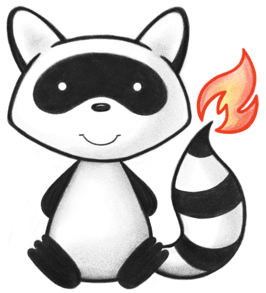
001package org.hl7.fhir.dstu3.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033import org.hl7.fhir.exceptions.FHIRException; 034 035public class Enumerations { 036 037// In here: 038// AbstractType: A type defined by FHIR that is an abstract type 039// AdministrativeGender: The gender of a person used for administrative purposes. 040// AgeUnits: A valueSet of UCUM codes for representing age value units. 041// BindingStrength: Indication of the degree of conformance expectations associated with a binding. 042// ConceptMapEquivalence: The degree of equivalence between concepts. 043// DataAbsentReason: Used to specify why the normally expected content of the data element is missing. 044// DataType: The type of an element - one of the FHIR data types. 045// DocumentReferenceStatus: The status of the document reference. 046// FHIRAllTypes: Either an abstract type, a resource or a data type. 047// FHIRDefinedType: Either a resource or a data type. 048// MessageEvent: One of the message events defined as part of FHIR. 049// NoteType: The presentation types of notes. 050// PublicationStatus: The lifecycle status of a Value Set or Concept Map. 051// RemittanceOutcome: The outcome of the processing. 052// ResourceType: One of the resource types defined as part of FHIR. 053// SearchParamType: Data types allowed to be used for search parameters. 054// SpecialValues: A set of generally useful codes defined so they can be included in value sets. 055 056 057 public enum AbstractType { 058 /** 059 * A place holder that means any kind of data type 060 */ 061 TYPE, 062 /** 063 * A place holder that means any kind of resource 064 */ 065 ANY, 066 /** 067 * added to help the parsers 068 */ 069 NULL; 070 public static AbstractType fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("Type".equals(codeString)) 074 return TYPE; 075 if ("Any".equals(codeString)) 076 return ANY; 077 throw new FHIRException("Unknown AbstractType code '"+codeString+"'"); 078 } 079 public String toCode() { 080 switch (this) { 081 case TYPE: return "Type"; 082 case ANY: return "Any"; 083 case NULL: return null; 084 default: return "?"; 085 } 086 } 087 public String getSystem() { 088 switch (this) { 089 case TYPE: return "http://hl7.org/fhir/abstract-types"; 090 case ANY: return "http://hl7.org/fhir/abstract-types"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getDefinition() { 096 switch (this) { 097 case TYPE: return "A place holder that means any kind of data type"; 098 case ANY: return "A place holder that means any kind of resource"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDisplay() { 104 switch (this) { 105 case TYPE: return "Type"; 106 case ANY: return "Any"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 } 112 113 public static class AbstractTypeEnumFactory implements EnumFactory<AbstractType> { 114 public AbstractType fromCode(String codeString) throws IllegalArgumentException { 115 if (codeString == null || "".equals(codeString)) 116 if (codeString == null || "".equals(codeString)) 117 return null; 118 if ("Type".equals(codeString)) 119 return AbstractType.TYPE; 120 if ("Any".equals(codeString)) 121 return AbstractType.ANY; 122 throw new IllegalArgumentException("Unknown AbstractType code '"+codeString+"'"); 123 } 124 public Enumeration<AbstractType> fromType(PrimitiveType<?> code) throws FHIRException { 125 if (code == null) 126 return null; 127 if (code.isEmpty()) 128 return new Enumeration<AbstractType>(this); 129 String codeString = code.asStringValue(); 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("Type".equals(codeString)) 133 return new Enumeration<AbstractType>(this, AbstractType.TYPE); 134 if ("Any".equals(codeString)) 135 return new Enumeration<AbstractType>(this, AbstractType.ANY); 136 throw new FHIRException("Unknown AbstractType code '"+codeString+"'"); 137 } 138 public String toCode(AbstractType code) { 139 if (code == AbstractType.TYPE) 140 return "Type"; 141 if (code == AbstractType.ANY) 142 return "Any"; 143 return "?"; 144 } 145 public String toSystem(AbstractType code) { 146 return code.getSystem(); 147 } 148 } 149 150 public enum AdministrativeGender { 151 /** 152 * Male 153 */ 154 MALE, 155 /** 156 * Female 157 */ 158 FEMALE, 159 /** 160 * Other 161 */ 162 OTHER, 163 /** 164 * Unknown 165 */ 166 UNKNOWN, 167 /** 168 * added to help the parsers 169 */ 170 NULL; 171 public static AdministrativeGender fromCode(String codeString) throws FHIRException { 172 if (codeString == null || "".equals(codeString)) 173 return null; 174 if ("male".equals(codeString)) 175 return MALE; 176 if ("female".equals(codeString)) 177 return FEMALE; 178 if ("other".equals(codeString)) 179 return OTHER; 180 if ("unknown".equals(codeString)) 181 return UNKNOWN; 182 throw new FHIRException("Unknown AdministrativeGender code '"+codeString+"'"); 183 } 184 public String toCode() { 185 switch (this) { 186 case MALE: return "male"; 187 case FEMALE: return "female"; 188 case OTHER: return "other"; 189 case UNKNOWN: return "unknown"; 190 case NULL: return null; 191 default: return "?"; 192 } 193 } 194 public String getSystem() { 195 switch (this) { 196 case MALE: return "http://hl7.org/fhir/administrative-gender"; 197 case FEMALE: return "http://hl7.org/fhir/administrative-gender"; 198 case OTHER: return "http://hl7.org/fhir/administrative-gender"; 199 case UNKNOWN: return "http://hl7.org/fhir/administrative-gender"; 200 case NULL: return null; 201 default: return "?"; 202 } 203 } 204 public String getDefinition() { 205 switch (this) { 206 case MALE: return "Male"; 207 case FEMALE: return "Female"; 208 case OTHER: return "Other"; 209 case UNKNOWN: return "Unknown"; 210 case NULL: return null; 211 default: return "?"; 212 } 213 } 214 public String getDisplay() { 215 switch (this) { 216 case MALE: return "Male"; 217 case FEMALE: return "Female"; 218 case OTHER: return "Other"; 219 case UNKNOWN: return "Unknown"; 220 case NULL: return null; 221 default: return "?"; 222 } 223 } 224 } 225 226 public static class AdministrativeGenderEnumFactory implements EnumFactory<AdministrativeGender> { 227 public AdministrativeGender fromCode(String codeString) throws IllegalArgumentException { 228 if (codeString == null || "".equals(codeString)) 229 if (codeString == null || "".equals(codeString)) 230 return null; 231 if ("male".equals(codeString)) 232 return AdministrativeGender.MALE; 233 if ("female".equals(codeString)) 234 return AdministrativeGender.FEMALE; 235 if ("other".equals(codeString)) 236 return AdministrativeGender.OTHER; 237 if ("unknown".equals(codeString)) 238 return AdministrativeGender.UNKNOWN; 239 throw new IllegalArgumentException("Unknown AdministrativeGender code '"+codeString+"'"); 240 } 241 public Enumeration<AdministrativeGender> fromType(PrimitiveType<?> code) throws FHIRException { 242 if (code == null) 243 return null; 244 if (code.isEmpty()) 245 return new Enumeration<AdministrativeGender>(this); 246 String codeString = code.asStringValue(); 247 if (codeString == null || "".equals(codeString)) 248 return null; 249 if ("male".equals(codeString)) 250 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.MALE); 251 if ("female".equals(codeString)) 252 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.FEMALE); 253 if ("other".equals(codeString)) 254 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.OTHER); 255 if ("unknown".equals(codeString)) 256 return new Enumeration<AdministrativeGender>(this, AdministrativeGender.UNKNOWN); 257 throw new FHIRException("Unknown AdministrativeGender code '"+codeString+"'"); 258 } 259 public String toCode(AdministrativeGender code) { 260 if (code == AdministrativeGender.MALE) 261 return "male"; 262 if (code == AdministrativeGender.FEMALE) 263 return "female"; 264 if (code == AdministrativeGender.OTHER) 265 return "other"; 266 if (code == AdministrativeGender.UNKNOWN) 267 return "unknown"; 268 return "?"; 269 } 270 public String toSystem(AdministrativeGender code) { 271 return code.getSystem(); 272 } 273 } 274 275 public enum AgeUnits { 276 /** 277 * null 278 */ 279 MIN, 280 /** 281 * null 282 */ 283 H, 284 /** 285 * null 286 */ 287 D, 288 /** 289 * null 290 */ 291 WK, 292 /** 293 * null 294 */ 295 MO, 296 /** 297 * null 298 */ 299 A, 300 /** 301 * added to help the parsers 302 */ 303 NULL; 304 public static AgeUnits fromCode(String codeString) throws FHIRException { 305 if (codeString == null || "".equals(codeString)) 306 return null; 307 if ("min".equals(codeString)) 308 return MIN; 309 if ("h".equals(codeString)) 310 return H; 311 if ("d".equals(codeString)) 312 return D; 313 if ("wk".equals(codeString)) 314 return WK; 315 if ("mo".equals(codeString)) 316 return MO; 317 if ("a".equals(codeString)) 318 return A; 319 throw new FHIRException("Unknown AgeUnits code '"+codeString+"'"); 320 } 321 public String toCode() { 322 switch (this) { 323 case MIN: return "min"; 324 case H: return "h"; 325 case D: return "d"; 326 case WK: return "wk"; 327 case MO: return "mo"; 328 case A: return "a"; 329 case NULL: return null; 330 default: return "?"; 331 } 332 } 333 public String getSystem() { 334 switch (this) { 335 case MIN: return "http://unitsofmeasure.org"; 336 case H: return "http://unitsofmeasure.org"; 337 case D: return "http://unitsofmeasure.org"; 338 case WK: return "http://unitsofmeasure.org"; 339 case MO: return "http://unitsofmeasure.org"; 340 case A: return "http://unitsofmeasure.org"; 341 case NULL: return null; 342 default: return "?"; 343 } 344 } 345 public String getDefinition() { 346 switch (this) { 347 case MIN: return ""; 348 case H: return ""; 349 case D: return ""; 350 case WK: return ""; 351 case MO: return ""; 352 case A: return ""; 353 case NULL: return null; 354 default: return "?"; 355 } 356 } 357 public String getDisplay() { 358 switch (this) { 359 case MIN: return "Minute"; 360 case H: return "Hour"; 361 case D: return "Day"; 362 case WK: return "Week"; 363 case MO: return "Month"; 364 case A: return "Year"; 365 case NULL: return null; 366 default: return "?"; 367 } 368 } 369 } 370 371 public static class AgeUnitsEnumFactory implements EnumFactory<AgeUnits> { 372 public AgeUnits fromCode(String codeString) throws IllegalArgumentException { 373 if (codeString == null || "".equals(codeString)) 374 if (codeString == null || "".equals(codeString)) 375 return null; 376 if ("min".equals(codeString)) 377 return AgeUnits.MIN; 378 if ("h".equals(codeString)) 379 return AgeUnits.H; 380 if ("d".equals(codeString)) 381 return AgeUnits.D; 382 if ("wk".equals(codeString)) 383 return AgeUnits.WK; 384 if ("mo".equals(codeString)) 385 return AgeUnits.MO; 386 if ("a".equals(codeString)) 387 return AgeUnits.A; 388 throw new IllegalArgumentException("Unknown AgeUnits code '"+codeString+"'"); 389 } 390 public Enumeration<AgeUnits> fromType(PrimitiveType<?> code) throws FHIRException { 391 if (code == null) 392 return null; 393 if (code.isEmpty()) 394 return new Enumeration<AgeUnits>(this); 395 String codeString = code.asStringValue(); 396 if (codeString == null || "".equals(codeString)) 397 return null; 398 if ("min".equals(codeString)) 399 return new Enumeration<AgeUnits>(this, AgeUnits.MIN); 400 if ("h".equals(codeString)) 401 return new Enumeration<AgeUnits>(this, AgeUnits.H); 402 if ("d".equals(codeString)) 403 return new Enumeration<AgeUnits>(this, AgeUnits.D); 404 if ("wk".equals(codeString)) 405 return new Enumeration<AgeUnits>(this, AgeUnits.WK); 406 if ("mo".equals(codeString)) 407 return new Enumeration<AgeUnits>(this, AgeUnits.MO); 408 if ("a".equals(codeString)) 409 return new Enumeration<AgeUnits>(this, AgeUnits.A); 410 throw new FHIRException("Unknown AgeUnits code '"+codeString+"'"); 411 } 412 public String toCode(AgeUnits code) { 413 if (code == AgeUnits.MIN) 414 return "min"; 415 if (code == AgeUnits.H) 416 return "h"; 417 if (code == AgeUnits.D) 418 return "d"; 419 if (code == AgeUnits.WK) 420 return "wk"; 421 if (code == AgeUnits.MO) 422 return "mo"; 423 if (code == AgeUnits.A) 424 return "a"; 425 return "?"; 426 } 427 public String toSystem(AgeUnits code) { 428 return code.getSystem(); 429 } 430 } 431 432 public enum BindingStrength { 433 /** 434 * To be conformant, the concept in this element SHALL be from the specified value set 435 */ 436 REQUIRED, 437 /** 438 * To be conformant, the concept in this element SHALL be from the specified value set if any of the codes within the value set can apply to the concept being communicated. If the value set does not cover the concept (based on human review), alternate codings (or, data type allowing, text) may be included instead. 439 */ 440 EXTENSIBLE, 441 /** 442 * Instances are encouraged to draw from the specified codes for interoperability purposes but are not required to do so to be considered conformant. 443 */ 444 PREFERRED, 445 /** 446 * Instances are not expected or even encouraged to draw from the specified value set. The value set merely provides examples of the types of concepts intended to be included. 447 */ 448 EXAMPLE, 449 /** 450 * added to help the parsers 451 */ 452 NULL; 453 public static BindingStrength fromCode(String codeString) throws FHIRException { 454 if (codeString == null || "".equals(codeString)) 455 return null; 456 if ("required".equals(codeString)) 457 return REQUIRED; 458 if ("extensible".equals(codeString)) 459 return EXTENSIBLE; 460 if ("preferred".equals(codeString)) 461 return PREFERRED; 462 if ("example".equals(codeString)) 463 return EXAMPLE; 464 throw new FHIRException("Unknown BindingStrength code '"+codeString+"'"); 465 } 466 public String toCode() { 467 switch (this) { 468 case REQUIRED: return "required"; 469 case EXTENSIBLE: return "extensible"; 470 case PREFERRED: return "preferred"; 471 case EXAMPLE: return "example"; 472 case NULL: return null; 473 default: return "?"; 474 } 475 } 476 public String getSystem() { 477 switch (this) { 478 case REQUIRED: return "http://hl7.org/fhir/binding-strength"; 479 case EXTENSIBLE: return "http://hl7.org/fhir/binding-strength"; 480 case PREFERRED: return "http://hl7.org/fhir/binding-strength"; 481 case EXAMPLE: return "http://hl7.org/fhir/binding-strength"; 482 case NULL: return null; 483 default: return "?"; 484 } 485 } 486 public String getDefinition() { 487 switch (this) { 488 case REQUIRED: return "To be conformant, the concept in this element SHALL be from the specified value set"; 489 case EXTENSIBLE: return "To be conformant, the concept in this element SHALL be from the specified value set if any of the codes within the value set can apply to the concept being communicated. If the value set does not cover the concept (based on human review), alternate codings (or, data type allowing, text) may be included instead."; 490 case PREFERRED: return "Instances are encouraged to draw from the specified codes for interoperability purposes but are not required to do so to be considered conformant."; 491 case EXAMPLE: return "Instances are not expected or even encouraged to draw from the specified value set. The value set merely provides examples of the types of concepts intended to be included."; 492 case NULL: return null; 493 default: return "?"; 494 } 495 } 496 public String getDisplay() { 497 switch (this) { 498 case REQUIRED: return "Required"; 499 case EXTENSIBLE: return "Extensible"; 500 case PREFERRED: return "Preferred"; 501 case EXAMPLE: return "Example"; 502 case NULL: return null; 503 default: return "?"; 504 } 505 } 506 } 507 508 public static class BindingStrengthEnumFactory implements EnumFactory<BindingStrength> { 509 public BindingStrength fromCode(String codeString) throws IllegalArgumentException { 510 if (codeString == null || "".equals(codeString)) 511 if (codeString == null || "".equals(codeString)) 512 return null; 513 if ("required".equals(codeString)) 514 return BindingStrength.REQUIRED; 515 if ("extensible".equals(codeString)) 516 return BindingStrength.EXTENSIBLE; 517 if ("preferred".equals(codeString)) 518 return BindingStrength.PREFERRED; 519 if ("example".equals(codeString)) 520 return BindingStrength.EXAMPLE; 521 throw new IllegalArgumentException("Unknown BindingStrength code '"+codeString+"'"); 522 } 523 public Enumeration<BindingStrength> fromType(PrimitiveType<?> code) throws FHIRException { 524 if (code == null) 525 return null; 526 if (code.isEmpty()) 527 return new Enumeration<BindingStrength>(this); 528 String codeString = code.asStringValue(); 529 if (codeString == null || "".equals(codeString)) 530 return null; 531 if ("required".equals(codeString)) 532 return new Enumeration<BindingStrength>(this, BindingStrength.REQUIRED); 533 if ("extensible".equals(codeString)) 534 return new Enumeration<BindingStrength>(this, BindingStrength.EXTENSIBLE); 535 if ("preferred".equals(codeString)) 536 return new Enumeration<BindingStrength>(this, BindingStrength.PREFERRED); 537 if ("example".equals(codeString)) 538 return new Enumeration<BindingStrength>(this, BindingStrength.EXAMPLE); 539 throw new FHIRException("Unknown BindingStrength code '"+codeString+"'"); 540 } 541 public String toCode(BindingStrength code) { 542 if (code == BindingStrength.REQUIRED) 543 return "required"; 544 if (code == BindingStrength.EXTENSIBLE) 545 return "extensible"; 546 if (code == BindingStrength.PREFERRED) 547 return "preferred"; 548 if (code == BindingStrength.EXAMPLE) 549 return "example"; 550 return "?"; 551 } 552 public String toSystem(BindingStrength code) { 553 return code.getSystem(); 554 } 555 } 556 557 public enum ConceptMapEquivalence { 558 /** 559 * The concepts are related to each other, and have at least some overlap in meaning, but the exact relationship is not known 560 */ 561 RELATEDTO, 562 /** 563 * The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical). 564 */ 565 EQUIVALENT, 566 /** 567 * The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical). 568 */ 569 EQUAL, 570 /** 571 * The target mapping is wider in meaning than the source concept. 572 */ 573 WIDER, 574 /** 575 * The target mapping subsumes the meaning of the source concept (e.g. the source is-a target). 576 */ 577 SUBSUMES, 578 /** 579 * The target mapping is narrower in meaning than the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally. 580 */ 581 NARROWER, 582 /** 583 * The target mapping specializes the meaning of the source concept (e.g. the target is-a source). 584 */ 585 SPECIALIZES, 586 /** 587 * The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally. 588 */ 589 INEXACT, 590 /** 591 * There is no match for this concept in the destination concept system. 592 */ 593 UNMATCHED, 594 /** 595 * This is an explicit assertion that there is no mapping between the source and target concept. 596 */ 597 DISJOINT, 598 /** 599 * added to help the parsers 600 */ 601 NULL; 602 public static ConceptMapEquivalence fromCode(String codeString) throws FHIRException { 603 if (codeString == null || "".equals(codeString)) 604 return null; 605 if ("relatedto".equals(codeString)) 606 return RELATEDTO; 607 if ("equivalent".equals(codeString)) 608 return EQUIVALENT; 609 if ("equal".equals(codeString)) 610 return EQUAL; 611 if ("wider".equals(codeString)) 612 return WIDER; 613 if ("subsumes".equals(codeString)) 614 return SUBSUMES; 615 if ("narrower".equals(codeString)) 616 return NARROWER; 617 if ("specializes".equals(codeString)) 618 return SPECIALIZES; 619 if ("inexact".equals(codeString)) 620 return INEXACT; 621 if ("unmatched".equals(codeString)) 622 return UNMATCHED; 623 if ("disjoint".equals(codeString)) 624 return DISJOINT; 625 throw new FHIRException("Unknown ConceptMapEquivalence code '"+codeString+"'"); 626 } 627 public String toCode() { 628 switch (this) { 629 case RELATEDTO: return "relatedto"; 630 case EQUIVALENT: return "equivalent"; 631 case EQUAL: return "equal"; 632 case WIDER: return "wider"; 633 case SUBSUMES: return "subsumes"; 634 case NARROWER: return "narrower"; 635 case SPECIALIZES: return "specializes"; 636 case INEXACT: return "inexact"; 637 case UNMATCHED: return "unmatched"; 638 case DISJOINT: return "disjoint"; 639 case NULL: return null; 640 default: return "?"; 641 } 642 } 643 public String getSystem() { 644 switch (this) { 645 case RELATEDTO: return "http://hl7.org/fhir/concept-map-equivalence"; 646 case EQUIVALENT: return "http://hl7.org/fhir/concept-map-equivalence"; 647 case EQUAL: return "http://hl7.org/fhir/concept-map-equivalence"; 648 case WIDER: return "http://hl7.org/fhir/concept-map-equivalence"; 649 case SUBSUMES: return "http://hl7.org/fhir/concept-map-equivalence"; 650 case NARROWER: return "http://hl7.org/fhir/concept-map-equivalence"; 651 case SPECIALIZES: return "http://hl7.org/fhir/concept-map-equivalence"; 652 case INEXACT: return "http://hl7.org/fhir/concept-map-equivalence"; 653 case UNMATCHED: return "http://hl7.org/fhir/concept-map-equivalence"; 654 case DISJOINT: return "http://hl7.org/fhir/concept-map-equivalence"; 655 case NULL: return null; 656 default: return "?"; 657 } 658 } 659 public String getDefinition() { 660 switch (this) { 661 case RELATEDTO: return "The concepts are related to each other, and have at least some overlap in meaning, but the exact relationship is not known"; 662 case EQUIVALENT: return "The definitions of the concepts mean the same thing (including when structural implications of meaning are considered) (i.e. extensionally identical)."; 663 case EQUAL: return "The definitions of the concepts are exactly the same (i.e. only grammatical differences) and structural implications of meaning are identical or irrelevant (i.e. intentionally identical)."; 664 case WIDER: return "The target mapping is wider in meaning than the source concept."; 665 case SUBSUMES: return "The target mapping subsumes the meaning of the source concept (e.g. the source is-a target)."; 666 case NARROWER: return "The target mapping is narrower in meaning than the source concept. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 667 case SPECIALIZES: return "The target mapping specializes the meaning of the source concept (e.g. the target is-a source)."; 668 case INEXACT: return "The target mapping overlaps with the source concept, but both source and target cover additional meaning, or the definitions are imprecise and it is uncertain whether they have the same boundaries to their meaning. The sense in which the mapping is narrower SHALL be described in the comments in this case, and applications should be careful when attempting to use these mappings operationally."; 669 case UNMATCHED: return "There is no match for this concept in the destination concept system."; 670 case DISJOINT: return "This is an explicit assertion that there is no mapping between the source and target concept."; 671 case NULL: return null; 672 default: return "?"; 673 } 674 } 675 public String getDisplay() { 676 switch (this) { 677 case RELATEDTO: return "Related To"; 678 case EQUIVALENT: return "Equivalent"; 679 case EQUAL: return "Equal"; 680 case WIDER: return "Wider"; 681 case SUBSUMES: return "Subsumes"; 682 case NARROWER: return "Narrower"; 683 case SPECIALIZES: return "Specializes"; 684 case INEXACT: return "Inexact"; 685 case UNMATCHED: return "Unmatched"; 686 case DISJOINT: return "Disjoint"; 687 case NULL: return null; 688 default: return "?"; 689 } 690 } 691 } 692 693 public static class ConceptMapEquivalenceEnumFactory implements EnumFactory<ConceptMapEquivalence> { 694 public ConceptMapEquivalence fromCode(String codeString) throws IllegalArgumentException { 695 if (codeString == null || "".equals(codeString)) 696 if (codeString == null || "".equals(codeString)) 697 return null; 698 if ("relatedto".equals(codeString)) 699 return ConceptMapEquivalence.RELATEDTO; 700 if ("equivalent".equals(codeString)) 701 return ConceptMapEquivalence.EQUIVALENT; 702 if ("equal".equals(codeString)) 703 return ConceptMapEquivalence.EQUAL; 704 if ("wider".equals(codeString)) 705 return ConceptMapEquivalence.WIDER; 706 if ("subsumes".equals(codeString)) 707 return ConceptMapEquivalence.SUBSUMES; 708 if ("narrower".equals(codeString)) 709 return ConceptMapEquivalence.NARROWER; 710 if ("specializes".equals(codeString)) 711 return ConceptMapEquivalence.SPECIALIZES; 712 if ("inexact".equals(codeString)) 713 return ConceptMapEquivalence.INEXACT; 714 if ("unmatched".equals(codeString)) 715 return ConceptMapEquivalence.UNMATCHED; 716 if ("disjoint".equals(codeString)) 717 return ConceptMapEquivalence.DISJOINT; 718 throw new IllegalArgumentException("Unknown ConceptMapEquivalence code '"+codeString+"'"); 719 } 720 public Enumeration<ConceptMapEquivalence> fromType(PrimitiveType<?> code) throws FHIRException { 721 if (code == null) 722 return null; 723 if (code.isEmpty()) 724 return new Enumeration<ConceptMapEquivalence>(this); 725 String codeString = code.asStringValue(); 726 if (codeString == null || "".equals(codeString)) 727 return null; 728 if ("relatedto".equals(codeString)) 729 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.RELATEDTO); 730 if ("equivalent".equals(codeString)) 731 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUIVALENT); 732 if ("equal".equals(codeString)) 733 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.EQUAL); 734 if ("wider".equals(codeString)) 735 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.WIDER); 736 if ("subsumes".equals(codeString)) 737 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SUBSUMES); 738 if ("narrower".equals(codeString)) 739 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.NARROWER); 740 if ("specializes".equals(codeString)) 741 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.SPECIALIZES); 742 if ("inexact".equals(codeString)) 743 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.INEXACT); 744 if ("unmatched".equals(codeString)) 745 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.UNMATCHED); 746 if ("disjoint".equals(codeString)) 747 return new Enumeration<ConceptMapEquivalence>(this, ConceptMapEquivalence.DISJOINT); 748 throw new FHIRException("Unknown ConceptMapEquivalence code '"+codeString+"'"); 749 } 750 public String toCode(ConceptMapEquivalence code) { 751 if (code == ConceptMapEquivalence.RELATEDTO) 752 return "relatedto"; 753 if (code == ConceptMapEquivalence.EQUIVALENT) 754 return "equivalent"; 755 if (code == ConceptMapEquivalence.EQUAL) 756 return "equal"; 757 if (code == ConceptMapEquivalence.WIDER) 758 return "wider"; 759 if (code == ConceptMapEquivalence.SUBSUMES) 760 return "subsumes"; 761 if (code == ConceptMapEquivalence.NARROWER) 762 return "narrower"; 763 if (code == ConceptMapEquivalence.SPECIALIZES) 764 return "specializes"; 765 if (code == ConceptMapEquivalence.INEXACT) 766 return "inexact"; 767 if (code == ConceptMapEquivalence.UNMATCHED) 768 return "unmatched"; 769 if (code == ConceptMapEquivalence.DISJOINT) 770 return "disjoint"; 771 return "?"; 772 } 773 public String toSystem(ConceptMapEquivalence code) { 774 return code.getSystem(); 775 } 776 } 777 778 public enum DataAbsentReason { 779 /** 780 * The value is not known. 781 */ 782 UNKNOWN, 783 /** 784 * The source human does not know the value. 785 */ 786 ASKED, 787 /** 788 * There is reason to expect (from the workflow) that the value may become known. 789 */ 790 TEMP, 791 /** 792 * The workflow didn't lead to this value being known. 793 */ 794 NOTASKED, 795 /** 796 * The information is not available due to security, privacy or related reasons. 797 */ 798 MASKED, 799 /** 800 * The source system wasn't capable of supporting this element. 801 */ 802 UNSUPPORTED, 803 /** 804 * The content of the data is represented in the resource narrative. 805 */ 806 ASTEXT, 807 /** 808 * Some system or workflow process error means that the information is not available. 809 */ 810 ERROR, 811 /** 812 * NaN, standing for not a number, is a numeric data type value representing an undefined or unrepresentable value. 813 */ 814 NAN, 815 /** 816 * The value is not available because the observation procedure (test, etc.) was not performed. 817 */ 818 NOTPERFORMED, 819 /** 820 * added to help the parsers 821 */ 822 NULL; 823 public static DataAbsentReason fromCode(String codeString) throws FHIRException { 824 if (codeString == null || "".equals(codeString)) 825 return null; 826 if ("unknown".equals(codeString)) 827 return UNKNOWN; 828 if ("asked".equals(codeString)) 829 return ASKED; 830 if ("temp".equals(codeString)) 831 return TEMP; 832 if ("not-asked".equals(codeString)) 833 return NOTASKED; 834 if ("masked".equals(codeString)) 835 return MASKED; 836 if ("unsupported".equals(codeString)) 837 return UNSUPPORTED; 838 if ("astext".equals(codeString)) 839 return ASTEXT; 840 if ("error".equals(codeString)) 841 return ERROR; 842 if ("NaN".equals(codeString)) 843 return NAN; 844 if ("not-performed".equals(codeString)) 845 return NOTPERFORMED; 846 throw new FHIRException("Unknown DataAbsentReason code '"+codeString+"'"); 847 } 848 public String toCode() { 849 switch (this) { 850 case UNKNOWN: return "unknown"; 851 case ASKED: return "asked"; 852 case TEMP: return "temp"; 853 case NOTASKED: return "not-asked"; 854 case MASKED: return "masked"; 855 case UNSUPPORTED: return "unsupported"; 856 case ASTEXT: return "astext"; 857 case ERROR: return "error"; 858 case NAN: return "NaN"; 859 case NOTPERFORMED: return "not-performed"; 860 case NULL: return null; 861 default: return "?"; 862 } 863 } 864 public String getSystem() { 865 switch (this) { 866 case UNKNOWN: return "http://hl7.org/fhir/data-absent-reason"; 867 case ASKED: return "http://hl7.org/fhir/data-absent-reason"; 868 case TEMP: return "http://hl7.org/fhir/data-absent-reason"; 869 case NOTASKED: return "http://hl7.org/fhir/data-absent-reason"; 870 case MASKED: return "http://hl7.org/fhir/data-absent-reason"; 871 case UNSUPPORTED: return "http://hl7.org/fhir/data-absent-reason"; 872 case ASTEXT: return "http://hl7.org/fhir/data-absent-reason"; 873 case ERROR: return "http://hl7.org/fhir/data-absent-reason"; 874 case NAN: return "http://hl7.org/fhir/data-absent-reason"; 875 case NOTPERFORMED: return "http://hl7.org/fhir/data-absent-reason"; 876 case NULL: return null; 877 default: return "?"; 878 } 879 } 880 public String getDefinition() { 881 switch (this) { 882 case UNKNOWN: return "The value is not known."; 883 case ASKED: return "The source human does not know the value."; 884 case TEMP: return "There is reason to expect (from the workflow) that the value may become known."; 885 case NOTASKED: return "The workflow didn't lead to this value being known."; 886 case MASKED: return "The information is not available due to security, privacy or related reasons."; 887 case UNSUPPORTED: return "The source system wasn't capable of supporting this element."; 888 case ASTEXT: return "The content of the data is represented in the resource narrative."; 889 case ERROR: return "Some system or workflow process error means that the information is not available."; 890 case NAN: return "NaN, standing for not a number, is a numeric data type value representing an undefined or unrepresentable value."; 891 case NOTPERFORMED: return "The value is not available because the observation procedure (test, etc.) was not performed."; 892 case NULL: return null; 893 default: return "?"; 894 } 895 } 896 public String getDisplay() { 897 switch (this) { 898 case UNKNOWN: return "Unknown"; 899 case ASKED: return "Asked"; 900 case TEMP: return "Temp"; 901 case NOTASKED: return "Not Asked"; 902 case MASKED: return "Masked"; 903 case UNSUPPORTED: return "Unsupported"; 904 case ASTEXT: return "As Text"; 905 case ERROR: return "Error"; 906 case NAN: return "Not a Number"; 907 case NOTPERFORMED: return "Not Performed"; 908 case NULL: return null; 909 default: return "?"; 910 } 911 } 912 } 913 914 public static class DataAbsentReasonEnumFactory implements EnumFactory<DataAbsentReason> { 915 public DataAbsentReason fromCode(String codeString) throws IllegalArgumentException { 916 if (codeString == null || "".equals(codeString)) 917 if (codeString == null || "".equals(codeString)) 918 return null; 919 if ("unknown".equals(codeString)) 920 return DataAbsentReason.UNKNOWN; 921 if ("asked".equals(codeString)) 922 return DataAbsentReason.ASKED; 923 if ("temp".equals(codeString)) 924 return DataAbsentReason.TEMP; 925 if ("not-asked".equals(codeString)) 926 return DataAbsentReason.NOTASKED; 927 if ("masked".equals(codeString)) 928 return DataAbsentReason.MASKED; 929 if ("unsupported".equals(codeString)) 930 return DataAbsentReason.UNSUPPORTED; 931 if ("astext".equals(codeString)) 932 return DataAbsentReason.ASTEXT; 933 if ("error".equals(codeString)) 934 return DataAbsentReason.ERROR; 935 if ("NaN".equals(codeString)) 936 return DataAbsentReason.NAN; 937 if ("not-performed".equals(codeString)) 938 return DataAbsentReason.NOTPERFORMED; 939 throw new IllegalArgumentException("Unknown DataAbsentReason code '"+codeString+"'"); 940 } 941 public Enumeration<DataAbsentReason> fromType(PrimitiveType<?> code) throws FHIRException { 942 if (code == null) 943 return null; 944 if (code.isEmpty()) 945 return new Enumeration<DataAbsentReason>(this); 946 String codeString = code.asStringValue(); 947 if (codeString == null || "".equals(codeString)) 948 return null; 949 if ("unknown".equals(codeString)) 950 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNKNOWN); 951 if ("asked".equals(codeString)) 952 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASKED); 953 if ("temp".equals(codeString)) 954 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.TEMP); 955 if ("not-asked".equals(codeString)) 956 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTASKED); 957 if ("masked".equals(codeString)) 958 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.MASKED); 959 if ("unsupported".equals(codeString)) 960 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.UNSUPPORTED); 961 if ("astext".equals(codeString)) 962 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ASTEXT); 963 if ("error".equals(codeString)) 964 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.ERROR); 965 if ("NaN".equals(codeString)) 966 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NAN); 967 if ("not-performed".equals(codeString)) 968 return new Enumeration<DataAbsentReason>(this, DataAbsentReason.NOTPERFORMED); 969 throw new FHIRException("Unknown DataAbsentReason code '"+codeString+"'"); 970 } 971 public String toCode(DataAbsentReason code) { 972 if (code == DataAbsentReason.UNKNOWN) 973 return "unknown"; 974 if (code == DataAbsentReason.ASKED) 975 return "asked"; 976 if (code == DataAbsentReason.TEMP) 977 return "temp"; 978 if (code == DataAbsentReason.NOTASKED) 979 return "not-asked"; 980 if (code == DataAbsentReason.MASKED) 981 return "masked"; 982 if (code == DataAbsentReason.UNSUPPORTED) 983 return "unsupported"; 984 if (code == DataAbsentReason.ASTEXT) 985 return "astext"; 986 if (code == DataAbsentReason.ERROR) 987 return "error"; 988 if (code == DataAbsentReason.NAN) 989 return "NaN"; 990 if (code == DataAbsentReason.NOTPERFORMED) 991 return "not-performed"; 992 return "?"; 993 } 994 public String toSystem(DataAbsentReason code) { 995 return code.getSystem(); 996 } 997 } 998 999 public enum DataType { 1000 /** 1001 * An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world. 1002 */ 1003 ADDRESS, 1004 /** 1005 * A duration of time during which an organism (or a process) has existed. 1006 */ 1007 AGE, 1008 /** 1009 * A text note which also contains information about who made the statement and when. 1010 */ 1011 ANNOTATION, 1012 /** 1013 * For referring to data content defined in other formats. 1014 */ 1015 ATTACHMENT, 1016 /** 1017 * Base definition for all elements that are defined inside a resource - but not those in a data type. 1018 */ 1019 BACKBONEELEMENT, 1020 /** 1021 * A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text. 1022 */ 1023 CODEABLECONCEPT, 1024 /** 1025 * A reference to a code defined by a terminology system. 1026 */ 1027 CODING, 1028 /** 1029 * Specifies contact information for a person or organization. 1030 */ 1031 CONTACTDETAIL, 1032 /** 1033 * Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 1034 */ 1035 CONTACTPOINT, 1036 /** 1037 * A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers. 1038 */ 1039 CONTRIBUTOR, 1040 /** 1041 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 1042 */ 1043 COUNT, 1044 /** 1045 * Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data. 1046 */ 1047 DATAREQUIREMENT, 1048 /** 1049 * A length - a value with a unit that is a physical distance. 1050 */ 1051 DISTANCE, 1052 /** 1053 * Indicates how the medication is/was taken or should be taken by the patient. 1054 */ 1055 DOSAGE, 1056 /** 1057 * A length of time. 1058 */ 1059 DURATION, 1060 /** 1061 * Base definition for all elements in a resource. 1062 */ 1063 ELEMENT, 1064 /** 1065 * Captures constraints on each element within the resource, profile, or extension. 1066 */ 1067 ELEMENTDEFINITION, 1068 /** 1069 * Optional Extension Element - found in all resources. 1070 */ 1071 EXTENSION, 1072 /** 1073 * A human's name with the ability to identify parts and usage. 1074 */ 1075 HUMANNAME, 1076 /** 1077 * A technical identifier - identifies some entity uniquely and unambiguously. 1078 */ 1079 IDENTIFIER, 1080 /** 1081 * The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource. 1082 */ 1083 META, 1084 /** 1085 * An amount of economic utility in some recognized currency. 1086 */ 1087 MONEY, 1088 /** 1089 * A human-readable formatted text, including images. 1090 */ 1091 NARRATIVE, 1092 /** 1093 * The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse. 1094 */ 1095 PARAMETERDEFINITION, 1096 /** 1097 * A time period defined by a start and end date and optionally time. 1098 */ 1099 PERIOD, 1100 /** 1101 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 1102 */ 1103 QUANTITY, 1104 /** 1105 * A set of ordered Quantities defined by a low and high limit. 1106 */ 1107 RANGE, 1108 /** 1109 * A relationship of two Quantity values - expressed as a numerator and a denominator. 1110 */ 1111 RATIO, 1112 /** 1113 * A reference from one resource to another. 1114 */ 1115 REFERENCE, 1116 /** 1117 * Related artifacts such as additional documentation, justification, or bibliographic references. 1118 */ 1119 RELATEDARTIFACT, 1120 /** 1121 * A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data. 1122 */ 1123 SAMPLEDDATA, 1124 /** 1125 * A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities. 1126 */ 1127 SIGNATURE, 1128 /** 1129 * null 1130 */ 1131 SIMPLEQUANTITY, 1132 /** 1133 * Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out. 1134 */ 1135 TIMING, 1136 /** 1137 * A description of a triggering event. 1138 */ 1139 TRIGGERDEFINITION, 1140 /** 1141 * Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care). 1142 */ 1143 USAGECONTEXT, 1144 /** 1145 * A stream of bytes 1146 */ 1147 BASE64BINARY, 1148 /** 1149 * Value of "true" or "false" 1150 */ 1151 BOOLEAN, 1152 /** 1153 * A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents 1154 */ 1155 CODE, 1156 /** 1157 * A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates. 1158 */ 1159 DATE, 1160 /** 1161 * A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates. 1162 */ 1163 DATETIME, 1164 /** 1165 * A rational number with implicit precision 1166 */ 1167 DECIMAL, 1168 /** 1169 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive. 1170 */ 1171 ID, 1172 /** 1173 * An instant in time - known at least to the second 1174 */ 1175 INSTANT, 1176 /** 1177 * A whole number 1178 */ 1179 INTEGER, 1180 /** 1181 * A string that may contain markdown syntax for optional processing by a mark down presentation engine 1182 */ 1183 MARKDOWN, 1184 /** 1185 * An OID represented as a URI 1186 */ 1187 OID, 1188 /** 1189 * An integer with a value that is positive (e.g. >0) 1190 */ 1191 POSITIVEINT, 1192 /** 1193 * A sequence of Unicode characters 1194 */ 1195 STRING, 1196 /** 1197 * A time during the day, with no date specified 1198 */ 1199 TIME, 1200 /** 1201 * An integer with a value that is not negative (e.g. >= 0) 1202 */ 1203 UNSIGNEDINT, 1204 /** 1205 * String of characters used to identify a name or a resource 1206 */ 1207 URI, 1208 /** 1209 * A UUID, represented as a URI 1210 */ 1211 UUID, 1212 /** 1213 * XHTML format, as defined by W3C, but restricted usage (mainly, no active content) 1214 */ 1215 XHTML, 1216 /** 1217 * added to help the parsers 1218 */ 1219 NULL; 1220 public static DataType fromCode(String codeString) throws FHIRException { 1221 if (codeString == null || "".equals(codeString)) 1222 return null; 1223 if ("Address".equals(codeString)) 1224 return ADDRESS; 1225 if ("Age".equals(codeString)) 1226 return AGE; 1227 if ("Annotation".equals(codeString)) 1228 return ANNOTATION; 1229 if ("Attachment".equals(codeString)) 1230 return ATTACHMENT; 1231 if ("BackboneElement".equals(codeString)) 1232 return BACKBONEELEMENT; 1233 if ("CodeableConcept".equals(codeString)) 1234 return CODEABLECONCEPT; 1235 if ("Coding".equals(codeString)) 1236 return CODING; 1237 if ("ContactDetail".equals(codeString)) 1238 return CONTACTDETAIL; 1239 if ("ContactPoint".equals(codeString)) 1240 return CONTACTPOINT; 1241 if ("Contributor".equals(codeString)) 1242 return CONTRIBUTOR; 1243 if ("Count".equals(codeString)) 1244 return COUNT; 1245 if ("DataRequirement".equals(codeString)) 1246 return DATAREQUIREMENT; 1247 if ("Distance".equals(codeString)) 1248 return DISTANCE; 1249 if ("Dosage".equals(codeString)) 1250 return DOSAGE; 1251 if ("Duration".equals(codeString)) 1252 return DURATION; 1253 if ("Element".equals(codeString)) 1254 return ELEMENT; 1255 if ("ElementDefinition".equals(codeString)) 1256 return ELEMENTDEFINITION; 1257 if ("Extension".equals(codeString)) 1258 return EXTENSION; 1259 if ("HumanName".equals(codeString)) 1260 return HUMANNAME; 1261 if ("Identifier".equals(codeString)) 1262 return IDENTIFIER; 1263 if ("Meta".equals(codeString)) 1264 return META; 1265 if ("Money".equals(codeString)) 1266 return MONEY; 1267 if ("Narrative".equals(codeString)) 1268 return NARRATIVE; 1269 if ("ParameterDefinition".equals(codeString)) 1270 return PARAMETERDEFINITION; 1271 if ("Period".equals(codeString)) 1272 return PERIOD; 1273 if ("Quantity".equals(codeString)) 1274 return QUANTITY; 1275 if ("Range".equals(codeString)) 1276 return RANGE; 1277 if ("Ratio".equals(codeString)) 1278 return RATIO; 1279 if ("Reference".equals(codeString)) 1280 return REFERENCE; 1281 if ("RelatedArtifact".equals(codeString)) 1282 return RELATEDARTIFACT; 1283 if ("SampledData".equals(codeString)) 1284 return SAMPLEDDATA; 1285 if ("Signature".equals(codeString)) 1286 return SIGNATURE; 1287 if ("SimpleQuantity".equals(codeString)) 1288 return SIMPLEQUANTITY; 1289 if ("Timing".equals(codeString)) 1290 return TIMING; 1291 if ("TriggerDefinition".equals(codeString)) 1292 return TRIGGERDEFINITION; 1293 if ("UsageContext".equals(codeString)) 1294 return USAGECONTEXT; 1295 if ("base64Binary".equals(codeString)) 1296 return BASE64BINARY; 1297 if ("boolean".equals(codeString)) 1298 return BOOLEAN; 1299 if ("code".equals(codeString)) 1300 return CODE; 1301 if ("date".equals(codeString)) 1302 return DATE; 1303 if ("dateTime".equals(codeString)) 1304 return DATETIME; 1305 if ("decimal".equals(codeString)) 1306 return DECIMAL; 1307 if ("id".equals(codeString)) 1308 return ID; 1309 if ("instant".equals(codeString)) 1310 return INSTANT; 1311 if ("integer".equals(codeString)) 1312 return INTEGER; 1313 if ("markdown".equals(codeString)) 1314 return MARKDOWN; 1315 if ("oid".equals(codeString)) 1316 return OID; 1317 if ("positiveInt".equals(codeString)) 1318 return POSITIVEINT; 1319 if ("string".equals(codeString)) 1320 return STRING; 1321 if ("time".equals(codeString)) 1322 return TIME; 1323 if ("unsignedInt".equals(codeString)) 1324 return UNSIGNEDINT; 1325 if ("uri".equals(codeString)) 1326 return URI; 1327 if ("uuid".equals(codeString)) 1328 return UUID; 1329 if ("xhtml".equals(codeString)) 1330 return XHTML; 1331 throw new FHIRException("Unknown DataType code '"+codeString+"'"); 1332 } 1333 public String toCode() { 1334 switch (this) { 1335 case ADDRESS: return "Address"; 1336 case AGE: return "Age"; 1337 case ANNOTATION: return "Annotation"; 1338 case ATTACHMENT: return "Attachment"; 1339 case BACKBONEELEMENT: return "BackboneElement"; 1340 case CODEABLECONCEPT: return "CodeableConcept"; 1341 case CODING: return "Coding"; 1342 case CONTACTDETAIL: return "ContactDetail"; 1343 case CONTACTPOINT: return "ContactPoint"; 1344 case CONTRIBUTOR: return "Contributor"; 1345 case COUNT: return "Count"; 1346 case DATAREQUIREMENT: return "DataRequirement"; 1347 case DISTANCE: return "Distance"; 1348 case DOSAGE: return "Dosage"; 1349 case DURATION: return "Duration"; 1350 case ELEMENT: return "Element"; 1351 case ELEMENTDEFINITION: return "ElementDefinition"; 1352 case EXTENSION: return "Extension"; 1353 case HUMANNAME: return "HumanName"; 1354 case IDENTIFIER: return "Identifier"; 1355 case META: return "Meta"; 1356 case MONEY: return "Money"; 1357 case NARRATIVE: return "Narrative"; 1358 case PARAMETERDEFINITION: return "ParameterDefinition"; 1359 case PERIOD: return "Period"; 1360 case QUANTITY: return "Quantity"; 1361 case RANGE: return "Range"; 1362 case RATIO: return "Ratio"; 1363 case REFERENCE: return "Reference"; 1364 case RELATEDARTIFACT: return "RelatedArtifact"; 1365 case SAMPLEDDATA: return "SampledData"; 1366 case SIGNATURE: return "Signature"; 1367 case SIMPLEQUANTITY: return "SimpleQuantity"; 1368 case TIMING: return "Timing"; 1369 case TRIGGERDEFINITION: return "TriggerDefinition"; 1370 case USAGECONTEXT: return "UsageContext"; 1371 case BASE64BINARY: return "base64Binary"; 1372 case BOOLEAN: return "boolean"; 1373 case CODE: return "code"; 1374 case DATE: return "date"; 1375 case DATETIME: return "dateTime"; 1376 case DECIMAL: return "decimal"; 1377 case ID: return "id"; 1378 case INSTANT: return "instant"; 1379 case INTEGER: return "integer"; 1380 case MARKDOWN: return "markdown"; 1381 case OID: return "oid"; 1382 case POSITIVEINT: return "positiveInt"; 1383 case STRING: return "string"; 1384 case TIME: return "time"; 1385 case UNSIGNEDINT: return "unsignedInt"; 1386 case URI: return "uri"; 1387 case UUID: return "uuid"; 1388 case XHTML: return "xhtml"; 1389 case NULL: return null; 1390 default: return "?"; 1391 } 1392 } 1393 public String getSystem() { 1394 switch (this) { 1395 case ADDRESS: return "http://hl7.org/fhir/data-types"; 1396 case AGE: return "http://hl7.org/fhir/data-types"; 1397 case ANNOTATION: return "http://hl7.org/fhir/data-types"; 1398 case ATTACHMENT: return "http://hl7.org/fhir/data-types"; 1399 case BACKBONEELEMENT: return "http://hl7.org/fhir/data-types"; 1400 case CODEABLECONCEPT: return "http://hl7.org/fhir/data-types"; 1401 case CODING: return "http://hl7.org/fhir/data-types"; 1402 case CONTACTDETAIL: return "http://hl7.org/fhir/data-types"; 1403 case CONTACTPOINT: return "http://hl7.org/fhir/data-types"; 1404 case CONTRIBUTOR: return "http://hl7.org/fhir/data-types"; 1405 case COUNT: return "http://hl7.org/fhir/data-types"; 1406 case DATAREQUIREMENT: return "http://hl7.org/fhir/data-types"; 1407 case DISTANCE: return "http://hl7.org/fhir/data-types"; 1408 case DOSAGE: return "http://hl7.org/fhir/data-types"; 1409 case DURATION: return "http://hl7.org/fhir/data-types"; 1410 case ELEMENT: return "http://hl7.org/fhir/data-types"; 1411 case ELEMENTDEFINITION: return "http://hl7.org/fhir/data-types"; 1412 case EXTENSION: return "http://hl7.org/fhir/data-types"; 1413 case HUMANNAME: return "http://hl7.org/fhir/data-types"; 1414 case IDENTIFIER: return "http://hl7.org/fhir/data-types"; 1415 case META: return "http://hl7.org/fhir/data-types"; 1416 case MONEY: return "http://hl7.org/fhir/data-types"; 1417 case NARRATIVE: return "http://hl7.org/fhir/data-types"; 1418 case PARAMETERDEFINITION: return "http://hl7.org/fhir/data-types"; 1419 case PERIOD: return "http://hl7.org/fhir/data-types"; 1420 case QUANTITY: return "http://hl7.org/fhir/data-types"; 1421 case RANGE: return "http://hl7.org/fhir/data-types"; 1422 case RATIO: return "http://hl7.org/fhir/data-types"; 1423 case REFERENCE: return "http://hl7.org/fhir/data-types"; 1424 case RELATEDARTIFACT: return "http://hl7.org/fhir/data-types"; 1425 case SAMPLEDDATA: return "http://hl7.org/fhir/data-types"; 1426 case SIGNATURE: return "http://hl7.org/fhir/data-types"; 1427 case SIMPLEQUANTITY: return "http://hl7.org/fhir/data-types"; 1428 case TIMING: return "http://hl7.org/fhir/data-types"; 1429 case TRIGGERDEFINITION: return "http://hl7.org/fhir/data-types"; 1430 case USAGECONTEXT: return "http://hl7.org/fhir/data-types"; 1431 case BASE64BINARY: return "http://hl7.org/fhir/data-types"; 1432 case BOOLEAN: return "http://hl7.org/fhir/data-types"; 1433 case CODE: return "http://hl7.org/fhir/data-types"; 1434 case DATE: return "http://hl7.org/fhir/data-types"; 1435 case DATETIME: return "http://hl7.org/fhir/data-types"; 1436 case DECIMAL: return "http://hl7.org/fhir/data-types"; 1437 case ID: return "http://hl7.org/fhir/data-types"; 1438 case INSTANT: return "http://hl7.org/fhir/data-types"; 1439 case INTEGER: return "http://hl7.org/fhir/data-types"; 1440 case MARKDOWN: return "http://hl7.org/fhir/data-types"; 1441 case OID: return "http://hl7.org/fhir/data-types"; 1442 case POSITIVEINT: return "http://hl7.org/fhir/data-types"; 1443 case STRING: return "http://hl7.org/fhir/data-types"; 1444 case TIME: return "http://hl7.org/fhir/data-types"; 1445 case UNSIGNEDINT: return "http://hl7.org/fhir/data-types"; 1446 case URI: return "http://hl7.org/fhir/data-types"; 1447 case UUID: return "http://hl7.org/fhir/data-types"; 1448 case XHTML: return "http://hl7.org/fhir/data-types"; 1449 case NULL: return null; 1450 default: return "?"; 1451 } 1452 } 1453 public String getDefinition() { 1454 switch (this) { 1455 case ADDRESS: return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 1456 case AGE: return "A duration of time during which an organism (or a process) has existed."; 1457 case ANNOTATION: return "A text note which also contains information about who made the statement and when."; 1458 case ATTACHMENT: return "For referring to data content defined in other formats."; 1459 case BACKBONEELEMENT: return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 1460 case CODEABLECONCEPT: return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 1461 case CODING: return "A reference to a code defined by a terminology system."; 1462 case CONTACTDETAIL: return "Specifies contact information for a person or organization."; 1463 case CONTACTPOINT: return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 1464 case CONTRIBUTOR: return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 1465 case COUNT: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 1466 case DATAREQUIREMENT: return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 1467 case DISTANCE: return "A length - a value with a unit that is a physical distance."; 1468 case DOSAGE: return "Indicates how the medication is/was taken or should be taken by the patient."; 1469 case DURATION: return "A length of time."; 1470 case ELEMENT: return "Base definition for all elements in a resource."; 1471 case ELEMENTDEFINITION: return "Captures constraints on each element within the resource, profile, or extension."; 1472 case EXTENSION: return "Optional Extension Element - found in all resources."; 1473 case HUMANNAME: return "A human's name with the ability to identify parts and usage."; 1474 case IDENTIFIER: return "A technical identifier - identifies some entity uniquely and unambiguously."; 1475 case META: return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource."; 1476 case MONEY: return "An amount of economic utility in some recognized currency."; 1477 case NARRATIVE: return "A human-readable formatted text, including images."; 1478 case PARAMETERDEFINITION: return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 1479 case PERIOD: return "A time period defined by a start and end date and optionally time."; 1480 case QUANTITY: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 1481 case RANGE: return "A set of ordered Quantities defined by a low and high limit."; 1482 case RATIO: return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 1483 case REFERENCE: return "A reference from one resource to another."; 1484 case RELATEDARTIFACT: return "Related artifacts such as additional documentation, justification, or bibliographic references."; 1485 case SAMPLEDDATA: return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 1486 case SIGNATURE: return "A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities."; 1487 case SIMPLEQUANTITY: return ""; 1488 case TIMING: return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 1489 case TRIGGERDEFINITION: return "A description of a triggering event."; 1490 case USAGECONTEXT: return "Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 1491 case BASE64BINARY: return "A stream of bytes"; 1492 case BOOLEAN: return "Value of \"true\" or \"false\""; 1493 case CODE: return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 1494 case DATE: return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 1495 case DATETIME: return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 1496 case DECIMAL: return "A rational number with implicit precision"; 1497 case ID: return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 1498 case INSTANT: return "An instant in time - known at least to the second"; 1499 case INTEGER: return "A whole number"; 1500 case MARKDOWN: return "A string that may contain markdown syntax for optional processing by a mark down presentation engine"; 1501 case OID: return "An OID represented as a URI"; 1502 case POSITIVEINT: return "An integer with a value that is positive (e.g. >0)"; 1503 case STRING: return "A sequence of Unicode characters"; 1504 case TIME: return "A time during the day, with no date specified"; 1505 case UNSIGNEDINT: return "An integer with a value that is not negative (e.g. >= 0)"; 1506 case URI: return "String of characters used to identify a name or a resource"; 1507 case UUID: return "A UUID, represented as a URI"; 1508 case XHTML: return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 1509 case NULL: return null; 1510 default: return "?"; 1511 } 1512 } 1513 public String getDisplay() { 1514 switch (this) { 1515 case ADDRESS: return "Address"; 1516 case AGE: return "Age"; 1517 case ANNOTATION: return "Annotation"; 1518 case ATTACHMENT: return "Attachment"; 1519 case BACKBONEELEMENT: return "BackboneElement"; 1520 case CODEABLECONCEPT: return "CodeableConcept"; 1521 case CODING: return "Coding"; 1522 case CONTACTDETAIL: return "ContactDetail"; 1523 case CONTACTPOINT: return "ContactPoint"; 1524 case CONTRIBUTOR: return "Contributor"; 1525 case COUNT: return "Count"; 1526 case DATAREQUIREMENT: return "DataRequirement"; 1527 case DISTANCE: return "Distance"; 1528 case DOSAGE: return "Dosage"; 1529 case DURATION: return "Duration"; 1530 case ELEMENT: return "Element"; 1531 case ELEMENTDEFINITION: return "ElementDefinition"; 1532 case EXTENSION: return "Extension"; 1533 case HUMANNAME: return "HumanName"; 1534 case IDENTIFIER: return "Identifier"; 1535 case META: return "Meta"; 1536 case MONEY: return "Money"; 1537 case NARRATIVE: return "Narrative"; 1538 case PARAMETERDEFINITION: return "ParameterDefinition"; 1539 case PERIOD: return "Period"; 1540 case QUANTITY: return "Quantity"; 1541 case RANGE: return "Range"; 1542 case RATIO: return "Ratio"; 1543 case REFERENCE: return "Reference"; 1544 case RELATEDARTIFACT: return "RelatedArtifact"; 1545 case SAMPLEDDATA: return "SampledData"; 1546 case SIGNATURE: return "Signature"; 1547 case SIMPLEQUANTITY: return "SimpleQuantity"; 1548 case TIMING: return "Timing"; 1549 case TRIGGERDEFINITION: return "TriggerDefinition"; 1550 case USAGECONTEXT: return "UsageContext"; 1551 case BASE64BINARY: return "base64Binary"; 1552 case BOOLEAN: return "boolean"; 1553 case CODE: return "code"; 1554 case DATE: return "date"; 1555 case DATETIME: return "dateTime"; 1556 case DECIMAL: return "decimal"; 1557 case ID: return "id"; 1558 case INSTANT: return "instant"; 1559 case INTEGER: return "integer"; 1560 case MARKDOWN: return "markdown"; 1561 case OID: return "oid"; 1562 case POSITIVEINT: return "positiveInt"; 1563 case STRING: return "string"; 1564 case TIME: return "time"; 1565 case UNSIGNEDINT: return "unsignedInt"; 1566 case URI: return "uri"; 1567 case UUID: return "uuid"; 1568 case XHTML: return "XHTML"; 1569 case NULL: return null; 1570 default: return "?"; 1571 } 1572 } 1573 } 1574 1575 public static class DataTypeEnumFactory implements EnumFactory<DataType> { 1576 public DataType fromCode(String codeString) throws IllegalArgumentException { 1577 if (codeString == null || "".equals(codeString)) 1578 if (codeString == null || "".equals(codeString)) 1579 return null; 1580 if ("Address".equals(codeString)) 1581 return DataType.ADDRESS; 1582 if ("Age".equals(codeString)) 1583 return DataType.AGE; 1584 if ("Annotation".equals(codeString)) 1585 return DataType.ANNOTATION; 1586 if ("Attachment".equals(codeString)) 1587 return DataType.ATTACHMENT; 1588 if ("BackboneElement".equals(codeString)) 1589 return DataType.BACKBONEELEMENT; 1590 if ("CodeableConcept".equals(codeString)) 1591 return DataType.CODEABLECONCEPT; 1592 if ("Coding".equals(codeString)) 1593 return DataType.CODING; 1594 if ("ContactDetail".equals(codeString)) 1595 return DataType.CONTACTDETAIL; 1596 if ("ContactPoint".equals(codeString)) 1597 return DataType.CONTACTPOINT; 1598 if ("Contributor".equals(codeString)) 1599 return DataType.CONTRIBUTOR; 1600 if ("Count".equals(codeString)) 1601 return DataType.COUNT; 1602 if ("DataRequirement".equals(codeString)) 1603 return DataType.DATAREQUIREMENT; 1604 if ("Distance".equals(codeString)) 1605 return DataType.DISTANCE; 1606 if ("Dosage".equals(codeString)) 1607 return DataType.DOSAGE; 1608 if ("Duration".equals(codeString)) 1609 return DataType.DURATION; 1610 if ("Element".equals(codeString)) 1611 return DataType.ELEMENT; 1612 if ("ElementDefinition".equals(codeString)) 1613 return DataType.ELEMENTDEFINITION; 1614 if ("Extension".equals(codeString)) 1615 return DataType.EXTENSION; 1616 if ("HumanName".equals(codeString)) 1617 return DataType.HUMANNAME; 1618 if ("Identifier".equals(codeString)) 1619 return DataType.IDENTIFIER; 1620 if ("Meta".equals(codeString)) 1621 return DataType.META; 1622 if ("Money".equals(codeString)) 1623 return DataType.MONEY; 1624 if ("Narrative".equals(codeString)) 1625 return DataType.NARRATIVE; 1626 if ("ParameterDefinition".equals(codeString)) 1627 return DataType.PARAMETERDEFINITION; 1628 if ("Period".equals(codeString)) 1629 return DataType.PERIOD; 1630 if ("Quantity".equals(codeString)) 1631 return DataType.QUANTITY; 1632 if ("Range".equals(codeString)) 1633 return DataType.RANGE; 1634 if ("Ratio".equals(codeString)) 1635 return DataType.RATIO; 1636 if ("Reference".equals(codeString)) 1637 return DataType.REFERENCE; 1638 if ("RelatedArtifact".equals(codeString)) 1639 return DataType.RELATEDARTIFACT; 1640 if ("SampledData".equals(codeString)) 1641 return DataType.SAMPLEDDATA; 1642 if ("Signature".equals(codeString)) 1643 return DataType.SIGNATURE; 1644 if ("SimpleQuantity".equals(codeString)) 1645 return DataType.SIMPLEQUANTITY; 1646 if ("Timing".equals(codeString)) 1647 return DataType.TIMING; 1648 if ("TriggerDefinition".equals(codeString)) 1649 return DataType.TRIGGERDEFINITION; 1650 if ("UsageContext".equals(codeString)) 1651 return DataType.USAGECONTEXT; 1652 if ("base64Binary".equals(codeString)) 1653 return DataType.BASE64BINARY; 1654 if ("boolean".equals(codeString)) 1655 return DataType.BOOLEAN; 1656 if ("code".equals(codeString)) 1657 return DataType.CODE; 1658 if ("date".equals(codeString)) 1659 return DataType.DATE; 1660 if ("dateTime".equals(codeString)) 1661 return DataType.DATETIME; 1662 if ("decimal".equals(codeString)) 1663 return DataType.DECIMAL; 1664 if ("id".equals(codeString)) 1665 return DataType.ID; 1666 if ("instant".equals(codeString)) 1667 return DataType.INSTANT; 1668 if ("integer".equals(codeString)) 1669 return DataType.INTEGER; 1670 if ("markdown".equals(codeString)) 1671 return DataType.MARKDOWN; 1672 if ("oid".equals(codeString)) 1673 return DataType.OID; 1674 if ("positiveInt".equals(codeString)) 1675 return DataType.POSITIVEINT; 1676 if ("string".equals(codeString)) 1677 return DataType.STRING; 1678 if ("time".equals(codeString)) 1679 return DataType.TIME; 1680 if ("unsignedInt".equals(codeString)) 1681 return DataType.UNSIGNEDINT; 1682 if ("uri".equals(codeString)) 1683 return DataType.URI; 1684 if ("uuid".equals(codeString)) 1685 return DataType.UUID; 1686 if ("xhtml".equals(codeString)) 1687 return DataType.XHTML; 1688 throw new IllegalArgumentException("Unknown DataType code '"+codeString+"'"); 1689 } 1690 public Enumeration<DataType> fromType(PrimitiveType<?> code) throws FHIRException { 1691 if (code == null) 1692 return null; 1693 if (code.isEmpty()) 1694 return new Enumeration<DataType>(this); 1695 String codeString = code.asStringValue(); 1696 if (codeString == null || "".equals(codeString)) 1697 return null; 1698 if ("Address".equals(codeString)) 1699 return new Enumeration<DataType>(this, DataType.ADDRESS); 1700 if ("Age".equals(codeString)) 1701 return new Enumeration<DataType>(this, DataType.AGE); 1702 if ("Annotation".equals(codeString)) 1703 return new Enumeration<DataType>(this, DataType.ANNOTATION); 1704 if ("Attachment".equals(codeString)) 1705 return new Enumeration<DataType>(this, DataType.ATTACHMENT); 1706 if ("BackboneElement".equals(codeString)) 1707 return new Enumeration<DataType>(this, DataType.BACKBONEELEMENT); 1708 if ("CodeableConcept".equals(codeString)) 1709 return new Enumeration<DataType>(this, DataType.CODEABLECONCEPT); 1710 if ("Coding".equals(codeString)) 1711 return new Enumeration<DataType>(this, DataType.CODING); 1712 if ("ContactDetail".equals(codeString)) 1713 return new Enumeration<DataType>(this, DataType.CONTACTDETAIL); 1714 if ("ContactPoint".equals(codeString)) 1715 return new Enumeration<DataType>(this, DataType.CONTACTPOINT); 1716 if ("Contributor".equals(codeString)) 1717 return new Enumeration<DataType>(this, DataType.CONTRIBUTOR); 1718 if ("Count".equals(codeString)) 1719 return new Enumeration<DataType>(this, DataType.COUNT); 1720 if ("DataRequirement".equals(codeString)) 1721 return new Enumeration<DataType>(this, DataType.DATAREQUIREMENT); 1722 if ("Distance".equals(codeString)) 1723 return new Enumeration<DataType>(this, DataType.DISTANCE); 1724 if ("Dosage".equals(codeString)) 1725 return new Enumeration<DataType>(this, DataType.DOSAGE); 1726 if ("Duration".equals(codeString)) 1727 return new Enumeration<DataType>(this, DataType.DURATION); 1728 if ("Element".equals(codeString)) 1729 return new Enumeration<DataType>(this, DataType.ELEMENT); 1730 if ("ElementDefinition".equals(codeString)) 1731 return new Enumeration<DataType>(this, DataType.ELEMENTDEFINITION); 1732 if ("Extension".equals(codeString)) 1733 return new Enumeration<DataType>(this, DataType.EXTENSION); 1734 if ("HumanName".equals(codeString)) 1735 return new Enumeration<DataType>(this, DataType.HUMANNAME); 1736 if ("Identifier".equals(codeString)) 1737 return new Enumeration<DataType>(this, DataType.IDENTIFIER); 1738 if ("Meta".equals(codeString)) 1739 return new Enumeration<DataType>(this, DataType.META); 1740 if ("Money".equals(codeString)) 1741 return new Enumeration<DataType>(this, DataType.MONEY); 1742 if ("Narrative".equals(codeString)) 1743 return new Enumeration<DataType>(this, DataType.NARRATIVE); 1744 if ("ParameterDefinition".equals(codeString)) 1745 return new Enumeration<DataType>(this, DataType.PARAMETERDEFINITION); 1746 if ("Period".equals(codeString)) 1747 return new Enumeration<DataType>(this, DataType.PERIOD); 1748 if ("Quantity".equals(codeString)) 1749 return new Enumeration<DataType>(this, DataType.QUANTITY); 1750 if ("Range".equals(codeString)) 1751 return new Enumeration<DataType>(this, DataType.RANGE); 1752 if ("Ratio".equals(codeString)) 1753 return new Enumeration<DataType>(this, DataType.RATIO); 1754 if ("Reference".equals(codeString)) 1755 return new Enumeration<DataType>(this, DataType.REFERENCE); 1756 if ("RelatedArtifact".equals(codeString)) 1757 return new Enumeration<DataType>(this, DataType.RELATEDARTIFACT); 1758 if ("SampledData".equals(codeString)) 1759 return new Enumeration<DataType>(this, DataType.SAMPLEDDATA); 1760 if ("Signature".equals(codeString)) 1761 return new Enumeration<DataType>(this, DataType.SIGNATURE); 1762 if ("SimpleQuantity".equals(codeString)) 1763 return new Enumeration<DataType>(this, DataType.SIMPLEQUANTITY); 1764 if ("Timing".equals(codeString)) 1765 return new Enumeration<DataType>(this, DataType.TIMING); 1766 if ("TriggerDefinition".equals(codeString)) 1767 return new Enumeration<DataType>(this, DataType.TRIGGERDEFINITION); 1768 if ("UsageContext".equals(codeString)) 1769 return new Enumeration<DataType>(this, DataType.USAGECONTEXT); 1770 if ("base64Binary".equals(codeString)) 1771 return new Enumeration<DataType>(this, DataType.BASE64BINARY); 1772 if ("boolean".equals(codeString)) 1773 return new Enumeration<DataType>(this, DataType.BOOLEAN); 1774 if ("code".equals(codeString)) 1775 return new Enumeration<DataType>(this, DataType.CODE); 1776 if ("date".equals(codeString)) 1777 return new Enumeration<DataType>(this, DataType.DATE); 1778 if ("dateTime".equals(codeString)) 1779 return new Enumeration<DataType>(this, DataType.DATETIME); 1780 if ("decimal".equals(codeString)) 1781 return new Enumeration<DataType>(this, DataType.DECIMAL); 1782 if ("id".equals(codeString)) 1783 return new Enumeration<DataType>(this, DataType.ID); 1784 if ("instant".equals(codeString)) 1785 return new Enumeration<DataType>(this, DataType.INSTANT); 1786 if ("integer".equals(codeString)) 1787 return new Enumeration<DataType>(this, DataType.INTEGER); 1788 if ("markdown".equals(codeString)) 1789 return new Enumeration<DataType>(this, DataType.MARKDOWN); 1790 if ("oid".equals(codeString)) 1791 return new Enumeration<DataType>(this, DataType.OID); 1792 if ("positiveInt".equals(codeString)) 1793 return new Enumeration<DataType>(this, DataType.POSITIVEINT); 1794 if ("string".equals(codeString)) 1795 return new Enumeration<DataType>(this, DataType.STRING); 1796 if ("time".equals(codeString)) 1797 return new Enumeration<DataType>(this, DataType.TIME); 1798 if ("unsignedInt".equals(codeString)) 1799 return new Enumeration<DataType>(this, DataType.UNSIGNEDINT); 1800 if ("uri".equals(codeString)) 1801 return new Enumeration<DataType>(this, DataType.URI); 1802 if ("uuid".equals(codeString)) 1803 return new Enumeration<DataType>(this, DataType.UUID); 1804 if ("xhtml".equals(codeString)) 1805 return new Enumeration<DataType>(this, DataType.XHTML); 1806 throw new FHIRException("Unknown DataType code '"+codeString+"'"); 1807 } 1808 public String toCode(DataType code) { 1809 if (code == DataType.ADDRESS) 1810 return "Address"; 1811 if (code == DataType.AGE) 1812 return "Age"; 1813 if (code == DataType.ANNOTATION) 1814 return "Annotation"; 1815 if (code == DataType.ATTACHMENT) 1816 return "Attachment"; 1817 if (code == DataType.BACKBONEELEMENT) 1818 return "BackboneElement"; 1819 if (code == DataType.CODEABLECONCEPT) 1820 return "CodeableConcept"; 1821 if (code == DataType.CODING) 1822 return "Coding"; 1823 if (code == DataType.CONTACTDETAIL) 1824 return "ContactDetail"; 1825 if (code == DataType.CONTACTPOINT) 1826 return "ContactPoint"; 1827 if (code == DataType.CONTRIBUTOR) 1828 return "Contributor"; 1829 if (code == DataType.COUNT) 1830 return "Count"; 1831 if (code == DataType.DATAREQUIREMENT) 1832 return "DataRequirement"; 1833 if (code == DataType.DISTANCE) 1834 return "Distance"; 1835 if (code == DataType.DOSAGE) 1836 return "Dosage"; 1837 if (code == DataType.DURATION) 1838 return "Duration"; 1839 if (code == DataType.ELEMENT) 1840 return "Element"; 1841 if (code == DataType.ELEMENTDEFINITION) 1842 return "ElementDefinition"; 1843 if (code == DataType.EXTENSION) 1844 return "Extension"; 1845 if (code == DataType.HUMANNAME) 1846 return "HumanName"; 1847 if (code == DataType.IDENTIFIER) 1848 return "Identifier"; 1849 if (code == DataType.META) 1850 return "Meta"; 1851 if (code == DataType.MONEY) 1852 return "Money"; 1853 if (code == DataType.NARRATIVE) 1854 return "Narrative"; 1855 if (code == DataType.PARAMETERDEFINITION) 1856 return "ParameterDefinition"; 1857 if (code == DataType.PERIOD) 1858 return "Period"; 1859 if (code == DataType.QUANTITY) 1860 return "Quantity"; 1861 if (code == DataType.RANGE) 1862 return "Range"; 1863 if (code == DataType.RATIO) 1864 return "Ratio"; 1865 if (code == DataType.REFERENCE) 1866 return "Reference"; 1867 if (code == DataType.RELATEDARTIFACT) 1868 return "RelatedArtifact"; 1869 if (code == DataType.SAMPLEDDATA) 1870 return "SampledData"; 1871 if (code == DataType.SIGNATURE) 1872 return "Signature"; 1873 if (code == DataType.SIMPLEQUANTITY) 1874 return "SimpleQuantity"; 1875 if (code == DataType.TIMING) 1876 return "Timing"; 1877 if (code == DataType.TRIGGERDEFINITION) 1878 return "TriggerDefinition"; 1879 if (code == DataType.USAGECONTEXT) 1880 return "UsageContext"; 1881 if (code == DataType.BASE64BINARY) 1882 return "base64Binary"; 1883 if (code == DataType.BOOLEAN) 1884 return "boolean"; 1885 if (code == DataType.CODE) 1886 return "code"; 1887 if (code == DataType.DATE) 1888 return "date"; 1889 if (code == DataType.DATETIME) 1890 return "dateTime"; 1891 if (code == DataType.DECIMAL) 1892 return "decimal"; 1893 if (code == DataType.ID) 1894 return "id"; 1895 if (code == DataType.INSTANT) 1896 return "instant"; 1897 if (code == DataType.INTEGER) 1898 return "integer"; 1899 if (code == DataType.MARKDOWN) 1900 return "markdown"; 1901 if (code == DataType.OID) 1902 return "oid"; 1903 if (code == DataType.POSITIVEINT) 1904 return "positiveInt"; 1905 if (code == DataType.STRING) 1906 return "string"; 1907 if (code == DataType.TIME) 1908 return "time"; 1909 if (code == DataType.UNSIGNEDINT) 1910 return "unsignedInt"; 1911 if (code == DataType.URI) 1912 return "uri"; 1913 if (code == DataType.UUID) 1914 return "uuid"; 1915 if (code == DataType.XHTML) 1916 return "xhtml"; 1917 return "?"; 1918 } 1919 public String toSystem(DataType code) { 1920 return code.getSystem(); 1921 } 1922 } 1923 1924 public enum DocumentReferenceStatus { 1925 /** 1926 * This is the current reference for this document. 1927 */ 1928 CURRENT, 1929 /** 1930 * This reference has been superseded by another reference. 1931 */ 1932 SUPERSEDED, 1933 /** 1934 * This reference was created in error. 1935 */ 1936 ENTEREDINERROR, 1937 /** 1938 * added to help the parsers 1939 */ 1940 NULL; 1941 public static DocumentReferenceStatus fromCode(String codeString) throws FHIRException { 1942 if (codeString == null || "".equals(codeString)) 1943 return null; 1944 if ("current".equals(codeString)) 1945 return CURRENT; 1946 if ("superseded".equals(codeString)) 1947 return SUPERSEDED; 1948 if ("entered-in-error".equals(codeString)) 1949 return ENTEREDINERROR; 1950 throw new FHIRException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 1951 } 1952 public String toCode() { 1953 switch (this) { 1954 case CURRENT: return "current"; 1955 case SUPERSEDED: return "superseded"; 1956 case ENTEREDINERROR: return "entered-in-error"; 1957 case NULL: return null; 1958 default: return "?"; 1959 } 1960 } 1961 public String getSystem() { 1962 switch (this) { 1963 case CURRENT: return "http://hl7.org/fhir/document-reference-status"; 1964 case SUPERSEDED: return "http://hl7.org/fhir/document-reference-status"; 1965 case ENTEREDINERROR: return "http://hl7.org/fhir/document-reference-status"; 1966 case NULL: return null; 1967 default: return "?"; 1968 } 1969 } 1970 public String getDefinition() { 1971 switch (this) { 1972 case CURRENT: return "This is the current reference for this document."; 1973 case SUPERSEDED: return "This reference has been superseded by another reference."; 1974 case ENTEREDINERROR: return "This reference was created in error."; 1975 case NULL: return null; 1976 default: return "?"; 1977 } 1978 } 1979 public String getDisplay() { 1980 switch (this) { 1981 case CURRENT: return "Current"; 1982 case SUPERSEDED: return "Superseded"; 1983 case ENTEREDINERROR: return "Entered in Error"; 1984 case NULL: return null; 1985 default: return "?"; 1986 } 1987 } 1988 } 1989 1990 public static class DocumentReferenceStatusEnumFactory implements EnumFactory<DocumentReferenceStatus> { 1991 public DocumentReferenceStatus fromCode(String codeString) throws IllegalArgumentException { 1992 if (codeString == null || "".equals(codeString)) 1993 if (codeString == null || "".equals(codeString)) 1994 return null; 1995 if ("current".equals(codeString)) 1996 return DocumentReferenceStatus.CURRENT; 1997 if ("superseded".equals(codeString)) 1998 return DocumentReferenceStatus.SUPERSEDED; 1999 if ("entered-in-error".equals(codeString)) 2000 return DocumentReferenceStatus.ENTEREDINERROR; 2001 throw new IllegalArgumentException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 2002 } 2003 public Enumeration<DocumentReferenceStatus> fromType(PrimitiveType<?> code) throws FHIRException { 2004 if (code == null) 2005 return null; 2006 if (code.isEmpty()) 2007 return new Enumeration<DocumentReferenceStatus>(this); 2008 String codeString = code.asStringValue(); 2009 if (codeString == null || "".equals(codeString)) 2010 return null; 2011 if ("current".equals(codeString)) 2012 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.CURRENT); 2013 if ("superseded".equals(codeString)) 2014 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.SUPERSEDED); 2015 if ("entered-in-error".equals(codeString)) 2016 return new Enumeration<DocumentReferenceStatus>(this, DocumentReferenceStatus.ENTEREDINERROR); 2017 throw new FHIRException("Unknown DocumentReferenceStatus code '"+codeString+"'"); 2018 } 2019 public String toCode(DocumentReferenceStatus code) { 2020 if (code == DocumentReferenceStatus.CURRENT) 2021 return "current"; 2022 if (code == DocumentReferenceStatus.SUPERSEDED) 2023 return "superseded"; 2024 if (code == DocumentReferenceStatus.ENTEREDINERROR) 2025 return "entered-in-error"; 2026 return "?"; 2027 } 2028 public String toSystem(DocumentReferenceStatus code) { 2029 return code.getSystem(); 2030 } 2031 } 2032 2033 public enum FHIRAllTypes { 2034 /** 2035 * An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world. 2036 */ 2037 ADDRESS, 2038 /** 2039 * A duration of time during which an organism (or a process) has existed. 2040 */ 2041 AGE, 2042 /** 2043 * A text note which also contains information about who made the statement and when. 2044 */ 2045 ANNOTATION, 2046 /** 2047 * For referring to data content defined in other formats. 2048 */ 2049 ATTACHMENT, 2050 /** 2051 * Base definition for all elements that are defined inside a resource - but not those in a data type. 2052 */ 2053 BACKBONEELEMENT, 2054 /** 2055 * A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text. 2056 */ 2057 CODEABLECONCEPT, 2058 /** 2059 * A reference to a code defined by a terminology system. 2060 */ 2061 CODING, 2062 /** 2063 * Specifies contact information for a person or organization. 2064 */ 2065 CONTACTDETAIL, 2066 /** 2067 * Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 2068 */ 2069 CONTACTPOINT, 2070 /** 2071 * A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers. 2072 */ 2073 CONTRIBUTOR, 2074 /** 2075 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 2076 */ 2077 COUNT, 2078 /** 2079 * Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data. 2080 */ 2081 DATAREQUIREMENT, 2082 /** 2083 * A length - a value with a unit that is a physical distance. 2084 */ 2085 DISTANCE, 2086 /** 2087 * Indicates how the medication is/was taken or should be taken by the patient. 2088 */ 2089 DOSAGE, 2090 /** 2091 * A length of time. 2092 */ 2093 DURATION, 2094 /** 2095 * Base definition for all elements in a resource. 2096 */ 2097 ELEMENT, 2098 /** 2099 * Captures constraints on each element within the resource, profile, or extension. 2100 */ 2101 ELEMENTDEFINITION, 2102 /** 2103 * Optional Extension Element - found in all resources. 2104 */ 2105 EXTENSION, 2106 /** 2107 * A human's name with the ability to identify parts and usage. 2108 */ 2109 HUMANNAME, 2110 /** 2111 * A technical identifier - identifies some entity uniquely and unambiguously. 2112 */ 2113 IDENTIFIER, 2114 /** 2115 * The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource. 2116 */ 2117 META, 2118 /** 2119 * An amount of economic utility in some recognized currency. 2120 */ 2121 MONEY, 2122 /** 2123 * A human-readable formatted text, including images. 2124 */ 2125 NARRATIVE, 2126 /** 2127 * The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse. 2128 */ 2129 PARAMETERDEFINITION, 2130 /** 2131 * A time period defined by a start and end date and optionally time. 2132 */ 2133 PERIOD, 2134 /** 2135 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 2136 */ 2137 QUANTITY, 2138 /** 2139 * A set of ordered Quantities defined by a low and high limit. 2140 */ 2141 RANGE, 2142 /** 2143 * A relationship of two Quantity values - expressed as a numerator and a denominator. 2144 */ 2145 RATIO, 2146 /** 2147 * A reference from one resource to another. 2148 */ 2149 REFERENCE, 2150 /** 2151 * Related artifacts such as additional documentation, justification, or bibliographic references. 2152 */ 2153 RELATEDARTIFACT, 2154 /** 2155 * A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data. 2156 */ 2157 SAMPLEDDATA, 2158 /** 2159 * A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities. 2160 */ 2161 SIGNATURE, 2162 /** 2163 * null 2164 */ 2165 SIMPLEQUANTITY, 2166 /** 2167 * Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out. 2168 */ 2169 TIMING, 2170 /** 2171 * A description of a triggering event. 2172 */ 2173 TRIGGERDEFINITION, 2174 /** 2175 * Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care). 2176 */ 2177 USAGECONTEXT, 2178 /** 2179 * A stream of bytes 2180 */ 2181 BASE64BINARY, 2182 /** 2183 * Value of "true" or "false" 2184 */ 2185 BOOLEAN, 2186 /** 2187 * A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents 2188 */ 2189 CODE, 2190 /** 2191 * A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates. 2192 */ 2193 DATE, 2194 /** 2195 * A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates. 2196 */ 2197 DATETIME, 2198 /** 2199 * A rational number with implicit precision 2200 */ 2201 DECIMAL, 2202 /** 2203 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive. 2204 */ 2205 ID, 2206 /** 2207 * An instant in time - known at least to the second 2208 */ 2209 INSTANT, 2210 /** 2211 * A whole number 2212 */ 2213 INTEGER, 2214 /** 2215 * A string that may contain markdown syntax for optional processing by a mark down presentation engine 2216 */ 2217 MARKDOWN, 2218 /** 2219 * An OID represented as a URI 2220 */ 2221 OID, 2222 /** 2223 * An integer with a value that is positive (e.g. >0) 2224 */ 2225 POSITIVEINT, 2226 /** 2227 * A sequence of Unicode characters 2228 */ 2229 STRING, 2230 /** 2231 * A time during the day, with no date specified 2232 */ 2233 TIME, 2234 /** 2235 * An integer with a value that is not negative (e.g. >= 0) 2236 */ 2237 UNSIGNEDINT, 2238 /** 2239 * String of characters used to identify a name or a resource 2240 */ 2241 URI, 2242 /** 2243 * A UUID, represented as a URI 2244 */ 2245 UUID, 2246 /** 2247 * XHTML format, as defined by W3C, but restricted usage (mainly, no active content) 2248 */ 2249 XHTML, 2250 /** 2251 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 2252 */ 2253 ACCOUNT, 2254 /** 2255 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 2256 */ 2257 ACTIVITYDEFINITION, 2258 /** 2259 * Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death. 2260 */ 2261 ADVERSEEVENT, 2262 /** 2263 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 2264 */ 2265 ALLERGYINTOLERANCE, 2266 /** 2267 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 2268 */ 2269 APPOINTMENT, 2270 /** 2271 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 2272 */ 2273 APPOINTMENTRESPONSE, 2274 /** 2275 * A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage. 2276 */ 2277 AUDITEVENT, 2278 /** 2279 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 2280 */ 2281 BASIC, 2282 /** 2283 * A binary resource can contain any content, whether text, image, pdf, zip archive, etc. 2284 */ 2285 BINARY, 2286 /** 2287 * Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 2288 */ 2289 BODYSITE, 2290 /** 2291 * A container for a collection of resources. 2292 */ 2293 BUNDLE, 2294 /** 2295 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 2296 */ 2297 CAPABILITYSTATEMENT, 2298 /** 2299 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 2300 */ 2301 CAREPLAN, 2302 /** 2303 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 2304 */ 2305 CARETEAM, 2306 /** 2307 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 2308 */ 2309 CHARGEITEM, 2310 /** 2311 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 2312 */ 2313 CLAIM, 2314 /** 2315 * This resource provides the adjudication details from the processing of a Claim resource. 2316 */ 2317 CLAIMRESPONSE, 2318 /** 2319 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 2320 */ 2321 CLINICALIMPRESSION, 2322 /** 2323 * A code system resource specifies a set of codes drawn from one or more code systems. 2324 */ 2325 CODESYSTEM, 2326 /** 2327 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 2328 */ 2329 COMMUNICATION, 2330 /** 2331 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 2332 */ 2333 COMMUNICATIONREQUEST, 2334 /** 2335 * A compartment definition that defines how resources are accessed on a server. 2336 */ 2337 COMPARTMENTDEFINITION, 2338 /** 2339 * A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained. 2340 */ 2341 COMPOSITION, 2342 /** 2343 * A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models. 2344 */ 2345 CONCEPTMAP, 2346 /** 2347 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 2348 */ 2349 CONDITION, 2350 /** 2351 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 2352 */ 2353 CONSENT, 2354 /** 2355 * A formal agreement between parties regarding the conduct of business, exchange of information or other matters. 2356 */ 2357 CONTRACT, 2358 /** 2359 * Financial instrument which may be used to reimburse or pay for health care products and services. 2360 */ 2361 COVERAGE, 2362 /** 2363 * The formal description of a single piece of information that can be gathered and reported. 2364 */ 2365 DATAELEMENT, 2366 /** 2367 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 2368 */ 2369 DETECTEDISSUE, 2370 /** 2371 * This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc. 2372 */ 2373 DEVICE, 2374 /** 2375 * The characteristics, operational status and capabilities of a medical-related component of a medical device. 2376 */ 2377 DEVICECOMPONENT, 2378 /** 2379 * Describes a measurement, calculation or setting capability of a medical device. 2380 */ 2381 DEVICEMETRIC, 2382 /** 2383 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 2384 */ 2385 DEVICEREQUEST, 2386 /** 2387 * A record of a device being used by a patient where the record is the result of a report from the patient or another clinician. 2388 */ 2389 DEVICEUSESTATEMENT, 2390 /** 2391 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 2392 */ 2393 DIAGNOSTICREPORT, 2394 /** 2395 * A collection of documents compiled for a purpose together with metadata that applies to the collection. 2396 */ 2397 DOCUMENTMANIFEST, 2398 /** 2399 * A reference to a document. 2400 */ 2401 DOCUMENTREFERENCE, 2402 /** 2403 * A resource that includes narrative, extensions, and contained resources. 2404 */ 2405 DOMAINRESOURCE, 2406 /** 2407 * The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 2408 */ 2409 ELIGIBILITYREQUEST, 2410 /** 2411 * This resource provides eligibility and plan details from the processing of an Eligibility resource. 2412 */ 2413 ELIGIBILITYRESPONSE, 2414 /** 2415 * An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient. 2416 */ 2417 ENCOUNTER, 2418 /** 2419 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 2420 */ 2421 ENDPOINT, 2422 /** 2423 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 2424 */ 2425 ENROLLMENTREQUEST, 2426 /** 2427 * This resource provides enrollment and plan details from the processing of an Enrollment resource. 2428 */ 2429 ENROLLMENTRESPONSE, 2430 /** 2431 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 2432 */ 2433 EPISODEOFCARE, 2434 /** 2435 * Resource to define constraints on the Expansion of a FHIR ValueSet. 2436 */ 2437 EXPANSIONPROFILE, 2438 /** 2439 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 2440 */ 2441 EXPLANATIONOFBENEFIT, 2442 /** 2443 * Significant health events and conditions for a person related to the patient relevant in the context of care for the patient. 2444 */ 2445 FAMILYMEMBERHISTORY, 2446 /** 2447 * Prospective warnings of potential issues when providing care to the patient. 2448 */ 2449 FLAG, 2450 /** 2451 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 2452 */ 2453 GOAL, 2454 /** 2455 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 2456 */ 2457 GRAPHDEFINITION, 2458 /** 2459 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 2460 */ 2461 GROUP, 2462 /** 2463 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 2464 */ 2465 GUIDANCERESPONSE, 2466 /** 2467 * The details of a healthcare service available at a location. 2468 */ 2469 HEALTHCARESERVICE, 2470 /** 2471 * A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection. 2472 */ 2473 IMAGINGMANIFEST, 2474 /** 2475 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 2476 */ 2477 IMAGINGSTUDY, 2478 /** 2479 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 2480 */ 2481 IMMUNIZATION, 2482 /** 2483 * A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification. 2484 */ 2485 IMMUNIZATIONRECOMMENDATION, 2486 /** 2487 * A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 2488 */ 2489 IMPLEMENTATIONGUIDE, 2490 /** 2491 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 2492 */ 2493 LIBRARY, 2494 /** 2495 * Identifies two or more records (resource instances) that are referring to the same real-world "occurrence". 2496 */ 2497 LINKAGE, 2498 /** 2499 * A set of information summarized from a list of other resources. 2500 */ 2501 LIST, 2502 /** 2503 * Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated. 2504 */ 2505 LOCATION, 2506 /** 2507 * The Measure resource provides the definition of a quality measure. 2508 */ 2509 MEASURE, 2510 /** 2511 * The MeasureReport resource contains the results of evaluating a measure. 2512 */ 2513 MEASUREREPORT, 2514 /** 2515 * A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference. 2516 */ 2517 MEDIA, 2518 /** 2519 * This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication. 2520 */ 2521 MEDICATION, 2522 /** 2523 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. 2524 */ 2525 MEDICATIONADMINISTRATION, 2526 /** 2527 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 2528 */ 2529 MEDICATIONDISPENSE, 2530 /** 2531 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 2532 */ 2533 MEDICATIONREQUEST, 2534 /** 2535 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 2536 2537The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 2538 */ 2539 MEDICATIONSTATEMENT, 2540 /** 2541 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 2542 */ 2543 MESSAGEDEFINITION, 2544 /** 2545 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 2546 */ 2547 MESSAGEHEADER, 2548 /** 2549 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 2550 */ 2551 NAMINGSYSTEM, 2552 /** 2553 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 2554 */ 2555 NUTRITIONORDER, 2556 /** 2557 * Measurements and simple assertions made about a patient, device or other subject. 2558 */ 2559 OBSERVATION, 2560 /** 2561 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 2562 */ 2563 OPERATIONDEFINITION, 2564 /** 2565 * A collection of error, warning or information messages that result from a system action. 2566 */ 2567 OPERATIONOUTCOME, 2568 /** 2569 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc. 2570 */ 2571 ORGANIZATION, 2572 /** 2573 * This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it. 2574 */ 2575 PARAMETERS, 2576 /** 2577 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 2578 */ 2579 PATIENT, 2580 /** 2581 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 2582 */ 2583 PAYMENTNOTICE, 2584 /** 2585 * This resource provides payment details and claim references supporting a bulk payment. 2586 */ 2587 PAYMENTRECONCILIATION, 2588 /** 2589 * Demographics and administrative information about a person independent of a specific health-related context. 2590 */ 2591 PERSON, 2592 /** 2593 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols. 2594 */ 2595 PLANDEFINITION, 2596 /** 2597 * A person who is directly or indirectly involved in the provisioning of healthcare. 2598 */ 2599 PRACTITIONER, 2600 /** 2601 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 2602 */ 2603 PRACTITIONERROLE, 2604 /** 2605 * An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy. 2606 */ 2607 PROCEDURE, 2608 /** 2609 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 2610 */ 2611 PROCEDUREREQUEST, 2612 /** 2613 * This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources. 2614 */ 2615 PROCESSREQUEST, 2616 /** 2617 * This resource provides processing status, errors and notes from the processing of a resource. 2618 */ 2619 PROCESSRESPONSE, 2620 /** 2621 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 2622 */ 2623 PROVENANCE, 2624 /** 2625 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 2626 */ 2627 QUESTIONNAIRE, 2628 /** 2629 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 2630 */ 2631 QUESTIONNAIRERESPONSE, 2632 /** 2633 * Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization. 2634 */ 2635 REFERRALREQUEST, 2636 /** 2637 * Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 2638 */ 2639 RELATEDPERSON, 2640 /** 2641 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 2642 */ 2643 REQUESTGROUP, 2644 /** 2645 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 2646 */ 2647 RESEARCHSTUDY, 2648 /** 2649 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 2650 */ 2651 RESEARCHSUBJECT, 2652 /** 2653 * This is the base resource type for everything. 2654 */ 2655 RESOURCE, 2656 /** 2657 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 2658 */ 2659 RISKASSESSMENT, 2660 /** 2661 * A container for slots of time that may be available for booking appointments. 2662 */ 2663 SCHEDULE, 2664 /** 2665 * A search parameter that defines a named search item that can be used to search/filter on a resource. 2666 */ 2667 SEARCHPARAMETER, 2668 /** 2669 * Raw data describing a biological sequence. 2670 */ 2671 SEQUENCE, 2672 /** 2673 * The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking. 2674 */ 2675 SERVICEDEFINITION, 2676 /** 2677 * A slot of time on a schedule that may be available for booking appointments. 2678 */ 2679 SLOT, 2680 /** 2681 * A sample to be used for analysis. 2682 */ 2683 SPECIMEN, 2684 /** 2685 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 2686 */ 2687 STRUCTUREDEFINITION, 2688 /** 2689 * A Map of relationships between 2 structures that can be used to transform data. 2690 */ 2691 STRUCTUREMAP, 2692 /** 2693 * The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined "channel" so that another system is able to take an appropriate action. 2694 */ 2695 SUBSCRIPTION, 2696 /** 2697 * A homogeneous material with a definite composition. 2698 */ 2699 SUBSTANCE, 2700 /** 2701 * Record of delivery of what is supplied. 2702 */ 2703 SUPPLYDELIVERY, 2704 /** 2705 * A record of a request for a medication, substance or device used in the healthcare setting. 2706 */ 2707 SUPPLYREQUEST, 2708 /** 2709 * A task to be performed. 2710 */ 2711 TASK, 2712 /** 2713 * A summary of information based on the results of executing a TestScript. 2714 */ 2715 TESTREPORT, 2716 /** 2717 * A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification. 2718 */ 2719 TESTSCRIPT, 2720 /** 2721 * A value set specifies a set of codes drawn from one or more code systems. 2722 */ 2723 VALUESET, 2724 /** 2725 * An authorization for the supply of glasses and/or contact lenses to a patient. 2726 */ 2727 VISIONPRESCRIPTION, 2728 /** 2729 * A place holder that means any kind of data type 2730 */ 2731 TYPE, 2732 /** 2733 * A place holder that means any kind of resource 2734 */ 2735 ANY, 2736 /** 2737 * added to help the parsers 2738 */ 2739 NULL; 2740 public static FHIRAllTypes fromCode(String codeString) throws FHIRException { 2741 if (codeString == null || "".equals(codeString)) 2742 return null; 2743 if ("Address".equals(codeString)) 2744 return ADDRESS; 2745 if ("Age".equals(codeString)) 2746 return AGE; 2747 if ("Annotation".equals(codeString)) 2748 return ANNOTATION; 2749 if ("Attachment".equals(codeString)) 2750 return ATTACHMENT; 2751 if ("BackboneElement".equals(codeString)) 2752 return BACKBONEELEMENT; 2753 if ("CodeableConcept".equals(codeString)) 2754 return CODEABLECONCEPT; 2755 if ("Coding".equals(codeString)) 2756 return CODING; 2757 if ("ContactDetail".equals(codeString)) 2758 return CONTACTDETAIL; 2759 if ("ContactPoint".equals(codeString)) 2760 return CONTACTPOINT; 2761 if ("Contributor".equals(codeString)) 2762 return CONTRIBUTOR; 2763 if ("Count".equals(codeString)) 2764 return COUNT; 2765 if ("DataRequirement".equals(codeString)) 2766 return DATAREQUIREMENT; 2767 if ("Distance".equals(codeString)) 2768 return DISTANCE; 2769 if ("Dosage".equals(codeString)) 2770 return DOSAGE; 2771 if ("Duration".equals(codeString)) 2772 return DURATION; 2773 if ("Element".equals(codeString)) 2774 return ELEMENT; 2775 if ("ElementDefinition".equals(codeString)) 2776 return ELEMENTDEFINITION; 2777 if ("Extension".equals(codeString)) 2778 return EXTENSION; 2779 if ("HumanName".equals(codeString)) 2780 return HUMANNAME; 2781 if ("Identifier".equals(codeString)) 2782 return IDENTIFIER; 2783 if ("Meta".equals(codeString)) 2784 return META; 2785 if ("Money".equals(codeString)) 2786 return MONEY; 2787 if ("Narrative".equals(codeString)) 2788 return NARRATIVE; 2789 if ("ParameterDefinition".equals(codeString)) 2790 return PARAMETERDEFINITION; 2791 if ("Period".equals(codeString)) 2792 return PERIOD; 2793 if ("Quantity".equals(codeString)) 2794 return QUANTITY; 2795 if ("Range".equals(codeString)) 2796 return RANGE; 2797 if ("Ratio".equals(codeString)) 2798 return RATIO; 2799 if ("Reference".equals(codeString)) 2800 return REFERENCE; 2801 if ("RelatedArtifact".equals(codeString)) 2802 return RELATEDARTIFACT; 2803 if ("SampledData".equals(codeString)) 2804 return SAMPLEDDATA; 2805 if ("Signature".equals(codeString)) 2806 return SIGNATURE; 2807 if ("SimpleQuantity".equals(codeString)) 2808 return SIMPLEQUANTITY; 2809 if ("Timing".equals(codeString)) 2810 return TIMING; 2811 if ("TriggerDefinition".equals(codeString)) 2812 return TRIGGERDEFINITION; 2813 if ("UsageContext".equals(codeString)) 2814 return USAGECONTEXT; 2815 if ("base64Binary".equals(codeString)) 2816 return BASE64BINARY; 2817 if ("boolean".equals(codeString)) 2818 return BOOLEAN; 2819 if ("code".equals(codeString)) 2820 return CODE; 2821 if ("date".equals(codeString)) 2822 return DATE; 2823 if ("dateTime".equals(codeString)) 2824 return DATETIME; 2825 if ("decimal".equals(codeString)) 2826 return DECIMAL; 2827 if ("id".equals(codeString)) 2828 return ID; 2829 if ("instant".equals(codeString)) 2830 return INSTANT; 2831 if ("integer".equals(codeString)) 2832 return INTEGER; 2833 if ("markdown".equals(codeString)) 2834 return MARKDOWN; 2835 if ("oid".equals(codeString)) 2836 return OID; 2837 if ("positiveInt".equals(codeString)) 2838 return POSITIVEINT; 2839 if ("string".equals(codeString)) 2840 return STRING; 2841 if ("time".equals(codeString)) 2842 return TIME; 2843 if ("unsignedInt".equals(codeString)) 2844 return UNSIGNEDINT; 2845 if ("uri".equals(codeString)) 2846 return URI; 2847 if ("uuid".equals(codeString)) 2848 return UUID; 2849 if ("xhtml".equals(codeString)) 2850 return XHTML; 2851 if ("Account".equals(codeString)) 2852 return ACCOUNT; 2853 if ("ActivityDefinition".equals(codeString)) 2854 return ACTIVITYDEFINITION; 2855 if ("AdverseEvent".equals(codeString)) 2856 return ADVERSEEVENT; 2857 if ("AllergyIntolerance".equals(codeString)) 2858 return ALLERGYINTOLERANCE; 2859 if ("Appointment".equals(codeString)) 2860 return APPOINTMENT; 2861 if ("AppointmentResponse".equals(codeString)) 2862 return APPOINTMENTRESPONSE; 2863 if ("AuditEvent".equals(codeString)) 2864 return AUDITEVENT; 2865 if ("Basic".equals(codeString)) 2866 return BASIC; 2867 if ("Binary".equals(codeString)) 2868 return BINARY; 2869 if ("BodySite".equals(codeString)) 2870 return BODYSITE; 2871 if ("Bundle".equals(codeString)) 2872 return BUNDLE; 2873 if ("CapabilityStatement".equals(codeString)) 2874 return CAPABILITYSTATEMENT; 2875 if ("CarePlan".equals(codeString)) 2876 return CAREPLAN; 2877 if ("CareTeam".equals(codeString)) 2878 return CARETEAM; 2879 if ("ChargeItem".equals(codeString)) 2880 return CHARGEITEM; 2881 if ("Claim".equals(codeString)) 2882 return CLAIM; 2883 if ("ClaimResponse".equals(codeString)) 2884 return CLAIMRESPONSE; 2885 if ("ClinicalImpression".equals(codeString)) 2886 return CLINICALIMPRESSION; 2887 if ("CodeSystem".equals(codeString)) 2888 return CODESYSTEM; 2889 if ("Communication".equals(codeString)) 2890 return COMMUNICATION; 2891 if ("CommunicationRequest".equals(codeString)) 2892 return COMMUNICATIONREQUEST; 2893 if ("CompartmentDefinition".equals(codeString)) 2894 return COMPARTMENTDEFINITION; 2895 if ("Composition".equals(codeString)) 2896 return COMPOSITION; 2897 if ("ConceptMap".equals(codeString)) 2898 return CONCEPTMAP; 2899 if ("Condition".equals(codeString)) 2900 return CONDITION; 2901 if ("Consent".equals(codeString)) 2902 return CONSENT; 2903 if ("Contract".equals(codeString)) 2904 return CONTRACT; 2905 if ("Coverage".equals(codeString)) 2906 return COVERAGE; 2907 if ("DataElement".equals(codeString)) 2908 return DATAELEMENT; 2909 if ("DetectedIssue".equals(codeString)) 2910 return DETECTEDISSUE; 2911 if ("Device".equals(codeString)) 2912 return DEVICE; 2913 if ("DeviceComponent".equals(codeString)) 2914 return DEVICECOMPONENT; 2915 if ("DeviceMetric".equals(codeString)) 2916 return DEVICEMETRIC; 2917 if ("DeviceRequest".equals(codeString)) 2918 return DEVICEREQUEST; 2919 if ("DeviceUseStatement".equals(codeString)) 2920 return DEVICEUSESTATEMENT; 2921 if ("DiagnosticReport".equals(codeString)) 2922 return DIAGNOSTICREPORT; 2923 if ("DocumentManifest".equals(codeString)) 2924 return DOCUMENTMANIFEST; 2925 if ("DocumentReference".equals(codeString)) 2926 return DOCUMENTREFERENCE; 2927 if ("DomainResource".equals(codeString)) 2928 return DOMAINRESOURCE; 2929 if ("EligibilityRequest".equals(codeString)) 2930 return ELIGIBILITYREQUEST; 2931 if ("EligibilityResponse".equals(codeString)) 2932 return ELIGIBILITYRESPONSE; 2933 if ("Encounter".equals(codeString)) 2934 return ENCOUNTER; 2935 if ("Endpoint".equals(codeString)) 2936 return ENDPOINT; 2937 if ("EnrollmentRequest".equals(codeString)) 2938 return ENROLLMENTREQUEST; 2939 if ("EnrollmentResponse".equals(codeString)) 2940 return ENROLLMENTRESPONSE; 2941 if ("EpisodeOfCare".equals(codeString)) 2942 return EPISODEOFCARE; 2943 if ("ExpansionProfile".equals(codeString)) 2944 return EXPANSIONPROFILE; 2945 if ("ExplanationOfBenefit".equals(codeString)) 2946 return EXPLANATIONOFBENEFIT; 2947 if ("FamilyMemberHistory".equals(codeString)) 2948 return FAMILYMEMBERHISTORY; 2949 if ("Flag".equals(codeString)) 2950 return FLAG; 2951 if ("Goal".equals(codeString)) 2952 return GOAL; 2953 if ("GraphDefinition".equals(codeString)) 2954 return GRAPHDEFINITION; 2955 if ("Group".equals(codeString)) 2956 return GROUP; 2957 if ("GuidanceResponse".equals(codeString)) 2958 return GUIDANCERESPONSE; 2959 if ("HealthcareService".equals(codeString)) 2960 return HEALTHCARESERVICE; 2961 if ("ImagingManifest".equals(codeString)) 2962 return IMAGINGMANIFEST; 2963 if ("ImagingStudy".equals(codeString)) 2964 return IMAGINGSTUDY; 2965 if ("Immunization".equals(codeString)) 2966 return IMMUNIZATION; 2967 if ("ImmunizationRecommendation".equals(codeString)) 2968 return IMMUNIZATIONRECOMMENDATION; 2969 if ("ImplementationGuide".equals(codeString)) 2970 return IMPLEMENTATIONGUIDE; 2971 if ("Library".equals(codeString)) 2972 return LIBRARY; 2973 if ("Linkage".equals(codeString)) 2974 return LINKAGE; 2975 if ("List".equals(codeString)) 2976 return LIST; 2977 if ("Location".equals(codeString)) 2978 return LOCATION; 2979 if ("Measure".equals(codeString)) 2980 return MEASURE; 2981 if ("MeasureReport".equals(codeString)) 2982 return MEASUREREPORT; 2983 if ("Media".equals(codeString)) 2984 return MEDIA; 2985 if ("Medication".equals(codeString)) 2986 return MEDICATION; 2987 if ("MedicationAdministration".equals(codeString)) 2988 return MEDICATIONADMINISTRATION; 2989 if ("MedicationDispense".equals(codeString)) 2990 return MEDICATIONDISPENSE; 2991 if ("MedicationRequest".equals(codeString)) 2992 return MEDICATIONREQUEST; 2993 if ("MedicationStatement".equals(codeString)) 2994 return MEDICATIONSTATEMENT; 2995 if ("MessageDefinition".equals(codeString)) 2996 return MESSAGEDEFINITION; 2997 if ("MessageHeader".equals(codeString)) 2998 return MESSAGEHEADER; 2999 if ("NamingSystem".equals(codeString)) 3000 return NAMINGSYSTEM; 3001 if ("NutritionOrder".equals(codeString)) 3002 return NUTRITIONORDER; 3003 if ("Observation".equals(codeString)) 3004 return OBSERVATION; 3005 if ("OperationDefinition".equals(codeString)) 3006 return OPERATIONDEFINITION; 3007 if ("OperationOutcome".equals(codeString)) 3008 return OPERATIONOUTCOME; 3009 if ("Organization".equals(codeString)) 3010 return ORGANIZATION; 3011 if ("Parameters".equals(codeString)) 3012 return PARAMETERS; 3013 if ("Patient".equals(codeString)) 3014 return PATIENT; 3015 if ("PaymentNotice".equals(codeString)) 3016 return PAYMENTNOTICE; 3017 if ("PaymentReconciliation".equals(codeString)) 3018 return PAYMENTRECONCILIATION; 3019 if ("Person".equals(codeString)) 3020 return PERSON; 3021 if ("PlanDefinition".equals(codeString)) 3022 return PLANDEFINITION; 3023 if ("Practitioner".equals(codeString)) 3024 return PRACTITIONER; 3025 if ("PractitionerRole".equals(codeString)) 3026 return PRACTITIONERROLE; 3027 if ("Procedure".equals(codeString)) 3028 return PROCEDURE; 3029 if ("ProcedureRequest".equals(codeString)) 3030 return PROCEDUREREQUEST; 3031 if ("ProcessRequest".equals(codeString)) 3032 return PROCESSREQUEST; 3033 if ("ProcessResponse".equals(codeString)) 3034 return PROCESSRESPONSE; 3035 if ("Provenance".equals(codeString)) 3036 return PROVENANCE; 3037 if ("Questionnaire".equals(codeString)) 3038 return QUESTIONNAIRE; 3039 if ("QuestionnaireResponse".equals(codeString)) 3040 return QUESTIONNAIRERESPONSE; 3041 if ("ReferralRequest".equals(codeString)) 3042 return REFERRALREQUEST; 3043 if ("RelatedPerson".equals(codeString)) 3044 return RELATEDPERSON; 3045 if ("RequestGroup".equals(codeString)) 3046 return REQUESTGROUP; 3047 if ("ResearchStudy".equals(codeString)) 3048 return RESEARCHSTUDY; 3049 if ("ResearchSubject".equals(codeString)) 3050 return RESEARCHSUBJECT; 3051 if ("Resource".equals(codeString)) 3052 return RESOURCE; 3053 if ("RiskAssessment".equals(codeString)) 3054 return RISKASSESSMENT; 3055 if ("Schedule".equals(codeString)) 3056 return SCHEDULE; 3057 if ("SearchParameter".equals(codeString)) 3058 return SEARCHPARAMETER; 3059 if ("Sequence".equals(codeString)) 3060 return SEQUENCE; 3061 if ("ServiceDefinition".equals(codeString)) 3062 return SERVICEDEFINITION; 3063 if ("Slot".equals(codeString)) 3064 return SLOT; 3065 if ("Specimen".equals(codeString)) 3066 return SPECIMEN; 3067 if ("StructureDefinition".equals(codeString)) 3068 return STRUCTUREDEFINITION; 3069 if ("StructureMap".equals(codeString)) 3070 return STRUCTUREMAP; 3071 if ("Subscription".equals(codeString)) 3072 return SUBSCRIPTION; 3073 if ("Substance".equals(codeString)) 3074 return SUBSTANCE; 3075 if ("SupplyDelivery".equals(codeString)) 3076 return SUPPLYDELIVERY; 3077 if ("SupplyRequest".equals(codeString)) 3078 return SUPPLYREQUEST; 3079 if ("Task".equals(codeString)) 3080 return TASK; 3081 if ("TestReport".equals(codeString)) 3082 return TESTREPORT; 3083 if ("TestScript".equals(codeString)) 3084 return TESTSCRIPT; 3085 if ("ValueSet".equals(codeString)) 3086 return VALUESET; 3087 if ("VisionPrescription".equals(codeString)) 3088 return VISIONPRESCRIPTION; 3089 if ("Type".equals(codeString)) 3090 return TYPE; 3091 if ("Any".equals(codeString)) 3092 return ANY; 3093 throw new FHIRException("Unknown FHIRAllTypes code '"+codeString+"'"); 3094 } 3095 public String toCode() { 3096 switch (this) { 3097 case ADDRESS: return "Address"; 3098 case AGE: return "Age"; 3099 case ANNOTATION: return "Annotation"; 3100 case ATTACHMENT: return "Attachment"; 3101 case BACKBONEELEMENT: return "BackboneElement"; 3102 case CODEABLECONCEPT: return "CodeableConcept"; 3103 case CODING: return "Coding"; 3104 case CONTACTDETAIL: return "ContactDetail"; 3105 case CONTACTPOINT: return "ContactPoint"; 3106 case CONTRIBUTOR: return "Contributor"; 3107 case COUNT: return "Count"; 3108 case DATAREQUIREMENT: return "DataRequirement"; 3109 case DISTANCE: return "Distance"; 3110 case DOSAGE: return "Dosage"; 3111 case DURATION: return "Duration"; 3112 case ELEMENT: return "Element"; 3113 case ELEMENTDEFINITION: return "ElementDefinition"; 3114 case EXTENSION: return "Extension"; 3115 case HUMANNAME: return "HumanName"; 3116 case IDENTIFIER: return "Identifier"; 3117 case META: return "Meta"; 3118 case MONEY: return "Money"; 3119 case NARRATIVE: return "Narrative"; 3120 case PARAMETERDEFINITION: return "ParameterDefinition"; 3121 case PERIOD: return "Period"; 3122 case QUANTITY: return "Quantity"; 3123 case RANGE: return "Range"; 3124 case RATIO: return "Ratio"; 3125 case REFERENCE: return "Reference"; 3126 case RELATEDARTIFACT: return "RelatedArtifact"; 3127 case SAMPLEDDATA: return "SampledData"; 3128 case SIGNATURE: return "Signature"; 3129 case SIMPLEQUANTITY: return "SimpleQuantity"; 3130 case TIMING: return "Timing"; 3131 case TRIGGERDEFINITION: return "TriggerDefinition"; 3132 case USAGECONTEXT: return "UsageContext"; 3133 case BASE64BINARY: return "base64Binary"; 3134 case BOOLEAN: return "boolean"; 3135 case CODE: return "code"; 3136 case DATE: return "date"; 3137 case DATETIME: return "dateTime"; 3138 case DECIMAL: return "decimal"; 3139 case ID: return "id"; 3140 case INSTANT: return "instant"; 3141 case INTEGER: return "integer"; 3142 case MARKDOWN: return "markdown"; 3143 case OID: return "oid"; 3144 case POSITIVEINT: return "positiveInt"; 3145 case STRING: return "string"; 3146 case TIME: return "time"; 3147 case UNSIGNEDINT: return "unsignedInt"; 3148 case URI: return "uri"; 3149 case UUID: return "uuid"; 3150 case XHTML: return "xhtml"; 3151 case ACCOUNT: return "Account"; 3152 case ACTIVITYDEFINITION: return "ActivityDefinition"; 3153 case ADVERSEEVENT: return "AdverseEvent"; 3154 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 3155 case APPOINTMENT: return "Appointment"; 3156 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 3157 case AUDITEVENT: return "AuditEvent"; 3158 case BASIC: return "Basic"; 3159 case BINARY: return "Binary"; 3160 case BODYSITE: return "BodySite"; 3161 case BUNDLE: return "Bundle"; 3162 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 3163 case CAREPLAN: return "CarePlan"; 3164 case CARETEAM: return "CareTeam"; 3165 case CHARGEITEM: return "ChargeItem"; 3166 case CLAIM: return "Claim"; 3167 case CLAIMRESPONSE: return "ClaimResponse"; 3168 case CLINICALIMPRESSION: return "ClinicalImpression"; 3169 case CODESYSTEM: return "CodeSystem"; 3170 case COMMUNICATION: return "Communication"; 3171 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 3172 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 3173 case COMPOSITION: return "Composition"; 3174 case CONCEPTMAP: return "ConceptMap"; 3175 case CONDITION: return "Condition"; 3176 case CONSENT: return "Consent"; 3177 case CONTRACT: return "Contract"; 3178 case COVERAGE: return "Coverage"; 3179 case DATAELEMENT: return "DataElement"; 3180 case DETECTEDISSUE: return "DetectedIssue"; 3181 case DEVICE: return "Device"; 3182 case DEVICECOMPONENT: return "DeviceComponent"; 3183 case DEVICEMETRIC: return "DeviceMetric"; 3184 case DEVICEREQUEST: return "DeviceRequest"; 3185 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 3186 case DIAGNOSTICREPORT: return "DiagnosticReport"; 3187 case DOCUMENTMANIFEST: return "DocumentManifest"; 3188 case DOCUMENTREFERENCE: return "DocumentReference"; 3189 case DOMAINRESOURCE: return "DomainResource"; 3190 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 3191 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 3192 case ENCOUNTER: return "Encounter"; 3193 case ENDPOINT: return "Endpoint"; 3194 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 3195 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 3196 case EPISODEOFCARE: return "EpisodeOfCare"; 3197 case EXPANSIONPROFILE: return "ExpansionProfile"; 3198 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 3199 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 3200 case FLAG: return "Flag"; 3201 case GOAL: return "Goal"; 3202 case GRAPHDEFINITION: return "GraphDefinition"; 3203 case GROUP: return "Group"; 3204 case GUIDANCERESPONSE: return "GuidanceResponse"; 3205 case HEALTHCARESERVICE: return "HealthcareService"; 3206 case IMAGINGMANIFEST: return "ImagingManifest"; 3207 case IMAGINGSTUDY: return "ImagingStudy"; 3208 case IMMUNIZATION: return "Immunization"; 3209 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 3210 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 3211 case LIBRARY: return "Library"; 3212 case LINKAGE: return "Linkage"; 3213 case LIST: return "List"; 3214 case LOCATION: return "Location"; 3215 case MEASURE: return "Measure"; 3216 case MEASUREREPORT: return "MeasureReport"; 3217 case MEDIA: return "Media"; 3218 case MEDICATION: return "Medication"; 3219 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 3220 case MEDICATIONDISPENSE: return "MedicationDispense"; 3221 case MEDICATIONREQUEST: return "MedicationRequest"; 3222 case MEDICATIONSTATEMENT: return "MedicationStatement"; 3223 case MESSAGEDEFINITION: return "MessageDefinition"; 3224 case MESSAGEHEADER: return "MessageHeader"; 3225 case NAMINGSYSTEM: return "NamingSystem"; 3226 case NUTRITIONORDER: return "NutritionOrder"; 3227 case OBSERVATION: return "Observation"; 3228 case OPERATIONDEFINITION: return "OperationDefinition"; 3229 case OPERATIONOUTCOME: return "OperationOutcome"; 3230 case ORGANIZATION: return "Organization"; 3231 case PARAMETERS: return "Parameters"; 3232 case PATIENT: return "Patient"; 3233 case PAYMENTNOTICE: return "PaymentNotice"; 3234 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 3235 case PERSON: return "Person"; 3236 case PLANDEFINITION: return "PlanDefinition"; 3237 case PRACTITIONER: return "Practitioner"; 3238 case PRACTITIONERROLE: return "PractitionerRole"; 3239 case PROCEDURE: return "Procedure"; 3240 case PROCEDUREREQUEST: return "ProcedureRequest"; 3241 case PROCESSREQUEST: return "ProcessRequest"; 3242 case PROCESSRESPONSE: return "ProcessResponse"; 3243 case PROVENANCE: return "Provenance"; 3244 case QUESTIONNAIRE: return "Questionnaire"; 3245 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 3246 case REFERRALREQUEST: return "ReferralRequest"; 3247 case RELATEDPERSON: return "RelatedPerson"; 3248 case REQUESTGROUP: return "RequestGroup"; 3249 case RESEARCHSTUDY: return "ResearchStudy"; 3250 case RESEARCHSUBJECT: return "ResearchSubject"; 3251 case RESOURCE: return "Resource"; 3252 case RISKASSESSMENT: return "RiskAssessment"; 3253 case SCHEDULE: return "Schedule"; 3254 case SEARCHPARAMETER: return "SearchParameter"; 3255 case SEQUENCE: return "Sequence"; 3256 case SERVICEDEFINITION: return "ServiceDefinition"; 3257 case SLOT: return "Slot"; 3258 case SPECIMEN: return "Specimen"; 3259 case STRUCTUREDEFINITION: return "StructureDefinition"; 3260 case STRUCTUREMAP: return "StructureMap"; 3261 case SUBSCRIPTION: return "Subscription"; 3262 case SUBSTANCE: return "Substance"; 3263 case SUPPLYDELIVERY: return "SupplyDelivery"; 3264 case SUPPLYREQUEST: return "SupplyRequest"; 3265 case TASK: return "Task"; 3266 case TESTREPORT: return "TestReport"; 3267 case TESTSCRIPT: return "TestScript"; 3268 case VALUESET: return "ValueSet"; 3269 case VISIONPRESCRIPTION: return "VisionPrescription"; 3270 case TYPE: return "Type"; 3271 case ANY: return "Any"; 3272 case NULL: return null; 3273 default: return "?"; 3274 } 3275 } 3276 public String getSystem() { 3277 switch (this) { 3278 case ADDRESS: return "http://hl7.org/fhir/data-types"; 3279 case AGE: return "http://hl7.org/fhir/data-types"; 3280 case ANNOTATION: return "http://hl7.org/fhir/data-types"; 3281 case ATTACHMENT: return "http://hl7.org/fhir/data-types"; 3282 case BACKBONEELEMENT: return "http://hl7.org/fhir/data-types"; 3283 case CODEABLECONCEPT: return "http://hl7.org/fhir/data-types"; 3284 case CODING: return "http://hl7.org/fhir/data-types"; 3285 case CONTACTDETAIL: return "http://hl7.org/fhir/data-types"; 3286 case CONTACTPOINT: return "http://hl7.org/fhir/data-types"; 3287 case CONTRIBUTOR: return "http://hl7.org/fhir/data-types"; 3288 case COUNT: return "http://hl7.org/fhir/data-types"; 3289 case DATAREQUIREMENT: return "http://hl7.org/fhir/data-types"; 3290 case DISTANCE: return "http://hl7.org/fhir/data-types"; 3291 case DOSAGE: return "http://hl7.org/fhir/data-types"; 3292 case DURATION: return "http://hl7.org/fhir/data-types"; 3293 case ELEMENT: return "http://hl7.org/fhir/data-types"; 3294 case ELEMENTDEFINITION: return "http://hl7.org/fhir/data-types"; 3295 case EXTENSION: return "http://hl7.org/fhir/data-types"; 3296 case HUMANNAME: return "http://hl7.org/fhir/data-types"; 3297 case IDENTIFIER: return "http://hl7.org/fhir/data-types"; 3298 case META: return "http://hl7.org/fhir/data-types"; 3299 case MONEY: return "http://hl7.org/fhir/data-types"; 3300 case NARRATIVE: return "http://hl7.org/fhir/data-types"; 3301 case PARAMETERDEFINITION: return "http://hl7.org/fhir/data-types"; 3302 case PERIOD: return "http://hl7.org/fhir/data-types"; 3303 case QUANTITY: return "http://hl7.org/fhir/data-types"; 3304 case RANGE: return "http://hl7.org/fhir/data-types"; 3305 case RATIO: return "http://hl7.org/fhir/data-types"; 3306 case REFERENCE: return "http://hl7.org/fhir/data-types"; 3307 case RELATEDARTIFACT: return "http://hl7.org/fhir/data-types"; 3308 case SAMPLEDDATA: return "http://hl7.org/fhir/data-types"; 3309 case SIGNATURE: return "http://hl7.org/fhir/data-types"; 3310 case SIMPLEQUANTITY: return "http://hl7.org/fhir/data-types"; 3311 case TIMING: return "http://hl7.org/fhir/data-types"; 3312 case TRIGGERDEFINITION: return "http://hl7.org/fhir/data-types"; 3313 case USAGECONTEXT: return "http://hl7.org/fhir/data-types"; 3314 case BASE64BINARY: return "http://hl7.org/fhir/data-types"; 3315 case BOOLEAN: return "http://hl7.org/fhir/data-types"; 3316 case CODE: return "http://hl7.org/fhir/data-types"; 3317 case DATE: return "http://hl7.org/fhir/data-types"; 3318 case DATETIME: return "http://hl7.org/fhir/data-types"; 3319 case DECIMAL: return "http://hl7.org/fhir/data-types"; 3320 case ID: return "http://hl7.org/fhir/data-types"; 3321 case INSTANT: return "http://hl7.org/fhir/data-types"; 3322 case INTEGER: return "http://hl7.org/fhir/data-types"; 3323 case MARKDOWN: return "http://hl7.org/fhir/data-types"; 3324 case OID: return "http://hl7.org/fhir/data-types"; 3325 case POSITIVEINT: return "http://hl7.org/fhir/data-types"; 3326 case STRING: return "http://hl7.org/fhir/data-types"; 3327 case TIME: return "http://hl7.org/fhir/data-types"; 3328 case UNSIGNEDINT: return "http://hl7.org/fhir/data-types"; 3329 case URI: return "http://hl7.org/fhir/data-types"; 3330 case UUID: return "http://hl7.org/fhir/data-types"; 3331 case XHTML: return "http://hl7.org/fhir/data-types"; 3332 case ACCOUNT: return "http://hl7.org/fhir/resource-types"; 3333 case ACTIVITYDEFINITION: return "http://hl7.org/fhir/resource-types"; 3334 case ADVERSEEVENT: return "http://hl7.org/fhir/resource-types"; 3335 case ALLERGYINTOLERANCE: return "http://hl7.org/fhir/resource-types"; 3336 case APPOINTMENT: return "http://hl7.org/fhir/resource-types"; 3337 case APPOINTMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 3338 case AUDITEVENT: return "http://hl7.org/fhir/resource-types"; 3339 case BASIC: return "http://hl7.org/fhir/resource-types"; 3340 case BINARY: return "http://hl7.org/fhir/resource-types"; 3341 case BODYSITE: return "http://hl7.org/fhir/resource-types"; 3342 case BUNDLE: return "http://hl7.org/fhir/resource-types"; 3343 case CAPABILITYSTATEMENT: return "http://hl7.org/fhir/resource-types"; 3344 case CAREPLAN: return "http://hl7.org/fhir/resource-types"; 3345 case CARETEAM: return "http://hl7.org/fhir/resource-types"; 3346 case CHARGEITEM: return "http://hl7.org/fhir/resource-types"; 3347 case CLAIM: return "http://hl7.org/fhir/resource-types"; 3348 case CLAIMRESPONSE: return "http://hl7.org/fhir/resource-types"; 3349 case CLINICALIMPRESSION: return "http://hl7.org/fhir/resource-types"; 3350 case CODESYSTEM: return "http://hl7.org/fhir/resource-types"; 3351 case COMMUNICATION: return "http://hl7.org/fhir/resource-types"; 3352 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 3353 case COMPARTMENTDEFINITION: return "http://hl7.org/fhir/resource-types"; 3354 case COMPOSITION: return "http://hl7.org/fhir/resource-types"; 3355 case CONCEPTMAP: return "http://hl7.org/fhir/resource-types"; 3356 case CONDITION: return "http://hl7.org/fhir/resource-types"; 3357 case CONSENT: return "http://hl7.org/fhir/resource-types"; 3358 case CONTRACT: return "http://hl7.org/fhir/resource-types"; 3359 case COVERAGE: return "http://hl7.org/fhir/resource-types"; 3360 case DATAELEMENT: return "http://hl7.org/fhir/resource-types"; 3361 case DETECTEDISSUE: return "http://hl7.org/fhir/resource-types"; 3362 case DEVICE: return "http://hl7.org/fhir/resource-types"; 3363 case DEVICECOMPONENT: return "http://hl7.org/fhir/resource-types"; 3364 case DEVICEMETRIC: return "http://hl7.org/fhir/resource-types"; 3365 case DEVICEREQUEST: return "http://hl7.org/fhir/resource-types"; 3366 case DEVICEUSESTATEMENT: return "http://hl7.org/fhir/resource-types"; 3367 case DIAGNOSTICREPORT: return "http://hl7.org/fhir/resource-types"; 3368 case DOCUMENTMANIFEST: return "http://hl7.org/fhir/resource-types"; 3369 case DOCUMENTREFERENCE: return "http://hl7.org/fhir/resource-types"; 3370 case DOMAINRESOURCE: return "http://hl7.org/fhir/resource-types"; 3371 case ELIGIBILITYREQUEST: return "http://hl7.org/fhir/resource-types"; 3372 case ELIGIBILITYRESPONSE: return "http://hl7.org/fhir/resource-types"; 3373 case ENCOUNTER: return "http://hl7.org/fhir/resource-types"; 3374 case ENDPOINT: return "http://hl7.org/fhir/resource-types"; 3375 case ENROLLMENTREQUEST: return "http://hl7.org/fhir/resource-types"; 3376 case ENROLLMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 3377 case EPISODEOFCARE: return "http://hl7.org/fhir/resource-types"; 3378 case EXPANSIONPROFILE: return "http://hl7.org/fhir/resource-types"; 3379 case EXPLANATIONOFBENEFIT: return "http://hl7.org/fhir/resource-types"; 3380 case FAMILYMEMBERHISTORY: return "http://hl7.org/fhir/resource-types"; 3381 case FLAG: return "http://hl7.org/fhir/resource-types"; 3382 case GOAL: return "http://hl7.org/fhir/resource-types"; 3383 case GRAPHDEFINITION: return "http://hl7.org/fhir/resource-types"; 3384 case GROUP: return "http://hl7.org/fhir/resource-types"; 3385 case GUIDANCERESPONSE: return "http://hl7.org/fhir/resource-types"; 3386 case HEALTHCARESERVICE: return "http://hl7.org/fhir/resource-types"; 3387 case IMAGINGMANIFEST: return "http://hl7.org/fhir/resource-types"; 3388 case IMAGINGSTUDY: return "http://hl7.org/fhir/resource-types"; 3389 case IMMUNIZATION: return "http://hl7.org/fhir/resource-types"; 3390 case IMMUNIZATIONRECOMMENDATION: return "http://hl7.org/fhir/resource-types"; 3391 case IMPLEMENTATIONGUIDE: return "http://hl7.org/fhir/resource-types"; 3392 case LIBRARY: return "http://hl7.org/fhir/resource-types"; 3393 case LINKAGE: return "http://hl7.org/fhir/resource-types"; 3394 case LIST: return "http://hl7.org/fhir/resource-types"; 3395 case LOCATION: return "http://hl7.org/fhir/resource-types"; 3396 case MEASURE: return "http://hl7.org/fhir/resource-types"; 3397 case MEASUREREPORT: return "http://hl7.org/fhir/resource-types"; 3398 case MEDIA: return "http://hl7.org/fhir/resource-types"; 3399 case MEDICATION: return "http://hl7.org/fhir/resource-types"; 3400 case MEDICATIONADMINISTRATION: return "http://hl7.org/fhir/resource-types"; 3401 case MEDICATIONDISPENSE: return "http://hl7.org/fhir/resource-types"; 3402 case MEDICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 3403 case MEDICATIONSTATEMENT: return "http://hl7.org/fhir/resource-types"; 3404 case MESSAGEDEFINITION: return "http://hl7.org/fhir/resource-types"; 3405 case MESSAGEHEADER: return "http://hl7.org/fhir/resource-types"; 3406 case NAMINGSYSTEM: return "http://hl7.org/fhir/resource-types"; 3407 case NUTRITIONORDER: return "http://hl7.org/fhir/resource-types"; 3408 case OBSERVATION: return "http://hl7.org/fhir/resource-types"; 3409 case OPERATIONDEFINITION: return "http://hl7.org/fhir/resource-types"; 3410 case OPERATIONOUTCOME: return "http://hl7.org/fhir/resource-types"; 3411 case ORGANIZATION: return "http://hl7.org/fhir/resource-types"; 3412 case PARAMETERS: return "http://hl7.org/fhir/resource-types"; 3413 case PATIENT: return "http://hl7.org/fhir/resource-types"; 3414 case PAYMENTNOTICE: return "http://hl7.org/fhir/resource-types"; 3415 case PAYMENTRECONCILIATION: return "http://hl7.org/fhir/resource-types"; 3416 case PERSON: return "http://hl7.org/fhir/resource-types"; 3417 case PLANDEFINITION: return "http://hl7.org/fhir/resource-types"; 3418 case PRACTITIONER: return "http://hl7.org/fhir/resource-types"; 3419 case PRACTITIONERROLE: return "http://hl7.org/fhir/resource-types"; 3420 case PROCEDURE: return "http://hl7.org/fhir/resource-types"; 3421 case PROCEDUREREQUEST: return "http://hl7.org/fhir/resource-types"; 3422 case PROCESSREQUEST: return "http://hl7.org/fhir/resource-types"; 3423 case PROCESSRESPONSE: return "http://hl7.org/fhir/resource-types"; 3424 case PROVENANCE: return "http://hl7.org/fhir/resource-types"; 3425 case QUESTIONNAIRE: return "http://hl7.org/fhir/resource-types"; 3426 case QUESTIONNAIRERESPONSE: return "http://hl7.org/fhir/resource-types"; 3427 case REFERRALREQUEST: return "http://hl7.org/fhir/resource-types"; 3428 case RELATEDPERSON: return "http://hl7.org/fhir/resource-types"; 3429 case REQUESTGROUP: return "http://hl7.org/fhir/resource-types"; 3430 case RESEARCHSTUDY: return "http://hl7.org/fhir/resource-types"; 3431 case RESEARCHSUBJECT: return "http://hl7.org/fhir/resource-types"; 3432 case RESOURCE: return "http://hl7.org/fhir/resource-types"; 3433 case RISKASSESSMENT: return "http://hl7.org/fhir/resource-types"; 3434 case SCHEDULE: return "http://hl7.org/fhir/resource-types"; 3435 case SEARCHPARAMETER: return "http://hl7.org/fhir/resource-types"; 3436 case SEQUENCE: return "http://hl7.org/fhir/resource-types"; 3437 case SERVICEDEFINITION: return "http://hl7.org/fhir/resource-types"; 3438 case SLOT: return "http://hl7.org/fhir/resource-types"; 3439 case SPECIMEN: return "http://hl7.org/fhir/resource-types"; 3440 case STRUCTUREDEFINITION: return "http://hl7.org/fhir/resource-types"; 3441 case STRUCTUREMAP: return "http://hl7.org/fhir/resource-types"; 3442 case SUBSCRIPTION: return "http://hl7.org/fhir/resource-types"; 3443 case SUBSTANCE: return "http://hl7.org/fhir/resource-types"; 3444 case SUPPLYDELIVERY: return "http://hl7.org/fhir/resource-types"; 3445 case SUPPLYREQUEST: return "http://hl7.org/fhir/resource-types"; 3446 case TASK: return "http://hl7.org/fhir/resource-types"; 3447 case TESTREPORT: return "http://hl7.org/fhir/resource-types"; 3448 case TESTSCRIPT: return "http://hl7.org/fhir/resource-types"; 3449 case VALUESET: return "http://hl7.org/fhir/resource-types"; 3450 case VISIONPRESCRIPTION: return "http://hl7.org/fhir/resource-types"; 3451 case TYPE: return "http://hl7.org/fhir/abstract-types"; 3452 case ANY: return "http://hl7.org/fhir/abstract-types"; 3453 case NULL: return null; 3454 default: return "?"; 3455 } 3456 } 3457 public String getDefinition() { 3458 switch (this) { 3459 case ADDRESS: return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 3460 case AGE: return "A duration of time during which an organism (or a process) has existed."; 3461 case ANNOTATION: return "A text note which also contains information about who made the statement and when."; 3462 case ATTACHMENT: return "For referring to data content defined in other formats."; 3463 case BACKBONEELEMENT: return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 3464 case CODEABLECONCEPT: return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 3465 case CODING: return "A reference to a code defined by a terminology system."; 3466 case CONTACTDETAIL: return "Specifies contact information for a person or organization."; 3467 case CONTACTPOINT: return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 3468 case CONTRIBUTOR: return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 3469 case COUNT: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 3470 case DATAREQUIREMENT: return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 3471 case DISTANCE: return "A length - a value with a unit that is a physical distance."; 3472 case DOSAGE: return "Indicates how the medication is/was taken or should be taken by the patient."; 3473 case DURATION: return "A length of time."; 3474 case ELEMENT: return "Base definition for all elements in a resource."; 3475 case ELEMENTDEFINITION: return "Captures constraints on each element within the resource, profile, or extension."; 3476 case EXTENSION: return "Optional Extension Element - found in all resources."; 3477 case HUMANNAME: return "A human's name with the ability to identify parts and usage."; 3478 case IDENTIFIER: return "A technical identifier - identifies some entity uniquely and unambiguously."; 3479 case META: return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource."; 3480 case MONEY: return "An amount of economic utility in some recognized currency."; 3481 case NARRATIVE: return "A human-readable formatted text, including images."; 3482 case PARAMETERDEFINITION: return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 3483 case PERIOD: return "A time period defined by a start and end date and optionally time."; 3484 case QUANTITY: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 3485 case RANGE: return "A set of ordered Quantities defined by a low and high limit."; 3486 case RATIO: return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 3487 case REFERENCE: return "A reference from one resource to another."; 3488 case RELATEDARTIFACT: return "Related artifacts such as additional documentation, justification, or bibliographic references."; 3489 case SAMPLEDDATA: return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 3490 case SIGNATURE: return "A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities."; 3491 case SIMPLEQUANTITY: return ""; 3492 case TIMING: return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 3493 case TRIGGERDEFINITION: return "A description of a triggering event."; 3494 case USAGECONTEXT: return "Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 3495 case BASE64BINARY: return "A stream of bytes"; 3496 case BOOLEAN: return "Value of \"true\" or \"false\""; 3497 case CODE: return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 3498 case DATE: return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 3499 case DATETIME: return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 3500 case DECIMAL: return "A rational number with implicit precision"; 3501 case ID: return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 3502 case INSTANT: return "An instant in time - known at least to the second"; 3503 case INTEGER: return "A whole number"; 3504 case MARKDOWN: return "A string that may contain markdown syntax for optional processing by a mark down presentation engine"; 3505 case OID: return "An OID represented as a URI"; 3506 case POSITIVEINT: return "An integer with a value that is positive (e.g. >0)"; 3507 case STRING: return "A sequence of Unicode characters"; 3508 case TIME: return "A time during the day, with no date specified"; 3509 case UNSIGNEDINT: return "An integer with a value that is not negative (e.g. >= 0)"; 3510 case URI: return "String of characters used to identify a name or a resource"; 3511 case UUID: return "A UUID, represented as a URI"; 3512 case XHTML: return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 3513 case ACCOUNT: return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 3514 case ACTIVITYDEFINITION: return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 3515 case ADVERSEEVENT: return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 3516 case ALLERGYINTOLERANCE: return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 3517 case APPOINTMENT: return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 3518 case APPOINTMENTRESPONSE: return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 3519 case AUDITEVENT: return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 3520 case BASIC: return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 3521 case BINARY: return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 3522 case BODYSITE: return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 3523 case BUNDLE: return "A container for a collection of resources."; 3524 case CAPABILITYSTATEMENT: return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 3525 case CAREPLAN: return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 3526 case CARETEAM: return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 3527 case CHARGEITEM: return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 3528 case CLAIM: return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 3529 case CLAIMRESPONSE: return "This resource provides the adjudication details from the processing of a Claim resource."; 3530 case CLINICALIMPRESSION: return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 3531 case CODESYSTEM: return "A code system resource specifies a set of codes drawn from one or more code systems."; 3532 case COMMUNICATION: return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 3533 case COMMUNICATIONREQUEST: return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 3534 case COMPARTMENTDEFINITION: return "A compartment definition that defines how resources are accessed on a server."; 3535 case COMPOSITION: return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 3536 case CONCEPTMAP: return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 3537 case CONDITION: return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 3538 case CONSENT: return "A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 3539 case CONTRACT: return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 3540 case COVERAGE: return "Financial instrument which may be used to reimburse or pay for health care products and services."; 3541 case DATAELEMENT: return "The formal description of a single piece of information that can be gathered and reported."; 3542 case DETECTEDISSUE: return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 3543 case DEVICE: return "This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 3544 case DEVICECOMPONENT: return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 3545 case DEVICEMETRIC: return "Describes a measurement, calculation or setting capability of a medical device."; 3546 case DEVICEREQUEST: return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 3547 case DEVICEUSESTATEMENT: return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 3548 case DIAGNOSTICREPORT: return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 3549 case DOCUMENTMANIFEST: return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 3550 case DOCUMENTREFERENCE: return "A reference to a document."; 3551 case DOMAINRESOURCE: return "A resource that includes narrative, extensions, and contained resources."; 3552 case ELIGIBILITYREQUEST: return "The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 3553 case ELIGIBILITYRESPONSE: return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 3554 case ENCOUNTER: return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 3555 case ENDPOINT: return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 3556 case ENROLLMENTREQUEST: return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 3557 case ENROLLMENTRESPONSE: return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 3558 case EPISODEOFCARE: return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 3559 case EXPANSIONPROFILE: return "Resource to define constraints on the Expansion of a FHIR ValueSet."; 3560 case EXPLANATIONOFBENEFIT: return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 3561 case FAMILYMEMBERHISTORY: return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 3562 case FLAG: return "Prospective warnings of potential issues when providing care to the patient."; 3563 case GOAL: return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 3564 case GRAPHDEFINITION: return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 3565 case GROUP: return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 3566 case GUIDANCERESPONSE: return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 3567 case HEALTHCARESERVICE: return "The details of a healthcare service available at a location."; 3568 case IMAGINGMANIFEST: return "A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection."; 3569 case IMAGINGSTUDY: return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 3570 case IMMUNIZATION: return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 3571 case IMMUNIZATIONRECOMMENDATION: return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 3572 case IMPLEMENTATIONGUIDE: return "A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 3573 case LIBRARY: return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 3574 case LINKAGE: return "Identifies two or more records (resource instances) that are referring to the same real-world \"occurrence\"."; 3575 case LIST: return "A set of information summarized from a list of other resources."; 3576 case LOCATION: return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 3577 case MEASURE: return "The Measure resource provides the definition of a quality measure."; 3578 case MEASUREREPORT: return "The MeasureReport resource contains the results of evaluating a measure."; 3579 case MEDIA: return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 3580 case MEDICATION: return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 3581 case MEDICATIONADMINISTRATION: return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 3582 case MEDICATIONDISPENSE: return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 3583 case MEDICATIONREQUEST: return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 3584 case MEDICATIONSTATEMENT: return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 3585 case MESSAGEDEFINITION: return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 3586 case MESSAGEHEADER: return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 3587 case NAMINGSYSTEM: return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 3588 case NUTRITIONORDER: return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 3589 case OBSERVATION: return "Measurements and simple assertions made about a patient, device or other subject."; 3590 case OPERATIONDEFINITION: return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 3591 case OPERATIONOUTCOME: return "A collection of error, warning or information messages that result from a system action."; 3592 case ORGANIZATION: return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 3593 case PARAMETERS: return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 3594 case PATIENT: return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 3595 case PAYMENTNOTICE: return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 3596 case PAYMENTRECONCILIATION: return "This resource provides payment details and claim references supporting a bulk payment."; 3597 case PERSON: return "Demographics and administrative information about a person independent of a specific health-related context."; 3598 case PLANDEFINITION: return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 3599 case PRACTITIONER: return "A person who is directly or indirectly involved in the provisioning of healthcare."; 3600 case PRACTITIONERROLE: return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 3601 case PROCEDURE: return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 3602 case PROCEDUREREQUEST: return "A record of a request for diagnostic investigations, treatments, or operations to be performed."; 3603 case PROCESSREQUEST: return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 3604 case PROCESSRESPONSE: return "This resource provides processing status, errors and notes from the processing of a resource."; 3605 case PROVENANCE: return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 3606 case QUESTIONNAIRE: return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 3607 case QUESTIONNAIRERESPONSE: return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 3608 case REFERRALREQUEST: return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 3609 case RELATEDPERSON: return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 3610 case REQUESTGROUP: return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 3611 case RESEARCHSTUDY: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 3612 case RESEARCHSUBJECT: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 3613 case RESOURCE: return "This is the base resource type for everything."; 3614 case RISKASSESSMENT: return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 3615 case SCHEDULE: return "A container for slots of time that may be available for booking appointments."; 3616 case SEARCHPARAMETER: return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 3617 case SEQUENCE: return "Raw data describing a biological sequence."; 3618 case SERVICEDEFINITION: return "The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking."; 3619 case SLOT: return "A slot of time on a schedule that may be available for booking appointments."; 3620 case SPECIMEN: return "A sample to be used for analysis."; 3621 case STRUCTUREDEFINITION: return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 3622 case STRUCTUREMAP: return "A Map of relationships between 2 structures that can be used to transform data."; 3623 case SUBSCRIPTION: return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 3624 case SUBSTANCE: return "A homogeneous material with a definite composition."; 3625 case SUPPLYDELIVERY: return "Record of delivery of what is supplied."; 3626 case SUPPLYREQUEST: return "A record of a request for a medication, substance or device used in the healthcare setting."; 3627 case TASK: return "A task to be performed."; 3628 case TESTREPORT: return "A summary of information based on the results of executing a TestScript."; 3629 case TESTSCRIPT: return "A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 3630 case VALUESET: return "A value set specifies a set of codes drawn from one or more code systems."; 3631 case VISIONPRESCRIPTION: return "An authorization for the supply of glasses and/or contact lenses to a patient."; 3632 case TYPE: return "A place holder that means any kind of data type"; 3633 case ANY: return "A place holder that means any kind of resource"; 3634 case NULL: return null; 3635 default: return "?"; 3636 } 3637 } 3638 public String getDisplay() { 3639 switch (this) { 3640 case ADDRESS: return "Address"; 3641 case AGE: return "Age"; 3642 case ANNOTATION: return "Annotation"; 3643 case ATTACHMENT: return "Attachment"; 3644 case BACKBONEELEMENT: return "BackboneElement"; 3645 case CODEABLECONCEPT: return "CodeableConcept"; 3646 case CODING: return "Coding"; 3647 case CONTACTDETAIL: return "ContactDetail"; 3648 case CONTACTPOINT: return "ContactPoint"; 3649 case CONTRIBUTOR: return "Contributor"; 3650 case COUNT: return "Count"; 3651 case DATAREQUIREMENT: return "DataRequirement"; 3652 case DISTANCE: return "Distance"; 3653 case DOSAGE: return "Dosage"; 3654 case DURATION: return "Duration"; 3655 case ELEMENT: return "Element"; 3656 case ELEMENTDEFINITION: return "ElementDefinition"; 3657 case EXTENSION: return "Extension"; 3658 case HUMANNAME: return "HumanName"; 3659 case IDENTIFIER: return "Identifier"; 3660 case META: return "Meta"; 3661 case MONEY: return "Money"; 3662 case NARRATIVE: return "Narrative"; 3663 case PARAMETERDEFINITION: return "ParameterDefinition"; 3664 case PERIOD: return "Period"; 3665 case QUANTITY: return "Quantity"; 3666 case RANGE: return "Range"; 3667 case RATIO: return "Ratio"; 3668 case REFERENCE: return "Reference"; 3669 case RELATEDARTIFACT: return "RelatedArtifact"; 3670 case SAMPLEDDATA: return "SampledData"; 3671 case SIGNATURE: return "Signature"; 3672 case SIMPLEQUANTITY: return "SimpleQuantity"; 3673 case TIMING: return "Timing"; 3674 case TRIGGERDEFINITION: return "TriggerDefinition"; 3675 case USAGECONTEXT: return "UsageContext"; 3676 case BASE64BINARY: return "base64Binary"; 3677 case BOOLEAN: return "boolean"; 3678 case CODE: return "code"; 3679 case DATE: return "date"; 3680 case DATETIME: return "dateTime"; 3681 case DECIMAL: return "decimal"; 3682 case ID: return "id"; 3683 case INSTANT: return "instant"; 3684 case INTEGER: return "integer"; 3685 case MARKDOWN: return "markdown"; 3686 case OID: return "oid"; 3687 case POSITIVEINT: return "positiveInt"; 3688 case STRING: return "string"; 3689 case TIME: return "time"; 3690 case UNSIGNEDINT: return "unsignedInt"; 3691 case URI: return "uri"; 3692 case UUID: return "uuid"; 3693 case XHTML: return "XHTML"; 3694 case ACCOUNT: return "Account"; 3695 case ACTIVITYDEFINITION: return "ActivityDefinition"; 3696 case ADVERSEEVENT: return "AdverseEvent"; 3697 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 3698 case APPOINTMENT: return "Appointment"; 3699 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 3700 case AUDITEVENT: return "AuditEvent"; 3701 case BASIC: return "Basic"; 3702 case BINARY: return "Binary"; 3703 case BODYSITE: return "BodySite"; 3704 case BUNDLE: return "Bundle"; 3705 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 3706 case CAREPLAN: return "CarePlan"; 3707 case CARETEAM: return "CareTeam"; 3708 case CHARGEITEM: return "ChargeItem"; 3709 case CLAIM: return "Claim"; 3710 case CLAIMRESPONSE: return "ClaimResponse"; 3711 case CLINICALIMPRESSION: return "ClinicalImpression"; 3712 case CODESYSTEM: return "CodeSystem"; 3713 case COMMUNICATION: return "Communication"; 3714 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 3715 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 3716 case COMPOSITION: return "Composition"; 3717 case CONCEPTMAP: return "ConceptMap"; 3718 case CONDITION: return "Condition"; 3719 case CONSENT: return "Consent"; 3720 case CONTRACT: return "Contract"; 3721 case COVERAGE: return "Coverage"; 3722 case DATAELEMENT: return "DataElement"; 3723 case DETECTEDISSUE: return "DetectedIssue"; 3724 case DEVICE: return "Device"; 3725 case DEVICECOMPONENT: return "DeviceComponent"; 3726 case DEVICEMETRIC: return "DeviceMetric"; 3727 case DEVICEREQUEST: return "DeviceRequest"; 3728 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 3729 case DIAGNOSTICREPORT: return "DiagnosticReport"; 3730 case DOCUMENTMANIFEST: return "DocumentManifest"; 3731 case DOCUMENTREFERENCE: return "DocumentReference"; 3732 case DOMAINRESOURCE: return "DomainResource"; 3733 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 3734 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 3735 case ENCOUNTER: return "Encounter"; 3736 case ENDPOINT: return "Endpoint"; 3737 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 3738 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 3739 case EPISODEOFCARE: return "EpisodeOfCare"; 3740 case EXPANSIONPROFILE: return "ExpansionProfile"; 3741 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 3742 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 3743 case FLAG: return "Flag"; 3744 case GOAL: return "Goal"; 3745 case GRAPHDEFINITION: return "GraphDefinition"; 3746 case GROUP: return "Group"; 3747 case GUIDANCERESPONSE: return "GuidanceResponse"; 3748 case HEALTHCARESERVICE: return "HealthcareService"; 3749 case IMAGINGMANIFEST: return "ImagingManifest"; 3750 case IMAGINGSTUDY: return "ImagingStudy"; 3751 case IMMUNIZATION: return "Immunization"; 3752 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 3753 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 3754 case LIBRARY: return "Library"; 3755 case LINKAGE: return "Linkage"; 3756 case LIST: return "List"; 3757 case LOCATION: return "Location"; 3758 case MEASURE: return "Measure"; 3759 case MEASUREREPORT: return "MeasureReport"; 3760 case MEDIA: return "Media"; 3761 case MEDICATION: return "Medication"; 3762 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 3763 case MEDICATIONDISPENSE: return "MedicationDispense"; 3764 case MEDICATIONREQUEST: return "MedicationRequest"; 3765 case MEDICATIONSTATEMENT: return "MedicationStatement"; 3766 case MESSAGEDEFINITION: return "MessageDefinition"; 3767 case MESSAGEHEADER: return "MessageHeader"; 3768 case NAMINGSYSTEM: return "NamingSystem"; 3769 case NUTRITIONORDER: return "NutritionOrder"; 3770 case OBSERVATION: return "Observation"; 3771 case OPERATIONDEFINITION: return "OperationDefinition"; 3772 case OPERATIONOUTCOME: return "OperationOutcome"; 3773 case ORGANIZATION: return "Organization"; 3774 case PARAMETERS: return "Parameters"; 3775 case PATIENT: return "Patient"; 3776 case PAYMENTNOTICE: return "PaymentNotice"; 3777 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 3778 case PERSON: return "Person"; 3779 case PLANDEFINITION: return "PlanDefinition"; 3780 case PRACTITIONER: return "Practitioner"; 3781 case PRACTITIONERROLE: return "PractitionerRole"; 3782 case PROCEDURE: return "Procedure"; 3783 case PROCEDUREREQUEST: return "ProcedureRequest"; 3784 case PROCESSREQUEST: return "ProcessRequest"; 3785 case PROCESSRESPONSE: return "ProcessResponse"; 3786 case PROVENANCE: return "Provenance"; 3787 case QUESTIONNAIRE: return "Questionnaire"; 3788 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 3789 case REFERRALREQUEST: return "ReferralRequest"; 3790 case RELATEDPERSON: return "RelatedPerson"; 3791 case REQUESTGROUP: return "RequestGroup"; 3792 case RESEARCHSTUDY: return "ResearchStudy"; 3793 case RESEARCHSUBJECT: return "ResearchSubject"; 3794 case RESOURCE: return "Resource"; 3795 case RISKASSESSMENT: return "RiskAssessment"; 3796 case SCHEDULE: return "Schedule"; 3797 case SEARCHPARAMETER: return "SearchParameter"; 3798 case SEQUENCE: return "Sequence"; 3799 case SERVICEDEFINITION: return "ServiceDefinition"; 3800 case SLOT: return "Slot"; 3801 case SPECIMEN: return "Specimen"; 3802 case STRUCTUREDEFINITION: return "StructureDefinition"; 3803 case STRUCTUREMAP: return "StructureMap"; 3804 case SUBSCRIPTION: return "Subscription"; 3805 case SUBSTANCE: return "Substance"; 3806 case SUPPLYDELIVERY: return "SupplyDelivery"; 3807 case SUPPLYREQUEST: return "SupplyRequest"; 3808 case TASK: return "Task"; 3809 case TESTREPORT: return "TestReport"; 3810 case TESTSCRIPT: return "TestScript"; 3811 case VALUESET: return "ValueSet"; 3812 case VISIONPRESCRIPTION: return "VisionPrescription"; 3813 case TYPE: return "Type"; 3814 case ANY: return "Any"; 3815 case NULL: return null; 3816 default: return "?"; 3817 } 3818 } 3819 } 3820 3821 public static class FHIRAllTypesEnumFactory implements EnumFactory<FHIRAllTypes> { 3822 public FHIRAllTypes fromCode(String codeString) throws IllegalArgumentException { 3823 if (codeString == null || "".equals(codeString)) 3824 if (codeString == null || "".equals(codeString)) 3825 return null; 3826 if ("Address".equals(codeString)) 3827 return FHIRAllTypes.ADDRESS; 3828 if ("Age".equals(codeString)) 3829 return FHIRAllTypes.AGE; 3830 if ("Annotation".equals(codeString)) 3831 return FHIRAllTypes.ANNOTATION; 3832 if ("Attachment".equals(codeString)) 3833 return FHIRAllTypes.ATTACHMENT; 3834 if ("BackboneElement".equals(codeString)) 3835 return FHIRAllTypes.BACKBONEELEMENT; 3836 if ("CodeableConcept".equals(codeString)) 3837 return FHIRAllTypes.CODEABLECONCEPT; 3838 if ("Coding".equals(codeString)) 3839 return FHIRAllTypes.CODING; 3840 if ("ContactDetail".equals(codeString)) 3841 return FHIRAllTypes.CONTACTDETAIL; 3842 if ("ContactPoint".equals(codeString)) 3843 return FHIRAllTypes.CONTACTPOINT; 3844 if ("Contributor".equals(codeString)) 3845 return FHIRAllTypes.CONTRIBUTOR; 3846 if ("Count".equals(codeString)) 3847 return FHIRAllTypes.COUNT; 3848 if ("DataRequirement".equals(codeString)) 3849 return FHIRAllTypes.DATAREQUIREMENT; 3850 if ("Distance".equals(codeString)) 3851 return FHIRAllTypes.DISTANCE; 3852 if ("Dosage".equals(codeString)) 3853 return FHIRAllTypes.DOSAGE; 3854 if ("Duration".equals(codeString)) 3855 return FHIRAllTypes.DURATION; 3856 if ("Element".equals(codeString)) 3857 return FHIRAllTypes.ELEMENT; 3858 if ("ElementDefinition".equals(codeString)) 3859 return FHIRAllTypes.ELEMENTDEFINITION; 3860 if ("Extension".equals(codeString)) 3861 return FHIRAllTypes.EXTENSION; 3862 if ("HumanName".equals(codeString)) 3863 return FHIRAllTypes.HUMANNAME; 3864 if ("Identifier".equals(codeString)) 3865 return FHIRAllTypes.IDENTIFIER; 3866 if ("Meta".equals(codeString)) 3867 return FHIRAllTypes.META; 3868 if ("Money".equals(codeString)) 3869 return FHIRAllTypes.MONEY; 3870 if ("Narrative".equals(codeString)) 3871 return FHIRAllTypes.NARRATIVE; 3872 if ("ParameterDefinition".equals(codeString)) 3873 return FHIRAllTypes.PARAMETERDEFINITION; 3874 if ("Period".equals(codeString)) 3875 return FHIRAllTypes.PERIOD; 3876 if ("Quantity".equals(codeString)) 3877 return FHIRAllTypes.QUANTITY; 3878 if ("Range".equals(codeString)) 3879 return FHIRAllTypes.RANGE; 3880 if ("Ratio".equals(codeString)) 3881 return FHIRAllTypes.RATIO; 3882 if ("Reference".equals(codeString)) 3883 return FHIRAllTypes.REFERENCE; 3884 if ("RelatedArtifact".equals(codeString)) 3885 return FHIRAllTypes.RELATEDARTIFACT; 3886 if ("SampledData".equals(codeString)) 3887 return FHIRAllTypes.SAMPLEDDATA; 3888 if ("Signature".equals(codeString)) 3889 return FHIRAllTypes.SIGNATURE; 3890 if ("SimpleQuantity".equals(codeString)) 3891 return FHIRAllTypes.SIMPLEQUANTITY; 3892 if ("Timing".equals(codeString)) 3893 return FHIRAllTypes.TIMING; 3894 if ("TriggerDefinition".equals(codeString)) 3895 return FHIRAllTypes.TRIGGERDEFINITION; 3896 if ("UsageContext".equals(codeString)) 3897 return FHIRAllTypes.USAGECONTEXT; 3898 if ("base64Binary".equals(codeString)) 3899 return FHIRAllTypes.BASE64BINARY; 3900 if ("boolean".equals(codeString)) 3901 return FHIRAllTypes.BOOLEAN; 3902 if ("code".equals(codeString)) 3903 return FHIRAllTypes.CODE; 3904 if ("date".equals(codeString)) 3905 return FHIRAllTypes.DATE; 3906 if ("dateTime".equals(codeString)) 3907 return FHIRAllTypes.DATETIME; 3908 if ("decimal".equals(codeString)) 3909 return FHIRAllTypes.DECIMAL; 3910 if ("id".equals(codeString)) 3911 return FHIRAllTypes.ID; 3912 if ("instant".equals(codeString)) 3913 return FHIRAllTypes.INSTANT; 3914 if ("integer".equals(codeString)) 3915 return FHIRAllTypes.INTEGER; 3916 if ("markdown".equals(codeString)) 3917 return FHIRAllTypes.MARKDOWN; 3918 if ("oid".equals(codeString)) 3919 return FHIRAllTypes.OID; 3920 if ("positiveInt".equals(codeString)) 3921 return FHIRAllTypes.POSITIVEINT; 3922 if ("string".equals(codeString)) 3923 return FHIRAllTypes.STRING; 3924 if ("time".equals(codeString)) 3925 return FHIRAllTypes.TIME; 3926 if ("unsignedInt".equals(codeString)) 3927 return FHIRAllTypes.UNSIGNEDINT; 3928 if ("uri".equals(codeString)) 3929 return FHIRAllTypes.URI; 3930 if ("uuid".equals(codeString)) 3931 return FHIRAllTypes.UUID; 3932 if ("xhtml".equals(codeString)) 3933 return FHIRAllTypes.XHTML; 3934 if ("Account".equals(codeString)) 3935 return FHIRAllTypes.ACCOUNT; 3936 if ("ActivityDefinition".equals(codeString)) 3937 return FHIRAllTypes.ACTIVITYDEFINITION; 3938 if ("AdverseEvent".equals(codeString)) 3939 return FHIRAllTypes.ADVERSEEVENT; 3940 if ("AllergyIntolerance".equals(codeString)) 3941 return FHIRAllTypes.ALLERGYINTOLERANCE; 3942 if ("Appointment".equals(codeString)) 3943 return FHIRAllTypes.APPOINTMENT; 3944 if ("AppointmentResponse".equals(codeString)) 3945 return FHIRAllTypes.APPOINTMENTRESPONSE; 3946 if ("AuditEvent".equals(codeString)) 3947 return FHIRAllTypes.AUDITEVENT; 3948 if ("Basic".equals(codeString)) 3949 return FHIRAllTypes.BASIC; 3950 if ("Binary".equals(codeString)) 3951 return FHIRAllTypes.BINARY; 3952 if ("BodySite".equals(codeString)) 3953 return FHIRAllTypes.BODYSITE; 3954 if ("Bundle".equals(codeString)) 3955 return FHIRAllTypes.BUNDLE; 3956 if ("CapabilityStatement".equals(codeString)) 3957 return FHIRAllTypes.CAPABILITYSTATEMENT; 3958 if ("CarePlan".equals(codeString)) 3959 return FHIRAllTypes.CAREPLAN; 3960 if ("CareTeam".equals(codeString)) 3961 return FHIRAllTypes.CARETEAM; 3962 if ("ChargeItem".equals(codeString)) 3963 return FHIRAllTypes.CHARGEITEM; 3964 if ("Claim".equals(codeString)) 3965 return FHIRAllTypes.CLAIM; 3966 if ("ClaimResponse".equals(codeString)) 3967 return FHIRAllTypes.CLAIMRESPONSE; 3968 if ("ClinicalImpression".equals(codeString)) 3969 return FHIRAllTypes.CLINICALIMPRESSION; 3970 if ("CodeSystem".equals(codeString)) 3971 return FHIRAllTypes.CODESYSTEM; 3972 if ("Communication".equals(codeString)) 3973 return FHIRAllTypes.COMMUNICATION; 3974 if ("CommunicationRequest".equals(codeString)) 3975 return FHIRAllTypes.COMMUNICATIONREQUEST; 3976 if ("CompartmentDefinition".equals(codeString)) 3977 return FHIRAllTypes.COMPARTMENTDEFINITION; 3978 if ("Composition".equals(codeString)) 3979 return FHIRAllTypes.COMPOSITION; 3980 if ("ConceptMap".equals(codeString)) 3981 return FHIRAllTypes.CONCEPTMAP; 3982 if ("Condition".equals(codeString)) 3983 return FHIRAllTypes.CONDITION; 3984 if ("Consent".equals(codeString)) 3985 return FHIRAllTypes.CONSENT; 3986 if ("Contract".equals(codeString)) 3987 return FHIRAllTypes.CONTRACT; 3988 if ("Coverage".equals(codeString)) 3989 return FHIRAllTypes.COVERAGE; 3990 if ("DataElement".equals(codeString)) 3991 return FHIRAllTypes.DATAELEMENT; 3992 if ("DetectedIssue".equals(codeString)) 3993 return FHIRAllTypes.DETECTEDISSUE; 3994 if ("Device".equals(codeString)) 3995 return FHIRAllTypes.DEVICE; 3996 if ("DeviceComponent".equals(codeString)) 3997 return FHIRAllTypes.DEVICECOMPONENT; 3998 if ("DeviceMetric".equals(codeString)) 3999 return FHIRAllTypes.DEVICEMETRIC; 4000 if ("DeviceRequest".equals(codeString)) 4001 return FHIRAllTypes.DEVICEREQUEST; 4002 if ("DeviceUseStatement".equals(codeString)) 4003 return FHIRAllTypes.DEVICEUSESTATEMENT; 4004 if ("DiagnosticReport".equals(codeString)) 4005 return FHIRAllTypes.DIAGNOSTICREPORT; 4006 if ("DocumentManifest".equals(codeString)) 4007 return FHIRAllTypes.DOCUMENTMANIFEST; 4008 if ("DocumentReference".equals(codeString)) 4009 return FHIRAllTypes.DOCUMENTREFERENCE; 4010 if ("DomainResource".equals(codeString)) 4011 return FHIRAllTypes.DOMAINRESOURCE; 4012 if ("EligibilityRequest".equals(codeString)) 4013 return FHIRAllTypes.ELIGIBILITYREQUEST; 4014 if ("EligibilityResponse".equals(codeString)) 4015 return FHIRAllTypes.ELIGIBILITYRESPONSE; 4016 if ("Encounter".equals(codeString)) 4017 return FHIRAllTypes.ENCOUNTER; 4018 if ("Endpoint".equals(codeString)) 4019 return FHIRAllTypes.ENDPOINT; 4020 if ("EnrollmentRequest".equals(codeString)) 4021 return FHIRAllTypes.ENROLLMENTREQUEST; 4022 if ("EnrollmentResponse".equals(codeString)) 4023 return FHIRAllTypes.ENROLLMENTRESPONSE; 4024 if ("EpisodeOfCare".equals(codeString)) 4025 return FHIRAllTypes.EPISODEOFCARE; 4026 if ("ExpansionProfile".equals(codeString)) 4027 return FHIRAllTypes.EXPANSIONPROFILE; 4028 if ("ExplanationOfBenefit".equals(codeString)) 4029 return FHIRAllTypes.EXPLANATIONOFBENEFIT; 4030 if ("FamilyMemberHistory".equals(codeString)) 4031 return FHIRAllTypes.FAMILYMEMBERHISTORY; 4032 if ("Flag".equals(codeString)) 4033 return FHIRAllTypes.FLAG; 4034 if ("Goal".equals(codeString)) 4035 return FHIRAllTypes.GOAL; 4036 if ("GraphDefinition".equals(codeString)) 4037 return FHIRAllTypes.GRAPHDEFINITION; 4038 if ("Group".equals(codeString)) 4039 return FHIRAllTypes.GROUP; 4040 if ("GuidanceResponse".equals(codeString)) 4041 return FHIRAllTypes.GUIDANCERESPONSE; 4042 if ("HealthcareService".equals(codeString)) 4043 return FHIRAllTypes.HEALTHCARESERVICE; 4044 if ("ImagingManifest".equals(codeString)) 4045 return FHIRAllTypes.IMAGINGMANIFEST; 4046 if ("ImagingStudy".equals(codeString)) 4047 return FHIRAllTypes.IMAGINGSTUDY; 4048 if ("Immunization".equals(codeString)) 4049 return FHIRAllTypes.IMMUNIZATION; 4050 if ("ImmunizationRecommendation".equals(codeString)) 4051 return FHIRAllTypes.IMMUNIZATIONRECOMMENDATION; 4052 if ("ImplementationGuide".equals(codeString)) 4053 return FHIRAllTypes.IMPLEMENTATIONGUIDE; 4054 if ("Library".equals(codeString)) 4055 return FHIRAllTypes.LIBRARY; 4056 if ("Linkage".equals(codeString)) 4057 return FHIRAllTypes.LINKAGE; 4058 if ("List".equals(codeString)) 4059 return FHIRAllTypes.LIST; 4060 if ("Location".equals(codeString)) 4061 return FHIRAllTypes.LOCATION; 4062 if ("Measure".equals(codeString)) 4063 return FHIRAllTypes.MEASURE; 4064 if ("MeasureReport".equals(codeString)) 4065 return FHIRAllTypes.MEASUREREPORT; 4066 if ("Media".equals(codeString)) 4067 return FHIRAllTypes.MEDIA; 4068 if ("Medication".equals(codeString)) 4069 return FHIRAllTypes.MEDICATION; 4070 if ("MedicationAdministration".equals(codeString)) 4071 return FHIRAllTypes.MEDICATIONADMINISTRATION; 4072 if ("MedicationDispense".equals(codeString)) 4073 return FHIRAllTypes.MEDICATIONDISPENSE; 4074 if ("MedicationRequest".equals(codeString)) 4075 return FHIRAllTypes.MEDICATIONREQUEST; 4076 if ("MedicationStatement".equals(codeString)) 4077 return FHIRAllTypes.MEDICATIONSTATEMENT; 4078 if ("MessageDefinition".equals(codeString)) 4079 return FHIRAllTypes.MESSAGEDEFINITION; 4080 if ("MessageHeader".equals(codeString)) 4081 return FHIRAllTypes.MESSAGEHEADER; 4082 if ("NamingSystem".equals(codeString)) 4083 return FHIRAllTypes.NAMINGSYSTEM; 4084 if ("NutritionOrder".equals(codeString)) 4085 return FHIRAllTypes.NUTRITIONORDER; 4086 if ("Observation".equals(codeString)) 4087 return FHIRAllTypes.OBSERVATION; 4088 if ("OperationDefinition".equals(codeString)) 4089 return FHIRAllTypes.OPERATIONDEFINITION; 4090 if ("OperationOutcome".equals(codeString)) 4091 return FHIRAllTypes.OPERATIONOUTCOME; 4092 if ("Organization".equals(codeString)) 4093 return FHIRAllTypes.ORGANIZATION; 4094 if ("Parameters".equals(codeString)) 4095 return FHIRAllTypes.PARAMETERS; 4096 if ("Patient".equals(codeString)) 4097 return FHIRAllTypes.PATIENT; 4098 if ("PaymentNotice".equals(codeString)) 4099 return FHIRAllTypes.PAYMENTNOTICE; 4100 if ("PaymentReconciliation".equals(codeString)) 4101 return FHIRAllTypes.PAYMENTRECONCILIATION; 4102 if ("Person".equals(codeString)) 4103 return FHIRAllTypes.PERSON; 4104 if ("PlanDefinition".equals(codeString)) 4105 return FHIRAllTypes.PLANDEFINITION; 4106 if ("Practitioner".equals(codeString)) 4107 return FHIRAllTypes.PRACTITIONER; 4108 if ("PractitionerRole".equals(codeString)) 4109 return FHIRAllTypes.PRACTITIONERROLE; 4110 if ("Procedure".equals(codeString)) 4111 return FHIRAllTypes.PROCEDURE; 4112 if ("ProcedureRequest".equals(codeString)) 4113 return FHIRAllTypes.PROCEDUREREQUEST; 4114 if ("ProcessRequest".equals(codeString)) 4115 return FHIRAllTypes.PROCESSREQUEST; 4116 if ("ProcessResponse".equals(codeString)) 4117 return FHIRAllTypes.PROCESSRESPONSE; 4118 if ("Provenance".equals(codeString)) 4119 return FHIRAllTypes.PROVENANCE; 4120 if ("Questionnaire".equals(codeString)) 4121 return FHIRAllTypes.QUESTIONNAIRE; 4122 if ("QuestionnaireResponse".equals(codeString)) 4123 return FHIRAllTypes.QUESTIONNAIRERESPONSE; 4124 if ("ReferralRequest".equals(codeString)) 4125 return FHIRAllTypes.REFERRALREQUEST; 4126 if ("RelatedPerson".equals(codeString)) 4127 return FHIRAllTypes.RELATEDPERSON; 4128 if ("RequestGroup".equals(codeString)) 4129 return FHIRAllTypes.REQUESTGROUP; 4130 if ("ResearchStudy".equals(codeString)) 4131 return FHIRAllTypes.RESEARCHSTUDY; 4132 if ("ResearchSubject".equals(codeString)) 4133 return FHIRAllTypes.RESEARCHSUBJECT; 4134 if ("Resource".equals(codeString)) 4135 return FHIRAllTypes.RESOURCE; 4136 if ("RiskAssessment".equals(codeString)) 4137 return FHIRAllTypes.RISKASSESSMENT; 4138 if ("Schedule".equals(codeString)) 4139 return FHIRAllTypes.SCHEDULE; 4140 if ("SearchParameter".equals(codeString)) 4141 return FHIRAllTypes.SEARCHPARAMETER; 4142 if ("Sequence".equals(codeString)) 4143 return FHIRAllTypes.SEQUENCE; 4144 if ("ServiceDefinition".equals(codeString)) 4145 return FHIRAllTypes.SERVICEDEFINITION; 4146 if ("Slot".equals(codeString)) 4147 return FHIRAllTypes.SLOT; 4148 if ("Specimen".equals(codeString)) 4149 return FHIRAllTypes.SPECIMEN; 4150 if ("StructureDefinition".equals(codeString)) 4151 return FHIRAllTypes.STRUCTUREDEFINITION; 4152 if ("StructureMap".equals(codeString)) 4153 return FHIRAllTypes.STRUCTUREMAP; 4154 if ("Subscription".equals(codeString)) 4155 return FHIRAllTypes.SUBSCRIPTION; 4156 if ("Substance".equals(codeString)) 4157 return FHIRAllTypes.SUBSTANCE; 4158 if ("SupplyDelivery".equals(codeString)) 4159 return FHIRAllTypes.SUPPLYDELIVERY; 4160 if ("SupplyRequest".equals(codeString)) 4161 return FHIRAllTypes.SUPPLYREQUEST; 4162 if ("Task".equals(codeString)) 4163 return FHIRAllTypes.TASK; 4164 if ("TestReport".equals(codeString)) 4165 return FHIRAllTypes.TESTREPORT; 4166 if ("TestScript".equals(codeString)) 4167 return FHIRAllTypes.TESTSCRIPT; 4168 if ("ValueSet".equals(codeString)) 4169 return FHIRAllTypes.VALUESET; 4170 if ("VisionPrescription".equals(codeString)) 4171 return FHIRAllTypes.VISIONPRESCRIPTION; 4172 if ("Type".equals(codeString)) 4173 return FHIRAllTypes.TYPE; 4174 if ("Any".equals(codeString)) 4175 return FHIRAllTypes.ANY; 4176 throw new IllegalArgumentException("Unknown FHIRAllTypes code '"+codeString+"'"); 4177 } 4178 public Enumeration<FHIRAllTypes> fromType(PrimitiveType<?> code) throws FHIRException { 4179 if (code == null) 4180 return null; 4181 if (code.isEmpty()) 4182 return new Enumeration<FHIRAllTypes>(this); 4183 String codeString = code.asStringValue(); 4184 if (codeString == null || "".equals(codeString)) 4185 return null; 4186 if ("Address".equals(codeString)) 4187 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ADDRESS); 4188 if ("Age".equals(codeString)) 4189 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.AGE); 4190 if ("Annotation".equals(codeString)) 4191 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ANNOTATION); 4192 if ("Attachment".equals(codeString)) 4193 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ATTACHMENT); 4194 if ("BackboneElement".equals(codeString)) 4195 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BACKBONEELEMENT); 4196 if ("CodeableConcept".equals(codeString)) 4197 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODEABLECONCEPT); 4198 if ("Coding".equals(codeString)) 4199 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODING); 4200 if ("ContactDetail".equals(codeString)) 4201 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTACTDETAIL); 4202 if ("ContactPoint".equals(codeString)) 4203 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTACTPOINT); 4204 if ("Contributor".equals(codeString)) 4205 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTRIBUTOR); 4206 if ("Count".equals(codeString)) 4207 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COUNT); 4208 if ("DataRequirement".equals(codeString)) 4209 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATAREQUIREMENT); 4210 if ("Distance".equals(codeString)) 4211 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DISTANCE); 4212 if ("Dosage".equals(codeString)) 4213 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOSAGE); 4214 if ("Duration".equals(codeString)) 4215 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DURATION); 4216 if ("Element".equals(codeString)) 4217 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELEMENT); 4218 if ("ElementDefinition".equals(codeString)) 4219 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELEMENTDEFINITION); 4220 if ("Extension".equals(codeString)) 4221 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXTENSION); 4222 if ("HumanName".equals(codeString)) 4223 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.HUMANNAME); 4224 if ("Identifier".equals(codeString)) 4225 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IDENTIFIER); 4226 if ("Meta".equals(codeString)) 4227 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.META); 4228 if ("Money".equals(codeString)) 4229 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MONEY); 4230 if ("Narrative".equals(codeString)) 4231 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NARRATIVE); 4232 if ("ParameterDefinition".equals(codeString)) 4233 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PARAMETERDEFINITION); 4234 if ("Period".equals(codeString)) 4235 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PERIOD); 4236 if ("Quantity".equals(codeString)) 4237 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUANTITY); 4238 if ("Range".equals(codeString)) 4239 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RANGE); 4240 if ("Ratio".equals(codeString)) 4241 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RATIO); 4242 if ("Reference".equals(codeString)) 4243 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REFERENCE); 4244 if ("RelatedArtifact".equals(codeString)) 4245 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RELATEDARTIFACT); 4246 if ("SampledData".equals(codeString)) 4247 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SAMPLEDDATA); 4248 if ("Signature".equals(codeString)) 4249 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SIGNATURE); 4250 if ("SimpleQuantity".equals(codeString)) 4251 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SIMPLEQUANTITY); 4252 if ("Timing".equals(codeString)) 4253 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TIMING); 4254 if ("TriggerDefinition".equals(codeString)) 4255 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TRIGGERDEFINITION); 4256 if ("UsageContext".equals(codeString)) 4257 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.USAGECONTEXT); 4258 if ("base64Binary".equals(codeString)) 4259 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BASE64BINARY); 4260 if ("boolean".equals(codeString)) 4261 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BOOLEAN); 4262 if ("code".equals(codeString)) 4263 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODE); 4264 if ("date".equals(codeString)) 4265 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATE); 4266 if ("dateTime".equals(codeString)) 4267 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATETIME); 4268 if ("decimal".equals(codeString)) 4269 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DECIMAL); 4270 if ("id".equals(codeString)) 4271 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ID); 4272 if ("instant".equals(codeString)) 4273 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INSTANT); 4274 if ("integer".equals(codeString)) 4275 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.INTEGER); 4276 if ("markdown".equals(codeString)) 4277 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MARKDOWN); 4278 if ("oid".equals(codeString)) 4279 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OID); 4280 if ("positiveInt".equals(codeString)) 4281 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.POSITIVEINT); 4282 if ("string".equals(codeString)) 4283 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRING); 4284 if ("time".equals(codeString)) 4285 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TIME); 4286 if ("unsignedInt".equals(codeString)) 4287 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.UNSIGNEDINT); 4288 if ("uri".equals(codeString)) 4289 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.URI); 4290 if ("uuid".equals(codeString)) 4291 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.UUID); 4292 if ("xhtml".equals(codeString)) 4293 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.XHTML); 4294 if ("Account".equals(codeString)) 4295 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ACCOUNT); 4296 if ("ActivityDefinition".equals(codeString)) 4297 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ACTIVITYDEFINITION); 4298 if ("AdverseEvent".equals(codeString)) 4299 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ADVERSEEVENT); 4300 if ("AllergyIntolerance".equals(codeString)) 4301 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ALLERGYINTOLERANCE); 4302 if ("Appointment".equals(codeString)) 4303 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.APPOINTMENT); 4304 if ("AppointmentResponse".equals(codeString)) 4305 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.APPOINTMENTRESPONSE); 4306 if ("AuditEvent".equals(codeString)) 4307 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.AUDITEVENT); 4308 if ("Basic".equals(codeString)) 4309 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BASIC); 4310 if ("Binary".equals(codeString)) 4311 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BINARY); 4312 if ("BodySite".equals(codeString)) 4313 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BODYSITE); 4314 if ("Bundle".equals(codeString)) 4315 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.BUNDLE); 4316 if ("CapabilityStatement".equals(codeString)) 4317 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CAPABILITYSTATEMENT); 4318 if ("CarePlan".equals(codeString)) 4319 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CAREPLAN); 4320 if ("CareTeam".equals(codeString)) 4321 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CARETEAM); 4322 if ("ChargeItem".equals(codeString)) 4323 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CHARGEITEM); 4324 if ("Claim".equals(codeString)) 4325 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLAIM); 4326 if ("ClaimResponse".equals(codeString)) 4327 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLAIMRESPONSE); 4328 if ("ClinicalImpression".equals(codeString)) 4329 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CLINICALIMPRESSION); 4330 if ("CodeSystem".equals(codeString)) 4331 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CODESYSTEM); 4332 if ("Communication".equals(codeString)) 4333 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMMUNICATION); 4334 if ("CommunicationRequest".equals(codeString)) 4335 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMMUNICATIONREQUEST); 4336 if ("CompartmentDefinition".equals(codeString)) 4337 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMPARTMENTDEFINITION); 4338 if ("Composition".equals(codeString)) 4339 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COMPOSITION); 4340 if ("ConceptMap".equals(codeString)) 4341 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONCEPTMAP); 4342 if ("Condition".equals(codeString)) 4343 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONDITION); 4344 if ("Consent".equals(codeString)) 4345 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONSENT); 4346 if ("Contract".equals(codeString)) 4347 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.CONTRACT); 4348 if ("Coverage".equals(codeString)) 4349 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.COVERAGE); 4350 if ("DataElement".equals(codeString)) 4351 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DATAELEMENT); 4352 if ("DetectedIssue".equals(codeString)) 4353 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DETECTEDISSUE); 4354 if ("Device".equals(codeString)) 4355 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICE); 4356 if ("DeviceComponent".equals(codeString)) 4357 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICECOMPONENT); 4358 if ("DeviceMetric".equals(codeString)) 4359 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEMETRIC); 4360 if ("DeviceRequest".equals(codeString)) 4361 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEREQUEST); 4362 if ("DeviceUseStatement".equals(codeString)) 4363 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DEVICEUSESTATEMENT); 4364 if ("DiagnosticReport".equals(codeString)) 4365 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DIAGNOSTICREPORT); 4366 if ("DocumentManifest".equals(codeString)) 4367 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOCUMENTMANIFEST); 4368 if ("DocumentReference".equals(codeString)) 4369 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOCUMENTREFERENCE); 4370 if ("DomainResource".equals(codeString)) 4371 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.DOMAINRESOURCE); 4372 if ("EligibilityRequest".equals(codeString)) 4373 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELIGIBILITYREQUEST); 4374 if ("EligibilityResponse".equals(codeString)) 4375 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ELIGIBILITYRESPONSE); 4376 if ("Encounter".equals(codeString)) 4377 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENCOUNTER); 4378 if ("Endpoint".equals(codeString)) 4379 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENDPOINT); 4380 if ("EnrollmentRequest".equals(codeString)) 4381 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENROLLMENTREQUEST); 4382 if ("EnrollmentResponse".equals(codeString)) 4383 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ENROLLMENTRESPONSE); 4384 if ("EpisodeOfCare".equals(codeString)) 4385 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EPISODEOFCARE); 4386 if ("ExpansionProfile".equals(codeString)) 4387 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXPANSIONPROFILE); 4388 if ("ExplanationOfBenefit".equals(codeString)) 4389 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.EXPLANATIONOFBENEFIT); 4390 if ("FamilyMemberHistory".equals(codeString)) 4391 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.FAMILYMEMBERHISTORY); 4392 if ("Flag".equals(codeString)) 4393 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.FLAG); 4394 if ("Goal".equals(codeString)) 4395 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GOAL); 4396 if ("GraphDefinition".equals(codeString)) 4397 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GRAPHDEFINITION); 4398 if ("Group".equals(codeString)) 4399 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GROUP); 4400 if ("GuidanceResponse".equals(codeString)) 4401 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.GUIDANCERESPONSE); 4402 if ("HealthcareService".equals(codeString)) 4403 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.HEALTHCARESERVICE); 4404 if ("ImagingManifest".equals(codeString)) 4405 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMAGINGMANIFEST); 4406 if ("ImagingStudy".equals(codeString)) 4407 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMAGINGSTUDY); 4408 if ("Immunization".equals(codeString)) 4409 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATION); 4410 if ("ImmunizationRecommendation".equals(codeString)) 4411 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMMUNIZATIONRECOMMENDATION); 4412 if ("ImplementationGuide".equals(codeString)) 4413 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.IMPLEMENTATIONGUIDE); 4414 if ("Library".equals(codeString)) 4415 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LIBRARY); 4416 if ("Linkage".equals(codeString)) 4417 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LINKAGE); 4418 if ("List".equals(codeString)) 4419 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LIST); 4420 if ("Location".equals(codeString)) 4421 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.LOCATION); 4422 if ("Measure".equals(codeString)) 4423 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEASURE); 4424 if ("MeasureReport".equals(codeString)) 4425 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEASUREREPORT); 4426 if ("Media".equals(codeString)) 4427 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDIA); 4428 if ("Medication".equals(codeString)) 4429 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATION); 4430 if ("MedicationAdministration".equals(codeString)) 4431 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONADMINISTRATION); 4432 if ("MedicationDispense".equals(codeString)) 4433 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONDISPENSE); 4434 if ("MedicationRequest".equals(codeString)) 4435 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONREQUEST); 4436 if ("MedicationStatement".equals(codeString)) 4437 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MEDICATIONSTATEMENT); 4438 if ("MessageDefinition".equals(codeString)) 4439 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MESSAGEDEFINITION); 4440 if ("MessageHeader".equals(codeString)) 4441 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.MESSAGEHEADER); 4442 if ("NamingSystem".equals(codeString)) 4443 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NAMINGSYSTEM); 4444 if ("NutritionOrder".equals(codeString)) 4445 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.NUTRITIONORDER); 4446 if ("Observation".equals(codeString)) 4447 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OBSERVATION); 4448 if ("OperationDefinition".equals(codeString)) 4449 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OPERATIONDEFINITION); 4450 if ("OperationOutcome".equals(codeString)) 4451 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.OPERATIONOUTCOME); 4452 if ("Organization".equals(codeString)) 4453 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ORGANIZATION); 4454 if ("Parameters".equals(codeString)) 4455 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PARAMETERS); 4456 if ("Patient".equals(codeString)) 4457 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PATIENT); 4458 if ("PaymentNotice".equals(codeString)) 4459 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PAYMENTNOTICE); 4460 if ("PaymentReconciliation".equals(codeString)) 4461 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PAYMENTRECONCILIATION); 4462 if ("Person".equals(codeString)) 4463 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PERSON); 4464 if ("PlanDefinition".equals(codeString)) 4465 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PLANDEFINITION); 4466 if ("Practitioner".equals(codeString)) 4467 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRACTITIONER); 4468 if ("PractitionerRole".equals(codeString)) 4469 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PRACTITIONERROLE); 4470 if ("Procedure".equals(codeString)) 4471 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCEDURE); 4472 if ("ProcedureRequest".equals(codeString)) 4473 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCEDUREREQUEST); 4474 if ("ProcessRequest".equals(codeString)) 4475 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCESSREQUEST); 4476 if ("ProcessResponse".equals(codeString)) 4477 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROCESSRESPONSE); 4478 if ("Provenance".equals(codeString)) 4479 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.PROVENANCE); 4480 if ("Questionnaire".equals(codeString)) 4481 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUESTIONNAIRE); 4482 if ("QuestionnaireResponse".equals(codeString)) 4483 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.QUESTIONNAIRERESPONSE); 4484 if ("ReferralRequest".equals(codeString)) 4485 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REFERRALREQUEST); 4486 if ("RelatedPerson".equals(codeString)) 4487 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RELATEDPERSON); 4488 if ("RequestGroup".equals(codeString)) 4489 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.REQUESTGROUP); 4490 if ("ResearchStudy".equals(codeString)) 4491 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHSTUDY); 4492 if ("ResearchSubject".equals(codeString)) 4493 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESEARCHSUBJECT); 4494 if ("Resource".equals(codeString)) 4495 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RESOURCE); 4496 if ("RiskAssessment".equals(codeString)) 4497 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.RISKASSESSMENT); 4498 if ("Schedule".equals(codeString)) 4499 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SCHEDULE); 4500 if ("SearchParameter".equals(codeString)) 4501 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SEARCHPARAMETER); 4502 if ("Sequence".equals(codeString)) 4503 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SEQUENCE); 4504 if ("ServiceDefinition".equals(codeString)) 4505 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SERVICEDEFINITION); 4506 if ("Slot".equals(codeString)) 4507 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SLOT); 4508 if ("Specimen".equals(codeString)) 4509 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SPECIMEN); 4510 if ("StructureDefinition".equals(codeString)) 4511 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRUCTUREDEFINITION); 4512 if ("StructureMap".equals(codeString)) 4513 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.STRUCTUREMAP); 4514 if ("Subscription".equals(codeString)) 4515 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSCRIPTION); 4516 if ("Substance".equals(codeString)) 4517 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUBSTANCE); 4518 if ("SupplyDelivery".equals(codeString)) 4519 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUPPLYDELIVERY); 4520 if ("SupplyRequest".equals(codeString)) 4521 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.SUPPLYREQUEST); 4522 if ("Task".equals(codeString)) 4523 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TASK); 4524 if ("TestReport".equals(codeString)) 4525 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TESTREPORT); 4526 if ("TestScript".equals(codeString)) 4527 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TESTSCRIPT); 4528 if ("ValueSet".equals(codeString)) 4529 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VALUESET); 4530 if ("VisionPrescription".equals(codeString)) 4531 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.VISIONPRESCRIPTION); 4532 if ("Type".equals(codeString)) 4533 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.TYPE); 4534 if ("Any".equals(codeString)) 4535 return new Enumeration<FHIRAllTypes>(this, FHIRAllTypes.ANY); 4536 throw new FHIRException("Unknown FHIRAllTypes code '"+codeString+"'"); 4537 } 4538 public String toCode(FHIRAllTypes code) { 4539 if (code == FHIRAllTypes.ADDRESS) 4540 return "Address"; 4541 if (code == FHIRAllTypes.AGE) 4542 return "Age"; 4543 if (code == FHIRAllTypes.ANNOTATION) 4544 return "Annotation"; 4545 if (code == FHIRAllTypes.ATTACHMENT) 4546 return "Attachment"; 4547 if (code == FHIRAllTypes.BACKBONEELEMENT) 4548 return "BackboneElement"; 4549 if (code == FHIRAllTypes.CODEABLECONCEPT) 4550 return "CodeableConcept"; 4551 if (code == FHIRAllTypes.CODING) 4552 return "Coding"; 4553 if (code == FHIRAllTypes.CONTACTDETAIL) 4554 return "ContactDetail"; 4555 if (code == FHIRAllTypes.CONTACTPOINT) 4556 return "ContactPoint"; 4557 if (code == FHIRAllTypes.CONTRIBUTOR) 4558 return "Contributor"; 4559 if (code == FHIRAllTypes.COUNT) 4560 return "Count"; 4561 if (code == FHIRAllTypes.DATAREQUIREMENT) 4562 return "DataRequirement"; 4563 if (code == FHIRAllTypes.DISTANCE) 4564 return "Distance"; 4565 if (code == FHIRAllTypes.DOSAGE) 4566 return "Dosage"; 4567 if (code == FHIRAllTypes.DURATION) 4568 return "Duration"; 4569 if (code == FHIRAllTypes.ELEMENT) 4570 return "Element"; 4571 if (code == FHIRAllTypes.ELEMENTDEFINITION) 4572 return "ElementDefinition"; 4573 if (code == FHIRAllTypes.EXTENSION) 4574 return "Extension"; 4575 if (code == FHIRAllTypes.HUMANNAME) 4576 return "HumanName"; 4577 if (code == FHIRAllTypes.IDENTIFIER) 4578 return "Identifier"; 4579 if (code == FHIRAllTypes.META) 4580 return "Meta"; 4581 if (code == FHIRAllTypes.MONEY) 4582 return "Money"; 4583 if (code == FHIRAllTypes.NARRATIVE) 4584 return "Narrative"; 4585 if (code == FHIRAllTypes.PARAMETERDEFINITION) 4586 return "ParameterDefinition"; 4587 if (code == FHIRAllTypes.PERIOD) 4588 return "Period"; 4589 if (code == FHIRAllTypes.QUANTITY) 4590 return "Quantity"; 4591 if (code == FHIRAllTypes.RANGE) 4592 return "Range"; 4593 if (code == FHIRAllTypes.RATIO) 4594 return "Ratio"; 4595 if (code == FHIRAllTypes.REFERENCE) 4596 return "Reference"; 4597 if (code == FHIRAllTypes.RELATEDARTIFACT) 4598 return "RelatedArtifact"; 4599 if (code == FHIRAllTypes.SAMPLEDDATA) 4600 return "SampledData"; 4601 if (code == FHIRAllTypes.SIGNATURE) 4602 return "Signature"; 4603 if (code == FHIRAllTypes.SIMPLEQUANTITY) 4604 return "SimpleQuantity"; 4605 if (code == FHIRAllTypes.TIMING) 4606 return "Timing"; 4607 if (code == FHIRAllTypes.TRIGGERDEFINITION) 4608 return "TriggerDefinition"; 4609 if (code == FHIRAllTypes.USAGECONTEXT) 4610 return "UsageContext"; 4611 if (code == FHIRAllTypes.BASE64BINARY) 4612 return "base64Binary"; 4613 if (code == FHIRAllTypes.BOOLEAN) 4614 return "boolean"; 4615 if (code == FHIRAllTypes.CODE) 4616 return "code"; 4617 if (code == FHIRAllTypes.DATE) 4618 return "date"; 4619 if (code == FHIRAllTypes.DATETIME) 4620 return "dateTime"; 4621 if (code == FHIRAllTypes.DECIMAL) 4622 return "decimal"; 4623 if (code == FHIRAllTypes.ID) 4624 return "id"; 4625 if (code == FHIRAllTypes.INSTANT) 4626 return "instant"; 4627 if (code == FHIRAllTypes.INTEGER) 4628 return "integer"; 4629 if (code == FHIRAllTypes.MARKDOWN) 4630 return "markdown"; 4631 if (code == FHIRAllTypes.OID) 4632 return "oid"; 4633 if (code == FHIRAllTypes.POSITIVEINT) 4634 return "positiveInt"; 4635 if (code == FHIRAllTypes.STRING) 4636 return "string"; 4637 if (code == FHIRAllTypes.TIME) 4638 return "time"; 4639 if (code == FHIRAllTypes.UNSIGNEDINT) 4640 return "unsignedInt"; 4641 if (code == FHIRAllTypes.URI) 4642 return "uri"; 4643 if (code == FHIRAllTypes.UUID) 4644 return "uuid"; 4645 if (code == FHIRAllTypes.XHTML) 4646 return "xhtml"; 4647 if (code == FHIRAllTypes.ACCOUNT) 4648 return "Account"; 4649 if (code == FHIRAllTypes.ACTIVITYDEFINITION) 4650 return "ActivityDefinition"; 4651 if (code == FHIRAllTypes.ADVERSEEVENT) 4652 return "AdverseEvent"; 4653 if (code == FHIRAllTypes.ALLERGYINTOLERANCE) 4654 return "AllergyIntolerance"; 4655 if (code == FHIRAllTypes.APPOINTMENT) 4656 return "Appointment"; 4657 if (code == FHIRAllTypes.APPOINTMENTRESPONSE) 4658 return "AppointmentResponse"; 4659 if (code == FHIRAllTypes.AUDITEVENT) 4660 return "AuditEvent"; 4661 if (code == FHIRAllTypes.BASIC) 4662 return "Basic"; 4663 if (code == FHIRAllTypes.BINARY) 4664 return "Binary"; 4665 if (code == FHIRAllTypes.BODYSITE) 4666 return "BodySite"; 4667 if (code == FHIRAllTypes.BUNDLE) 4668 return "Bundle"; 4669 if (code == FHIRAllTypes.CAPABILITYSTATEMENT) 4670 return "CapabilityStatement"; 4671 if (code == FHIRAllTypes.CAREPLAN) 4672 return "CarePlan"; 4673 if (code == FHIRAllTypes.CARETEAM) 4674 return "CareTeam"; 4675 if (code == FHIRAllTypes.CHARGEITEM) 4676 return "ChargeItem"; 4677 if (code == FHIRAllTypes.CLAIM) 4678 return "Claim"; 4679 if (code == FHIRAllTypes.CLAIMRESPONSE) 4680 return "ClaimResponse"; 4681 if (code == FHIRAllTypes.CLINICALIMPRESSION) 4682 return "ClinicalImpression"; 4683 if (code == FHIRAllTypes.CODESYSTEM) 4684 return "CodeSystem"; 4685 if (code == FHIRAllTypes.COMMUNICATION) 4686 return "Communication"; 4687 if (code == FHIRAllTypes.COMMUNICATIONREQUEST) 4688 return "CommunicationRequest"; 4689 if (code == FHIRAllTypes.COMPARTMENTDEFINITION) 4690 return "CompartmentDefinition"; 4691 if (code == FHIRAllTypes.COMPOSITION) 4692 return "Composition"; 4693 if (code == FHIRAllTypes.CONCEPTMAP) 4694 return "ConceptMap"; 4695 if (code == FHIRAllTypes.CONDITION) 4696 return "Condition"; 4697 if (code == FHIRAllTypes.CONSENT) 4698 return "Consent"; 4699 if (code == FHIRAllTypes.CONTRACT) 4700 return "Contract"; 4701 if (code == FHIRAllTypes.COVERAGE) 4702 return "Coverage"; 4703 if (code == FHIRAllTypes.DATAELEMENT) 4704 return "DataElement"; 4705 if (code == FHIRAllTypes.DETECTEDISSUE) 4706 return "DetectedIssue"; 4707 if (code == FHIRAllTypes.DEVICE) 4708 return "Device"; 4709 if (code == FHIRAllTypes.DEVICECOMPONENT) 4710 return "DeviceComponent"; 4711 if (code == FHIRAllTypes.DEVICEMETRIC) 4712 return "DeviceMetric"; 4713 if (code == FHIRAllTypes.DEVICEREQUEST) 4714 return "DeviceRequest"; 4715 if (code == FHIRAllTypes.DEVICEUSESTATEMENT) 4716 return "DeviceUseStatement"; 4717 if (code == FHIRAllTypes.DIAGNOSTICREPORT) 4718 return "DiagnosticReport"; 4719 if (code == FHIRAllTypes.DOCUMENTMANIFEST) 4720 return "DocumentManifest"; 4721 if (code == FHIRAllTypes.DOCUMENTREFERENCE) 4722 return "DocumentReference"; 4723 if (code == FHIRAllTypes.DOMAINRESOURCE) 4724 return "DomainResource"; 4725 if (code == FHIRAllTypes.ELIGIBILITYREQUEST) 4726 return "EligibilityRequest"; 4727 if (code == FHIRAllTypes.ELIGIBILITYRESPONSE) 4728 return "EligibilityResponse"; 4729 if (code == FHIRAllTypes.ENCOUNTER) 4730 return "Encounter"; 4731 if (code == FHIRAllTypes.ENDPOINT) 4732 return "Endpoint"; 4733 if (code == FHIRAllTypes.ENROLLMENTREQUEST) 4734 return "EnrollmentRequest"; 4735 if (code == FHIRAllTypes.ENROLLMENTRESPONSE) 4736 return "EnrollmentResponse"; 4737 if (code == FHIRAllTypes.EPISODEOFCARE) 4738 return "EpisodeOfCare"; 4739 if (code == FHIRAllTypes.EXPANSIONPROFILE) 4740 return "ExpansionProfile"; 4741 if (code == FHIRAllTypes.EXPLANATIONOFBENEFIT) 4742 return "ExplanationOfBenefit"; 4743 if (code == FHIRAllTypes.FAMILYMEMBERHISTORY) 4744 return "FamilyMemberHistory"; 4745 if (code == FHIRAllTypes.FLAG) 4746 return "Flag"; 4747 if (code == FHIRAllTypes.GOAL) 4748 return "Goal"; 4749 if (code == FHIRAllTypes.GRAPHDEFINITION) 4750 return "GraphDefinition"; 4751 if (code == FHIRAllTypes.GROUP) 4752 return "Group"; 4753 if (code == FHIRAllTypes.GUIDANCERESPONSE) 4754 return "GuidanceResponse"; 4755 if (code == FHIRAllTypes.HEALTHCARESERVICE) 4756 return "HealthcareService"; 4757 if (code == FHIRAllTypes.IMAGINGMANIFEST) 4758 return "ImagingManifest"; 4759 if (code == FHIRAllTypes.IMAGINGSTUDY) 4760 return "ImagingStudy"; 4761 if (code == FHIRAllTypes.IMMUNIZATION) 4762 return "Immunization"; 4763 if (code == FHIRAllTypes.IMMUNIZATIONRECOMMENDATION) 4764 return "ImmunizationRecommendation"; 4765 if (code == FHIRAllTypes.IMPLEMENTATIONGUIDE) 4766 return "ImplementationGuide"; 4767 if (code == FHIRAllTypes.LIBRARY) 4768 return "Library"; 4769 if (code == FHIRAllTypes.LINKAGE) 4770 return "Linkage"; 4771 if (code == FHIRAllTypes.LIST) 4772 return "List"; 4773 if (code == FHIRAllTypes.LOCATION) 4774 return "Location"; 4775 if (code == FHIRAllTypes.MEASURE) 4776 return "Measure"; 4777 if (code == FHIRAllTypes.MEASUREREPORT) 4778 return "MeasureReport"; 4779 if (code == FHIRAllTypes.MEDIA) 4780 return "Media"; 4781 if (code == FHIRAllTypes.MEDICATION) 4782 return "Medication"; 4783 if (code == FHIRAllTypes.MEDICATIONADMINISTRATION) 4784 return "MedicationAdministration"; 4785 if (code == FHIRAllTypes.MEDICATIONDISPENSE) 4786 return "MedicationDispense"; 4787 if (code == FHIRAllTypes.MEDICATIONREQUEST) 4788 return "MedicationRequest"; 4789 if (code == FHIRAllTypes.MEDICATIONSTATEMENT) 4790 return "MedicationStatement"; 4791 if (code == FHIRAllTypes.MESSAGEDEFINITION) 4792 return "MessageDefinition"; 4793 if (code == FHIRAllTypes.MESSAGEHEADER) 4794 return "MessageHeader"; 4795 if (code == FHIRAllTypes.NAMINGSYSTEM) 4796 return "NamingSystem"; 4797 if (code == FHIRAllTypes.NUTRITIONORDER) 4798 return "NutritionOrder"; 4799 if (code == FHIRAllTypes.OBSERVATION) 4800 return "Observation"; 4801 if (code == FHIRAllTypes.OPERATIONDEFINITION) 4802 return "OperationDefinition"; 4803 if (code == FHIRAllTypes.OPERATIONOUTCOME) 4804 return "OperationOutcome"; 4805 if (code == FHIRAllTypes.ORGANIZATION) 4806 return "Organization"; 4807 if (code == FHIRAllTypes.PARAMETERS) 4808 return "Parameters"; 4809 if (code == FHIRAllTypes.PATIENT) 4810 return "Patient"; 4811 if (code == FHIRAllTypes.PAYMENTNOTICE) 4812 return "PaymentNotice"; 4813 if (code == FHIRAllTypes.PAYMENTRECONCILIATION) 4814 return "PaymentReconciliation"; 4815 if (code == FHIRAllTypes.PERSON) 4816 return "Person"; 4817 if (code == FHIRAllTypes.PLANDEFINITION) 4818 return "PlanDefinition"; 4819 if (code == FHIRAllTypes.PRACTITIONER) 4820 return "Practitioner"; 4821 if (code == FHIRAllTypes.PRACTITIONERROLE) 4822 return "PractitionerRole"; 4823 if (code == FHIRAllTypes.PROCEDURE) 4824 return "Procedure"; 4825 if (code == FHIRAllTypes.PROCEDUREREQUEST) 4826 return "ProcedureRequest"; 4827 if (code == FHIRAllTypes.PROCESSREQUEST) 4828 return "ProcessRequest"; 4829 if (code == FHIRAllTypes.PROCESSRESPONSE) 4830 return "ProcessResponse"; 4831 if (code == FHIRAllTypes.PROVENANCE) 4832 return "Provenance"; 4833 if (code == FHIRAllTypes.QUESTIONNAIRE) 4834 return "Questionnaire"; 4835 if (code == FHIRAllTypes.QUESTIONNAIRERESPONSE) 4836 return "QuestionnaireResponse"; 4837 if (code == FHIRAllTypes.REFERRALREQUEST) 4838 return "ReferralRequest"; 4839 if (code == FHIRAllTypes.RELATEDPERSON) 4840 return "RelatedPerson"; 4841 if (code == FHIRAllTypes.REQUESTGROUP) 4842 return "RequestGroup"; 4843 if (code == FHIRAllTypes.RESEARCHSTUDY) 4844 return "ResearchStudy"; 4845 if (code == FHIRAllTypes.RESEARCHSUBJECT) 4846 return "ResearchSubject"; 4847 if (code == FHIRAllTypes.RESOURCE) 4848 return "Resource"; 4849 if (code == FHIRAllTypes.RISKASSESSMENT) 4850 return "RiskAssessment"; 4851 if (code == FHIRAllTypes.SCHEDULE) 4852 return "Schedule"; 4853 if (code == FHIRAllTypes.SEARCHPARAMETER) 4854 return "SearchParameter"; 4855 if (code == FHIRAllTypes.SEQUENCE) 4856 return "Sequence"; 4857 if (code == FHIRAllTypes.SERVICEDEFINITION) 4858 return "ServiceDefinition"; 4859 if (code == FHIRAllTypes.SLOT) 4860 return "Slot"; 4861 if (code == FHIRAllTypes.SPECIMEN) 4862 return "Specimen"; 4863 if (code == FHIRAllTypes.STRUCTUREDEFINITION) 4864 return "StructureDefinition"; 4865 if (code == FHIRAllTypes.STRUCTUREMAP) 4866 return "StructureMap"; 4867 if (code == FHIRAllTypes.SUBSCRIPTION) 4868 return "Subscription"; 4869 if (code == FHIRAllTypes.SUBSTANCE) 4870 return "Substance"; 4871 if (code == FHIRAllTypes.SUPPLYDELIVERY) 4872 return "SupplyDelivery"; 4873 if (code == FHIRAllTypes.SUPPLYREQUEST) 4874 return "SupplyRequest"; 4875 if (code == FHIRAllTypes.TASK) 4876 return "Task"; 4877 if (code == FHIRAllTypes.TESTREPORT) 4878 return "TestReport"; 4879 if (code == FHIRAllTypes.TESTSCRIPT) 4880 return "TestScript"; 4881 if (code == FHIRAllTypes.VALUESET) 4882 return "ValueSet"; 4883 if (code == FHIRAllTypes.VISIONPRESCRIPTION) 4884 return "VisionPrescription"; 4885 if (code == FHIRAllTypes.TYPE) 4886 return "Type"; 4887 if (code == FHIRAllTypes.ANY) 4888 return "Any"; 4889 return "?"; 4890 } 4891 public String toSystem(FHIRAllTypes code) { 4892 return code.getSystem(); 4893 } 4894 } 4895 4896 public enum FHIRDefinedType { 4897 /** 4898 * An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world. 4899 */ 4900 ADDRESS, 4901 /** 4902 * A duration of time during which an organism (or a process) has existed. 4903 */ 4904 AGE, 4905 /** 4906 * A text note which also contains information about who made the statement and when. 4907 */ 4908 ANNOTATION, 4909 /** 4910 * For referring to data content defined in other formats. 4911 */ 4912 ATTACHMENT, 4913 /** 4914 * Base definition for all elements that are defined inside a resource - but not those in a data type. 4915 */ 4916 BACKBONEELEMENT, 4917 /** 4918 * A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text. 4919 */ 4920 CODEABLECONCEPT, 4921 /** 4922 * A reference to a code defined by a terminology system. 4923 */ 4924 CODING, 4925 /** 4926 * Specifies contact information for a person or organization. 4927 */ 4928 CONTACTDETAIL, 4929 /** 4930 * Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc. 4931 */ 4932 CONTACTPOINT, 4933 /** 4934 * A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers. 4935 */ 4936 CONTRIBUTOR, 4937 /** 4938 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 4939 */ 4940 COUNT, 4941 /** 4942 * Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data. 4943 */ 4944 DATAREQUIREMENT, 4945 /** 4946 * A length - a value with a unit that is a physical distance. 4947 */ 4948 DISTANCE, 4949 /** 4950 * Indicates how the medication is/was taken or should be taken by the patient. 4951 */ 4952 DOSAGE, 4953 /** 4954 * A length of time. 4955 */ 4956 DURATION, 4957 /** 4958 * Base definition for all elements in a resource. 4959 */ 4960 ELEMENT, 4961 /** 4962 * Captures constraints on each element within the resource, profile, or extension. 4963 */ 4964 ELEMENTDEFINITION, 4965 /** 4966 * Optional Extension Element - found in all resources. 4967 */ 4968 EXTENSION, 4969 /** 4970 * A human's name with the ability to identify parts and usage. 4971 */ 4972 HUMANNAME, 4973 /** 4974 * A technical identifier - identifies some entity uniquely and unambiguously. 4975 */ 4976 IDENTIFIER, 4977 /** 4978 * The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource. 4979 */ 4980 META, 4981 /** 4982 * An amount of economic utility in some recognized currency. 4983 */ 4984 MONEY, 4985 /** 4986 * A human-readable formatted text, including images. 4987 */ 4988 NARRATIVE, 4989 /** 4990 * The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse. 4991 */ 4992 PARAMETERDEFINITION, 4993 /** 4994 * A time period defined by a start and end date and optionally time. 4995 */ 4996 PERIOD, 4997 /** 4998 * A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies. 4999 */ 5000 QUANTITY, 5001 /** 5002 * A set of ordered Quantities defined by a low and high limit. 5003 */ 5004 RANGE, 5005 /** 5006 * A relationship of two Quantity values - expressed as a numerator and a denominator. 5007 */ 5008 RATIO, 5009 /** 5010 * A reference from one resource to another. 5011 */ 5012 REFERENCE, 5013 /** 5014 * Related artifacts such as additional documentation, justification, or bibliographic references. 5015 */ 5016 RELATEDARTIFACT, 5017 /** 5018 * A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data. 5019 */ 5020 SAMPLEDDATA, 5021 /** 5022 * A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities. 5023 */ 5024 SIGNATURE, 5025 /** 5026 * null 5027 */ 5028 SIMPLEQUANTITY, 5029 /** 5030 * Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out. 5031 */ 5032 TIMING, 5033 /** 5034 * A description of a triggering event. 5035 */ 5036 TRIGGERDEFINITION, 5037 /** 5038 * Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care). 5039 */ 5040 USAGECONTEXT, 5041 /** 5042 * A stream of bytes 5043 */ 5044 BASE64BINARY, 5045 /** 5046 * Value of "true" or "false" 5047 */ 5048 BOOLEAN, 5049 /** 5050 * A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents 5051 */ 5052 CODE, 5053 /** 5054 * A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates. 5055 */ 5056 DATE, 5057 /** 5058 * A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates. 5059 */ 5060 DATETIME, 5061 /** 5062 * A rational number with implicit precision 5063 */ 5064 DECIMAL, 5065 /** 5066 * Any combination of letters, numerals, "-" and ".", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive. 5067 */ 5068 ID, 5069 /** 5070 * An instant in time - known at least to the second 5071 */ 5072 INSTANT, 5073 /** 5074 * A whole number 5075 */ 5076 INTEGER, 5077 /** 5078 * A string that may contain markdown syntax for optional processing by a mark down presentation engine 5079 */ 5080 MARKDOWN, 5081 /** 5082 * An OID represented as a URI 5083 */ 5084 OID, 5085 /** 5086 * An integer with a value that is positive (e.g. >0) 5087 */ 5088 POSITIVEINT, 5089 /** 5090 * A sequence of Unicode characters 5091 */ 5092 STRING, 5093 /** 5094 * A time during the day, with no date specified 5095 */ 5096 TIME, 5097 /** 5098 * An integer with a value that is not negative (e.g. >= 0) 5099 */ 5100 UNSIGNEDINT, 5101 /** 5102 * String of characters used to identify a name or a resource 5103 */ 5104 URI, 5105 /** 5106 * A UUID, represented as a URI 5107 */ 5108 UUID, 5109 /** 5110 * XHTML format, as defined by W3C, but restricted usage (mainly, no active content) 5111 */ 5112 XHTML, 5113 /** 5114 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 5115 */ 5116 ACCOUNT, 5117 /** 5118 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 5119 */ 5120 ACTIVITYDEFINITION, 5121 /** 5122 * Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death. 5123 */ 5124 ADVERSEEVENT, 5125 /** 5126 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 5127 */ 5128 ALLERGYINTOLERANCE, 5129 /** 5130 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 5131 */ 5132 APPOINTMENT, 5133 /** 5134 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 5135 */ 5136 APPOINTMENTRESPONSE, 5137 /** 5138 * A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage. 5139 */ 5140 AUDITEVENT, 5141 /** 5142 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 5143 */ 5144 BASIC, 5145 /** 5146 * A binary resource can contain any content, whether text, image, pdf, zip archive, etc. 5147 */ 5148 BINARY, 5149 /** 5150 * Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 5151 */ 5152 BODYSITE, 5153 /** 5154 * A container for a collection of resources. 5155 */ 5156 BUNDLE, 5157 /** 5158 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 5159 */ 5160 CAPABILITYSTATEMENT, 5161 /** 5162 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 5163 */ 5164 CAREPLAN, 5165 /** 5166 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 5167 */ 5168 CARETEAM, 5169 /** 5170 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 5171 */ 5172 CHARGEITEM, 5173 /** 5174 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 5175 */ 5176 CLAIM, 5177 /** 5178 * This resource provides the adjudication details from the processing of a Claim resource. 5179 */ 5180 CLAIMRESPONSE, 5181 /** 5182 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 5183 */ 5184 CLINICALIMPRESSION, 5185 /** 5186 * A code system resource specifies a set of codes drawn from one or more code systems. 5187 */ 5188 CODESYSTEM, 5189 /** 5190 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 5191 */ 5192 COMMUNICATION, 5193 /** 5194 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 5195 */ 5196 COMMUNICATIONREQUEST, 5197 /** 5198 * A compartment definition that defines how resources are accessed on a server. 5199 */ 5200 COMPARTMENTDEFINITION, 5201 /** 5202 * A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained. 5203 */ 5204 COMPOSITION, 5205 /** 5206 * A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models. 5207 */ 5208 CONCEPTMAP, 5209 /** 5210 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 5211 */ 5212 CONDITION, 5213 /** 5214 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 5215 */ 5216 CONSENT, 5217 /** 5218 * A formal agreement between parties regarding the conduct of business, exchange of information or other matters. 5219 */ 5220 CONTRACT, 5221 /** 5222 * Financial instrument which may be used to reimburse or pay for health care products and services. 5223 */ 5224 COVERAGE, 5225 /** 5226 * The formal description of a single piece of information that can be gathered and reported. 5227 */ 5228 DATAELEMENT, 5229 /** 5230 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 5231 */ 5232 DETECTEDISSUE, 5233 /** 5234 * This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc. 5235 */ 5236 DEVICE, 5237 /** 5238 * The characteristics, operational status and capabilities of a medical-related component of a medical device. 5239 */ 5240 DEVICECOMPONENT, 5241 /** 5242 * Describes a measurement, calculation or setting capability of a medical device. 5243 */ 5244 DEVICEMETRIC, 5245 /** 5246 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 5247 */ 5248 DEVICEREQUEST, 5249 /** 5250 * A record of a device being used by a patient where the record is the result of a report from the patient or another clinician. 5251 */ 5252 DEVICEUSESTATEMENT, 5253 /** 5254 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 5255 */ 5256 DIAGNOSTICREPORT, 5257 /** 5258 * A collection of documents compiled for a purpose together with metadata that applies to the collection. 5259 */ 5260 DOCUMENTMANIFEST, 5261 /** 5262 * A reference to a document. 5263 */ 5264 DOCUMENTREFERENCE, 5265 /** 5266 * A resource that includes narrative, extensions, and contained resources. 5267 */ 5268 DOMAINRESOURCE, 5269 /** 5270 * The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 5271 */ 5272 ELIGIBILITYREQUEST, 5273 /** 5274 * This resource provides eligibility and plan details from the processing of an Eligibility resource. 5275 */ 5276 ELIGIBILITYRESPONSE, 5277 /** 5278 * An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient. 5279 */ 5280 ENCOUNTER, 5281 /** 5282 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 5283 */ 5284 ENDPOINT, 5285 /** 5286 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 5287 */ 5288 ENROLLMENTREQUEST, 5289 /** 5290 * This resource provides enrollment and plan details from the processing of an Enrollment resource. 5291 */ 5292 ENROLLMENTRESPONSE, 5293 /** 5294 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 5295 */ 5296 EPISODEOFCARE, 5297 /** 5298 * Resource to define constraints on the Expansion of a FHIR ValueSet. 5299 */ 5300 EXPANSIONPROFILE, 5301 /** 5302 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 5303 */ 5304 EXPLANATIONOFBENEFIT, 5305 /** 5306 * Significant health events and conditions for a person related to the patient relevant in the context of care for the patient. 5307 */ 5308 FAMILYMEMBERHISTORY, 5309 /** 5310 * Prospective warnings of potential issues when providing care to the patient. 5311 */ 5312 FLAG, 5313 /** 5314 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 5315 */ 5316 GOAL, 5317 /** 5318 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 5319 */ 5320 GRAPHDEFINITION, 5321 /** 5322 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 5323 */ 5324 GROUP, 5325 /** 5326 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 5327 */ 5328 GUIDANCERESPONSE, 5329 /** 5330 * The details of a healthcare service available at a location. 5331 */ 5332 HEALTHCARESERVICE, 5333 /** 5334 * A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection. 5335 */ 5336 IMAGINGMANIFEST, 5337 /** 5338 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 5339 */ 5340 IMAGINGSTUDY, 5341 /** 5342 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 5343 */ 5344 IMMUNIZATION, 5345 /** 5346 * A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification. 5347 */ 5348 IMMUNIZATIONRECOMMENDATION, 5349 /** 5350 * A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 5351 */ 5352 IMPLEMENTATIONGUIDE, 5353 /** 5354 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 5355 */ 5356 LIBRARY, 5357 /** 5358 * Identifies two or more records (resource instances) that are referring to the same real-world "occurrence". 5359 */ 5360 LINKAGE, 5361 /** 5362 * A set of information summarized from a list of other resources. 5363 */ 5364 LIST, 5365 /** 5366 * Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated. 5367 */ 5368 LOCATION, 5369 /** 5370 * The Measure resource provides the definition of a quality measure. 5371 */ 5372 MEASURE, 5373 /** 5374 * The MeasureReport resource contains the results of evaluating a measure. 5375 */ 5376 MEASUREREPORT, 5377 /** 5378 * A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference. 5379 */ 5380 MEDIA, 5381 /** 5382 * This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication. 5383 */ 5384 MEDICATION, 5385 /** 5386 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. 5387 */ 5388 MEDICATIONADMINISTRATION, 5389 /** 5390 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 5391 */ 5392 MEDICATIONDISPENSE, 5393 /** 5394 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 5395 */ 5396 MEDICATIONREQUEST, 5397 /** 5398 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 5399 5400The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 5401 */ 5402 MEDICATIONSTATEMENT, 5403 /** 5404 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 5405 */ 5406 MESSAGEDEFINITION, 5407 /** 5408 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 5409 */ 5410 MESSAGEHEADER, 5411 /** 5412 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 5413 */ 5414 NAMINGSYSTEM, 5415 /** 5416 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 5417 */ 5418 NUTRITIONORDER, 5419 /** 5420 * Measurements and simple assertions made about a patient, device or other subject. 5421 */ 5422 OBSERVATION, 5423 /** 5424 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 5425 */ 5426 OPERATIONDEFINITION, 5427 /** 5428 * A collection of error, warning or information messages that result from a system action. 5429 */ 5430 OPERATIONOUTCOME, 5431 /** 5432 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc. 5433 */ 5434 ORGANIZATION, 5435 /** 5436 * This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it. 5437 */ 5438 PARAMETERS, 5439 /** 5440 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 5441 */ 5442 PATIENT, 5443 /** 5444 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 5445 */ 5446 PAYMENTNOTICE, 5447 /** 5448 * This resource provides payment details and claim references supporting a bulk payment. 5449 */ 5450 PAYMENTRECONCILIATION, 5451 /** 5452 * Demographics and administrative information about a person independent of a specific health-related context. 5453 */ 5454 PERSON, 5455 /** 5456 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols. 5457 */ 5458 PLANDEFINITION, 5459 /** 5460 * A person who is directly or indirectly involved in the provisioning of healthcare. 5461 */ 5462 PRACTITIONER, 5463 /** 5464 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 5465 */ 5466 PRACTITIONERROLE, 5467 /** 5468 * An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy. 5469 */ 5470 PROCEDURE, 5471 /** 5472 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 5473 */ 5474 PROCEDUREREQUEST, 5475 /** 5476 * This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources. 5477 */ 5478 PROCESSREQUEST, 5479 /** 5480 * This resource provides processing status, errors and notes from the processing of a resource. 5481 */ 5482 PROCESSRESPONSE, 5483 /** 5484 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 5485 */ 5486 PROVENANCE, 5487 /** 5488 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 5489 */ 5490 QUESTIONNAIRE, 5491 /** 5492 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 5493 */ 5494 QUESTIONNAIRERESPONSE, 5495 /** 5496 * Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization. 5497 */ 5498 REFERRALREQUEST, 5499 /** 5500 * Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 5501 */ 5502 RELATEDPERSON, 5503 /** 5504 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 5505 */ 5506 REQUESTGROUP, 5507 /** 5508 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 5509 */ 5510 RESEARCHSTUDY, 5511 /** 5512 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 5513 */ 5514 RESEARCHSUBJECT, 5515 /** 5516 * This is the base resource type for everything. 5517 */ 5518 RESOURCE, 5519 /** 5520 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 5521 */ 5522 RISKASSESSMENT, 5523 /** 5524 * A container for slots of time that may be available for booking appointments. 5525 */ 5526 SCHEDULE, 5527 /** 5528 * A search parameter that defines a named search item that can be used to search/filter on a resource. 5529 */ 5530 SEARCHPARAMETER, 5531 /** 5532 * Raw data describing a biological sequence. 5533 */ 5534 SEQUENCE, 5535 /** 5536 * The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking. 5537 */ 5538 SERVICEDEFINITION, 5539 /** 5540 * A slot of time on a schedule that may be available for booking appointments. 5541 */ 5542 SLOT, 5543 /** 5544 * A sample to be used for analysis. 5545 */ 5546 SPECIMEN, 5547 /** 5548 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 5549 */ 5550 STRUCTUREDEFINITION, 5551 /** 5552 * A Map of relationships between 2 structures that can be used to transform data. 5553 */ 5554 STRUCTUREMAP, 5555 /** 5556 * The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined "channel" so that another system is able to take an appropriate action. 5557 */ 5558 SUBSCRIPTION, 5559 /** 5560 * A homogeneous material with a definite composition. 5561 */ 5562 SUBSTANCE, 5563 /** 5564 * Record of delivery of what is supplied. 5565 */ 5566 SUPPLYDELIVERY, 5567 /** 5568 * A record of a request for a medication, substance or device used in the healthcare setting. 5569 */ 5570 SUPPLYREQUEST, 5571 /** 5572 * A task to be performed. 5573 */ 5574 TASK, 5575 /** 5576 * A summary of information based on the results of executing a TestScript. 5577 */ 5578 TESTREPORT, 5579 /** 5580 * A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification. 5581 */ 5582 TESTSCRIPT, 5583 /** 5584 * A value set specifies a set of codes drawn from one or more code systems. 5585 */ 5586 VALUESET, 5587 /** 5588 * An authorization for the supply of glasses and/or contact lenses to a patient. 5589 */ 5590 VISIONPRESCRIPTION, 5591 /** 5592 * added to help the parsers 5593 */ 5594 NULL; 5595 public static FHIRDefinedType fromCode(String codeString) throws FHIRException { 5596 if (codeString == null || "".equals(codeString)) 5597 return null; 5598 if ("Address".equals(codeString)) 5599 return ADDRESS; 5600 if ("Age".equals(codeString)) 5601 return AGE; 5602 if ("Annotation".equals(codeString)) 5603 return ANNOTATION; 5604 if ("Attachment".equals(codeString)) 5605 return ATTACHMENT; 5606 if ("BackboneElement".equals(codeString)) 5607 return BACKBONEELEMENT; 5608 if ("CodeableConcept".equals(codeString)) 5609 return CODEABLECONCEPT; 5610 if ("Coding".equals(codeString)) 5611 return CODING; 5612 if ("ContactDetail".equals(codeString)) 5613 return CONTACTDETAIL; 5614 if ("ContactPoint".equals(codeString)) 5615 return CONTACTPOINT; 5616 if ("Contributor".equals(codeString)) 5617 return CONTRIBUTOR; 5618 if ("Count".equals(codeString)) 5619 return COUNT; 5620 if ("DataRequirement".equals(codeString)) 5621 return DATAREQUIREMENT; 5622 if ("Distance".equals(codeString)) 5623 return DISTANCE; 5624 if ("Dosage".equals(codeString)) 5625 return DOSAGE; 5626 if ("Duration".equals(codeString)) 5627 return DURATION; 5628 if ("Element".equals(codeString)) 5629 return ELEMENT; 5630 if ("ElementDefinition".equals(codeString)) 5631 return ELEMENTDEFINITION; 5632 if ("Extension".equals(codeString)) 5633 return EXTENSION; 5634 if ("HumanName".equals(codeString)) 5635 return HUMANNAME; 5636 if ("Identifier".equals(codeString)) 5637 return IDENTIFIER; 5638 if ("Meta".equals(codeString)) 5639 return META; 5640 if ("Money".equals(codeString)) 5641 return MONEY; 5642 if ("Narrative".equals(codeString)) 5643 return NARRATIVE; 5644 if ("ParameterDefinition".equals(codeString)) 5645 return PARAMETERDEFINITION; 5646 if ("Period".equals(codeString)) 5647 return PERIOD; 5648 if ("Quantity".equals(codeString)) 5649 return QUANTITY; 5650 if ("Range".equals(codeString)) 5651 return RANGE; 5652 if ("Ratio".equals(codeString)) 5653 return RATIO; 5654 if ("Reference".equals(codeString)) 5655 return REFERENCE; 5656 if ("RelatedArtifact".equals(codeString)) 5657 return RELATEDARTIFACT; 5658 if ("SampledData".equals(codeString)) 5659 return SAMPLEDDATA; 5660 if ("Signature".equals(codeString)) 5661 return SIGNATURE; 5662 if ("SimpleQuantity".equals(codeString)) 5663 return SIMPLEQUANTITY; 5664 if ("Timing".equals(codeString)) 5665 return TIMING; 5666 if ("TriggerDefinition".equals(codeString)) 5667 return TRIGGERDEFINITION; 5668 if ("UsageContext".equals(codeString)) 5669 return USAGECONTEXT; 5670 if ("base64Binary".equals(codeString)) 5671 return BASE64BINARY; 5672 if ("boolean".equals(codeString)) 5673 return BOOLEAN; 5674 if ("code".equals(codeString)) 5675 return CODE; 5676 if ("date".equals(codeString)) 5677 return DATE; 5678 if ("dateTime".equals(codeString)) 5679 return DATETIME; 5680 if ("decimal".equals(codeString)) 5681 return DECIMAL; 5682 if ("id".equals(codeString)) 5683 return ID; 5684 if ("instant".equals(codeString)) 5685 return INSTANT; 5686 if ("integer".equals(codeString)) 5687 return INTEGER; 5688 if ("markdown".equals(codeString)) 5689 return MARKDOWN; 5690 if ("oid".equals(codeString)) 5691 return OID; 5692 if ("positiveInt".equals(codeString)) 5693 return POSITIVEINT; 5694 if ("string".equals(codeString)) 5695 return STRING; 5696 if ("time".equals(codeString)) 5697 return TIME; 5698 if ("unsignedInt".equals(codeString)) 5699 return UNSIGNEDINT; 5700 if ("uri".equals(codeString)) 5701 return URI; 5702 if ("uuid".equals(codeString)) 5703 return UUID; 5704 if ("xhtml".equals(codeString)) 5705 return XHTML; 5706 if ("Account".equals(codeString)) 5707 return ACCOUNT; 5708 if ("ActivityDefinition".equals(codeString)) 5709 return ACTIVITYDEFINITION; 5710 if ("AdverseEvent".equals(codeString)) 5711 return ADVERSEEVENT; 5712 if ("AllergyIntolerance".equals(codeString)) 5713 return ALLERGYINTOLERANCE; 5714 if ("Appointment".equals(codeString)) 5715 return APPOINTMENT; 5716 if ("AppointmentResponse".equals(codeString)) 5717 return APPOINTMENTRESPONSE; 5718 if ("AuditEvent".equals(codeString)) 5719 return AUDITEVENT; 5720 if ("Basic".equals(codeString)) 5721 return BASIC; 5722 if ("Binary".equals(codeString)) 5723 return BINARY; 5724 if ("BodySite".equals(codeString)) 5725 return BODYSITE; 5726 if ("Bundle".equals(codeString)) 5727 return BUNDLE; 5728 if ("CapabilityStatement".equals(codeString)) 5729 return CAPABILITYSTATEMENT; 5730 if ("CarePlan".equals(codeString)) 5731 return CAREPLAN; 5732 if ("CareTeam".equals(codeString)) 5733 return CARETEAM; 5734 if ("ChargeItem".equals(codeString)) 5735 return CHARGEITEM; 5736 if ("Claim".equals(codeString)) 5737 return CLAIM; 5738 if ("ClaimResponse".equals(codeString)) 5739 return CLAIMRESPONSE; 5740 if ("ClinicalImpression".equals(codeString)) 5741 return CLINICALIMPRESSION; 5742 if ("CodeSystem".equals(codeString)) 5743 return CODESYSTEM; 5744 if ("Communication".equals(codeString)) 5745 return COMMUNICATION; 5746 if ("CommunicationRequest".equals(codeString)) 5747 return COMMUNICATIONREQUEST; 5748 if ("CompartmentDefinition".equals(codeString)) 5749 return COMPARTMENTDEFINITION; 5750 if ("Composition".equals(codeString)) 5751 return COMPOSITION; 5752 if ("ConceptMap".equals(codeString)) 5753 return CONCEPTMAP; 5754 if ("Condition".equals(codeString)) 5755 return CONDITION; 5756 if ("Consent".equals(codeString)) 5757 return CONSENT; 5758 if ("Contract".equals(codeString)) 5759 return CONTRACT; 5760 if ("Coverage".equals(codeString)) 5761 return COVERAGE; 5762 if ("DataElement".equals(codeString)) 5763 return DATAELEMENT; 5764 if ("DetectedIssue".equals(codeString)) 5765 return DETECTEDISSUE; 5766 if ("Device".equals(codeString)) 5767 return DEVICE; 5768 if ("DeviceComponent".equals(codeString)) 5769 return DEVICECOMPONENT; 5770 if ("DeviceMetric".equals(codeString)) 5771 return DEVICEMETRIC; 5772 if ("DeviceRequest".equals(codeString)) 5773 return DEVICEREQUEST; 5774 if ("DeviceUseStatement".equals(codeString)) 5775 return DEVICEUSESTATEMENT; 5776 if ("DiagnosticReport".equals(codeString)) 5777 return DIAGNOSTICREPORT; 5778 if ("DocumentManifest".equals(codeString)) 5779 return DOCUMENTMANIFEST; 5780 if ("DocumentReference".equals(codeString)) 5781 return DOCUMENTREFERENCE; 5782 if ("DomainResource".equals(codeString)) 5783 return DOMAINRESOURCE; 5784 if ("EligibilityRequest".equals(codeString)) 5785 return ELIGIBILITYREQUEST; 5786 if ("EligibilityResponse".equals(codeString)) 5787 return ELIGIBILITYRESPONSE; 5788 if ("Encounter".equals(codeString)) 5789 return ENCOUNTER; 5790 if ("Endpoint".equals(codeString)) 5791 return ENDPOINT; 5792 if ("EnrollmentRequest".equals(codeString)) 5793 return ENROLLMENTREQUEST; 5794 if ("EnrollmentResponse".equals(codeString)) 5795 return ENROLLMENTRESPONSE; 5796 if ("EpisodeOfCare".equals(codeString)) 5797 return EPISODEOFCARE; 5798 if ("ExpansionProfile".equals(codeString)) 5799 return EXPANSIONPROFILE; 5800 if ("ExplanationOfBenefit".equals(codeString)) 5801 return EXPLANATIONOFBENEFIT; 5802 if ("FamilyMemberHistory".equals(codeString)) 5803 return FAMILYMEMBERHISTORY; 5804 if ("Flag".equals(codeString)) 5805 return FLAG; 5806 if ("Goal".equals(codeString)) 5807 return GOAL; 5808 if ("GraphDefinition".equals(codeString)) 5809 return GRAPHDEFINITION; 5810 if ("Group".equals(codeString)) 5811 return GROUP; 5812 if ("GuidanceResponse".equals(codeString)) 5813 return GUIDANCERESPONSE; 5814 if ("HealthcareService".equals(codeString)) 5815 return HEALTHCARESERVICE; 5816 if ("ImagingManifest".equals(codeString)) 5817 return IMAGINGMANIFEST; 5818 if ("ImagingStudy".equals(codeString)) 5819 return IMAGINGSTUDY; 5820 if ("Immunization".equals(codeString)) 5821 return IMMUNIZATION; 5822 if ("ImmunizationRecommendation".equals(codeString)) 5823 return IMMUNIZATIONRECOMMENDATION; 5824 if ("ImplementationGuide".equals(codeString)) 5825 return IMPLEMENTATIONGUIDE; 5826 if ("Library".equals(codeString)) 5827 return LIBRARY; 5828 if ("Linkage".equals(codeString)) 5829 return LINKAGE; 5830 if ("List".equals(codeString)) 5831 return LIST; 5832 if ("Location".equals(codeString)) 5833 return LOCATION; 5834 if ("Measure".equals(codeString)) 5835 return MEASURE; 5836 if ("MeasureReport".equals(codeString)) 5837 return MEASUREREPORT; 5838 if ("Media".equals(codeString)) 5839 return MEDIA; 5840 if ("Medication".equals(codeString)) 5841 return MEDICATION; 5842 if ("MedicationAdministration".equals(codeString)) 5843 return MEDICATIONADMINISTRATION; 5844 if ("MedicationDispense".equals(codeString)) 5845 return MEDICATIONDISPENSE; 5846 if ("MedicationRequest".equals(codeString)) 5847 return MEDICATIONREQUEST; 5848 if ("MedicationStatement".equals(codeString)) 5849 return MEDICATIONSTATEMENT; 5850 if ("MessageDefinition".equals(codeString)) 5851 return MESSAGEDEFINITION; 5852 if ("MessageHeader".equals(codeString)) 5853 return MESSAGEHEADER; 5854 if ("NamingSystem".equals(codeString)) 5855 return NAMINGSYSTEM; 5856 if ("NutritionOrder".equals(codeString)) 5857 return NUTRITIONORDER; 5858 if ("Observation".equals(codeString)) 5859 return OBSERVATION; 5860 if ("OperationDefinition".equals(codeString)) 5861 return OPERATIONDEFINITION; 5862 if ("OperationOutcome".equals(codeString)) 5863 return OPERATIONOUTCOME; 5864 if ("Organization".equals(codeString)) 5865 return ORGANIZATION; 5866 if ("Parameters".equals(codeString)) 5867 return PARAMETERS; 5868 if ("Patient".equals(codeString)) 5869 return PATIENT; 5870 if ("PaymentNotice".equals(codeString)) 5871 return PAYMENTNOTICE; 5872 if ("PaymentReconciliation".equals(codeString)) 5873 return PAYMENTRECONCILIATION; 5874 if ("Person".equals(codeString)) 5875 return PERSON; 5876 if ("PlanDefinition".equals(codeString)) 5877 return PLANDEFINITION; 5878 if ("Practitioner".equals(codeString)) 5879 return PRACTITIONER; 5880 if ("PractitionerRole".equals(codeString)) 5881 return PRACTITIONERROLE; 5882 if ("Procedure".equals(codeString)) 5883 return PROCEDURE; 5884 if ("ProcedureRequest".equals(codeString)) 5885 return PROCEDUREREQUEST; 5886 if ("ProcessRequest".equals(codeString)) 5887 return PROCESSREQUEST; 5888 if ("ProcessResponse".equals(codeString)) 5889 return PROCESSRESPONSE; 5890 if ("Provenance".equals(codeString)) 5891 return PROVENANCE; 5892 if ("Questionnaire".equals(codeString)) 5893 return QUESTIONNAIRE; 5894 if ("QuestionnaireResponse".equals(codeString)) 5895 return QUESTIONNAIRERESPONSE; 5896 if ("ReferralRequest".equals(codeString)) 5897 return REFERRALREQUEST; 5898 if ("RelatedPerson".equals(codeString)) 5899 return RELATEDPERSON; 5900 if ("RequestGroup".equals(codeString)) 5901 return REQUESTGROUP; 5902 if ("ResearchStudy".equals(codeString)) 5903 return RESEARCHSTUDY; 5904 if ("ResearchSubject".equals(codeString)) 5905 return RESEARCHSUBJECT; 5906 if ("Resource".equals(codeString)) 5907 return RESOURCE; 5908 if ("RiskAssessment".equals(codeString)) 5909 return RISKASSESSMENT; 5910 if ("Schedule".equals(codeString)) 5911 return SCHEDULE; 5912 if ("SearchParameter".equals(codeString)) 5913 return SEARCHPARAMETER; 5914 if ("Sequence".equals(codeString)) 5915 return SEQUENCE; 5916 if ("ServiceDefinition".equals(codeString)) 5917 return SERVICEDEFINITION; 5918 if ("Slot".equals(codeString)) 5919 return SLOT; 5920 if ("Specimen".equals(codeString)) 5921 return SPECIMEN; 5922 if ("StructureDefinition".equals(codeString)) 5923 return STRUCTUREDEFINITION; 5924 if ("StructureMap".equals(codeString)) 5925 return STRUCTUREMAP; 5926 if ("Subscription".equals(codeString)) 5927 return SUBSCRIPTION; 5928 if ("Substance".equals(codeString)) 5929 return SUBSTANCE; 5930 if ("SupplyDelivery".equals(codeString)) 5931 return SUPPLYDELIVERY; 5932 if ("SupplyRequest".equals(codeString)) 5933 return SUPPLYREQUEST; 5934 if ("Task".equals(codeString)) 5935 return TASK; 5936 if ("TestReport".equals(codeString)) 5937 return TESTREPORT; 5938 if ("TestScript".equals(codeString)) 5939 return TESTSCRIPT; 5940 if ("ValueSet".equals(codeString)) 5941 return VALUESET; 5942 if ("VisionPrescription".equals(codeString)) 5943 return VISIONPRESCRIPTION; 5944 throw new FHIRException("Unknown FHIRDefinedType code '"+codeString+"'"); 5945 } 5946 public String toCode() { 5947 switch (this) { 5948 case ADDRESS: return "Address"; 5949 case AGE: return "Age"; 5950 case ANNOTATION: return "Annotation"; 5951 case ATTACHMENT: return "Attachment"; 5952 case BACKBONEELEMENT: return "BackboneElement"; 5953 case CODEABLECONCEPT: return "CodeableConcept"; 5954 case CODING: return "Coding"; 5955 case CONTACTDETAIL: return "ContactDetail"; 5956 case CONTACTPOINT: return "ContactPoint"; 5957 case CONTRIBUTOR: return "Contributor"; 5958 case COUNT: return "Count"; 5959 case DATAREQUIREMENT: return "DataRequirement"; 5960 case DISTANCE: return "Distance"; 5961 case DOSAGE: return "Dosage"; 5962 case DURATION: return "Duration"; 5963 case ELEMENT: return "Element"; 5964 case ELEMENTDEFINITION: return "ElementDefinition"; 5965 case EXTENSION: return "Extension"; 5966 case HUMANNAME: return "HumanName"; 5967 case IDENTIFIER: return "Identifier"; 5968 case META: return "Meta"; 5969 case MONEY: return "Money"; 5970 case NARRATIVE: return "Narrative"; 5971 case PARAMETERDEFINITION: return "ParameterDefinition"; 5972 case PERIOD: return "Period"; 5973 case QUANTITY: return "Quantity"; 5974 case RANGE: return "Range"; 5975 case RATIO: return "Ratio"; 5976 case REFERENCE: return "Reference"; 5977 case RELATEDARTIFACT: return "RelatedArtifact"; 5978 case SAMPLEDDATA: return "SampledData"; 5979 case SIGNATURE: return "Signature"; 5980 case SIMPLEQUANTITY: return "SimpleQuantity"; 5981 case TIMING: return "Timing"; 5982 case TRIGGERDEFINITION: return "TriggerDefinition"; 5983 case USAGECONTEXT: return "UsageContext"; 5984 case BASE64BINARY: return "base64Binary"; 5985 case BOOLEAN: return "boolean"; 5986 case CODE: return "code"; 5987 case DATE: return "date"; 5988 case DATETIME: return "dateTime"; 5989 case DECIMAL: return "decimal"; 5990 case ID: return "id"; 5991 case INSTANT: return "instant"; 5992 case INTEGER: return "integer"; 5993 case MARKDOWN: return "markdown"; 5994 case OID: return "oid"; 5995 case POSITIVEINT: return "positiveInt"; 5996 case STRING: return "string"; 5997 case TIME: return "time"; 5998 case UNSIGNEDINT: return "unsignedInt"; 5999 case URI: return "uri"; 6000 case UUID: return "uuid"; 6001 case XHTML: return "xhtml"; 6002 case ACCOUNT: return "Account"; 6003 case ACTIVITYDEFINITION: return "ActivityDefinition"; 6004 case ADVERSEEVENT: return "AdverseEvent"; 6005 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 6006 case APPOINTMENT: return "Appointment"; 6007 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 6008 case AUDITEVENT: return "AuditEvent"; 6009 case BASIC: return "Basic"; 6010 case BINARY: return "Binary"; 6011 case BODYSITE: return "BodySite"; 6012 case BUNDLE: return "Bundle"; 6013 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 6014 case CAREPLAN: return "CarePlan"; 6015 case CARETEAM: return "CareTeam"; 6016 case CHARGEITEM: return "ChargeItem"; 6017 case CLAIM: return "Claim"; 6018 case CLAIMRESPONSE: return "ClaimResponse"; 6019 case CLINICALIMPRESSION: return "ClinicalImpression"; 6020 case CODESYSTEM: return "CodeSystem"; 6021 case COMMUNICATION: return "Communication"; 6022 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 6023 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 6024 case COMPOSITION: return "Composition"; 6025 case CONCEPTMAP: return "ConceptMap"; 6026 case CONDITION: return "Condition"; 6027 case CONSENT: return "Consent"; 6028 case CONTRACT: return "Contract"; 6029 case COVERAGE: return "Coverage"; 6030 case DATAELEMENT: return "DataElement"; 6031 case DETECTEDISSUE: return "DetectedIssue"; 6032 case DEVICE: return "Device"; 6033 case DEVICECOMPONENT: return "DeviceComponent"; 6034 case DEVICEMETRIC: return "DeviceMetric"; 6035 case DEVICEREQUEST: return "DeviceRequest"; 6036 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 6037 case DIAGNOSTICREPORT: return "DiagnosticReport"; 6038 case DOCUMENTMANIFEST: return "DocumentManifest"; 6039 case DOCUMENTREFERENCE: return "DocumentReference"; 6040 case DOMAINRESOURCE: return "DomainResource"; 6041 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 6042 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 6043 case ENCOUNTER: return "Encounter"; 6044 case ENDPOINT: return "Endpoint"; 6045 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 6046 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 6047 case EPISODEOFCARE: return "EpisodeOfCare"; 6048 case EXPANSIONPROFILE: return "ExpansionProfile"; 6049 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 6050 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 6051 case FLAG: return "Flag"; 6052 case GOAL: return "Goal"; 6053 case GRAPHDEFINITION: return "GraphDefinition"; 6054 case GROUP: return "Group"; 6055 case GUIDANCERESPONSE: return "GuidanceResponse"; 6056 case HEALTHCARESERVICE: return "HealthcareService"; 6057 case IMAGINGMANIFEST: return "ImagingManifest"; 6058 case IMAGINGSTUDY: return "ImagingStudy"; 6059 case IMMUNIZATION: return "Immunization"; 6060 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 6061 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 6062 case LIBRARY: return "Library"; 6063 case LINKAGE: return "Linkage"; 6064 case LIST: return "List"; 6065 case LOCATION: return "Location"; 6066 case MEASURE: return "Measure"; 6067 case MEASUREREPORT: return "MeasureReport"; 6068 case MEDIA: return "Media"; 6069 case MEDICATION: return "Medication"; 6070 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 6071 case MEDICATIONDISPENSE: return "MedicationDispense"; 6072 case MEDICATIONREQUEST: return "MedicationRequest"; 6073 case MEDICATIONSTATEMENT: return "MedicationStatement"; 6074 case MESSAGEDEFINITION: return "MessageDefinition"; 6075 case MESSAGEHEADER: return "MessageHeader"; 6076 case NAMINGSYSTEM: return "NamingSystem"; 6077 case NUTRITIONORDER: return "NutritionOrder"; 6078 case OBSERVATION: return "Observation"; 6079 case OPERATIONDEFINITION: return "OperationDefinition"; 6080 case OPERATIONOUTCOME: return "OperationOutcome"; 6081 case ORGANIZATION: return "Organization"; 6082 case PARAMETERS: return "Parameters"; 6083 case PATIENT: return "Patient"; 6084 case PAYMENTNOTICE: return "PaymentNotice"; 6085 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 6086 case PERSON: return "Person"; 6087 case PLANDEFINITION: return "PlanDefinition"; 6088 case PRACTITIONER: return "Practitioner"; 6089 case PRACTITIONERROLE: return "PractitionerRole"; 6090 case PROCEDURE: return "Procedure"; 6091 case PROCEDUREREQUEST: return "ProcedureRequest"; 6092 case PROCESSREQUEST: return "ProcessRequest"; 6093 case PROCESSRESPONSE: return "ProcessResponse"; 6094 case PROVENANCE: return "Provenance"; 6095 case QUESTIONNAIRE: return "Questionnaire"; 6096 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 6097 case REFERRALREQUEST: return "ReferralRequest"; 6098 case RELATEDPERSON: return "RelatedPerson"; 6099 case REQUESTGROUP: return "RequestGroup"; 6100 case RESEARCHSTUDY: return "ResearchStudy"; 6101 case RESEARCHSUBJECT: return "ResearchSubject"; 6102 case RESOURCE: return "Resource"; 6103 case RISKASSESSMENT: return "RiskAssessment"; 6104 case SCHEDULE: return "Schedule"; 6105 case SEARCHPARAMETER: return "SearchParameter"; 6106 case SEQUENCE: return "Sequence"; 6107 case SERVICEDEFINITION: return "ServiceDefinition"; 6108 case SLOT: return "Slot"; 6109 case SPECIMEN: return "Specimen"; 6110 case STRUCTUREDEFINITION: return "StructureDefinition"; 6111 case STRUCTUREMAP: return "StructureMap"; 6112 case SUBSCRIPTION: return "Subscription"; 6113 case SUBSTANCE: return "Substance"; 6114 case SUPPLYDELIVERY: return "SupplyDelivery"; 6115 case SUPPLYREQUEST: return "SupplyRequest"; 6116 case TASK: return "Task"; 6117 case TESTREPORT: return "TestReport"; 6118 case TESTSCRIPT: return "TestScript"; 6119 case VALUESET: return "ValueSet"; 6120 case VISIONPRESCRIPTION: return "VisionPrescription"; 6121 case NULL: return null; 6122 default: return "?"; 6123 } 6124 } 6125 public String getSystem() { 6126 switch (this) { 6127 case ADDRESS: return "http://hl7.org/fhir/data-types"; 6128 case AGE: return "http://hl7.org/fhir/data-types"; 6129 case ANNOTATION: return "http://hl7.org/fhir/data-types"; 6130 case ATTACHMENT: return "http://hl7.org/fhir/data-types"; 6131 case BACKBONEELEMENT: return "http://hl7.org/fhir/data-types"; 6132 case CODEABLECONCEPT: return "http://hl7.org/fhir/data-types"; 6133 case CODING: return "http://hl7.org/fhir/data-types"; 6134 case CONTACTDETAIL: return "http://hl7.org/fhir/data-types"; 6135 case CONTACTPOINT: return "http://hl7.org/fhir/data-types"; 6136 case CONTRIBUTOR: return "http://hl7.org/fhir/data-types"; 6137 case COUNT: return "http://hl7.org/fhir/data-types"; 6138 case DATAREQUIREMENT: return "http://hl7.org/fhir/data-types"; 6139 case DISTANCE: return "http://hl7.org/fhir/data-types"; 6140 case DOSAGE: return "http://hl7.org/fhir/data-types"; 6141 case DURATION: return "http://hl7.org/fhir/data-types"; 6142 case ELEMENT: return "http://hl7.org/fhir/data-types"; 6143 case ELEMENTDEFINITION: return "http://hl7.org/fhir/data-types"; 6144 case EXTENSION: return "http://hl7.org/fhir/data-types"; 6145 case HUMANNAME: return "http://hl7.org/fhir/data-types"; 6146 case IDENTIFIER: return "http://hl7.org/fhir/data-types"; 6147 case META: return "http://hl7.org/fhir/data-types"; 6148 case MONEY: return "http://hl7.org/fhir/data-types"; 6149 case NARRATIVE: return "http://hl7.org/fhir/data-types"; 6150 case PARAMETERDEFINITION: return "http://hl7.org/fhir/data-types"; 6151 case PERIOD: return "http://hl7.org/fhir/data-types"; 6152 case QUANTITY: return "http://hl7.org/fhir/data-types"; 6153 case RANGE: return "http://hl7.org/fhir/data-types"; 6154 case RATIO: return "http://hl7.org/fhir/data-types"; 6155 case REFERENCE: return "http://hl7.org/fhir/data-types"; 6156 case RELATEDARTIFACT: return "http://hl7.org/fhir/data-types"; 6157 case SAMPLEDDATA: return "http://hl7.org/fhir/data-types"; 6158 case SIGNATURE: return "http://hl7.org/fhir/data-types"; 6159 case SIMPLEQUANTITY: return "http://hl7.org/fhir/data-types"; 6160 case TIMING: return "http://hl7.org/fhir/data-types"; 6161 case TRIGGERDEFINITION: return "http://hl7.org/fhir/data-types"; 6162 case USAGECONTEXT: return "http://hl7.org/fhir/data-types"; 6163 case BASE64BINARY: return "http://hl7.org/fhir/data-types"; 6164 case BOOLEAN: return "http://hl7.org/fhir/data-types"; 6165 case CODE: return "http://hl7.org/fhir/data-types"; 6166 case DATE: return "http://hl7.org/fhir/data-types"; 6167 case DATETIME: return "http://hl7.org/fhir/data-types"; 6168 case DECIMAL: return "http://hl7.org/fhir/data-types"; 6169 case ID: return "http://hl7.org/fhir/data-types"; 6170 case INSTANT: return "http://hl7.org/fhir/data-types"; 6171 case INTEGER: return "http://hl7.org/fhir/data-types"; 6172 case MARKDOWN: return "http://hl7.org/fhir/data-types"; 6173 case OID: return "http://hl7.org/fhir/data-types"; 6174 case POSITIVEINT: return "http://hl7.org/fhir/data-types"; 6175 case STRING: return "http://hl7.org/fhir/data-types"; 6176 case TIME: return "http://hl7.org/fhir/data-types"; 6177 case UNSIGNEDINT: return "http://hl7.org/fhir/data-types"; 6178 case URI: return "http://hl7.org/fhir/data-types"; 6179 case UUID: return "http://hl7.org/fhir/data-types"; 6180 case XHTML: return "http://hl7.org/fhir/data-types"; 6181 case ACCOUNT: return "http://hl7.org/fhir/resource-types"; 6182 case ACTIVITYDEFINITION: return "http://hl7.org/fhir/resource-types"; 6183 case ADVERSEEVENT: return "http://hl7.org/fhir/resource-types"; 6184 case ALLERGYINTOLERANCE: return "http://hl7.org/fhir/resource-types"; 6185 case APPOINTMENT: return "http://hl7.org/fhir/resource-types"; 6186 case APPOINTMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 6187 case AUDITEVENT: return "http://hl7.org/fhir/resource-types"; 6188 case BASIC: return "http://hl7.org/fhir/resource-types"; 6189 case BINARY: return "http://hl7.org/fhir/resource-types"; 6190 case BODYSITE: return "http://hl7.org/fhir/resource-types"; 6191 case BUNDLE: return "http://hl7.org/fhir/resource-types"; 6192 case CAPABILITYSTATEMENT: return "http://hl7.org/fhir/resource-types"; 6193 case CAREPLAN: return "http://hl7.org/fhir/resource-types"; 6194 case CARETEAM: return "http://hl7.org/fhir/resource-types"; 6195 case CHARGEITEM: return "http://hl7.org/fhir/resource-types"; 6196 case CLAIM: return "http://hl7.org/fhir/resource-types"; 6197 case CLAIMRESPONSE: return "http://hl7.org/fhir/resource-types"; 6198 case CLINICALIMPRESSION: return "http://hl7.org/fhir/resource-types"; 6199 case CODESYSTEM: return "http://hl7.org/fhir/resource-types"; 6200 case COMMUNICATION: return "http://hl7.org/fhir/resource-types"; 6201 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 6202 case COMPARTMENTDEFINITION: return "http://hl7.org/fhir/resource-types"; 6203 case COMPOSITION: return "http://hl7.org/fhir/resource-types"; 6204 case CONCEPTMAP: return "http://hl7.org/fhir/resource-types"; 6205 case CONDITION: return "http://hl7.org/fhir/resource-types"; 6206 case CONSENT: return "http://hl7.org/fhir/resource-types"; 6207 case CONTRACT: return "http://hl7.org/fhir/resource-types"; 6208 case COVERAGE: return "http://hl7.org/fhir/resource-types"; 6209 case DATAELEMENT: return "http://hl7.org/fhir/resource-types"; 6210 case DETECTEDISSUE: return "http://hl7.org/fhir/resource-types"; 6211 case DEVICE: return "http://hl7.org/fhir/resource-types"; 6212 case DEVICECOMPONENT: return "http://hl7.org/fhir/resource-types"; 6213 case DEVICEMETRIC: return "http://hl7.org/fhir/resource-types"; 6214 case DEVICEREQUEST: return "http://hl7.org/fhir/resource-types"; 6215 case DEVICEUSESTATEMENT: return "http://hl7.org/fhir/resource-types"; 6216 case DIAGNOSTICREPORT: return "http://hl7.org/fhir/resource-types"; 6217 case DOCUMENTMANIFEST: return "http://hl7.org/fhir/resource-types"; 6218 case DOCUMENTREFERENCE: return "http://hl7.org/fhir/resource-types"; 6219 case DOMAINRESOURCE: return "http://hl7.org/fhir/resource-types"; 6220 case ELIGIBILITYREQUEST: return "http://hl7.org/fhir/resource-types"; 6221 case ELIGIBILITYRESPONSE: return "http://hl7.org/fhir/resource-types"; 6222 case ENCOUNTER: return "http://hl7.org/fhir/resource-types"; 6223 case ENDPOINT: return "http://hl7.org/fhir/resource-types"; 6224 case ENROLLMENTREQUEST: return "http://hl7.org/fhir/resource-types"; 6225 case ENROLLMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 6226 case EPISODEOFCARE: return "http://hl7.org/fhir/resource-types"; 6227 case EXPANSIONPROFILE: return "http://hl7.org/fhir/resource-types"; 6228 case EXPLANATIONOFBENEFIT: return "http://hl7.org/fhir/resource-types"; 6229 case FAMILYMEMBERHISTORY: return "http://hl7.org/fhir/resource-types"; 6230 case FLAG: return "http://hl7.org/fhir/resource-types"; 6231 case GOAL: return "http://hl7.org/fhir/resource-types"; 6232 case GRAPHDEFINITION: return "http://hl7.org/fhir/resource-types"; 6233 case GROUP: return "http://hl7.org/fhir/resource-types"; 6234 case GUIDANCERESPONSE: return "http://hl7.org/fhir/resource-types"; 6235 case HEALTHCARESERVICE: return "http://hl7.org/fhir/resource-types"; 6236 case IMAGINGMANIFEST: return "http://hl7.org/fhir/resource-types"; 6237 case IMAGINGSTUDY: return "http://hl7.org/fhir/resource-types"; 6238 case IMMUNIZATION: return "http://hl7.org/fhir/resource-types"; 6239 case IMMUNIZATIONRECOMMENDATION: return "http://hl7.org/fhir/resource-types"; 6240 case IMPLEMENTATIONGUIDE: return "http://hl7.org/fhir/resource-types"; 6241 case LIBRARY: return "http://hl7.org/fhir/resource-types"; 6242 case LINKAGE: return "http://hl7.org/fhir/resource-types"; 6243 case LIST: return "http://hl7.org/fhir/resource-types"; 6244 case LOCATION: return "http://hl7.org/fhir/resource-types"; 6245 case MEASURE: return "http://hl7.org/fhir/resource-types"; 6246 case MEASUREREPORT: return "http://hl7.org/fhir/resource-types"; 6247 case MEDIA: return "http://hl7.org/fhir/resource-types"; 6248 case MEDICATION: return "http://hl7.org/fhir/resource-types"; 6249 case MEDICATIONADMINISTRATION: return "http://hl7.org/fhir/resource-types"; 6250 case MEDICATIONDISPENSE: return "http://hl7.org/fhir/resource-types"; 6251 case MEDICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 6252 case MEDICATIONSTATEMENT: return "http://hl7.org/fhir/resource-types"; 6253 case MESSAGEDEFINITION: return "http://hl7.org/fhir/resource-types"; 6254 case MESSAGEHEADER: return "http://hl7.org/fhir/resource-types"; 6255 case NAMINGSYSTEM: return "http://hl7.org/fhir/resource-types"; 6256 case NUTRITIONORDER: return "http://hl7.org/fhir/resource-types"; 6257 case OBSERVATION: return "http://hl7.org/fhir/resource-types"; 6258 case OPERATIONDEFINITION: return "http://hl7.org/fhir/resource-types"; 6259 case OPERATIONOUTCOME: return "http://hl7.org/fhir/resource-types"; 6260 case ORGANIZATION: return "http://hl7.org/fhir/resource-types"; 6261 case PARAMETERS: return "http://hl7.org/fhir/resource-types"; 6262 case PATIENT: return "http://hl7.org/fhir/resource-types"; 6263 case PAYMENTNOTICE: return "http://hl7.org/fhir/resource-types"; 6264 case PAYMENTRECONCILIATION: return "http://hl7.org/fhir/resource-types"; 6265 case PERSON: return "http://hl7.org/fhir/resource-types"; 6266 case PLANDEFINITION: return "http://hl7.org/fhir/resource-types"; 6267 case PRACTITIONER: return "http://hl7.org/fhir/resource-types"; 6268 case PRACTITIONERROLE: return "http://hl7.org/fhir/resource-types"; 6269 case PROCEDURE: return "http://hl7.org/fhir/resource-types"; 6270 case PROCEDUREREQUEST: return "http://hl7.org/fhir/resource-types"; 6271 case PROCESSREQUEST: return "http://hl7.org/fhir/resource-types"; 6272 case PROCESSRESPONSE: return "http://hl7.org/fhir/resource-types"; 6273 case PROVENANCE: return "http://hl7.org/fhir/resource-types"; 6274 case QUESTIONNAIRE: return "http://hl7.org/fhir/resource-types"; 6275 case QUESTIONNAIRERESPONSE: return "http://hl7.org/fhir/resource-types"; 6276 case REFERRALREQUEST: return "http://hl7.org/fhir/resource-types"; 6277 case RELATEDPERSON: return "http://hl7.org/fhir/resource-types"; 6278 case REQUESTGROUP: return "http://hl7.org/fhir/resource-types"; 6279 case RESEARCHSTUDY: return "http://hl7.org/fhir/resource-types"; 6280 case RESEARCHSUBJECT: return "http://hl7.org/fhir/resource-types"; 6281 case RESOURCE: return "http://hl7.org/fhir/resource-types"; 6282 case RISKASSESSMENT: return "http://hl7.org/fhir/resource-types"; 6283 case SCHEDULE: return "http://hl7.org/fhir/resource-types"; 6284 case SEARCHPARAMETER: return "http://hl7.org/fhir/resource-types"; 6285 case SEQUENCE: return "http://hl7.org/fhir/resource-types"; 6286 case SERVICEDEFINITION: return "http://hl7.org/fhir/resource-types"; 6287 case SLOT: return "http://hl7.org/fhir/resource-types"; 6288 case SPECIMEN: return "http://hl7.org/fhir/resource-types"; 6289 case STRUCTUREDEFINITION: return "http://hl7.org/fhir/resource-types"; 6290 case STRUCTUREMAP: return "http://hl7.org/fhir/resource-types"; 6291 case SUBSCRIPTION: return "http://hl7.org/fhir/resource-types"; 6292 case SUBSTANCE: return "http://hl7.org/fhir/resource-types"; 6293 case SUPPLYDELIVERY: return "http://hl7.org/fhir/resource-types"; 6294 case SUPPLYREQUEST: return "http://hl7.org/fhir/resource-types"; 6295 case TASK: return "http://hl7.org/fhir/resource-types"; 6296 case TESTREPORT: return "http://hl7.org/fhir/resource-types"; 6297 case TESTSCRIPT: return "http://hl7.org/fhir/resource-types"; 6298 case VALUESET: return "http://hl7.org/fhir/resource-types"; 6299 case VISIONPRESCRIPTION: return "http://hl7.org/fhir/resource-types"; 6300 case NULL: return null; 6301 default: return "?"; 6302 } 6303 } 6304 public String getDefinition() { 6305 switch (this) { 6306 case ADDRESS: return "An address expressed using postal conventions (as opposed to GPS or other location definition formats). This data type may be used to convey addresses for use in delivering mail as well as for visiting locations which might not be valid for mail delivery. There are a variety of postal address formats defined around the world."; 6307 case AGE: return "A duration of time during which an organism (or a process) has existed."; 6308 case ANNOTATION: return "A text note which also contains information about who made the statement and when."; 6309 case ATTACHMENT: return "For referring to data content defined in other formats."; 6310 case BACKBONEELEMENT: return "Base definition for all elements that are defined inside a resource - but not those in a data type."; 6311 case CODEABLECONCEPT: return "A concept that may be defined by a formal reference to a terminology or ontology or may be provided by text."; 6312 case CODING: return "A reference to a code defined by a terminology system."; 6313 case CONTACTDETAIL: return "Specifies contact information for a person or organization."; 6314 case CONTACTPOINT: return "Details for all kinds of technology mediated contact points for a person or organization, including telephone, email, etc."; 6315 case CONTRIBUTOR: return "A contributor to the content of a knowledge asset, including authors, editors, reviewers, and endorsers."; 6316 case COUNT: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 6317 case DATAREQUIREMENT: return "Describes a required data item for evaluation in terms of the type of data, and optional code or date-based filters of the data."; 6318 case DISTANCE: return "A length - a value with a unit that is a physical distance."; 6319 case DOSAGE: return "Indicates how the medication is/was taken or should be taken by the patient."; 6320 case DURATION: return "A length of time."; 6321 case ELEMENT: return "Base definition for all elements in a resource."; 6322 case ELEMENTDEFINITION: return "Captures constraints on each element within the resource, profile, or extension."; 6323 case EXTENSION: return "Optional Extension Element - found in all resources."; 6324 case HUMANNAME: return "A human's name with the ability to identify parts and usage."; 6325 case IDENTIFIER: return "A technical identifier - identifies some entity uniquely and unambiguously."; 6326 case META: return "The metadata about a resource. This is content in the resource that is maintained by the infrastructure. Changes to the content may not always be associated with version changes to the resource."; 6327 case MONEY: return "An amount of economic utility in some recognized currency."; 6328 case NARRATIVE: return "A human-readable formatted text, including images."; 6329 case PARAMETERDEFINITION: return "The parameters to the module. This collection specifies both the input and output parameters. Input parameters are provided by the caller as part of the $evaluate operation. Output parameters are included in the GuidanceResponse."; 6330 case PERIOD: return "A time period defined by a start and end date and optionally time."; 6331 case QUANTITY: return "A measured amount (or an amount that can potentially be measured). Note that measured amounts include amounts that are not precisely quantified, including amounts involving arbitrary units and floating currencies."; 6332 case RANGE: return "A set of ordered Quantities defined by a low and high limit."; 6333 case RATIO: return "A relationship of two Quantity values - expressed as a numerator and a denominator."; 6334 case REFERENCE: return "A reference from one resource to another."; 6335 case RELATEDARTIFACT: return "Related artifacts such as additional documentation, justification, or bibliographic references."; 6336 case SAMPLEDDATA: return "A series of measurements taken by a device, with upper and lower limits. There may be more than one dimension in the data."; 6337 case SIGNATURE: return "A digital signature along with supporting context. The signature may be electronic/cryptographic in nature, or a graphical image representing a hand-written signature, or a signature process. Different signature approaches have different utilities."; 6338 case SIMPLEQUANTITY: return ""; 6339 case TIMING: return "Specifies an event that may occur multiple times. Timing schedules are used to record when things are planned, expected or requested to occur. The most common usage is in dosage instructions for medications. They are also used when planning care of various kinds, and may be used for reporting the schedule to which past regular activities were carried out."; 6340 case TRIGGERDEFINITION: return "A description of a triggering event."; 6341 case USAGECONTEXT: return "Specifies clinical/business/etc metadata that can be used to retrieve, index and/or categorize an artifact. This metadata can either be specific to the applicable population (e.g., age category, DRG) or the specific context of care (e.g., venue, care setting, provider of care)."; 6342 case BASE64BINARY: return "A stream of bytes"; 6343 case BOOLEAN: return "Value of \"true\" or \"false\""; 6344 case CODE: return "A string which has at least one character and no leading or trailing whitespace and where there is no whitespace other than single spaces in the contents"; 6345 case DATE: return "A date or partial date (e.g. just year or year + month). There is no time zone. The format is a union of the schema types gYear, gYearMonth and date. Dates SHALL be valid dates."; 6346 case DATETIME: return "A date, date-time or partial date (e.g. just year or year + month). If hours and minutes are specified, a time zone SHALL be populated. The format is a union of the schema types gYear, gYearMonth, date and dateTime. Seconds must be provided due to schema type constraints but may be zero-filled and may be ignored. Dates SHALL be valid dates."; 6347 case DECIMAL: return "A rational number with implicit precision"; 6348 case ID: return "Any combination of letters, numerals, \"-\" and \".\", with a length limit of 64 characters. (This might be an integer, an unprefixed OID, UUID or any other identifier pattern that meets these constraints.) Ids are case-insensitive."; 6349 case INSTANT: return "An instant in time - known at least to the second"; 6350 case INTEGER: return "A whole number"; 6351 case MARKDOWN: return "A string that may contain markdown syntax for optional processing by a mark down presentation engine"; 6352 case OID: return "An OID represented as a URI"; 6353 case POSITIVEINT: return "An integer with a value that is positive (e.g. >0)"; 6354 case STRING: return "A sequence of Unicode characters"; 6355 case TIME: return "A time during the day, with no date specified"; 6356 case UNSIGNEDINT: return "An integer with a value that is not negative (e.g. >= 0)"; 6357 case URI: return "String of characters used to identify a name or a resource"; 6358 case UUID: return "A UUID, represented as a URI"; 6359 case XHTML: return "XHTML format, as defined by W3C, but restricted usage (mainly, no active content)"; 6360 case ACCOUNT: return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 6361 case ACTIVITYDEFINITION: return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 6362 case ADVERSEEVENT: return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 6363 case ALLERGYINTOLERANCE: return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 6364 case APPOINTMENT: return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 6365 case APPOINTMENTRESPONSE: return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 6366 case AUDITEVENT: return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 6367 case BASIC: return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 6368 case BINARY: return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 6369 case BODYSITE: return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 6370 case BUNDLE: return "A container for a collection of resources."; 6371 case CAPABILITYSTATEMENT: return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 6372 case CAREPLAN: return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 6373 case CARETEAM: return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 6374 case CHARGEITEM: return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 6375 case CLAIM: return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 6376 case CLAIMRESPONSE: return "This resource provides the adjudication details from the processing of a Claim resource."; 6377 case CLINICALIMPRESSION: return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 6378 case CODESYSTEM: return "A code system resource specifies a set of codes drawn from one or more code systems."; 6379 case COMMUNICATION: return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 6380 case COMMUNICATIONREQUEST: return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 6381 case COMPARTMENTDEFINITION: return "A compartment definition that defines how resources are accessed on a server."; 6382 case COMPOSITION: return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 6383 case CONCEPTMAP: return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 6384 case CONDITION: return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 6385 case CONSENT: return "A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 6386 case CONTRACT: return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 6387 case COVERAGE: return "Financial instrument which may be used to reimburse or pay for health care products and services."; 6388 case DATAELEMENT: return "The formal description of a single piece of information that can be gathered and reported."; 6389 case DETECTEDISSUE: return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 6390 case DEVICE: return "This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 6391 case DEVICECOMPONENT: return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 6392 case DEVICEMETRIC: return "Describes a measurement, calculation or setting capability of a medical device."; 6393 case DEVICEREQUEST: return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 6394 case DEVICEUSESTATEMENT: return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 6395 case DIAGNOSTICREPORT: return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 6396 case DOCUMENTMANIFEST: return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 6397 case DOCUMENTREFERENCE: return "A reference to a document."; 6398 case DOMAINRESOURCE: return "A resource that includes narrative, extensions, and contained resources."; 6399 case ELIGIBILITYREQUEST: return "The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 6400 case ELIGIBILITYRESPONSE: return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 6401 case ENCOUNTER: return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 6402 case ENDPOINT: return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 6403 case ENROLLMENTREQUEST: return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 6404 case ENROLLMENTRESPONSE: return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 6405 case EPISODEOFCARE: return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 6406 case EXPANSIONPROFILE: return "Resource to define constraints on the Expansion of a FHIR ValueSet."; 6407 case EXPLANATIONOFBENEFIT: return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 6408 case FAMILYMEMBERHISTORY: return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 6409 case FLAG: return "Prospective warnings of potential issues when providing care to the patient."; 6410 case GOAL: return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 6411 case GRAPHDEFINITION: return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 6412 case GROUP: return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 6413 case GUIDANCERESPONSE: return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 6414 case HEALTHCARESERVICE: return "The details of a healthcare service available at a location."; 6415 case IMAGINGMANIFEST: return "A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection."; 6416 case IMAGINGSTUDY: return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 6417 case IMMUNIZATION: return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 6418 case IMMUNIZATIONRECOMMENDATION: return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 6419 case IMPLEMENTATIONGUIDE: return "A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 6420 case LIBRARY: return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 6421 case LINKAGE: return "Identifies two or more records (resource instances) that are referring to the same real-world \"occurrence\"."; 6422 case LIST: return "A set of information summarized from a list of other resources."; 6423 case LOCATION: return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 6424 case MEASURE: return "The Measure resource provides the definition of a quality measure."; 6425 case MEASUREREPORT: return "The MeasureReport resource contains the results of evaluating a measure."; 6426 case MEDIA: return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 6427 case MEDICATION: return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 6428 case MEDICATIONADMINISTRATION: return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 6429 case MEDICATIONDISPENSE: return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 6430 case MEDICATIONREQUEST: return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 6431 case MEDICATIONSTATEMENT: return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 6432 case MESSAGEDEFINITION: return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 6433 case MESSAGEHEADER: return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 6434 case NAMINGSYSTEM: return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 6435 case NUTRITIONORDER: return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 6436 case OBSERVATION: return "Measurements and simple assertions made about a patient, device or other subject."; 6437 case OPERATIONDEFINITION: return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 6438 case OPERATIONOUTCOME: return "A collection of error, warning or information messages that result from a system action."; 6439 case ORGANIZATION: return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 6440 case PARAMETERS: return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 6441 case PATIENT: return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 6442 case PAYMENTNOTICE: return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 6443 case PAYMENTRECONCILIATION: return "This resource provides payment details and claim references supporting a bulk payment."; 6444 case PERSON: return "Demographics and administrative information about a person independent of a specific health-related context."; 6445 case PLANDEFINITION: return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 6446 case PRACTITIONER: return "A person who is directly or indirectly involved in the provisioning of healthcare."; 6447 case PRACTITIONERROLE: return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 6448 case PROCEDURE: return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 6449 case PROCEDUREREQUEST: return "A record of a request for diagnostic investigations, treatments, or operations to be performed."; 6450 case PROCESSREQUEST: return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 6451 case PROCESSRESPONSE: return "This resource provides processing status, errors and notes from the processing of a resource."; 6452 case PROVENANCE: return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 6453 case QUESTIONNAIRE: return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 6454 case QUESTIONNAIRERESPONSE: return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 6455 case REFERRALREQUEST: return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 6456 case RELATEDPERSON: return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 6457 case REQUESTGROUP: return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 6458 case RESEARCHSTUDY: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 6459 case RESEARCHSUBJECT: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 6460 case RESOURCE: return "This is the base resource type for everything."; 6461 case RISKASSESSMENT: return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 6462 case SCHEDULE: return "A container for slots of time that may be available for booking appointments."; 6463 case SEARCHPARAMETER: return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 6464 case SEQUENCE: return "Raw data describing a biological sequence."; 6465 case SERVICEDEFINITION: return "The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking."; 6466 case SLOT: return "A slot of time on a schedule that may be available for booking appointments."; 6467 case SPECIMEN: return "A sample to be used for analysis."; 6468 case STRUCTUREDEFINITION: return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 6469 case STRUCTUREMAP: return "A Map of relationships between 2 structures that can be used to transform data."; 6470 case SUBSCRIPTION: return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 6471 case SUBSTANCE: return "A homogeneous material with a definite composition."; 6472 case SUPPLYDELIVERY: return "Record of delivery of what is supplied."; 6473 case SUPPLYREQUEST: return "A record of a request for a medication, substance or device used in the healthcare setting."; 6474 case TASK: return "A task to be performed."; 6475 case TESTREPORT: return "A summary of information based on the results of executing a TestScript."; 6476 case TESTSCRIPT: return "A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 6477 case VALUESET: return "A value set specifies a set of codes drawn from one or more code systems."; 6478 case VISIONPRESCRIPTION: return "An authorization for the supply of glasses and/or contact lenses to a patient."; 6479 case NULL: return null; 6480 default: return "?"; 6481 } 6482 } 6483 public String getDisplay() { 6484 switch (this) { 6485 case ADDRESS: return "Address"; 6486 case AGE: return "Age"; 6487 case ANNOTATION: return "Annotation"; 6488 case ATTACHMENT: return "Attachment"; 6489 case BACKBONEELEMENT: return "BackboneElement"; 6490 case CODEABLECONCEPT: return "CodeableConcept"; 6491 case CODING: return "Coding"; 6492 case CONTACTDETAIL: return "ContactDetail"; 6493 case CONTACTPOINT: return "ContactPoint"; 6494 case CONTRIBUTOR: return "Contributor"; 6495 case COUNT: return "Count"; 6496 case DATAREQUIREMENT: return "DataRequirement"; 6497 case DISTANCE: return "Distance"; 6498 case DOSAGE: return "Dosage"; 6499 case DURATION: return "Duration"; 6500 case ELEMENT: return "Element"; 6501 case ELEMENTDEFINITION: return "ElementDefinition"; 6502 case EXTENSION: return "Extension"; 6503 case HUMANNAME: return "HumanName"; 6504 case IDENTIFIER: return "Identifier"; 6505 case META: return "Meta"; 6506 case MONEY: return "Money"; 6507 case NARRATIVE: return "Narrative"; 6508 case PARAMETERDEFINITION: return "ParameterDefinition"; 6509 case PERIOD: return "Period"; 6510 case QUANTITY: return "Quantity"; 6511 case RANGE: return "Range"; 6512 case RATIO: return "Ratio"; 6513 case REFERENCE: return "Reference"; 6514 case RELATEDARTIFACT: return "RelatedArtifact"; 6515 case SAMPLEDDATA: return "SampledData"; 6516 case SIGNATURE: return "Signature"; 6517 case SIMPLEQUANTITY: return "SimpleQuantity"; 6518 case TIMING: return "Timing"; 6519 case TRIGGERDEFINITION: return "TriggerDefinition"; 6520 case USAGECONTEXT: return "UsageContext"; 6521 case BASE64BINARY: return "base64Binary"; 6522 case BOOLEAN: return "boolean"; 6523 case CODE: return "code"; 6524 case DATE: return "date"; 6525 case DATETIME: return "dateTime"; 6526 case DECIMAL: return "decimal"; 6527 case ID: return "id"; 6528 case INSTANT: return "instant"; 6529 case INTEGER: return "integer"; 6530 case MARKDOWN: return "markdown"; 6531 case OID: return "oid"; 6532 case POSITIVEINT: return "positiveInt"; 6533 case STRING: return "string"; 6534 case TIME: return "time"; 6535 case UNSIGNEDINT: return "unsignedInt"; 6536 case URI: return "uri"; 6537 case UUID: return "uuid"; 6538 case XHTML: return "XHTML"; 6539 case ACCOUNT: return "Account"; 6540 case ACTIVITYDEFINITION: return "ActivityDefinition"; 6541 case ADVERSEEVENT: return "AdverseEvent"; 6542 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 6543 case APPOINTMENT: return "Appointment"; 6544 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 6545 case AUDITEVENT: return "AuditEvent"; 6546 case BASIC: return "Basic"; 6547 case BINARY: return "Binary"; 6548 case BODYSITE: return "BodySite"; 6549 case BUNDLE: return "Bundle"; 6550 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 6551 case CAREPLAN: return "CarePlan"; 6552 case CARETEAM: return "CareTeam"; 6553 case CHARGEITEM: return "ChargeItem"; 6554 case CLAIM: return "Claim"; 6555 case CLAIMRESPONSE: return "ClaimResponse"; 6556 case CLINICALIMPRESSION: return "ClinicalImpression"; 6557 case CODESYSTEM: return "CodeSystem"; 6558 case COMMUNICATION: return "Communication"; 6559 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 6560 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 6561 case COMPOSITION: return "Composition"; 6562 case CONCEPTMAP: return "ConceptMap"; 6563 case CONDITION: return "Condition"; 6564 case CONSENT: return "Consent"; 6565 case CONTRACT: return "Contract"; 6566 case COVERAGE: return "Coverage"; 6567 case DATAELEMENT: return "DataElement"; 6568 case DETECTEDISSUE: return "DetectedIssue"; 6569 case DEVICE: return "Device"; 6570 case DEVICECOMPONENT: return "DeviceComponent"; 6571 case DEVICEMETRIC: return "DeviceMetric"; 6572 case DEVICEREQUEST: return "DeviceRequest"; 6573 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 6574 case DIAGNOSTICREPORT: return "DiagnosticReport"; 6575 case DOCUMENTMANIFEST: return "DocumentManifest"; 6576 case DOCUMENTREFERENCE: return "DocumentReference"; 6577 case DOMAINRESOURCE: return "DomainResource"; 6578 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 6579 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 6580 case ENCOUNTER: return "Encounter"; 6581 case ENDPOINT: return "Endpoint"; 6582 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 6583 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 6584 case EPISODEOFCARE: return "EpisodeOfCare"; 6585 case EXPANSIONPROFILE: return "ExpansionProfile"; 6586 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 6587 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 6588 case FLAG: return "Flag"; 6589 case GOAL: return "Goal"; 6590 case GRAPHDEFINITION: return "GraphDefinition"; 6591 case GROUP: return "Group"; 6592 case GUIDANCERESPONSE: return "GuidanceResponse"; 6593 case HEALTHCARESERVICE: return "HealthcareService"; 6594 case IMAGINGMANIFEST: return "ImagingManifest"; 6595 case IMAGINGSTUDY: return "ImagingStudy"; 6596 case IMMUNIZATION: return "Immunization"; 6597 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 6598 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 6599 case LIBRARY: return "Library"; 6600 case LINKAGE: return "Linkage"; 6601 case LIST: return "List"; 6602 case LOCATION: return "Location"; 6603 case MEASURE: return "Measure"; 6604 case MEASUREREPORT: return "MeasureReport"; 6605 case MEDIA: return "Media"; 6606 case MEDICATION: return "Medication"; 6607 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 6608 case MEDICATIONDISPENSE: return "MedicationDispense"; 6609 case MEDICATIONREQUEST: return "MedicationRequest"; 6610 case MEDICATIONSTATEMENT: return "MedicationStatement"; 6611 case MESSAGEDEFINITION: return "MessageDefinition"; 6612 case MESSAGEHEADER: return "MessageHeader"; 6613 case NAMINGSYSTEM: return "NamingSystem"; 6614 case NUTRITIONORDER: return "NutritionOrder"; 6615 case OBSERVATION: return "Observation"; 6616 case OPERATIONDEFINITION: return "OperationDefinition"; 6617 case OPERATIONOUTCOME: return "OperationOutcome"; 6618 case ORGANIZATION: return "Organization"; 6619 case PARAMETERS: return "Parameters"; 6620 case PATIENT: return "Patient"; 6621 case PAYMENTNOTICE: return "PaymentNotice"; 6622 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 6623 case PERSON: return "Person"; 6624 case PLANDEFINITION: return "PlanDefinition"; 6625 case PRACTITIONER: return "Practitioner"; 6626 case PRACTITIONERROLE: return "PractitionerRole"; 6627 case PROCEDURE: return "Procedure"; 6628 case PROCEDUREREQUEST: return "ProcedureRequest"; 6629 case PROCESSREQUEST: return "ProcessRequest"; 6630 case PROCESSRESPONSE: return "ProcessResponse"; 6631 case PROVENANCE: return "Provenance"; 6632 case QUESTIONNAIRE: return "Questionnaire"; 6633 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 6634 case REFERRALREQUEST: return "ReferralRequest"; 6635 case RELATEDPERSON: return "RelatedPerson"; 6636 case REQUESTGROUP: return "RequestGroup"; 6637 case RESEARCHSTUDY: return "ResearchStudy"; 6638 case RESEARCHSUBJECT: return "ResearchSubject"; 6639 case RESOURCE: return "Resource"; 6640 case RISKASSESSMENT: return "RiskAssessment"; 6641 case SCHEDULE: return "Schedule"; 6642 case SEARCHPARAMETER: return "SearchParameter"; 6643 case SEQUENCE: return "Sequence"; 6644 case SERVICEDEFINITION: return "ServiceDefinition"; 6645 case SLOT: return "Slot"; 6646 case SPECIMEN: return "Specimen"; 6647 case STRUCTUREDEFINITION: return "StructureDefinition"; 6648 case STRUCTUREMAP: return "StructureMap"; 6649 case SUBSCRIPTION: return "Subscription"; 6650 case SUBSTANCE: return "Substance"; 6651 case SUPPLYDELIVERY: return "SupplyDelivery"; 6652 case SUPPLYREQUEST: return "SupplyRequest"; 6653 case TASK: return "Task"; 6654 case TESTREPORT: return "TestReport"; 6655 case TESTSCRIPT: return "TestScript"; 6656 case VALUESET: return "ValueSet"; 6657 case VISIONPRESCRIPTION: return "VisionPrescription"; 6658 case NULL: return null; 6659 default: return "?"; 6660 } 6661 } 6662 } 6663 6664 public static class FHIRDefinedTypeEnumFactory implements EnumFactory<FHIRDefinedType> { 6665 public FHIRDefinedType fromCode(String codeString) throws IllegalArgumentException { 6666 if (codeString == null || "".equals(codeString)) 6667 if (codeString == null || "".equals(codeString)) 6668 return null; 6669 if ("Address".equals(codeString)) 6670 return FHIRDefinedType.ADDRESS; 6671 if ("Age".equals(codeString)) 6672 return FHIRDefinedType.AGE; 6673 if ("Annotation".equals(codeString)) 6674 return FHIRDefinedType.ANNOTATION; 6675 if ("Attachment".equals(codeString)) 6676 return FHIRDefinedType.ATTACHMENT; 6677 if ("BackboneElement".equals(codeString)) 6678 return FHIRDefinedType.BACKBONEELEMENT; 6679 if ("CodeableConcept".equals(codeString)) 6680 return FHIRDefinedType.CODEABLECONCEPT; 6681 if ("Coding".equals(codeString)) 6682 return FHIRDefinedType.CODING; 6683 if ("ContactDetail".equals(codeString)) 6684 return FHIRDefinedType.CONTACTDETAIL; 6685 if ("ContactPoint".equals(codeString)) 6686 return FHIRDefinedType.CONTACTPOINT; 6687 if ("Contributor".equals(codeString)) 6688 return FHIRDefinedType.CONTRIBUTOR; 6689 if ("Count".equals(codeString)) 6690 return FHIRDefinedType.COUNT; 6691 if ("DataRequirement".equals(codeString)) 6692 return FHIRDefinedType.DATAREQUIREMENT; 6693 if ("Distance".equals(codeString)) 6694 return FHIRDefinedType.DISTANCE; 6695 if ("Dosage".equals(codeString)) 6696 return FHIRDefinedType.DOSAGE; 6697 if ("Duration".equals(codeString)) 6698 return FHIRDefinedType.DURATION; 6699 if ("Element".equals(codeString)) 6700 return FHIRDefinedType.ELEMENT; 6701 if ("ElementDefinition".equals(codeString)) 6702 return FHIRDefinedType.ELEMENTDEFINITION; 6703 if ("Extension".equals(codeString)) 6704 return FHIRDefinedType.EXTENSION; 6705 if ("HumanName".equals(codeString)) 6706 return FHIRDefinedType.HUMANNAME; 6707 if ("Identifier".equals(codeString)) 6708 return FHIRDefinedType.IDENTIFIER; 6709 if ("Meta".equals(codeString)) 6710 return FHIRDefinedType.META; 6711 if ("Money".equals(codeString)) 6712 return FHIRDefinedType.MONEY; 6713 if ("Narrative".equals(codeString)) 6714 return FHIRDefinedType.NARRATIVE; 6715 if ("ParameterDefinition".equals(codeString)) 6716 return FHIRDefinedType.PARAMETERDEFINITION; 6717 if ("Period".equals(codeString)) 6718 return FHIRDefinedType.PERIOD; 6719 if ("Quantity".equals(codeString)) 6720 return FHIRDefinedType.QUANTITY; 6721 if ("Range".equals(codeString)) 6722 return FHIRDefinedType.RANGE; 6723 if ("Ratio".equals(codeString)) 6724 return FHIRDefinedType.RATIO; 6725 if ("Reference".equals(codeString)) 6726 return FHIRDefinedType.REFERENCE; 6727 if ("RelatedArtifact".equals(codeString)) 6728 return FHIRDefinedType.RELATEDARTIFACT; 6729 if ("SampledData".equals(codeString)) 6730 return FHIRDefinedType.SAMPLEDDATA; 6731 if ("Signature".equals(codeString)) 6732 return FHIRDefinedType.SIGNATURE; 6733 if ("SimpleQuantity".equals(codeString)) 6734 return FHIRDefinedType.SIMPLEQUANTITY; 6735 if ("Timing".equals(codeString)) 6736 return FHIRDefinedType.TIMING; 6737 if ("TriggerDefinition".equals(codeString)) 6738 return FHIRDefinedType.TRIGGERDEFINITION; 6739 if ("UsageContext".equals(codeString)) 6740 return FHIRDefinedType.USAGECONTEXT; 6741 if ("base64Binary".equals(codeString)) 6742 return FHIRDefinedType.BASE64BINARY; 6743 if ("boolean".equals(codeString)) 6744 return FHIRDefinedType.BOOLEAN; 6745 if ("code".equals(codeString)) 6746 return FHIRDefinedType.CODE; 6747 if ("date".equals(codeString)) 6748 return FHIRDefinedType.DATE; 6749 if ("dateTime".equals(codeString)) 6750 return FHIRDefinedType.DATETIME; 6751 if ("decimal".equals(codeString)) 6752 return FHIRDefinedType.DECIMAL; 6753 if ("id".equals(codeString)) 6754 return FHIRDefinedType.ID; 6755 if ("instant".equals(codeString)) 6756 return FHIRDefinedType.INSTANT; 6757 if ("integer".equals(codeString)) 6758 return FHIRDefinedType.INTEGER; 6759 if ("markdown".equals(codeString)) 6760 return FHIRDefinedType.MARKDOWN; 6761 if ("oid".equals(codeString)) 6762 return FHIRDefinedType.OID; 6763 if ("positiveInt".equals(codeString)) 6764 return FHIRDefinedType.POSITIVEINT; 6765 if ("string".equals(codeString)) 6766 return FHIRDefinedType.STRING; 6767 if ("time".equals(codeString)) 6768 return FHIRDefinedType.TIME; 6769 if ("unsignedInt".equals(codeString)) 6770 return FHIRDefinedType.UNSIGNEDINT; 6771 if ("uri".equals(codeString)) 6772 return FHIRDefinedType.URI; 6773 if ("uuid".equals(codeString)) 6774 return FHIRDefinedType.UUID; 6775 if ("xhtml".equals(codeString)) 6776 return FHIRDefinedType.XHTML; 6777 if ("Account".equals(codeString)) 6778 return FHIRDefinedType.ACCOUNT; 6779 if ("ActivityDefinition".equals(codeString)) 6780 return FHIRDefinedType.ACTIVITYDEFINITION; 6781 if ("AdverseEvent".equals(codeString)) 6782 return FHIRDefinedType.ADVERSEEVENT; 6783 if ("AllergyIntolerance".equals(codeString)) 6784 return FHIRDefinedType.ALLERGYINTOLERANCE; 6785 if ("Appointment".equals(codeString)) 6786 return FHIRDefinedType.APPOINTMENT; 6787 if ("AppointmentResponse".equals(codeString)) 6788 return FHIRDefinedType.APPOINTMENTRESPONSE; 6789 if ("AuditEvent".equals(codeString)) 6790 return FHIRDefinedType.AUDITEVENT; 6791 if ("Basic".equals(codeString)) 6792 return FHIRDefinedType.BASIC; 6793 if ("Binary".equals(codeString)) 6794 return FHIRDefinedType.BINARY; 6795 if ("BodySite".equals(codeString)) 6796 return FHIRDefinedType.BODYSITE; 6797 if ("Bundle".equals(codeString)) 6798 return FHIRDefinedType.BUNDLE; 6799 if ("CapabilityStatement".equals(codeString)) 6800 return FHIRDefinedType.CAPABILITYSTATEMENT; 6801 if ("CarePlan".equals(codeString)) 6802 return FHIRDefinedType.CAREPLAN; 6803 if ("CareTeam".equals(codeString)) 6804 return FHIRDefinedType.CARETEAM; 6805 if ("ChargeItem".equals(codeString)) 6806 return FHIRDefinedType.CHARGEITEM; 6807 if ("Claim".equals(codeString)) 6808 return FHIRDefinedType.CLAIM; 6809 if ("ClaimResponse".equals(codeString)) 6810 return FHIRDefinedType.CLAIMRESPONSE; 6811 if ("ClinicalImpression".equals(codeString)) 6812 return FHIRDefinedType.CLINICALIMPRESSION; 6813 if ("CodeSystem".equals(codeString)) 6814 return FHIRDefinedType.CODESYSTEM; 6815 if ("Communication".equals(codeString)) 6816 return FHIRDefinedType.COMMUNICATION; 6817 if ("CommunicationRequest".equals(codeString)) 6818 return FHIRDefinedType.COMMUNICATIONREQUEST; 6819 if ("CompartmentDefinition".equals(codeString)) 6820 return FHIRDefinedType.COMPARTMENTDEFINITION; 6821 if ("Composition".equals(codeString)) 6822 return FHIRDefinedType.COMPOSITION; 6823 if ("ConceptMap".equals(codeString)) 6824 return FHIRDefinedType.CONCEPTMAP; 6825 if ("Condition".equals(codeString)) 6826 return FHIRDefinedType.CONDITION; 6827 if ("Consent".equals(codeString)) 6828 return FHIRDefinedType.CONSENT; 6829 if ("Contract".equals(codeString)) 6830 return FHIRDefinedType.CONTRACT; 6831 if ("Coverage".equals(codeString)) 6832 return FHIRDefinedType.COVERAGE; 6833 if ("DataElement".equals(codeString)) 6834 return FHIRDefinedType.DATAELEMENT; 6835 if ("DetectedIssue".equals(codeString)) 6836 return FHIRDefinedType.DETECTEDISSUE; 6837 if ("Device".equals(codeString)) 6838 return FHIRDefinedType.DEVICE; 6839 if ("DeviceComponent".equals(codeString)) 6840 return FHIRDefinedType.DEVICECOMPONENT; 6841 if ("DeviceMetric".equals(codeString)) 6842 return FHIRDefinedType.DEVICEMETRIC; 6843 if ("DeviceRequest".equals(codeString)) 6844 return FHIRDefinedType.DEVICEREQUEST; 6845 if ("DeviceUseStatement".equals(codeString)) 6846 return FHIRDefinedType.DEVICEUSESTATEMENT; 6847 if ("DiagnosticReport".equals(codeString)) 6848 return FHIRDefinedType.DIAGNOSTICREPORT; 6849 if ("DocumentManifest".equals(codeString)) 6850 return FHIRDefinedType.DOCUMENTMANIFEST; 6851 if ("DocumentReference".equals(codeString)) 6852 return FHIRDefinedType.DOCUMENTREFERENCE; 6853 if ("DomainResource".equals(codeString)) 6854 return FHIRDefinedType.DOMAINRESOURCE; 6855 if ("EligibilityRequest".equals(codeString)) 6856 return FHIRDefinedType.ELIGIBILITYREQUEST; 6857 if ("EligibilityResponse".equals(codeString)) 6858 return FHIRDefinedType.ELIGIBILITYRESPONSE; 6859 if ("Encounter".equals(codeString)) 6860 return FHIRDefinedType.ENCOUNTER; 6861 if ("Endpoint".equals(codeString)) 6862 return FHIRDefinedType.ENDPOINT; 6863 if ("EnrollmentRequest".equals(codeString)) 6864 return FHIRDefinedType.ENROLLMENTREQUEST; 6865 if ("EnrollmentResponse".equals(codeString)) 6866 return FHIRDefinedType.ENROLLMENTRESPONSE; 6867 if ("EpisodeOfCare".equals(codeString)) 6868 return FHIRDefinedType.EPISODEOFCARE; 6869 if ("ExpansionProfile".equals(codeString)) 6870 return FHIRDefinedType.EXPANSIONPROFILE; 6871 if ("ExplanationOfBenefit".equals(codeString)) 6872 return FHIRDefinedType.EXPLANATIONOFBENEFIT; 6873 if ("FamilyMemberHistory".equals(codeString)) 6874 return FHIRDefinedType.FAMILYMEMBERHISTORY; 6875 if ("Flag".equals(codeString)) 6876 return FHIRDefinedType.FLAG; 6877 if ("Goal".equals(codeString)) 6878 return FHIRDefinedType.GOAL; 6879 if ("GraphDefinition".equals(codeString)) 6880 return FHIRDefinedType.GRAPHDEFINITION; 6881 if ("Group".equals(codeString)) 6882 return FHIRDefinedType.GROUP; 6883 if ("GuidanceResponse".equals(codeString)) 6884 return FHIRDefinedType.GUIDANCERESPONSE; 6885 if ("HealthcareService".equals(codeString)) 6886 return FHIRDefinedType.HEALTHCARESERVICE; 6887 if ("ImagingManifest".equals(codeString)) 6888 return FHIRDefinedType.IMAGINGMANIFEST; 6889 if ("ImagingStudy".equals(codeString)) 6890 return FHIRDefinedType.IMAGINGSTUDY; 6891 if ("Immunization".equals(codeString)) 6892 return FHIRDefinedType.IMMUNIZATION; 6893 if ("ImmunizationRecommendation".equals(codeString)) 6894 return FHIRDefinedType.IMMUNIZATIONRECOMMENDATION; 6895 if ("ImplementationGuide".equals(codeString)) 6896 return FHIRDefinedType.IMPLEMENTATIONGUIDE; 6897 if ("Library".equals(codeString)) 6898 return FHIRDefinedType.LIBRARY; 6899 if ("Linkage".equals(codeString)) 6900 return FHIRDefinedType.LINKAGE; 6901 if ("List".equals(codeString)) 6902 return FHIRDefinedType.LIST; 6903 if ("Location".equals(codeString)) 6904 return FHIRDefinedType.LOCATION; 6905 if ("Measure".equals(codeString)) 6906 return FHIRDefinedType.MEASURE; 6907 if ("MeasureReport".equals(codeString)) 6908 return FHIRDefinedType.MEASUREREPORT; 6909 if ("Media".equals(codeString)) 6910 return FHIRDefinedType.MEDIA; 6911 if ("Medication".equals(codeString)) 6912 return FHIRDefinedType.MEDICATION; 6913 if ("MedicationAdministration".equals(codeString)) 6914 return FHIRDefinedType.MEDICATIONADMINISTRATION; 6915 if ("MedicationDispense".equals(codeString)) 6916 return FHIRDefinedType.MEDICATIONDISPENSE; 6917 if ("MedicationRequest".equals(codeString)) 6918 return FHIRDefinedType.MEDICATIONREQUEST; 6919 if ("MedicationStatement".equals(codeString)) 6920 return FHIRDefinedType.MEDICATIONSTATEMENT; 6921 if ("MessageDefinition".equals(codeString)) 6922 return FHIRDefinedType.MESSAGEDEFINITION; 6923 if ("MessageHeader".equals(codeString)) 6924 return FHIRDefinedType.MESSAGEHEADER; 6925 if ("NamingSystem".equals(codeString)) 6926 return FHIRDefinedType.NAMINGSYSTEM; 6927 if ("NutritionOrder".equals(codeString)) 6928 return FHIRDefinedType.NUTRITIONORDER; 6929 if ("Observation".equals(codeString)) 6930 return FHIRDefinedType.OBSERVATION; 6931 if ("OperationDefinition".equals(codeString)) 6932 return FHIRDefinedType.OPERATIONDEFINITION; 6933 if ("OperationOutcome".equals(codeString)) 6934 return FHIRDefinedType.OPERATIONOUTCOME; 6935 if ("Organization".equals(codeString)) 6936 return FHIRDefinedType.ORGANIZATION; 6937 if ("Parameters".equals(codeString)) 6938 return FHIRDefinedType.PARAMETERS; 6939 if ("Patient".equals(codeString)) 6940 return FHIRDefinedType.PATIENT; 6941 if ("PaymentNotice".equals(codeString)) 6942 return FHIRDefinedType.PAYMENTNOTICE; 6943 if ("PaymentReconciliation".equals(codeString)) 6944 return FHIRDefinedType.PAYMENTRECONCILIATION; 6945 if ("Person".equals(codeString)) 6946 return FHIRDefinedType.PERSON; 6947 if ("PlanDefinition".equals(codeString)) 6948 return FHIRDefinedType.PLANDEFINITION; 6949 if ("Practitioner".equals(codeString)) 6950 return FHIRDefinedType.PRACTITIONER; 6951 if ("PractitionerRole".equals(codeString)) 6952 return FHIRDefinedType.PRACTITIONERROLE; 6953 if ("Procedure".equals(codeString)) 6954 return FHIRDefinedType.PROCEDURE; 6955 if ("ProcedureRequest".equals(codeString)) 6956 return FHIRDefinedType.PROCEDUREREQUEST; 6957 if ("ProcessRequest".equals(codeString)) 6958 return FHIRDefinedType.PROCESSREQUEST; 6959 if ("ProcessResponse".equals(codeString)) 6960 return FHIRDefinedType.PROCESSRESPONSE; 6961 if ("Provenance".equals(codeString)) 6962 return FHIRDefinedType.PROVENANCE; 6963 if ("Questionnaire".equals(codeString)) 6964 return FHIRDefinedType.QUESTIONNAIRE; 6965 if ("QuestionnaireResponse".equals(codeString)) 6966 return FHIRDefinedType.QUESTIONNAIRERESPONSE; 6967 if ("ReferralRequest".equals(codeString)) 6968 return FHIRDefinedType.REFERRALREQUEST; 6969 if ("RelatedPerson".equals(codeString)) 6970 return FHIRDefinedType.RELATEDPERSON; 6971 if ("RequestGroup".equals(codeString)) 6972 return FHIRDefinedType.REQUESTGROUP; 6973 if ("ResearchStudy".equals(codeString)) 6974 return FHIRDefinedType.RESEARCHSTUDY; 6975 if ("ResearchSubject".equals(codeString)) 6976 return FHIRDefinedType.RESEARCHSUBJECT; 6977 if ("Resource".equals(codeString)) 6978 return FHIRDefinedType.RESOURCE; 6979 if ("RiskAssessment".equals(codeString)) 6980 return FHIRDefinedType.RISKASSESSMENT; 6981 if ("Schedule".equals(codeString)) 6982 return FHIRDefinedType.SCHEDULE; 6983 if ("SearchParameter".equals(codeString)) 6984 return FHIRDefinedType.SEARCHPARAMETER; 6985 if ("Sequence".equals(codeString)) 6986 return FHIRDefinedType.SEQUENCE; 6987 if ("ServiceDefinition".equals(codeString)) 6988 return FHIRDefinedType.SERVICEDEFINITION; 6989 if ("Slot".equals(codeString)) 6990 return FHIRDefinedType.SLOT; 6991 if ("Specimen".equals(codeString)) 6992 return FHIRDefinedType.SPECIMEN; 6993 if ("StructureDefinition".equals(codeString)) 6994 return FHIRDefinedType.STRUCTUREDEFINITION; 6995 if ("StructureMap".equals(codeString)) 6996 return FHIRDefinedType.STRUCTUREMAP; 6997 if ("Subscription".equals(codeString)) 6998 return FHIRDefinedType.SUBSCRIPTION; 6999 if ("Substance".equals(codeString)) 7000 return FHIRDefinedType.SUBSTANCE; 7001 if ("SupplyDelivery".equals(codeString)) 7002 return FHIRDefinedType.SUPPLYDELIVERY; 7003 if ("SupplyRequest".equals(codeString)) 7004 return FHIRDefinedType.SUPPLYREQUEST; 7005 if ("Task".equals(codeString)) 7006 return FHIRDefinedType.TASK; 7007 if ("TestReport".equals(codeString)) 7008 return FHIRDefinedType.TESTREPORT; 7009 if ("TestScript".equals(codeString)) 7010 return FHIRDefinedType.TESTSCRIPT; 7011 if ("ValueSet".equals(codeString)) 7012 return FHIRDefinedType.VALUESET; 7013 if ("VisionPrescription".equals(codeString)) 7014 return FHIRDefinedType.VISIONPRESCRIPTION; 7015 throw new IllegalArgumentException("Unknown FHIRDefinedType code '"+codeString+"'"); 7016 } 7017 public Enumeration<FHIRDefinedType> fromType(PrimitiveType<?> code) throws FHIRException { 7018 if (code == null) 7019 return null; 7020 if (code.isEmpty()) 7021 return new Enumeration<FHIRDefinedType>(this); 7022 String codeString = code.asStringValue(); 7023 if (codeString == null || "".equals(codeString)) 7024 return null; 7025 if ("Address".equals(codeString)) 7026 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADDRESS); 7027 if ("Age".equals(codeString)) 7028 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AGE); 7029 if ("Annotation".equals(codeString)) 7030 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ANNOTATION); 7031 if ("Attachment".equals(codeString)) 7032 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ATTACHMENT); 7033 if ("BackboneElement".equals(codeString)) 7034 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BACKBONEELEMENT); 7035 if ("CodeableConcept".equals(codeString)) 7036 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODEABLECONCEPT); 7037 if ("Coding".equals(codeString)) 7038 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODING); 7039 if ("ContactDetail".equals(codeString)) 7040 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTDETAIL); 7041 if ("ContactPoint".equals(codeString)) 7042 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTACTPOINT); 7043 if ("Contributor".equals(codeString)) 7044 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRIBUTOR); 7045 if ("Count".equals(codeString)) 7046 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COUNT); 7047 if ("DataRequirement".equals(codeString)) 7048 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATAREQUIREMENT); 7049 if ("Distance".equals(codeString)) 7050 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DISTANCE); 7051 if ("Dosage".equals(codeString)) 7052 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOSAGE); 7053 if ("Duration".equals(codeString)) 7054 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DURATION); 7055 if ("Element".equals(codeString)) 7056 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENT); 7057 if ("ElementDefinition".equals(codeString)) 7058 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELEMENTDEFINITION); 7059 if ("Extension".equals(codeString)) 7060 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXTENSION); 7061 if ("HumanName".equals(codeString)) 7062 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HUMANNAME); 7063 if ("Identifier".equals(codeString)) 7064 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IDENTIFIER); 7065 if ("Meta".equals(codeString)) 7066 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.META); 7067 if ("Money".equals(codeString)) 7068 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MONEY); 7069 if ("Narrative".equals(codeString)) 7070 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NARRATIVE); 7071 if ("ParameterDefinition".equals(codeString)) 7072 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERDEFINITION); 7073 if ("Period".equals(codeString)) 7074 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERIOD); 7075 if ("Quantity".equals(codeString)) 7076 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUANTITY); 7077 if ("Range".equals(codeString)) 7078 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RANGE); 7079 if ("Ratio".equals(codeString)) 7080 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RATIO); 7081 if ("Reference".equals(codeString)) 7082 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REFERENCE); 7083 if ("RelatedArtifact".equals(codeString)) 7084 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDARTIFACT); 7085 if ("SampledData".equals(codeString)) 7086 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SAMPLEDDATA); 7087 if ("Signature".equals(codeString)) 7088 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIGNATURE); 7089 if ("SimpleQuantity".equals(codeString)) 7090 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SIMPLEQUANTITY); 7091 if ("Timing".equals(codeString)) 7092 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIMING); 7093 if ("TriggerDefinition".equals(codeString)) 7094 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TRIGGERDEFINITION); 7095 if ("UsageContext".equals(codeString)) 7096 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.USAGECONTEXT); 7097 if ("base64Binary".equals(codeString)) 7098 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASE64BINARY); 7099 if ("boolean".equals(codeString)) 7100 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BOOLEAN); 7101 if ("code".equals(codeString)) 7102 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODE); 7103 if ("date".equals(codeString)) 7104 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATE); 7105 if ("dateTime".equals(codeString)) 7106 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATETIME); 7107 if ("decimal".equals(codeString)) 7108 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DECIMAL); 7109 if ("id".equals(codeString)) 7110 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ID); 7111 if ("instant".equals(codeString)) 7112 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INSTANT); 7113 if ("integer".equals(codeString)) 7114 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.INTEGER); 7115 if ("markdown".equals(codeString)) 7116 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MARKDOWN); 7117 if ("oid".equals(codeString)) 7118 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OID); 7119 if ("positiveInt".equals(codeString)) 7120 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.POSITIVEINT); 7121 if ("string".equals(codeString)) 7122 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRING); 7123 if ("time".equals(codeString)) 7124 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TIME); 7125 if ("unsignedInt".equals(codeString)) 7126 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UNSIGNEDINT); 7127 if ("uri".equals(codeString)) 7128 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.URI); 7129 if ("uuid".equals(codeString)) 7130 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.UUID); 7131 if ("xhtml".equals(codeString)) 7132 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.XHTML); 7133 if ("Account".equals(codeString)) 7134 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACCOUNT); 7135 if ("ActivityDefinition".equals(codeString)) 7136 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ACTIVITYDEFINITION); 7137 if ("AdverseEvent".equals(codeString)) 7138 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ADVERSEEVENT); 7139 if ("AllergyIntolerance".equals(codeString)) 7140 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ALLERGYINTOLERANCE); 7141 if ("Appointment".equals(codeString)) 7142 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENT); 7143 if ("AppointmentResponse".equals(codeString)) 7144 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.APPOINTMENTRESPONSE); 7145 if ("AuditEvent".equals(codeString)) 7146 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.AUDITEVENT); 7147 if ("Basic".equals(codeString)) 7148 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BASIC); 7149 if ("Binary".equals(codeString)) 7150 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BINARY); 7151 if ("BodySite".equals(codeString)) 7152 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BODYSITE); 7153 if ("Bundle".equals(codeString)) 7154 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.BUNDLE); 7155 if ("CapabilityStatement".equals(codeString)) 7156 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAPABILITYSTATEMENT); 7157 if ("CarePlan".equals(codeString)) 7158 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CAREPLAN); 7159 if ("CareTeam".equals(codeString)) 7160 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CARETEAM); 7161 if ("ChargeItem".equals(codeString)) 7162 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CHARGEITEM); 7163 if ("Claim".equals(codeString)) 7164 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIM); 7165 if ("ClaimResponse".equals(codeString)) 7166 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLAIMRESPONSE); 7167 if ("ClinicalImpression".equals(codeString)) 7168 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CLINICALIMPRESSION); 7169 if ("CodeSystem".equals(codeString)) 7170 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CODESYSTEM); 7171 if ("Communication".equals(codeString)) 7172 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATION); 7173 if ("CommunicationRequest".equals(codeString)) 7174 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMMUNICATIONREQUEST); 7175 if ("CompartmentDefinition".equals(codeString)) 7176 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPARTMENTDEFINITION); 7177 if ("Composition".equals(codeString)) 7178 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COMPOSITION); 7179 if ("ConceptMap".equals(codeString)) 7180 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONCEPTMAP); 7181 if ("Condition".equals(codeString)) 7182 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONDITION); 7183 if ("Consent".equals(codeString)) 7184 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONSENT); 7185 if ("Contract".equals(codeString)) 7186 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.CONTRACT); 7187 if ("Coverage".equals(codeString)) 7188 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.COVERAGE); 7189 if ("DataElement".equals(codeString)) 7190 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DATAELEMENT); 7191 if ("DetectedIssue".equals(codeString)) 7192 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DETECTEDISSUE); 7193 if ("Device".equals(codeString)) 7194 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICE); 7195 if ("DeviceComponent".equals(codeString)) 7196 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICECOMPONENT); 7197 if ("DeviceMetric".equals(codeString)) 7198 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEMETRIC); 7199 if ("DeviceRequest".equals(codeString)) 7200 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEREQUEST); 7201 if ("DeviceUseStatement".equals(codeString)) 7202 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DEVICEUSESTATEMENT); 7203 if ("DiagnosticReport".equals(codeString)) 7204 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DIAGNOSTICREPORT); 7205 if ("DocumentManifest".equals(codeString)) 7206 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTMANIFEST); 7207 if ("DocumentReference".equals(codeString)) 7208 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOCUMENTREFERENCE); 7209 if ("DomainResource".equals(codeString)) 7210 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.DOMAINRESOURCE); 7211 if ("EligibilityRequest".equals(codeString)) 7212 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELIGIBILITYREQUEST); 7213 if ("EligibilityResponse".equals(codeString)) 7214 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ELIGIBILITYRESPONSE); 7215 if ("Encounter".equals(codeString)) 7216 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENCOUNTER); 7217 if ("Endpoint".equals(codeString)) 7218 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENDPOINT); 7219 if ("EnrollmentRequest".equals(codeString)) 7220 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTREQUEST); 7221 if ("EnrollmentResponse".equals(codeString)) 7222 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ENROLLMENTRESPONSE); 7223 if ("EpisodeOfCare".equals(codeString)) 7224 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EPISODEOFCARE); 7225 if ("ExpansionProfile".equals(codeString)) 7226 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPANSIONPROFILE); 7227 if ("ExplanationOfBenefit".equals(codeString)) 7228 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.EXPLANATIONOFBENEFIT); 7229 if ("FamilyMemberHistory".equals(codeString)) 7230 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FAMILYMEMBERHISTORY); 7231 if ("Flag".equals(codeString)) 7232 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.FLAG); 7233 if ("Goal".equals(codeString)) 7234 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GOAL); 7235 if ("GraphDefinition".equals(codeString)) 7236 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GRAPHDEFINITION); 7237 if ("Group".equals(codeString)) 7238 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GROUP); 7239 if ("GuidanceResponse".equals(codeString)) 7240 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.GUIDANCERESPONSE); 7241 if ("HealthcareService".equals(codeString)) 7242 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.HEALTHCARESERVICE); 7243 if ("ImagingManifest".equals(codeString)) 7244 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMAGINGMANIFEST); 7245 if ("ImagingStudy".equals(codeString)) 7246 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMAGINGSTUDY); 7247 if ("Immunization".equals(codeString)) 7248 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATION); 7249 if ("ImmunizationRecommendation".equals(codeString)) 7250 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMMUNIZATIONRECOMMENDATION); 7251 if ("ImplementationGuide".equals(codeString)) 7252 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.IMPLEMENTATIONGUIDE); 7253 if ("Library".equals(codeString)) 7254 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIBRARY); 7255 if ("Linkage".equals(codeString)) 7256 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LINKAGE); 7257 if ("List".equals(codeString)) 7258 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LIST); 7259 if ("Location".equals(codeString)) 7260 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.LOCATION); 7261 if ("Measure".equals(codeString)) 7262 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEASURE); 7263 if ("MeasureReport".equals(codeString)) 7264 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEASUREREPORT); 7265 if ("Media".equals(codeString)) 7266 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDIA); 7267 if ("Medication".equals(codeString)) 7268 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATION); 7269 if ("MedicationAdministration".equals(codeString)) 7270 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONADMINISTRATION); 7271 if ("MedicationDispense".equals(codeString)) 7272 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONDISPENSE); 7273 if ("MedicationRequest".equals(codeString)) 7274 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONREQUEST); 7275 if ("MedicationStatement".equals(codeString)) 7276 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MEDICATIONSTATEMENT); 7277 if ("MessageDefinition".equals(codeString)) 7278 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEDEFINITION); 7279 if ("MessageHeader".equals(codeString)) 7280 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.MESSAGEHEADER); 7281 if ("NamingSystem".equals(codeString)) 7282 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NAMINGSYSTEM); 7283 if ("NutritionOrder".equals(codeString)) 7284 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.NUTRITIONORDER); 7285 if ("Observation".equals(codeString)) 7286 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OBSERVATION); 7287 if ("OperationDefinition".equals(codeString)) 7288 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONDEFINITION); 7289 if ("OperationOutcome".equals(codeString)) 7290 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.OPERATIONOUTCOME); 7291 if ("Organization".equals(codeString)) 7292 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.ORGANIZATION); 7293 if ("Parameters".equals(codeString)) 7294 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PARAMETERS); 7295 if ("Patient".equals(codeString)) 7296 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PATIENT); 7297 if ("PaymentNotice".equals(codeString)) 7298 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTNOTICE); 7299 if ("PaymentReconciliation".equals(codeString)) 7300 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PAYMENTRECONCILIATION); 7301 if ("Person".equals(codeString)) 7302 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PERSON); 7303 if ("PlanDefinition".equals(codeString)) 7304 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PLANDEFINITION); 7305 if ("Practitioner".equals(codeString)) 7306 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONER); 7307 if ("PractitionerRole".equals(codeString)) 7308 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PRACTITIONERROLE); 7309 if ("Procedure".equals(codeString)) 7310 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCEDURE); 7311 if ("ProcedureRequest".equals(codeString)) 7312 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCEDUREREQUEST); 7313 if ("ProcessRequest".equals(codeString)) 7314 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCESSREQUEST); 7315 if ("ProcessResponse".equals(codeString)) 7316 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROCESSRESPONSE); 7317 if ("Provenance".equals(codeString)) 7318 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.PROVENANCE); 7319 if ("Questionnaire".equals(codeString)) 7320 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRE); 7321 if ("QuestionnaireResponse".equals(codeString)) 7322 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.QUESTIONNAIRERESPONSE); 7323 if ("ReferralRequest".equals(codeString)) 7324 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REFERRALREQUEST); 7325 if ("RelatedPerson".equals(codeString)) 7326 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RELATEDPERSON); 7327 if ("RequestGroup".equals(codeString)) 7328 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.REQUESTGROUP); 7329 if ("ResearchStudy".equals(codeString)) 7330 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHSTUDY); 7331 if ("ResearchSubject".equals(codeString)) 7332 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESEARCHSUBJECT); 7333 if ("Resource".equals(codeString)) 7334 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RESOURCE); 7335 if ("RiskAssessment".equals(codeString)) 7336 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.RISKASSESSMENT); 7337 if ("Schedule".equals(codeString)) 7338 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SCHEDULE); 7339 if ("SearchParameter".equals(codeString)) 7340 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SEARCHPARAMETER); 7341 if ("Sequence".equals(codeString)) 7342 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SEQUENCE); 7343 if ("ServiceDefinition".equals(codeString)) 7344 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SERVICEDEFINITION); 7345 if ("Slot".equals(codeString)) 7346 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SLOT); 7347 if ("Specimen".equals(codeString)) 7348 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SPECIMEN); 7349 if ("StructureDefinition".equals(codeString)) 7350 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREDEFINITION); 7351 if ("StructureMap".equals(codeString)) 7352 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.STRUCTUREMAP); 7353 if ("Subscription".equals(codeString)) 7354 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSCRIPTION); 7355 if ("Substance".equals(codeString)) 7356 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUBSTANCE); 7357 if ("SupplyDelivery".equals(codeString)) 7358 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYDELIVERY); 7359 if ("SupplyRequest".equals(codeString)) 7360 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.SUPPLYREQUEST); 7361 if ("Task".equals(codeString)) 7362 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TASK); 7363 if ("TestReport".equals(codeString)) 7364 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTREPORT); 7365 if ("TestScript".equals(codeString)) 7366 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.TESTSCRIPT); 7367 if ("ValueSet".equals(codeString)) 7368 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VALUESET); 7369 if ("VisionPrescription".equals(codeString)) 7370 return new Enumeration<FHIRDefinedType>(this, FHIRDefinedType.VISIONPRESCRIPTION); 7371 throw new FHIRException("Unknown FHIRDefinedType code '"+codeString+"'"); 7372 } 7373 public String toCode(FHIRDefinedType code) { 7374 if (code == FHIRDefinedType.ADDRESS) 7375 return "Address"; 7376 if (code == FHIRDefinedType.AGE) 7377 return "Age"; 7378 if (code == FHIRDefinedType.ANNOTATION) 7379 return "Annotation"; 7380 if (code == FHIRDefinedType.ATTACHMENT) 7381 return "Attachment"; 7382 if (code == FHIRDefinedType.BACKBONEELEMENT) 7383 return "BackboneElement"; 7384 if (code == FHIRDefinedType.CODEABLECONCEPT) 7385 return "CodeableConcept"; 7386 if (code == FHIRDefinedType.CODING) 7387 return "Coding"; 7388 if (code == FHIRDefinedType.CONTACTDETAIL) 7389 return "ContactDetail"; 7390 if (code == FHIRDefinedType.CONTACTPOINT) 7391 return "ContactPoint"; 7392 if (code == FHIRDefinedType.CONTRIBUTOR) 7393 return "Contributor"; 7394 if (code == FHIRDefinedType.COUNT) 7395 return "Count"; 7396 if (code == FHIRDefinedType.DATAREQUIREMENT) 7397 return "DataRequirement"; 7398 if (code == FHIRDefinedType.DISTANCE) 7399 return "Distance"; 7400 if (code == FHIRDefinedType.DOSAGE) 7401 return "Dosage"; 7402 if (code == FHIRDefinedType.DURATION) 7403 return "Duration"; 7404 if (code == FHIRDefinedType.ELEMENT) 7405 return "Element"; 7406 if (code == FHIRDefinedType.ELEMENTDEFINITION) 7407 return "ElementDefinition"; 7408 if (code == FHIRDefinedType.EXTENSION) 7409 return "Extension"; 7410 if (code == FHIRDefinedType.HUMANNAME) 7411 return "HumanName"; 7412 if (code == FHIRDefinedType.IDENTIFIER) 7413 return "Identifier"; 7414 if (code == FHIRDefinedType.META) 7415 return "Meta"; 7416 if (code == FHIRDefinedType.MONEY) 7417 return "Money"; 7418 if (code == FHIRDefinedType.NARRATIVE) 7419 return "Narrative"; 7420 if (code == FHIRDefinedType.PARAMETERDEFINITION) 7421 return "ParameterDefinition"; 7422 if (code == FHIRDefinedType.PERIOD) 7423 return "Period"; 7424 if (code == FHIRDefinedType.QUANTITY) 7425 return "Quantity"; 7426 if (code == FHIRDefinedType.RANGE) 7427 return "Range"; 7428 if (code == FHIRDefinedType.RATIO) 7429 return "Ratio"; 7430 if (code == FHIRDefinedType.REFERENCE) 7431 return "Reference"; 7432 if (code == FHIRDefinedType.RELATEDARTIFACT) 7433 return "RelatedArtifact"; 7434 if (code == FHIRDefinedType.SAMPLEDDATA) 7435 return "SampledData"; 7436 if (code == FHIRDefinedType.SIGNATURE) 7437 return "Signature"; 7438 if (code == FHIRDefinedType.SIMPLEQUANTITY) 7439 return "SimpleQuantity"; 7440 if (code == FHIRDefinedType.TIMING) 7441 return "Timing"; 7442 if (code == FHIRDefinedType.TRIGGERDEFINITION) 7443 return "TriggerDefinition"; 7444 if (code == FHIRDefinedType.USAGECONTEXT) 7445 return "UsageContext"; 7446 if (code == FHIRDefinedType.BASE64BINARY) 7447 return "base64Binary"; 7448 if (code == FHIRDefinedType.BOOLEAN) 7449 return "boolean"; 7450 if (code == FHIRDefinedType.CODE) 7451 return "code"; 7452 if (code == FHIRDefinedType.DATE) 7453 return "date"; 7454 if (code == FHIRDefinedType.DATETIME) 7455 return "dateTime"; 7456 if (code == FHIRDefinedType.DECIMAL) 7457 return "decimal"; 7458 if (code == FHIRDefinedType.ID) 7459 return "id"; 7460 if (code == FHIRDefinedType.INSTANT) 7461 return "instant"; 7462 if (code == FHIRDefinedType.INTEGER) 7463 return "integer"; 7464 if (code == FHIRDefinedType.MARKDOWN) 7465 return "markdown"; 7466 if (code == FHIRDefinedType.OID) 7467 return "oid"; 7468 if (code == FHIRDefinedType.POSITIVEINT) 7469 return "positiveInt"; 7470 if (code == FHIRDefinedType.STRING) 7471 return "string"; 7472 if (code == FHIRDefinedType.TIME) 7473 return "time"; 7474 if (code == FHIRDefinedType.UNSIGNEDINT) 7475 return "unsignedInt"; 7476 if (code == FHIRDefinedType.URI) 7477 return "uri"; 7478 if (code == FHIRDefinedType.UUID) 7479 return "uuid"; 7480 if (code == FHIRDefinedType.XHTML) 7481 return "xhtml"; 7482 if (code == FHIRDefinedType.ACCOUNT) 7483 return "Account"; 7484 if (code == FHIRDefinedType.ACTIVITYDEFINITION) 7485 return "ActivityDefinition"; 7486 if (code == FHIRDefinedType.ADVERSEEVENT) 7487 return "AdverseEvent"; 7488 if (code == FHIRDefinedType.ALLERGYINTOLERANCE) 7489 return "AllergyIntolerance"; 7490 if (code == FHIRDefinedType.APPOINTMENT) 7491 return "Appointment"; 7492 if (code == FHIRDefinedType.APPOINTMENTRESPONSE) 7493 return "AppointmentResponse"; 7494 if (code == FHIRDefinedType.AUDITEVENT) 7495 return "AuditEvent"; 7496 if (code == FHIRDefinedType.BASIC) 7497 return "Basic"; 7498 if (code == FHIRDefinedType.BINARY) 7499 return "Binary"; 7500 if (code == FHIRDefinedType.BODYSITE) 7501 return "BodySite"; 7502 if (code == FHIRDefinedType.BUNDLE) 7503 return "Bundle"; 7504 if (code == FHIRDefinedType.CAPABILITYSTATEMENT) 7505 return "CapabilityStatement"; 7506 if (code == FHIRDefinedType.CAREPLAN) 7507 return "CarePlan"; 7508 if (code == FHIRDefinedType.CARETEAM) 7509 return "CareTeam"; 7510 if (code == FHIRDefinedType.CHARGEITEM) 7511 return "ChargeItem"; 7512 if (code == FHIRDefinedType.CLAIM) 7513 return "Claim"; 7514 if (code == FHIRDefinedType.CLAIMRESPONSE) 7515 return "ClaimResponse"; 7516 if (code == FHIRDefinedType.CLINICALIMPRESSION) 7517 return "ClinicalImpression"; 7518 if (code == FHIRDefinedType.CODESYSTEM) 7519 return "CodeSystem"; 7520 if (code == FHIRDefinedType.COMMUNICATION) 7521 return "Communication"; 7522 if (code == FHIRDefinedType.COMMUNICATIONREQUEST) 7523 return "CommunicationRequest"; 7524 if (code == FHIRDefinedType.COMPARTMENTDEFINITION) 7525 return "CompartmentDefinition"; 7526 if (code == FHIRDefinedType.COMPOSITION) 7527 return "Composition"; 7528 if (code == FHIRDefinedType.CONCEPTMAP) 7529 return "ConceptMap"; 7530 if (code == FHIRDefinedType.CONDITION) 7531 return "Condition"; 7532 if (code == FHIRDefinedType.CONSENT) 7533 return "Consent"; 7534 if (code == FHIRDefinedType.CONTRACT) 7535 return "Contract"; 7536 if (code == FHIRDefinedType.COVERAGE) 7537 return "Coverage"; 7538 if (code == FHIRDefinedType.DATAELEMENT) 7539 return "DataElement"; 7540 if (code == FHIRDefinedType.DETECTEDISSUE) 7541 return "DetectedIssue"; 7542 if (code == FHIRDefinedType.DEVICE) 7543 return "Device"; 7544 if (code == FHIRDefinedType.DEVICECOMPONENT) 7545 return "DeviceComponent"; 7546 if (code == FHIRDefinedType.DEVICEMETRIC) 7547 return "DeviceMetric"; 7548 if (code == FHIRDefinedType.DEVICEREQUEST) 7549 return "DeviceRequest"; 7550 if (code == FHIRDefinedType.DEVICEUSESTATEMENT) 7551 return "DeviceUseStatement"; 7552 if (code == FHIRDefinedType.DIAGNOSTICREPORT) 7553 return "DiagnosticReport"; 7554 if (code == FHIRDefinedType.DOCUMENTMANIFEST) 7555 return "DocumentManifest"; 7556 if (code == FHIRDefinedType.DOCUMENTREFERENCE) 7557 return "DocumentReference"; 7558 if (code == FHIRDefinedType.DOMAINRESOURCE) 7559 return "DomainResource"; 7560 if (code == FHIRDefinedType.ELIGIBILITYREQUEST) 7561 return "EligibilityRequest"; 7562 if (code == FHIRDefinedType.ELIGIBILITYRESPONSE) 7563 return "EligibilityResponse"; 7564 if (code == FHIRDefinedType.ENCOUNTER) 7565 return "Encounter"; 7566 if (code == FHIRDefinedType.ENDPOINT) 7567 return "Endpoint"; 7568 if (code == FHIRDefinedType.ENROLLMENTREQUEST) 7569 return "EnrollmentRequest"; 7570 if (code == FHIRDefinedType.ENROLLMENTRESPONSE) 7571 return "EnrollmentResponse"; 7572 if (code == FHIRDefinedType.EPISODEOFCARE) 7573 return "EpisodeOfCare"; 7574 if (code == FHIRDefinedType.EXPANSIONPROFILE) 7575 return "ExpansionProfile"; 7576 if (code == FHIRDefinedType.EXPLANATIONOFBENEFIT) 7577 return "ExplanationOfBenefit"; 7578 if (code == FHIRDefinedType.FAMILYMEMBERHISTORY) 7579 return "FamilyMemberHistory"; 7580 if (code == FHIRDefinedType.FLAG) 7581 return "Flag"; 7582 if (code == FHIRDefinedType.GOAL) 7583 return "Goal"; 7584 if (code == FHIRDefinedType.GRAPHDEFINITION) 7585 return "GraphDefinition"; 7586 if (code == FHIRDefinedType.GROUP) 7587 return "Group"; 7588 if (code == FHIRDefinedType.GUIDANCERESPONSE) 7589 return "GuidanceResponse"; 7590 if (code == FHIRDefinedType.HEALTHCARESERVICE) 7591 return "HealthcareService"; 7592 if (code == FHIRDefinedType.IMAGINGMANIFEST) 7593 return "ImagingManifest"; 7594 if (code == FHIRDefinedType.IMAGINGSTUDY) 7595 return "ImagingStudy"; 7596 if (code == FHIRDefinedType.IMMUNIZATION) 7597 return "Immunization"; 7598 if (code == FHIRDefinedType.IMMUNIZATIONRECOMMENDATION) 7599 return "ImmunizationRecommendation"; 7600 if (code == FHIRDefinedType.IMPLEMENTATIONGUIDE) 7601 return "ImplementationGuide"; 7602 if (code == FHIRDefinedType.LIBRARY) 7603 return "Library"; 7604 if (code == FHIRDefinedType.LINKAGE) 7605 return "Linkage"; 7606 if (code == FHIRDefinedType.LIST) 7607 return "List"; 7608 if (code == FHIRDefinedType.LOCATION) 7609 return "Location"; 7610 if (code == FHIRDefinedType.MEASURE) 7611 return "Measure"; 7612 if (code == FHIRDefinedType.MEASUREREPORT) 7613 return "MeasureReport"; 7614 if (code == FHIRDefinedType.MEDIA) 7615 return "Media"; 7616 if (code == FHIRDefinedType.MEDICATION) 7617 return "Medication"; 7618 if (code == FHIRDefinedType.MEDICATIONADMINISTRATION) 7619 return "MedicationAdministration"; 7620 if (code == FHIRDefinedType.MEDICATIONDISPENSE) 7621 return "MedicationDispense"; 7622 if (code == FHIRDefinedType.MEDICATIONREQUEST) 7623 return "MedicationRequest"; 7624 if (code == FHIRDefinedType.MEDICATIONSTATEMENT) 7625 return "MedicationStatement"; 7626 if (code == FHIRDefinedType.MESSAGEDEFINITION) 7627 return "MessageDefinition"; 7628 if (code == FHIRDefinedType.MESSAGEHEADER) 7629 return "MessageHeader"; 7630 if (code == FHIRDefinedType.NAMINGSYSTEM) 7631 return "NamingSystem"; 7632 if (code == FHIRDefinedType.NUTRITIONORDER) 7633 return "NutritionOrder"; 7634 if (code == FHIRDefinedType.OBSERVATION) 7635 return "Observation"; 7636 if (code == FHIRDefinedType.OPERATIONDEFINITION) 7637 return "OperationDefinition"; 7638 if (code == FHIRDefinedType.OPERATIONOUTCOME) 7639 return "OperationOutcome"; 7640 if (code == FHIRDefinedType.ORGANIZATION) 7641 return "Organization"; 7642 if (code == FHIRDefinedType.PARAMETERS) 7643 return "Parameters"; 7644 if (code == FHIRDefinedType.PATIENT) 7645 return "Patient"; 7646 if (code == FHIRDefinedType.PAYMENTNOTICE) 7647 return "PaymentNotice"; 7648 if (code == FHIRDefinedType.PAYMENTRECONCILIATION) 7649 return "PaymentReconciliation"; 7650 if (code == FHIRDefinedType.PERSON) 7651 return "Person"; 7652 if (code == FHIRDefinedType.PLANDEFINITION) 7653 return "PlanDefinition"; 7654 if (code == FHIRDefinedType.PRACTITIONER) 7655 return "Practitioner"; 7656 if (code == FHIRDefinedType.PRACTITIONERROLE) 7657 return "PractitionerRole"; 7658 if (code == FHIRDefinedType.PROCEDURE) 7659 return "Procedure"; 7660 if (code == FHIRDefinedType.PROCEDUREREQUEST) 7661 return "ProcedureRequest"; 7662 if (code == FHIRDefinedType.PROCESSREQUEST) 7663 return "ProcessRequest"; 7664 if (code == FHIRDefinedType.PROCESSRESPONSE) 7665 return "ProcessResponse"; 7666 if (code == FHIRDefinedType.PROVENANCE) 7667 return "Provenance"; 7668 if (code == FHIRDefinedType.QUESTIONNAIRE) 7669 return "Questionnaire"; 7670 if (code == FHIRDefinedType.QUESTIONNAIRERESPONSE) 7671 return "QuestionnaireResponse"; 7672 if (code == FHIRDefinedType.REFERRALREQUEST) 7673 return "ReferralRequest"; 7674 if (code == FHIRDefinedType.RELATEDPERSON) 7675 return "RelatedPerson"; 7676 if (code == FHIRDefinedType.REQUESTGROUP) 7677 return "RequestGroup"; 7678 if (code == FHIRDefinedType.RESEARCHSTUDY) 7679 return "ResearchStudy"; 7680 if (code == FHIRDefinedType.RESEARCHSUBJECT) 7681 return "ResearchSubject"; 7682 if (code == FHIRDefinedType.RESOURCE) 7683 return "Resource"; 7684 if (code == FHIRDefinedType.RISKASSESSMENT) 7685 return "RiskAssessment"; 7686 if (code == FHIRDefinedType.SCHEDULE) 7687 return "Schedule"; 7688 if (code == FHIRDefinedType.SEARCHPARAMETER) 7689 return "SearchParameter"; 7690 if (code == FHIRDefinedType.SEQUENCE) 7691 return "Sequence"; 7692 if (code == FHIRDefinedType.SERVICEDEFINITION) 7693 return "ServiceDefinition"; 7694 if (code == FHIRDefinedType.SLOT) 7695 return "Slot"; 7696 if (code == FHIRDefinedType.SPECIMEN) 7697 return "Specimen"; 7698 if (code == FHIRDefinedType.STRUCTUREDEFINITION) 7699 return "StructureDefinition"; 7700 if (code == FHIRDefinedType.STRUCTUREMAP) 7701 return "StructureMap"; 7702 if (code == FHIRDefinedType.SUBSCRIPTION) 7703 return "Subscription"; 7704 if (code == FHIRDefinedType.SUBSTANCE) 7705 return "Substance"; 7706 if (code == FHIRDefinedType.SUPPLYDELIVERY) 7707 return "SupplyDelivery"; 7708 if (code == FHIRDefinedType.SUPPLYREQUEST) 7709 return "SupplyRequest"; 7710 if (code == FHIRDefinedType.TASK) 7711 return "Task"; 7712 if (code == FHIRDefinedType.TESTREPORT) 7713 return "TestReport"; 7714 if (code == FHIRDefinedType.TESTSCRIPT) 7715 return "TestScript"; 7716 if (code == FHIRDefinedType.VALUESET) 7717 return "ValueSet"; 7718 if (code == FHIRDefinedType.VISIONPRESCRIPTION) 7719 return "VisionPrescription"; 7720 return "?"; 7721 } 7722 public String toSystem(FHIRDefinedType code) { 7723 return code.getSystem(); 7724 } 7725 } 7726 7727 public enum MessageEvent { 7728 /** 7729 * The definition of a code system is used to create a simple collection of codes suitable for use for data entry or validation. An expanded code system will be returned, or an error message. 7730 */ 7731 CODESYSTEMEXPAND, 7732 /** 7733 * Change the status of a Medication Administration to show that it is complete. 7734 */ 7735 MEDICATIONADMINISTRATIONCOMPLETE, 7736 /** 7737 * Someone wishes to record that the record of administration of a medication is in error and should be ignored. 7738 */ 7739 MEDICATIONADMINISTRATIONNULLIFICATION, 7740 /** 7741 * Indicates that a medication has been recorded against the patient's record. 7742 */ 7743 MEDICATIONADMINISTRATIONRECORDING, 7744 /** 7745 * Update a Medication Administration record. 7746 */ 7747 MEDICATIONADMINISTRATIONUPDATE, 7748 /** 7749 * Notification of a change to an administrative resource (either create or update). Note that there is no delete, though some administrative resources have status or period elements for this use. 7750 */ 7751 ADMINNOTIFY, 7752 /** 7753 * Notification to convey information. 7754 */ 7755 COMMUNICATIONREQUEST, 7756 /** 7757 * Provide a diagnostic report, or update a previously provided diagnostic report. 7758 */ 7759 DIAGNOSTICREPORTPROVIDE, 7760 /** 7761 * Provide a simple observation or update a previously provided simple observation. 7762 */ 7763 OBSERVATIONPROVIDE, 7764 /** 7765 * Notification that two patient records actually identify the same patient. 7766 */ 7767 PATIENTLINK, 7768 /** 7769 * Notification that previous advice that two patient records concern the same patient is now considered incorrect. 7770 */ 7771 PATIENTUNLINK, 7772 /** 7773 * The definition of a value set is used to create a simple collection of codes suitable for use for data entry or validation. An expanded value set will be returned, or an error message. 7774 */ 7775 VALUESETEXPAND, 7776 /** 7777 * added to help the parsers 7778 */ 7779 NULL; 7780 public static MessageEvent fromCode(String codeString) throws FHIRException { 7781 if (codeString == null || "".equals(codeString)) 7782 return null; 7783 if ("CodeSystem-expand".equals(codeString)) 7784 return CODESYSTEMEXPAND; 7785 if ("MedicationAdministration-Complete".equals(codeString)) 7786 return MEDICATIONADMINISTRATIONCOMPLETE; 7787 if ("MedicationAdministration-Nullification".equals(codeString)) 7788 return MEDICATIONADMINISTRATIONNULLIFICATION; 7789 if ("MedicationAdministration-Recording".equals(codeString)) 7790 return MEDICATIONADMINISTRATIONRECORDING; 7791 if ("MedicationAdministration-Update".equals(codeString)) 7792 return MEDICATIONADMINISTRATIONUPDATE; 7793 if ("admin-notify".equals(codeString)) 7794 return ADMINNOTIFY; 7795 if ("communication-request".equals(codeString)) 7796 return COMMUNICATIONREQUEST; 7797 if ("diagnosticreport-provide".equals(codeString)) 7798 return DIAGNOSTICREPORTPROVIDE; 7799 if ("observation-provide".equals(codeString)) 7800 return OBSERVATIONPROVIDE; 7801 if ("patient-link".equals(codeString)) 7802 return PATIENTLINK; 7803 if ("patient-unlink".equals(codeString)) 7804 return PATIENTUNLINK; 7805 if ("valueset-expand".equals(codeString)) 7806 return VALUESETEXPAND; 7807 throw new FHIRException("Unknown MessageEvent code '"+codeString+"'"); 7808 } 7809 public String toCode() { 7810 switch (this) { 7811 case CODESYSTEMEXPAND: return "CodeSystem-expand"; 7812 case MEDICATIONADMINISTRATIONCOMPLETE: return "MedicationAdministration-Complete"; 7813 case MEDICATIONADMINISTRATIONNULLIFICATION: return "MedicationAdministration-Nullification"; 7814 case MEDICATIONADMINISTRATIONRECORDING: return "MedicationAdministration-Recording"; 7815 case MEDICATIONADMINISTRATIONUPDATE: return "MedicationAdministration-Update"; 7816 case ADMINNOTIFY: return "admin-notify"; 7817 case COMMUNICATIONREQUEST: return "communication-request"; 7818 case DIAGNOSTICREPORTPROVIDE: return "diagnosticreport-provide"; 7819 case OBSERVATIONPROVIDE: return "observation-provide"; 7820 case PATIENTLINK: return "patient-link"; 7821 case PATIENTUNLINK: return "patient-unlink"; 7822 case VALUESETEXPAND: return "valueset-expand"; 7823 case NULL: return null; 7824 default: return "?"; 7825 } 7826 } 7827 public String getSystem() { 7828 switch (this) { 7829 case CODESYSTEMEXPAND: return "http://hl7.org/fhir/message-events"; 7830 case MEDICATIONADMINISTRATIONCOMPLETE: return "http://hl7.org/fhir/message-events"; 7831 case MEDICATIONADMINISTRATIONNULLIFICATION: return "http://hl7.org/fhir/message-events"; 7832 case MEDICATIONADMINISTRATIONRECORDING: return "http://hl7.org/fhir/message-events"; 7833 case MEDICATIONADMINISTRATIONUPDATE: return "http://hl7.org/fhir/message-events"; 7834 case ADMINNOTIFY: return "http://hl7.org/fhir/message-events"; 7835 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/message-events"; 7836 case DIAGNOSTICREPORTPROVIDE: return "http://hl7.org/fhir/message-events"; 7837 case OBSERVATIONPROVIDE: return "http://hl7.org/fhir/message-events"; 7838 case PATIENTLINK: return "http://hl7.org/fhir/message-events"; 7839 case PATIENTUNLINK: return "http://hl7.org/fhir/message-events"; 7840 case VALUESETEXPAND: return "http://hl7.org/fhir/message-events"; 7841 case NULL: return null; 7842 default: return "?"; 7843 } 7844 } 7845 public String getDefinition() { 7846 switch (this) { 7847 case CODESYSTEMEXPAND: return "The definition of a code system is used to create a simple collection of codes suitable for use for data entry or validation. An expanded code system will be returned, or an error message."; 7848 case MEDICATIONADMINISTRATIONCOMPLETE: return "Change the status of a Medication Administration to show that it is complete."; 7849 case MEDICATIONADMINISTRATIONNULLIFICATION: return "Someone wishes to record that the record of administration of a medication is in error and should be ignored."; 7850 case MEDICATIONADMINISTRATIONRECORDING: return "Indicates that a medication has been recorded against the patient's record."; 7851 case MEDICATIONADMINISTRATIONUPDATE: return "Update a Medication Administration record."; 7852 case ADMINNOTIFY: return "Notification of a change to an administrative resource (either create or update). Note that there is no delete, though some administrative resources have status or period elements for this use."; 7853 case COMMUNICATIONREQUEST: return "Notification to convey information."; 7854 case DIAGNOSTICREPORTPROVIDE: return "Provide a diagnostic report, or update a previously provided diagnostic report."; 7855 case OBSERVATIONPROVIDE: return "Provide a simple observation or update a previously provided simple observation."; 7856 case PATIENTLINK: return "Notification that two patient records actually identify the same patient."; 7857 case PATIENTUNLINK: return "Notification that previous advice that two patient records concern the same patient is now considered incorrect."; 7858 case VALUESETEXPAND: return "The definition of a value set is used to create a simple collection of codes suitable for use for data entry or validation. An expanded value set will be returned, or an error message."; 7859 case NULL: return null; 7860 default: return "?"; 7861 } 7862 } 7863 public String getDisplay() { 7864 switch (this) { 7865 case CODESYSTEMEXPAND: return "CodeSystem-expand"; 7866 case MEDICATIONADMINISTRATIONCOMPLETE: return "MedicationAdministration-Complete"; 7867 case MEDICATIONADMINISTRATIONNULLIFICATION: return "MedicationAdministration-Nullification"; 7868 case MEDICATIONADMINISTRATIONRECORDING: return "MedicationAdministration-Recording"; 7869 case MEDICATIONADMINISTRATIONUPDATE: return "MedicationAdministration-Update"; 7870 case ADMINNOTIFY: return "admin-notify"; 7871 case COMMUNICATIONREQUEST: return "communication-request"; 7872 case DIAGNOSTICREPORTPROVIDE: return "diagnosticreport-provide"; 7873 case OBSERVATIONPROVIDE: return "observation-provide"; 7874 case PATIENTLINK: return "patient-link"; 7875 case PATIENTUNLINK: return "patient-unlink"; 7876 case VALUESETEXPAND: return "valueset-expand"; 7877 case NULL: return null; 7878 default: return "?"; 7879 } 7880 } 7881 } 7882 7883 public static class MessageEventEnumFactory implements EnumFactory<MessageEvent> { 7884 public MessageEvent fromCode(String codeString) throws IllegalArgumentException { 7885 if (codeString == null || "".equals(codeString)) 7886 if (codeString == null || "".equals(codeString)) 7887 return null; 7888 if ("CodeSystem-expand".equals(codeString)) 7889 return MessageEvent.CODESYSTEMEXPAND; 7890 if ("MedicationAdministration-Complete".equals(codeString)) 7891 return MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE; 7892 if ("MedicationAdministration-Nullification".equals(codeString)) 7893 return MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION; 7894 if ("MedicationAdministration-Recording".equals(codeString)) 7895 return MessageEvent.MEDICATIONADMINISTRATIONRECORDING; 7896 if ("MedicationAdministration-Update".equals(codeString)) 7897 return MessageEvent.MEDICATIONADMINISTRATIONUPDATE; 7898 if ("admin-notify".equals(codeString)) 7899 return MessageEvent.ADMINNOTIFY; 7900 if ("communication-request".equals(codeString)) 7901 return MessageEvent.COMMUNICATIONREQUEST; 7902 if ("diagnosticreport-provide".equals(codeString)) 7903 return MessageEvent.DIAGNOSTICREPORTPROVIDE; 7904 if ("observation-provide".equals(codeString)) 7905 return MessageEvent.OBSERVATIONPROVIDE; 7906 if ("patient-link".equals(codeString)) 7907 return MessageEvent.PATIENTLINK; 7908 if ("patient-unlink".equals(codeString)) 7909 return MessageEvent.PATIENTUNLINK; 7910 if ("valueset-expand".equals(codeString)) 7911 return MessageEvent.VALUESETEXPAND; 7912 throw new IllegalArgumentException("Unknown MessageEvent code '"+codeString+"'"); 7913 } 7914 public Enumeration<MessageEvent> fromType(PrimitiveType<?> code) throws FHIRException { 7915 if (code == null) 7916 return null; 7917 if (code.isEmpty()) 7918 return new Enumeration<MessageEvent>(this); 7919 String codeString = code.asStringValue(); 7920 if (codeString == null || "".equals(codeString)) 7921 return null; 7922 if ("CodeSystem-expand".equals(codeString)) 7923 return new Enumeration<MessageEvent>(this, MessageEvent.CODESYSTEMEXPAND); 7924 if ("MedicationAdministration-Complete".equals(codeString)) 7925 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE); 7926 if ("MedicationAdministration-Nullification".equals(codeString)) 7927 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION); 7928 if ("MedicationAdministration-Recording".equals(codeString)) 7929 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONRECORDING); 7930 if ("MedicationAdministration-Update".equals(codeString)) 7931 return new Enumeration<MessageEvent>(this, MessageEvent.MEDICATIONADMINISTRATIONUPDATE); 7932 if ("admin-notify".equals(codeString)) 7933 return new Enumeration<MessageEvent>(this, MessageEvent.ADMINNOTIFY); 7934 if ("communication-request".equals(codeString)) 7935 return new Enumeration<MessageEvent>(this, MessageEvent.COMMUNICATIONREQUEST); 7936 if ("diagnosticreport-provide".equals(codeString)) 7937 return new Enumeration<MessageEvent>(this, MessageEvent.DIAGNOSTICREPORTPROVIDE); 7938 if ("observation-provide".equals(codeString)) 7939 return new Enumeration<MessageEvent>(this, MessageEvent.OBSERVATIONPROVIDE); 7940 if ("patient-link".equals(codeString)) 7941 return new Enumeration<MessageEvent>(this, MessageEvent.PATIENTLINK); 7942 if ("patient-unlink".equals(codeString)) 7943 return new Enumeration<MessageEvent>(this, MessageEvent.PATIENTUNLINK); 7944 if ("valueset-expand".equals(codeString)) 7945 return new Enumeration<MessageEvent>(this, MessageEvent.VALUESETEXPAND); 7946 throw new FHIRException("Unknown MessageEvent code '"+codeString+"'"); 7947 } 7948 public String toCode(MessageEvent code) { 7949 if (code == MessageEvent.CODESYSTEMEXPAND) 7950 return "CodeSystem-expand"; 7951 if (code == MessageEvent.MEDICATIONADMINISTRATIONCOMPLETE) 7952 return "MedicationAdministration-Complete"; 7953 if (code == MessageEvent.MEDICATIONADMINISTRATIONNULLIFICATION) 7954 return "MedicationAdministration-Nullification"; 7955 if (code == MessageEvent.MEDICATIONADMINISTRATIONRECORDING) 7956 return "MedicationAdministration-Recording"; 7957 if (code == MessageEvent.MEDICATIONADMINISTRATIONUPDATE) 7958 return "MedicationAdministration-Update"; 7959 if (code == MessageEvent.ADMINNOTIFY) 7960 return "admin-notify"; 7961 if (code == MessageEvent.COMMUNICATIONREQUEST) 7962 return "communication-request"; 7963 if (code == MessageEvent.DIAGNOSTICREPORTPROVIDE) 7964 return "diagnosticreport-provide"; 7965 if (code == MessageEvent.OBSERVATIONPROVIDE) 7966 return "observation-provide"; 7967 if (code == MessageEvent.PATIENTLINK) 7968 return "patient-link"; 7969 if (code == MessageEvent.PATIENTUNLINK) 7970 return "patient-unlink"; 7971 if (code == MessageEvent.VALUESETEXPAND) 7972 return "valueset-expand"; 7973 return "?"; 7974 } 7975 public String toSystem(MessageEvent code) { 7976 return code.getSystem(); 7977 } 7978 } 7979 7980 public enum NoteType { 7981 /** 7982 * Display the note. 7983 */ 7984 DISPLAY, 7985 /** 7986 * Print the note on the form. 7987 */ 7988 PRINT, 7989 /** 7990 * Print the note for the operator. 7991 */ 7992 PRINTOPER, 7993 /** 7994 * added to help the parsers 7995 */ 7996 NULL; 7997 public static NoteType fromCode(String codeString) throws FHIRException { 7998 if (codeString == null || "".equals(codeString)) 7999 return null; 8000 if ("display".equals(codeString)) 8001 return DISPLAY; 8002 if ("print".equals(codeString)) 8003 return PRINT; 8004 if ("printoper".equals(codeString)) 8005 return PRINTOPER; 8006 throw new FHIRException("Unknown NoteType code '"+codeString+"'"); 8007 } 8008 public String toCode() { 8009 switch (this) { 8010 case DISPLAY: return "display"; 8011 case PRINT: return "print"; 8012 case PRINTOPER: return "printoper"; 8013 case NULL: return null; 8014 default: return "?"; 8015 } 8016 } 8017 public String getSystem() { 8018 switch (this) { 8019 case DISPLAY: return "http://hl7.org/fhir/note-type"; 8020 case PRINT: return "http://hl7.org/fhir/note-type"; 8021 case PRINTOPER: return "http://hl7.org/fhir/note-type"; 8022 case NULL: return null; 8023 default: return "?"; 8024 } 8025 } 8026 public String getDefinition() { 8027 switch (this) { 8028 case DISPLAY: return "Display the note."; 8029 case PRINT: return "Print the note on the form."; 8030 case PRINTOPER: return "Print the note for the operator."; 8031 case NULL: return null; 8032 default: return "?"; 8033 } 8034 } 8035 public String getDisplay() { 8036 switch (this) { 8037 case DISPLAY: return "Display"; 8038 case PRINT: return "Print (Form)"; 8039 case PRINTOPER: return "Print (Operator)"; 8040 case NULL: return null; 8041 default: return "?"; 8042 } 8043 } 8044 } 8045 8046 public static class NoteTypeEnumFactory implements EnumFactory<NoteType> { 8047 public NoteType fromCode(String codeString) throws IllegalArgumentException { 8048 if (codeString == null || "".equals(codeString)) 8049 if (codeString == null || "".equals(codeString)) 8050 return null; 8051 if ("display".equals(codeString)) 8052 return NoteType.DISPLAY; 8053 if ("print".equals(codeString)) 8054 return NoteType.PRINT; 8055 if ("printoper".equals(codeString)) 8056 return NoteType.PRINTOPER; 8057 throw new IllegalArgumentException("Unknown NoteType code '"+codeString+"'"); 8058 } 8059 public Enumeration<NoteType> fromType(PrimitiveType<?> code) throws FHIRException { 8060 if (code == null) 8061 return null; 8062 if (code.isEmpty()) 8063 return new Enumeration<NoteType>(this); 8064 String codeString = code.asStringValue(); 8065 if (codeString == null || "".equals(codeString)) 8066 return null; 8067 if ("display".equals(codeString)) 8068 return new Enumeration<NoteType>(this, NoteType.DISPLAY); 8069 if ("print".equals(codeString)) 8070 return new Enumeration<NoteType>(this, NoteType.PRINT); 8071 if ("printoper".equals(codeString)) 8072 return new Enumeration<NoteType>(this, NoteType.PRINTOPER); 8073 throw new FHIRException("Unknown NoteType code '"+codeString+"'"); 8074 } 8075 public String toCode(NoteType code) { 8076 if (code == NoteType.DISPLAY) 8077 return "display"; 8078 if (code == NoteType.PRINT) 8079 return "print"; 8080 if (code == NoteType.PRINTOPER) 8081 return "printoper"; 8082 return "?"; 8083 } 8084 public String toSystem(NoteType code) { 8085 return code.getSystem(); 8086 } 8087 } 8088 8089 public enum PublicationStatus { 8090 /** 8091 * This resource is still under development and is not yet considered to be ready for normal use. 8092 */ 8093 DRAFT, 8094 /** 8095 * This resource is ready for normal use. 8096 */ 8097 ACTIVE, 8098 /** 8099 * This resource has been withdrawn or superseded and should no longer be used. 8100 */ 8101 RETIRED, 8102 /** 8103 * The authoring system does not know which of the status values currently applies for this resource. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 8104 */ 8105 UNKNOWN, 8106 /** 8107 * added to help the parsers 8108 */ 8109 NULL; 8110 public static PublicationStatus fromCode(String codeString) throws FHIRException { 8111 if (codeString == null || "".equals(codeString)) 8112 return null; 8113 if ("draft".equals(codeString)) 8114 return DRAFT; 8115 if ("active".equals(codeString)) 8116 return ACTIVE; 8117 if ("retired".equals(codeString)) 8118 return RETIRED; 8119 if ("unknown".equals(codeString)) 8120 return UNKNOWN; 8121 throw new FHIRException("Unknown PublicationStatus code '"+codeString+"'"); 8122 } 8123 public String toCode() { 8124 switch (this) { 8125 case DRAFT: return "draft"; 8126 case ACTIVE: return "active"; 8127 case RETIRED: return "retired"; 8128 case UNKNOWN: return "unknown"; 8129 case NULL: return null; 8130 default: return "?"; 8131 } 8132 } 8133 public String getSystem() { 8134 switch (this) { 8135 case DRAFT: return "http://hl7.org/fhir/publication-status"; 8136 case ACTIVE: return "http://hl7.org/fhir/publication-status"; 8137 case RETIRED: return "http://hl7.org/fhir/publication-status"; 8138 case UNKNOWN: return "http://hl7.org/fhir/publication-status"; 8139 case NULL: return null; 8140 default: return "?"; 8141 } 8142 } 8143 public String getDefinition() { 8144 switch (this) { 8145 case DRAFT: return "This resource is still under development and is not yet considered to be ready for normal use."; 8146 case ACTIVE: return "This resource is ready for normal use."; 8147 case RETIRED: return "This resource has been withdrawn or superseded and should no longer be used."; 8148 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this resource. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 8149 case NULL: return null; 8150 default: return "?"; 8151 } 8152 } 8153 public String getDisplay() { 8154 switch (this) { 8155 case DRAFT: return "Draft"; 8156 case ACTIVE: return "Active"; 8157 case RETIRED: return "Retired"; 8158 case UNKNOWN: return "Unknown"; 8159 case NULL: return null; 8160 default: return "?"; 8161 } 8162 } 8163 } 8164 8165 public static class PublicationStatusEnumFactory implements EnumFactory<PublicationStatus> { 8166 public PublicationStatus fromCode(String codeString) throws IllegalArgumentException { 8167 if (codeString == null || "".equals(codeString)) 8168 if (codeString == null || "".equals(codeString)) 8169 return null; 8170 if ("draft".equals(codeString)) 8171 return PublicationStatus.DRAFT; 8172 if ("active".equals(codeString)) 8173 return PublicationStatus.ACTIVE; 8174 if ("retired".equals(codeString)) 8175 return PublicationStatus.RETIRED; 8176 if ("unknown".equals(codeString)) 8177 return PublicationStatus.UNKNOWN; 8178 throw new IllegalArgumentException("Unknown PublicationStatus code '"+codeString+"'"); 8179 } 8180 public Enumeration<PublicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 8181 if (code == null) 8182 return null; 8183 if (code.isEmpty()) 8184 return new Enumeration<PublicationStatus>(this); 8185 String codeString = code.asStringValue(); 8186 if (codeString == null || "".equals(codeString)) 8187 return null; 8188 if ("draft".equals(codeString)) 8189 return new Enumeration<PublicationStatus>(this, PublicationStatus.DRAFT); 8190 if ("active".equals(codeString)) 8191 return new Enumeration<PublicationStatus>(this, PublicationStatus.ACTIVE); 8192 if ("retired".equals(codeString)) 8193 return new Enumeration<PublicationStatus>(this, PublicationStatus.RETIRED); 8194 if ("unknown".equals(codeString)) 8195 return new Enumeration<PublicationStatus>(this, PublicationStatus.UNKNOWN); 8196 throw new FHIRException("Unknown PublicationStatus code '"+codeString+"'"); 8197 } 8198 public String toCode(PublicationStatus code) { 8199 if (code == PublicationStatus.DRAFT) 8200 return "draft"; 8201 if (code == PublicationStatus.ACTIVE) 8202 return "active"; 8203 if (code == PublicationStatus.RETIRED) 8204 return "retired"; 8205 if (code == PublicationStatus.UNKNOWN) 8206 return "unknown"; 8207 return "?"; 8208 } 8209 public String toSystem(PublicationStatus code) { 8210 return code.getSystem(); 8211 } 8212 } 8213 8214 public enum RemittanceOutcome { 8215 /** 8216 * The processing has completed without errors 8217 */ 8218 COMPLETE, 8219 /** 8220 * One or more errors have been detected in the Claim 8221 */ 8222 ERROR, 8223 /** 8224 * No errors have been detected in the Claim and some of the adjudication has been performed. 8225 */ 8226 PARTIAL, 8227 /** 8228 * added to help the parsers 8229 */ 8230 NULL; 8231 public static RemittanceOutcome fromCode(String codeString) throws FHIRException { 8232 if (codeString == null || "".equals(codeString)) 8233 return null; 8234 if ("complete".equals(codeString)) 8235 return COMPLETE; 8236 if ("error".equals(codeString)) 8237 return ERROR; 8238 if ("partial".equals(codeString)) 8239 return PARTIAL; 8240 throw new FHIRException("Unknown RemittanceOutcome code '"+codeString+"'"); 8241 } 8242 public String toCode() { 8243 switch (this) { 8244 case COMPLETE: return "complete"; 8245 case ERROR: return "error"; 8246 case PARTIAL: return "partial"; 8247 case NULL: return null; 8248 default: return "?"; 8249 } 8250 } 8251 public String getSystem() { 8252 switch (this) { 8253 case COMPLETE: return "http://hl7.org/fhir/remittance-outcome"; 8254 case ERROR: return "http://hl7.org/fhir/remittance-outcome"; 8255 case PARTIAL: return "http://hl7.org/fhir/remittance-outcome"; 8256 case NULL: return null; 8257 default: return "?"; 8258 } 8259 } 8260 public String getDefinition() { 8261 switch (this) { 8262 case COMPLETE: return "The processing has completed without errors"; 8263 case ERROR: return "One or more errors have been detected in the Claim"; 8264 case PARTIAL: return "No errors have been detected in the Claim and some of the adjudication has been performed."; 8265 case NULL: return null; 8266 default: return "?"; 8267 } 8268 } 8269 public String getDisplay() { 8270 switch (this) { 8271 case COMPLETE: return "Processing Complete"; 8272 case ERROR: return "Error"; 8273 case PARTIAL: return "Partial Processing"; 8274 case NULL: return null; 8275 default: return "?"; 8276 } 8277 } 8278 } 8279 8280 public static class RemittanceOutcomeEnumFactory implements EnumFactory<RemittanceOutcome> { 8281 public RemittanceOutcome fromCode(String codeString) throws IllegalArgumentException { 8282 if (codeString == null || "".equals(codeString)) 8283 if (codeString == null || "".equals(codeString)) 8284 return null; 8285 if ("complete".equals(codeString)) 8286 return RemittanceOutcome.COMPLETE; 8287 if ("error".equals(codeString)) 8288 return RemittanceOutcome.ERROR; 8289 if ("partial".equals(codeString)) 8290 return RemittanceOutcome.PARTIAL; 8291 throw new IllegalArgumentException("Unknown RemittanceOutcome code '"+codeString+"'"); 8292 } 8293 public Enumeration<RemittanceOutcome> fromType(PrimitiveType<?> code) throws FHIRException { 8294 if (code == null) 8295 return null; 8296 if (code.isEmpty()) 8297 return new Enumeration<RemittanceOutcome>(this); 8298 String codeString = code.asStringValue(); 8299 if (codeString == null || "".equals(codeString)) 8300 return null; 8301 if ("complete".equals(codeString)) 8302 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.COMPLETE); 8303 if ("error".equals(codeString)) 8304 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.ERROR); 8305 if ("partial".equals(codeString)) 8306 return new Enumeration<RemittanceOutcome>(this, RemittanceOutcome.PARTIAL); 8307 throw new FHIRException("Unknown RemittanceOutcome code '"+codeString+"'"); 8308 } 8309 public String toCode(RemittanceOutcome code) { 8310 if (code == RemittanceOutcome.COMPLETE) 8311 return "complete"; 8312 if (code == RemittanceOutcome.ERROR) 8313 return "error"; 8314 if (code == RemittanceOutcome.PARTIAL) 8315 return "partial"; 8316 return "?"; 8317 } 8318 public String toSystem(RemittanceOutcome code) { 8319 return code.getSystem(); 8320 } 8321 } 8322 8323 public enum ResourceType { 8324 /** 8325 * A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc. 8326 */ 8327 ACCOUNT, 8328 /** 8329 * This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context. 8330 */ 8331 ACTIVITYDEFINITION, 8332 /** 8333 * Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death. 8334 */ 8335 ADVERSEEVENT, 8336 /** 8337 * Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance. 8338 */ 8339 ALLERGYINTOLERANCE, 8340 /** 8341 * A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s). 8342 */ 8343 APPOINTMENT, 8344 /** 8345 * A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection. 8346 */ 8347 APPOINTMENTRESPONSE, 8348 /** 8349 * A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage. 8350 */ 8351 AUDITEVENT, 8352 /** 8353 * Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification. 8354 */ 8355 BASIC, 8356 /** 8357 * A binary resource can contain any content, whether text, image, pdf, zip archive, etc. 8358 */ 8359 BINARY, 8360 /** 8361 * Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case. 8362 */ 8363 BODYSITE, 8364 /** 8365 * A container for a collection of resources. 8366 */ 8367 BUNDLE, 8368 /** 8369 * A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation. 8370 */ 8371 CAPABILITYSTATEMENT, 8372 /** 8373 * Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions. 8374 */ 8375 CAREPLAN, 8376 /** 8377 * The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient. 8378 */ 8379 CARETEAM, 8380 /** 8381 * The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation. 8382 */ 8383 CHARGEITEM, 8384 /** 8385 * A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery. 8386 */ 8387 CLAIM, 8388 /** 8389 * This resource provides the adjudication details from the processing of a Claim resource. 8390 */ 8391 CLAIMRESPONSE, 8392 /** 8393 * A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called "ClinicalImpression" rather than "ClinicalAssessment" to avoid confusion with the recording of assessment tools such as Apgar score. 8394 */ 8395 CLINICALIMPRESSION, 8396 /** 8397 * A code system resource specifies a set of codes drawn from one or more code systems. 8398 */ 8399 CODESYSTEM, 8400 /** 8401 * An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition. 8402 */ 8403 COMMUNICATION, 8404 /** 8405 * A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition. 8406 */ 8407 COMMUNICATIONREQUEST, 8408 /** 8409 * A compartment definition that defines how resources are accessed on a server. 8410 */ 8411 COMPARTMENTDEFINITION, 8412 /** 8413 * A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained. 8414 */ 8415 COMPOSITION, 8416 /** 8417 * A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models. 8418 */ 8419 CONCEPTMAP, 8420 /** 8421 * A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern. 8422 */ 8423 CONDITION, 8424 /** 8425 * A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time. 8426 */ 8427 CONSENT, 8428 /** 8429 * A formal agreement between parties regarding the conduct of business, exchange of information or other matters. 8430 */ 8431 CONTRACT, 8432 /** 8433 * Financial instrument which may be used to reimburse or pay for health care products and services. 8434 */ 8435 COVERAGE, 8436 /** 8437 * The formal description of a single piece of information that can be gathered and reported. 8438 */ 8439 DATAELEMENT, 8440 /** 8441 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc. 8442 */ 8443 DETECTEDISSUE, 8444 /** 8445 * This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc. 8446 */ 8447 DEVICE, 8448 /** 8449 * The characteristics, operational status and capabilities of a medical-related component of a medical device. 8450 */ 8451 DEVICECOMPONENT, 8452 /** 8453 * Describes a measurement, calculation or setting capability of a medical device. 8454 */ 8455 DEVICEMETRIC, 8456 /** 8457 * Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker. 8458 */ 8459 DEVICEREQUEST, 8460 /** 8461 * A record of a device being used by a patient where the record is the result of a report from the patient or another clinician. 8462 */ 8463 DEVICEUSESTATEMENT, 8464 /** 8465 * The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports. 8466 */ 8467 DIAGNOSTICREPORT, 8468 /** 8469 * A collection of documents compiled for a purpose together with metadata that applies to the collection. 8470 */ 8471 DOCUMENTMANIFEST, 8472 /** 8473 * A reference to a document. 8474 */ 8475 DOCUMENTREFERENCE, 8476 /** 8477 * A resource that includes narrative, extensions, and contained resources. 8478 */ 8479 DOMAINRESOURCE, 8480 /** 8481 * The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy. 8482 */ 8483 ELIGIBILITYREQUEST, 8484 /** 8485 * This resource provides eligibility and plan details from the processing of an Eligibility resource. 8486 */ 8487 ELIGIBILITYRESPONSE, 8488 /** 8489 * An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient. 8490 */ 8491 ENCOUNTER, 8492 /** 8493 * The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information. 8494 */ 8495 ENDPOINT, 8496 /** 8497 * This resource provides the insurance enrollment details to the insurer regarding a specified coverage. 8498 */ 8499 ENROLLMENTREQUEST, 8500 /** 8501 * This resource provides enrollment and plan details from the processing of an Enrollment resource. 8502 */ 8503 ENROLLMENTRESPONSE, 8504 /** 8505 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 8506 */ 8507 EPISODEOFCARE, 8508 /** 8509 * Resource to define constraints on the Expansion of a FHIR ValueSet. 8510 */ 8511 EXPANSIONPROFILE, 8512 /** 8513 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 8514 */ 8515 EXPLANATIONOFBENEFIT, 8516 /** 8517 * Significant health events and conditions for a person related to the patient relevant in the context of care for the patient. 8518 */ 8519 FAMILYMEMBERHISTORY, 8520 /** 8521 * Prospective warnings of potential issues when providing care to the patient. 8522 */ 8523 FLAG, 8524 /** 8525 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 8526 */ 8527 GOAL, 8528 /** 8529 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 8530 */ 8531 GRAPHDEFINITION, 8532 /** 8533 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 8534 */ 8535 GROUP, 8536 /** 8537 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 8538 */ 8539 GUIDANCERESPONSE, 8540 /** 8541 * The details of a healthcare service available at a location. 8542 */ 8543 HEALTHCARESERVICE, 8544 /** 8545 * A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection. 8546 */ 8547 IMAGINGMANIFEST, 8548 /** 8549 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 8550 */ 8551 IMAGINGSTUDY, 8552 /** 8553 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 8554 */ 8555 IMMUNIZATION, 8556 /** 8557 * A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification. 8558 */ 8559 IMMUNIZATIONRECOMMENDATION, 8560 /** 8561 * A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 8562 */ 8563 IMPLEMENTATIONGUIDE, 8564 /** 8565 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 8566 */ 8567 LIBRARY, 8568 /** 8569 * Identifies two or more records (resource instances) that are referring to the same real-world "occurrence". 8570 */ 8571 LINKAGE, 8572 /** 8573 * A set of information summarized from a list of other resources. 8574 */ 8575 LIST, 8576 /** 8577 * Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated. 8578 */ 8579 LOCATION, 8580 /** 8581 * The Measure resource provides the definition of a quality measure. 8582 */ 8583 MEASURE, 8584 /** 8585 * The MeasureReport resource contains the results of evaluating a measure. 8586 */ 8587 MEASUREREPORT, 8588 /** 8589 * A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference. 8590 */ 8591 MEDIA, 8592 /** 8593 * This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication. 8594 */ 8595 MEDICATION, 8596 /** 8597 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. 8598 */ 8599 MEDICATIONADMINISTRATION, 8600 /** 8601 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 8602 */ 8603 MEDICATIONDISPENSE, 8604 /** 8605 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 8606 */ 8607 MEDICATIONREQUEST, 8608 /** 8609 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 8610 8611The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 8612 */ 8613 MEDICATIONSTATEMENT, 8614 /** 8615 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 8616 */ 8617 MESSAGEDEFINITION, 8618 /** 8619 * The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle. 8620 */ 8621 MESSAGEHEADER, 8622 /** 8623 * A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a "System" used within the Identifier and Coding data types. 8624 */ 8625 NAMINGSYSTEM, 8626 /** 8627 * A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident. 8628 */ 8629 NUTRITIONORDER, 8630 /** 8631 * Measurements and simple assertions made about a patient, device or other subject. 8632 */ 8633 OBSERVATION, 8634 /** 8635 * A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction). 8636 */ 8637 OPERATIONDEFINITION, 8638 /** 8639 * A collection of error, warning or information messages that result from a system action. 8640 */ 8641 OPERATIONOUTCOME, 8642 /** 8643 * A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc. 8644 */ 8645 ORGANIZATION, 8646 /** 8647 * This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it. 8648 */ 8649 PARAMETERS, 8650 /** 8651 * Demographics and other administrative information about an individual or animal receiving care or other health-related services. 8652 */ 8653 PATIENT, 8654 /** 8655 * This resource provides the status of the payment for goods and services rendered, and the request and response resource references. 8656 */ 8657 PAYMENTNOTICE, 8658 /** 8659 * This resource provides payment details and claim references supporting a bulk payment. 8660 */ 8661 PAYMENTRECONCILIATION, 8662 /** 8663 * Demographics and administrative information about a person independent of a specific health-related context. 8664 */ 8665 PERSON, 8666 /** 8667 * This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols. 8668 */ 8669 PLANDEFINITION, 8670 /** 8671 * A person who is directly or indirectly involved in the provisioning of healthcare. 8672 */ 8673 PRACTITIONER, 8674 /** 8675 * A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time. 8676 */ 8677 PRACTITIONERROLE, 8678 /** 8679 * An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy. 8680 */ 8681 PROCEDURE, 8682 /** 8683 * A record of a request for diagnostic investigations, treatments, or operations to be performed. 8684 */ 8685 PROCEDUREREQUEST, 8686 /** 8687 * This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources. 8688 */ 8689 PROCESSREQUEST, 8690 /** 8691 * This resource provides processing status, errors and notes from the processing of a resource. 8692 */ 8693 PROCESSRESPONSE, 8694 /** 8695 * Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies. 8696 */ 8697 PROVENANCE, 8698 /** 8699 * A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection. 8700 */ 8701 QUESTIONNAIRE, 8702 /** 8703 * A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to. 8704 */ 8705 QUESTIONNAIRERESPONSE, 8706 /** 8707 * Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization. 8708 */ 8709 REFERRALREQUEST, 8710 /** 8711 * Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process. 8712 */ 8713 RELATEDPERSON, 8714 /** 8715 * A group of related requests that can be used to capture intended activities that have inter-dependencies such as "give this medication after that one". 8716 */ 8717 REQUESTGROUP, 8718 /** 8719 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 8720 */ 8721 RESEARCHSTUDY, 8722 /** 8723 * A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects. 8724 */ 8725 RESEARCHSUBJECT, 8726 /** 8727 * This is the base resource type for everything. 8728 */ 8729 RESOURCE, 8730 /** 8731 * An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome. 8732 */ 8733 RISKASSESSMENT, 8734 /** 8735 * A container for slots of time that may be available for booking appointments. 8736 */ 8737 SCHEDULE, 8738 /** 8739 * A search parameter that defines a named search item that can be used to search/filter on a resource. 8740 */ 8741 SEARCHPARAMETER, 8742 /** 8743 * Raw data describing a biological sequence. 8744 */ 8745 SEQUENCE, 8746 /** 8747 * The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking. 8748 */ 8749 SERVICEDEFINITION, 8750 /** 8751 * A slot of time on a schedule that may be available for booking appointments. 8752 */ 8753 SLOT, 8754 /** 8755 * A sample to be used for analysis. 8756 */ 8757 SPECIMEN, 8758 /** 8759 * A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types. 8760 */ 8761 STRUCTUREDEFINITION, 8762 /** 8763 * A Map of relationships between 2 structures that can be used to transform data. 8764 */ 8765 STRUCTUREMAP, 8766 /** 8767 * The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined "channel" so that another system is able to take an appropriate action. 8768 */ 8769 SUBSCRIPTION, 8770 /** 8771 * A homogeneous material with a definite composition. 8772 */ 8773 SUBSTANCE, 8774 /** 8775 * Record of delivery of what is supplied. 8776 */ 8777 SUPPLYDELIVERY, 8778 /** 8779 * A record of a request for a medication, substance or device used in the healthcare setting. 8780 */ 8781 SUPPLYREQUEST, 8782 /** 8783 * A task to be performed. 8784 */ 8785 TASK, 8786 /** 8787 * A summary of information based on the results of executing a TestScript. 8788 */ 8789 TESTREPORT, 8790 /** 8791 * A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification. 8792 */ 8793 TESTSCRIPT, 8794 /** 8795 * A value set specifies a set of codes drawn from one or more code systems. 8796 */ 8797 VALUESET, 8798 /** 8799 * An authorization for the supply of glasses and/or contact lenses to a patient. 8800 */ 8801 VISIONPRESCRIPTION, 8802 /** 8803 * added to help the parsers 8804 */ 8805 NULL; 8806 public static ResourceType fromCode(String codeString) throws FHIRException { 8807 if (codeString == null || "".equals(codeString)) 8808 return null; 8809 if ("Account".equals(codeString)) 8810 return ACCOUNT; 8811 if ("ActivityDefinition".equals(codeString)) 8812 return ACTIVITYDEFINITION; 8813 if ("AdverseEvent".equals(codeString)) 8814 return ADVERSEEVENT; 8815 if ("AllergyIntolerance".equals(codeString)) 8816 return ALLERGYINTOLERANCE; 8817 if ("Appointment".equals(codeString)) 8818 return APPOINTMENT; 8819 if ("AppointmentResponse".equals(codeString)) 8820 return APPOINTMENTRESPONSE; 8821 if ("AuditEvent".equals(codeString)) 8822 return AUDITEVENT; 8823 if ("Basic".equals(codeString)) 8824 return BASIC; 8825 if ("Binary".equals(codeString)) 8826 return BINARY; 8827 if ("BodySite".equals(codeString)) 8828 return BODYSITE; 8829 if ("Bundle".equals(codeString)) 8830 return BUNDLE; 8831 if ("CapabilityStatement".equals(codeString)) 8832 return CAPABILITYSTATEMENT; 8833 if ("CarePlan".equals(codeString)) 8834 return CAREPLAN; 8835 if ("CareTeam".equals(codeString)) 8836 return CARETEAM; 8837 if ("ChargeItem".equals(codeString)) 8838 return CHARGEITEM; 8839 if ("Claim".equals(codeString)) 8840 return CLAIM; 8841 if ("ClaimResponse".equals(codeString)) 8842 return CLAIMRESPONSE; 8843 if ("ClinicalImpression".equals(codeString)) 8844 return CLINICALIMPRESSION; 8845 if ("CodeSystem".equals(codeString)) 8846 return CODESYSTEM; 8847 if ("Communication".equals(codeString)) 8848 return COMMUNICATION; 8849 if ("CommunicationRequest".equals(codeString)) 8850 return COMMUNICATIONREQUEST; 8851 if ("CompartmentDefinition".equals(codeString)) 8852 return COMPARTMENTDEFINITION; 8853 if ("Composition".equals(codeString)) 8854 return COMPOSITION; 8855 if ("ConceptMap".equals(codeString)) 8856 return CONCEPTMAP; 8857 if ("Condition".equals(codeString)) 8858 return CONDITION; 8859 if ("Consent".equals(codeString)) 8860 return CONSENT; 8861 if ("Contract".equals(codeString)) 8862 return CONTRACT; 8863 if ("Coverage".equals(codeString)) 8864 return COVERAGE; 8865 if ("DataElement".equals(codeString)) 8866 return DATAELEMENT; 8867 if ("DetectedIssue".equals(codeString)) 8868 return DETECTEDISSUE; 8869 if ("Device".equals(codeString)) 8870 return DEVICE; 8871 if ("DeviceComponent".equals(codeString)) 8872 return DEVICECOMPONENT; 8873 if ("DeviceMetric".equals(codeString)) 8874 return DEVICEMETRIC; 8875 if ("DeviceRequest".equals(codeString)) 8876 return DEVICEREQUEST; 8877 if ("DeviceUseStatement".equals(codeString)) 8878 return DEVICEUSESTATEMENT; 8879 if ("DiagnosticReport".equals(codeString)) 8880 return DIAGNOSTICREPORT; 8881 if ("DocumentManifest".equals(codeString)) 8882 return DOCUMENTMANIFEST; 8883 if ("DocumentReference".equals(codeString)) 8884 return DOCUMENTREFERENCE; 8885 if ("DomainResource".equals(codeString)) 8886 return DOMAINRESOURCE; 8887 if ("EligibilityRequest".equals(codeString)) 8888 return ELIGIBILITYREQUEST; 8889 if ("EligibilityResponse".equals(codeString)) 8890 return ELIGIBILITYRESPONSE; 8891 if ("Encounter".equals(codeString)) 8892 return ENCOUNTER; 8893 if ("Endpoint".equals(codeString)) 8894 return ENDPOINT; 8895 if ("EnrollmentRequest".equals(codeString)) 8896 return ENROLLMENTREQUEST; 8897 if ("EnrollmentResponse".equals(codeString)) 8898 return ENROLLMENTRESPONSE; 8899 if ("EpisodeOfCare".equals(codeString)) 8900 return EPISODEOFCARE; 8901 if ("ExpansionProfile".equals(codeString)) 8902 return EXPANSIONPROFILE; 8903 if ("ExplanationOfBenefit".equals(codeString)) 8904 return EXPLANATIONOFBENEFIT; 8905 if ("FamilyMemberHistory".equals(codeString)) 8906 return FAMILYMEMBERHISTORY; 8907 if ("Flag".equals(codeString)) 8908 return FLAG; 8909 if ("Goal".equals(codeString)) 8910 return GOAL; 8911 if ("GraphDefinition".equals(codeString)) 8912 return GRAPHDEFINITION; 8913 if ("Group".equals(codeString)) 8914 return GROUP; 8915 if ("GuidanceResponse".equals(codeString)) 8916 return GUIDANCERESPONSE; 8917 if ("HealthcareService".equals(codeString)) 8918 return HEALTHCARESERVICE; 8919 if ("ImagingManifest".equals(codeString)) 8920 return IMAGINGMANIFEST; 8921 if ("ImagingStudy".equals(codeString)) 8922 return IMAGINGSTUDY; 8923 if ("Immunization".equals(codeString)) 8924 return IMMUNIZATION; 8925 if ("ImmunizationRecommendation".equals(codeString)) 8926 return IMMUNIZATIONRECOMMENDATION; 8927 if ("ImplementationGuide".equals(codeString)) 8928 return IMPLEMENTATIONGUIDE; 8929 if ("Library".equals(codeString)) 8930 return LIBRARY; 8931 if ("Linkage".equals(codeString)) 8932 return LINKAGE; 8933 if ("List".equals(codeString)) 8934 return LIST; 8935 if ("Location".equals(codeString)) 8936 return LOCATION; 8937 if ("Measure".equals(codeString)) 8938 return MEASURE; 8939 if ("MeasureReport".equals(codeString)) 8940 return MEASUREREPORT; 8941 if ("Media".equals(codeString)) 8942 return MEDIA; 8943 if ("Medication".equals(codeString)) 8944 return MEDICATION; 8945 if ("MedicationAdministration".equals(codeString)) 8946 return MEDICATIONADMINISTRATION; 8947 if ("MedicationDispense".equals(codeString)) 8948 return MEDICATIONDISPENSE; 8949 if ("MedicationRequest".equals(codeString)) 8950 return MEDICATIONREQUEST; 8951 if ("MedicationStatement".equals(codeString)) 8952 return MEDICATIONSTATEMENT; 8953 if ("MessageDefinition".equals(codeString)) 8954 return MESSAGEDEFINITION; 8955 if ("MessageHeader".equals(codeString)) 8956 return MESSAGEHEADER; 8957 if ("NamingSystem".equals(codeString)) 8958 return NAMINGSYSTEM; 8959 if ("NutritionOrder".equals(codeString)) 8960 return NUTRITIONORDER; 8961 if ("Observation".equals(codeString)) 8962 return OBSERVATION; 8963 if ("OperationDefinition".equals(codeString)) 8964 return OPERATIONDEFINITION; 8965 if ("OperationOutcome".equals(codeString)) 8966 return OPERATIONOUTCOME; 8967 if ("Organization".equals(codeString)) 8968 return ORGANIZATION; 8969 if ("Parameters".equals(codeString)) 8970 return PARAMETERS; 8971 if ("Patient".equals(codeString)) 8972 return PATIENT; 8973 if ("PaymentNotice".equals(codeString)) 8974 return PAYMENTNOTICE; 8975 if ("PaymentReconciliation".equals(codeString)) 8976 return PAYMENTRECONCILIATION; 8977 if ("Person".equals(codeString)) 8978 return PERSON; 8979 if ("PlanDefinition".equals(codeString)) 8980 return PLANDEFINITION; 8981 if ("Practitioner".equals(codeString)) 8982 return PRACTITIONER; 8983 if ("PractitionerRole".equals(codeString)) 8984 return PRACTITIONERROLE; 8985 if ("Procedure".equals(codeString)) 8986 return PROCEDURE; 8987 if ("ProcedureRequest".equals(codeString)) 8988 return PROCEDUREREQUEST; 8989 if ("ProcessRequest".equals(codeString)) 8990 return PROCESSREQUEST; 8991 if ("ProcessResponse".equals(codeString)) 8992 return PROCESSRESPONSE; 8993 if ("Provenance".equals(codeString)) 8994 return PROVENANCE; 8995 if ("Questionnaire".equals(codeString)) 8996 return QUESTIONNAIRE; 8997 if ("QuestionnaireResponse".equals(codeString)) 8998 return QUESTIONNAIRERESPONSE; 8999 if ("ReferralRequest".equals(codeString)) 9000 return REFERRALREQUEST; 9001 if ("RelatedPerson".equals(codeString)) 9002 return RELATEDPERSON; 9003 if ("RequestGroup".equals(codeString)) 9004 return REQUESTGROUP; 9005 if ("ResearchStudy".equals(codeString)) 9006 return RESEARCHSTUDY; 9007 if ("ResearchSubject".equals(codeString)) 9008 return RESEARCHSUBJECT; 9009 if ("Resource".equals(codeString)) 9010 return RESOURCE; 9011 if ("RiskAssessment".equals(codeString)) 9012 return RISKASSESSMENT; 9013 if ("Schedule".equals(codeString)) 9014 return SCHEDULE; 9015 if ("SearchParameter".equals(codeString)) 9016 return SEARCHPARAMETER; 9017 if ("Sequence".equals(codeString)) 9018 return SEQUENCE; 9019 if ("ServiceDefinition".equals(codeString)) 9020 return SERVICEDEFINITION; 9021 if ("Slot".equals(codeString)) 9022 return SLOT; 9023 if ("Specimen".equals(codeString)) 9024 return SPECIMEN; 9025 if ("StructureDefinition".equals(codeString)) 9026 return STRUCTUREDEFINITION; 9027 if ("StructureMap".equals(codeString)) 9028 return STRUCTUREMAP; 9029 if ("Subscription".equals(codeString)) 9030 return SUBSCRIPTION; 9031 if ("Substance".equals(codeString)) 9032 return SUBSTANCE; 9033 if ("SupplyDelivery".equals(codeString)) 9034 return SUPPLYDELIVERY; 9035 if ("SupplyRequest".equals(codeString)) 9036 return SUPPLYREQUEST; 9037 if ("Task".equals(codeString)) 9038 return TASK; 9039 if ("TestReport".equals(codeString)) 9040 return TESTREPORT; 9041 if ("TestScript".equals(codeString)) 9042 return TESTSCRIPT; 9043 if ("ValueSet".equals(codeString)) 9044 return VALUESET; 9045 if ("VisionPrescription".equals(codeString)) 9046 return VISIONPRESCRIPTION; 9047 throw new FHIRException("Unknown ResourceType code '"+codeString+"'"); 9048 } 9049 public String toCode() { 9050 switch (this) { 9051 case ACCOUNT: return "Account"; 9052 case ACTIVITYDEFINITION: return "ActivityDefinition"; 9053 case ADVERSEEVENT: return "AdverseEvent"; 9054 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 9055 case APPOINTMENT: return "Appointment"; 9056 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 9057 case AUDITEVENT: return "AuditEvent"; 9058 case BASIC: return "Basic"; 9059 case BINARY: return "Binary"; 9060 case BODYSITE: return "BodySite"; 9061 case BUNDLE: return "Bundle"; 9062 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 9063 case CAREPLAN: return "CarePlan"; 9064 case CARETEAM: return "CareTeam"; 9065 case CHARGEITEM: return "ChargeItem"; 9066 case CLAIM: return "Claim"; 9067 case CLAIMRESPONSE: return "ClaimResponse"; 9068 case CLINICALIMPRESSION: return "ClinicalImpression"; 9069 case CODESYSTEM: return "CodeSystem"; 9070 case COMMUNICATION: return "Communication"; 9071 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 9072 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 9073 case COMPOSITION: return "Composition"; 9074 case CONCEPTMAP: return "ConceptMap"; 9075 case CONDITION: return "Condition"; 9076 case CONSENT: return "Consent"; 9077 case CONTRACT: return "Contract"; 9078 case COVERAGE: return "Coverage"; 9079 case DATAELEMENT: return "DataElement"; 9080 case DETECTEDISSUE: return "DetectedIssue"; 9081 case DEVICE: return "Device"; 9082 case DEVICECOMPONENT: return "DeviceComponent"; 9083 case DEVICEMETRIC: return "DeviceMetric"; 9084 case DEVICEREQUEST: return "DeviceRequest"; 9085 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 9086 case DIAGNOSTICREPORT: return "DiagnosticReport"; 9087 case DOCUMENTMANIFEST: return "DocumentManifest"; 9088 case DOCUMENTREFERENCE: return "DocumentReference"; 9089 case DOMAINRESOURCE: return "DomainResource"; 9090 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 9091 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 9092 case ENCOUNTER: return "Encounter"; 9093 case ENDPOINT: return "Endpoint"; 9094 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 9095 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 9096 case EPISODEOFCARE: return "EpisodeOfCare"; 9097 case EXPANSIONPROFILE: return "ExpansionProfile"; 9098 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 9099 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 9100 case FLAG: return "Flag"; 9101 case GOAL: return "Goal"; 9102 case GRAPHDEFINITION: return "GraphDefinition"; 9103 case GROUP: return "Group"; 9104 case GUIDANCERESPONSE: return "GuidanceResponse"; 9105 case HEALTHCARESERVICE: return "HealthcareService"; 9106 case IMAGINGMANIFEST: return "ImagingManifest"; 9107 case IMAGINGSTUDY: return "ImagingStudy"; 9108 case IMMUNIZATION: return "Immunization"; 9109 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 9110 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 9111 case LIBRARY: return "Library"; 9112 case LINKAGE: return "Linkage"; 9113 case LIST: return "List"; 9114 case LOCATION: return "Location"; 9115 case MEASURE: return "Measure"; 9116 case MEASUREREPORT: return "MeasureReport"; 9117 case MEDIA: return "Media"; 9118 case MEDICATION: return "Medication"; 9119 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 9120 case MEDICATIONDISPENSE: return "MedicationDispense"; 9121 case MEDICATIONREQUEST: return "MedicationRequest"; 9122 case MEDICATIONSTATEMENT: return "MedicationStatement"; 9123 case MESSAGEDEFINITION: return "MessageDefinition"; 9124 case MESSAGEHEADER: return "MessageHeader"; 9125 case NAMINGSYSTEM: return "NamingSystem"; 9126 case NUTRITIONORDER: return "NutritionOrder"; 9127 case OBSERVATION: return "Observation"; 9128 case OPERATIONDEFINITION: return "OperationDefinition"; 9129 case OPERATIONOUTCOME: return "OperationOutcome"; 9130 case ORGANIZATION: return "Organization"; 9131 case PARAMETERS: return "Parameters"; 9132 case PATIENT: return "Patient"; 9133 case PAYMENTNOTICE: return "PaymentNotice"; 9134 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 9135 case PERSON: return "Person"; 9136 case PLANDEFINITION: return "PlanDefinition"; 9137 case PRACTITIONER: return "Practitioner"; 9138 case PRACTITIONERROLE: return "PractitionerRole"; 9139 case PROCEDURE: return "Procedure"; 9140 case PROCEDUREREQUEST: return "ProcedureRequest"; 9141 case PROCESSREQUEST: return "ProcessRequest"; 9142 case PROCESSRESPONSE: return "ProcessResponse"; 9143 case PROVENANCE: return "Provenance"; 9144 case QUESTIONNAIRE: return "Questionnaire"; 9145 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 9146 case REFERRALREQUEST: return "ReferralRequest"; 9147 case RELATEDPERSON: return "RelatedPerson"; 9148 case REQUESTGROUP: return "RequestGroup"; 9149 case RESEARCHSTUDY: return "ResearchStudy"; 9150 case RESEARCHSUBJECT: return "ResearchSubject"; 9151 case RESOURCE: return "Resource"; 9152 case RISKASSESSMENT: return "RiskAssessment"; 9153 case SCHEDULE: return "Schedule"; 9154 case SEARCHPARAMETER: return "SearchParameter"; 9155 case SEQUENCE: return "Sequence"; 9156 case SERVICEDEFINITION: return "ServiceDefinition"; 9157 case SLOT: return "Slot"; 9158 case SPECIMEN: return "Specimen"; 9159 case STRUCTUREDEFINITION: return "StructureDefinition"; 9160 case STRUCTUREMAP: return "StructureMap"; 9161 case SUBSCRIPTION: return "Subscription"; 9162 case SUBSTANCE: return "Substance"; 9163 case SUPPLYDELIVERY: return "SupplyDelivery"; 9164 case SUPPLYREQUEST: return "SupplyRequest"; 9165 case TASK: return "Task"; 9166 case TESTREPORT: return "TestReport"; 9167 case TESTSCRIPT: return "TestScript"; 9168 case VALUESET: return "ValueSet"; 9169 case VISIONPRESCRIPTION: return "VisionPrescription"; 9170 case NULL: return null; 9171 default: return "?"; 9172 } 9173 } 9174 public String getSystem() { 9175 switch (this) { 9176 case ACCOUNT: return "http://hl7.org/fhir/resource-types"; 9177 case ACTIVITYDEFINITION: return "http://hl7.org/fhir/resource-types"; 9178 case ADVERSEEVENT: return "http://hl7.org/fhir/resource-types"; 9179 case ALLERGYINTOLERANCE: return "http://hl7.org/fhir/resource-types"; 9180 case APPOINTMENT: return "http://hl7.org/fhir/resource-types"; 9181 case APPOINTMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 9182 case AUDITEVENT: return "http://hl7.org/fhir/resource-types"; 9183 case BASIC: return "http://hl7.org/fhir/resource-types"; 9184 case BINARY: return "http://hl7.org/fhir/resource-types"; 9185 case BODYSITE: return "http://hl7.org/fhir/resource-types"; 9186 case BUNDLE: return "http://hl7.org/fhir/resource-types"; 9187 case CAPABILITYSTATEMENT: return "http://hl7.org/fhir/resource-types"; 9188 case CAREPLAN: return "http://hl7.org/fhir/resource-types"; 9189 case CARETEAM: return "http://hl7.org/fhir/resource-types"; 9190 case CHARGEITEM: return "http://hl7.org/fhir/resource-types"; 9191 case CLAIM: return "http://hl7.org/fhir/resource-types"; 9192 case CLAIMRESPONSE: return "http://hl7.org/fhir/resource-types"; 9193 case CLINICALIMPRESSION: return "http://hl7.org/fhir/resource-types"; 9194 case CODESYSTEM: return "http://hl7.org/fhir/resource-types"; 9195 case COMMUNICATION: return "http://hl7.org/fhir/resource-types"; 9196 case COMMUNICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 9197 case COMPARTMENTDEFINITION: return "http://hl7.org/fhir/resource-types"; 9198 case COMPOSITION: return "http://hl7.org/fhir/resource-types"; 9199 case CONCEPTMAP: return "http://hl7.org/fhir/resource-types"; 9200 case CONDITION: return "http://hl7.org/fhir/resource-types"; 9201 case CONSENT: return "http://hl7.org/fhir/resource-types"; 9202 case CONTRACT: return "http://hl7.org/fhir/resource-types"; 9203 case COVERAGE: return "http://hl7.org/fhir/resource-types"; 9204 case DATAELEMENT: return "http://hl7.org/fhir/resource-types"; 9205 case DETECTEDISSUE: return "http://hl7.org/fhir/resource-types"; 9206 case DEVICE: return "http://hl7.org/fhir/resource-types"; 9207 case DEVICECOMPONENT: return "http://hl7.org/fhir/resource-types"; 9208 case DEVICEMETRIC: return "http://hl7.org/fhir/resource-types"; 9209 case DEVICEREQUEST: return "http://hl7.org/fhir/resource-types"; 9210 case DEVICEUSESTATEMENT: return "http://hl7.org/fhir/resource-types"; 9211 case DIAGNOSTICREPORT: return "http://hl7.org/fhir/resource-types"; 9212 case DOCUMENTMANIFEST: return "http://hl7.org/fhir/resource-types"; 9213 case DOCUMENTREFERENCE: return "http://hl7.org/fhir/resource-types"; 9214 case DOMAINRESOURCE: return "http://hl7.org/fhir/resource-types"; 9215 case ELIGIBILITYREQUEST: return "http://hl7.org/fhir/resource-types"; 9216 case ELIGIBILITYRESPONSE: return "http://hl7.org/fhir/resource-types"; 9217 case ENCOUNTER: return "http://hl7.org/fhir/resource-types"; 9218 case ENDPOINT: return "http://hl7.org/fhir/resource-types"; 9219 case ENROLLMENTREQUEST: return "http://hl7.org/fhir/resource-types"; 9220 case ENROLLMENTRESPONSE: return "http://hl7.org/fhir/resource-types"; 9221 case EPISODEOFCARE: return "http://hl7.org/fhir/resource-types"; 9222 case EXPANSIONPROFILE: return "http://hl7.org/fhir/resource-types"; 9223 case EXPLANATIONOFBENEFIT: return "http://hl7.org/fhir/resource-types"; 9224 case FAMILYMEMBERHISTORY: return "http://hl7.org/fhir/resource-types"; 9225 case FLAG: return "http://hl7.org/fhir/resource-types"; 9226 case GOAL: return "http://hl7.org/fhir/resource-types"; 9227 case GRAPHDEFINITION: return "http://hl7.org/fhir/resource-types"; 9228 case GROUP: return "http://hl7.org/fhir/resource-types"; 9229 case GUIDANCERESPONSE: return "http://hl7.org/fhir/resource-types"; 9230 case HEALTHCARESERVICE: return "http://hl7.org/fhir/resource-types"; 9231 case IMAGINGMANIFEST: return "http://hl7.org/fhir/resource-types"; 9232 case IMAGINGSTUDY: return "http://hl7.org/fhir/resource-types"; 9233 case IMMUNIZATION: return "http://hl7.org/fhir/resource-types"; 9234 case IMMUNIZATIONRECOMMENDATION: return "http://hl7.org/fhir/resource-types"; 9235 case IMPLEMENTATIONGUIDE: return "http://hl7.org/fhir/resource-types"; 9236 case LIBRARY: return "http://hl7.org/fhir/resource-types"; 9237 case LINKAGE: return "http://hl7.org/fhir/resource-types"; 9238 case LIST: return "http://hl7.org/fhir/resource-types"; 9239 case LOCATION: return "http://hl7.org/fhir/resource-types"; 9240 case MEASURE: return "http://hl7.org/fhir/resource-types"; 9241 case MEASUREREPORT: return "http://hl7.org/fhir/resource-types"; 9242 case MEDIA: return "http://hl7.org/fhir/resource-types"; 9243 case MEDICATION: return "http://hl7.org/fhir/resource-types"; 9244 case MEDICATIONADMINISTRATION: return "http://hl7.org/fhir/resource-types"; 9245 case MEDICATIONDISPENSE: return "http://hl7.org/fhir/resource-types"; 9246 case MEDICATIONREQUEST: return "http://hl7.org/fhir/resource-types"; 9247 case MEDICATIONSTATEMENT: return "http://hl7.org/fhir/resource-types"; 9248 case MESSAGEDEFINITION: return "http://hl7.org/fhir/resource-types"; 9249 case MESSAGEHEADER: return "http://hl7.org/fhir/resource-types"; 9250 case NAMINGSYSTEM: return "http://hl7.org/fhir/resource-types"; 9251 case NUTRITIONORDER: return "http://hl7.org/fhir/resource-types"; 9252 case OBSERVATION: return "http://hl7.org/fhir/resource-types"; 9253 case OPERATIONDEFINITION: return "http://hl7.org/fhir/resource-types"; 9254 case OPERATIONOUTCOME: return "http://hl7.org/fhir/resource-types"; 9255 case ORGANIZATION: return "http://hl7.org/fhir/resource-types"; 9256 case PARAMETERS: return "http://hl7.org/fhir/resource-types"; 9257 case PATIENT: return "http://hl7.org/fhir/resource-types"; 9258 case PAYMENTNOTICE: return "http://hl7.org/fhir/resource-types"; 9259 case PAYMENTRECONCILIATION: return "http://hl7.org/fhir/resource-types"; 9260 case PERSON: return "http://hl7.org/fhir/resource-types"; 9261 case PLANDEFINITION: return "http://hl7.org/fhir/resource-types"; 9262 case PRACTITIONER: return "http://hl7.org/fhir/resource-types"; 9263 case PRACTITIONERROLE: return "http://hl7.org/fhir/resource-types"; 9264 case PROCEDURE: return "http://hl7.org/fhir/resource-types"; 9265 case PROCEDUREREQUEST: return "http://hl7.org/fhir/resource-types"; 9266 case PROCESSREQUEST: return "http://hl7.org/fhir/resource-types"; 9267 case PROCESSRESPONSE: return "http://hl7.org/fhir/resource-types"; 9268 case PROVENANCE: return "http://hl7.org/fhir/resource-types"; 9269 case QUESTIONNAIRE: return "http://hl7.org/fhir/resource-types"; 9270 case QUESTIONNAIRERESPONSE: return "http://hl7.org/fhir/resource-types"; 9271 case REFERRALREQUEST: return "http://hl7.org/fhir/resource-types"; 9272 case RELATEDPERSON: return "http://hl7.org/fhir/resource-types"; 9273 case REQUESTGROUP: return "http://hl7.org/fhir/resource-types"; 9274 case RESEARCHSTUDY: return "http://hl7.org/fhir/resource-types"; 9275 case RESEARCHSUBJECT: return "http://hl7.org/fhir/resource-types"; 9276 case RESOURCE: return "http://hl7.org/fhir/resource-types"; 9277 case RISKASSESSMENT: return "http://hl7.org/fhir/resource-types"; 9278 case SCHEDULE: return "http://hl7.org/fhir/resource-types"; 9279 case SEARCHPARAMETER: return "http://hl7.org/fhir/resource-types"; 9280 case SEQUENCE: return "http://hl7.org/fhir/resource-types"; 9281 case SERVICEDEFINITION: return "http://hl7.org/fhir/resource-types"; 9282 case SLOT: return "http://hl7.org/fhir/resource-types"; 9283 case SPECIMEN: return "http://hl7.org/fhir/resource-types"; 9284 case STRUCTUREDEFINITION: return "http://hl7.org/fhir/resource-types"; 9285 case STRUCTUREMAP: return "http://hl7.org/fhir/resource-types"; 9286 case SUBSCRIPTION: return "http://hl7.org/fhir/resource-types"; 9287 case SUBSTANCE: return "http://hl7.org/fhir/resource-types"; 9288 case SUPPLYDELIVERY: return "http://hl7.org/fhir/resource-types"; 9289 case SUPPLYREQUEST: return "http://hl7.org/fhir/resource-types"; 9290 case TASK: return "http://hl7.org/fhir/resource-types"; 9291 case TESTREPORT: return "http://hl7.org/fhir/resource-types"; 9292 case TESTSCRIPT: return "http://hl7.org/fhir/resource-types"; 9293 case VALUESET: return "http://hl7.org/fhir/resource-types"; 9294 case VISIONPRESCRIPTION: return "http://hl7.org/fhir/resource-types"; 9295 case NULL: return null; 9296 default: return "?"; 9297 } 9298 } 9299 public String getDefinition() { 9300 switch (this) { 9301 case ACCOUNT: return "A financial tool for tracking value accrued for a particular purpose. In the healthcare field, used to track charges for a patient, cost centers, etc."; 9302 case ACTIVITYDEFINITION: return "This resource allows for the definition of some activity to be performed, independent of a particular patient, practitioner, or other performance context."; 9303 case ADVERSEEVENT: return "Actual or potential/avoided event causing unintended physical injury resulting from or contributed to by medical care, a research study or other healthcare setting factors that requires additional monitoring, treatment, or hospitalization, or that results in death."; 9304 case ALLERGYINTOLERANCE: return "Risk of harmful or undesirable, physiological response which is unique to an individual and associated with exposure to a substance."; 9305 case APPOINTMENT: return "A booking of a healthcare event among patient(s), practitioner(s), related person(s) and/or device(s) for a specific date/time. This may result in one or more Encounter(s)."; 9306 case APPOINTMENTRESPONSE: return "A reply to an appointment request for a patient and/or practitioner(s), such as a confirmation or rejection."; 9307 case AUDITEVENT: return "A record of an event made for purposes of maintaining a security log. Typical uses include detection of intrusion attempts and monitoring for inappropriate usage."; 9308 case BASIC: return "Basic is used for handling concepts not yet defined in FHIR, narrative-only resources that don't map to an existing resource, and custom resources not appropriate for inclusion in the FHIR specification."; 9309 case BINARY: return "A binary resource can contain any content, whether text, image, pdf, zip archive, etc."; 9310 case BODYSITE: return "Record details about the anatomical location of a specimen or body part. This resource may be used when a coded concept does not provide the necessary detail needed for the use case."; 9311 case BUNDLE: return "A container for a collection of resources."; 9312 case CAPABILITYSTATEMENT: return "A Capability Statement documents a set of capabilities (behaviors) of a FHIR Server that may be used as a statement of actual server functionality or a statement of required or desired server implementation."; 9313 case CAREPLAN: return "Describes the intention of how one or more practitioners intend to deliver care for a particular patient, group or community for a period of time, possibly limited to care for a specific condition or set of conditions."; 9314 case CARETEAM: return "The Care Team includes all the people and organizations who plan to participate in the coordination and delivery of care for a patient."; 9315 case CHARGEITEM: return "The resource ChargeItem describes the provision of healthcare provider products for a certain patient, therefore referring not only to the product, but containing in addition details of the provision, like date, time, amounts and participating organizations and persons. Main Usage of the ChargeItem is to enable the billing process and internal cost allocation."; 9316 case CLAIM: return "A provider issued list of services and products provided, or to be provided, to a patient which is provided to an insurer for payment recovery."; 9317 case CLAIMRESPONSE: return "This resource provides the adjudication details from the processing of a Claim resource."; 9318 case CLINICALIMPRESSION: return "A record of a clinical assessment performed to determine what problem(s) may affect the patient and before planning the treatments or management strategies that are best to manage a patient's condition. Assessments are often 1:1 with a clinical consultation / encounter, but this varies greatly depending on the clinical workflow. This resource is called \"ClinicalImpression\" rather than \"ClinicalAssessment\" to avoid confusion with the recording of assessment tools such as Apgar score."; 9319 case CODESYSTEM: return "A code system resource specifies a set of codes drawn from one or more code systems."; 9320 case COMMUNICATION: return "An occurrence of information being transmitted; e.g. an alert that was sent to a responsible provider, a public health agency was notified about a reportable condition."; 9321 case COMMUNICATIONREQUEST: return "A request to convey information; e.g. the CDS system proposes that an alert be sent to a responsible provider, the CDS system proposes that the public health agency be notified about a reportable condition."; 9322 case COMPARTMENTDEFINITION: return "A compartment definition that defines how resources are accessed on a server."; 9323 case COMPOSITION: return "A set of healthcare-related information that is assembled together into a single logical document that provides a single coherent statement of meaning, establishes its own context and that has clinical attestation with regard to who is making the statement. While a Composition defines the structure, it does not actually contain the content: rather the full content of a document is contained in a Bundle, of which the Composition is the first resource contained."; 9324 case CONCEPTMAP: return "A statement of relationships from one set of concepts to one or more other concepts - either code systems or data elements, or classes in class models."; 9325 case CONDITION: return "A clinical condition, problem, diagnosis, or other event, situation, issue, or clinical concept that has risen to a level of concern."; 9326 case CONSENT: return "A record of a healthcare consumer?s policy choices, which permits or denies identified recipient(s) or recipient role(s) to perform one or more actions within a given policy context, for specific purposes and periods of time."; 9327 case CONTRACT: return "A formal agreement between parties regarding the conduct of business, exchange of information or other matters."; 9328 case COVERAGE: return "Financial instrument which may be used to reimburse or pay for health care products and services."; 9329 case DATAELEMENT: return "The formal description of a single piece of information that can be gathered and reported."; 9330 case DETECTEDISSUE: return "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, Ineffective treatment frequency, Procedure-condition conflict, etc."; 9331 case DEVICE: return "This resource identifies an instance or a type of a manufactured item that is used in the provision of healthcare without being substantially changed through that activity. The device may be a medical or non-medical device. Medical devices include durable (reusable) medical equipment, implantable devices, as well as disposable equipment used for diagnostic, treatment, and research for healthcare and public health. Non-medical devices may include items such as a machine, cellphone, computer, application, etc."; 9332 case DEVICECOMPONENT: return "The characteristics, operational status and capabilities of a medical-related component of a medical device."; 9333 case DEVICEMETRIC: return "Describes a measurement, calculation or setting capability of a medical device."; 9334 case DEVICEREQUEST: return "Represents a request for a patient to employ a medical device. The device may be an implantable device, or an external assistive device, such as a walker."; 9335 case DEVICEUSESTATEMENT: return "A record of a device being used by a patient where the record is the result of a report from the patient or another clinician."; 9336 case DIAGNOSTICREPORT: return "The findings and interpretation of diagnostic tests performed on patients, groups of patients, devices, and locations, and/or specimens derived from these. The report includes clinical context such as requesting and provider information, and some mix of atomic results, images, textual and coded interpretations, and formatted representation of diagnostic reports."; 9337 case DOCUMENTMANIFEST: return "A collection of documents compiled for a purpose together with metadata that applies to the collection."; 9338 case DOCUMENTREFERENCE: return "A reference to a document."; 9339 case DOMAINRESOURCE: return "A resource that includes narrative, extensions, and contained resources."; 9340 case ELIGIBILITYREQUEST: return "The EligibilityRequest provides patient and insurance coverage information to an insurer for them to respond, in the form of an EligibilityResponse, with information regarding whether the stated coverage is valid and in-force and optionally to provide the insurance details of the policy."; 9341 case ELIGIBILITYRESPONSE: return "This resource provides eligibility and plan details from the processing of an Eligibility resource."; 9342 case ENCOUNTER: return "An interaction between a patient and healthcare provider(s) for the purpose of providing healthcare service(s) or assessing the health status of a patient."; 9343 case ENDPOINT: return "The technical details of an endpoint that can be used for electronic services, such as for web services providing XDS.b or a REST endpoint for another FHIR server. This may include any security context information."; 9344 case ENROLLMENTREQUEST: return "This resource provides the insurance enrollment details to the insurer regarding a specified coverage."; 9345 case ENROLLMENTRESPONSE: return "This resource provides enrollment and plan details from the processing of an Enrollment resource."; 9346 case EPISODEOFCARE: return "An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time."; 9347 case EXPANSIONPROFILE: return "Resource to define constraints on the Expansion of a FHIR ValueSet."; 9348 case EXPLANATIONOFBENEFIT: return "This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided."; 9349 case FAMILYMEMBERHISTORY: return "Significant health events and conditions for a person related to the patient relevant in the context of care for the patient."; 9350 case FLAG: return "Prospective warnings of potential issues when providing care to the patient."; 9351 case GOAL: return "Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc."; 9352 case GRAPHDEFINITION: return "A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set."; 9353 case GROUP: return "Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization."; 9354 case GUIDANCERESPONSE: return "A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken."; 9355 case HEALTHCARESERVICE: return "The details of a healthcare service available at a location."; 9356 case IMAGINGMANIFEST: return "A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection."; 9357 case IMAGINGSTUDY: return "Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities."; 9358 case IMMUNIZATION: return "Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed."; 9359 case IMMUNIZATIONRECOMMENDATION: return "A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification."; 9360 case IMPLEMENTATIONGUIDE: return "A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts."; 9361 case LIBRARY: return "The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets."; 9362 case LINKAGE: return "Identifies two or more records (resource instances) that are referring to the same real-world \"occurrence\"."; 9363 case LIST: return "A set of information summarized from a list of other resources."; 9364 case LOCATION: return "Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated."; 9365 case MEASURE: return "The Measure resource provides the definition of a quality measure."; 9366 case MEASUREREPORT: return "The MeasureReport resource contains the results of evaluating a measure."; 9367 case MEDIA: return "A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference."; 9368 case MEDICATION: return "This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication."; 9369 case MEDICATIONADMINISTRATION: return "Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner."; 9370 case MEDICATIONDISPENSE: return "Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order."; 9371 case MEDICATIONREQUEST: return "An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called \"MedicationRequest\" rather than \"MedicationPrescription\" or \"MedicationOrder\" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns."; 9372 case MEDICATIONSTATEMENT: return "A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains \r\rThe primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information."; 9373 case MESSAGEDEFINITION: return "Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted."; 9374 case MESSAGEHEADER: return "The header for a message exchange that is either requesting or responding to an action. The reference(s) that are the subject of the action as well as other information related to the action are typically transmitted in a bundle in which the MessageHeader resource instance is the first resource in the bundle."; 9375 case NAMINGSYSTEM: return "A curated namespace that issues unique symbols within that namespace for the identification of concepts, people, devices, etc. Represents a \"System\" used within the Identifier and Coding data types."; 9376 case NUTRITIONORDER: return "A request to supply a diet, formula feeding (enteral) or oral nutritional supplement to a patient/resident."; 9377 case OBSERVATION: return "Measurements and simple assertions made about a patient, device or other subject."; 9378 case OPERATIONDEFINITION: return "A formal computable definition of an operation (on the RESTful interface) or a named query (using the search interaction)."; 9379 case OPERATIONOUTCOME: return "A collection of error, warning or information messages that result from a system action."; 9380 case ORGANIZATION: return "A formally or informally recognized grouping of people or organizations formed for the purpose of achieving some form of collective action. Includes companies, institutions, corporations, departments, community groups, healthcare practice groups, etc."; 9381 case PARAMETERS: return "This special resource type is used to represent an operation request and response (operations.html). It has no other use, and there is no RESTful endpoint associated with it."; 9382 case PATIENT: return "Demographics and other administrative information about an individual or animal receiving care or other health-related services."; 9383 case PAYMENTNOTICE: return "This resource provides the status of the payment for goods and services rendered, and the request and response resource references."; 9384 case PAYMENTRECONCILIATION: return "This resource provides payment details and claim references supporting a bulk payment."; 9385 case PERSON: return "Demographics and administrative information about a person independent of a specific health-related context."; 9386 case PLANDEFINITION: return "This resource allows for the definition of various types of plans as a sharable, consumable, and executable artifact. The resource is general enough to support the description of a broad range of clinical artifacts such as clinical decision support rules, order sets and protocols."; 9387 case PRACTITIONER: return "A person who is directly or indirectly involved in the provisioning of healthcare."; 9388 case PRACTITIONERROLE: return "A specific set of Roles/Locations/specialties/services that a practitioner may perform at an organization for a period of time."; 9389 case PROCEDURE: return "An action that is or was performed on a patient. This can be a physical intervention like an operation, or less invasive like counseling or hypnotherapy."; 9390 case PROCEDUREREQUEST: return "A record of a request for diagnostic investigations, treatments, or operations to be performed."; 9391 case PROCESSREQUEST: return "This resource provides the target, request and response, and action details for an action to be performed by the target on or about existing resources."; 9392 case PROCESSRESPONSE: return "This resource provides processing status, errors and notes from the processing of a resource."; 9393 case PROVENANCE: return "Provenance of a resource is a record that describes entities and processes involved in producing and delivering or otherwise influencing that resource. Provenance provides a critical foundation for assessing authenticity, enabling trust, and allowing reproducibility. Provenance assertions are a form of contextual metadata and can themselves become important records with their own provenance. Provenance statement indicates clinical significance in terms of confidence in authenticity, reliability, and trustworthiness, integrity, and stage in lifecycle (e.g. Document Completion - has the artifact been legally authenticated), all of which may impact security, privacy, and trust policies."; 9394 case QUESTIONNAIRE: return "A structured set of questions intended to guide the collection of answers from end-users. Questionnaires provide detailed control over order, presentation, phraseology and grouping to allow coherent, consistent data collection."; 9395 case QUESTIONNAIRERESPONSE: return "A structured set of questions and their answers. The questions are ordered and grouped into coherent subsets, corresponding to the structure of the grouping of the questionnaire being responded to."; 9396 case REFERRALREQUEST: return "Used to record and send details about a request for referral service or transfer of a patient to the care of another provider or provider organization."; 9397 case RELATEDPERSON: return "Information about a person that is involved in the care for a patient, but who is not the target of healthcare, nor has a formal responsibility in the care process."; 9398 case REQUESTGROUP: return "A group of related requests that can be used to capture intended activities that have inter-dependencies such as \"give this medication after that one\"."; 9399 case RESEARCHSTUDY: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 9400 case RESEARCHSUBJECT: return "A process where a researcher or organization plans and then executes a series of steps intended to increase the field of healthcare-related knowledge. This includes studies of safety, efficacy, comparative effectiveness and other information about medications, devices, therapies and other interventional and investigative techniques. A ResearchStudy involves the gathering of information about human or animal subjects."; 9401 case RESOURCE: return "This is the base resource type for everything."; 9402 case RISKASSESSMENT: return "An assessment of the likely outcome(s) for a patient or other subject as well as the likelihood of each outcome."; 9403 case SCHEDULE: return "A container for slots of time that may be available for booking appointments."; 9404 case SEARCHPARAMETER: return "A search parameter that defines a named search item that can be used to search/filter on a resource."; 9405 case SEQUENCE: return "Raw data describing a biological sequence."; 9406 case SERVICEDEFINITION: return "The ServiceDefinition describes a unit of decision support functionality that is made available as a service, such as immunization modules or drug-drug interaction checking."; 9407 case SLOT: return "A slot of time on a schedule that may be available for booking appointments."; 9408 case SPECIMEN: return "A sample to be used for analysis."; 9409 case STRUCTUREDEFINITION: return "A definition of a FHIR structure. This resource is used to describe the underlying resources, data types defined in FHIR, and also for describing extensions and constraints on resources and data types."; 9410 case STRUCTUREMAP: return "A Map of relationships between 2 structures that can be used to transform data."; 9411 case SUBSCRIPTION: return "The subscription resource is used to define a push based subscription from a server to another system. Once a subscription is registered with the server, the server checks every resource that is created or updated, and if the resource matches the given criteria, it sends a message on the defined \"channel\" so that another system is able to take an appropriate action."; 9412 case SUBSTANCE: return "A homogeneous material with a definite composition."; 9413 case SUPPLYDELIVERY: return "Record of delivery of what is supplied."; 9414 case SUPPLYREQUEST: return "A record of a request for a medication, substance or device used in the healthcare setting."; 9415 case TASK: return "A task to be performed."; 9416 case TESTREPORT: return "A summary of information based on the results of executing a TestScript."; 9417 case TESTSCRIPT: return "A structured set of tests against a FHIR server implementation to determine compliance against the FHIR specification."; 9418 case VALUESET: return "A value set specifies a set of codes drawn from one or more code systems."; 9419 case VISIONPRESCRIPTION: return "An authorization for the supply of glasses and/or contact lenses to a patient."; 9420 case NULL: return null; 9421 default: return "?"; 9422 } 9423 } 9424 public String getDisplay() { 9425 switch (this) { 9426 case ACCOUNT: return "Account"; 9427 case ACTIVITYDEFINITION: return "ActivityDefinition"; 9428 case ADVERSEEVENT: return "AdverseEvent"; 9429 case ALLERGYINTOLERANCE: return "AllergyIntolerance"; 9430 case APPOINTMENT: return "Appointment"; 9431 case APPOINTMENTRESPONSE: return "AppointmentResponse"; 9432 case AUDITEVENT: return "AuditEvent"; 9433 case BASIC: return "Basic"; 9434 case BINARY: return "Binary"; 9435 case BODYSITE: return "BodySite"; 9436 case BUNDLE: return "Bundle"; 9437 case CAPABILITYSTATEMENT: return "CapabilityStatement"; 9438 case CAREPLAN: return "CarePlan"; 9439 case CARETEAM: return "CareTeam"; 9440 case CHARGEITEM: return "ChargeItem"; 9441 case CLAIM: return "Claim"; 9442 case CLAIMRESPONSE: return "ClaimResponse"; 9443 case CLINICALIMPRESSION: return "ClinicalImpression"; 9444 case CODESYSTEM: return "CodeSystem"; 9445 case COMMUNICATION: return "Communication"; 9446 case COMMUNICATIONREQUEST: return "CommunicationRequest"; 9447 case COMPARTMENTDEFINITION: return "CompartmentDefinition"; 9448 case COMPOSITION: return "Composition"; 9449 case CONCEPTMAP: return "ConceptMap"; 9450 case CONDITION: return "Condition"; 9451 case CONSENT: return "Consent"; 9452 case CONTRACT: return "Contract"; 9453 case COVERAGE: return "Coverage"; 9454 case DATAELEMENT: return "DataElement"; 9455 case DETECTEDISSUE: return "DetectedIssue"; 9456 case DEVICE: return "Device"; 9457 case DEVICECOMPONENT: return "DeviceComponent"; 9458 case DEVICEMETRIC: return "DeviceMetric"; 9459 case DEVICEREQUEST: return "DeviceRequest"; 9460 case DEVICEUSESTATEMENT: return "DeviceUseStatement"; 9461 case DIAGNOSTICREPORT: return "DiagnosticReport"; 9462 case DOCUMENTMANIFEST: return "DocumentManifest"; 9463 case DOCUMENTREFERENCE: return "DocumentReference"; 9464 case DOMAINRESOURCE: return "DomainResource"; 9465 case ELIGIBILITYREQUEST: return "EligibilityRequest"; 9466 case ELIGIBILITYRESPONSE: return "EligibilityResponse"; 9467 case ENCOUNTER: return "Encounter"; 9468 case ENDPOINT: return "Endpoint"; 9469 case ENROLLMENTREQUEST: return "EnrollmentRequest"; 9470 case ENROLLMENTRESPONSE: return "EnrollmentResponse"; 9471 case EPISODEOFCARE: return "EpisodeOfCare"; 9472 case EXPANSIONPROFILE: return "ExpansionProfile"; 9473 case EXPLANATIONOFBENEFIT: return "ExplanationOfBenefit"; 9474 case FAMILYMEMBERHISTORY: return "FamilyMemberHistory"; 9475 case FLAG: return "Flag"; 9476 case GOAL: return "Goal"; 9477 case GRAPHDEFINITION: return "GraphDefinition"; 9478 case GROUP: return "Group"; 9479 case GUIDANCERESPONSE: return "GuidanceResponse"; 9480 case HEALTHCARESERVICE: return "HealthcareService"; 9481 case IMAGINGMANIFEST: return "ImagingManifest"; 9482 case IMAGINGSTUDY: return "ImagingStudy"; 9483 case IMMUNIZATION: return "Immunization"; 9484 case IMMUNIZATIONRECOMMENDATION: return "ImmunizationRecommendation"; 9485 case IMPLEMENTATIONGUIDE: return "ImplementationGuide"; 9486 case LIBRARY: return "Library"; 9487 case LINKAGE: return "Linkage"; 9488 case LIST: return "List"; 9489 case LOCATION: return "Location"; 9490 case MEASURE: return "Measure"; 9491 case MEASUREREPORT: return "MeasureReport"; 9492 case MEDIA: return "Media"; 9493 case MEDICATION: return "Medication"; 9494 case MEDICATIONADMINISTRATION: return "MedicationAdministration"; 9495 case MEDICATIONDISPENSE: return "MedicationDispense"; 9496 case MEDICATIONREQUEST: return "MedicationRequest"; 9497 case MEDICATIONSTATEMENT: return "MedicationStatement"; 9498 case MESSAGEDEFINITION: return "MessageDefinition"; 9499 case MESSAGEHEADER: return "MessageHeader"; 9500 case NAMINGSYSTEM: return "NamingSystem"; 9501 case NUTRITIONORDER: return "NutritionOrder"; 9502 case OBSERVATION: return "Observation"; 9503 case OPERATIONDEFINITION: return "OperationDefinition"; 9504 case OPERATIONOUTCOME: return "OperationOutcome"; 9505 case ORGANIZATION: return "Organization"; 9506 case PARAMETERS: return "Parameters"; 9507 case PATIENT: return "Patient"; 9508 case PAYMENTNOTICE: return "PaymentNotice"; 9509 case PAYMENTRECONCILIATION: return "PaymentReconciliation"; 9510 case PERSON: return "Person"; 9511 case PLANDEFINITION: return "PlanDefinition"; 9512 case PRACTITIONER: return "Practitioner"; 9513 case PRACTITIONERROLE: return "PractitionerRole"; 9514 case PROCEDURE: return "Procedure"; 9515 case PROCEDUREREQUEST: return "ProcedureRequest"; 9516 case PROCESSREQUEST: return "ProcessRequest"; 9517 case PROCESSRESPONSE: return "ProcessResponse"; 9518 case PROVENANCE: return "Provenance"; 9519 case QUESTIONNAIRE: return "Questionnaire"; 9520 case QUESTIONNAIRERESPONSE: return "QuestionnaireResponse"; 9521 case REFERRALREQUEST: return "ReferralRequest"; 9522 case RELATEDPERSON: return "RelatedPerson"; 9523 case REQUESTGROUP: return "RequestGroup"; 9524 case RESEARCHSTUDY: return "ResearchStudy"; 9525 case RESEARCHSUBJECT: return "ResearchSubject"; 9526 case RESOURCE: return "Resource"; 9527 case RISKASSESSMENT: return "RiskAssessment"; 9528 case SCHEDULE: return "Schedule"; 9529 case SEARCHPARAMETER: return "SearchParameter"; 9530 case SEQUENCE: return "Sequence"; 9531 case SERVICEDEFINITION: return "ServiceDefinition"; 9532 case SLOT: return "Slot"; 9533 case SPECIMEN: return "Specimen"; 9534 case STRUCTUREDEFINITION: return "StructureDefinition"; 9535 case STRUCTUREMAP: return "StructureMap"; 9536 case SUBSCRIPTION: return "Subscription"; 9537 case SUBSTANCE: return "Substance"; 9538 case SUPPLYDELIVERY: return "SupplyDelivery"; 9539 case SUPPLYREQUEST: return "SupplyRequest"; 9540 case TASK: return "Task"; 9541 case TESTREPORT: return "TestReport"; 9542 case TESTSCRIPT: return "TestScript"; 9543 case VALUESET: return "ValueSet"; 9544 case VISIONPRESCRIPTION: return "VisionPrescription"; 9545 case NULL: return null; 9546 default: return "?"; 9547 } 9548 } 9549 } 9550 9551 public static class ResourceTypeEnumFactory implements EnumFactory<ResourceType> { 9552 public ResourceType fromCode(String codeString) throws IllegalArgumentException { 9553 if (codeString == null || "".equals(codeString)) 9554 if (codeString == null || "".equals(codeString)) 9555 return null; 9556 if ("Account".equals(codeString)) 9557 return ResourceType.ACCOUNT; 9558 if ("ActivityDefinition".equals(codeString)) 9559 return ResourceType.ACTIVITYDEFINITION; 9560 if ("AdverseEvent".equals(codeString)) 9561 return ResourceType.ADVERSEEVENT; 9562 if ("AllergyIntolerance".equals(codeString)) 9563 return ResourceType.ALLERGYINTOLERANCE; 9564 if ("Appointment".equals(codeString)) 9565 return ResourceType.APPOINTMENT; 9566 if ("AppointmentResponse".equals(codeString)) 9567 return ResourceType.APPOINTMENTRESPONSE; 9568 if ("AuditEvent".equals(codeString)) 9569 return ResourceType.AUDITEVENT; 9570 if ("Basic".equals(codeString)) 9571 return ResourceType.BASIC; 9572 if ("Binary".equals(codeString)) 9573 return ResourceType.BINARY; 9574 if ("BodySite".equals(codeString)) 9575 return ResourceType.BODYSITE; 9576 if ("Bundle".equals(codeString)) 9577 return ResourceType.BUNDLE; 9578 if ("CapabilityStatement".equals(codeString)) 9579 return ResourceType.CAPABILITYSTATEMENT; 9580 if ("CarePlan".equals(codeString)) 9581 return ResourceType.CAREPLAN; 9582 if ("CareTeam".equals(codeString)) 9583 return ResourceType.CARETEAM; 9584 if ("ChargeItem".equals(codeString)) 9585 return ResourceType.CHARGEITEM; 9586 if ("Claim".equals(codeString)) 9587 return ResourceType.CLAIM; 9588 if ("ClaimResponse".equals(codeString)) 9589 return ResourceType.CLAIMRESPONSE; 9590 if ("ClinicalImpression".equals(codeString)) 9591 return ResourceType.CLINICALIMPRESSION; 9592 if ("CodeSystem".equals(codeString)) 9593 return ResourceType.CODESYSTEM; 9594 if ("Communication".equals(codeString)) 9595 return ResourceType.COMMUNICATION; 9596 if ("CommunicationRequest".equals(codeString)) 9597 return ResourceType.COMMUNICATIONREQUEST; 9598 if ("CompartmentDefinition".equals(codeString)) 9599 return ResourceType.COMPARTMENTDEFINITION; 9600 if ("Composition".equals(codeString)) 9601 return ResourceType.COMPOSITION; 9602 if ("ConceptMap".equals(codeString)) 9603 return ResourceType.CONCEPTMAP; 9604 if ("Condition".equals(codeString)) 9605 return ResourceType.CONDITION; 9606 if ("Consent".equals(codeString)) 9607 return ResourceType.CONSENT; 9608 if ("Contract".equals(codeString)) 9609 return ResourceType.CONTRACT; 9610 if ("Coverage".equals(codeString)) 9611 return ResourceType.COVERAGE; 9612 if ("DataElement".equals(codeString)) 9613 return ResourceType.DATAELEMENT; 9614 if ("DetectedIssue".equals(codeString)) 9615 return ResourceType.DETECTEDISSUE; 9616 if ("Device".equals(codeString)) 9617 return ResourceType.DEVICE; 9618 if ("DeviceComponent".equals(codeString)) 9619 return ResourceType.DEVICECOMPONENT; 9620 if ("DeviceMetric".equals(codeString)) 9621 return ResourceType.DEVICEMETRIC; 9622 if ("DeviceRequest".equals(codeString)) 9623 return ResourceType.DEVICEREQUEST; 9624 if ("DeviceUseStatement".equals(codeString)) 9625 return ResourceType.DEVICEUSESTATEMENT; 9626 if ("DiagnosticReport".equals(codeString)) 9627 return ResourceType.DIAGNOSTICREPORT; 9628 if ("DocumentManifest".equals(codeString)) 9629 return ResourceType.DOCUMENTMANIFEST; 9630 if ("DocumentReference".equals(codeString)) 9631 return ResourceType.DOCUMENTREFERENCE; 9632 if ("DomainResource".equals(codeString)) 9633 return ResourceType.DOMAINRESOURCE; 9634 if ("EligibilityRequest".equals(codeString)) 9635 return ResourceType.ELIGIBILITYREQUEST; 9636 if ("EligibilityResponse".equals(codeString)) 9637 return ResourceType.ELIGIBILITYRESPONSE; 9638 if ("Encounter".equals(codeString)) 9639 return ResourceType.ENCOUNTER; 9640 if ("Endpoint".equals(codeString)) 9641 return ResourceType.ENDPOINT; 9642 if ("EnrollmentRequest".equals(codeString)) 9643 return ResourceType.ENROLLMENTREQUEST; 9644 if ("EnrollmentResponse".equals(codeString)) 9645 return ResourceType.ENROLLMENTRESPONSE; 9646 if ("EpisodeOfCare".equals(codeString)) 9647 return ResourceType.EPISODEOFCARE; 9648 if ("ExpansionProfile".equals(codeString)) 9649 return ResourceType.EXPANSIONPROFILE; 9650 if ("ExplanationOfBenefit".equals(codeString)) 9651 return ResourceType.EXPLANATIONOFBENEFIT; 9652 if ("FamilyMemberHistory".equals(codeString)) 9653 return ResourceType.FAMILYMEMBERHISTORY; 9654 if ("Flag".equals(codeString)) 9655 return ResourceType.FLAG; 9656 if ("Goal".equals(codeString)) 9657 return ResourceType.GOAL; 9658 if ("GraphDefinition".equals(codeString)) 9659 return ResourceType.GRAPHDEFINITION; 9660 if ("Group".equals(codeString)) 9661 return ResourceType.GROUP; 9662 if ("GuidanceResponse".equals(codeString)) 9663 return ResourceType.GUIDANCERESPONSE; 9664 if ("HealthcareService".equals(codeString)) 9665 return ResourceType.HEALTHCARESERVICE; 9666 if ("ImagingManifest".equals(codeString)) 9667 return ResourceType.IMAGINGMANIFEST; 9668 if ("ImagingStudy".equals(codeString)) 9669 return ResourceType.IMAGINGSTUDY; 9670 if ("Immunization".equals(codeString)) 9671 return ResourceType.IMMUNIZATION; 9672 if ("ImmunizationRecommendation".equals(codeString)) 9673 return ResourceType.IMMUNIZATIONRECOMMENDATION; 9674 if ("ImplementationGuide".equals(codeString)) 9675 return ResourceType.IMPLEMENTATIONGUIDE; 9676 if ("Library".equals(codeString)) 9677 return ResourceType.LIBRARY; 9678 if ("Linkage".equals(codeString)) 9679 return ResourceType.LINKAGE; 9680 if ("List".equals(codeString)) 9681 return ResourceType.LIST; 9682 if ("Location".equals(codeString)) 9683 return ResourceType.LOCATION; 9684 if ("Measure".equals(codeString)) 9685 return ResourceType.MEASURE; 9686 if ("MeasureReport".equals(codeString)) 9687 return ResourceType.MEASUREREPORT; 9688 if ("Media".equals(codeString)) 9689 return ResourceType.MEDIA; 9690 if ("Medication".equals(codeString)) 9691 return ResourceType.MEDICATION; 9692 if ("MedicationAdministration".equals(codeString)) 9693 return ResourceType.MEDICATIONADMINISTRATION; 9694 if ("MedicationDispense".equals(codeString)) 9695 return ResourceType.MEDICATIONDISPENSE; 9696 if ("MedicationRequest".equals(codeString)) 9697 return ResourceType.MEDICATIONREQUEST; 9698 if ("MedicationStatement".equals(codeString)) 9699 return ResourceType.MEDICATIONSTATEMENT; 9700 if ("MessageDefinition".equals(codeString)) 9701 return ResourceType.MESSAGEDEFINITION; 9702 if ("MessageHeader".equals(codeString)) 9703 return ResourceType.MESSAGEHEADER; 9704 if ("NamingSystem".equals(codeString)) 9705 return ResourceType.NAMINGSYSTEM; 9706 if ("NutritionOrder".equals(codeString)) 9707 return ResourceType.NUTRITIONORDER; 9708 if ("Observation".equals(codeString)) 9709 return ResourceType.OBSERVATION; 9710 if ("OperationDefinition".equals(codeString)) 9711 return ResourceType.OPERATIONDEFINITION; 9712 if ("OperationOutcome".equals(codeString)) 9713 return ResourceType.OPERATIONOUTCOME; 9714 if ("Organization".equals(codeString)) 9715 return ResourceType.ORGANIZATION; 9716 if ("Parameters".equals(codeString)) 9717 return ResourceType.PARAMETERS; 9718 if ("Patient".equals(codeString)) 9719 return ResourceType.PATIENT; 9720 if ("PaymentNotice".equals(codeString)) 9721 return ResourceType.PAYMENTNOTICE; 9722 if ("PaymentReconciliation".equals(codeString)) 9723 return ResourceType.PAYMENTRECONCILIATION; 9724 if ("Person".equals(codeString)) 9725 return ResourceType.PERSON; 9726 if ("PlanDefinition".equals(codeString)) 9727 return ResourceType.PLANDEFINITION; 9728 if ("Practitioner".equals(codeString)) 9729 return ResourceType.PRACTITIONER; 9730 if ("PractitionerRole".equals(codeString)) 9731 return ResourceType.PRACTITIONERROLE; 9732 if ("Procedure".equals(codeString)) 9733 return ResourceType.PROCEDURE; 9734 if ("ProcedureRequest".equals(codeString)) 9735 return ResourceType.PROCEDUREREQUEST; 9736 if ("ProcessRequest".equals(codeString)) 9737 return ResourceType.PROCESSREQUEST; 9738 if ("ProcessResponse".equals(codeString)) 9739 return ResourceType.PROCESSRESPONSE; 9740 if ("Provenance".equals(codeString)) 9741 return ResourceType.PROVENANCE; 9742 if ("Questionnaire".equals(codeString)) 9743 return ResourceType.QUESTIONNAIRE; 9744 if ("QuestionnaireResponse".equals(codeString)) 9745 return ResourceType.QUESTIONNAIRERESPONSE; 9746 if ("ReferralRequest".equals(codeString)) 9747 return ResourceType.REFERRALREQUEST; 9748 if ("RelatedPerson".equals(codeString)) 9749 return ResourceType.RELATEDPERSON; 9750 if ("RequestGroup".equals(codeString)) 9751 return ResourceType.REQUESTGROUP; 9752 if ("ResearchStudy".equals(codeString)) 9753 return ResourceType.RESEARCHSTUDY; 9754 if ("ResearchSubject".equals(codeString)) 9755 return ResourceType.RESEARCHSUBJECT; 9756 if ("Resource".equals(codeString)) 9757 return ResourceType.RESOURCE; 9758 if ("RiskAssessment".equals(codeString)) 9759 return ResourceType.RISKASSESSMENT; 9760 if ("Schedule".equals(codeString)) 9761 return ResourceType.SCHEDULE; 9762 if ("SearchParameter".equals(codeString)) 9763 return ResourceType.SEARCHPARAMETER; 9764 if ("Sequence".equals(codeString)) 9765 return ResourceType.SEQUENCE; 9766 if ("ServiceDefinition".equals(codeString)) 9767 return ResourceType.SERVICEDEFINITION; 9768 if ("Slot".equals(codeString)) 9769 return ResourceType.SLOT; 9770 if ("Specimen".equals(codeString)) 9771 return ResourceType.SPECIMEN; 9772 if ("StructureDefinition".equals(codeString)) 9773 return ResourceType.STRUCTUREDEFINITION; 9774 if ("StructureMap".equals(codeString)) 9775 return ResourceType.STRUCTUREMAP; 9776 if ("Subscription".equals(codeString)) 9777 return ResourceType.SUBSCRIPTION; 9778 if ("Substance".equals(codeString)) 9779 return ResourceType.SUBSTANCE; 9780 if ("SupplyDelivery".equals(codeString)) 9781 return ResourceType.SUPPLYDELIVERY; 9782 if ("SupplyRequest".equals(codeString)) 9783 return ResourceType.SUPPLYREQUEST; 9784 if ("Task".equals(codeString)) 9785 return ResourceType.TASK; 9786 if ("TestReport".equals(codeString)) 9787 return ResourceType.TESTREPORT; 9788 if ("TestScript".equals(codeString)) 9789 return ResourceType.TESTSCRIPT; 9790 if ("ValueSet".equals(codeString)) 9791 return ResourceType.VALUESET; 9792 if ("VisionPrescription".equals(codeString)) 9793 return ResourceType.VISIONPRESCRIPTION; 9794 throw new IllegalArgumentException("Unknown ResourceType code '"+codeString+"'"); 9795 } 9796 public Enumeration<ResourceType> fromType(PrimitiveType<?> code) throws FHIRException { 9797 if (code == null) 9798 return null; 9799 if (code.isEmpty()) 9800 return new Enumeration<ResourceType>(this); 9801 String codeString = code.asStringValue(); 9802 if (codeString == null || "".equals(codeString)) 9803 return null; 9804 if ("Account".equals(codeString)) 9805 return new Enumeration<ResourceType>(this, ResourceType.ACCOUNT); 9806 if ("ActivityDefinition".equals(codeString)) 9807 return new Enumeration<ResourceType>(this, ResourceType.ACTIVITYDEFINITION); 9808 if ("AdverseEvent".equals(codeString)) 9809 return new Enumeration<ResourceType>(this, ResourceType.ADVERSEEVENT); 9810 if ("AllergyIntolerance".equals(codeString)) 9811 return new Enumeration<ResourceType>(this, ResourceType.ALLERGYINTOLERANCE); 9812 if ("Appointment".equals(codeString)) 9813 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENT); 9814 if ("AppointmentResponse".equals(codeString)) 9815 return new Enumeration<ResourceType>(this, ResourceType.APPOINTMENTRESPONSE); 9816 if ("AuditEvent".equals(codeString)) 9817 return new Enumeration<ResourceType>(this, ResourceType.AUDITEVENT); 9818 if ("Basic".equals(codeString)) 9819 return new Enumeration<ResourceType>(this, ResourceType.BASIC); 9820 if ("Binary".equals(codeString)) 9821 return new Enumeration<ResourceType>(this, ResourceType.BINARY); 9822 if ("BodySite".equals(codeString)) 9823 return new Enumeration<ResourceType>(this, ResourceType.BODYSITE); 9824 if ("Bundle".equals(codeString)) 9825 return new Enumeration<ResourceType>(this, ResourceType.BUNDLE); 9826 if ("CapabilityStatement".equals(codeString)) 9827 return new Enumeration<ResourceType>(this, ResourceType.CAPABILITYSTATEMENT); 9828 if ("CarePlan".equals(codeString)) 9829 return new Enumeration<ResourceType>(this, ResourceType.CAREPLAN); 9830 if ("CareTeam".equals(codeString)) 9831 return new Enumeration<ResourceType>(this, ResourceType.CARETEAM); 9832 if ("ChargeItem".equals(codeString)) 9833 return new Enumeration<ResourceType>(this, ResourceType.CHARGEITEM); 9834 if ("Claim".equals(codeString)) 9835 return new Enumeration<ResourceType>(this, ResourceType.CLAIM); 9836 if ("ClaimResponse".equals(codeString)) 9837 return new Enumeration<ResourceType>(this, ResourceType.CLAIMRESPONSE); 9838 if ("ClinicalImpression".equals(codeString)) 9839 return new Enumeration<ResourceType>(this, ResourceType.CLINICALIMPRESSION); 9840 if ("CodeSystem".equals(codeString)) 9841 return new Enumeration<ResourceType>(this, ResourceType.CODESYSTEM); 9842 if ("Communication".equals(codeString)) 9843 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATION); 9844 if ("CommunicationRequest".equals(codeString)) 9845 return new Enumeration<ResourceType>(this, ResourceType.COMMUNICATIONREQUEST); 9846 if ("CompartmentDefinition".equals(codeString)) 9847 return new Enumeration<ResourceType>(this, ResourceType.COMPARTMENTDEFINITION); 9848 if ("Composition".equals(codeString)) 9849 return new Enumeration<ResourceType>(this, ResourceType.COMPOSITION); 9850 if ("ConceptMap".equals(codeString)) 9851 return new Enumeration<ResourceType>(this, ResourceType.CONCEPTMAP); 9852 if ("Condition".equals(codeString)) 9853 return new Enumeration<ResourceType>(this, ResourceType.CONDITION); 9854 if ("Consent".equals(codeString)) 9855 return new Enumeration<ResourceType>(this, ResourceType.CONSENT); 9856 if ("Contract".equals(codeString)) 9857 return new Enumeration<ResourceType>(this, ResourceType.CONTRACT); 9858 if ("Coverage".equals(codeString)) 9859 return new Enumeration<ResourceType>(this, ResourceType.COVERAGE); 9860 if ("DataElement".equals(codeString)) 9861 return new Enumeration<ResourceType>(this, ResourceType.DATAELEMENT); 9862 if ("DetectedIssue".equals(codeString)) 9863 return new Enumeration<ResourceType>(this, ResourceType.DETECTEDISSUE); 9864 if ("Device".equals(codeString)) 9865 return new Enumeration<ResourceType>(this, ResourceType.DEVICE); 9866 if ("DeviceComponent".equals(codeString)) 9867 return new Enumeration<ResourceType>(this, ResourceType.DEVICECOMPONENT); 9868 if ("DeviceMetric".equals(codeString)) 9869 return new Enumeration<ResourceType>(this, ResourceType.DEVICEMETRIC); 9870 if ("DeviceRequest".equals(codeString)) 9871 return new Enumeration<ResourceType>(this, ResourceType.DEVICEREQUEST); 9872 if ("DeviceUseStatement".equals(codeString)) 9873 return new Enumeration<ResourceType>(this, ResourceType.DEVICEUSESTATEMENT); 9874 if ("DiagnosticReport".equals(codeString)) 9875 return new Enumeration<ResourceType>(this, ResourceType.DIAGNOSTICREPORT); 9876 if ("DocumentManifest".equals(codeString)) 9877 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTMANIFEST); 9878 if ("DocumentReference".equals(codeString)) 9879 return new Enumeration<ResourceType>(this, ResourceType.DOCUMENTREFERENCE); 9880 if ("DomainResource".equals(codeString)) 9881 return new Enumeration<ResourceType>(this, ResourceType.DOMAINRESOURCE); 9882 if ("EligibilityRequest".equals(codeString)) 9883 return new Enumeration<ResourceType>(this, ResourceType.ELIGIBILITYREQUEST); 9884 if ("EligibilityResponse".equals(codeString)) 9885 return new Enumeration<ResourceType>(this, ResourceType.ELIGIBILITYRESPONSE); 9886 if ("Encounter".equals(codeString)) 9887 return new Enumeration<ResourceType>(this, ResourceType.ENCOUNTER); 9888 if ("Endpoint".equals(codeString)) 9889 return new Enumeration<ResourceType>(this, ResourceType.ENDPOINT); 9890 if ("EnrollmentRequest".equals(codeString)) 9891 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTREQUEST); 9892 if ("EnrollmentResponse".equals(codeString)) 9893 return new Enumeration<ResourceType>(this, ResourceType.ENROLLMENTRESPONSE); 9894 if ("EpisodeOfCare".equals(codeString)) 9895 return new Enumeration<ResourceType>(this, ResourceType.EPISODEOFCARE); 9896 if ("ExpansionProfile".equals(codeString)) 9897 return new Enumeration<ResourceType>(this, ResourceType.EXPANSIONPROFILE); 9898 if ("ExplanationOfBenefit".equals(codeString)) 9899 return new Enumeration<ResourceType>(this, ResourceType.EXPLANATIONOFBENEFIT); 9900 if ("FamilyMemberHistory".equals(codeString)) 9901 return new Enumeration<ResourceType>(this, ResourceType.FAMILYMEMBERHISTORY); 9902 if ("Flag".equals(codeString)) 9903 return new Enumeration<ResourceType>(this, ResourceType.FLAG); 9904 if ("Goal".equals(codeString)) 9905 return new Enumeration<ResourceType>(this, ResourceType.GOAL); 9906 if ("GraphDefinition".equals(codeString)) 9907 return new Enumeration<ResourceType>(this, ResourceType.GRAPHDEFINITION); 9908 if ("Group".equals(codeString)) 9909 return new Enumeration<ResourceType>(this, ResourceType.GROUP); 9910 if ("GuidanceResponse".equals(codeString)) 9911 return new Enumeration<ResourceType>(this, ResourceType.GUIDANCERESPONSE); 9912 if ("HealthcareService".equals(codeString)) 9913 return new Enumeration<ResourceType>(this, ResourceType.HEALTHCARESERVICE); 9914 if ("ImagingManifest".equals(codeString)) 9915 return new Enumeration<ResourceType>(this, ResourceType.IMAGINGMANIFEST); 9916 if ("ImagingStudy".equals(codeString)) 9917 return new Enumeration<ResourceType>(this, ResourceType.IMAGINGSTUDY); 9918 if ("Immunization".equals(codeString)) 9919 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATION); 9920 if ("ImmunizationRecommendation".equals(codeString)) 9921 return new Enumeration<ResourceType>(this, ResourceType.IMMUNIZATIONRECOMMENDATION); 9922 if ("ImplementationGuide".equals(codeString)) 9923 return new Enumeration<ResourceType>(this, ResourceType.IMPLEMENTATIONGUIDE); 9924 if ("Library".equals(codeString)) 9925 return new Enumeration<ResourceType>(this, ResourceType.LIBRARY); 9926 if ("Linkage".equals(codeString)) 9927 return new Enumeration<ResourceType>(this, ResourceType.LINKAGE); 9928 if ("List".equals(codeString)) 9929 return new Enumeration<ResourceType>(this, ResourceType.LIST); 9930 if ("Location".equals(codeString)) 9931 return new Enumeration<ResourceType>(this, ResourceType.LOCATION); 9932 if ("Measure".equals(codeString)) 9933 return new Enumeration<ResourceType>(this, ResourceType.MEASURE); 9934 if ("MeasureReport".equals(codeString)) 9935 return new Enumeration<ResourceType>(this, ResourceType.MEASUREREPORT); 9936 if ("Media".equals(codeString)) 9937 return new Enumeration<ResourceType>(this, ResourceType.MEDIA); 9938 if ("Medication".equals(codeString)) 9939 return new Enumeration<ResourceType>(this, ResourceType.MEDICATION); 9940 if ("MedicationAdministration".equals(codeString)) 9941 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONADMINISTRATION); 9942 if ("MedicationDispense".equals(codeString)) 9943 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONDISPENSE); 9944 if ("MedicationRequest".equals(codeString)) 9945 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONREQUEST); 9946 if ("MedicationStatement".equals(codeString)) 9947 return new Enumeration<ResourceType>(this, ResourceType.MEDICATIONSTATEMENT); 9948 if ("MessageDefinition".equals(codeString)) 9949 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEDEFINITION); 9950 if ("MessageHeader".equals(codeString)) 9951 return new Enumeration<ResourceType>(this, ResourceType.MESSAGEHEADER); 9952 if ("NamingSystem".equals(codeString)) 9953 return new Enumeration<ResourceType>(this, ResourceType.NAMINGSYSTEM); 9954 if ("NutritionOrder".equals(codeString)) 9955 return new Enumeration<ResourceType>(this, ResourceType.NUTRITIONORDER); 9956 if ("Observation".equals(codeString)) 9957 return new Enumeration<ResourceType>(this, ResourceType.OBSERVATION); 9958 if ("OperationDefinition".equals(codeString)) 9959 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONDEFINITION); 9960 if ("OperationOutcome".equals(codeString)) 9961 return new Enumeration<ResourceType>(this, ResourceType.OPERATIONOUTCOME); 9962 if ("Organization".equals(codeString)) 9963 return new Enumeration<ResourceType>(this, ResourceType.ORGANIZATION); 9964 if ("Parameters".equals(codeString)) 9965 return new Enumeration<ResourceType>(this, ResourceType.PARAMETERS); 9966 if ("Patient".equals(codeString)) 9967 return new Enumeration<ResourceType>(this, ResourceType.PATIENT); 9968 if ("PaymentNotice".equals(codeString)) 9969 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTNOTICE); 9970 if ("PaymentReconciliation".equals(codeString)) 9971 return new Enumeration<ResourceType>(this, ResourceType.PAYMENTRECONCILIATION); 9972 if ("Person".equals(codeString)) 9973 return new Enumeration<ResourceType>(this, ResourceType.PERSON); 9974 if ("PlanDefinition".equals(codeString)) 9975 return new Enumeration<ResourceType>(this, ResourceType.PLANDEFINITION); 9976 if ("Practitioner".equals(codeString)) 9977 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONER); 9978 if ("PractitionerRole".equals(codeString)) 9979 return new Enumeration<ResourceType>(this, ResourceType.PRACTITIONERROLE); 9980 if ("Procedure".equals(codeString)) 9981 return new Enumeration<ResourceType>(this, ResourceType.PROCEDURE); 9982 if ("ProcedureRequest".equals(codeString)) 9983 return new Enumeration<ResourceType>(this, ResourceType.PROCEDUREREQUEST); 9984 if ("ProcessRequest".equals(codeString)) 9985 return new Enumeration<ResourceType>(this, ResourceType.PROCESSREQUEST); 9986 if ("ProcessResponse".equals(codeString)) 9987 return new Enumeration<ResourceType>(this, ResourceType.PROCESSRESPONSE); 9988 if ("Provenance".equals(codeString)) 9989 return new Enumeration<ResourceType>(this, ResourceType.PROVENANCE); 9990 if ("Questionnaire".equals(codeString)) 9991 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRE); 9992 if ("QuestionnaireResponse".equals(codeString)) 9993 return new Enumeration<ResourceType>(this, ResourceType.QUESTIONNAIRERESPONSE); 9994 if ("ReferralRequest".equals(codeString)) 9995 return new Enumeration<ResourceType>(this, ResourceType.REFERRALREQUEST); 9996 if ("RelatedPerson".equals(codeString)) 9997 return new Enumeration<ResourceType>(this, ResourceType.RELATEDPERSON); 9998 if ("RequestGroup".equals(codeString)) 9999 return new Enumeration<ResourceType>(this, ResourceType.REQUESTGROUP); 10000 if ("ResearchStudy".equals(codeString)) 10001 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHSTUDY); 10002 if ("ResearchSubject".equals(codeString)) 10003 return new Enumeration<ResourceType>(this, ResourceType.RESEARCHSUBJECT); 10004 if ("Resource".equals(codeString)) 10005 return new Enumeration<ResourceType>(this, ResourceType.RESOURCE); 10006 if ("RiskAssessment".equals(codeString)) 10007 return new Enumeration<ResourceType>(this, ResourceType.RISKASSESSMENT); 10008 if ("Schedule".equals(codeString)) 10009 return new Enumeration<ResourceType>(this, ResourceType.SCHEDULE); 10010 if ("SearchParameter".equals(codeString)) 10011 return new Enumeration<ResourceType>(this, ResourceType.SEARCHPARAMETER); 10012 if ("Sequence".equals(codeString)) 10013 return new Enumeration<ResourceType>(this, ResourceType.SEQUENCE); 10014 if ("ServiceDefinition".equals(codeString)) 10015 return new Enumeration<ResourceType>(this, ResourceType.SERVICEDEFINITION); 10016 if ("Slot".equals(codeString)) 10017 return new Enumeration<ResourceType>(this, ResourceType.SLOT); 10018 if ("Specimen".equals(codeString)) 10019 return new Enumeration<ResourceType>(this, ResourceType.SPECIMEN); 10020 if ("StructureDefinition".equals(codeString)) 10021 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREDEFINITION); 10022 if ("StructureMap".equals(codeString)) 10023 return new Enumeration<ResourceType>(this, ResourceType.STRUCTUREMAP); 10024 if ("Subscription".equals(codeString)) 10025 return new Enumeration<ResourceType>(this, ResourceType.SUBSCRIPTION); 10026 if ("Substance".equals(codeString)) 10027 return new Enumeration<ResourceType>(this, ResourceType.SUBSTANCE); 10028 if ("SupplyDelivery".equals(codeString)) 10029 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYDELIVERY); 10030 if ("SupplyRequest".equals(codeString)) 10031 return new Enumeration<ResourceType>(this, ResourceType.SUPPLYREQUEST); 10032 if ("Task".equals(codeString)) 10033 return new Enumeration<ResourceType>(this, ResourceType.TASK); 10034 if ("TestReport".equals(codeString)) 10035 return new Enumeration<ResourceType>(this, ResourceType.TESTREPORT); 10036 if ("TestScript".equals(codeString)) 10037 return new Enumeration<ResourceType>(this, ResourceType.TESTSCRIPT); 10038 if ("ValueSet".equals(codeString)) 10039 return new Enumeration<ResourceType>(this, ResourceType.VALUESET); 10040 if ("VisionPrescription".equals(codeString)) 10041 return new Enumeration<ResourceType>(this, ResourceType.VISIONPRESCRIPTION); 10042 throw new FHIRException("Unknown ResourceType code '"+codeString+"'"); 10043 } 10044 public String toCode(ResourceType code) { 10045 if (code == ResourceType.ACCOUNT) 10046 return "Account"; 10047 if (code == ResourceType.ACTIVITYDEFINITION) 10048 return "ActivityDefinition"; 10049 if (code == ResourceType.ADVERSEEVENT) 10050 return "AdverseEvent"; 10051 if (code == ResourceType.ALLERGYINTOLERANCE) 10052 return "AllergyIntolerance"; 10053 if (code == ResourceType.APPOINTMENT) 10054 return "Appointment"; 10055 if (code == ResourceType.APPOINTMENTRESPONSE) 10056 return "AppointmentResponse"; 10057 if (code == ResourceType.AUDITEVENT) 10058 return "AuditEvent"; 10059 if (code == ResourceType.BASIC) 10060 return "Basic"; 10061 if (code == ResourceType.BINARY) 10062 return "Binary"; 10063 if (code == ResourceType.BODYSITE) 10064 return "BodySite"; 10065 if (code == ResourceType.BUNDLE) 10066 return "Bundle"; 10067 if (code == ResourceType.CAPABILITYSTATEMENT) 10068 return "CapabilityStatement"; 10069 if (code == ResourceType.CAREPLAN) 10070 return "CarePlan"; 10071 if (code == ResourceType.CARETEAM) 10072 return "CareTeam"; 10073 if (code == ResourceType.CHARGEITEM) 10074 return "ChargeItem"; 10075 if (code == ResourceType.CLAIM) 10076 return "Claim"; 10077 if (code == ResourceType.CLAIMRESPONSE) 10078 return "ClaimResponse"; 10079 if (code == ResourceType.CLINICALIMPRESSION) 10080 return "ClinicalImpression"; 10081 if (code == ResourceType.CODESYSTEM) 10082 return "CodeSystem"; 10083 if (code == ResourceType.COMMUNICATION) 10084 return "Communication"; 10085 if (code == ResourceType.COMMUNICATIONREQUEST) 10086 return "CommunicationRequest"; 10087 if (code == ResourceType.COMPARTMENTDEFINITION) 10088 return "CompartmentDefinition"; 10089 if (code == ResourceType.COMPOSITION) 10090 return "Composition"; 10091 if (code == ResourceType.CONCEPTMAP) 10092 return "ConceptMap"; 10093 if (code == ResourceType.CONDITION) 10094 return "Condition"; 10095 if (code == ResourceType.CONSENT) 10096 return "Consent"; 10097 if (code == ResourceType.CONTRACT) 10098 return "Contract"; 10099 if (code == ResourceType.COVERAGE) 10100 return "Coverage"; 10101 if (code == ResourceType.DATAELEMENT) 10102 return "DataElement"; 10103 if (code == ResourceType.DETECTEDISSUE) 10104 return "DetectedIssue"; 10105 if (code == ResourceType.DEVICE) 10106 return "Device"; 10107 if (code == ResourceType.DEVICECOMPONENT) 10108 return "DeviceComponent"; 10109 if (code == ResourceType.DEVICEMETRIC) 10110 return "DeviceMetric"; 10111 if (code == ResourceType.DEVICEREQUEST) 10112 return "DeviceRequest"; 10113 if (code == ResourceType.DEVICEUSESTATEMENT) 10114 return "DeviceUseStatement"; 10115 if (code == ResourceType.DIAGNOSTICREPORT) 10116 return "DiagnosticReport"; 10117 if (code == ResourceType.DOCUMENTMANIFEST) 10118 return "DocumentManifest"; 10119 if (code == ResourceType.DOCUMENTREFERENCE) 10120 return "DocumentReference"; 10121 if (code == ResourceType.DOMAINRESOURCE) 10122 return "DomainResource"; 10123 if (code == ResourceType.ELIGIBILITYREQUEST) 10124 return "EligibilityRequest"; 10125 if (code == ResourceType.ELIGIBILITYRESPONSE) 10126 return "EligibilityResponse"; 10127 if (code == ResourceType.ENCOUNTER) 10128 return "Encounter"; 10129 if (code == ResourceType.ENDPOINT) 10130 return "Endpoint"; 10131 if (code == ResourceType.ENROLLMENTREQUEST) 10132 return "EnrollmentRequest"; 10133 if (code == ResourceType.ENROLLMENTRESPONSE) 10134 return "EnrollmentResponse"; 10135 if (code == ResourceType.EPISODEOFCARE) 10136 return "EpisodeOfCare"; 10137 if (code == ResourceType.EXPANSIONPROFILE) 10138 return "ExpansionProfile"; 10139 if (code == ResourceType.EXPLANATIONOFBENEFIT) 10140 return "ExplanationOfBenefit"; 10141 if (code == ResourceType.FAMILYMEMBERHISTORY) 10142 return "FamilyMemberHistory"; 10143 if (code == ResourceType.FLAG) 10144 return "Flag"; 10145 if (code == ResourceType.GOAL) 10146 return "Goal"; 10147 if (code == ResourceType.GRAPHDEFINITION) 10148 return "GraphDefinition"; 10149 if (code == ResourceType.GROUP) 10150 return "Group"; 10151 if (code == ResourceType.GUIDANCERESPONSE) 10152 return "GuidanceResponse"; 10153 if (code == ResourceType.HEALTHCARESERVICE) 10154 return "HealthcareService"; 10155 if (code == ResourceType.IMAGINGMANIFEST) 10156 return "ImagingManifest"; 10157 if (code == ResourceType.IMAGINGSTUDY) 10158 return "ImagingStudy"; 10159 if (code == ResourceType.IMMUNIZATION) 10160 return "Immunization"; 10161 if (code == ResourceType.IMMUNIZATIONRECOMMENDATION) 10162 return "ImmunizationRecommendation"; 10163 if (code == ResourceType.IMPLEMENTATIONGUIDE) 10164 return "ImplementationGuide"; 10165 if (code == ResourceType.LIBRARY) 10166 return "Library"; 10167 if (code == ResourceType.LINKAGE) 10168 return "Linkage"; 10169 if (code == ResourceType.LIST) 10170 return "List"; 10171 if (code == ResourceType.LOCATION) 10172 return "Location"; 10173 if (code == ResourceType.MEASURE) 10174 return "Measure"; 10175 if (code == ResourceType.MEASUREREPORT) 10176 return "MeasureReport"; 10177 if (code == ResourceType.MEDIA) 10178 return "Media"; 10179 if (code == ResourceType.MEDICATION) 10180 return "Medication"; 10181 if (code == ResourceType.MEDICATIONADMINISTRATION) 10182 return "MedicationAdministration"; 10183 if (code == ResourceType.MEDICATIONDISPENSE) 10184 return "MedicationDispense"; 10185 if (code == ResourceType.MEDICATIONREQUEST) 10186 return "MedicationRequest"; 10187 if (code == ResourceType.MEDICATIONSTATEMENT) 10188 return "MedicationStatement"; 10189 if (code == ResourceType.MESSAGEDEFINITION) 10190 return "MessageDefinition"; 10191 if (code == ResourceType.MESSAGEHEADER) 10192 return "MessageHeader"; 10193 if (code == ResourceType.NAMINGSYSTEM) 10194 return "NamingSystem"; 10195 if (code == ResourceType.NUTRITIONORDER) 10196 return "NutritionOrder"; 10197 if (code == ResourceType.OBSERVATION) 10198 return "Observation"; 10199 if (code == ResourceType.OPERATIONDEFINITION) 10200 return "OperationDefinition"; 10201 if (code == ResourceType.OPERATIONOUTCOME) 10202 return "OperationOutcome"; 10203 if (code == ResourceType.ORGANIZATION) 10204 return "Organization"; 10205 if (code == ResourceType.PARAMETERS) 10206 return "Parameters"; 10207 if (code == ResourceType.PATIENT) 10208 return "Patient"; 10209 if (code == ResourceType.PAYMENTNOTICE) 10210 return "PaymentNotice"; 10211 if (code == ResourceType.PAYMENTRECONCILIATION) 10212 return "PaymentReconciliation"; 10213 if (code == ResourceType.PERSON) 10214 return "Person"; 10215 if (code == ResourceType.PLANDEFINITION) 10216 return "PlanDefinition"; 10217 if (code == ResourceType.PRACTITIONER) 10218 return "Practitioner"; 10219 if (code == ResourceType.PRACTITIONERROLE) 10220 return "PractitionerRole"; 10221 if (code == ResourceType.PROCEDURE) 10222 return "Procedure"; 10223 if (code == ResourceType.PROCEDUREREQUEST) 10224 return "ProcedureRequest"; 10225 if (code == ResourceType.PROCESSREQUEST) 10226 return "ProcessRequest"; 10227 if (code == ResourceType.PROCESSRESPONSE) 10228 return "ProcessResponse"; 10229 if (code == ResourceType.PROVENANCE) 10230 return "Provenance"; 10231 if (code == ResourceType.QUESTIONNAIRE) 10232 return "Questionnaire"; 10233 if (code == ResourceType.QUESTIONNAIRERESPONSE) 10234 return "QuestionnaireResponse"; 10235 if (code == ResourceType.REFERRALREQUEST) 10236 return "ReferralRequest"; 10237 if (code == ResourceType.RELATEDPERSON) 10238 return "RelatedPerson"; 10239 if (code == ResourceType.REQUESTGROUP) 10240 return "RequestGroup"; 10241 if (code == ResourceType.RESEARCHSTUDY) 10242 return "ResearchStudy"; 10243 if (code == ResourceType.RESEARCHSUBJECT) 10244 return "ResearchSubject"; 10245 if (code == ResourceType.RESOURCE) 10246 return "Resource"; 10247 if (code == ResourceType.RISKASSESSMENT) 10248 return "RiskAssessment"; 10249 if (code == ResourceType.SCHEDULE) 10250 return "Schedule"; 10251 if (code == ResourceType.SEARCHPARAMETER) 10252 return "SearchParameter"; 10253 if (code == ResourceType.SEQUENCE) 10254 return "Sequence"; 10255 if (code == ResourceType.SERVICEDEFINITION) 10256 return "ServiceDefinition"; 10257 if (code == ResourceType.SLOT) 10258 return "Slot"; 10259 if (code == ResourceType.SPECIMEN) 10260 return "Specimen"; 10261 if (code == ResourceType.STRUCTUREDEFINITION) 10262 return "StructureDefinition"; 10263 if (code == ResourceType.STRUCTUREMAP) 10264 return "StructureMap"; 10265 if (code == ResourceType.SUBSCRIPTION) 10266 return "Subscription"; 10267 if (code == ResourceType.SUBSTANCE) 10268 return "Substance"; 10269 if (code == ResourceType.SUPPLYDELIVERY) 10270 return "SupplyDelivery"; 10271 if (code == ResourceType.SUPPLYREQUEST) 10272 return "SupplyRequest"; 10273 if (code == ResourceType.TASK) 10274 return "Task"; 10275 if (code == ResourceType.TESTREPORT) 10276 return "TestReport"; 10277 if (code == ResourceType.TESTSCRIPT) 10278 return "TestScript"; 10279 if (code == ResourceType.VALUESET) 10280 return "ValueSet"; 10281 if (code == ResourceType.VISIONPRESCRIPTION) 10282 return "VisionPrescription"; 10283 return "?"; 10284 } 10285 public String toSystem(ResourceType code) { 10286 return code.getSystem(); 10287 } 10288 } 10289 10290 public enum SearchParamType { 10291 /** 10292 * Search parameter SHALL be a number (a whole number, or a decimal). 10293 */ 10294 NUMBER, 10295 /** 10296 * Search parameter is on a date/time. The date format is the standard XML format, though other formats may be supported. 10297 */ 10298 DATE, 10299 /** 10300 * Search parameter is a simple string, like a name part. Search is case-insensitive and accent-insensitive. May match just the start of a string. String parameters may contain spaces. 10301 */ 10302 STRING, 10303 /** 10304 * Search parameter on a coded element or identifier. May be used to search through the text, displayname, code and code/codesystem (for codes) and label, system and key (for identifier). Its value is either a string or a pair of namespace and value, separated by a "|", depending on the modifier used. 10305 */ 10306 TOKEN, 10307 /** 10308 * A reference to another resource. 10309 */ 10310 REFERENCE, 10311 /** 10312 * A composite search parameter that combines a search on two values together. 10313 */ 10314 COMPOSITE, 10315 /** 10316 * A search parameter that searches on a quantity. 10317 */ 10318 QUANTITY, 10319 /** 10320 * A search parameter that searches on a URI (RFC 3986). 10321 */ 10322 URI, 10323 /** 10324 * added to help the parsers 10325 */ 10326 NULL; 10327 public static SearchParamType fromCode(String codeString) throws FHIRException { 10328 if (codeString == null || "".equals(codeString)) 10329 return null; 10330 if ("number".equals(codeString)) 10331 return NUMBER; 10332 if ("date".equals(codeString)) 10333 return DATE; 10334 if ("string".equals(codeString)) 10335 return STRING; 10336 if ("token".equals(codeString)) 10337 return TOKEN; 10338 if ("reference".equals(codeString)) 10339 return REFERENCE; 10340 if ("composite".equals(codeString)) 10341 return COMPOSITE; 10342 if ("quantity".equals(codeString)) 10343 return QUANTITY; 10344 if ("uri".equals(codeString)) 10345 return URI; 10346 throw new FHIRException("Unknown SearchParamType code '"+codeString+"'"); 10347 } 10348 public String toCode() { 10349 switch (this) { 10350 case NUMBER: return "number"; 10351 case DATE: return "date"; 10352 case STRING: return "string"; 10353 case TOKEN: return "token"; 10354 case REFERENCE: return "reference"; 10355 case COMPOSITE: return "composite"; 10356 case QUANTITY: return "quantity"; 10357 case URI: return "uri"; 10358 case NULL: return null; 10359 default: return "?"; 10360 } 10361 } 10362 public String getSystem() { 10363 switch (this) { 10364 case NUMBER: return "http://hl7.org/fhir/search-param-type"; 10365 case DATE: return "http://hl7.org/fhir/search-param-type"; 10366 case STRING: return "http://hl7.org/fhir/search-param-type"; 10367 case TOKEN: return "http://hl7.org/fhir/search-param-type"; 10368 case REFERENCE: return "http://hl7.org/fhir/search-param-type"; 10369 case COMPOSITE: return "http://hl7.org/fhir/search-param-type"; 10370 case QUANTITY: return "http://hl7.org/fhir/search-param-type"; 10371 case URI: return "http://hl7.org/fhir/search-param-type"; 10372 case NULL: return null; 10373 default: return "?"; 10374 } 10375 } 10376 public String getDefinition() { 10377 switch (this) { 10378 case NUMBER: return "Search parameter SHALL be a number (a whole number, or a decimal)."; 10379 case DATE: return "Search parameter is on a date/time. The date format is the standard XML format, though other formats may be supported."; 10380 case STRING: return "Search parameter is a simple string, like a name part. Search is case-insensitive and accent-insensitive. May match just the start of a string. String parameters may contain spaces."; 10381 case TOKEN: return "Search parameter on a coded element or identifier. May be used to search through the text, displayname, code and code/codesystem (for codes) and label, system and key (for identifier). Its value is either a string or a pair of namespace and value, separated by a \"|\", depending on the modifier used."; 10382 case REFERENCE: return "A reference to another resource."; 10383 case COMPOSITE: return "A composite search parameter that combines a search on two values together."; 10384 case QUANTITY: return "A search parameter that searches on a quantity."; 10385 case URI: return "A search parameter that searches on a URI (RFC 3986)."; 10386 case NULL: return null; 10387 default: return "?"; 10388 } 10389 } 10390 public String getDisplay() { 10391 switch (this) { 10392 case NUMBER: return "Number"; 10393 case DATE: return "Date/DateTime"; 10394 case STRING: return "String"; 10395 case TOKEN: return "Token"; 10396 case REFERENCE: return "Reference"; 10397 case COMPOSITE: return "Composite"; 10398 case QUANTITY: return "Quantity"; 10399 case URI: return "URI"; 10400 case NULL: return null; 10401 default: return "?"; 10402 } 10403 } 10404 } 10405 10406 public static class SearchParamTypeEnumFactory implements EnumFactory<SearchParamType> { 10407 public SearchParamType fromCode(String codeString) throws IllegalArgumentException { 10408 if (codeString == null || "".equals(codeString)) 10409 if (codeString == null || "".equals(codeString)) 10410 return null; 10411 if ("number".equals(codeString)) 10412 return SearchParamType.NUMBER; 10413 if ("date".equals(codeString)) 10414 return SearchParamType.DATE; 10415 if ("string".equals(codeString)) 10416 return SearchParamType.STRING; 10417 if ("token".equals(codeString)) 10418 return SearchParamType.TOKEN; 10419 if ("reference".equals(codeString)) 10420 return SearchParamType.REFERENCE; 10421 if ("composite".equals(codeString)) 10422 return SearchParamType.COMPOSITE; 10423 if ("quantity".equals(codeString)) 10424 return SearchParamType.QUANTITY; 10425 if ("uri".equals(codeString)) 10426 return SearchParamType.URI; 10427 throw new IllegalArgumentException("Unknown SearchParamType code '"+codeString+"'"); 10428 } 10429 public Enumeration<SearchParamType> fromType(PrimitiveType<?> code) throws FHIRException { 10430 if (code == null) 10431 return null; 10432 if (code.isEmpty()) 10433 return new Enumeration<SearchParamType>(this); 10434 String codeString = code.asStringValue(); 10435 if (codeString == null || "".equals(codeString)) 10436 return null; 10437 if ("number".equals(codeString)) 10438 return new Enumeration<SearchParamType>(this, SearchParamType.NUMBER); 10439 if ("date".equals(codeString)) 10440 return new Enumeration<SearchParamType>(this, SearchParamType.DATE); 10441 if ("string".equals(codeString)) 10442 return new Enumeration<SearchParamType>(this, SearchParamType.STRING); 10443 if ("token".equals(codeString)) 10444 return new Enumeration<SearchParamType>(this, SearchParamType.TOKEN); 10445 if ("reference".equals(codeString)) 10446 return new Enumeration<SearchParamType>(this, SearchParamType.REFERENCE); 10447 if ("composite".equals(codeString)) 10448 return new Enumeration<SearchParamType>(this, SearchParamType.COMPOSITE); 10449 if ("quantity".equals(codeString)) 10450 return new Enumeration<SearchParamType>(this, SearchParamType.QUANTITY); 10451 if ("uri".equals(codeString)) 10452 return new Enumeration<SearchParamType>(this, SearchParamType.URI); 10453 throw new FHIRException("Unknown SearchParamType code '"+codeString+"'"); 10454 } 10455 public String toCode(SearchParamType code) { 10456 if (code == SearchParamType.NUMBER) 10457 return "number"; 10458 if (code == SearchParamType.DATE) 10459 return "date"; 10460 if (code == SearchParamType.STRING) 10461 return "string"; 10462 if (code == SearchParamType.TOKEN) 10463 return "token"; 10464 if (code == SearchParamType.REFERENCE) 10465 return "reference"; 10466 if (code == SearchParamType.COMPOSITE) 10467 return "composite"; 10468 if (code == SearchParamType.QUANTITY) 10469 return "quantity"; 10470 if (code == SearchParamType.URI) 10471 return "uri"; 10472 return "?"; 10473 } 10474 public String toSystem(SearchParamType code) { 10475 return code.getSystem(); 10476 } 10477 } 10478 10479 public enum SpecialValues { 10480 /** 10481 * Boolean true. 10482 */ 10483 TRUE, 10484 /** 10485 * Boolean false. 10486 */ 10487 FALSE, 10488 /** 10489 * The content is greater than zero, but too small to be quantified. 10490 */ 10491 TRACE, 10492 /** 10493 * The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material. 10494 */ 10495 SUFFICIENT, 10496 /** 10497 * The value is no longer available. 10498 */ 10499 WITHDRAWN, 10500 /** 10501 * The are no known applicable values in this context. 10502 */ 10503 NILKNOWN, 10504 /** 10505 * added to help the parsers 10506 */ 10507 NULL; 10508 public static SpecialValues fromCode(String codeString) throws FHIRException { 10509 if (codeString == null || "".equals(codeString)) 10510 return null; 10511 if ("true".equals(codeString)) 10512 return TRUE; 10513 if ("false".equals(codeString)) 10514 return FALSE; 10515 if ("trace".equals(codeString)) 10516 return TRACE; 10517 if ("sufficient".equals(codeString)) 10518 return SUFFICIENT; 10519 if ("withdrawn".equals(codeString)) 10520 return WITHDRAWN; 10521 if ("nil-known".equals(codeString)) 10522 return NILKNOWN; 10523 throw new FHIRException("Unknown SpecialValues code '"+codeString+"'"); 10524 } 10525 public String toCode() { 10526 switch (this) { 10527 case TRUE: return "true"; 10528 case FALSE: return "false"; 10529 case TRACE: return "trace"; 10530 case SUFFICIENT: return "sufficient"; 10531 case WITHDRAWN: return "withdrawn"; 10532 case NILKNOWN: return "nil-known"; 10533 case NULL: return null; 10534 default: return "?"; 10535 } 10536 } 10537 public String getSystem() { 10538 switch (this) { 10539 case TRUE: return "http://hl7.org/fhir/special-values"; 10540 case FALSE: return "http://hl7.org/fhir/special-values"; 10541 case TRACE: return "http://hl7.org/fhir/special-values"; 10542 case SUFFICIENT: return "http://hl7.org/fhir/special-values"; 10543 case WITHDRAWN: return "http://hl7.org/fhir/special-values"; 10544 case NILKNOWN: return "http://hl7.org/fhir/special-values"; 10545 case NULL: return null; 10546 default: return "?"; 10547 } 10548 } 10549 public String getDefinition() { 10550 switch (this) { 10551 case TRUE: return "Boolean true."; 10552 case FALSE: return "Boolean false."; 10553 case TRACE: return "The content is greater than zero, but too small to be quantified."; 10554 case SUFFICIENT: return "The specific quantity is not known, but is known to be non-zero and is not specified because it makes up the bulk of the material."; 10555 case WITHDRAWN: return "The value is no longer available."; 10556 case NILKNOWN: return "The are no known applicable values in this context."; 10557 case NULL: return null; 10558 default: return "?"; 10559 } 10560 } 10561 public String getDisplay() { 10562 switch (this) { 10563 case TRUE: return "true"; 10564 case FALSE: return "false"; 10565 case TRACE: return "Trace Amount Detected"; 10566 case SUFFICIENT: return "Sufficient Quantity"; 10567 case WITHDRAWN: return "Value Withdrawn"; 10568 case NILKNOWN: return "Nil Known"; 10569 case NULL: return null; 10570 default: return "?"; 10571 } 10572 } 10573 } 10574 10575 public static class SpecialValuesEnumFactory implements EnumFactory<SpecialValues> { 10576 public SpecialValues fromCode(String codeString) throws IllegalArgumentException { 10577 if (codeString == null || "".equals(codeString)) 10578 if (codeString == null || "".equals(codeString)) 10579 return null; 10580 if ("true".equals(codeString)) 10581 return SpecialValues.TRUE; 10582 if ("false".equals(codeString)) 10583 return SpecialValues.FALSE; 10584 if ("trace".equals(codeString)) 10585 return SpecialValues.TRACE; 10586 if ("sufficient".equals(codeString)) 10587 return SpecialValues.SUFFICIENT; 10588 if ("withdrawn".equals(codeString)) 10589 return SpecialValues.WITHDRAWN; 10590 if ("nil-known".equals(codeString)) 10591 return SpecialValues.NILKNOWN; 10592 throw new IllegalArgumentException("Unknown SpecialValues code '"+codeString+"'"); 10593 } 10594 public Enumeration<SpecialValues> fromType(PrimitiveType<?> code) throws FHIRException { 10595 if (code == null) 10596 return null; 10597 if (code.isEmpty()) 10598 return new Enumeration<SpecialValues>(this); 10599 String codeString = code.asStringValue(); 10600 if (codeString == null || "".equals(codeString)) 10601 return null; 10602 if ("true".equals(codeString)) 10603 return new Enumeration<SpecialValues>(this, SpecialValues.TRUE); 10604 if ("false".equals(codeString)) 10605 return new Enumeration<SpecialValues>(this, SpecialValues.FALSE); 10606 if ("trace".equals(codeString)) 10607 return new Enumeration<SpecialValues>(this, SpecialValues.TRACE); 10608 if ("sufficient".equals(codeString)) 10609 return new Enumeration<SpecialValues>(this, SpecialValues.SUFFICIENT); 10610 if ("withdrawn".equals(codeString)) 10611 return new Enumeration<SpecialValues>(this, SpecialValues.WITHDRAWN); 10612 if ("nil-known".equals(codeString)) 10613 return new Enumeration<SpecialValues>(this, SpecialValues.NILKNOWN); 10614 throw new FHIRException("Unknown SpecialValues code '"+codeString+"'"); 10615 } 10616 public String toCode(SpecialValues code) { 10617 if (code == SpecialValues.TRUE) 10618 return "true"; 10619 if (code == SpecialValues.FALSE) 10620 return "false"; 10621 if (code == SpecialValues.TRACE) 10622 return "trace"; 10623 if (code == SpecialValues.SUFFICIENT) 10624 return "sufficient"; 10625 if (code == SpecialValues.WITHDRAWN) 10626 return "withdrawn"; 10627 if (code == SpecialValues.NILKNOWN) 10628 return "nil-known"; 10629 return "?"; 10630 } 10631 public String toSystem(SpecialValues code) { 10632 return code.getSystem(); 10633 } 10634 } 10635 10636 10637}