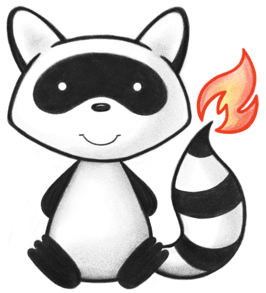
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * Resource to define constraints on the Expansion of a FHIR ValueSet. 053 */ 054@ResourceDef(name="ExpansionProfile", profile="http://hl7.org/fhir/Profile/ExpansionProfile") 055@ChildOrder(names={"url", "identifier", "version", "name", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "fixedVersion", "excludedSystem", "includeDesignations", "designation", "includeDefinition", "activeOnly", "excludeNested", "excludeNotForUI", "excludePostCoordinated", "displayLanguage", "limitedExpansion"}) 056public class ExpansionProfile extends MetadataResource { 057 058 public enum SystemVersionProcessingMode { 059 /** 060 * Use this version of the code system if a value set doesn't specify a version 061 */ 062 DEFAULT, 063 /** 064 * Use this version of the code system. If a value set specifies a different version, the expansion operation should fail 065 */ 066 CHECK, 067 /** 068 * Use this version of the code system irrespective of which version is specified by a value set. Note that this has obvious safety issues, in that it may result in a value set expansion giving a different list of codes that is both wrong and unsafe, and implementers should only use this capability reluctantly. It primarily exists to deal with situations where specifications have fallen into decay as time passes. If a version is override, the version used SHALL explicitly be represented in the expansion parameters 069 */ 070 OVERRIDE, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static SystemVersionProcessingMode fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("default".equals(codeString)) 079 return DEFAULT; 080 if ("check".equals(codeString)) 081 return CHECK; 082 if ("override".equals(codeString)) 083 return OVERRIDE; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown SystemVersionProcessingMode code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case DEFAULT: return "default"; 092 case CHECK: return "check"; 093 case OVERRIDE: return "override"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case DEFAULT: return "http://hl7.org/fhir/system-version-processing-mode"; 101 case CHECK: return "http://hl7.org/fhir/system-version-processing-mode"; 102 case OVERRIDE: return "http://hl7.org/fhir/system-version-processing-mode"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getDefinition() { 108 switch (this) { 109 case DEFAULT: return "Use this version of the code system if a value set doesn't specify a version"; 110 case CHECK: return "Use this version of the code system. If a value set specifies a different version, the expansion operation should fail"; 111 case OVERRIDE: return "Use this version of the code system irrespective of which version is specified by a value set. Note that this has obvious safety issues, in that it may result in a value set expansion giving a different list of codes that is both wrong and unsafe, and implementers should only use this capability reluctantly. It primarily exists to deal with situations where specifications have fallen into decay as time passes. If a version is override, the version used SHALL explicitly be represented in the expansion parameters"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDisplay() { 117 switch (this) { 118 case DEFAULT: return "Default Version"; 119 case CHECK: return "Check ValueSet Version"; 120 case OVERRIDE: return "Override ValueSet Version"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 } 126 127 public static class SystemVersionProcessingModeEnumFactory implements EnumFactory<SystemVersionProcessingMode> { 128 public SystemVersionProcessingMode fromCode(String codeString) throws IllegalArgumentException { 129 if (codeString == null || "".equals(codeString)) 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("default".equals(codeString)) 133 return SystemVersionProcessingMode.DEFAULT; 134 if ("check".equals(codeString)) 135 return SystemVersionProcessingMode.CHECK; 136 if ("override".equals(codeString)) 137 return SystemVersionProcessingMode.OVERRIDE; 138 throw new IllegalArgumentException("Unknown SystemVersionProcessingMode code '"+codeString+"'"); 139 } 140 public Enumeration<SystemVersionProcessingMode> fromType(PrimitiveType<?> code) throws FHIRException { 141 if (code == null) 142 return null; 143 if (code.isEmpty()) 144 return new Enumeration<SystemVersionProcessingMode>(this); 145 String codeString = code.asStringValue(); 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("default".equals(codeString)) 149 return new Enumeration<SystemVersionProcessingMode>(this, SystemVersionProcessingMode.DEFAULT); 150 if ("check".equals(codeString)) 151 return new Enumeration<SystemVersionProcessingMode>(this, SystemVersionProcessingMode.CHECK); 152 if ("override".equals(codeString)) 153 return new Enumeration<SystemVersionProcessingMode>(this, SystemVersionProcessingMode.OVERRIDE); 154 throw new FHIRException("Unknown SystemVersionProcessingMode code '"+codeString+"'"); 155 } 156 public String toCode(SystemVersionProcessingMode code) { 157 if (code == SystemVersionProcessingMode.DEFAULT) 158 return "default"; 159 if (code == SystemVersionProcessingMode.CHECK) 160 return "check"; 161 if (code == SystemVersionProcessingMode.OVERRIDE) 162 return "override"; 163 return "?"; 164 } 165 public String toSystem(SystemVersionProcessingMode code) { 166 return code.getSystem(); 167 } 168 } 169 170 @Block() 171 public static class ExpansionProfileFixedVersionComponent extends BackboneElement implements IBaseBackboneElement { 172 /** 173 * The specific system for which to fix the version. 174 */ 175 @Child(name = "system", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 176 @Description(shortDefinition="System to have its version fixed", formalDefinition="The specific system for which to fix the version." ) 177 protected UriType system; 178 179 /** 180 * The version of the code system from which codes in the expansion should be included. 181 */ 182 @Child(name = "version", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 183 @Description(shortDefinition="Specific version of the code system referred to", formalDefinition="The version of the code system from which codes in the expansion should be included." ) 184 protected StringType version; 185 186 /** 187 * How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile. 188 */ 189 @Child(name = "mode", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 190 @Description(shortDefinition="default | check | override", formalDefinition="How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile." ) 191 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/system-version-processing-mode") 192 protected Enumeration<SystemVersionProcessingMode> mode; 193 194 private static final long serialVersionUID = 1818466753L; 195 196 /** 197 * Constructor 198 */ 199 public ExpansionProfileFixedVersionComponent() { 200 super(); 201 } 202 203 /** 204 * Constructor 205 */ 206 public ExpansionProfileFixedVersionComponent(UriType system, StringType version, Enumeration<SystemVersionProcessingMode> mode) { 207 super(); 208 this.system = system; 209 this.version = version; 210 this.mode = mode; 211 } 212 213 /** 214 * @return {@link #system} (The specific system for which to fix the version.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 215 */ 216 public UriType getSystemElement() { 217 if (this.system == null) 218 if (Configuration.errorOnAutoCreate()) 219 throw new Error("Attempt to auto-create ExpansionProfileFixedVersionComponent.system"); 220 else if (Configuration.doAutoCreate()) 221 this.system = new UriType(); // bb 222 return this.system; 223 } 224 225 public boolean hasSystemElement() { 226 return this.system != null && !this.system.isEmpty(); 227 } 228 229 public boolean hasSystem() { 230 return this.system != null && !this.system.isEmpty(); 231 } 232 233 /** 234 * @param value {@link #system} (The specific system for which to fix the version.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 235 */ 236 public ExpansionProfileFixedVersionComponent setSystemElement(UriType value) { 237 this.system = value; 238 return this; 239 } 240 241 /** 242 * @return The specific system for which to fix the version. 243 */ 244 public String getSystem() { 245 return this.system == null ? null : this.system.getValue(); 246 } 247 248 /** 249 * @param value The specific system for which to fix the version. 250 */ 251 public ExpansionProfileFixedVersionComponent setSystem(String value) { 252 if (this.system == null) 253 this.system = new UriType(); 254 this.system.setValue(value); 255 return this; 256 } 257 258 /** 259 * @return {@link #version} (The version of the code system from which codes in the expansion should be included.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 260 */ 261 public StringType getVersionElement() { 262 if (this.version == null) 263 if (Configuration.errorOnAutoCreate()) 264 throw new Error("Attempt to auto-create ExpansionProfileFixedVersionComponent.version"); 265 else if (Configuration.doAutoCreate()) 266 this.version = new StringType(); // bb 267 return this.version; 268 } 269 270 public boolean hasVersionElement() { 271 return this.version != null && !this.version.isEmpty(); 272 } 273 274 public boolean hasVersion() { 275 return this.version != null && !this.version.isEmpty(); 276 } 277 278 /** 279 * @param value {@link #version} (The version of the code system from which codes in the expansion should be included.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 280 */ 281 public ExpansionProfileFixedVersionComponent setVersionElement(StringType value) { 282 this.version = value; 283 return this; 284 } 285 286 /** 287 * @return The version of the code system from which codes in the expansion should be included. 288 */ 289 public String getVersion() { 290 return this.version == null ? null : this.version.getValue(); 291 } 292 293 /** 294 * @param value The version of the code system from which codes in the expansion should be included. 295 */ 296 public ExpansionProfileFixedVersionComponent setVersion(String value) { 297 if (this.version == null) 298 this.version = new StringType(); 299 this.version.setValue(value); 300 return this; 301 } 302 303 /** 304 * @return {@link #mode} (How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 305 */ 306 public Enumeration<SystemVersionProcessingMode> getModeElement() { 307 if (this.mode == null) 308 if (Configuration.errorOnAutoCreate()) 309 throw new Error("Attempt to auto-create ExpansionProfileFixedVersionComponent.mode"); 310 else if (Configuration.doAutoCreate()) 311 this.mode = new Enumeration<SystemVersionProcessingMode>(new SystemVersionProcessingModeEnumFactory()); // bb 312 return this.mode; 313 } 314 315 public boolean hasModeElement() { 316 return this.mode != null && !this.mode.isEmpty(); 317 } 318 319 public boolean hasMode() { 320 return this.mode != null && !this.mode.isEmpty(); 321 } 322 323 /** 324 * @param value {@link #mode} (How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 325 */ 326 public ExpansionProfileFixedVersionComponent setModeElement(Enumeration<SystemVersionProcessingMode> value) { 327 this.mode = value; 328 return this; 329 } 330 331 /** 332 * @return How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile. 333 */ 334 public SystemVersionProcessingMode getMode() { 335 return this.mode == null ? null : this.mode.getValue(); 336 } 337 338 /** 339 * @param value How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile. 340 */ 341 public ExpansionProfileFixedVersionComponent setMode(SystemVersionProcessingMode value) { 342 if (this.mode == null) 343 this.mode = new Enumeration<SystemVersionProcessingMode>(new SystemVersionProcessingModeEnumFactory()); 344 this.mode.setValue(value); 345 return this; 346 } 347 348 protected void listChildren(List<Property> children) { 349 super.listChildren(children); 350 children.add(new Property("system", "uri", "The specific system for which to fix the version.", 0, 1, system)); 351 children.add(new Property("version", "string", "The version of the code system from which codes in the expansion should be included.", 0, 1, version)); 352 children.add(new Property("mode", "code", "How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile.", 0, 1, mode)); 353 } 354 355 @Override 356 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 357 switch (_hash) { 358 case -887328209: /*system*/ return new Property("system", "uri", "The specific system for which to fix the version.", 0, 1, system); 359 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system from which codes in the expansion should be included.", 0, 1, version); 360 case 3357091: /*mode*/ return new Property("mode", "code", "How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile.", 0, 1, mode); 361 default: return super.getNamedProperty(_hash, _name, _checkValid); 362 } 363 364 } 365 366 @Override 367 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 368 switch (hash) { 369 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 370 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 371 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<SystemVersionProcessingMode> 372 default: return super.getProperty(hash, name, checkValid); 373 } 374 375 } 376 377 @Override 378 public Base setProperty(int hash, String name, Base value) throws FHIRException { 379 switch (hash) { 380 case -887328209: // system 381 this.system = castToUri(value); // UriType 382 return value; 383 case 351608024: // version 384 this.version = castToString(value); // StringType 385 return value; 386 case 3357091: // mode 387 value = new SystemVersionProcessingModeEnumFactory().fromType(castToCode(value)); 388 this.mode = (Enumeration) value; // Enumeration<SystemVersionProcessingMode> 389 return value; 390 default: return super.setProperty(hash, name, value); 391 } 392 393 } 394 395 @Override 396 public Base setProperty(String name, Base value) throws FHIRException { 397 if (name.equals("system")) { 398 this.system = castToUri(value); // UriType 399 } else if (name.equals("version")) { 400 this.version = castToString(value); // StringType 401 } else if (name.equals("mode")) { 402 value = new SystemVersionProcessingModeEnumFactory().fromType(castToCode(value)); 403 this.mode = (Enumeration) value; // Enumeration<SystemVersionProcessingMode> 404 } else 405 return super.setProperty(name, value); 406 return value; 407 } 408 409 @Override 410 public Base makeProperty(int hash, String name) throws FHIRException { 411 switch (hash) { 412 case -887328209: return getSystemElement(); 413 case 351608024: return getVersionElement(); 414 case 3357091: return getModeElement(); 415 default: return super.makeProperty(hash, name); 416 } 417 418 } 419 420 @Override 421 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 422 switch (hash) { 423 case -887328209: /*system*/ return new String[] {"uri"}; 424 case 351608024: /*version*/ return new String[] {"string"}; 425 case 3357091: /*mode*/ return new String[] {"code"}; 426 default: return super.getTypesForProperty(hash, name); 427 } 428 429 } 430 431 @Override 432 public Base addChild(String name) throws FHIRException { 433 if (name.equals("system")) { 434 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.system"); 435 } 436 else if (name.equals("version")) { 437 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.version"); 438 } 439 else if (name.equals("mode")) { 440 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.mode"); 441 } 442 else 443 return super.addChild(name); 444 } 445 446 public ExpansionProfileFixedVersionComponent copy() { 447 ExpansionProfileFixedVersionComponent dst = new ExpansionProfileFixedVersionComponent(); 448 copyValues(dst); 449 dst.system = system == null ? null : system.copy(); 450 dst.version = version == null ? null : version.copy(); 451 dst.mode = mode == null ? null : mode.copy(); 452 return dst; 453 } 454 455 @Override 456 public boolean equalsDeep(Base other_) { 457 if (!super.equalsDeep(other_)) 458 return false; 459 if (!(other_ instanceof ExpansionProfileFixedVersionComponent)) 460 return false; 461 ExpansionProfileFixedVersionComponent o = (ExpansionProfileFixedVersionComponent) other_; 462 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) && compareDeep(mode, o.mode, true) 463 ; 464 } 465 466 @Override 467 public boolean equalsShallow(Base other_) { 468 if (!super.equalsShallow(other_)) 469 return false; 470 if (!(other_ instanceof ExpansionProfileFixedVersionComponent)) 471 return false; 472 ExpansionProfileFixedVersionComponent o = (ExpansionProfileFixedVersionComponent) other_; 473 return compareValues(system, o.system, true) && compareValues(version, o.version, true) && compareValues(mode, o.mode, true) 474 ; 475 } 476 477 public boolean isEmpty() { 478 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version, mode); 479 } 480 481 public String fhirType() { 482 return "ExpansionProfile.fixedVersion"; 483 484 } 485 486 } 487 488 @Block() 489 public static class ExpansionProfileExcludedSystemComponent extends BackboneElement implements IBaseBackboneElement { 490 /** 491 * An absolute URI which is the code system to be excluded. 492 */ 493 @Child(name = "system", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 494 @Description(shortDefinition="The specific code system to be excluded", formalDefinition="An absolute URI which is the code system to be excluded." ) 495 protected UriType system; 496 497 /** 498 * The version of the code system from which codes in the expansion should be excluded. 499 */ 500 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 501 @Description(shortDefinition="Specific version of the code system referred to", formalDefinition="The version of the code system from which codes in the expansion should be excluded." ) 502 protected StringType version; 503 504 private static final long serialVersionUID = 1145288774L; 505 506 /** 507 * Constructor 508 */ 509 public ExpansionProfileExcludedSystemComponent() { 510 super(); 511 } 512 513 /** 514 * Constructor 515 */ 516 public ExpansionProfileExcludedSystemComponent(UriType system) { 517 super(); 518 this.system = system; 519 } 520 521 /** 522 * @return {@link #system} (An absolute URI which is the code system to be excluded.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 523 */ 524 public UriType getSystemElement() { 525 if (this.system == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create ExpansionProfileExcludedSystemComponent.system"); 528 else if (Configuration.doAutoCreate()) 529 this.system = new UriType(); // bb 530 return this.system; 531 } 532 533 public boolean hasSystemElement() { 534 return this.system != null && !this.system.isEmpty(); 535 } 536 537 public boolean hasSystem() { 538 return this.system != null && !this.system.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #system} (An absolute URI which is the code system to be excluded.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 543 */ 544 public ExpansionProfileExcludedSystemComponent setSystemElement(UriType value) { 545 this.system = value; 546 return this; 547 } 548 549 /** 550 * @return An absolute URI which is the code system to be excluded. 551 */ 552 public String getSystem() { 553 return this.system == null ? null : this.system.getValue(); 554 } 555 556 /** 557 * @param value An absolute URI which is the code system to be excluded. 558 */ 559 public ExpansionProfileExcludedSystemComponent setSystem(String value) { 560 if (this.system == null) 561 this.system = new UriType(); 562 this.system.setValue(value); 563 return this; 564 } 565 566 /** 567 * @return {@link #version} (The version of the code system from which codes in the expansion should be excluded.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 568 */ 569 public StringType getVersionElement() { 570 if (this.version == null) 571 if (Configuration.errorOnAutoCreate()) 572 throw new Error("Attempt to auto-create ExpansionProfileExcludedSystemComponent.version"); 573 else if (Configuration.doAutoCreate()) 574 this.version = new StringType(); // bb 575 return this.version; 576 } 577 578 public boolean hasVersionElement() { 579 return this.version != null && !this.version.isEmpty(); 580 } 581 582 public boolean hasVersion() { 583 return this.version != null && !this.version.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #version} (The version of the code system from which codes in the expansion should be excluded.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 588 */ 589 public ExpansionProfileExcludedSystemComponent setVersionElement(StringType value) { 590 this.version = value; 591 return this; 592 } 593 594 /** 595 * @return The version of the code system from which codes in the expansion should be excluded. 596 */ 597 public String getVersion() { 598 return this.version == null ? null : this.version.getValue(); 599 } 600 601 /** 602 * @param value The version of the code system from which codes in the expansion should be excluded. 603 */ 604 public ExpansionProfileExcludedSystemComponent setVersion(String value) { 605 if (Utilities.noString(value)) 606 this.version = null; 607 else { 608 if (this.version == null) 609 this.version = new StringType(); 610 this.version.setValue(value); 611 } 612 return this; 613 } 614 615 protected void listChildren(List<Property> children) { 616 super.listChildren(children); 617 children.add(new Property("system", "uri", "An absolute URI which is the code system to be excluded.", 0, 1, system)); 618 children.add(new Property("version", "string", "The version of the code system from which codes in the expansion should be excluded.", 0, 1, version)); 619 } 620 621 @Override 622 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 623 switch (_hash) { 624 case -887328209: /*system*/ return new Property("system", "uri", "An absolute URI which is the code system to be excluded.", 0, 1, system); 625 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system from which codes in the expansion should be excluded.", 0, 1, version); 626 default: return super.getNamedProperty(_hash, _name, _checkValid); 627 } 628 629 } 630 631 @Override 632 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 633 switch (hash) { 634 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 635 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 636 default: return super.getProperty(hash, name, checkValid); 637 } 638 639 } 640 641 @Override 642 public Base setProperty(int hash, String name, Base value) throws FHIRException { 643 switch (hash) { 644 case -887328209: // system 645 this.system = castToUri(value); // UriType 646 return value; 647 case 351608024: // version 648 this.version = castToString(value); // StringType 649 return value; 650 default: return super.setProperty(hash, name, value); 651 } 652 653 } 654 655 @Override 656 public Base setProperty(String name, Base value) throws FHIRException { 657 if (name.equals("system")) { 658 this.system = castToUri(value); // UriType 659 } else if (name.equals("version")) { 660 this.version = castToString(value); // StringType 661 } else 662 return super.setProperty(name, value); 663 return value; 664 } 665 666 @Override 667 public Base makeProperty(int hash, String name) throws FHIRException { 668 switch (hash) { 669 case -887328209: return getSystemElement(); 670 case 351608024: return getVersionElement(); 671 default: return super.makeProperty(hash, name); 672 } 673 674 } 675 676 @Override 677 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 678 switch (hash) { 679 case -887328209: /*system*/ return new String[] {"uri"}; 680 case 351608024: /*version*/ return new String[] {"string"}; 681 default: return super.getTypesForProperty(hash, name); 682 } 683 684 } 685 686 @Override 687 public Base addChild(String name) throws FHIRException { 688 if (name.equals("system")) { 689 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.system"); 690 } 691 else if (name.equals("version")) { 692 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.version"); 693 } 694 else 695 return super.addChild(name); 696 } 697 698 public ExpansionProfileExcludedSystemComponent copy() { 699 ExpansionProfileExcludedSystemComponent dst = new ExpansionProfileExcludedSystemComponent(); 700 copyValues(dst); 701 dst.system = system == null ? null : system.copy(); 702 dst.version = version == null ? null : version.copy(); 703 return dst; 704 } 705 706 @Override 707 public boolean equalsDeep(Base other_) { 708 if (!super.equalsDeep(other_)) 709 return false; 710 if (!(other_ instanceof ExpansionProfileExcludedSystemComponent)) 711 return false; 712 ExpansionProfileExcludedSystemComponent o = (ExpansionProfileExcludedSystemComponent) other_; 713 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true); 714 } 715 716 @Override 717 public boolean equalsShallow(Base other_) { 718 if (!super.equalsShallow(other_)) 719 return false; 720 if (!(other_ instanceof ExpansionProfileExcludedSystemComponent)) 721 return false; 722 ExpansionProfileExcludedSystemComponent o = (ExpansionProfileExcludedSystemComponent) other_; 723 return compareValues(system, o.system, true) && compareValues(version, o.version, true); 724 } 725 726 public boolean isEmpty() { 727 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version); 728 } 729 730 public String fhirType() { 731 return "ExpansionProfile.excludedSystem"; 732 733 } 734 735 } 736 737 @Block() 738 public static class ExpansionProfileDesignationComponent extends BackboneElement implements IBaseBackboneElement { 739 /** 740 * Designations to be included. 741 */ 742 @Child(name = "include", type = {}, order=1, min=0, max=1, modifier=false, summary=true) 743 @Description(shortDefinition="Designations to be included", formalDefinition="Designations to be included." ) 744 protected DesignationIncludeComponent include; 745 746 /** 747 * Designations to be excluded. 748 */ 749 @Child(name = "exclude", type = {}, order=2, min=0, max=1, modifier=false, summary=true) 750 @Description(shortDefinition="Designations to be excluded", formalDefinition="Designations to be excluded." ) 751 protected DesignationExcludeComponent exclude; 752 753 private static final long serialVersionUID = -2080476436L; 754 755 /** 756 * Constructor 757 */ 758 public ExpansionProfileDesignationComponent() { 759 super(); 760 } 761 762 /** 763 * @return {@link #include} (Designations to be included.) 764 */ 765 public DesignationIncludeComponent getInclude() { 766 if (this.include == null) 767 if (Configuration.errorOnAutoCreate()) 768 throw new Error("Attempt to auto-create ExpansionProfileDesignationComponent.include"); 769 else if (Configuration.doAutoCreate()) 770 this.include = new DesignationIncludeComponent(); // cc 771 return this.include; 772 } 773 774 public boolean hasInclude() { 775 return this.include != null && !this.include.isEmpty(); 776 } 777 778 /** 779 * @param value {@link #include} (Designations to be included.) 780 */ 781 public ExpansionProfileDesignationComponent setInclude(DesignationIncludeComponent value) { 782 this.include = value; 783 return this; 784 } 785 786 /** 787 * @return {@link #exclude} (Designations to be excluded.) 788 */ 789 public DesignationExcludeComponent getExclude() { 790 if (this.exclude == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create ExpansionProfileDesignationComponent.exclude"); 793 else if (Configuration.doAutoCreate()) 794 this.exclude = new DesignationExcludeComponent(); // cc 795 return this.exclude; 796 } 797 798 public boolean hasExclude() { 799 return this.exclude != null && !this.exclude.isEmpty(); 800 } 801 802 /** 803 * @param value {@link #exclude} (Designations to be excluded.) 804 */ 805 public ExpansionProfileDesignationComponent setExclude(DesignationExcludeComponent value) { 806 this.exclude = value; 807 return this; 808 } 809 810 protected void listChildren(List<Property> children) { 811 super.listChildren(children); 812 children.add(new Property("include", "", "Designations to be included.", 0, 1, include)); 813 children.add(new Property("exclude", "", "Designations to be excluded.", 0, 1, exclude)); 814 } 815 816 @Override 817 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 818 switch (_hash) { 819 case 1942574248: /*include*/ return new Property("include", "", "Designations to be included.", 0, 1, include); 820 case -1321148966: /*exclude*/ return new Property("exclude", "", "Designations to be excluded.", 0, 1, exclude); 821 default: return super.getNamedProperty(_hash, _name, _checkValid); 822 } 823 824 } 825 826 @Override 827 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 828 switch (hash) { 829 case 1942574248: /*include*/ return this.include == null ? new Base[0] : new Base[] {this.include}; // DesignationIncludeComponent 830 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : new Base[] {this.exclude}; // DesignationExcludeComponent 831 default: return super.getProperty(hash, name, checkValid); 832 } 833 834 } 835 836 @Override 837 public Base setProperty(int hash, String name, Base value) throws FHIRException { 838 switch (hash) { 839 case 1942574248: // include 840 this.include = (DesignationIncludeComponent) value; // DesignationIncludeComponent 841 return value; 842 case -1321148966: // exclude 843 this.exclude = (DesignationExcludeComponent) value; // DesignationExcludeComponent 844 return value; 845 default: return super.setProperty(hash, name, value); 846 } 847 848 } 849 850 @Override 851 public Base setProperty(String name, Base value) throws FHIRException { 852 if (name.equals("include")) { 853 this.include = (DesignationIncludeComponent) value; // DesignationIncludeComponent 854 } else if (name.equals("exclude")) { 855 this.exclude = (DesignationExcludeComponent) value; // DesignationExcludeComponent 856 } else 857 return super.setProperty(name, value); 858 return value; 859 } 860 861 @Override 862 public Base makeProperty(int hash, String name) throws FHIRException { 863 switch (hash) { 864 case 1942574248: return getInclude(); 865 case -1321148966: return getExclude(); 866 default: return super.makeProperty(hash, name); 867 } 868 869 } 870 871 @Override 872 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 873 switch (hash) { 874 case 1942574248: /*include*/ return new String[] {}; 875 case -1321148966: /*exclude*/ return new String[] {}; 876 default: return super.getTypesForProperty(hash, name); 877 } 878 879 } 880 881 @Override 882 public Base addChild(String name) throws FHIRException { 883 if (name.equals("include")) { 884 this.include = new DesignationIncludeComponent(); 885 return this.include; 886 } 887 else if (name.equals("exclude")) { 888 this.exclude = new DesignationExcludeComponent(); 889 return this.exclude; 890 } 891 else 892 return super.addChild(name); 893 } 894 895 public ExpansionProfileDesignationComponent copy() { 896 ExpansionProfileDesignationComponent dst = new ExpansionProfileDesignationComponent(); 897 copyValues(dst); 898 dst.include = include == null ? null : include.copy(); 899 dst.exclude = exclude == null ? null : exclude.copy(); 900 return dst; 901 } 902 903 @Override 904 public boolean equalsDeep(Base other_) { 905 if (!super.equalsDeep(other_)) 906 return false; 907 if (!(other_ instanceof ExpansionProfileDesignationComponent)) 908 return false; 909 ExpansionProfileDesignationComponent o = (ExpansionProfileDesignationComponent) other_; 910 return compareDeep(include, o.include, true) && compareDeep(exclude, o.exclude, true); 911 } 912 913 @Override 914 public boolean equalsShallow(Base other_) { 915 if (!super.equalsShallow(other_)) 916 return false; 917 if (!(other_ instanceof ExpansionProfileDesignationComponent)) 918 return false; 919 ExpansionProfileDesignationComponent o = (ExpansionProfileDesignationComponent) other_; 920 return true; 921 } 922 923 public boolean isEmpty() { 924 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(include, exclude); 925 } 926 927 public String fhirType() { 928 return "ExpansionProfile.designation"; 929 930 } 931 932 } 933 934 @Block() 935 public static class DesignationIncludeComponent extends BackboneElement implements IBaseBackboneElement { 936 /** 937 * A data group for each designation to be included. 938 */ 939 @Child(name = "designation", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 940 @Description(shortDefinition="The designation to be included", formalDefinition="A data group for each designation to be included." ) 941 protected List<DesignationIncludeDesignationComponent> designation; 942 943 private static final long serialVersionUID = -1989669274L; 944 945 /** 946 * Constructor 947 */ 948 public DesignationIncludeComponent() { 949 super(); 950 } 951 952 /** 953 * @return {@link #designation} (A data group for each designation to be included.) 954 */ 955 public List<DesignationIncludeDesignationComponent> getDesignation() { 956 if (this.designation == null) 957 this.designation = new ArrayList<DesignationIncludeDesignationComponent>(); 958 return this.designation; 959 } 960 961 /** 962 * @return Returns a reference to <code>this</code> for easy method chaining 963 */ 964 public DesignationIncludeComponent setDesignation(List<DesignationIncludeDesignationComponent> theDesignation) { 965 this.designation = theDesignation; 966 return this; 967 } 968 969 public boolean hasDesignation() { 970 if (this.designation == null) 971 return false; 972 for (DesignationIncludeDesignationComponent item : this.designation) 973 if (!item.isEmpty()) 974 return true; 975 return false; 976 } 977 978 public DesignationIncludeDesignationComponent addDesignation() { //3 979 DesignationIncludeDesignationComponent t = new DesignationIncludeDesignationComponent(); 980 if (this.designation == null) 981 this.designation = new ArrayList<DesignationIncludeDesignationComponent>(); 982 this.designation.add(t); 983 return t; 984 } 985 986 public DesignationIncludeComponent addDesignation(DesignationIncludeDesignationComponent t) { //3 987 if (t == null) 988 return this; 989 if (this.designation == null) 990 this.designation = new ArrayList<DesignationIncludeDesignationComponent>(); 991 this.designation.add(t); 992 return this; 993 } 994 995 /** 996 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist 997 */ 998 public DesignationIncludeDesignationComponent getDesignationFirstRep() { 999 if (getDesignation().isEmpty()) { 1000 addDesignation(); 1001 } 1002 return getDesignation().get(0); 1003 } 1004 1005 protected void listChildren(List<Property> children) { 1006 super.listChildren(children); 1007 children.add(new Property("designation", "", "A data group for each designation to be included.", 0, java.lang.Integer.MAX_VALUE, designation)); 1008 } 1009 1010 @Override 1011 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1012 switch (_hash) { 1013 case -900931593: /*designation*/ return new Property("designation", "", "A data group for each designation to be included.", 0, java.lang.Integer.MAX_VALUE, designation); 1014 default: return super.getNamedProperty(_hash, _name, _checkValid); 1015 } 1016 1017 } 1018 1019 @Override 1020 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1021 switch (hash) { 1022 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // DesignationIncludeDesignationComponent 1023 default: return super.getProperty(hash, name, checkValid); 1024 } 1025 1026 } 1027 1028 @Override 1029 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1030 switch (hash) { 1031 case -900931593: // designation 1032 this.getDesignation().add((DesignationIncludeDesignationComponent) value); // DesignationIncludeDesignationComponent 1033 return value; 1034 default: return super.setProperty(hash, name, value); 1035 } 1036 1037 } 1038 1039 @Override 1040 public Base setProperty(String name, Base value) throws FHIRException { 1041 if (name.equals("designation")) { 1042 this.getDesignation().add((DesignationIncludeDesignationComponent) value); 1043 } else 1044 return super.setProperty(name, value); 1045 return value; 1046 } 1047 1048 @Override 1049 public Base makeProperty(int hash, String name) throws FHIRException { 1050 switch (hash) { 1051 case -900931593: return addDesignation(); 1052 default: return super.makeProperty(hash, name); 1053 } 1054 1055 } 1056 1057 @Override 1058 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1059 switch (hash) { 1060 case -900931593: /*designation*/ return new String[] {}; 1061 default: return super.getTypesForProperty(hash, name); 1062 } 1063 1064 } 1065 1066 @Override 1067 public Base addChild(String name) throws FHIRException { 1068 if (name.equals("designation")) { 1069 return addDesignation(); 1070 } 1071 else 1072 return super.addChild(name); 1073 } 1074 1075 public DesignationIncludeComponent copy() { 1076 DesignationIncludeComponent dst = new DesignationIncludeComponent(); 1077 copyValues(dst); 1078 if (designation != null) { 1079 dst.designation = new ArrayList<DesignationIncludeDesignationComponent>(); 1080 for (DesignationIncludeDesignationComponent i : designation) 1081 dst.designation.add(i.copy()); 1082 }; 1083 return dst; 1084 } 1085 1086 @Override 1087 public boolean equalsDeep(Base other_) { 1088 if (!super.equalsDeep(other_)) 1089 return false; 1090 if (!(other_ instanceof DesignationIncludeComponent)) 1091 return false; 1092 DesignationIncludeComponent o = (DesignationIncludeComponent) other_; 1093 return compareDeep(designation, o.designation, true); 1094 } 1095 1096 @Override 1097 public boolean equalsShallow(Base other_) { 1098 if (!super.equalsShallow(other_)) 1099 return false; 1100 if (!(other_ instanceof DesignationIncludeComponent)) 1101 return false; 1102 DesignationIncludeComponent o = (DesignationIncludeComponent) other_; 1103 return true; 1104 } 1105 1106 public boolean isEmpty() { 1107 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(designation); 1108 } 1109 1110 public String fhirType() { 1111 return "ExpansionProfile.designation.include"; 1112 1113 } 1114 1115 } 1116 1117 @Block() 1118 public static class DesignationIncludeDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1119 /** 1120 * The language this designation is defined for. 1121 */ 1122 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1123 @Description(shortDefinition="Human language of the designation to be included", formalDefinition="The language this designation is defined for." ) 1124 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 1125 protected CodeType language; 1126 1127 /** 1128 * Which kinds of designation to include in the expansion. 1129 */ 1130 @Child(name = "use", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=true) 1131 @Description(shortDefinition="What kind of Designation to include", formalDefinition="Which kinds of designation to include in the expansion." ) 1132 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1133 protected Coding use; 1134 1135 private static final long serialVersionUID = 242239292L; 1136 1137 /** 1138 * Constructor 1139 */ 1140 public DesignationIncludeDesignationComponent() { 1141 super(); 1142 } 1143 1144 /** 1145 * @return {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1146 */ 1147 public CodeType getLanguageElement() { 1148 if (this.language == null) 1149 if (Configuration.errorOnAutoCreate()) 1150 throw new Error("Attempt to auto-create DesignationIncludeDesignationComponent.language"); 1151 else if (Configuration.doAutoCreate()) 1152 this.language = new CodeType(); // bb 1153 return this.language; 1154 } 1155 1156 public boolean hasLanguageElement() { 1157 return this.language != null && !this.language.isEmpty(); 1158 } 1159 1160 public boolean hasLanguage() { 1161 return this.language != null && !this.language.isEmpty(); 1162 } 1163 1164 /** 1165 * @param value {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1166 */ 1167 public DesignationIncludeDesignationComponent setLanguageElement(CodeType value) { 1168 this.language = value; 1169 return this; 1170 } 1171 1172 /** 1173 * @return The language this designation is defined for. 1174 */ 1175 public String getLanguage() { 1176 return this.language == null ? null : this.language.getValue(); 1177 } 1178 1179 /** 1180 * @param value The language this designation is defined for. 1181 */ 1182 public DesignationIncludeDesignationComponent setLanguage(String value) { 1183 if (Utilities.noString(value)) 1184 this.language = null; 1185 else { 1186 if (this.language == null) 1187 this.language = new CodeType(); 1188 this.language.setValue(value); 1189 } 1190 return this; 1191 } 1192 1193 /** 1194 * @return {@link #use} (Which kinds of designation to include in the expansion.) 1195 */ 1196 public Coding getUse() { 1197 if (this.use == null) 1198 if (Configuration.errorOnAutoCreate()) 1199 throw new Error("Attempt to auto-create DesignationIncludeDesignationComponent.use"); 1200 else if (Configuration.doAutoCreate()) 1201 this.use = new Coding(); // cc 1202 return this.use; 1203 } 1204 1205 public boolean hasUse() { 1206 return this.use != null && !this.use.isEmpty(); 1207 } 1208 1209 /** 1210 * @param value {@link #use} (Which kinds of designation to include in the expansion.) 1211 */ 1212 public DesignationIncludeDesignationComponent setUse(Coding value) { 1213 this.use = value; 1214 return this; 1215 } 1216 1217 protected void listChildren(List<Property> children) { 1218 super.listChildren(children); 1219 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 1220 children.add(new Property("use", "Coding", "Which kinds of designation to include in the expansion.", 0, 1, use)); 1221 } 1222 1223 @Override 1224 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1225 switch (_hash) { 1226 case -1613589672: /*language*/ return new Property("language", "code", "The language this designation is defined for.", 0, 1, language); 1227 case 116103: /*use*/ return new Property("use", "Coding", "Which kinds of designation to include in the expansion.", 0, 1, use); 1228 default: return super.getNamedProperty(_hash, _name, _checkValid); 1229 } 1230 1231 } 1232 1233 @Override 1234 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1235 switch (hash) { 1236 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 1237 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Coding 1238 default: return super.getProperty(hash, name, checkValid); 1239 } 1240 1241 } 1242 1243 @Override 1244 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1245 switch (hash) { 1246 case -1613589672: // language 1247 this.language = castToCode(value); // CodeType 1248 return value; 1249 case 116103: // use 1250 this.use = castToCoding(value); // Coding 1251 return value; 1252 default: return super.setProperty(hash, name, value); 1253 } 1254 1255 } 1256 1257 @Override 1258 public Base setProperty(String name, Base value) throws FHIRException { 1259 if (name.equals("language")) { 1260 this.language = castToCode(value); // CodeType 1261 } else if (name.equals("use")) { 1262 this.use = castToCoding(value); // Coding 1263 } else 1264 return super.setProperty(name, value); 1265 return value; 1266 } 1267 1268 @Override 1269 public Base makeProperty(int hash, String name) throws FHIRException { 1270 switch (hash) { 1271 case -1613589672: return getLanguageElement(); 1272 case 116103: return getUse(); 1273 default: return super.makeProperty(hash, name); 1274 } 1275 1276 } 1277 1278 @Override 1279 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1280 switch (hash) { 1281 case -1613589672: /*language*/ return new String[] {"code"}; 1282 case 116103: /*use*/ return new String[] {"Coding"}; 1283 default: return super.getTypesForProperty(hash, name); 1284 } 1285 1286 } 1287 1288 @Override 1289 public Base addChild(String name) throws FHIRException { 1290 if (name.equals("language")) { 1291 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.language"); 1292 } 1293 else if (name.equals("use")) { 1294 this.use = new Coding(); 1295 return this.use; 1296 } 1297 else 1298 return super.addChild(name); 1299 } 1300 1301 public DesignationIncludeDesignationComponent copy() { 1302 DesignationIncludeDesignationComponent dst = new DesignationIncludeDesignationComponent(); 1303 copyValues(dst); 1304 dst.language = language == null ? null : language.copy(); 1305 dst.use = use == null ? null : use.copy(); 1306 return dst; 1307 } 1308 1309 @Override 1310 public boolean equalsDeep(Base other_) { 1311 if (!super.equalsDeep(other_)) 1312 return false; 1313 if (!(other_ instanceof DesignationIncludeDesignationComponent)) 1314 return false; 1315 DesignationIncludeDesignationComponent o = (DesignationIncludeDesignationComponent) other_; 1316 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true); 1317 } 1318 1319 @Override 1320 public boolean equalsShallow(Base other_) { 1321 if (!super.equalsShallow(other_)) 1322 return false; 1323 if (!(other_ instanceof DesignationIncludeDesignationComponent)) 1324 return false; 1325 DesignationIncludeDesignationComponent o = (DesignationIncludeDesignationComponent) other_; 1326 return compareValues(language, o.language, true); 1327 } 1328 1329 public boolean isEmpty() { 1330 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use); 1331 } 1332 1333 public String fhirType() { 1334 return "ExpansionProfile.designation.include.designation"; 1335 1336 } 1337 1338 } 1339 1340 @Block() 1341 public static class DesignationExcludeComponent extends BackboneElement implements IBaseBackboneElement { 1342 /** 1343 * A data group for each designation to be excluded. 1344 */ 1345 @Child(name = "designation", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1346 @Description(shortDefinition="The designation to be excluded", formalDefinition="A data group for each designation to be excluded." ) 1347 protected List<DesignationExcludeDesignationComponent> designation; 1348 1349 private static final long serialVersionUID = 1045849752L; 1350 1351 /** 1352 * Constructor 1353 */ 1354 public DesignationExcludeComponent() { 1355 super(); 1356 } 1357 1358 /** 1359 * @return {@link #designation} (A data group for each designation to be excluded.) 1360 */ 1361 public List<DesignationExcludeDesignationComponent> getDesignation() { 1362 if (this.designation == null) 1363 this.designation = new ArrayList<DesignationExcludeDesignationComponent>(); 1364 return this.designation; 1365 } 1366 1367 /** 1368 * @return Returns a reference to <code>this</code> for easy method chaining 1369 */ 1370 public DesignationExcludeComponent setDesignation(List<DesignationExcludeDesignationComponent> theDesignation) { 1371 this.designation = theDesignation; 1372 return this; 1373 } 1374 1375 public boolean hasDesignation() { 1376 if (this.designation == null) 1377 return false; 1378 for (DesignationExcludeDesignationComponent item : this.designation) 1379 if (!item.isEmpty()) 1380 return true; 1381 return false; 1382 } 1383 1384 public DesignationExcludeDesignationComponent addDesignation() { //3 1385 DesignationExcludeDesignationComponent t = new DesignationExcludeDesignationComponent(); 1386 if (this.designation == null) 1387 this.designation = new ArrayList<DesignationExcludeDesignationComponent>(); 1388 this.designation.add(t); 1389 return t; 1390 } 1391 1392 public DesignationExcludeComponent addDesignation(DesignationExcludeDesignationComponent t) { //3 1393 if (t == null) 1394 return this; 1395 if (this.designation == null) 1396 this.designation = new ArrayList<DesignationExcludeDesignationComponent>(); 1397 this.designation.add(t); 1398 return this; 1399 } 1400 1401 /** 1402 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist 1403 */ 1404 public DesignationExcludeDesignationComponent getDesignationFirstRep() { 1405 if (getDesignation().isEmpty()) { 1406 addDesignation(); 1407 } 1408 return getDesignation().get(0); 1409 } 1410 1411 protected void listChildren(List<Property> children) { 1412 super.listChildren(children); 1413 children.add(new Property("designation", "", "A data group for each designation to be excluded.", 0, java.lang.Integer.MAX_VALUE, designation)); 1414 } 1415 1416 @Override 1417 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1418 switch (_hash) { 1419 case -900931593: /*designation*/ return new Property("designation", "", "A data group for each designation to be excluded.", 0, java.lang.Integer.MAX_VALUE, designation); 1420 default: return super.getNamedProperty(_hash, _name, _checkValid); 1421 } 1422 1423 } 1424 1425 @Override 1426 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1427 switch (hash) { 1428 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // DesignationExcludeDesignationComponent 1429 default: return super.getProperty(hash, name, checkValid); 1430 } 1431 1432 } 1433 1434 @Override 1435 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1436 switch (hash) { 1437 case -900931593: // designation 1438 this.getDesignation().add((DesignationExcludeDesignationComponent) value); // DesignationExcludeDesignationComponent 1439 return value; 1440 default: return super.setProperty(hash, name, value); 1441 } 1442 1443 } 1444 1445 @Override 1446 public Base setProperty(String name, Base value) throws FHIRException { 1447 if (name.equals("designation")) { 1448 this.getDesignation().add((DesignationExcludeDesignationComponent) value); 1449 } else 1450 return super.setProperty(name, value); 1451 return value; 1452 } 1453 1454 @Override 1455 public Base makeProperty(int hash, String name) throws FHIRException { 1456 switch (hash) { 1457 case -900931593: return addDesignation(); 1458 default: return super.makeProperty(hash, name); 1459 } 1460 1461 } 1462 1463 @Override 1464 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1465 switch (hash) { 1466 case -900931593: /*designation*/ return new String[] {}; 1467 default: return super.getTypesForProperty(hash, name); 1468 } 1469 1470 } 1471 1472 @Override 1473 public Base addChild(String name) throws FHIRException { 1474 if (name.equals("designation")) { 1475 return addDesignation(); 1476 } 1477 else 1478 return super.addChild(name); 1479 } 1480 1481 public DesignationExcludeComponent copy() { 1482 DesignationExcludeComponent dst = new DesignationExcludeComponent(); 1483 copyValues(dst); 1484 if (designation != null) { 1485 dst.designation = new ArrayList<DesignationExcludeDesignationComponent>(); 1486 for (DesignationExcludeDesignationComponent i : designation) 1487 dst.designation.add(i.copy()); 1488 }; 1489 return dst; 1490 } 1491 1492 @Override 1493 public boolean equalsDeep(Base other_) { 1494 if (!super.equalsDeep(other_)) 1495 return false; 1496 if (!(other_ instanceof DesignationExcludeComponent)) 1497 return false; 1498 DesignationExcludeComponent o = (DesignationExcludeComponent) other_; 1499 return compareDeep(designation, o.designation, true); 1500 } 1501 1502 @Override 1503 public boolean equalsShallow(Base other_) { 1504 if (!super.equalsShallow(other_)) 1505 return false; 1506 if (!(other_ instanceof DesignationExcludeComponent)) 1507 return false; 1508 DesignationExcludeComponent o = (DesignationExcludeComponent) other_; 1509 return true; 1510 } 1511 1512 public boolean isEmpty() { 1513 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(designation); 1514 } 1515 1516 public String fhirType() { 1517 return "ExpansionProfile.designation.exclude"; 1518 1519 } 1520 1521 } 1522 1523 @Block() 1524 public static class DesignationExcludeDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1525 /** 1526 * The language this designation is defined for. 1527 */ 1528 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1529 @Description(shortDefinition="Human language of the designation to be excluded", formalDefinition="The language this designation is defined for." ) 1530 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 1531 protected CodeType language; 1532 1533 /** 1534 * Which kinds of designation to exclude from the expansion. 1535 */ 1536 @Child(name = "use", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=true) 1537 @Description(shortDefinition="What kind of Designation to exclude", formalDefinition="Which kinds of designation to exclude from the expansion." ) 1538 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1539 protected Coding use; 1540 1541 private static final long serialVersionUID = 242239292L; 1542 1543 /** 1544 * Constructor 1545 */ 1546 public DesignationExcludeDesignationComponent() { 1547 super(); 1548 } 1549 1550 /** 1551 * @return {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1552 */ 1553 public CodeType getLanguageElement() { 1554 if (this.language == null) 1555 if (Configuration.errorOnAutoCreate()) 1556 throw new Error("Attempt to auto-create DesignationExcludeDesignationComponent.language"); 1557 else if (Configuration.doAutoCreate()) 1558 this.language = new CodeType(); // bb 1559 return this.language; 1560 } 1561 1562 public boolean hasLanguageElement() { 1563 return this.language != null && !this.language.isEmpty(); 1564 } 1565 1566 public boolean hasLanguage() { 1567 return this.language != null && !this.language.isEmpty(); 1568 } 1569 1570 /** 1571 * @param value {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1572 */ 1573 public DesignationExcludeDesignationComponent setLanguageElement(CodeType value) { 1574 this.language = value; 1575 return this; 1576 } 1577 1578 /** 1579 * @return The language this designation is defined for. 1580 */ 1581 public String getLanguage() { 1582 return this.language == null ? null : this.language.getValue(); 1583 } 1584 1585 /** 1586 * @param value The language this designation is defined for. 1587 */ 1588 public DesignationExcludeDesignationComponent setLanguage(String value) { 1589 if (Utilities.noString(value)) 1590 this.language = null; 1591 else { 1592 if (this.language == null) 1593 this.language = new CodeType(); 1594 this.language.setValue(value); 1595 } 1596 return this; 1597 } 1598 1599 /** 1600 * @return {@link #use} (Which kinds of designation to exclude from the expansion.) 1601 */ 1602 public Coding getUse() { 1603 if (this.use == null) 1604 if (Configuration.errorOnAutoCreate()) 1605 throw new Error("Attempt to auto-create DesignationExcludeDesignationComponent.use"); 1606 else if (Configuration.doAutoCreate()) 1607 this.use = new Coding(); // cc 1608 return this.use; 1609 } 1610 1611 public boolean hasUse() { 1612 return this.use != null && !this.use.isEmpty(); 1613 } 1614 1615 /** 1616 * @param value {@link #use} (Which kinds of designation to exclude from the expansion.) 1617 */ 1618 public DesignationExcludeDesignationComponent setUse(Coding value) { 1619 this.use = value; 1620 return this; 1621 } 1622 1623 protected void listChildren(List<Property> children) { 1624 super.listChildren(children); 1625 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 1626 children.add(new Property("use", "Coding", "Which kinds of designation to exclude from the expansion.", 0, 1, use)); 1627 } 1628 1629 @Override 1630 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1631 switch (_hash) { 1632 case -1613589672: /*language*/ return new Property("language", "code", "The language this designation is defined for.", 0, 1, language); 1633 case 116103: /*use*/ return new Property("use", "Coding", "Which kinds of designation to exclude from the expansion.", 0, 1, use); 1634 default: return super.getNamedProperty(_hash, _name, _checkValid); 1635 } 1636 1637 } 1638 1639 @Override 1640 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1641 switch (hash) { 1642 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 1643 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Coding 1644 default: return super.getProperty(hash, name, checkValid); 1645 } 1646 1647 } 1648 1649 @Override 1650 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1651 switch (hash) { 1652 case -1613589672: // language 1653 this.language = castToCode(value); // CodeType 1654 return value; 1655 case 116103: // use 1656 this.use = castToCoding(value); // Coding 1657 return value; 1658 default: return super.setProperty(hash, name, value); 1659 } 1660 1661 } 1662 1663 @Override 1664 public Base setProperty(String name, Base value) throws FHIRException { 1665 if (name.equals("language")) { 1666 this.language = castToCode(value); // CodeType 1667 } else if (name.equals("use")) { 1668 this.use = castToCoding(value); // Coding 1669 } else 1670 return super.setProperty(name, value); 1671 return value; 1672 } 1673 1674 @Override 1675 public Base makeProperty(int hash, String name) throws FHIRException { 1676 switch (hash) { 1677 case -1613589672: return getLanguageElement(); 1678 case 116103: return getUse(); 1679 default: return super.makeProperty(hash, name); 1680 } 1681 1682 } 1683 1684 @Override 1685 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1686 switch (hash) { 1687 case -1613589672: /*language*/ return new String[] {"code"}; 1688 case 116103: /*use*/ return new String[] {"Coding"}; 1689 default: return super.getTypesForProperty(hash, name); 1690 } 1691 1692 } 1693 1694 @Override 1695 public Base addChild(String name) throws FHIRException { 1696 if (name.equals("language")) { 1697 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.language"); 1698 } 1699 else if (name.equals("use")) { 1700 this.use = new Coding(); 1701 return this.use; 1702 } 1703 else 1704 return super.addChild(name); 1705 } 1706 1707 public DesignationExcludeDesignationComponent copy() { 1708 DesignationExcludeDesignationComponent dst = new DesignationExcludeDesignationComponent(); 1709 copyValues(dst); 1710 dst.language = language == null ? null : language.copy(); 1711 dst.use = use == null ? null : use.copy(); 1712 return dst; 1713 } 1714 1715 @Override 1716 public boolean equalsDeep(Base other_) { 1717 if (!super.equalsDeep(other_)) 1718 return false; 1719 if (!(other_ instanceof DesignationExcludeDesignationComponent)) 1720 return false; 1721 DesignationExcludeDesignationComponent o = (DesignationExcludeDesignationComponent) other_; 1722 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true); 1723 } 1724 1725 @Override 1726 public boolean equalsShallow(Base other_) { 1727 if (!super.equalsShallow(other_)) 1728 return false; 1729 if (!(other_ instanceof DesignationExcludeDesignationComponent)) 1730 return false; 1731 DesignationExcludeDesignationComponent o = (DesignationExcludeDesignationComponent) other_; 1732 return compareValues(language, o.language, true); 1733 } 1734 1735 public boolean isEmpty() { 1736 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use); 1737 } 1738 1739 public String fhirType() { 1740 return "ExpansionProfile.designation.exclude.designation"; 1741 1742 } 1743 1744 } 1745 1746 /** 1747 * A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance. 1748 */ 1749 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 1750 @Description(shortDefinition="Additional identifier for the expansion profile", formalDefinition="A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1751 protected Identifier identifier; 1752 1753 /** 1754 * Fix use of a particular code system to a particular version. 1755 */ 1756 @Child(name = "fixedVersion", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1757 @Description(shortDefinition="Fix use of a code system to a particular version", formalDefinition="Fix use of a particular code system to a particular version." ) 1758 protected List<ExpansionProfileFixedVersionComponent> fixedVersion; 1759 1760 /** 1761 * Code system, or a particular version of a code system to be excluded from value set expansions. 1762 */ 1763 @Child(name = "excludedSystem", type = {}, order=2, min=0, max=1, modifier=false, summary=true) 1764 @Description(shortDefinition="Systems/Versions to be exclude", formalDefinition="Code system, or a particular version of a code system to be excluded from value set expansions." ) 1765 protected ExpansionProfileExcludedSystemComponent excludedSystem; 1766 1767 /** 1768 * Controls whether concept designations are to be included or excluded in value set expansions. 1769 */ 1770 @Child(name = "includeDesignations", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1771 @Description(shortDefinition="Whether the expansion should include concept designations", formalDefinition="Controls whether concept designations are to be included or excluded in value set expansions." ) 1772 protected BooleanType includeDesignations; 1773 1774 /** 1775 * A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations. 1776 */ 1777 @Child(name = "designation", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 1778 @Description(shortDefinition="When the expansion profile imposes designation contraints", formalDefinition="A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations." ) 1779 protected ExpansionProfileDesignationComponent designation; 1780 1781 /** 1782 * Controls whether the value set definition is included or excluded in value set expansions. 1783 */ 1784 @Child(name = "includeDefinition", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1785 @Description(shortDefinition="Include or exclude the value set definition in the expansion", formalDefinition="Controls whether the value set definition is included or excluded in value set expansions." ) 1786 protected BooleanType includeDefinition; 1787 1788 /** 1789 * Controls whether inactive concepts are included or excluded in value set expansions. 1790 */ 1791 @Child(name = "activeOnly", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=true) 1792 @Description(shortDefinition="Include or exclude inactive concepts in the expansion", formalDefinition="Controls whether inactive concepts are included or excluded in value set expansions." ) 1793 protected BooleanType activeOnly; 1794 1795 /** 1796 * Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains). 1797 */ 1798 @Child(name = "excludeNested", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1799 @Description(shortDefinition="Nested codes in the expansion or not", formalDefinition="Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains)." ) 1800 protected BooleanType excludeNested; 1801 1802 /** 1803 * Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces. 1804 */ 1805 @Child(name = "excludeNotForUI", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1806 @Description(shortDefinition="Include or exclude codes which cannot be rendered in user interfaces in the value set expansion", formalDefinition="Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces." ) 1807 protected BooleanType excludeNotForUI; 1808 1809 /** 1810 * Controls whether or not the value set expansion includes post coordinated codes. 1811 */ 1812 @Child(name = "excludePostCoordinated", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1813 @Description(shortDefinition="Include or exclude codes which are post coordinated expressions in the value set expansion", formalDefinition="Controls whether or not the value set expansion includes post coordinated codes." ) 1814 protected BooleanType excludePostCoordinated; 1815 1816 /** 1817 * Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display. 1818 */ 1819 @Child(name = "displayLanguage", type = {CodeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1820 @Description(shortDefinition="Specify the language for the display element of codes in the value set expansion", formalDefinition="Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display." ) 1821 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 1822 protected CodeType displayLanguage; 1823 1824 /** 1825 * If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html). 1826 */ 1827 @Child(name = "limitedExpansion", type = {BooleanType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1828 @Description(shortDefinition="Controls behaviour of the value set expand operation when value sets are too large to be completely expanded", formalDefinition="If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html)." ) 1829 protected BooleanType limitedExpansion; 1830 1831 private static final long serialVersionUID = 1067457001L; 1832 1833 /** 1834 * Constructor 1835 */ 1836 public ExpansionProfile() { 1837 super(); 1838 } 1839 1840 /** 1841 * Constructor 1842 */ 1843 public ExpansionProfile(Enumeration<PublicationStatus> status) { 1844 super(); 1845 this.status = status; 1846 } 1847 1848 /** 1849 * @return {@link #url} (An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1850 */ 1851 public UriType getUrlElement() { 1852 if (this.url == null) 1853 if (Configuration.errorOnAutoCreate()) 1854 throw new Error("Attempt to auto-create ExpansionProfile.url"); 1855 else if (Configuration.doAutoCreate()) 1856 this.url = new UriType(); // bb 1857 return this.url; 1858 } 1859 1860 public boolean hasUrlElement() { 1861 return this.url != null && !this.url.isEmpty(); 1862 } 1863 1864 public boolean hasUrl() { 1865 return this.url != null && !this.url.isEmpty(); 1866 } 1867 1868 /** 1869 * @param value {@link #url} (An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1870 */ 1871 public ExpansionProfile setUrlElement(UriType value) { 1872 this.url = value; 1873 return this; 1874 } 1875 1876 /** 1877 * @return An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions). 1878 */ 1879 public String getUrl() { 1880 return this.url == null ? null : this.url.getValue(); 1881 } 1882 1883 /** 1884 * @param value An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions). 1885 */ 1886 public ExpansionProfile setUrl(String value) { 1887 if (Utilities.noString(value)) 1888 this.url = null; 1889 else { 1890 if (this.url == null) 1891 this.url = new UriType(); 1892 this.url.setValue(value); 1893 } 1894 return this; 1895 } 1896 1897 /** 1898 * @return {@link #identifier} (A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1899 */ 1900 public Identifier getIdentifier() { 1901 if (this.identifier == null) 1902 if (Configuration.errorOnAutoCreate()) 1903 throw new Error("Attempt to auto-create ExpansionProfile.identifier"); 1904 else if (Configuration.doAutoCreate()) 1905 this.identifier = new Identifier(); // cc 1906 return this.identifier; 1907 } 1908 1909 public boolean hasIdentifier() { 1910 return this.identifier != null && !this.identifier.isEmpty(); 1911 } 1912 1913 /** 1914 * @param value {@link #identifier} (A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1915 */ 1916 public ExpansionProfile setIdentifier(Identifier value) { 1917 this.identifier = value; 1918 return this; 1919 } 1920 1921 /** 1922 * @return {@link #version} (The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1923 */ 1924 public StringType getVersionElement() { 1925 if (this.version == null) 1926 if (Configuration.errorOnAutoCreate()) 1927 throw new Error("Attempt to auto-create ExpansionProfile.version"); 1928 else if (Configuration.doAutoCreate()) 1929 this.version = new StringType(); // bb 1930 return this.version; 1931 } 1932 1933 public boolean hasVersionElement() { 1934 return this.version != null && !this.version.isEmpty(); 1935 } 1936 1937 public boolean hasVersion() { 1938 return this.version != null && !this.version.isEmpty(); 1939 } 1940 1941 /** 1942 * @param value {@link #version} (The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1943 */ 1944 public ExpansionProfile setVersionElement(StringType value) { 1945 this.version = value; 1946 return this; 1947 } 1948 1949 /** 1950 * @return The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1951 */ 1952 public String getVersion() { 1953 return this.version == null ? null : this.version.getValue(); 1954 } 1955 1956 /** 1957 * @param value The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1958 */ 1959 public ExpansionProfile setVersion(String value) { 1960 if (Utilities.noString(value)) 1961 this.version = null; 1962 else { 1963 if (this.version == null) 1964 this.version = new StringType(); 1965 this.version.setValue(value); 1966 } 1967 return this; 1968 } 1969 1970 /** 1971 * @return {@link #name} (A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1972 */ 1973 public StringType getNameElement() { 1974 if (this.name == null) 1975 if (Configuration.errorOnAutoCreate()) 1976 throw new Error("Attempt to auto-create ExpansionProfile.name"); 1977 else if (Configuration.doAutoCreate()) 1978 this.name = new StringType(); // bb 1979 return this.name; 1980 } 1981 1982 public boolean hasNameElement() { 1983 return this.name != null && !this.name.isEmpty(); 1984 } 1985 1986 public boolean hasName() { 1987 return this.name != null && !this.name.isEmpty(); 1988 } 1989 1990 /** 1991 * @param value {@link #name} (A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1992 */ 1993 public ExpansionProfile setNameElement(StringType value) { 1994 this.name = value; 1995 return this; 1996 } 1997 1998 /** 1999 * @return A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2000 */ 2001 public String getName() { 2002 return this.name == null ? null : this.name.getValue(); 2003 } 2004 2005 /** 2006 * @param value A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2007 */ 2008 public ExpansionProfile setName(String value) { 2009 if (Utilities.noString(value)) 2010 this.name = null; 2011 else { 2012 if (this.name == null) 2013 this.name = new StringType(); 2014 this.name.setValue(value); 2015 } 2016 return this; 2017 } 2018 2019 /** 2020 * @return {@link #status} (The status of this expansion profile. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2021 */ 2022 public Enumeration<PublicationStatus> getStatusElement() { 2023 if (this.status == null) 2024 if (Configuration.errorOnAutoCreate()) 2025 throw new Error("Attempt to auto-create ExpansionProfile.status"); 2026 else if (Configuration.doAutoCreate()) 2027 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2028 return this.status; 2029 } 2030 2031 public boolean hasStatusElement() { 2032 return this.status != null && !this.status.isEmpty(); 2033 } 2034 2035 public boolean hasStatus() { 2036 return this.status != null && !this.status.isEmpty(); 2037 } 2038 2039 /** 2040 * @param value {@link #status} (The status of this expansion profile. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2041 */ 2042 public ExpansionProfile setStatusElement(Enumeration<PublicationStatus> value) { 2043 this.status = value; 2044 return this; 2045 } 2046 2047 /** 2048 * @return The status of this expansion profile. Enables tracking the life-cycle of the content. 2049 */ 2050 public PublicationStatus getStatus() { 2051 return this.status == null ? null : this.status.getValue(); 2052 } 2053 2054 /** 2055 * @param value The status of this expansion profile. Enables tracking the life-cycle of the content. 2056 */ 2057 public ExpansionProfile setStatus(PublicationStatus value) { 2058 if (this.status == null) 2059 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2060 this.status.setValue(value); 2061 return this; 2062 } 2063 2064 /** 2065 * @return {@link #experimental} (A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2066 */ 2067 public BooleanType getExperimentalElement() { 2068 if (this.experimental == null) 2069 if (Configuration.errorOnAutoCreate()) 2070 throw new Error("Attempt to auto-create ExpansionProfile.experimental"); 2071 else if (Configuration.doAutoCreate()) 2072 this.experimental = new BooleanType(); // bb 2073 return this.experimental; 2074 } 2075 2076 public boolean hasExperimentalElement() { 2077 return this.experimental != null && !this.experimental.isEmpty(); 2078 } 2079 2080 public boolean hasExperimental() { 2081 return this.experimental != null && !this.experimental.isEmpty(); 2082 } 2083 2084 /** 2085 * @param value {@link #experimental} (A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2086 */ 2087 public ExpansionProfile setExperimentalElement(BooleanType value) { 2088 this.experimental = value; 2089 return this; 2090 } 2091 2092 /** 2093 * @return A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2094 */ 2095 public boolean getExperimental() { 2096 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2097 } 2098 2099 /** 2100 * @param value A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2101 */ 2102 public ExpansionProfile setExperimental(boolean value) { 2103 if (this.experimental == null) 2104 this.experimental = new BooleanType(); 2105 this.experimental.setValue(value); 2106 return this; 2107 } 2108 2109 /** 2110 * @return {@link #date} (The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2111 */ 2112 public DateTimeType getDateElement() { 2113 if (this.date == null) 2114 if (Configuration.errorOnAutoCreate()) 2115 throw new Error("Attempt to auto-create ExpansionProfile.date"); 2116 else if (Configuration.doAutoCreate()) 2117 this.date = new DateTimeType(); // bb 2118 return this.date; 2119 } 2120 2121 public boolean hasDateElement() { 2122 return this.date != null && !this.date.isEmpty(); 2123 } 2124 2125 public boolean hasDate() { 2126 return this.date != null && !this.date.isEmpty(); 2127 } 2128 2129 /** 2130 * @param value {@link #date} (The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2131 */ 2132 public ExpansionProfile setDateElement(DateTimeType value) { 2133 this.date = value; 2134 return this; 2135 } 2136 2137 /** 2138 * @return The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes. 2139 */ 2140 public Date getDate() { 2141 return this.date == null ? null : this.date.getValue(); 2142 } 2143 2144 /** 2145 * @param value The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes. 2146 */ 2147 public ExpansionProfile setDate(Date value) { 2148 if (value == null) 2149 this.date = null; 2150 else { 2151 if (this.date == null) 2152 this.date = new DateTimeType(); 2153 this.date.setValue(value); 2154 } 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #publisher} (The name of the individual or organization that published the expansion profile.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2160 */ 2161 public StringType getPublisherElement() { 2162 if (this.publisher == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create ExpansionProfile.publisher"); 2165 else if (Configuration.doAutoCreate()) 2166 this.publisher = new StringType(); // bb 2167 return this.publisher; 2168 } 2169 2170 public boolean hasPublisherElement() { 2171 return this.publisher != null && !this.publisher.isEmpty(); 2172 } 2173 2174 public boolean hasPublisher() { 2175 return this.publisher != null && !this.publisher.isEmpty(); 2176 } 2177 2178 /** 2179 * @param value {@link #publisher} (The name of the individual or organization that published the expansion profile.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2180 */ 2181 public ExpansionProfile setPublisherElement(StringType value) { 2182 this.publisher = value; 2183 return this; 2184 } 2185 2186 /** 2187 * @return The name of the individual or organization that published the expansion profile. 2188 */ 2189 public String getPublisher() { 2190 return this.publisher == null ? null : this.publisher.getValue(); 2191 } 2192 2193 /** 2194 * @param value The name of the individual or organization that published the expansion profile. 2195 */ 2196 public ExpansionProfile setPublisher(String value) { 2197 if (Utilities.noString(value)) 2198 this.publisher = null; 2199 else { 2200 if (this.publisher == null) 2201 this.publisher = new StringType(); 2202 this.publisher.setValue(value); 2203 } 2204 return this; 2205 } 2206 2207 /** 2208 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2209 */ 2210 public List<ContactDetail> getContact() { 2211 if (this.contact == null) 2212 this.contact = new ArrayList<ContactDetail>(); 2213 return this.contact; 2214 } 2215 2216 /** 2217 * @return Returns a reference to <code>this</code> for easy method chaining 2218 */ 2219 public ExpansionProfile setContact(List<ContactDetail> theContact) { 2220 this.contact = theContact; 2221 return this; 2222 } 2223 2224 public boolean hasContact() { 2225 if (this.contact == null) 2226 return false; 2227 for (ContactDetail item : this.contact) 2228 if (!item.isEmpty()) 2229 return true; 2230 return false; 2231 } 2232 2233 public ContactDetail addContact() { //3 2234 ContactDetail t = new ContactDetail(); 2235 if (this.contact == null) 2236 this.contact = new ArrayList<ContactDetail>(); 2237 this.contact.add(t); 2238 return t; 2239 } 2240 2241 public ExpansionProfile addContact(ContactDetail t) { //3 2242 if (t == null) 2243 return this; 2244 if (this.contact == null) 2245 this.contact = new ArrayList<ContactDetail>(); 2246 this.contact.add(t); 2247 return this; 2248 } 2249 2250 /** 2251 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 2252 */ 2253 public ContactDetail getContactFirstRep() { 2254 if (getContact().isEmpty()) { 2255 addContact(); 2256 } 2257 return getContact().get(0); 2258 } 2259 2260 /** 2261 * @return {@link #description} (A free text natural language description of the expansion profile from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2262 */ 2263 public MarkdownType getDescriptionElement() { 2264 if (this.description == null) 2265 if (Configuration.errorOnAutoCreate()) 2266 throw new Error("Attempt to auto-create ExpansionProfile.description"); 2267 else if (Configuration.doAutoCreate()) 2268 this.description = new MarkdownType(); // bb 2269 return this.description; 2270 } 2271 2272 public boolean hasDescriptionElement() { 2273 return this.description != null && !this.description.isEmpty(); 2274 } 2275 2276 public boolean hasDescription() { 2277 return this.description != null && !this.description.isEmpty(); 2278 } 2279 2280 /** 2281 * @param value {@link #description} (A free text natural language description of the expansion profile from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2282 */ 2283 public ExpansionProfile setDescriptionElement(MarkdownType value) { 2284 this.description = value; 2285 return this; 2286 } 2287 2288 /** 2289 * @return A free text natural language description of the expansion profile from a consumer's perspective. 2290 */ 2291 public String getDescription() { 2292 return this.description == null ? null : this.description.getValue(); 2293 } 2294 2295 /** 2296 * @param value A free text natural language description of the expansion profile from a consumer's perspective. 2297 */ 2298 public ExpansionProfile setDescription(String value) { 2299 if (value == null) 2300 this.description = null; 2301 else { 2302 if (this.description == null) 2303 this.description = new MarkdownType(); 2304 this.description.setValue(value); 2305 } 2306 return this; 2307 } 2308 2309 /** 2310 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate expansion profile instances.) 2311 */ 2312 public List<UsageContext> getUseContext() { 2313 if (this.useContext == null) 2314 this.useContext = new ArrayList<UsageContext>(); 2315 return this.useContext; 2316 } 2317 2318 /** 2319 * @return Returns a reference to <code>this</code> for easy method chaining 2320 */ 2321 public ExpansionProfile setUseContext(List<UsageContext> theUseContext) { 2322 this.useContext = theUseContext; 2323 return this; 2324 } 2325 2326 public boolean hasUseContext() { 2327 if (this.useContext == null) 2328 return false; 2329 for (UsageContext item : this.useContext) 2330 if (!item.isEmpty()) 2331 return true; 2332 return false; 2333 } 2334 2335 public UsageContext addUseContext() { //3 2336 UsageContext t = new UsageContext(); 2337 if (this.useContext == null) 2338 this.useContext = new ArrayList<UsageContext>(); 2339 this.useContext.add(t); 2340 return t; 2341 } 2342 2343 public ExpansionProfile addUseContext(UsageContext t) { //3 2344 if (t == null) 2345 return this; 2346 if (this.useContext == null) 2347 this.useContext = new ArrayList<UsageContext>(); 2348 this.useContext.add(t); 2349 return this; 2350 } 2351 2352 /** 2353 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 2354 */ 2355 public UsageContext getUseContextFirstRep() { 2356 if (getUseContext().isEmpty()) { 2357 addUseContext(); 2358 } 2359 return getUseContext().get(0); 2360 } 2361 2362 /** 2363 * @return {@link #jurisdiction} (A legal or geographic region in which the expansion profile is intended to be used.) 2364 */ 2365 public List<CodeableConcept> getJurisdiction() { 2366 if (this.jurisdiction == null) 2367 this.jurisdiction = new ArrayList<CodeableConcept>(); 2368 return this.jurisdiction; 2369 } 2370 2371 /** 2372 * @return Returns a reference to <code>this</code> for easy method chaining 2373 */ 2374 public ExpansionProfile setJurisdiction(List<CodeableConcept> theJurisdiction) { 2375 this.jurisdiction = theJurisdiction; 2376 return this; 2377 } 2378 2379 public boolean hasJurisdiction() { 2380 if (this.jurisdiction == null) 2381 return false; 2382 for (CodeableConcept item : this.jurisdiction) 2383 if (!item.isEmpty()) 2384 return true; 2385 return false; 2386 } 2387 2388 public CodeableConcept addJurisdiction() { //3 2389 CodeableConcept t = new CodeableConcept(); 2390 if (this.jurisdiction == null) 2391 this.jurisdiction = new ArrayList<CodeableConcept>(); 2392 this.jurisdiction.add(t); 2393 return t; 2394 } 2395 2396 public ExpansionProfile addJurisdiction(CodeableConcept t) { //3 2397 if (t == null) 2398 return this; 2399 if (this.jurisdiction == null) 2400 this.jurisdiction = new ArrayList<CodeableConcept>(); 2401 this.jurisdiction.add(t); 2402 return this; 2403 } 2404 2405 /** 2406 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 2407 */ 2408 public CodeableConcept getJurisdictionFirstRep() { 2409 if (getJurisdiction().isEmpty()) { 2410 addJurisdiction(); 2411 } 2412 return getJurisdiction().get(0); 2413 } 2414 2415 /** 2416 * @return {@link #fixedVersion} (Fix use of a particular code system to a particular version.) 2417 */ 2418 public List<ExpansionProfileFixedVersionComponent> getFixedVersion() { 2419 if (this.fixedVersion == null) 2420 this.fixedVersion = new ArrayList<ExpansionProfileFixedVersionComponent>(); 2421 return this.fixedVersion; 2422 } 2423 2424 /** 2425 * @return Returns a reference to <code>this</code> for easy method chaining 2426 */ 2427 public ExpansionProfile setFixedVersion(List<ExpansionProfileFixedVersionComponent> theFixedVersion) { 2428 this.fixedVersion = theFixedVersion; 2429 return this; 2430 } 2431 2432 public boolean hasFixedVersion() { 2433 if (this.fixedVersion == null) 2434 return false; 2435 for (ExpansionProfileFixedVersionComponent item : this.fixedVersion) 2436 if (!item.isEmpty()) 2437 return true; 2438 return false; 2439 } 2440 2441 public ExpansionProfileFixedVersionComponent addFixedVersion() { //3 2442 ExpansionProfileFixedVersionComponent t = new ExpansionProfileFixedVersionComponent(); 2443 if (this.fixedVersion == null) 2444 this.fixedVersion = new ArrayList<ExpansionProfileFixedVersionComponent>(); 2445 this.fixedVersion.add(t); 2446 return t; 2447 } 2448 2449 public ExpansionProfile addFixedVersion(ExpansionProfileFixedVersionComponent t) { //3 2450 if (t == null) 2451 return this; 2452 if (this.fixedVersion == null) 2453 this.fixedVersion = new ArrayList<ExpansionProfileFixedVersionComponent>(); 2454 this.fixedVersion.add(t); 2455 return this; 2456 } 2457 2458 /** 2459 * @return The first repetition of repeating field {@link #fixedVersion}, creating it if it does not already exist 2460 */ 2461 public ExpansionProfileFixedVersionComponent getFixedVersionFirstRep() { 2462 if (getFixedVersion().isEmpty()) { 2463 addFixedVersion(); 2464 } 2465 return getFixedVersion().get(0); 2466 } 2467 2468 /** 2469 * @return {@link #excludedSystem} (Code system, or a particular version of a code system to be excluded from value set expansions.) 2470 */ 2471 public ExpansionProfileExcludedSystemComponent getExcludedSystem() { 2472 if (this.excludedSystem == null) 2473 if (Configuration.errorOnAutoCreate()) 2474 throw new Error("Attempt to auto-create ExpansionProfile.excludedSystem"); 2475 else if (Configuration.doAutoCreate()) 2476 this.excludedSystem = new ExpansionProfileExcludedSystemComponent(); // cc 2477 return this.excludedSystem; 2478 } 2479 2480 public boolean hasExcludedSystem() { 2481 return this.excludedSystem != null && !this.excludedSystem.isEmpty(); 2482 } 2483 2484 /** 2485 * @param value {@link #excludedSystem} (Code system, or a particular version of a code system to be excluded from value set expansions.) 2486 */ 2487 public ExpansionProfile setExcludedSystem(ExpansionProfileExcludedSystemComponent value) { 2488 this.excludedSystem = value; 2489 return this; 2490 } 2491 2492 /** 2493 * @return {@link #includeDesignations} (Controls whether concept designations are to be included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getIncludeDesignations" gives direct access to the value 2494 */ 2495 public BooleanType getIncludeDesignationsElement() { 2496 if (this.includeDesignations == null) 2497 if (Configuration.errorOnAutoCreate()) 2498 throw new Error("Attempt to auto-create ExpansionProfile.includeDesignations"); 2499 else if (Configuration.doAutoCreate()) 2500 this.includeDesignations = new BooleanType(); // bb 2501 return this.includeDesignations; 2502 } 2503 2504 public boolean hasIncludeDesignationsElement() { 2505 return this.includeDesignations != null && !this.includeDesignations.isEmpty(); 2506 } 2507 2508 public boolean hasIncludeDesignations() { 2509 return this.includeDesignations != null && !this.includeDesignations.isEmpty(); 2510 } 2511 2512 /** 2513 * @param value {@link #includeDesignations} (Controls whether concept designations are to be included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getIncludeDesignations" gives direct access to the value 2514 */ 2515 public ExpansionProfile setIncludeDesignationsElement(BooleanType value) { 2516 this.includeDesignations = value; 2517 return this; 2518 } 2519 2520 /** 2521 * @return Controls whether concept designations are to be included or excluded in value set expansions. 2522 */ 2523 public boolean getIncludeDesignations() { 2524 return this.includeDesignations == null || this.includeDesignations.isEmpty() ? false : this.includeDesignations.getValue(); 2525 } 2526 2527 /** 2528 * @param value Controls whether concept designations are to be included or excluded in value set expansions. 2529 */ 2530 public ExpansionProfile setIncludeDesignations(boolean value) { 2531 if (this.includeDesignations == null) 2532 this.includeDesignations = new BooleanType(); 2533 this.includeDesignations.setValue(value); 2534 return this; 2535 } 2536 2537 /** 2538 * @return {@link #designation} (A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations.) 2539 */ 2540 public ExpansionProfileDesignationComponent getDesignation() { 2541 if (this.designation == null) 2542 if (Configuration.errorOnAutoCreate()) 2543 throw new Error("Attempt to auto-create ExpansionProfile.designation"); 2544 else if (Configuration.doAutoCreate()) 2545 this.designation = new ExpansionProfileDesignationComponent(); // cc 2546 return this.designation; 2547 } 2548 2549 public boolean hasDesignation() { 2550 return this.designation != null && !this.designation.isEmpty(); 2551 } 2552 2553 /** 2554 * @param value {@link #designation} (A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations.) 2555 */ 2556 public ExpansionProfile setDesignation(ExpansionProfileDesignationComponent value) { 2557 this.designation = value; 2558 return this; 2559 } 2560 2561 /** 2562 * @return {@link #includeDefinition} (Controls whether the value set definition is included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getIncludeDefinition" gives direct access to the value 2563 */ 2564 public BooleanType getIncludeDefinitionElement() { 2565 if (this.includeDefinition == null) 2566 if (Configuration.errorOnAutoCreate()) 2567 throw new Error("Attempt to auto-create ExpansionProfile.includeDefinition"); 2568 else if (Configuration.doAutoCreate()) 2569 this.includeDefinition = new BooleanType(); // bb 2570 return this.includeDefinition; 2571 } 2572 2573 public boolean hasIncludeDefinitionElement() { 2574 return this.includeDefinition != null && !this.includeDefinition.isEmpty(); 2575 } 2576 2577 public boolean hasIncludeDefinition() { 2578 return this.includeDefinition != null && !this.includeDefinition.isEmpty(); 2579 } 2580 2581 /** 2582 * @param value {@link #includeDefinition} (Controls whether the value set definition is included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getIncludeDefinition" gives direct access to the value 2583 */ 2584 public ExpansionProfile setIncludeDefinitionElement(BooleanType value) { 2585 this.includeDefinition = value; 2586 return this; 2587 } 2588 2589 /** 2590 * @return Controls whether the value set definition is included or excluded in value set expansions. 2591 */ 2592 public boolean getIncludeDefinition() { 2593 return this.includeDefinition == null || this.includeDefinition.isEmpty() ? false : this.includeDefinition.getValue(); 2594 } 2595 2596 /** 2597 * @param value Controls whether the value set definition is included or excluded in value set expansions. 2598 */ 2599 public ExpansionProfile setIncludeDefinition(boolean value) { 2600 if (this.includeDefinition == null) 2601 this.includeDefinition = new BooleanType(); 2602 this.includeDefinition.setValue(value); 2603 return this; 2604 } 2605 2606 /** 2607 * @return {@link #activeOnly} (Controls whether inactive concepts are included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getActiveOnly" gives direct access to the value 2608 */ 2609 public BooleanType getActiveOnlyElement() { 2610 if (this.activeOnly == null) 2611 if (Configuration.errorOnAutoCreate()) 2612 throw new Error("Attempt to auto-create ExpansionProfile.activeOnly"); 2613 else if (Configuration.doAutoCreate()) 2614 this.activeOnly = new BooleanType(); // bb 2615 return this.activeOnly; 2616 } 2617 2618 public boolean hasActiveOnlyElement() { 2619 return this.activeOnly != null && !this.activeOnly.isEmpty(); 2620 } 2621 2622 public boolean hasActiveOnly() { 2623 return this.activeOnly != null && !this.activeOnly.isEmpty(); 2624 } 2625 2626 /** 2627 * @param value {@link #activeOnly} (Controls whether inactive concepts are included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getActiveOnly" gives direct access to the value 2628 */ 2629 public ExpansionProfile setActiveOnlyElement(BooleanType value) { 2630 this.activeOnly = value; 2631 return this; 2632 } 2633 2634 /** 2635 * @return Controls whether inactive concepts are included or excluded in value set expansions. 2636 */ 2637 public boolean getActiveOnly() { 2638 return this.activeOnly == null || this.activeOnly.isEmpty() ? false : this.activeOnly.getValue(); 2639 } 2640 2641 /** 2642 * @param value Controls whether inactive concepts are included or excluded in value set expansions. 2643 */ 2644 public ExpansionProfile setActiveOnly(boolean value) { 2645 if (this.activeOnly == null) 2646 this.activeOnly = new BooleanType(); 2647 this.activeOnly.setValue(value); 2648 return this; 2649 } 2650 2651 /** 2652 * @return {@link #excludeNested} (Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains).). This is the underlying object with id, value and extensions. The accessor "getExcludeNested" gives direct access to the value 2653 */ 2654 public BooleanType getExcludeNestedElement() { 2655 if (this.excludeNested == null) 2656 if (Configuration.errorOnAutoCreate()) 2657 throw new Error("Attempt to auto-create ExpansionProfile.excludeNested"); 2658 else if (Configuration.doAutoCreate()) 2659 this.excludeNested = new BooleanType(); // bb 2660 return this.excludeNested; 2661 } 2662 2663 public boolean hasExcludeNestedElement() { 2664 return this.excludeNested != null && !this.excludeNested.isEmpty(); 2665 } 2666 2667 public boolean hasExcludeNested() { 2668 return this.excludeNested != null && !this.excludeNested.isEmpty(); 2669 } 2670 2671 /** 2672 * @param value {@link #excludeNested} (Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains).). This is the underlying object with id, value and extensions. The accessor "getExcludeNested" gives direct access to the value 2673 */ 2674 public ExpansionProfile setExcludeNestedElement(BooleanType value) { 2675 this.excludeNested = value; 2676 return this; 2677 } 2678 2679 /** 2680 * @return Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains). 2681 */ 2682 public boolean getExcludeNested() { 2683 return this.excludeNested == null || this.excludeNested.isEmpty() ? false : this.excludeNested.getValue(); 2684 } 2685 2686 /** 2687 * @param value Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains). 2688 */ 2689 public ExpansionProfile setExcludeNested(boolean value) { 2690 if (this.excludeNested == null) 2691 this.excludeNested = new BooleanType(); 2692 this.excludeNested.setValue(value); 2693 return this; 2694 } 2695 2696 /** 2697 * @return {@link #excludeNotForUI} (Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces.). This is the underlying object with id, value and extensions. The accessor "getExcludeNotForUI" gives direct access to the value 2698 */ 2699 public BooleanType getExcludeNotForUIElement() { 2700 if (this.excludeNotForUI == null) 2701 if (Configuration.errorOnAutoCreate()) 2702 throw new Error("Attempt to auto-create ExpansionProfile.excludeNotForUI"); 2703 else if (Configuration.doAutoCreate()) 2704 this.excludeNotForUI = new BooleanType(); // bb 2705 return this.excludeNotForUI; 2706 } 2707 2708 public boolean hasExcludeNotForUIElement() { 2709 return this.excludeNotForUI != null && !this.excludeNotForUI.isEmpty(); 2710 } 2711 2712 public boolean hasExcludeNotForUI() { 2713 return this.excludeNotForUI != null && !this.excludeNotForUI.isEmpty(); 2714 } 2715 2716 /** 2717 * @param value {@link #excludeNotForUI} (Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces.). This is the underlying object with id, value and extensions. The accessor "getExcludeNotForUI" gives direct access to the value 2718 */ 2719 public ExpansionProfile setExcludeNotForUIElement(BooleanType value) { 2720 this.excludeNotForUI = value; 2721 return this; 2722 } 2723 2724 /** 2725 * @return Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces. 2726 */ 2727 public boolean getExcludeNotForUI() { 2728 return this.excludeNotForUI == null || this.excludeNotForUI.isEmpty() ? false : this.excludeNotForUI.getValue(); 2729 } 2730 2731 /** 2732 * @param value Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces. 2733 */ 2734 public ExpansionProfile setExcludeNotForUI(boolean value) { 2735 if (this.excludeNotForUI == null) 2736 this.excludeNotForUI = new BooleanType(); 2737 this.excludeNotForUI.setValue(value); 2738 return this; 2739 } 2740 2741 /** 2742 * @return {@link #excludePostCoordinated} (Controls whether or not the value set expansion includes post coordinated codes.). This is the underlying object with id, value and extensions. The accessor "getExcludePostCoordinated" gives direct access to the value 2743 */ 2744 public BooleanType getExcludePostCoordinatedElement() { 2745 if (this.excludePostCoordinated == null) 2746 if (Configuration.errorOnAutoCreate()) 2747 throw new Error("Attempt to auto-create ExpansionProfile.excludePostCoordinated"); 2748 else if (Configuration.doAutoCreate()) 2749 this.excludePostCoordinated = new BooleanType(); // bb 2750 return this.excludePostCoordinated; 2751 } 2752 2753 public boolean hasExcludePostCoordinatedElement() { 2754 return this.excludePostCoordinated != null && !this.excludePostCoordinated.isEmpty(); 2755 } 2756 2757 public boolean hasExcludePostCoordinated() { 2758 return this.excludePostCoordinated != null && !this.excludePostCoordinated.isEmpty(); 2759 } 2760 2761 /** 2762 * @param value {@link #excludePostCoordinated} (Controls whether or not the value set expansion includes post coordinated codes.). This is the underlying object with id, value and extensions. The accessor "getExcludePostCoordinated" gives direct access to the value 2763 */ 2764 public ExpansionProfile setExcludePostCoordinatedElement(BooleanType value) { 2765 this.excludePostCoordinated = value; 2766 return this; 2767 } 2768 2769 /** 2770 * @return Controls whether or not the value set expansion includes post coordinated codes. 2771 */ 2772 public boolean getExcludePostCoordinated() { 2773 return this.excludePostCoordinated == null || this.excludePostCoordinated.isEmpty() ? false : this.excludePostCoordinated.getValue(); 2774 } 2775 2776 /** 2777 * @param value Controls whether or not the value set expansion includes post coordinated codes. 2778 */ 2779 public ExpansionProfile setExcludePostCoordinated(boolean value) { 2780 if (this.excludePostCoordinated == null) 2781 this.excludePostCoordinated = new BooleanType(); 2782 this.excludePostCoordinated.setValue(value); 2783 return this; 2784 } 2785 2786 /** 2787 * @return {@link #displayLanguage} (Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display.). This is the underlying object with id, value and extensions. The accessor "getDisplayLanguage" gives direct access to the value 2788 */ 2789 public CodeType getDisplayLanguageElement() { 2790 if (this.displayLanguage == null) 2791 if (Configuration.errorOnAutoCreate()) 2792 throw new Error("Attempt to auto-create ExpansionProfile.displayLanguage"); 2793 else if (Configuration.doAutoCreate()) 2794 this.displayLanguage = new CodeType(); // bb 2795 return this.displayLanguage; 2796 } 2797 2798 public boolean hasDisplayLanguageElement() { 2799 return this.displayLanguage != null && !this.displayLanguage.isEmpty(); 2800 } 2801 2802 public boolean hasDisplayLanguage() { 2803 return this.displayLanguage != null && !this.displayLanguage.isEmpty(); 2804 } 2805 2806 /** 2807 * @param value {@link #displayLanguage} (Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display.). This is the underlying object with id, value and extensions. The accessor "getDisplayLanguage" gives direct access to the value 2808 */ 2809 public ExpansionProfile setDisplayLanguageElement(CodeType value) { 2810 this.displayLanguage = value; 2811 return this; 2812 } 2813 2814 /** 2815 * @return Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display. 2816 */ 2817 public String getDisplayLanguage() { 2818 return this.displayLanguage == null ? null : this.displayLanguage.getValue(); 2819 } 2820 2821 /** 2822 * @param value Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display. 2823 */ 2824 public ExpansionProfile setDisplayLanguage(String value) { 2825 if (Utilities.noString(value)) 2826 this.displayLanguage = null; 2827 else { 2828 if (this.displayLanguage == null) 2829 this.displayLanguage = new CodeType(); 2830 this.displayLanguage.setValue(value); 2831 } 2832 return this; 2833 } 2834 2835 /** 2836 * @return {@link #limitedExpansion} (If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html).). This is the underlying object with id, value and extensions. The accessor "getLimitedExpansion" gives direct access to the value 2837 */ 2838 public BooleanType getLimitedExpansionElement() { 2839 if (this.limitedExpansion == null) 2840 if (Configuration.errorOnAutoCreate()) 2841 throw new Error("Attempt to auto-create ExpansionProfile.limitedExpansion"); 2842 else if (Configuration.doAutoCreate()) 2843 this.limitedExpansion = new BooleanType(); // bb 2844 return this.limitedExpansion; 2845 } 2846 2847 public boolean hasLimitedExpansionElement() { 2848 return this.limitedExpansion != null && !this.limitedExpansion.isEmpty(); 2849 } 2850 2851 public boolean hasLimitedExpansion() { 2852 return this.limitedExpansion != null && !this.limitedExpansion.isEmpty(); 2853 } 2854 2855 /** 2856 * @param value {@link #limitedExpansion} (If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html).). This is the underlying object with id, value and extensions. The accessor "getLimitedExpansion" gives direct access to the value 2857 */ 2858 public ExpansionProfile setLimitedExpansionElement(BooleanType value) { 2859 this.limitedExpansion = value; 2860 return this; 2861 } 2862 2863 /** 2864 * @return If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html). 2865 */ 2866 public boolean getLimitedExpansion() { 2867 return this.limitedExpansion == null || this.limitedExpansion.isEmpty() ? false : this.limitedExpansion.getValue(); 2868 } 2869 2870 /** 2871 * @param value If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html). 2872 */ 2873 public ExpansionProfile setLimitedExpansion(boolean value) { 2874 if (this.limitedExpansion == null) 2875 this.limitedExpansion = new BooleanType(); 2876 this.limitedExpansion.setValue(value); 2877 return this; 2878 } 2879 2880 protected void listChildren(List<Property> children) { 2881 super.listChildren(children); 2882 children.add(new Property("url", "uri", "An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 2883 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier)); 2884 children.add(new Property("version", "string", "The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2885 children.add(new Property("name", "string", "A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2886 children.add(new Property("status", "code", "The status of this expansion profile. Enables tracking the life-cycle of the content.", 0, 1, status)); 2887 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 2888 children.add(new Property("date", "dateTime", "The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes.", 0, 1, date)); 2889 children.add(new Property("publisher", "string", "The name of the individual or organization that published the expansion profile.", 0, 1, publisher)); 2890 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2891 children.add(new Property("description", "markdown", "A free text natural language description of the expansion profile from a consumer's perspective.", 0, 1, description)); 2892 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate expansion profile instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2893 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the expansion profile is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2894 children.add(new Property("fixedVersion", "", "Fix use of a particular code system to a particular version.", 0, java.lang.Integer.MAX_VALUE, fixedVersion)); 2895 children.add(new Property("excludedSystem", "", "Code system, or a particular version of a code system to be excluded from value set expansions.", 0, 1, excludedSystem)); 2896 children.add(new Property("includeDesignations", "boolean", "Controls whether concept designations are to be included or excluded in value set expansions.", 0, 1, includeDesignations)); 2897 children.add(new Property("designation", "", "A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations.", 0, 1, designation)); 2898 children.add(new Property("includeDefinition", "boolean", "Controls whether the value set definition is included or excluded in value set expansions.", 0, 1, includeDefinition)); 2899 children.add(new Property("activeOnly", "boolean", "Controls whether inactive concepts are included or excluded in value set expansions.", 0, 1, activeOnly)); 2900 children.add(new Property("excludeNested", "boolean", "Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains).", 0, 1, excludeNested)); 2901 children.add(new Property("excludeNotForUI", "boolean", "Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces.", 0, 1, excludeNotForUI)); 2902 children.add(new Property("excludePostCoordinated", "boolean", "Controls whether or not the value set expansion includes post coordinated codes.", 0, 1, excludePostCoordinated)); 2903 children.add(new Property("displayLanguage", "code", "Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display.", 0, 1, displayLanguage)); 2904 children.add(new Property("limitedExpansion", "boolean", "If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html).", 0, 1, limitedExpansion)); 2905 } 2906 2907 @Override 2908 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2909 switch (_hash) { 2910 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 2911 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier); 2912 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2913 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2914 case -892481550: /*status*/ return new Property("status", "code", "The status of this expansion profile. Enables tracking the life-cycle of the content.", 0, 1, status); 2915 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 2916 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes.", 0, 1, date); 2917 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the expansion profile.", 0, 1, publisher); 2918 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2919 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the expansion profile from a consumer's perspective.", 0, 1, description); 2920 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate expansion profile instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2921 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the expansion profile is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2922 case 807748292: /*fixedVersion*/ return new Property("fixedVersion", "", "Fix use of a particular code system to a particular version.", 0, java.lang.Integer.MAX_VALUE, fixedVersion); 2923 case 2125282457: /*excludedSystem*/ return new Property("excludedSystem", "", "Code system, or a particular version of a code system to be excluded from value set expansions.", 0, 1, excludedSystem); 2924 case 461507620: /*includeDesignations*/ return new Property("includeDesignations", "boolean", "Controls whether concept designations are to be included or excluded in value set expansions.", 0, 1, includeDesignations); 2925 case -900931593: /*designation*/ return new Property("designation", "", "A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations.", 0, 1, designation); 2926 case 127972379: /*includeDefinition*/ return new Property("includeDefinition", "boolean", "Controls whether the value set definition is included or excluded in value set expansions.", 0, 1, includeDefinition); 2927 case 2043813842: /*activeOnly*/ return new Property("activeOnly", "boolean", "Controls whether inactive concepts are included or excluded in value set expansions.", 0, 1, activeOnly); 2928 case 424992625: /*excludeNested*/ return new Property("excludeNested", "boolean", "Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains).", 0, 1, excludeNested); 2929 case 667582980: /*excludeNotForUI*/ return new Property("excludeNotForUI", "boolean", "Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces.", 0, 1, excludeNotForUI); 2930 case 563335154: /*excludePostCoordinated*/ return new Property("excludePostCoordinated", "boolean", "Controls whether or not the value set expansion includes post coordinated codes.", 0, 1, excludePostCoordinated); 2931 case 1486237242: /*displayLanguage*/ return new Property("displayLanguage", "code", "Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display.", 0, 1, displayLanguage); 2932 case 597771333: /*limitedExpansion*/ return new Property("limitedExpansion", "boolean", "If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html).", 0, 1, limitedExpansion); 2933 default: return super.getNamedProperty(_hash, _name, _checkValid); 2934 } 2935 2936 } 2937 2938 @Override 2939 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2940 switch (hash) { 2941 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2942 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2943 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2944 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2945 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2946 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2947 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2948 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2949 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2950 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2951 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2952 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2953 case 807748292: /*fixedVersion*/ return this.fixedVersion == null ? new Base[0] : this.fixedVersion.toArray(new Base[this.fixedVersion.size()]); // ExpansionProfileFixedVersionComponent 2954 case 2125282457: /*excludedSystem*/ return this.excludedSystem == null ? new Base[0] : new Base[] {this.excludedSystem}; // ExpansionProfileExcludedSystemComponent 2955 case 461507620: /*includeDesignations*/ return this.includeDesignations == null ? new Base[0] : new Base[] {this.includeDesignations}; // BooleanType 2956 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : new Base[] {this.designation}; // ExpansionProfileDesignationComponent 2957 case 127972379: /*includeDefinition*/ return this.includeDefinition == null ? new Base[0] : new Base[] {this.includeDefinition}; // BooleanType 2958 case 2043813842: /*activeOnly*/ return this.activeOnly == null ? new Base[0] : new Base[] {this.activeOnly}; // BooleanType 2959 case 424992625: /*excludeNested*/ return this.excludeNested == null ? new Base[0] : new Base[] {this.excludeNested}; // BooleanType 2960 case 667582980: /*excludeNotForUI*/ return this.excludeNotForUI == null ? new Base[0] : new Base[] {this.excludeNotForUI}; // BooleanType 2961 case 563335154: /*excludePostCoordinated*/ return this.excludePostCoordinated == null ? new Base[0] : new Base[] {this.excludePostCoordinated}; // BooleanType 2962 case 1486237242: /*displayLanguage*/ return this.displayLanguage == null ? new Base[0] : new Base[] {this.displayLanguage}; // CodeType 2963 case 597771333: /*limitedExpansion*/ return this.limitedExpansion == null ? new Base[0] : new Base[] {this.limitedExpansion}; // BooleanType 2964 default: return super.getProperty(hash, name, checkValid); 2965 } 2966 2967 } 2968 2969 @Override 2970 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2971 switch (hash) { 2972 case 116079: // url 2973 this.url = castToUri(value); // UriType 2974 return value; 2975 case -1618432855: // identifier 2976 this.identifier = castToIdentifier(value); // Identifier 2977 return value; 2978 case 351608024: // version 2979 this.version = castToString(value); // StringType 2980 return value; 2981 case 3373707: // name 2982 this.name = castToString(value); // StringType 2983 return value; 2984 case -892481550: // status 2985 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2986 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2987 return value; 2988 case -404562712: // experimental 2989 this.experimental = castToBoolean(value); // BooleanType 2990 return value; 2991 case 3076014: // date 2992 this.date = castToDateTime(value); // DateTimeType 2993 return value; 2994 case 1447404028: // publisher 2995 this.publisher = castToString(value); // StringType 2996 return value; 2997 case 951526432: // contact 2998 this.getContact().add(castToContactDetail(value)); // ContactDetail 2999 return value; 3000 case -1724546052: // description 3001 this.description = castToMarkdown(value); // MarkdownType 3002 return value; 3003 case -669707736: // useContext 3004 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3005 return value; 3006 case -507075711: // jurisdiction 3007 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3008 return value; 3009 case 807748292: // fixedVersion 3010 this.getFixedVersion().add((ExpansionProfileFixedVersionComponent) value); // ExpansionProfileFixedVersionComponent 3011 return value; 3012 case 2125282457: // excludedSystem 3013 this.excludedSystem = (ExpansionProfileExcludedSystemComponent) value; // ExpansionProfileExcludedSystemComponent 3014 return value; 3015 case 461507620: // includeDesignations 3016 this.includeDesignations = castToBoolean(value); // BooleanType 3017 return value; 3018 case -900931593: // designation 3019 this.designation = (ExpansionProfileDesignationComponent) value; // ExpansionProfileDesignationComponent 3020 return value; 3021 case 127972379: // includeDefinition 3022 this.includeDefinition = castToBoolean(value); // BooleanType 3023 return value; 3024 case 2043813842: // activeOnly 3025 this.activeOnly = castToBoolean(value); // BooleanType 3026 return value; 3027 case 424992625: // excludeNested 3028 this.excludeNested = castToBoolean(value); // BooleanType 3029 return value; 3030 case 667582980: // excludeNotForUI 3031 this.excludeNotForUI = castToBoolean(value); // BooleanType 3032 return value; 3033 case 563335154: // excludePostCoordinated 3034 this.excludePostCoordinated = castToBoolean(value); // BooleanType 3035 return value; 3036 case 1486237242: // displayLanguage 3037 this.displayLanguage = castToCode(value); // CodeType 3038 return value; 3039 case 597771333: // limitedExpansion 3040 this.limitedExpansion = castToBoolean(value); // BooleanType 3041 return value; 3042 default: return super.setProperty(hash, name, value); 3043 } 3044 3045 } 3046 3047 @Override 3048 public Base setProperty(String name, Base value) throws FHIRException { 3049 if (name.equals("url")) { 3050 this.url = castToUri(value); // UriType 3051 } else if (name.equals("identifier")) { 3052 this.identifier = castToIdentifier(value); // Identifier 3053 } else if (name.equals("version")) { 3054 this.version = castToString(value); // StringType 3055 } else if (name.equals("name")) { 3056 this.name = castToString(value); // StringType 3057 } else if (name.equals("status")) { 3058 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3059 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3060 } else if (name.equals("experimental")) { 3061 this.experimental = castToBoolean(value); // BooleanType 3062 } else if (name.equals("date")) { 3063 this.date = castToDateTime(value); // DateTimeType 3064 } else if (name.equals("publisher")) { 3065 this.publisher = castToString(value); // StringType 3066 } else if (name.equals("contact")) { 3067 this.getContact().add(castToContactDetail(value)); 3068 } else if (name.equals("description")) { 3069 this.description = castToMarkdown(value); // MarkdownType 3070 } else if (name.equals("useContext")) { 3071 this.getUseContext().add(castToUsageContext(value)); 3072 } else if (name.equals("jurisdiction")) { 3073 this.getJurisdiction().add(castToCodeableConcept(value)); 3074 } else if (name.equals("fixedVersion")) { 3075 this.getFixedVersion().add((ExpansionProfileFixedVersionComponent) value); 3076 } else if (name.equals("excludedSystem")) { 3077 this.excludedSystem = (ExpansionProfileExcludedSystemComponent) value; // ExpansionProfileExcludedSystemComponent 3078 } else if (name.equals("includeDesignations")) { 3079 this.includeDesignations = castToBoolean(value); // BooleanType 3080 } else if (name.equals("designation")) { 3081 this.designation = (ExpansionProfileDesignationComponent) value; // ExpansionProfileDesignationComponent 3082 } else if (name.equals("includeDefinition")) { 3083 this.includeDefinition = castToBoolean(value); // BooleanType 3084 } else if (name.equals("activeOnly")) { 3085 this.activeOnly = castToBoolean(value); // BooleanType 3086 } else if (name.equals("excludeNested")) { 3087 this.excludeNested = castToBoolean(value); // BooleanType 3088 } else if (name.equals("excludeNotForUI")) { 3089 this.excludeNotForUI = castToBoolean(value); // BooleanType 3090 } else if (name.equals("excludePostCoordinated")) { 3091 this.excludePostCoordinated = castToBoolean(value); // BooleanType 3092 } else if (name.equals("displayLanguage")) { 3093 this.displayLanguage = castToCode(value); // CodeType 3094 } else if (name.equals("limitedExpansion")) { 3095 this.limitedExpansion = castToBoolean(value); // BooleanType 3096 } else 3097 return super.setProperty(name, value); 3098 return value; 3099 } 3100 3101 @Override 3102 public Base makeProperty(int hash, String name) throws FHIRException { 3103 switch (hash) { 3104 case 116079: return getUrlElement(); 3105 case -1618432855: return getIdentifier(); 3106 case 351608024: return getVersionElement(); 3107 case 3373707: return getNameElement(); 3108 case -892481550: return getStatusElement(); 3109 case -404562712: return getExperimentalElement(); 3110 case 3076014: return getDateElement(); 3111 case 1447404028: return getPublisherElement(); 3112 case 951526432: return addContact(); 3113 case -1724546052: return getDescriptionElement(); 3114 case -669707736: return addUseContext(); 3115 case -507075711: return addJurisdiction(); 3116 case 807748292: return addFixedVersion(); 3117 case 2125282457: return getExcludedSystem(); 3118 case 461507620: return getIncludeDesignationsElement(); 3119 case -900931593: return getDesignation(); 3120 case 127972379: return getIncludeDefinitionElement(); 3121 case 2043813842: return getActiveOnlyElement(); 3122 case 424992625: return getExcludeNestedElement(); 3123 case 667582980: return getExcludeNotForUIElement(); 3124 case 563335154: return getExcludePostCoordinatedElement(); 3125 case 1486237242: return getDisplayLanguageElement(); 3126 case 597771333: return getLimitedExpansionElement(); 3127 default: return super.makeProperty(hash, name); 3128 } 3129 3130 } 3131 3132 @Override 3133 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3134 switch (hash) { 3135 case 116079: /*url*/ return new String[] {"uri"}; 3136 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3137 case 351608024: /*version*/ return new String[] {"string"}; 3138 case 3373707: /*name*/ return new String[] {"string"}; 3139 case -892481550: /*status*/ return new String[] {"code"}; 3140 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3141 case 3076014: /*date*/ return new String[] {"dateTime"}; 3142 case 1447404028: /*publisher*/ return new String[] {"string"}; 3143 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3144 case -1724546052: /*description*/ return new String[] {"markdown"}; 3145 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3146 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3147 case 807748292: /*fixedVersion*/ return new String[] {}; 3148 case 2125282457: /*excludedSystem*/ return new String[] {}; 3149 case 461507620: /*includeDesignations*/ return new String[] {"boolean"}; 3150 case -900931593: /*designation*/ return new String[] {}; 3151 case 127972379: /*includeDefinition*/ return new String[] {"boolean"}; 3152 case 2043813842: /*activeOnly*/ return new String[] {"boolean"}; 3153 case 424992625: /*excludeNested*/ return new String[] {"boolean"}; 3154 case 667582980: /*excludeNotForUI*/ return new String[] {"boolean"}; 3155 case 563335154: /*excludePostCoordinated*/ return new String[] {"boolean"}; 3156 case 1486237242: /*displayLanguage*/ return new String[] {"code"}; 3157 case 597771333: /*limitedExpansion*/ return new String[] {"boolean"}; 3158 default: return super.getTypesForProperty(hash, name); 3159 } 3160 3161 } 3162 3163 @Override 3164 public Base addChild(String name) throws FHIRException { 3165 if (name.equals("url")) { 3166 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.url"); 3167 } 3168 else if (name.equals("identifier")) { 3169 this.identifier = new Identifier(); 3170 return this.identifier; 3171 } 3172 else if (name.equals("version")) { 3173 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.version"); 3174 } 3175 else if (name.equals("name")) { 3176 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.name"); 3177 } 3178 else if (name.equals("status")) { 3179 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.status"); 3180 } 3181 else if (name.equals("experimental")) { 3182 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.experimental"); 3183 } 3184 else if (name.equals("date")) { 3185 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.date"); 3186 } 3187 else if (name.equals("publisher")) { 3188 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.publisher"); 3189 } 3190 else if (name.equals("contact")) { 3191 return addContact(); 3192 } 3193 else if (name.equals("description")) { 3194 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.description"); 3195 } 3196 else if (name.equals("useContext")) { 3197 return addUseContext(); 3198 } 3199 else if (name.equals("jurisdiction")) { 3200 return addJurisdiction(); 3201 } 3202 else if (name.equals("fixedVersion")) { 3203 return addFixedVersion(); 3204 } 3205 else if (name.equals("excludedSystem")) { 3206 this.excludedSystem = new ExpansionProfileExcludedSystemComponent(); 3207 return this.excludedSystem; 3208 } 3209 else if (name.equals("includeDesignations")) { 3210 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.includeDesignations"); 3211 } 3212 else if (name.equals("designation")) { 3213 this.designation = new ExpansionProfileDesignationComponent(); 3214 return this.designation; 3215 } 3216 else if (name.equals("includeDefinition")) { 3217 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.includeDefinition"); 3218 } 3219 else if (name.equals("activeOnly")) { 3220 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.activeOnly"); 3221 } 3222 else if (name.equals("excludeNested")) { 3223 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.excludeNested"); 3224 } 3225 else if (name.equals("excludeNotForUI")) { 3226 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.excludeNotForUI"); 3227 } 3228 else if (name.equals("excludePostCoordinated")) { 3229 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.excludePostCoordinated"); 3230 } 3231 else if (name.equals("displayLanguage")) { 3232 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.displayLanguage"); 3233 } 3234 else if (name.equals("limitedExpansion")) { 3235 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.limitedExpansion"); 3236 } 3237 else 3238 return super.addChild(name); 3239 } 3240 3241 public String fhirType() { 3242 return "ExpansionProfile"; 3243 3244 } 3245 3246 public ExpansionProfile copy() { 3247 ExpansionProfile dst = new ExpansionProfile(); 3248 copyValues(dst); 3249 dst.url = url == null ? null : url.copy(); 3250 dst.identifier = identifier == null ? null : identifier.copy(); 3251 dst.version = version == null ? null : version.copy(); 3252 dst.name = name == null ? null : name.copy(); 3253 dst.status = status == null ? null : status.copy(); 3254 dst.experimental = experimental == null ? null : experimental.copy(); 3255 dst.date = date == null ? null : date.copy(); 3256 dst.publisher = publisher == null ? null : publisher.copy(); 3257 if (contact != null) { 3258 dst.contact = new ArrayList<ContactDetail>(); 3259 for (ContactDetail i : contact) 3260 dst.contact.add(i.copy()); 3261 }; 3262 dst.description = description == null ? null : description.copy(); 3263 if (useContext != null) { 3264 dst.useContext = new ArrayList<UsageContext>(); 3265 for (UsageContext i : useContext) 3266 dst.useContext.add(i.copy()); 3267 }; 3268 if (jurisdiction != null) { 3269 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3270 for (CodeableConcept i : jurisdiction) 3271 dst.jurisdiction.add(i.copy()); 3272 }; 3273 if (fixedVersion != null) { 3274 dst.fixedVersion = new ArrayList<ExpansionProfileFixedVersionComponent>(); 3275 for (ExpansionProfileFixedVersionComponent i : fixedVersion) 3276 dst.fixedVersion.add(i.copy()); 3277 }; 3278 dst.excludedSystem = excludedSystem == null ? null : excludedSystem.copy(); 3279 dst.includeDesignations = includeDesignations == null ? null : includeDesignations.copy(); 3280 dst.designation = designation == null ? null : designation.copy(); 3281 dst.includeDefinition = includeDefinition == null ? null : includeDefinition.copy(); 3282 dst.activeOnly = activeOnly == null ? null : activeOnly.copy(); 3283 dst.excludeNested = excludeNested == null ? null : excludeNested.copy(); 3284 dst.excludeNotForUI = excludeNotForUI == null ? null : excludeNotForUI.copy(); 3285 dst.excludePostCoordinated = excludePostCoordinated == null ? null : excludePostCoordinated.copy(); 3286 dst.displayLanguage = displayLanguage == null ? null : displayLanguage.copy(); 3287 dst.limitedExpansion = limitedExpansion == null ? null : limitedExpansion.copy(); 3288 return dst; 3289 } 3290 3291 protected ExpansionProfile typedCopy() { 3292 return copy(); 3293 } 3294 3295 @Override 3296 public boolean equalsDeep(Base other_) { 3297 if (!super.equalsDeep(other_)) 3298 return false; 3299 if (!(other_ instanceof ExpansionProfile)) 3300 return false; 3301 ExpansionProfile o = (ExpansionProfile) other_; 3302 return compareDeep(identifier, o.identifier, true) && compareDeep(fixedVersion, o.fixedVersion, true) 3303 && compareDeep(excludedSystem, o.excludedSystem, true) && compareDeep(includeDesignations, o.includeDesignations, true) 3304 && compareDeep(designation, o.designation, true) && compareDeep(includeDefinition, o.includeDefinition, true) 3305 && compareDeep(activeOnly, o.activeOnly, true) && compareDeep(excludeNested, o.excludeNested, true) 3306 && compareDeep(excludeNotForUI, o.excludeNotForUI, true) && compareDeep(excludePostCoordinated, o.excludePostCoordinated, true) 3307 && compareDeep(displayLanguage, o.displayLanguage, true) && compareDeep(limitedExpansion, o.limitedExpansion, true) 3308 ; 3309 } 3310 3311 @Override 3312 public boolean equalsShallow(Base other_) { 3313 if (!super.equalsShallow(other_)) 3314 return false; 3315 if (!(other_ instanceof ExpansionProfile)) 3316 return false; 3317 ExpansionProfile o = (ExpansionProfile) other_; 3318 return compareValues(includeDesignations, o.includeDesignations, true) && compareValues(includeDefinition, o.includeDefinition, true) 3319 && compareValues(activeOnly, o.activeOnly, true) && compareValues(excludeNested, o.excludeNested, true) 3320 && compareValues(excludeNotForUI, o.excludeNotForUI, true) && compareValues(excludePostCoordinated, o.excludePostCoordinated, true) 3321 && compareValues(displayLanguage, o.displayLanguage, true) && compareValues(limitedExpansion, o.limitedExpansion, true) 3322 ; 3323 } 3324 3325 public boolean isEmpty() { 3326 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, fixedVersion, excludedSystem 3327 , includeDesignations, designation, includeDefinition, activeOnly, excludeNested, excludeNotForUI 3328 , excludePostCoordinated, displayLanguage, limitedExpansion); 3329 } 3330 3331 @Override 3332 public ResourceType getResourceType() { 3333 return ResourceType.ExpansionProfile; 3334 } 3335 3336 /** 3337 * Search parameter: <b>date</b> 3338 * <p> 3339 * Description: <b>The expansion profile publication date</b><br> 3340 * Type: <b>date</b><br> 3341 * Path: <b>ExpansionProfile.date</b><br> 3342 * </p> 3343 */ 3344 @SearchParamDefinition(name="date", path="ExpansionProfile.date", description="The expansion profile publication date", type="date" ) 3345 public static final String SP_DATE = "date"; 3346 /** 3347 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3348 * <p> 3349 * Description: <b>The expansion profile publication date</b><br> 3350 * Type: <b>date</b><br> 3351 * Path: <b>ExpansionProfile.date</b><br> 3352 * </p> 3353 */ 3354 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3355 3356 /** 3357 * Search parameter: <b>identifier</b> 3358 * <p> 3359 * Description: <b>External identifier for the expansion profile</b><br> 3360 * Type: <b>token</b><br> 3361 * Path: <b>ExpansionProfile.identifier</b><br> 3362 * </p> 3363 */ 3364 @SearchParamDefinition(name="identifier", path="ExpansionProfile.identifier", description="External identifier for the expansion profile", type="token" ) 3365 public static final String SP_IDENTIFIER = "identifier"; 3366 /** 3367 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3368 * <p> 3369 * Description: <b>External identifier for the expansion profile</b><br> 3370 * Type: <b>token</b><br> 3371 * Path: <b>ExpansionProfile.identifier</b><br> 3372 * </p> 3373 */ 3374 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3375 3376 /** 3377 * Search parameter: <b>jurisdiction</b> 3378 * <p> 3379 * Description: <b>Intended jurisdiction for the expansion profile</b><br> 3380 * Type: <b>token</b><br> 3381 * Path: <b>ExpansionProfile.jurisdiction</b><br> 3382 * </p> 3383 */ 3384 @SearchParamDefinition(name="jurisdiction", path="ExpansionProfile.jurisdiction", description="Intended jurisdiction for the expansion profile", type="token" ) 3385 public static final String SP_JURISDICTION = "jurisdiction"; 3386 /** 3387 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3388 * <p> 3389 * Description: <b>Intended jurisdiction for the expansion profile</b><br> 3390 * Type: <b>token</b><br> 3391 * Path: <b>ExpansionProfile.jurisdiction</b><br> 3392 * </p> 3393 */ 3394 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3395 3396 /** 3397 * Search parameter: <b>name</b> 3398 * <p> 3399 * Description: <b>Computationally friendly name of the expansion profile</b><br> 3400 * Type: <b>string</b><br> 3401 * Path: <b>ExpansionProfile.name</b><br> 3402 * </p> 3403 */ 3404 @SearchParamDefinition(name="name", path="ExpansionProfile.name", description="Computationally friendly name of the expansion profile", type="string" ) 3405 public static final String SP_NAME = "name"; 3406 /** 3407 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3408 * <p> 3409 * Description: <b>Computationally friendly name of the expansion profile</b><br> 3410 * Type: <b>string</b><br> 3411 * Path: <b>ExpansionProfile.name</b><br> 3412 * </p> 3413 */ 3414 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3415 3416 /** 3417 * Search parameter: <b>description</b> 3418 * <p> 3419 * Description: <b>The description of the expansion profile</b><br> 3420 * Type: <b>string</b><br> 3421 * Path: <b>ExpansionProfile.description</b><br> 3422 * </p> 3423 */ 3424 @SearchParamDefinition(name="description", path="ExpansionProfile.description", description="The description of the expansion profile", type="string" ) 3425 public static final String SP_DESCRIPTION = "description"; 3426 /** 3427 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3428 * <p> 3429 * Description: <b>The description of the expansion profile</b><br> 3430 * Type: <b>string</b><br> 3431 * Path: <b>ExpansionProfile.description</b><br> 3432 * </p> 3433 */ 3434 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3435 3436 /** 3437 * Search parameter: <b>publisher</b> 3438 * <p> 3439 * Description: <b>Name of the publisher of the expansion profile</b><br> 3440 * Type: <b>string</b><br> 3441 * Path: <b>ExpansionProfile.publisher</b><br> 3442 * </p> 3443 */ 3444 @SearchParamDefinition(name="publisher", path="ExpansionProfile.publisher", description="Name of the publisher of the expansion profile", type="string" ) 3445 public static final String SP_PUBLISHER = "publisher"; 3446 /** 3447 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3448 * <p> 3449 * Description: <b>Name of the publisher of the expansion profile</b><br> 3450 * Type: <b>string</b><br> 3451 * Path: <b>ExpansionProfile.publisher</b><br> 3452 * </p> 3453 */ 3454 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3455 3456 /** 3457 * Search parameter: <b>version</b> 3458 * <p> 3459 * Description: <b>The business version of the expansion profile</b><br> 3460 * Type: <b>token</b><br> 3461 * Path: <b>ExpansionProfile.version</b><br> 3462 * </p> 3463 */ 3464 @SearchParamDefinition(name="version", path="ExpansionProfile.version", description="The business version of the expansion profile", type="token" ) 3465 public static final String SP_VERSION = "version"; 3466 /** 3467 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3468 * <p> 3469 * Description: <b>The business version of the expansion profile</b><br> 3470 * Type: <b>token</b><br> 3471 * Path: <b>ExpansionProfile.version</b><br> 3472 * </p> 3473 */ 3474 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3475 3476 /** 3477 * Search parameter: <b>url</b> 3478 * <p> 3479 * Description: <b>The uri that identifies the expansion profile</b><br> 3480 * Type: <b>uri</b><br> 3481 * Path: <b>ExpansionProfile.url</b><br> 3482 * </p> 3483 */ 3484 @SearchParamDefinition(name="url", path="ExpansionProfile.url", description="The uri that identifies the expansion profile", type="uri" ) 3485 public static final String SP_URL = "url"; 3486 /** 3487 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3488 * <p> 3489 * Description: <b>The uri that identifies the expansion profile</b><br> 3490 * Type: <b>uri</b><br> 3491 * Path: <b>ExpansionProfile.url</b><br> 3492 * </p> 3493 */ 3494 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3495 3496 /** 3497 * Search parameter: <b>status</b> 3498 * <p> 3499 * Description: <b>The current status of the expansion profile</b><br> 3500 * Type: <b>token</b><br> 3501 * Path: <b>ExpansionProfile.status</b><br> 3502 * </p> 3503 */ 3504 @SearchParamDefinition(name="status", path="ExpansionProfile.status", description="The current status of the expansion profile", type="token" ) 3505 public static final String SP_STATUS = "status"; 3506 /** 3507 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3508 * <p> 3509 * Description: <b>The current status of the expansion profile</b><br> 3510 * Type: <b>token</b><br> 3511 * Path: <b>ExpansionProfile.status</b><br> 3512 * </p> 3513 */ 3514 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3515 3516 3517}