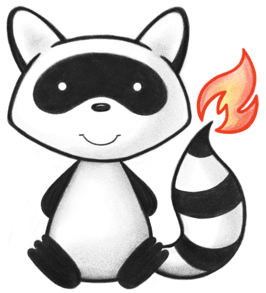
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 040 041import ca.uhn.fhir.model.api.annotation.Block; 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * An association between a patient and an organization / healthcare provider(s) during which time encounters may occur. The managing organization assumes a level of responsibility for the patient during this time. 048 */ 049@ResourceDef(name="EpisodeOfCare", profile="http://hl7.org/fhir/Profile/EpisodeOfCare") 050public class EpisodeOfCare extends DomainResource { 051 052 public enum EpisodeOfCareStatus { 053 /** 054 * This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services. 055 */ 056 PLANNED, 057 /** 058 * This episode has been placed on a waitlist, pending the episode being made active (or cancelled). 059 */ 060 WAITLIST, 061 /** 062 * This episode of care is current. 063 */ 064 ACTIVE, 065 /** 066 * This episode of care is on hold, the organization has limited responsibility for the patient (such as while on respite). 067 */ 068 ONHOLD, 069 /** 070 * This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as "closed", "completed" or other similar terms. 071 */ 072 FINISHED, 073 /** 074 * The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow. 075 */ 076 CANCELLED, 077 /** 078 * This instance should not have been part of this patient's medical record. 079 */ 080 ENTEREDINERROR, 081 /** 082 * added to help the parsers with the generic types 083 */ 084 NULL; 085 public static EpisodeOfCareStatus fromCode(String codeString) throws FHIRException { 086 if (codeString == null || "".equals(codeString)) 087 return null; 088 if ("planned".equals(codeString)) 089 return PLANNED; 090 if ("waitlist".equals(codeString)) 091 return WAITLIST; 092 if ("active".equals(codeString)) 093 return ACTIVE; 094 if ("onhold".equals(codeString)) 095 return ONHOLD; 096 if ("finished".equals(codeString)) 097 return FINISHED; 098 if ("cancelled".equals(codeString)) 099 return CANCELLED; 100 if ("entered-in-error".equals(codeString)) 101 return ENTEREDINERROR; 102 if (Configuration.isAcceptInvalidEnums()) 103 return null; 104 else 105 throw new FHIRException("Unknown EpisodeOfCareStatus code '"+codeString+"'"); 106 } 107 public String toCode() { 108 switch (this) { 109 case PLANNED: return "planned"; 110 case WAITLIST: return "waitlist"; 111 case ACTIVE: return "active"; 112 case ONHOLD: return "onhold"; 113 case FINISHED: return "finished"; 114 case CANCELLED: return "cancelled"; 115 case ENTEREDINERROR: return "entered-in-error"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 public String getSystem() { 121 switch (this) { 122 case PLANNED: return "http://hl7.org/fhir/episode-of-care-status"; 123 case WAITLIST: return "http://hl7.org/fhir/episode-of-care-status"; 124 case ACTIVE: return "http://hl7.org/fhir/episode-of-care-status"; 125 case ONHOLD: return "http://hl7.org/fhir/episode-of-care-status"; 126 case FINISHED: return "http://hl7.org/fhir/episode-of-care-status"; 127 case CANCELLED: return "http://hl7.org/fhir/episode-of-care-status"; 128 case ENTEREDINERROR: return "http://hl7.org/fhir/episode-of-care-status"; 129 case NULL: return null; 130 default: return "?"; 131 } 132 } 133 public String getDefinition() { 134 switch (this) { 135 case PLANNED: return "This episode of care is planned to start at the date specified in the period.start. During this status, an organization may perform assessments to determine if the patient is eligible to receive services, or be organizing to make resources available to provide care services."; 136 case WAITLIST: return "This episode has been placed on a waitlist, pending the episode being made active (or cancelled)."; 137 case ACTIVE: return "This episode of care is current."; 138 case ONHOLD: return "This episode of care is on hold, the organization has limited responsibility for the patient (such as while on respite)."; 139 case FINISHED: return "This episode of care is finished and the organization is not expecting to be providing further care to the patient. Can also be known as \"closed\", \"completed\" or other similar terms."; 140 case CANCELLED: return "The episode of care was cancelled, or withdrawn from service, often selected during the planned stage as the patient may have gone elsewhere, or the circumstances have changed and the organization is unable to provide the care. It indicates that services terminated outside the planned/expected workflow."; 141 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 public String getDisplay() { 147 switch (this) { 148 case PLANNED: return "Planned"; 149 case WAITLIST: return "Waitlist"; 150 case ACTIVE: return "Active"; 151 case ONHOLD: return "On Hold"; 152 case FINISHED: return "Finished"; 153 case CANCELLED: return "Cancelled"; 154 case ENTEREDINERROR: return "Entered in Error"; 155 case NULL: return null; 156 default: return "?"; 157 } 158 } 159 } 160 161 public static class EpisodeOfCareStatusEnumFactory implements EnumFactory<EpisodeOfCareStatus> { 162 public EpisodeOfCareStatus fromCode(String codeString) throws IllegalArgumentException { 163 if (codeString == null || "".equals(codeString)) 164 if (codeString == null || "".equals(codeString)) 165 return null; 166 if ("planned".equals(codeString)) 167 return EpisodeOfCareStatus.PLANNED; 168 if ("waitlist".equals(codeString)) 169 return EpisodeOfCareStatus.WAITLIST; 170 if ("active".equals(codeString)) 171 return EpisodeOfCareStatus.ACTIVE; 172 if ("onhold".equals(codeString)) 173 return EpisodeOfCareStatus.ONHOLD; 174 if ("finished".equals(codeString)) 175 return EpisodeOfCareStatus.FINISHED; 176 if ("cancelled".equals(codeString)) 177 return EpisodeOfCareStatus.CANCELLED; 178 if ("entered-in-error".equals(codeString)) 179 return EpisodeOfCareStatus.ENTEREDINERROR; 180 throw new IllegalArgumentException("Unknown EpisodeOfCareStatus code '"+codeString+"'"); 181 } 182 public Enumeration<EpisodeOfCareStatus> fromType(PrimitiveType<?> code) throws FHIRException { 183 if (code == null) 184 return null; 185 if (code.isEmpty()) 186 return new Enumeration<EpisodeOfCareStatus>(this); 187 String codeString = code.asStringValue(); 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("planned".equals(codeString)) 191 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.PLANNED); 192 if ("waitlist".equals(codeString)) 193 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.WAITLIST); 194 if ("active".equals(codeString)) 195 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ACTIVE); 196 if ("onhold".equals(codeString)) 197 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ONHOLD); 198 if ("finished".equals(codeString)) 199 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.FINISHED); 200 if ("cancelled".equals(codeString)) 201 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.CANCELLED); 202 if ("entered-in-error".equals(codeString)) 203 return new Enumeration<EpisodeOfCareStatus>(this, EpisodeOfCareStatus.ENTEREDINERROR); 204 throw new FHIRException("Unknown EpisodeOfCareStatus code '"+codeString+"'"); 205 } 206 public String toCode(EpisodeOfCareStatus code) { 207 if (code == EpisodeOfCareStatus.NULL) 208 return null; 209 if (code == EpisodeOfCareStatus.PLANNED) 210 return "planned"; 211 if (code == EpisodeOfCareStatus.WAITLIST) 212 return "waitlist"; 213 if (code == EpisodeOfCareStatus.ACTIVE) 214 return "active"; 215 if (code == EpisodeOfCareStatus.ONHOLD) 216 return "onhold"; 217 if (code == EpisodeOfCareStatus.FINISHED) 218 return "finished"; 219 if (code == EpisodeOfCareStatus.CANCELLED) 220 return "cancelled"; 221 if (code == EpisodeOfCareStatus.ENTEREDINERROR) 222 return "entered-in-error"; 223 return "?"; 224 } 225 public String toSystem(EpisodeOfCareStatus code) { 226 return code.getSystem(); 227 } 228 } 229 230 @Block() 231 public static class EpisodeOfCareStatusHistoryComponent extends BackboneElement implements IBaseBackboneElement { 232 /** 233 * planned | waitlist | active | onhold | finished | cancelled. 234 */ 235 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 236 @Description(shortDefinition="planned | waitlist | active | onhold | finished | cancelled | entered-in-error", formalDefinition="planned | waitlist | active | onhold | finished | cancelled." ) 237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/episode-of-care-status") 238 protected Enumeration<EpisodeOfCareStatus> status; 239 240 /** 241 * The period during this EpisodeOfCare that the specific status applied. 242 */ 243 @Child(name = "period", type = {Period.class}, order=2, min=1, max=1, modifier=false, summary=false) 244 @Description(shortDefinition="Duration the EpisodeOfCare was in the specified status", formalDefinition="The period during this EpisodeOfCare that the specific status applied." ) 245 protected Period period; 246 247 private static final long serialVersionUID = -1192432864L; 248 249 /** 250 * Constructor 251 */ 252 public EpisodeOfCareStatusHistoryComponent() { 253 super(); 254 } 255 256 /** 257 * Constructor 258 */ 259 public EpisodeOfCareStatusHistoryComponent(Enumeration<EpisodeOfCareStatus> status, Period period) { 260 super(); 261 this.status = status; 262 this.period = period; 263 } 264 265 /** 266 * @return {@link #status} (planned | waitlist | active | onhold | finished | cancelled.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 267 */ 268 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 269 if (this.status == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.status"); 272 else if (Configuration.doAutoCreate()) 273 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 274 return this.status; 275 } 276 277 public boolean hasStatusElement() { 278 return this.status != null && !this.status.isEmpty(); 279 } 280 281 public boolean hasStatus() { 282 return this.status != null && !this.status.isEmpty(); 283 } 284 285 /** 286 * @param value {@link #status} (planned | waitlist | active | onhold | finished | cancelled.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 287 */ 288 public EpisodeOfCareStatusHistoryComponent setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 289 this.status = value; 290 return this; 291 } 292 293 /** 294 * @return planned | waitlist | active | onhold | finished | cancelled. 295 */ 296 public EpisodeOfCareStatus getStatus() { 297 return this.status == null ? null : this.status.getValue(); 298 } 299 300 /** 301 * @param value planned | waitlist | active | onhold | finished | cancelled. 302 */ 303 public EpisodeOfCareStatusHistoryComponent setStatus(EpisodeOfCareStatus value) { 304 if (this.status == null) 305 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 306 this.status.setValue(value); 307 return this; 308 } 309 310 /** 311 * @return {@link #period} (The period during this EpisodeOfCare that the specific status applied.) 312 */ 313 public Period getPeriod() { 314 if (this.period == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create EpisodeOfCareStatusHistoryComponent.period"); 317 else if (Configuration.doAutoCreate()) 318 this.period = new Period(); // cc 319 return this.period; 320 } 321 322 public boolean hasPeriod() { 323 return this.period != null && !this.period.isEmpty(); 324 } 325 326 /** 327 * @param value {@link #period} (The period during this EpisodeOfCare that the specific status applied.) 328 */ 329 public EpisodeOfCareStatusHistoryComponent setPeriod(Period value) { 330 this.period = value; 331 return this; 332 } 333 334 protected void listChildren(List<Property> children) { 335 super.listChildren(children); 336 children.add(new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status)); 337 children.add(new Property("period", "Period", "The period during this EpisodeOfCare that the specific status applied.", 0, 1, period)); 338 } 339 340 @Override 341 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 342 switch (_hash) { 343 case -892481550: /*status*/ return new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status); 344 case -991726143: /*period*/ return new Property("period", "Period", "The period during this EpisodeOfCare that the specific status applied.", 0, 1, period); 345 default: return super.getNamedProperty(_hash, _name, _checkValid); 346 } 347 348 } 349 350 @Override 351 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 352 switch (hash) { 353 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EpisodeOfCareStatus> 354 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 355 default: return super.getProperty(hash, name, checkValid); 356 } 357 358 } 359 360 @Override 361 public Base setProperty(int hash, String name, Base value) throws FHIRException { 362 switch (hash) { 363 case -892481550: // status 364 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 365 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 366 return value; 367 case -991726143: // period 368 this.period = castToPeriod(value); // Period 369 return value; 370 default: return super.setProperty(hash, name, value); 371 } 372 373 } 374 375 @Override 376 public Base setProperty(String name, Base value) throws FHIRException { 377 if (name.equals("status")) { 378 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 379 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 380 } else if (name.equals("period")) { 381 this.period = castToPeriod(value); // Period 382 } else 383 return super.setProperty(name, value); 384 return value; 385 } 386 387 @Override 388 public Base makeProperty(int hash, String name) throws FHIRException { 389 switch (hash) { 390 case -892481550: return getStatusElement(); 391 case -991726143: return getPeriod(); 392 default: return super.makeProperty(hash, name); 393 } 394 395 } 396 397 @Override 398 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 399 switch (hash) { 400 case -892481550: /*status*/ return new String[] {"code"}; 401 case -991726143: /*period*/ return new String[] {"Period"}; 402 default: return super.getTypesForProperty(hash, name); 403 } 404 405 } 406 407 @Override 408 public Base addChild(String name) throws FHIRException { 409 if (name.equals("status")) { 410 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 411 } 412 else if (name.equals("period")) { 413 this.period = new Period(); 414 return this.period; 415 } 416 else 417 return super.addChild(name); 418 } 419 420 public EpisodeOfCareStatusHistoryComponent copy() { 421 EpisodeOfCareStatusHistoryComponent dst = new EpisodeOfCareStatusHistoryComponent(); 422 copyValues(dst); 423 dst.status = status == null ? null : status.copy(); 424 dst.period = period == null ? null : period.copy(); 425 return dst; 426 } 427 428 @Override 429 public boolean equalsDeep(Base other_) { 430 if (!super.equalsDeep(other_)) 431 return false; 432 if (!(other_ instanceof EpisodeOfCareStatusHistoryComponent)) 433 return false; 434 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other_; 435 return compareDeep(status, o.status, true) && compareDeep(period, o.period, true); 436 } 437 438 @Override 439 public boolean equalsShallow(Base other_) { 440 if (!super.equalsShallow(other_)) 441 return false; 442 if (!(other_ instanceof EpisodeOfCareStatusHistoryComponent)) 443 return false; 444 EpisodeOfCareStatusHistoryComponent o = (EpisodeOfCareStatusHistoryComponent) other_; 445 return compareValues(status, o.status, true); 446 } 447 448 public boolean isEmpty() { 449 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(status, period); 450 } 451 452 public String fhirType() { 453 return "EpisodeOfCare.statusHistory"; 454 455 } 456 457 } 458 459 @Block() 460 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 461 /** 462 * A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for. 463 */ 464 @Child(name = "condition", type = {Condition.class}, order=1, min=1, max=1, modifier=false, summary=true) 465 @Description(shortDefinition="Conditions/problems/diagnoses this episode of care is for", formalDefinition="A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for." ) 466 protected Reference condition; 467 468 /** 469 * The actual object that is the target of the reference (A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.) 470 */ 471 protected Condition conditionTarget; 472 473 /** 474 * Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?). 475 */ 476 @Child(name = "role", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 477 @Description(shortDefinition="Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?)", formalDefinition="Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?)." ) 478 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/diagnosis-role") 479 protected CodeableConcept role; 480 481 /** 482 * Ranking of the diagnosis (for each role type). 483 */ 484 @Child(name = "rank", type = {PositiveIntType.class}, order=3, min=0, max=1, modifier=false, summary=true) 485 @Description(shortDefinition="Ranking of the diagnosis (for each role type)", formalDefinition="Ranking of the diagnosis (for each role type)." ) 486 protected PositiveIntType rank; 487 488 private static final long serialVersionUID = 249445632L; 489 490 /** 491 * Constructor 492 */ 493 public DiagnosisComponent() { 494 super(); 495 } 496 497 /** 498 * Constructor 499 */ 500 public DiagnosisComponent(Reference condition) { 501 super(); 502 this.condition = condition; 503 } 504 505 /** 506 * @return {@link #condition} (A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.) 507 */ 508 public Reference getCondition() { 509 if (this.condition == null) 510 if (Configuration.errorOnAutoCreate()) 511 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 512 else if (Configuration.doAutoCreate()) 513 this.condition = new Reference(); // cc 514 return this.condition; 515 } 516 517 public boolean hasCondition() { 518 return this.condition != null && !this.condition.isEmpty(); 519 } 520 521 /** 522 * @param value {@link #condition} (A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.) 523 */ 524 public DiagnosisComponent setCondition(Reference value) { 525 this.condition = value; 526 return this; 527 } 528 529 /** 530 * @return {@link #condition} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.) 531 */ 532 public Condition getConditionTarget() { 533 if (this.conditionTarget == null) 534 if (Configuration.errorOnAutoCreate()) 535 throw new Error("Attempt to auto-create DiagnosisComponent.condition"); 536 else if (Configuration.doAutoCreate()) 537 this.conditionTarget = new Condition(); // aa 538 return this.conditionTarget; 539 } 540 541 /** 542 * @param value {@link #condition} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.) 543 */ 544 public DiagnosisComponent setConditionTarget(Condition value) { 545 this.conditionTarget = value; 546 return this; 547 } 548 549 /** 550 * @return {@link #role} (Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).) 551 */ 552 public CodeableConcept getRole() { 553 if (this.role == null) 554 if (Configuration.errorOnAutoCreate()) 555 throw new Error("Attempt to auto-create DiagnosisComponent.role"); 556 else if (Configuration.doAutoCreate()) 557 this.role = new CodeableConcept(); // cc 558 return this.role; 559 } 560 561 public boolean hasRole() { 562 return this.role != null && !this.role.isEmpty(); 563 } 564 565 /** 566 * @param value {@link #role} (Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).) 567 */ 568 public DiagnosisComponent setRole(CodeableConcept value) { 569 this.role = value; 570 return this; 571 } 572 573 /** 574 * @return {@link #rank} (Ranking of the diagnosis (for each role type).). This is the underlying object with id, value and extensions. The accessor "getRank" gives direct access to the value 575 */ 576 public PositiveIntType getRankElement() { 577 if (this.rank == null) 578 if (Configuration.errorOnAutoCreate()) 579 throw new Error("Attempt to auto-create DiagnosisComponent.rank"); 580 else if (Configuration.doAutoCreate()) 581 this.rank = new PositiveIntType(); // bb 582 return this.rank; 583 } 584 585 public boolean hasRankElement() { 586 return this.rank != null && !this.rank.isEmpty(); 587 } 588 589 public boolean hasRank() { 590 return this.rank != null && !this.rank.isEmpty(); 591 } 592 593 /** 594 * @param value {@link #rank} (Ranking of the diagnosis (for each role type).). This is the underlying object with id, value and extensions. The accessor "getRank" gives direct access to the value 595 */ 596 public DiagnosisComponent setRankElement(PositiveIntType value) { 597 this.rank = value; 598 return this; 599 } 600 601 /** 602 * @return Ranking of the diagnosis (for each role type). 603 */ 604 public int getRank() { 605 return this.rank == null || this.rank.isEmpty() ? 0 : this.rank.getValue(); 606 } 607 608 /** 609 * @param value Ranking of the diagnosis (for each role type). 610 */ 611 public DiagnosisComponent setRank(int value) { 612 if (this.rank == null) 613 this.rank = new PositiveIntType(); 614 this.rank.setValue(value); 615 return this; 616 } 617 618 protected void listChildren(List<Property> children) { 619 super.listChildren(children); 620 children.add(new Property("condition", "Reference(Condition)", "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.", 0, 1, condition)); 621 children.add(new Property("role", "CodeableConcept", "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).", 0, 1, role)); 622 children.add(new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, rank)); 623 } 624 625 @Override 626 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 627 switch (_hash) { 628 case -861311717: /*condition*/ return new Property("condition", "Reference(Condition)", "A list of conditions/problems/diagnoses that this episode of care is intended to be providing care for.", 0, 1, condition); 629 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Role that this diagnosis has within the episode of care (e.g. admission, billing, discharge ?).", 0, 1, role); 630 case 3492908: /*rank*/ return new Property("rank", "positiveInt", "Ranking of the diagnosis (for each role type).", 0, 1, rank); 631 default: return super.getNamedProperty(_hash, _name, _checkValid); 632 } 633 634 } 635 636 @Override 637 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 638 switch (hash) { 639 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : new Base[] {this.condition}; // Reference 640 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 641 case 3492908: /*rank*/ return this.rank == null ? new Base[0] : new Base[] {this.rank}; // PositiveIntType 642 default: return super.getProperty(hash, name, checkValid); 643 } 644 645 } 646 647 @Override 648 public Base setProperty(int hash, String name, Base value) throws FHIRException { 649 switch (hash) { 650 case -861311717: // condition 651 this.condition = castToReference(value); // Reference 652 return value; 653 case 3506294: // role 654 this.role = castToCodeableConcept(value); // CodeableConcept 655 return value; 656 case 3492908: // rank 657 this.rank = castToPositiveInt(value); // PositiveIntType 658 return value; 659 default: return super.setProperty(hash, name, value); 660 } 661 662 } 663 664 @Override 665 public Base setProperty(String name, Base value) throws FHIRException { 666 if (name.equals("condition")) { 667 this.condition = castToReference(value); // Reference 668 } else if (name.equals("role")) { 669 this.role = castToCodeableConcept(value); // CodeableConcept 670 } else if (name.equals("rank")) { 671 this.rank = castToPositiveInt(value); // PositiveIntType 672 } else 673 return super.setProperty(name, value); 674 return value; 675 } 676 677 @Override 678 public Base makeProperty(int hash, String name) throws FHIRException { 679 switch (hash) { 680 case -861311717: return getCondition(); 681 case 3506294: return getRole(); 682 case 3492908: return getRankElement(); 683 default: return super.makeProperty(hash, name); 684 } 685 686 } 687 688 @Override 689 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 690 switch (hash) { 691 case -861311717: /*condition*/ return new String[] {"Reference"}; 692 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 693 case 3492908: /*rank*/ return new String[] {"positiveInt"}; 694 default: return super.getTypesForProperty(hash, name); 695 } 696 697 } 698 699 @Override 700 public Base addChild(String name) throws FHIRException { 701 if (name.equals("condition")) { 702 this.condition = new Reference(); 703 return this.condition; 704 } 705 else if (name.equals("role")) { 706 this.role = new CodeableConcept(); 707 return this.role; 708 } 709 else if (name.equals("rank")) { 710 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.rank"); 711 } 712 else 713 return super.addChild(name); 714 } 715 716 public DiagnosisComponent copy() { 717 DiagnosisComponent dst = new DiagnosisComponent(); 718 copyValues(dst); 719 dst.condition = condition == null ? null : condition.copy(); 720 dst.role = role == null ? null : role.copy(); 721 dst.rank = rank == null ? null : rank.copy(); 722 return dst; 723 } 724 725 @Override 726 public boolean equalsDeep(Base other_) { 727 if (!super.equalsDeep(other_)) 728 return false; 729 if (!(other_ instanceof DiagnosisComponent)) 730 return false; 731 DiagnosisComponent o = (DiagnosisComponent) other_; 732 return compareDeep(condition, o.condition, true) && compareDeep(role, o.role, true) && compareDeep(rank, o.rank, true) 733 ; 734 } 735 736 @Override 737 public boolean equalsShallow(Base other_) { 738 if (!super.equalsShallow(other_)) 739 return false; 740 if (!(other_ instanceof DiagnosisComponent)) 741 return false; 742 DiagnosisComponent o = (DiagnosisComponent) other_; 743 return compareValues(rank, o.rank, true); 744 } 745 746 public boolean isEmpty() { 747 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(condition, role, rank); 748 } 749 750 public String fhirType() { 751 return "EpisodeOfCare.diagnosis"; 752 753 } 754 755 } 756 757 /** 758 * The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes. 759 */ 760 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 761 @Description(shortDefinition="Business Identifier(s) relevant for this EpisodeOfCare", formalDefinition="The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes." ) 762 protected List<Identifier> identifier; 763 764 /** 765 * planned | waitlist | active | onhold | finished | cancelled. 766 */ 767 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 768 @Description(shortDefinition="planned | waitlist | active | onhold | finished | cancelled | entered-in-error", formalDefinition="planned | waitlist | active | onhold | finished | cancelled." ) 769 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/episode-of-care-status") 770 protected Enumeration<EpisodeOfCareStatus> status; 771 772 /** 773 * The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource). 774 */ 775 @Child(name = "statusHistory", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 776 @Description(shortDefinition="Past list of status codes (the current status may be included to cover the start date of the status)", formalDefinition="The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource)." ) 777 protected List<EpisodeOfCareStatusHistoryComponent> statusHistory; 778 779 /** 780 * A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care. 781 */ 782 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 783 @Description(shortDefinition="Type/class - e.g. specialist referral, disease management", formalDefinition="A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care." ) 784 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/episodeofcare-type") 785 protected List<CodeableConcept> type; 786 787 /** 788 * The list of diagnosis relevant to this episode of care. 789 */ 790 @Child(name = "diagnosis", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 791 @Description(shortDefinition="The list of diagnosis relevant to this episode of care", formalDefinition="The list of diagnosis relevant to this episode of care." ) 792 protected List<DiagnosisComponent> diagnosis; 793 794 /** 795 * The patient who is the focus of this episode of care. 796 */ 797 @Child(name = "patient", type = {Patient.class}, order=5, min=1, max=1, modifier=false, summary=true) 798 @Description(shortDefinition="The patient who is the focus of this episode of care", formalDefinition="The patient who is the focus of this episode of care." ) 799 protected Reference patient; 800 801 /** 802 * The actual object that is the target of the reference (The patient who is the focus of this episode of care.) 803 */ 804 protected Patient patientTarget; 805 806 /** 807 * The organization that has assumed the specific responsibilities for the specified duration. 808 */ 809 @Child(name = "managingOrganization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=true) 810 @Description(shortDefinition="Organization that assumes care", formalDefinition="The organization that has assumed the specific responsibilities for the specified duration." ) 811 protected Reference managingOrganization; 812 813 /** 814 * The actual object that is the target of the reference (The organization that has assumed the specific responsibilities for the specified duration.) 815 */ 816 protected Organization managingOrganizationTarget; 817 818 /** 819 * The interval during which the managing organization assumes the defined responsibility. 820 */ 821 @Child(name = "period", type = {Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 822 @Description(shortDefinition="Interval during responsibility is assumed", formalDefinition="The interval during which the managing organization assumes the defined responsibility." ) 823 protected Period period; 824 825 /** 826 * Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals. 827 */ 828 @Child(name = "referralRequest", type = {ReferralRequest.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 829 @Description(shortDefinition="Originating Referral Request(s)", formalDefinition="Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals." ) 830 protected List<Reference> referralRequest; 831 /** 832 * The actual objects that are the target of the reference (Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.) 833 */ 834 protected List<ReferralRequest> referralRequestTarget; 835 836 837 /** 838 * The practitioner that is the care manager/care co-ordinator for this patient. 839 */ 840 @Child(name = "careManager", type = {Practitioner.class}, order=9, min=0, max=1, modifier=false, summary=false) 841 @Description(shortDefinition="Care manager/care co-ordinator for the patient", formalDefinition="The practitioner that is the care manager/care co-ordinator for this patient." ) 842 protected Reference careManager; 843 844 /** 845 * The actual object that is the target of the reference (The practitioner that is the care manager/care co-ordinator for this patient.) 846 */ 847 protected Practitioner careManagerTarget; 848 849 /** 850 * The list of practitioners that may be facilitating this episode of care for specific purposes. 851 */ 852 @Child(name = "team", type = {CareTeam.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 853 @Description(shortDefinition="Other practitioners facilitating this episode of care", formalDefinition="The list of practitioners that may be facilitating this episode of care for specific purposes." ) 854 protected List<Reference> team; 855 /** 856 * The actual objects that are the target of the reference (The list of practitioners that may be facilitating this episode of care for specific purposes.) 857 */ 858 protected List<CareTeam> teamTarget; 859 860 861 /** 862 * The set of accounts that may be used for billing for this EpisodeOfCare. 863 */ 864 @Child(name = "account", type = {Account.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 865 @Description(shortDefinition="The set of accounts that may be used for billing for this EpisodeOfCare", formalDefinition="The set of accounts that may be used for billing for this EpisodeOfCare." ) 866 protected List<Reference> account; 867 /** 868 * The actual objects that are the target of the reference (The set of accounts that may be used for billing for this EpisodeOfCare.) 869 */ 870 protected List<Account> accountTarget; 871 872 873 private static final long serialVersionUID = 76719771L; 874 875 /** 876 * Constructor 877 */ 878 public EpisodeOfCare() { 879 super(); 880 } 881 882 /** 883 * Constructor 884 */ 885 public EpisodeOfCare(Enumeration<EpisodeOfCareStatus> status, Reference patient) { 886 super(); 887 this.status = status; 888 this.patient = patient; 889 } 890 891 /** 892 * @return {@link #identifier} (The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.) 893 */ 894 public List<Identifier> getIdentifier() { 895 if (this.identifier == null) 896 this.identifier = new ArrayList<Identifier>(); 897 return this.identifier; 898 } 899 900 /** 901 * @return Returns a reference to <code>this</code> for easy method chaining 902 */ 903 public EpisodeOfCare setIdentifier(List<Identifier> theIdentifier) { 904 this.identifier = theIdentifier; 905 return this; 906 } 907 908 public boolean hasIdentifier() { 909 if (this.identifier == null) 910 return false; 911 for (Identifier item : this.identifier) 912 if (!item.isEmpty()) 913 return true; 914 return false; 915 } 916 917 public Identifier addIdentifier() { //3 918 Identifier t = new Identifier(); 919 if (this.identifier == null) 920 this.identifier = new ArrayList<Identifier>(); 921 this.identifier.add(t); 922 return t; 923 } 924 925 public EpisodeOfCare addIdentifier(Identifier t) { //3 926 if (t == null) 927 return this; 928 if (this.identifier == null) 929 this.identifier = new ArrayList<Identifier>(); 930 this.identifier.add(t); 931 return this; 932 } 933 934 /** 935 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 936 */ 937 public Identifier getIdentifierFirstRep() { 938 if (getIdentifier().isEmpty()) { 939 addIdentifier(); 940 } 941 return getIdentifier().get(0); 942 } 943 944 /** 945 * @return {@link #status} (planned | waitlist | active | onhold | finished | cancelled.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 946 */ 947 public Enumeration<EpisodeOfCareStatus> getStatusElement() { 948 if (this.status == null) 949 if (Configuration.errorOnAutoCreate()) 950 throw new Error("Attempt to auto-create EpisodeOfCare.status"); 951 else if (Configuration.doAutoCreate()) 952 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); // bb 953 return this.status; 954 } 955 956 public boolean hasStatusElement() { 957 return this.status != null && !this.status.isEmpty(); 958 } 959 960 public boolean hasStatus() { 961 return this.status != null && !this.status.isEmpty(); 962 } 963 964 /** 965 * @param value {@link #status} (planned | waitlist | active | onhold | finished | cancelled.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 966 */ 967 public EpisodeOfCare setStatusElement(Enumeration<EpisodeOfCareStatus> value) { 968 this.status = value; 969 return this; 970 } 971 972 /** 973 * @return planned | waitlist | active | onhold | finished | cancelled. 974 */ 975 public EpisodeOfCareStatus getStatus() { 976 return this.status == null ? null : this.status.getValue(); 977 } 978 979 /** 980 * @param value planned | waitlist | active | onhold | finished | cancelled. 981 */ 982 public EpisodeOfCare setStatus(EpisodeOfCareStatus value) { 983 if (this.status == null) 984 this.status = new Enumeration<EpisodeOfCareStatus>(new EpisodeOfCareStatusEnumFactory()); 985 this.status.setValue(value); 986 return this; 987 } 988 989 /** 990 * @return {@link #statusHistory} (The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).) 991 */ 992 public List<EpisodeOfCareStatusHistoryComponent> getStatusHistory() { 993 if (this.statusHistory == null) 994 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 995 return this.statusHistory; 996 } 997 998 /** 999 * @return Returns a reference to <code>this</code> for easy method chaining 1000 */ 1001 public EpisodeOfCare setStatusHistory(List<EpisodeOfCareStatusHistoryComponent> theStatusHistory) { 1002 this.statusHistory = theStatusHistory; 1003 return this; 1004 } 1005 1006 public boolean hasStatusHistory() { 1007 if (this.statusHistory == null) 1008 return false; 1009 for (EpisodeOfCareStatusHistoryComponent item : this.statusHistory) 1010 if (!item.isEmpty()) 1011 return true; 1012 return false; 1013 } 1014 1015 public EpisodeOfCareStatusHistoryComponent addStatusHistory() { //3 1016 EpisodeOfCareStatusHistoryComponent t = new EpisodeOfCareStatusHistoryComponent(); 1017 if (this.statusHistory == null) 1018 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1019 this.statusHistory.add(t); 1020 return t; 1021 } 1022 1023 public EpisodeOfCare addStatusHistory(EpisodeOfCareStatusHistoryComponent t) { //3 1024 if (t == null) 1025 return this; 1026 if (this.statusHistory == null) 1027 this.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1028 this.statusHistory.add(t); 1029 return this; 1030 } 1031 1032 /** 1033 * @return The first repetition of repeating field {@link #statusHistory}, creating it if it does not already exist 1034 */ 1035 public EpisodeOfCareStatusHistoryComponent getStatusHistoryFirstRep() { 1036 if (getStatusHistory().isEmpty()) { 1037 addStatusHistory(); 1038 } 1039 return getStatusHistory().get(0); 1040 } 1041 1042 /** 1043 * @return {@link #type} (A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.) 1044 */ 1045 public List<CodeableConcept> getType() { 1046 if (this.type == null) 1047 this.type = new ArrayList<CodeableConcept>(); 1048 return this.type; 1049 } 1050 1051 /** 1052 * @return Returns a reference to <code>this</code> for easy method chaining 1053 */ 1054 public EpisodeOfCare setType(List<CodeableConcept> theType) { 1055 this.type = theType; 1056 return this; 1057 } 1058 1059 public boolean hasType() { 1060 if (this.type == null) 1061 return false; 1062 for (CodeableConcept item : this.type) 1063 if (!item.isEmpty()) 1064 return true; 1065 return false; 1066 } 1067 1068 public CodeableConcept addType() { //3 1069 CodeableConcept t = new CodeableConcept(); 1070 if (this.type == null) 1071 this.type = new ArrayList<CodeableConcept>(); 1072 this.type.add(t); 1073 return t; 1074 } 1075 1076 public EpisodeOfCare addType(CodeableConcept t) { //3 1077 if (t == null) 1078 return this; 1079 if (this.type == null) 1080 this.type = new ArrayList<CodeableConcept>(); 1081 this.type.add(t); 1082 return this; 1083 } 1084 1085 /** 1086 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 1087 */ 1088 public CodeableConcept getTypeFirstRep() { 1089 if (getType().isEmpty()) { 1090 addType(); 1091 } 1092 return getType().get(0); 1093 } 1094 1095 /** 1096 * @return {@link #diagnosis} (The list of diagnosis relevant to this episode of care.) 1097 */ 1098 public List<DiagnosisComponent> getDiagnosis() { 1099 if (this.diagnosis == null) 1100 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1101 return this.diagnosis; 1102 } 1103 1104 /** 1105 * @return Returns a reference to <code>this</code> for easy method chaining 1106 */ 1107 public EpisodeOfCare setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 1108 this.diagnosis = theDiagnosis; 1109 return this; 1110 } 1111 1112 public boolean hasDiagnosis() { 1113 if (this.diagnosis == null) 1114 return false; 1115 for (DiagnosisComponent item : this.diagnosis) 1116 if (!item.isEmpty()) 1117 return true; 1118 return false; 1119 } 1120 1121 public DiagnosisComponent addDiagnosis() { //3 1122 DiagnosisComponent t = new DiagnosisComponent(); 1123 if (this.diagnosis == null) 1124 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1125 this.diagnosis.add(t); 1126 return t; 1127 } 1128 1129 public EpisodeOfCare addDiagnosis(DiagnosisComponent t) { //3 1130 if (t == null) 1131 return this; 1132 if (this.diagnosis == null) 1133 this.diagnosis = new ArrayList<DiagnosisComponent>(); 1134 this.diagnosis.add(t); 1135 return this; 1136 } 1137 1138 /** 1139 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist 1140 */ 1141 public DiagnosisComponent getDiagnosisFirstRep() { 1142 if (getDiagnosis().isEmpty()) { 1143 addDiagnosis(); 1144 } 1145 return getDiagnosis().get(0); 1146 } 1147 1148 /** 1149 * @return {@link #patient} (The patient who is the focus of this episode of care.) 1150 */ 1151 public Reference getPatient() { 1152 if (this.patient == null) 1153 if (Configuration.errorOnAutoCreate()) 1154 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1155 else if (Configuration.doAutoCreate()) 1156 this.patient = new Reference(); // cc 1157 return this.patient; 1158 } 1159 1160 public boolean hasPatient() { 1161 return this.patient != null && !this.patient.isEmpty(); 1162 } 1163 1164 /** 1165 * @param value {@link #patient} (The patient who is the focus of this episode of care.) 1166 */ 1167 public EpisodeOfCare setPatient(Reference value) { 1168 this.patient = value; 1169 return this; 1170 } 1171 1172 /** 1173 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient who is the focus of this episode of care.) 1174 */ 1175 public Patient getPatientTarget() { 1176 if (this.patientTarget == null) 1177 if (Configuration.errorOnAutoCreate()) 1178 throw new Error("Attempt to auto-create EpisodeOfCare.patient"); 1179 else if (Configuration.doAutoCreate()) 1180 this.patientTarget = new Patient(); // aa 1181 return this.patientTarget; 1182 } 1183 1184 /** 1185 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient who is the focus of this episode of care.) 1186 */ 1187 public EpisodeOfCare setPatientTarget(Patient value) { 1188 this.patientTarget = value; 1189 return this; 1190 } 1191 1192 /** 1193 * @return {@link #managingOrganization} (The organization that has assumed the specific responsibilities for the specified duration.) 1194 */ 1195 public Reference getManagingOrganization() { 1196 if (this.managingOrganization == null) 1197 if (Configuration.errorOnAutoCreate()) 1198 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1199 else if (Configuration.doAutoCreate()) 1200 this.managingOrganization = new Reference(); // cc 1201 return this.managingOrganization; 1202 } 1203 1204 public boolean hasManagingOrganization() { 1205 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1206 } 1207 1208 /** 1209 * @param value {@link #managingOrganization} (The organization that has assumed the specific responsibilities for the specified duration.) 1210 */ 1211 public EpisodeOfCare setManagingOrganization(Reference value) { 1212 this.managingOrganization = value; 1213 return this; 1214 } 1215 1216 /** 1217 * @return {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization that has assumed the specific responsibilities for the specified duration.) 1218 */ 1219 public Organization getManagingOrganizationTarget() { 1220 if (this.managingOrganizationTarget == null) 1221 if (Configuration.errorOnAutoCreate()) 1222 throw new Error("Attempt to auto-create EpisodeOfCare.managingOrganization"); 1223 else if (Configuration.doAutoCreate()) 1224 this.managingOrganizationTarget = new Organization(); // aa 1225 return this.managingOrganizationTarget; 1226 } 1227 1228 /** 1229 * @param value {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization that has assumed the specific responsibilities for the specified duration.) 1230 */ 1231 public EpisodeOfCare setManagingOrganizationTarget(Organization value) { 1232 this.managingOrganizationTarget = value; 1233 return this; 1234 } 1235 1236 /** 1237 * @return {@link #period} (The interval during which the managing organization assumes the defined responsibility.) 1238 */ 1239 public Period getPeriod() { 1240 if (this.period == null) 1241 if (Configuration.errorOnAutoCreate()) 1242 throw new Error("Attempt to auto-create EpisodeOfCare.period"); 1243 else if (Configuration.doAutoCreate()) 1244 this.period = new Period(); // cc 1245 return this.period; 1246 } 1247 1248 public boolean hasPeriod() { 1249 return this.period != null && !this.period.isEmpty(); 1250 } 1251 1252 /** 1253 * @param value {@link #period} (The interval during which the managing organization assumes the defined responsibility.) 1254 */ 1255 public EpisodeOfCare setPeriod(Period value) { 1256 this.period = value; 1257 return this; 1258 } 1259 1260 /** 1261 * @return {@link #referralRequest} (Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.) 1262 */ 1263 public List<Reference> getReferralRequest() { 1264 if (this.referralRequest == null) 1265 this.referralRequest = new ArrayList<Reference>(); 1266 return this.referralRequest; 1267 } 1268 1269 /** 1270 * @return Returns a reference to <code>this</code> for easy method chaining 1271 */ 1272 public EpisodeOfCare setReferralRequest(List<Reference> theReferralRequest) { 1273 this.referralRequest = theReferralRequest; 1274 return this; 1275 } 1276 1277 public boolean hasReferralRequest() { 1278 if (this.referralRequest == null) 1279 return false; 1280 for (Reference item : this.referralRequest) 1281 if (!item.isEmpty()) 1282 return true; 1283 return false; 1284 } 1285 1286 public Reference addReferralRequest() { //3 1287 Reference t = new Reference(); 1288 if (this.referralRequest == null) 1289 this.referralRequest = new ArrayList<Reference>(); 1290 this.referralRequest.add(t); 1291 return t; 1292 } 1293 1294 public EpisodeOfCare addReferralRequest(Reference t) { //3 1295 if (t == null) 1296 return this; 1297 if (this.referralRequest == null) 1298 this.referralRequest = new ArrayList<Reference>(); 1299 this.referralRequest.add(t); 1300 return this; 1301 } 1302 1303 /** 1304 * @return The first repetition of repeating field {@link #referralRequest}, creating it if it does not already exist 1305 */ 1306 public Reference getReferralRequestFirstRep() { 1307 if (getReferralRequest().isEmpty()) { 1308 addReferralRequest(); 1309 } 1310 return getReferralRequest().get(0); 1311 } 1312 1313 /** 1314 * @deprecated Use Reference#setResource(IBaseResource) instead 1315 */ 1316 @Deprecated 1317 public List<ReferralRequest> getReferralRequestTarget() { 1318 if (this.referralRequestTarget == null) 1319 this.referralRequestTarget = new ArrayList<ReferralRequest>(); 1320 return this.referralRequestTarget; 1321 } 1322 1323 /** 1324 * @deprecated Use Reference#setResource(IBaseResource) instead 1325 */ 1326 @Deprecated 1327 public ReferralRequest addReferralRequestTarget() { 1328 ReferralRequest r = new ReferralRequest(); 1329 if (this.referralRequestTarget == null) 1330 this.referralRequestTarget = new ArrayList<ReferralRequest>(); 1331 this.referralRequestTarget.add(r); 1332 return r; 1333 } 1334 1335 /** 1336 * @return {@link #careManager} (The practitioner that is the care manager/care co-ordinator for this patient.) 1337 */ 1338 public Reference getCareManager() { 1339 if (this.careManager == null) 1340 if (Configuration.errorOnAutoCreate()) 1341 throw new Error("Attempt to auto-create EpisodeOfCare.careManager"); 1342 else if (Configuration.doAutoCreate()) 1343 this.careManager = new Reference(); // cc 1344 return this.careManager; 1345 } 1346 1347 public boolean hasCareManager() { 1348 return this.careManager != null && !this.careManager.isEmpty(); 1349 } 1350 1351 /** 1352 * @param value {@link #careManager} (The practitioner that is the care manager/care co-ordinator for this patient.) 1353 */ 1354 public EpisodeOfCare setCareManager(Reference value) { 1355 this.careManager = value; 1356 return this; 1357 } 1358 1359 /** 1360 * @return {@link #careManager} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The practitioner that is the care manager/care co-ordinator for this patient.) 1361 */ 1362 public Practitioner getCareManagerTarget() { 1363 if (this.careManagerTarget == null) 1364 if (Configuration.errorOnAutoCreate()) 1365 throw new Error("Attempt to auto-create EpisodeOfCare.careManager"); 1366 else if (Configuration.doAutoCreate()) 1367 this.careManagerTarget = new Practitioner(); // aa 1368 return this.careManagerTarget; 1369 } 1370 1371 /** 1372 * @param value {@link #careManager} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The practitioner that is the care manager/care co-ordinator for this patient.) 1373 */ 1374 public EpisodeOfCare setCareManagerTarget(Practitioner value) { 1375 this.careManagerTarget = value; 1376 return this; 1377 } 1378 1379 /** 1380 * @return {@link #team} (The list of practitioners that may be facilitating this episode of care for specific purposes.) 1381 */ 1382 public List<Reference> getTeam() { 1383 if (this.team == null) 1384 this.team = new ArrayList<Reference>(); 1385 return this.team; 1386 } 1387 1388 /** 1389 * @return Returns a reference to <code>this</code> for easy method chaining 1390 */ 1391 public EpisodeOfCare setTeam(List<Reference> theTeam) { 1392 this.team = theTeam; 1393 return this; 1394 } 1395 1396 public boolean hasTeam() { 1397 if (this.team == null) 1398 return false; 1399 for (Reference item : this.team) 1400 if (!item.isEmpty()) 1401 return true; 1402 return false; 1403 } 1404 1405 public Reference addTeam() { //3 1406 Reference t = new Reference(); 1407 if (this.team == null) 1408 this.team = new ArrayList<Reference>(); 1409 this.team.add(t); 1410 return t; 1411 } 1412 1413 public EpisodeOfCare addTeam(Reference t) { //3 1414 if (t == null) 1415 return this; 1416 if (this.team == null) 1417 this.team = new ArrayList<Reference>(); 1418 this.team.add(t); 1419 return this; 1420 } 1421 1422 /** 1423 * @return The first repetition of repeating field {@link #team}, creating it if it does not already exist 1424 */ 1425 public Reference getTeamFirstRep() { 1426 if (getTeam().isEmpty()) { 1427 addTeam(); 1428 } 1429 return getTeam().get(0); 1430 } 1431 1432 /** 1433 * @deprecated Use Reference#setResource(IBaseResource) instead 1434 */ 1435 @Deprecated 1436 public List<CareTeam> getTeamTarget() { 1437 if (this.teamTarget == null) 1438 this.teamTarget = new ArrayList<CareTeam>(); 1439 return this.teamTarget; 1440 } 1441 1442 /** 1443 * @deprecated Use Reference#setResource(IBaseResource) instead 1444 */ 1445 @Deprecated 1446 public CareTeam addTeamTarget() { 1447 CareTeam r = new CareTeam(); 1448 if (this.teamTarget == null) 1449 this.teamTarget = new ArrayList<CareTeam>(); 1450 this.teamTarget.add(r); 1451 return r; 1452 } 1453 1454 /** 1455 * @return {@link #account} (The set of accounts that may be used for billing for this EpisodeOfCare.) 1456 */ 1457 public List<Reference> getAccount() { 1458 if (this.account == null) 1459 this.account = new ArrayList<Reference>(); 1460 return this.account; 1461 } 1462 1463 /** 1464 * @return Returns a reference to <code>this</code> for easy method chaining 1465 */ 1466 public EpisodeOfCare setAccount(List<Reference> theAccount) { 1467 this.account = theAccount; 1468 return this; 1469 } 1470 1471 public boolean hasAccount() { 1472 if (this.account == null) 1473 return false; 1474 for (Reference item : this.account) 1475 if (!item.isEmpty()) 1476 return true; 1477 return false; 1478 } 1479 1480 public Reference addAccount() { //3 1481 Reference t = new Reference(); 1482 if (this.account == null) 1483 this.account = new ArrayList<Reference>(); 1484 this.account.add(t); 1485 return t; 1486 } 1487 1488 public EpisodeOfCare addAccount(Reference t) { //3 1489 if (t == null) 1490 return this; 1491 if (this.account == null) 1492 this.account = new ArrayList<Reference>(); 1493 this.account.add(t); 1494 return this; 1495 } 1496 1497 /** 1498 * @return The first repetition of repeating field {@link #account}, creating it if it does not already exist 1499 */ 1500 public Reference getAccountFirstRep() { 1501 if (getAccount().isEmpty()) { 1502 addAccount(); 1503 } 1504 return getAccount().get(0); 1505 } 1506 1507 /** 1508 * @deprecated Use Reference#setResource(IBaseResource) instead 1509 */ 1510 @Deprecated 1511 public List<Account> getAccountTarget() { 1512 if (this.accountTarget == null) 1513 this.accountTarget = new ArrayList<Account>(); 1514 return this.accountTarget; 1515 } 1516 1517 /** 1518 * @deprecated Use Reference#setResource(IBaseResource) instead 1519 */ 1520 @Deprecated 1521 public Account addAccountTarget() { 1522 Account r = new Account(); 1523 if (this.accountTarget == null) 1524 this.accountTarget = new ArrayList<Account>(); 1525 this.accountTarget.add(r); 1526 return r; 1527 } 1528 1529 protected void listChildren(List<Property> children) { 1530 super.listChildren(children); 1531 children.add(new Property("identifier", "Identifier", "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1532 children.add(new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status)); 1533 children.add(new Property("statusHistory", "", "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 0, java.lang.Integer.MAX_VALUE, statusHistory)); 1534 children.add(new Property("type", "CodeableConcept", "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.", 0, java.lang.Integer.MAX_VALUE, type)); 1535 children.add(new Property("diagnosis", "", "The list of diagnosis relevant to this episode of care.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 1536 children.add(new Property("patient", "Reference(Patient)", "The patient who is the focus of this episode of care.", 0, 1, patient)); 1537 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization that has assumed the specific responsibilities for the specified duration.", 0, 1, managingOrganization)); 1538 children.add(new Property("period", "Period", "The interval during which the managing organization assumes the defined responsibility.", 0, 1, period)); 1539 children.add(new Property("referralRequest", "Reference(ReferralRequest)", "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, java.lang.Integer.MAX_VALUE, referralRequest)); 1540 children.add(new Property("careManager", "Reference(Practitioner)", "The practitioner that is the care manager/care co-ordinator for this patient.", 0, 1, careManager)); 1541 children.add(new Property("team", "Reference(CareTeam)", "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, java.lang.Integer.MAX_VALUE, team)); 1542 children.add(new Property("account", "Reference(Account)", "The set of accounts that may be used for billing for this EpisodeOfCare.", 0, java.lang.Integer.MAX_VALUE, account)); 1543 } 1544 1545 @Override 1546 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1547 switch (_hash) { 1548 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The EpisodeOfCare may be known by different identifiers for different contexts of use, such as when an external agency is tracking the Episode for funding purposes.", 0, java.lang.Integer.MAX_VALUE, identifier); 1549 case -892481550: /*status*/ return new Property("status", "code", "planned | waitlist | active | onhold | finished | cancelled.", 0, 1, status); 1550 case -986695614: /*statusHistory*/ return new Property("statusHistory", "", "The history of statuses that the EpisodeOfCare has been through (without requiring processing the history of the resource).", 0, java.lang.Integer.MAX_VALUE, statusHistory); 1551 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A classification of the type of episode of care; e.g. specialist referral, disease management, type of funded care.", 0, java.lang.Integer.MAX_VALUE, type); 1552 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "The list of diagnosis relevant to this episode of care.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 1553 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient who is the focus of this episode of care.", 0, 1, patient); 1554 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization that has assumed the specific responsibilities for the specified duration.", 0, 1, managingOrganization); 1555 case -991726143: /*period*/ return new Property("period", "Period", "The interval during which the managing organization assumes the defined responsibility.", 0, 1, period); 1556 case -310299598: /*referralRequest*/ return new Property("referralRequest", "Reference(ReferralRequest)", "Referral Request(s) that are fulfilled by this EpisodeOfCare, incoming referrals.", 0, java.lang.Integer.MAX_VALUE, referralRequest); 1557 case -1147746468: /*careManager*/ return new Property("careManager", "Reference(Practitioner)", "The practitioner that is the care manager/care co-ordinator for this patient.", 0, 1, careManager); 1558 case 3555933: /*team*/ return new Property("team", "Reference(CareTeam)", "The list of practitioners that may be facilitating this episode of care for specific purposes.", 0, java.lang.Integer.MAX_VALUE, team); 1559 case -1177318867: /*account*/ return new Property("account", "Reference(Account)", "The set of accounts that may be used for billing for this EpisodeOfCare.", 0, java.lang.Integer.MAX_VALUE, account); 1560 default: return super.getNamedProperty(_hash, _name, _checkValid); 1561 } 1562 1563 } 1564 1565 @Override 1566 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1567 switch (hash) { 1568 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1569 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<EpisodeOfCareStatus> 1570 case -986695614: /*statusHistory*/ return this.statusHistory == null ? new Base[0] : this.statusHistory.toArray(new Base[this.statusHistory.size()]); // EpisodeOfCareStatusHistoryComponent 1571 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1572 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 1573 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1574 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 1575 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 1576 case -310299598: /*referralRequest*/ return this.referralRequest == null ? new Base[0] : this.referralRequest.toArray(new Base[this.referralRequest.size()]); // Reference 1577 case -1147746468: /*careManager*/ return this.careManager == null ? new Base[0] : new Base[] {this.careManager}; // Reference 1578 case 3555933: /*team*/ return this.team == null ? new Base[0] : this.team.toArray(new Base[this.team.size()]); // Reference 1579 case -1177318867: /*account*/ return this.account == null ? new Base[0] : this.account.toArray(new Base[this.account.size()]); // Reference 1580 default: return super.getProperty(hash, name, checkValid); 1581 } 1582 1583 } 1584 1585 @Override 1586 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1587 switch (hash) { 1588 case -1618432855: // identifier 1589 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1590 return value; 1591 case -892481550: // status 1592 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 1593 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1594 return value; 1595 case -986695614: // statusHistory 1596 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); // EpisodeOfCareStatusHistoryComponent 1597 return value; 1598 case 3575610: // type 1599 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1600 return value; 1601 case 1196993265: // diagnosis 1602 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 1603 return value; 1604 case -791418107: // patient 1605 this.patient = castToReference(value); // Reference 1606 return value; 1607 case -2058947787: // managingOrganization 1608 this.managingOrganization = castToReference(value); // Reference 1609 return value; 1610 case -991726143: // period 1611 this.period = castToPeriod(value); // Period 1612 return value; 1613 case -310299598: // referralRequest 1614 this.getReferralRequest().add(castToReference(value)); // Reference 1615 return value; 1616 case -1147746468: // careManager 1617 this.careManager = castToReference(value); // Reference 1618 return value; 1619 case 3555933: // team 1620 this.getTeam().add(castToReference(value)); // Reference 1621 return value; 1622 case -1177318867: // account 1623 this.getAccount().add(castToReference(value)); // Reference 1624 return value; 1625 default: return super.setProperty(hash, name, value); 1626 } 1627 1628 } 1629 1630 @Override 1631 public Base setProperty(String name, Base value) throws FHIRException { 1632 if (name.equals("identifier")) { 1633 this.getIdentifier().add(castToIdentifier(value)); 1634 } else if (name.equals("status")) { 1635 value = new EpisodeOfCareStatusEnumFactory().fromType(castToCode(value)); 1636 this.status = (Enumeration) value; // Enumeration<EpisodeOfCareStatus> 1637 } else if (name.equals("statusHistory")) { 1638 this.getStatusHistory().add((EpisodeOfCareStatusHistoryComponent) value); 1639 } else if (name.equals("type")) { 1640 this.getType().add(castToCodeableConcept(value)); 1641 } else if (name.equals("diagnosis")) { 1642 this.getDiagnosis().add((DiagnosisComponent) value); 1643 } else if (name.equals("patient")) { 1644 this.patient = castToReference(value); // Reference 1645 } else if (name.equals("managingOrganization")) { 1646 this.managingOrganization = castToReference(value); // Reference 1647 } else if (name.equals("period")) { 1648 this.period = castToPeriod(value); // Period 1649 } else if (name.equals("referralRequest")) { 1650 this.getReferralRequest().add(castToReference(value)); 1651 } else if (name.equals("careManager")) { 1652 this.careManager = castToReference(value); // Reference 1653 } else if (name.equals("team")) { 1654 this.getTeam().add(castToReference(value)); 1655 } else if (name.equals("account")) { 1656 this.getAccount().add(castToReference(value)); 1657 } else 1658 return super.setProperty(name, value); 1659 return value; 1660 } 1661 1662 @Override 1663 public Base makeProperty(int hash, String name) throws FHIRException { 1664 switch (hash) { 1665 case -1618432855: return addIdentifier(); 1666 case -892481550: return getStatusElement(); 1667 case -986695614: return addStatusHistory(); 1668 case 3575610: return addType(); 1669 case 1196993265: return addDiagnosis(); 1670 case -791418107: return getPatient(); 1671 case -2058947787: return getManagingOrganization(); 1672 case -991726143: return getPeriod(); 1673 case -310299598: return addReferralRequest(); 1674 case -1147746468: return getCareManager(); 1675 case 3555933: return addTeam(); 1676 case -1177318867: return addAccount(); 1677 default: return super.makeProperty(hash, name); 1678 } 1679 1680 } 1681 1682 @Override 1683 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1684 switch (hash) { 1685 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1686 case -892481550: /*status*/ return new String[] {"code"}; 1687 case -986695614: /*statusHistory*/ return new String[] {}; 1688 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1689 case 1196993265: /*diagnosis*/ return new String[] {}; 1690 case -791418107: /*patient*/ return new String[] {"Reference"}; 1691 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1692 case -991726143: /*period*/ return new String[] {"Period"}; 1693 case -310299598: /*referralRequest*/ return new String[] {"Reference"}; 1694 case -1147746468: /*careManager*/ return new String[] {"Reference"}; 1695 case 3555933: /*team*/ return new String[] {"Reference"}; 1696 case -1177318867: /*account*/ return new String[] {"Reference"}; 1697 default: return super.getTypesForProperty(hash, name); 1698 } 1699 1700 } 1701 1702 @Override 1703 public Base addChild(String name) throws FHIRException { 1704 if (name.equals("identifier")) { 1705 return addIdentifier(); 1706 } 1707 else if (name.equals("status")) { 1708 throw new FHIRException("Cannot call addChild on a singleton property EpisodeOfCare.status"); 1709 } 1710 else if (name.equals("statusHistory")) { 1711 return addStatusHistory(); 1712 } 1713 else if (name.equals("type")) { 1714 return addType(); 1715 } 1716 else if (name.equals("diagnosis")) { 1717 return addDiagnosis(); 1718 } 1719 else if (name.equals("patient")) { 1720 this.patient = new Reference(); 1721 return this.patient; 1722 } 1723 else if (name.equals("managingOrganization")) { 1724 this.managingOrganization = new Reference(); 1725 return this.managingOrganization; 1726 } 1727 else if (name.equals("period")) { 1728 this.period = new Period(); 1729 return this.period; 1730 } 1731 else if (name.equals("referralRequest")) { 1732 return addReferralRequest(); 1733 } 1734 else if (name.equals("careManager")) { 1735 this.careManager = new Reference(); 1736 return this.careManager; 1737 } 1738 else if (name.equals("team")) { 1739 return addTeam(); 1740 } 1741 else if (name.equals("account")) { 1742 return addAccount(); 1743 } 1744 else 1745 return super.addChild(name); 1746 } 1747 1748 public String fhirType() { 1749 return "EpisodeOfCare"; 1750 1751 } 1752 1753 public EpisodeOfCare copy() { 1754 EpisodeOfCare dst = new EpisodeOfCare(); 1755 copyValues(dst); 1756 if (identifier != null) { 1757 dst.identifier = new ArrayList<Identifier>(); 1758 for (Identifier i : identifier) 1759 dst.identifier.add(i.copy()); 1760 }; 1761 dst.status = status == null ? null : status.copy(); 1762 if (statusHistory != null) { 1763 dst.statusHistory = new ArrayList<EpisodeOfCareStatusHistoryComponent>(); 1764 for (EpisodeOfCareStatusHistoryComponent i : statusHistory) 1765 dst.statusHistory.add(i.copy()); 1766 }; 1767 if (type != null) { 1768 dst.type = new ArrayList<CodeableConcept>(); 1769 for (CodeableConcept i : type) 1770 dst.type.add(i.copy()); 1771 }; 1772 if (diagnosis != null) { 1773 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 1774 for (DiagnosisComponent i : diagnosis) 1775 dst.diagnosis.add(i.copy()); 1776 }; 1777 dst.patient = patient == null ? null : patient.copy(); 1778 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1779 dst.period = period == null ? null : period.copy(); 1780 if (referralRequest != null) { 1781 dst.referralRequest = new ArrayList<Reference>(); 1782 for (Reference i : referralRequest) 1783 dst.referralRequest.add(i.copy()); 1784 }; 1785 dst.careManager = careManager == null ? null : careManager.copy(); 1786 if (team != null) { 1787 dst.team = new ArrayList<Reference>(); 1788 for (Reference i : team) 1789 dst.team.add(i.copy()); 1790 }; 1791 if (account != null) { 1792 dst.account = new ArrayList<Reference>(); 1793 for (Reference i : account) 1794 dst.account.add(i.copy()); 1795 }; 1796 return dst; 1797 } 1798 1799 protected EpisodeOfCare typedCopy() { 1800 return copy(); 1801 } 1802 1803 @Override 1804 public boolean equalsDeep(Base other_) { 1805 if (!super.equalsDeep(other_)) 1806 return false; 1807 if (!(other_ instanceof EpisodeOfCare)) 1808 return false; 1809 EpisodeOfCare o = (EpisodeOfCare) other_; 1810 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(statusHistory, o.statusHistory, true) 1811 && compareDeep(type, o.type, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(patient, o.patient, true) 1812 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(period, o.period, true) 1813 && compareDeep(referralRequest, o.referralRequest, true) && compareDeep(careManager, o.careManager, true) 1814 && compareDeep(team, o.team, true) && compareDeep(account, o.account, true); 1815 } 1816 1817 @Override 1818 public boolean equalsShallow(Base other_) { 1819 if (!super.equalsShallow(other_)) 1820 return false; 1821 if (!(other_ instanceof EpisodeOfCare)) 1822 return false; 1823 EpisodeOfCare o = (EpisodeOfCare) other_; 1824 return compareValues(status, o.status, true); 1825 } 1826 1827 public boolean isEmpty() { 1828 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, statusHistory 1829 , type, diagnosis, patient, managingOrganization, period, referralRequest, careManager 1830 , team, account); 1831 } 1832 1833 @Override 1834 public ResourceType getResourceType() { 1835 return ResourceType.EpisodeOfCare; 1836 } 1837 1838 /** 1839 * Search parameter: <b>date</b> 1840 * <p> 1841 * Description: <b>The provided date search value falls within the episode of care's period</b><br> 1842 * Type: <b>date</b><br> 1843 * Path: <b>EpisodeOfCare.period</b><br> 1844 * </p> 1845 */ 1846 @SearchParamDefinition(name="date", path="EpisodeOfCare.period", description="The provided date search value falls within the episode of care's period", type="date" ) 1847 public static final String SP_DATE = "date"; 1848 /** 1849 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1850 * <p> 1851 * Description: <b>The provided date search value falls within the episode of care's period</b><br> 1852 * Type: <b>date</b><br> 1853 * Path: <b>EpisodeOfCare.period</b><br> 1854 * </p> 1855 */ 1856 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1857 1858 /** 1859 * Search parameter: <b>identifier</b> 1860 * <p> 1861 * Description: <b>Business Identifier(s) relevant for this EpisodeOfCare</b><br> 1862 * Type: <b>token</b><br> 1863 * Path: <b>EpisodeOfCare.identifier</b><br> 1864 * </p> 1865 */ 1866 @SearchParamDefinition(name="identifier", path="EpisodeOfCare.identifier", description="Business Identifier(s) relevant for this EpisodeOfCare", type="token" ) 1867 public static final String SP_IDENTIFIER = "identifier"; 1868 /** 1869 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1870 * <p> 1871 * Description: <b>Business Identifier(s) relevant for this EpisodeOfCare</b><br> 1872 * Type: <b>token</b><br> 1873 * Path: <b>EpisodeOfCare.identifier</b><br> 1874 * </p> 1875 */ 1876 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1877 1878 /** 1879 * Search parameter: <b>condition</b> 1880 * <p> 1881 * Description: <b>Conditions/problems/diagnoses this episode of care is for</b><br> 1882 * Type: <b>reference</b><br> 1883 * Path: <b>EpisodeOfCare.diagnosis.condition</b><br> 1884 * </p> 1885 */ 1886 @SearchParamDefinition(name="condition", path="EpisodeOfCare.diagnosis.condition", description="Conditions/problems/diagnoses this episode of care is for", type="reference", target={Condition.class } ) 1887 public static final String SP_CONDITION = "condition"; 1888 /** 1889 * <b>Fluent Client</b> search parameter constant for <b>condition</b> 1890 * <p> 1891 * Description: <b>Conditions/problems/diagnoses this episode of care is for</b><br> 1892 * Type: <b>reference</b><br> 1893 * Path: <b>EpisodeOfCare.diagnosis.condition</b><br> 1894 * </p> 1895 */ 1896 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONDITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONDITION); 1897 1898/** 1899 * Constant for fluent queries to be used to add include statements. Specifies 1900 * the path value of "<b>EpisodeOfCare:condition</b>". 1901 */ 1902 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONDITION = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:condition").toLocked(); 1903 1904 /** 1905 * Search parameter: <b>incomingreferral</b> 1906 * <p> 1907 * Description: <b>Incoming Referral Request</b><br> 1908 * Type: <b>reference</b><br> 1909 * Path: <b>EpisodeOfCare.referralRequest</b><br> 1910 * </p> 1911 */ 1912 @SearchParamDefinition(name="incomingreferral", path="EpisodeOfCare.referralRequest", description="Incoming Referral Request", type="reference", target={ReferralRequest.class } ) 1913 public static final String SP_INCOMINGREFERRAL = "incomingreferral"; 1914 /** 1915 * <b>Fluent Client</b> search parameter constant for <b>incomingreferral</b> 1916 * <p> 1917 * Description: <b>Incoming Referral Request</b><br> 1918 * Type: <b>reference</b><br> 1919 * Path: <b>EpisodeOfCare.referralRequest</b><br> 1920 * </p> 1921 */ 1922 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INCOMINGREFERRAL = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INCOMINGREFERRAL); 1923 1924/** 1925 * Constant for fluent queries to be used to add include statements. Specifies 1926 * the path value of "<b>EpisodeOfCare:incomingreferral</b>". 1927 */ 1928 public static final ca.uhn.fhir.model.api.Include INCLUDE_INCOMINGREFERRAL = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:incomingreferral").toLocked(); 1929 1930 /** 1931 * Search parameter: <b>patient</b> 1932 * <p> 1933 * Description: <b>The patient who is the focus of this episode of care</b><br> 1934 * Type: <b>reference</b><br> 1935 * Path: <b>EpisodeOfCare.patient</b><br> 1936 * </p> 1937 */ 1938 @SearchParamDefinition(name="patient", path="EpisodeOfCare.patient", description="The patient who is the focus of this episode of care", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1939 public static final String SP_PATIENT = "patient"; 1940 /** 1941 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1942 * <p> 1943 * Description: <b>The patient who is the focus of this episode of care</b><br> 1944 * Type: <b>reference</b><br> 1945 * Path: <b>EpisodeOfCare.patient</b><br> 1946 * </p> 1947 */ 1948 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1949 1950/** 1951 * Constant for fluent queries to be used to add include statements. Specifies 1952 * the path value of "<b>EpisodeOfCare:patient</b>". 1953 */ 1954 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:patient").toLocked(); 1955 1956 /** 1957 * Search parameter: <b>organization</b> 1958 * <p> 1959 * Description: <b>The organization that has assumed the specific responsibilities of this EpisodeOfCare</b><br> 1960 * Type: <b>reference</b><br> 1961 * Path: <b>EpisodeOfCare.managingOrganization</b><br> 1962 * </p> 1963 */ 1964 @SearchParamDefinition(name="organization", path="EpisodeOfCare.managingOrganization", description="The organization that has assumed the specific responsibilities of this EpisodeOfCare", type="reference", target={Organization.class } ) 1965 public static final String SP_ORGANIZATION = "organization"; 1966 /** 1967 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1968 * <p> 1969 * Description: <b>The organization that has assumed the specific responsibilities of this EpisodeOfCare</b><br> 1970 * Type: <b>reference</b><br> 1971 * Path: <b>EpisodeOfCare.managingOrganization</b><br> 1972 * </p> 1973 */ 1974 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 1975 1976/** 1977 * Constant for fluent queries to be used to add include statements. Specifies 1978 * the path value of "<b>EpisodeOfCare:organization</b>". 1979 */ 1980 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:organization").toLocked(); 1981 1982 /** 1983 * Search parameter: <b>type</b> 1984 * <p> 1985 * Description: <b>Type/class - e.g. specialist referral, disease management</b><br> 1986 * Type: <b>token</b><br> 1987 * Path: <b>EpisodeOfCare.type</b><br> 1988 * </p> 1989 */ 1990 @SearchParamDefinition(name="type", path="EpisodeOfCare.type", description="Type/class - e.g. specialist referral, disease management", type="token" ) 1991 public static final String SP_TYPE = "type"; 1992 /** 1993 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1994 * <p> 1995 * Description: <b>Type/class - e.g. specialist referral, disease management</b><br> 1996 * Type: <b>token</b><br> 1997 * Path: <b>EpisodeOfCare.type</b><br> 1998 * </p> 1999 */ 2000 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2001 2002 /** 2003 * Search parameter: <b>care-manager</b> 2004 * <p> 2005 * Description: <b>Care manager/care co-ordinator for the patient</b><br> 2006 * Type: <b>reference</b><br> 2007 * Path: <b>EpisodeOfCare.careManager</b><br> 2008 * </p> 2009 */ 2010 @SearchParamDefinition(name="care-manager", path="EpisodeOfCare.careManager", description="Care manager/care co-ordinator for the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 2011 public static final String SP_CARE_MANAGER = "care-manager"; 2012 /** 2013 * <b>Fluent Client</b> search parameter constant for <b>care-manager</b> 2014 * <p> 2015 * Description: <b>Care manager/care co-ordinator for the patient</b><br> 2016 * Type: <b>reference</b><br> 2017 * Path: <b>EpisodeOfCare.careManager</b><br> 2018 * </p> 2019 */ 2020 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_MANAGER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_MANAGER); 2021 2022/** 2023 * Constant for fluent queries to be used to add include statements. Specifies 2024 * the path value of "<b>EpisodeOfCare:care-manager</b>". 2025 */ 2026 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_MANAGER = new ca.uhn.fhir.model.api.Include("EpisodeOfCare:care-manager").toLocked(); 2027 2028 /** 2029 * Search parameter: <b>status</b> 2030 * <p> 2031 * Description: <b>The current status of the Episode of Care as provided (does not check the status history collection)</b><br> 2032 * Type: <b>token</b><br> 2033 * Path: <b>EpisodeOfCare.status</b><br> 2034 * </p> 2035 */ 2036 @SearchParamDefinition(name="status", path="EpisodeOfCare.status", description="The current status of the Episode of Care as provided (does not check the status history collection)", type="token" ) 2037 public static final String SP_STATUS = "status"; 2038 /** 2039 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2040 * <p> 2041 * Description: <b>The current status of the Episode of Care as provided (does not check the status history collection)</b><br> 2042 * Type: <b>token</b><br> 2043 * Path: <b>EpisodeOfCare.status</b><br> 2044 * </p> 2045 */ 2046 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2047 2048 2049}