 649 } 650 } 651 public String getSystem() { 652 switch (this) { 653 case RELATEDTO: return "http://hl7.org/fhir/concept-map-equivalence"; 654 case EQUIVALENT: return "http://hl7.org/fhir/concept-map-equivalence"; 655 case EQUAL: return "http://hl7.org/fhir/concept-map-equivalence"; 656 case WIDER: return "http://hl7.org/fhir/concept-map-equivalence"; 657 case SUBSUMES: return "http://hl7.org/fhir/concept-map-equivalence"; 658 case NARROWER: return "http://hl7.org/fhir/concept-map-equivalence"; 659 case SPECIALIZES: return "http://hl7.org/fhir/concept-map-equivalence"; 660 case INEXACT: return "http://hl7.org/fhir/concept-map-equivalence"; 661 case UNMATCHED: return "http://hl7.org/fhir/concept-map-equivalence"; 662 case DISJOINT: return "http://hl7.org/fhir/concept-map-equivalence"; 663 case NULL: return null; 664 default: return "?"; 665 } 666 } 667 public String getDefinition() { 668 switch (this) { 669 case RELATEDTO: return "The concepts are related to each other, and have at least some overlap in meaning, but the exact relationship is not known"; 670 case EQUIVALENT: return "The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical)."; 671 case EQUAL: return "The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical)."; 672 case WIDER: return "The target mapping is wider in meaning than the source concept."; 673 case SUBSUMES: return "The target mapping subsumes the meaning of the source concept (e.g. the source is-a target)."; 674 case NARROWER: return "The target mapping is narrower in meaning than the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 675 case SPECIALIZES: return "The target mapping specializes the meaning of the source concept (e.g. the target is-a source)."; 676 case INEXACT: return "The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 677 case UNMATCHED: return "There is no match for this concept in the destination concept system."; 678 case DISJOINT: return "This is an explicit assertion that there is no mapping between the source and target concept."; 679 case NULL: return null; 680 default: return "?"; 681 } 682 } 683 public String getDisplay() { 684 switch (this) { 685 case RELATEDTO: return "Related To"; 686 case EQUIVALENT: return "Equivalent"; 687 case EQUAL: return "Equal"; 688 case WIDER: return "Wider"; 689 case SUBSUMES: return "Subsumes"; 690 case NARROWER: return "Narrower"; 691 case SPECIALIZES: return "Specializes"; 692 case INEXACT: return "Inexact"; 693 case UNMATCHED: return "Unmatched"; 694 case DISJOINT: return "Disjoint"; 695 case NULL: return null; 696 default: return "?"; 697 } 698 } 699 } 700 701 public static class ConceptMapEquivalenceEnumFactory implements EnumFactory<ConceptMapEquivalence> { 702 public ConceptMapEquivalence fromCode(String codeString) throws IllegalArgumentException { 703 if (codeString == null || "".equals(codeString)) 704 if (codeString == null || "".equals(codeString)) 705 return null; 706 if ("relatedto".equals(codeString)) 707 return ConceptMapEquivalence.RELATEDTO; 708 if ("equivalent".equals(codeString)) 709 return ConceptMapEquivalence.EQUIVALENT; 710 if ("equal".equals(codeString)) 711 return ConceptMapEquivalence.EQUAL; 712 if ("wider".equals(codeString)) 713 return ConceptMapEquivalence.WIDER; 714 if ("subsumes".equals(codeString)) 715 return ConceptMapEquivalence.SUBSUMES; 716 if ("narrower".equals(codeString)) 717 return ConceptMapEquivalence.NARROWER; 718 if ("specializes".equals(codeString)) 719 return ConceptMapEquivalence.SPECIALIZES; 720 if ("inexact".equals(codeString)) 721 return ConceptMapEquivalence.INEXACT; 722 if ("unmatched".equals(codeString)) 723 return ConceptMapEquivalence.UNMATCHED; 724 if ("disjoint".equals(codeString)) 725 return ConceptMapEquivalence.DISJOINT; 726 throw new IllegalArgumentException("Unknown ConceptMapEquivalence code '"+codeString+"'"); 727 } 728 public Enumeration<ConceptMapEquivalence> fromType(PrimitiveType<?> code) throws FHIRException { 729 if (code == null) 730 return null; 731 if (code.isEmpty()) 732 return new Enumeration<ConceptMapEquivalence>(this); 733 String codeString = code.asStringValue(); 734 if (codeString == null || "".equals(codeString)) 735 return null; 736 if ("relatedto".equals(codeString)) 737 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.RELATEDTO); 738 if ("equivalent".equals(codeString)) 739 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUIVALENT); 740 if ("equal".equals(codeString)) 741 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUAL); 742 if ("wider".equals(codeString)) 743 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.WIDER); 744 if ("subsumes".equals(codeString)) 745 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SUBSUMES); 746 if ("narrower".equals(codeString)) 747 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NARROWER); 748 if ("specializes".equals(codeString)) 749 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SPECIALIZES); 750 if ("inexact".equals(codeString)) 751 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.INEXACT); 752 if ("unmatched".equals(codeString)) 753 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.UNMATCHED); 754 if ("disjoint".equals(codeString)) 755 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.DISJOINT); 756 throw new FHIRException("Unknown ConceptMapEquivalence code '"+codeString+"'"); 757 } 758 public String toCode(ConceptMapEquivalence code) { 759 if (code == ConceptMapEquivalence.NULL) 760 return null; 761 if (code == ConceptMapEquivalence.RELATEDTO) 762 return "relatedto"; 763 if (code == ConceptMapEquivalence.EQUIVALENT) 764 return "equivalent"; 765 if (code == ConceptMapEquivalence.EQUAL) 766 return "equal"; 767 if (code == ConceptMapEquivalence.WIDER) 768 return "wider"; 769 if (code == ConceptMapEquivalence.SUBSUMES) 770 return "subsumes"; 771 if (code == ConceptMapEquivalence.NARROWER) 772 return "narrower"; 773 if (code == ConceptMapEquivalence.SPECIALIZES) 774 return "specializes"; 775 if (code == ConceptMapEquivalence.INEXACT) 776 return "inexact"; 777 if (code == ConceptMapEquivalence.UNMATCHED) 778 return "unmatched"; 779 if (code == ConceptMapEquivalence.DISJOINT) 780 return "disjoint"; 781 return "?"; 782 } 783 public String toSystem(ConceptMapEquivalence code) { 784 return code.getSystem(); 785 } 786 } 787 788 public enum DataAbsentReason { 789 /** 790 * The value is not known. 791 */ 792 UNKNOWN, 793 /** 794 * The source human does not know the value. 795 */ 796 ASKED, 797 /** 798 * There is reason to expect (from the workflow) that the value may become known. 799 */ 800 TEMP, 801 /** 802 * The workflow didn't lead to this value being known. 803 */ 804 NOTASKED, 805 /** 806 * The information is not available due to security, privacy or related reasons. 807 */ 808 MASKED, 809 /** 810 * The source system wasn't capable of supporting this element. 811 */ 812 UNSUPPORTED, 813 /** 814 * The content of the data is represented in the resource narrative. 815 */ 816 ASTEXT, 817 /** 818 * Some system or workflow process error means that the information is not available. 819 */ 820 ERROR, 821 /** 822 * NaN, standing for not a number, is a numeric data type value representing an undefined or unrepresentable value. 823 */ 824 NAN, 825 /** 826 * The value is not available because the observation procedure (test, etc.) was not performed. 827 */ 828 NOTPERFORMED, 829 /** 830 * added to help the parsers 831 */ 832 NULL; 833 public static DataAbsentReason fromCode(String codeString) throws FHIRException { 834 if (codeString == null || "".equals(codeString)) 835 return null; 836 if ("unknown".equals(codeString)) 837 return UNKNOWN; 838 if ("asked".equals(codeString)) 839 return ASKED; 840 if ("temp".equals(codeString)) 841 return TEMP; 842 if ("not-asked".equals(codeString)) 843 return NOTASKED; 844 if ("masked".equals(codeString)) 845 return MASKED; 846 if ("unsupported".equals(codeString)) 847 return UNSUPPORTED; 848 if ("astext".equals(codeString)) 849 return ASTEXT; 850 if ("error".equals(codeString)) 851 return ERROR; 852 if ("NaN".equals(codeString)) 853 return NAN; 854 if ("not-performed".equals(codeString)) 855 return NOTPERFORMED; 856 throw new FHIRException("Unknown DataAbsentReason code '"+codeString+"'"); 857 } 858 public String toCode() { 859 switch (this) { 860 case UNKNOWN: return "unknown"; 861 case ASKED: return "asked"; 862 case TEMP: return "temp"; 863 case NOTASKED: return "not-asked"; 864 case MASKED: return "masked"; 865 case UNSUPPORTED: return "unsupported"; 866 case ASTEXT: return "astext"; 867 case ERROR: return "error"; 868 case NAN: return "NaN"; 869 case NOTPERFORMED: return "not-performed"; 870 case NULL: return null; 871 default: return "?"; 872 } 873 } 874 public String getSystem() { 875 switch (this) { 876 case UNKNOWN: return "http://hl7.org/fhir/data-absent-reason"; 877 case ASKED: return "http://hl7.org/fhir/data-absent-reason"; 878 case TEMP: return "http://hl7.org/fhir/data-absent-reason"; 879 case NOTASKED: return "http://hl7.org/fhir/data-absent-reason"; 880 case MASKED: return "http://hl7.org/fhir/data-absent-reason"; 881 case UNSUPPORTED: return "http://hl7.org/fhir/data-absent-reason"; 882 case ASTEXT: return "http://hl7.org/fhir/data-absent-reason"; 883 case ERROR: return "http://hl7.org/fhir/data-absent-reason"; 884 case NAN: return "http://hl7.org/fhir/data-absent-reason"; 885 case NOTPERFORMED: return "http://hl7.org/fhir/data-absent-reason"; 886 case NULL: return null; 887 default: return "?"; 888 } 889 } 890 public String getDefinition() { 891 switch (this) { 892 case UNKNOWN: return "The value is not known."; 893 case ASKED: return "The source human does not know the value."; 894 case TEMP: return "There is reason to expect (from the workflow) that the value may become known."; 895 case NOTASKED: return "The workflow didn't lead to this value being known."; 896 case MASKED: return "The information is not available due to security, privacy or related reasons."; 897 case UNSUPPORTED: return "The source system wasn't capable of supporting this element."; 898 case ASTEXT: return "The content of the data is represented in the resource narrative."; 899 case ERROR: return "Some system or workflow process error means that the information is not available."; 900 case NAN: return "NaN, standing for not a number, is a numeric data type value representing an undefined or unrepresentable value."; 901 case NOTPERFORMED: return "The value is not available because the observation procedure (test, etc.) was not performed."; 902 case NULL: return null; 903 default: return "?"; 904 } 905 } 906 public String getDisplay() { 907 switch (this) { 908 case UNKNOWN: return "Unknown"; 909 case ASKED: return "Asked"; 910 case TEMP: return "Temp"; 911 case NOTASKED: return "Not Asked"; 912 case MASKED: return "Masked"; 913 case UNSUPPORTED: return "Unsupported"; 914 case ASTEXT: return "As Text"; 915 case ERROR: return "Error"; 916 case NAN: return "Not a Number"; 917 case NOTPERFORMED: return "Not Performed"; 918 case NULL: return null; 919 default: return "?"; 920 } 921 } 922 } 923 924 public static class DataAbsentReasonEnumFactory implements EnumFactory<DataAbsentReason> { 925 public DataAbsentReason fromCode(String codeString) throws IllegalArgumentException { 926 if (codeString == null || "".equals(codeString)) 927 if (codeString == null || "".equals(codeString)) 928 return null; 929 if ("unknown".equals(codeString)) 930 return DataAbsentReason.UNKNOWN; 931 if ("asked".equals(codeString)) 932 return DataAbsentReason.ASKED; 933 if ("temp".equals(codeString)) 934 return DataAbsentReason.TEMP; 935 if ("not-asked".equals(codeString)) 936 return DataAbsentReason.NOTASKED; 937 if ("masked".equals(codeString)) 938 return DataAbsentReason.MASKED; 939 if ("unsupported".equals(codeString)) 940 return DataAbsentReason.UNSUPPORTED; 941 if ("astext".equals(codeString)) 942 return DataAbsentReason.ASTEXT; 943 if ("error".equals(codeString)) 944 return DataAbsentReason.ERROR; 945 if ("NaN".equals(codeString)) 946 return DataAbsentReason.NAN; 947 if ("not-performed".equals(codeString)) 948 return DataAbsentReason.NOTPERFORMED; 949 throw new IllegalArgumentException("Unknown DataAbsentReason code '"+codeString+"'"); 950 } 951 public Enumeration<DataAbsentReason> fromType(PrimitiveType<?> code) throws FHIRException { 952 if (code == null) 953 return null; 954 if (code.isEmpty()) 955 return new Enumeration<DataAbsentReason>(this); 956 String codeString = code.asStringValue(); 957 if (codeString == null || "".equals(codeString)) 958 return null; 959 if ("unknown".equals(codeString)) 960 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNKNOWN); 961 if ("asked".equals(codeString)) 962 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASKED); 963 if ("temp".equals(codeString)) 964 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.TEMP); 965 if ("not-asked".equals(codeString)) 966 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTASKED); 967 if ("masked".equals(codeString)) 968 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.MASKED); 969 if ("unsupported".equals(codeString)) 970 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNSUPPORTED); 971 if ("astext".equals(codeString)) 972 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASTEXT); 973 if ("error".equals(codeString)) 974 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ERROR); 975 if ("NaN".equals(codeString)) 976 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NAN); 977 if ("not-performed".equals(codeString)) 978 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTPERFORMED); 979 throw new FHIRException("Unknown DataAbsentReason code '"+codeString+"'"); 980 } 981 public String toCode(DataAbsentReason code) { 982 if (code == DataAbsentReason.NULL) 983 return null; 984 if (code == DataAbsentReason.UNKNOWN) 985 return "unknown"; 986 if (code == DataAbsentReason.ASKED) 987 return "asked"; 988 if (code == DataAbsentReason.TEMP) 989 return "temp"; 990 if (code == DataAbsentReason.NOTASKED) 991 return "not-asked"; 992 if (code == DataAbsentReason.MASKED) 993 return "masked"; 994 if (code == DataAbsentReason.UNSUPPORTED) 995 return "unsupported"; 996 if (code == DataAbsentReason.ASTEXT) 997 return "astext"; 998 if (code == DataAbsentReason.ERROR) 999 return "error"; 1000 if (code == DataAbsentReason.NAN) 1001 return "NaN"; 1002 if (code == DataAbsentReason.NOTPERFORMED) 1003 return "not-performed"; 1004 return "?"; 1005 } 1006 public String toSystem(DataAbsentReason code) { 1007 return code.getSystem(); 1008 } 1009 } 1010 1011 public enum DataType { 1012 /** 1013 * An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world. 1014 */ 1015 ADDRESS, 1016 /** 1017 * A duration of time during which an organism (or a process) has existed. 1018 */ 1019 AGE, 1020 /** 1021 * A text note which also contains information about who made the statement and when. 1022 */ 1023 ANNOTATION, 1024 /** 1025 * For referring to data content defined in other formats. 1026 */ 1027 ATTACHMENT, 1028 /** 1029 * Base definition for all elements that are defined inside a resource - but not those in a data type. 1030 */ 1031 BACKBONEELEMENT, 1032 /** 1033 * A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text. 1034 */ 1035 CODEABLECONCEPT, 1036 /** 1037 * A reference to a code defined by a terminology system. 1038 */ 1039 CODING, 1040 /** 1041 * Specifies contact information for a person or organization. 1042 */ 1043 CONTACTDETAIL, 1044 /** 1045 * Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 1046 */ 1047 CONTACTPOINT, 1048 /** 1049 * A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers. 1050 */ 1051 CONTRIBUTOR, 1052 /** 1053 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 1054 */ 1055 COUNT, 1056 /** 1057 * Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data. 1058 */ 1059 DATAREQUIREMENT, 1060 /** 1061 * A length - a value with a unit that is a physical distance. 1062 */ 1063 DISTANCE, 1064 /** 1065 * Indicates how the medication is/was taken or should be taken by the patient. 1066 */ 1067 DOSAGE, 1068 /** 1069 * A length of time. 1070 */ 1071 DURATION, 1072 /** 1073 * Base definition for all elements in a resource. 1074 */ 1075 ELEMENT, 1076 /** 1077 * Captures constraints on each element within the resource, profile, or extension. 1078 */ 1079 ELEMENTDEFINITION, 1080 /** 1081 * Optional Extension Element - found in all resources. 1082 */ 1083 EXTENSION, 1084 /** 1085 * A human's name with the ability to identify parts and usage. 1086 */ 1087 HUMANNAME, 1088 /** 1089 * A technical identifier - identifies some entity uniquely and unambiguously. 1090 */ 1091 IDENTIFIER, 1092 /** 1093 * The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource. 1094 */ 1095 META, 1096 /** 1097 * An amount of economic utility in some recognized currency. 1098 */ 1099 MONEY, 1100 /** 1101 * A human-readable formatted text, including images. 1102 */ 1103 NARRATIVE, 1104 /** 1105 * The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse. 1106 */ 1107 PARAMETERDEFINITION, 1108 /** 1109 * A time period defined by a start and end date and optionally time. 1110 */ 1111 PERIOD, 1112 /** 1113 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 1114 */ 1115 QUANTITY, 1116 /** 1117 * A set of ordered Quantities defined by a low and high limit. 1118 */ 1119 RANGE, 1120 /** 1121 * A relationship of two Quantity values - expressed as a numerator and a denominator. 1122 */ 1123 RATIO, 1124 /** 1125 * A reference from one resource to another. 1126 */ 1127 REFERENCE, 1128 /** 1129 * Related artifacts such as additional documentation, justification, or bibliographic references. 1130 */ 1131 RELATEDARTIFACT, 1132 /** 1133 * A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data. 1134 */ 1135 SAMPLEDDATA, 1136 /** 1137 * A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities. 1138 */ 1139 SIGNATURE, 1140 /** 1141 * null 1142 */ 1143 SIMPLEQUANTITY, 1144 /** 1145 * Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out. 1146 */ 1147 TIMING, 1148 /** 1149 * A description of a triggering event. 1150 */ 1151 TRIGGERDEFINITION, 1152 /** 1153 * Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care). 1154 */ 1155 USAGECONTEXT, 1156 /** 1157 * A stream of bytes 1158 */ 1159 BASE64BINARY, 1160 /** 1161 * Value of "true" or "false" 1162 */ 1163 BOOLEAN, 1164 /** 1165 * A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents 1166 */ 1167 CODE, 1168 /** 1169 * A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates. 1170 */ 1171 DATE, 1172 /** 1173 * A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates. 1174 */ 1175 DATETIME, 1176 /** 1177 * A rational number with implicit precision 1178 */ 1179 DECIMAL, 1180 /** 1181 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive. 1182 */ 1183 ID, 1184 /** 1185 * An instant in time - known at least to the second 1186 */ 1187 INSTANT, 1188 /** 1189 * A whole number 1190 */ 1191 INTEGER, 1192 /** 1193 * A string that may contain markdown syntax for optional processing by a mark down presentation engine 1194 */ 1195 MARKDOWN, 1196 /** 1197 * An OID represented as a URI 1198 */ 1199 OID, 1200 /** 1201 * An integer with a value that is positive (e.g. >0) 1202 */ 1203 POSITIVEINT, 1204 /** 1205 * A sequence of Unicode characters 1206 */ 1207 STRING, 1208 /** 1209 * A time during the day, with no date specified 1210 */ 1211 TIME, 1212 /** 1213 * An integer with a value that is not negative (e.g. >= 0) 1214 */ 1215 UNSIGNEDINT, 1216 /** 1217 * String of characters used to identify a name or a resource 1218 */ 1219 URI, 1220 /** 1221 * A UUID, represented as a URI 1222 */ 1223 UUID, 1224 /** 1225 * XHTML format, as defined by W3C, but restricted usage (mainly, no active content) 1226 */ 1227 XHTML, 1228 /** 1229 * added to help the parsers 1230 */ 1231 NULL; 1232 public static DataType fromCode(String codeString) throws FHIRException { 1233 if (codeString == null || "".equals(codeString)) 1234 return null; 1235 if ("Address".equals(codeString)) 1236 return ADDRESS; 1237 if ("Age".equals(codeString)) 1238 return AGE; 1239 if ("Annotation".equals(codeString)) 1240 return ANNOTATION; 1241 if ("Attachment".equals(codeString)) 1242 return ATTACHMENT; 1243 if ("BackboneElement".equals(codeString)) 1244 return BACKBONEELEMENT; 1245 if ("CodeableConcept".equals(codeString)) 1246 return CODEABLECONCEPT; 1247 if ("Coding".equals(codeString)) 1248 return CODING; 1249 if ("ContactDetail".equals(codeString)) 1250 return CONTACTDETAIL; 1251 if ("ContactPoint".equals(codeString)) 1252 return CONTACTPOINT; 1253 if ("Contributor".equals(codeString)) 1254 return CONTRIBUTOR; 1255 if ("Count".equals(codeString)) 1256 return COUNT; 1257 if ("DataRequirement".equals(codeString)) 1258 return DATAREQUIREMENT; 1259 if ("Distance".equals(codeString)) 1260 return DISTANCE; 1261 if ("Dosage".equals(codeString)) 1262 return DOSAGE; 1263 if ("Duration".equals(codeString)) 1264 return DURATION; 1265 if ("Element".equals(codeString)) 1266 return ELEMENT; 1267 if ("ElementDefinition".equals(codeString)) 1268 return ELEMENTDEFINITION; 1269 if ("Extension".equals(codeString)) 1270 return EXTENSION; 1271 if ("HumanName".equals(codeString)) 1272 return HUMANNAME; 1273 if ("Identifier".equals(codeString)) 1274 return IDENTIFIER; 1275 if ("Meta".equals(codeString)) 1276 return META; 1277 if ("Money".equals(codeString)) 1278 return MONEY; 1279 if ("Narrative".equals(codeString)) 1280 return NARRATIVE; 1281 if ("ParameterDefinition".equals(codeString)) 1282 return PARAMETERDEFINITION; 1283 if ("Period".equals(codeString)) 1284 return PERIOD; 1285 if ("Quantity".equals(codeString)) 1286 return QUANTITY; 1287 if ("Range".equals(codeString)) 1288 return RANGE; 1289 if ("Ratio".equals(codeString)) 1290 return RATIO; 1291 if ("Reference".equals(codeString)) 1292 return REFERENCE; 1293 if ("RelatedArtifact".equals(codeString)) 1294 return RELATEDARTIFACT; 1295 if ("SampledData".equals(codeString)) 1296 return SAMPLEDDATA; 1297 if ("Signature".equals(codeString)) 1298 return SIGNATURE; 1299 if ("SimpleQuantity".equals(codeString)) 1300 return SIMPLEQUANTITY; 1301 if ("Timing".equals(codeString)) 1302 return TIMING; 1303 if ("TriggerDefinition".equals(codeString)) 1304 return TRIGGERDEFINITION; 1305 if ("UsageContext".equals(codeString)) 1306 return USAGECONTEXT; 1307 if ("base64Binary".equals(codeString)) 1308 return BASE64BINARY; 1309 if ("boolean".equals(codeString)) 1310 return BOOLEAN; 1311 if ("code".equals(codeString)) 1312 return CODE; 1313 if ("date".equals(codeString)) 1314 return DATE; 1315 if ("dateTime".equals(codeString)) 1316 return DATETIME; 1317 if ("decimal".equals(codeString)) 1318 return DECIMAL; 1319 if ("id".equals(codeString)) 1320 return ID; 1321 if ("instant".equals(codeString)) 1322 return INSTANT; 1323 if ("integer".equals(codeString)) 1324 return INTEGER; 1325 if ("markdown".equals(codeString)) 1326 return MARKDOWN; 1327 if ("oid".equals(codeString)) 1328 return OID; 1329 if ("positiveInt".equals(codeString)) 1330 return POSITIVEINT; 1331 if ("string".equals(codeString)) 1332 return STRING; 1333 if ("time".equals(codeString)) 1334 return TIME; 1335 if ("unsignedInt".equals(codeString)) 1336 return UNSIGNEDINT; 1337 if ("uri".equals(codeString)) 1338 return URI; 1339 if ("uuid".equals(codeString)) 1340 return UUID; 1341 if ("xhtml".equals(codeString)) 1342 return XHTML; 1343 throw new FHIRException("Unknown DataType code '"+codeString+"'"); 1344 } 1345 public String toCode() { 1346 switch (this) { 1347 case ADDRESS: return "Address"; 1348 case AGE: return "Age"; 1349 case ANNOTATION: return "Annotation"; 1350 case ATTACHMENT: return "Attachment"; 1351 case BACKBONEELEMENT: return "BackboneElement"; 1352 case CODEABLECONCEPT: return "CodeableConcept"; 1353 case CODING: return "Coding"; 1354 case CONTACTDETAIL: return "ContactDetail"; 1355 case CONTACTPOINT: return "ContactPoint"; 1356 case CONTRIBUTOR: return "Contributor"; 1357 case COUNT: return "Count"; 1358 case DATAREQUIREMENT: return "DataRequirement"; 1359 case DISTANCE: return "Distance"; 1360 case DOSAGE: return "Dosage"; 1361 case DURATION: return "Duration"; 1362 case ELEMENT: return "Element"; 1363 case ELEMENTDEFINITION: return "ElementDefinition"; 1364 case EXTENSION: return "Extension"; 1365 case HUMANNAME: return "HumanName"; 1366 case IDENTIFIER: return "Identifier"; 1367 case META: return "Meta"; 1368 case MONEY: return "Money"; 1369 case NARRATIVE: return "Narrative"; 1370 case PARAMETERDEFINITION: return "ParameterDefinition"; 1371 case PERIOD: return "Period"; 1372 case QUANTITY: return "Quantity"; 1373 case RANGE: return "Range"; 1374 case RATIO: return "Ratio"; 1375 case REFERENCE: return "Reference"; 1376 case RELATEDARTIFACT: return "RelatedArtifact"; 1377 case SAMPLEDDATA: return "SampledData"; 1378 case SIGNATURE: return "Signature"; 1379 case SIMPLEQUANTITY: return "SimpleQuantity"; 1380 case TIMING: return "Timing"; 1381 case TRIGGERDEFINITION: return "TriggerDefinition"; 1382 case USAGECONTEXT: return "UsageContext"; 1383 case BASE64BINARY: return "base64Binary"; 1384 case BOOLEAN: return "boolean"; 1385 case CODE: return "code"; 1386 case DATE: return "date"; 1387 case DATETIME: return "dateTime"; 1388 case DECIMAL: return "decimal"; 1389 case ID: return "id"; 1390 case INSTANT: return "instant"; 1391 case INTEGER: return "integer"; 1392 case MARKDOWN: return "markdown"; 1393 case OID: return "oid"; 1394 case POSITIVEINT: return "positiveInt"; 1395 case STRING: return "string"; 1396 case TIME: return "time"; 1397 case UNSIGNEDINT: return "unsignedInt"; 1398 case URI: return "uri"; 1399 case UUID: return "uuid"; 1400 case XHTML: return "xhtml"; 1401 case NULL: return null; 1402 default: return "?"; 1403 } 1404 } 1405 public String getSystem() { 1406 switch (this) { 1407 case ADDRESS: return "http://hl7.org/fhir/data-types"; 1408 case AGE: return "http://hl7.org/fhir/data-types"; 1409 case ANNOTATION: return "http://hl7.org/fhir/data-types"; 1410 case ATTACHMENT: return "http://hl7.org/fhir/data-types"; 1411 case BACKBONEELEMENT: return "http://hl7.org/fhir/data-types"; 1412 case CODEABLECONCEPT: return "http://hl7.org/fhir/data-types"; 1413 case CODING: return "http://hl7.org/fhir/data-types"; 1414 case CONTACTDETAIL: return "http://hl7.org/fhir/data-types"; 1415 case CONTACTPOINT: return "http://hl7.org/fhir/data-types"; 1416 case CONTRIBUTOR: return "http://hl7.org/fhir/data-types"; 1417 case COUNT: return "http://hl7.org/fhir/data-types"; 1418 case DATAREQUIREMENT: return "http://hl7.org/fhir/data-types"; 1419 case DISTANCE: return "http://hl7.org/fhir/data-types"; 1420 case DOSAGE: return "http://hl7.org/fhir/data-types"; 1421 case DURATION: return "http://hl7.org/fhir/data-types"; 1422 case ELEMENT: return "http://hl7.org/fhir/data-types"; 1423 case ELEMENTDEFINITION: return "http://hl7.org/fhir/data-types"; 1424 case EXTENSION: return "http://hl7.org/fhir/data-types"; 1425 case HUMANNAME: return "http://hl7.org/fhir/data-types"; 1426 case IDENTIFIER: return "http://hl7.org/fhir/data-types"; 1427 case META: return "http://hl7.org/fhir/data-types"; 1428 case MONEY: return "http://hl7.org/fhir/data-types"; 1429 case NARRATIVE: return "http://hl7.org/fhir/data-types"; 1430 case PARAMETERDEFINITION: return "http://hl7.org/fhir/data-types"; 1431 case PERIOD: return "http://hl7.org/fhir/data-types"; 1432 case QUANTITY: return "http://hl7.org/fhir/data-types"; 1433 case RANGE: return "http://hl7.org/fhir/data-types"; 1434 case RATIO: return "http://hl7.org/fhir/data-types"; 1435 case REFERENCE: return "http://hl7.org/fhir/data-types"; 1436 case RELATEDARTIFACT: return "http://hl7.org/fhir/data-types"; 1437 case SAMPLEDDATA: return "http://hl7.org/fhir/data-types"; 1438 case SIGNATURE: return "http://hl7.org/fhir/data-types"; 1439 case SIMPLEQUANTITY: return "http://hl7.org/fhir/data-types"; 1440 case TIMING: return "http://hl7.org/fhir/data-types"; 1441 case TRIGGERDEFINITION: return "http://hl7.org/fhir/data-types"; 1442 case USAGECONTEXT: return "http://hl7.org/fhir/data-types"; 1443 case BASE64BINARY: return "http://hl7.org/fhir/data-types"; 1444 case BOOLEAN: return "http://hl7.org/fhir/data-types"; 1445 case CODE: return "http://hl7.org/fhir/data-types"; 1446 case DATE: return "http://hl7.org/fhir/data-types"; 1447 case DATETIME: return "http://hl7.org/fhir/data-types"; 1448 case DECIMAL: return "http://hl7.org/fhir/data-types"; 1449 case ID: return "http://hl7.org/fhir/data-types"; 1450 case INSTANT: return "http://hl7.org/fhir/data-types"; 1451 case INTEGER: return "http://hl7.org/fhir/data-types"; 1452 case MARKDOWN: return "http://hl7.org/fhir/data-types"; 1453 case OID: return "http://hl7.org/fhir/data-types"; 1454 case POSITIVEINT: return "http://hl7.org/fhir/data-types"; 1455 case STRING: return "http://hl7.org/fhir/data-types"; 1456 case TIME: return "http://hl7.org/fhir/data-types"; 1457 case UNSIGNEDINT: return "http://hl7.org/fhir/data-types"; 1458 case URI: return "http://hl7.org/fhir/data-types"; 1459 case UUID: return "http://hl7.org/fhir/data-types"; 1460 case XHTML: return "http://hl7.org/fhir/data-types"; 1461 case NULL: return null; 1462 default: return "?"; 1463 } 1464 } 1465 public String getDefinition() { 1466 switch (this) { 1467 case ADDRESS: return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 1468 case AGE: return "A duration of time during which an organism (or a process) has existed."; 1469 case ANNOTATION: return "A text note which also contains information about who made the statement and when."; 1470 case ATTACHMENT: return "For referring to data content defined in other formats."; 1471 case BACKBONEELEMENT: return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 1472 case CODEABLECONCEPT: return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 1473 case CODING: return "A reference to a code defined by a terminology system."; 1474 case CONTACTDETAIL: return "Specifies contact information for a person or organization."; 1475 case CONTACTPOINT: return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 1476 case CONTRIBUTOR: return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 1477 case COUNT: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 1478 case DATAREQUIREMENT: return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 1479 case DISTANCE: return "A length - a value with a unit that is a physical distance."; 1480 case DOSAGE: return "Indicates how the medication is/was taken or should be taken by the patient."; 1481 case DURATION: return "A length of time."; 1482 case ELEMENT: return "Base definition for all elements in a resource."; 1483 case ELEMENTDEFINITION: return "Captures constraints on each element within the resource, profile, or extension."; 1484 case EXTENSION: return "Optional Extension Element - found in all resources."; 1485 case HUMANNAME: return "A human's name with the ability to identify parts and usage."; 1486 case IDENTIFIER: return "A technical identifier - identifies some entity uniquely and unambiguously."; 1487 case META: return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource."; 1488 case MONEY: return "An amount of economic utility in some recognized currency."; 1489 case NARRATIVE: return "A human-readable formatted text, including images."; 1490 case PARAMETERDEFINITION: return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 1491 case PERIOD: return "A time period defined by a start and end date and optionally time."; 1492 case QUANTITY: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 1493 case RANGE: return "A set of ordered Quantities defined by a low and high limit."; 1494 case RATIO: return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 1495 case REFERENCE: return "A reference from one resource to another."; 1496 case RELATEDARTIFACT: return "Related artifacts such as additional documentation, justification, or bibliographic references."; 1497 case SAMPLEDDATA: return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 1498 case SIGNATURE: return "A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities."; 1499 case SIMPLEQUANTITY: return ""; 1500 case TIMING: return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 1501 case TRIGGERDEFINITION: return "A description of a triggering event."; 1502 case USAGECONTEXT: return "Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 1503 case BASE64BINARY: return "A stream of bytes"; 1504 case BOOLEAN: return "Value of \"true\" or \"false\""; 1505 case CODE: return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 1506 case DATE: return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 1507 case DATETIME: return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 1508 case DECIMAL: return "A rational number with implicit precision"; 1509 case ID: return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 1510 case INSTANT: return "An instant in time - known at least to the second"; 1511 case INTEGER: return "A whole number"; 1512 case MARKDOWN: return "A string that may contain markdown syntax for optional processing by a mark down presentation engine"; 1513 case OID: return "An OID represented as a URI"; 1514 case POSITIVEINT: return "An integer with a value that is positive (e.g. >0)"; 1515 case STRING: return "A sequence of Unicode characters"; 1516 case TIME: return "A time during the day, with no date specified"; 1517 case UNSIGNEDINT: return "An integer with a value that is not negative (e.g. >= 0)"; 1518 case URI: return "String of characters used to identify a name or a resource"; 1519 case UUID: return "A UUID, represented as a URI"; 1520 case XHTML: return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 1521 case NULL: return null; 1522 default: return "?"; 1523 } 1524 } 1525 public String getDisplay() { 1526 switch (this) { 1527 case ADDRESS: return "Address"; 1528 case AGE: return "Age"; 1529 case ANNOTATION: return "Annotation"; 1530 case ATTACHMENT: return "Attachment"; 1531 case BACKBONEELEMENT: return "BackboneElement"; 1532 case CODEABLECONCEPT: return "CodeableConcept"; 1533 case CODING: return "Coding"; 1534 case CONTACTDETAIL: return "ContactDetail"; 1535 case CONTACTPOINT: return "ContactPoint"; 1536 case CONTRIBUTOR: return "Contributor"; 1537 case COUNT: return "Count"; 1538 case DATAREQUIREMENT: return "DataRequirement"; 1539 case DISTANCE: return "Distance"; 1540 case DOSAGE: return "Dosage"; 1541 case DURATION: return "Duration"; 1542 case ELEMENT: return "Element"; 1543 case ELEMENTDEFINITION: return "ElementDefinition"; 1544 case EXTENSION: return "Extension"; 1545 case HUMANNAME: return "HumanName"; 1546 case IDENTIFIER: return "Identifier"; 1547 case META: return "Meta"; 1548 case MONEY: return "Money"; 1549 case NARRATIVE: return "Narrative"; 1550 case PARAMETERDEFINITION: return "ParameterDefinition"; 1551 case PERIOD: return "Period"; 1552 case QUANTITY: return "Quantity"; 1553 case RANGE: return "Range"; 1554 case RATIO: return "Ratio"; 1555 case REFERENCE: return "Reference"; 1556 case RELATEDARTIFACT: return "RelatedArtifact"; 1557 case SAMPLEDDATA: return "SampledData"; 1558 case SIGNATURE: return "Signature"; 1559 case SIMPLEQUANTITY: return "SimpleQuantity"; 1560 case TIMING: return "Timing"; 1561 case TRIGGERDEFINITION: return "TriggerDefinition"; 1562 case USAGECONTEXT: return "UsageContext"; 1563 case BASE64BINARY: return "base64Binary"; 1564 case BOOLEAN: return "boolean"; 1565 case CODE: return "code"; 1566 case DATE: return "date"; 1567 case DATETIME: return "dateTime"; 1568 case DECIMAL: return "decimal"; 1569 case ID: return "id"; 1570 case INSTANT: return "instant"; 1571 case INTEGER: return "integer"; 1572 case MARKDOWN: return "markdown"; 1573 case OID: return "oid"; 1574 case POSITIVEINT: return "positiveInt"; 1575 case STRING: return "string"; 1576 case TIME: return "time"; 1577 case UNSIGNEDINT: return "unsignedInt"; 1578 case URI: return "uri"; 1579 case UUID: return "uuid"; 1580 case XHTML: return "XHTML"; 1581 case NULL: return null; 1582 default: return "?"; 1583 } 1584 } 1585 } 1586 1587 public static class DataTypeEnumFactory implements EnumFactory<DataType> { 1588 public DataType fromCode(String codeString) throws IllegalArgumentException { 1589 if (codeString == null || "".equals(codeString)) 1590 if (codeString == null || "".equals(codeString)) 1591 return null; 1592 if ("Address".equals(codeString)) 1593 return DataType.ADDRESS; 1594 if ("Age".equals(codeString)) 1595 return DataType.AGE; 1596 if ("Annotation".equals(codeString)) 1597 return DataType.ANNOTATION; 1598 if ("Attachment".equals(codeString)) 1599 return DataType.ATTACHMENT; 1600 if ("BackboneElement".equals(codeString)) 1601 return DataType.BACKBONEELEMENT; 1602 if ("CodeableConcept".equals(codeString)) 1603 return DataType.CODEABLECONCEPT; 1604 if ("Coding".equals(codeString)) 1605 return DataType.CODING; 1606 if ("ContactDetail".equals(codeString)) 1607 return DataType.CONTACTDETAIL; 1608 if ("ContactPoint".equals(codeString)) 1609 return DataType.CONTACTPOINT; 1610 if ("Contributor".equals(codeString)) 1611 return DataType.CONTRIBUTOR; 1612 if ("Count".equals(codeString)) 1613 return DataType.COUNT; 1614 if ("DataRequirement".equals(codeString)) 1615 return DataType.DATAREQUIREMENT; 1616 if ("Distance".equals(codeString)) 1617 return DataType.DISTANCE; 1618 if ("Dosage".equals(codeString)) 1619 return DataType.DOSAGE; 1620 if ("Duration".equals(codeString)) 1621 return DataType.DURATION; 1622 if ("Element".equals(codeString)) 1623 return DataType.ELEMENT; 1624 if ("ElementDefinition".equals(codeString)) 1625 return DataType.ELEMENTDEFINITION; 1626 if ("Extension".equals(codeString)) 1627 return DataType.EXTENSION; 1628 if ("HumanName".equals(codeString)) 1629 return DataType.HUMANNAME; 1630 if ("Identifier".equals(codeString)) 1631 return DataType.IDENTIFIER; 1632 if ("Meta".equals(codeString)) 1633 return DataType.META; 1634 if ("Money".equals(codeString)) 1635 return DataType.MONEY; 1636 if ("Narrative".equals(codeString)) 1637 return DataType.NARRATIVE; 1638 if ("ParameterDefinition".equals(codeString)) 1639 return DataType.PARAMETERDEFINITION; 1640 if ("Period".equals(codeString)) 1641 return DataType.PERIOD; 1642 if ("Quantity".equals(codeString)) 1643 return DataType.QUANTITY; 1644 if ("Range".equals(codeString)) 1645 return DataType.RANGE; 1646 if ("Ratio".equals(codeString)) 1647 return DataType.RATIO; 1648 if ("Reference".equals(codeString)) 1649 return DataType.REFERENCE; 1650 if ("RelatedArtifact".equals(codeString)) 1651 return DataType.RELATEDARTIFACT; 1652 if ("SampledData".equals(codeString)) 1653 return DataType.SAMPLEDDATA; 1654 if ("Signature".equals(codeString)) 1655 return DataType.SIGNATURE; 1656 if ("SimpleQuantity".equals(codeString)) 1657 return DataType.SIMPLEQUANTITY; 1658 if ("Timing".equals(codeString)) 1659 return DataType.TIMING; 1660 if ("TriggerDefinition".equals(codeString)) 1661 return DataType.TRIGGERDEFINITION; 1662 if ("UsageContext".equals(codeString)) 1663 return DataType.USAGECONTEXT; 1664 if ("base64Binary".equals(codeString)) 1665 return DataType.BASE64BINARY; 1666 if ("boolean".equals(codeString)) 1667 return DataType.BOOLEAN; 1668 if ("code".equals(codeString)) 1669 return DataType.CODE; 1670 if ("date".equals(codeString)) 1671 return DataType.DATE; 1672 if ("dateTime".equals(codeString)) 1673 return DataType.DATETIME; 1674 if ("decimal".equals(codeString)) 1675 return DataType.DECIMAL; 1676 if ("id".equals(codeString)) 1677 return DataType.ID; 1678 if ("instant".equals(codeString)) 1679 return DataType.INSTANT; 1680 if ("integer".equals(codeString)) 1681 return DataType.INTEGER; 1682 if ("markdown".equals(codeString)) 1683 return DataType.MARKDOWN; 1684 if ("oid".equals(codeString)) 1685 return DataType.OID; 1686 if ("positiveInt".equals(codeString)) 1687 return DataType.POSITIVEINT; 1688 if ("string".equals(codeString)) 1689 return DataType.STRING; 1690 if ("time".equals(codeString)) 1691 return DataType.TIME; 1692 if ("unsignedInt".equals(codeString)) 1693 return DataType.UNSIGNEDINT; 1694 if ("uri".equals(codeString)) 1695 return DataType.URI; 1696 if ("uuid".equals(codeString)) 1697 return DataType.UUID; 1698 if ("xhtml".equals(codeString)) 1699 return DataType.XHTML; 1700 throw new IllegalArgumentException("Unknown DataType code '"+codeString+"'"); 1701 } 1702 public Enumeration<DataType> fromType(PrimitiveType<?> code) throws FHIRException { 1703 if (code == null) 1704 return null; 1705 if (code.isEmpty()) 1706 return new Enumeration<DataType>(this); 1707 String codeString = code.asStringValue(); 1708 if (codeString == null || "".equals(codeString)) 1709 return null; 1710 if ("Address".equals(codeString)) 1711 return new Enumeration<DataType>(this, DataType.ADDRESS); 1712 if ("Age".equals(codeString)) 1713 return new Enumeration<DataType>(this, DataType.AGE); 1714 if ("Annotation".equals(codeString)) 1715 return new Enumeration<DataType>(this, DataType.ANNOTATION); 1716 if ("Attachment".equals(codeString)) 1717 return new Enumeration<DataType>(this, DataType.ATTACHMENT); 1718 if ("BackboneElement".equals(codeString)) 1719 return new Enumeration<DataType>(this, DataType.BACKBONEELEMENT); 1720 if ("CodeableConcept".equals(codeString)) 1721 return new Enumeration<DataType>(this, DataType.CODEABLECONCEPT); 1722 if ("Coding".equals(codeString)) 1723 return new Enumeration<DataType>(this, DataType.CODING); 1724 if ("ContactDetail".equals(codeString)) 1725 return new Enumeration<DataType>(this, DataType.CONTACTDETAIL); 1726 if ("ContactPoint".equals(codeString)) 1727 return new Enumeration<DataType>(this, DataType.CONTACTPOINT); 1728 if ("Contributor".equals(codeString)) 1729 return new Enumeration<DataType>(this, DataType.CONTRIBUTOR); 1730 if ("Count".equals(codeString)) 1731 return new Enumeration<DataType>(this, DataType.COUNT); 1732 if ("DataRequirement".equals(codeString)) 1733 return new Enumeration<DataType>(this, DataType.DATAREQUIREMENT); 1734 if ("Distance".equals(codeString)) 1735 return new Enumeration<DataType>(this, DataType.DISTANCE); 1736 if ("Dosage".equals(codeString)) 1737 return new Enumeration<DataType>(this, DataType.DOSAGE); 1738 if ("Duration".equals(codeString)) 1739 return new Enumeration<DataType>(this, DataType.DURATION); 1740 if ("Element".equals(codeString)) 1741 return new Enumeration<DataType>(this, DataType.ELEMENT); 1742 if ("ElementDefinition".equals(codeString)) 1743 return new Enumeration<DataType>(this, DataType.ELEMENTDEFINITION); 1744 if ("Extension".equals(codeString)) 1745 return new Enumeration<DataType>(this, DataType.EXTENSION); 1746 if ("HumanName".equals(codeString)) 1747 return new Enumeration<DataType>(this, DataType.HUMANNAME); 1748 if ("Identifier".equals(codeString)) 1749 return new Enumeration<DataType>(this, DataType.IDENTIFIER); 1750 if ("Meta".equals(codeString)) 1751 return new Enumeration<DataType>(this, DataType.META); 1752 if ("Money".equals(codeString)) 1753 return new Enumeration<DataType>(this, DataType.MONEY); 1754 if ("Narrative".equals(codeString)) 1755 return new Enumeration<DataType>(this, DataType.NARRATIVE); 1756 if ("ParameterDefinition".equals(codeString)) 1757 return new Enumeration<DataType>(this, DataType.PARAMETERDEFINITION); 1758 if ("Period".equals(codeString)) 1759 return new Enumeration<DataType>(this, DataType.PERIOD); 1760 if ("Quantity".equals(codeString)) 1761 return new Enumeration<DataType>(this, DataType.QUANTITY); 1762 if ("Range".equals(codeString)) 1763 return new Enumeration<DataType>(this, DataType.RANGE); 1764 if ("Ratio".equals(codeString)) 1765 return new Enumeration<DataType>(this, DataType.RATIO); 1766 if ("Reference".equals(codeString)) 1767 return new Enumeration<DataType>(this, DataType.REFERENCE); 1768 if ("RelatedArtifact".equals(codeString)) 1769 return new Enumeration<DataType>(this, DataType.RELATEDARTIFACT); 1770 if ("SampledData".equals(codeString)) 1771 return new Enumeration<DataType>(this, DataType.SAMPLEDDATA); 1772 if ("Signature".equals(codeString)) 1773 return new Enumeration<DataType>(this, DataType.SIGNATURE); 1774 if ("SimpleQuantity".equals(codeString)) 1775 return new Enumeration<DataType>(this, DataType.SIMPLEQUANTITY); 1776 if ("Timing".equals(codeString)) 1777 return new Enumeration<DataType>(this, DataType.TIMING); 1778 if ("TriggerDefinition".equals(codeString)) 1779 return new Enumeration<DataType>(this, DataType.TRIGGERDEFINITION); 1780 if ("UsageContext".equals(codeString)) 1781 return new Enumeration<DataType>(this, DataType.USAGECONTEXT); 1782 if ("base64Binary".equals(codeString)) 1783 return new Enumeration<DataType>(this, DataType.BASE64BINARY); 1784 if ("boolean".equals(codeString)) 1785 return new Enumeration<DataType>(this, DataType.BOOLEAN); 1786 if ("code".equals(codeString)) 1787 return new Enumeration<DataType>(this, DataType.CODE); 1788 if ("date".equals(codeString)) 1789 return new Enumeration<DataType>(this, DataType.DATE); 1790 if ("dateTime".equals(codeString)) 1791 return new Enumeration<DataType>(this, DataType.DATETIME); 1792 if ("decimal".equals(codeString)) 1793 return new Enumeration<DataType>(this, DataType.DECIMAL); 1794 if ("id".equals(codeString)) 1795 return new Enumeration<DataType>(this, DataType.ID); 1796 if ("instant".equals(codeString)) 1797 return new Enumeration<DataType>(this, DataType.INSTANT); 1798 if ("integer".equals(codeString)) 1799 return new Enumeration<DataType>(this, DataType.INTEGER); 1800 if ("markdown".equals(codeString)) 1801 return new Enumeration<DataType>(this, DataType.MARKDOWN); 1802 if ("oid".equals(codeString)) 1803 return new Enumeration<DataType>(this, DataType.OID); 1804 if ("positiveInt".equals(codeString)) 1805 return new Enumeration<DataType>(this, DataType.POSITIVEINT); 1806 if ("string".equals(codeString)) 1807 return new Enumeration<DataType>(this, DataType.STRING); 1808 if ("time".equals(codeString)) 1809 return new Enumeration<DataType>(this, DataType.TIME); 1810 if ("unsignedInt".equals(codeString)) 1811 return new Enumeration<DataType>(this, DataType.UNSIGNEDINT); 1812 if ("uri".equals(codeString)) 1813 return new Enumeration<DataType>(this, DataType.URI); 1814 if ("uuid".equals(codeString)) 1815 return new Enumeration<DataType>(this, DataType.UUID); 1816 if ("xhtml".equals(codeString)) 1817 return new Enumeration<DataType>(this, DataType.XHTML); 1818 throw new FHIRException("Unknown DataType code '"+codeString+"'"); 1819 } 1820 public String toCode(DataType code) { 1821 if (code == DataType.NULL) 1822 return null; 1823 if (code == DataType.ADDRESS) 1824 return "Address"; 1825 if (code == DataType.AGE) 1826 return "Age"; 1827 if (code == DataType.ANNOTATION) 1828 return "Annotation"; 1829 if (code == DataType.ATTACHMENT) 1830 return "Attachment"; 1831 if (code == DataType.BACKBONEELEMENT) 1832 return "BackboneElement"; 1833 if (code == DataType.CODEABLECONCEPT) 1834 return "CodeableConcept"; 1835 if (code == DataType.CODING) 1836 return "Coding"; 1837 if (code == DataType.CONTACTDETAIL) 1838 return "ContactDetail"; 1839 if (code == DataType.CONTACTPOINT) 1840 return "ContactPoint"; 1841 if (code == DataType.CONTRIBUTOR) 1842 return "Contributor"; 1843 if (code == DataType.COUNT) 1844 return "Count"; 1845 if (code == DataType.DATAREQUIREMENT) 1846 return "DataRequirement"; 1847 if (code == DataType.DISTANCE) 1848 return "Distance"; 1849 if (code == DataType.DOSAGE) 1850 return "Dosage"; 1851 if (code == DataType.DURATION) 1852 return "Duration"; 1853 if (code == DataType.ELEMENT) 1854 return "Element"; 1855 if (code == DataType.ELEMENTDEFINITION) 1856 return "ElementDefinition"; 1857 if (code == DataType.EXTENSION) 1858 return "Extension"; 1859 if (code == DataType.HUMANNAME) 1860 return "HumanName"; 1861 if (code == DataType.IDENTIFIER) 1862 return "Identifier"; 1863 if (code == DataType.META) 1864 return "Meta"; 1865 if (code == DataType.MONEY) 1866 return "Money"; 1867 if (code == DataType.NARRATIVE) 1868 return "Narrative"; 1869 if (code == DataType.PARAMETERDEFINITION) 1870 return "ParameterDefinition"; 1871 if (code == DataType.PERIOD) 1872 return "Period"; 1873 if (code == DataType.QUANTITY) 1874 return "Quantity"; 1875 if (code == DataType.RANGE) 1876 return "Range"; 1877 if (code == DataType.RATIO) 1878 return "Ratio"; 1879 if (code == DataType.REFERENCE) 1880 return "Reference"; 1881 if (code == DataType.RELATEDARTIFACT) 1882 return "RelatedArtifact"; 1883 if (code == DataType.SAMPLEDDATA) 1884 return "SampledData"; 1885 if (code == DataType.SIGNATURE) 1886 return "Signature"; 1887 if (code == DataType.SIMPLEQUANTITY) 1888 return "SimpleQuantity"; 1889 if (code == DataType.TIMING) 1890 return "Timing"; 1891 if (code == DataType.TRIGGERDEFINITION) 1892 return "TriggerDefinition"; 1893 if (code == DataType.USAGECONTEXT) 1894 return "UsageContext"; 1895 if (code == DataType.BASE64BINARY) 1896 return "base64Binary"; 1897 if (code == DataType.BOOLEAN) 1898 return "boolean"; 1899 if (code == DataType.CODE) 1900 return "code"; 1901 if (code == DataType.DATE) 1902 return "date"; 1903 if (code == DataType.DATETIME) 1904 return "dateTime"; 1905 if (code == DataType.DECIMAL) 1906 return "decimal"; 1907 if (code == DataType.ID) 1908 return "id"; 1909 if (code == DataType.INSTANT) 1910 return "instant"; 1911 if (code == DataType.INTEGER) 1912 return "integer"; 1913 if (code == DataType.MARKDOWN) 1914 return "markdown"; 1915 if (code == DataType.OID) 1916 return "oid"; 1917 if (code == DataType.POSITIVEINT) 1918 return "positiveInt"; 1919 if (code == DataType.STRING) 1920 return "string"; 1921 if (code == DataType.TIME) 1922 return "time"; 1923 if (code == DataType.UNSIGNEDINT) 1924 return "unsignedInt"; 1925 if (code == DataType.URI) 1926 return "uri"; 1927 if (code == DataType.UUID) 1928 return "uuid"; 1929 if (code == DataType.XHTML) 1930 return "xhtml"; 1931 return "?"; 1932 } 1933 public String toSystem(DataType code) { 1934 return code.getSystem(); 1935 } 1936 } 1937 1938 public enum DocumentReferenceStatus { 1939 /** 1940 * This is the current reference for this document. 1941 */ 1942 CURRENT, 1943 /** 1944 * This reference has been superseded by another reference. 1945 */ 1946 SUPERSEDED, 1947 /** 1948 * This reference was created in error. 1949 */ 1950 ENTEREDINERROR, 1951 /** 1952 * added to help the parsers 1953 */ 1954 NULL; 1955 public static DocumentReferenceStatus fromCode(String codeString) throws FHIRException { 1956 if (codeString == null || "".equals(codeString)) 1957 return null; 1958 if ("current".equals(codeString)) 1959 return CURRENT; 1960 if ("superseded".equals(codeString)) 1961 return SUPERSEDED; 1962 if ("entered-in-error".equals(codeString)) 1963 return ENTEREDINERROR; 1964 throw new FHIRException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 1965 } 1966 public String toCode() { 1967 switch (this) { 1968 case CURRENT: return "current"; 1969 case SUPERSEDED: return "superseded"; 1970 case ENTEREDINERROR: return "entered-in-error"; 1971 case NULL: return null; 1972 default: return "?"; 1973 } 1974 } 1975 public String getSystem() { 1976 switch (this) { 1977 case CURRENT: return "http://hl7.org/fhir/document-reference-status"; 1978 case SUPERSEDED: return "http://hl7.org/fhir/document-reference-status"; 1979 case ENTEREDINERROR: return "http://hl7.org/fhir/document-reference-status"; 1980 case NULL: return null; 1981 default: return "?"; 1982 } 1983 } 1984 public String getDefinition() { 1985 switch (this) { 1986 case CURRENT: return "This is the current reference for this document."; 1987 case SUPERSEDED: return "This reference has been superseded by another reference."; 1988 case ENTEREDINERROR: return "This reference was created in error."; 1989 case NULL: return null; 1990 default: return "?"; 1991 } 1992 } 1993 public String getDisplay() { 1994 switch (this) { 1995 case CURRENT: return "Current"; 1996 case SUPERSEDED: return "Superseded"; 1997 case ENTEREDINERROR: return "Entered in Error"; 1998 case NULL: return null; 1999 default: return "?"; 2000 } 2001 } 2002 } 2003 2004 public static class DocumentReferenceStatusEnumFactory implements EnumFactory<DocumentReferenceStatus> { 2005 public DocumentReferenceStatus fromCode(String codeString) throws IllegalArgumentException { 2006 if (codeString == null || "".equals(codeString)) 2007 if (codeString == null || "".equals(codeString)) 2008 return null; 2009 if ("current".equals(codeString)) 2010 return DocumentReferenceStatus.CURRENT; 2011 if ("superseded".equals(codeString)) 2012 return DocumentReferenceStatus.SUPERSEDED; 2013 if ("entered-in-error".equals(codeString)) 2014 return DocumentReferenceStatus.ENTEREDINERROR; 2015 throw new IllegalArgumentException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 2016 } 2017 public Enumeration<DocumentReferenceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 2018 if (code == null) 2019 return null; 2020 if (code.isEmpty()) 2021 return new Enumeration<DocumentReferenceStatus>(this); 2022 String codeString = code.asStringValue(); 2023 if (codeString == null || "".equals(codeString)) 2024 return null; 2025 if ("current".equals(codeString)) 2026 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.CURRENT); 2027 if ("superseded".equals(codeString)) 2028 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.SUPERSEDED); 2029 if ("entered-in-error".equals(codeString)) 2030 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.ENTEREDINERROR); 2031 throw new FHIRException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 2032 } 2033 public String toCode(DocumentReferenceStatus code) { 2034 if (code == DocumentReferenceStatus.NULL) 2035 return null; 2036 if (code == DocumentReferenceStatus.CURRENT) 2037 return "current"; 2038 if (code == DocumentReferenceStatus.SUPERSEDED) 2039 return "superseded"; 2040 if (code == DocumentReferenceStatus.ENTEREDINERROR) 2041 return "entered-in-error"; 2042 return "?"; 2043 } 2044 public String toSystem(DocumentReferenceStatus code) { 2045 return code.getSystem(); 2046 } 2047 } 2048 2049 public enum FHIRAllTypes { 2050 /** 2051 * An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world. 2052 */ 2053 ADDRESS, 2054 /** 2055 * A duration of time during which an organism (or a process) has existed. 2056 */ 2057 AGE, 2058 /** 2059 * A text note which also contains information about who made the statement and when. 2060 */ 2061 ANNOTATION, 2062 /** 2063 * For referring to data content defined in other formats. 2064 */ 2065 ATTACHMENT, 2066 /** 2067 * Base definition for all elements that are defined inside a resource - but not those in a data type. 2068 */ 2069 BACKBONEELEMENT, 2070 /** 2071 * A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text. 2072 */ 2073 CODEABLECONCEPT, 2074 /** 2075 * A reference to a code defined by a terminology system. 2076 */ 2077 CODING, 2078 /** 2079 * Specifies contact information for a person or organization. 2080 */ 2081 CONTACTDETAIL, 2082 /** 2083 * Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 2084 */ 2085 CONTACTPOINT, 2086 /** 2087 * A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers. 2088 */ 2089 CONTRIBUTOR, 2090 /** 2091 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 2092 */ 2093 COUNT, 2094 /** 2095 * Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data. 2096 */ 2097 DATAREQUIREMENT, 2098 /** 2099 * A length - a value with a unit that is a physical distance. 2100 */ 2101 DISTANCE, 2102 /** 2103 * Indicates how the medication is/was taken or should be taken by the patient. 2104 */ 2105 DOSAGE, 2106 /** 2107 * A length of time. 2108 */ 2109 DURATION, 2110 /** 2111 * Base definition for all elements in a resource. 2112 */ 2113 ELEMENT, 2114 /** 2115 * Captures constraints on each element within the resource, profile, or extension. 2116 */ 2117 ELEMENTDEFINITION, 2118 /** 2119 * Optional Extension Element - found in all resources. 2120 */ 2121 EXTENSION, 2122 /** 2123 * A human's name with the ability to identify parts and usage. 2124 */ 2125 HUMANNAME, 2126 /** 2127 * A technical identifier - identifies some entity uniquely and unambiguously. 2128 */ 2129 IDENTIFIER, 2130 /** 2131 * The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource. 2132 */ 2133 META, 2134 /** 2135 * An amount of economic utility in some recognized currency. 2136 */ 2137 MONEY, 2138 /** 2139 * A human-readable formatted text, including images. 2140 */ 2141 NARRATIVE, 2142 /** 2143 * The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse. 2144 */ 2145 PARAMETERDEFINITION, 2146 /** 2147 * A time period defined by a start and end date and optionally time. 2148 */ 2149 PERIOD, 2150 /** 2151 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 2152 */ 2153 QUANTITY, 2154 /** 2155 * A set of ordered Quantities defined by a low and high limit. 2156 */ 2157 RANGE, 2158 /** 2159 * A relationship of two Quantity values - expressed as a numerator and a denominator. 2160 */ 2161 RATIO, 2162 /** 2163 * A reference from one resource to another. 2164 */ 2165 REFERENCE, 2166 /** 2167 * Related artifacts such as additional documentation, justification, or bibliographic references. 2168 */ 2169 RELATEDARTIFACT, 2170 /** 2171 * A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data. 2172 */ 2173 SAMPLEDDATA, 2174 /** 2175 * A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities. 2176 */ 2177 SIGNATURE, 2178 /** 2179 * null 2180 */ 2181 SIMPLEQUANTITY, 2182 /** 2183 * Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out. 2184 */ 2185 TIMING, 2186 /** 2187 * A description of a triggering event. 2188 */ 2189 TRIGGERDEFINITION, 2190 /** 2191 * Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care). 2192 */ 2193 USAGECONTEXT, 2194 /** 2195 * A stream of bytes 2196 */ 2197 BASE64BINARY, 2198 /** 2199 * Value of "true" or "false" 2200 */ 2201 BOOLEAN, 2202 /** 2203 * A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents 2204 */ 2205 CODE, 2206 /** 2207 * A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates. 2208 */ 2209 DATE, 2210 /** 2211 * A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates. 2212 */ 2213 DATETIME, 2214 /** 2215 * A rational number with implicit precision 2216 */ 2217 DECIMAL, 2218 /** 2219 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive. 2220 */ 2221 ID, 2222 /** 2223 * An instant in time - known at least to the second 2224 */ 2225 INSTANT, 2226 /** 2227 * A whole number 2228 */ 2229 INTEGER, 2230 /** 2231 * A string that may contain markdown syntax for optional processing by a mark down presentation engine 2232 */ 2233 MARKDOWN, 2234 /** 2235 * An OID represented as a URI 2236 */ 2237 OID, 2238 /** 2239 * An integer with a value that is positive (e.g. >0) 2240 */ 2241 POSITIVEINT, 2242 /** 2243 * A sequence of Unicode characters 2244 */ 2245 STRING, 2246 /** 2247 * A time during the day, with no date specified 2248 */ 2249 TIME, 2250 /** 2251 * An integer with a value that is not negative (e.g. >= 0) 2252 */ 2253 UNSIGNEDINT, 2254 /** 2255 * String of characters used to identify a name or a resource 2256 */ 2257 URI, 2258 /** 2259 * A UUID, represented as a URI 2260 */ 2261 UUID, 2262 /** 2263 * XHTML format, as defined by W3C, but restricted usage (mainly, no active content) 2264 */ 2265 XHTML, 2266 /** 2267 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 2268 */ 2269 ACCOUNT, 2270 /** 2271 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 2272 */ 2273 ACTIVITYDEFINITION, 2274 /** 2275 * Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death. 2276 */ 2277 ADVERSEEVENT, 2278 /** 2279 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 2280 */ 2281 ALLERGYINTOLERANCE, 2282 /** 2283 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 2284 */ 2285 APPOINTMENT, 2286 /** 2287 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 2288 */ 2289 APPOINTMENTRESPONSE, 2290 /** 2291 * A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage. 2292 */ 2293 AUDITEVENT, 2294 /** 2295 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 2296 */ 2297 BASIC, 2298 /** 2299 * A binary resource can contain any content, whether text, image, pdf, zip archive, etc. 2300 */ 2301 BINARY, 2302 /** 2303 * Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 2304 */ 2305 BODYSITE, 2306 /** 2307 * A container for a collection of resources. 2308 */ 2309 BUNDLE, 2310 /** 2311 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 2312 */ 2313 CAPABILITYSTATEMENT, 2314 /** 2315 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 2316 */ 2317 CAREPLAN, 2318 /** 2319 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 2320 */ 2321 CARETEAM, 2322 /** 2323 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 2324 */ 2325 CHARGEITEM, 2326 /** 2327 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 2328 */ 2329 CLAIM, 2330 /** 2331 * This resource provides the adjudication details from the processing of a Claim resource. 2332 */ 2333 CLAIMRESPONSE, 2334 /** 2335 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 2336 */ 2337 CLINICALIMPRESSION, 2338 /** 2339 * A code system resource specifies a set of codes drawn from one or more code systems. 2340 */ 2341 CODESYSTEM, 2342 /** 2343 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 2344 */ 2345 COMMUNICATION, 2346 /** 2347 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 2348 */ 2349 COMMUNICATIONREQUEST, 2350 /** 2351 * A compartment definition that defines how resources are accessed on a server. 2352 */ 2353 COMPARTMENTDEFINITION, 2354 /** 2355 * A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained. 2356 */ 2357 COMPOSITION, 2358 /** 2359 * A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models. 2360 */ 2361 CONCEPTMAP, 2362 /** 2363 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 2364 */ 2365 CONDITION, 2366 /** 2367 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 2368 */ 2369 CONSENT, 2370 /** 2371 * A formal agreement between parties regarding the conduct of business, exchange of information or other matters. 2372 */ 2373 CONTRACT, 2374 /** 2375 * Financial instrument which may be used to reimburse or pay for health care products and services. 2376 */ 2377 COVERAGE, 2378 /** 2379 * The formal description of a single piece of information that can be gathered and reported. 2380 */ 2381 DATAELEMENT, 2382 /** 2383 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 2384 */ 2385 DETECTEDISSUE, 2386 /** 2387 * This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc. 2388 */ 2389 DEVICE, 2390 /** 2391 * The characteristics, operational status and capabilities of a medical-related component of a medical device. 2392 */ 2393 DEVICECOMPONENT, 2394 /** 2395 * Describes a measurement, calculation or setting capability of a medical device. 2396 */ 2397 DEVICEMETRIC, 2398 /** 2399 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 2400 */ 2401 DEVICEREQUEST, 2402 /** 2403 * A record of a device being used by a patient where the record is the result of a report from the patient or another clinician. 2404 */ 2405 DEVICEUSESTATEMENT, 2406 /** 2407 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 2408 */ 2409 DIAGNOSTICREPORT, 2410 /** 2411 * A collection of documents compiled for a purpose together with metadata that applies to the collection. 2412 */ 2413 DOCUMENTMANIFEST, 2414 /** 2415 * A reference to a document. 2416 */ 2417 DOCUMENTREFERENCE, 2418 /** 2419 * A resource that includes narrative, extensions, and contained resources. 2420 */ 2421 DOMAINRESOURCE, 2422 /** 2423 * The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 2424 */ 2425 ELIGIBILITYREQUEST, 2426 /** 2427 * This resource provides eligibility and plan details from the processing of an Eligibility resource. 2428 */ 2429 ELIGIBILITYRESPONSE, 2430 /** 2431 * An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient. 2432 */ 2433 ENCOUNTER, 2434 /** 2435 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 2436 */ 2437 ENDPOINT, 2438 /** 2439 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 2440 */ 2441 ENROLLMENTREQUEST, 2442 /** 2443 * This resource provides enrollment and plan details from the processing of an Enrollment resource. 2444 */ 2445 ENROLLMENTRESPONSE, 2446 /** 2447 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 2448 */ 2449 EPISODEOFCARE, 2450 /** 2451 * Resource to define constraints on the Expansion of a FHIR ValueSet. 2452 */ 2453 EXPANSIONPROFILE, 2454 /** 2455 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 2456 */ 2457 EXPLANATIONOFBENEFIT, 2458 /** 2459 * Significant health events and conditions for a person related to the patient relevant in the context of care for the patient. 2460 */ 2461 FAMILYMEMBERHISTORY, 2462 /** 2463 * Prospective warnings of potential issues when providing care to the patient. 2464 */ 2465 FLAG, 2466 /** 2467 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 2468 */ 2469 GOAL, 2470 /** 2471 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 2472 */ 2473 GRAPHDEFINITION, 2474 /** 2475 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 2476 */ 2477 GROUP, 2478 /** 2479 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 2480 */ 2481 GUIDANCERESPONSE, 2482 /** 2483 * The details of a healthcare service available at a location. 2484 */ 2485 HEALTHCARESERVICE, 2486 /** 2487 * A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection. 2488 */ 2489 IMAGINGMANIFEST, 2490 /** 2491 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 2492 */ 2493 IMAGINGSTUDY, 2494 /** 2495 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 2496 */ 2497 IMMUNIZATION, 2498 /** 2499 * A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification. 2500 */ 2501 IMMUNIZATIONRECOMMENDATION, 2502 /** 2503 * A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 2504 */ 2505 IMPLEMENTATIONGUIDE, 2506 /** 2507 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 2508 */ 2509 LIBRARY, 2510 /** 2511 * Identifies two or more records (resource instances) that are referring to the same real-world "occurrence". 2512 */ 2513 LINKAGE, 2514 /** 2515 * A set of information summarized from a list of other resources. 2516 */ 2517 LIST, 2518 /** 2519 * Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated. 2520 */ 2521 LOCATION, 2522 /** 2523 * The Measure resource provides the definition of a quality measure. 2524 */ 2525 MEASURE, 2526 /** 2527 * The MeasureReport resource contains the results of evaluating a measure. 2528 */ 2529 MEASUREREPORT, 2530 /** 2531 * A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference. 2532 */ 2533 MEDIA, 2534 /** 2535 * This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication. 2536 */ 2537 MEDICATION, 2538 /** 2539 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. 2540 */ 2541 MEDICATIONADMINISTRATION, 2542 /** 2543 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 2544 */ 2545 MEDICATIONDISPENSE, 2546 /** 2547 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 2548 */ 2549 MEDICATIONREQUEST, 2550 /** 2551 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 2552 2553The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 2554 */ 2555 MEDICATIONSTATEMENT, 2556 /** 2557 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 2558 */ 2559 MESSAGEDEFINITION, 2560 /** 2561 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 2562 */ 2563 MESSAGEHEADER, 2564 /** 2565 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 2566 */ 2567 NAMINGSYSTEM, 2568 /** 2569 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 2570 */ 2571 NUTRITIONORDER, 2572 /** 2573 * Measurements and simple assertions made about a patient, device or other subject. 2574 */ 2575 OBSERVATION, 2576 /** 2577 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 2578 */ 2579 OPERATIONDEFINITION, 2580 /** 2581 * A collection of error, warning or information messages that result from a system action. 2582 */ 2583 OPERATIONOUTCOME, 2584 /** 2585 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc. 2586 */ 2587 ORGANIZATION, 2588 /** 2589 * This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it. 2590 */ 2591 PARAMETERS, 2592 /** 2593 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 2594 */ 2595 PATIENT, 2596 /** 2597 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 2598 */ 2599 PAYMENTNOTICE, 2600 /** 2601 * This resource provides payment details and claim references supporting a bulk payment. 2602 */ 2603 PAYMENTRECONCILIATION, 2604 /** 2605 * Demographics and administrative information about a person independent of a specific health-related context. 2606 */ 2607 PERSON, 2608 /** 2609 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols. 2610 */ 2611 PLANDEFINITION, 2612 /** 2613 * A person who is directly or indirectly involved in the provisioning of healthcare. 2614 */ 2615 PRACTITIONER, 2616 /** 2617 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 2618 */ 2619 PRACTITIONERROLE, 2620 /** 2621 * An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy. 2622 */ 2623 PROCEDURE, 2624 /** 2625 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 2626 */ 2627 PROCEDUREREQUEST, 2628 /** 2629 * This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources. 2630 */ 2631 PROCESSREQUEST, 2632 /** 2633 * This resource provides processing status, errors and notes from the processing of a resource. 2634 */ 2635 PROCESSRESPONSE, 2636 /** 2637 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 2638 */ 2639 PROVENANCE, 2640 /** 2641 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 2642 */ 2643 QUESTIONNAIRE, 2644 /** 2645 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 2646 */ 2647 QUESTIONNAIRERESPONSE, 2648 /** 2649 * Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization. 2650 */ 2651 REFERRALREQUEST, 2652 /** 2653 * Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 2654 */ 2655 RELATEDPERSON, 2656 /** 2657 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 2658 */ 2659 REQUESTGROUP, 2660 /** 2661 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 2662 */ 2663 RESEARCHSTUDY, 2664 /** 2665 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 2666 */ 2667 RESEARCHSUBJECT, 2668 /** 2669 * This is the base resource type for everything. 2670 */ 2671 RESOURCE, 2672 /** 2673 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 2674 */ 2675 RISKASSESSMENT, 2676 /** 2677 * A container for slots of time that may be available for booking appointments. 2678 */ 2679 SCHEDULE, 2680 /** 2681 * A search parameter that defines a named search item that can be used to search/filter on a resource. 2682 */ 2683 SEARCHPARAMETER, 2684 /** 2685 * Raw data describing a biological sequence. 2686 */ 2687 SEQUENCE, 2688 /** 2689 * The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking. 2690 */ 2691 SERVICEDEFINITION, 2692 /** 2693 * A slot of time on a schedule that may be available for booking appointments. 2694 */ 2695 SLOT, 2696 /** 2697 * A sample to be used for analysis. 2698 */ 2699 SPECIMEN, 2700 /** 2701 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 2702 */ 2703 STRUCTUREDEFINITION, 2704 /** 2705 * A Map of relationships between 2 structures that can be used to transform data. 2706 */ 2707 STRUCTUREMAP, 2708 /** 2709 * The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined "channel" so that another system is able to take an appropriate action. 2710 */ 2711 SUBSCRIPTION, 2712 /** 2713 * A homogeneous material with a definite composition. 2714 */ 2715 SUBSTANCE, 2716 /** 2717 * Record of delivery of what is supplied. 2718 */ 2719 SUPPLYDELIVERY, 2720 /** 2721 * A record of a request for a medication, substance or device used in the healthcare setting. 2722 */ 2723 SUPPLYREQUEST, 2724 /** 2725 * A task to be performed. 2726 */ 2727 TASK, 2728 /** 2729 * A summary of information based on the results of executing a TestScript. 2730 */ 2731 TESTREPORT, 2732 /** 2733 * A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification. 2734 */ 2735 TESTSCRIPT, 2736 /** 2737 * A value set specifies a set of codes drawn from one or more code systems. 2738 */ 2739 VALUESET, 2740 /** 2741 * An authorization for the supply of glasses and/or contact lenses to a patient. 2742 */ 2743 VISIONPRESCRIPTION, 2744 /** 2745 * A place holder that means any kind of data type 2746 */ 2747 TYPE, 2748 /** 2749 * A place holder that means any kind of resource 2750 */ 2751 ANY, 2752 /** 2753 * added to help the parsers 2754 */ 2755 NULL; 2756 public static FHIRAllTypes fromCode(String codeString) throws FHIRException { 2757 if (codeString == null || "".equals(codeString)) 2758 return null; 2759 if ("Address".equals(codeString)) 2760 return ADDRESS; 2761 if ("Age".equals(codeString)) 2762 return AGE; 2763 if ("Annotation".equals(codeString)) 2764 return ANNOTATION; 2765 if ("Attachment".equals(codeString)) 2766 return ATTACHMENT; 2767 if ("BackboneElement".equals(codeString)) 2768 return BACKBONEELEMENT; 2769 if ("CodeableConcept".equals(codeString)) 2770 return CODEABLECONCEPT; 2771 if ("Coding".equals(codeString)) 2772 return CODING; 2773 if ("ContactDetail".equals(codeString)) 2774 return CONTACTDETAIL; 2775 if ("ContactPoint".equals(codeString)) 2776 return CONTACTPOINT; 2777 if ("Contributor".equals(codeString)) 2778 return CONTRIBUTOR; 2779 if ("Count".equals(codeString)) 2780 return COUNT; 2781 if ("DataRequirement".equals(codeString)) 2782 return DATAREQUIREMENT; 2783 if ("Distance".equals(codeString)) 2784 return DISTANCE; 2785 if ("Dosage".equals(codeString)) 2786 return DOSAGE; 2787 if ("Duration".equals(codeString)) 2788 return DURATION; 2789 if ("Element".equals(codeString)) 2790 return ELEMENT; 2791 if ("ElementDefinition".equals(codeString)) 2792 return ELEMENTDEFINITION; 2793 if ("Extension".equals(codeString)) 2794 return EXTENSION; 2795 if ("HumanName".equals(codeString)) 2796 return HUMANNAME; 2797 if ("Identifier".equals(codeString)) 2798 return IDENTIFIER; 2799 if ("Meta".equals(codeString)) 2800 return META; 2801 if ("Money".equals(codeString)) 2802 return MONEY; 2803 if ("Narrative".equals(codeString)) 2804 return NARRATIVE; 2805 if ("ParameterDefinition".equals(codeString)) 2806 return PARAMETERDEFINITION; 2807 if ("Period".equals(codeString)) 2808 return PERIOD; 2809 if ("Quantity".equals(codeString)) 2810 return QUANTITY; 2811 if ("Range".equals(codeString)) 2812 return RANGE; 2813 if ("Ratio".equals(codeString)) 2814 return RATIO; 2815 if ("Reference".equals(codeString)) 2816 return REFERENCE; 2817 if ("RelatedArtifact".equals(codeString)) 2818 return RELATEDARTIFACT; 2819 if ("SampledData".equals(codeString)) 2820 return SAMPLEDDATA; 2821 if ("Signature".equals(codeString)) 2822 return SIGNATURE; 2823 if ("SimpleQuantity".equals(codeString)) 2824 return SIMPLEQUANTITY; 2825 if ("Timing".equals(codeString)) 2826 return TIMING; 2827 if ("TriggerDefinition".equals(codeString)) 2828 return TRIGGERDEFINITION; 2829 if ("UsageContext".equals(codeString)) 2830 return USAGECONTEXT; 2831 if ("base64Binary".equals(codeString)) 2832 return BASE64BINARY; 2833 if ("boolean".equals(codeString)) 2834 return BOOLEAN; 2835 if ("code".equals(codeString)) 2836 return CODE; 2837 if ("date".equals(codeString)) 2838 return DATE; 2839 if ("dateTime".equals(codeString)) 2840 return DATETIME; 2841 if ("decimal".equals(codeString)) 2842 return DECIMAL; 2843 if ("id".equals(codeString)) 2844 return ID; 2845 if ("instant".equals(codeString)) 2846 return INSTANT; 2847 if ("integer".equals(codeString)) 2848 return INTEGER; 2849 if ("markdown".equals(codeString)) 2850 return MARKDOWN; 2851 if ("oid".equals(codeString)) 2852 return OID; 2853 if ("positiveInt".equals(codeString)) 2854 return POSITIVEINT; 2855 if ("string".equals(codeString)) 2856 return STRING; 2857 if ("time".equals(codeString)) 2858 return TIME; 2859 if ("unsignedInt".equals(codeString)) 2860 return UNSIGNEDINT; 2861 if ("uri".equals(codeString)) 2862 return URI; 2863 if ("uuid".equals(codeString)) 2864 return UUID; 2865 if ("xhtml".equals(codeString)) 2866 return XHTML; 2867 if ("Account".equals(codeString)) 2868 return ACCOUNT; 2869 if ("ActivityDefinition".equals(codeString)) 2870 return ACTIVITYDEFINITION; 2871 if ("AdverseEvent".equals(codeString)) 2872 return ADVERSEEVENT; 2873 if ("AllergyIntolerance".equals(codeString)) 2874 return ALLERGYINTOLERANCE; 2875 if ("Appointment".equals(codeString)) 2876 return APPOINTMENT; 2877 if ("AppointmentResponse".equals(codeString)) 2878 return APPOINTMENTRESPONSE; 2879 if ("AuditEvent".equals(codeString)) 2880 return AUDITEVENT; 2881 if ("Basic".equals(codeString)) 2882 return BASIC; 2883 if ("Binary".equals(codeString)) 2884 return BINARY; 2885 if ("BodySite".equals(codeString)) 2886 return BODYSITE; 2887 if ("Bundle".equals(codeString)) 2888 return BUNDLE; 2889 if ("CapabilityStatement".equals(codeString)) 2890 return CAPABILITYSTATEMENT; 2891 if ("CarePlan".equals(codeString)) 2892 return CAREPLAN; 2893 if ("CareTeam".equals(codeString)) 2894 return CARETEAM; 2895 if ("ChargeItem".equals(codeString)) 2896 return CHARGEITEM; 2897 if ("Claim".equals(codeString)) 2898 return CLAIM; 2899 if ("ClaimResponse".equals(codeString)) 2900 return CLAIMRESPONSE; 2901 if ("ClinicalImpression".equals(codeString)) 2902 return CLINICALIMPRESSION; 2903 if ("CodeSystem".equals(codeString)) 2904 return CODESYSTEM; 2905 if ("Communication".equals(codeString)) 2906 return COMMUNICATION; 2907 if ("CommunicationRequest".equals(codeString)) 2908 return COMMUNICATIONREQUEST; 2909 if ("CompartmentDefinition".equals(codeString)) 2910 return COMPARTMENTDEFINITION; 2911 if ("Composition".equals(codeString)) 2912 return COMPOSITION; 2913 if ("ConceptMap".equals(codeString)) 2914 return CONCEPTMAP; 2915 if ("Condition".equals(codeString)) 2916 return CONDITION; 2917 if ("Consent".equals(codeString)) 2918 return CONSENT; 2919 if ("Contract".equals(codeString)) 2920 return CONTRACT; 2921 if ("Coverage".equals(codeString)) 2922 return COVERAGE; 2923 if ("DataElement".equals(codeString)) 2924 return DATAELEMENT; 2925 if ("DetectedIssue".equals(codeString)) 2926 return DETECTEDISSUE; 2927 if ("Device".equals(codeString)) 2928 return DEVICE; 2929 if ("DeviceComponent".equals(codeString)) 2930 return DEVICECOMPONENT; 2931 if ("DeviceMetric".equals(codeString)) 2932 return DEVICEMETRIC; 2933 if ("DeviceRequest".equals(codeString)) 2934 return DEVICEREQUEST; 2935 if ("DeviceUseStatement".equals(codeString)) 2936 return DEVICEUSESTATEMENT; 2937 if ("DiagnosticReport".equals(codeString)) 2938 return DIAGNOSTICREPORT; 2939 if ("DocumentManifest".equals(codeString)) 2940 return DOCUMENTMANIFEST; 2941 if ("DocumentReference".equals(codeString)) 2942 return DOCUMENTREFERENCE; 2943 if ("DomainResource".equals(codeString)) 2944 return DOMAINRESOURCE; 2945 if ("EligibilityRequest".equals(codeString)) 2946 return ELIGIBILITYREQUEST; 2947 if ("EligibilityResponse".equals(codeString)) 2948 return ELIGIBILITYRESPONSE; 2949 if ("Encounter".equals(codeString)) 2950 return ENCOUNTER; 2951 if ("Endpoint".equals(codeString)) 2952 return ENDPOINT; 2953 if ("EnrollmentRequest".equals(codeString)) 2954 return ENROLLMENTREQUEST; 2955 if ("EnrollmentResponse".equals(codeString)) 2956 return ENROLLMENTRESPONSE; 2957 if ("EpisodeOfCare".equals(codeString)) 2958 return EPISODEOFCARE; 2959 if ("ExpansionProfile".equals(codeString)) 2960 return EXPANSIONPROFILE; 2961 if ("ExplanationOfBenefit".equals(codeString)) 2962 return EXPLANATIONOFBENEFIT; 2963 if ("FamilyMemberHistory".equals(codeString)) 2964 return FAMILYMEMBERHISTORY; 2965 if ("Flag".equals(codeString)) 2966 return FLAG; 2967 if ("Goal".equals(codeString)) 2968 return GOAL; 2969 if ("GraphDefinition".equals(codeString)) 2970 return GRAPHDEFINITION; 2971 if ("Group".equals(codeString)) 2972 return GROUP; 2973 if ("GuidanceResponse".equals(codeString)) 2974 return GUIDANCERESPONSE; 2975 if ("HealthcareService".equals(codeString)) 2976 return HEALTHCARESERVICE; 2977 if ("ImagingManifest".equals(codeString)) 2978 return IMAGINGMANIFEST; 2979 if ("ImagingStudy".equals(codeString)) 2980 return IMAGINGSTUDY; 2981 if ("Immunization".equals(codeString)) 2982 return IMMUNIZATION; 2983 if ("ImmunizationRecommendation".equals(codeString)) 2984 return IMMUNIZATIONRECOMMENDATION; 2985 if ("ImplementationGuide".equals(codeString)) 2986 return IMPLEMENTATIONGUIDE; 2987 if ("Library".equals(codeString)) 2988 return LIBRARY; 2989 if ("Linkage".equals(codeString)) 2990 return LINKAGE; 2991 if ("List".equals(codeString)) 2992 return LIST; 2993 if ("Location".equals(codeString)) 2994 return LOCATION; 2995 if ("Measure".equals(codeString)) 2996 return MEASURE; 2997 if ("MeasureReport".equals(codeString)) 2998 return MEASUREREPORT; 2999 if ("Media".equals(codeString)) 3000 return MEDIA; 3001 if ("Medication".equals(codeString)) 3002 return MEDICATION; 3003 if ("MedicationAdministration".equals(codeString)) 3004 return MEDICATIONADMINISTRATION; 3005 if ("MedicationDispense".equals(codeString)) 3006 return MEDICATIONDISPENSE; 3007 if ("MedicationRequest".equals(codeString)) 3008 return MEDICATIONREQUEST; 3009 if ("MedicationStatement".equals(codeString)) 3010 return MEDICATIONSTATEMENT; 3011 if ("MessageDefinition".equals(codeString)) 3012 return MESSAGEDEFINITION; 3013 if ("MessageHeader".equals(codeString)) 3014 return MESSAGEHEADER; 3015 if ("NamingSystem".equals(codeString)) 3016 return NAMINGSYSTEM; 3017 if ("NutritionOrder".equals(codeString)) 3018 return NUTRITIONORDER; 3019 if ("Observation".equals(codeString)) 3020 return OBSERVATION; 3021 if ("OperationDefinition".equals(codeString)) 3022 return OPERATIONDEFINITION; 3023 if ("OperationOutcome".equals(codeString)) 3024 return OPERATIONOUTCOME; 3025 if ("Organization".equals(codeString)) 3026 return ORGANIZATION; 3027 if ("Parameters".equals(codeString)) 3028 return PARAMETERS; 3029 if ("Patient".equals(codeString)) 3030 return PATIENT; 3031 if ("PaymentNotice".equals(codeString)) 3032 return PAYMENTNOTICE; 3033 if ("PaymentReconciliation".equals(codeString)) 3034 return PAYMENTRECONCILIATION; 3035 if ("Person".equals(codeString)) 3036 return PERSON; 3037 if ("PlanDefinition".equals(codeString)) 3038 return PLANDEFINITION; 3039 if ("Practitioner".equals(codeString)) 3040 return PRACTITIONER; 3041 if ("PractitionerRole".equals(codeString)) 3042 return PRACTITIONERROLE; 3043 if ("Procedure".equals(codeString)) 3044 return PROCEDURE; 3045 if ("ProcedureRequest".equals(codeString)) 3046 return PROCEDUREREQUEST; 3047 if ("ProcessRequest".equals(codeString)) 3048 return PROCESSREQUEST; 3049 if ("ProcessResponse".equals(codeString)) 3050 return PROCESSRESPONSE; 3051 if ("Provenance".equals(codeString)) 3052 return PROVENANCE; 3053 if ("Questionnaire".equals(codeString)) 3054 return QUESTIONNAIRE; 3055 if ("QuestionnaireResponse".equals(codeString)) 3056 return QUESTIONNAIRERESPONSE; 3057 if ("ReferralRequest".equals(codeString)) 3058 return REFERRALREQUEST; 3059 if ("RelatedPerson".equals(codeString)) 3060 return RELATEDPERSON; 3061 if ("RequestGroup".equals(codeString)) 3062 return REQUESTGROUP; 3063 if ("ResearchStudy".equals(codeString)) 3064 return RESEARCHSTUDY; 3065 if ("ResearchSubject".equals(codeString)) 3066 return RESEARCHSUBJECT; 3067 if ("Resource".equals(codeString)) 3068 return RESOURCE; 3069 if ("RiskAssessment".equals(codeString)) 3070 return RISKASSESSMENT; 3071 if ("Schedule".equals(codeString)) 3072 return SCHEDULE; 3073 if ("SearchParameter".equals(codeString)) 3074 return SEARCHPARAMETER; 3075 if ("Sequence".equals(codeString)) 3076 return SEQUENCE; 3077 if ("ServiceDefinition".equals(codeString)) 3078 return SERVICEDEFINITION; 3079 if ("Slot".equals(codeString)) 3080 return SLOT; 3081 if ("Specimen".equals(codeString)) 3082 return SPECIMEN; 3083 if ("StructureDefinition".equals(codeString)) 3084 return STRUCTUREDEFINITION; 3085 if ("StructureMap".equals(codeString)) 3086 return STRUCTUREMAP; 3087 if ("Subscription".equals(codeString)) 3088 return SUBSCRIPTION; 3089 if ("Substance".equals(codeString)) 3090 return SUBSTANCE; 3091 if ("SupplyDelivery".equals(codeString)) 3092 return SUPPLYDELIVERY; 3093 if ("SupplyRequest".equals(codeString)) 3094 return SUPPLYREQUEST; 3095 if ("Task".equals(codeString)) 3096 return TASK; 3097 if ("TestReport".equals(codeString)) 3098 return TESTREPORT; 3099 if ("TestScript".equals(codeString)) 3100 return TESTSCRIPT; 3101 if ("ValueSet".equals(codeString)) 3102 return VALUESET; 3103 if ("VisionPrescription".equals(codeString)) 3104 return VISIONPRESCRIPTION; 3105 if ("Type".equals(codeString)) 3106 return TYPE; 3107 if ("Any".equals(codeString)) 3108 return ANY; 3109 throw new FHIRException("Unknown FHIRAllTypes code '"+codeString+"'"); 3110 } 3111 public String toCode() { 3112 switch (this) { 3113 case ADDRESS: return "Address"; 3114 case AGE: return "Age"; 3115 case ANNOTATION: return "Annotation"; 3116 case ATTACHMENT: return "Attachment"; 3117 case BACKBONEELEMENT: return "BackboneElement"; 3118 case CODEABLECONCEPT: return "CodeableConcept"; 3119 case CODING: return "Coding"; 3120 case CONTACTDETAIL: return "ContactDetail"; 3121 case CONTACTPOINT: return "ContactPoint"; 3122 case CONTRIBUTOR: return "Contributor"; 3123 case COUNT: return "Count"; 3124 case DATAREQUIREMENT: return "DataRequirement"; 3125 case DISTANCE: return "Distance"; 3126 case DOSAGE: return "Dosage"; 3127 case DURATION: return "Duration"; 3128 case ELEMENT: return "Element"; 3129 case ELEMENTDEFINITION: return "ElementDefinition"; 3130 case EXTENSION: return "Extension"; 3131 case HUMANNAME: return "HumanName"; 3132 case IDENTIFIER: return "Identifier"; 3133 case META: return "Meta"; 3134 case MONEY: return "Money"; 3135 case NARRATIVE: return "Narrative"; 3136 case PARAMETERDEFINITION: return "ParameterDefinition"; 3137 case PERIOD: return "Period"; 3138 case QUANTITY: return "Quantity"; 3139 case RANGE: return "Range"; 3140 case RATIO: return "Ratio"; 3141 case REFERENCE: return "Reference"; 3142 case RELATEDARTIFACT: return "RelatedArtifact"; 3143 case SAMPLEDDATA: return "SampledData"; 3144 case SIGNATURE: return "Signature"; 3145 case SIMPLEQUANTITY: return "SimpleQuantity"; 3146 case TIMING: return "Timing"; 3147 case TRIGGERDEFINITION: return "TriggerDefinition"; 3148 case USAGECONTEXT: return "UsageContext"; 3149 case BASE64BINARY: return "base64Binary"; 3150 case BOOLEAN: return "boolean"; 3151 case CODE: return "code"; 3152 case DATE: return "date"; 3153 case DATETIME: return "dateTime"; 3154 case DECIMAL: return "decimal"; 3155 case ID: return "id"; 3156 case INSTANT: return "instant"; 3157 case INTEGER: return "integer"; 3158 case MARKDOWN: return "markdown"; 3159 case OID: return "oid"; 3160 case POSITIVEINT: return "positiveInt"; 3161 case STRING: return "string"; 3162 case TIME: return "time"; 3163 case UNSIGNEDINT: return "unsignedInt"; 3164 case URI: return "uri"; 3165 case UUID: return "uuid"; 3166 case XHTML: return "xhtml"; 3167 case ACCOUNT: return "Account"; 3168 case ACTIVITYDEFINITION: return "ActivityDefinition"; 3169 case ADVERSEEVENT: return "AdverseEvent"; 3170 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 3171 case APPOINTMENT: return "Appointment"; 3172 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 3173 case AUDITEVENT: return "AuditEvent"; 3174 case BASIC: return "Basic"; 3175 case BINARY: return "Binary"; 3176 case BODYSITE: return "BodySite"; 3177 case BUNDLE: return "Bundle"; 3178 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 3179 case CAREPLAN: return "CarePlan"; 3180 case CARETEAM: return "CareTeam"; 3181 case CHARGEITEM: return "ChargeItem"; 3182 case CLAIM: return "Claim"; 3183 case CLAIMRESPONSE: return "ClaimResponse"; 3184 case CLINICALIMPRESSION: return "ClinicalImpression"; 3185 case CODESYSTEM: return "CodeSystem"; 3186 case COMMUNICATION: return "Communication"; 3187 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 3188 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 3189 case COMPOSITION: return "Composition"; 3190 case CONCEPTMAP: return "ConceptMap"; 3191 case CONDITION: return "Condition"; 3192 case CONSENT: return "Consent"; 3193 case CONTRACT: return "Contract"; 3194 case COVERAGE: return "Coverage"; 3195 case DATAELEMENT: return "DataElement"; 3196 case DETECTEDISSUE: return "DetectedIssue"; 3197 case DEVICE: return "Device"; 3198 case DEVICECOMPONENT: return "DeviceComponent"; 3199 case DEVICEMETRIC: return "DeviceMetric"; 3200 case DEVICEREQUEST: return "DeviceRequest"; 3201 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 3202 case DIAGNOSTICREPORT: return "DiagnosticReport"; 3203 case DOCUMENTMANIFEST: return "DocumentManifest"; 3204 case DOCUMENTREFERENCE: return "DocumentReference"; 3205 case DOMAINRESOURCE: return "DomainResource"; 3206 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 3207 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 3208 case ENCOUNTER: return "Encounter"; 3209 case ENDPOINT: return "Endpoint"; 3210 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 3211 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 3212 case EPISODEOFCARE: return "EpisodeOfCare"; 3213 case EXPANSIONPROFILE: return "ExpansionProfile"; 3214 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 3215 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 3216 case FLAG: return "Flag"; 3217 case GOAL: return "Goal"; 3218 case GRAPHDEFINITION: return "GraphDefinition"; 3219 case GROUP: return "Group"; 3220 case GUIDANCERESPONSE: return "GuidanceResponse"; 3221 case HEALTHCARESERVICE: return "HealthcareService"; 3222 case IMAGINGMANIFEST: return "ImagingManifest"; 3223 case IMAGINGSTUDY: return "ImagingStudy"; 3224 case IMMUNIZATION: return "Immunization"; 3225 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 3226 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 3227 case LIBRARY: return "Library"; 3228 case LINKAGE: return "Linkage"; 3229 case LIST: return "List"; 3230 case LOCATION: return "Location"; 3231 case MEASURE: return "Measure"; 3232 case MEASUREREPORT: return "MeasureReport"; 3233 case MEDIA: return "Media"; 3234 case MEDICATION: return "Medication"; 3235 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 3236 case MEDICATIONDISPENSE: return "MedicationDispense"; 3237 case MEDICATIONREQUEST: return "MedicationRequest"; 3238 case MEDICATIONSTATEMENT: return "MedicationStatement"; 3239 case MESSAGEDEFINITION: return "MessageDefinition"; 3240 case MESSAGEHEADER: return "MessageHeader"; 3241 case NAMINGSYSTEM: return "NamingSystem"; 3242 case NUTRITIONORDER: return "NutritionOrder"; 3243 case OBSERVATION: return "Observation"; 3244 case OPERATIONDEFINITION: return "OperationDefinition"; 3245 case OPERATIONOUTCOME: return "OperationOutcome"; 3246 case ORGANIZATION: return "Organization"; 3247 case PARAMETERS: return "Parameters"; 3248 case PATIENT: return "Patient"; 3249 case PAYMENTNOTICE: return "PaymentNotice"; 3250 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 3251 case PERSON: return "Person"; 3252 case PLANDEFINITION: return "PlanDefinition"; 3253 case PRACTITIONER: return "Practitioner"; 3254 case PRACTITIONERROLE: return "PractitionerRole"; 3255 case PROCEDURE: return "Procedure"; 3256 case PROCEDUREREQUEST: return "ProcedureRequest"; 3257 case PROCESSREQUEST: return "ProcessRequest"; 3258 case PROCESSRESPONSE: return "ProcessResponse"; 3259 case PROVENANCE: return "Provenance"; 3260 case QUESTIONNAIRE: return "Questionnaire"; 3261 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 3262 case REFERRALREQUEST: return "ReferralRequest"; 3263 case RELATEDPERSON: return "RelatedPerson"; 3264 case REQUESTGROUP: return "RequestGroup"; 3265 case RESEARCHSTUDY: return "ResearchStudy"; 3266 case RESEARCHSUBJECT: return "ResearchSubject"; 3267 case RESOURCE: return "Resource"; 3268 case RISKASSESSMENT: return "RiskAssessment"; 3269 case SCHEDULE: return "Schedule"; 3270 case SEARCHPARAMETER: return "SearchParameter"; 3271 case SEQUENCE: return "Sequence"; 3272 case SERVICEDEFINITION: return "ServiceDefinition"; 3273 case SLOT: return "Slot"; 3274 case SPECIMEN: return "Specimen"; 3275 case STRUCTUREDEFINITION: return "StructureDefinition"; 3276 case STRUCTUREMAP: return "StructureMap"; 3277 case SUBSCRIPTION: return "Subscription"; 3278 case SUBSTANCE: return "Substance"; 3279 case SUPPLYDELIVERY: return "SupplyDelivery"; 3280 case SUPPLYREQUEST: return "SupplyRequest"; 3281 case TASK: return "Task"; 3282 case TESTREPORT: return "TestReport"; 3283 case TESTSCRIPT: return "TestScript"; 3284 case VALUESET: return "ValueSet"; 3285 case VISIONPRESCRIPTION: return "VisionPrescription"; 3286 case TYPE: return "Type"; 3287 case ANY: return "Any"; 3288 case NULL: return null; 3289 default: return "?"; 3290 } 3291 } 3292 public String getSystem() { 3293 switch (this) { 3294 case ADDRESS: return "http://hl7.org/fhir/data-types"; 3295 case AGE: return "http://hl7.org/fhir/data-types"; 3296 case ANNOTATION: return "http://hl7.org/fhir/data-types"; 3297 case ATTACHMENT: return "http://hl7.org/fhir/data-types"; 3298 case BACKBONEELEMENT: return "http://hl7.org/fhir/data-types"; 3299 case CODEABLECONCEPT: return "http://hl7.org/fhir/data-types"; 3300 case CODING: return "http://hl7.org/fhir/data-types"; 3301 case CONTACTDETAIL: return "http://hl7.org/fhir/data-types"; 3302 case CONTACTPOINT: return "http://hl7.org/fhir/data-types"; 3303 case CONTRIBUTOR: return "http://hl7.org/fhir/data-types"; 3304 case COUNT: return "http://hl7.org/fhir/data-types"; 3305 case DATAREQUIREMENT: return "http://hl7.org/fhir/data-types"; 3306 case DISTANCE: return "http://hl7.org/fhir/data-types"; 3307 case DOSAGE: return "http://hl7.org/fhir/data-types"; 3308 case DURATION: return "http://hl7.org/fhir/data-types"; 3309 case ELEMENT: return "http://hl7.org/fhir/data-types"; 3310 case ELEMENTDEFINITION: return "http://hl7.org/fhir/data-types"; 3311 case EXTENSION: return "http://hl7.org/fhir/data-types"; 3312 case HUMANNAME: return "http://hl7.org/fhir/data-types"; 3313 case IDENTIFIER: return "http://hl7.org/fhir/data-types"; 3314 case META: return "http://hl7.org/fhir/data-types"; 3315 case MONEY: return "http://hl7.org/fhir/data-types"; 3316 case NARRATIVE: return "http://hl7.org/fhir/data-types"; 3317 case PARAMETERDEFINITION: return "http://hl7.org/fhir/data-types"; 3318 case PERIOD: return "http://hl7.org/fhir/data-types"; 3319 case QUANTITY: return "http://hl7.org/fhir/data-types"; 3320 case RANGE: return "http://hl7.org/fhir/data-types"; 3321 case RATIO: return "http://hl7.org/fhir/data-types"; 3322 case REFERENCE: return "http://hl7.org/fhir/data-types"; 3323 case RELATEDARTIFACT: return "http://hl7.org/fhir/data-types"; 3324 case SAMPLEDDATA: return "http://hl7.org/fhir/data-types"; 3325 case SIGNATURE: return "http://hl7.org/fhir/data-types"; 3326 case SIMPLEQUANTITY: return "http://hl7.org/fhir/data-types"; 3327 case TIMING: return "http://hl7.org/fhir/data-types"; 3328 case TRIGGERDEFINITION: return "http://hl7.org/fhir/data-types"; 3329 case USAGECONTEXT: return "http://hl7.org/fhir/data-types"; 3330 case BASE64BINARY: return "http://hl7.org/fhir/data-types"; 3331 case BOOLEAN: return "http://hl7.org/fhir/data-types"; 3332 case CODE: return "http://hl7.org/fhir/data-types"; 3333 case DATE: return "http://hl7.org/fhir/data-types"; 3334 case DATETIME: return "http://hl7.org/fhir/data-types"; 3335 case DECIMAL: return "http://hl7.org/fhir/data-types"; 3336 case ID: return "http://hl7.org/fhir/data-types"; 3337 case INSTANT: return "http://hl7.org/fhir/data-types"; 3338 case INTEGER: return "http://hl7.org/fhir/data-types"; 3339 case MARKDOWN: return "http://hl7.org/fhir/data-types"; 3340 case OID: return "http://hl7.org/fhir/data-types"; 3341 case POSITIVEINT: return "http://hl7.org/fhir/data-types"; 3342 case STRING: return "http://hl7.org/fhir/data-types"; 3343 case TIME: return "http://hl7.org/fhir/data-types"; 3344 case UNSIGNEDINT: return "http://hl7.org/fhir/data-types"; 3345 case URI: return "http://hl7.org/fhir/data-types"; 3346 case UUID: return "http://hl7.org/fhir/data-types"; 3347 case XHTML: return "http://hl7.org/fhir/data-types"; 3348 case ACCOUNT: return "http://hl7.org/fhir/resource-types"; 3349 case ACTIVITYDEFINITION: return "http://hl7.org/fhir/resource-types"; 3350 case ADVERSEEVENT: return "http://hl7.org/fhir/resource-types"; 3351 case ALLERGYINTOLERANCE: return "http://hl7.org/fhir/resource-types"; 3352 case APPOINTMENT: return "http://hl7.org/fhir/resource-types"; 3353 case APPOINTMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 3354 case AUDITEVENT: return "http://hl7.org/fhir/resource-types"; 3355 case BASIC: return "http://hl7.org/fhir/resource-types"; 3356 case BINARY: return "http://hl7.org/fhir/resource-types"; 3357 case BODYSITE: return "http://hl7.org/fhir/resource-types"; 3358 case BUNDLE: return "http://hl7.org/fhir/resource-types"; 3359 case CAPABILITYSTATEMENT: return "http://hl7.org/fhir/resource-types"; 3360 case CAREPLAN: return "http://hl7.org/fhir/resource-types"; 3361 case CARETEAM: return "http://hl7.org/fhir/resource-types"; 3362 case CHARGEITEM: return "http://hl7.org/fhir/resource-types"; 3363 case CLAIM: return "http://hl7.org/fhir/resource-types"; 3364 case CLAIMRESPONSE: return "http://hl7.org/fhir/resource-types"; 3365 case CLINICALIMPRESSION: return "http://hl7.org/fhir/resource-types"; 3366 case CODESYSTEM: return "http://hl7.org/fhir/resource-types"; 3367 case COMMUNICATION: return "http://hl7.org/fhir/resource-types"; 3368 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 3369 case COMPARTMENTDEFINITION: return "http://hl7.org/fhir/resource-types"; 3370 case COMPOSITION: return "http://hl7.org/fhir/resource-types"; 3371 case CONCEPTMAP: return "http://hl7.org/fhir/resource-types"; 3372 case CONDITION: return "http://hl7.org/fhir/resource-types"; 3373 case CONSENT: return "http://hl7.org/fhir/resource-types"; 3374 case CONTRACT: return "http://hl7.org/fhir/resource-types"; 3375 case COVERAGE: return "http://hl7.org/fhir/resource-types"; 3376 case DATAELEMENT: return "http://hl7.org/fhir/resource-types"; 3377 case DETECTEDISSUE: return "http://hl7.org/fhir/resource-types"; 3378 case DEVICE: return "http://hl7.org/fhir/resource-types"; 3379 case DEVICECOMPONENT: return "http://hl7.org/fhir/resource-types"; 3380 case DEVICEMETRIC: return "http://hl7.org/fhir/resource-types"; 3381 case DEVICEREQUEST: return "http://hl7.org/fhir/resource-types"; 3382 case DEVICEUSESTATEMENT: return "http://hl7.org/fhir/resource-types"; 3383 case DIAGNOSTICREPORT: return "http://hl7.org/fhir/resource-types"; 3384 case DOCUMENTMANIFEST: return "http://hl7.org/fhir/resource-types"; 3385 case DOCUMENTREFERENCE: return "http://hl7.org/fhir/resource-types"; 3386 case DOMAINRESOURCE: return "http://hl7.org/fhir/resource-types"; 3387 case ELIGIBILITYREQUEST: return "http://hl7.org/fhir/resource-types"; 3388 case ELIGIBILITYRESPONSE: return "http://hl7.org/fhir/resource-types"; 3389 case ENCOUNTER: return "http://hl7.org/fhir/resource-types"; 3390 case ENDPOINT: return "http://hl7.org/fhir/resource-types"; 3391 case ENROLLMENTREQUEST: return "http://hl7.org/fhir/resource-types"; 3392 case ENROLLMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 3393 case EPISODEOFCARE: return "http://hl7.org/fhir/resource-types"; 3394 case EXPANSIONPROFILE: return "http://hl7.org/fhir/resource-types"; 3395 case EXPLANATIONOFBENEFIT: return "http://hl7.org/fhir/resource-types"; 3396 case FAMILYMEMBERHISTORY: return "http://hl7.org/fhir/resource-types"; 3397 case FLAG: return "http://hl7.org/fhir/resource-types"; 3398 case GOAL: return "http://hl7.org/fhir/resource-types"; 3399 case GRAPHDEFINITION: return "http://hl7.org/fhir/resource-types"; 3400 case GROUP: return "http://hl7.org/fhir/resource-types"; 3401 case GUIDANCERESPONSE: return "http://hl7.org/fhir/resource-types"; 3402 case HEALTHCARESERVICE: return "http://hl7.org/fhir/resource-types"; 3403 case IMAGINGMANIFEST: return "http://hl7.org/fhir/resource-types"; 3404 case IMAGINGSTUDY: return "http://hl7.org/fhir/resource-types"; 3405 case IMMUNIZATION: return "http://hl7.org/fhir/resource-types"; 3406 case IMMUNIZATIONRECOMMENDATION: return "http://hl7.org/fhir/resource-types"; 3407 case IMPLEMENTATIONGUIDE: return "http://hl7.org/fhir/resource-types"; 3408 case LIBRARY: return "http://hl7.org/fhir/resource-types"; 3409 case LINKAGE: return "http://hl7.org/fhir/resource-types"; 3410 case LIST: return "http://hl7.org/fhir/resource-types"; 3411 case LOCATION: return "http://hl7.org/fhir/resource-types"; 3412 case MEASURE: return "http://hl7.org/fhir/resource-types"; 3413 case MEASUREREPORT: return "http://hl7.org/fhir/resource-types"; 3414 case MEDIA: return "http://hl7.org/fhir/resource-types"; 3415 case MEDICATION: return "http://hl7.org/fhir/resource-types"; 3416 case MEDICATIONADMINISTRATION: return "http://hl7.org/fhir/resource-types"; 3417 case MEDICATIONDISPENSE: return "http://hl7.org/fhir/resource-types"; 3418 case MEDICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 3419 case MEDICATIONSTATEMENT: return "http://hl7.org/fhir/resource-types"; 3420 case MESSAGEDEFINITION: return "http://hl7.org/fhir/resource-types"; 3421 case MESSAGEHEADER: return "http://hl7.org/fhir/resource-types"; 3422 case NAMINGSYSTEM: return "http://hl7.org/fhir/resource-types"; 3423 case NUTRITIONORDER: return "http://hl7.org/fhir/resource-types"; 3424 case OBSERVATION: return "http://hl7.org/fhir/resource-types"; 3425 case OPERATIONDEFINITION: return "http://hl7.org/fhir/resource-types"; 3426 case OPERATIONOUTCOME: return "http://hl7.org/fhir/resource-types"; 3427 case ORGANIZATION: return "http://hl7.org/fhir/resource-types"; 3428 case PARAMETERS: return "http://hl7.org/fhir/resource-types"; 3429 case PATIENT: return "http://hl7.org/fhir/resource-types"; 3430 case PAYMENTNOTICE: return "http://hl7.org/fhir/resource-types"; 3431 case PAYMENTRECONCILIATION: return "http://hl7.org/fhir/resource-types"; 3432 case PERSON: return "http://hl7.org/fhir/resource-types"; 3433 case PLANDEFINITION: return "http://hl7.org/fhir/resource-types"; 3434 case PRACTITIONER: return "http://hl7.org/fhir/resource-types"; 3435 case PRACTITIONERROLE: return "http://hl7.org/fhir/resource-types"; 3436 case PROCEDURE: return "http://hl7.org/fhir/resource-types"; 3437 case PROCEDUREREQUEST: return "http://hl7.org/fhir/resource-types"; 3438 case PROCESSREQUEST: return "http://hl7.org/fhir/resource-types"; 3439 case PROCESSRESPONSE: return "http://hl7.org/fhir/resource-types"; 3440 case PROVENANCE: return "http://hl7.org/fhir/resource-types"; 3441 case QUESTIONNAIRE: return "http://hl7.org/fhir/resource-types"; 3442 case QUESTIONNAIRERESPONSE: return "http://hl7.org/fhir/resource-types"; 3443 case REFERRALREQUEST: return "http://hl7.org/fhir/resource-types"; 3444 case RELATEDPERSON: return "http://hl7.org/fhir/resource-types"; 3445 case REQUESTGROUP: return "http://hl7.org/fhir/resource-types"; 3446 case RESEARCHSTUDY: return "http://hl7.org/fhir/resource-types"; 3447 case RESEARCHSUBJECT: return "http://hl7.org/fhir/resource-types"; 3448 case RESOURCE: return "http://hl7.org/fhir/resource-types"; 3449 case RISKASSESSMENT: return "http://hl7.org/fhir/resource-types"; 3450 case SCHEDULE: return "http://hl7.org/fhir/resource-types"; 3451 case SEARCHPARAMETER: return "http://hl7.org/fhir/resource-types"; 3452 case SEQUENCE: return "http://hl7.org/fhir/resource-types"; 3453 case SERVICEDEFINITION: return "http://hl7.org/fhir/resource-types"; 3454 case SLOT: return "http://hl7.org/fhir/resource-types"; 3455 case SPECIMEN: return "http://hl7.org/fhir/resource-types"; 3456 case STRUCTUREDEFINITION: return "http://hl7.org/fhir/resource-types"; 3457 case STRUCTUREMAP: return "http://hl7.org/fhir/resource-types"; 3458 case SUBSCRIPTION: return "http://hl7.org/fhir/resource-types"; 3459 case SUBSTANCE: return "http://hl7.org/fhir/resource-types"; 3460 case SUPPLYDELIVERY: return "http://hl7.org/fhir/resource-types"; 3461 case SUPPLYREQUEST: return "http://hl7.org/fhir/resource-types"; 3462 case TASK: return "http://hl7.org/fhir/resource-types"; 3463 case TESTREPORT: return "http://hl7.org/fhir/resource-types"; 3464 case TESTSCRIPT: return "http://hl7.org/fhir/resource-types"; 3465 case VALUESET: return "http://hl7.org/fhir/resource-types"; 3466 case VISIONPRESCRIPTION: return "http://hl7.org/fhir/resource-types"; 3467 case TYPE: return "http://hl7.org/fhir/abstract-types"; 3468 case ANY: return "http://hl7.org/fhir/abstract-types"; 3469 case NULL: return null; 3470 default: return "?"; 3471 } 3472 } 3473 public String getDefinition() { 3474 switch (this) { 3475 case ADDRESS: return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 3476 case AGE: return "A duration of time during which an organism (or a process) has existed."; 3477 case ANNOTATION: return "A text note which also contains information about who made the statement and when."; 3478 case ATTACHMENT: return "For referring to data content defined in other formats."; 3479 case BACKBONEELEMENT: return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 3480 case CODEABLECONCEPT: return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 3481 case CODING: return "A reference to a code defined by a terminology system."; 3482 case CONTACTDETAIL: return "Specifies contact information for a person or organization."; 3483 case CONTACTPOINT: return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 3484 case CONTRIBUTOR: return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 3485 case COUNT: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 3486 case DATAREQUIREMENT: return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 3487 case DISTANCE: return "A length - a value with a unit that is a physical distance."; 3488 case DOSAGE: return "Indicates how the medication is/was taken or should be taken by the patient."; 3489 case DURATION: return "A length of time."; 3490 case ELEMENT: return "Base definition for all elements in a resource."; 3491 case ELEMENTDEFINITION: return "Captures constraints on each element within the resource, profile, or extension."; 3492 case EXTENSION: return "Optional Extension Element - found in all resources."; 3493 case HUMANNAME: return "A human's name with the ability to identify parts and usage."; 3494 case IDENTIFIER: return "A technical identifier - identifies some entity uniquely and unambiguously."; 3495 case META: return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource."; 3496 case MONEY: return "An amount of economic utility in some recognized currency."; 3497 case NARRATIVE: return "A human-readable formatted text, including images."; 3498 case PARAMETERDEFINITION: return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 3499 case PERIOD: return "A time period defined by a start and end date and optionally time."; 3500 case QUANTITY: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 3501 case RANGE: return "A set of ordered Quantities defined by a low and high limit."; 3502 case RATIO: return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 3503 case REFERENCE: return "A reference from one resource to another."; 3504 case RELATEDARTIFACT: return "Related artifacts such as additional documentation, justification, or bibliographic references."; 3505 case SAMPLEDDATA: return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 3506 case SIGNATURE: return "A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities."; 3507 case SIMPLEQUANTITY: return ""; 3508 case TIMING: return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 3509 case TRIGGERDEFINITION: return "A description of a triggering event."; 3510 case USAGECONTEXT: return "Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 3511 case BASE64BINARY: return "A stream of bytes"; 3512 case BOOLEAN: return "Value of \"true\" or \"false\""; 3513 case CODE: return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 3514 case DATE: return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 3515 case DATETIME: return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 3516 case DECIMAL: return "A rational number with implicit precision"; 3517 case ID: return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 3518 case INSTANT: return "An instant in time - known at least to the second"; 3519 case INTEGER: return "A whole number"; 3520 case MARKDOWN: return "A string that may contain markdown syntax for optional processing by a mark down presentation engine"; 3521 case OID: return "An OID represented as a URI"; 3522 case POSITIVEINT: return "An integer with a value that is positive (e.g. >0)"; 3523 case STRING: return "A sequence of Unicode characters"; 3524 case TIME: return "A time during the day, with no date specified"; 3525 case UNSIGNEDINT: return "An integer with a value that is not negative (e.g. >= 0)"; 3526 case URI: return "String of characters used to identify a name or a resource"; 3527 case UUID: return "A UUID, represented as a URI"; 3528 case XHTML: return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 3529 case ACCOUNT: return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 3530 case ACTIVITYDEFINITION: return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 3531 case ADVERSEEVENT: return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 3532 case ALLERGYINTOLERANCE: return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 3533 case APPOINTMENT: return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 3534 case APPOINTMENTRESPONSE: return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 3535 case AUDITEVENT: return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 3536 case BASIC: return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 3537 case BINARY: return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 3538 case BODYSITE: return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 3539 case BUNDLE: return "A container for a collection of resources."; 3540 case CAPABILITYSTATEMENT: return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 3541 case CAREPLAN: return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 3542 case CARETEAM: return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 3543 case CHARGEITEM: return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 3544 case CLAIM: return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 3545 case CLAIMRESPONSE: return "This resource provides the adjudication details from the processing of a Claim resource."; 3546 case CLINICALIMPRESSION: return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 3547 case CODESYSTEM: return "A code system resource specifies a set of codes drawn from one or more code systems."; 3548 case COMMUNICATION: return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 3549 case COMMUNICATIONREQUEST: return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 3550 case COMPARTMENTDEFINITION: return "A compartment definition that defines how resources are accessed on a server."; 3551 case COMPOSITION: return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 3552 case CONCEPTMAP: return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 3553 case CONDITION: return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 3554 case CONSENT: return "A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 3555 case CONTRACT: return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 3556 case COVERAGE: return "Financial instrument which may be used to reimburse or pay for health care products and services."; 3557 case DATAELEMENT: return "The formal description of a single piece of information that can be gathered and reported."; 3558 case DETECTEDISSUE: return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 3559 case DEVICE: return "This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 3560 case DEVICECOMPONENT: return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 3561 case DEVICEMETRIC: return "Describes a measurement, calculation or setting capability of a medical device."; 3562 case DEVICEREQUEST: return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 3563 case DEVICEUSESTATEMENT: return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 3564 case DIAGNOSTICREPORT: return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 3565 case DOCUMENTMANIFEST: return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 3566 case DOCUMENTREFERENCE: return "A reference to a document."; 3567 case DOMAINRESOURCE: return "A resource that includes narrative, extensions, and contained resources."; 3568 case ELIGIBILITYREQUEST: return "The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 3569 case ELIGIBILITYRESPONSE: return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 3570 case ENCOUNTER: return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 3571 case ENDPOINT: return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 3572 case ENROLLMENTREQUEST: return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 3573 case ENROLLMENTRESPONSE: return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 3574 case EPISODEOFCARE: return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 3575 case EXPANSIONPROFILE: return "Resource to define constraints on the Expansion of a FHIR ValueSet."; 3576 case EXPLANATIONOFBENEFIT: return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 3577 case FAMILYMEMBERHISTORY: return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 3578 case FLAG: return "Prospective warnings of potential issues when providing care to the patient."; 3579 case GOAL: return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 3580 case GRAPHDEFINITION: return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 3581 case GROUP: return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 3582 case GUIDANCERESPONSE: return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 3583 case HEALTHCARESERVICE: return "The details of a healthcare service available at a location."; 3584 case IMAGINGMANIFEST: return "A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection."; 3585 case IMAGINGSTUDY: return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 3586 case IMMUNIZATION: return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 3587 case IMMUNIZATIONRECOMMENDATION: return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 3588 case IMPLEMENTATIONGUIDE: return "A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 3589 case LIBRARY: return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 3590 case LINKAGE: return "Identifies two or more records (resource instances) that are referring to the same real-world \"occurrence\"."; 3591 case LIST: return "A set of information summarized from a list of other resources."; 3592 case LOCATION: return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 3593 case MEASURE: return "The Measure resource provides the definition of a quality measure."; 3594 case MEASUREREPORT: return "The MeasureReport resource contains the results of evaluating a measure."; 3595 case MEDIA: return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 3596 case MEDICATION: return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 3597 case MEDICATIONADMINISTRATION: return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 3598 case MEDICATIONDISPENSE: return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 3599 case MEDICATIONREQUEST: return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 3600 case MEDICATIONSTATEMENT: return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 3601 case MESSAGEDEFINITION: return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 3602 case MESSAGEHEADER: return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 3603 case NAMINGSYSTEM: return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 3604 case NUTRITIONORDER: return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 3605 case OBSERVATION: return "Measurements and simple assertions made about a patient, device or other subject."; 3606 case OPERATIONDEFINITION: return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 3607 case OPERATIONOUTCOME: return "A collection of error, warning or information messages that result from a system action."; 3608 case ORGANIZATION: return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 3609 case PARAMETERS: return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 3610 case PATIENT: return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 3611 case PAYMENTNOTICE: return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 3612 case PAYMENTRECONCILIATION: return "This resource provides payment details and claim references supporting a bulk payment."; 3613 case PERSON: return "Demographics and administrative information about a person independent of a specific health-related context."; 3614 case PLANDEFINITION: return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 3615 case PRACTITIONER: return "A person who is directly or indirectly involved in the provisioning of healthcare."; 3616 case PRACTITIONERROLE: return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 3617 case PROCEDURE: return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 3618 case PROCEDUREREQUEST: return "A record of a request for diagnostic investigations, treatments, or operations to be performed."; 3619 case PROCESSREQUEST: return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 3620 case PROCESSRESPONSE: return "This resource provides processing status, errors and notes from the processing of a resource."; 3621 case PROVENANCE: return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 3622 case QUESTIONNAIRE: return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 3623 case QUESTIONNAIRERESPONSE: return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 3624 case REFERRALREQUEST: return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 3625 case RELATEDPERSON: return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 3626 case REQUESTGROUP: return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 3627 case RESEARCHSTUDY: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 3628 case RESEARCHSUBJECT: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 3629 case RESOURCE: return "This is the base resource type for everything."; 3630 case RISKASSESSMENT: return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 3631 case SCHEDULE: return "A container for slots of time that may be available for booking appointments."; 3632 case SEARCHPARAMETER: return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 3633 case SEQUENCE: return "Raw data describing a biological sequence."; 3634 case SERVICEDEFINITION: return "The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking."; 3635 case SLOT: return "A slot of time on a schedule that may be available for booking appointments."; 3636 case SPECIMEN: return "A sample to be used for analysis."; 3637 case STRUCTUREDEFINITION: return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 3638 case STRUCTUREMAP: return "A Map of relationships between 2 structures that can be used to transform data."; 3639 case SUBSCRIPTION: return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 3640 case SUBSTANCE: return "A homogeneous material with a definite composition."; 3641 case SUPPLYDELIVERY: return "Record of delivery of what is supplied."; 3642 case SUPPLYREQUEST: return "A record of a request for a medication, substance or device used in the healthcare setting."; 3643 case TASK: return "A task to be performed."; 3644 case TESTREPORT: return "A summary of information based on the results of executing a TestScript."; 3645 case TESTSCRIPT: return "A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 3646 case VALUESET: return "A value set specifies a set of codes drawn from one or more code systems."; 3647 case VISIONPRESCRIPTION: return "An authorization for the supply of glasses and/or contact lenses to a patient."; 3648 case TYPE: return "A place holder that means any kind of data type"; 3649 case ANY: return "A place holder that means any kind of resource"; 3650 case NULL: return null; 3651 default: return "?"; 3652 } 3653 } 3654 public String getDisplay() { 3655 switch (this) { 3656 case ADDRESS: return "Address"; 3657 case AGE: return "Age"; 3658 case ANNOTATION: return "Annotation"; 3659 case ATTACHMENT: return "Attachment"; 3660 case BACKBONEELEMENT: return "BackboneElement"; 3661 case CODEABLECONCEPT: return "CodeableConcept"; 3662 case CODING: return "Coding"; 3663 case CONTACTDETAIL: return "ContactDetail"; 3664 case CONTACTPOINT: return "ContactPoint"; 3665 case CONTRIBUTOR: return "Contributor"; 3666 case COUNT: return "Count"; 3667 case DATAREQUIREMENT: return "DataRequirement"; 3668 case DISTANCE: return "Distance"; 3669 case DOSAGE: return "Dosage"; 3670 case DURATION: return "Duration"; 3671 case ELEMENT: return "Element"; 3672 case ELEMENTDEFINITION: return "ElementDefinition"; 3673 case EXTENSION: return "Extension"; 3674 case HUMANNAME: return "HumanName"; 3675 case IDENTIFIER: return "Identifier"; 3676 case META: return "Meta"; 3677 case MONEY: return "Money"; 3678 case NARRATIVE: return "Narrative"; 3679 case PARAMETERDEFINITION: return "ParameterDefinition"; 3680 case PERIOD: return "Period"; 3681 case QUANTITY: return "Quantity"; 3682 case RANGE: return "Range"; 3683 case RATIO: return "Ratio"; 3684 case REFERENCE: return "Reference"; 3685 case RELATEDARTIFACT: return "RelatedArtifact"; 3686 case SAMPLEDDATA: return "SampledData"; 3687 case SIGNATURE: return "Signature"; 3688 case SIMPLEQUANTITY: return "SimpleQuantity"; 3689 case TIMING: return "Timing"; 3690 case TRIGGERDEFINITION: return "TriggerDefinition"; 3691 case USAGECONTEXT: return "UsageContext"; 3692 case BASE64BINARY: return "base64Binary"; 3693 case BOOLEAN: return "boolean"; 3694 case CODE: return "code"; 3695 case DATE: return "date"; 3696 case DATETIME: return "dateTime"; 3697 case DECIMAL: return "decimal"; 3698 case ID: return "id"; 3699 case INSTANT: return "instant"; 3700 case INTEGER: return "integer"; 3701 case MARKDOWN: return "markdown"; 3702 case OID: return "oid"; 3703 case POSITIVEINT: return "positiveInt"; 3704 case STRING: return "string"; 3705 case TIME: return "time"; 3706 case UNSIGNEDINT: return "unsignedInt"; 3707 case URI: return "uri"; 3708 case UUID: return "uuid"; 3709 case XHTML: return "XHTML"; 3710 case ACCOUNT: return "Account"; 3711 case ACTIVITYDEFINITION: return "ActivityDefinition"; 3712 case ADVERSEEVENT: return "AdverseEvent"; 3713 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 3714 case APPOINTMENT: return "Appointment"; 3715 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 3716 case AUDITEVENT: return "AuditEvent"; 3717 case BASIC: return "Basic"; 3718 case BINARY: return "Binary"; 3719 case BODYSITE: return "BodySite"; 3720 case BUNDLE: return "Bundle"; 3721 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 3722 case CAREPLAN: return "CarePlan"; 3723 case CARETEAM: return "CareTeam"; 3724 case CHARGEITEM: return "ChargeItem"; 3725 case CLAIM: return "Claim"; 3726 case CLAIMRESPONSE: return "ClaimResponse"; 3727 case CLINICALIMPRESSION: return "ClinicalImpression"; 3728 case CODESYSTEM: return "CodeSystem"; 3729 case COMMUNICATION: return "Communication"; 3730 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 3731 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 3732 case COMPOSITION: return "Composition"; 3733 case CONCEPTMAP: return "ConceptMap"; 3734 case CONDITION: return "Condition"; 3735 case CONSENT: return "Consent"; 3736 case CONTRACT: return "Contract"; 3737 case COVERAGE: return "Coverage"; 3738 case DATAELEMENT: return "DataElement"; 3739 case DETECTEDISSUE: return "DetectedIssue"; 3740 case DEVICE: return "Device"; 3741 case DEVICECOMPONENT: return "DeviceComponent"; 3742 case DEVICEMETRIC: return "DeviceMetric"; 3743 case DEVICEREQUEST: return "DeviceRequest"; 3744 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 3745 case DIAGNOSTICREPORT: return "DiagnosticReport"; 3746 case DOCUMENTMANIFEST: return "DocumentManifest"; 3747 case DOCUMENTREFERENCE: return "DocumentReference"; 3748 case DOMAINRESOURCE: return "DomainResource"; 3749 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 3750 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 3751 case ENCOUNTER: return "Encounter"; 3752 case ENDPOINT: return "Endpoint"; 3753 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 3754 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 3755 case EPISODEOFCARE: return "EpisodeOfCare"; 3756 case EXPANSIONPROFILE: return "ExpansionProfile"; 3757 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 3758 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 3759 case FLAG: return "Flag"; 3760 case GOAL: return "Goal"; 3761 case GRAPHDEFINITION: return "GraphDefinition"; 3762 case GROUP: return "Group"; 3763 case GUIDANCERESPONSE: return "GuidanceResponse"; 3764 case HEALTHCARESERVICE: return "HealthcareService"; 3765 case IMAGINGMANIFEST: return "ImagingManifest"; 3766 case IMAGINGSTUDY: return "ImagingStudy"; 3767 case IMMUNIZATION: return "Immunization"; 3768 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 3769 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 3770 case LIBRARY: return "Library"; 3771 case LINKAGE: return "Linkage"; 3772 case LIST: return "List"; 3773 case LOCATION: return "Location"; 3774 case MEASURE: return "Measure"; 3775 case MEASUREREPORT: return "MeasureReport"; 3776 case MEDIA: return "Media"; 3777 case MEDICATION: return "Medication"; 3778 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 3779 case MEDICATIONDISPENSE: return "MedicationDispense"; 3780 case MEDICATIONREQUEST: return "MedicationRequest"; 3781 case MEDICATIONSTATEMENT: return "MedicationStatement"; 3782 case MESSAGEDEFINITION: return "MessageDefinition"; 3783 case MESSAGEHEADER: return "MessageHeader"; 3784 case NAMINGSYSTEM: return "NamingSystem"; 3785 case NUTRITIONORDER: return "NutritionOrder"; 3786 case OBSERVATION: return "Observation"; 3787 case OPERATIONDEFINITION: return "OperationDefinition"; 3788 case OPERATIONOUTCOME: return "OperationOutcome"; 3789 case ORGANIZATION: return "Organization"; 3790 case PARAMETERS: return "Parameters"; 3791 case PATIENT: return "Patient"; 3792 case PAYMENTNOTICE: return "PaymentNotice"; 3793 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 3794 case PERSON: return "Person"; 3795 case PLANDEFINITION: return "PlanDefinition"; 3796 case PRACTITIONER: return "Practitioner"; 3797 case PRACTITIONERROLE: return "PractitionerRole"; 3798 case PROCEDURE: return "Procedure"; 3799 case PROCEDUREREQUEST: return "ProcedureRequest"; 3800 case PROCESSREQUEST: return "ProcessRequest"; 3801 case PROCESSRESPONSE: return "ProcessResponse"; 3802 case PROVENANCE: return "Provenance"; 3803 case QUESTIONNAIRE: return "Questionnaire"; 3804 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 3805 case REFERRALREQUEST: return "ReferralRequest"; 3806 case RELATEDPERSON: return "RelatedPerson"; 3807 case REQUESTGROUP: return "RequestGroup"; 3808 case RESEARCHSTUDY: return "ResearchStudy"; 3809 case RESEARCHSUBJECT: return "ResearchSubject"; 3810 case RESOURCE: return "Resource"; 3811 case RISKASSESSMENT: return "RiskAssessment"; 3812 case SCHEDULE: return "Schedule"; 3813 case SEARCHPARAMETER: return "SearchParameter"; 3814 case SEQUENCE: return "Sequence"; 3815 case SERVICEDEFINITION: return "ServiceDefinition"; 3816 case SLOT: return "Slot"; 3817 case SPECIMEN: return "Specimen"; 3818 case STRUCTUREDEFINITION: return "StructureDefinition"; 3819 case STRUCTUREMAP: return "StructureMap"; 3820 case SUBSCRIPTION: return "Subscription"; 3821 case SUBSTANCE: return "Substance"; 3822 case SUPPLYDELIVERY: return "SupplyDelivery"; 3823 case SUPPLYREQUEST: return "SupplyRequest"; 3824 case TASK: return "Task"; 3825 case TESTREPORT: return "TestReport"; 3826 case TESTSCRIPT: return "TestScript"; 3827 case VALUESET: return "ValueSet"; 3828 case VISIONPRESCRIPTION: return "VisionPrescription"; 3829 case TYPE: return "Type"; 3830 case ANY: return "Any"; 3831 case NULL: return null; 3832 default: return "?"; 3833 } 3834 } 3835 } 3836 3837 public static class FHIRAllTypesEnumFactory implements EnumFactory<FHIRAllTypes> { 3838 public FHIRAllTypes fromCode(String codeString) throws IllegalArgumentException { 3839 if (codeString == null || "".equals(codeString)) 3840 if (codeString == null || "".equals(codeString)) 3841 return null; 3842 if ("Address".equals(codeString)) 3843 return FHIRAllTypes.ADDRESS; 3844 if ("Age".equals(codeString)) 3845 return FHIRAllTypes.AGE; 3846 if ("Annotation".equals(codeString)) 3847 return FHIRAllTypes.ANNOTATION; 3848 if ("Attachment".equals(codeString)) 3849 return FHIRAllTypes.ATTACHMENT; 3850 if ("BackboneElement".equals(codeString)) 3851 return FHIRAllTypes.BACKBONEELEMENT; 3852 if ("CodeableConcept".equals(codeString)) 3853 return FHIRAllTypes.CODEABLECONCEPT; 3854 if ("Coding".equals(codeString)) 3855 return FHIRAllTypes.CODING; 3856 if ("ContactDetail".equals(codeString)) 3857 return FHIRAllTypes.CONTACTDETAIL; 3858 if ("ContactPoint".equals(codeString)) 3859 return FHIRAllTypes.CONTACTPOINT; 3860 if ("Contributor".equals(codeString)) 3861 return FHIRAllTypes.CONTRIBUTOR; 3862 if ("Count".equals(codeString)) 3863 return FHIRAllTypes.COUNT; 3864 if ("DataRequirement".equals(codeString)) 3865 return FHIRAllTypes.DATAREQUIREMENT; 3866 if ("Distance".equals(codeString)) 3867 return FHIRAllTypes.DISTANCE; 3868 if ("Dosage".equals(codeString)) 3869 return FHIRAllTypes.DOSAGE; 3870 if ("Duration".equals(codeString)) 3871 return FHIRAllTypes.DURATION; 3872 if ("Element".equals(codeString)) 3873 return FHIRAllTypes.ELEMENT; 3874 if ("ElementDefinition".equals(codeString)) 3875 return FHIRAllTypes.ELEMENTDEFINITION; 3876 if ("Extension".equals(codeString)) 3877 return FHIRAllTypes.EXTENSION; 3878 if ("HumanName".equals(codeString)) 3879 return FHIRAllTypes.HUMANNAME; 3880 if ("Identifier".equals(codeString)) 3881 return FHIRAllTypes.IDENTIFIER; 3882 if ("Meta".equals(codeString)) 3883 return FHIRAllTypes.META; 3884 if ("Money".equals(codeString)) 3885 return FHIRAllTypes.MONEY; 3886 if ("Narrative".equals(codeString)) 3887 return FHIRAllTypes.NARRATIVE; 3888 if ("ParameterDefinition".equals(codeString)) 3889 return FHIRAllTypes.PARAMETERDEFINITION; 3890 if ("Period".equals(codeString)) 3891 return FHIRAllTypes.PERIOD; 3892 if ("Quantity".equals(codeString)) 3893 return FHIRAllTypes.QUANTITY; 3894 if ("Range".equals(codeString)) 3895 return FHIRAllTypes.RANGE; 3896 if ("Ratio".equals(codeString)) 3897 return FHIRAllTypes.RATIO; 3898 if ("Reference".equals(codeString)) 3899 return FHIRAllTypes.REFERENCE; 3900 if ("RelatedArtifact".equals(codeString)) 3901 return FHIRAllTypes.RELATEDARTIFACT; 3902 if ("SampledData".equals(codeString)) 3903 return FHIRAllTypes.SAMPLEDDATA; 3904 if ("Signature".equals(codeString)) 3905 return FHIRAllTypes.SIGNATURE; 3906 if ("SimpleQuantity".equals(codeString)) 3907 return FHIRAllTypes.SIMPLEQUANTITY; 3908 if ("Timing".equals(codeString)) 3909 return FHIRAllTypes.TIMING; 3910 if ("TriggerDefinition".equals(codeString)) 3911 return FHIRAllTypes.TRIGGERDEFINITION; 3912 if ("UsageContext".equals(codeString)) 3913 return FHIRAllTypes.USAGECONTEXT; 3914 if ("base64Binary".equals(codeString)) 3915 return FHIRAllTypes.BASE64BINARY; 3916 if ("boolean".equals(codeString)) 3917 return FHIRAllTypes.BOOLEAN; 3918 if ("code".equals(codeString)) 3919 return FHIRAllTypes.CODE; 3920 if ("date".equals(codeString)) 3921 return FHIRAllTypes.DATE; 3922 if ("dateTime".equals(codeString)) 3923 return FHIRAllTypes.DATETIME; 3924 if ("decimal".equals(codeString)) 3925 return FHIRAllTypes.DECIMAL; 3926 if ("id".equals(codeString)) 3927 return FHIRAllTypes.ID; 3928 if ("instant".equals(codeString)) 3929 return FHIRAllTypes.INSTANT; 3930 if ("integer".equals(codeString)) 3931 return FHIRAllTypes.INTEGER; 3932 if ("markdown".equals(codeString)) 3933 return FHIRAllTypes.MARKDOWN; 3934 if ("oid".equals(codeString)) 3935 return FHIRAllTypes.OID; 3936 if ("positiveInt".equals(codeString)) 3937 return FHIRAllTypes.POSITIVEINT; 3938 if ("string".equals(codeString)) 3939 return FHIRAllTypes.STRING; 3940 if ("time".equals(codeString)) 3941 return FHIRAllTypes.TIME; 3942 if ("unsignedInt".equals(codeString)) 3943 return FHIRAllTypes.UNSIGNEDINT; 3944 if ("uri".equals(codeString)) 3945 return FHIRAllTypes.URI; 3946 if ("uuid".equals(codeString)) 3947 return FHIRAllTypes.UUID; 3948 if ("xhtml".equals(codeString)) 3949 return FHIRAllTypes.XHTML; 3950 if ("Account".equals(codeString)) 3951 return FHIRAllTypes.ACCOUNT; 3952 if ("ActivityDefinition".equals(codeString)) 3953 return FHIRAllTypes.ACTIVITYDEFINITION; 3954 if ("AdverseEvent".equals(codeString)) 3955 return FHIRAllTypes.ADVERSEEVENT; 3956 if ("AllergyIntolerance".equals(codeString)) 3957 return FHIRAllTypes.ALLERGYINTOLERANCE; 3958 if ("Appointment".equals(codeString)) 3959 return FHIRAllTypes.APPOINTMENT; 3960 if ("AppointmentResponse".equals(codeString)) 3961 return FHIRAllTypes.APPOINTMENTRESPONSE; 3962 if ("AuditEvent".equals(codeString)) 3963 return FHIRAllTypes.AUDITEVENT; 3964 if ("Basic".equals(codeString)) 3965 return FHIRAllTypes.BASIC; 3966 if ("Binary".equals(codeString)) 3967 return FHIRAllTypes.BINARY; 3968 if ("BodySite".equals(codeString)) 3969 return FHIRAllTypes.BODYSITE; 3970 if ("Bundle".equals(codeString)) 3971 return FHIRAllTypes.BUNDLE; 3972 if ("CapabilityStatement".equals(codeString)) 3973 return FHIRAllTypes.CAPABILITYSTATEMENT; 3974 if ("CarePlan".equals(codeString)) 3975 return FHIRAllTypes.CAREPLAN; 3976 if ("CareTeam".equals(codeString)) 3977 return FHIRAllTypes.CARETEAM; 3978 if ("ChargeItem".equals(codeString)) 3979 return FHIRAllTypes.CHARGEITEM; 3980 if ("Claim".equals(codeString)) 3981 return FHIRAllTypes.CLAIM; 3982 if ("ClaimResponse".equals(codeString)) 3983 return FHIRAllTypes.CLAIMRESPONSE; 3984 if ("ClinicalImpression".equals(codeString)) 3985 return FHIRAllTypes.CLINICALIMPRESSION; 3986 if ("CodeSystem".equals(codeString)) 3987 return FHIRAllTypes.CODESYSTEM; 3988 if ("Communication".equals(codeString)) 3989 return FHIRAllTypes.COMMUNICATION; 3990 if ("CommunicationRequest".equals(codeString)) 3991 return FHIRAllTypes.COMMUNICATIONREQUEST; 3992 if ("CompartmentDefinition".equals(codeString)) 3993 return FHIRAllTypes.COMPARTMENTDEFINITION; 3994 if ("Composition".equals(codeString)) 3995 return FHIRAllTypes.COMPOSITION; 3996 if ("ConceptMap".equals(codeString)) 3997 return FHIRAllTypes.CONCEPTMAP; 3998 if ("Condition".equals(codeString)) 3999 return FHIRAllTypes.CONDITION; 4000 if ("Consent".equals(codeString)) 4001 return FHIRAllTypes.CONSENT; 4002 if ("Contract".equals(codeString)) 4003 return FHIRAllTypes.CONTRACT; 4004 if ("Coverage".equals(codeString)) 4005 return FHIRAllTypes.COVERAGE; 4006 if ("DataElement".equals(codeString)) 4007 return FHIRAllTypes.DATAELEMENT; 4008 if ("DetectedIssue".equals(codeString)) 4009 return FHIRAllTypes.DETECTEDISSUE; 4010 if ("Device".equals(codeString)) 4011 return FHIRAllTypes.DEVICE; 4012 if ("DeviceComponent".equals(codeString)) 4013 return FHIRAllTypes.DEVICECOMPONENT; 4014 if ("DeviceMetric".equals(codeString)) 4015 return FHIRAllTypes.DEVICEMETRIC; 4016 if ("DeviceRequest".equals(codeString)) 4017 return FHIRAllTypes.DEVICEREQUEST; 4018 if ("DeviceUseStatement".equals(codeString)) 4019 return FHIRAllTypes.DEVICEUSESTATEMENT; 4020 if ("DiagnosticReport".equals(codeString)) 4021 return FHIRAllTypes.DIAGNOSTICREPORT; 4022 if ("DocumentManifest".equals(codeString)) 4023 return FHIRAllTypes.DOCUMENTMANIFEST; 4024 if ("DocumentReference".equals(codeString)) 4025 return FHIRAllTypes.DOCUMENTREFERENCE; 4026 if ("DomainResource".equals(codeString)) 4027 return FHIRAllTypes.DOMAINRESOURCE; 4028 if ("EligibilityRequest".equals(codeString)) 4029 return FHIRAllTypes.ELIGIBILITYREQUEST; 4030 if ("EligibilityResponse".equals(codeString)) 4031 return FHIRAllTypes.ELIGIBILITYRESPONSE; 4032 if ("Encounter".equals(codeString)) 4033 return FHIRAllTypes.ENCOUNTER; 4034 if ("Endpoint".equals(codeString)) 4035 return FHIRAllTypes.ENDPOINT; 4036 if ("EnrollmentRequest".equals(codeString)) 4037 return FHIRAllTypes.ENROLLMENTREQUEST; 4038 if ("EnrollmentResponse".equals(codeString)) 4039 return FHIRAllTypes.ENROLLMENTRESPONSE; 4040 if ("EpisodeOfCare".equals(codeString)) 4041 return FHIRAllTypes.EPISODEOFCARE; 4042 if ("ExpansionProfile".equals(codeString)) 4043 return FHIRAllTypes.EXPANSIONPROFILE; 4044 if ("ExplanationOfBenefit".equals(codeString)) 4045 return FHIRAllTypes.EXPLANATIONOFBENEFIT; 4046 if ("FamilyMemberHistory".equals(codeString)) 4047 return FHIRAllTypes.FAMILYMEMBERHISTORY; 4048 if ("Flag".equals(codeString)) 4049 return FHIRAllTypes.FLAG; 4050 if ("Goal".equals(codeString)) 4051 return FHIRAllTypes.GOAL; 4052 if ("GraphDefinition".equals(codeString)) 4053 return FHIRAllTypes.GRAPHDEFINITION; 4054 if ("Group".equals(codeString)) 4055 return FHIRAllTypes.GROUP; 4056 if ("GuidanceResponse".equals(codeString)) 4057 return FHIRAllTypes.GUIDANCERESPONSE; 4058 if ("HealthcareService".equals(codeString)) 4059 return FHIRAllTypes.HEALTHCARESERVICE; 4060 if ("ImagingManifest".equals(codeString)) 4061 return FHIRAllTypes.IMAGINGMANIFEST; 4062 if ("ImagingStudy".equals(codeString)) 4063 return FHIRAllTypes.IMAGINGSTUDY; 4064 if ("Immunization".equals(codeString)) 4065 return FHIRAllTypes.IMMUNIZATION; 4066 if ("ImmunizationRecommendation".equals(codeString)) 4067 return FHIRAllTypes.IMMUNIZATIONRECOMMENDATION; 4068 if ("ImplementationGuide".equals(codeString)) 4069 return FHIRAllTypes.IMPLEMENTATIONGUIDE; 4070 if ("Library".equals(codeString)) 4071 return FHIRAllTypes.LIBRARY; 4072 if ("Linkage".equals(codeString)) 4073 return FHIRAllTypes.LINKAGE; 4074 if ("List".equals(codeString)) 4075 return FHIRAllTypes.LIST; 4076 if ("Location".equals(codeString)) 4077 return FHIRAllTypes.LOCATION; 4078 if ("Measure".equals(codeString)) 4079 return FHIRAllTypes.MEASURE; 4080 if ("MeasureReport".equals(codeString)) 4081 return FHIRAllTypes.MEASUREREPORT; 4082 if ("Media".equals(codeString)) 4083 return FHIRAllTypes.MEDIA; 4084 if ("Medication".equals(codeString)) 4085 return FHIRAllTypes.MEDICATION; 4086 if ("MedicationAdministration".equals(codeString)) 4087 return FHIRAllTypes.MEDICATIONADMINISTRATION; 4088 if ("MedicationDispense".equals(codeString)) 4089 return FHIRAllTypes.MEDICATIONDISPENSE; 4090 if ("MedicationRequest".equals(codeString)) 4091 return FHIRAllTypes.MEDICATIONREQUEST; 4092 if ("MedicationStatement".equals(codeString)) 4093 return FHIRAllTypes.MEDICATIONSTATEMENT; 4094 if ("MessageDefinition".equals(codeString)) 4095 return FHIRAllTypes.MESSAGEDEFINITION; 4096 if ("MessageHeader".equals(codeString)) 4097 return FHIRAllTypes.MESSAGEHEADER; 4098 if ("NamingSystem".equals(codeString)) 4099 return FHIRAllTypes.NAMINGSYSTEM; 4100 if ("NutritionOrder".equals(codeString)) 4101 return FHIRAllTypes.NUTRITIONORDER; 4102 if ("Observation".equals(codeString)) 4103 return FHIRAllTypes.OBSERVATION; 4104 if ("OperationDefinition".equals(codeString)) 4105 return FHIRAllTypes.OPERATIONDEFINITION; 4106 if ("OperationOutcome".equals(codeString)) 4107 return FHIRAllTypes.OPERATIONOUTCOME; 4108 if ("Organization".equals(codeString)) 4109 return FHIRAllTypes.ORGANIZATION; 4110 if ("Parameters".equals(codeString)) 4111 return FHIRAllTypes.PARAMETERS; 4112 if ("Patient".equals(codeString)) 4113 return FHIRAllTypes.PATIENT; 4114 if ("PaymentNotice".equals(codeString)) 4115 return FHIRAllTypes.PAYMENTNOTICE; 4116 if ("PaymentReconciliation".equals(codeString)) 4117 return FHIRAllTypes.PAYMENTRECONCILIATION; 4118 if ("Person".equals(codeString)) 4119 return FHIRAllTypes.PERSON; 4120 if ("PlanDefinition".equals(codeString)) 4121 return FHIRAllTypes.PLANDEFINITION; 4122 if ("Practitioner".equals(codeString)) 4123 return FHIRAllTypes.PRACTITIONER; 4124 if ("PractitionerRole".equals(codeString)) 4125 return FHIRAllTypes.PRACTITIONERROLE; 4126 if ("Procedure".equals(codeString)) 4127 return FHIRAllTypes.PROCEDURE; 4128 if ("ProcedureRequest".equals(codeString)) 4129 return FHIRAllTypes.PROCEDUREREQUEST; 4130 if ("ProcessRequest".equals(codeString)) 4131 return FHIRAllTypes.PROCESSREQUEST; 4132 if ("ProcessResponse".equals(codeString)) 4133 return FHIRAllTypes.PROCESSRESPONSE; 4134 if ("Provenance".equals(codeString)) 4135 return FHIRAllTypes.PROVENANCE; 4136 if ("Questionnaire".equals(codeString)) 4137 return FHIRAllTypes.QUESTIONNAIRE; 4138 if ("QuestionnaireResponse".equals(codeString)) 4139 return FHIRAllTypes.QUESTIONNAIRERESPONSE; 4140 if ("ReferralRequest".equals(codeString)) 4141 return FHIRAllTypes.REFERRALREQUEST; 4142 if ("RelatedPerson".equals(codeString)) 4143 return FHIRAllTypes.RELATEDPERSON; 4144 if ("RequestGroup".equals(codeString)) 4145 return FHIRAllTypes.REQUESTGROUP; 4146 if ("ResearchStudy".equals(codeString)) 4147 return FHIRAllTypes.RESEARCHSTUDY; 4148 if ("ResearchSubject".equals(codeString)) 4149 return FHIRAllTypes.RESEARCHSUBJECT; 4150 if ("Resource".equals(codeString)) 4151 return FHIRAllTypes.RESOURCE; 4152 if ("RiskAssessment".equals(codeString)) 4153 return FHIRAllTypes.RISKASSESSMENT; 4154 if ("Schedule".equals(codeString)) 4155 return FHIRAllTypes.SCHEDULE; 4156 if ("SearchParameter".equals(codeString)) 4157 return FHIRAllTypes.SEARCHPARAMETER; 4158 if ("Sequence".equals(codeString)) 4159 return FHIRAllTypes.SEQUENCE; 4160 if ("ServiceDefinition".equals(codeString)) 4161 return FHIRAllTypes.SERVICEDEFINITION; 4162 if ("Slot".equals(codeString)) 4163 return FHIRAllTypes.SLOT; 4164 if ("Specimen".equals(codeString)) 4165 return FHIRAllTypes.SPECIMEN; 4166 if ("StructureDefinition".equals(codeString)) 4167 return FHIRAllTypes.STRUCTUREDEFINITION; 4168 if ("StructureMap".equals(codeString)) 4169 return FHIRAllTypes.STRUCTUREMAP; 4170 if ("Subscription".equals(codeString)) 4171 return FHIRAllTypes.SUBSCRIPTION; 4172 if ("Substance".equals(codeString)) 4173 return FHIRAllTypes.SUBSTANCE; 4174 if ("SupplyDelivery".equals(codeString)) 4175 return FHIRAllTypes.SUPPLYDELIVERY; 4176 if ("SupplyRequest".equals(codeString)) 4177 return FHIRAllTypes.SUPPLYREQUEST; 4178 if ("Task".equals(codeString)) 4179 return FHIRAllTypes.TASK; 4180 if ("TestReport".equals(codeString)) 4181 return FHIRAllTypes.TESTREPORT; 4182 if ("TestScript".equals(codeString)) 4183 return FHIRAllTypes.TESTSCRIPT; 4184 if ("ValueSet".equals(codeString)) 4185 return FHIRAllTypes.VALUESET; 4186 if ("VisionPrescription".equals(codeString)) 4187 return FHIRAllTypes.VISIONPRESCRIPTION; 4188 if ("Type".equals(codeString)) 4189 return FHIRAllTypes.TYPE; 4190 if ("Any".equals(codeString)) 4191 return FHIRAllTypes.ANY; 4192 throw new IllegalArgumentException("Unknown FHIRAllTypes code '"+codeString+"'"); 4193 } 4194 public Enumeration<FHIRAllTypes> fromType(PrimitiveType<?> code) throws FHIRException { 4195 if (code == null) 4196 return null; 4197 if (code.isEmpty()) 4198 return new Enumeration<FHIRAllTypes>(this); 4199 String codeString = code.asStringValue(); 4200 if (codeString == null || "".equals(codeString)) 4201 return null; 4202 if ("Address".equals(codeString)) 4203 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ADDRESS); 4204 if ("Age".equals(codeString)) 4205 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.AGE); 4206 if ("Annotation".equals(codeString)) 4207 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ANNOTATION); 4208 if ("Attachment".equals(codeString)) 4209 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ATTACHMENT); 4210 if ("BackboneElement".equals(codeString)) 4211 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BACKBONEELEMENT); 4212 if ("CodeableConcept".equals(codeString)) 4213 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODEABLECONCEPT); 4214 if ("Coding".equals(codeString)) 4215 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODING); 4216 if ("ContactDetail".equals(codeString)) 4217 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTACTDETAIL); 4218 if ("ContactPoint".equals(codeString)) 4219 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTACTPOINT); 4220 if ("Contributor".equals(codeString)) 4221 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTRIBUTOR); 4222 if ("Count".equals(codeString)) 4223 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COUNT); 4224 if ("DataRequirement".equals(codeString)) 4225 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATAREQUIREMENT); 4226 if ("Distance".equals(codeString)) 4227 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DISTANCE); 4228 if ("Dosage".equals(codeString)) 4229 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOSAGE); 4230 if ("Duration".equals(codeString)) 4231 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DURATION); 4232 if ("Element".equals(codeString)) 4233 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELEMENT); 4234 if ("ElementDefinition".equals(codeString)) 4235 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELEMENTDEFINITION); 4236 if ("Extension".equals(codeString)) 4237 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXTENSION); 4238 if ("HumanName".equals(codeString)) 4239 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.HUMANNAME); 4240 if ("Identifier".equals(codeString)) 4241 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IDENTIFIER); 4242 if ("Meta".equals(codeString)) 4243 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.META); 4244 if ("Money".equals(codeString)) 4245 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MONEY); 4246 if ("Narrative".equals(codeString)) 4247 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NARRATIVE); 4248 if ("ParameterDefinition".equals(codeString)) 4249 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PARAMETERDEFINITION); 4250 if ("Period".equals(codeString)) 4251 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PERIOD); 4252 if ("Quantity".equals(codeString)) 4253 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUANTITY); 4254 if ("Range".equals(codeString)) 4255 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RANGE); 4256 if ("Ratio".equals(codeString)) 4257 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RATIO); 4258 if ("Reference".equals(codeString)) 4259 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REFERENCE); 4260 if ("RelatedArtifact".equals(codeString)) 4261 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RELATEDARTIFACT); 4262 if ("SampledData".equals(codeString)) 4263 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SAMPLEDDATA); 4264 if ("Signature".equals(codeString)) 4265 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SIGNATURE); 4266 if ("SimpleQuantity".equals(codeString)) 4267 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SIMPLEQUANTITY); 4268 if ("Timing".equals(codeString)) 4269 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TIMING); 4270 if ("TriggerDefinition".equals(codeString)) 4271 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TRIGGERDEFINITION); 4272 if ("UsageContext".equals(codeString)) 4273 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.USAGECONTEXT); 4274 if ("base64Binary".equals(codeString)) 4275 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BASE64BINARY); 4276 if ("boolean".equals(codeString)) 4277 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BOOLEAN); 4278 if ("code".equals(codeString)) 4279 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODE); 4280 if ("date".equals(codeString)) 4281 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATE); 4282 if ("dateTime".equals(codeString)) 4283 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATETIME); 4284 if ("decimal".equals(codeString)) 4285 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DECIMAL); 4286 if ("id".equals(codeString)) 4287 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ID); 4288 if ("instant".equals(codeString)) 4289 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INSTANT); 4290 if ("integer".equals(codeString)) 4291 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INTEGER); 4292 if ("markdown".equals(codeString)) 4293 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MARKDOWN); 4294 if ("oid".equals(codeString)) 4295 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OID); 4296 if ("positiveInt".equals(codeString)) 4297 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.POSITIVEINT); 4298 if ("string".equals(codeString)) 4299 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRING); 4300 if ("time".equals(codeString)) 4301 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TIME); 4302 if ("unsignedInt".equals(codeString)) 4303 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.UNSIGNEDINT); 4304 if ("uri".equals(codeString)) 4305 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.URI); 4306 if ("uuid".equals(codeString)) 4307 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.UUID); 4308 if ("xhtml".equals(codeString)) 4309 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.XHTML); 4310 if ("Account".equals(codeString)) 4311 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ACCOUNT); 4312 if ("ActivityDefinition".equals(codeString)) 4313 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ACTIVITYDEFINITION); 4314 if ("AdverseEvent".equals(codeString)) 4315 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ADVERSEEVENT); 4316 if ("AllergyIntolerance".equals(codeString)) 4317 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ALLERGYINTOLERANCE); 4318 if ("Appointment".equals(codeString)) 4319 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.APPOINTMENT); 4320 if ("AppointmentResponse".equals(codeString)) 4321 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.APPOINTMENTRESPONSE); 4322 if ("AuditEvent".equals(codeString)) 4323 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.AUDITEVENT); 4324 if ("Basic".equals(codeString)) 4325 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BASIC); 4326 if ("Binary".equals(codeString)) 4327 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BINARY); 4328 if ("BodySite".equals(codeString)) 4329 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BODYSITE); 4330 if ("Bundle".equals(codeString)) 4331 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BUNDLE); 4332 if ("CapabilityStatement".equals(codeString)) 4333 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CAPABILITYSTATEMENT); 4334 if ("CarePlan".equals(codeString)) 4335 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CAREPLAN); 4336 if ("CareTeam".equals(codeString)) 4337 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CARETEAM); 4338 if ("ChargeItem".equals(codeString)) 4339 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CHARGEITEM); 4340 if ("Claim".equals(codeString)) 4341 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLAIM); 4342 if ("ClaimResponse".equals(codeString)) 4343 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLAIMRESPONSE); 4344 if ("ClinicalImpression".equals(codeString)) 4345 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLINICALIMPRESSION); 4346 if ("CodeSystem".equals(codeString)) 4347 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODESYSTEM); 4348 if ("Communication".equals(codeString)) 4349 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMMUNICATION); 4350 if ("CommunicationRequest".equals(codeString)) 4351 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMMUNICATIONREQUEST); 4352 if ("CompartmentDefinition".equals(codeString)) 4353 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMPARTMENTDEFINITION); 4354 if ("Composition".equals(codeString)) 4355 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMPOSITION); 4356 if ("ConceptMap".equals(codeString)) 4357 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONCEPTMAP); 4358 if ("Condition".equals(codeString)) 4359 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONDITION); 4360 if ("Consent".equals(codeString)) 4361 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONSENT); 4362 if ("Contract".equals(codeString)) 4363 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTRACT); 4364 if ("Coverage".equals(codeString)) 4365 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COVERAGE); 4366 if ("DataElement".equals(codeString)) 4367 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATAELEMENT); 4368 if ("DetectedIssue".equals(codeString)) 4369 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DETECTEDISSUE); 4370 if ("Device".equals(codeString)) 4371 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICE); 4372 if ("DeviceComponent".equals(codeString)) 4373 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICECOMPONENT); 4374 if ("DeviceMetric".equals(codeString)) 4375 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEMETRIC); 4376 if ("DeviceRequest".equals(codeString)) 4377 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEREQUEST); 4378 if ("DeviceUseStatement".equals(codeString)) 4379 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEUSESTATEMENT); 4380 if ("DiagnosticReport".equals(codeString)) 4381 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DIAGNOSTICREPORT); 4382 if ("DocumentManifest".equals(codeString)) 4383 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOCUMENTMANIFEST); 4384 if ("DocumentReference".equals(codeString)) 4385 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOCUMENTREFERENCE); 4386 if ("DomainResource".equals(codeString)) 4387 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOMAINRESOURCE); 4388 if ("EligibilityRequest".equals(codeString)) 4389 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELIGIBILITYREQUEST); 4390 if ("EligibilityResponse".equals(codeString)) 4391 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELIGIBILITYRESPONSE); 4392 if ("Encounter".equals(codeString)) 4393 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENCOUNTER); 4394 if ("Endpoint".equals(codeString)) 4395 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENDPOINT); 4396 if ("EnrollmentRequest".equals(codeString)) 4397 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENROLLMENTREQUEST); 4398 if ("EnrollmentResponse".equals(codeString)) 4399 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENROLLMENTRESPONSE); 4400 if ("EpisodeOfCare".equals(codeString)) 4401 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EPISODEOFCARE); 4402 if ("ExpansionProfile".equals(codeString)) 4403 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXPANSIONPROFILE); 4404 if ("ExplanationOfBenefit".equals(codeString)) 4405 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXPLANATIONOFBENEFIT); 4406 if ("FamilyMemberHistory".equals(codeString)) 4407 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.FAMILYMEMBERHISTORY); 4408 if ("Flag".equals(codeString)) 4409 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.FLAG); 4410 if ("Goal".equals(codeString)) 4411 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GOAL); 4412 if ("GraphDefinition".equals(codeString)) 4413 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GRAPHDEFINITION); 4414 if ("Group".equals(codeString)) 4415 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GROUP); 4416 if ("GuidanceResponse".equals(codeString)) 4417 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GUIDANCERESPONSE); 4418 if ("HealthcareService".equals(codeString)) 4419 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.HEALTHCARESERVICE); 4420 if ("ImagingManifest".equals(codeString)) 4421 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMAGINGMANIFEST); 4422 if ("ImagingStudy".equals(codeString)) 4423 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMAGINGSTUDY); 4424 if ("Immunization".equals(codeString)) 4425 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATION); 4426 if ("ImmunizationRecommendation".equals(codeString)) 4427 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATIONRECOMMENDATION); 4428 if ("ImplementationGuide".equals(codeString)) 4429 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMPLEMENTATIONGUIDE); 4430 if ("Library".equals(codeString)) 4431 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LIBRARY); 4432 if ("Linkage".equals(codeString)) 4433 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LINKAGE); 4434 if ("List".equals(codeString)) 4435 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LIST); 4436 if ("Location".equals(codeString)) 4437 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LOCATION); 4438 if ("Measure".equals(codeString)) 4439 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEASURE); 4440 if ("MeasureReport".equals(codeString)) 4441 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEASUREREPORT); 4442 if ("Media".equals(codeString)) 4443 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDIA); 4444 if ("Medication".equals(codeString)) 4445 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATION); 4446 if ("MedicationAdministration".equals(codeString)) 4447 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONADMINISTRATION); 4448 if ("MedicationDispense".equals(codeString)) 4449 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONDISPENSE); 4450 if ("MedicationRequest".equals(codeString)) 4451 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONREQUEST); 4452 if ("MedicationStatement".equals(codeString)) 4453 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONSTATEMENT); 4454 if ("MessageDefinition".equals(codeString)) 4455 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MESSAGEDEFINITION); 4456 if ("MessageHeader".equals(codeString)) 4457 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MESSAGEHEADER); 4458 if ("NamingSystem".equals(codeString)) 4459 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NAMINGSYSTEM); 4460 if ("NutritionOrder".equals(codeString)) 4461 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NUTRITIONORDER); 4462 if ("Observation".equals(codeString)) 4463 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OBSERVATION); 4464 if ("OperationDefinition".equals(codeString)) 4465 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OPERATIONDEFINITION); 4466 if ("OperationOutcome".equals(codeString)) 4467 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OPERATIONOUTCOME); 4468 if ("Organization".equals(codeString)) 4469 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ORGANIZATION); 4470 if ("Parameters".equals(codeString)) 4471 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PARAMETERS); 4472 if ("Patient".equals(codeString)) 4473 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PATIENT); 4474 if ("PaymentNotice".equals(codeString)) 4475 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PAYMENTNOTICE); 4476 if ("PaymentReconciliation".equals(codeString)) 4477 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PAYMENTRECONCILIATION); 4478 if ("Person".equals(codeString)) 4479 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PERSON); 4480 if ("PlanDefinition".equals(codeString)) 4481 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PLANDEFINITION); 4482 if ("Practitioner".equals(codeString)) 4483 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRACTITIONER); 4484 if ("PractitionerRole".equals(codeString)) 4485 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRACTITIONERROLE); 4486 if ("Procedure".equals(codeString)) 4487 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCEDURE); 4488 if ("ProcedureRequest".equals(codeString)) 4489 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCEDUREREQUEST); 4490 if ("ProcessRequest".equals(codeString)) 4491 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCESSREQUEST); 4492 if ("ProcessResponse".equals(codeString)) 4493 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCESSRESPONSE); 4494 if ("Provenance".equals(codeString)) 4495 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROVENANCE); 4496 if ("Questionnaire".equals(codeString)) 4497 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUESTIONNAIRE); 4498 if ("QuestionnaireResponse".equals(codeString)) 4499 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUESTIONNAIRERESPONSE); 4500 if ("ReferralRequest".equals(codeString)) 4501 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REFERRALREQUEST); 4502 if ("RelatedPerson".equals(codeString)) 4503 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RELATEDPERSON); 4504 if ("RequestGroup".equals(codeString)) 4505 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REQUESTGROUP); 4506 if ("ResearchStudy".equals(codeString)) 4507 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHSTUDY); 4508 if ("ResearchSubject".equals(codeString)) 4509 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHSUBJECT); 4510 if ("Resource".equals(codeString)) 4511 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESOURCE); 4512 if ("RiskAssessment".equals(codeString)) 4513 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RISKASSESSMENT); 4514 if ("Schedule".equals(codeString)) 4515 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SCHEDULE); 4516 if ("SearchParameter".equals(codeString)) 4517 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SEARCHPARAMETER); 4518 if ("Sequence".equals(codeString)) 4519 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SEQUENCE); 4520 if ("ServiceDefinition".equals(codeString)) 4521 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SERVICEDEFINITION); 4522 if ("Slot".equals(codeString)) 4523 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SLOT); 4524 if ("Specimen".equals(codeString)) 4525 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SPECIMEN); 4526 if ("StructureDefinition".equals(codeString)) 4527 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRUCTUREDEFINITION); 4528 if ("StructureMap".equals(codeString)) 4529 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRUCTUREMAP); 4530 if ("Subscription".equals(codeString)) 4531 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSCRIPTION); 4532 if ("Substance".equals(codeString)) 4533 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCE); 4534 if ("SupplyDelivery".equals(codeString)) 4535 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUPPLYDELIVERY); 4536 if ("SupplyRequest".equals(codeString)) 4537 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUPPLYREQUEST); 4538 if ("Task".equals(codeString)) 4539 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TASK); 4540 if ("TestReport".equals(codeString)) 4541 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TESTREPORT); 4542 if ("TestScript".equals(codeString)) 4543 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TESTSCRIPT); 4544 if ("ValueSet".equals(codeString)) 4545 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VALUESET); 4546 if ("VisionPrescription".equals(codeString)) 4547 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VISIONPRESCRIPTION); 4548 if ("Type".equals(codeString)) 4549 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TYPE); 4550 if ("Any".equals(codeString)) 4551 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ANY); 4552 throw new FHIRException("Unknown FHIRAllTypes code '"+codeString+"'"); 4553 } 4554 public String toCode(FHIRAllTypes code) { 4555 if (code == FHIRAllTypes.NULL) 4556 return null; 4557 if (code == FHIRAllTypes.ADDRESS) 4558 return "Address"; 4559 if (code == FHIRAllTypes.AGE) 4560 return "Age"; 4561 if (code == FHIRAllTypes.ANNOTATION) 4562 return "Annotation"; 4563 if (code == FHIRAllTypes.ATTACHMENT) 4564 return "Attachment"; 4565 if (code == FHIRAllTypes.BACKBONEELEMENT) 4566 return "BackboneElement"; 4567 if (code == FHIRAllTypes.CODEABLECONCEPT) 4568 return "CodeableConcept"; 4569 if (code == FHIRAllTypes.CODING) 4570 return "Coding"; 4571 if (code == FHIRAllTypes.CONTACTDETAIL) 4572 return "ContactDetail"; 4573 if (code == FHIRAllTypes.CONTACTPOINT) 4574 return "ContactPoint"; 4575 if (code == FHIRAllTypes.CONTRIBUTOR) 4576 return "Contributor"; 4577 if (code == FHIRAllTypes.COUNT) 4578 return "Count"; 4579 if (code == FHIRAllTypes.DATAREQUIREMENT) 4580 return "DataRequirement"; 4581 if (code == FHIRAllTypes.DISTANCE) 4582 return "Distance"; 4583 if (code == FHIRAllTypes.DOSAGE) 4584 return "Dosage"; 4585 if (code == FHIRAllTypes.DURATION) 4586 return "Duration"; 4587 if (code == FHIRAllTypes.ELEMENT) 4588 return "Element"; 4589 if (code == FHIRAllTypes.ELEMENTDEFINITION) 4590 return "ElementDefinition"; 4591 if (code == FHIRAllTypes.EXTENSION) 4592 return "Extension"; 4593 if (code == FHIRAllTypes.HUMANNAME) 4594 return "HumanName"; 4595 if (code == FHIRAllTypes.IDENTIFIER) 4596 return "Identifier"; 4597 if (code == FHIRAllTypes.META) 4598 return "Meta"; 4599 if (code == FHIRAllTypes.MONEY) 4600 return "Money"; 4601 if (code == FHIRAllTypes.NARRATIVE) 4602 return "Narrative"; 4603 if (code == FHIRAllTypes.PARAMETERDEFINITION) 4604 return "ParameterDefinition"; 4605 if (code == FHIRAllTypes.PERIOD) 4606 return "Period"; 4607 if (code == FHIRAllTypes.QUANTITY) 4608 return "Quantity"; 4609 if (code == FHIRAllTypes.RANGE) 4610 return "Range"; 4611 if (code == FHIRAllTypes.RATIO) 4612 return "Ratio"; 4613 if (code == FHIRAllTypes.REFERENCE) 4614 return "Reference"; 4615 if (code == FHIRAllTypes.RELATEDARTIFACT) 4616 return "RelatedArtifact"; 4617 if (code == FHIRAllTypes.SAMPLEDDATA) 4618 return "SampledData"; 4619 if (code == FHIRAllTypes.SIGNATURE) 4620 return "Signature"; 4621 if (code == FHIRAllTypes.SIMPLEQUANTITY) 4622 return "SimpleQuantity"; 4623 if (code == FHIRAllTypes.TIMING) 4624 return "Timing"; 4625 if (code == FHIRAllTypes.TRIGGERDEFINITION) 4626 return "TriggerDefinition"; 4627 if (code == FHIRAllTypes.USAGECONTEXT) 4628 return "UsageContext"; 4629 if (code == FHIRAllTypes.BASE64BINARY) 4630 return "base64Binary"; 4631 if (code == FHIRAllTypes.BOOLEAN) 4632 return "boolean"; 4633 if (code == FHIRAllTypes.CODE) 4634 return "code"; 4635 if (code == FHIRAllTypes.DATE) 4636 return "date"; 4637 if (code == FHIRAllTypes.DATETIME) 4638 return "dateTime"; 4639 if (code == FHIRAllTypes.DECIMAL) 4640 return "decimal"; 4641 if (code == FHIRAllTypes.ID) 4642 return "id"; 4643 if (code == FHIRAllTypes.INSTANT) 4644 return "instant"; 4645 if (code == FHIRAllTypes.INTEGER) 4646 return "integer"; 4647 if (code == FHIRAllTypes.MARKDOWN) 4648 return "markdown"; 4649 if (code == FHIRAllTypes.OID) 4650 return "oid"; 4651 if (code == FHIRAllTypes.POSITIVEINT) 4652 return "positiveInt"; 4653 if (code == FHIRAllTypes.STRING) 4654 return "string"; 4655 if (code == FHIRAllTypes.TIME) 4656 return "time"; 4657 if (code == FHIRAllTypes.UNSIGNEDINT) 4658 return "unsignedInt"; 4659 if (code == FHIRAllTypes.URI) 4660 return "uri"; 4661 if (code == FHIRAllTypes.UUID) 4662 return "uuid"; 4663 if (code == FHIRAllTypes.XHTML) 4664 return "xhtml"; 4665 if (code == FHIRAllTypes.ACCOUNT) 4666 return "Account"; 4667 if (code == FHIRAllTypes.ACTIVITYDEFINITION) 4668 return "ActivityDefinition"; 4669 if (code == FHIRAllTypes.ADVERSEEVENT) 4670 return "AdverseEvent"; 4671 if (code == FHIRAllTypes.ALLERGYINTOLERANCE) 4672 return "AllergyIntolerance"; 4673 if (code == FHIRAllTypes.APPOINTMENT) 4674 return "Appointment"; 4675 if (code == FHIRAllTypes.APPOINTMENTRESPONSE) 4676 return "AppointmentResponse"; 4677 if (code == FHIRAllTypes.AUDITEVENT) 4678 return "AuditEvent"; 4679 if (code == FHIRAllTypes.BASIC) 4680 return "Basic"; 4681 if (code == FHIRAllTypes.BINARY) 4682 return "Binary"; 4683 if (code == FHIRAllTypes.BODYSITE) 4684 return "BodySite"; 4685 if (code == FHIRAllTypes.BUNDLE) 4686 return "Bundle"; 4687 if (code == FHIRAllTypes.CAPABILITYSTATEMENT) 4688 return "CapabilityStatement"; 4689 if (code == FHIRAllTypes.CAREPLAN) 4690 return "CarePlan"; 4691 if (code == FHIRAllTypes.CARETEAM) 4692 return "CareTeam"; 4693 if (code == FHIRAllTypes.CHARGEITEM) 4694 return "ChargeItem"; 4695 if (code == FHIRAllTypes.CLAIM) 4696 return "Claim"; 4697 if (code == FHIRAllTypes.CLAIMRESPONSE) 4698 return "ClaimResponse"; 4699 if (code == FHIRAllTypes.CLINICALIMPRESSION) 4700 return "ClinicalImpression"; 4701 if (code == FHIRAllTypes.CODESYSTEM) 4702 return "CodeSystem"; 4703 if (code == FHIRAllTypes.COMMUNICATION) 4704 return "Communication"; 4705 if (code == FHIRAllTypes.COMMUNICATIONREQUEST) 4706 return "CommunicationRequest"; 4707 if (code == FHIRAllTypes.COMPARTMENTDEFINITION) 4708 return "CompartmentDefinition"; 4709 if (code == FHIRAllTypes.COMPOSITION) 4710 return "Composition"; 4711 if (code == FHIRAllTypes.CONCEPTMAP) 4712 return "ConceptMap"; 4713 if (code == FHIRAllTypes.CONDITION) 4714 return "Condition"; 4715 if (code == FHIRAllTypes.CONSENT) 4716 return "Consent"; 4717 if (code == FHIRAllTypes.CONTRACT) 4718 return "Contract"; 4719 if (code == FHIRAllTypes.COVERAGE) 4720 return "Coverage"; 4721 if (code == FHIRAllTypes.DATAELEMENT) 4722 return "DataElement"; 4723 if (code == FHIRAllTypes.DETECTEDISSUE) 4724 return "DetectedIssue"; 4725 if (code == FHIRAllTypes.DEVICE) 4726 return "Device"; 4727 if (code == FHIRAllTypes.DEVICECOMPONENT) 4728 return "DeviceComponent"; 4729 if (code == FHIRAllTypes.DEVICEMETRIC) 4730 return "DeviceMetric"; 4731 if (code == FHIRAllTypes.DEVICEREQUEST) 4732 return "DeviceRequest"; 4733 if (code == FHIRAllTypes.DEVICEUSESTATEMENT) 4734 return "DeviceUseStatement"; 4735 if (code == FHIRAllTypes.DIAGNOSTICREPORT) 4736 return "DiagnosticReport"; 4737 if (code == FHIRAllTypes.DOCUMENTMANIFEST) 4738 return "DocumentManifest"; 4739 if (code == FHIRAllTypes.DOCUMENTREFERENCE) 4740 return "DocumentReference"; 4741 if (code == FHIRAllTypes.DOMAINRESOURCE) 4742 return "DomainResource"; 4743 if (code == FHIRAllTypes.ELIGIBILITYREQUEST) 4744 return "EligibilityRequest"; 4745 if (code == FHIRAllTypes.ELIGIBILITYRESPONSE) 4746 return "EligibilityResponse"; 4747 if (code == FHIRAllTypes.ENCOUNTER) 4748 return "Encounter"; 4749 if (code == FHIRAllTypes.ENDPOINT) 4750 return "Endpoint"; 4751 if (code == FHIRAllTypes.ENROLLMENTREQUEST) 4752 return "EnrollmentRequest"; 4753 if (code == FHIRAllTypes.ENROLLMENTRESPONSE) 4754 return "EnrollmentResponse"; 4755 if (code == FHIRAllTypes.EPISODEOFCARE) 4756 return "EpisodeOfCare"; 4757 if (code == FHIRAllTypes.EXPANSIONPROFILE) 4758 return "ExpansionProfile"; 4759 if (code == FHIRAllTypes.EXPLANATIONOFBENEFIT) 4760 return "ExplanationOfBenefit"; 4761 if (code == FHIRAllTypes.FAMILYMEMBERHISTORY) 4762 return "FamilyMemberHistory"; 4763 if (code == FHIRAllTypes.FLAG) 4764 return "Flag"; 4765 if (code == FHIRAllTypes.GOAL) 4766 return "Goal"; 4767 if (code == FHIRAllTypes.GRAPHDEFINITION) 4768 return "GraphDefinition"; 4769 if (code == FHIRAllTypes.GROUP) 4770 return "Group"; 4771 if (code == FHIRAllTypes.GUIDANCERESPONSE) 4772 return "GuidanceResponse"; 4773 if (code == FHIRAllTypes.HEALTHCARESERVICE) 4774 return "HealthcareService"; 4775 if (code == FHIRAllTypes.IMAGINGMANIFEST) 4776 return "ImagingManifest"; 4777 if (code == FHIRAllTypes.IMAGINGSTUDY) 4778 return "ImagingStudy"; 4779 if (code == FHIRAllTypes.IMMUNIZATION) 4780 return "Immunization"; 4781 if (code == FHIRAllTypes.IMMUNIZATIONRECOMMENDATION) 4782 return "ImmunizationRecommendation"; 4783 if (code == FHIRAllTypes.IMPLEMENTATIONGUIDE) 4784 return "ImplementationGuide"; 4785 if (code == FHIRAllTypes.LIBRARY) 4786 return "Library"; 4787 if (code == FHIRAllTypes.LINKAGE) 4788 return "Linkage"; 4789 if (code == FHIRAllTypes.LIST) 4790 return "List"; 4791 if (code == FHIRAllTypes.LOCATION) 4792 return "Location"; 4793 if (code == FHIRAllTypes.MEASURE) 4794 return "Measure"; 4795 if (code == FHIRAllTypes.MEASUREREPORT) 4796 return "MeasureReport"; 4797 if (code == FHIRAllTypes.MEDIA) 4798 return "Media"; 4799 if (code == FHIRAllTypes.MEDICATION) 4800 return "Medication"; 4801 if (code == FHIRAllTypes.MEDICATIONADMINISTRATION) 4802 return "MedicationAdministration"; 4803 if (code == FHIRAllTypes.MEDICATIONDISPENSE) 4804 return "MedicationDispense"; 4805 if (code == FHIRAllTypes.MEDICATIONREQUEST) 4806 return "MedicationRequest"; 4807 if (code == FHIRAllTypes.MEDICATIONSTATEMENT) 4808 return "MedicationStatement"; 4809 if (code == FHIRAllTypes.MESSAGEDEFINITION) 4810 return "MessageDefinition"; 4811 if (code == FHIRAllTypes.MESSAGEHEADER) 4812 return "MessageHeader"; 4813 if (code == FHIRAllTypes.NAMINGSYSTEM) 4814 return "NamingSystem"; 4815 if (code == FHIRAllTypes.NUTRITIONORDER) 4816 return "NutritionOrder"; 4817 if (code == FHIRAllTypes.OBSERVATION) 4818 return "Observation"; 4819 if (code == FHIRAllTypes.OPERATIONDEFINITION) 4820 return "OperationDefinition"; 4821 if (code == FHIRAllTypes.OPERATIONOUTCOME) 4822 return "OperationOutcome"; 4823 if (code == FHIRAllTypes.ORGANIZATION) 4824 return "Organization"; 4825 if (code == FHIRAllTypes.PARAMETERS) 4826 return "Parameters"; 4827 if (code == FHIRAllTypes.PATIENT) 4828 return "Patient"; 4829 if (code == FHIRAllTypes.PAYMENTNOTICE) 4830 return "PaymentNotice"; 4831 if (code == FHIRAllTypes.PAYMENTRECONCILIATION) 4832 return "PaymentReconciliation"; 4833 if (code == FHIRAllTypes.PERSON) 4834 return "Person"; 4835 if (code == FHIRAllTypes.PLANDEFINITION) 4836 return "PlanDefinition"; 4837 if (code == FHIRAllTypes.PRACTITIONER) 4838 return "Practitioner"; 4839 if (code == FHIRAllTypes.PRACTITIONERROLE) 4840 return "PractitionerRole"; 4841 if (code == FHIRAllTypes.PROCEDURE) 4842 return "Procedure"; 4843 if (code == FHIRAllTypes.PROCEDUREREQUEST) 4844 return "ProcedureRequest"; 4845 if (code == FHIRAllTypes.PROCESSREQUEST) 4846 return "ProcessRequest"; 4847 if (code == FHIRAllTypes.PROCESSRESPONSE) 4848 return "ProcessResponse"; 4849 if (code == FHIRAllTypes.PROVENANCE) 4850 return "Provenance"; 4851 if (code == FHIRAllTypes.QUESTIONNAIRE) 4852 return "Questionnaire"; 4853 if (code == FHIRAllTypes.QUESTIONNAIRERESPONSE) 4854 return "QuestionnaireResponse"; 4855 if (code == FHIRAllTypes.REFERRALREQUEST) 4856 return "ReferralRequest"; 4857 if (code == FHIRAllTypes.RELATEDPERSON) 4858 return "RelatedPerson"; 4859 if (code == FHIRAllTypes.REQUESTGROUP) 4860 return "RequestGroup"; 4861 if (code == FHIRAllTypes.RESEARCHSTUDY) 4862 return "ResearchStudy"; 4863 if (code == FHIRAllTypes.RESEARCHSUBJECT) 4864 return "ResearchSubject"; 4865 if (code == FHIRAllTypes.RESOURCE) 4866 return "Resource"; 4867 if (code == FHIRAllTypes.RISKASSESSMENT) 4868 return "RiskAssessment"; 4869 if (code == FHIRAllTypes.SCHEDULE) 4870 return "Schedule"; 4871 if (code == FHIRAllTypes.SEARCHPARAMETER) 4872 return "SearchParameter"; 4873 if (code == FHIRAllTypes.SEQUENCE) 4874 return "Sequence"; 4875 if (code == FHIRAllTypes.SERVICEDEFINITION) 4876 return "ServiceDefinition"; 4877 if (code == FHIRAllTypes.SLOT) 4878 return "Slot"; 4879 if (code == FHIRAllTypes.SPECIMEN) 4880 return "Specimen"; 4881 if (code == FHIRAllTypes.STRUCTUREDEFINITION) 4882 return "StructureDefinition"; 4883 if (code == FHIRAllTypes.STRUCTUREMAP) 4884 return "StructureMap"; 4885 if (code == FHIRAllTypes.SUBSCRIPTION) 4886 return "Subscription"; 4887 if (code == FHIRAllTypes.SUBSTANCE) 4888 return "Substance"; 4889 if (code == FHIRAllTypes.SUPPLYDELIVERY) 4890 return "SupplyDelivery"; 4891 if (code == FHIRAllTypes.SUPPLYREQUEST) 4892 return "SupplyRequest"; 4893 if (code == FHIRAllTypes.TASK) 4894 return "Task"; 4895 if (code == FHIRAllTypes.TESTREPORT) 4896 return "TestReport"; 4897 if (code == FHIRAllTypes.TESTSCRIPT) 4898 return "TestScript"; 4899 if (code == FHIRAllTypes.VALUESET) 4900 return "ValueSet"; 4901 if (code == FHIRAllTypes.VISIONPRESCRIPTION) 4902 return "VisionPrescription"; 4903 if (code == FHIRAllTypes.TYPE) 4904 return "Type"; 4905 if (code == FHIRAllTypes.ANY) 4906 return "Any"; 4907 return "?"; 4908 } 4909 public String toSystem(FHIRAllTypes code) { 4910 return code.getSystem(); 4911 } 4912 } 4913 4914 public enum FHIRDefinedType { 4915 /** 4916 * An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world. 4917 */ 4918 ADDRESS, 4919 /** 4920 * A duration of time during which an organism (or a process) has existed. 4921 */ 4922 AGE, 4923 /** 4924 * A text note which also contains information about who made the statement and when. 4925 */ 4926 ANNOTATION, 4927 /** 4928 * For referring to data content defined in other formats. 4929 */ 4930 ATTACHMENT, 4931 /** 4932 * Base definition for all elements that are defined inside a resource - but not those in a data type. 4933 */ 4934 BACKBONEELEMENT, 4935 /** 4936 * A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text. 4937 */ 4938 CODEABLECONCEPT, 4939 /** 4940 * A reference to a code defined by a terminology system. 4941 */ 4942 CODING, 4943 /** 4944 * Specifies contact information for a person or organization. 4945 */ 4946 CONTACTDETAIL, 4947 /** 4948 * Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 4949 */ 4950 CONTACTPOINT, 4951 /** 4952 * A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers. 4953 */ 4954 CONTRIBUTOR, 4955 /** 4956 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 4957 */ 4958 COUNT, 4959 /** 4960 * Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data. 4961 */ 4962 DATAREQUIREMENT, 4963 /** 4964 * A length - a value with a unit that is a physical distance. 4965 */ 4966 DISTANCE, 4967 /** 4968 * Indicates how the medication is/was taken or should be taken by the patient. 4969 */ 4970 DOSAGE, 4971 /** 4972 * A length of time. 4973 */ 4974 DURATION, 4975 /** 4976 * Base definition for all elements in a resource. 4977 */ 4978 ELEMENT, 4979 /** 4980 * Captures constraints on each element within the resource, profile, or extension. 4981 */ 4982 ELEMENTDEFINITION, 4983 /** 4984 * Optional Extension Element - found in all resources. 4985 */ 4986 EXTENSION, 4987 /** 4988 * A human's name with the ability to identify parts and usage. 4989 */ 4990 HUMANNAME, 4991 /** 4992 * A technical identifier - identifies some entity uniquely and unambiguously. 4993 */ 4994 IDENTIFIER, 4995 /** 4996 * The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource. 4997 */ 4998 META, 4999 /** 5000 * An amount of economic utility in some recognized currency. 5001 */ 5002 MONEY, 5003 /** 5004 * A human-readable formatted text, including images. 5005 */ 5006 NARRATIVE, 5007 /** 5008 * The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse. 5009 */ 5010 PARAMETERDEFINITION, 5011 /** 5012 * A time period defined by a start and end date and optionally time. 5013 */ 5014 PERIOD, 5015 /** 5016 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 5017 */ 5018 QUANTITY, 5019 /** 5020 * A set of ordered Quantities defined by a low and high limit. 5021 */ 5022 RANGE, 5023 /** 5024 * A relationship of two Quantity values - expressed as a numerator and a denominator. 5025 */ 5026 RATIO, 5027 /** 5028 * A reference from one resource to another. 5029 */ 5030 REFERENCE, 5031 /** 5032 * Related artifacts such as additional documentation, justification, or bibliographic references. 5033 */ 5034 RELATEDARTIFACT, 5035 /** 5036 * A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data. 5037 */ 5038 SAMPLEDDATA, 5039 /** 5040 * A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities. 5041 */ 5042 SIGNATURE, 5043 /** 5044 * null 5045 */ 5046 SIMPLEQUANTITY, 5047 /** 5048 * Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out. 5049 */ 5050 TIMING, 5051 /** 5052 * A description of a triggering event. 5053 */ 5054 TRIGGERDEFINITION, 5055 /** 5056 * Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care). 5057 */ 5058 USAGECONTEXT, 5059 /** 5060 * A stream of bytes 5061 */ 5062 BASE64BINARY, 5063 /** 5064 * Value of "true" or "false" 5065 */ 5066 BOOLEAN, 5067 /** 5068 * A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents 5069 */ 5070 CODE, 5071 /** 5072 * A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates. 5073 */ 5074 DATE, 5075 /** 5076 * A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates. 5077 */ 5078 DATETIME, 5079 /** 5080 * A rational number with implicit precision 5081 */ 5082 DECIMAL, 5083 /** 5084 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive. 5085 */ 5086 ID, 5087 /** 5088 * An instant in time - known at least to the second 5089 */ 5090 INSTANT, 5091 /** 5092 * A whole number 5093 */ 5094 INTEGER, 5095 /** 5096 * A string that may contain markdown syntax for optional processing by a mark down presentation engine 5097 */ 5098 MARKDOWN, 5099 /** 5100 * An OID represented as a URI 5101 */ 5102 OID, 5103 /** 5104 * An integer with a value that is positive (e.g. >0) 5105 */ 5106 POSITIVEINT, 5107 /** 5108 * A sequence of Unicode characters 5109 */ 5110 STRING, 5111 /** 5112 * A time during the day, with no date specified 5113 */ 5114 TIME, 5115 /** 5116 * An integer with a value that is not negative (e.g. >= 0) 5117 */ 5118 UNSIGNEDINT, 5119 /** 5120 * String of characters used to identify a name or a resource 5121 */ 5122 URI, 5123 /** 5124 * A UUID, represented as a URI 5125 */ 5126 UUID, 5127 /** 5128 * XHTML format, as defined by W3C, but restricted usage (mainly, no active content) 5129 */ 5130 XHTML, 5131 /** 5132 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 5133 */ 5134 ACCOUNT, 5135 /** 5136 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 5137 */ 5138 ACTIVITYDEFINITION, 5139 /** 5140 * Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death. 5141 */ 5142 ADVERSEEVENT, 5143 /** 5144 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 5145 */ 5146 ALLERGYINTOLERANCE, 5147 /** 5148 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 5149 */ 5150 APPOINTMENT, 5151 /** 5152 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 5153 */ 5154 APPOINTMENTRESPONSE, 5155 /** 5156 * A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage. 5157 */ 5158 AUDITEVENT, 5159 /** 5160 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 5161 */ 5162 BASIC, 5163 /** 5164 * A binary resource can contain any content, whether text, image, pdf, zip archive, etc. 5165 */ 5166 BINARY, 5167 /** 5168 * Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 5169 */ 5170 BODYSITE, 5171 /** 5172 * A container for a collection of resources. 5173 */ 5174 BUNDLE, 5175 /** 5176 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 5177 */ 5178 CAPABILITYSTATEMENT, 5179 /** 5180 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 5181 */ 5182 CAREPLAN, 5183 /** 5184 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 5185 */ 5186 CARETEAM, 5187 /** 5188 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 5189 */ 5190 CHARGEITEM, 5191 /** 5192 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 5193 */ 5194 CLAIM, 5195 /** 5196 * This resource provides the adjudication details from the processing of a Claim resource. 5197 */ 5198 CLAIMRESPONSE, 5199 /** 5200 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 5201 */ 5202 CLINICALIMPRESSION, 5203 /** 5204 * A code system resource specifies a set of codes drawn from one or more code systems. 5205 */ 5206 CODESYSTEM, 5207 /** 5208 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 5209 */ 5210 COMMUNICATION, 5211 /** 5212 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 5213 */ 5214 COMMUNICATIONREQUEST, 5215 /** 5216 * A compartment definition that defines how resources are accessed on a server. 5217 */ 5218 COMPARTMENTDEFINITION, 5219 /** 5220 * A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained. 5221 */ 5222 COMPOSITION, 5223 /** 5224 * A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models. 5225 */ 5226 CONCEPTMAP, 5227 /** 5228 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 5229 */ 5230 CONDITION, 5231 /** 5232 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 5233 */ 5234 CONSENT, 5235 /** 5236 * A formal agreement between parties regarding the conduct of business, exchange of information or other matters. 5237 */ 5238 CONTRACT, 5239 /** 5240 * Financial instrument which may be used to reimburse or pay for health care products and services. 5241 */ 5242 COVERAGE, 5243 /** 5244 * The formal description of a single piece of information that can be gathered and reported. 5245 */ 5246 DATAELEMENT, 5247 /** 5248 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 5249 */ 5250 DETECTEDISSUE, 5251 /** 5252 * This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc. 5253 */ 5254 DEVICE, 5255 /** 5256 * The characteristics, operational status and capabilities of a medical-related component of a medical device. 5257 */ 5258 DEVICECOMPONENT, 5259 /** 5260 * Describes a measurement, calculation or setting capability of a medical device. 5261 */ 5262 DEVICEMETRIC, 5263 /** 5264 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 5265 */ 5266 DEVICEREQUEST, 5267 /** 5268 * A record of a device being used by a patient where the record is the result of a report from the patient or another clinician. 5269 */ 5270 DEVICEUSESTATEMENT, 5271 /** 5272 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 5273 */ 5274 DIAGNOSTICREPORT, 5275 /** 5276 * A collection of documents compiled for a purpose together with metadata that applies to the collection. 5277 */ 5278 DOCUMENTMANIFEST, 5279 /** 5280 * A reference to a document. 5281 */ 5282 DOCUMENTREFERENCE, 5283 /** 5284 * A resource that includes narrative, extensions, and contained resources. 5285 */ 5286 DOMAINRESOURCE, 5287 /** 5288 * The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 5289 */ 5290 ELIGIBILITYREQUEST, 5291 /** 5292 * This resource provides eligibility and plan details from the processing of an Eligibility resource. 5293 */ 5294 ELIGIBILITYRESPONSE, 5295 /** 5296 * An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient. 5297 */ 5298 ENCOUNTER, 5299 /** 5300 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 5301 */ 5302 ENDPOINT, 5303 /** 5304 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 5305 */ 5306 ENROLLMENTREQUEST, 5307 /** 5308 * This resource provides enrollment and plan details from the processing of an Enrollment resource. 5309 */ 5310 ENROLLMENTRESPONSE, 5311 /** 5312 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 5313 */ 5314 EPISODEOFCARE, 5315 /** 5316 * Resource to define constraints on the Expansion of a FHIR ValueSet. 5317 */ 5318 EXPANSIONPROFILE, 5319 /** 5320 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 5321 */ 5322 EXPLANATIONOFBENEFIT, 5323 /** 5324 * Significant health events and conditions for a person related to the patient relevant in the context of care for the patient. 5325 */ 5326 FAMILYMEMBERHISTORY, 5327 /** 5328 * Prospective warnings of potential issues when providing care to the patient. 5329 */ 5330 FLAG, 5331 /** 5332 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 5333 */ 5334 GOAL, 5335 /** 5336 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 5337 */ 5338 GRAPHDEFINITION, 5339 /** 5340 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 5341 */ 5342 GROUP, 5343 /** 5344 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 5345 */ 5346 GUIDANCERESPONSE, 5347 /** 5348 * The details of a healthcare service available at a location. 5349 */ 5350 HEALTHCARESERVICE, 5351 /** 5352 * A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection. 5353 */ 5354 IMAGINGMANIFEST, 5355 /** 5356 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 5357 */ 5358 IMAGINGSTUDY, 5359 /** 5360 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 5361 */ 5362 IMMUNIZATION, 5363 /** 5364 * A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification. 5365 */ 5366 IMMUNIZATIONRECOMMENDATION, 5367 /** 5368 * A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 5369 */ 5370 IMPLEMENTATIONGUIDE, 5371 /** 5372 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 5373 */ 5374 LIBRARY, 5375 /** 5376 * Identifies two or more records (resource instances) that are referring to the same real-world "occurrence". 5377 */ 5378 LINKAGE, 5379 /** 5380 * A set of information summarized from a list of other resources. 5381 */ 5382 LIST, 5383 /** 5384 * Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated. 5385 */ 5386 LOCATION, 5387 /** 5388 * The Measure resource provides the definition of a quality measure. 5389 */ 5390 MEASURE, 5391 /** 5392 * The MeasureReport resource contains the results of evaluating a measure. 5393 */ 5394 MEASUREREPORT, 5395 /** 5396 * A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference. 5397 */ 5398 MEDIA, 5399 /** 5400 * This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication. 5401 */ 5402 MEDICATION, 5403 /** 5404 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. 5405 */ 5406 MEDICATIONADMINISTRATION, 5407 /** 5408 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 5409 */ 5410 MEDICATIONDISPENSE, 5411 /** 5412 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 5413 */ 5414 MEDICATIONREQUEST, 5415 /** 5416 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 5417 5418The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 5419 */ 5420 MEDICATIONSTATEMENT, 5421 /** 5422 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 5423 */ 5424 MESSAGEDEFINITION, 5425 /** 5426 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 5427 */ 5428 MESSAGEHEADER, 5429 /** 5430 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 5431 */ 5432 NAMINGSYSTEM, 5433 /** 5434 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 5435 */ 5436 NUTRITIONORDER, 5437 /** 5438 * Measurements and simple assertions made about a patient, device or other subject. 5439 */ 5440 OBSERVATION, 5441 /** 5442 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 5443 */ 5444 OPERATIONDEFINITION, 5445 /** 5446 * A collection of error, warning or information messages that result from a system action. 5447 */ 5448 OPERATIONOUTCOME, 5449 /** 5450 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc. 5451 */ 5452 ORGANIZATION, 5453 /** 5454 * This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it. 5455 */ 5456 PARAMETERS, 5457 /** 5458 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 5459 */ 5460 PATIENT, 5461 /** 5462 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 5463 */ 5464 PAYMENTNOTICE, 5465 /** 5466 * This resource provides payment details and claim references supporting a bulk payment. 5467 */ 5468 PAYMENTRECONCILIATION, 5469 /** 5470 * Demographics and administrative information about a person independent of a specific health-related context. 5471 */ 5472 PERSON, 5473 /** 5474 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols. 5475 */ 5476 PLANDEFINITION, 5477 /** 5478 * A person who is directly or indirectly involved in the provisioning of healthcare. 5479 */ 5480 PRACTITIONER, 5481 /** 5482 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 5483 */ 5484 PRACTITIONERROLE, 5485 /** 5486 * An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy. 5487 */ 5488 PROCEDURE, 5489 /** 5490 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 5491 */ 5492 PROCEDUREREQUEST, 5493 /** 5494 * This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources. 5495 */ 5496 PROCESSREQUEST, 5497 /** 5498 * This resource provides processing status, errors and notes from the processing of a resource. 5499 */ 5500 PROCESSRESPONSE, 5501 /** 5502 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 5503 */ 5504 PROVENANCE, 5505 /** 5506 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 5507 */ 5508 QUESTIONNAIRE, 5509 /** 5510 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 5511 */ 5512 QUESTIONNAIRERESPONSE, 5513 /** 5514 * Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization. 5515 */ 5516 REFERRALREQUEST, 5517 /** 5518 * Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 5519 */ 5520 RELATEDPERSON, 5521 /** 5522 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 5523 */ 5524 REQUESTGROUP, 5525 /** 5526 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 5527 */ 5528 RESEARCHSTUDY, 5529 /** 5530 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 5531 */ 5532 RESEARCHSUBJECT, 5533 /** 5534 * This is the base resource type for everything. 5535 */ 5536 RESOURCE, 5537 /** 5538 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 5539 */ 5540 RISKASSESSMENT, 5541 /** 5542 * A container for slots of time that may be available for booking appointments. 5543 */ 5544 SCHEDULE, 5545 /** 5546 * A search parameter that defines a named search item that can be used to search/filter on a resource. 5547 */ 5548 SEARCHPARAMETER, 5549 /** 5550 * Raw data describing a biological sequence. 5551 */ 5552 SEQUENCE, 5553 /** 5554 * The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking. 5555 */ 5556 SERVICEDEFINITION, 5557 /** 5558 * A slot of time on a schedule that may be available for booking appointments. 5559 */ 5560 SLOT, 5561 /** 5562 * A sample to be used for analysis. 5563 */ 5564 SPECIMEN, 5565 /** 5566 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 5567 */ 5568 STRUCTUREDEFINITION, 5569 /** 5570 * A Map of relationships between 2 structures that can be used to transform data. 5571 */ 5572 STRUCTUREMAP, 5573 /** 5574 * The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined "channel" so that another system is able to take an appropriate action. 5575 */ 5576 SUBSCRIPTION, 5577 /** 5578 * A homogeneous material with a definite composition. 5579 */ 5580 SUBSTANCE, 5581 /** 5582 * Record of delivery of what is supplied. 5583 */ 5584 SUPPLYDELIVERY, 5585 /** 5586 * A record of a request for a medication, substance or device used in the healthcare setting. 5587 */ 5588 SUPPLYREQUEST, 5589 /** 5590 * A task to be performed. 5591 */ 5592 TASK, 5593 /** 5594 * A summary of information based on the results of executing a TestScript. 5595 */ 5596 TESTREPORT, 5597 /** 5598 * A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification. 5599 */ 5600 TESTSCRIPT, 5601 /** 5602 * A value set specifies a set of codes drawn from one or more code systems. 5603 */ 5604 VALUESET, 5605 /** 5606 * An authorization for the supply of glasses and/or contact lenses to a patient. 5607 */ 5608 VISIONPRESCRIPTION, 5609 /** 5610 * added to help the parsers 5611 */ 5612 NULL; 5613 public static FHIRDefinedType fromCode(String codeString) throws FHIRException { 5614 if (codeString == null || "".equals(codeString)) 5615 return null; 5616 if ("Address".equals(codeString)) 5617 return ADDRESS; 5618 if ("Age".equals(codeString)) 5619 return AGE; 5620 if ("Annotation".equals(codeString)) 5621 return ANNOTATION; 5622 if ("Attachment".equals(codeString)) 5623 return ATTACHMENT; 5624 if ("BackboneElement".equals(codeString)) 5625 return BACKBONEELEMENT; 5626 if ("CodeableConcept".equals(codeString)) 5627 return CODEABLECONCEPT; 5628 if ("Coding".equals(codeString)) 5629 return CODING; 5630 if ("ContactDetail".equals(codeString)) 5631 return CONTACTDETAIL; 5632 if ("ContactPoint".equals(codeString)) 5633 return CONTACTPOINT; 5634 if ("Contributor".equals(codeString)) 5635 return CONTRIBUTOR; 5636 if ("Count".equals(codeString)) 5637 return COUNT; 5638 if ("DataRequirement".equals(codeString)) 5639 return DATAREQUIREMENT; 5640 if ("Distance".equals(codeString)) 5641 return DISTANCE; 5642 if ("Dosage".equals(codeString)) 5643 return DOSAGE; 5644 if ("Duration".equals(codeString)) 5645 return DURATION; 5646 if ("Element".equals(codeString)) 5647 return ELEMENT; 5648 if ("ElementDefinition".equals(codeString)) 5649 return ELEMENTDEFINITION; 5650 if ("Extension".equals(codeString)) 5651 return EXTENSION; 5652 if ("HumanName".equals(codeString)) 5653 return HUMANNAME; 5654 if ("Identifier".equals(codeString)) 5655 return IDENTIFIER; 5656 if ("Meta".equals(codeString)) 5657 return META; 5658 if ("Money".equals(codeString)) 5659 return MONEY; 5660 if ("Narrative".equals(codeString)) 5661 return NARRATIVE; 5662 if ("ParameterDefinition".equals(codeString)) 5663 return PARAMETERDEFINITION; 5664 if ("Period".equals(codeString)) 5665 return PERIOD; 5666 if ("Quantity".equals(codeString)) 5667 return QUANTITY; 5668 if ("Range".equals(codeString)) 5669 return RANGE; 5670 if ("Ratio".equals(codeString)) 5671 return RATIO; 5672 if ("Reference".equals(codeString)) 5673 return REFERENCE; 5674 if ("RelatedArtifact".equals(codeString)) 5675 return RELATEDARTIFACT; 5676 if ("SampledData".equals(codeString)) 5677 return SAMPLEDDATA; 5678 if ("Signature".equals(codeString)) 5679 return SIGNATURE; 5680 if ("SimpleQuantity".equals(codeString)) 5681 return SIMPLEQUANTITY; 5682 if ("Timing".equals(codeString)) 5683 return TIMING; 5684 if ("TriggerDefinition".equals(codeString)) 5685 return TRIGGERDEFINITION; 5686 if ("UsageContext".equals(codeString)) 5687 return USAGECONTEXT; 5688 if ("base64Binary".equals(codeString)) 5689 return BASE64BINARY; 5690 if ("boolean".equals(codeString)) 5691 return BOOLEAN; 5692 if ("code".equals(codeString)) 5693 return CODE; 5694 if ("date".equals(codeString)) 5695 return DATE; 5696 if ("dateTime".equals(codeString)) 5697 return DATETIME; 5698 if ("decimal".equals(codeString)) 5699 return DECIMAL; 5700 if ("id".equals(codeString)) 5701 return ID; 5702 if ("instant".equals(codeString)) 5703 return INSTANT; 5704 if ("integer".equals(codeString)) 5705 return INTEGER; 5706 if ("markdown".equals(codeString)) 5707 return MARKDOWN; 5708 if ("oid".equals(codeString)) 5709 return OID; 5710 if ("positiveInt".equals(codeString)) 5711 return POSITIVEINT; 5712 if ("string".equals(codeString)) 5713 return STRING; 5714 if ("time".equals(codeString)) 5715 return TIME; 5716 if ("unsignedInt".equals(codeString)) 5717 return UNSIGNEDINT; 5718 if ("uri".equals(codeString)) 5719 return URI; 5720 if ("uuid".equals(codeString)) 5721 return UUID; 5722 if ("xhtml".equals(codeString)) 5723 return XHTML; 5724 if ("Account".equals(codeString)) 5725 return ACCOUNT; 5726 if ("ActivityDefinition".equals(codeString)) 5727 return ACTIVITYDEFINITION; 5728 if ("AdverseEvent".equals(codeString)) 5729 return ADVERSEEVENT; 5730 if ("AllergyIntolerance".equals(codeString)) 5731 return ALLERGYINTOLERANCE; 5732 if ("Appointment".equals(codeString)) 5733 return APPOINTMENT; 5734 if ("AppointmentResponse".equals(codeString)) 5735 return APPOINTMENTRESPONSE; 5736 if ("AuditEvent".equals(codeString)) 5737 return AUDITEVENT; 5738 if ("Basic".equals(codeString)) 5739 return BASIC; 5740 if ("Binary".equals(codeString)) 5741 return BINARY; 5742 if ("BodySite".equals(codeString)) 5743 return BODYSITE; 5744 if ("Bundle".equals(codeString)) 5745 return BUNDLE; 5746 if ("CapabilityStatement".equals(codeString)) 5747 return CAPABILITYSTATEMENT; 5748 if ("CarePlan".equals(codeString)) 5749 return CAREPLAN; 5750 if ("CareTeam".equals(codeString)) 5751 return CARETEAM; 5752 if ("ChargeItem".equals(codeString)) 5753 return CHARGEITEM; 5754 if ("Claim".equals(codeString)) 5755 return CLAIM; 5756 if ("ClaimResponse".equals(codeString)) 5757 return CLAIMRESPONSE; 5758 if ("ClinicalImpression".equals(codeString)) 5759 return CLINICALIMPRESSION; 5760 if ("CodeSystem".equals(codeString)) 5761 return CODESYSTEM; 5762 if ("Communication".equals(codeString)) 5763 return COMMUNICATION; 5764 if ("CommunicationRequest".equals(codeString)) 5765 return COMMUNICATIONREQUEST; 5766 if ("CompartmentDefinition".equals(codeString)) 5767 return COMPARTMENTDEFINITION; 5768 if ("Composition".equals(codeString)) 5769 return COMPOSITION; 5770 if ("ConceptMap".equals(codeString)) 5771 return CONCEPTMAP; 5772 if ("Condition".equals(codeString)) 5773 return CONDITION; 5774 if ("Consent".equals(codeString)) 5775 return CONSENT; 5776 if ("Contract".equals(codeString)) 5777 return CONTRACT; 5778 if ("Coverage".equals(codeString)) 5779 return COVERAGE; 5780 if ("DataElement".equals(codeString)) 5781 return DATAELEMENT; 5782 if ("DetectedIssue".equals(codeString)) 5783 return DETECTEDISSUE; 5784 if ("Device".equals(codeString)) 5785 return DEVICE; 5786 if ("DeviceComponent".equals(codeString)) 5787 return DEVICECOMPONENT; 5788 if ("DeviceMetric".equals(codeString)) 5789 return DEVICEMETRIC; 5790 if ("DeviceRequest".equals(codeString)) 5791 return DEVICEREQUEST; 5792 if ("DeviceUseStatement".equals(codeString)) 5793 return DEVICEUSESTATEMENT; 5794 if ("DiagnosticReport".equals(codeString)) 5795 return DIAGNOSTICREPORT; 5796 if ("DocumentManifest".equals(codeString)) 5797 return DOCUMENTMANIFEST; 5798 if ("DocumentReference".equals(codeString)) 5799 return DOCUMENTREFERENCE; 5800 if ("DomainResource".equals(codeString)) 5801 return DOMAINRESOURCE; 5802 if ("EligibilityRequest".equals(codeString)) 5803 return ELIGIBILITYREQUEST; 5804 if ("EligibilityResponse".equals(codeString)) 5805 return ELIGIBILITYRESPONSE; 5806 if ("Encounter".equals(codeString)) 5807 return ENCOUNTER; 5808 if ("Endpoint".equals(codeString)) 5809 return ENDPOINT; 5810 if ("EnrollmentRequest".equals(codeString)) 5811 return ENROLLMENTREQUEST; 5812 if ("EnrollmentResponse".equals(codeString)) 5813 return ENROLLMENTRESPONSE; 5814 if ("EpisodeOfCare".equals(codeString)) 5815 return EPISODEOFCARE; 5816 if ("ExpansionProfile".equals(codeString)) 5817 return EXPANSIONPROFILE; 5818 if ("ExplanationOfBenefit".equals(codeString)) 5819 return EXPLANATIONOFBENEFIT; 5820 if ("FamilyMemberHistory".equals(codeString)) 5821 return FAMILYMEMBERHISTORY; 5822 if ("Flag".equals(codeString)) 5823 return FLAG; 5824 if ("Goal".equals(codeString)) 5825 return GOAL; 5826 if ("GraphDefinition".equals(codeString)) 5827 return GRAPHDEFINITION; 5828 if ("Group".equals(codeString)) 5829 return GROUP; 5830 if ("GuidanceResponse".equals(codeString)) 5831 return GUIDANCERESPONSE; 5832 if ("HealthcareService".equals(codeString)) 5833 return HEALTHCARESERVICE; 5834 if ("ImagingManifest".equals(codeString)) 5835 return IMAGINGMANIFEST; 5836 if ("ImagingStudy".equals(codeString)) 5837 return IMAGINGSTUDY; 5838 if ("Immunization".equals(codeString)) 5839 return IMMUNIZATION; 5840 if ("ImmunizationRecommendation".equals(codeString)) 5841 return IMMUNIZATIONRECOMMENDATION; 5842 if ("ImplementationGuide".equals(codeString)) 5843 return IMPLEMENTATIONGUIDE; 5844 if ("Library".equals(codeString)) 5845 return LIBRARY; 5846 if ("Linkage".equals(codeString)) 5847 return LINKAGE; 5848 if ("List".equals(codeString)) 5849 return LIST; 5850 if ("Location".equals(codeString)) 5851 return LOCATION; 5852 if ("Measure".equals(codeString)) 5853 return MEASURE; 5854 if ("MeasureReport".equals(codeString)) 5855 return MEASUREREPORT; 5856 if ("Media".equals(codeString)) 5857 return MEDIA; 5858 if ("Medication".equals(codeString)) 5859 return MEDICATION; 5860 if ("MedicationAdministration".equals(codeString)) 5861 return MEDICATIONADMINISTRATION; 5862 if ("MedicationDispense".equals(codeString)) 5863 return MEDICATIONDISPENSE; 5864 if ("MedicationRequest".equals(codeString)) 5865 return MEDICATIONREQUEST; 5866 if ("MedicationStatement".equals(codeString)) 5867 return MEDICATIONSTATEMENT; 5868 if ("MessageDefinition".equals(codeString)) 5869 return MESSAGEDEFINITION; 5870 if ("MessageHeader".equals(codeString)) 5871 return MESSAGEHEADER; 5872 if ("NamingSystem".equals(codeString)) 5873 return NAMINGSYSTEM; 5874 if ("NutritionOrder".equals(codeString)) 5875 return NUTRITIONORDER; 5876 if ("Observation".equals(codeString)) 5877 return OBSERVATION; 5878 if ("OperationDefinition".equals(codeString)) 5879 return OPERATIONDEFINITION; 5880 if ("OperationOutcome".equals(codeString)) 5881 return OPERATIONOUTCOME; 5882 if ("Organization".equals(codeString)) 5883 return ORGANIZATION; 5884 if ("Parameters".equals(codeString)) 5885 return PARAMETERS; 5886 if ("Patient".equals(codeString)) 5887 return PATIENT; 5888 if ("PaymentNotice".equals(codeString)) 5889 return PAYMENTNOTICE; 5890 if ("PaymentReconciliation".equals(codeString)) 5891 return PAYMENTRECONCILIATION; 5892 if ("Person".equals(codeString)) 5893 return PERSON; 5894 if ("PlanDefinition".equals(codeString)) 5895 return PLANDEFINITION; 5896 if ("Practitioner".equals(codeString)) 5897 return PRACTITIONER; 5898 if ("PractitionerRole".equals(codeString)) 5899 return PRACTITIONERROLE; 5900 if ("Procedure".equals(codeString)) 5901 return PROCEDURE; 5902 if ("ProcedureRequest".equals(codeString)) 5903 return PROCEDUREREQUEST; 5904 if ("ProcessRequest".equals(codeString)) 5905 return PROCESSREQUEST; 5906 if ("ProcessResponse".equals(codeString)) 5907 return PROCESSRESPONSE; 5908 if ("Provenance".equals(codeString)) 5909 return PROVENANCE; 5910 if ("Questionnaire".equals(codeString)) 5911 return QUESTIONNAIRE; 5912 if ("QuestionnaireResponse".equals(codeString)) 5913 return QUESTIONNAIRERESPONSE; 5914 if ("ReferralRequest".equals(codeString)) 5915 return REFERRALREQUEST; 5916 if ("RelatedPerson".equals(codeString)) 5917 return RELATEDPERSON; 5918 if ("RequestGroup".equals(codeString)) 5919 return REQUESTGROUP; 5920 if ("ResearchStudy".equals(codeString)) 5921 return RESEARCHSTUDY; 5922 if ("ResearchSubject".equals(codeString)) 5923 return RESEARCHSUBJECT; 5924 if ("Resource".equals(codeString)) 5925 return RESOURCE; 5926 if ("RiskAssessment".equals(codeString)) 5927 return RISKASSESSMENT; 5928 if ("Schedule".equals(codeString)) 5929 return SCHEDULE; 5930 if ("SearchParameter".equals(codeString)) 5931 return SEARCHPARAMETER; 5932 if ("Sequence".equals(codeString)) 5933 return SEQUENCE; 5934 if ("ServiceDefinition".equals(codeString)) 5935 return SERVICEDEFINITION; 5936 if ("Slot".equals(codeString)) 5937 return SLOT; 5938 if ("Specimen".equals(codeString)) 5939 return SPECIMEN; 5940 if ("StructureDefinition".equals(codeString)) 5941 return STRUCTUREDEFINITION; 5942 if ("StructureMap".equals(codeString)) 5943 return STRUCTUREMAP; 5944 if ("Subscription".equals(codeString)) 5945 return SUBSCRIPTION; 5946 if ("Substance".equals(codeString)) 5947 return SUBSTANCE; 5948 if ("SupplyDelivery".equals(codeString)) 5949 return SUPPLYDELIVERY; 5950 if ("SupplyRequest".equals(codeString)) 5951 return SUPPLYREQUEST; 5952 if ("Task".equals(codeString)) 5953 return TASK; 5954 if ("TestReport".equals(codeString)) 5955 return TESTREPORT; 5956 if ("TestScript".equals(codeString)) 5957 return TESTSCRIPT; 5958 if ("ValueSet".equals(codeString)) 5959 return VALUESET; 5960 if ("VisionPrescription".equals(codeString)) 5961 return VISIONPRESCRIPTION; 5962 throw new FHIRException("Unknown FHIRDefinedType code '"+codeString+"'"); 5963 } 5964 public String toCode() { 5965 switch (this) { 5966 case ADDRESS: return "Address"; 5967 case AGE: return "Age"; 5968 case ANNOTATION: return "Annotation"; 5969 case ATTACHMENT: return "Attachment"; 5970 case BACKBONEELEMENT: return "BackboneElement"; 5971 case CODEABLECONCEPT: return "CodeableConcept"; 5972 case CODING: return "Coding"; 5973 case CONTACTDETAIL: return "ContactDetail"; 5974 case CONTACTPOINT: return "ContactPoint"; 5975 case CONTRIBUTOR: return "Contributor"; 5976 case COUNT: return "Count"; 5977 case DATAREQUIREMENT: return "DataRequirement"; 5978 case DISTANCE: return "Distance"; 5979 case DOSAGE: return "Dosage"; 5980 case DURATION: return "Duration"; 5981 case ELEMENT: return "Element"; 5982 case ELEMENTDEFINITION: return "ElementDefinition"; 5983 case EXTENSION: return "Extension"; 5984 case HUMANNAME: return "HumanName"; 5985 case IDENTIFIER: return "Identifier"; 5986 case META: return "Meta"; 5987 case MONEY: return "Money"; 5988 case NARRATIVE: return "Narrative"; 5989 case PARAMETERDEFINITION: return "ParameterDefinition"; 5990 case PERIOD: return "Period"; 5991 case QUANTITY: return "Quantity"; 5992 case RANGE: return "Range"; 5993 case RATIO: return "Ratio"; 5994 case REFERENCE: return "Reference"; 5995 case RELATEDARTIFACT: return "RelatedArtifact"; 5996 case SAMPLEDDATA: return "SampledData"; 5997 case SIGNATURE: return "Signature"; 5998 case SIMPLEQUANTITY: return "SimpleQuantity"; 5999 case TIMING: return "Timing"; 6000 case TRIGGERDEFINITION: return "TriggerDefinition"; 6001 case USAGECONTEXT: return "UsageContext"; 6002 case BASE64BINARY: return "base64Binary"; 6003 case BOOLEAN: return "boolean"; 6004 case CODE: return "code"; 6005 case DATE: return "date"; 6006 case DATETIME: return "dateTime"; 6007 case DECIMAL: return "decimal"; 6008 case ID: return "id"; 6009 case INSTANT: return "instant"; 6010 case INTEGER: return "integer"; 6011 case MARKDOWN: return "markdown"; 6012 case OID: return "oid"; 6013 case POSITIVEINT: return "positiveInt"; 6014 case STRING: return "string"; 6015 case TIME: return "time"; 6016 case UNSIGNEDINT: return "unsignedInt"; 6017 case URI: return "uri"; 6018 case UUID: return "uuid"; 6019 case XHTML: return "xhtml"; 6020 case ACCOUNT: return "Account"; 6021 case ACTIVITYDEFINITION: return "ActivityDefinition"; 6022 case ADVERSEEVENT: return "AdverseEvent"; 6023 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 6024 case APPOINTMENT: return "Appointment"; 6025 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 6026 case AUDITEVENT: return "AuditEvent"; 6027 case BASIC: return "Basic"; 6028 case BINARY: return "Binary"; 6029 case BODYSITE: return "BodySite"; 6030 case BUNDLE: return "Bundle"; 6031 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 6032 case CAREPLAN: return "CarePlan"; 6033 case CARETEAM: return "CareTeam"; 6034 case CHARGEITEM: return "ChargeItem"; 6035 case CLAIM: return "Claim"; 6036 case CLAIMRESPONSE: return "ClaimResponse"; 6037 case CLINICALIMPRESSION: return "ClinicalImpression"; 6038 case CODESYSTEM: return "CodeSystem"; 6039 case COMMUNICATION: return "Communication"; 6040 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 6041 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 6042 case COMPOSITION: return "Composition"; 6043 case CONCEPTMAP: return "ConceptMap"; 6044 case CONDITION: return "Condition"; 6045 case CONSENT: return "Consent"; 6046 case CONTRACT: return "Contract"; 6047 case COVERAGE: return "Coverage"; 6048 case DATAELEMENT: return "DataElement"; 6049 case DETECTEDISSUE: return "DetectedIssue"; 6050 case DEVICE: return "Device"; 6051 case DEVICECOMPONENT: return "DeviceComponent"; 6052 case DEVICEMETRIC: return "DeviceMetric"; 6053 case DEVICEREQUEST: return "DeviceRequest"; 6054 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 6055 case DIAGNOSTICREPORT: return "DiagnosticReport"; 6056 case DOCUMENTMANIFEST: return "DocumentManifest"; 6057 case DOCUMENTREFERENCE: return "DocumentReference"; 6058 case DOMAINRESOURCE: return "DomainResource"; 6059 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 6060 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 6061 case ENCOUNTER: return "Encounter"; 6062 case ENDPOINT: return "Endpoint"; 6063 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 6064 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 6065 case EPISODEOFCARE: return "EpisodeOfCare"; 6066 case EXPANSIONPROFILE: return "ExpansionProfile"; 6067 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 6068 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 6069 case FLAG: return "Flag"; 6070 case GOAL: return "Goal"; 6071 case GRAPHDEFINITION: return "GraphDefinition"; 6072 case GROUP: return "Group"; 6073 case GUIDANCERESPONSE: return "GuidanceResponse"; 6074 case HEALTHCARESERVICE: return "HealthcareService"; 6075 case IMAGINGMANIFEST: return "ImagingManifest"; 6076 case IMAGINGSTUDY: return "ImagingStudy"; 6077 case IMMUNIZATION: return "Immunization"; 6078 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 6079 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 6080 case LIBRARY: return "Library"; 6081 case LINKAGE: return "Linkage"; 6082 case LIST: return "List"; 6083 case LOCATION: return "Location"; 6084 case MEASURE: return "Measure"; 6085 case MEASUREREPORT: return "MeasureReport"; 6086 case MEDIA: return "Media"; 6087 case MEDICATION: return "Medication"; 6088 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 6089 case MEDICATIONDISPENSE: return "MedicationDispense"; 6090 case MEDICATIONREQUEST: return "MedicationRequest"; 6091 case MEDICATIONSTATEMENT: return "MedicationStatement"; 6092 case MESSAGEDEFINITION: return "MessageDefinition"; 6093 case MESSAGEHEADER: return "MessageHeader"; 6094 case NAMINGSYSTEM: return "NamingSystem"; 6095 case NUTRITIONORDER: return "NutritionOrder"; 6096 case OBSERVATION: return "Observation"; 6097 case OPERATIONDEFINITION: return "OperationDefinition"; 6098 case OPERATIONOUTCOME: return "OperationOutcome"; 6099 case ORGANIZATION: return "Organization"; 6100 case PARAMETERS: return "Parameters"; 6101 case PATIENT: return "Patient"; 6102 case PAYMENTNOTICE: return "PaymentNotice"; 6103 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 6104 case PERSON: return "Person"; 6105 case PLANDEFINITION: return "PlanDefinition"; 6106 case PRACTITIONER: return "Practitioner"; 6107 case PRACTITIONERROLE: return "PractitionerRole"; 6108 case PROCEDURE: return "Procedure"; 6109 case PROCEDUREREQUEST: return "ProcedureRequest"; 6110 case PROCESSREQUEST: return "ProcessRequest"; 6111 case PROCESSRESPONSE: return "ProcessResponse"; 6112 case PROVENANCE: return "Provenance"; 6113 case QUESTIONNAIRE: return "Questionnaire"; 6114 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 6115 case REFERRALREQUEST: return "ReferralRequest"; 6116 case RELATEDPERSON: return "RelatedPerson"; 6117 case REQUESTGROUP: return "RequestGroup"; 6118 case RESEARCHSTUDY: return "ResearchStudy"; 6119 case RESEARCHSUBJECT: return "ResearchSubject"; 6120 case RESOURCE: return "Resource"; 6121 case RISKASSESSMENT: return "RiskAssessment"; 6122 case SCHEDULE: return "Schedule"; 6123 case SEARCHPARAMETER: return "SearchParameter"; 6124 case SEQUENCE: return "Sequence"; 6125 case SERVICEDEFINITION: return "ServiceDefinition"; 6126 case SLOT: return "Slot"; 6127 case SPECIMEN: return "Specimen"; 6128 case STRUCTUREDEFINITION: return "StructureDefinition"; 6129 case STRUCTUREMAP: return "StructureMap"; 6130 case SUBSCRIPTION: return "Subscription"; 6131 case SUBSTANCE: return "Substance"; 6132 case SUPPLYDELIVERY: return "SupplyDelivery"; 6133 case SUPPLYREQUEST: return "SupplyRequest"; 6134 case TASK: return "Task"; 6135 case TESTREPORT: return "TestReport"; 6136 case TESTSCRIPT: return "TestScript"; 6137 case VALUESET: return "ValueSet"; 6138 case VISIONPRESCRIPTION: return "VisionPrescription"; 6139 case NULL: return null; 6140 default: return "?"; 6141 } 6142 } 6143 public String getSystem() { 6144 switch (this) { 6145 case ADDRESS: return "http://hl7.org/fhir/data-types"; 6146 case AGE: return "http://hl7.org/fhir/data-types"; 6147 case ANNOTATION: return "http://hl7.org/fhir/data-types"; 6148 case ATTACHMENT: return "http://hl7.org/fhir/data-types"; 6149 case BACKBONEELEMENT: return "http://hl7.org/fhir/data-types"; 6150 case CODEABLECONCEPT: return "http://hl7.org/fhir/data-types"; 6151 case CODING: return "http://hl7.org/fhir/data-types"; 6152 case CONTACTDETAIL: return "http://hl7.org/fhir/data-types"; 6153 case CONTACTPOINT: return "http://hl7.org/fhir/data-types"; 6154 case CONTRIBUTOR: return "http://hl7.org/fhir/data-types"; 6155 case COUNT: return "http://hl7.org/fhir/data-types"; 6156 case DATAREQUIREMENT: return "http://hl7.org/fhir/data-types"; 6157 case DISTANCE: return "http://hl7.org/fhir/data-types"; 6158 case DOSAGE: return "http://hl7.org/fhir/data-types"; 6159 case DURATION: return "http://hl7.org/fhir/data-types"; 6160 case ELEMENT: return "http://hl7.org/fhir/data-types"; 6161 case ELEMENTDEFINITION: return "http://hl7.org/fhir/data-types"; 6162 case EXTENSION: return "http://hl7.org/fhir/data-types"; 6163 case HUMANNAME: return "http://hl7.org/fhir/data-types"; 6164 case IDENTIFIER: return "http://hl7.org/fhir/data-types"; 6165 case META: return "http://hl7.org/fhir/data-types"; 6166 case MONEY: return "http://hl7.org/fhir/data-types"; 6167 case NARRATIVE: return "http://hl7.org/fhir/data-types"; 6168 case PARAMETERDEFINITION: return "http://hl7.org/fhir/data-types"; 6169 case PERIOD: return "http://hl7.org/fhir/data-types"; 6170 case QUANTITY: return "http://hl7.org/fhir/data-types"; 6171 case RANGE: return "http://hl7.org/fhir/data-types"; 6172 case RATIO: return "http://hl7.org/fhir/data-types"; 6173 case REFERENCE: return "http://hl7.org/fhir/data-types"; 6174 case RELATEDARTIFACT: return "http://hl7.org/fhir/data-types"; 6175 case SAMPLEDDATA: return "http://hl7.org/fhir/data-types"; 6176 case SIGNATURE: return "http://hl7.org/fhir/data-types"; 6177 case SIMPLEQUANTITY: return "http://hl7.org/fhir/data-types"; 6178 case TIMING: return "http://hl7.org/fhir/data-types"; 6179 case TRIGGERDEFINITION: return "http://hl7.org/fhir/data-types"; 6180 case USAGECONTEXT: return "http://hl7.org/fhir/data-types"; 6181 case BASE64BINARY: return "http://hl7.org/fhir/data-types"; 6182 case BOOLEAN: return "http://hl7.org/fhir/data-types"; 6183 case CODE: return "http://hl7.org/fhir/data-types"; 6184 case DATE: return "http://hl7.org/fhir/data-types"; 6185 case DATETIME: return "http://hl7.org/fhir/data-types"; 6186 case DECIMAL: return "http://hl7.org/fhir/data-types"; 6187 case ID: return "http://hl7.org/fhir/data-types"; 6188 case INSTANT: return "http://hl7.org/fhir/data-types"; 6189 case INTEGER: return "http://hl7.org/fhir/data-types"; 6190 case MARKDOWN: return "http://hl7.org/fhir/data-types"; 6191 case OID: return "http://hl7.org/fhir/data-types"; 6192 case POSITIVEINT: return "http://hl7.org/fhir/data-types"; 6193 case STRING: return "http://hl7.org/fhir/data-types"; 6194 case TIME: return "http://hl7.org/fhir/data-types"; 6195 case UNSIGNEDINT: return "http://hl7.org/fhir/data-types"; 6196 case URI: return "http://hl7.org/fhir/data-types"; 6197 case UUID: return "http://hl7.org/fhir/data-types"; 6198 case XHTML: return "http://hl7.org/fhir/data-types"; 6199 case ACCOUNT: return "http://hl7.org/fhir/resource-types"; 6200 case ACTIVITYDEFINITION: return "http://hl7.org/fhir/resource-types"; 6201 case ADVERSEEVENT: return "http://hl7.org/fhir/resource-types"; 6202 case ALLERGYINTOLERANCE: return "http://hl7.org/fhir/resource-types"; 6203 case APPOINTMENT: return "http://hl7.org/fhir/resource-types"; 6204 case APPOINTMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 6205 case AUDITEVENT: return "http://hl7.org/fhir/resource-types"; 6206 case BASIC: return "http://hl7.org/fhir/resource-types"; 6207 case BINARY: return "http://hl7.org/fhir/resource-types"; 6208 case BODYSITE: return "http://hl7.org/fhir/resource-types"; 6209 case BUNDLE: return "http://hl7.org/fhir/resource-types"; 6210 case CAPABILITYSTATEMENT: return "http://hl7.org/fhir/resource-types"; 6211 case CAREPLAN: return "http://hl7.org/fhir/resource-types"; 6212 case CARETEAM: return "http://hl7.org/fhir/resource-types"; 6213 case CHARGEITEM: return "http://hl7.org/fhir/resource-types"; 6214 case CLAIM: return "http://hl7.org/fhir/resource-types"; 6215 case CLAIMRESPONSE: return "http://hl7.org/fhir/resource-types"; 6216 case CLINICALIMPRESSION: return "http://hl7.org/fhir/resource-types"; 6217 case CODESYSTEM: return "http://hl7.org/fhir/resource-types"; 6218 case COMMUNICATION: return "http://hl7.org/fhir/resource-types"; 6219 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 6220 case COMPARTMENTDEFINITION: return "http://hl7.org/fhir/resource-types"; 6221 case COMPOSITION: return "http://hl7.org/fhir/resource-types"; 6222 case CONCEPTMAP: return "http://hl7.org/fhir/resource-types"; 6223 case CONDITION: return "http://hl7.org/fhir/resource-types"; 6224 case CONSENT: return "http://hl7.org/fhir/resource-types"; 6225 case CONTRACT: return "http://hl7.org/fhir/resource-types"; 6226 case COVERAGE: return "http://hl7.org/fhir/resource-types"; 6227 case DATAELEMENT: return "http://hl7.org/fhir/resource-types"; 6228 case DETECTEDISSUE: return "http://hl7.org/fhir/resource-types"; 6229 case DEVICE: return "http://hl7.org/fhir/resource-types"; 6230 case DEVICECOMPONENT: return "http://hl7.org/fhir/resource-types"; 6231 case DEVICEMETRIC: return "http://hl7.org/fhir/resource-types"; 6232 case DEVICEREQUEST: return "http://hl7.org/fhir/resource-types"; 6233 case DEVICEUSESTATEMENT: return "http://hl7.org/fhir/resource-types"; 6234 case DIAGNOSTICREPORT: return "http://hl7.org/fhir/resource-types"; 6235 case DOCUMENTMANIFEST: return "http://hl7.org/fhir/resource-types"; 6236 case DOCUMENTREFERENCE: return "http://hl7.org/fhir/resource-types"; 6237 case DOMAINRESOURCE: return "http://hl7.org/fhir/resource-types"; 6238 case ELIGIBILITYREQUEST: return "http://hl7.org/fhir/resource-types"; 6239 case ELIGIBILITYRESPONSE: return "http://hl7.org/fhir/resource-types"; 6240 case ENCOUNTER: return "http://hl7.org/fhir/resource-types"; 6241 case ENDPOINT: return "http://hl7.org/fhir/resource-types"; 6242 case ENROLLMENTREQUEST: return "http://hl7.org/fhir/resource-types"; 6243 case ENROLLMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 6244 case EPISODEOFCARE: return "http://hl7.org/fhir/resource-types"; 6245 case EXPANSIONPROFILE: return "http://hl7.org/fhir/resource-types"; 6246 case EXPLANATIONOFBENEFIT: return "http://hl7.org/fhir/resource-types"; 6247 case FAMILYMEMBERHISTORY: return "http://hl7.org/fhir/resource-types"; 6248 case FLAG: return "http://hl7.org/fhir/resource-types"; 6249 case GOAL: return "http://hl7.org/fhir/resource-types"; 6250 case GRAPHDEFINITION: return "http://hl7.org/fhir/resource-types"; 6251 case GROUP: return "http://hl7.org/fhir/resource-types"; 6252 case GUIDANCERESPONSE: return "http://hl7.org/fhir/resource-types"; 6253 case HEALTHCARESERVICE: return "http://hl7.org/fhir/resource-types"; 6254 case IMAGINGMANIFEST: return "http://hl7.org/fhir/resource-types"; 6255 case IMAGINGSTUDY: return "http://hl7.org/fhir/resource-types"; 6256 case IMMUNIZATION: return "http://hl7.org/fhir/resource-types"; 6257 case IMMUNIZATIONRECOMMENDATION: return "http://hl7.org/fhir/resource-types"; 6258 case IMPLEMENTATIONGUIDE: return "http://hl7.org/fhir/resource-types"; 6259 case LIBRARY: return "http://hl7.org/fhir/resource-types"; 6260 case LINKAGE: return "http://hl7.org/fhir/resource-types"; 6261 case LIST: return "http://hl7.org/fhir/resource-types"; 6262 case LOCATION: return "http://hl7.org/fhir/resource-types"; 6263 case MEASURE: return "http://hl7.org/fhir/resource-types"; 6264 case MEASUREREPORT: return "http://hl7.org/fhir/resource-types"; 6265 case MEDIA: return "http://hl7.org/fhir/resource-types"; 6266 case MEDICATION: return "http://hl7.org/fhir/resource-types"; 6267 case MEDICATIONADMINISTRATION: return "http://hl7.org/fhir/resource-types"; 6268 case MEDICATIONDISPENSE: return "http://hl7.org/fhir/resource-types"; 6269 case MEDICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 6270 case MEDICATIONSTATEMENT: return "http://hl7.org/fhir/resource-types"; 6271 case MESSAGEDEFINITION: return "http://hl7.org/fhir/resource-types"; 6272 case MESSAGEHEADER: return "http://hl7.org/fhir/resource-types"; 6273 case NAMINGSYSTEM: return "http://hl7.org/fhir/resource-types"; 6274 case NUTRITIONORDER: return "http://hl7.org/fhir/resource-types"; 6275 case OBSERVATION: return "http://hl7.org/fhir/resource-types"; 6276 case OPERATIONDEFINITION: return "http://hl7.org/fhir/resource-types"; 6277 case OPERATIONOUTCOME: return "http://hl7.org/fhir/resource-types"; 6278 case ORGANIZATION: return "http://hl7.org/fhir/resource-types"; 6279 case PARAMETERS: return "http://hl7.org/fhir/resource-types"; 6280 case PATIENT: return "http://hl7.org/fhir/resource-types"; 6281 case PAYMENTNOTICE: return "http://hl7.org/fhir/resource-types"; 6282 case PAYMENTRECONCILIATION: return "http://hl7.org/fhir/resource-types"; 6283 case PERSON: return "http://hl7.org/fhir/resource-types"; 6284 case PLANDEFINITION: return "http://hl7.org/fhir/resource-types"; 6285 case PRACTITIONER: return "http://hl7.org/fhir/resource-types"; 6286 case PRACTITIONERROLE: return "http://hl7.org/fhir/resource-types"; 6287 case PROCEDURE: return "http://hl7.org/fhir/resource-types"; 6288 case PROCEDUREREQUEST: return "http://hl7.org/fhir/resource-types"; 6289 case PROCESSREQUEST: return "http://hl7.org/fhir/resource-types"; 6290 case PROCESSRESPONSE: return "http://hl7.org/fhir/resource-types"; 6291 case PROVENANCE: return "http://hl7.org/fhir/resource-types"; 6292 case QUESTIONNAIRE: return "http://hl7.org/fhir/resource-types"; 6293 case QUESTIONNAIRERESPONSE: return "http://hl7.org/fhir/resource-types"; 6294 case REFERRALREQUEST: return "http://hl7.org/fhir/resource-types"; 6295 case RELATEDPERSON: return "http://hl7.org/fhir/resource-types"; 6296 case REQUESTGROUP: return "http://hl7.org/fhir/resource-types"; 6297 case RESEARCHSTUDY: return "http://hl7.org/fhir/resource-types"; 6298 case RESEARCHSUBJECT: return "http://hl7.org/fhir/resource-types"; 6299 case RESOURCE: return "http://hl7.org/fhir/resource-types"; 6300 case RISKASSESSMENT: return "http://hl7.org/fhir/resource-types"; 6301 case SCHEDULE: return "http://hl7.org/fhir/resource-types"; 6302 case SEARCHPARAMETER: return "http://hl7.org/fhir/resource-types"; 6303 case SEQUENCE: return "http://hl7.org/fhir/resource-types"; 6304 case SERVICEDEFINITION: return "http://hl7.org/fhir/resource-types"; 6305 case SLOT: return "http://hl7.org/fhir/resource-types"; 6306 case SPECIMEN: return "http://hl7.org/fhir/resource-types"; 6307 case STRUCTUREDEFINITION: return "http://hl7.org/fhir/resource-types"; 6308 case STRUCTUREMAP: return "http://hl7.org/fhir/resource-types"; 6309 case SUBSCRIPTION: return "http://hl7.org/fhir/resource-types"; 6310 case SUBSTANCE: return "http://hl7.org/fhir/resource-types"; 6311 case SUPPLYDELIVERY: return "http://hl7.org/fhir/resource-types"; 6312 case SUPPLYREQUEST: return "http://hl7.org/fhir/resource-types"; 6313 case TASK: return "http://hl7.org/fhir/resource-types"; 6314 case TESTREPORT: return "http://hl7.org/fhir/resource-types"; 6315 case TESTSCRIPT: return "http://hl7.org/fhir/resource-types"; 6316 case VALUESET: return "http://hl7.org/fhir/resource-types"; 6317 case VISIONPRESCRIPTION: return "http://hl7.org/fhir/resource-types"; 6318 case NULL: return null; 6319 default: return "?"; 6320 } 6321 } 6322 public String getDefinition() { 6323 switch (this) { 6324 case ADDRESS: return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 6325 case AGE: return "A duration of time during which an organism (or a process) has existed."; 6326 case ANNOTATION: return "A text note which also contains information about who made the statement and when."; 6327 case ATTACHMENT: return "For referring to data content defined in other formats."; 6328 case BACKBONEELEMENT: return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 6329 case CODEABLECONCEPT: return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 6330 case CODING: return "A reference to a code defined by a terminology system."; 6331 case CONTACTDETAIL: return "Specifies contact information for a person or organization."; 6332 case CONTACTPOINT: return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 6333 case CONTRIBUTOR: return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 6334 case COUNT: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 6335 case DATAREQUIREMENT: return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 6336 case DISTANCE: return "A length - a value with a unit that is a physical distance."; 6337 case DOSAGE: return "Indicates how the medication is/was taken or should be taken by the patient."; 6338 case DURATION: return "A length of time."; 6339 case ELEMENT: return "Base definition for all elements in a resource."; 6340 case ELEMENTDEFINITION: return "Captures constraints on each element within the resource, profile, or extension."; 6341 case EXTENSION: return "Optional Extension Element - found in all resources."; 6342 case HUMANNAME: return "A human's name with the ability to identify parts and usage."; 6343 case IDENTIFIER: return "A technical identifier - identifies some entity uniquely and unambiguously."; 6344 case META: return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource."; 6345 case MONEY: return "An amount of economic utility in some recognized currency."; 6346 case NARRATIVE: return "A human-readable formatted text, including images."; 6347 case PARAMETERDEFINITION: return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 6348 case PERIOD: return "A time period defined by a start and end date and optionally time."; 6349 case QUANTITY: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 6350 case RANGE: return "A set of ordered Quantities defined by a low and high limit."; 6351 case RATIO: return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 6352 case REFERENCE: return "A reference from one resource to another."; 6353 case RELATEDARTIFACT: return "Related artifacts such as additional documentation, justification, or bibliographic references."; 6354 case SAMPLEDDATA: return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 6355 case SIGNATURE: return "A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities."; 6356 case SIMPLEQUANTITY: return ""; 6357 case TIMING: return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 6358 case TRIGGERDEFINITION: return "A description of a triggering event."; 6359 case USAGECONTEXT: return "Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 6360 case BASE64BINARY: return "A stream of bytes"; 6361 case BOOLEAN: return "Value of \"true\" or \"false\""; 6362 case CODE: return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 6363 case DATE: return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 6364 case DATETIME: return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 6365 case DECIMAL: return "A rational number with implicit precision"; 6366 case ID: return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 6367 case INSTANT: return "An instant in time - known at least to the second"; 6368 case INTEGER: return "A whole number"; 6369 case MARKDOWN: return "A string that may contain markdown syntax for optional processing by a mark down presentation engine"; 6370 case OID: return "An OID represented as a URI"; 6371 case POSITIVEINT: return "An integer with a value that is positive (e.g. >0)"; 6372 case STRING: return "A sequence of Unicode characters"; 6373 case TIME: return "A time during the day, with no date specified"; 6374 case UNSIGNEDINT: return "An integer with a value that is not negative (e.g. >= 0)"; 6375 case URI: return "String of characters used to identify a name or a resource"; 6376 case UUID: return "A UUID, represented as a URI"; 6377 case XHTML: return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 6378 case ACCOUNT: return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 6379 case ACTIVITYDEFINITION: return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 6380 case ADVERSEEVENT: return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 6381 case ALLERGYINTOLERANCE: return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 6382 case APPOINTMENT: return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 6383 case APPOINTMENTRESPONSE: return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 6384 case AUDITEVENT: return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 6385 case BASIC: return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 6386 case BINARY: return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 6387 case BODYSITE: return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 6388 case BUNDLE: return "A container for a collection of resources."; 6389 case CAPABILITYSTATEMENT: return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 6390 case CAREPLAN: return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 6391 case CARETEAM: return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 6392 case CHARGEITEM: return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 6393 case CLAIM: return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 6394 case CLAIMRESPONSE: return "This resource provides the adjudication details from the processing of a Claim resource."; 6395 case CLINICALIMPRESSION: return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 6396 case CODESYSTEM: return "A code system resource specifies a set of codes drawn from one or more code systems."; 6397 case COMMUNICATION: return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 6398 case COMMUNICATIONREQUEST: return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 6399 case COMPARTMENTDEFINITION: return "A compartment definition that defines how resources are accessed on a server."; 6400 case COMPOSITION: return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 6401 case CONCEPTMAP: return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 6402 case CONDITION: return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 6403 case CONSENT: return "A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 6404 case CONTRACT: return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 6405 case COVERAGE: return "Financial instrument which may be used to reimburse or pay for health care products and services."; 6406 case DATAELEMENT: return "The formal description of a single piece of information that can be gathered and reported."; 6407 case DETECTEDISSUE: return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 6408 case DEVICE: return "This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 6409 case DEVICECOMPONENT: return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 6410 case DEVICEMETRIC: return "Describes a measurement, calculation or setting capability of a medical device."; 6411 case DEVICEREQUEST: return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 6412 case DEVICEUSESTATEMENT: return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 6413 case DIAGNOSTICREPORT: return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 6414 case DOCUMENTMANIFEST: return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 6415 case DOCUMENTREFERENCE: return "A reference to a document."; 6416 case DOMAINRESOURCE: return "A resource that includes narrative, extensions, and contained resources."; 6417 case ELIGIBILITYREQUEST: return "The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 6418 case ELIGIBILITYRESPONSE: return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 6419 case ENCOUNTER: return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 6420 case ENDPOINT: return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 6421 case ENROLLMENTREQUEST: return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 6422 case ENROLLMENTRESPONSE: return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 6423 case EPISODEOFCARE: return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 6424 case EXPANSIONPROFILE: return "Resource to define constraints on the Expansion of a FHIR ValueSet."; 6425 case EXPLANATIONOFBENEFIT: return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 6426 case FAMILYMEMBERHISTORY: return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 6427 case FLAG: return "Prospective warnings of potential issues when providing care to the patient."; 6428 case GOAL: return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 6429 case GRAPHDEFINITION: return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 6430 case GROUP: return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 6431 case GUIDANCERESPONSE: return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 6432 case HEALTHCARESERVICE: return "The details of a healthcare service available at a location."; 6433 case IMAGINGMANIFEST: return "A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection."; 6434 case IMAGINGSTUDY: return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 6435 case IMMUNIZATION: return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 6436 case IMMUNIZATIONRECOMMENDATION: return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 6437 case IMPLEMENTATIONGUIDE: return "A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 6438 case LIBRARY: return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 6439 case LINKAGE: return "Identifies two or more records (resource instances) that are referring to the same real-world \"occurrence\"."; 6440 case LIST: return "A set of information summarized from a list of other resources."; 6441 case LOCATION: return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 6442 case MEASURE: return "The Measure resource provides the definition of a quality measure."; 6443 case MEASUREREPORT: return "The MeasureReport resource contains the results of evaluating a measure."; 6444 case MEDIA: return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 6445 case MEDICATION: return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 6446 case MEDICATIONADMINISTRATION: return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 6447 case MEDICATIONDISPENSE: return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 6448 case MEDICATIONREQUEST: return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 6449 case MEDICATIONSTATEMENT: return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 6450 case MESSAGEDEFINITION: return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 6451 case MESSAGEHEADER: return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 6452 case NAMINGSYSTEM: return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 6453 case NUTRITIONORDER: return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 6454 case OBSERVATION: return "Measurements and simple assertions made about a patient, device or other subject."; 6455 case OPERATIONDEFINITION: return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 6456 case OPERATIONOUTCOME: return "A collection of error, warning or information messages that result from a system action."; 6457 case ORGANIZATION: return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 6458 case PARAMETERS: return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 6459 case PATIENT: return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 6460 case PAYMENTNOTICE: return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 6461 case PAYMENTRECONCILIATION: return "This resource provides payment details and claim references supporting a bulk payment."; 6462 case PERSON: return "Demographics and administrative information about a person independent of a specific health-related context."; 6463 case PLANDEFINITION: return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 6464 case PRACTITIONER: return "A person who is directly or indirectly involved in the provisioning of healthcare."; 6465 case PRACTITIONERROLE: return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 6466 case PROCEDURE: return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 6467 case PROCEDUREREQUEST: return "A record of a request for diagnostic investigations, treatments, or operations to be performed."; 6468 case PROCESSREQUEST: return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 6469 case PROCESSRESPONSE: return "This resource provides processing status, errors and notes from the processing of a resource."; 6470 case PROVENANCE: return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 6471 case QUESTIONNAIRE: return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 6472 case QUESTIONNAIRERESPONSE: return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 6473 case REFERRALREQUEST: return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 6474 case RELATEDPERSON: return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 6475 case REQUESTGROUP: return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 6476 case RESEARCHSTUDY: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 6477 case RESEARCHSUBJECT: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 6478 case RESOURCE: return "This is the base resource type for everything."; 6479 case RISKASSESSMENT: return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 6480 case SCHEDULE: return "A container for slots of time that may be available for booking appointments."; 6481 case SEARCHPARAMETER: return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 6482 case SEQUENCE: return "Raw data describing a biological sequence."; 6483 case SERVICEDEFINITION: return "The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking."; 6484 case SLOT: return "A slot of time on a schedule that may be available for booking appointments."; 6485 case SPECIMEN: return "A sample to be used for analysis."; 6486 case STRUCTUREDEFINITION: return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 6487 case STRUCTUREMAP: return "A Map of relationships between 2 structures that can be used to transform data."; 6488 case SUBSCRIPTION: return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 6489 case SUBSTANCE: return "A homogeneous material with a definite composition."; 6490 case SUPPLYDELIVERY: return "Record of delivery of what is supplied."; 6491 case SUPPLYREQUEST: return "A record of a request for a medication, substance or device used in the healthcare setting."; 6492 case TASK: return "A task to be performed."; 6493 case TESTREPORT: return "A summary of information based on the results of executing a TestScript."; 6494 case TESTSCRIPT: return "A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 6495 case VALUESET: return "A value set specifies a set of codes drawn from one or more code systems."; 6496 case VISIONPRESCRIPTION: return "An authorization for the supply of glasses and/or contact lenses to a patient."; 6497 case NULL: return null; 6498 default: return "?"; 6499 } 6500 } 6501 public String getDisplay() { 6502 switch (this) { 6503 case ADDRESS: return "Address"; 6504 case AGE: return "Age"; 6505 case ANNOTATION: return "Annotation"; 6506 case ATTACHMENT: return "Attachment"; 6507 case BACKBONEELEMENT: return "BackboneElement"; 6508 case CODEABLECONCEPT: return "CodeableConcept"; 6509 case CODING: return "Coding"; 6510 case CONTACTDETAIL: return "ContactDetail"; 6511 case CONTACTPOINT: return "ContactPoint"; 6512 case CONTRIBUTOR: return "Contributor"; 6513 case COUNT: return "Count"; 6514 case DATAREQUIREMENT: return "DataRequirement"; 6515 case DISTANCE: return "Distance"; 6516 case DOSAGE: return "Dosage"; 6517 case DURATION: return "Duration"; 6518 case ELEMENT: return "Element"; 6519 case ELEMENTDEFINITION: return "ElementDefinition"; 6520 case EXTENSION: return "Extension"; 6521 case HUMANNAME: return "HumanName"; 6522 case IDENTIFIER: return "Identifier"; 6523 case META: return "Meta"; 6524 case MONEY: return "Money"; 6525 case NARRATIVE: return "Narrative"; 6526 case PARAMETERDEFINITION: return "ParameterDefinition"; 6527 case PERIOD: return "Period"; 6528 case QUANTITY: return "Quantity"; 6529 case RANGE: return "Range"; 6530 case RATIO: return "Ratio"; 6531 case REFERENCE: return "Reference"; 6532 case RELATEDARTIFACT: return "RelatedArtifact"; 6533 case SAMPLEDDATA: return "SampledData"; 6534 case SIGNATURE: return "Signature"; 6535 case SIMPLEQUANTITY: return "SimpleQuantity"; 6536 case TIMING: return "Timing"; 6537 case TRIGGERDEFINITION: return "TriggerDefinition"; 6538 case USAGECONTEXT: return "UsageContext"; 6539 case BASE64BINARY: return "base64Binary"; 6540 case BOOLEAN: return "boolean"; 6541 case CODE: return "code"; 6542 case DATE: return "date"; 6543 case DATETIME: return "dateTime"; 6544 case DECIMAL: return "decimal"; 6545 case ID: return "id"; 6546 case INSTANT: return "instant"; 6547 case INTEGER: return "integer"; 6548 case MARKDOWN: return "markdown"; 6549 case OID: return "oid"; 6550 case POSITIVEINT: return "positiveInt"; 6551 case STRING: return "string"; 6552 case TIME: return "time"; 6553 case UNSIGNEDINT: return "unsignedInt"; 6554 case URI: return "uri"; 6555 case UUID: return "uuid"; 6556 case XHTML: return "XHTML"; 6557 case ACCOUNT: return "Account"; 6558 case ACTIVITYDEFINITION: return "ActivityDefinition"; 6559 case ADVERSEEVENT: return "AdverseEvent"; 6560 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 6561 case APPOINTMENT: return "Appointment"; 6562 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 6563 case AUDITEVENT: return "AuditEvent"; 6564 case BASIC: return "Basic"; 6565 case BINARY: return "Binary"; 6566 case BODYSITE: return "BodySite"; 6567 case BUNDLE: return "Bundle"; 6568 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 6569 case CAREPLAN: return "CarePlan"; 6570 case CARETEAM: return "CareTeam"; 6571 case CHARGEITEM: return "ChargeItem"; 6572 case CLAIM: return "Claim"; 6573 case CLAIMRESPONSE: return "ClaimResponse"; 6574 case CLINICALIMPRESSION: return "ClinicalImpression"; 6575 case CODESYSTEM: return "CodeSystem"; 6576 case COMMUNICATION: return "Communication"; 6577 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 6578 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 6579 case COMPOSITION: return "Composition"; 6580 case CONCEPTMAP: return "ConceptMap"; 6581 case CONDITION: return "Condition"; 6582 case CONSENT: return "Consent"; 6583 case CONTRACT: return "Contract"; 6584 case COVERAGE: return "Coverage"; 6585 case DATAELEMENT: return "DataElement"; 6586 case DETECTEDISSUE: return "DetectedIssue"; 6587 case DEVICE: return "Device"; 6588 case DEVICECOMPONENT: return "DeviceComponent"; 6589 case DEVICEMETRIC: return "DeviceMetric"; 6590 case DEVICEREQUEST: return "DeviceRequest"; 6591 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 6592 case DIAGNOSTICREPORT: return "DiagnosticReport"; 6593 case DOCUMENTMANIFEST: return "DocumentManifest"; 6594 case DOCUMENTREFERENCE: return "DocumentReference"; 6595 case DOMAINRESOURCE: return "DomainResource"; 6596 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 6597 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 6598 case ENCOUNTER: return "Encounter"; 6599 case ENDPOINT: return "Endpoint"; 6600 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 6601 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 6602 case EPISODEOFCARE: return "EpisodeOfCare"; 6603 case EXPANSIONPROFILE: return "ExpansionProfile"; 6604 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 6605 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 6606 case FLAG: return "Flag"; 6607 case GOAL: return "Goal"; 6608 case GRAPHDEFINITION: return "GraphDefinition"; 6609 case GROUP: return "Group"; 6610 case GUIDANCERESPONSE: return "GuidanceResponse"; 6611 case HEALTHCARESERVICE: return "HealthcareService"; 6612 case IMAGINGMANIFEST: return "ImagingManifest"; 6613 case IMAGINGSTUDY: return "ImagingStudy"; 6614 case IMMUNIZATION: return "Immunization"; 6615 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 6616 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 6617 case LIBRARY: return "Library"; 6618 case LINKAGE: return "Linkage"; 6619 case LIST: return "List"; 6620 case LOCATION: return "Location"; 6621 case MEASURE: return "Measure"; 6622 case MEASUREREPORT: return "MeasureReport"; 6623 case MEDIA: return "Media"; 6624 case MEDICATION: return "Medication"; 6625 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 6626 case MEDICATIONDISPENSE: return "MedicationDispense"; 6627 case MEDICATIONREQUEST: return "MedicationRequest"; 6628 case MEDICATIONSTATEMENT: return "MedicationStatement"; 6629 case MESSAGEDEFINITION: return "MessageDefinition"; 6630 case MESSAGEHEADER: return "MessageHeader"; 6631 case NAMINGSYSTEM: return "NamingSystem"; 6632 case NUTRITIONORDER: return "NutritionOrder"; 6633 case OBSERVATION: return "Observation"; 6634 case OPERATIONDEFINITION: return "OperationDefinition"; 6635 case OPERATIONOUTCOME: return "OperationOutcome"; 6636 case ORGANIZATION: return "Organization"; 6637 case PARAMETERS: return "Parameters"; 6638 case PATIENT: return "Patient"; 6639 case PAYMENTNOTICE: return "PaymentNotice"; 6640 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 6641 case PERSON: return "Person"; 6642 case PLANDEFINITION: return "PlanDefinition"; 6643 case PRACTITIONER: return "Practitioner"; 6644 case PRACTITIONERROLE: return "PractitionerRole"; 6645 case PROCEDURE: return "Procedure"; 6646 case PROCEDUREREQUEST: return "ProcedureRequest"; 6647 case PROCESSREQUEST: return "ProcessRequest"; 6648 case PROCESSRESPONSE: return "ProcessResponse"; 6649 case PROVENANCE: return "Provenance"; 6650 case QUESTIONNAIRE: return "Questionnaire"; 6651 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 6652 case REFERRALREQUEST: return "ReferralRequest"; 6653 case RELATEDPERSON: return "RelatedPerson"; 6654 case REQUESTGROUP: return "RequestGroup"; 6655 case RESEARCHSTUDY: return "ResearchStudy"; 6656 case RESEARCHSUBJECT: return "ResearchSubject"; 6657 case RESOURCE: return "Resource"; 6658 case RISKASSESSMENT: return "RiskAssessment"; 6659 case SCHEDULE: return "Schedule"; 6660 case SEARCHPARAMETER: return "SearchParameter"; 6661 case SEQUENCE: return "Sequence"; 6662 case SERVICEDEFINITION: return "ServiceDefinition"; 6663 case SLOT: return "Slot"; 6664 case SPECIMEN: return "Specimen"; 6665 case STRUCTUREDEFINITION: return "StructureDefinition"; 6666 case STRUCTUREMAP: return "StructureMap"; 6667 case SUBSCRIPTION: return "Subscription"; 6668 case SUBSTANCE: return "Substance"; 6669 case SUPPLYDELIVERY: return "SupplyDelivery"; 6670 case SUPPLYREQUEST: return "SupplyRequest"; 6671 case TASK: return "Task"; 6672 case TESTREPORT: return "TestReport"; 6673 case TESTSCRIPT: return "TestScript"; 6674 case VALUESET: return "ValueSet"; 6675 case VISIONPRESCRIPTION: return "VisionPrescription"; 6676 case NULL: return null; 6677 default: return "?"; 6678 } 6679 } 6680 } 6681 6682 public static class FHIRDefinedTypeEnumFactory implements EnumFactory<FHIRDefinedType> { 6683 public FHIRDefinedType fromCode(String codeString) throws IllegalArgumentException { 6684 if (codeString == null || "".equals(codeString)) 6685 if (codeString == null || "".equals(codeString)) 6686 return null; 6687 if ("Address".equals(codeString)) 6688 return FHIRDefinedType.ADDRESS; 6689 if ("Age".equals(codeString)) 6690 return FHIRDefinedType.AGE; 6691 if ("Annotation".equals(codeString)) 6692 return FHIRDefinedType.ANNOTATION; 6693 if ("Attachment".equals(codeString)) 6694 return FHIRDefinedType.ATTACHMENT; 6695 if ("BackboneElement".equals(codeString)) 6696 return FHIRDefinedType.BACKBONEELEMENT; 6697 if ("CodeableConcept".equals(codeString)) 6698 return FHIRDefinedType.CODEABLECONCEPT; 6699 if ("Coding".equals(codeString)) 6700 return FHIRDefinedType.CODING; 6701 if ("ContactDetail".equals(codeString)) 6702 return FHIRDefinedType.CONTACTDETAIL; 6703 if ("ContactPoint".equals(codeString)) 6704 return FHIRDefinedType.CONTACTPOINT; 6705 if ("Contributor".equals(codeString)) 6706 return FHIRDefinedType.CONTRIBUTOR; 6707 if ("Count".equals(codeString)) 6708 return FHIRDefinedType.COUNT; 6709 if ("DataRequirement".equals(codeString)) 6710 return FHIRDefinedType.DATAREQUIREMENT; 6711 if ("Distance".equals(codeString)) 6712 return FHIRDefinedType.DISTANCE; 6713 if ("Dosage".equals(codeString)) 6714 return FHIRDefinedType.DOSAGE; 6715 if ("Duration".equals(codeString)) 6716 return FHIRDefinedType.DURATION; 6717 if ("Element".equals(codeString)) 6718 return FHIRDefinedType.ELEMENT; 6719 if ("ElementDefinition".equals(codeString)) 6720 return FHIRDefinedType.ELEMENTDEFINITION; 6721 if ("Extension".equals(codeString)) 6722 return FHIRDefinedType.EXTENSION; 6723 if ("HumanName".equals(codeString)) 6724 return FHIRDefinedType.HUMANNAME; 6725 if ("Identifier".equals(codeString)) 6726 return FHIRDefinedType.IDENTIFIER; 6727 if ("Meta".equals(codeString)) 6728 return FHIRDefinedType.META; 6729 if ("Money".equals(codeString)) 6730 return FHIRDefinedType.MONEY; 6731 if ("Narrative".equals(codeString)) 6732 return FHIRDefinedType.NARRATIVE; 6733 if ("ParameterDefinition".equals(codeString)) 6734 return FHIRDefinedType.PARAMETERDEFINITION; 6735 if ("Period".equals(codeString)) 6736 return FHIRDefinedType.PERIOD; 6737 if ("Quantity".equals(codeString)) 6738 return FHIRDefinedType.QUANTITY; 6739 if ("Range".equals(codeString)) 6740 return FHIRDefinedType.RANGE; 6741 if ("Ratio".equals(codeString)) 6742 return FHIRDefinedType.RATIO; 6743 if ("Reference".equals(codeString)) 6744 return FHIRDefinedType.REFERENCE; 6745 if ("RelatedArtifact".equals(codeString)) 6746 return FHIRDefinedType.RELATEDARTIFACT; 6747 if ("SampledData".equals(codeString)) 6748 return FHIRDefinedType.SAMPLEDDATA; 6749 if ("Signature".equals(codeString)) 6750 return FHIRDefinedType.SIGNATURE; 6751 if ("SimpleQuantity".equals(codeString)) 6752 return FHIRDefinedType.SIMPLEQUANTITY; 6753 if ("Timing".equals(codeString)) 6754 return FHIRDefinedType.TIMING; 6755 if ("TriggerDefinition".equals(codeString)) 6756 return FHIRDefinedType.TRIGGERDEFINITION; 6757 if ("UsageContext".equals(codeString)) 6758 return FHIRDefinedType.USAGECONTEXT; 6759 if ("base64Binary".equals(codeString)) 6760 return FHIRDefinedType.BASE64BINARY; 6761 if ("boolean".equals(codeString)) 6762 return FHIRDefinedType.BOOLEAN; 6763 if ("code".equals(codeString)) 6764 return FHIRDefinedType.CODE; 6765 if ("date".equals(codeString)) 6766 return FHIRDefinedType.DATE; 6767 if ("dateTime".equals(codeString)) 6768 return FHIRDefinedType.DATETIME; 6769 if ("decimal".equals(codeString)) 6770 return FHIRDefinedType.DECIMAL; 6771 if ("id".equals(codeString)) 6772 return FHIRDefinedType.ID; 6773 if ("instant".equals(codeString)) 6774 return FHIRDefinedType.INSTANT; 6775 if ("integer".equals(codeString)) 6776 return FHIRDefinedType.INTEGER; 6777 if ("markdown".equals(codeString)) 6778 return FHIRDefinedType.MARKDOWN; 6779 if ("oid".equals(codeString)) 6780 return FHIRDefinedType.OID; 6781 if ("positiveInt".equals(codeString)) 6782 return FHIRDefinedType.POSITIVEINT; 6783 if ("string".equals(codeString)) 6784 return FHIRDefinedType.STRING; 6785 if ("time".equals(codeString)) 6786 return FHIRDefinedType.TIME; 6787 if ("unsignedInt".equals(codeString)) 6788 return FHIRDefinedType.UNSIGNEDINT; 6789 if ("uri".equals(codeString)) 6790 return FHIRDefinedType.URI; 6791 if ("uuid".equals(codeString)) 6792 return FHIRDefinedType.UUID; 6793 if ("xhtml".equals(codeString)) 6794 return FHIRDefinedType.XHTML; 6795 if ("Account".equals(codeString)) 6796 return FHIRDefinedType.ACCOUNT; 6797 if ("ActivityDefinition".equals(codeString)) 6798 return FHIRDefinedType.ACTIVITYDEFINITION; 6799 if ("AdverseEvent".equals(codeString)) 6800 return FHIRDefinedType.ADVERSEEVENT; 6801 if ("AllergyIntolerance".equals(codeString)) 6802 return FHIRDefinedType.ALLERGYINTOLERANCE; 6803 if ("Appointment".equals(codeString)) 6804 return FHIRDefinedType.APPOINTMENT; 6805 if ("AppointmentResponse".equals(codeString)) 6806 return FHIRDefinedType.APPOINTMENTRESPONSE; 6807 if ("AuditEvent".equals(codeString)) 6808 return FHIRDefinedType.AUDITEVENT; 6809 if ("Basic".equals(codeString)) 6810 return FHIRDefinedType.BASIC; 6811 if ("Binary".equals(codeString)) 6812 return FHIRDefinedType.BINARY; 6813 if ("BodySite".equals(codeString)) 6814 return FHIRDefinedType.BODYSITE; 6815 if ("Bundle".equals(codeString)) 6816 return FHIRDefinedType.BUNDLE; 6817 if ("CapabilityStatement".equals(codeString)) 6818 return FHIRDefinedType.CAPABILITYSTATEMENT; 6819 if ("CarePlan".equals(codeString)) 6820 return FHIRDefinedType.CAREPLAN; 6821 if ("CareTeam".equals(codeString)) 6822 return FHIRDefinedType.CARETEAM; 6823 if ("ChargeItem".equals(codeString)) 6824 return FHIRDefinedType.CHARGEITEM; 6825 if ("Claim".equals(codeString)) 6826 return FHIRDefinedType.CLAIM; 6827 if ("ClaimResponse".equals(codeString)) 6828 return FHIRDefinedType.CLAIMRESPONSE; 6829 if ("ClinicalImpression".equals(codeString)) 6830 return FHIRDefinedType.CLINICALIMPRESSION; 6831 if ("CodeSystem".equals(codeString)) 6832 return FHIRDefinedType.CODESYSTEM; 6833 if ("Communication".equals(codeString)) 6834 return FHIRDefinedType.COMMUNICATION; 6835 if ("CommunicationRequest".equals(codeString)) 6836 return FHIRDefinedType.COMMUNICATIONREQUEST; 6837 if ("CompartmentDefinition".equals(codeString)) 6838 return FHIRDefinedType.COMPARTMENTDEFINITION; 6839 if ("Composition".equals(codeString)) 6840 return FHIRDefinedType.COMPOSITION; 6841 if ("ConceptMap".equals(codeString)) 6842 return FHIRDefinedType.CONCEPTMAP; 6843 if ("Condition".equals(codeString)) 6844 return FHIRDefinedType.CONDITION; 6845 if ("Consent".equals(codeString)) 6846 return FHIRDefinedType.CONSENT; 6847 if ("Contract".equals(codeString)) 6848 return FHIRDefinedType.CONTRACT; 6849 if ("Coverage".equals(codeString)) 6850 return FHIRDefinedType.COVERAGE; 6851 if ("DataElement".equals(codeString)) 6852 return FHIRDefinedType.DATAELEMENT; 6853 if ("DetectedIssue".equals(codeString)) 6854 return FHIRDefinedType.DETECTEDISSUE; 6855 if ("Device".equals(codeString)) 6856 return FHIRDefinedType.DEVICE; 6857 if ("DeviceComponent".equals(codeString)) 6858 return FHIRDefinedType.DEVICECOMPONENT; 6859 if ("DeviceMetric".equals(codeString)) 6860 return FHIRDefinedType.DEVICEMETRIC; 6861 if ("DeviceRequest".equals(codeString)) 6862 return FHIRDefinedType.DEVICEREQUEST; 6863 if ("DeviceUseStatement".equals(codeString)) 6864 return FHIRDefinedType.DEVICEUSESTATEMENT; 6865 if ("DiagnosticReport".equals(codeString)) 6866 return FHIRDefinedType.DIAGNOSTICREPORT; 6867 if ("DocumentManifest".equals(codeString)) 6868 return FHIRDefinedType.DOCUMENTMANIFEST; 6869 if ("DocumentReference".equals(codeString)) 6870 return FHIRDefinedType.DOCUMENTREFERENCE; 6871 if ("DomainResource".equals(codeString)) 6872 return FHIRDefinedType.DOMAINRESOURCE; 6873 if ("EligibilityRequest".equals(codeString)) 6874 return FHIRDefinedType.ELIGIBILITYREQUEST; 6875 if ("EligibilityResponse".equals(codeString)) 6876 return FHIRDefinedType.ELIGIBILITYRESPONSE; 6877 if ("Encounter".equals(codeString)) 6878 return FHIRDefinedType.ENCOUNTER; 6879 if ("Endpoint".equals(codeString)) 6880 return FHIRDefinedType.ENDPOINT; 6881 if ("EnrollmentRequest".equals(codeString)) 6882 return FHIRDefinedType.ENROLLMENTREQUEST; 6883 if ("EnrollmentResponse".equals(codeString)) 6884 return FHIRDefinedType.ENROLLMENTRESPONSE; 6885 if ("EpisodeOfCare".equals(codeString)) 6886 return FHIRDefinedType.EPISODEOFCARE; 6887 if ("ExpansionProfile".equals(codeString)) 6888 return FHIRDefinedType.EXPANSIONPROFILE; 6889 if ("ExplanationOfBenefit".equals(codeString)) 6890 return FHIRDefinedType.EXPLANATIONOFBENEFIT; 6891 if ("FamilyMemberHistory".equals(codeString)) 6892 return FHIRDefinedType.FAMILYMEMBERHISTORY; 6893 if ("Flag".equals(codeString)) 6894 return FHIRDefinedType.FLAG; 6895 if ("Goal".equals(codeString)) 6896 return FHIRDefinedType.GOAL; 6897 if ("GraphDefinition".equals(codeString)) 6898 return FHIRDefinedType.GRAPHDEFINITION; 6899 if ("Group".equals(codeString)) 6900 return FHIRDefinedType.GROUP; 6901 if ("GuidanceResponse".equals(codeString)) 6902 return FHIRDefinedType.GUIDANCERESPONSE; 6903 if ("HealthcareService".equals(codeString)) 6904 return FHIRDefinedType.HEALTHCARESERVICE; 6905 if ("ImagingManifest".equals(codeString)) 6906 return FHIRDefinedType.IMAGINGMANIFEST; 6907 if ("ImagingStudy".equals(codeString)) 6908 return FHIRDefinedType.IMAGINGSTUDY; 6909 if ("Immunization".equals(codeString)) 6910 return FHIRDefinedType.IMMUNIZATION; 6911 if ("ImmunizationRecommendation".equals(codeString)) 6912 return FHIRDefinedType.IMMUNIZATIONRECOMMENDATION; 6913 if ("ImplementationGuide".equals(codeString)) 6914 return FHIRDefinedType.IMPLEMENTATIONGUIDE; 6915 if ("Library".equals(codeString)) 6916 return FHIRDefinedType.LIBRARY; 6917 if ("Linkage".equals(codeString)) 6918 return FHIRDefinedType.LINKAGE; 6919 if ("List".equals(codeString)) 6920 return FHIRDefinedType.LIST; 6921 if ("Location".equals(codeString)) 6922 return FHIRDefinedType.LOCATION; 6923 if ("Measure".equals(codeString)) 6924 return FHIRDefinedType.MEASURE; 6925 if ("MeasureReport".equals(codeString)) 6926 return FHIRDefinedType.MEASUREREPORT; 6927 if ("Media".equals(codeString)) 6928 return FHIRDefinedType.MEDIA; 6929 if ("Medication".equals(codeString)) 6930 return FHIRDefinedType.MEDICATION; 6931 if ("MedicationAdministration".equals(codeString)) 6932 return FHIRDefinedType.MEDICATIONADMINISTRATION; 6933 if ("MedicationDispense".equals(codeString)) 6934 return FHIRDefinedType.MEDICATIONDISPENSE; 6935 if ("MedicationRequest".equals(codeString)) 6936 return FHIRDefinedType.MEDICATIONREQUEST; 6937 if ("MedicationStatement".equals(codeString)) 6938 return FHIRDefinedType.MEDICATIONSTATEMENT; 6939 if ("MessageDefinition".equals(codeString)) 6940 return FHIRDefinedType.MESSAGEDEFINITION; 6941 if ("MessageHeader".equals(codeString)) 6942 return FHIRDefinedType.MESSAGEHEADER; 6943 if ("NamingSystem".equals(codeString)) 6944 return FHIRDefinedType.NAMINGSYSTEM; 6945 if ("NutritionOrder".equals(codeString)) 6946 return FHIRDefinedType.NUTRITIONORDER; 6947 if ("Observation".equals(codeString)) 6948 return FHIRDefinedType.OBSERVATION; 6949 if ("OperationDefinition".equals(codeString)) 6950 return FHIRDefinedType.OPERATIONDEFINITION; 6951 if ("OperationOutcome".equals(codeString)) 6952 return FHIRDefinedType.OPERATIONOUTCOME; 6953 if ("Organization".equals(codeString)) 6954 return FHIRDefinedType.ORGANIZATION; 6955 if ("Parameters".equals(codeString)) 6956 return FHIRDefinedType.PARAMETERS; 6957 if ("Patient".equals(codeString)) 6958 return FHIRDefinedType.PATIENT; 6959 if ("PaymentNotice".equals(codeString)) 6960 return FHIRDefinedType.PAYMENTNOTICE; 6961 if ("PaymentReconciliation".equals(codeString)) 6962 return FHIRDefinedType.PAYMENTRECONCILIATION; 6963 if ("Person".equals(codeString)) 6964 return FHIRDefinedType.PERSON; 6965 if ("PlanDefinition".equals(codeString)) 6966 return FHIRDefinedType.PLANDEFINITION; 6967 if ("Practitioner".equals(codeString)) 6968 return FHIRDefinedType.PRACTITIONER; 6969 if ("PractitionerRole".equals(codeString)) 6970 return FHIRDefinedType.PRACTITIONERROLE; 6971 if ("Procedure".equals(codeString)) 6972 return FHIRDefinedType.PROCEDURE; 6973 if ("ProcedureRequest".equals(codeString)) 6974 return FHIRDefinedType.PROCEDUREREQUEST; 6975 if ("ProcessRequest".equals(codeString)) 6976 return FHIRDefinedType.PROCESSREQUEST; 6977 if ("ProcessResponse".equals(codeString)) 6978 return FHIRDefinedType.PROCESSRESPONSE; 6979 if ("Provenance".equals(codeString)) 6980 return FHIRDefinedType.PROVENANCE; 6981 if ("Questionnaire".equals(codeString)) 6982 return FHIRDefinedType.QUESTIONNAIRE; 6983 if ("QuestionnaireResponse".equals(codeString)) 6984 return FHIRDefinedType.QUESTIONNAIRERESPONSE; 6985 if ("ReferralRequest".equals(codeString)) 6986 return FHIRDefinedType.REFERRALREQUEST; 6987 if ("RelatedPerson".equals(codeString)) 6988 return FHIRDefinedType.RELATEDPERSON; 6989 if ("RequestGroup".equals(codeString)) 6990 return FHIRDefinedType.REQUESTGROUP; 6991 if ("ResearchStudy".equals(codeString)) 6992 return FHIRDefinedType.RESEARCHSTUDY; 6993 if ("ResearchSubject".equals(codeString)) 6994 return FHIRDefinedType.RESEARCHSUBJECT; 6995 if ("Resource".equals(codeString)) 6996 return FHIRDefinedType.RESOURCE; 6997 if ("RiskAssessment".equals(codeString)) 6998 return FHIRDefinedType.RISKASSESSMENT; 6999 if ("Schedule".equals(codeString)) 7000 return FHIRDefinedType.SCHEDULE; 7001 if ("SearchParameter".equals(codeString)) 7002 return FHIRDefinedType.SEARCHPARAMETER; 7003 if ("Sequence".equals(codeString)) 7004 return FHIRDefinedType.SEQUENCE; 7005 if ("ServiceDefinition".equals(codeString)) 7006 return FHIRDefinedType.SERVICEDEFINITION; 7007 if ("Slot".equals(codeString)) 7008 return FHIRDefinedType.SLOT; 7009 if ("Specimen".equals(codeString)) 7010 return FHIRDefinedType.SPECIMEN; 7011 if ("StructureDefinition".equals(codeString)) 7012 return FHIRDefinedType.STRUCTUREDEFINITION; 7013 if ("StructureMap".equals(codeString)) 7014 return FHIRDefinedType.STRUCTUREMAP; 7015 if ("Subscription".equals(codeString)) 7016 return FHIRDefinedType.SUBSCRIPTION; 7017 if ("Substance".equals(codeString)) 7018 return FHIRDefinedType.SUBSTANCE; 7019 if ("SupplyDelivery".equals(codeString)) 7020 return FHIRDefinedType.SUPPLYDELIVERY; 7021 if ("SupplyRequest".equals(codeString)) 7022 return FHIRDefinedType.SUPPLYREQUEST; 7023 if ("Task".equals(codeString)) 7024 return FHIRDefinedType.TASK; 7025 if ("TestReport".equals(codeString)) 7026 return FHIRDefinedType.TESTREPORT; 7027 if ("TestScript".equals(codeString)) 7028 return FHIRDefinedType.TESTSCRIPT; 7029 if ("ValueSet".equals(codeString)) 7030 return FHIRDefinedType.VALUESET; 7031 if ("VisionPrescription".equals(codeString)) 7032 return FHIRDefinedType.VISIONPRESCRIPTION; 7033 throw new IllegalArgumentException("Unknown FHIRDefinedType code '"+codeString+"'"); 7034 } 7035 public Enumeration<FHIRDefinedType> fromType(PrimitiveType<?> code) throws FHIRException { 7036 if (code == null) 7037 return null; 7038 if (code.isEmpty()) 7039 return new Enumeration<FHIRDefinedType>(this); 7040 String codeString = code.asStringValue(); 7041 if (codeString == null || "".equals(codeString)) 7042 return null; 7043 if ("Address".equals(codeString)) 7044 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADDRESS); 7045 if ("Age".equals(codeString)) 7046 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AGE); 7047 if ("Annotation".equals(codeString)) 7048 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ANNOTATION); 7049 if ("Attachment".equals(codeString)) 7050 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ATTACHMENT); 7051 if ("BackboneElement".equals(codeString)) 7052 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BACKBONEELEMENT); 7053 if ("CodeableConcept".equals(codeString)) 7054 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODEABLECONCEPT); 7055 if ("Coding".equals(codeString)) 7056 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODING); 7057 if ("ContactDetail".equals(codeString)) 7058 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTDETAIL); 7059 if ("ContactPoint".equals(codeString)) 7060 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTPOINT); 7061 if ("Contributor".equals(codeString)) 7062 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRIBUTOR); 7063 if ("Count".equals(codeString)) 7064 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COUNT); 7065 if ("DataRequirement".equals(codeString)) 7066 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATAREQUIREMENT); 7067 if ("Distance".equals(codeString)) 7068 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DISTANCE); 7069 if ("Dosage".equals(codeString)) 7070 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOSAGE); 7071 if ("Duration".equals(codeString)) 7072 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DURATION); 7073 if ("Element".equals(codeString)) 7074 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENT); 7075 if ("ElementDefinition".equals(codeString)) 7076 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENTDEFINITION); 7077 if ("Extension".equals(codeString)) 7078 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXTENSION); 7079 if ("HumanName".equals(codeString)) 7080 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HUMANNAME); 7081 if ("Identifier".equals(codeString)) 7082 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IDENTIFIER); 7083 if ("Meta".equals(codeString)) 7084 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.META); 7085 if ("Money".equals(codeString)) 7086 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MONEY); 7087 if ("Narrative".equals(codeString)) 7088 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NARRATIVE); 7089 if ("ParameterDefinition".equals(codeString)) 7090 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERDEFINITION); 7091 if ("Period".equals(codeString)) 7092 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERIOD); 7093 if ("Quantity".equals(codeString)) 7094 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUANTITY); 7095 if ("Range".equals(codeString)) 7096 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RANGE); 7097 if ("Ratio".equals(codeString)) 7098 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RATIO); 7099 if ("Reference".equals(codeString)) 7100 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REFERENCE); 7101 if ("RelatedArtifact".equals(codeString)) 7102 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDARTIFACT); 7103 if ("SampledData".equals(codeString)) 7104 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SAMPLEDDATA); 7105 if ("Signature".equals(codeString)) 7106 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIGNATURE); 7107 if ("SimpleQuantity".equals(codeString)) 7108 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIMPLEQUANTITY); 7109 if ("Timing".equals(codeString)) 7110 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIMING); 7111 if ("TriggerDefinition".equals(codeString)) 7112 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TRIGGERDEFINITION); 7113 if ("UsageContext".equals(codeString)) 7114 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.USAGECONTEXT); 7115 if ("base64Binary".equals(codeString)) 7116 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASE64BINARY); 7117 if ("boolean".equals(codeString)) 7118 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BOOLEAN); 7119 if ("code".equals(codeString)) 7120 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODE); 7121 if ("date".equals(codeString)) 7122 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATE); 7123 if ("dateTime".equals(codeString)) 7124 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATETIME); 7125 if ("decimal".equals(codeString)) 7126 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DECIMAL); 7127 if ("id".equals(codeString)) 7128 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ID); 7129 if ("instant".equals(codeString)) 7130 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INSTANT); 7131 if ("integer".equals(codeString)) 7132 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INTEGER); 7133 if ("markdown".equals(codeString)) 7134 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MARKDOWN); 7135 if ("oid".equals(codeString)) 7136 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OID); 7137 if ("positiveInt".equals(codeString)) 7138 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.POSITIVEINT); 7139 if ("string".equals(codeString)) 7140 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRING); 7141 if ("time".equals(codeString)) 7142 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIME); 7143 if ("unsignedInt".equals(codeString)) 7144 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UNSIGNEDINT); 7145 if ("uri".equals(codeString)) 7146 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.URI); 7147 if ("uuid".equals(codeString)) 7148 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UUID); 7149 if ("xhtml".equals(codeString)) 7150 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.XHTML); 7151 if ("Account".equals(codeString)) 7152 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACCOUNT); 7153 if ("ActivityDefinition".equals(codeString)) 7154 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACTIVITYDEFINITION); 7155 if ("AdverseEvent".equals(codeString)) 7156 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADVERSEEVENT); 7157 if ("AllergyIntolerance".equals(codeString)) 7158 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ALLERGYINTOLERANCE); 7159 if ("Appointment".equals(codeString)) 7160 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENT); 7161 if ("AppointmentResponse".equals(codeString)) 7162 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENTRESPONSE); 7163 if ("AuditEvent".equals(codeString)) 7164 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AUDITEVENT); 7165 if ("Basic".equals(codeString)) 7166 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASIC); 7167 if ("Binary".equals(codeString)) 7168 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BINARY); 7169 if ("BodySite".equals(codeString)) 7170 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BODYSITE); 7171 if ("Bundle".equals(codeString)) 7172 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BUNDLE); 7173 if ("CapabilityStatement".equals(codeString)) 7174 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAPABILITYSTATEMENT); 7175 if ("CarePlan".equals(codeString)) 7176 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAREPLAN); 7177 if ("CareTeam".equals(codeString)) 7178 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CARETEAM); 7179 if ("ChargeItem".equals(codeString)) 7180 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CHARGEITEM); 7181 if ("Claim".equals(codeString)) 7182 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIM); 7183 if ("ClaimResponse".equals(codeString)) 7184 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIMRESPONSE); 7185 if ("ClinicalImpression".equals(codeString)) 7186 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLINICALIMPRESSION); 7187 if ("CodeSystem".equals(codeString)) 7188 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODESYSTEM); 7189 if ("Communication".equals(codeString)) 7190 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATION); 7191 if ("CommunicationRequest".equals(codeString)) 7192 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATIONREQUEST); 7193 if ("CompartmentDefinition".equals(codeString)) 7194 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPARTMENTDEFINITION); 7195 if ("Composition".equals(codeString)) 7196 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPOSITION); 7197 if ("ConceptMap".equals(codeString)) 7198 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONCEPTMAP); 7199 if ("Condition".equals(codeString)) 7200 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONDITION); 7201 if ("Consent".equals(codeString)) 7202 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONSENT); 7203 if ("Contract".equals(codeString)) 7204 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRACT); 7205 if ("Coverage".equals(codeString)) 7206 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGE); 7207 if ("DataElement".equals(codeString)) 7208 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATAELEMENT); 7209 if ("DetectedIssue".equals(codeString)) 7210 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DETECTEDISSUE); 7211 if ("Device".equals(codeString)) 7212 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICE); 7213 if ("DeviceComponent".equals(codeString)) 7214 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICECOMPONENT); 7215 if ("DeviceMetric".equals(codeString)) 7216 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEMETRIC); 7217 if ("DeviceRequest".equals(codeString)) 7218 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEREQUEST); 7219 if ("DeviceUseStatement".equals(codeString)) 7220 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEUSESTATEMENT); 7221 if ("DiagnosticReport".equals(codeString)) 7222 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DIAGNOSTICREPORT); 7223 if ("DocumentManifest".equals(codeString)) 7224 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTMANIFEST); 7225 if ("DocumentReference".equals(codeString)) 7226 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTREFERENCE); 7227 if ("DomainResource".equals(codeString)) 7228 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOMAINRESOURCE); 7229 if ("EligibilityRequest".equals(codeString)) 7230 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELIGIBILITYREQUEST); 7231 if ("EligibilityResponse".equals(codeString)) 7232 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELIGIBILITYRESPONSE); 7233 if ("Encounter".equals(codeString)) 7234 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENCOUNTER); 7235 if ("Endpoint".equals(codeString)) 7236 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENDPOINT); 7237 if ("EnrollmentRequest".equals(codeString)) 7238 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTREQUEST); 7239 if ("EnrollmentResponse".equals(codeString)) 7240 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTRESPONSE); 7241 if ("EpisodeOfCare".equals(codeString)) 7242 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EPISODEOFCARE); 7243 if ("ExpansionProfile".equals(codeString)) 7244 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPANSIONPROFILE); 7245 if ("ExplanationOfBenefit".equals(codeString)) 7246 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPLANATIONOFBENEFIT); 7247 if ("FamilyMemberHistory".equals(codeString)) 7248 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FAMILYMEMBERHISTORY); 7249 if ("Flag".equals(codeString)) 7250 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FLAG); 7251 if ("Goal".equals(codeString)) 7252 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GOAL); 7253 if ("GraphDefinition".equals(codeString)) 7254 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GRAPHDEFINITION); 7255 if ("Group".equals(codeString)) 7256 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GROUP); 7257 if ("GuidanceResponse".equals(codeString)) 7258 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GUIDANCERESPONSE); 7259 if ("HealthcareService".equals(codeString)) 7260 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HEALTHCARESERVICE); 7261 if ("ImagingManifest".equals(codeString)) 7262 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMAGINGMANIFEST); 7263 if ("ImagingStudy".equals(codeString)) 7264 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMAGINGSTUDY); 7265 if ("Immunization".equals(codeString)) 7266 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATION); 7267 if ("ImmunizationRecommendation".equals(codeString)) 7268 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATIONRECOMMENDATION); 7269 if ("ImplementationGuide".equals(codeString)) 7270 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMPLEMENTATIONGUIDE); 7271 if ("Library".equals(codeString)) 7272 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIBRARY); 7273 if ("Linkage".equals(codeString)) 7274 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LINKAGE); 7275 if ("List".equals(codeString)) 7276 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIST); 7277 if ("Location".equals(codeString)) 7278 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LOCATION); 7279 if ("Measure".equals(codeString)) 7280 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEASURE); 7281 if ("MeasureReport".equals(codeString)) 7282 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEASUREREPORT); 7283 if ("Media".equals(codeString)) 7284 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDIA); 7285 if ("Medication".equals(codeString)) 7286 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATION); 7287 if ("MedicationAdministration".equals(codeString)) 7288 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONADMINISTRATION); 7289 if ("MedicationDispense".equals(codeString)) 7290 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONDISPENSE); 7291 if ("MedicationRequest".equals(codeString)) 7292 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONREQUEST); 7293 if ("MedicationStatement".equals(codeString)) 7294 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONSTATEMENT); 7295 if ("MessageDefinition".equals(codeString)) 7296 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEDEFINITION); 7297 if ("MessageHeader".equals(codeString)) 7298 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEHEADER); 7299 if ("NamingSystem".equals(codeString)) 7300 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NAMINGSYSTEM); 7301 if ("NutritionOrder".equals(codeString)) 7302 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NUTRITIONORDER); 7303 if ("Observation".equals(codeString)) 7304 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OBSERVATION); 7305 if ("OperationDefinition".equals(codeString)) 7306 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONDEFINITION); 7307 if ("OperationOutcome".equals(codeString)) 7308 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONOUTCOME); 7309 if ("Organization".equals(codeString)) 7310 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORGANIZATION); 7311 if ("Parameters".equals(codeString)) 7312 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERS); 7313 if ("Patient".equals(codeString)) 7314 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PATIENT); 7315 if ("PaymentNotice".equals(codeString)) 7316 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTNOTICE); 7317 if ("PaymentReconciliation".equals(codeString)) 7318 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTRECONCILIATION); 7319 if ("Person".equals(codeString)) 7320 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERSON); 7321 if ("PlanDefinition".equals(codeString)) 7322 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PLANDEFINITION); 7323 if ("Practitioner".equals(codeString)) 7324 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONER); 7325 if ("PractitionerRole".equals(codeString)) 7326 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONERROLE); 7327 if ("Procedure".equals(codeString)) 7328 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCEDURE); 7329 if ("ProcedureRequest".equals(codeString)) 7330 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCEDUREREQUEST); 7331 if ("ProcessRequest".equals(codeString)) 7332 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCESSREQUEST); 7333 if ("ProcessResponse".equals(codeString)) 7334 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCESSRESPONSE); 7335 if ("Provenance".equals(codeString)) 7336 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROVENANCE); 7337 if ("Questionnaire".equals(codeString)) 7338 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRE); 7339 if ("QuestionnaireResponse".equals(codeString)) 7340 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRERESPONSE); 7341 if ("ReferralRequest".equals(codeString)) 7342 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REFERRALREQUEST); 7343 if ("RelatedPerson".equals(codeString)) 7344 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDPERSON); 7345 if ("RequestGroup".equals(codeString)) 7346 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REQUESTGROUP); 7347 if ("ResearchStudy".equals(codeString)) 7348 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHSTUDY); 7349 if ("ResearchSubject".equals(codeString)) 7350 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHSUBJECT); 7351 if ("Resource".equals(codeString)) 7352 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESOURCE); 7353 if ("RiskAssessment".equals(codeString)) 7354 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RISKASSESSMENT); 7355 if ("Schedule".equals(codeString)) 7356 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SCHEDULE); 7357 if ("SearchParameter".equals(codeString)) 7358 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SEARCHPARAMETER); 7359 if ("Sequence".equals(codeString)) 7360 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SEQUENCE); 7361 if ("ServiceDefinition".equals(codeString)) 7362 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SERVICEDEFINITION); 7363 if ("Slot".equals(codeString)) 7364 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SLOT); 7365 if ("Specimen".equals(codeString)) 7366 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SPECIMEN); 7367 if ("StructureDefinition".equals(codeString)) 7368 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREDEFINITION); 7369 if ("StructureMap".equals(codeString)) 7370 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREMAP); 7371 if ("Subscription".equals(codeString)) 7372 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSCRIPTION); 7373 if ("Substance".equals(codeString)) 7374 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCE); 7375 if ("SupplyDelivery".equals(codeString)) 7376 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYDELIVERY); 7377 if ("SupplyRequest".equals(codeString)) 7378 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYREQUEST); 7379 if ("Task".equals(codeString)) 7380 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TASK); 7381 if ("TestReport".equals(codeString)) 7382 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTREPORT); 7383 if ("TestScript".equals(codeString)) 7384 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTSCRIPT); 7385 if ("ValueSet".equals(codeString)) 7386 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VALUESET); 7387 if ("VisionPrescription".equals(codeString)) 7388 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VISIONPRESCRIPTION); 7389 throw new FHIRException("Unknown FHIRDefinedType code '"+codeString+"'"); 7390 } 7391 public String toCode(FHIRDefinedType code) { 7392 if (code == FHIRDefinedType.NULL) 7393 return null; 7394 if (code == FHIRDefinedType.ADDRESS) 7395 return "Address"; 7396 if (code == FHIRDefinedType.AGE) 7397 return "Age"; 7398 if (code == FHIRDefinedType.ANNOTATION) 7399 return "Annotation"; 7400 if (code == FHIRDefinedType.ATTACHMENT) 7401 return "Attachment"; 7402 if (code == FHIRDefinedType.BACKBONEELEMENT) 7403 return "BackboneElement"; 7404 if (code == FHIRDefinedType.CODEABLECONCEPT) 7405 return "CodeableConcept"; 7406 if (code == FHIRDefinedType.CODING) 7407 return "Coding"; 7408 if (code == FHIRDefinedType.CONTACTDETAIL) 7409 return "ContactDetail"; 7410 if (code == FHIRDefinedType.CONTACTPOINT) 7411 return "ContactPoint"; 7412 if (code == FHIRDefinedType.CONTRIBUTOR) 7413 return "Contributor"; 7414 if (code == FHIRDefinedType.COUNT) 7415 return "Count"; 7416 if (code == FHIRDefinedType.DATAREQUIREMENT) 7417 return "DataRequirement"; 7418 if (code == FHIRDefinedType.DISTANCE) 7419 return "Distance"; 7420 if (code == FHIRDefinedType.DOSAGE) 7421 return "Dosage"; 7422 if (code == FHIRDefinedType.DURATION) 7423 return "Duration"; 7424 if (code == FHIRDefinedType.ELEMENT) 7425 return "Element"; 7426 if (code == FHIRDefinedType.ELEMENTDEFINITION) 7427 return "ElementDefinition"; 7428 if (code == FHIRDefinedType.EXTENSION) 7429 return "Extension"; 7430 if (code == FHIRDefinedType.HUMANNAME) 7431 return "HumanName"; 7432 if (code == FHIRDefinedType.IDENTIFIER) 7433 return "Identifier"; 7434 if (code == FHIRDefinedType.META) 7435 return "Meta"; 7436 if (code == FHIRDefinedType.MONEY) 7437 return "Money"; 7438 if (code == FHIRDefinedType.NARRATIVE) 7439 return "Narrative"; 7440 if (code == FHIRDefinedType.PARAMETERDEFINITION) 7441 return "ParameterDefinition"; 7442 if (code == FHIRDefinedType.PERIOD) 7443 return "Period"; 7444 if (code == FHIRDefinedType.QUANTITY) 7445 return "Quantity"; 7446 if (code == FHIRDefinedType.RANGE) 7447 return "Range"; 7448 if (code == FHIRDefinedType.RATIO) 7449 return "Ratio"; 7450 if (code == FHIRDefinedType.REFERENCE) 7451 return "Reference"; 7452 if (code == FHIRDefinedType.RELATEDARTIFACT) 7453 return "RelatedArtifact"; 7454 if (code == FHIRDefinedType.SAMPLEDDATA) 7455 return "SampledData"; 7456 if (code == FHIRDefinedType.SIGNATURE) 7457 return "Signature"; 7458 if (code == FHIRDefinedType.SIMPLEQUANTITY) 7459 return "SimpleQuantity"; 7460 if (code == FHIRDefinedType.TIMING) 7461 return "Timing"; 7462 if (code == FHIRDefinedType.TRIGGERDEFINITION) 7463 return "TriggerDefinition"; 7464 if (code == FHIRDefinedType.USAGECONTEXT) 7465 return "UsageContext"; 7466 if (code == FHIRDefinedType.BASE64BINARY) 7467 return "base64Binary"; 7468 if (code == FHIRDefinedType.BOOLEAN) 7469 return "boolean"; 7470 if (code == FHIRDefinedType.CODE) 7471 return "code"; 7472 if (code == FHIRDefinedType.DATE) 7473 return "date"; 7474 if (code == FHIRDefinedType.DATETIME) 7475 return "dateTime"; 7476 if (code == FHIRDefinedType.DECIMAL) 7477 return "decimal"; 7478 if (code == FHIRDefinedType.ID) 7479 return "id"; 7480 if (code == FHIRDefinedType.INSTANT) 7481 return "instant"; 7482 if (code == FHIRDefinedType.INTEGER) 7483 return "integer"; 7484 if (code == FHIRDefinedType.MARKDOWN) 7485 return "markdown"; 7486 if (code == FHIRDefinedType.OID) 7487 return "oid"; 7488 if (code == FHIRDefinedType.POSITIVEINT) 7489 return "positiveInt"; 7490 if (code == FHIRDefinedType.STRING) 7491 return "string"; 7492 if (code == FHIRDefinedType.TIME) 7493 return "time"; 7494 if (code == FHIRDefinedType.UNSIGNEDINT) 7495 return "unsignedInt"; 7496 if (code == FHIRDefinedType.URI) 7497 return "uri"; 7498 if (code == FHIRDefinedType.UUID) 7499 return "uuid"; 7500 if (code == FHIRDefinedType.XHTML) 7501 return "xhtml"; 7502 if (code == FHIRDefinedType.ACCOUNT) 7503 return "Account"; 7504 if (code == FHIRDefinedType.ACTIVITYDEFINITION) 7505 return "ActivityDefinition"; 7506 if (code == FHIRDefinedType.ADVERSEEVENT) 7507 return "AdverseEvent"; 7508 if (code == FHIRDefinedType.ALLERGYINTOLERANCE) 7509 return "AllergyIntolerance"; 7510 if (code == FHIRDefinedType.APPOINTMENT) 7511 return "Appointment"; 7512 if (code == FHIRDefinedType.APPOINTMENTRESPONSE) 7513 return "AppointmentResponse"; 7514 if (code == FHIRDefinedType.AUDITEVENT) 7515 return "AuditEvent"; 7516 if (code == FHIRDefinedType.BASIC) 7517 return "Basic"; 7518 if (code == FHIRDefinedType.BINARY) 7519 return "Binary"; 7520 if (code == FHIRDefinedType.BODYSITE) 7521 return "BodySite"; 7522 if (code == FHIRDefinedType.BUNDLE) 7523 return "Bundle"; 7524 if (code == FHIRDefinedType.CAPABILITYSTATEMENT) 7525 return "CapabilityStatement"; 7526 if (code == FHIRDefinedType.CAREPLAN) 7527 return "CarePlan"; 7528 if (code == FHIRDefinedType.CARETEAM) 7529 return "CareTeam"; 7530 if (code == FHIRDefinedType.CHARGEITEM) 7531 return "ChargeItem"; 7532 if (code == FHIRDefinedType.CLAIM) 7533 return "Claim"; 7534 if (code == FHIRDefinedType.CLAIMRESPONSE) 7535 return "ClaimResponse"; 7536 if (code == FHIRDefinedType.CLINICALIMPRESSION) 7537 return "ClinicalImpression"; 7538 if (code == FHIRDefinedType.CODESYSTEM) 7539 return "CodeSystem"; 7540 if (code == FHIRDefinedType.COMMUNICATION) 7541 return "Communication"; 7542 if (code == FHIRDefinedType.COMMUNICATIONREQUEST) 7543 return "CommunicationRequest"; 7544 if (code == FHIRDefinedType.COMPARTMENTDEFINITION) 7545 return "CompartmentDefinition"; 7546 if (code == FHIRDefinedType.COMPOSITION) 7547 return "Composition"; 7548 if (code == FHIRDefinedType.CONCEPTMAP) 7549 return "ConceptMap"; 7550 if (code == FHIRDefinedType.CONDITION) 7551 return "Condition"; 7552 if (code == FHIRDefinedType.CONSENT) 7553 return "Consent"; 7554 if (code == FHIRDefinedType.CONTRACT) 7555 return "Contract"; 7556 if (code == FHIRDefinedType.COVERAGE) 7557 return "Coverage"; 7558 if (code == FHIRDefinedType.DATAELEMENT) 7559 return "DataElement"; 7560 if (code == FHIRDefinedType.DETECTEDISSUE) 7561 return "DetectedIssue"; 7562 if (code == FHIRDefinedType.DEVICE) 7563 return "Device"; 7564 if (code == FHIRDefinedType.DEVICECOMPONENT) 7565 return "DeviceComponent"; 7566 if (code == FHIRDefinedType.DEVICEMETRIC) 7567 return "DeviceMetric"; 7568 if (code == FHIRDefinedType.DEVICEREQUEST) 7569 return "DeviceRequest"; 7570 if (code == FHIRDefinedType.DEVICEUSESTATEMENT) 7571 return "DeviceUseStatement"; 7572 if (code == FHIRDefinedType.DIAGNOSTICREPORT) 7573 return "DiagnosticReport"; 7574 if (code == FHIRDefinedType.DOCUMENTMANIFEST) 7575 return "DocumentManifest"; 7576 if (code == FHIRDefinedType.DOCUMENTREFERENCE) 7577 return "DocumentReference"; 7578 if (code == FHIRDefinedType.DOMAINRESOURCE) 7579 return "DomainResource"; 7580 if (code == FHIRDefinedType.ELIGIBILITYREQUEST) 7581 return "EligibilityRequest"; 7582 if (code == FHIRDefinedType.ELIGIBILITYRESPONSE) 7583 return "EligibilityResponse"; 7584 if (code == FHIRDefinedType.ENCOUNTER) 7585 return "Encounter"; 7586 if (code == FHIRDefinedType.ENDPOINT) 7587 return "Endpoint"; 7588 if (code == FHIRDefinedType.ENROLLMENTREQUEST) 7589 return "EnrollmentRequest"; 7590 if (code == FHIRDefinedType.ENROLLMENTRESPONSE) 7591 return "EnrollmentResponse"; 7592 if (code == FHIRDefinedType.EPISODEOFCARE) 7593 return "EpisodeOfCare"; 7594 if (code == FHIRDefinedType.EXPANSIONPROFILE) 7595 return "ExpansionProfile"; 7596 if (code == FHIRDefinedType.EXPLANATIONOFBENEFIT) 7597 return "ExplanationOfBenefit"; 7598 if (code == FHIRDefinedType.FAMILYMEMBERHISTORY) 7599 return "FamilyMemberHistory"; 7600 if (code == FHIRDefinedType.FLAG) 7601 return "Flag"; 7602 if (code == FHIRDefinedType.GOAL) 7603 return "Goal"; 7604 if (code == FHIRDefinedType.GRAPHDEFINITION) 7605 return "GraphDefinition"; 7606 if (code == FHIRDefinedType.GROUP) 7607 return "Group"; 7608 if (code == FHIRDefinedType.GUIDANCERESPONSE) 7609 return "GuidanceResponse"; 7610 if (code == FHIRDefinedType.HEALTHCARESERVICE) 7611 return "HealthcareService"; 7612 if (code == FHIRDefinedType.IMAGINGMANIFEST) 7613 return "ImagingManifest"; 7614 if (code == FHIRDefinedType.IMAGINGSTUDY) 7615 return "ImagingStudy"; 7616 if (code == FHIRDefinedType.IMMUNIZATION) 7617 return "Immunization"; 7618 if (code == FHIRDefinedType.IMMUNIZATIONRECOMMENDATION) 7619 return "ImmunizationRecommendation"; 7620 if (code == FHIRDefinedType.IMPLEMENTATIONGUIDE) 7621 return "ImplementationGuide"; 7622 if (code == FHIRDefinedType.LIBRARY) 7623 return "Library"; 7624 if (code == FHIRDefinedType.LINKAGE) 7625 return "Linkage"; 7626 if (code == FHIRDefinedType.LIST) 7627 return "List"; 7628 if (code == FHIRDefinedType.LOCATION) 7629 return "Location"; 7630 if (code == FHIRDefinedType.MEASURE) 7631 return "Measure"; 7632 if (code == FHIRDefinedType.MEASUREREPORT) 7633 return "MeasureReport"; 7634 if (code == FHIRDefinedType.MEDIA) 7635 return "Media"; 7636 if (code == FHIRDefinedType.MEDICATION) 7637 return "Medication"; 7638 if (code == FHIRDefinedType.MEDICATIONADMINISTRATION) 7639 return "MedicationAdministration"; 7640 if (code == FHIRDefinedType.MEDICATIONDISPENSE) 7641 return "MedicationDispense"; 7642 if (code == FHIRDefinedType.MEDICATIONREQUEST) 7643 return "MedicationRequest"; 7644 if (code == FHIRDefinedType.MEDICATIONSTATEMENT) 7645 return "MedicationStatement"; 7646 if (code == FHIRDefinedType.MESSAGEDEFINITION) 7647 return "MessageDefinition"; 7648 if (code == FHIRDefinedType.MESSAGEHEADER) 7649 return "MessageHeader"; 7650 if (code == FHIRDefinedType.NAMINGSYSTEM) 7651 return "NamingSystem"; 7652 if (code == FHIRDefinedType.NUTRITIONORDER) 7653 return "NutritionOrder"; 7654 if (code == FHIRDefinedType.OBSERVATION) 7655 return "Observation"; 7656 if (code == FHIRDefinedType.OPERATIONDEFINITION) 7657 return "OperationDefinition"; 7658 if (code == FHIRDefinedType.OPERATIONOUTCOME) 7659 return "OperationOutcome"; 7660 if (code == FHIRDefinedType.ORGANIZATION) 7661 return "Organization"; 7662 if (code == FHIRDefinedType.PARAMETERS) 7663 return "Parameters"; 7664 if (code == FHIRDefinedType.PATIENT) 7665 return "Patient"; 7666 if (code == FHIRDefinedType.PAYMENTNOTICE) 7667 return "PaymentNotice"; 7668 if (code == FHIRDefinedType.PAYMENTRECONCILIATION) 7669 return "PaymentReconciliation"; 7670 if (code == FHIRDefinedType.PERSON) 7671 return "Person"; 7672 if (code == FHIRDefinedType.PLANDEFINITION) 7673 return "PlanDefinition"; 7674 if (code == FHIRDefinedType.PRACTITIONER) 7675 return "Practitioner"; 7676 if (code == FHIRDefinedType.PRACTITIONERROLE) 7677 return "PractitionerRole"; 7678 if (code == FHIRDefinedType.PROCEDURE) 7679 return "Procedure"; 7680 if (code == FHIRDefinedType.PROCEDUREREQUEST) 7681 return "ProcedureRequest"; 7682 if (code == FHIRDefinedType.PROCESSREQUEST) 7683 return "ProcessRequest"; 7684 if (code == FHIRDefinedType.PROCESSRESPONSE) 7685 return "ProcessResponse"; 7686 if (code == FHIRDefinedType.PROVENANCE) 7687 return "Provenance"; 7688 if (code == FHIRDefinedType.QUESTIONNAIRE) 7689 return "Questionnaire"; 7690 if (code == FHIRDefinedType.QUESTIONNAIRERESPONSE) 7691 return "QuestionnaireResponse"; 7692 if (code == FHIRDefinedType.REFERRALREQUEST) 7693 return "ReferralRequest"; 7694 if (code == FHIRDefinedType.RELATEDPERSON) 7695 return "RelatedPerson"; 7696 if (code == FHIRDefinedType.REQUESTGROUP) 7697 return "RequestGroup"; 7698 if (code == FHIRDefinedType.RESEARCHSTUDY) 7699 return "ResearchStudy"; 7700 if (code == FHIRDefinedType.RESEARCHSUBJECT) 7701 return "ResearchSubject"; 7702 if (code == FHIRDefinedType.RESOURCE) 7703 return "Resource"; 7704 if (code == FHIRDefinedType.RISKASSESSMENT) 7705 return "RiskAssessment"; 7706 if (code == FHIRDefinedType.SCHEDULE) 7707 return "Schedule"; 7708 if (code == FHIRDefinedType.SEARCHPARAMETER) 7709 return "SearchParameter"; 7710 if (code == FHIRDefinedType.SEQUENCE) 7711 return "Sequence"; 7712 if (code == FHIRDefinedType.SERVICEDEFINITION) 7713 return "ServiceDefinition"; 7714 if (code == FHIRDefinedType.SLOT) 7715 return "Slot"; 7716 if (code == FHIRDefinedType.SPECIMEN) 7717 return "Specimen"; 7718 if (code == FHIRDefinedType.STRUCTUREDEFINITION) 7719 return "StructureDefinition"; 7720 if (code == FHIRDefinedType.STRUCTUREMAP) 7721 return "StructureMap"; 7722 if (code == FHIRDefinedType.SUBSCRIPTION) 7723 return "Subscription"; 7724 if (code == FHIRDefinedType.SUBSTANCE) 7725 return "Substance"; 7726 if (code == FHIRDefinedType.SUPPLYDELIVERY) 7727 return "SupplyDelivery"; 7728 if (code == FHIRDefinedType.SUPPLYREQUEST) 7729 return "SupplyRequest"; 7730 if (code == FHIRDefinedType.TASK) 7731 return "Task"; 7732 if (code == FHIRDefinedType.TESTREPORT) 7733 return "TestReport"; 7734 if (code == FHIRDefinedType.TESTSCRIPT) 7735 return "TestScript"; 7736 if (code == FHIRDefinedType.VALUESET) 7737 return "ValueSet"; 7738 if (code == FHIRDefinedType.VISIONPRESCRIPTION) 7739 return "VisionPrescription"; 7740 return "?"; 7741 } 7742 public String toSystem(FHIRDefinedType code) { 7743 return code.getSystem(); 7744 } 7745 } 7746 7747 public enum MessageEvent { 7748 /** 7749 * The definition of a code system is used to create a simple collection of codes suitable for use for data entry or validation. An expanded code system will be returned, or an error message. 7750 */ 7751 CODESYSTEMEXPAND, 7752 /** 7753 * Change the status of a Medication Administration to show that it is complete. 7754 */ 7755 MEDICATIONADMINISTRATIONCOMPLETE, 7756 /** 7757 * Someone wishes to record that the record of administration of a medication is in error and should be ignored. 7758 */ 7759 MEDICATIONADMINISTRATIONNULLIFICATION, 7760 /** 7761 * Indicates that a medication has been recorded against the patient's record. 7762 */ 7763 MEDICATIONADMINISTRATIONRECORDING, 7764 /** 7765 * Update a Medication Administration record. 7766 */ 7767 MEDICATIONADMINISTRATIONUPDATE, 7768 /** 7769 * Notification of a change to an administrative resource (either create or update). Note that there is no delete, though some administrative resources have status or period elements for this use. 7770 */ 7771 ADMINNOTIFY, 7772 /** 7773 * Notification to convey information. 7774 */ 7775 COMMUNICATIONREQUEST, 7776 /** 7777 * Provide a diagnostic report, or update a previously provided diagnostic report. 7778 */ 7779 DIAGNOSTICREPORTPROVIDE, 7780 /** 7781 * Provide a simple observation or update a previously provided simple observation. 7782 */ 7783 OBSERVATIONPROVIDE, 7784 /** 7785 * Notification that two patient records actually identify the same patient. 7786 */ 7787 PATIENTLINK, 7788 /** 7789 * Notification that previous advice that two patient records concern the same patient is now considered incorrect. 7790 */ 7791 PATIENTUNLINK, 7792 /** 7793 * The definition of a value set is used to create a simple collection of codes suitable for use for data entry or validation. An expanded value set will be returned, or an error message. 7794 */ 7795 VALUESETEXPAND, 7796 /** 7797 * added to help the parsers 7798 */ 7799 NULL; 7800 public static MessageEvent fromCode(String codeString) throws FHIRException { 7801 if (codeString == null || "".equals(codeString)) 7802 return null; 7803 if ("CodeSystem-expand".equals(codeString)) 7804 return CODESYSTEMEXPAND; 7805 if ("MedicationAdministration-Complete".equals(codeString)) 7806 return MEDICATIONADMINISTRATIONCOMPLETE; 7807 if ("MedicationAdministration-Nullification".equals(codeString)) 7808 return MEDICATIONADMINISTRATIONNULLIFICATION; 7809 if ("MedicationAdministration-Recording".equals(codeString)) 7810 return MEDICATIONADMINISTRATIONRECORDING; 7811 if ("MedicationAdministration-Update".equals(codeString)) 7812 return MEDICATIONADMINISTRATIONUPDATE; 7813 if ("admin-notify".equals(codeString)) 7814 return ADMINNOTIFY; 7815 if ("communication-request".equals(codeString)) 7816 return COMMUNICATIONREQUEST; 7817 if ("diagnosticreport-provide".equals(codeString)) 7818 return DIAGNOSTICREPORTPROVIDE; 7819 if ("observation-provide".equals(codeString)) 7820 return OBSERVATIONPROVIDE; 7821 if ("patient-link".equals(codeString)) 7822 return PATIENTLINK; 7823 if ("patient-unlink".equals(codeString)) 7824 return PATIENTUNLINK; 7825 if ("valueset-expand".equals(codeString)) 7826 return VALUESETEXPAND; 7827 throw new FHIRException("Unknown MessageEvent code '"+codeString+"'"); 7828 } 7829 public String toCode() { 7830 switch (this) { 7831 case CODESYSTEMEXPAND: return "CodeSystem-expand"; 7832 case MEDICATIONADMINISTRATIONCOMPLETE: return "MedicationAdministration-Complete"; 7833 case MEDICATIONADMINISTRATIONNULLIFICATION: return "MedicationAdministration-Nullification"; 7834 case MEDICATIONADMINISTRATIONRECORDING: return "MedicationAdministration-Recording"; 7835 case MEDICATIONADMINISTRATIONUPDATE: return "MedicationAdministration-Update"; 7836 case ADMINNOTIFY: return "admin-notify"; 7837 case COMMUNICATIONREQUEST: return "communication-request"; 7838 case DIAGNOSTICREPORTPROVIDE: return "diagnosticreport-provide"; 7839 case OBSERVATIONPROVIDE: return "observation-provide"; 7840 case PATIENTLINK: return "patient-link"; 7841 case PATIENTUNLINK: return "patient-unlink"; 7842 case VALUESETEXPAND: return "valueset-expand"; 7843 case NULL: return null; 7844 default: return "?"; 7845 } 7846 } 7847 public String getSystem() { 7848 switch (this) { 7849 case CODESYSTEMEXPAND: return "http://hl7.org/fhir/message-events"; 7850 case MEDICATIONADMINISTRATIONCOMPLETE: return "http://hl7.org/fhir/message-events"; 7851 case MEDICATIONADMINISTRATIONNULLIFICATION: return "http://hl7.org/fhir/message-events"; 7852 case MEDICATIONADMINISTRATIONRECORDING: return "http://hl7.org/fhir/message-events"; 7853 case MEDICATIONADMINISTRATIONUPDATE: return "http://hl7.org/fhir/message-events"; 7854 case ADMINNOTIFY: return "http://hl7.org/fhir/message-events"; 7855 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/message-events"; 7856 case DIAGNOSTICREPORTPROVIDE: return "http://hl7.org/fhir/message-events"; 7857 case OBSERVATIONPROVIDE: return "http://hl7.org/fhir/message-events"; 7858 case PATIENTLINK: return "http://hl7.org/fhir/message-events"; 7859 case PATIENTUNLINK: return "http://hl7.org/fhir/message-events"; 7860 case VALUESETEXPAND: return "http://hl7.org/fhir/message-events"; 7861 case NULL: return null; 7862 default: return "?"; 7863 } 7864 } 7865 public String getDefinition() { 7866 switch (this) { 7867 case CODESYSTEMEXPAND: return "The definition of a code system is used to create a simple collection of codes suitable for use for data entry or validation. An expanded code system will be returned, or an error message."; 7868 case MEDICATIONADMINISTRATIONCOMPLETE: return "Change the status of a Medication Administration to show that it is complete."; 7869 case MEDICATIONADMINISTRATIONNULLIFICATION: return "Someone wishes to record that the record of administration of a medication is in error and should be ignored."; 7870 case MEDICATIONADMINISTRATIONRECORDING: return "Indicates that a medication has been recorded against the patient's record."; 7871 case MEDICATIONADMINISTRATIONUPDATE: return "Update a Medication Administration record."; 7872 case ADMINNOTIFY: return "Notification of a change to an administrative resource (either create or update). Note that there is no delete, though some administrative resources have status or period elements for this use."; 7873 case COMMUNICATIONREQUEST: return "Notification to convey information."; 7874 case DIAGNOSTICREPORTPROVIDE: return "Provide a diagnostic report, or update a previously provided diagnostic report."; 7875 case OBSERVATIONPROVIDE: return "Provide a simple observation or update a previously provided simple observation."; 7876 case PATIENTLINK: return "Notification that two patient records actually identify the same patient."; 7877 case PATIENTUNLINK: return "Notification that previous advice that two patient records concern the same patient is now considered incorrect."; 7878 case VALUESETEXPAND: return "The definition of a value set is used to create a simple collection of codes suitable for use for data entry or validation. An expanded value set will be returned, or an error message."; 7879 case NULL: return null; 7880 default: return "?"; 7881 } 7882 } 7883 public String getDisplay() { 7884 switch (this) { 7885 case CODESYSTEMEXPAND: return "CodeSystem-expand"; 7886 case MEDICATIONADMINISTRATIONCOMPLETE: return "MedicationAdministration-Complete"; 7887 case MEDICATIONADMINISTRATIONNULLIFICATION: return "MedicationAdministration-Nullification"; 7888 case MEDICATIONADMINISTRATIONRECORDING: return "MedicationAdministration-Recording"; 7889 case MEDICATIONADMINISTRATIONUPDATE: return "MedicationAdministration-Update"; 7890 case ADMINNOTIFY: return "admin-notify"; 7891 case COMMUNICATIONREQUEST: return "communication-request"; 7892 case DIAGNOSTICREPORTPROVIDE: return "diagnosticreport-provide"; 7893 case OBSERVATIONPROVIDE: return "observation-provide"; 7894 case PATIENTLINK: return "patient-link"; 7895 case PATIENTUNLINK: return "patient-unlink"; 7896 case VALUESETEXPAND: return "valueset-expand"; 7897 case NULL: return null; 7898 default: return "?"; 7899 } 7900 } 7901 } 7902 7903 public static class MessageEventEnumFactory implements EnumFactory<MessageEvent> { 7904 public MessageEvent fromCode(String codeString) throws IllegalArgumentException { 7905 if (codeString == null || "".equals(codeString)) 7906 if (codeString == null || "".equals(codeString)) 7907 return null; 7908 if ("CodeSystem-expand".equals(codeString)) 7909 return MessageEvent.CODESYSTEMEXPAND; 7910 if ("MedicationAdministration-Complete".equals(codeString)) 7911 return MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE; 7912 if ("MedicationAdministration-Nullification".equals(codeString)) 7913 return MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION; 7914 if ("MedicationAdministration-Recording".equals(codeString)) 7915 return MessageEvent.MEDICATIONADMINISTRATIONRECORDING; 7916 if ("MedicationAdministration-Update".equals(codeString)) 7917 return MessageEvent.MEDICATIONADMINISTRATIONUPDATE; 7918 if ("admin-notify".equals(codeString)) 7919 return MessageEvent.ADMINNOTIFY; 7920 if ("communication-request".equals(codeString)) 7921 return MessageEvent.COMMUNICATIONREQUEST; 7922 if ("diagnosticreport-provide".equals(codeString)) 7923 return MessageEvent.DIAGNOSTICREPORTPROVIDE; 7924 if ("observation-provide".equals(codeString)) 7925 return MessageEvent.OBSERVATIONPROVIDE; 7926 if ("patient-link".equals(codeString)) 7927 return MessageEvent.PATIENTLINK; 7928 if ("patient-unlink".equals(codeString)) 7929 return MessageEvent.PATIENTUNLINK; 7930 if ("valueset-expand".equals(codeString)) 7931 return MessageEvent.VALUESETEXPAND; 7932 throw new IllegalArgumentException("Unknown MessageEvent code '"+codeString+"'"); 7933 } 7934 public Enumeration<MessageEvent> fromType(PrimitiveType<?> code) throws FHIRException { 7935 if (code == null) 7936 return null; 7937 if (code.isEmpty()) 7938 return new Enumeration<MessageEvent>(this); 7939 String codeString = code.asStringValue(); 7940 if (codeString == null || "".equals(codeString)) 7941 return null; 7942 if ("CodeSystem-expand".equals(codeString)) 7943 return new Enumeration<MessageEvent>(this, MessageEvent.CODESYSTEMEXPAND); 7944 if ("MedicationAdministration-Complete".equals(codeString)) 7945 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE); 7946 if ("MedicationAdministration-Nullification".equals(codeString)) 7947 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION); 7948 if ("MedicationAdministration-Recording".equals(codeString)) 7949 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONRECORDING); 7950 if ("MedicationAdministration-Update".equals(codeString)) 7951 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONUPDATE); 7952 if ("admin-notify".equals(codeString)) 7953 return new Enumeration<MessageEvent>(this, MessageEvent.ADMINNOTIFY); 7954 if ("communication-request".equals(codeString)) 7955 return new Enumeration<MessageEvent>(this, MessageEvent.COMMUNICATIONREQUEST); 7956 if ("diagnosticreport-provide".equals(codeString)) 7957 return new Enumeration<MessageEvent>(this, MessageEvent.DIAGNOSTICREPORTPROVIDE); 7958 if ("observation-provide".equals(codeString)) 7959 return new Enumeration<MessageEvent>(this, MessageEvent.OBSERVATIONPROVIDE); 7960 if ("patient-link".equals(codeString)) 7961 return new Enumeration<MessageEvent>(this, MessageEvent.PATIENTLINK); 7962 if ("patient-unlink".equals(codeString)) 7963 return new Enumeration<MessageEvent>(this, MessageEvent.PATIENTUNLINK); 7964 if ("valueset-expand".equals(codeString)) 7965 return new Enumeration<MessageEvent>(this, MessageEvent.VALUESETEXPAND); 7966 throw new FHIRException("Unknown MessageEvent code '"+codeString+"'"); 7967 } 7968 public String toCode(MessageEvent code) { 7969 if (code == MessageEvent.NULL) 7970 return null; 7971 if (code == MessageEvent.CODESYSTEMEXPAND) 7972 return "CodeSystem-expand"; 7973 if (code == MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE) 7974 return "MedicationAdministration-Complete"; 7975 if (code == MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION) 7976 return "MedicationAdministration-Nullification"; 7977 if (code == MessageEvent.MEDICATIONADMINISTRATIONRECORDING) 7978 return "MedicationAdministration-Recording"; 7979 if (code == MessageEvent.MEDICATIONADMINISTRATIONUPDATE) 7980 return "MedicationAdministration-Update"; 7981 if (code == MessageEvent.ADMINNOTIFY) 7982 return "admin-notify"; 7983 if (code == MessageEvent.COMMUNICATIONREQUEST) 7984 return "communication-request"; 7985 if (code == MessageEvent.DIAGNOSTICREPORTPROVIDE) 7986 return "diagnosticreport-provide"; 7987 if (code == MessageEvent.OBSERVATIONPROVIDE) 7988 return "observation-provide"; 7989 if (code == MessageEvent.PATIENTLINK) 7990 return "patient-link"; 7991 if (code == MessageEvent.PATIENTUNLINK) 7992 return "patient-unlink"; 7993 if (code == MessageEvent.VALUESETEXPAND) 7994 return "valueset-expand"; 7995 return "?"; 7996 } 7997 public String toSystem(MessageEvent code) { 7998 return code.getSystem(); 7999 } 8000 } 8001 8002 public enum NoteType { 8003 /** 8004 * Display the note. 8005 */ 8006 DISPLAY, 8007 /** 8008 * Print the note on the form. 8009 */ 8010 PRINT, 8011 /** 8012 * Print the note for the operator. 8013 */ 8014 PRINTOPER, 8015 /** 8016 * added to help the parsers 8017 */ 8018 NULL; 8019 public static NoteType fromCode(String codeString) throws FHIRException { 8020 if (codeString == null || "".equals(codeString)) 8021 return null; 8022 if ("display".equals(codeString)) 8023 return DISPLAY; 8024 if ("print".equals(codeString)) 8025 return PRINT; 8026 if ("printoper".equals(codeString)) 8027 return PRINTOPER; 8028 throw new FHIRException("Unknown NoteType code '"+codeString+"'"); 8029 } 8030 public String toCode() { 8031 switch (this) { 8032 case DISPLAY: return "display"; 8033 case PRINT: return "print"; 8034 case PRINTOPER: return "printoper"; 8035 case NULL: return null; 8036 default: return "?"; 8037 } 8038 } 8039 public String getSystem() { 8040 switch (this) { 8041 case DISPLAY: return "http://hl7.org/fhir/note-type"; 8042 case PRINT: return "http://hl7.org/fhir/note-type"; 8043 case PRINTOPER: return "http://hl7.org/fhir/note-type"; 8044 case NULL: return null; 8045 default: return "?"; 8046 } 8047 } 8048 public String getDefinition() { 8049 switch (this) { 8050 case DISPLAY: return "Display the note."; 8051 case PRINT: return "Print the note on the form."; 8052 case PRINTOPER: return "Print the note for the operator."; 8053 case NULL: return null; 8054 default: return "?"; 8055 } 8056 } 8057 public String getDisplay() { 8058 switch (this) { 8059 case DISPLAY: return "Display"; 8060 case PRINT: return "Print (Form)"; 8061 case PRINTOPER: return "Print (Operator)"; 8062 case NULL: return null; 8063 default: return "?"; 8064 } 8065 } 8066 } 8067 8068 public static class NoteTypeEnumFactory implements EnumFactory<NoteType> { 8069 public NoteType fromCode(String codeString) throws IllegalArgumentException { 8070 if (codeString == null || "".equals(codeString)) 8071 if (codeString == null || "".equals(codeString)) 8072 return null; 8073 if ("display".equals(codeString)) 8074 return NoteType.DISPLAY; 8075 if ("print".equals(codeString)) 8076 return NoteType.PRINT; 8077 if ("printoper".equals(codeString)) 8078 return NoteType.PRINTOPER; 8079 throw new IllegalArgumentException("Unknown NoteType code '"+codeString+"'"); 8080 } 8081 public Enumeration<NoteType> fromType(PrimitiveType<?> code) throws FHIRException { 8082 if (code == null) 8083 return null; 8084 if (code.isEmpty()) 8085 return new Enumeration<NoteType>(this); 8086 String codeString = code.asStringValue(); 8087 if (codeString == null || "".equals(codeString)) 8088 return null; 8089 if ("display".equals(codeString)) 8090 return new Enumeration<NoteType>(this, NoteType.DISPLAY); 8091 if ("print".equals(codeString)) 8092 return new Enumeration<NoteType>(this, NoteType.PRINT); 8093 if ("printoper".equals(codeString)) 8094 return new Enumeration<NoteType>(this, NoteType.PRINTOPER); 8095 throw new FHIRException("Unknown NoteType code '"+codeString+"'"); 8096 } 8097 public String toCode(NoteType code) { 8098 if (code == NoteType.NULL) 8099 return null; 8100 if (code == NoteType.DISPLAY) 8101 return "display"; 8102 if (code == NoteType.PRINT) 8103 return "print"; 8104 if (code == NoteType.PRINTOPER) 8105 return "printoper"; 8106 return "?"; 8107 } 8108 public String toSystem(NoteType code) { 8109 return code.getSystem(); 8110 } 8111 } 8112 8113 public enum PublicationStatus { 8114 /** 8115 * This resource is still under development and is not yet considered to be ready for normal use. 8116 */ 8117 DRAFT, 8118 /** 8119 * This resource is ready for normal use. 8120 */ 8121 ACTIVE, 8122 /** 8123 * This resource has been withdrawn or superseded and should no longer be used. 8124 */ 8125 RETIRED, 8126 /** 8127 * The authoring system does not know which of the status values currently applies for this resource. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 8128 */ 8129 UNKNOWN, 8130 /** 8131 * added to help the parsers 8132 */ 8133 NULL; 8134 public static PublicationStatus fromCode(String codeString) throws FHIRException { 8135 if (codeString == null || "".equals(codeString)) 8136 return null; 8137 if ("draft".equals(codeString)) 8138 return DRAFT; 8139 if ("active".equals(codeString)) 8140 return ACTIVE; 8141 if ("retired".equals(codeString)) 8142 return RETIRED; 8143 if ("unknown".equals(codeString)) 8144 return UNKNOWN; 8145 throw new FHIRException("Unknown PublicationStatus code '"+codeString+"'"); 8146 } 8147 public String toCode() { 8148 switch (this) { 8149 case DRAFT: return "draft"; 8150 case ACTIVE: return "active"; 8151 case RETIRED: return "retired"; 8152 case UNKNOWN: return "unknown"; 8153 case NULL: return null; 8154 default: return "?"; 8155 } 8156 } 8157 public String getSystem() { 8158 switch (this) { 8159 case DRAFT: return "http://hl7.org/fhir/publication-status"; 8160 case ACTIVE: return "http://hl7.org/fhir/publication-status"; 8161 case RETIRED: return "http://hl7.org/fhir/publication-status"; 8162 case UNKNOWN: return "http://hl7.org/fhir/publication-status"; 8163 case NULL: return null; 8164 default: return "?"; 8165 } 8166 } 8167 public String getDefinition() { 8168 switch (this) { 8169 case DRAFT: return "This resource is still under development and is not yet considered to be ready for normal use."; 8170 case ACTIVE: return "This resource is ready for normal use."; 8171 case RETIRED: return "This resource has been withdrawn or superseded and should no longer be used."; 8172 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this resource. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 8173 case NULL: return null; 8174 default: return "?"; 8175 } 8176 } 8177 public String getDisplay() { 8178 switch (this) { 8179 case DRAFT: return "Draft"; 8180 case ACTIVE: return "Active"; 8181 case RETIRED: return "Retired"; 8182 case UNKNOWN: return "Unknown"; 8183 case NULL: return null; 8184 default: return "?"; 8185 } 8186 } 8187 } 8188 8189 public static class PublicationStatusEnumFactory implements EnumFactory<PublicationStatus> { 8190 public PublicationStatus fromCode(String codeString) throws IllegalArgumentException { 8191 if (codeString == null || "".equals(codeString)) 8192 if (codeString == null || "".equals(codeString)) 8193 return null; 8194 if ("draft".equals(codeString)) 8195 return PublicationStatus.DRAFT; 8196 if ("active".equals(codeString)) 8197 return PublicationStatus.ACTIVE; 8198 if ("retired".equals(codeString)) 8199 return PublicationStatus.RETIRED; 8200 if ("unknown".equals(codeString)) 8201 return PublicationStatus.UNKNOWN; 8202 throw new IllegalArgumentException("Unknown PublicationStatus code '"+codeString+"'"); 8203 } 8204 public Enumeration<PublicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 8205 if (code == null) 8206 return null; 8207 if (code.isEmpty()) 8208 return new Enumeration<PublicationStatus>(this); 8209 String codeString = code.asStringValue(); 8210 if (codeString == null || "".equals(codeString)) 8211 return null; 8212 if ("draft".equals(codeString)) 8213 return new Enumeration<PublicationStatus>(this, PublicationStatus.DRAFT); 8214 if ("active".equals(codeString)) 8215 return new Enumeration<PublicationStatus>(this, PublicationStatus.ACTIVE); 8216 if ("retired".equals(codeString)) 8217 return new Enumeration<PublicationStatus>(this, PublicationStatus.RETIRED); 8218 if ("unknown".equals(codeString)) 8219 return new Enumeration<PublicationStatus>(this, PublicationStatus.UNKNOWN); 8220 throw new FHIRException("Unknown PublicationStatus code '"+codeString+"'"); 8221 } 8222 public String toCode(PublicationStatus code) { 8223 if (code == PublicationStatus.NULL) 8224 return null; 8225 if (code == PublicationStatus.DRAFT) 8226 return "draft"; 8227 if (code == PublicationStatus.ACTIVE) 8228 return "active"; 8229 if (code == PublicationStatus.RETIRED) 8230 return "retired"; 8231 if (code == PublicationStatus.UNKNOWN) 8232 return "unknown"; 8233 return "?"; 8234 } 8235 public String toSystem(PublicationStatus code) { 8236 return code.getSystem(); 8237 } 8238 } 8239 8240 public enum RemittanceOutcome { 8241 /** 8242 * The processing has completed without errors 8243 */ 8244 COMPLETE, 8245 /** 8246 * One or more errors have been detected in the Claim 8247 */ 8248 ERROR, 8249 /** 8250 * No errors have been detected in the Claim and some of the adjudication has been performed. 8251 */ 8252 PARTIAL, 8253 /** 8254 * added to help the parsers 8255 */ 8256 NULL; 8257 public static RemittanceOutcome fromCode(String codeString) throws FHIRException { 8258 if (codeString == null || "".equals(codeString)) 8259 return null; 8260 if ("complete".equals(codeString)) 8261 return COMPLETE; 8262 if ("error".equals(codeString)) 8263 return ERROR; 8264 if ("partial".equals(codeString)) 8265 return PARTIAL; 8266 throw new FHIRException("Unknown RemittanceOutcome code '"+codeString+"'"); 8267 } 8268 public String toCode() { 8269 switch (this) { 8270 case COMPLETE: return "complete"; 8271 case ERROR: return "error"; 8272 case PARTIAL: return "partial"; 8273 case NULL: return null; 8274 default: return "?"; 8275 } 8276 } 8277 public String getSystem() { 8278 switch (this) { 8279 case COMPLETE: return "http://hl7.org/fhir/remittance-outcome"; 8280 case ERROR: return "http://hl7.org/fhir/remittance-outcome"; 8281 case PARTIAL: return "http://hl7.org/fhir/remittance-outcome"; 8282 case NULL: return null; 8283 default: return "?"; 8284 } 8285 } 8286 public String getDefinition() { 8287 switch (this) { 8288 case COMPLETE: return "The processing has completed without errors"; 8289 case ERROR: return "One or more errors have been detected in the Claim"; 8290 case PARTIAL: return "No errors have been detected in the Claim and some of the adjudication has been performed."; 8291 case NULL: return null; 8292 default: return "?"; 8293 } 8294 } 8295 public String getDisplay() { 8296 switch (this) { 8297 case COMPLETE: return "Processing Complete"; 8298 case ERROR: return "Error"; 8299 case PARTIAL: return "Partial Processing"; 8300 case NULL: return null; 8301 default: return "?"; 8302 } 8303 } 8304 } 8305 8306 public static class RemittanceOutcomeEnumFactory implements EnumFactory<RemittanceOutcome> { 8307 public RemittanceOutcome fromCode(String codeString) throws IllegalArgumentException { 8308 if (codeString == null || "".equals(codeString)) 8309 if (codeString == null || "".equals(codeString)) 8310 return null; 8311 if ("complete".equals(codeString)) 8312 return RemittanceOutcome.COMPLETE; 8313 if ("error".equals(codeString)) 8314 return RemittanceOutcome.ERROR; 8315 if ("partial".equals(codeString)) 8316 return RemittanceOutcome.PARTIAL; 8317 throw new IllegalArgumentException("Unknown RemittanceOutcome code '"+codeString+"'"); 8318 } 8319 public Enumeration<RemittanceOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 8320 if (code == null) 8321 return null; 8322 if (code.isEmpty()) 8323 return new Enumeration<RemittanceOutcome>(this); 8324 String codeString = code.asStringValue(); 8325 if (codeString == null || "".equals(codeString)) 8326 return null; 8327 if ("complete".equals(codeString)) 8328 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.COMPLETE); 8329 if ("error".equals(codeString)) 8330 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.ERROR); 8331 if ("partial".equals(codeString)) 8332 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.PARTIAL); 8333 throw new FHIRException("Unknown RemittanceOutcome code '"+codeString+"'"); 8334 } 8335 public String toCode(RemittanceOutcome code) { 8336 if (code == RemittanceOutcome.NULL) 8337 return null; 8338 if (code == RemittanceOutcome.COMPLETE) 8339 return "complete"; 8340 if (code == RemittanceOutcome.ERROR) 8341 return "error"; 8342 if (code == RemittanceOutcome.PARTIAL) 8343 return "partial"; 8344 return "?"; 8345 } 8346 public String toSystem(RemittanceOutcome code) { 8347 return code.getSystem(); 8348 } 8349 } 8350 8351 public enum ResourceType { 8352 /** 8353 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 8354 */ 8355 ACCOUNT, 8356 /** 8357 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 8358 */ 8359 ACTIVITYDEFINITION, 8360 /** 8361 * Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death. 8362 */ 8363 ADVERSEEVENT, 8364 /** 8365 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 8366 */ 8367 ALLERGYINTOLERANCE, 8368 /** 8369 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 8370 */ 8371 APPOINTMENT, 8372 /** 8373 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 8374 */ 8375 APPOINTMENTRESPONSE, 8376 /** 8377 * A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage. 8378 */ 8379 AUDITEVENT, 8380 /** 8381 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 8382 */ 8383 BASIC, 8384 /** 8385 * A binary resource can contain any content, whether text, image, pdf, zip archive, etc. 8386 */ 8387 BINARY, 8388 /** 8389 * Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 8390 */ 8391 BODYSITE, 8392 /** 8393 * A container for a collection of resources. 8394 */ 8395 BUNDLE, 8396 /** 8397 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 8398 */ 8399 CAPABILITYSTATEMENT, 8400 /** 8401 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 8402 */ 8403 CAREPLAN, 8404 /** 8405 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 8406 */ 8407 CARETEAM, 8408 /** 8409 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 8410 */ 8411 CHARGEITEM, 8412 /** 8413 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 8414 */ 8415 CLAIM, 8416 /** 8417 * This resource provides the adjudication details from the processing of a Claim resource. 8418 */ 8419 CLAIMRESPONSE, 8420 /** 8421 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 8422 */ 8423 CLINICALIMPRESSION, 8424 /** 8425 * A code system resource specifies a set of codes drawn from one or more code systems. 8426 */ 8427 CODESYSTEM, 8428 /** 8429 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 8430 */ 8431 COMMUNICATION, 8432 /** 8433 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 8434 */ 8435 COMMUNICATIONREQUEST, 8436 /** 8437 * A compartment definition that defines how resources are accessed on a server. 8438 */ 8439 COMPARTMENTDEFINITION, 8440 /** 8441 * A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained. 8442 */ 8443 COMPOSITION, 8444 /** 8445 * A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models. 8446 */ 8447 CONCEPTMAP, 8448 /** 8449 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 8450 */ 8451 CONDITION, 8452 /** 8453 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 8454 */ 8455 CONSENT, 8456 /** 8457 * A formal agreement between parties regarding the conduct of business, exchange of information or other matters. 8458 */ 8459 CONTRACT, 8460 /** 8461 * Financial instrument which may be used to reimburse or pay for health care products and services. 8462 */ 8463 COVERAGE, 8464 /** 8465 * The formal description of a single piece of information that can be gathered and reported. 8466 */ 8467 DATAELEMENT, 8468 /** 8469 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 8470 */ 8471 DETECTEDISSUE, 8472 /** 8473 * This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc. 8474 */ 8475 DEVICE, 8476 /** 8477 * The characteristics, operational status and capabilities of a medical-related component of a medical device. 8478 */ 8479 DEVICECOMPONENT, 8480 /** 8481 * Describes a measurement, calculation or setting capability of a medical device. 8482 */ 8483 DEVICEMETRIC, 8484 /** 8485 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 8486 */ 8487 DEVICEREQUEST, 8488 /** 8489 * A record of a device being used by a patient where the record is the result of a report from the patient or another clinician. 8490 */ 8491 DEVICEUSESTATEMENT, 8492 /** 8493 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 8494 */ 8495 DIAGNOSTICREPORT, 8496 /** 8497 * A collection of documents compiled for a purpose together with metadata that applies to the collection. 8498 */ 8499 DOCUMENTMANIFEST, 8500 /** 8501 * A reference to a document. 8502 */ 8503 DOCUMENTREFERENCE, 8504 /** 8505 * A resource that includes narrative, extensions, and contained resources. 8506 */ 8507 DOMAINRESOURCE, 8508 /** 8509 * The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 8510 */ 8511 ELIGIBILITYREQUEST, 8512 /** 8513 * This resource provides eligibility and plan details from the processing of an Eligibility resource. 8514 */ 8515 ELIGIBILITYRESPONSE, 8516 /** 8517 * An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient. 8518 */ 8519 ENCOUNTER, 8520 /** 8521 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 8522 */ 8523 ENDPOINT, 8524 /** 8525 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 8526 */ 8527 ENROLLMENTREQUEST, 8528 /** 8529 * This resource provides enrollment and plan details from the processing of an Enrollment resource. 8530 */ 8531 ENROLLMENTRESPONSE, 8532 /** 8533 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 8534 */ 8535 EPISODEOFCARE, 8536 /** 8537 * Resource to define constraints on the Expansion of a FHIR ValueSet. 8538 */ 8539 EXPANSIONPROFILE, 8540 /** 8541 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 8542 */ 8543 EXPLANATIONOFBENEFIT, 8544 /** 8545 * Significant health events and conditions for a person related to the patient relevant in the context of care for the patient. 8546 */ 8547 FAMILYMEMBERHISTORY, 8548 /** 8549 * Prospective warnings of potential issues when providing care to the patient. 8550 */ 8551 FLAG, 8552 /** 8553 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 8554 */ 8555 GOAL, 8556 /** 8557 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 8558 */ 8559 GRAPHDEFINITION, 8560 /** 8561 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 8562 */ 8563 GROUP, 8564 /** 8565 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 8566 */ 8567 GUIDANCERESPONSE, 8568 /** 8569 * The details of a healthcare service available at a location. 8570 */ 8571 HEALTHCARESERVICE, 8572 /** 8573 * A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection. 8574 */ 8575 IMAGINGMANIFEST, 8576 /** 8577 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 8578 */ 8579 IMAGINGSTUDY, 8580 /** 8581 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 8582 */ 8583 IMMUNIZATION, 8584 /** 8585 * A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification. 8586 */ 8587 IMMUNIZATIONRECOMMENDATION, 8588 /** 8589 * A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 8590 */ 8591 IMPLEMENTATIONGUIDE, 8592 /** 8593 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 8594 */ 8595 LIBRARY, 8596 /** 8597 * Identifies two or more records (resource instances) that are referring to the same real-world "occurrence". 8598 */ 8599 LINKAGE, 8600 /** 8601 * A set of information summarized from a list of other resources. 8602 */ 8603 LIST, 8604 /** 8605 * Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated. 8606 */ 8607 LOCATION, 8608 /** 8609 * The Measure resource provides the definition of a quality measure. 8610 */ 8611 MEASURE, 8612 /** 8613 * The MeasureReport resource contains the results of evaluating a measure. 8614 */ 8615 MEASUREREPORT, 8616 /** 8617 * A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference. 8618 */ 8619 MEDIA, 8620 /** 8621 * This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication. 8622 */ 8623 MEDICATION, 8624 /** 8625 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. 8626 */ 8627 MEDICATIONADMINISTRATION, 8628 /** 8629 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 8630 */ 8631 MEDICATIONDISPENSE, 8632 /** 8633 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 8634 */ 8635 MEDICATIONREQUEST, 8636 /** 8637 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 8638 8639The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 8640 */ 8641 MEDICATIONSTATEMENT, 8642 /** 8643 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 8644 */ 8645 MESSAGEDEFINITION, 8646 /** 8647 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 8648 */ 8649 MESSAGEHEADER, 8650 /** 8651 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 8652 */ 8653 NAMINGSYSTEM, 8654 /** 8655 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 8656 */ 8657 NUTRITIONORDER, 8658 /** 8659 * Measurements and simple assertions made about a patient, device or other subject. 8660 */ 8661 OBSERVATION, 8662 /** 8663 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 8664 */ 8665 OPERATIONDEFINITION, 8666 /** 8667 * A collection of error, warning or information messages that result from a system action. 8668 */ 8669 OPERATIONOUTCOME, 8670 /** 8671 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc. 8672 */ 8673 ORGANIZATION, 8674 /** 8675 * This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it. 8676 */ 8677 PARAMETERS, 8678 /** 8679 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 8680 */ 8681 PATIENT, 8682 /** 8683 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 8684 */ 8685 PAYMENTNOTICE, 8686 /** 8687 * This resource provides payment details and claim references supporting a bulk payment. 8688 */ 8689 PAYMENTRECONCILIATION, 8690 /** 8691 * Demographics and administrative information about a person independent of a specific health-related context. 8692 */ 8693 PERSON, 8694 /** 8695 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols. 8696 */ 8697 PLANDEFINITION, 8698 /** 8699 * A person who is directly or indirectly involved in the provisioning of healthcare. 8700 */ 8701 PRACTITIONER, 8702 /** 8703 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 8704 */ 8705 PRACTITIONERROLE, 8706 /** 8707 * An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy. 8708 */ 8709 PROCEDURE, 8710 /** 8711 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 8712 */ 8713 PROCEDUREREQUEST, 8714 /** 8715 * This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources. 8716 */ 8717 PROCESSREQUEST, 8718 /** 8719 * This resource provides processing status, errors and notes from the processing of a resource. 8720 */ 8721 PROCESSRESPONSE, 8722 /** 8723 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 8724 */ 8725 PROVENANCE, 8726 /** 8727 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 8728 */ 8729 QUESTIONNAIRE, 8730 /** 8731 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 8732 */ 8733 QUESTIONNAIRERESPONSE, 8734 /** 8735 * Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization. 8736 */ 8737 REFERRALREQUEST, 8738 /** 8739 * Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 8740 */ 8741 RELATEDPERSON, 8742 /** 8743 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 8744 */ 8745 REQUESTGROUP, 8746 /** 8747 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 8748 */ 8749 RESEARCHSTUDY, 8750 /** 8751 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 8752 */ 8753 RESEARCHSUBJECT, 8754 /** 8755 * This is the base resource type for everything. 8756 */ 8757 RESOURCE, 8758 /** 8759 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 8760 */ 8761 RISKASSESSMENT, 8762 /** 8763 * A container for slots of time that may be available for booking appointments. 8764 */ 8765 SCHEDULE, 8766 /** 8767 * A search parameter that defines a named search item that can be used to search/filter on a resource. 8768 */ 8769 SEARCHPARAMETER, 8770 /** 8771 * Raw data describing a biological sequence. 8772 */ 8773 SEQUENCE, 8774 /** 8775 * The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking. 8776 */ 8777 SERVICEDEFINITION, 8778 /** 8779 * A slot of time on a schedule that may be available for booking appointments. 8780 */ 8781 SLOT, 8782 /** 8783 * A sample to be used for analysis. 8784 */ 8785 SPECIMEN, 8786 /** 8787 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 8788 */ 8789 STRUCTUREDEFINITION, 8790 /** 8791 * A Map of relationships between 2 structures that can be used to transform data. 8792 */ 8793 STRUCTUREMAP, 8794 /** 8795 * The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined "channel" so that another system is able to take an appropriate action. 8796 */ 8797 SUBSCRIPTION, 8798 /** 8799 * A homogeneous material with a definite composition. 8800 */ 8801 SUBSTANCE, 8802 /** 8803 * Record of delivery of what is supplied. 8804 */ 8805 SUPPLYDELIVERY, 8806 /** 8807 * A record of a request for a medication, substance or device used in the healthcare setting. 8808 */ 8809 SUPPLYREQUEST, 8810 /** 8811 * A task to be performed. 8812 */ 8813 TASK, 8814 /** 8815 * A summary of information based on the results of executing a TestScript. 8816 */ 8817 TESTREPORT, 8818 /** 8819 * A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification. 8820 */ 8821 TESTSCRIPT, 8822 /** 8823 * A value set specifies a set of codes drawn from one or more code systems. 8824 */ 8825 VALUESET, 8826 /** 8827 * An authorization for the supply of glasses and/or contact lenses to a patient. 8828 */ 8829 VISIONPRESCRIPTION, 8830 /** 8831 * added to help the parsers 8832 */ 8833 NULL; 8834 public static ResourceType fromCode(String codeString) throws FHIRException { 8835 if (codeString == null || "".equals(codeString)) 8836 return null; 8837 if ("Account".equals(codeString)) 8838 return ACCOUNT; 8839 if ("ActivityDefinition".equals(codeString)) 8840 return ACTIVITYDEFINITION; 8841 if ("AdverseEvent".equals(codeString)) 8842 return ADVERSEEVENT; 8843 if ("AllergyIntolerance".equals(codeString)) 8844 return ALLERGYINTOLERANCE; 8845 if ("Appointment".equals(codeString)) 8846 return APPOINTMENT; 8847 if ("AppointmentResponse".equals(codeString)) 8848 return APPOINTMENTRESPONSE; 8849 if ("AuditEvent".equals(codeString)) 8850 return AUDITEVENT; 8851 if ("Basic".equals(codeString)) 8852 return BASIC; 8853 if ("Binary".equals(codeString)) 8854 return BINARY; 8855 if ("BodySite".equals(codeString)) 8856 return BODYSITE; 8857 if ("Bundle".equals(codeString)) 8858 return BUNDLE; 8859 if ("CapabilityStatement".equals(codeString)) 8860 return CAPABILITYSTATEMENT; 8861 if ("CarePlan".equals(codeString)) 8862 return CAREPLAN; 8863 if ("CareTeam".equals(codeString)) 8864 return CARETEAM; 8865 if ("ChargeItem".equals(codeString)) 8866 return CHARGEITEM; 8867 if ("Claim".equals(codeString)) 8868 return CLAIM; 8869 if ("ClaimResponse".equals(codeString)) 8870 return CLAIMRESPONSE; 8871 if ("ClinicalImpression".equals(codeString)) 8872 return CLINICALIMPRESSION; 8873 if ("CodeSystem".equals(codeString)) 8874 return CODESYSTEM; 8875 if ("Communication".equals(codeString)) 8876 return COMMUNICATION; 8877 if ("CommunicationRequest".equals(codeString)) 8878 return COMMUNICATIONREQUEST; 8879 if ("CompartmentDefinition".equals(codeString)) 8880 return COMPARTMENTDEFINITION; 8881 if ("Composition".equals(codeString)) 8882 return COMPOSITION; 8883 if ("ConceptMap".equals(codeString)) 8884 return CONCEPTMAP; 8885 if ("Condition".equals(codeString)) 8886 return CONDITION; 8887 if ("Consent".equals(codeString)) 8888 return CONSENT; 8889 if ("Contract".equals(codeString)) 8890 return CONTRACT; 8891 if ("Coverage".equals(codeString)) 8892 return COVERAGE; 8893 if ("DataElement".equals(codeString)) 8894 return DATAELEMENT; 8895 if ("DetectedIssue".equals(codeString)) 8896 return DETECTEDISSUE; 8897 if ("Device".equals(codeString)) 8898 return DEVICE; 8899 if ("DeviceComponent".equals(codeString)) 8900 return DEVICECOMPONENT; 8901 if ("DeviceMetric".equals(codeString)) 8902 return DEVICEMETRIC; 8903 if ("DeviceRequest".equals(codeString)) 8904 return DEVICEREQUEST; 8905 if ("DeviceUseStatement".equals(codeString)) 8906 return DEVICEUSESTATEMENT; 8907 if ("DiagnosticReport".equals(codeString)) 8908 return DIAGNOSTICREPORT; 8909 if ("DocumentManifest".equals(codeString)) 8910 return DOCUMENTMANIFEST; 8911 if ("DocumentReference".equals(codeString)) 8912 return DOCUMENTREFERENCE; 8913 if ("DomainResource".equals(codeString)) 8914 return DOMAINRESOURCE; 8915 if ("EligibilityRequest".equals(codeString)) 8916 return ELIGIBILITYREQUEST; 8917 if ("EligibilityResponse".equals(codeString)) 8918 return ELIGIBILITYRESPONSE; 8919 if ("Encounter".equals(codeString)) 8920 return ENCOUNTER; 8921 if ("Endpoint".equals(codeString)) 8922 return ENDPOINT; 8923 if ("EnrollmentRequest".equals(codeString)) 8924 return ENROLLMENTREQUEST; 8925 if ("EnrollmentResponse".equals(codeString)) 8926 return ENROLLMENTRESPONSE; 8927 if ("EpisodeOfCare".equals(codeString)) 8928 return EPISODEOFCARE; 8929 if ("ExpansionProfile".equals(codeString)) 8930 return EXPANSIONPROFILE; 8931 if ("ExplanationOfBenefit".equals(codeString)) 8932 return EXPLANATIONOFBENEFIT; 8933 if ("FamilyMemberHistory".equals(codeString)) 8934 return FAMILYMEMBERHISTORY; 8935 if ("Flag".equals(codeString)) 8936 return FLAG; 8937 if ("Goal".equals(codeString)) 8938 return GOAL; 8939 if ("GraphDefinition".equals(codeString)) 8940 return GRAPHDEFINITION; 8941 if ("Group".equals(codeString)) 8942 return GROUP; 8943 if ("GuidanceResponse".equals(codeString)) 8944 return GUIDANCERESPONSE; 8945 if ("HealthcareService".equals(codeString)) 8946 return HEALTHCARESERVICE; 8947 if ("ImagingManifest".equals(codeString)) 8948 return IMAGINGMANIFEST; 8949 if ("ImagingStudy".equals(codeString)) 8950 return IMAGINGSTUDY; 8951 if ("Immunization".equals(codeString)) 8952 return IMMUNIZATION; 8953 if ("ImmunizationRecommendation".equals(codeString)) 8954 return IMMUNIZATIONRECOMMENDATION; 8955 if ("ImplementationGuide".equals(codeString)) 8956 return IMPLEMENTATIONGUIDE; 8957 if ("Library".equals(codeString)) 8958 return LIBRARY; 8959 if ("Linkage".equals(codeString)) 8960 return LINKAGE; 8961 if ("List".equals(codeString)) 8962 return LIST; 8963 if ("Location".equals(codeString)) 8964 return LOCATION; 8965 if ("Measure".equals(codeString)) 8966 return MEASURE; 8967 if ("MeasureReport".equals(codeString)) 8968 return MEASUREREPORT; 8969 if ("Media".equals(codeString)) 8970 return MEDIA; 8971 if ("Medication".equals(codeString)) 8972 return MEDICATION; 8973 if ("MedicationAdministration".equals(codeString)) 8974 return MEDICATIONADMINISTRATION; 8975 if ("MedicationDispense".equals(codeString)) 8976 return MEDICATIONDISPENSE; 8977 if ("MedicationRequest".equals(codeString)) 8978 return MEDICATIONREQUEST; 8979 if ("MedicationStatement".equals(codeString)) 8980 return MEDICATIONSTATEMENT; 8981 if ("MessageDefinition".equals(codeString)) 8982 return MESSAGEDEFINITION; 8983 if ("MessageHeader".equals(codeString)) 8984 return MESSAGEHEADER; 8985 if ("NamingSystem".equals(codeString)) 8986 return NAMINGSYSTEM; 8987 if ("NutritionOrder".equals(codeString)) 8988 return NUTRITIONORDER; 8989 if ("Observation".equals(codeString)) 8990 return OBSERVATION; 8991 if ("OperationDefinition".equals(codeString)) 8992 return OPERATIONDEFINITION; 8993 if ("OperationOutcome".equals(codeString)) 8994 return OPERATIONOUTCOME; 8995 if ("Organization".equals(codeString)) 8996 return ORGANIZATION; 8997 if ("Parameters".equals(codeString)) 8998 return PARAMETERS; 8999 if ("Patient".equals(codeString)) 9000 return PATIENT; 9001 if ("PaymentNotice".equals(codeString)) 9002 return PAYMENTNOTICE; 9003 if ("PaymentReconciliation".equals(codeString)) 9004 return PAYMENTRECONCILIATION; 9005 if ("Person".equals(codeString)) 9006 return PERSON; 9007 if ("PlanDefinition".equals(codeString)) 9008 return PLANDEFINITION; 9009 if ("Practitioner".equals(codeString)) 9010 return PRACTITIONER; 9011 if ("PractitionerRole".equals(codeString)) 9012 return PRACTITIONERROLE; 9013 if ("Procedure".equals(codeString)) 9014 return PROCEDURE; 9015 if ("ProcedureRequest".equals(codeString)) 9016 return PROCEDUREREQUEST; 9017 if ("ProcessRequest".equals(codeString)) 9018 return PROCESSREQUEST; 9019 if ("ProcessResponse".equals(codeString)) 9020 return PROCESSRESPONSE; 9021 if ("Provenance".equals(codeString)) 9022 return PROVENANCE; 9023 if ("Questionnaire".equals(codeString)) 9024 return QUESTIONNAIRE; 9025 if ("QuestionnaireResponse".equals(codeString)) 9026 return QUESTIONNAIRERESPONSE; 9027 if ("ReferralRequest".equals(codeString)) 9028 return REFERRALREQUEST; 9029 if ("RelatedPerson".equals(codeString)) 9030 return RELATEDPERSON; 9031 if ("RequestGroup".equals(codeString)) 9032 return REQUESTGROUP; 9033 if ("ResearchStudy".equals(codeString)) 9034 return RESEARCHSTUDY; 9035 if ("ResearchSubject".equals(codeString)) 9036 return RESEARCHSUBJECT; 9037 if ("Resource".equals(codeString)) 9038 return RESOURCE; 9039 if ("RiskAssessment".equals(codeString)) 9040 return RISKASSESSMENT; 9041 if ("Schedule".equals(codeString)) 9042 return SCHEDULE; 9043 if ("SearchParameter".equals(codeString)) 9044 return SEARCHPARAMETER; 9045 if ("Sequence".equals(codeString)) 9046 return SEQUENCE; 9047 if ("ServiceDefinition".equals(codeString)) 9048 return SERVICEDEFINITION; 9049 if ("Slot".equals(codeString)) 9050 return SLOT; 9051 if ("Specimen".equals(codeString)) 9052 return SPECIMEN; 9053 if ("StructureDefinition".equals(codeString)) 9054 return STRUCTUREDEFINITION; 9055 if ("StructureMap".equals(codeString)) 9056 return STRUCTUREMAP; 9057 if ("Subscription".equals(codeString)) 9058 return SUBSCRIPTION; 9059 if ("Substance".equals(codeString)) 9060 return SUBSTANCE; 9061 if ("SupplyDelivery".equals(codeString)) 9062 return SUPPLYDELIVERY; 9063 if ("SupplyRequest".equals(codeString)) 9064 return SUPPLYREQUEST; 9065 if ("Task".equals(codeString)) 9066 return TASK; 9067 if ("TestReport".equals(codeString)) 9068 return TESTREPORT; 9069 if ("TestScript".equals(codeString)) 9070 return TESTSCRIPT; 9071 if ("ValueSet".equals(codeString)) 9072 return VALUESET; 9073 if ("VisionPrescription".equals(codeString)) 9074 return VISIONPRESCRIPTION; 9075 throw new FHIRException("Unknown ResourceType code '"+codeString+"'"); 9076 } 9077 public String toCode() { 9078 switch (this) { 9079 case ACCOUNT: return "Account"; 9080 case ACTIVITYDEFINITION: return "ActivityDefinition"; 9081 case ADVERSEEVENT: return "AdverseEvent"; 9082 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 9083 case APPOINTMENT: return "Appointment"; 9084 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 9085 case AUDITEVENT: return "AuditEvent"; 9086 case BASIC: return "Basic"; 9087 case BINARY: return "Binary"; 9088 case BODYSITE: return "BodySite"; 9089 case BUNDLE: return "Bundle"; 9090 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 9091 case CAREPLAN: return "CarePlan"; 9092 case CARETEAM: return "CareTeam"; 9093 case CHARGEITEM: return "ChargeItem"; 9094 case CLAIM: return "Claim"; 9095 case CLAIMRESPONSE: return "ClaimResponse"; 9096 case CLINICALIMPRESSION: return "ClinicalImpression"; 9097 case CODESYSTEM: return "CodeSystem"; 9098 case COMMUNICATION: return "Communication"; 9099 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 9100 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 9101 case COMPOSITION: return "Composition"; 9102 case CONCEPTMAP: return "ConceptMap"; 9103 case CONDITION: return "Condition"; 9104 case CONSENT: return "Consent"; 9105 case CONTRACT: return "Contract"; 9106 case COVERAGE: return "Coverage"; 9107 case DATAELEMENT: return "DataElement"; 9108 case DETECTEDISSUE: return "DetectedIssue"; 9109 case DEVICE: return "Device"; 9110 case DEVICECOMPONENT: return "DeviceComponent"; 9111 case DEVICEMETRIC: return "DeviceMetric"; 9112 case DEVICEREQUEST: return "DeviceRequest"; 9113 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 9114 case DIAGNOSTICREPORT: return "DiagnosticReport"; 9115 case DOCUMENTMANIFEST: return "DocumentManifest"; 9116 case DOCUMENTREFERENCE: return "DocumentReference"; 9117 case DOMAINRESOURCE: return "DomainResource"; 9118 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 9119 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 9120 case ENCOUNTER: return "Encounter"; 9121 case ENDPOINT: return "Endpoint"; 9122 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 9123 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 9124 case EPISODEOFCARE: return "EpisodeOfCare"; 9125 case EXPANSIONPROFILE: return "ExpansionProfile"; 9126 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 9127 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 9128 case FLAG: return "Flag"; 9129 case GOAL: return "Goal"; 9130 case GRAPHDEFINITION: return "GraphDefinition"; 9131 case GROUP: return "Group"; 9132 case GUIDANCERESPONSE: return "GuidanceResponse"; 9133 case HEALTHCARESERVICE: return "HealthcareService"; 9134 case IMAGINGMANIFEST: return "ImagingManifest"; 9135 case IMAGINGSTUDY: return "ImagingStudy"; 9136 case IMMUNIZATION: return "Immunization"; 9137 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 9138 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 9139 case LIBRARY: return "Library"; 9140 case LINKAGE: return "Linkage"; 9141 case LIST: return "List"; 9142 case LOCATION: return "Location"; 9143 case MEASURE: return "Measure"; 9144 case MEASUREREPORT: return "MeasureReport"; 9145 case MEDIA: return "Media"; 9146 case MEDICATION: return "Medication"; 9147 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 9148 case MEDICATIONDISPENSE: return "MedicationDispense"; 9149 case MEDICATIONREQUEST: return "MedicationRequest"; 9150 case MEDICATIONSTATEMENT: return "MedicationStatement"; 9151 case MESSAGEDEFINITION: return "MessageDefinition"; 9152 case MESSAGEHEADER: return "MessageHeader"; 9153 case NAMINGSYSTEM: return "NamingSystem"; 9154 case NUTRITIONORDER: return "NutritionOrder"; 9155 case OBSERVATION: return "Observation"; 9156 case OPERATIONDEFINITION: return "OperationDefinition"; 9157 case OPERATIONOUTCOME: return "OperationOutcome"; 9158 case ORGANIZATION: return "Organization"; 9159 case PARAMETERS: return "Parameters"; 9160 case PATIENT: return "Patient"; 9161 case PAYMENTNOTICE: return "PaymentNotice"; 9162 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 9163 case PERSON: return "Person"; 9164 case PLANDEFINITION: return "PlanDefinition"; 9165 case PRACTITIONER: return "Practitioner"; 9166 case PRACTITIONERROLE: return "PractitionerRole"; 9167 case PROCEDURE: return "Procedure"; 9168 case PROCEDUREREQUEST: return "ProcedureRequest"; 9169 case PROCESSREQUEST: return "ProcessRequest"; 9170 case PROCESSRESPONSE: return "ProcessResponse"; 9171 case PROVENANCE: return "Provenance"; 9172 case QUESTIONNAIRE: return "Questionnaire"; 9173 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 9174 case REFERRALREQUEST: return "ReferralRequest"; 9175 case RELATEDPERSON: return "RelatedPerson"; 9176 case REQUESTGROUP: return "RequestGroup"; 9177 case RESEARCHSTUDY: return "ResearchStudy"; 9178 case RESEARCHSUBJECT: return "ResearchSubject"; 9179 case RESOURCE: return "Resource"; 9180 case RISKASSESSMENT: return "RiskAssessment"; 9181 case SCHEDULE: return "Schedule"; 9182 case SEARCHPARAMETER: return "SearchParameter"; 9183 case SEQUENCE: return "Sequence"; 9184 case SERVICEDEFINITION: return "ServiceDefinition"; 9185 case SLOT: return "Slot"; 9186 case SPECIMEN: return "Specimen"; 9187 case STRUCTUREDEFINITION: return "StructureDefinition"; 9188 case STRUCTUREMAP: return "StructureMap"; 9189 case SUBSCRIPTION: return "Subscription"; 9190 case SUBSTANCE: return "Substance"; 9191 case SUPPLYDELIVERY: return "SupplyDelivery"; 9192 case SUPPLYREQUEST: return "SupplyRequest"; 9193 case TASK: return "Task"; 9194 case TESTREPORT: return "TestReport"; 9195 case TESTSCRIPT: return "TestScript"; 9196 case VALUESET: return "ValueSet"; 9197 case VISIONPRESCRIPTION: return "VisionPrescription"; 9198 case NULL: return null; 9199 default: return "?"; 9200 } 9201 } 9202 public String getSystem() { 9203 switch (this) { 9204 case ACCOUNT: return "http://hl7.org/fhir/resource-types"; 9205 case ACTIVITYDEFINITION: return "http://hl7.org/fhir/resource-types"; 9206 case ADVERSEEVENT: return "http://hl7.org/fhir/resource-types"; 9207 case ALLERGYINTOLERANCE: return "http://hl7.org/fhir/resource-types"; 9208 case APPOINTMENT: return "http://hl7.org/fhir/resource-types"; 9209 case APPOINTMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 9210 case AUDITEVENT: return "http://hl7.org/fhir/resource-types"; 9211 case BASIC: return "http://hl7.org/fhir/resource-types"; 9212 case BINARY: return "http://hl7.org/fhir/resource-types"; 9213 case BODYSITE: return "http://hl7.org/fhir/resource-types"; 9214 case BUNDLE: return "http://hl7.org/fhir/resource-types"; 9215 case CAPABILITYSTATEMENT: return "http://hl7.org/fhir/resource-types"; 9216 case CAREPLAN: return "http://hl7.org/fhir/resource-types"; 9217 case CARETEAM: return "http://hl7.org/fhir/resource-types"; 9218 case CHARGEITEM: return "http://hl7.org/fhir/resource-types"; 9219 case CLAIM: return "http://hl7.org/fhir/resource-types"; 9220 case CLAIMRESPONSE: return "http://hl7.org/fhir/resource-types"; 9221 case CLINICALIMPRESSION: return "http://hl7.org/fhir/resource-types"; 9222 case CODESYSTEM: return "http://hl7.org/fhir/resource-types"; 9223 case COMMUNICATION: return "http://hl7.org/fhir/resource-types"; 9224 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 9225 case COMPARTMENTDEFINITION: return "http://hl7.org/fhir/resource-types"; 9226 case COMPOSITION: return "http://hl7.org/fhir/resource-types"; 9227 case CONCEPTMAP: return "http://hl7.org/fhir/resource-types"; 9228 case CONDITION: return "http://hl7.org/fhir/resource-types"; 9229 case CONSENT: return "http://hl7.org/fhir/resource-types"; 9230 case CONTRACT: return "http://hl7.org/fhir/resource-types"; 9231 case COVERAGE: return "http://hl7.org/fhir/resource-types"; 9232 case DATAELEMENT: return "http://hl7.org/fhir/resource-types"; 9233 case DETECTEDISSUE: return "http://hl7.org/fhir/resource-types"; 9234 case DEVICE: return "http://hl7.org/fhir/resource-types"; 9235 case DEVICECOMPONENT: return "http://hl7.org/fhir/resource-types"; 9236 case DEVICEMETRIC: return "http://hl7.org/fhir/resource-types"; 9237 case DEVICEREQUEST: return "http://hl7.org/fhir/resource-types"; 9238 case DEVICEUSESTATEMENT: return "http://hl7.org/fhir/resource-types"; 9239 case DIAGNOSTICREPORT: return "http://hl7.org/fhir/resource-types"; 9240 case DOCUMENTMANIFEST: return "http://hl7.org/fhir/resource-types"; 9241 case DOCUMENTREFERENCE: return "http://hl7.org/fhir/resource-types"; 9242 case DOMAINRESOURCE: return "http://hl7.org/fhir/resource-types"; 9243 case ELIGIBILITYREQUEST: return "http://hl7.org/fhir/resource-types"; 9244 case ELIGIBILITYRESPONSE: return "http://hl7.org/fhir/resource-types"; 9245 case ENCOUNTER: return "http://hl7.org/fhir/resource-types"; 9246 case ENDPOINT: return "http://hl7.org/fhir/resource-types"; 9247 case ENROLLMENTREQUEST: return "http://hl7.org/fhir/resource-types"; 9248 case ENROLLMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 9249 case EPISODEOFCARE: return "http://hl7.org/fhir/resource-types"; 9250 case EXPANSIONPROFILE: return "http://hl7.org/fhir/resource-types"; 9251 case EXPLANATIONOFBENEFIT: return "http://hl7.org/fhir/resource-types"; 9252 case FAMILYMEMBERHISTORY: return "http://hl7.org/fhir/resource-types"; 9253 case FLAG: return "http://hl7.org/fhir/resource-types"; 9254 case GOAL: return "http://hl7.org/fhir/resource-types"; 9255 case GRAPHDEFINITION: return "http://hl7.org/fhir/resource-types"; 9256 case GROUP: return "http://hl7.org/fhir/resource-types"; 9257 case GUIDANCERESPONSE: return "http://hl7.org/fhir/resource-types"; 9258 case HEALTHCARESERVICE: return "http://hl7.org/fhir/resource-types"; 9259 case IMAGINGMANIFEST: return "http://hl7.org/fhir/resource-types"; 9260 case IMAGINGSTUDY: return "http://hl7.org/fhir/resource-types"; 9261 case IMMUNIZATION: return "http://hl7.org/fhir/resource-types"; 9262 case IMMUNIZATIONRECOMMENDATION: return "http://hl7.org/fhir/resource-types"; 9263 case IMPLEMENTATIONGUIDE: return "http://hl7.org/fhir/resource-types"; 9264 case LIBRARY: return "http://hl7.org/fhir/resource-types"; 9265 case LINKAGE: return "http://hl7.org/fhir/resource-types"; 9266 case LIST: return "http://hl7.org/fhir/resource-types"; 9267 case LOCATION: return "http://hl7.org/fhir/resource-types"; 9268 case MEASURE: return "http://hl7.org/fhir/resource-types"; 9269 case MEASUREREPORT: return "http://hl7.org/fhir/resource-types"; 9270 case MEDIA: return "http://hl7.org/fhir/resource-types"; 9271 case MEDICATION: return "http://hl7.org/fhir/resource-types"; 9272 case MEDICATIONADMINISTRATION: return "http://hl7.org/fhir/resource-types"; 9273 case MEDICATIONDISPENSE: return "http://hl7.org/fhir/resource-types"; 9274 case MEDICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 9275 case MEDICATIONSTATEMENT: return "http://hl7.org/fhir/resource-types"; 9276 case MESSAGEDEFINITION: return "http://hl7.org/fhir/resource-types"; 9277 case MESSAGEHEADER: return "http://hl7.org/fhir/resource-types"; 9278 case NAMINGSYSTEM: return "http://hl7.org/fhir/resource-types"; 9279 case NUTRITIONORDER: return "http://hl7.org/fhir/resource-types"; 9280 case OBSERVATION: return "http://hl7.org/fhir/resource-types"; 9281 case OPERATIONDEFINITION: return "http://hl7.org/fhir/resource-types"; 9282 case OPERATIONOUTCOME: return "http://hl7.org/fhir/resource-types"; 9283 case ORGANIZATION: return "http://hl7.org/fhir/resource-types"; 9284 case PARAMETERS: return "http://hl7.org/fhir/resource-types"; 9285 case PATIENT: return "http://hl7.org/fhir/resource-types"; 9286 case PAYMENTNOTICE: return "http://hl7.org/fhir/resource-types"; 9287 case PAYMENTRECONCILIATION: return "http://hl7.org/fhir/resource-types"; 9288 case PERSON: return "http://hl7.org/fhir/resource-types"; 9289 case PLANDEFINITION: return "http://hl7.org/fhir/resource-types"; 9290 case PRACTITIONER: return "http://hl7.org/fhir/resource-types"; 9291 case PRACTITIONERROLE: return "http://hl7.org/fhir/resource-types"; 9292 case PROCEDURE: return "http://hl7.org/fhir/resource-types"; 9293 case PROCEDUREREQUEST: return "http://hl7.org/fhir/resource-types"; 9294 case PROCESSREQUEST: return "http://hl7.org/fhir/resource-types"; 9295 case PROCESSRESPONSE: return "http://hl7.org/fhir/resource-types"; 9296 case PROVENANCE: return "http://hl7.org/fhir/resource-types"; 9297 case QUESTIONNAIRE: return "http://hl7.org/fhir/resource-types"; 9298 case QUESTIONNAIRERESPONSE: return "http://hl7.org/fhir/resource-types"; 9299 case REFERRALREQUEST: return "http://hl7.org/fhir/resource-types"; 9300 case RELATEDPERSON: return "http://hl7.org/fhir/resource-types"; 9301 case REQUESTGROUP: return "http://hl7.org/fhir/resource-types"; 9302 case RESEARCHSTUDY: return "http://hl7.org/fhir/resource-types"; 9303 case RESEARCHSUBJECT: return "http://hl7.org/fhir/resource-types"; 9304 case RESOURCE: return "http://hl7.org/fhir/resource-types"; 9305 case RISKASSESSMENT: return "http://hl7.org/fhir/resource-types"; 9306 case SCHEDULE: return "http://hl7.org/fhir/resource-types"; 9307 case SEARCHPARAMETER: return "http://hl7.org/fhir/resource-types"; 9308 case SEQUENCE: return "http://hl7.org/fhir/resource-types"; 9309 case SERVICEDEFINITION: return "http://hl7.org/fhir/resource-types"; 9310 case SLOT: return "http://hl7.org/fhir/resource-types"; 9311 case SPECIMEN: return "http://hl7.org/fhir/resource-types"; 9312 case STRUCTUREDEFINITION: return "http://hl7.org/fhir/resource-types"; 9313 case STRUCTUREMAP: return "http://hl7.org/fhir/resource-types"; 9314 case SUBSCRIPTION: return "http://hl7.org/fhir/resource-types"; 9315 case SUBSTANCE: return "http://hl7.org/fhir/resource-types"; 9316 case SUPPLYDELIVERY: return "http://hl7.org/fhir/resource-types"; 9317 case SUPPLYREQUEST: return "http://hl7.org/fhir/resource-types"; 9318 case TASK: return "http://hl7.org/fhir/resource-types"; 9319 case TESTREPORT: return "http://hl7.org/fhir/resource-types"; 9320 case TESTSCRIPT: return "http://hl7.org/fhir/resource-types"; 9321 case VALUESET: return "http://hl7.org/fhir/resource-types"; 9322 case VISIONPRESCRIPTION: return "http://hl7.org/fhir/resource-types"; 9323 case NULL: return null; 9324 default: return "?"; 9325 } 9326 } 9327 public String getDefinition() { 9328 switch (this) { 9329 case ACCOUNT: return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 9330 case ACTIVITYDEFINITION: return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 9331 case ADVERSEEVENT: return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 9332 case ALLERGYINTOLERANCE: return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 9333 case APPOINTMENT: return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 9334 case APPOINTMENTRESPONSE: return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 9335 case AUDITEVENT: return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 9336 case BASIC: return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 9337 case BINARY: return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 9338 case BODYSITE: return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 9339 case BUNDLE: return "A container for a collection of resources."; 9340 case CAPABILITYSTATEMENT: return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 9341 case CAREPLAN: return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 9342 case CARETEAM: return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 9343 case CHARGEITEM: return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 9344 case CLAIM: return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 9345 case CLAIMRESPONSE: return "This resource provides the adjudication details from the processing of a Claim resource."; 9346 case CLINICALIMPRESSION: return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 9347 case CODESYSTEM: return "A code system resource specifies a set of codes drawn from one or more code systems."; 9348 case COMMUNICATION: return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 9349 case COMMUNICATIONREQUEST: return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 9350 case COMPARTMENTDEFINITION: return "A compartment definition that defines how resources are accessed on a server."; 9351 case COMPOSITION: return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 9352 case CONCEPTMAP: return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 9353 case CONDITION: return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 9354 case CONSENT: return "A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 9355 case CONTRACT: return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 9356 case COVERAGE: return "Financial instrument which may be used to reimburse or pay for health care products and services."; 9357 case DATAELEMENT: return "The formal description of a single piece of information that can be gathered and reported."; 9358 case DETECTEDISSUE: return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 9359 case DEVICE: return "This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 9360 case DEVICECOMPONENT: return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 9361 case DEVICEMETRIC: return "Describes a measurement, calculation or setting capability of a medical device."; 9362 case DEVICEREQUEST: return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 9363 case DEVICEUSESTATEMENT: return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 9364 case DIAGNOSTICREPORT: return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 9365 case DOCUMENTMANIFEST: return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 9366 case DOCUMENTREFERENCE: return "A reference to a document."; 9367 case DOMAINRESOURCE: return "A resource that includes narrative, extensions, and contained resources."; 9368 case ELIGIBILITYREQUEST: return "The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 9369 case ELIGIBILITYRESPONSE: return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 9370 case ENCOUNTER: return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 9371 case ENDPOINT: return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 9372 case ENROLLMENTREQUEST: return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 9373 case ENROLLMENTRESPONSE: return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 9374 case EPISODEOFCARE: return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 9375 case EXPANSIONPROFILE: return "Resource to define constraints on the Expansion of a FHIR ValueSet."; 9376 case EXPLANATIONOFBENEFIT: return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 9377 case FAMILYMEMBERHISTORY: return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 9378 case FLAG: return "Prospective warnings of potential issues when providing care to the patient."; 9379 case GOAL: return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 9380 case GRAPHDEFINITION: return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 9381 case GROUP: return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 9382 case GUIDANCERESPONSE: return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 9383 case HEALTHCARESERVICE: return "The details of a healthcare service available at a location."; 9384 case IMAGINGMANIFEST: return "A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection."; 9385 case IMAGINGSTUDY: return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 9386 case IMMUNIZATION: return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 9387 case IMMUNIZATIONRECOMMENDATION: return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 9388 case IMPLEMENTATIONGUIDE: return "A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 9389 case LIBRARY: return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 9390 case LINKAGE: return "Identifies two or more records (resource instances) that are referring to the same real-world \"occurrence\"."; 9391 case LIST: return "A set of information summarized from a list of other resources."; 9392 case LOCATION: return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 9393 case MEASURE: return "The Measure resource provides the definition of a quality measure."; 9394 case MEASUREREPORT: return "The MeasureReport resource contains the results of evaluating a measure."; 9395 case MEDIA: return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 9396 case MEDICATION: return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 9397 case MEDICATIONADMINISTRATION: return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 9398 case MEDICATIONDISPENSE: return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 9399 case MEDICATIONREQUEST: return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 9400 case MEDICATIONSTATEMENT: return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 9401 case MESSAGEDEFINITION: return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 9402 case MESSAGEHEADER: return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 9403 case NAMINGSYSTEM: return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 9404 case NUTRITIONORDER: return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 9405 case OBSERVATION: return "Measurements and simple assertions made about a patient, device or other subject."; 9406 case OPERATIONDEFINITION: return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 9407 case OPERATIONOUTCOME: return "A collection of error, warning or information messages that result from a system action."; 9408 case ORGANIZATION: return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 9409 case PARAMETERS: return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 9410 case PATIENT: return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 9411 case PAYMENTNOTICE: return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 9412 case PAYMENTRECONCILIATION: return "This resource provides payment details and claim references supporting a bulk payment."; 9413 case PERSON: return "Demographics and administrative information about a person independent of a specific health-related context."; 9414 case PLANDEFINITION: return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 9415 case PRACTITIONER: return "A person who is directly or indirectly involved in the provisioning of healthcare."; 9416 case PRACTITIONERROLE: return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 9417 case PROCEDURE: return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 9418 case PROCEDUREREQUEST: return "A record of a request for diagnostic investigations, treatments, or operations to be performed."; 9419 case PROCESSREQUEST: return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 9420 case PROCESSRESPONSE: return "This resource provides processing status, errors and notes from the processing of a resource."; 9421 case PROVENANCE: return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 9422 case QUESTIONNAIRE: return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 9423 case QUESTIONNAIRERESPONSE: return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 9424 case REFERRALREQUEST: return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 9425 case RELATEDPERSON: return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 9426 case REQUESTGROUP: return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 9427 case RESEARCHSTUDY: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 9428 case RESEARCHSUBJECT: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 9429 case RESOURCE: return "This is the base resource type for everything."; 9430 case RISKASSESSMENT: return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 9431 case SCHEDULE: return "A container for slots of time that may be available for booking appointments."; 9432 case SEARCHPARAMETER: return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 9433 case SEQUENCE: return "Raw data describing a biological sequence."; 9434 case SERVICEDEFINITION: return "The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking."; 9435 case SLOT: return "A slot of time on a schedule that may be available for booking appointments."; 9436 case SPECIMEN: return "A sample to be used for analysis."; 9437 case STRUCTUREDEFINITION: return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 9438 case STRUCTUREMAP: return "A Map of relationships between 2 structures that can be used to transform data."; 9439 case SUBSCRIPTION: return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 9440 case SUBSTANCE: return "A homogeneous material with a definite composition."; 9441 case SUPPLYDELIVERY: return "Record of delivery of what is supplied."; 9442 case SUPPLYREQUEST: return "A record of a request for a medication, substance or device used in the healthcare setting."; 9443 case TASK: return "A task to be performed."; 9444 case TESTREPORT: return "A summary of information based on the results of executing a TestScript."; 9445 case TESTSCRIPT: return "A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 9446 case VALUESET: return "A value set specifies a set of codes drawn from one or more code systems."; 9447 case VISIONPRESCRIPTION: return "An authorization for the supply of glasses and/or contact lenses to a patient."; 9448 case NULL: return null; 9449 default: return "?"; 9450 } 9451 } 9452 public String getDisplay() { 9453 switch (this) { 9454 case ACCOUNT: return "Account"; 9455 case ACTIVITYDEFINITION: return "ActivityDefinition"; 9456 case ADVERSEEVENT: return "AdverseEvent"; 9457 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 9458 case APPOINTMENT: return "Appointment"; 9459 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 9460 case AUDITEVENT: return "AuditEvent"; 9461 case BASIC: return "Basic"; 9462 case BINARY: return "Binary"; 9463 case BODYSITE: return "BodySite"; 9464 case BUNDLE: return "Bundle"; 9465 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 9466 case CAREPLAN: return "CarePlan"; 9467 case CARETEAM: return "CareTeam"; 9468 case CHARGEITEM: return "ChargeItem"; 9469 case CLAIM: return "Claim"; 9470 case CLAIMRESPONSE: return "ClaimResponse"; 9471 case CLINICALIMPRESSION: return "ClinicalImpression"; 9472 case CODESYSTEM: return "CodeSystem"; 9473 case COMMUNICATION: return "Communication"; 9474 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 9475 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 9476 case COMPOSITION: return "Composition"; 9477 case CONCEPTMAP: return "ConceptMap"; 9478 case CONDITION: return "Condition"; 9479 case CONSENT: return "Consent"; 9480 case CONTRACT: return "Contract"; 9481 case COVERAGE: return "Coverage"; 9482 case DATAELEMENT: return "DataElement"; 9483 case DETECTEDISSUE: return "DetectedIssue"; 9484 case DEVICE: return "Device"; 9485 case DEVICECOMPONENT: return "DeviceComponent"; 9486 case DEVICEMETRIC: return "DeviceMetric"; 9487 case DEVICEREQUEST: return "DeviceRequest"; 9488 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 9489 case DIAGNOSTICREPORT: return "DiagnosticReport"; 9490 case DOCUMENTMANIFEST: return "DocumentManifest"; 9491 case DOCUMENTREFERENCE: return "DocumentReference"; 9492 case DOMAINRESOURCE: return "DomainResource"; 9493 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 9494 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 9495 case ENCOUNTER: return "Encounter"; 9496 case ENDPOINT: return "Endpoint"; 9497 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 9498 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 9499 case EPISODEOFCARE: return "EpisodeOfCare"; 9500 case EXPANSIONPROFILE: return "ExpansionProfile"; 9501 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 9502 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 9503 case FLAG: return "Flag"; 9504 case GOAL: return "Goal"; 9505 case GRAPHDEFINITION: return "GraphDefinition"; 9506 case GROUP: return "Group"; 9507 case GUIDANCERESPONSE: return "GuidanceResponse"; 9508 case HEALTHCARESERVICE: return "HealthcareService"; 9509 case IMAGINGMANIFEST: return "ImagingManifest"; 9510 case IMAGINGSTUDY: return "ImagingStudy"; 9511 case IMMUNIZATION: return "Immunization"; 9512 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 9513 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 9514 case LIBRARY: return "Library"; 9515 case LINKAGE: return "Linkage"; 9516 case LIST: return "List"; 9517 case LOCATION: return "Location"; 9518 case MEASURE: return "Measure"; 9519 case MEASUREREPORT: return "MeasureReport"; 9520 case MEDIA: return "Media"; 9521 case MEDICATION: return "Medication"; 9522 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 9523 case MEDICATIONDISPENSE: return "MedicationDispense"; 9524 case MEDICATIONREQUEST: return "MedicationRequest"; 9525 case MEDICATIONSTATEMENT: return "MedicationStatement"; 9526 case MESSAGEDEFINITION: return "MessageDefinition"; 9527 case MESSAGEHEADER: return "MessageHeader"; 9528 case NAMINGSYSTEM: return "NamingSystem"; 9529 case NUTRITIONORDER: return "NutritionOrder"; 9530 case OBSERVATION: return "Observation"; 9531 case OPERATIONDEFINITION: return "OperationDefinition"; 9532 case OPERATIONOUTCOME: return "OperationOutcome"; 9533 case ORGANIZATION: return "Organization"; 9534 case PARAMETERS: return "Parameters"; 9535 case PATIENT: return "Patient"; 9536 case PAYMENTNOTICE: return "PaymentNotice"; 9537 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 9538 case PERSON: return "Person"; 9539 case PLANDEFINITION: return "PlanDefinition"; 9540 case PRACTITIONER: return "Practitioner"; 9541 case PRACTITIONERROLE: return "PractitionerRole"; 9542 case PROCEDURE: return "Procedure"; 9543 case PROCEDUREREQUEST: return "ProcedureRequest"; 9544 case PROCESSREQUEST: return "ProcessRequest"; 9545 case PROCESSRESPONSE: return "ProcessResponse"; 9546 case PROVENANCE: return "Provenance"; 9547 case QUESTIONNAIRE: return "Questionnaire"; 9548 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 9549 case REFERRALREQUEST: return "ReferralRequest"; 9550 case RELATEDPERSON: return "RelatedPerson"; 9551 case REQUESTGROUP: return "RequestGroup"; 9552 case RESEARCHSTUDY: return "ResearchStudy"; 9553 case RESEARCHSUBJECT: return "ResearchSubject"; 9554 case RESOURCE: return "Resource"; 9555 case RISKASSESSMENT: return "RiskAssessment"; 9556 case SCHEDULE: return "Schedule"; 9557 case SEARCHPARAMETER: return "SearchParameter"; 9558 case SEQUENCE: return "Sequence"; 9559 case SERVICEDEFINITION: return "ServiceDefinition"; 9560 case SLOT: return "Slot"; 9561 case SPECIMEN: return "Specimen"; 9562 case STRUCTUREDEFINITION: return "StructureDefinition"; 9563 case STRUCTUREMAP: return "StructureMap"; 9564 case SUBSCRIPTION: return "Subscription"; 9565 case SUBSTANCE: return "Substance"; 9566 case SUPPLYDELIVERY: return "SupplyDelivery"; 9567 case SUPPLYREQUEST: return "SupplyRequest"; 9568 case TASK: return "Task"; 9569 case TESTREPORT: return "TestReport"; 9570 case TESTSCRIPT: return "TestScript"; 9571 case VALUESET: return "ValueSet"; 9572 case VISIONPRESCRIPTION: return "VisionPrescription"; 9573 case NULL: return null; 9574 default: return "?"; 9575 } 9576 } 9577 } 9578 9579 public static class ResourceTypeEnumFactory implements EnumFactory<ResourceType> { 9580 public ResourceType fromCode(String codeString) throws IllegalArgumentException { 9581 if (codeString == null || "".equals(codeString)) 9582 if (codeString == null || "".equals(codeString)) 9583 return null; 9584 if ("Account".equals(codeString)) 9585 return ResourceType.ACCOUNT; 9586 if ("ActivityDefinition".equals(codeString)) 9587 return ResourceType.ACTIVITYDEFINITION; 9588 if ("AdverseEvent".equals(codeString)) 9589 return ResourceType.ADVERSEEVENT; 9590 if ("AllergyIntolerance".equals(codeString)) 9591 return ResourceType.ALLERGYINTOLERANCE; 9592 if ("Appointment".equals(codeString)) 9593 return ResourceType.APPOINTMENT; 9594 if ("AppointmentResponse".equals(codeString)) 9595 return ResourceType.APPOINTMENTRESPONSE; 9596 if ("AuditEvent".equals(codeString)) 9597 return ResourceType.AUDITEVENT; 9598 if ("Basic".equals(codeString)) 9599 return ResourceType.BASIC; 9600 if ("Binary".equals(codeString)) 9601 return ResourceType.BINARY; 9602 if ("BodySite".equals(codeString)) 9603 return ResourceType.BODYSITE; 9604 if ("Bundle".equals(codeString)) 9605 return ResourceType.BUNDLE; 9606 if ("CapabilityStatement".equals(codeString)) 9607 return ResourceType.CAPABILITYSTATEMENT; 9608 if ("CarePlan".equals(codeString)) 9609 return ResourceType.CAREPLAN; 9610 if ("CareTeam".equals(codeString)) 9611 return ResourceType.CARETEAM; 9612 if ("ChargeItem".equals(codeString)) 9613 return ResourceType.CHARGEITEM; 9614 if ("Claim".equals(codeString)) 9615 return ResourceType.CLAIM; 9616 if ("ClaimResponse".equals(codeString)) 9617 return ResourceType.CLAIMRESPONSE; 9618 if ("ClinicalImpression".equals(codeString)) 9619 return ResourceType.CLINICALIMPRESSION; 9620 if ("CodeSystem".equals(codeString)) 9621 return ResourceType.CODESYSTEM; 9622 if ("Communication".equals(codeString)) 9623 return ResourceType.COMMUNICATION; 9624 if ("CommunicationRequest".equals(codeString)) 9625 return ResourceType.COMMUNICATIONREQUEST; 9626 if ("CompartmentDefinition".equals(codeString)) 9627 return ResourceType.COMPARTMENTDEFINITION; 9628 if ("Composition".equals(codeString)) 9629 return ResourceType.COMPOSITION; 9630 if ("ConceptMap".equals(codeString)) 9631 return ResourceType.CONCEPTMAP; 9632 if ("Condition".equals(codeString)) 9633 return ResourceType.CONDITION; 9634 if ("Consent".equals(codeString)) 9635 return ResourceType.CONSENT; 9636 if ("Contract".equals(codeString)) 9637 return ResourceType.CONTRACT; 9638 if ("Coverage".equals(codeString)) 9639 return ResourceType.COVERAGE; 9640 if ("DataElement".equals(codeString)) 9641 return ResourceType.DATAELEMENT; 9642 if ("DetectedIssue".equals(codeString)) 9643 return ResourceType.DETECTEDISSUE; 9644 if ("Device".equals(codeString)) 9645 return ResourceType.DEVICE; 9646 if ("DeviceComponent".equals(codeString)) 9647 return ResourceType.DEVICECOMPONENT; 9648 if ("DeviceMetric".equals(codeString)) 9649 return ResourceType.DEVICEMETRIC; 9650 if ("DeviceRequest".equals(codeString)) 9651 return ResourceType.DEVICEREQUEST; 9652 if ("DeviceUseStatement".equals(codeString)) 9653 return ResourceType.DEVICEUSESTATEMENT; 9654 if ("DiagnosticReport".equals(codeString)) 9655 return ResourceType.DIAGNOSTICREPORT; 9656 if ("DocumentManifest".equals(codeString)) 9657 return ResourceType.DOCUMENTMANIFEST; 9658 if ("DocumentReference".equals(codeString)) 9659 return ResourceType.DOCUMENTREFERENCE; 9660 if ("DomainResource".equals(codeString)) 9661 return ResourceType.DOMAINRESOURCE; 9662 if ("EligibilityRequest".equals(codeString)) 9663 return ResourceType.ELIGIBILITYREQUEST; 9664 if ("EligibilityResponse".equals(codeString)) 9665 return ResourceType.ELIGIBILITYRESPONSE; 9666 if ("Encounter".equals(codeString)) 9667 return ResourceType.ENCOUNTER; 9668 if ("Endpoint".equals(codeString)) 9669 return ResourceType.ENDPOINT; 9670 if ("EnrollmentRequest".equals(codeString)) 9671 return ResourceType.ENROLLMENTREQUEST; 9672 if ("EnrollmentResponse".equals(codeString)) 9673 return ResourceType.ENROLLMENTRESPONSE; 9674 if ("EpisodeOfCare".equals(codeString)) 9675 return ResourceType.EPISODEOFCARE; 9676 if ("ExpansionProfile".equals(codeString)) 9677 return ResourceType.EXPANSIONPROFILE; 9678 if ("ExplanationOfBenefit".equals(codeString)) 9679 return ResourceType.EXPLANATIONOFBENEFIT; 9680 if ("FamilyMemberHistory".equals(codeString)) 9681 return ResourceType.FAMILYMEMBERHISTORY; 9682 if ("Flag".equals(codeString)) 9683 return ResourceType.FLAG; 9684 if ("Goal".equals(codeString)) 9685 return ResourceType.GOAL; 9686 if ("GraphDefinition".equals(codeString)) 9687 return ResourceType.GRAPHDEFINITION; 9688 if ("Group".equals(codeString)) 9689 return ResourceType.GROUP; 9690 if ("GuidanceResponse".equals(codeString)) 9691 return ResourceType.GUIDANCERESPONSE; 9692 if ("HealthcareService".equals(codeString)) 9693 return ResourceType.HEALTHCARESERVICE; 9694 if ("ImagingManifest".equals(codeString)) 9695 return ResourceType.IMAGINGMANIFEST; 9696 if ("ImagingStudy".equals(codeString)) 9697 return ResourceType.IMAGINGSTUDY; 9698 if ("Immunization".equals(codeString)) 9699 return ResourceType.IMMUNIZATION; 9700 if ("ImmunizationRecommendation".equals(codeString)) 9701 return ResourceType.IMMUNIZATIONRECOMMENDATION; 9702 if ("ImplementationGuide".equals(codeString)) 9703 return ResourceType.IMPLEMENTATIONGUIDE; 9704 if ("Library".equals(codeString)) 9705 return ResourceType.LIBRARY; 9706 if ("Linkage".equals(codeString)) 9707 return ResourceType.LINKAGE; 9708 if ("List".equals(codeString)) 9709 return ResourceType.LIST; 9710 if ("Location".equals(codeString)) 9711 return ResourceType.LOCATION; 9712 if ("Measure".equals(codeString)) 9713 return ResourceType.MEASURE; 9714 if ("MeasureReport".equals(codeString)) 9715 return ResourceType.MEASUREREPORT; 9716 if ("Media".equals(codeString)) 9717 return ResourceType.MEDIA; 9718 if ("Medication".equals(codeString)) 9719 return ResourceType.MEDICATION; 9720 if ("MedicationAdministration".equals(codeString)) 9721 return ResourceType.MEDICATIONADMINISTRATION; 9722 if ("MedicationDispense".equals(codeString)) 9723 return ResourceType.MEDICATIONDISPENSE; 9724 if ("MedicationRequest".equals(codeString)) 9725 return ResourceType.MEDICATIONREQUEST; 9726 if ("MedicationStatement".equals(codeString)) 9727 return ResourceType.MEDICATIONSTATEMENT; 9728 if ("MessageDefinition".equals(codeString)) 9729 return ResourceType.MESSAGEDEFINITION; 9730 if ("MessageHeader".equals(codeString)) 9731 return ResourceType.MESSAGEHEADER; 9732 if ("NamingSystem".equals(codeString)) 9733 return ResourceType.NAMINGSYSTEM; 9734 if ("NutritionOrder".equals(codeString)) 9735 return ResourceType.NUTRITIONORDER; 9736 if ("Observation".equals(codeString)) 9737 return ResourceType.OBSERVATION; 9738 if ("OperationDefinition".equals(codeString)) 9739 return ResourceType.OPERATIONDEFINITION; 9740 if ("OperationOutcome".equals(codeString)) 9741 return ResourceType.OPERATIONOUTCOME; 9742 if ("Organization".equals(codeString)) 9743 return ResourceType.ORGANIZATION; 9744 if ("Parameters".equals(codeString)) 9745 return ResourceType.PARAMETERS; 9746 if ("Patient".equals(codeString)) 9747 return ResourceType.PATIENT; 9748 if ("PaymentNotice".equals(codeString)) 9749 return ResourceType.PAYMENTNOTICE; 9750 if ("PaymentReconciliation".equals(codeString)) 9751 return ResourceType.PAYMENTRECONCILIATION; 9752 if ("Person".equals(codeString)) 9753 return ResourceType.PERSON; 9754 if ("PlanDefinition".equals(codeString)) 9755 return ResourceType.PLANDEFINITION; 9756 if ("Practitioner".equals(codeString)) 9757 return ResourceType.PRACTITIONER; 9758 if ("PractitionerRole".equals(codeString)) 9759 return ResourceType.PRACTITIONERROLE; 9760 if ("Procedure".equals(codeString)) 9761 return ResourceType.PROCEDURE; 9762 if ("ProcedureRequest".equals(codeString)) 9763 return ResourceType.PROCEDUREREQUEST; 9764 if ("ProcessRequest".equals(codeString)) 9765 return ResourceType.PROCESSREQUEST; 9766 if ("ProcessResponse".equals(codeString)) 9767 return ResourceType.PROCESSRESPONSE; 9768 if ("Provenance".equals(codeString)) 9769 return ResourceType.PROVENANCE; 9770 if ("Questionnaire".equals(codeString)) 9771 return ResourceType.QUESTIONNAIRE; 9772 if ("QuestionnaireResponse".equals(codeString)) 9773 return ResourceType.QUESTIONNAIRERESPONSE; 9774 if ("ReferralRequest".equals(codeString)) 9775 return ResourceType.REFERRALREQUEST; 9776 if ("RelatedPerson".equals(codeString)) 9777 return ResourceType.RELATEDPERSON; 9778 if ("RequestGroup".equals(codeString)) 9779 return ResourceType.REQUESTGROUP; 9780 if ("ResearchStudy".equals(codeString)) 9781 return ResourceType.RESEARCHSTUDY; 9782 if ("ResearchSubject".equals(codeString)) 9783 return ResourceType.RESEARCHSUBJECT; 9784 if ("Resource".equals(codeString)) 9785 return ResourceType.RESOURCE; 9786 if ("RiskAssessment".equals(codeString)) 9787 return ResourceType.RISKASSESSMENT; 9788 if ("Schedule".equals(codeString)) 9789 return ResourceType.SCHEDULE; 9790 if ("SearchParameter".equals(codeString)) 9791 return ResourceType.SEARCHPARAMETER; 9792 if ("Sequence".equals(codeString)) 9793 return ResourceType.SEQUENCE; 9794 if ("ServiceDefinition".equals(codeString)) 9795 return ResourceType.SERVICEDEFINITION; 9796 if ("Slot".equals(codeString)) 9797 return ResourceType.SLOT; 9798 if ("Specimen".equals(codeString)) 9799 return ResourceType.SPECIMEN; 9800 if ("StructureDefinition".equals(codeString)) 9801 return ResourceType.STRUCTUREDEFINITION; 9802 if ("StructureMap".equals(codeString)) 9803 return ResourceType.STRUCTUREMAP; 9804 if ("Subscription".equals(codeString)) 9805 return ResourceType.SUBSCRIPTION; 9806 if ("Substance".equals(codeString)) 9807 return ResourceType.SUBSTANCE; 9808 if ("SupplyDelivery".equals(codeString)) 9809 return ResourceType.SUPPLYDELIVERY; 9810 if ("SupplyRequest".equals(codeString)) 9811 return ResourceType.SUPPLYREQUEST; 9812 if ("Task".equals(codeString)) 9813 return ResourceType.TASK; 9814 if ("TestReport".equals(codeString)) 9815 return ResourceType.TESTREPORT; 9816 if ("TestScript".equals(codeString)) 9817 return ResourceType.TESTSCRIPT; 9818 if ("ValueSet".equals(codeString)) 9819 return ResourceType.VALUESET; 9820 if ("VisionPrescription".equals(codeString)) 9821 return ResourceType.VISIONPRESCRIPTION; 9822 throw new IllegalArgumentException("Unknown ResourceType code '"+codeString+"'"); 9823 } 9824 public Enumeration<ResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 9825 if (code == null) 9826 return null; 9827 if (code.isEmpty()) 9828 return new Enumeration<ResourceType>(this); 9829 String codeString = code.asStringValue(); 9830 if (codeString == null || "".equals(codeString)) 9831 return null; 9832 if ("Account".equals(codeString)) 9833 return new Enumeration<ResourceType>(this, ResourceType.ACCOUNT); 9834 if ("ActivityDefinition".equals(codeString)) 9835 return new Enumeration<ResourceType>(this, ResourceType.ACTIVITYDEFINITION); 9836 if ("AdverseEvent".equals(codeString)) 9837 return new Enumeration<ResourceType>(this, ResourceType.ADVERSEEVENT); 9838 if ("AllergyIntolerance".equals(codeString)) 9839 return new Enumeration<ResourceType>(this, ResourceType.ALLERGYINTOLERANCE); 9840 if ("Appointment".equals(codeString)) 9841 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENT); 9842 if ("AppointmentResponse".equals(codeString)) 9843 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENTRESPONSE); 9844 if ("AuditEvent".equals(codeString)) 9845 return new Enumeration<ResourceType>(this, ResourceType.AUDITEVENT); 9846 if ("Basic".equals(codeString)) 9847 return new Enumeration<ResourceType>(this, ResourceType.BASIC); 9848 if ("Binary".equals(codeString)) 9849 return new Enumeration<ResourceType>(this, ResourceType.BINARY); 9850 if ("BodySite".equals(codeString)) 9851 return new Enumeration<ResourceType>(this, ResourceType.BODYSITE); 9852 if ("Bundle".equals(codeString)) 9853 return new Enumeration<ResourceType>(this, ResourceType.BUNDLE); 9854 if ("CapabilityStatement".equals(codeString)) 9855 return new Enumeration<ResourceType>(this, ResourceType.CAPABILITYSTATEMENT); 9856 if ("CarePlan".equals(codeString)) 9857 return new Enumeration<ResourceType>(this, ResourceType.CAREPLAN); 9858 if ("CareTeam".equals(codeString)) 9859 return new Enumeration<ResourceType>(this, ResourceType.CARETEAM); 9860 if ("ChargeItem".equals(codeString)) 9861 return new Enumeration<ResourceType>(this, ResourceType.CHARGEITEM); 9862 if ("Claim".equals(codeString)) 9863 return new Enumeration<ResourceType>(this, ResourceType.CLAIM); 9864 if ("ClaimResponse".equals(codeString)) 9865 return new Enumeration<ResourceType>(this, ResourceType.CLAIMRESPONSE); 9866 if ("ClinicalImpression".equals(codeString)) 9867 return new Enumeration<ResourceType>(this, ResourceType.CLINICALIMPRESSION); 9868 if ("CodeSystem".equals(codeString)) 9869 return new Enumeration<ResourceType>(this, ResourceType.CODESYSTEM); 9870 if ("Communication".equals(codeString)) 9871 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATION); 9872 if ("CommunicationRequest".equals(codeString)) 9873 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATIONREQUEST); 9874 if ("CompartmentDefinition".equals(codeString)) 9875 return new Enumeration<ResourceType>(this, ResourceType.COMPARTMENTDEFINITION); 9876 if ("Composition".equals(codeString)) 9877 return new Enumeration<ResourceType>(this, ResourceType.COMPOSITION); 9878 if ("ConceptMap".equals(codeString)) 9879 return new Enumeration<ResourceType>(this, ResourceType.CONCEPTMAP); 9880 if ("Condition".equals(codeString)) 9881 return new Enumeration<ResourceType>(this, ResourceType.CONDITION); 9882 if ("Consent".equals(codeString)) 9883 return new Enumeration<ResourceType>(this, ResourceType.CONSENT); 9884 if ("Contract".equals(codeString)) 9885 return new Enumeration<ResourceType>(this, ResourceType.CONTRACT); 9886 if ("Coverage".equals(codeString)) 9887 return new Enumeration<ResourceType>(this, ResourceType.COVERAGE); 9888 if ("DataElement".equals(codeString)) 9889 return new Enumeration<ResourceType>(this, ResourceType.DATAELEMENT); 9890 if ("DetectedIssue".equals(codeString)) 9891 return new Enumeration<ResourceType>(this, ResourceType.DETECTEDISSUE); 9892 if ("Device".equals(codeString)) 9893 return new Enumeration<ResourceType>(this, ResourceType.DEVICE); 9894 if ("DeviceComponent".equals(codeString)) 9895 return new Enumeration<ResourceType>(this, ResourceType.DEVICECOMPONENT); 9896 if ("DeviceMetric".equals(codeString)) 9897 return new Enumeration<ResourceType>(this, ResourceType.DEVICEMETRIC); 9898 if ("DeviceRequest".equals(codeString)) 9899 return new Enumeration<ResourceType>(this, ResourceType.DEVICEREQUEST); 9900 if ("DeviceUseStatement".equals(codeString)) 9901 return new Enumeration<ResourceType>(this, ResourceType.DEVICEUSESTATEMENT); 9902 if ("DiagnosticReport".equals(codeString)) 9903 return new Enumeration<ResourceType>(this, ResourceType.DIAGNOSTICREPORT); 9904 if ("DocumentManifest".equals(codeString)) 9905 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTMANIFEST); 9906 if ("DocumentReference".equals(codeString)) 9907 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTREFERENCE); 9908 if ("DomainResource".equals(codeString)) 9909 return new Enumeration<ResourceType>(this, ResourceType.DOMAINRESOURCE); 9910 if ("EligibilityRequest".equals(codeString)) 9911 return new Enumeration<ResourceType>(this, ResourceType.ELIGIBILITYREQUEST); 9912 if ("EligibilityResponse".equals(codeString)) 9913 return new Enumeration<ResourceType>(this, ResourceType.ELIGIBILITYRESPONSE); 9914 if ("Encounter".equals(codeString)) 9915 return new Enumeration<ResourceType>(this, ResourceType.ENCOUNTER); 9916 if ("Endpoint".equals(codeString)) 9917 return new Enumeration<ResourceType>(this, ResourceType.ENDPOINT); 9918 if ("EnrollmentRequest".equals(codeString)) 9919 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTREQUEST); 9920 if ("EnrollmentResponse".equals(codeString)) 9921 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTRESPONSE); 9922 if ("EpisodeOfCare".equals(codeString)) 9923 return new Enumeration<ResourceType>(this, ResourceType.EPISODEOFCARE); 9924 if ("ExpansionProfile".equals(codeString)) 9925 return new Enumeration<ResourceType>(this, ResourceType.EXPANSIONPROFILE); 9926 if ("ExplanationOfBenefit".equals(codeString)) 9927 return new Enumeration<ResourceType>(this, ResourceType.EXPLANATIONOFBENEFIT); 9928 if ("FamilyMemberHistory".equals(codeString)) 9929 return new Enumeration<ResourceType>(this, ResourceType.FAMILYMEMBERHISTORY); 9930 if ("Flag".equals(codeString)) 9931 return new Enumeration<ResourceType>(this, ResourceType.FLAG); 9932 if ("Goal".equals(codeString)) 9933 return new Enumeration<ResourceType>(this, ResourceType.GOAL); 9934 if ("GraphDefinition".equals(codeString)) 9935 return new Enumeration<ResourceType>(this, ResourceType.GRAPHDEFINITION); 9936 if ("Group".equals(codeString)) 9937 return new Enumeration<ResourceType>(this, ResourceType.GROUP); 9938 if ("GuidanceResponse".equals(codeString)) 9939 return new Enumeration<ResourceType>(this, ResourceType.GUIDANCERESPONSE); 9940 if ("HealthcareService".equals(codeString)) 9941 return new Enumeration<ResourceType>(this, ResourceType.HEALTHCARESERVICE); 9942 if ("ImagingManifest".equals(codeString)) 9943 return new Enumeration<ResourceType>(this, ResourceType.IMAGINGMANIFEST); 9944 if ("ImagingStudy".equals(codeString)) 9945 return new Enumeration<ResourceType>(this, ResourceType.IMAGINGSTUDY); 9946 if ("Immunization".equals(codeString)) 9947 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATION); 9948 if ("ImmunizationRecommendation".equals(codeString)) 9949 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATIONRECOMMENDATION); 9950 if ("ImplementationGuide".equals(codeString)) 9951 return new Enumeration<ResourceType>(this, ResourceType.IMPLEMENTATIONGUIDE); 9952 if ("Library".equals(codeString)) 9953 return new Enumeration<ResourceType>(this, ResourceType.LIBRARY); 9954 if ("Linkage".equals(codeString)) 9955 return new Enumeration<ResourceType>(this, ResourceType.LINKAGE); 9956 if ("List".equals(codeString)) 9957 return new Enumeration<ResourceType>(this, ResourceType.LIST); 9958 if ("Location".equals(codeString)) 9959 return new Enumeration<ResourceType>(this, ResourceType.LOCATION); 9960 if ("Measure".equals(codeString)) 9961 return new Enumeration<ResourceType>(this, ResourceType.MEASURE); 9962 if ("MeasureReport".equals(codeString)) 9963 return new Enumeration<ResourceType>(this, ResourceType.MEASUREREPORT); 9964 if ("Media".equals(codeString)) 9965 return new Enumeration<ResourceType>(this, ResourceType.MEDIA); 9966 if ("Medication".equals(codeString)) 9967 return new Enumeration<ResourceType>(this, ResourceType.MEDICATION); 9968 if ("MedicationAdministration".equals(codeString)) 9969 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONADMINISTRATION); 9970 if ("MedicationDispense".equals(codeString)) 9971 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONDISPENSE); 9972 if ("MedicationRequest".equals(codeString)) 9973 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONREQUEST); 9974 if ("MedicationStatement".equals(codeString)) 9975 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONSTATEMENT); 9976 if ("MessageDefinition".equals(codeString)) 9977 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEDEFINITION); 9978 if ("MessageHeader".equals(codeString)) 9979 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEHEADER); 9980 if ("NamingSystem".equals(codeString)) 9981 return new Enumeration<ResourceType>(this, ResourceType.NAMINGSYSTEM); 9982 if ("NutritionOrder".equals(codeString)) 9983 return new Enumeration<ResourceType>(this, ResourceType.NUTRITIONORDER); 9984 if ("Observation".equals(codeString)) 9985 return new Enumeration<ResourceType>(this, ResourceType.OBSERVATION); 9986 if ("OperationDefinition".equals(codeString)) 9987 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONDEFINITION); 9988 if ("OperationOutcome".equals(codeString)) 9989 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONOUTCOME); 9990 if ("Organization".equals(codeString)) 9991 return new Enumeration<ResourceType>(this, ResourceType.ORGANIZATION); 9992 if ("Parameters".equals(codeString)) 9993 return new Enumeration<ResourceType>(this, ResourceType.PARAMETERS); 9994 if ("Patient".equals(codeString)) 9995 return new Enumeration<ResourceType>(this, ResourceType.PATIENT); 9996 if ("PaymentNotice".equals(codeString)) 9997 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTNOTICE); 9998 if ("PaymentReconciliation".equals(codeString)) 9999 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTRECONCILIATION); 10000 if ("Person".equals(codeString)) 10001 return new Enumeration<ResourceType>(this, ResourceType.PERSON); 10002 if ("PlanDefinition".equals(codeString)) 10003 return new Enumeration<ResourceType>(this, ResourceType.PLANDEFINITION); 10004 if ("Practitioner".equals(codeString)) 10005 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONER); 10006 if ("PractitionerRole".equals(codeString)) 10007 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONERROLE); 10008 if ("Procedure".equals(codeString)) 10009 return new Enumeration<ResourceType>(this, ResourceType.PROCEDURE); 10010 if ("ProcedureRequest".equals(codeString)) 10011 return new Enumeration<ResourceType>(this, ResourceType.PROCEDUREREQUEST); 10012 if ("ProcessRequest".equals(codeString)) 10013 return new Enumeration<ResourceType>(this, ResourceType.PROCESSREQUEST); 10014 if ("ProcessResponse".equals(codeString)) 10015 return new Enumeration<ResourceType>(this, ResourceType.PROCESSRESPONSE); 10016 if ("Provenance".equals(codeString)) 10017 return new Enumeration<ResourceType>(this, ResourceType.PROVENANCE); 10018 if ("Questionnaire".equals(codeString)) 10019 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRE); 10020 if ("QuestionnaireResponse".equals(codeString)) 10021 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRERESPONSE); 10022 if ("ReferralRequest".equals(codeString)) 10023 return new Enumeration<ResourceType>(this, ResourceType.REFERRALREQUEST); 10024 if ("RelatedPerson".equals(codeString)) 10025 return new Enumeration<ResourceType>(this, ResourceType.RELATEDPERSON); 10026 if ("RequestGroup".equals(codeString)) 10027 return new Enumeration<ResourceType>(this, ResourceType.REQUESTGROUP); 10028 if ("ResearchStudy".equals(codeString)) 10029 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHSTUDY); 10030 if ("ResearchSubject".equals(codeString)) 10031 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHSUBJECT); 10032 if ("Resource".equals(codeString)) 10033 return new Enumeration<ResourceType>(this, ResourceType.RESOURCE); 10034 if ("RiskAssessment".equals(codeString)) 10035 return new Enumeration<ResourceType>(this, ResourceType.RISKASSESSMENT); 10036 if ("Schedule".equals(codeString)) 10037 return new Enumeration<ResourceType>(this, ResourceType.SCHEDULE); 10038 if ("SearchParameter".equals(codeString)) 10039 return new Enumeration<ResourceType>(this, ResourceType.SEARCHPARAMETER); 10040 if ("Sequence".equals(codeString)) 10041 return new Enumeration<ResourceType>(this, ResourceType.SEQUENCE); 10042 if ("ServiceDefinition".equals(codeString)) 10043 return new Enumeration<ResourceType>(this, ResourceType.SERVICEDEFINITION); 10044 if ("Slot".equals(codeString)) 10045 return new Enumeration<ResourceType>(this, ResourceType.SLOT); 10046 if ("Specimen".equals(codeString)) 10047 return new Enumeration<ResourceType>(this, ResourceType.SPECIMEN); 10048 if ("StructureDefinition".equals(codeString)) 10049 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREDEFINITION); 10050 if ("StructureMap".equals(codeString)) 10051 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREMAP); 10052 if ("Subscription".equals(codeString)) 10053 return new Enumeration<ResourceType>(this, ResourceType.SUBSCRIPTION); 10054 if ("Substance".equals(codeString)) 10055 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCE); 10056 if ("SupplyDelivery".equals(codeString)) 10057 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYDELIVERY); 10058 if ("SupplyRequest".equals(codeString)) 10059 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYREQUEST); 10060 if ("Task".equals(codeString)) 10061 return new Enumeration<ResourceType>(this, ResourceType.TASK); 10062 if ("TestReport".equals(codeString)) 10063 return new Enumeration<ResourceType>(this, ResourceType.TESTREPORT); 10064 if ("TestScript".equals(codeString)) 10065 return new Enumeration<ResourceType>(this, ResourceType.TESTSCRIPT); 10066 if ("ValueSet".equals(codeString)) 10067 return new Enumeration<ResourceType>(this, ResourceType.VALUESET); 10068 if ("VisionPrescription".equals(codeString)) 10069 return new Enumeration<ResourceType>(this, ResourceType.VISIONPRESCRIPTION); 10070 throw new FHIRException("Unknown ResourceType code '"+codeString+"'"); 10071 } 10072 public String toCode(ResourceType code) { 10073 if (code == ResourceType.NULL) 10074 return null; 10075 if (code == ResourceType.ACCOUNT) 10076 return "Account"; 10077 if (code == ResourceType.ACTIVITYDEFINITION) 10078 return "ActivityDefinition"; 10079 if (code == ResourceType.ADVERSEEVENT) 10080 return "AdverseEvent"; 10081 if (code == ResourceType.ALLERGYINTOLERANCE) 10082 return "AllergyIntolerance"; 10083 if (code == ResourceType.APPOINTMENT) 10084 return "Appointment"; 10085 if (code == ResourceType.APPOINTMENTRESPONSE) 10086 return "AppointmentResponse"; 10087 if (code == ResourceType.AUDITEVENT) 10088 return "AuditEvent"; 10089 if (code == ResourceType.BASIC) 10090 return "Basic"; 10091 if (code == ResourceType.BINARY) 10092 return "Binary"; 10093 if (code == ResourceType.BODYSITE) 10094 return "BodySite"; 10095 if (code == ResourceType.BUNDLE) 10096 return "Bundle"; 10097 if (code == ResourceType.CAPABILITYSTATEMENT) 10098 return "CapabilityStatement"; 10099 if (code == ResourceType.CAREPLAN) 10100 return "CarePlan"; 10101 if (code == ResourceType.CARETEAM) 10102 return "CareTeam"; 10103 if (code == ResourceType.CHARGEITEM) 10104 return "ChargeItem"; 10105 if (code == ResourceType.CLAIM) 10106 return "Claim"; 10107 if (code == ResourceType.CLAIMRESPONSE) 10108 return "ClaimResponse"; 10109 if (code == ResourceType.CLINICALIMPRESSION) 10110 return "ClinicalImpression"; 10111 if (code == ResourceType.CODESYSTEM) 10112 return "CodeSystem"; 10113 if (code == ResourceType.COMMUNICATION) 10114 return "Communication"; 10115 if (code == ResourceType.COMMUNICATIONREQUEST) 10116 return "CommunicationRequest"; 10117 if (code == ResourceType.COMPARTMENTDEFINITION) 10118 return "CompartmentDefinition"; 10119 if (code == ResourceType.COMPOSITION) 10120 return "Composition"; 10121 if (code == ResourceType.CONCEPTMAP) 10122 return "ConceptMap"; 10123 if (code == ResourceType.CONDITION) 10124 return "Condition"; 10125 if (code == ResourceType.CONSENT) 10126 return "Consent"; 10127 if (code == ResourceType.CONTRACT) 10128 return "Contract"; 10129 if (code == ResourceType.COVERAGE) 10130 return "Coverage"; 10131 if (code == ResourceType.DATAELEMENT) 10132 return "DataElement"; 10133 if (code == ResourceType.DETECTEDISSUE) 10134 return "DetectedIssue"; 10135 if (code == ResourceType.DEVICE) 10136 return "Device"; 10137 if (code == ResourceType.DEVICECOMPONENT) 10138 return "DeviceComponent"; 10139 if (code == ResourceType.DEVICEMETRIC) 10140 return "DeviceMetric"; 10141 if (code == ResourceType.DEVICEREQUEST) 10142 return "DeviceRequest"; 10143 if (code == ResourceType.DEVICEUSESTATEMENT) 10144 return "DeviceUseStatement"; 10145 if (code == ResourceType.DIAGNOSTICREPORT) 10146 return "DiagnosticReport"; 10147 if (code == ResourceType.DOCUMENTMANIFEST) 10148 return "DocumentManifest"; 10149 if (code == ResourceType.DOCUMENTREFERENCE) 10150 return "DocumentReference"; 10151 if (code == ResourceType.DOMAINRESOURCE) 10152 return "DomainResource"; 10153 if (code == ResourceType.ELIGIBILITYREQUEST) 10154 return "EligibilityRequest"; 10155 if (code == ResourceType.ELIGIBILITYRESPONSE) 10156 return "EligibilityResponse"; 10157 if (code == ResourceType.ENCOUNTER) 10158 return "Encounter"; 10159 if (code == ResourceType.ENDPOINT) 10160 return "Endpoint"; 10161 if (code == ResourceType.ENROLLMENTREQUEST) 10162 return "EnrollmentRequest"; 10163 if (code == ResourceType.ENROLLMENTRESPONSE) 10164 return "EnrollmentResponse"; 10165 if (code == ResourceType.EPISODEOFCARE) 10166 return "EpisodeOfCare"; 10167 if (code == ResourceType.EXPANSIONPROFILE) 10168 return "ExpansionProfile"; 10169 if (code == ResourceType.EXPLANATIONOFBENEFIT) 10170 return "ExplanationOfBenefit"; 10171 if (code == ResourceType.FAMILYMEMBERHISTORY) 10172 return "FamilyMemberHistory"; 10173 if (code == ResourceType.FLAG) 10174 return "Flag"; 10175 if (code == ResourceType.GOAL) 10176 return "Goal"; 10177 if (code == ResourceType.GRAPHDEFINITION) 10178 return "GraphDefinition"; 10179 if (code == ResourceType.GROUP) 10180 return "Group"; 10181 if (code == ResourceType.GUIDANCERESPONSE) 10182 return "GuidanceResponse"; 10183 if (code == ResourceType.HEALTHCARESERVICE) 10184 return "HealthcareService"; 10185 if (code == ResourceType.IMAGINGMANIFEST) 10186 return "ImagingManifest"; 10187 if (code == ResourceType.IMAGINGSTUDY) 10188 return "ImagingStudy"; 10189 if (code == ResourceType.IMMUNIZATION) 10190 return "Immunization"; 10191 if (code == ResourceType.IMMUNIZATIONRECOMMENDATION) 10192 return "ImmunizationRecommendation"; 10193 if (code == ResourceType.IMPLEMENTATIONGUIDE) 10194 return "ImplementationGuide"; 10195 if (code == ResourceType.LIBRARY) 10196 return "Library"; 10197 if (code == ResourceType.LINKAGE) 10198 return "Linkage"; 10199 if (code == ResourceType.LIST) 10200 return "List"; 10201 if (code == ResourceType.LOCATION) 10202 return "Location"; 10203 if (code == ResourceType.MEASURE) 10204 return "Measure"; 10205 if (code == ResourceType.MEASUREREPORT) 10206 return "MeasureReport"; 10207 if (code == ResourceType.MEDIA) 10208 return "Media"; 10209 if (code == ResourceType.MEDICATION) 10210 return "Medication"; 10211 if (code == ResourceType.MEDICATIONADMINISTRATION) 10212 return "MedicationAdministration"; 10213 if (code == ResourceType.MEDICATIONDISPENSE) 10214 return "MedicationDispense"; 10215 if (code == ResourceType.MEDICATIONREQUEST) 10216 return "MedicationRequest"; 10217 if (code == ResourceType.MEDICATIONSTATEMENT) 10218 return "MedicationStatement"; 10219 if (code == ResourceType.MESSAGEDEFINITION) 10220 return "MessageDefinition"; 10221 if (code == ResourceType.MESSAGEHEADER) 10222 return "MessageHeader"; 10223 if (code == ResourceType.NAMINGSYSTEM) 10224 return "NamingSystem"; 10225 if (code == ResourceType.NUTRITIONORDER) 10226 return "NutritionOrder"; 10227 if (code == ResourceType.OBSERVATION) 10228 return "Observation"; 10229 if (code == ResourceType.OPERATIONDEFINITION) 10230 return "OperationDefinition"; 10231 if (code == ResourceType.OPERATIONOUTCOME) 10232 return "OperationOutcome"; 10233 if (code == ResourceType.ORGANIZATION) 10234 return "Organization"; 10235 if (code == ResourceType.PARAMETERS) 10236 return "Parameters"; 10237 if (code == ResourceType.PATIENT) 10238 return "Patient"; 10239 if (code == ResourceType.PAYMENTNOTICE) 10240 return "PaymentNotice"; 10241 if (code == ResourceType.PAYMENTRECONCILIATION) 10242 return "PaymentReconciliation"; 10243 if (code == ResourceType.PERSON) 10244 return "Person"; 10245 if (code == ResourceType.PLANDEFINITION) 10246 return "PlanDefinition"; 10247 if (code == ResourceType.PRACTITIONER) 10248 return "Practitioner"; 10249 if (code == ResourceType.PRACTITIONERROLE) 10250 return "PractitionerRole"; 10251 if (code == ResourceType.PROCEDURE) 10252 return "Procedure"; 10253 if (code == ResourceType.PROCEDUREREQUEST) 10254 return "ProcedureRequest"; 10255 if (code == ResourceType.PROCESSREQUEST) 10256 return "ProcessRequest"; 10257 if (code == ResourceType.PROCESSRESPONSE) 10258 return "ProcessResponse"; 10259 if (code == ResourceType.PROVENANCE) 10260 return "Provenance"; 10261 if (code == ResourceType.QUESTIONNAIRE) 10262 return "Questionnaire"; 10263 if (code == ResourceType.QUESTIONNAIRERESPONSE) 10264 return "QuestionnaireResponse"; 10265 if (code == ResourceType.REFERRALREQUEST) 10266 return "ReferralRequest"; 10267 if (code == ResourceType.RELATEDPERSON) 10268 return "RelatedPerson"; 10269 if (code == ResourceType.REQUESTGROUP) 10270 return "RequestGroup"; 10271 if (code == ResourceType.RESEARCHSTUDY) 10272 return "ResearchStudy"; 10273 if (code == ResourceType.RESEARCHSUBJECT) 10274 return "ResearchSubject"; 10275 if (code == ResourceType.RESOURCE) 10276 return "Resource"; 10277 if (code == ResourceType.RISKASSESSMENT) 10278 return "RiskAssessment"; 10279 if (code == ResourceType.SCHEDULE) 10280 return "Schedule"; 10281 if (code == ResourceType.SEARCHPARAMETER) 10282 return "SearchParameter"; 10283 if (code == ResourceType.SEQUENCE) 10284 return "Sequence"; 10285 if (code == ResourceType.SERVICEDEFINITION) 10286 return "ServiceDefinition"; 10287 if (code == ResourceType.SLOT) 10288 return "Slot"; 10289 if (code == ResourceType.SPECIMEN) 10290 return "Specimen"; 10291 if (code == ResourceType.STRUCTUREDEFINITION) 10292 return "StructureDefinition"; 10293 if (code == ResourceType.STRUCTUREMAP) 10294 return "StructureMap"; 10295 if (code == ResourceType.SUBSCRIPTION) 10296 return "Subscription"; 10297 if (code == ResourceType.SUBSTANCE) 10298 return "Substance"; 10299 if (code == ResourceType.SUPPLYDELIVERY) 10300 return "SupplyDelivery"; 10301 if (code == ResourceType.SUPPLYREQUEST) 10302 return "SupplyRequest"; 10303 if (code == ResourceType.TASK) 10304 return "Task"; 10305 if (code == ResourceType.TESTREPORT) 10306 return "TestReport"; 10307 if (code == ResourceType.TESTSCRIPT) 10308 return "TestScript"; 10309 if (code == ResourceType.VALUESET) 10310 return "ValueSet"; 10311 if (code == ResourceType.VISIONPRESCRIPTION) 10312 return "VisionPrescription"; 10313 return "?"; 10314 } 10315 public String toSystem(ResourceType code) { 10316 return code.getSystem(); 10317 } 10318 } 10319 10320 public enum SearchParamType { 10321 /** 10322 * Search parameter SHALL be a number (a whole number, or a decimal). 10323 */ 10324 NUMBER, 10325 /** 10326 * Search parameter is on a date/time. The date format is the standard XML format, though other formats may be supported. 10327 */ 10328 DATE, 10329 /** 10330 * Search parameter is a simple string, like a name part. Search is case-insensitive and accent-insensitive. May match just the start of a string. String parameters may contain spaces. 10331 */ 10332 STRING, 10333 /** 10334 * Search parameter on a coded element or identifier. May be used to search through the text, displayname, code and code/codesystem (for codes) and label, system and key (for identifier). Its value is either a string or a pair of namespace and value, separated by a "|", depending on the modifier used. 10335 */ 10336 TOKEN, 10337 /** 10338 * A reference to another resource. 10339 */ 10340 REFERENCE, 10341 /** 10342 * A composite search parameter that combines a search on two values together. 10343 */ 10344 COMPOSITE, 10345 /** 10346 * A search parameter that searches on a quantity. 10347 */ 10348 QUANTITY, 10349 /** 10350 * A search parameter that searches on a URI (RFC 3986). 10351 */ 10352 URI, 10353 /** 10354 * added to help the parsers 10355 */ 10356 NULL; 10357 public static SearchParamType fromCode(String codeString) throws FHIRException { 10358 if (codeString == null || "".equals(codeString)) 10359 return null; 10360 if ("number".equals(codeString)) 10361 return NUMBER; 10362 if ("date".equals(codeString)) 10363 return DATE; 10364 if ("string".equals(codeString)) 10365 return STRING; 10366 if ("token".equals(codeString)) 10367 return TOKEN; 10368 if ("reference".equals(codeString)) 10369 return REFERENCE; 10370 if ("composite".equals(codeString)) 10371 return COMPOSITE; 10372 if ("quantity".equals(codeString)) 10373 return QUANTITY; 10374 if ("uri".equals(codeString)) 10375 return URI; 10376 throw new FHIRException("Unknown SearchParamType code '"+codeString+"'"); 10377 } 10378 public String toCode() { 10379 switch (this) { 10380 case NUMBER: return "number"; 10381 case DATE: return "date"; 10382 case STRING: return "string"; 10383 case TOKEN: return "token"; 10384 case REFERENCE: return "reference"; 10385 case COMPOSITE: return "composite"; 10386 case QUANTITY: return "quantity"; 10387 case URI: return "uri"; 10388 case NULL: return null; 10389 default: return "?"; 10390 } 10391 } 10392 public String getSystem() { 10393 switch (this) { 10394 case NUMBER: return "http://hl7.org/fhir/search-param-type"; 10395 case DATE: return "http://hl7.org/fhir/search-param-type"; 10396 case STRING: return "http://hl7.org/fhir/search-param-type"; 10397 case TOKEN: return "http://hl7.org/fhir/search-param-type"; 10398 case REFERENCE: return "http://hl7.org/fhir/search-param-type"; 10399 case COMPOSITE: return "http://hl7.org/fhir/search-param-type"; 10400 case QUANTITY: return "http://hl7.org/fhir/search-param-type"; 10401 case URI: return "http://hl7.org/fhir/search-param-type"; 10402 case NULL: return null; 10403 default: return "?"; 10404 } 10405 } 10406 public String getDefinition() { 10407 switch (this) { 10408 case NUMBER: return "Search parameter SHALL be a number (a whole number, or a decimal)."; 10409 case DATE: return "Search parameter is on a date/time. The date format is the standard XML format, though other formats may be supported."; 10410 case STRING: return "Search parameter is a simple string, like a name part. Search is case-insensitive and accent-insensitive. May match just the start of a string. String parameters may contain spaces."; 10411 case TOKEN: return "Search parameter on a coded element or identifier. May be used to search through the text, displayname, code and code/codesystem (for codes) and label, system and key (for identifier). Its value is either a string or a pair of namespace and value, separated by a \"|\", depending on the modifier used."; 10412 case REFERENCE: return "A reference to another resource."; 10413 case COMPOSITE: return "A composite search parameter that combines a search on two values together."; 10414 case QUANTITY: return "A search parameter that searches on a quantity."; 10415 case URI: return "A search parameter that searches on a URI (RFC 3986)."; 10416 case NULL: return null; 10417 default: return "?"; 10418 } 10419 } 10420 public String getDisplay() { 10421 switch (this) { 10422 case NUMBER: return "Number"; 10423 case DATE: return "Date/DateTime"; 10424 case STRING: return "String"; 10425 case TOKEN: return "Token"; 10426 case REFERENCE: return "Reference"; 10427 case COMPOSITE: return "Composite"; 10428 case QUANTITY: return "Quantity"; 10429 case URI: return "URI"; 10430 case NULL: return null; 10431 default: return "?"; 10432 } 10433 } 10434 } 10435 10436 public static class SearchParamTypeEnumFactory implements EnumFactory<SearchParamType> { 10437 public SearchParamType fromCode(String codeString) throws IllegalArgumentException { 10438 if (codeString == null || "".equals(codeString)) 10439 if (codeString == null || "".equals(codeString)) 10440 return null; 10441 if ("number".equals(codeString)) 10442 return SearchParamType.NUMBER; 10443 if ("date".equals(codeString)) 10444 return SearchParamType.DATE; 10445 if ("string".equals(codeString)) 10446 return SearchParamType.STRING; 10447 if ("token".equals(codeString)) 10448 return SearchParamType.TOKEN; 10449 if ("reference".equals(codeString)) 10450 return SearchParamType.REFERENCE; 10451 if ("composite".equals(codeString)) 10452 return SearchParamType.COMPOSITE; 10453 if ("quantity".equals(codeString)) 10454 return SearchParamType.QUANTITY; 10455 if ("uri".equals(codeString)) 10456 return SearchParamType.URI; 10457 throw new IllegalArgumentException("Unknown SearchParamType code '"+codeString+"'"); 10458 } 10459 public Enumeration<SearchParamType> fromType(PrimitiveType<?> code) throws FHIRException { 10460 if (code == null) 10461 return null; 10462 if (code.isEmpty()) 10463 return new Enumeration<SearchParamType>(this); 10464 String codeString = code.asStringValue(); 10465 if (codeString == null || "".equals(codeString)) 10466 return null; 10467 if ("number".equals(codeString)) 10468 return new Enumeration<SearchParamType>(this, SearchParamType.NUMBER); 10469 if ("date".equals(codeString)) 10470 return new Enumeration<SearchParamType>(this, SearchParamType.DATE); 10471 if ("string".equals(codeString)) 10472 return new Enumeration<SearchParamType>(this, SearchParamType.STRING); 10473 if ("token".equals(codeString)) 10474 return new Enumeration<SearchParamType>(this, SearchParamType.TOKEN); 10475 if ("reference".equals(codeString)) 10476 return new Enumeration<SearchParamType>(this, SearchParamType.REFERENCE); 10477 if ("composite".equals(codeString)) 10478 return new Enumeration<SearchParamType>(this, SearchParamType.COMPOSITE); 10479 if ("quantity".equals(codeString)) 10480 return new Enumeration<SearchParamType>(this, SearchParamType.QUANTITY); 10481 if ("uri".equals(codeString)) 10482 return new Enumeration<SearchParamType>(this, SearchParamType.URI); 10483 throw new FHIRException("Unknown SearchParamType code '"+codeString+"'"); 10484 } 10485 public String toCode(SearchParamType code) { 10486 if (code == SearchParamType.NULL) 10487 return null; 10488 if (code == SearchParamType.NUMBER) 10489 return "number"; 10490 if (code == SearchParamType.DATE) 10491 return "date"; 10492 if (code == SearchParamType.STRING) 10493 return "string"; 10494 if (code == SearchParamType.TOKEN) 10495 return "token"; 10496 if (code == SearchParamType.REFERENCE) 10497 return "reference"; 10498 if (code == SearchParamType.COMPOSITE) 10499 return "composite"; 10500 if (code == SearchParamType.QUANTITY) 10501 return "quantity"; 10502 if (code == SearchParamType.URI) 10503 return "uri"; 10504 return "?"; 10505 } 10506 public String toSystem(SearchParamType code) { 10507 return code.getSystem(); 10508 } 10509 } 10510 10511 public enum SpecialValues { 10512 /** 10513 * Boolean true. 10514 */ 10515 TRUE, 10516 /** 10517 * Boolean false. 10518 */ 10519 FALSE, 10520 /** 10521 * The content is greater than zero, but too small to be quantified. 10522 */ 10523 TRACE, 10524 /** 10525 * The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material. 10526 */ 10527 SUFFICIENT, 10528 /** 10529 * The value is no longer available. 10530 */ 10531 WITHDRAWN, 10532 /** 10533 * The are no known applicable values in this context. 10534 */ 10535 NILKNOWN, 10536 /** 10537 * added to help the parsers 10538 */ 10539 NULL; 10540 public static SpecialValues fromCode(String codeString) throws FHIRException { 10541 if (codeString == null || "".equals(codeString)) 10542 return null; 10543 if ("true".equals(codeString)) 10544 return TRUE; 10545 if ("false".equals(codeString)) 10546 return FALSE; 10547 if ("trace".equals(codeString)) 10548 return TRACE; 10549 if ("sufficient".equals(codeString)) 10550 return SUFFICIENT; 10551 if ("withdrawn".equals(codeString)) 10552 return WITHDRAWN; 10553 if ("nil-known".equals(codeString)) 10554 return NILKNOWN; 10555 throw new FHIRException("Unknown SpecialValues code '"+codeString+"'"); 10556 } 10557 public String toCode() { 10558 switch (this) { 10559 case TRUE: return "true"; 10560 case FALSE: return "false"; 10561 case TRACE: return "trace"; 10562 case SUFFICIENT: return "sufficient"; 10563 case WITHDRAWN: return "withdrawn"; 10564 case NILKNOWN: return "nil-known"; 10565 case NULL: return null; 10566 default: return "?"; 10567 } 10568 } 10569 public String getSystem() { 10570 switch (this) { 10571 case TRUE: return "http://hl7.org/fhir/special-values"; 10572 case FALSE: return "http://hl7.org/fhir/special-values"; 10573 case TRACE: return "http://hl7.org/fhir/special-values"; 10574 case SUFFICIENT: return "http://hl7.org/fhir/special-values"; 10575 case WITHDRAWN: return "http://hl7.org/fhir/special-values"; 10576 case NILKNOWN: return "http://hl7.org/fhir/special-values"; 10577 case NULL: return null; 10578 default: return "?"; 10579 } 10580 } 10581 public String getDefinition() { 10582 switch (this) { 10583 case TRUE: return "Boolean true."; 10584 case FALSE: return "Boolean false."; 10585 case TRACE: return "The content is greater than zero, but too small to be quantified."; 10586 case SUFFICIENT: return "The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material."; 10587 case WITHDRAWN: return "The value is no longer available."; 10588 case NILKNOWN: return "The are no known applicable values in this context."; 10589 case NULL: return null; 10590 default: return "?"; 10591 } 10592 } 10593 public String getDisplay() { 10594 switch (this) { 10595 case TRUE: return "true"; 10596 case FALSE: return "false"; 10597 case TRACE: return "Trace Amount Detected"; 10598 case SUFFICIENT: return "Sufficient Quantity"; 10599 case WITHDRAWN: return "Value Withdrawn"; 10600 case NILKNOWN: return "Nil Known"; 10601 case NULL: return null; 10602 default: return "?"; 10603 } 10604 } 10605 } 10606 10607 public static class SpecialValuesEnumFactory implements EnumFactory<SpecialValues> { 10608 public SpecialValues fromCode(String codeString) throws IllegalArgumentException { 10609 if (codeString == null || "".equals(codeString)) 10610 if (codeString == null || "".equals(codeString)) 10611 return null; 10612 if ("true".equals(codeString)) 10613 return SpecialValues.TRUE; 10614 if ("false".equals(codeString)) 10615 return SpecialValues.FALSE; 10616 if ("trace".equals(codeString)) 10617 return SpecialValues.TRACE; 10618 if ("sufficient".equals(codeString)) 10619 return SpecialValues.SUFFICIENT; 10620 if ("withdrawn".equals(codeString)) 10621 return SpecialValues.WITHDRAWN; 10622 if ("nil-known".equals(codeString)) 10623 return SpecialValues.NILKNOWN; 10624 throw new IllegalArgumentException("Unknown SpecialValues code '"+codeString+"'"); 10625 } 10626 public Enumeration<SpecialValues> fromType(PrimitiveType<?> code) throws FHIRException { 10627 if (code == null) 10628 return null; 10629 if (code.isEmpty()) 10630 return new Enumeration<SpecialValues>(this); 10631 String codeString = code.asStringValue(); 10632 if (codeString == null || "".equals(codeString)) 10633 return null; 10634 if ("true".equals(codeString)) 10635 return new Enumeration<SpecialValues>(this, SpecialValues.TRUE); 10636 if ("false".equals(codeString)) 10637 return new Enumeration<SpecialValues>(this, SpecialValues.FALSE); 10638 if ("trace".equals(codeString)) 10639 return new Enumeration<SpecialValues>(this, SpecialValues.TRACE); 10640 if ("sufficient".equals(codeString)) 10641 return new Enumeration<SpecialValues>(this, SpecialValues.SUFFICIENT); 10642 if ("withdrawn".equals(codeString)) 10643 return new Enumeration<SpecialValues>(this, SpecialValues.WITHDRAWN); 10644 if ("nil-known".equals(codeString)) 10645 return new Enumeration<SpecialValues>(this, SpecialValues.NILKNOWN); 10646 throw new FHIRException("Unknown SpecialValues code '"+codeString+"'"); 10647 } 10648 public String toCode(SpecialValues code) { 10649 if (code == SpecialValues.NULL) 10650 return null; 10651 if (code == SpecialValues.TRUE) 10652 return "true"; 10653 if (code == SpecialValues.FALSE) 10654 return "false"; 10655 if (code == SpecialValues.TRACE) 10656 return "trace"; 10657 if (code == SpecialValues.SUFFICIENT) 10658 return "sufficient"; 10659 if (code == SpecialValues.WITHDRAWN) 10660 return "withdrawn"; 10661 if (code == SpecialValues.NILKNOWN) 10662 return "nil-known"; 10663 return "?"; 10664 } 10665 public String toSystem(SpecialValues code) { 10666 return code.getSystem(); 10667 } 10668 } 10669 10670 10671}