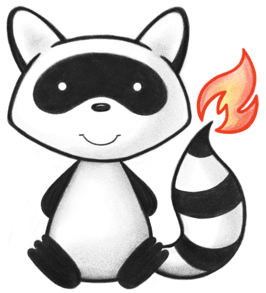
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * Resource to define constraints on the Expansion of a FHIR ValueSet. 053 */ 054@ResourceDef(name="ExpansionProfile", profile="http://hl7.org/fhir/Profile/ExpansionProfile") 055@ChildOrder(names={"url", "identifier", "version", "name", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "fixedVersion", "excludedSystem", "includeDesignations", "designation", "includeDefinition", "activeOnly", "excludeNested", "excludeNotForUI", "excludePostCoordinated", "displayLanguage", "limitedExpansion"}) 056public class ExpansionProfile extends MetadataResource { 057 058 public enum SystemVersionProcessingMode { 059 /** 060 * Use this version of the code system if a value set doesn't specify a version 061 */ 062 DEFAULT, 063 /** 064 * Use this version of the code system. If a value set specifies a different version, the expansion operation should fail 065 */ 066 CHECK, 067 /** 068 * Use this version of the code system irrespective of which version is specified by a value set. Note that this has obvious safety issues, in that it may result in a value set expansion giving a different list of codes that is both wrong and unsafe, and implementers should only use this capability reluctantly. It primarily exists to deal with situations where specifications have fallen into decay as time passes. If a version is override, the version used SHALL explicitly be represented in the expansion parameters 069 */ 070 OVERRIDE, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static SystemVersionProcessingMode fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("default".equals(codeString)) 079 return DEFAULT; 080 if ("check".equals(codeString)) 081 return CHECK; 082 if ("override".equals(codeString)) 083 return OVERRIDE; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown SystemVersionProcessingMode code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case DEFAULT: return "default"; 092 case CHECK: return "check"; 093 case OVERRIDE: return "override"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case DEFAULT: return "http://hl7.org/fhir/system-version-processing-mode"; 101 case CHECK: return "http://hl7.org/fhir/system-version-processing-mode"; 102 case OVERRIDE: return "http://hl7.org/fhir/system-version-processing-mode"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getDefinition() { 108 switch (this) { 109 case DEFAULT: return "Use this version of the code system if a value set doesn't specify a version"; 110 case CHECK: return "Use this version of the code system. If a value set specifies a different version, the expansion operation should fail"; 111 case OVERRIDE: return "Use this version of the code system irrespective of which version is specified by a value set. Note that this has obvious safety issues, in that it may result in a value set expansion giving a different list of codes that is both wrong and unsafe, and implementers should only use this capability reluctantly. It primarily exists to deal with situations where specifications have fallen into decay as time passes. If a version is override, the version used SHALL explicitly be represented in the expansion parameters"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDisplay() { 117 switch (this) { 118 case DEFAULT: return "Default Version"; 119 case CHECK: return "Check ValueSet Version"; 120 case OVERRIDE: return "Override ValueSet Version"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 } 126 127 public static class SystemVersionProcessingModeEnumFactory implements EnumFactory<SystemVersionProcessingMode> { 128 public SystemVersionProcessingMode fromCode(String codeString) throws IllegalArgumentException { 129 if (codeString == null || "".equals(codeString)) 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("default".equals(codeString)) 133 return SystemVersionProcessingMode.DEFAULT; 134 if ("check".equals(codeString)) 135 return SystemVersionProcessingMode.CHECK; 136 if ("override".equals(codeString)) 137 return SystemVersionProcessingMode.OVERRIDE; 138 throw new IllegalArgumentException("Unknown SystemVersionProcessingMode code '"+codeString+"'"); 139 } 140 public Enumeration<SystemVersionProcessingMode> fromType(PrimitiveType<?> code) throws FHIRException { 141 if (code == null) 142 return null; 143 if (code.isEmpty()) 144 return new Enumeration<SystemVersionProcessingMode>(this); 145 String codeString = code.asStringValue(); 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("default".equals(codeString)) 149 return new Enumeration<SystemVersionProcessingMode>(this, SystemVersionProcessingMode.DEFAULT); 150 if ("check".equals(codeString)) 151 return new Enumeration<SystemVersionProcessingMode>(this, SystemVersionProcessingMode.CHECK); 152 if ("override".equals(codeString)) 153 return new Enumeration<SystemVersionProcessingMode>(this, SystemVersionProcessingMode.OVERRIDE); 154 throw new FHIRException("Unknown SystemVersionProcessingMode code '"+codeString+"'"); 155 } 156 public String toCode(SystemVersionProcessingMode code) { 157 if (code == SystemVersionProcessingMode.NULL) 158 return null; 159 if (code == SystemVersionProcessingMode.DEFAULT) 160 return "default"; 161 if (code == SystemVersionProcessingMode.CHECK) 162 return "check"; 163 if (code == SystemVersionProcessingMode.OVERRIDE) 164 return "override"; 165 return "?"; 166 } 167 public String toSystem(SystemVersionProcessingMode code) { 168 return code.getSystem(); 169 } 170 } 171 172 @Block() 173 public static class ExpansionProfileFixedVersionComponent extends BackboneElement implements IBaseBackboneElement { 174 /** 175 * The specific system for which to fix the version. 176 */ 177 @Child(name = "system", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 178 @Description(shortDefinition="System to have its version fixed", formalDefinition="The specific system for which to fix the version." ) 179 protected UriType system; 180 181 /** 182 * The version of the code system from which codes in the expansion should be included. 183 */ 184 @Child(name = "version", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 185 @Description(shortDefinition="Specific version of the code system referred to", formalDefinition="The version of the code system from which codes in the expansion should be included." ) 186 protected StringType version; 187 188 /** 189 * How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile. 190 */ 191 @Child(name = "mode", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 192 @Description(shortDefinition="default | check | override", formalDefinition="How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/system-version-processing-mode") 194 protected Enumeration<SystemVersionProcessingMode> mode; 195 196 private static final long serialVersionUID = 1818466753L; 197 198 /** 199 * Constructor 200 */ 201 public ExpansionProfileFixedVersionComponent() { 202 super(); 203 } 204 205 /** 206 * Constructor 207 */ 208 public ExpansionProfileFixedVersionComponent(UriType system, StringType version, Enumeration<SystemVersionProcessingMode> mode) { 209 super(); 210 this.system = system; 211 this.version = version; 212 this.mode = mode; 213 } 214 215 /** 216 * @return {@link #system} (The specific system for which to fix the version.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 217 */ 218 public UriType getSystemElement() { 219 if (this.system == null) 220 if (Configuration.errorOnAutoCreate()) 221 throw new Error("Attempt to auto-create ExpansionProfileFixedVersionComponent.system"); 222 else if (Configuration.doAutoCreate()) 223 this.system = new UriType(); // bb 224 return this.system; 225 } 226 227 public boolean hasSystemElement() { 228 return this.system != null && !this.system.isEmpty(); 229 } 230 231 public boolean hasSystem() { 232 return this.system != null && !this.system.isEmpty(); 233 } 234 235 /** 236 * @param value {@link #system} (The specific system for which to fix the version.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 237 */ 238 public ExpansionProfileFixedVersionComponent setSystemElement(UriType value) { 239 this.system = value; 240 return this; 241 } 242 243 /** 244 * @return The specific system for which to fix the version. 245 */ 246 public String getSystem() { 247 return this.system == null ? null : this.system.getValue(); 248 } 249 250 /** 251 * @param value The specific system for which to fix the version. 252 */ 253 public ExpansionProfileFixedVersionComponent setSystem(String value) { 254 if (this.system == null) 255 this.system = new UriType(); 256 this.system.setValue(value); 257 return this; 258 } 259 260 /** 261 * @return {@link #version} (The version of the code system from which codes in the expansion should be included.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 262 */ 263 public StringType getVersionElement() { 264 if (this.version == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create ExpansionProfileFixedVersionComponent.version"); 267 else if (Configuration.doAutoCreate()) 268 this.version = new StringType(); // bb 269 return this.version; 270 } 271 272 public boolean hasVersionElement() { 273 return this.version != null && !this.version.isEmpty(); 274 } 275 276 public boolean hasVersion() { 277 return this.version != null && !this.version.isEmpty(); 278 } 279 280 /** 281 * @param value {@link #version} (The version of the code system from which codes in the expansion should be included.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 282 */ 283 public ExpansionProfileFixedVersionComponent setVersionElement(StringType value) { 284 this.version = value; 285 return this; 286 } 287 288 /** 289 * @return The version of the code system from which codes in the expansion should be included. 290 */ 291 public String getVersion() { 292 return this.version == null ? null : this.version.getValue(); 293 } 294 295 /** 296 * @param value The version of the code system from which codes in the expansion should be included. 297 */ 298 public ExpansionProfileFixedVersionComponent setVersion(String value) { 299 if (this.version == null) 300 this.version = new StringType(); 301 this.version.setValue(value); 302 return this; 303 } 304 305 /** 306 * @return {@link #mode} (How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 307 */ 308 public Enumeration<SystemVersionProcessingMode> getModeElement() { 309 if (this.mode == null) 310 if (Configuration.errorOnAutoCreate()) 311 throw new Error("Attempt to auto-create ExpansionProfileFixedVersionComponent.mode"); 312 else if (Configuration.doAutoCreate()) 313 this.mode = new Enumeration<SystemVersionProcessingMode>(new SystemVersionProcessingModeEnumFactory()); // bb 314 return this.mode; 315 } 316 317 public boolean hasModeElement() { 318 return this.mode != null && !this.mode.isEmpty(); 319 } 320 321 public boolean hasMode() { 322 return this.mode != null && !this.mode.isEmpty(); 323 } 324 325 /** 326 * @param value {@link #mode} (How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 327 */ 328 public ExpansionProfileFixedVersionComponent setModeElement(Enumeration<SystemVersionProcessingMode> value) { 329 this.mode = value; 330 return this; 331 } 332 333 /** 334 * @return How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile. 335 */ 336 public SystemVersionProcessingMode getMode() { 337 return this.mode == null ? null : this.mode.getValue(); 338 } 339 340 /** 341 * @param value How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile. 342 */ 343 public ExpansionProfileFixedVersionComponent setMode(SystemVersionProcessingMode value) { 344 if (this.mode == null) 345 this.mode = new Enumeration<SystemVersionProcessingMode>(new SystemVersionProcessingModeEnumFactory()); 346 this.mode.setValue(value); 347 return this; 348 } 349 350 protected void listChildren(List<Property> children) { 351 super.listChildren(children); 352 children.add(new Property("system", "uri", "The specific system for which to fix the version.", 0, 1, system)); 353 children.add(new Property("version", "string", "The version of the code system from which codes in the expansion should be included.", 0, 1, version)); 354 children.add(new Property("mode", "code", "How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile.", 0, 1, mode)); 355 } 356 357 @Override 358 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 359 switch (_hash) { 360 case -887328209: /*system*/ return new Property("system", "uri", "The specific system for which to fix the version.", 0, 1, system); 361 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system from which codes in the expansion should be included.", 0, 1, version); 362 case 3357091: /*mode*/ return new Property("mode", "code", "How to manage the intersection between a fixed version in a value set, and this fixed version of the system in the expansion profile.", 0, 1, mode); 363 default: return super.getNamedProperty(_hash, _name, _checkValid); 364 } 365 366 } 367 368 @Override 369 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 370 switch (hash) { 371 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 372 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 373 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<SystemVersionProcessingMode> 374 default: return super.getProperty(hash, name, checkValid); 375 } 376 377 } 378 379 @Override 380 public Base setProperty(int hash, String name, Base value) throws FHIRException { 381 switch (hash) { 382 case -887328209: // system 383 this.system = castToUri(value); // UriType 384 return value; 385 case 351608024: // version 386 this.version = castToString(value); // StringType 387 return value; 388 case 3357091: // mode 389 value = new SystemVersionProcessingModeEnumFactory().fromType(castToCode(value)); 390 this.mode = (Enumeration) value; // Enumeration<SystemVersionProcessingMode> 391 return value; 392 default: return super.setProperty(hash, name, value); 393 } 394 395 } 396 397 @Override 398 public Base setProperty(String name, Base value) throws FHIRException { 399 if (name.equals("system")) { 400 this.system = castToUri(value); // UriType 401 } else if (name.equals("version")) { 402 this.version = castToString(value); // StringType 403 } else if (name.equals("mode")) { 404 value = new SystemVersionProcessingModeEnumFactory().fromType(castToCode(value)); 405 this.mode = (Enumeration) value; // Enumeration<SystemVersionProcessingMode> 406 } else 407 return super.setProperty(name, value); 408 return value; 409 } 410 411 @Override 412 public Base makeProperty(int hash, String name) throws FHIRException { 413 switch (hash) { 414 case -887328209: return getSystemElement(); 415 case 351608024: return getVersionElement(); 416 case 3357091: return getModeElement(); 417 default: return super.makeProperty(hash, name); 418 } 419 420 } 421 422 @Override 423 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 424 switch (hash) { 425 case -887328209: /*system*/ return new String[] {"uri"}; 426 case 351608024: /*version*/ return new String[] {"string"}; 427 case 3357091: /*mode*/ return new String[] {"code"}; 428 default: return super.getTypesForProperty(hash, name); 429 } 430 431 } 432 433 @Override 434 public Base addChild(String name) throws FHIRException { 435 if (name.equals("system")) { 436 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.system"); 437 } 438 else if (name.equals("version")) { 439 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.version"); 440 } 441 else if (name.equals("mode")) { 442 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.mode"); 443 } 444 else 445 return super.addChild(name); 446 } 447 448 public ExpansionProfileFixedVersionComponent copy() { 449 ExpansionProfileFixedVersionComponent dst = new ExpansionProfileFixedVersionComponent(); 450 copyValues(dst); 451 dst.system = system == null ? null : system.copy(); 452 dst.version = version == null ? null : version.copy(); 453 dst.mode = mode == null ? null : mode.copy(); 454 return dst; 455 } 456 457 @Override 458 public boolean equalsDeep(Base other_) { 459 if (!super.equalsDeep(other_)) 460 return false; 461 if (!(other_ instanceof ExpansionProfileFixedVersionComponent)) 462 return false; 463 ExpansionProfileFixedVersionComponent o = (ExpansionProfileFixedVersionComponent) other_; 464 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true) && compareDeep(mode, o.mode, true) 465 ; 466 } 467 468 @Override 469 public boolean equalsShallow(Base other_) { 470 if (!super.equalsShallow(other_)) 471 return false; 472 if (!(other_ instanceof ExpansionProfileFixedVersionComponent)) 473 return false; 474 ExpansionProfileFixedVersionComponent o = (ExpansionProfileFixedVersionComponent) other_; 475 return compareValues(system, o.system, true) && compareValues(version, o.version, true) && compareValues(mode, o.mode, true) 476 ; 477 } 478 479 public boolean isEmpty() { 480 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version, mode); 481 } 482 483 public String fhirType() { 484 return "ExpansionProfile.fixedVersion"; 485 486 } 487 488 } 489 490 @Block() 491 public static class ExpansionProfileExcludedSystemComponent extends BackboneElement implements IBaseBackboneElement { 492 /** 493 * An absolute URI which is the code system to be excluded. 494 */ 495 @Child(name = "system", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 496 @Description(shortDefinition="The specific code system to be excluded", formalDefinition="An absolute URI which is the code system to be excluded." ) 497 protected UriType system; 498 499 /** 500 * The version of the code system from which codes in the expansion should be excluded. 501 */ 502 @Child(name = "version", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 503 @Description(shortDefinition="Specific version of the code system referred to", formalDefinition="The version of the code system from which codes in the expansion should be excluded." ) 504 protected StringType version; 505 506 private static final long serialVersionUID = 1145288774L; 507 508 /** 509 * Constructor 510 */ 511 public ExpansionProfileExcludedSystemComponent() { 512 super(); 513 } 514 515 /** 516 * Constructor 517 */ 518 public ExpansionProfileExcludedSystemComponent(UriType system) { 519 super(); 520 this.system = system; 521 } 522 523 /** 524 * @return {@link #system} (An absolute URI which is the code system to be excluded.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 525 */ 526 public UriType getSystemElement() { 527 if (this.system == null) 528 if (Configuration.errorOnAutoCreate()) 529 throw new Error("Attempt to auto-create ExpansionProfileExcludedSystemComponent.system"); 530 else if (Configuration.doAutoCreate()) 531 this.system = new UriType(); // bb 532 return this.system; 533 } 534 535 public boolean hasSystemElement() { 536 return this.system != null && !this.system.isEmpty(); 537 } 538 539 public boolean hasSystem() { 540 return this.system != null && !this.system.isEmpty(); 541 } 542 543 /** 544 * @param value {@link #system} (An absolute URI which is the code system to be excluded.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 545 */ 546 public ExpansionProfileExcludedSystemComponent setSystemElement(UriType value) { 547 this.system = value; 548 return this; 549 } 550 551 /** 552 * @return An absolute URI which is the code system to be excluded. 553 */ 554 public String getSystem() { 555 return this.system == null ? null : this.system.getValue(); 556 } 557 558 /** 559 * @param value An absolute URI which is the code system to be excluded. 560 */ 561 public ExpansionProfileExcludedSystemComponent setSystem(String value) { 562 if (this.system == null) 563 this.system = new UriType(); 564 this.system.setValue(value); 565 return this; 566 } 567 568 /** 569 * @return {@link #version} (The version of the code system from which codes in the expansion should be excluded.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 570 */ 571 public StringType getVersionElement() { 572 if (this.version == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create ExpansionProfileExcludedSystemComponent.version"); 575 else if (Configuration.doAutoCreate()) 576 this.version = new StringType(); // bb 577 return this.version; 578 } 579 580 public boolean hasVersionElement() { 581 return this.version != null && !this.version.isEmpty(); 582 } 583 584 public boolean hasVersion() { 585 return this.version != null && !this.version.isEmpty(); 586 } 587 588 /** 589 * @param value {@link #version} (The version of the code system from which codes in the expansion should be excluded.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 590 */ 591 public ExpansionProfileExcludedSystemComponent setVersionElement(StringType value) { 592 this.version = value; 593 return this; 594 } 595 596 /** 597 * @return The version of the code system from which codes in the expansion should be excluded. 598 */ 599 public String getVersion() { 600 return this.version == null ? null : this.version.getValue(); 601 } 602 603 /** 604 * @param value The version of the code system from which codes in the expansion should be excluded. 605 */ 606 public ExpansionProfileExcludedSystemComponent setVersion(String value) { 607 if (Utilities.noString(value)) 608 this.version = null; 609 else { 610 if (this.version == null) 611 this.version = new StringType(); 612 this.version.setValue(value); 613 } 614 return this; 615 } 616 617 protected void listChildren(List<Property> children) { 618 super.listChildren(children); 619 children.add(new Property("system", "uri", "An absolute URI which is the code system to be excluded.", 0, 1, system)); 620 children.add(new Property("version", "string", "The version of the code system from which codes in the expansion should be excluded.", 0, 1, version)); 621 } 622 623 @Override 624 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 625 switch (_hash) { 626 case -887328209: /*system*/ return new Property("system", "uri", "An absolute URI which is the code system to be excluded.", 0, 1, system); 627 case 351608024: /*version*/ return new Property("version", "string", "The version of the code system from which codes in the expansion should be excluded.", 0, 1, version); 628 default: return super.getNamedProperty(_hash, _name, _checkValid); 629 } 630 631 } 632 633 @Override 634 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 635 switch (hash) { 636 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 637 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 638 default: return super.getProperty(hash, name, checkValid); 639 } 640 641 } 642 643 @Override 644 public Base setProperty(int hash, String name, Base value) throws FHIRException { 645 switch (hash) { 646 case -887328209: // system 647 this.system = castToUri(value); // UriType 648 return value; 649 case 351608024: // version 650 this.version = castToString(value); // StringType 651 return value; 652 default: return super.setProperty(hash, name, value); 653 } 654 655 } 656 657 @Override 658 public Base setProperty(String name, Base value) throws FHIRException { 659 if (name.equals("system")) { 660 this.system = castToUri(value); // UriType 661 } else if (name.equals("version")) { 662 this.version = castToString(value); // StringType 663 } else 664 return super.setProperty(name, value); 665 return value; 666 } 667 668 @Override 669 public Base makeProperty(int hash, String name) throws FHIRException { 670 switch (hash) { 671 case -887328209: return getSystemElement(); 672 case 351608024: return getVersionElement(); 673 default: return super.makeProperty(hash, name); 674 } 675 676 } 677 678 @Override 679 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 680 switch (hash) { 681 case -887328209: /*system*/ return new String[] {"uri"}; 682 case 351608024: /*version*/ return new String[] {"string"}; 683 default: return super.getTypesForProperty(hash, name); 684 } 685 686 } 687 688 @Override 689 public Base addChild(String name) throws FHIRException { 690 if (name.equals("system")) { 691 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.system"); 692 } 693 else if (name.equals("version")) { 694 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.version"); 695 } 696 else 697 return super.addChild(name); 698 } 699 700 public ExpansionProfileExcludedSystemComponent copy() { 701 ExpansionProfileExcludedSystemComponent dst = new ExpansionProfileExcludedSystemComponent(); 702 copyValues(dst); 703 dst.system = system == null ? null : system.copy(); 704 dst.version = version == null ? null : version.copy(); 705 return dst; 706 } 707 708 @Override 709 public boolean equalsDeep(Base other_) { 710 if (!super.equalsDeep(other_)) 711 return false; 712 if (!(other_ instanceof ExpansionProfileExcludedSystemComponent)) 713 return false; 714 ExpansionProfileExcludedSystemComponent o = (ExpansionProfileExcludedSystemComponent) other_; 715 return compareDeep(system, o.system, true) && compareDeep(version, o.version, true); 716 } 717 718 @Override 719 public boolean equalsShallow(Base other_) { 720 if (!super.equalsShallow(other_)) 721 return false; 722 if (!(other_ instanceof ExpansionProfileExcludedSystemComponent)) 723 return false; 724 ExpansionProfileExcludedSystemComponent o = (ExpansionProfileExcludedSystemComponent) other_; 725 return compareValues(system, o.system, true) && compareValues(version, o.version, true); 726 } 727 728 public boolean isEmpty() { 729 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(system, version); 730 } 731 732 public String fhirType() { 733 return "ExpansionProfile.excludedSystem"; 734 735 } 736 737 } 738 739 @Block() 740 public static class ExpansionProfileDesignationComponent extends BackboneElement implements IBaseBackboneElement { 741 /** 742 * Designations to be included. 743 */ 744 @Child(name = "include", type = {}, order=1, min=0, max=1, modifier=false, summary=true) 745 @Description(shortDefinition="Designations to be included", formalDefinition="Designations to be included." ) 746 protected DesignationIncludeComponent include; 747 748 /** 749 * Designations to be excluded. 750 */ 751 @Child(name = "exclude", type = {}, order=2, min=0, max=1, modifier=false, summary=true) 752 @Description(shortDefinition="Designations to be excluded", formalDefinition="Designations to be excluded." ) 753 protected DesignationExcludeComponent exclude; 754 755 private static final long serialVersionUID = -2080476436L; 756 757 /** 758 * Constructor 759 */ 760 public ExpansionProfileDesignationComponent() { 761 super(); 762 } 763 764 /** 765 * @return {@link #include} (Designations to be included.) 766 */ 767 public DesignationIncludeComponent getInclude() { 768 if (this.include == null) 769 if (Configuration.errorOnAutoCreate()) 770 throw new Error("Attempt to auto-create ExpansionProfileDesignationComponent.include"); 771 else if (Configuration.doAutoCreate()) 772 this.include = new DesignationIncludeComponent(); // cc 773 return this.include; 774 } 775 776 public boolean hasInclude() { 777 return this.include != null && !this.include.isEmpty(); 778 } 779 780 /** 781 * @param value {@link #include} (Designations to be included.) 782 */ 783 public ExpansionProfileDesignationComponent setInclude(DesignationIncludeComponent value) { 784 this.include = value; 785 return this; 786 } 787 788 /** 789 * @return {@link #exclude} (Designations to be excluded.) 790 */ 791 public DesignationExcludeComponent getExclude() { 792 if (this.exclude == null) 793 if (Configuration.errorOnAutoCreate()) 794 throw new Error("Attempt to auto-create ExpansionProfileDesignationComponent.exclude"); 795 else if (Configuration.doAutoCreate()) 796 this.exclude = new DesignationExcludeComponent(); // cc 797 return this.exclude; 798 } 799 800 public boolean hasExclude() { 801 return this.exclude != null && !this.exclude.isEmpty(); 802 } 803 804 /** 805 * @param value {@link #exclude} (Designations to be excluded.) 806 */ 807 public ExpansionProfileDesignationComponent setExclude(DesignationExcludeComponent value) { 808 this.exclude = value; 809 return this; 810 } 811 812 protected void listChildren(List<Property> children) { 813 super.listChildren(children); 814 children.add(new Property("include", "", "Designations to be included.", 0, 1, include)); 815 children.add(new Property("exclude", "", "Designations to be excluded.", 0, 1, exclude)); 816 } 817 818 @Override 819 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 820 switch (_hash) { 821 case 1942574248: /*include*/ return new Property("include", "", "Designations to be included.", 0, 1, include); 822 case -1321148966: /*exclude*/ return new Property("exclude", "", "Designations to be excluded.", 0, 1, exclude); 823 default: return super.getNamedProperty(_hash, _name, _checkValid); 824 } 825 826 } 827 828 @Override 829 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 830 switch (hash) { 831 case 1942574248: /*include*/ return this.include == null ? new Base[0] : new Base[] {this.include}; // DesignationIncludeComponent 832 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : new Base[] {this.exclude}; // DesignationExcludeComponent 833 default: return super.getProperty(hash, name, checkValid); 834 } 835 836 } 837 838 @Override 839 public Base setProperty(int hash, String name, Base value) throws FHIRException { 840 switch (hash) { 841 case 1942574248: // include 842 this.include = (DesignationIncludeComponent) value; // DesignationIncludeComponent 843 return value; 844 case -1321148966: // exclude 845 this.exclude = (DesignationExcludeComponent) value; // DesignationExcludeComponent 846 return value; 847 default: return super.setProperty(hash, name, value); 848 } 849 850 } 851 852 @Override 853 public Base setProperty(String name, Base value) throws FHIRException { 854 if (name.equals("include")) { 855 this.include = (DesignationIncludeComponent) value; // DesignationIncludeComponent 856 } else if (name.equals("exclude")) { 857 this.exclude = (DesignationExcludeComponent) value; // DesignationExcludeComponent 858 } else 859 return super.setProperty(name, value); 860 return value; 861 } 862 863 @Override 864 public Base makeProperty(int hash, String name) throws FHIRException { 865 switch (hash) { 866 case 1942574248: return getInclude(); 867 case -1321148966: return getExclude(); 868 default: return super.makeProperty(hash, name); 869 } 870 871 } 872 873 @Override 874 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 875 switch (hash) { 876 case 1942574248: /*include*/ return new String[] {}; 877 case -1321148966: /*exclude*/ return new String[] {}; 878 default: return super.getTypesForProperty(hash, name); 879 } 880 881 } 882 883 @Override 884 public Base addChild(String name) throws FHIRException { 885 if (name.equals("include")) { 886 this.include = new DesignationIncludeComponent(); 887 return this.include; 888 } 889 else if (name.equals("exclude")) { 890 this.exclude = new DesignationExcludeComponent(); 891 return this.exclude; 892 } 893 else 894 return super.addChild(name); 895 } 896 897 public ExpansionProfileDesignationComponent copy() { 898 ExpansionProfileDesignationComponent dst = new ExpansionProfileDesignationComponent(); 899 copyValues(dst); 900 dst.include = include == null ? null : include.copy(); 901 dst.exclude = exclude == null ? null : exclude.copy(); 902 return dst; 903 } 904 905 @Override 906 public boolean equalsDeep(Base other_) { 907 if (!super.equalsDeep(other_)) 908 return false; 909 if (!(other_ instanceof ExpansionProfileDesignationComponent)) 910 return false; 911 ExpansionProfileDesignationComponent o = (ExpansionProfileDesignationComponent) other_; 912 return compareDeep(include, o.include, true) && compareDeep(exclude, o.exclude, true); 913 } 914 915 @Override 916 public boolean equalsShallow(Base other_) { 917 if (!super.equalsShallow(other_)) 918 return false; 919 if (!(other_ instanceof ExpansionProfileDesignationComponent)) 920 return false; 921 ExpansionProfileDesignationComponent o = (ExpansionProfileDesignationComponent) other_; 922 return true; 923 } 924 925 public boolean isEmpty() { 926 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(include, exclude); 927 } 928 929 public String fhirType() { 930 return "ExpansionProfile.designation"; 931 932 } 933 934 } 935 936 @Block() 937 public static class DesignationIncludeComponent extends BackboneElement implements IBaseBackboneElement { 938 /** 939 * A data group for each designation to be included. 940 */ 941 @Child(name = "designation", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 942 @Description(shortDefinition="The designation to be included", formalDefinition="A data group for each designation to be included." ) 943 protected List<DesignationIncludeDesignationComponent> designation; 944 945 private static final long serialVersionUID = -1989669274L; 946 947 /** 948 * Constructor 949 */ 950 public DesignationIncludeComponent() { 951 super(); 952 } 953 954 /** 955 * @return {@link #designation} (A data group for each designation to be included.) 956 */ 957 public List<DesignationIncludeDesignationComponent> getDesignation() { 958 if (this.designation == null) 959 this.designation = new ArrayList<DesignationIncludeDesignationComponent>(); 960 return this.designation; 961 } 962 963 /** 964 * @return Returns a reference to <code>this</code> for easy method chaining 965 */ 966 public DesignationIncludeComponent setDesignation(List<DesignationIncludeDesignationComponent> theDesignation) { 967 this.designation = theDesignation; 968 return this; 969 } 970 971 public boolean hasDesignation() { 972 if (this.designation == null) 973 return false; 974 for (DesignationIncludeDesignationComponent item : this.designation) 975 if (!item.isEmpty()) 976 return true; 977 return false; 978 } 979 980 public DesignationIncludeDesignationComponent addDesignation() { //3 981 DesignationIncludeDesignationComponent t = new DesignationIncludeDesignationComponent(); 982 if (this.designation == null) 983 this.designation = new ArrayList<DesignationIncludeDesignationComponent>(); 984 this.designation.add(t); 985 return t; 986 } 987 988 public DesignationIncludeComponent addDesignation(DesignationIncludeDesignationComponent t) { //3 989 if (t == null) 990 return this; 991 if (this.designation == null) 992 this.designation = new ArrayList<DesignationIncludeDesignationComponent>(); 993 this.designation.add(t); 994 return this; 995 } 996 997 /** 998 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist 999 */ 1000 public DesignationIncludeDesignationComponent getDesignationFirstRep() { 1001 if (getDesignation().isEmpty()) { 1002 addDesignation(); 1003 } 1004 return getDesignation().get(0); 1005 } 1006 1007 protected void listChildren(List<Property> children) { 1008 super.listChildren(children); 1009 children.add(new Property("designation", "", "A data group for each designation to be included.", 0, java.lang.Integer.MAX_VALUE, designation)); 1010 } 1011 1012 @Override 1013 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1014 switch (_hash) { 1015 case -900931593: /*designation*/ return new Property("designation", "", "A data group for each designation to be included.", 0, java.lang.Integer.MAX_VALUE, designation); 1016 default: return super.getNamedProperty(_hash, _name, _checkValid); 1017 } 1018 1019 } 1020 1021 @Override 1022 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1023 switch (hash) { 1024 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // DesignationIncludeDesignationComponent 1025 default: return super.getProperty(hash, name, checkValid); 1026 } 1027 1028 } 1029 1030 @Override 1031 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1032 switch (hash) { 1033 case -900931593: // designation 1034 this.getDesignation().add((DesignationIncludeDesignationComponent) value); // DesignationIncludeDesignationComponent 1035 return value; 1036 default: return super.setProperty(hash, name, value); 1037 } 1038 1039 } 1040 1041 @Override 1042 public Base setProperty(String name, Base value) throws FHIRException { 1043 if (name.equals("designation")) { 1044 this.getDesignation().add((DesignationIncludeDesignationComponent) value); 1045 } else 1046 return super.setProperty(name, value); 1047 return value; 1048 } 1049 1050 @Override 1051 public Base makeProperty(int hash, String name) throws FHIRException { 1052 switch (hash) { 1053 case -900931593: return addDesignation(); 1054 default: return super.makeProperty(hash, name); 1055 } 1056 1057 } 1058 1059 @Override 1060 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1061 switch (hash) { 1062 case -900931593: /*designation*/ return new String[] {}; 1063 default: return super.getTypesForProperty(hash, name); 1064 } 1065 1066 } 1067 1068 @Override 1069 public Base addChild(String name) throws FHIRException { 1070 if (name.equals("designation")) { 1071 return addDesignation(); 1072 } 1073 else 1074 return super.addChild(name); 1075 } 1076 1077 public DesignationIncludeComponent copy() { 1078 DesignationIncludeComponent dst = new DesignationIncludeComponent(); 1079 copyValues(dst); 1080 if (designation != null) { 1081 dst.designation = new ArrayList<DesignationIncludeDesignationComponent>(); 1082 for (DesignationIncludeDesignationComponent i : designation) 1083 dst.designation.add(i.copy()); 1084 }; 1085 return dst; 1086 } 1087 1088 @Override 1089 public boolean equalsDeep(Base other_) { 1090 if (!super.equalsDeep(other_)) 1091 return false; 1092 if (!(other_ instanceof DesignationIncludeComponent)) 1093 return false; 1094 DesignationIncludeComponent o = (DesignationIncludeComponent) other_; 1095 return compareDeep(designation, o.designation, true); 1096 } 1097 1098 @Override 1099 public boolean equalsShallow(Base other_) { 1100 if (!super.equalsShallow(other_)) 1101 return false; 1102 if (!(other_ instanceof DesignationIncludeComponent)) 1103 return false; 1104 DesignationIncludeComponent o = (DesignationIncludeComponent) other_; 1105 return true; 1106 } 1107 1108 public boolean isEmpty() { 1109 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(designation); 1110 } 1111 1112 public String fhirType() { 1113 return "ExpansionProfile.designation.include"; 1114 1115 } 1116 1117 } 1118 1119 @Block() 1120 public static class DesignationIncludeDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1121 /** 1122 * The language this designation is defined for. 1123 */ 1124 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1125 @Description(shortDefinition="Human language of the designation to be included", formalDefinition="The language this designation is defined for." ) 1126 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 1127 protected CodeType language; 1128 1129 /** 1130 * Which kinds of designation to include in the expansion. 1131 */ 1132 @Child(name = "use", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=true) 1133 @Description(shortDefinition="What kind of Designation to include", formalDefinition="Which kinds of designation to include in the expansion." ) 1134 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1135 protected Coding use; 1136 1137 private static final long serialVersionUID = 242239292L; 1138 1139 /** 1140 * Constructor 1141 */ 1142 public DesignationIncludeDesignationComponent() { 1143 super(); 1144 } 1145 1146 /** 1147 * @return {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1148 */ 1149 public CodeType getLanguageElement() { 1150 if (this.language == null) 1151 if (Configuration.errorOnAutoCreate()) 1152 throw new Error("Attempt to auto-create DesignationIncludeDesignationComponent.language"); 1153 else if (Configuration.doAutoCreate()) 1154 this.language = new CodeType(); // bb 1155 return this.language; 1156 } 1157 1158 public boolean hasLanguageElement() { 1159 return this.language != null && !this.language.isEmpty(); 1160 } 1161 1162 public boolean hasLanguage() { 1163 return this.language != null && !this.language.isEmpty(); 1164 } 1165 1166 /** 1167 * @param value {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1168 */ 1169 public DesignationIncludeDesignationComponent setLanguageElement(CodeType value) { 1170 this.language = value; 1171 return this; 1172 } 1173 1174 /** 1175 * @return The language this designation is defined for. 1176 */ 1177 public String getLanguage() { 1178 return this.language == null ? null : this.language.getValue(); 1179 } 1180 1181 /** 1182 * @param value The language this designation is defined for. 1183 */ 1184 public DesignationIncludeDesignationComponent setLanguage(String value) { 1185 if (Utilities.noString(value)) 1186 this.language = null; 1187 else { 1188 if (this.language == null) 1189 this.language = new CodeType(); 1190 this.language.setValue(value); 1191 } 1192 return this; 1193 } 1194 1195 /** 1196 * @return {@link #use} (Which kinds of designation to include in the expansion.) 1197 */ 1198 public Coding getUse() { 1199 if (this.use == null) 1200 if (Configuration.errorOnAutoCreate()) 1201 throw new Error("Attempt to auto-create DesignationIncludeDesignationComponent.use"); 1202 else if (Configuration.doAutoCreate()) 1203 this.use = new Coding(); // cc 1204 return this.use; 1205 } 1206 1207 public boolean hasUse() { 1208 return this.use != null && !this.use.isEmpty(); 1209 } 1210 1211 /** 1212 * @param value {@link #use} (Which kinds of designation to include in the expansion.) 1213 */ 1214 public DesignationIncludeDesignationComponent setUse(Coding value) { 1215 this.use = value; 1216 return this; 1217 } 1218 1219 protected void listChildren(List<Property> children) { 1220 super.listChildren(children); 1221 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 1222 children.add(new Property("use", "Coding", "Which kinds of designation to include in the expansion.", 0, 1, use)); 1223 } 1224 1225 @Override 1226 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1227 switch (_hash) { 1228 case -1613589672: /*language*/ return new Property("language", "code", "The language this designation is defined for.", 0, 1, language); 1229 case 116103: /*use*/ return new Property("use", "Coding", "Which kinds of designation to include in the expansion.", 0, 1, use); 1230 default: return super.getNamedProperty(_hash, _name, _checkValid); 1231 } 1232 1233 } 1234 1235 @Override 1236 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1237 switch (hash) { 1238 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 1239 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Coding 1240 default: return super.getProperty(hash, name, checkValid); 1241 } 1242 1243 } 1244 1245 @Override 1246 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1247 switch (hash) { 1248 case -1613589672: // language 1249 this.language = castToCode(value); // CodeType 1250 return value; 1251 case 116103: // use 1252 this.use = castToCoding(value); // Coding 1253 return value; 1254 default: return super.setProperty(hash, name, value); 1255 } 1256 1257 } 1258 1259 @Override 1260 public Base setProperty(String name, Base value) throws FHIRException { 1261 if (name.equals("language")) { 1262 this.language = castToCode(value); // CodeType 1263 } else if (name.equals("use")) { 1264 this.use = castToCoding(value); // Coding 1265 } else 1266 return super.setProperty(name, value); 1267 return value; 1268 } 1269 1270 @Override 1271 public Base makeProperty(int hash, String name) throws FHIRException { 1272 switch (hash) { 1273 case -1613589672: return getLanguageElement(); 1274 case 116103: return getUse(); 1275 default: return super.makeProperty(hash, name); 1276 } 1277 1278 } 1279 1280 @Override 1281 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1282 switch (hash) { 1283 case -1613589672: /*language*/ return new String[] {"code"}; 1284 case 116103: /*use*/ return new String[] {"Coding"}; 1285 default: return super.getTypesForProperty(hash, name); 1286 } 1287 1288 } 1289 1290 @Override 1291 public Base addChild(String name) throws FHIRException { 1292 if (name.equals("language")) { 1293 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.language"); 1294 } 1295 else if (name.equals("use")) { 1296 this.use = new Coding(); 1297 return this.use; 1298 } 1299 else 1300 return super.addChild(name); 1301 } 1302 1303 public DesignationIncludeDesignationComponent copy() { 1304 DesignationIncludeDesignationComponent dst = new DesignationIncludeDesignationComponent(); 1305 copyValues(dst); 1306 dst.language = language == null ? null : language.copy(); 1307 dst.use = use == null ? null : use.copy(); 1308 return dst; 1309 } 1310 1311 @Override 1312 public boolean equalsDeep(Base other_) { 1313 if (!super.equalsDeep(other_)) 1314 return false; 1315 if (!(other_ instanceof DesignationIncludeDesignationComponent)) 1316 return false; 1317 DesignationIncludeDesignationComponent o = (DesignationIncludeDesignationComponent) other_; 1318 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true); 1319 } 1320 1321 @Override 1322 public boolean equalsShallow(Base other_) { 1323 if (!super.equalsShallow(other_)) 1324 return false; 1325 if (!(other_ instanceof DesignationIncludeDesignationComponent)) 1326 return false; 1327 DesignationIncludeDesignationComponent o = (DesignationIncludeDesignationComponent) other_; 1328 return compareValues(language, o.language, true); 1329 } 1330 1331 public boolean isEmpty() { 1332 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use); 1333 } 1334 1335 public String fhirType() { 1336 return "ExpansionProfile.designation.include.designation"; 1337 1338 } 1339 1340 } 1341 1342 @Block() 1343 public static class DesignationExcludeComponent extends BackboneElement implements IBaseBackboneElement { 1344 /** 1345 * A data group for each designation to be excluded. 1346 */ 1347 @Child(name = "designation", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1348 @Description(shortDefinition="The designation to be excluded", formalDefinition="A data group for each designation to be excluded." ) 1349 protected List<DesignationExcludeDesignationComponent> designation; 1350 1351 private static final long serialVersionUID = 1045849752L; 1352 1353 /** 1354 * Constructor 1355 */ 1356 public DesignationExcludeComponent() { 1357 super(); 1358 } 1359 1360 /** 1361 * @return {@link #designation} (A data group for each designation to be excluded.) 1362 */ 1363 public List<DesignationExcludeDesignationComponent> getDesignation() { 1364 if (this.designation == null) 1365 this.designation = new ArrayList<DesignationExcludeDesignationComponent>(); 1366 return this.designation; 1367 } 1368 1369 /** 1370 * @return Returns a reference to <code>this</code> for easy method chaining 1371 */ 1372 public DesignationExcludeComponent setDesignation(List<DesignationExcludeDesignationComponent> theDesignation) { 1373 this.designation = theDesignation; 1374 return this; 1375 } 1376 1377 public boolean hasDesignation() { 1378 if (this.designation == null) 1379 return false; 1380 for (DesignationExcludeDesignationComponent item : this.designation) 1381 if (!item.isEmpty()) 1382 return true; 1383 return false; 1384 } 1385 1386 public DesignationExcludeDesignationComponent addDesignation() { //3 1387 DesignationExcludeDesignationComponent t = new DesignationExcludeDesignationComponent(); 1388 if (this.designation == null) 1389 this.designation = new ArrayList<DesignationExcludeDesignationComponent>(); 1390 this.designation.add(t); 1391 return t; 1392 } 1393 1394 public DesignationExcludeComponent addDesignation(DesignationExcludeDesignationComponent t) { //3 1395 if (t == null) 1396 return this; 1397 if (this.designation == null) 1398 this.designation = new ArrayList<DesignationExcludeDesignationComponent>(); 1399 this.designation.add(t); 1400 return this; 1401 } 1402 1403 /** 1404 * @return The first repetition of repeating field {@link #designation}, creating it if it does not already exist 1405 */ 1406 public DesignationExcludeDesignationComponent getDesignationFirstRep() { 1407 if (getDesignation().isEmpty()) { 1408 addDesignation(); 1409 } 1410 return getDesignation().get(0); 1411 } 1412 1413 protected void listChildren(List<Property> children) { 1414 super.listChildren(children); 1415 children.add(new Property("designation", "", "A data group for each designation to be excluded.", 0, java.lang.Integer.MAX_VALUE, designation)); 1416 } 1417 1418 @Override 1419 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1420 switch (_hash) { 1421 case -900931593: /*designation*/ return new Property("designation", "", "A data group for each designation to be excluded.", 0, java.lang.Integer.MAX_VALUE, designation); 1422 default: return super.getNamedProperty(_hash, _name, _checkValid); 1423 } 1424 1425 } 1426 1427 @Override 1428 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1429 switch (hash) { 1430 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : this.designation.toArray(new Base[this.designation.size()]); // DesignationExcludeDesignationComponent 1431 default: return super.getProperty(hash, name, checkValid); 1432 } 1433 1434 } 1435 1436 @Override 1437 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1438 switch (hash) { 1439 case -900931593: // designation 1440 this.getDesignation().add((DesignationExcludeDesignationComponent) value); // DesignationExcludeDesignationComponent 1441 return value; 1442 default: return super.setProperty(hash, name, value); 1443 } 1444 1445 } 1446 1447 @Override 1448 public Base setProperty(String name, Base value) throws FHIRException { 1449 if (name.equals("designation")) { 1450 this.getDesignation().add((DesignationExcludeDesignationComponent) value); 1451 } else 1452 return super.setProperty(name, value); 1453 return value; 1454 } 1455 1456 @Override 1457 public Base makeProperty(int hash, String name) throws FHIRException { 1458 switch (hash) { 1459 case -900931593: return addDesignation(); 1460 default: return super.makeProperty(hash, name); 1461 } 1462 1463 } 1464 1465 @Override 1466 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1467 switch (hash) { 1468 case -900931593: /*designation*/ return new String[] {}; 1469 default: return super.getTypesForProperty(hash, name); 1470 } 1471 1472 } 1473 1474 @Override 1475 public Base addChild(String name) throws FHIRException { 1476 if (name.equals("designation")) { 1477 return addDesignation(); 1478 } 1479 else 1480 return super.addChild(name); 1481 } 1482 1483 public DesignationExcludeComponent copy() { 1484 DesignationExcludeComponent dst = new DesignationExcludeComponent(); 1485 copyValues(dst); 1486 if (designation != null) { 1487 dst.designation = new ArrayList<DesignationExcludeDesignationComponent>(); 1488 for (DesignationExcludeDesignationComponent i : designation) 1489 dst.designation.add(i.copy()); 1490 }; 1491 return dst; 1492 } 1493 1494 @Override 1495 public boolean equalsDeep(Base other_) { 1496 if (!super.equalsDeep(other_)) 1497 return false; 1498 if (!(other_ instanceof DesignationExcludeComponent)) 1499 return false; 1500 DesignationExcludeComponent o = (DesignationExcludeComponent) other_; 1501 return compareDeep(designation, o.designation, true); 1502 } 1503 1504 @Override 1505 public boolean equalsShallow(Base other_) { 1506 if (!super.equalsShallow(other_)) 1507 return false; 1508 if (!(other_ instanceof DesignationExcludeComponent)) 1509 return false; 1510 DesignationExcludeComponent o = (DesignationExcludeComponent) other_; 1511 return true; 1512 } 1513 1514 public boolean isEmpty() { 1515 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(designation); 1516 } 1517 1518 public String fhirType() { 1519 return "ExpansionProfile.designation.exclude"; 1520 1521 } 1522 1523 } 1524 1525 @Block() 1526 public static class DesignationExcludeDesignationComponent extends BackboneElement implements IBaseBackboneElement { 1527 /** 1528 * The language this designation is defined for. 1529 */ 1530 @Child(name = "language", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1531 @Description(shortDefinition="Human language of the designation to be excluded", formalDefinition="The language this designation is defined for." ) 1532 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 1533 protected CodeType language; 1534 1535 /** 1536 * Which kinds of designation to exclude from the expansion. 1537 */ 1538 @Child(name = "use", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=true) 1539 @Description(shortDefinition="What kind of Designation to exclude", formalDefinition="Which kinds of designation to exclude from the expansion." ) 1540 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/designation-use") 1541 protected Coding use; 1542 1543 private static final long serialVersionUID = 242239292L; 1544 1545 /** 1546 * Constructor 1547 */ 1548 public DesignationExcludeDesignationComponent() { 1549 super(); 1550 } 1551 1552 /** 1553 * @return {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1554 */ 1555 public CodeType getLanguageElement() { 1556 if (this.language == null) 1557 if (Configuration.errorOnAutoCreate()) 1558 throw new Error("Attempt to auto-create DesignationExcludeDesignationComponent.language"); 1559 else if (Configuration.doAutoCreate()) 1560 this.language = new CodeType(); // bb 1561 return this.language; 1562 } 1563 1564 public boolean hasLanguageElement() { 1565 return this.language != null && !this.language.isEmpty(); 1566 } 1567 1568 public boolean hasLanguage() { 1569 return this.language != null && !this.language.isEmpty(); 1570 } 1571 1572 /** 1573 * @param value {@link #language} (The language this designation is defined for.). This is the underlying object with id, value and extensions. The accessor "getLanguage" gives direct access to the value 1574 */ 1575 public DesignationExcludeDesignationComponent setLanguageElement(CodeType value) { 1576 this.language = value; 1577 return this; 1578 } 1579 1580 /** 1581 * @return The language this designation is defined for. 1582 */ 1583 public String getLanguage() { 1584 return this.language == null ? null : this.language.getValue(); 1585 } 1586 1587 /** 1588 * @param value The language this designation is defined for. 1589 */ 1590 public DesignationExcludeDesignationComponent setLanguage(String value) { 1591 if (Utilities.noString(value)) 1592 this.language = null; 1593 else { 1594 if (this.language == null) 1595 this.language = new CodeType(); 1596 this.language.setValue(value); 1597 } 1598 return this; 1599 } 1600 1601 /** 1602 * @return {@link #use} (Which kinds of designation to exclude from the expansion.) 1603 */ 1604 public Coding getUse() { 1605 if (this.use == null) 1606 if (Configuration.errorOnAutoCreate()) 1607 throw new Error("Attempt to auto-create DesignationExcludeDesignationComponent.use"); 1608 else if (Configuration.doAutoCreate()) 1609 this.use = new Coding(); // cc 1610 return this.use; 1611 } 1612 1613 public boolean hasUse() { 1614 return this.use != null && !this.use.isEmpty(); 1615 } 1616 1617 /** 1618 * @param value {@link #use} (Which kinds of designation to exclude from the expansion.) 1619 */ 1620 public DesignationExcludeDesignationComponent setUse(Coding value) { 1621 this.use = value; 1622 return this; 1623 } 1624 1625 protected void listChildren(List<Property> children) { 1626 super.listChildren(children); 1627 children.add(new Property("language", "code", "The language this designation is defined for.", 0, 1, language)); 1628 children.add(new Property("use", "Coding", "Which kinds of designation to exclude from the expansion.", 0, 1, use)); 1629 } 1630 1631 @Override 1632 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1633 switch (_hash) { 1634 case -1613589672: /*language*/ return new Property("language", "code", "The language this designation is defined for.", 0, 1, language); 1635 case 116103: /*use*/ return new Property("use", "Coding", "Which kinds of designation to exclude from the expansion.", 0, 1, use); 1636 default: return super.getNamedProperty(_hash, _name, _checkValid); 1637 } 1638 1639 } 1640 1641 @Override 1642 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1643 switch (hash) { 1644 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeType 1645 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Coding 1646 default: return super.getProperty(hash, name, checkValid); 1647 } 1648 1649 } 1650 1651 @Override 1652 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1653 switch (hash) { 1654 case -1613589672: // language 1655 this.language = castToCode(value); // CodeType 1656 return value; 1657 case 116103: // use 1658 this.use = castToCoding(value); // Coding 1659 return value; 1660 default: return super.setProperty(hash, name, value); 1661 } 1662 1663 } 1664 1665 @Override 1666 public Base setProperty(String name, Base value) throws FHIRException { 1667 if (name.equals("language")) { 1668 this.language = castToCode(value); // CodeType 1669 } else if (name.equals("use")) { 1670 this.use = castToCoding(value); // Coding 1671 } else 1672 return super.setProperty(name, value); 1673 return value; 1674 } 1675 1676 @Override 1677 public Base makeProperty(int hash, String name) throws FHIRException { 1678 switch (hash) { 1679 case -1613589672: return getLanguageElement(); 1680 case 116103: return getUse(); 1681 default: return super.makeProperty(hash, name); 1682 } 1683 1684 } 1685 1686 @Override 1687 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1688 switch (hash) { 1689 case -1613589672: /*language*/ return new String[] {"code"}; 1690 case 116103: /*use*/ return new String[] {"Coding"}; 1691 default: return super.getTypesForProperty(hash, name); 1692 } 1693 1694 } 1695 1696 @Override 1697 public Base addChild(String name) throws FHIRException { 1698 if (name.equals("language")) { 1699 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.language"); 1700 } 1701 else if (name.equals("use")) { 1702 this.use = new Coding(); 1703 return this.use; 1704 } 1705 else 1706 return super.addChild(name); 1707 } 1708 1709 public DesignationExcludeDesignationComponent copy() { 1710 DesignationExcludeDesignationComponent dst = new DesignationExcludeDesignationComponent(); 1711 copyValues(dst); 1712 dst.language = language == null ? null : language.copy(); 1713 dst.use = use == null ? null : use.copy(); 1714 return dst; 1715 } 1716 1717 @Override 1718 public boolean equalsDeep(Base other_) { 1719 if (!super.equalsDeep(other_)) 1720 return false; 1721 if (!(other_ instanceof DesignationExcludeDesignationComponent)) 1722 return false; 1723 DesignationExcludeDesignationComponent o = (DesignationExcludeDesignationComponent) other_; 1724 return compareDeep(language, o.language, true) && compareDeep(use, o.use, true); 1725 } 1726 1727 @Override 1728 public boolean equalsShallow(Base other_) { 1729 if (!super.equalsShallow(other_)) 1730 return false; 1731 if (!(other_ instanceof DesignationExcludeDesignationComponent)) 1732 return false; 1733 DesignationExcludeDesignationComponent o = (DesignationExcludeDesignationComponent) other_; 1734 return compareValues(language, o.language, true); 1735 } 1736 1737 public boolean isEmpty() { 1738 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(language, use); 1739 } 1740 1741 public String fhirType() { 1742 return "ExpansionProfile.designation.exclude.designation"; 1743 1744 } 1745 1746 } 1747 1748 /** 1749 * A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance. 1750 */ 1751 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 1752 @Description(shortDefinition="Additional identifier for the expansion profile", formalDefinition="A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1753 protected Identifier identifier; 1754 1755 /** 1756 * Fix use of a particular code system to a particular version. 1757 */ 1758 @Child(name = "fixedVersion", type = {}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1759 @Description(shortDefinition="Fix use of a code system to a particular version", formalDefinition="Fix use of a particular code system to a particular version." ) 1760 protected List<ExpansionProfileFixedVersionComponent> fixedVersion; 1761 1762 /** 1763 * Code system, or a particular version of a code system to be excluded from value set expansions. 1764 */ 1765 @Child(name = "excludedSystem", type = {}, order=2, min=0, max=1, modifier=false, summary=true) 1766 @Description(shortDefinition="Systems/Versions to be exclude", formalDefinition="Code system, or a particular version of a code system to be excluded from value set expansions." ) 1767 protected ExpansionProfileExcludedSystemComponent excludedSystem; 1768 1769 /** 1770 * Controls whether concept designations are to be included or excluded in value set expansions. 1771 */ 1772 @Child(name = "includeDesignations", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1773 @Description(shortDefinition="Whether the expansion should include concept designations", formalDefinition="Controls whether concept designations are to be included or excluded in value set expansions." ) 1774 protected BooleanType includeDesignations; 1775 1776 /** 1777 * A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations. 1778 */ 1779 @Child(name = "designation", type = {}, order=4, min=0, max=1, modifier=false, summary=true) 1780 @Description(shortDefinition="When the expansion profile imposes designation contraints", formalDefinition="A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations." ) 1781 protected ExpansionProfileDesignationComponent designation; 1782 1783 /** 1784 * Controls whether the value set definition is included or excluded in value set expansions. 1785 */ 1786 @Child(name = "includeDefinition", type = {BooleanType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1787 @Description(shortDefinition="Include or exclude the value set definition in the expansion", formalDefinition="Controls whether the value set definition is included or excluded in value set expansions." ) 1788 protected BooleanType includeDefinition; 1789 1790 /** 1791 * Controls whether inactive concepts are included or excluded in value set expansions. 1792 */ 1793 @Child(name = "activeOnly", type = {BooleanType.class}, order=6, min=0, max=1, modifier=false, summary=true) 1794 @Description(shortDefinition="Include or exclude inactive concepts in the expansion", formalDefinition="Controls whether inactive concepts are included or excluded in value set expansions." ) 1795 protected BooleanType activeOnly; 1796 1797 /** 1798 * Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains). 1799 */ 1800 @Child(name = "excludeNested", type = {BooleanType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1801 @Description(shortDefinition="Nested codes in the expansion or not", formalDefinition="Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains)." ) 1802 protected BooleanType excludeNested; 1803 1804 /** 1805 * Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces. 1806 */ 1807 @Child(name = "excludeNotForUI", type = {BooleanType.class}, order=8, min=0, max=1, modifier=false, summary=true) 1808 @Description(shortDefinition="Include or exclude codes which cannot be rendered in user interfaces in the value set expansion", formalDefinition="Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces." ) 1809 protected BooleanType excludeNotForUI; 1810 1811 /** 1812 * Controls whether or not the value set expansion includes post coordinated codes. 1813 */ 1814 @Child(name = "excludePostCoordinated", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=true) 1815 @Description(shortDefinition="Include or exclude codes which are post coordinated expressions in the value set expansion", formalDefinition="Controls whether or not the value set expansion includes post coordinated codes." ) 1816 protected BooleanType excludePostCoordinated; 1817 1818 /** 1819 * Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display. 1820 */ 1821 @Child(name = "displayLanguage", type = {CodeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 1822 @Description(shortDefinition="Specify the language for the display element of codes in the value set expansion", formalDefinition="Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display." ) 1823 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 1824 protected CodeType displayLanguage; 1825 1826 /** 1827 * If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html). 1828 */ 1829 @Child(name = "limitedExpansion", type = {BooleanType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1830 @Description(shortDefinition="Controls behaviour of the value set expand operation when value sets are too large to be completely expanded", formalDefinition="If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html)." ) 1831 protected BooleanType limitedExpansion; 1832 1833 private static final long serialVersionUID = 1067457001L; 1834 1835 /** 1836 * Constructor 1837 */ 1838 public ExpansionProfile() { 1839 super(); 1840 } 1841 1842 /** 1843 * Constructor 1844 */ 1845 public ExpansionProfile(Enumeration<PublicationStatus> status) { 1846 super(); 1847 this.status = status; 1848 } 1849 1850 /** 1851 * @return {@link #url} (An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1852 */ 1853 public UriType getUrlElement() { 1854 if (this.url == null) 1855 if (Configuration.errorOnAutoCreate()) 1856 throw new Error("Attempt to auto-create ExpansionProfile.url"); 1857 else if (Configuration.doAutoCreate()) 1858 this.url = new UriType(); // bb 1859 return this.url; 1860 } 1861 1862 public boolean hasUrlElement() { 1863 return this.url != null && !this.url.isEmpty(); 1864 } 1865 1866 public boolean hasUrl() { 1867 return this.url != null && !this.url.isEmpty(); 1868 } 1869 1870 /** 1871 * @param value {@link #url} (An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1872 */ 1873 public ExpansionProfile setUrlElement(UriType value) { 1874 this.url = value; 1875 return this; 1876 } 1877 1878 /** 1879 * @return An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions). 1880 */ 1881 public String getUrl() { 1882 return this.url == null ? null : this.url.getValue(); 1883 } 1884 1885 /** 1886 * @param value An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions). 1887 */ 1888 public ExpansionProfile setUrl(String value) { 1889 if (Utilities.noString(value)) 1890 this.url = null; 1891 else { 1892 if (this.url == null) 1893 this.url = new UriType(); 1894 this.url.setValue(value); 1895 } 1896 return this; 1897 } 1898 1899 /** 1900 * @return {@link #identifier} (A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1901 */ 1902 public Identifier getIdentifier() { 1903 if (this.identifier == null) 1904 if (Configuration.errorOnAutoCreate()) 1905 throw new Error("Attempt to auto-create ExpansionProfile.identifier"); 1906 else if (Configuration.doAutoCreate()) 1907 this.identifier = new Identifier(); // cc 1908 return this.identifier; 1909 } 1910 1911 public boolean hasIdentifier() { 1912 return this.identifier != null && !this.identifier.isEmpty(); 1913 } 1914 1915 /** 1916 * @param value {@link #identifier} (A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1917 */ 1918 public ExpansionProfile setIdentifier(Identifier value) { 1919 this.identifier = value; 1920 return this; 1921 } 1922 1923 /** 1924 * @return {@link #version} (The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1925 */ 1926 public StringType getVersionElement() { 1927 if (this.version == null) 1928 if (Configuration.errorOnAutoCreate()) 1929 throw new Error("Attempt to auto-create ExpansionProfile.version"); 1930 else if (Configuration.doAutoCreate()) 1931 this.version = new StringType(); // bb 1932 return this.version; 1933 } 1934 1935 public boolean hasVersionElement() { 1936 return this.version != null && !this.version.isEmpty(); 1937 } 1938 1939 public boolean hasVersion() { 1940 return this.version != null && !this.version.isEmpty(); 1941 } 1942 1943 /** 1944 * @param value {@link #version} (The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1945 */ 1946 public ExpansionProfile setVersionElement(StringType value) { 1947 this.version = value; 1948 return this; 1949 } 1950 1951 /** 1952 * @return The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1953 */ 1954 public String getVersion() { 1955 return this.version == null ? null : this.version.getValue(); 1956 } 1957 1958 /** 1959 * @param value The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1960 */ 1961 public ExpansionProfile setVersion(String value) { 1962 if (Utilities.noString(value)) 1963 this.version = null; 1964 else { 1965 if (this.version == null) 1966 this.version = new StringType(); 1967 this.version.setValue(value); 1968 } 1969 return this; 1970 } 1971 1972 /** 1973 * @return {@link #name} (A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1974 */ 1975 public StringType getNameElement() { 1976 if (this.name == null) 1977 if (Configuration.errorOnAutoCreate()) 1978 throw new Error("Attempt to auto-create ExpansionProfile.name"); 1979 else if (Configuration.doAutoCreate()) 1980 this.name = new StringType(); // bb 1981 return this.name; 1982 } 1983 1984 public boolean hasNameElement() { 1985 return this.name != null && !this.name.isEmpty(); 1986 } 1987 1988 public boolean hasName() { 1989 return this.name != null && !this.name.isEmpty(); 1990 } 1991 1992 /** 1993 * @param value {@link #name} (A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1994 */ 1995 public ExpansionProfile setNameElement(StringType value) { 1996 this.name = value; 1997 return this; 1998 } 1999 2000 /** 2001 * @return A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2002 */ 2003 public String getName() { 2004 return this.name == null ? null : this.name.getValue(); 2005 } 2006 2007 /** 2008 * @param value A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2009 */ 2010 public ExpansionProfile setName(String value) { 2011 if (Utilities.noString(value)) 2012 this.name = null; 2013 else { 2014 if (this.name == null) 2015 this.name = new StringType(); 2016 this.name.setValue(value); 2017 } 2018 return this; 2019 } 2020 2021 /** 2022 * @return {@link #status} (The status of this expansion profile. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2023 */ 2024 public Enumeration<PublicationStatus> getStatusElement() { 2025 if (this.status == null) 2026 if (Configuration.errorOnAutoCreate()) 2027 throw new Error("Attempt to auto-create ExpansionProfile.status"); 2028 else if (Configuration.doAutoCreate()) 2029 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2030 return this.status; 2031 } 2032 2033 public boolean hasStatusElement() { 2034 return this.status != null && !this.status.isEmpty(); 2035 } 2036 2037 public boolean hasStatus() { 2038 return this.status != null && !this.status.isEmpty(); 2039 } 2040 2041 /** 2042 * @param value {@link #status} (The status of this expansion profile. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2043 */ 2044 public ExpansionProfile setStatusElement(Enumeration<PublicationStatus> value) { 2045 this.status = value; 2046 return this; 2047 } 2048 2049 /** 2050 * @return The status of this expansion profile. Enables tracking the life-cycle of the content. 2051 */ 2052 public PublicationStatus getStatus() { 2053 return this.status == null ? null : this.status.getValue(); 2054 } 2055 2056 /** 2057 * @param value The status of this expansion profile. Enables tracking the life-cycle of the content. 2058 */ 2059 public ExpansionProfile setStatus(PublicationStatus value) { 2060 if (this.status == null) 2061 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2062 this.status.setValue(value); 2063 return this; 2064 } 2065 2066 /** 2067 * @return {@link #experimental} (A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2068 */ 2069 public BooleanType getExperimentalElement() { 2070 if (this.experimental == null) 2071 if (Configuration.errorOnAutoCreate()) 2072 throw new Error("Attempt to auto-create ExpansionProfile.experimental"); 2073 else if (Configuration.doAutoCreate()) 2074 this.experimental = new BooleanType(); // bb 2075 return this.experimental; 2076 } 2077 2078 public boolean hasExperimentalElement() { 2079 return this.experimental != null && !this.experimental.isEmpty(); 2080 } 2081 2082 public boolean hasExperimental() { 2083 return this.experimental != null && !this.experimental.isEmpty(); 2084 } 2085 2086 /** 2087 * @param value {@link #experimental} (A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2088 */ 2089 public ExpansionProfile setExperimentalElement(BooleanType value) { 2090 this.experimental = value; 2091 return this; 2092 } 2093 2094 /** 2095 * @return A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2096 */ 2097 public boolean getExperimental() { 2098 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2099 } 2100 2101 /** 2102 * @param value A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2103 */ 2104 public ExpansionProfile setExperimental(boolean value) { 2105 if (this.experimental == null) 2106 this.experimental = new BooleanType(); 2107 this.experimental.setValue(value); 2108 return this; 2109 } 2110 2111 /** 2112 * @return {@link #date} (The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2113 */ 2114 public DateTimeType getDateElement() { 2115 if (this.date == null) 2116 if (Configuration.errorOnAutoCreate()) 2117 throw new Error("Attempt to auto-create ExpansionProfile.date"); 2118 else if (Configuration.doAutoCreate()) 2119 this.date = new DateTimeType(); // bb 2120 return this.date; 2121 } 2122 2123 public boolean hasDateElement() { 2124 return this.date != null && !this.date.isEmpty(); 2125 } 2126 2127 public boolean hasDate() { 2128 return this.date != null && !this.date.isEmpty(); 2129 } 2130 2131 /** 2132 * @param value {@link #date} (The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2133 */ 2134 public ExpansionProfile setDateElement(DateTimeType value) { 2135 this.date = value; 2136 return this; 2137 } 2138 2139 /** 2140 * @return The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes. 2141 */ 2142 public Date getDate() { 2143 return this.date == null ? null : this.date.getValue(); 2144 } 2145 2146 /** 2147 * @param value The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes. 2148 */ 2149 public ExpansionProfile setDate(Date value) { 2150 if (value == null) 2151 this.date = null; 2152 else { 2153 if (this.date == null) 2154 this.date = new DateTimeType(); 2155 this.date.setValue(value); 2156 } 2157 return this; 2158 } 2159 2160 /** 2161 * @return {@link #publisher} (The name of the individual or organization that published the expansion profile.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2162 */ 2163 public StringType getPublisherElement() { 2164 if (this.publisher == null) 2165 if (Configuration.errorOnAutoCreate()) 2166 throw new Error("Attempt to auto-create ExpansionProfile.publisher"); 2167 else if (Configuration.doAutoCreate()) 2168 this.publisher = new StringType(); // bb 2169 return this.publisher; 2170 } 2171 2172 public boolean hasPublisherElement() { 2173 return this.publisher != null && !this.publisher.isEmpty(); 2174 } 2175 2176 public boolean hasPublisher() { 2177 return this.publisher != null && !this.publisher.isEmpty(); 2178 } 2179 2180 /** 2181 * @param value {@link #publisher} (The name of the individual or organization that published the expansion profile.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2182 */ 2183 public ExpansionProfile setPublisherElement(StringType value) { 2184 this.publisher = value; 2185 return this; 2186 } 2187 2188 /** 2189 * @return The name of the individual or organization that published the expansion profile. 2190 */ 2191 public String getPublisher() { 2192 return this.publisher == null ? null : this.publisher.getValue(); 2193 } 2194 2195 /** 2196 * @param value The name of the individual or organization that published the expansion profile. 2197 */ 2198 public ExpansionProfile setPublisher(String value) { 2199 if (Utilities.noString(value)) 2200 this.publisher = null; 2201 else { 2202 if (this.publisher == null) 2203 this.publisher = new StringType(); 2204 this.publisher.setValue(value); 2205 } 2206 return this; 2207 } 2208 2209 /** 2210 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2211 */ 2212 public List<ContactDetail> getContact() { 2213 if (this.contact == null) 2214 this.contact = new ArrayList<ContactDetail>(); 2215 return this.contact; 2216 } 2217 2218 /** 2219 * @return Returns a reference to <code>this</code> for easy method chaining 2220 */ 2221 public ExpansionProfile setContact(List<ContactDetail> theContact) { 2222 this.contact = theContact; 2223 return this; 2224 } 2225 2226 public boolean hasContact() { 2227 if (this.contact == null) 2228 return false; 2229 for (ContactDetail item : this.contact) 2230 if (!item.isEmpty()) 2231 return true; 2232 return false; 2233 } 2234 2235 public ContactDetail addContact() { //3 2236 ContactDetail t = new ContactDetail(); 2237 if (this.contact == null) 2238 this.contact = new ArrayList<ContactDetail>(); 2239 this.contact.add(t); 2240 return t; 2241 } 2242 2243 public ExpansionProfile addContact(ContactDetail t) { //3 2244 if (t == null) 2245 return this; 2246 if (this.contact == null) 2247 this.contact = new ArrayList<ContactDetail>(); 2248 this.contact.add(t); 2249 return this; 2250 } 2251 2252 /** 2253 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 2254 */ 2255 public ContactDetail getContactFirstRep() { 2256 if (getContact().isEmpty()) { 2257 addContact(); 2258 } 2259 return getContact().get(0); 2260 } 2261 2262 /** 2263 * @return {@link #description} (A free text natural language description of the expansion profile from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2264 */ 2265 public MarkdownType getDescriptionElement() { 2266 if (this.description == null) 2267 if (Configuration.errorOnAutoCreate()) 2268 throw new Error("Attempt to auto-create ExpansionProfile.description"); 2269 else if (Configuration.doAutoCreate()) 2270 this.description = new MarkdownType(); // bb 2271 return this.description; 2272 } 2273 2274 public boolean hasDescriptionElement() { 2275 return this.description != null && !this.description.isEmpty(); 2276 } 2277 2278 public boolean hasDescription() { 2279 return this.description != null && !this.description.isEmpty(); 2280 } 2281 2282 /** 2283 * @param value {@link #description} (A free text natural language description of the expansion profile from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2284 */ 2285 public ExpansionProfile setDescriptionElement(MarkdownType value) { 2286 this.description = value; 2287 return this; 2288 } 2289 2290 /** 2291 * @return A free text natural language description of the expansion profile from a consumer's perspective. 2292 */ 2293 public String getDescription() { 2294 return this.description == null ? null : this.description.getValue(); 2295 } 2296 2297 /** 2298 * @param value A free text natural language description of the expansion profile from a consumer's perspective. 2299 */ 2300 public ExpansionProfile setDescription(String value) { 2301 if (value == null) 2302 this.description = null; 2303 else { 2304 if (this.description == null) 2305 this.description = new MarkdownType(); 2306 this.description.setValue(value); 2307 } 2308 return this; 2309 } 2310 2311 /** 2312 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate expansion profile instances.) 2313 */ 2314 public List<UsageContext> getUseContext() { 2315 if (this.useContext == null) 2316 this.useContext = new ArrayList<UsageContext>(); 2317 return this.useContext; 2318 } 2319 2320 /** 2321 * @return Returns a reference to <code>this</code> for easy method chaining 2322 */ 2323 public ExpansionProfile setUseContext(List<UsageContext> theUseContext) { 2324 this.useContext = theUseContext; 2325 return this; 2326 } 2327 2328 public boolean hasUseContext() { 2329 if (this.useContext == null) 2330 return false; 2331 for (UsageContext item : this.useContext) 2332 if (!item.isEmpty()) 2333 return true; 2334 return false; 2335 } 2336 2337 public UsageContext addUseContext() { //3 2338 UsageContext t = new UsageContext(); 2339 if (this.useContext == null) 2340 this.useContext = new ArrayList<UsageContext>(); 2341 this.useContext.add(t); 2342 return t; 2343 } 2344 2345 public ExpansionProfile addUseContext(UsageContext t) { //3 2346 if (t == null) 2347 return this; 2348 if (this.useContext == null) 2349 this.useContext = new ArrayList<UsageContext>(); 2350 this.useContext.add(t); 2351 return this; 2352 } 2353 2354 /** 2355 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 2356 */ 2357 public UsageContext getUseContextFirstRep() { 2358 if (getUseContext().isEmpty()) { 2359 addUseContext(); 2360 } 2361 return getUseContext().get(0); 2362 } 2363 2364 /** 2365 * @return {@link #jurisdiction} (A legal or geographic region in which the expansion profile is intended to be used.) 2366 */ 2367 public List<CodeableConcept> getJurisdiction() { 2368 if (this.jurisdiction == null) 2369 this.jurisdiction = new ArrayList<CodeableConcept>(); 2370 return this.jurisdiction; 2371 } 2372 2373 /** 2374 * @return Returns a reference to <code>this</code> for easy method chaining 2375 */ 2376 public ExpansionProfile setJurisdiction(List<CodeableConcept> theJurisdiction) { 2377 this.jurisdiction = theJurisdiction; 2378 return this; 2379 } 2380 2381 public boolean hasJurisdiction() { 2382 if (this.jurisdiction == null) 2383 return false; 2384 for (CodeableConcept item : this.jurisdiction) 2385 if (!item.isEmpty()) 2386 return true; 2387 return false; 2388 } 2389 2390 public CodeableConcept addJurisdiction() { //3 2391 CodeableConcept t = new CodeableConcept(); 2392 if (this.jurisdiction == null) 2393 this.jurisdiction = new ArrayList<CodeableConcept>(); 2394 this.jurisdiction.add(t); 2395 return t; 2396 } 2397 2398 public ExpansionProfile addJurisdiction(CodeableConcept t) { //3 2399 if (t == null) 2400 return this; 2401 if (this.jurisdiction == null) 2402 this.jurisdiction = new ArrayList<CodeableConcept>(); 2403 this.jurisdiction.add(t); 2404 return this; 2405 } 2406 2407 /** 2408 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 2409 */ 2410 public CodeableConcept getJurisdictionFirstRep() { 2411 if (getJurisdiction().isEmpty()) { 2412 addJurisdiction(); 2413 } 2414 return getJurisdiction().get(0); 2415 } 2416 2417 /** 2418 * @return {@link #fixedVersion} (Fix use of a particular code system to a particular version.) 2419 */ 2420 public List<ExpansionProfileFixedVersionComponent> getFixedVersion() { 2421 if (this.fixedVersion == null) 2422 this.fixedVersion = new ArrayList<ExpansionProfileFixedVersionComponent>(); 2423 return this.fixedVersion; 2424 } 2425 2426 /** 2427 * @return Returns a reference to <code>this</code> for easy method chaining 2428 */ 2429 public ExpansionProfile setFixedVersion(List<ExpansionProfileFixedVersionComponent> theFixedVersion) { 2430 this.fixedVersion = theFixedVersion; 2431 return this; 2432 } 2433 2434 public boolean hasFixedVersion() { 2435 if (this.fixedVersion == null) 2436 return false; 2437 for (ExpansionProfileFixedVersionComponent item : this.fixedVersion) 2438 if (!item.isEmpty()) 2439 return true; 2440 return false; 2441 } 2442 2443 public ExpansionProfileFixedVersionComponent addFixedVersion() { //3 2444 ExpansionProfileFixedVersionComponent t = new ExpansionProfileFixedVersionComponent(); 2445 if (this.fixedVersion == null) 2446 this.fixedVersion = new ArrayList<ExpansionProfileFixedVersionComponent>(); 2447 this.fixedVersion.add(t); 2448 return t; 2449 } 2450 2451 public ExpansionProfile addFixedVersion(ExpansionProfileFixedVersionComponent t) { //3 2452 if (t == null) 2453 return this; 2454 if (this.fixedVersion == null) 2455 this.fixedVersion = new ArrayList<ExpansionProfileFixedVersionComponent>(); 2456 this.fixedVersion.add(t); 2457 return this; 2458 } 2459 2460 /** 2461 * @return The first repetition of repeating field {@link #fixedVersion}, creating it if it does not already exist 2462 */ 2463 public ExpansionProfileFixedVersionComponent getFixedVersionFirstRep() { 2464 if (getFixedVersion().isEmpty()) { 2465 addFixedVersion(); 2466 } 2467 return getFixedVersion().get(0); 2468 } 2469 2470 /** 2471 * @return {@link #excludedSystem} (Code system, or a particular version of a code system to be excluded from value set expansions.) 2472 */ 2473 public ExpansionProfileExcludedSystemComponent getExcludedSystem() { 2474 if (this.excludedSystem == null) 2475 if (Configuration.errorOnAutoCreate()) 2476 throw new Error("Attempt to auto-create ExpansionProfile.excludedSystem"); 2477 else if (Configuration.doAutoCreate()) 2478 this.excludedSystem = new ExpansionProfileExcludedSystemComponent(); // cc 2479 return this.excludedSystem; 2480 } 2481 2482 public boolean hasExcludedSystem() { 2483 return this.excludedSystem != null && !this.excludedSystem.isEmpty(); 2484 } 2485 2486 /** 2487 * @param value {@link #excludedSystem} (Code system, or a particular version of a code system to be excluded from value set expansions.) 2488 */ 2489 public ExpansionProfile setExcludedSystem(ExpansionProfileExcludedSystemComponent value) { 2490 this.excludedSystem = value; 2491 return this; 2492 } 2493 2494 /** 2495 * @return {@link #includeDesignations} (Controls whether concept designations are to be included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getIncludeDesignations" gives direct access to the value 2496 */ 2497 public BooleanType getIncludeDesignationsElement() { 2498 if (this.includeDesignations == null) 2499 if (Configuration.errorOnAutoCreate()) 2500 throw new Error("Attempt to auto-create ExpansionProfile.includeDesignations"); 2501 else if (Configuration.doAutoCreate()) 2502 this.includeDesignations = new BooleanType(); // bb 2503 return this.includeDesignations; 2504 } 2505 2506 public boolean hasIncludeDesignationsElement() { 2507 return this.includeDesignations != null && !this.includeDesignations.isEmpty(); 2508 } 2509 2510 public boolean hasIncludeDesignations() { 2511 return this.includeDesignations != null && !this.includeDesignations.isEmpty(); 2512 } 2513 2514 /** 2515 * @param value {@link #includeDesignations} (Controls whether concept designations are to be included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getIncludeDesignations" gives direct access to the value 2516 */ 2517 public ExpansionProfile setIncludeDesignationsElement(BooleanType value) { 2518 this.includeDesignations = value; 2519 return this; 2520 } 2521 2522 /** 2523 * @return Controls whether concept designations are to be included or excluded in value set expansions. 2524 */ 2525 public boolean getIncludeDesignations() { 2526 return this.includeDesignations == null || this.includeDesignations.isEmpty() ? false : this.includeDesignations.getValue(); 2527 } 2528 2529 /** 2530 * @param value Controls whether concept designations are to be included or excluded in value set expansions. 2531 */ 2532 public ExpansionProfile setIncludeDesignations(boolean value) { 2533 if (this.includeDesignations == null) 2534 this.includeDesignations = new BooleanType(); 2535 this.includeDesignations.setValue(value); 2536 return this; 2537 } 2538 2539 /** 2540 * @return {@link #designation} (A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations.) 2541 */ 2542 public ExpansionProfileDesignationComponent getDesignation() { 2543 if (this.designation == null) 2544 if (Configuration.errorOnAutoCreate()) 2545 throw new Error("Attempt to auto-create ExpansionProfile.designation"); 2546 else if (Configuration.doAutoCreate()) 2547 this.designation = new ExpansionProfileDesignationComponent(); // cc 2548 return this.designation; 2549 } 2550 2551 public boolean hasDesignation() { 2552 return this.designation != null && !this.designation.isEmpty(); 2553 } 2554 2555 /** 2556 * @param value {@link #designation} (A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations.) 2557 */ 2558 public ExpansionProfile setDesignation(ExpansionProfileDesignationComponent value) { 2559 this.designation = value; 2560 return this; 2561 } 2562 2563 /** 2564 * @return {@link #includeDefinition} (Controls whether the value set definition is included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getIncludeDefinition" gives direct access to the value 2565 */ 2566 public BooleanType getIncludeDefinitionElement() { 2567 if (this.includeDefinition == null) 2568 if (Configuration.errorOnAutoCreate()) 2569 throw new Error("Attempt to auto-create ExpansionProfile.includeDefinition"); 2570 else if (Configuration.doAutoCreate()) 2571 this.includeDefinition = new BooleanType(); // bb 2572 return this.includeDefinition; 2573 } 2574 2575 public boolean hasIncludeDefinitionElement() { 2576 return this.includeDefinition != null && !this.includeDefinition.isEmpty(); 2577 } 2578 2579 public boolean hasIncludeDefinition() { 2580 return this.includeDefinition != null && !this.includeDefinition.isEmpty(); 2581 } 2582 2583 /** 2584 * @param value {@link #includeDefinition} (Controls whether the value set definition is included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getIncludeDefinition" gives direct access to the value 2585 */ 2586 public ExpansionProfile setIncludeDefinitionElement(BooleanType value) { 2587 this.includeDefinition = value; 2588 return this; 2589 } 2590 2591 /** 2592 * @return Controls whether the value set definition is included or excluded in value set expansions. 2593 */ 2594 public boolean getIncludeDefinition() { 2595 return this.includeDefinition == null || this.includeDefinition.isEmpty() ? false : this.includeDefinition.getValue(); 2596 } 2597 2598 /** 2599 * @param value Controls whether the value set definition is included or excluded in value set expansions. 2600 */ 2601 public ExpansionProfile setIncludeDefinition(boolean value) { 2602 if (this.includeDefinition == null) 2603 this.includeDefinition = new BooleanType(); 2604 this.includeDefinition.setValue(value); 2605 return this; 2606 } 2607 2608 /** 2609 * @return {@link #activeOnly} (Controls whether inactive concepts are included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getActiveOnly" gives direct access to the value 2610 */ 2611 public BooleanType getActiveOnlyElement() { 2612 if (this.activeOnly == null) 2613 if (Configuration.errorOnAutoCreate()) 2614 throw new Error("Attempt to auto-create ExpansionProfile.activeOnly"); 2615 else if (Configuration.doAutoCreate()) 2616 this.activeOnly = new BooleanType(); // bb 2617 return this.activeOnly; 2618 } 2619 2620 public boolean hasActiveOnlyElement() { 2621 return this.activeOnly != null && !this.activeOnly.isEmpty(); 2622 } 2623 2624 public boolean hasActiveOnly() { 2625 return this.activeOnly != null && !this.activeOnly.isEmpty(); 2626 } 2627 2628 /** 2629 * @param value {@link #activeOnly} (Controls whether inactive concepts are included or excluded in value set expansions.). This is the underlying object with id, value and extensions. The accessor "getActiveOnly" gives direct access to the value 2630 */ 2631 public ExpansionProfile setActiveOnlyElement(BooleanType value) { 2632 this.activeOnly = value; 2633 return this; 2634 } 2635 2636 /** 2637 * @return Controls whether inactive concepts are included or excluded in value set expansions. 2638 */ 2639 public boolean getActiveOnly() { 2640 return this.activeOnly == null || this.activeOnly.isEmpty() ? false : this.activeOnly.getValue(); 2641 } 2642 2643 /** 2644 * @param value Controls whether inactive concepts are included or excluded in value set expansions. 2645 */ 2646 public ExpansionProfile setActiveOnly(boolean value) { 2647 if (this.activeOnly == null) 2648 this.activeOnly = new BooleanType(); 2649 this.activeOnly.setValue(value); 2650 return this; 2651 } 2652 2653 /** 2654 * @return {@link #excludeNested} (Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains).). This is the underlying object with id, value and extensions. The accessor "getExcludeNested" gives direct access to the value 2655 */ 2656 public BooleanType getExcludeNestedElement() { 2657 if (this.excludeNested == null) 2658 if (Configuration.errorOnAutoCreate()) 2659 throw new Error("Attempt to auto-create ExpansionProfile.excludeNested"); 2660 else if (Configuration.doAutoCreate()) 2661 this.excludeNested = new BooleanType(); // bb 2662 return this.excludeNested; 2663 } 2664 2665 public boolean hasExcludeNestedElement() { 2666 return this.excludeNested != null && !this.excludeNested.isEmpty(); 2667 } 2668 2669 public boolean hasExcludeNested() { 2670 return this.excludeNested != null && !this.excludeNested.isEmpty(); 2671 } 2672 2673 /** 2674 * @param value {@link #excludeNested} (Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains).). This is the underlying object with id, value and extensions. The accessor "getExcludeNested" gives direct access to the value 2675 */ 2676 public ExpansionProfile setExcludeNestedElement(BooleanType value) { 2677 this.excludeNested = value; 2678 return this; 2679 } 2680 2681 /** 2682 * @return Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains). 2683 */ 2684 public boolean getExcludeNested() { 2685 return this.excludeNested == null || this.excludeNested.isEmpty() ? false : this.excludeNested.getValue(); 2686 } 2687 2688 /** 2689 * @param value Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains). 2690 */ 2691 public ExpansionProfile setExcludeNested(boolean value) { 2692 if (this.excludeNested == null) 2693 this.excludeNested = new BooleanType(); 2694 this.excludeNested.setValue(value); 2695 return this; 2696 } 2697 2698 /** 2699 * @return {@link #excludeNotForUI} (Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces.). This is the underlying object with id, value and extensions. The accessor "getExcludeNotForUI" gives direct access to the value 2700 */ 2701 public BooleanType getExcludeNotForUIElement() { 2702 if (this.excludeNotForUI == null) 2703 if (Configuration.errorOnAutoCreate()) 2704 throw new Error("Attempt to auto-create ExpansionProfile.excludeNotForUI"); 2705 else if (Configuration.doAutoCreate()) 2706 this.excludeNotForUI = new BooleanType(); // bb 2707 return this.excludeNotForUI; 2708 } 2709 2710 public boolean hasExcludeNotForUIElement() { 2711 return this.excludeNotForUI != null && !this.excludeNotForUI.isEmpty(); 2712 } 2713 2714 public boolean hasExcludeNotForUI() { 2715 return this.excludeNotForUI != null && !this.excludeNotForUI.isEmpty(); 2716 } 2717 2718 /** 2719 * @param value {@link #excludeNotForUI} (Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces.). This is the underlying object with id, value and extensions. The accessor "getExcludeNotForUI" gives direct access to the value 2720 */ 2721 public ExpansionProfile setExcludeNotForUIElement(BooleanType value) { 2722 this.excludeNotForUI = value; 2723 return this; 2724 } 2725 2726 /** 2727 * @return Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces. 2728 */ 2729 public boolean getExcludeNotForUI() { 2730 return this.excludeNotForUI == null || this.excludeNotForUI.isEmpty() ? false : this.excludeNotForUI.getValue(); 2731 } 2732 2733 /** 2734 * @param value Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces. 2735 */ 2736 public ExpansionProfile setExcludeNotForUI(boolean value) { 2737 if (this.excludeNotForUI == null) 2738 this.excludeNotForUI = new BooleanType(); 2739 this.excludeNotForUI.setValue(value); 2740 return this; 2741 } 2742 2743 /** 2744 * @return {@link #excludePostCoordinated} (Controls whether or not the value set expansion includes post coordinated codes.). This is the underlying object with id, value and extensions. The accessor "getExcludePostCoordinated" gives direct access to the value 2745 */ 2746 public BooleanType getExcludePostCoordinatedElement() { 2747 if (this.excludePostCoordinated == null) 2748 if (Configuration.errorOnAutoCreate()) 2749 throw new Error("Attempt to auto-create ExpansionProfile.excludePostCoordinated"); 2750 else if (Configuration.doAutoCreate()) 2751 this.excludePostCoordinated = new BooleanType(); // bb 2752 return this.excludePostCoordinated; 2753 } 2754 2755 public boolean hasExcludePostCoordinatedElement() { 2756 return this.excludePostCoordinated != null && !this.excludePostCoordinated.isEmpty(); 2757 } 2758 2759 public boolean hasExcludePostCoordinated() { 2760 return this.excludePostCoordinated != null && !this.excludePostCoordinated.isEmpty(); 2761 } 2762 2763 /** 2764 * @param value {@link #excludePostCoordinated} (Controls whether or not the value set expansion includes post coordinated codes.). This is the underlying object with id, value and extensions. The accessor "getExcludePostCoordinated" gives direct access to the value 2765 */ 2766 public ExpansionProfile setExcludePostCoordinatedElement(BooleanType value) { 2767 this.excludePostCoordinated = value; 2768 return this; 2769 } 2770 2771 /** 2772 * @return Controls whether or not the value set expansion includes post coordinated codes. 2773 */ 2774 public boolean getExcludePostCoordinated() { 2775 return this.excludePostCoordinated == null || this.excludePostCoordinated.isEmpty() ? false : this.excludePostCoordinated.getValue(); 2776 } 2777 2778 /** 2779 * @param value Controls whether or not the value set expansion includes post coordinated codes. 2780 */ 2781 public ExpansionProfile setExcludePostCoordinated(boolean value) { 2782 if (this.excludePostCoordinated == null) 2783 this.excludePostCoordinated = new BooleanType(); 2784 this.excludePostCoordinated.setValue(value); 2785 return this; 2786 } 2787 2788 /** 2789 * @return {@link #displayLanguage} (Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display.). This is the underlying object with id, value and extensions. The accessor "getDisplayLanguage" gives direct access to the value 2790 */ 2791 public CodeType getDisplayLanguageElement() { 2792 if (this.displayLanguage == null) 2793 if (Configuration.errorOnAutoCreate()) 2794 throw new Error("Attempt to auto-create ExpansionProfile.displayLanguage"); 2795 else if (Configuration.doAutoCreate()) 2796 this.displayLanguage = new CodeType(); // bb 2797 return this.displayLanguage; 2798 } 2799 2800 public boolean hasDisplayLanguageElement() { 2801 return this.displayLanguage != null && !this.displayLanguage.isEmpty(); 2802 } 2803 2804 public boolean hasDisplayLanguage() { 2805 return this.displayLanguage != null && !this.displayLanguage.isEmpty(); 2806 } 2807 2808 /** 2809 * @param value {@link #displayLanguage} (Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display.). This is the underlying object with id, value and extensions. The accessor "getDisplayLanguage" gives direct access to the value 2810 */ 2811 public ExpansionProfile setDisplayLanguageElement(CodeType value) { 2812 this.displayLanguage = value; 2813 return this; 2814 } 2815 2816 /** 2817 * @return Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display. 2818 */ 2819 public String getDisplayLanguage() { 2820 return this.displayLanguage == null ? null : this.displayLanguage.getValue(); 2821 } 2822 2823 /** 2824 * @param value Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display. 2825 */ 2826 public ExpansionProfile setDisplayLanguage(String value) { 2827 if (Utilities.noString(value)) 2828 this.displayLanguage = null; 2829 else { 2830 if (this.displayLanguage == null) 2831 this.displayLanguage = new CodeType(); 2832 this.displayLanguage.setValue(value); 2833 } 2834 return this; 2835 } 2836 2837 /** 2838 * @return {@link #limitedExpansion} (If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html).). This is the underlying object with id, value and extensions. The accessor "getLimitedExpansion" gives direct access to the value 2839 */ 2840 public BooleanType getLimitedExpansionElement() { 2841 if (this.limitedExpansion == null) 2842 if (Configuration.errorOnAutoCreate()) 2843 throw new Error("Attempt to auto-create ExpansionProfile.limitedExpansion"); 2844 else if (Configuration.doAutoCreate()) 2845 this.limitedExpansion = new BooleanType(); // bb 2846 return this.limitedExpansion; 2847 } 2848 2849 public boolean hasLimitedExpansionElement() { 2850 return this.limitedExpansion != null && !this.limitedExpansion.isEmpty(); 2851 } 2852 2853 public boolean hasLimitedExpansion() { 2854 return this.limitedExpansion != null && !this.limitedExpansion.isEmpty(); 2855 } 2856 2857 /** 2858 * @param value {@link #limitedExpansion} (If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html).). This is the underlying object with id, value and extensions. The accessor "getLimitedExpansion" gives direct access to the value 2859 */ 2860 public ExpansionProfile setLimitedExpansionElement(BooleanType value) { 2861 this.limitedExpansion = value; 2862 return this; 2863 } 2864 2865 /** 2866 * @return If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html). 2867 */ 2868 public boolean getLimitedExpansion() { 2869 return this.limitedExpansion == null || this.limitedExpansion.isEmpty() ? false : this.limitedExpansion.getValue(); 2870 } 2871 2872 /** 2873 * @param value If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html). 2874 */ 2875 public ExpansionProfile setLimitedExpansion(boolean value) { 2876 if (this.limitedExpansion == null) 2877 this.limitedExpansion = new BooleanType(); 2878 this.limitedExpansion.setValue(value); 2879 return this; 2880 } 2881 2882 protected void listChildren(List<Property> children) { 2883 super.listChildren(children); 2884 children.add(new Property("url", "uri", "An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 2885 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier)); 2886 children.add(new Property("version", "string", "The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2887 children.add(new Property("name", "string", "A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2888 children.add(new Property("status", "code", "The status of this expansion profile. Enables tracking the life-cycle of the content.", 0, 1, status)); 2889 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 2890 children.add(new Property("date", "dateTime", "The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes.", 0, 1, date)); 2891 children.add(new Property("publisher", "string", "The name of the individual or organization that published the expansion profile.", 0, 1, publisher)); 2892 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2893 children.add(new Property("description", "markdown", "A free text natural language description of the expansion profile from a consumer's perspective.", 0, 1, description)); 2894 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate expansion profile instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2895 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the expansion profile is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2896 children.add(new Property("fixedVersion", "", "Fix use of a particular code system to a particular version.", 0, java.lang.Integer.MAX_VALUE, fixedVersion)); 2897 children.add(new Property("excludedSystem", "", "Code system, or a particular version of a code system to be excluded from value set expansions.", 0, 1, excludedSystem)); 2898 children.add(new Property("includeDesignations", "boolean", "Controls whether concept designations are to be included or excluded in value set expansions.", 0, 1, includeDesignations)); 2899 children.add(new Property("designation", "", "A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations.", 0, 1, designation)); 2900 children.add(new Property("includeDefinition", "boolean", "Controls whether the value set definition is included or excluded in value set expansions.", 0, 1, includeDefinition)); 2901 children.add(new Property("activeOnly", "boolean", "Controls whether inactive concepts are included or excluded in value set expansions.", 0, 1, activeOnly)); 2902 children.add(new Property("excludeNested", "boolean", "Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains).", 0, 1, excludeNested)); 2903 children.add(new Property("excludeNotForUI", "boolean", "Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces.", 0, 1, excludeNotForUI)); 2904 children.add(new Property("excludePostCoordinated", "boolean", "Controls whether or not the value set expansion includes post coordinated codes.", 0, 1, excludePostCoordinated)); 2905 children.add(new Property("displayLanguage", "code", "Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display.", 0, 1, displayLanguage)); 2906 children.add(new Property("limitedExpansion", "boolean", "If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html).", 0, 1, limitedExpansion)); 2907 } 2908 2909 @Override 2910 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2911 switch (_hash) { 2912 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this expansion profile when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this expansion profile is (or will be) published. The URL SHOULD include the major version of the expansion profile. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 2913 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this expansion profile when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier); 2914 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the expansion profile when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the expansion profile author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2915 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the expansion profile. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2916 case -892481550: /*status*/ return new Property("status", "code", "The status of this expansion profile. Enables tracking the life-cycle of the content.", 0, 1, status); 2917 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this expansion profile is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 2918 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the expansion profile was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the expansion profile changes.", 0, 1, date); 2919 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the expansion profile.", 0, 1, publisher); 2920 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2921 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the expansion profile from a consumer's perspective.", 0, 1, description); 2922 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate expansion profile instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2923 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the expansion profile is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2924 case 807748292: /*fixedVersion*/ return new Property("fixedVersion", "", "Fix use of a particular code system to a particular version.", 0, java.lang.Integer.MAX_VALUE, fixedVersion); 2925 case 2125282457: /*excludedSystem*/ return new Property("excludedSystem", "", "Code system, or a particular version of a code system to be excluded from value set expansions.", 0, 1, excludedSystem); 2926 case 461507620: /*includeDesignations*/ return new Property("includeDesignations", "boolean", "Controls whether concept designations are to be included or excluded in value set expansions.", 0, 1, includeDesignations); 2927 case -900931593: /*designation*/ return new Property("designation", "", "A set of criteria that provide the constraints imposed on the value set expansion by including or excluding designations.", 0, 1, designation); 2928 case 127972379: /*includeDefinition*/ return new Property("includeDefinition", "boolean", "Controls whether the value set definition is included or excluded in value set expansions.", 0, 1, includeDefinition); 2929 case 2043813842: /*activeOnly*/ return new Property("activeOnly", "boolean", "Controls whether inactive concepts are included or excluded in value set expansions.", 0, 1, activeOnly); 2930 case 424992625: /*excludeNested*/ return new Property("excludeNested", "boolean", "Controls whether or not the value set expansion nests codes or not (i.e. ValueSet.expansion.contains.contains).", 0, 1, excludeNested); 2931 case 667582980: /*excludeNotForUI*/ return new Property("excludeNotForUI", "boolean", "Controls whether or not the value set expansion includes codes which cannot be displayed in user interfaces.", 0, 1, excludeNotForUI); 2932 case 563335154: /*excludePostCoordinated*/ return new Property("excludePostCoordinated", "boolean", "Controls whether or not the value set expansion includes post coordinated codes.", 0, 1, excludePostCoordinated); 2933 case 1486237242: /*displayLanguage*/ return new Property("displayLanguage", "code", "Specifies the language to be used for description in the expansions i.e. the language to be used for ValueSet.expansion.contains.display.", 0, 1, displayLanguage); 2934 case 597771333: /*limitedExpansion*/ return new Property("limitedExpansion", "boolean", "If the value set being expanded is incomplete (because it is too big to expand), return a limited expansion (a subset) with an indicator that expansion is incomplete, using the extension [http://hl7.org/fhir/StructureDefinition/valueset-toocostly](extension-valueset-toocostly.html).", 0, 1, limitedExpansion); 2935 default: return super.getNamedProperty(_hash, _name, _checkValid); 2936 } 2937 2938 } 2939 2940 @Override 2941 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2942 switch (hash) { 2943 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2944 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2945 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2946 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2947 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2948 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2949 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2950 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2951 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2952 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2953 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2954 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2955 case 807748292: /*fixedVersion*/ return this.fixedVersion == null ? new Base[0] : this.fixedVersion.toArray(new Base[this.fixedVersion.size()]); // ExpansionProfileFixedVersionComponent 2956 case 2125282457: /*excludedSystem*/ return this.excludedSystem == null ? new Base[0] : new Base[] {this.excludedSystem}; // ExpansionProfileExcludedSystemComponent 2957 case 461507620: /*includeDesignations*/ return this.includeDesignations == null ? new Base[0] : new Base[] {this.includeDesignations}; // BooleanType 2958 case -900931593: /*designation*/ return this.designation == null ? new Base[0] : new Base[] {this.designation}; // ExpansionProfileDesignationComponent 2959 case 127972379: /*includeDefinition*/ return this.includeDefinition == null ? new Base[0] : new Base[] {this.includeDefinition}; // BooleanType 2960 case 2043813842: /*activeOnly*/ return this.activeOnly == null ? new Base[0] : new Base[] {this.activeOnly}; // BooleanType 2961 case 424992625: /*excludeNested*/ return this.excludeNested == null ? new Base[0] : new Base[] {this.excludeNested}; // BooleanType 2962 case 667582980: /*excludeNotForUI*/ return this.excludeNotForUI == null ? new Base[0] : new Base[] {this.excludeNotForUI}; // BooleanType 2963 case 563335154: /*excludePostCoordinated*/ return this.excludePostCoordinated == null ? new Base[0] : new Base[] {this.excludePostCoordinated}; // BooleanType 2964 case 1486237242: /*displayLanguage*/ return this.displayLanguage == null ? new Base[0] : new Base[] {this.displayLanguage}; // CodeType 2965 case 597771333: /*limitedExpansion*/ return this.limitedExpansion == null ? new Base[0] : new Base[] {this.limitedExpansion}; // BooleanType 2966 default: return super.getProperty(hash, name, checkValid); 2967 } 2968 2969 } 2970 2971 @Override 2972 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2973 switch (hash) { 2974 case 116079: // url 2975 this.url = castToUri(value); // UriType 2976 return value; 2977 case -1618432855: // identifier 2978 this.identifier = castToIdentifier(value); // Identifier 2979 return value; 2980 case 351608024: // version 2981 this.version = castToString(value); // StringType 2982 return value; 2983 case 3373707: // name 2984 this.name = castToString(value); // StringType 2985 return value; 2986 case -892481550: // status 2987 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2988 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2989 return value; 2990 case -404562712: // experimental 2991 this.experimental = castToBoolean(value); // BooleanType 2992 return value; 2993 case 3076014: // date 2994 this.date = castToDateTime(value); // DateTimeType 2995 return value; 2996 case 1447404028: // publisher 2997 this.publisher = castToString(value); // StringType 2998 return value; 2999 case 951526432: // contact 3000 this.getContact().add(castToContactDetail(value)); // ContactDetail 3001 return value; 3002 case -1724546052: // description 3003 this.description = castToMarkdown(value); // MarkdownType 3004 return value; 3005 case -669707736: // useContext 3006 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3007 return value; 3008 case -507075711: // jurisdiction 3009 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3010 return value; 3011 case 807748292: // fixedVersion 3012 this.getFixedVersion().add((ExpansionProfileFixedVersionComponent) value); // ExpansionProfileFixedVersionComponent 3013 return value; 3014 case 2125282457: // excludedSystem 3015 this.excludedSystem = (ExpansionProfileExcludedSystemComponent) value; // ExpansionProfileExcludedSystemComponent 3016 return value; 3017 case 461507620: // includeDesignations 3018 this.includeDesignations = castToBoolean(value); // BooleanType 3019 return value; 3020 case -900931593: // designation 3021 this.designation = (ExpansionProfileDesignationComponent) value; // ExpansionProfileDesignationComponent 3022 return value; 3023 case 127972379: // includeDefinition 3024 this.includeDefinition = castToBoolean(value); // BooleanType 3025 return value; 3026 case 2043813842: // activeOnly 3027 this.activeOnly = castToBoolean(value); // BooleanType 3028 return value; 3029 case 424992625: // excludeNested 3030 this.excludeNested = castToBoolean(value); // BooleanType 3031 return value; 3032 case 667582980: // excludeNotForUI 3033 this.excludeNotForUI = castToBoolean(value); // BooleanType 3034 return value; 3035 case 563335154: // excludePostCoordinated 3036 this.excludePostCoordinated = castToBoolean(value); // BooleanType 3037 return value; 3038 case 1486237242: // displayLanguage 3039 this.displayLanguage = castToCode(value); // CodeType 3040 return value; 3041 case 597771333: // limitedExpansion 3042 this.limitedExpansion = castToBoolean(value); // BooleanType 3043 return value; 3044 default: return super.setProperty(hash, name, value); 3045 } 3046 3047 } 3048 3049 @Override 3050 public Base setProperty(String name, Base value) throws FHIRException { 3051 if (name.equals("url")) { 3052 this.url = castToUri(value); // UriType 3053 } else if (name.equals("identifier")) { 3054 this.identifier = castToIdentifier(value); // Identifier 3055 } else if (name.equals("version")) { 3056 this.version = castToString(value); // StringType 3057 } else if (name.equals("name")) { 3058 this.name = castToString(value); // StringType 3059 } else if (name.equals("status")) { 3060 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3061 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3062 } else if (name.equals("experimental")) { 3063 this.experimental = castToBoolean(value); // BooleanType 3064 } else if (name.equals("date")) { 3065 this.date = castToDateTime(value); // DateTimeType 3066 } else if (name.equals("publisher")) { 3067 this.publisher = castToString(value); // StringType 3068 } else if (name.equals("contact")) { 3069 this.getContact().add(castToContactDetail(value)); 3070 } else if (name.equals("description")) { 3071 this.description = castToMarkdown(value); // MarkdownType 3072 } else if (name.equals("useContext")) { 3073 this.getUseContext().add(castToUsageContext(value)); 3074 } else if (name.equals("jurisdiction")) { 3075 this.getJurisdiction().add(castToCodeableConcept(value)); 3076 } else if (name.equals("fixedVersion")) { 3077 this.getFixedVersion().add((ExpansionProfileFixedVersionComponent) value); 3078 } else if (name.equals("excludedSystem")) { 3079 this.excludedSystem = (ExpansionProfileExcludedSystemComponent) value; // ExpansionProfileExcludedSystemComponent 3080 } else if (name.equals("includeDesignations")) { 3081 this.includeDesignations = castToBoolean(value); // BooleanType 3082 } else if (name.equals("designation")) { 3083 this.designation = (ExpansionProfileDesignationComponent) value; // ExpansionProfileDesignationComponent 3084 } else if (name.equals("includeDefinition")) { 3085 this.includeDefinition = castToBoolean(value); // BooleanType 3086 } else if (name.equals("activeOnly")) { 3087 this.activeOnly = castToBoolean(value); // BooleanType 3088 } else if (name.equals("excludeNested")) { 3089 this.excludeNested = castToBoolean(value); // BooleanType 3090 } else if (name.equals("excludeNotForUI")) { 3091 this.excludeNotForUI = castToBoolean(value); // BooleanType 3092 } else if (name.equals("excludePostCoordinated")) { 3093 this.excludePostCoordinated = castToBoolean(value); // BooleanType 3094 } else if (name.equals("displayLanguage")) { 3095 this.displayLanguage = castToCode(value); // CodeType 3096 } else if (name.equals("limitedExpansion")) { 3097 this.limitedExpansion = castToBoolean(value); // BooleanType 3098 } else 3099 return super.setProperty(name, value); 3100 return value; 3101 } 3102 3103 @Override 3104 public Base makeProperty(int hash, String name) throws FHIRException { 3105 switch (hash) { 3106 case 116079: return getUrlElement(); 3107 case -1618432855: return getIdentifier(); 3108 case 351608024: return getVersionElement(); 3109 case 3373707: return getNameElement(); 3110 case -892481550: return getStatusElement(); 3111 case -404562712: return getExperimentalElement(); 3112 case 3076014: return getDateElement(); 3113 case 1447404028: return getPublisherElement(); 3114 case 951526432: return addContact(); 3115 case -1724546052: return getDescriptionElement(); 3116 case -669707736: return addUseContext(); 3117 case -507075711: return addJurisdiction(); 3118 case 807748292: return addFixedVersion(); 3119 case 2125282457: return getExcludedSystem(); 3120 case 461507620: return getIncludeDesignationsElement(); 3121 case -900931593: return getDesignation(); 3122 case 127972379: return getIncludeDefinitionElement(); 3123 case 2043813842: return getActiveOnlyElement(); 3124 case 424992625: return getExcludeNestedElement(); 3125 case 667582980: return getExcludeNotForUIElement(); 3126 case 563335154: return getExcludePostCoordinatedElement(); 3127 case 1486237242: return getDisplayLanguageElement(); 3128 case 597771333: return getLimitedExpansionElement(); 3129 default: return super.makeProperty(hash, name); 3130 } 3131 3132 } 3133 3134 @Override 3135 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3136 switch (hash) { 3137 case 116079: /*url*/ return new String[] {"uri"}; 3138 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3139 case 351608024: /*version*/ return new String[] {"string"}; 3140 case 3373707: /*name*/ return new String[] {"string"}; 3141 case -892481550: /*status*/ return new String[] {"code"}; 3142 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3143 case 3076014: /*date*/ return new String[] {"dateTime"}; 3144 case 1447404028: /*publisher*/ return new String[] {"string"}; 3145 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3146 case -1724546052: /*description*/ return new String[] {"markdown"}; 3147 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3148 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3149 case 807748292: /*fixedVersion*/ return new String[] {}; 3150 case 2125282457: /*excludedSystem*/ return new String[] {}; 3151 case 461507620: /*includeDesignations*/ return new String[] {"boolean"}; 3152 case -900931593: /*designation*/ return new String[] {}; 3153 case 127972379: /*includeDefinition*/ return new String[] {"boolean"}; 3154 case 2043813842: /*activeOnly*/ return new String[] {"boolean"}; 3155 case 424992625: /*excludeNested*/ return new String[] {"boolean"}; 3156 case 667582980: /*excludeNotForUI*/ return new String[] {"boolean"}; 3157 case 563335154: /*excludePostCoordinated*/ return new String[] {"boolean"}; 3158 case 1486237242: /*displayLanguage*/ return new String[] {"code"}; 3159 case 597771333: /*limitedExpansion*/ return new String[] {"boolean"}; 3160 default: return super.getTypesForProperty(hash, name); 3161 } 3162 3163 } 3164 3165 @Override 3166 public Base addChild(String name) throws FHIRException { 3167 if (name.equals("url")) { 3168 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.url"); 3169 } 3170 else if (name.equals("identifier")) { 3171 this.identifier = new Identifier(); 3172 return this.identifier; 3173 } 3174 else if (name.equals("version")) { 3175 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.version"); 3176 } 3177 else if (name.equals("name")) { 3178 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.name"); 3179 } 3180 else if (name.equals("status")) { 3181 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.status"); 3182 } 3183 else if (name.equals("experimental")) { 3184 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.experimental"); 3185 } 3186 else if (name.equals("date")) { 3187 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.date"); 3188 } 3189 else if (name.equals("publisher")) { 3190 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.publisher"); 3191 } 3192 else if (name.equals("contact")) { 3193 return addContact(); 3194 } 3195 else if (name.equals("description")) { 3196 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.description"); 3197 } 3198 else if (name.equals("useContext")) { 3199 return addUseContext(); 3200 } 3201 else if (name.equals("jurisdiction")) { 3202 return addJurisdiction(); 3203 } 3204 else if (name.equals("fixedVersion")) { 3205 return addFixedVersion(); 3206 } 3207 else if (name.equals("excludedSystem")) { 3208 this.excludedSystem = new ExpansionProfileExcludedSystemComponent(); 3209 return this.excludedSystem; 3210 } 3211 else if (name.equals("includeDesignations")) { 3212 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.includeDesignations"); 3213 } 3214 else if (name.equals("designation")) { 3215 this.designation = new ExpansionProfileDesignationComponent(); 3216 return this.designation; 3217 } 3218 else if (name.equals("includeDefinition")) { 3219 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.includeDefinition"); 3220 } 3221 else if (name.equals("activeOnly")) { 3222 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.activeOnly"); 3223 } 3224 else if (name.equals("excludeNested")) { 3225 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.excludeNested"); 3226 } 3227 else if (name.equals("excludeNotForUI")) { 3228 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.excludeNotForUI"); 3229 } 3230 else if (name.equals("excludePostCoordinated")) { 3231 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.excludePostCoordinated"); 3232 } 3233 else if (name.equals("displayLanguage")) { 3234 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.displayLanguage"); 3235 } 3236 else if (name.equals("limitedExpansion")) { 3237 throw new FHIRException("Cannot call addChild on a singleton property ExpansionProfile.limitedExpansion"); 3238 } 3239 else 3240 return super.addChild(name); 3241 } 3242 3243 public String fhirType() { 3244 return "ExpansionProfile"; 3245 3246 } 3247 3248 public ExpansionProfile copy() { 3249 ExpansionProfile dst = new ExpansionProfile(); 3250 copyValues(dst); 3251 dst.url = url == null ? null : url.copy(); 3252 dst.identifier = identifier == null ? null : identifier.copy(); 3253 dst.version = version == null ? null : version.copy(); 3254 dst.name = name == null ? null : name.copy(); 3255 dst.status = status == null ? null : status.copy(); 3256 dst.experimental = experimental == null ? null : experimental.copy(); 3257 dst.date = date == null ? null : date.copy(); 3258 dst.publisher = publisher == null ? null : publisher.copy(); 3259 if (contact != null) { 3260 dst.contact = new ArrayList<ContactDetail>(); 3261 for (ContactDetail i : contact) 3262 dst.contact.add(i.copy()); 3263 }; 3264 dst.description = description == null ? null : description.copy(); 3265 if (useContext != null) { 3266 dst.useContext = new ArrayList<UsageContext>(); 3267 for (UsageContext i : useContext) 3268 dst.useContext.add(i.copy()); 3269 }; 3270 if (jurisdiction != null) { 3271 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3272 for (CodeableConcept i : jurisdiction) 3273 dst.jurisdiction.add(i.copy()); 3274 }; 3275 if (fixedVersion != null) { 3276 dst.fixedVersion = new ArrayList<ExpansionProfileFixedVersionComponent>(); 3277 for (ExpansionProfileFixedVersionComponent i : fixedVersion) 3278 dst.fixedVersion.add(i.copy()); 3279 }; 3280 dst.excludedSystem = excludedSystem == null ? null : excludedSystem.copy(); 3281 dst.includeDesignations = includeDesignations == null ? null : includeDesignations.copy(); 3282 dst.designation = designation == null ? null : designation.copy(); 3283 dst.includeDefinition = includeDefinition == null ? null : includeDefinition.copy(); 3284 dst.activeOnly = activeOnly == null ? null : activeOnly.copy(); 3285 dst.excludeNested = excludeNested == null ? null : excludeNested.copy(); 3286 dst.excludeNotForUI = excludeNotForUI == null ? null : excludeNotForUI.copy(); 3287 dst.excludePostCoordinated = excludePostCoordinated == null ? null : excludePostCoordinated.copy(); 3288 dst.displayLanguage = displayLanguage == null ? null : displayLanguage.copy(); 3289 dst.limitedExpansion = limitedExpansion == null ? null : limitedExpansion.copy(); 3290 return dst; 3291 } 3292 3293 protected ExpansionProfile typedCopy() { 3294 return copy(); 3295 } 3296 3297 @Override 3298 public boolean equalsDeep(Base other_) { 3299 if (!super.equalsDeep(other_)) 3300 return false; 3301 if (!(other_ instanceof ExpansionProfile)) 3302 return false; 3303 ExpansionProfile o = (ExpansionProfile) other_; 3304 return compareDeep(identifier, o.identifier, true) && compareDeep(fixedVersion, o.fixedVersion, true) 3305 && compareDeep(excludedSystem, o.excludedSystem, true) && compareDeep(includeDesignations, o.includeDesignations, true) 3306 && compareDeep(designation, o.designation, true) && compareDeep(includeDefinition, o.includeDefinition, true) 3307 && compareDeep(activeOnly, o.activeOnly, true) && compareDeep(excludeNested, o.excludeNested, true) 3308 && compareDeep(excludeNotForUI, o.excludeNotForUI, true) && compareDeep(excludePostCoordinated, o.excludePostCoordinated, true) 3309 && compareDeep(displayLanguage, o.displayLanguage, true) && compareDeep(limitedExpansion, o.limitedExpansion, true) 3310 ; 3311 } 3312 3313 @Override 3314 public boolean equalsShallow(Base other_) { 3315 if (!super.equalsShallow(other_)) 3316 return false; 3317 if (!(other_ instanceof ExpansionProfile)) 3318 return false; 3319 ExpansionProfile o = (ExpansionProfile) other_; 3320 return compareValues(includeDesignations, o.includeDesignations, true) && compareValues(includeDefinition, o.includeDefinition, true) 3321 && compareValues(activeOnly, o.activeOnly, true) && compareValues(excludeNested, o.excludeNested, true) 3322 && compareValues(excludeNotForUI, o.excludeNotForUI, true) && compareValues(excludePostCoordinated, o.excludePostCoordinated, true) 3323 && compareValues(displayLanguage, o.displayLanguage, true) && compareValues(limitedExpansion, o.limitedExpansion, true) 3324 ; 3325 } 3326 3327 public boolean isEmpty() { 3328 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, fixedVersion, excludedSystem 3329 , includeDesignations, designation, includeDefinition, activeOnly, excludeNested, excludeNotForUI 3330 , excludePostCoordinated, displayLanguage, limitedExpansion); 3331 } 3332 3333 @Override 3334 public ResourceType getResourceType() { 3335 return ResourceType.ExpansionProfile; 3336 } 3337 3338 /** 3339 * Search parameter: <b>date</b> 3340 * <p> 3341 * Description: <b>The expansion profile publication date</b><br> 3342 * Type: <b>date</b><br> 3343 * Path: <b>ExpansionProfile.date</b><br> 3344 * </p> 3345 */ 3346 @SearchParamDefinition(name="date", path="ExpansionProfile.date", description="The expansion profile publication date", type="date" ) 3347 public static final String SP_DATE = "date"; 3348 /** 3349 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3350 * <p> 3351 * Description: <b>The expansion profile publication date</b><br> 3352 * Type: <b>date</b><br> 3353 * Path: <b>ExpansionProfile.date</b><br> 3354 * </p> 3355 */ 3356 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3357 3358 /** 3359 * Search parameter: <b>identifier</b> 3360 * <p> 3361 * Description: <b>External identifier for the expansion profile</b><br> 3362 * Type: <b>token</b><br> 3363 * Path: <b>ExpansionProfile.identifier</b><br> 3364 * </p> 3365 */ 3366 @SearchParamDefinition(name="identifier", path="ExpansionProfile.identifier", description="External identifier for the expansion profile", type="token" ) 3367 public static final String SP_IDENTIFIER = "identifier"; 3368 /** 3369 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3370 * <p> 3371 * Description: <b>External identifier for the expansion profile</b><br> 3372 * Type: <b>token</b><br> 3373 * Path: <b>ExpansionProfile.identifier</b><br> 3374 * </p> 3375 */ 3376 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3377 3378 /** 3379 * Search parameter: <b>jurisdiction</b> 3380 * <p> 3381 * Description: <b>Intended jurisdiction for the expansion profile</b><br> 3382 * Type: <b>token</b><br> 3383 * Path: <b>ExpansionProfile.jurisdiction</b><br> 3384 * </p> 3385 */ 3386 @SearchParamDefinition(name="jurisdiction", path="ExpansionProfile.jurisdiction", description="Intended jurisdiction for the expansion profile", type="token" ) 3387 public static final String SP_JURISDICTION = "jurisdiction"; 3388 /** 3389 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3390 * <p> 3391 * Description: <b>Intended jurisdiction for the expansion profile</b><br> 3392 * Type: <b>token</b><br> 3393 * Path: <b>ExpansionProfile.jurisdiction</b><br> 3394 * </p> 3395 */ 3396 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3397 3398 /** 3399 * Search parameter: <b>name</b> 3400 * <p> 3401 * Description: <b>Computationally friendly name of the expansion profile</b><br> 3402 * Type: <b>string</b><br> 3403 * Path: <b>ExpansionProfile.name</b><br> 3404 * </p> 3405 */ 3406 @SearchParamDefinition(name="name", path="ExpansionProfile.name", description="Computationally friendly name of the expansion profile", type="string" ) 3407 public static final String SP_NAME = "name"; 3408 /** 3409 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3410 * <p> 3411 * Description: <b>Computationally friendly name of the expansion profile</b><br> 3412 * Type: <b>string</b><br> 3413 * Path: <b>ExpansionProfile.name</b><br> 3414 * </p> 3415 */ 3416 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3417 3418 /** 3419 * Search parameter: <b>description</b> 3420 * <p> 3421 * Description: <b>The description of the expansion profile</b><br> 3422 * Type: <b>string</b><br> 3423 * Path: <b>ExpansionProfile.description</b><br> 3424 * </p> 3425 */ 3426 @SearchParamDefinition(name="description", path="ExpansionProfile.description", description="The description of the expansion profile", type="string" ) 3427 public static final String SP_DESCRIPTION = "description"; 3428 /** 3429 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3430 * <p> 3431 * Description: <b>The description of the expansion profile</b><br> 3432 * Type: <b>string</b><br> 3433 * Path: <b>ExpansionProfile.description</b><br> 3434 * </p> 3435 */ 3436 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3437 3438 /** 3439 * Search parameter: <b>publisher</b> 3440 * <p> 3441 * Description: <b>Name of the publisher of the expansion profile</b><br> 3442 * Type: <b>string</b><br> 3443 * Path: <b>ExpansionProfile.publisher</b><br> 3444 * </p> 3445 */ 3446 @SearchParamDefinition(name="publisher", path="ExpansionProfile.publisher", description="Name of the publisher of the expansion profile", type="string" ) 3447 public static final String SP_PUBLISHER = "publisher"; 3448 /** 3449 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3450 * <p> 3451 * Description: <b>Name of the publisher of the expansion profile</b><br> 3452 * Type: <b>string</b><br> 3453 * Path: <b>ExpansionProfile.publisher</b><br> 3454 * </p> 3455 */ 3456 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3457 3458 /** 3459 * Search parameter: <b>version</b> 3460 * <p> 3461 * Description: <b>The business version of the expansion profile</b><br> 3462 * Type: <b>token</b><br> 3463 * Path: <b>ExpansionProfile.version</b><br> 3464 * </p> 3465 */ 3466 @SearchParamDefinition(name="version", path="ExpansionProfile.version", description="The business version of the expansion profile", type="token" ) 3467 public static final String SP_VERSION = "version"; 3468 /** 3469 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3470 * <p> 3471 * Description: <b>The business version of the expansion profile</b><br> 3472 * Type: <b>token</b><br> 3473 * Path: <b>ExpansionProfile.version</b><br> 3474 * </p> 3475 */ 3476 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3477 3478 /** 3479 * Search parameter: <b>url</b> 3480 * <p> 3481 * Description: <b>The uri that identifies the expansion profile</b><br> 3482 * Type: <b>uri</b><br> 3483 * Path: <b>ExpansionProfile.url</b><br> 3484 * </p> 3485 */ 3486 @SearchParamDefinition(name="url", path="ExpansionProfile.url", description="The uri that identifies the expansion profile", type="uri" ) 3487 public static final String SP_URL = "url"; 3488 /** 3489 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3490 * <p> 3491 * Description: <b>The uri that identifies the expansion profile</b><br> 3492 * Type: <b>uri</b><br> 3493 * Path: <b>ExpansionProfile.url</b><br> 3494 * </p> 3495 */ 3496 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3497 3498 /** 3499 * Search parameter: <b>status</b> 3500 * <p> 3501 * Description: <b>The current status of the expansion profile</b><br> 3502 * Type: <b>token</b><br> 3503 * Path: <b>ExpansionProfile.status</b><br> 3504 * </p> 3505 */ 3506 @SearchParamDefinition(name="status", path="ExpansionProfile.status", description="The current status of the expansion profile", type="token" ) 3507 public static final String SP_STATUS = "status"; 3508 /** 3509 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3510 * <p> 3511 * Description: <b>The current status of the expansion profile</b><br> 3512 * Type: <b>token</b><br> 3513 * Path: <b>ExpansionProfile.status</b><br> 3514 * </p> 3515 */ 3516 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3517 3518 3519}