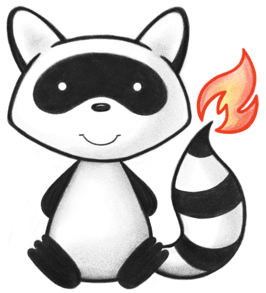
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 051 */ 052@ResourceDef(name="ExplanationOfBenefit", profile="http://hl7.org/fhir/Profile/ExplanationOfBenefit") 053public class ExplanationOfBenefit extends DomainResource { 054 055 public enum ExplanationOfBenefitStatus { 056 /** 057 * The resource instance is currently in-force. 058 */ 059 ACTIVE, 060 /** 061 * The resource instance is withdrawn, rescinded or reversed. 062 */ 063 CANCELLED, 064 /** 065 * A new resource instance the contents of which is not complete. 066 */ 067 DRAFT, 068 /** 069 * The resource instance was entered in error. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static ExplanationOfBenefitStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("cancelled".equals(codeString)) 082 return CANCELLED; 083 if ("draft".equals(codeString)) 084 return DRAFT; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: return "active"; 095 case CANCELLED: return "cancelled"; 096 case DRAFT: return "draft"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case ACTIVE: return "http://hl7.org/fhir/explanationofbenefit-status"; 105 case CANCELLED: return "http://hl7.org/fhir/explanationofbenefit-status"; 106 case DRAFT: return "http://hl7.org/fhir/explanationofbenefit-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/explanationofbenefit-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case ACTIVE: return "The resource instance is currently in-force."; 115 case CANCELLED: return "The resource instance is withdrawn, rescinded or reversed."; 116 case DRAFT: return "A new resource instance the contents of which is not complete."; 117 case ENTEREDINERROR: return "The resource instance was entered in error."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case ACTIVE: return "Active"; 125 case CANCELLED: return "Cancelled"; 126 case DRAFT: return "Draft"; 127 case ENTEREDINERROR: return "Entered In Error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class ExplanationOfBenefitStatusEnumFactory implements EnumFactory<ExplanationOfBenefitStatus> { 135 public ExplanationOfBenefitStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("active".equals(codeString)) 140 return ExplanationOfBenefitStatus.ACTIVE; 141 if ("cancelled".equals(codeString)) 142 return ExplanationOfBenefitStatus.CANCELLED; 143 if ("draft".equals(codeString)) 144 return ExplanationOfBenefitStatus.DRAFT; 145 if ("entered-in-error".equals(codeString)) 146 return ExplanationOfBenefitStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 148 } 149 public Enumeration<ExplanationOfBenefitStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<ExplanationOfBenefitStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("active".equals(codeString)) 158 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ACTIVE); 159 if ("cancelled".equals(codeString)) 160 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.CANCELLED); 161 if ("draft".equals(codeString)) 162 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.DRAFT); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 166 } 167 public String toCode(ExplanationOfBenefitStatus code) { 168 if (code == ExplanationOfBenefitStatus.NULL) 169 return null; 170 if (code == ExplanationOfBenefitStatus.ACTIVE) 171 return "active"; 172 if (code == ExplanationOfBenefitStatus.CANCELLED) 173 return "cancelled"; 174 if (code == ExplanationOfBenefitStatus.DRAFT) 175 return "draft"; 176 if (code == ExplanationOfBenefitStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 return "?"; 179 } 180 public String toSystem(ExplanationOfBenefitStatus code) { 181 return code.getSystem(); 182 } 183 } 184 185 @Block() 186 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 187 /** 188 * Other claims which are related to this claim such as prior claim versions or for related services. 189 */ 190 @Child(name = "claim", type = {Claim.class}, order=1, min=0, max=1, modifier=false, summary=false) 191 @Description(shortDefinition="Reference to the related claim", formalDefinition="Other claims which are related to this claim such as prior claim versions or for related services." ) 192 protected Reference claim; 193 194 /** 195 * The actual object that is the target of the reference (Other claims which are related to this claim such as prior claim versions or for related services.) 196 */ 197 protected Claim claimTarget; 198 199 /** 200 * For example prior or umbrella. 201 */ 202 @Child(name = "relationship", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 203 @Description(shortDefinition="How the reference claim is related", formalDefinition="For example prior or umbrella." ) 204 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-claim-relationship") 205 protected CodeableConcept relationship; 206 207 /** 208 * An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # . 209 */ 210 @Child(name = "reference", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 211 @Description(shortDefinition="Related file or case reference", formalDefinition="An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # ." ) 212 protected Identifier reference; 213 214 private static final long serialVersionUID = -379338905L; 215 216 /** 217 * Constructor 218 */ 219 public RelatedClaimComponent() { 220 super(); 221 } 222 223 /** 224 * @return {@link #claim} (Other claims which are related to this claim such as prior claim versions or for related services.) 225 */ 226 public Reference getClaim() { 227 if (this.claim == null) 228 if (Configuration.errorOnAutoCreate()) 229 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 230 else if (Configuration.doAutoCreate()) 231 this.claim = new Reference(); // cc 232 return this.claim; 233 } 234 235 public boolean hasClaim() { 236 return this.claim != null && !this.claim.isEmpty(); 237 } 238 239 /** 240 * @param value {@link #claim} (Other claims which are related to this claim such as prior claim versions or for related services.) 241 */ 242 public RelatedClaimComponent setClaim(Reference value) { 243 this.claim = value; 244 return this; 245 } 246 247 /** 248 * @return {@link #claim} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Other claims which are related to this claim such as prior claim versions or for related services.) 249 */ 250 public Claim getClaimTarget() { 251 if (this.claimTarget == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 254 else if (Configuration.doAutoCreate()) 255 this.claimTarget = new Claim(); // aa 256 return this.claimTarget; 257 } 258 259 /** 260 * @param value {@link #claim} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Other claims which are related to this claim such as prior claim versions or for related services.) 261 */ 262 public RelatedClaimComponent setClaimTarget(Claim value) { 263 this.claimTarget = value; 264 return this; 265 } 266 267 /** 268 * @return {@link #relationship} (For example prior or umbrella.) 269 */ 270 public CodeableConcept getRelationship() { 271 if (this.relationship == null) 272 if (Configuration.errorOnAutoCreate()) 273 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 274 else if (Configuration.doAutoCreate()) 275 this.relationship = new CodeableConcept(); // cc 276 return this.relationship; 277 } 278 279 public boolean hasRelationship() { 280 return this.relationship != null && !this.relationship.isEmpty(); 281 } 282 283 /** 284 * @param value {@link #relationship} (For example prior or umbrella.) 285 */ 286 public RelatedClaimComponent setRelationship(CodeableConcept value) { 287 this.relationship = value; 288 return this; 289 } 290 291 /** 292 * @return {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .) 293 */ 294 public Identifier getReference() { 295 if (this.reference == null) 296 if (Configuration.errorOnAutoCreate()) 297 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 298 else if (Configuration.doAutoCreate()) 299 this.reference = new Identifier(); // cc 300 return this.reference; 301 } 302 303 public boolean hasReference() { 304 return this.reference != null && !this.reference.isEmpty(); 305 } 306 307 /** 308 * @param value {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .) 309 */ 310 public RelatedClaimComponent setReference(Identifier value) { 311 this.reference = value; 312 return this; 313 } 314 315 protected void listChildren(List<Property> children) { 316 super.listChildren(children); 317 children.add(new Property("claim", "Reference(Claim)", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, 1, claim)); 318 children.add(new Property("relationship", "CodeableConcept", "For example prior or umbrella.", 0, 1, relationship)); 319 children.add(new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .", 0, 1, reference)); 320 } 321 322 @Override 323 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 324 switch (_hash) { 325 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, 1, claim); 326 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "For example prior or umbrella.", 0, 1, relationship); 327 case -925155509: /*reference*/ return new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .", 0, 1, reference); 328 default: return super.getNamedProperty(_hash, _name, _checkValid); 329 } 330 331 } 332 333 @Override 334 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 335 switch (hash) { 336 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 337 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 338 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Identifier 339 default: return super.getProperty(hash, name, checkValid); 340 } 341 342 } 343 344 @Override 345 public Base setProperty(int hash, String name, Base value) throws FHIRException { 346 switch (hash) { 347 case 94742588: // claim 348 this.claim = castToReference(value); // Reference 349 return value; 350 case -261851592: // relationship 351 this.relationship = castToCodeableConcept(value); // CodeableConcept 352 return value; 353 case -925155509: // reference 354 this.reference = castToIdentifier(value); // Identifier 355 return value; 356 default: return super.setProperty(hash, name, value); 357 } 358 359 } 360 361 @Override 362 public Base setProperty(String name, Base value) throws FHIRException { 363 if (name.equals("claim")) { 364 this.claim = castToReference(value); // Reference 365 } else if (name.equals("relationship")) { 366 this.relationship = castToCodeableConcept(value); // CodeableConcept 367 } else if (name.equals("reference")) { 368 this.reference = castToIdentifier(value); // Identifier 369 } else 370 return super.setProperty(name, value); 371 return value; 372 } 373 374 @Override 375 public Base makeProperty(int hash, String name) throws FHIRException { 376 switch (hash) { 377 case 94742588: return getClaim(); 378 case -261851592: return getRelationship(); 379 case -925155509: return getReference(); 380 default: return super.makeProperty(hash, name); 381 } 382 383 } 384 385 @Override 386 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 387 switch (hash) { 388 case 94742588: /*claim*/ return new String[] {"Reference"}; 389 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 390 case -925155509: /*reference*/ return new String[] {"Identifier"}; 391 default: return super.getTypesForProperty(hash, name); 392 } 393 394 } 395 396 @Override 397 public Base addChild(String name) throws FHIRException { 398 if (name.equals("claim")) { 399 this.claim = new Reference(); 400 return this.claim; 401 } 402 else if (name.equals("relationship")) { 403 this.relationship = new CodeableConcept(); 404 return this.relationship; 405 } 406 else if (name.equals("reference")) { 407 this.reference = new Identifier(); 408 return this.reference; 409 } 410 else 411 return super.addChild(name); 412 } 413 414 public RelatedClaimComponent copy() { 415 RelatedClaimComponent dst = new RelatedClaimComponent(); 416 copyValues(dst); 417 dst.claim = claim == null ? null : claim.copy(); 418 dst.relationship = relationship == null ? null : relationship.copy(); 419 dst.reference = reference == null ? null : reference.copy(); 420 return dst; 421 } 422 423 @Override 424 public boolean equalsDeep(Base other_) { 425 if (!super.equalsDeep(other_)) 426 return false; 427 if (!(other_ instanceof RelatedClaimComponent)) 428 return false; 429 RelatedClaimComponent o = (RelatedClaimComponent) other_; 430 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) && compareDeep(reference, o.reference, true) 431 ; 432 } 433 434 @Override 435 public boolean equalsShallow(Base other_) { 436 if (!super.equalsShallow(other_)) 437 return false; 438 if (!(other_ instanceof RelatedClaimComponent)) 439 return false; 440 RelatedClaimComponent o = (RelatedClaimComponent) other_; 441 return true; 442 } 443 444 public boolean isEmpty() { 445 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference 446 ); 447 } 448 449 public String fhirType() { 450 return "ExplanationOfBenefit.related"; 451 452 } 453 454 } 455 456 @Block() 457 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 458 /** 459 * Type of Party to be reimbursed: Subscriber, provider, other. 460 */ 461 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 462 @Description(shortDefinition="Type of party: Subscriber, Provider, other", formalDefinition="Type of Party to be reimbursed: Subscriber, provider, other." ) 463 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 464 protected CodeableConcept type; 465 466 /** 467 * organization | patient | practitioner | relatedperson. 468 */ 469 @Child(name = "resourceType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 470 @Description(shortDefinition="organization | patient | practitioner | relatedperson", formalDefinition="organization | patient | practitioner | relatedperson." ) 471 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-type-link") 472 protected CodeableConcept resourceType; 473 474 /** 475 * Party to be reimbursed: Subscriber, provider, other. 476 */ 477 @Child(name = "party", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=false) 478 @Description(shortDefinition="Party to receive the payable", formalDefinition="Party to be reimbursed: Subscriber, provider, other." ) 479 protected Reference party; 480 481 /** 482 * The actual object that is the target of the reference (Party to be reimbursed: Subscriber, provider, other.) 483 */ 484 protected Resource partyTarget; 485 486 private static final long serialVersionUID = 1146157718L; 487 488 /** 489 * Constructor 490 */ 491 public PayeeComponent() { 492 super(); 493 } 494 495 /** 496 * @return {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 497 */ 498 public CodeableConcept getType() { 499 if (this.type == null) 500 if (Configuration.errorOnAutoCreate()) 501 throw new Error("Attempt to auto-create PayeeComponent.type"); 502 else if (Configuration.doAutoCreate()) 503 this.type = new CodeableConcept(); // cc 504 return this.type; 505 } 506 507 public boolean hasType() { 508 return this.type != null && !this.type.isEmpty(); 509 } 510 511 /** 512 * @param value {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 513 */ 514 public PayeeComponent setType(CodeableConcept value) { 515 this.type = value; 516 return this; 517 } 518 519 /** 520 * @return {@link #resourceType} (organization | patient | practitioner | relatedperson.) 521 */ 522 public CodeableConcept getResourceType() { 523 if (this.resourceType == null) 524 if (Configuration.errorOnAutoCreate()) 525 throw new Error("Attempt to auto-create PayeeComponent.resourceType"); 526 else if (Configuration.doAutoCreate()) 527 this.resourceType = new CodeableConcept(); // cc 528 return this.resourceType; 529 } 530 531 public boolean hasResourceType() { 532 return this.resourceType != null && !this.resourceType.isEmpty(); 533 } 534 535 /** 536 * @param value {@link #resourceType} (organization | patient | practitioner | relatedperson.) 537 */ 538 public PayeeComponent setResourceType(CodeableConcept value) { 539 this.resourceType = value; 540 return this; 541 } 542 543 /** 544 * @return {@link #party} (Party to be reimbursed: Subscriber, provider, other.) 545 */ 546 public Reference getParty() { 547 if (this.party == null) 548 if (Configuration.errorOnAutoCreate()) 549 throw new Error("Attempt to auto-create PayeeComponent.party"); 550 else if (Configuration.doAutoCreate()) 551 this.party = new Reference(); // cc 552 return this.party; 553 } 554 555 public boolean hasParty() { 556 return this.party != null && !this.party.isEmpty(); 557 } 558 559 /** 560 * @param value {@link #party} (Party to be reimbursed: Subscriber, provider, other.) 561 */ 562 public PayeeComponent setParty(Reference value) { 563 this.party = value; 564 return this; 565 } 566 567 /** 568 * @return {@link #party} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Party to be reimbursed: Subscriber, provider, other.) 569 */ 570 public Resource getPartyTarget() { 571 return this.partyTarget; 572 } 573 574 /** 575 * @param value {@link #party} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Party to be reimbursed: Subscriber, provider, other.) 576 */ 577 public PayeeComponent setPartyTarget(Resource value) { 578 this.partyTarget = value; 579 return this; 580 } 581 582 protected void listChildren(List<Property> children) { 583 super.listChildren(children); 584 children.add(new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type)); 585 children.add(new Property("resourceType", "CodeableConcept", "organization | patient | practitioner | relatedperson.", 0, 1, resourceType)); 586 children.add(new Property("party", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, party)); 587 } 588 589 @Override 590 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 591 switch (_hash) { 592 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type); 593 case -384364440: /*resourceType*/ return new Property("resourceType", "CodeableConcept", "organization | patient | practitioner | relatedperson.", 0, 1, resourceType); 594 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, party); 595 default: return super.getNamedProperty(_hash, _name, _checkValid); 596 } 597 598 } 599 600 @Override 601 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 602 switch (hash) { 603 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 604 case -384364440: /*resourceType*/ return this.resourceType == null ? new Base[0] : new Base[] {this.resourceType}; // CodeableConcept 605 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 606 default: return super.getProperty(hash, name, checkValid); 607 } 608 609 } 610 611 @Override 612 public Base setProperty(int hash, String name, Base value) throws FHIRException { 613 switch (hash) { 614 case 3575610: // type 615 this.type = castToCodeableConcept(value); // CodeableConcept 616 return value; 617 case -384364440: // resourceType 618 this.resourceType = castToCodeableConcept(value); // CodeableConcept 619 return value; 620 case 106437350: // party 621 this.party = castToReference(value); // Reference 622 return value; 623 default: return super.setProperty(hash, name, value); 624 } 625 626 } 627 628 @Override 629 public Base setProperty(String name, Base value) throws FHIRException { 630 if (name.equals("type")) { 631 this.type = castToCodeableConcept(value); // CodeableConcept 632 } else if (name.equals("resourceType")) { 633 this.resourceType = castToCodeableConcept(value); // CodeableConcept 634 } else if (name.equals("party")) { 635 this.party = castToReference(value); // Reference 636 } else 637 return super.setProperty(name, value); 638 return value; 639 } 640 641 @Override 642 public Base makeProperty(int hash, String name) throws FHIRException { 643 switch (hash) { 644 case 3575610: return getType(); 645 case -384364440: return getResourceType(); 646 case 106437350: return getParty(); 647 default: return super.makeProperty(hash, name); 648 } 649 650 } 651 652 @Override 653 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 654 switch (hash) { 655 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 656 case -384364440: /*resourceType*/ return new String[] {"CodeableConcept"}; 657 case 106437350: /*party*/ return new String[] {"Reference"}; 658 default: return super.getTypesForProperty(hash, name); 659 } 660 661 } 662 663 @Override 664 public Base addChild(String name) throws FHIRException { 665 if (name.equals("type")) { 666 this.type = new CodeableConcept(); 667 return this.type; 668 } 669 else if (name.equals("resourceType")) { 670 this.resourceType = new CodeableConcept(); 671 return this.resourceType; 672 } 673 else if (name.equals("party")) { 674 this.party = new Reference(); 675 return this.party; 676 } 677 else 678 return super.addChild(name); 679 } 680 681 public PayeeComponent copy() { 682 PayeeComponent dst = new PayeeComponent(); 683 copyValues(dst); 684 dst.type = type == null ? null : type.copy(); 685 dst.resourceType = resourceType == null ? null : resourceType.copy(); 686 dst.party = party == null ? null : party.copy(); 687 return dst; 688 } 689 690 @Override 691 public boolean equalsDeep(Base other_) { 692 if (!super.equalsDeep(other_)) 693 return false; 694 if (!(other_ instanceof PayeeComponent)) 695 return false; 696 PayeeComponent o = (PayeeComponent) other_; 697 return compareDeep(type, o.type, true) && compareDeep(resourceType, o.resourceType, true) && compareDeep(party, o.party, true) 698 ; 699 } 700 701 @Override 702 public boolean equalsShallow(Base other_) { 703 if (!super.equalsShallow(other_)) 704 return false; 705 if (!(other_ instanceof PayeeComponent)) 706 return false; 707 PayeeComponent o = (PayeeComponent) other_; 708 return true; 709 } 710 711 public boolean isEmpty() { 712 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resourceType, party 713 ); 714 } 715 716 public String fhirType() { 717 return "ExplanationOfBenefit.payee"; 718 719 } 720 721 } 722 723 @Block() 724 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 725 /** 726 * Sequence of the information element which serves to provide a link. 727 */ 728 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 729 @Description(shortDefinition="Information instance identifier", formalDefinition="Sequence of the information element which serves to provide a link." ) 730 protected PositiveIntType sequence; 731 732 /** 733 * The general class of the information supplied: information; exception; accident, employment; onset, etc. 734 */ 735 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 736 @Description(shortDefinition="General class of information", formalDefinition="The general class of the information supplied: information; exception; accident, employment; onset, etc." ) 737 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-informationcategory") 738 protected CodeableConcept category; 739 740 /** 741 * System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication. 742 */ 743 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 744 @Description(shortDefinition="Type of information", formalDefinition="System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication." ) 745 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-exception") 746 protected CodeableConcept code; 747 748 /** 749 * The date when or period to which this information refers. 750 */ 751 @Child(name = "timing", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 752 @Description(shortDefinition="When it occurred", formalDefinition="The date when or period to which this information refers." ) 753 protected Type timing; 754 755 /** 756 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 757 */ 758 @Child(name = "value", type = {StringType.class, Quantity.class, Attachment.class, Reference.class}, order=5, min=0, max=1, modifier=false, summary=false) 759 @Description(shortDefinition="Additional Data or supporting information", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 760 protected Type value; 761 762 /** 763 * For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content. 764 */ 765 @Child(name = "reason", type = {Coding.class}, order=6, min=0, max=1, modifier=false, summary=false) 766 @Description(shortDefinition="Reason associated with the information", formalDefinition="For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content." ) 767 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/missing-tooth-reason") 768 protected Coding reason; 769 770 private static final long serialVersionUID = -410136661L; 771 772 /** 773 * Constructor 774 */ 775 public SupportingInformationComponent() { 776 super(); 777 } 778 779 /** 780 * Constructor 781 */ 782 public SupportingInformationComponent(PositiveIntType sequence, CodeableConcept category) { 783 super(); 784 this.sequence = sequence; 785 this.category = category; 786 } 787 788 /** 789 * @return {@link #sequence} (Sequence of the information element which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 790 */ 791 public PositiveIntType getSequenceElement() { 792 if (this.sequence == null) 793 if (Configuration.errorOnAutoCreate()) 794 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 795 else if (Configuration.doAutoCreate()) 796 this.sequence = new PositiveIntType(); // bb 797 return this.sequence; 798 } 799 800 public boolean hasSequenceElement() { 801 return this.sequence != null && !this.sequence.isEmpty(); 802 } 803 804 public boolean hasSequence() { 805 return this.sequence != null && !this.sequence.isEmpty(); 806 } 807 808 /** 809 * @param value {@link #sequence} (Sequence of the information element which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 810 */ 811 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 812 this.sequence = value; 813 return this; 814 } 815 816 /** 817 * @return Sequence of the information element which serves to provide a link. 818 */ 819 public int getSequence() { 820 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 821 } 822 823 /** 824 * @param value Sequence of the information element which serves to provide a link. 825 */ 826 public SupportingInformationComponent setSequence(int value) { 827 if (this.sequence == null) 828 this.sequence = new PositiveIntType(); 829 this.sequence.setValue(value); 830 return this; 831 } 832 833 /** 834 * @return {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 835 */ 836 public CodeableConcept getCategory() { 837 if (this.category == null) 838 if (Configuration.errorOnAutoCreate()) 839 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 840 else if (Configuration.doAutoCreate()) 841 this.category = new CodeableConcept(); // cc 842 return this.category; 843 } 844 845 public boolean hasCategory() { 846 return this.category != null && !this.category.isEmpty(); 847 } 848 849 /** 850 * @param value {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 851 */ 852 public SupportingInformationComponent setCategory(CodeableConcept value) { 853 this.category = value; 854 return this; 855 } 856 857 /** 858 * @return {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.) 859 */ 860 public CodeableConcept getCode() { 861 if (this.code == null) 862 if (Configuration.errorOnAutoCreate()) 863 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 864 else if (Configuration.doAutoCreate()) 865 this.code = new CodeableConcept(); // cc 866 return this.code; 867 } 868 869 public boolean hasCode() { 870 return this.code != null && !this.code.isEmpty(); 871 } 872 873 /** 874 * @param value {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.) 875 */ 876 public SupportingInformationComponent setCode(CodeableConcept value) { 877 this.code = value; 878 return this; 879 } 880 881 /** 882 * @return {@link #timing} (The date when or period to which this information refers.) 883 */ 884 public Type getTiming() { 885 return this.timing; 886 } 887 888 /** 889 * @return {@link #timing} (The date when or period to which this information refers.) 890 */ 891 public DateType getTimingDateType() throws FHIRException { 892 if (this.timing == null) 893 return null; 894 if (!(this.timing instanceof DateType)) 895 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 896 return (DateType) this.timing; 897 } 898 899 public boolean hasTimingDateType() { 900 return this != null && this.timing instanceof DateType; 901 } 902 903 /** 904 * @return {@link #timing} (The date when or period to which this information refers.) 905 */ 906 public Period getTimingPeriod() throws FHIRException { 907 if (this.timing == null) 908 return null; 909 if (!(this.timing instanceof Period)) 910 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 911 return (Period) this.timing; 912 } 913 914 public boolean hasTimingPeriod() { 915 return this != null && this.timing instanceof Period; 916 } 917 918 public boolean hasTiming() { 919 return this.timing != null && !this.timing.isEmpty(); 920 } 921 922 /** 923 * @param value {@link #timing} (The date when or period to which this information refers.) 924 */ 925 public SupportingInformationComponent setTiming(Type value) throws FHIRFormatError { 926 if (value != null && !(value instanceof DateType || value instanceof Period)) 927 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.information.timing[x]: "+value.fhirType()); 928 this.timing = value; 929 return this; 930 } 931 932 /** 933 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 934 */ 935 public Type getValue() { 936 return this.value; 937 } 938 939 /** 940 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 941 */ 942 public StringType getValueStringType() throws FHIRException { 943 if (this.value == null) 944 return null; 945 if (!(this.value instanceof StringType)) 946 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 947 return (StringType) this.value; 948 } 949 950 public boolean hasValueStringType() { 951 return this != null && this.value instanceof StringType; 952 } 953 954 /** 955 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 956 */ 957 public Quantity getValueQuantity() throws FHIRException { 958 if (this.value == null) 959 return null; 960 if (!(this.value instanceof Quantity)) 961 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 962 return (Quantity) this.value; 963 } 964 965 public boolean hasValueQuantity() { 966 return this != null && this.value instanceof Quantity; 967 } 968 969 /** 970 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 971 */ 972 public Attachment getValueAttachment() throws FHIRException { 973 if (this.value == null) 974 return null; 975 if (!(this.value instanceof Attachment)) 976 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 977 return (Attachment) this.value; 978 } 979 980 public boolean hasValueAttachment() { 981 return this != null && this.value instanceof Attachment; 982 } 983 984 /** 985 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 986 */ 987 public Reference getValueReference() throws FHIRException { 988 if (this.value == null) 989 return null; 990 if (!(this.value instanceof Reference)) 991 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 992 return (Reference) this.value; 993 } 994 995 public boolean hasValueReference() { 996 return this != null && this.value instanceof Reference; 997 } 998 999 public boolean hasValue() { 1000 return this.value != null && !this.value.isEmpty(); 1001 } 1002 1003 /** 1004 * @param value {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1005 */ 1006 public SupportingInformationComponent setValue(Type value) throws FHIRFormatError { 1007 if (value != null && !(value instanceof StringType || value instanceof Quantity || value instanceof Attachment || value instanceof Reference)) 1008 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.information.value[x]: "+value.fhirType()); 1009 this.value = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return {@link #reason} (For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.) 1015 */ 1016 public Coding getReason() { 1017 if (this.reason == null) 1018 if (Configuration.errorOnAutoCreate()) 1019 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1020 else if (Configuration.doAutoCreate()) 1021 this.reason = new Coding(); // cc 1022 return this.reason; 1023 } 1024 1025 public boolean hasReason() { 1026 return this.reason != null && !this.reason.isEmpty(); 1027 } 1028 1029 /** 1030 * @param value {@link #reason} (For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.) 1031 */ 1032 public SupportingInformationComponent setReason(Coding value) { 1033 this.reason = value; 1034 return this; 1035 } 1036 1037 protected void listChildren(List<Property> children) { 1038 super.listChildren(children); 1039 children.add(new Property("sequence", "positiveInt", "Sequence of the information element which serves to provide a link.", 0, 1, sequence)); 1040 children.add(new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category)); 1041 children.add(new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.", 0, 1, code)); 1042 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing)); 1043 children.add(new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value)); 1044 children.add(new Property("reason", "Coding", "For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.", 0, 1, reason)); 1045 } 1046 1047 @Override 1048 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1049 switch (_hash) { 1050 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of the information element which serves to provide a link.", 0, 1, sequence); 1051 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category); 1052 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.", 0, 1, code); 1053 case 164632566: /*timing[x]*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1054 case -873664438: /*timing*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1055 case 807935768: /*timingDate*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1056 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1057 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1058 case 111972721: /*value*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1059 case -1424603934: /*valueString*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1060 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1061 case -475566732: /*valueAttachment*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1062 case 1755241690: /*valueReference*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1063 case -934964668: /*reason*/ return new Property("reason", "Coding", "For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.", 0, 1, reason); 1064 default: return super.getNamedProperty(_hash, _name, _checkValid); 1065 } 1066 1067 } 1068 1069 @Override 1070 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1071 switch (hash) { 1072 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1073 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1074 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1075 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 1076 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 1077 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Coding 1078 default: return super.getProperty(hash, name, checkValid); 1079 } 1080 1081 } 1082 1083 @Override 1084 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1085 switch (hash) { 1086 case 1349547969: // sequence 1087 this.sequence = castToPositiveInt(value); // PositiveIntType 1088 return value; 1089 case 50511102: // category 1090 this.category = castToCodeableConcept(value); // CodeableConcept 1091 return value; 1092 case 3059181: // code 1093 this.code = castToCodeableConcept(value); // CodeableConcept 1094 return value; 1095 case -873664438: // timing 1096 this.timing = castToType(value); // Type 1097 return value; 1098 case 111972721: // value 1099 this.value = castToType(value); // Type 1100 return value; 1101 case -934964668: // reason 1102 this.reason = castToCoding(value); // Coding 1103 return value; 1104 default: return super.setProperty(hash, name, value); 1105 } 1106 1107 } 1108 1109 @Override 1110 public Base setProperty(String name, Base value) throws FHIRException { 1111 if (name.equals("sequence")) { 1112 this.sequence = castToPositiveInt(value); // PositiveIntType 1113 } else if (name.equals("category")) { 1114 this.category = castToCodeableConcept(value); // CodeableConcept 1115 } else if (name.equals("code")) { 1116 this.code = castToCodeableConcept(value); // CodeableConcept 1117 } else if (name.equals("timing[x]")) { 1118 this.timing = castToType(value); // Type 1119 } else if (name.equals("value[x]")) { 1120 this.value = castToType(value); // Type 1121 } else if (name.equals("reason")) { 1122 this.reason = castToCoding(value); // Coding 1123 } else 1124 return super.setProperty(name, value); 1125 return value; 1126 } 1127 1128 @Override 1129 public Base makeProperty(int hash, String name) throws FHIRException { 1130 switch (hash) { 1131 case 1349547969: return getSequenceElement(); 1132 case 50511102: return getCategory(); 1133 case 3059181: return getCode(); 1134 case 164632566: return getTiming(); 1135 case -873664438: return getTiming(); 1136 case -1410166417: return getValue(); 1137 case 111972721: return getValue(); 1138 case -934964668: return getReason(); 1139 default: return super.makeProperty(hash, name); 1140 } 1141 1142 } 1143 1144 @Override 1145 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1146 switch (hash) { 1147 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1148 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1149 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1150 case -873664438: /*timing*/ return new String[] {"date", "Period"}; 1151 case 111972721: /*value*/ return new String[] {"string", "Quantity", "Attachment", "Reference"}; 1152 case -934964668: /*reason*/ return new String[] {"Coding"}; 1153 default: return super.getTypesForProperty(hash, name); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base addChild(String name) throws FHIRException { 1160 if (name.equals("sequence")) { 1161 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 1162 } 1163 else if (name.equals("category")) { 1164 this.category = new CodeableConcept(); 1165 return this.category; 1166 } 1167 else if (name.equals("code")) { 1168 this.code = new CodeableConcept(); 1169 return this.code; 1170 } 1171 else if (name.equals("timingDate")) { 1172 this.timing = new DateType(); 1173 return this.timing; 1174 } 1175 else if (name.equals("timingPeriod")) { 1176 this.timing = new Period(); 1177 return this.timing; 1178 } 1179 else if (name.equals("valueString")) { 1180 this.value = new StringType(); 1181 return this.value; 1182 } 1183 else if (name.equals("valueQuantity")) { 1184 this.value = new Quantity(); 1185 return this.value; 1186 } 1187 else if (name.equals("valueAttachment")) { 1188 this.value = new Attachment(); 1189 return this.value; 1190 } 1191 else if (name.equals("valueReference")) { 1192 this.value = new Reference(); 1193 return this.value; 1194 } 1195 else if (name.equals("reason")) { 1196 this.reason = new Coding(); 1197 return this.reason; 1198 } 1199 else 1200 return super.addChild(name); 1201 } 1202 1203 public SupportingInformationComponent copy() { 1204 SupportingInformationComponent dst = new SupportingInformationComponent(); 1205 copyValues(dst); 1206 dst.sequence = sequence == null ? null : sequence.copy(); 1207 dst.category = category == null ? null : category.copy(); 1208 dst.code = code == null ? null : code.copy(); 1209 dst.timing = timing == null ? null : timing.copy(); 1210 dst.value = value == null ? null : value.copy(); 1211 dst.reason = reason == null ? null : reason.copy(); 1212 return dst; 1213 } 1214 1215 @Override 1216 public boolean equalsDeep(Base other_) { 1217 if (!super.equalsDeep(other_)) 1218 return false; 1219 if (!(other_ instanceof SupportingInformationComponent)) 1220 return false; 1221 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1222 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1223 && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) && compareDeep(reason, o.reason, true) 1224 ; 1225 } 1226 1227 @Override 1228 public boolean equalsShallow(Base other_) { 1229 if (!super.equalsShallow(other_)) 1230 return false; 1231 if (!(other_ instanceof SupportingInformationComponent)) 1232 return false; 1233 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1234 return compareValues(sequence, o.sequence, true); 1235 } 1236 1237 public boolean isEmpty() { 1238 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code 1239 , timing, value, reason); 1240 } 1241 1242 public String fhirType() { 1243 return "ExplanationOfBenefit.information"; 1244 1245 } 1246 1247 } 1248 1249 @Block() 1250 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 1251 /** 1252 * Sequence of careteam which serves to order and provide a link. 1253 */ 1254 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1255 @Description(shortDefinition="Number to covey order of careteam", formalDefinition="Sequence of careteam which serves to order and provide a link." ) 1256 protected PositiveIntType sequence; 1257 1258 /** 1259 * The members of the team who provided the overall service. 1260 */ 1261 @Child(name = "provider", type = {Practitioner.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 1262 @Description(shortDefinition="Member of the Care Team", formalDefinition="The members of the team who provided the overall service." ) 1263 protected Reference provider; 1264 1265 /** 1266 * The actual object that is the target of the reference (The members of the team who provided the overall service.) 1267 */ 1268 protected Resource providerTarget; 1269 1270 /** 1271 * The practitioner who is billing and responsible for the claimed services rendered to the patient. 1272 */ 1273 @Child(name = "responsible", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1274 @Description(shortDefinition="Billing practitioner", formalDefinition="The practitioner who is billing and responsible for the claimed services rendered to the patient." ) 1275 protected BooleanType responsible; 1276 1277 /** 1278 * The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team. 1279 */ 1280 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1281 @Description(shortDefinition="Role on the team", formalDefinition="The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team." ) 1282 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-careteamrole") 1283 protected CodeableConcept role; 1284 1285 /** 1286 * The qualification which is applicable for this service. 1287 */ 1288 @Child(name = "qualification", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1289 @Description(shortDefinition="Type, classification or Specialization", formalDefinition="The qualification which is applicable for this service." ) 1290 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provider-qualification") 1291 protected CodeableConcept qualification; 1292 1293 private static final long serialVersionUID = 1758966968L; 1294 1295 /** 1296 * Constructor 1297 */ 1298 public CareTeamComponent() { 1299 super(); 1300 } 1301 1302 /** 1303 * Constructor 1304 */ 1305 public CareTeamComponent(PositiveIntType sequence, Reference provider) { 1306 super(); 1307 this.sequence = sequence; 1308 this.provider = provider; 1309 } 1310 1311 /** 1312 * @return {@link #sequence} (Sequence of careteam which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1313 */ 1314 public PositiveIntType getSequenceElement() { 1315 if (this.sequence == null) 1316 if (Configuration.errorOnAutoCreate()) 1317 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 1318 else if (Configuration.doAutoCreate()) 1319 this.sequence = new PositiveIntType(); // bb 1320 return this.sequence; 1321 } 1322 1323 public boolean hasSequenceElement() { 1324 return this.sequence != null && !this.sequence.isEmpty(); 1325 } 1326 1327 public boolean hasSequence() { 1328 return this.sequence != null && !this.sequence.isEmpty(); 1329 } 1330 1331 /** 1332 * @param value {@link #sequence} (Sequence of careteam which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1333 */ 1334 public CareTeamComponent setSequenceElement(PositiveIntType value) { 1335 this.sequence = value; 1336 return this; 1337 } 1338 1339 /** 1340 * @return Sequence of careteam which serves to order and provide a link. 1341 */ 1342 public int getSequence() { 1343 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1344 } 1345 1346 /** 1347 * @param value Sequence of careteam which serves to order and provide a link. 1348 */ 1349 public CareTeamComponent setSequence(int value) { 1350 if (this.sequence == null) 1351 this.sequence = new PositiveIntType(); 1352 this.sequence.setValue(value); 1353 return this; 1354 } 1355 1356 /** 1357 * @return {@link #provider} (The members of the team who provided the overall service.) 1358 */ 1359 public Reference getProvider() { 1360 if (this.provider == null) 1361 if (Configuration.errorOnAutoCreate()) 1362 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 1363 else if (Configuration.doAutoCreate()) 1364 this.provider = new Reference(); // cc 1365 return this.provider; 1366 } 1367 1368 public boolean hasProvider() { 1369 return this.provider != null && !this.provider.isEmpty(); 1370 } 1371 1372 /** 1373 * @param value {@link #provider} (The members of the team who provided the overall service.) 1374 */ 1375 public CareTeamComponent setProvider(Reference value) { 1376 this.provider = value; 1377 return this; 1378 } 1379 1380 /** 1381 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The members of the team who provided the overall service.) 1382 */ 1383 public Resource getProviderTarget() { 1384 return this.providerTarget; 1385 } 1386 1387 /** 1388 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The members of the team who provided the overall service.) 1389 */ 1390 public CareTeamComponent setProviderTarget(Resource value) { 1391 this.providerTarget = value; 1392 return this; 1393 } 1394 1395 /** 1396 * @return {@link #responsible} (The practitioner who is billing and responsible for the claimed services rendered to the patient.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1397 */ 1398 public BooleanType getResponsibleElement() { 1399 if (this.responsible == null) 1400 if (Configuration.errorOnAutoCreate()) 1401 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1402 else if (Configuration.doAutoCreate()) 1403 this.responsible = new BooleanType(); // bb 1404 return this.responsible; 1405 } 1406 1407 public boolean hasResponsibleElement() { 1408 return this.responsible != null && !this.responsible.isEmpty(); 1409 } 1410 1411 public boolean hasResponsible() { 1412 return this.responsible != null && !this.responsible.isEmpty(); 1413 } 1414 1415 /** 1416 * @param value {@link #responsible} (The practitioner who is billing and responsible for the claimed services rendered to the patient.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1417 */ 1418 public CareTeamComponent setResponsibleElement(BooleanType value) { 1419 this.responsible = value; 1420 return this; 1421 } 1422 1423 /** 1424 * @return The practitioner who is billing and responsible for the claimed services rendered to the patient. 1425 */ 1426 public boolean getResponsible() { 1427 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1428 } 1429 1430 /** 1431 * @param value The practitioner who is billing and responsible for the claimed services rendered to the patient. 1432 */ 1433 public CareTeamComponent setResponsible(boolean value) { 1434 if (this.responsible == null) 1435 this.responsible = new BooleanType(); 1436 this.responsible.setValue(value); 1437 return this; 1438 } 1439 1440 /** 1441 * @return {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.) 1442 */ 1443 public CodeableConcept getRole() { 1444 if (this.role == null) 1445 if (Configuration.errorOnAutoCreate()) 1446 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1447 else if (Configuration.doAutoCreate()) 1448 this.role = new CodeableConcept(); // cc 1449 return this.role; 1450 } 1451 1452 public boolean hasRole() { 1453 return this.role != null && !this.role.isEmpty(); 1454 } 1455 1456 /** 1457 * @param value {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.) 1458 */ 1459 public CareTeamComponent setRole(CodeableConcept value) { 1460 this.role = value; 1461 return this; 1462 } 1463 1464 /** 1465 * @return {@link #qualification} (The qualification which is applicable for this service.) 1466 */ 1467 public CodeableConcept getQualification() { 1468 if (this.qualification == null) 1469 if (Configuration.errorOnAutoCreate()) 1470 throw new Error("Attempt to auto-create CareTeamComponent.qualification"); 1471 else if (Configuration.doAutoCreate()) 1472 this.qualification = new CodeableConcept(); // cc 1473 return this.qualification; 1474 } 1475 1476 public boolean hasQualification() { 1477 return this.qualification != null && !this.qualification.isEmpty(); 1478 } 1479 1480 /** 1481 * @param value {@link #qualification} (The qualification which is applicable for this service.) 1482 */ 1483 public CareTeamComponent setQualification(CodeableConcept value) { 1484 this.qualification = value; 1485 return this; 1486 } 1487 1488 protected void listChildren(List<Property> children) { 1489 super.listChildren(children); 1490 children.add(new Property("sequence", "positiveInt", "Sequence of careteam which serves to order and provide a link.", 0, 1, sequence)); 1491 children.add(new Property("provider", "Reference(Practitioner|Organization)", "The members of the team who provided the overall service.", 0, 1, provider)); 1492 children.add(new Property("responsible", "boolean", "The practitioner who is billing and responsible for the claimed services rendered to the patient.", 0, 1, responsible)); 1493 children.add(new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.", 0, 1, role)); 1494 children.add(new Property("qualification", "CodeableConcept", "The qualification which is applicable for this service.", 0, 1, qualification)); 1495 } 1496 1497 @Override 1498 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1499 switch (_hash) { 1500 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of careteam which serves to order and provide a link.", 0, 1, sequence); 1501 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|Organization)", "The members of the team who provided the overall service.", 0, 1, provider); 1502 case 1847674614: /*responsible*/ return new Property("responsible", "boolean", "The practitioner who is billing and responsible for the claimed services rendered to the patient.", 0, 1, responsible); 1503 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.", 0, 1, role); 1504 case -631333393: /*qualification*/ return new Property("qualification", "CodeableConcept", "The qualification which is applicable for this service.", 0, 1, qualification); 1505 default: return super.getNamedProperty(_hash, _name, _checkValid); 1506 } 1507 1508 } 1509 1510 @Override 1511 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1512 switch (hash) { 1513 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1514 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1515 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // BooleanType 1516 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1517 case -631333393: /*qualification*/ return this.qualification == null ? new Base[0] : new Base[] {this.qualification}; // CodeableConcept 1518 default: return super.getProperty(hash, name, checkValid); 1519 } 1520 1521 } 1522 1523 @Override 1524 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1525 switch (hash) { 1526 case 1349547969: // sequence 1527 this.sequence = castToPositiveInt(value); // PositiveIntType 1528 return value; 1529 case -987494927: // provider 1530 this.provider = castToReference(value); // Reference 1531 return value; 1532 case 1847674614: // responsible 1533 this.responsible = castToBoolean(value); // BooleanType 1534 return value; 1535 case 3506294: // role 1536 this.role = castToCodeableConcept(value); // CodeableConcept 1537 return value; 1538 case -631333393: // qualification 1539 this.qualification = castToCodeableConcept(value); // CodeableConcept 1540 return value; 1541 default: return super.setProperty(hash, name, value); 1542 } 1543 1544 } 1545 1546 @Override 1547 public Base setProperty(String name, Base value) throws FHIRException { 1548 if (name.equals("sequence")) { 1549 this.sequence = castToPositiveInt(value); // PositiveIntType 1550 } else if (name.equals("provider")) { 1551 this.provider = castToReference(value); // Reference 1552 } else if (name.equals("responsible")) { 1553 this.responsible = castToBoolean(value); // BooleanType 1554 } else if (name.equals("role")) { 1555 this.role = castToCodeableConcept(value); // CodeableConcept 1556 } else if (name.equals("qualification")) { 1557 this.qualification = castToCodeableConcept(value); // CodeableConcept 1558 } else 1559 return super.setProperty(name, value); 1560 return value; 1561 } 1562 1563 @Override 1564 public Base makeProperty(int hash, String name) throws FHIRException { 1565 switch (hash) { 1566 case 1349547969: return getSequenceElement(); 1567 case -987494927: return getProvider(); 1568 case 1847674614: return getResponsibleElement(); 1569 case 3506294: return getRole(); 1570 case -631333393: return getQualification(); 1571 default: return super.makeProperty(hash, name); 1572 } 1573 1574 } 1575 1576 @Override 1577 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1578 switch (hash) { 1579 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1580 case -987494927: /*provider*/ return new String[] {"Reference"}; 1581 case 1847674614: /*responsible*/ return new String[] {"boolean"}; 1582 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1583 case -631333393: /*qualification*/ return new String[] {"CodeableConcept"}; 1584 default: return super.getTypesForProperty(hash, name); 1585 } 1586 1587 } 1588 1589 @Override 1590 public Base addChild(String name) throws FHIRException { 1591 if (name.equals("sequence")) { 1592 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 1593 } 1594 else if (name.equals("provider")) { 1595 this.provider = new Reference(); 1596 return this.provider; 1597 } 1598 else if (name.equals("responsible")) { 1599 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.responsible"); 1600 } 1601 else if (name.equals("role")) { 1602 this.role = new CodeableConcept(); 1603 return this.role; 1604 } 1605 else if (name.equals("qualification")) { 1606 this.qualification = new CodeableConcept(); 1607 return this.qualification; 1608 } 1609 else 1610 return super.addChild(name); 1611 } 1612 1613 public CareTeamComponent copy() { 1614 CareTeamComponent dst = new CareTeamComponent(); 1615 copyValues(dst); 1616 dst.sequence = sequence == null ? null : sequence.copy(); 1617 dst.provider = provider == null ? null : provider.copy(); 1618 dst.responsible = responsible == null ? null : responsible.copy(); 1619 dst.role = role == null ? null : role.copy(); 1620 dst.qualification = qualification == null ? null : qualification.copy(); 1621 return dst; 1622 } 1623 1624 @Override 1625 public boolean equalsDeep(Base other_) { 1626 if (!super.equalsDeep(other_)) 1627 return false; 1628 if (!(other_ instanceof CareTeamComponent)) 1629 return false; 1630 CareTeamComponent o = (CareTeamComponent) other_; 1631 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) && compareDeep(responsible, o.responsible, true) 1632 && compareDeep(role, o.role, true) && compareDeep(qualification, o.qualification, true); 1633 } 1634 1635 @Override 1636 public boolean equalsShallow(Base other_) { 1637 if (!super.equalsShallow(other_)) 1638 return false; 1639 if (!(other_ instanceof CareTeamComponent)) 1640 return false; 1641 CareTeamComponent o = (CareTeamComponent) other_; 1642 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true) 1643 ; 1644 } 1645 1646 public boolean isEmpty() { 1647 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible 1648 , role, qualification); 1649 } 1650 1651 public String fhirType() { 1652 return "ExplanationOfBenefit.careTeam"; 1653 1654 } 1655 1656 } 1657 1658 @Block() 1659 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1660 /** 1661 * Sequence of diagnosis which serves to provide a link. 1662 */ 1663 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1664 @Description(shortDefinition="Number to covey order of diagnosis", formalDefinition="Sequence of diagnosis which serves to provide a link." ) 1665 protected PositiveIntType sequence; 1666 1667 /** 1668 * The diagnosis. 1669 */ 1670 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1671 @Description(shortDefinition="Patient's diagnosis", formalDefinition="The diagnosis." ) 1672 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1673 protected Type diagnosis; 1674 1675 /** 1676 * The type of the Diagnosis, for example: admitting, primary, secondary, discharge. 1677 */ 1678 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1679 @Description(shortDefinition="Timing or nature of the diagnosis", formalDefinition="The type of the Diagnosis, for example: admitting, primary, secondary, discharge." ) 1680 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosistype") 1681 protected List<CodeableConcept> type; 1682 1683 /** 1684 * The package billing code, for example DRG, based on the assigned grouping code system. 1685 */ 1686 @Child(name = "packageCode", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1687 @Description(shortDefinition="Package billing code", formalDefinition="The package billing code, for example DRG, based on the assigned grouping code system." ) 1688 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 1689 protected CodeableConcept packageCode; 1690 1691 private static final long serialVersionUID = -350960873L; 1692 1693 /** 1694 * Constructor 1695 */ 1696 public DiagnosisComponent() { 1697 super(); 1698 } 1699 1700 /** 1701 * Constructor 1702 */ 1703 public DiagnosisComponent(PositiveIntType sequence, Type diagnosis) { 1704 super(); 1705 this.sequence = sequence; 1706 this.diagnosis = diagnosis; 1707 } 1708 1709 /** 1710 * @return {@link #sequence} (Sequence of diagnosis which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1711 */ 1712 public PositiveIntType getSequenceElement() { 1713 if (this.sequence == null) 1714 if (Configuration.errorOnAutoCreate()) 1715 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 1716 else if (Configuration.doAutoCreate()) 1717 this.sequence = new PositiveIntType(); // bb 1718 return this.sequence; 1719 } 1720 1721 public boolean hasSequenceElement() { 1722 return this.sequence != null && !this.sequence.isEmpty(); 1723 } 1724 1725 public boolean hasSequence() { 1726 return this.sequence != null && !this.sequence.isEmpty(); 1727 } 1728 1729 /** 1730 * @param value {@link #sequence} (Sequence of diagnosis which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1731 */ 1732 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 1733 this.sequence = value; 1734 return this; 1735 } 1736 1737 /** 1738 * @return Sequence of diagnosis which serves to provide a link. 1739 */ 1740 public int getSequence() { 1741 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1742 } 1743 1744 /** 1745 * @param value Sequence of diagnosis which serves to provide a link. 1746 */ 1747 public DiagnosisComponent setSequence(int value) { 1748 if (this.sequence == null) 1749 this.sequence = new PositiveIntType(); 1750 this.sequence.setValue(value); 1751 return this; 1752 } 1753 1754 /** 1755 * @return {@link #diagnosis} (The diagnosis.) 1756 */ 1757 public Type getDiagnosis() { 1758 return this.diagnosis; 1759 } 1760 1761 /** 1762 * @return {@link #diagnosis} (The diagnosis.) 1763 */ 1764 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 1765 if (this.diagnosis == null) 1766 return null; 1767 if (!(this.diagnosis instanceof CodeableConcept)) 1768 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1769 return (CodeableConcept) this.diagnosis; 1770 } 1771 1772 public boolean hasDiagnosisCodeableConcept() { 1773 return this != null && this.diagnosis instanceof CodeableConcept; 1774 } 1775 1776 /** 1777 * @return {@link #diagnosis} (The diagnosis.) 1778 */ 1779 public Reference getDiagnosisReference() throws FHIRException { 1780 if (this.diagnosis == null) 1781 return null; 1782 if (!(this.diagnosis instanceof Reference)) 1783 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1784 return (Reference) this.diagnosis; 1785 } 1786 1787 public boolean hasDiagnosisReference() { 1788 return this != null && this.diagnosis instanceof Reference; 1789 } 1790 1791 public boolean hasDiagnosis() { 1792 return this.diagnosis != null && !this.diagnosis.isEmpty(); 1793 } 1794 1795 /** 1796 * @param value {@link #diagnosis} (The diagnosis.) 1797 */ 1798 public DiagnosisComponent setDiagnosis(Type value) throws FHIRFormatError { 1799 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1800 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.diagnosis.diagnosis[x]: "+value.fhirType()); 1801 this.diagnosis = value; 1802 return this; 1803 } 1804 1805 /** 1806 * @return {@link #type} (The type of the Diagnosis, for example: admitting, primary, secondary, discharge.) 1807 */ 1808 public List<CodeableConcept> getType() { 1809 if (this.type == null) 1810 this.type = new ArrayList<CodeableConcept>(); 1811 return this.type; 1812 } 1813 1814 /** 1815 * @return Returns a reference to <code>this</code> for easy method chaining 1816 */ 1817 public DiagnosisComponent setType(List<CodeableConcept> theType) { 1818 this.type = theType; 1819 return this; 1820 } 1821 1822 public boolean hasType() { 1823 if (this.type == null) 1824 return false; 1825 for (CodeableConcept item : this.type) 1826 if (!item.isEmpty()) 1827 return true; 1828 return false; 1829 } 1830 1831 public CodeableConcept addType() { //3 1832 CodeableConcept t = new CodeableConcept(); 1833 if (this.type == null) 1834 this.type = new ArrayList<CodeableConcept>(); 1835 this.type.add(t); 1836 return t; 1837 } 1838 1839 public DiagnosisComponent addType(CodeableConcept t) { //3 1840 if (t == null) 1841 return this; 1842 if (this.type == null) 1843 this.type = new ArrayList<CodeableConcept>(); 1844 this.type.add(t); 1845 return this; 1846 } 1847 1848 /** 1849 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 1850 */ 1851 public CodeableConcept getTypeFirstRep() { 1852 if (getType().isEmpty()) { 1853 addType(); 1854 } 1855 return getType().get(0); 1856 } 1857 1858 /** 1859 * @return {@link #packageCode} (The package billing code, for example DRG, based on the assigned grouping code system.) 1860 */ 1861 public CodeableConcept getPackageCode() { 1862 if (this.packageCode == null) 1863 if (Configuration.errorOnAutoCreate()) 1864 throw new Error("Attempt to auto-create DiagnosisComponent.packageCode"); 1865 else if (Configuration.doAutoCreate()) 1866 this.packageCode = new CodeableConcept(); // cc 1867 return this.packageCode; 1868 } 1869 1870 public boolean hasPackageCode() { 1871 return this.packageCode != null && !this.packageCode.isEmpty(); 1872 } 1873 1874 /** 1875 * @param value {@link #packageCode} (The package billing code, for example DRG, based on the assigned grouping code system.) 1876 */ 1877 public DiagnosisComponent setPackageCode(CodeableConcept value) { 1878 this.packageCode = value; 1879 return this; 1880 } 1881 1882 protected void listChildren(List<Property> children) { 1883 super.listChildren(children); 1884 children.add(new Property("sequence", "positiveInt", "Sequence of diagnosis which serves to provide a link.", 0, 1, sequence)); 1885 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis)); 1886 children.add(new Property("type", "CodeableConcept", "The type of the Diagnosis, for example: admitting, primary, secondary, discharge.", 0, java.lang.Integer.MAX_VALUE, type)); 1887 children.add(new Property("packageCode", "CodeableConcept", "The package billing code, for example DRG, based on the assigned grouping code system.", 0, 1, packageCode)); 1888 } 1889 1890 @Override 1891 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1892 switch (_hash) { 1893 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of diagnosis which serves to provide a link.", 0, 1, sequence); 1894 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 1895 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 1896 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 1897 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 1898 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the Diagnosis, for example: admitting, primary, secondary, discharge.", 0, java.lang.Integer.MAX_VALUE, type); 1899 case 908444499: /*packageCode*/ return new Property("packageCode", "CodeableConcept", "The package billing code, for example DRG, based on the assigned grouping code system.", 0, 1, packageCode); 1900 default: return super.getNamedProperty(_hash, _name, _checkValid); 1901 } 1902 1903 } 1904 1905 @Override 1906 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1907 switch (hash) { 1908 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1909 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // Type 1910 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1911 case 908444499: /*packageCode*/ return this.packageCode == null ? new Base[0] : new Base[] {this.packageCode}; // CodeableConcept 1912 default: return super.getProperty(hash, name, checkValid); 1913 } 1914 1915 } 1916 1917 @Override 1918 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1919 switch (hash) { 1920 case 1349547969: // sequence 1921 this.sequence = castToPositiveInt(value); // PositiveIntType 1922 return value; 1923 case 1196993265: // diagnosis 1924 this.diagnosis = castToType(value); // Type 1925 return value; 1926 case 3575610: // type 1927 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1928 return value; 1929 case 908444499: // packageCode 1930 this.packageCode = castToCodeableConcept(value); // CodeableConcept 1931 return value; 1932 default: return super.setProperty(hash, name, value); 1933 } 1934 1935 } 1936 1937 @Override 1938 public Base setProperty(String name, Base value) throws FHIRException { 1939 if (name.equals("sequence")) { 1940 this.sequence = castToPositiveInt(value); // PositiveIntType 1941 } else if (name.equals("diagnosis[x]")) { 1942 this.diagnosis = castToType(value); // Type 1943 } else if (name.equals("type")) { 1944 this.getType().add(castToCodeableConcept(value)); 1945 } else if (name.equals("packageCode")) { 1946 this.packageCode = castToCodeableConcept(value); // CodeableConcept 1947 } else 1948 return super.setProperty(name, value); 1949 return value; 1950 } 1951 1952 @Override 1953 public Base makeProperty(int hash, String name) throws FHIRException { 1954 switch (hash) { 1955 case 1349547969: return getSequenceElement(); 1956 case -1487009809: return getDiagnosis(); 1957 case 1196993265: return getDiagnosis(); 1958 case 3575610: return addType(); 1959 case 908444499: return getPackageCode(); 1960 default: return super.makeProperty(hash, name); 1961 } 1962 1963 } 1964 1965 @Override 1966 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1967 switch (hash) { 1968 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1969 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 1970 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1971 case 908444499: /*packageCode*/ return new String[] {"CodeableConcept"}; 1972 default: return super.getTypesForProperty(hash, name); 1973 } 1974 1975 } 1976 1977 @Override 1978 public Base addChild(String name) throws FHIRException { 1979 if (name.equals("sequence")) { 1980 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 1981 } 1982 else if (name.equals("diagnosisCodeableConcept")) { 1983 this.diagnosis = new CodeableConcept(); 1984 return this.diagnosis; 1985 } 1986 else if (name.equals("diagnosisReference")) { 1987 this.diagnosis = new Reference(); 1988 return this.diagnosis; 1989 } 1990 else if (name.equals("type")) { 1991 return addType(); 1992 } 1993 else if (name.equals("packageCode")) { 1994 this.packageCode = new CodeableConcept(); 1995 return this.packageCode; 1996 } 1997 else 1998 return super.addChild(name); 1999 } 2000 2001 public DiagnosisComponent copy() { 2002 DiagnosisComponent dst = new DiagnosisComponent(); 2003 copyValues(dst); 2004 dst.sequence = sequence == null ? null : sequence.copy(); 2005 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2006 if (type != null) { 2007 dst.type = new ArrayList<CodeableConcept>(); 2008 for (CodeableConcept i : type) 2009 dst.type.add(i.copy()); 2010 }; 2011 dst.packageCode = packageCode == null ? null : packageCode.copy(); 2012 return dst; 2013 } 2014 2015 @Override 2016 public boolean equalsDeep(Base other_) { 2017 if (!super.equalsDeep(other_)) 2018 return false; 2019 if (!(other_ instanceof DiagnosisComponent)) 2020 return false; 2021 DiagnosisComponent o = (DiagnosisComponent) other_; 2022 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(type, o.type, true) 2023 && compareDeep(packageCode, o.packageCode, true); 2024 } 2025 2026 @Override 2027 public boolean equalsShallow(Base other_) { 2028 if (!super.equalsShallow(other_)) 2029 return false; 2030 if (!(other_ instanceof DiagnosisComponent)) 2031 return false; 2032 DiagnosisComponent o = (DiagnosisComponent) other_; 2033 return compareValues(sequence, o.sequence, true); 2034 } 2035 2036 public boolean isEmpty() { 2037 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type 2038 , packageCode); 2039 } 2040 2041 public String fhirType() { 2042 return "ExplanationOfBenefit.diagnosis"; 2043 2044 } 2045 2046 } 2047 2048 @Block() 2049 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2050 /** 2051 * Sequence of procedures which serves to order and provide a link. 2052 */ 2053 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2054 @Description(shortDefinition="Procedure sequence for reference", formalDefinition="Sequence of procedures which serves to order and provide a link." ) 2055 protected PositiveIntType sequence; 2056 2057 /** 2058 * Date and optionally time the procedure was performed . 2059 */ 2060 @Child(name = "date", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2061 @Description(shortDefinition="When the procedure was performed", formalDefinition="Date and optionally time the procedure was performed ." ) 2062 protected DateTimeType date; 2063 2064 /** 2065 * The procedure code. 2066 */ 2067 @Child(name = "procedure", type = {CodeableConcept.class, Procedure.class}, order=3, min=1, max=1, modifier=false, summary=false) 2068 @Description(shortDefinition="Patient's list of procedures performed", formalDefinition="The procedure code." ) 2069 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10-procedures") 2070 protected Type procedure; 2071 2072 private static final long serialVersionUID = 864307347L; 2073 2074 /** 2075 * Constructor 2076 */ 2077 public ProcedureComponent() { 2078 super(); 2079 } 2080 2081 /** 2082 * Constructor 2083 */ 2084 public ProcedureComponent(PositiveIntType sequence, Type procedure) { 2085 super(); 2086 this.sequence = sequence; 2087 this.procedure = procedure; 2088 } 2089 2090 /** 2091 * @return {@link #sequence} (Sequence of procedures which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2092 */ 2093 public PositiveIntType getSequenceElement() { 2094 if (this.sequence == null) 2095 if (Configuration.errorOnAutoCreate()) 2096 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2097 else if (Configuration.doAutoCreate()) 2098 this.sequence = new PositiveIntType(); // bb 2099 return this.sequence; 2100 } 2101 2102 public boolean hasSequenceElement() { 2103 return this.sequence != null && !this.sequence.isEmpty(); 2104 } 2105 2106 public boolean hasSequence() { 2107 return this.sequence != null && !this.sequence.isEmpty(); 2108 } 2109 2110 /** 2111 * @param value {@link #sequence} (Sequence of procedures which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2112 */ 2113 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2114 this.sequence = value; 2115 return this; 2116 } 2117 2118 /** 2119 * @return Sequence of procedures which serves to order and provide a link. 2120 */ 2121 public int getSequence() { 2122 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2123 } 2124 2125 /** 2126 * @param value Sequence of procedures which serves to order and provide a link. 2127 */ 2128 public ProcedureComponent setSequence(int value) { 2129 if (this.sequence == null) 2130 this.sequence = new PositiveIntType(); 2131 this.sequence.setValue(value); 2132 return this; 2133 } 2134 2135 /** 2136 * @return {@link #date} (Date and optionally time the procedure was performed .). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2137 */ 2138 public DateTimeType getDateElement() { 2139 if (this.date == null) 2140 if (Configuration.errorOnAutoCreate()) 2141 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2142 else if (Configuration.doAutoCreate()) 2143 this.date = new DateTimeType(); // bb 2144 return this.date; 2145 } 2146 2147 public boolean hasDateElement() { 2148 return this.date != null && !this.date.isEmpty(); 2149 } 2150 2151 public boolean hasDate() { 2152 return this.date != null && !this.date.isEmpty(); 2153 } 2154 2155 /** 2156 * @param value {@link #date} (Date and optionally time the procedure was performed .). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2157 */ 2158 public ProcedureComponent setDateElement(DateTimeType value) { 2159 this.date = value; 2160 return this; 2161 } 2162 2163 /** 2164 * @return Date and optionally time the procedure was performed . 2165 */ 2166 public Date getDate() { 2167 return this.date == null ? null : this.date.getValue(); 2168 } 2169 2170 /** 2171 * @param value Date and optionally time the procedure was performed . 2172 */ 2173 public ProcedureComponent setDate(Date value) { 2174 if (value == null) 2175 this.date = null; 2176 else { 2177 if (this.date == null) 2178 this.date = new DateTimeType(); 2179 this.date.setValue(value); 2180 } 2181 return this; 2182 } 2183 2184 /** 2185 * @return {@link #procedure} (The procedure code.) 2186 */ 2187 public Type getProcedure() { 2188 return this.procedure; 2189 } 2190 2191 /** 2192 * @return {@link #procedure} (The procedure code.) 2193 */ 2194 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2195 if (this.procedure == null) 2196 return null; 2197 if (!(this.procedure instanceof CodeableConcept)) 2198 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2199 return (CodeableConcept) this.procedure; 2200 } 2201 2202 public boolean hasProcedureCodeableConcept() { 2203 return this != null && this.procedure instanceof CodeableConcept; 2204 } 2205 2206 /** 2207 * @return {@link #procedure} (The procedure code.) 2208 */ 2209 public Reference getProcedureReference() throws FHIRException { 2210 if (this.procedure == null) 2211 return null; 2212 if (!(this.procedure instanceof Reference)) 2213 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2214 return (Reference) this.procedure; 2215 } 2216 2217 public boolean hasProcedureReference() { 2218 return this != null && this.procedure instanceof Reference; 2219 } 2220 2221 public boolean hasProcedure() { 2222 return this.procedure != null && !this.procedure.isEmpty(); 2223 } 2224 2225 /** 2226 * @param value {@link #procedure} (The procedure code.) 2227 */ 2228 public ProcedureComponent setProcedure(Type value) throws FHIRFormatError { 2229 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2230 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.procedure.procedure[x]: "+value.fhirType()); 2231 this.procedure = value; 2232 return this; 2233 } 2234 2235 protected void listChildren(List<Property> children) { 2236 super.listChildren(children); 2237 children.add(new Property("sequence", "positiveInt", "Sequence of procedures which serves to order and provide a link.", 0, 1, sequence)); 2238 children.add(new Property("date", "dateTime", "Date and optionally time the procedure was performed .", 0, 1, date)); 2239 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure)); 2240 } 2241 2242 @Override 2243 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2244 switch (_hash) { 2245 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of procedures which serves to order and provide a link.", 0, 1, sequence); 2246 case 3076014: /*date*/ return new Property("date", "dateTime", "Date and optionally time the procedure was performed .", 0, 1, date); 2247 case 1640074445: /*procedure[x]*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2248 case -1095204141: /*procedure*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2249 case -1284783026: /*procedureCodeableConcept*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2250 case 881809848: /*procedureReference*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2251 default: return super.getNamedProperty(_hash, _name, _checkValid); 2252 } 2253 2254 } 2255 2256 @Override 2257 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2258 switch (hash) { 2259 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2260 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2261 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // Type 2262 default: return super.getProperty(hash, name, checkValid); 2263 } 2264 2265 } 2266 2267 @Override 2268 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2269 switch (hash) { 2270 case 1349547969: // sequence 2271 this.sequence = castToPositiveInt(value); // PositiveIntType 2272 return value; 2273 case 3076014: // date 2274 this.date = castToDateTime(value); // DateTimeType 2275 return value; 2276 case -1095204141: // procedure 2277 this.procedure = castToType(value); // Type 2278 return value; 2279 default: return super.setProperty(hash, name, value); 2280 } 2281 2282 } 2283 2284 @Override 2285 public Base setProperty(String name, Base value) throws FHIRException { 2286 if (name.equals("sequence")) { 2287 this.sequence = castToPositiveInt(value); // PositiveIntType 2288 } else if (name.equals("date")) { 2289 this.date = castToDateTime(value); // DateTimeType 2290 } else if (name.equals("procedure[x]")) { 2291 this.procedure = castToType(value); // Type 2292 } else 2293 return super.setProperty(name, value); 2294 return value; 2295 } 2296 2297 @Override 2298 public Base makeProperty(int hash, String name) throws FHIRException { 2299 switch (hash) { 2300 case 1349547969: return getSequenceElement(); 2301 case 3076014: return getDateElement(); 2302 case 1640074445: return getProcedure(); 2303 case -1095204141: return getProcedure(); 2304 default: return super.makeProperty(hash, name); 2305 } 2306 2307 } 2308 2309 @Override 2310 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2311 switch (hash) { 2312 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2313 case 3076014: /*date*/ return new String[] {"dateTime"}; 2314 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept", "Reference"}; 2315 default: return super.getTypesForProperty(hash, name); 2316 } 2317 2318 } 2319 2320 @Override 2321 public Base addChild(String name) throws FHIRException { 2322 if (name.equals("sequence")) { 2323 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 2324 } 2325 else if (name.equals("date")) { 2326 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 2327 } 2328 else if (name.equals("procedureCodeableConcept")) { 2329 this.procedure = new CodeableConcept(); 2330 return this.procedure; 2331 } 2332 else if (name.equals("procedureReference")) { 2333 this.procedure = new Reference(); 2334 return this.procedure; 2335 } 2336 else 2337 return super.addChild(name); 2338 } 2339 2340 public ProcedureComponent copy() { 2341 ProcedureComponent dst = new ProcedureComponent(); 2342 copyValues(dst); 2343 dst.sequence = sequence == null ? null : sequence.copy(); 2344 dst.date = date == null ? null : date.copy(); 2345 dst.procedure = procedure == null ? null : procedure.copy(); 2346 return dst; 2347 } 2348 2349 @Override 2350 public boolean equalsDeep(Base other_) { 2351 if (!super.equalsDeep(other_)) 2352 return false; 2353 if (!(other_ instanceof ProcedureComponent)) 2354 return false; 2355 ProcedureComponent o = (ProcedureComponent) other_; 2356 return compareDeep(sequence, o.sequence, true) && compareDeep(date, o.date, true) && compareDeep(procedure, o.procedure, true) 2357 ; 2358 } 2359 2360 @Override 2361 public boolean equalsShallow(Base other_) { 2362 if (!super.equalsShallow(other_)) 2363 return false; 2364 if (!(other_ instanceof ProcedureComponent)) 2365 return false; 2366 ProcedureComponent o = (ProcedureComponent) other_; 2367 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 2368 } 2369 2370 public boolean isEmpty() { 2371 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, date, procedure 2372 ); 2373 } 2374 2375 public String fhirType() { 2376 return "ExplanationOfBenefit.procedure"; 2377 2378 } 2379 2380 } 2381 2382 @Block() 2383 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 2384 /** 2385 * Reference to the program or plan identification, underwriter or payor. 2386 */ 2387 @Child(name = "coverage", type = {Coverage.class}, order=1, min=0, max=1, modifier=false, summary=false) 2388 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the program or plan identification, underwriter or payor." ) 2389 protected Reference coverage; 2390 2391 /** 2392 * The actual object that is the target of the reference (Reference to the program or plan identification, underwriter or payor.) 2393 */ 2394 protected Coverage coverageTarget; 2395 2396 /** 2397 * A list of references from the Insurer to which these services pertain. 2398 */ 2399 @Child(name = "preAuthRef", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2400 @Description(shortDefinition="Pre-Authorization/Determination Reference", formalDefinition="A list of references from the Insurer to which these services pertain." ) 2401 protected List<StringType> preAuthRef; 2402 2403 private static final long serialVersionUID = -870298727L; 2404 2405 /** 2406 * Constructor 2407 */ 2408 public InsuranceComponent() { 2409 super(); 2410 } 2411 2412 /** 2413 * @return {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 2414 */ 2415 public Reference getCoverage() { 2416 if (this.coverage == null) 2417 if (Configuration.errorOnAutoCreate()) 2418 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2419 else if (Configuration.doAutoCreate()) 2420 this.coverage = new Reference(); // cc 2421 return this.coverage; 2422 } 2423 2424 public boolean hasCoverage() { 2425 return this.coverage != null && !this.coverage.isEmpty(); 2426 } 2427 2428 /** 2429 * @param value {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 2430 */ 2431 public InsuranceComponent setCoverage(Reference value) { 2432 this.coverage = value; 2433 return this; 2434 } 2435 2436 /** 2437 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 2438 */ 2439 public Coverage getCoverageTarget() { 2440 if (this.coverageTarget == null) 2441 if (Configuration.errorOnAutoCreate()) 2442 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2443 else if (Configuration.doAutoCreate()) 2444 this.coverageTarget = new Coverage(); // aa 2445 return this.coverageTarget; 2446 } 2447 2448 /** 2449 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 2450 */ 2451 public InsuranceComponent setCoverageTarget(Coverage value) { 2452 this.coverageTarget = value; 2453 return this; 2454 } 2455 2456 /** 2457 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2458 */ 2459 public List<StringType> getPreAuthRef() { 2460 if (this.preAuthRef == null) 2461 this.preAuthRef = new ArrayList<StringType>(); 2462 return this.preAuthRef; 2463 } 2464 2465 /** 2466 * @return Returns a reference to <code>this</code> for easy method chaining 2467 */ 2468 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 2469 this.preAuthRef = thePreAuthRef; 2470 return this; 2471 } 2472 2473 public boolean hasPreAuthRef() { 2474 if (this.preAuthRef == null) 2475 return false; 2476 for (StringType item : this.preAuthRef) 2477 if (!item.isEmpty()) 2478 return true; 2479 return false; 2480 } 2481 2482 /** 2483 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2484 */ 2485 public StringType addPreAuthRefElement() {//2 2486 StringType t = new StringType(); 2487 if (this.preAuthRef == null) 2488 this.preAuthRef = new ArrayList<StringType>(); 2489 this.preAuthRef.add(t); 2490 return t; 2491 } 2492 2493 /** 2494 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2495 */ 2496 public InsuranceComponent addPreAuthRef(String value) { //1 2497 StringType t = new StringType(); 2498 t.setValue(value); 2499 if (this.preAuthRef == null) 2500 this.preAuthRef = new ArrayList<StringType>(); 2501 this.preAuthRef.add(t); 2502 return this; 2503 } 2504 2505 /** 2506 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2507 */ 2508 public boolean hasPreAuthRef(String value) { 2509 if (this.preAuthRef == null) 2510 return false; 2511 for (StringType v : this.preAuthRef) 2512 if (v.getValue().equals(value)) // string 2513 return true; 2514 return false; 2515 } 2516 2517 protected void listChildren(List<Property> children) { 2518 super.listChildren(children); 2519 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage)); 2520 children.add(new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 2521 } 2522 2523 @Override 2524 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2525 switch (_hash) { 2526 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage); 2527 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 2528 default: return super.getNamedProperty(_hash, _name, _checkValid); 2529 } 2530 2531 } 2532 2533 @Override 2534 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2535 switch (hash) { 2536 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 2537 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 2538 default: return super.getProperty(hash, name, checkValid); 2539 } 2540 2541 } 2542 2543 @Override 2544 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2545 switch (hash) { 2546 case -351767064: // coverage 2547 this.coverage = castToReference(value); // Reference 2548 return value; 2549 case 522246568: // preAuthRef 2550 this.getPreAuthRef().add(castToString(value)); // StringType 2551 return value; 2552 default: return super.setProperty(hash, name, value); 2553 } 2554 2555 } 2556 2557 @Override 2558 public Base setProperty(String name, Base value) throws FHIRException { 2559 if (name.equals("coverage")) { 2560 this.coverage = castToReference(value); // Reference 2561 } else if (name.equals("preAuthRef")) { 2562 this.getPreAuthRef().add(castToString(value)); 2563 } else 2564 return super.setProperty(name, value); 2565 return value; 2566 } 2567 2568 @Override 2569 public Base makeProperty(int hash, String name) throws FHIRException { 2570 switch (hash) { 2571 case -351767064: return getCoverage(); 2572 case 522246568: return addPreAuthRefElement(); 2573 default: return super.makeProperty(hash, name); 2574 } 2575 2576 } 2577 2578 @Override 2579 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2580 switch (hash) { 2581 case -351767064: /*coverage*/ return new String[] {"Reference"}; 2582 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 2583 default: return super.getTypesForProperty(hash, name); 2584 } 2585 2586 } 2587 2588 @Override 2589 public Base addChild(String name) throws FHIRException { 2590 if (name.equals("coverage")) { 2591 this.coverage = new Reference(); 2592 return this.coverage; 2593 } 2594 else if (name.equals("preAuthRef")) { 2595 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.preAuthRef"); 2596 } 2597 else 2598 return super.addChild(name); 2599 } 2600 2601 public InsuranceComponent copy() { 2602 InsuranceComponent dst = new InsuranceComponent(); 2603 copyValues(dst); 2604 dst.coverage = coverage == null ? null : coverage.copy(); 2605 if (preAuthRef != null) { 2606 dst.preAuthRef = new ArrayList<StringType>(); 2607 for (StringType i : preAuthRef) 2608 dst.preAuthRef.add(i.copy()); 2609 }; 2610 return dst; 2611 } 2612 2613 @Override 2614 public boolean equalsDeep(Base other_) { 2615 if (!super.equalsDeep(other_)) 2616 return false; 2617 if (!(other_ instanceof InsuranceComponent)) 2618 return false; 2619 InsuranceComponent o = (InsuranceComponent) other_; 2620 return compareDeep(coverage, o.coverage, true) && compareDeep(preAuthRef, o.preAuthRef, true); 2621 } 2622 2623 @Override 2624 public boolean equalsShallow(Base other_) { 2625 if (!super.equalsShallow(other_)) 2626 return false; 2627 if (!(other_ instanceof InsuranceComponent)) 2628 return false; 2629 InsuranceComponent o = (InsuranceComponent) other_; 2630 return compareValues(preAuthRef, o.preAuthRef, true); 2631 } 2632 2633 public boolean isEmpty() { 2634 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, preAuthRef); 2635 } 2636 2637 public String fhirType() { 2638 return "ExplanationOfBenefit.insurance"; 2639 2640 } 2641 2642 } 2643 2644 @Block() 2645 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 2646 /** 2647 * Date of an accident which these services are addressing. 2648 */ 2649 @Child(name = "date", type = {DateType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2650 @Description(shortDefinition="When the accident occurred", formalDefinition="Date of an accident which these services are addressing." ) 2651 protected DateType date; 2652 2653 /** 2654 * Type of accident: work, auto, etc. 2655 */ 2656 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2657 @Description(shortDefinition="The nature of the accident", formalDefinition="Type of accident: work, auto, etc." ) 2658 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActIncidentCode") 2659 protected CodeableConcept type; 2660 2661 /** 2662 * Where the accident occurred. 2663 */ 2664 @Child(name = "location", type = {Address.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=false) 2665 @Description(shortDefinition="Accident Place", formalDefinition="Where the accident occurred." ) 2666 protected Type location; 2667 2668 private static final long serialVersionUID = 622904984L; 2669 2670 /** 2671 * Constructor 2672 */ 2673 public AccidentComponent() { 2674 super(); 2675 } 2676 2677 /** 2678 * @return {@link #date} (Date of an accident which these services are addressing.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2679 */ 2680 public DateType getDateElement() { 2681 if (this.date == null) 2682 if (Configuration.errorOnAutoCreate()) 2683 throw new Error("Attempt to auto-create AccidentComponent.date"); 2684 else if (Configuration.doAutoCreate()) 2685 this.date = new DateType(); // bb 2686 return this.date; 2687 } 2688 2689 public boolean hasDateElement() { 2690 return this.date != null && !this.date.isEmpty(); 2691 } 2692 2693 public boolean hasDate() { 2694 return this.date != null && !this.date.isEmpty(); 2695 } 2696 2697 /** 2698 * @param value {@link #date} (Date of an accident which these services are addressing.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2699 */ 2700 public AccidentComponent setDateElement(DateType value) { 2701 this.date = value; 2702 return this; 2703 } 2704 2705 /** 2706 * @return Date of an accident which these services are addressing. 2707 */ 2708 public Date getDate() { 2709 return this.date == null ? null : this.date.getValue(); 2710 } 2711 2712 /** 2713 * @param value Date of an accident which these services are addressing. 2714 */ 2715 public AccidentComponent setDate(Date value) { 2716 if (value == null) 2717 this.date = null; 2718 else { 2719 if (this.date == null) 2720 this.date = new DateType(); 2721 this.date.setValue(value); 2722 } 2723 return this; 2724 } 2725 2726 /** 2727 * @return {@link #type} (Type of accident: work, auto, etc.) 2728 */ 2729 public CodeableConcept getType() { 2730 if (this.type == null) 2731 if (Configuration.errorOnAutoCreate()) 2732 throw new Error("Attempt to auto-create AccidentComponent.type"); 2733 else if (Configuration.doAutoCreate()) 2734 this.type = new CodeableConcept(); // cc 2735 return this.type; 2736 } 2737 2738 public boolean hasType() { 2739 return this.type != null && !this.type.isEmpty(); 2740 } 2741 2742 /** 2743 * @param value {@link #type} (Type of accident: work, auto, etc.) 2744 */ 2745 public AccidentComponent setType(CodeableConcept value) { 2746 this.type = value; 2747 return this; 2748 } 2749 2750 /** 2751 * @return {@link #location} (Where the accident occurred.) 2752 */ 2753 public Type getLocation() { 2754 return this.location; 2755 } 2756 2757 /** 2758 * @return {@link #location} (Where the accident occurred.) 2759 */ 2760 public Address getLocationAddress() throws FHIRException { 2761 if (this.location == null) 2762 return null; 2763 if (!(this.location instanceof Address)) 2764 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 2765 return (Address) this.location; 2766 } 2767 2768 public boolean hasLocationAddress() { 2769 return this != null && this.location instanceof Address; 2770 } 2771 2772 /** 2773 * @return {@link #location} (Where the accident occurred.) 2774 */ 2775 public Reference getLocationReference() throws FHIRException { 2776 if (this.location == null) 2777 return null; 2778 if (!(this.location instanceof Reference)) 2779 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 2780 return (Reference) this.location; 2781 } 2782 2783 public boolean hasLocationReference() { 2784 return this != null && this.location instanceof Reference; 2785 } 2786 2787 public boolean hasLocation() { 2788 return this.location != null && !this.location.isEmpty(); 2789 } 2790 2791 /** 2792 * @param value {@link #location} (Where the accident occurred.) 2793 */ 2794 public AccidentComponent setLocation(Type value) throws FHIRFormatError { 2795 if (value != null && !(value instanceof Address || value instanceof Reference)) 2796 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.accident.location[x]: "+value.fhirType()); 2797 this.location = value; 2798 return this; 2799 } 2800 2801 protected void listChildren(List<Property> children) { 2802 super.listChildren(children); 2803 children.add(new Property("date", "date", "Date of an accident which these services are addressing.", 0, 1, date)); 2804 children.add(new Property("type", "CodeableConcept", "Type of accident: work, auto, etc.", 0, 1, type)); 2805 children.add(new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location)); 2806 } 2807 2808 @Override 2809 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2810 switch (_hash) { 2811 case 3076014: /*date*/ return new Property("date", "date", "Date of an accident which these services are addressing.", 0, 1, date); 2812 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of accident: work, auto, etc.", 0, 1, type); 2813 case 552316075: /*location[x]*/ return new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location); 2814 case 1901043637: /*location*/ return new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location); 2815 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location); 2816 case 755866390: /*locationReference*/ return new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location); 2817 default: return super.getNamedProperty(_hash, _name, _checkValid); 2818 } 2819 2820 } 2821 2822 @Override 2823 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2824 switch (hash) { 2825 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 2826 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2827 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Type 2828 default: return super.getProperty(hash, name, checkValid); 2829 } 2830 2831 } 2832 2833 @Override 2834 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2835 switch (hash) { 2836 case 3076014: // date 2837 this.date = castToDate(value); // DateType 2838 return value; 2839 case 3575610: // type 2840 this.type = castToCodeableConcept(value); // CodeableConcept 2841 return value; 2842 case 1901043637: // location 2843 this.location = castToType(value); // Type 2844 return value; 2845 default: return super.setProperty(hash, name, value); 2846 } 2847 2848 } 2849 2850 @Override 2851 public Base setProperty(String name, Base value) throws FHIRException { 2852 if (name.equals("date")) { 2853 this.date = castToDate(value); // DateType 2854 } else if (name.equals("type")) { 2855 this.type = castToCodeableConcept(value); // CodeableConcept 2856 } else if (name.equals("location[x]")) { 2857 this.location = castToType(value); // Type 2858 } else 2859 return super.setProperty(name, value); 2860 return value; 2861 } 2862 2863 @Override 2864 public Base makeProperty(int hash, String name) throws FHIRException { 2865 switch (hash) { 2866 case 3076014: return getDateElement(); 2867 case 3575610: return getType(); 2868 case 552316075: return getLocation(); 2869 case 1901043637: return getLocation(); 2870 default: return super.makeProperty(hash, name); 2871 } 2872 2873 } 2874 2875 @Override 2876 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2877 switch (hash) { 2878 case 3076014: /*date*/ return new String[] {"date"}; 2879 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2880 case 1901043637: /*location*/ return new String[] {"Address", "Reference"}; 2881 default: return super.getTypesForProperty(hash, name); 2882 } 2883 2884 } 2885 2886 @Override 2887 public Base addChild(String name) throws FHIRException { 2888 if (name.equals("date")) { 2889 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 2890 } 2891 else if (name.equals("type")) { 2892 this.type = new CodeableConcept(); 2893 return this.type; 2894 } 2895 else if (name.equals("locationAddress")) { 2896 this.location = new Address(); 2897 return this.location; 2898 } 2899 else if (name.equals("locationReference")) { 2900 this.location = new Reference(); 2901 return this.location; 2902 } 2903 else 2904 return super.addChild(name); 2905 } 2906 2907 public AccidentComponent copy() { 2908 AccidentComponent dst = new AccidentComponent(); 2909 copyValues(dst); 2910 dst.date = date == null ? null : date.copy(); 2911 dst.type = type == null ? null : type.copy(); 2912 dst.location = location == null ? null : location.copy(); 2913 return dst; 2914 } 2915 2916 @Override 2917 public boolean equalsDeep(Base other_) { 2918 if (!super.equalsDeep(other_)) 2919 return false; 2920 if (!(other_ instanceof AccidentComponent)) 2921 return false; 2922 AccidentComponent o = (AccidentComponent) other_; 2923 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) && compareDeep(location, o.location, true) 2924 ; 2925 } 2926 2927 @Override 2928 public boolean equalsShallow(Base other_) { 2929 if (!super.equalsShallow(other_)) 2930 return false; 2931 if (!(other_ instanceof AccidentComponent)) 2932 return false; 2933 AccidentComponent o = (AccidentComponent) other_; 2934 return compareValues(date, o.date, true); 2935 } 2936 2937 public boolean isEmpty() { 2938 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 2939 } 2940 2941 public String fhirType() { 2942 return "ExplanationOfBenefit.accident"; 2943 2944 } 2945 2946 } 2947 2948 @Block() 2949 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 2950 /** 2951 * A service line number. 2952 */ 2953 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2954 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 2955 protected PositiveIntType sequence; 2956 2957 /** 2958 * Careteam applicable for this service or product line. 2959 */ 2960 @Child(name = "careTeamLinkId", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2961 @Description(shortDefinition="Applicable careteam members", formalDefinition="Careteam applicable for this service or product line." ) 2962 protected List<PositiveIntType> careTeamLinkId; 2963 2964 /** 2965 * Diagnosis applicable for this service or product line. 2966 */ 2967 @Child(name = "diagnosisLinkId", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2968 @Description(shortDefinition="Applicable diagnoses", formalDefinition="Diagnosis applicable for this service or product line." ) 2969 protected List<PositiveIntType> diagnosisLinkId; 2970 2971 /** 2972 * Procedures applicable for this service or product line. 2973 */ 2974 @Child(name = "procedureLinkId", type = {PositiveIntType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2975 @Description(shortDefinition="Applicable procedures", formalDefinition="Procedures applicable for this service or product line." ) 2976 protected List<PositiveIntType> procedureLinkId; 2977 2978 /** 2979 * Exceptions, special conditions and supporting information pplicable for this service or product line. 2980 */ 2981 @Child(name = "informationLinkId", type = {PositiveIntType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2982 @Description(shortDefinition="Applicable exception and supporting information", formalDefinition="Exceptions, special conditions and supporting information pplicable for this service or product line." ) 2983 protected List<PositiveIntType> informationLinkId; 2984 2985 /** 2986 * The type of reveneu or cost center providing the product and/or service. 2987 */ 2988 @Child(name = "revenue", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 2989 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 2990 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 2991 protected CodeableConcept revenue; 2992 2993 /** 2994 * Health Care Service Type Codes to identify the classification of service or benefits. 2995 */ 2996 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 2997 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 2998 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 2999 protected CodeableConcept category; 3000 3001 /** 3002 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 3003 */ 3004 @Child(name = "service", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 3005 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 3006 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3007 protected CodeableConcept service; 3008 3009 /** 3010 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 3011 */ 3012 @Child(name = "modifier", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3013 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 3014 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 3015 protected List<CodeableConcept> modifier; 3016 3017 /** 3018 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 3019 */ 3020 @Child(name = "programCode", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3021 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 3022 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 3023 protected List<CodeableConcept> programCode; 3024 3025 /** 3026 * The date or dates when the enclosed suite of services were performed or completed. 3027 */ 3028 @Child(name = "serviced", type = {DateType.class, Period.class}, order=11, min=0, max=1, modifier=false, summary=false) 3029 @Description(shortDefinition="Date or dates of Service", formalDefinition="The date or dates when the enclosed suite of services were performed or completed." ) 3030 protected Type serviced; 3031 3032 /** 3033 * Where the service was provided. 3034 */ 3035 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=12, min=0, max=1, modifier=false, summary=false) 3036 @Description(shortDefinition="Place of service", formalDefinition="Where the service was provided." ) 3037 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 3038 protected Type location; 3039 3040 /** 3041 * The number of repetitions of a service or product. 3042 */ 3043 @Child(name = "quantity", type = {SimpleQuantity.class}, order=13, min=0, max=1, modifier=false, summary=false) 3044 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 3045 protected SimpleQuantity quantity; 3046 3047 /** 3048 * If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group. 3049 */ 3050 @Child(name = "unitPrice", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 3051 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group." ) 3052 protected Money unitPrice; 3053 3054 /** 3055 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3056 */ 3057 @Child(name = "factor", type = {DecimalType.class}, order=15, min=0, max=1, modifier=false, summary=false) 3058 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 3059 protected DecimalType factor; 3060 3061 /** 3062 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 3063 */ 3064 @Child(name = "net", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 3065 @Description(shortDefinition="Total item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 3066 protected Money net; 3067 3068 /** 3069 * List of Unique Device Identifiers associated with this line item. 3070 */ 3071 @Child(name = "udi", type = {Device.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3072 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 3073 protected List<Reference> udi; 3074 /** 3075 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 3076 */ 3077 protected List<Device> udiTarget; 3078 3079 3080 /** 3081 * Physical service site on the patient (limb, tooth, etc). 3082 */ 3083 @Child(name = "bodySite", type = {CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=false) 3084 @Description(shortDefinition="Service Location", formalDefinition="Physical service site on the patient (limb, tooth, etc)." ) 3085 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 3086 protected CodeableConcept bodySite; 3087 3088 /** 3089 * A region or surface of the site, eg. limb region or tooth surface(s). 3090 */ 3091 @Child(name = "subSite", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3092 @Description(shortDefinition="Service Sub-location", formalDefinition="A region or surface of the site, eg. limb region or tooth surface(s)." ) 3093 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 3094 protected List<CodeableConcept> subSite; 3095 3096 /** 3097 * A billed item may include goods or services provided in multiple encounters. 3098 */ 3099 @Child(name = "encounter", type = {Encounter.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3100 @Description(shortDefinition="Encounters related to this billed item", formalDefinition="A billed item may include goods or services provided in multiple encounters." ) 3101 protected List<Reference> encounter; 3102 /** 3103 * The actual objects that are the target of the reference (A billed item may include goods or services provided in multiple encounters.) 3104 */ 3105 protected List<Encounter> encounterTarget; 3106 3107 3108 /** 3109 * A list of note references to the notes provided below. 3110 */ 3111 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3112 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 3113 protected List<PositiveIntType> noteNumber; 3114 3115 /** 3116 * The adjudications results. 3117 */ 3118 @Child(name = "adjudication", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3119 @Description(shortDefinition="Adjudication details", formalDefinition="The adjudications results." ) 3120 protected List<AdjudicationComponent> adjudication; 3121 3122 /** 3123 * Second tier of goods and services. 3124 */ 3125 @Child(name = "detail", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3126 @Description(shortDefinition="Additional items", formalDefinition="Second tier of goods and services." ) 3127 protected List<DetailComponent> detail; 3128 3129 private static final long serialVersionUID = -1567825229L; 3130 3131 /** 3132 * Constructor 3133 */ 3134 public ItemComponent() { 3135 super(); 3136 } 3137 3138 /** 3139 * Constructor 3140 */ 3141 public ItemComponent(PositiveIntType sequence) { 3142 super(); 3143 this.sequence = sequence; 3144 } 3145 3146 /** 3147 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3148 */ 3149 public PositiveIntType getSequenceElement() { 3150 if (this.sequence == null) 3151 if (Configuration.errorOnAutoCreate()) 3152 throw new Error("Attempt to auto-create ItemComponent.sequence"); 3153 else if (Configuration.doAutoCreate()) 3154 this.sequence = new PositiveIntType(); // bb 3155 return this.sequence; 3156 } 3157 3158 public boolean hasSequenceElement() { 3159 return this.sequence != null && !this.sequence.isEmpty(); 3160 } 3161 3162 public boolean hasSequence() { 3163 return this.sequence != null && !this.sequence.isEmpty(); 3164 } 3165 3166 /** 3167 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3168 */ 3169 public ItemComponent setSequenceElement(PositiveIntType value) { 3170 this.sequence = value; 3171 return this; 3172 } 3173 3174 /** 3175 * @return A service line number. 3176 */ 3177 public int getSequence() { 3178 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3179 } 3180 3181 /** 3182 * @param value A service line number. 3183 */ 3184 public ItemComponent setSequence(int value) { 3185 if (this.sequence == null) 3186 this.sequence = new PositiveIntType(); 3187 this.sequence.setValue(value); 3188 return this; 3189 } 3190 3191 /** 3192 * @return {@link #careTeamLinkId} (Careteam applicable for this service or product line.) 3193 */ 3194 public List<PositiveIntType> getCareTeamLinkId() { 3195 if (this.careTeamLinkId == null) 3196 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3197 return this.careTeamLinkId; 3198 } 3199 3200 /** 3201 * @return Returns a reference to <code>this</code> for easy method chaining 3202 */ 3203 public ItemComponent setCareTeamLinkId(List<PositiveIntType> theCareTeamLinkId) { 3204 this.careTeamLinkId = theCareTeamLinkId; 3205 return this; 3206 } 3207 3208 public boolean hasCareTeamLinkId() { 3209 if (this.careTeamLinkId == null) 3210 return false; 3211 for (PositiveIntType item : this.careTeamLinkId) 3212 if (!item.isEmpty()) 3213 return true; 3214 return false; 3215 } 3216 3217 /** 3218 * @return {@link #careTeamLinkId} (Careteam applicable for this service or product line.) 3219 */ 3220 public PositiveIntType addCareTeamLinkIdElement() {//2 3221 PositiveIntType t = new PositiveIntType(); 3222 if (this.careTeamLinkId == null) 3223 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3224 this.careTeamLinkId.add(t); 3225 return t; 3226 } 3227 3228 /** 3229 * @param value {@link #careTeamLinkId} (Careteam applicable for this service or product line.) 3230 */ 3231 public ItemComponent addCareTeamLinkId(int value) { //1 3232 PositiveIntType t = new PositiveIntType(); 3233 t.setValue(value); 3234 if (this.careTeamLinkId == null) 3235 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3236 this.careTeamLinkId.add(t); 3237 return this; 3238 } 3239 3240 /** 3241 * @param value {@link #careTeamLinkId} (Careteam applicable for this service or product line.) 3242 */ 3243 public boolean hasCareTeamLinkId(int value) { 3244 if (this.careTeamLinkId == null) 3245 return false; 3246 for (PositiveIntType v : this.careTeamLinkId) 3247 if (v.getValue().equals(value)) // positiveInt 3248 return true; 3249 return false; 3250 } 3251 3252 /** 3253 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3254 */ 3255 public List<PositiveIntType> getDiagnosisLinkId() { 3256 if (this.diagnosisLinkId == null) 3257 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3258 return this.diagnosisLinkId; 3259 } 3260 3261 /** 3262 * @return Returns a reference to <code>this</code> for easy method chaining 3263 */ 3264 public ItemComponent setDiagnosisLinkId(List<PositiveIntType> theDiagnosisLinkId) { 3265 this.diagnosisLinkId = theDiagnosisLinkId; 3266 return this; 3267 } 3268 3269 public boolean hasDiagnosisLinkId() { 3270 if (this.diagnosisLinkId == null) 3271 return false; 3272 for (PositiveIntType item : this.diagnosisLinkId) 3273 if (!item.isEmpty()) 3274 return true; 3275 return false; 3276 } 3277 3278 /** 3279 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3280 */ 3281 public PositiveIntType addDiagnosisLinkIdElement() {//2 3282 PositiveIntType t = new PositiveIntType(); 3283 if (this.diagnosisLinkId == null) 3284 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3285 this.diagnosisLinkId.add(t); 3286 return t; 3287 } 3288 3289 /** 3290 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3291 */ 3292 public ItemComponent addDiagnosisLinkId(int value) { //1 3293 PositiveIntType t = new PositiveIntType(); 3294 t.setValue(value); 3295 if (this.diagnosisLinkId == null) 3296 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3297 this.diagnosisLinkId.add(t); 3298 return this; 3299 } 3300 3301 /** 3302 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3303 */ 3304 public boolean hasDiagnosisLinkId(int value) { 3305 if (this.diagnosisLinkId == null) 3306 return false; 3307 for (PositiveIntType v : this.diagnosisLinkId) 3308 if (v.getValue().equals(value)) // positiveInt 3309 return true; 3310 return false; 3311 } 3312 3313 /** 3314 * @return {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3315 */ 3316 public List<PositiveIntType> getProcedureLinkId() { 3317 if (this.procedureLinkId == null) 3318 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3319 return this.procedureLinkId; 3320 } 3321 3322 /** 3323 * @return Returns a reference to <code>this</code> for easy method chaining 3324 */ 3325 public ItemComponent setProcedureLinkId(List<PositiveIntType> theProcedureLinkId) { 3326 this.procedureLinkId = theProcedureLinkId; 3327 return this; 3328 } 3329 3330 public boolean hasProcedureLinkId() { 3331 if (this.procedureLinkId == null) 3332 return false; 3333 for (PositiveIntType item : this.procedureLinkId) 3334 if (!item.isEmpty()) 3335 return true; 3336 return false; 3337 } 3338 3339 /** 3340 * @return {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3341 */ 3342 public PositiveIntType addProcedureLinkIdElement() {//2 3343 PositiveIntType t = new PositiveIntType(); 3344 if (this.procedureLinkId == null) 3345 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3346 this.procedureLinkId.add(t); 3347 return t; 3348 } 3349 3350 /** 3351 * @param value {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3352 */ 3353 public ItemComponent addProcedureLinkId(int value) { //1 3354 PositiveIntType t = new PositiveIntType(); 3355 t.setValue(value); 3356 if (this.procedureLinkId == null) 3357 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3358 this.procedureLinkId.add(t); 3359 return this; 3360 } 3361 3362 /** 3363 * @param value {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3364 */ 3365 public boolean hasProcedureLinkId(int value) { 3366 if (this.procedureLinkId == null) 3367 return false; 3368 for (PositiveIntType v : this.procedureLinkId) 3369 if (v.getValue().equals(value)) // positiveInt 3370 return true; 3371 return false; 3372 } 3373 3374 /** 3375 * @return {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3376 */ 3377 public List<PositiveIntType> getInformationLinkId() { 3378 if (this.informationLinkId == null) 3379 this.informationLinkId = new ArrayList<PositiveIntType>(); 3380 return this.informationLinkId; 3381 } 3382 3383 /** 3384 * @return Returns a reference to <code>this</code> for easy method chaining 3385 */ 3386 public ItemComponent setInformationLinkId(List<PositiveIntType> theInformationLinkId) { 3387 this.informationLinkId = theInformationLinkId; 3388 return this; 3389 } 3390 3391 public boolean hasInformationLinkId() { 3392 if (this.informationLinkId == null) 3393 return false; 3394 for (PositiveIntType item : this.informationLinkId) 3395 if (!item.isEmpty()) 3396 return true; 3397 return false; 3398 } 3399 3400 /** 3401 * @return {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3402 */ 3403 public PositiveIntType addInformationLinkIdElement() {//2 3404 PositiveIntType t = new PositiveIntType(); 3405 if (this.informationLinkId == null) 3406 this.informationLinkId = new ArrayList<PositiveIntType>(); 3407 this.informationLinkId.add(t); 3408 return t; 3409 } 3410 3411 /** 3412 * @param value {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3413 */ 3414 public ItemComponent addInformationLinkId(int value) { //1 3415 PositiveIntType t = new PositiveIntType(); 3416 t.setValue(value); 3417 if (this.informationLinkId == null) 3418 this.informationLinkId = new ArrayList<PositiveIntType>(); 3419 this.informationLinkId.add(t); 3420 return this; 3421 } 3422 3423 /** 3424 * @param value {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3425 */ 3426 public boolean hasInformationLinkId(int value) { 3427 if (this.informationLinkId == null) 3428 return false; 3429 for (PositiveIntType v : this.informationLinkId) 3430 if (v.getValue().equals(value)) // positiveInt 3431 return true; 3432 return false; 3433 } 3434 3435 /** 3436 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 3437 */ 3438 public CodeableConcept getRevenue() { 3439 if (this.revenue == null) 3440 if (Configuration.errorOnAutoCreate()) 3441 throw new Error("Attempt to auto-create ItemComponent.revenue"); 3442 else if (Configuration.doAutoCreate()) 3443 this.revenue = new CodeableConcept(); // cc 3444 return this.revenue; 3445 } 3446 3447 public boolean hasRevenue() { 3448 return this.revenue != null && !this.revenue.isEmpty(); 3449 } 3450 3451 /** 3452 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 3453 */ 3454 public ItemComponent setRevenue(CodeableConcept value) { 3455 this.revenue = value; 3456 return this; 3457 } 3458 3459 /** 3460 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 3461 */ 3462 public CodeableConcept getCategory() { 3463 if (this.category == null) 3464 if (Configuration.errorOnAutoCreate()) 3465 throw new Error("Attempt to auto-create ItemComponent.category"); 3466 else if (Configuration.doAutoCreate()) 3467 this.category = new CodeableConcept(); // cc 3468 return this.category; 3469 } 3470 3471 public boolean hasCategory() { 3472 return this.category != null && !this.category.isEmpty(); 3473 } 3474 3475 /** 3476 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 3477 */ 3478 public ItemComponent setCategory(CodeableConcept value) { 3479 this.category = value; 3480 return this; 3481 } 3482 3483 /** 3484 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 3485 */ 3486 public CodeableConcept getService() { 3487 if (this.service == null) 3488 if (Configuration.errorOnAutoCreate()) 3489 throw new Error("Attempt to auto-create ItemComponent.service"); 3490 else if (Configuration.doAutoCreate()) 3491 this.service = new CodeableConcept(); // cc 3492 return this.service; 3493 } 3494 3495 public boolean hasService() { 3496 return this.service != null && !this.service.isEmpty(); 3497 } 3498 3499 /** 3500 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 3501 */ 3502 public ItemComponent setService(CodeableConcept value) { 3503 this.service = value; 3504 return this; 3505 } 3506 3507 /** 3508 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 3509 */ 3510 public List<CodeableConcept> getModifier() { 3511 if (this.modifier == null) 3512 this.modifier = new ArrayList<CodeableConcept>(); 3513 return this.modifier; 3514 } 3515 3516 /** 3517 * @return Returns a reference to <code>this</code> for easy method chaining 3518 */ 3519 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 3520 this.modifier = theModifier; 3521 return this; 3522 } 3523 3524 public boolean hasModifier() { 3525 if (this.modifier == null) 3526 return false; 3527 for (CodeableConcept item : this.modifier) 3528 if (!item.isEmpty()) 3529 return true; 3530 return false; 3531 } 3532 3533 public CodeableConcept addModifier() { //3 3534 CodeableConcept t = new CodeableConcept(); 3535 if (this.modifier == null) 3536 this.modifier = new ArrayList<CodeableConcept>(); 3537 this.modifier.add(t); 3538 return t; 3539 } 3540 3541 public ItemComponent addModifier(CodeableConcept t) { //3 3542 if (t == null) 3543 return this; 3544 if (this.modifier == null) 3545 this.modifier = new ArrayList<CodeableConcept>(); 3546 this.modifier.add(t); 3547 return this; 3548 } 3549 3550 /** 3551 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 3552 */ 3553 public CodeableConcept getModifierFirstRep() { 3554 if (getModifier().isEmpty()) { 3555 addModifier(); 3556 } 3557 return getModifier().get(0); 3558 } 3559 3560 /** 3561 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 3562 */ 3563 public List<CodeableConcept> getProgramCode() { 3564 if (this.programCode == null) 3565 this.programCode = new ArrayList<CodeableConcept>(); 3566 return this.programCode; 3567 } 3568 3569 /** 3570 * @return Returns a reference to <code>this</code> for easy method chaining 3571 */ 3572 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 3573 this.programCode = theProgramCode; 3574 return this; 3575 } 3576 3577 public boolean hasProgramCode() { 3578 if (this.programCode == null) 3579 return false; 3580 for (CodeableConcept item : this.programCode) 3581 if (!item.isEmpty()) 3582 return true; 3583 return false; 3584 } 3585 3586 public CodeableConcept addProgramCode() { //3 3587 CodeableConcept t = new CodeableConcept(); 3588 if (this.programCode == null) 3589 this.programCode = new ArrayList<CodeableConcept>(); 3590 this.programCode.add(t); 3591 return t; 3592 } 3593 3594 public ItemComponent addProgramCode(CodeableConcept t) { //3 3595 if (t == null) 3596 return this; 3597 if (this.programCode == null) 3598 this.programCode = new ArrayList<CodeableConcept>(); 3599 this.programCode.add(t); 3600 return this; 3601 } 3602 3603 /** 3604 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 3605 */ 3606 public CodeableConcept getProgramCodeFirstRep() { 3607 if (getProgramCode().isEmpty()) { 3608 addProgramCode(); 3609 } 3610 return getProgramCode().get(0); 3611 } 3612 3613 /** 3614 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3615 */ 3616 public Type getServiced() { 3617 return this.serviced; 3618 } 3619 3620 /** 3621 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3622 */ 3623 public DateType getServicedDateType() throws FHIRException { 3624 if (this.serviced == null) 3625 return null; 3626 if (!(this.serviced instanceof DateType)) 3627 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 3628 return (DateType) this.serviced; 3629 } 3630 3631 public boolean hasServicedDateType() { 3632 return this != null && this.serviced instanceof DateType; 3633 } 3634 3635 /** 3636 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3637 */ 3638 public Period getServicedPeriod() throws FHIRException { 3639 if (this.serviced == null) 3640 return null; 3641 if (!(this.serviced instanceof Period)) 3642 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 3643 return (Period) this.serviced; 3644 } 3645 3646 public boolean hasServicedPeriod() { 3647 return this != null && this.serviced instanceof Period; 3648 } 3649 3650 public boolean hasServiced() { 3651 return this.serviced != null && !this.serviced.isEmpty(); 3652 } 3653 3654 /** 3655 * @param value {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3656 */ 3657 public ItemComponent setServiced(Type value) throws FHIRFormatError { 3658 if (value != null && !(value instanceof DateType || value instanceof Period)) 3659 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.item.serviced[x]: "+value.fhirType()); 3660 this.serviced = value; 3661 return this; 3662 } 3663 3664 /** 3665 * @return {@link #location} (Where the service was provided.) 3666 */ 3667 public Type getLocation() { 3668 return this.location; 3669 } 3670 3671 /** 3672 * @return {@link #location} (Where the service was provided.) 3673 */ 3674 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 3675 if (this.location == null) 3676 return null; 3677 if (!(this.location instanceof CodeableConcept)) 3678 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 3679 return (CodeableConcept) this.location; 3680 } 3681 3682 public boolean hasLocationCodeableConcept() { 3683 return this != null && this.location instanceof CodeableConcept; 3684 } 3685 3686 /** 3687 * @return {@link #location} (Where the service was provided.) 3688 */ 3689 public Address getLocationAddress() throws FHIRException { 3690 if (this.location == null) 3691 return null; 3692 if (!(this.location instanceof Address)) 3693 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3694 return (Address) this.location; 3695 } 3696 3697 public boolean hasLocationAddress() { 3698 return this != null && this.location instanceof Address; 3699 } 3700 3701 /** 3702 * @return {@link #location} (Where the service was provided.) 3703 */ 3704 public Reference getLocationReference() throws FHIRException { 3705 if (this.location == null) 3706 return null; 3707 if (!(this.location instanceof Reference)) 3708 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3709 return (Reference) this.location; 3710 } 3711 3712 public boolean hasLocationReference() { 3713 return this != null && this.location instanceof Reference; 3714 } 3715 3716 public boolean hasLocation() { 3717 return this.location != null && !this.location.isEmpty(); 3718 } 3719 3720 /** 3721 * @param value {@link #location} (Where the service was provided.) 3722 */ 3723 public ItemComponent setLocation(Type value) throws FHIRFormatError { 3724 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 3725 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.item.location[x]: "+value.fhirType()); 3726 this.location = value; 3727 return this; 3728 } 3729 3730 /** 3731 * @return {@link #quantity} (The number of repetitions of a service or product.) 3732 */ 3733 public SimpleQuantity getQuantity() { 3734 if (this.quantity == null) 3735 if (Configuration.errorOnAutoCreate()) 3736 throw new Error("Attempt to auto-create ItemComponent.quantity"); 3737 else if (Configuration.doAutoCreate()) 3738 this.quantity = new SimpleQuantity(); // cc 3739 return this.quantity; 3740 } 3741 3742 public boolean hasQuantity() { 3743 return this.quantity != null && !this.quantity.isEmpty(); 3744 } 3745 3746 /** 3747 * @param value {@link #quantity} (The number of repetitions of a service or product.) 3748 */ 3749 public ItemComponent setQuantity(SimpleQuantity value) { 3750 this.quantity = value; 3751 return this; 3752 } 3753 3754 /** 3755 * @return {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 3756 */ 3757 public Money getUnitPrice() { 3758 if (this.unitPrice == null) 3759 if (Configuration.errorOnAutoCreate()) 3760 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 3761 else if (Configuration.doAutoCreate()) 3762 this.unitPrice = new Money(); // cc 3763 return this.unitPrice; 3764 } 3765 3766 public boolean hasUnitPrice() { 3767 return this.unitPrice != null && !this.unitPrice.isEmpty(); 3768 } 3769 3770 /** 3771 * @param value {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 3772 */ 3773 public ItemComponent setUnitPrice(Money value) { 3774 this.unitPrice = value; 3775 return this; 3776 } 3777 3778 /** 3779 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 3780 */ 3781 public DecimalType getFactorElement() { 3782 if (this.factor == null) 3783 if (Configuration.errorOnAutoCreate()) 3784 throw new Error("Attempt to auto-create ItemComponent.factor"); 3785 else if (Configuration.doAutoCreate()) 3786 this.factor = new DecimalType(); // bb 3787 return this.factor; 3788 } 3789 3790 public boolean hasFactorElement() { 3791 return this.factor != null && !this.factor.isEmpty(); 3792 } 3793 3794 public boolean hasFactor() { 3795 return this.factor != null && !this.factor.isEmpty(); 3796 } 3797 3798 /** 3799 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 3800 */ 3801 public ItemComponent setFactorElement(DecimalType value) { 3802 this.factor = value; 3803 return this; 3804 } 3805 3806 /** 3807 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3808 */ 3809 public BigDecimal getFactor() { 3810 return this.factor == null ? null : this.factor.getValue(); 3811 } 3812 3813 /** 3814 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3815 */ 3816 public ItemComponent setFactor(BigDecimal value) { 3817 if (value == null) 3818 this.factor = null; 3819 else { 3820 if (this.factor == null) 3821 this.factor = new DecimalType(); 3822 this.factor.setValue(value); 3823 } 3824 return this; 3825 } 3826 3827 /** 3828 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3829 */ 3830 public ItemComponent setFactor(long value) { 3831 this.factor = new DecimalType(); 3832 this.factor.setValue(value); 3833 return this; 3834 } 3835 3836 /** 3837 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3838 */ 3839 public ItemComponent setFactor(double value) { 3840 this.factor = new DecimalType(); 3841 this.factor.setValue(value); 3842 return this; 3843 } 3844 3845 /** 3846 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 3847 */ 3848 public Money getNet() { 3849 if (this.net == null) 3850 if (Configuration.errorOnAutoCreate()) 3851 throw new Error("Attempt to auto-create ItemComponent.net"); 3852 else if (Configuration.doAutoCreate()) 3853 this.net = new Money(); // cc 3854 return this.net; 3855 } 3856 3857 public boolean hasNet() { 3858 return this.net != null && !this.net.isEmpty(); 3859 } 3860 3861 /** 3862 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 3863 */ 3864 public ItemComponent setNet(Money value) { 3865 this.net = value; 3866 return this; 3867 } 3868 3869 /** 3870 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 3871 */ 3872 public List<Reference> getUdi() { 3873 if (this.udi == null) 3874 this.udi = new ArrayList<Reference>(); 3875 return this.udi; 3876 } 3877 3878 /** 3879 * @return Returns a reference to <code>this</code> for easy method chaining 3880 */ 3881 public ItemComponent setUdi(List<Reference> theUdi) { 3882 this.udi = theUdi; 3883 return this; 3884 } 3885 3886 public boolean hasUdi() { 3887 if (this.udi == null) 3888 return false; 3889 for (Reference item : this.udi) 3890 if (!item.isEmpty()) 3891 return true; 3892 return false; 3893 } 3894 3895 public Reference addUdi() { //3 3896 Reference t = new Reference(); 3897 if (this.udi == null) 3898 this.udi = new ArrayList<Reference>(); 3899 this.udi.add(t); 3900 return t; 3901 } 3902 3903 public ItemComponent addUdi(Reference t) { //3 3904 if (t == null) 3905 return this; 3906 if (this.udi == null) 3907 this.udi = new ArrayList<Reference>(); 3908 this.udi.add(t); 3909 return this; 3910 } 3911 3912 /** 3913 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 3914 */ 3915 public Reference getUdiFirstRep() { 3916 if (getUdi().isEmpty()) { 3917 addUdi(); 3918 } 3919 return getUdi().get(0); 3920 } 3921 3922 /** 3923 * @deprecated Use Reference#setResource(IBaseResource) instead 3924 */ 3925 @Deprecated 3926 public List<Device> getUdiTarget() { 3927 if (this.udiTarget == null) 3928 this.udiTarget = new ArrayList<Device>(); 3929 return this.udiTarget; 3930 } 3931 3932 /** 3933 * @deprecated Use Reference#setResource(IBaseResource) instead 3934 */ 3935 @Deprecated 3936 public Device addUdiTarget() { 3937 Device r = new Device(); 3938 if (this.udiTarget == null) 3939 this.udiTarget = new ArrayList<Device>(); 3940 this.udiTarget.add(r); 3941 return r; 3942 } 3943 3944 /** 3945 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, etc).) 3946 */ 3947 public CodeableConcept getBodySite() { 3948 if (this.bodySite == null) 3949 if (Configuration.errorOnAutoCreate()) 3950 throw new Error("Attempt to auto-create ItemComponent.bodySite"); 3951 else if (Configuration.doAutoCreate()) 3952 this.bodySite = new CodeableConcept(); // cc 3953 return this.bodySite; 3954 } 3955 3956 public boolean hasBodySite() { 3957 return this.bodySite != null && !this.bodySite.isEmpty(); 3958 } 3959 3960 /** 3961 * @param value {@link #bodySite} (Physical service site on the patient (limb, tooth, etc).) 3962 */ 3963 public ItemComponent setBodySite(CodeableConcept value) { 3964 this.bodySite = value; 3965 return this; 3966 } 3967 3968 /** 3969 * @return {@link #subSite} (A region or surface of the site, eg. limb region or tooth surface(s).) 3970 */ 3971 public List<CodeableConcept> getSubSite() { 3972 if (this.subSite == null) 3973 this.subSite = new ArrayList<CodeableConcept>(); 3974 return this.subSite; 3975 } 3976 3977 /** 3978 * @return Returns a reference to <code>this</code> for easy method chaining 3979 */ 3980 public ItemComponent setSubSite(List<CodeableConcept> theSubSite) { 3981 this.subSite = theSubSite; 3982 return this; 3983 } 3984 3985 public boolean hasSubSite() { 3986 if (this.subSite == null) 3987 return false; 3988 for (CodeableConcept item : this.subSite) 3989 if (!item.isEmpty()) 3990 return true; 3991 return false; 3992 } 3993 3994 public CodeableConcept addSubSite() { //3 3995 CodeableConcept t = new CodeableConcept(); 3996 if (this.subSite == null) 3997 this.subSite = new ArrayList<CodeableConcept>(); 3998 this.subSite.add(t); 3999 return t; 4000 } 4001 4002 public ItemComponent addSubSite(CodeableConcept t) { //3 4003 if (t == null) 4004 return this; 4005 if (this.subSite == null) 4006 this.subSite = new ArrayList<CodeableConcept>(); 4007 this.subSite.add(t); 4008 return this; 4009 } 4010 4011 /** 4012 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist 4013 */ 4014 public CodeableConcept getSubSiteFirstRep() { 4015 if (getSubSite().isEmpty()) { 4016 addSubSite(); 4017 } 4018 return getSubSite().get(0); 4019 } 4020 4021 /** 4022 * @return {@link #encounter} (A billed item may include goods or services provided in multiple encounters.) 4023 */ 4024 public List<Reference> getEncounter() { 4025 if (this.encounter == null) 4026 this.encounter = new ArrayList<Reference>(); 4027 return this.encounter; 4028 } 4029 4030 /** 4031 * @return Returns a reference to <code>this</code> for easy method chaining 4032 */ 4033 public ItemComponent setEncounter(List<Reference> theEncounter) { 4034 this.encounter = theEncounter; 4035 return this; 4036 } 4037 4038 public boolean hasEncounter() { 4039 if (this.encounter == null) 4040 return false; 4041 for (Reference item : this.encounter) 4042 if (!item.isEmpty()) 4043 return true; 4044 return false; 4045 } 4046 4047 public Reference addEncounter() { //3 4048 Reference t = new Reference(); 4049 if (this.encounter == null) 4050 this.encounter = new ArrayList<Reference>(); 4051 this.encounter.add(t); 4052 return t; 4053 } 4054 4055 public ItemComponent addEncounter(Reference t) { //3 4056 if (t == null) 4057 return this; 4058 if (this.encounter == null) 4059 this.encounter = new ArrayList<Reference>(); 4060 this.encounter.add(t); 4061 return this; 4062 } 4063 4064 /** 4065 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist 4066 */ 4067 public Reference getEncounterFirstRep() { 4068 if (getEncounter().isEmpty()) { 4069 addEncounter(); 4070 } 4071 return getEncounter().get(0); 4072 } 4073 4074 /** 4075 * @deprecated Use Reference#setResource(IBaseResource) instead 4076 */ 4077 @Deprecated 4078 public List<Encounter> getEncounterTarget() { 4079 if (this.encounterTarget == null) 4080 this.encounterTarget = new ArrayList<Encounter>(); 4081 return this.encounterTarget; 4082 } 4083 4084 /** 4085 * @deprecated Use Reference#setResource(IBaseResource) instead 4086 */ 4087 @Deprecated 4088 public Encounter addEncounterTarget() { 4089 Encounter r = new Encounter(); 4090 if (this.encounterTarget == null) 4091 this.encounterTarget = new ArrayList<Encounter>(); 4092 this.encounterTarget.add(r); 4093 return r; 4094 } 4095 4096 /** 4097 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 4098 */ 4099 public List<PositiveIntType> getNoteNumber() { 4100 if (this.noteNumber == null) 4101 this.noteNumber = new ArrayList<PositiveIntType>(); 4102 return this.noteNumber; 4103 } 4104 4105 /** 4106 * @return Returns a reference to <code>this</code> for easy method chaining 4107 */ 4108 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 4109 this.noteNumber = theNoteNumber; 4110 return this; 4111 } 4112 4113 public boolean hasNoteNumber() { 4114 if (this.noteNumber == null) 4115 return false; 4116 for (PositiveIntType item : this.noteNumber) 4117 if (!item.isEmpty()) 4118 return true; 4119 return false; 4120 } 4121 4122 /** 4123 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 4124 */ 4125 public PositiveIntType addNoteNumberElement() {//2 4126 PositiveIntType t = new PositiveIntType(); 4127 if (this.noteNumber == null) 4128 this.noteNumber = new ArrayList<PositiveIntType>(); 4129 this.noteNumber.add(t); 4130 return t; 4131 } 4132 4133 /** 4134 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 4135 */ 4136 public ItemComponent addNoteNumber(int value) { //1 4137 PositiveIntType t = new PositiveIntType(); 4138 t.setValue(value); 4139 if (this.noteNumber == null) 4140 this.noteNumber = new ArrayList<PositiveIntType>(); 4141 this.noteNumber.add(t); 4142 return this; 4143 } 4144 4145 /** 4146 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 4147 */ 4148 public boolean hasNoteNumber(int value) { 4149 if (this.noteNumber == null) 4150 return false; 4151 for (PositiveIntType v : this.noteNumber) 4152 if (v.getValue().equals(value)) // positiveInt 4153 return true; 4154 return false; 4155 } 4156 4157 /** 4158 * @return {@link #adjudication} (The adjudications results.) 4159 */ 4160 public List<AdjudicationComponent> getAdjudication() { 4161 if (this.adjudication == null) 4162 this.adjudication = new ArrayList<AdjudicationComponent>(); 4163 return this.adjudication; 4164 } 4165 4166 /** 4167 * @return Returns a reference to <code>this</code> for easy method chaining 4168 */ 4169 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 4170 this.adjudication = theAdjudication; 4171 return this; 4172 } 4173 4174 public boolean hasAdjudication() { 4175 if (this.adjudication == null) 4176 return false; 4177 for (AdjudicationComponent item : this.adjudication) 4178 if (!item.isEmpty()) 4179 return true; 4180 return false; 4181 } 4182 4183 public AdjudicationComponent addAdjudication() { //3 4184 AdjudicationComponent t = new AdjudicationComponent(); 4185 if (this.adjudication == null) 4186 this.adjudication = new ArrayList<AdjudicationComponent>(); 4187 this.adjudication.add(t); 4188 return t; 4189 } 4190 4191 public ItemComponent addAdjudication(AdjudicationComponent t) { //3 4192 if (t == null) 4193 return this; 4194 if (this.adjudication == null) 4195 this.adjudication = new ArrayList<AdjudicationComponent>(); 4196 this.adjudication.add(t); 4197 return this; 4198 } 4199 4200 /** 4201 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 4202 */ 4203 public AdjudicationComponent getAdjudicationFirstRep() { 4204 if (getAdjudication().isEmpty()) { 4205 addAdjudication(); 4206 } 4207 return getAdjudication().get(0); 4208 } 4209 4210 /** 4211 * @return {@link #detail} (Second tier of goods and services.) 4212 */ 4213 public List<DetailComponent> getDetail() { 4214 if (this.detail == null) 4215 this.detail = new ArrayList<DetailComponent>(); 4216 return this.detail; 4217 } 4218 4219 /** 4220 * @return Returns a reference to <code>this</code> for easy method chaining 4221 */ 4222 public ItemComponent setDetail(List<DetailComponent> theDetail) { 4223 this.detail = theDetail; 4224 return this; 4225 } 4226 4227 public boolean hasDetail() { 4228 if (this.detail == null) 4229 return false; 4230 for (DetailComponent item : this.detail) 4231 if (!item.isEmpty()) 4232 return true; 4233 return false; 4234 } 4235 4236 public DetailComponent addDetail() { //3 4237 DetailComponent t = new DetailComponent(); 4238 if (this.detail == null) 4239 this.detail = new ArrayList<DetailComponent>(); 4240 this.detail.add(t); 4241 return t; 4242 } 4243 4244 public ItemComponent addDetail(DetailComponent t) { //3 4245 if (t == null) 4246 return this; 4247 if (this.detail == null) 4248 this.detail = new ArrayList<DetailComponent>(); 4249 this.detail.add(t); 4250 return this; 4251 } 4252 4253 /** 4254 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 4255 */ 4256 public DetailComponent getDetailFirstRep() { 4257 if (getDetail().isEmpty()) { 4258 addDetail(); 4259 } 4260 return getDetail().get(0); 4261 } 4262 4263 protected void listChildren(List<Property> children) { 4264 super.listChildren(children); 4265 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 4266 children.add(new Property("careTeamLinkId", "positiveInt", "Careteam applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, careTeamLinkId)); 4267 children.add(new Property("diagnosisLinkId", "positiveInt", "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId)); 4268 children.add(new Property("procedureLinkId", "positiveInt", "Procedures applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, procedureLinkId)); 4269 children.add(new Property("informationLinkId", "positiveInt", "Exceptions, special conditions and supporting information pplicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, informationLinkId)); 4270 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 4271 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 4272 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 4273 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 4274 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 4275 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 4276 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location)); 4277 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 4278 children.add(new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice)); 4279 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 4280 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 4281 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 4282 children.add(new Property("bodySite", "CodeableConcept", "Physical service site on the patient (limb, tooth, etc).", 0, 1, bodySite)); 4283 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the site, eg. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 4284 children.add(new Property("encounter", "Reference(Encounter)", "A billed item may include goods or services provided in multiple encounters.", 0, java.lang.Integer.MAX_VALUE, encounter)); 4285 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 4286 children.add(new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 4287 children.add(new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail)); 4288 } 4289 4290 @Override 4291 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4292 switch (_hash) { 4293 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 4294 case -186757789: /*careTeamLinkId*/ return new Property("careTeamLinkId", "positiveInt", "Careteam applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, careTeamLinkId); 4295 case -1659207418: /*diagnosisLinkId*/ return new Property("diagnosisLinkId", "positiveInt", "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId); 4296 case -532846744: /*procedureLinkId*/ return new Property("procedureLinkId", "positiveInt", "Procedures applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, procedureLinkId); 4297 case 1965585153: /*informationLinkId*/ return new Property("informationLinkId", "positiveInt", "Exceptions, special conditions and supporting information pplicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, informationLinkId); 4298 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 4299 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 4300 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 4301 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 4302 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 4303 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4304 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4305 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4306 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4307 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4308 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4309 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4310 case -1280020865: /*locationAddress*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4311 case 755866390: /*locationReference*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4312 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 4313 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice); 4314 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 4315 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 4316 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 4317 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Physical service site on the patient (limb, tooth, etc).", 0, 1, bodySite); 4318 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the site, eg. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 4319 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "A billed item may include goods or services provided in multiple encounters.", 0, java.lang.Integer.MAX_VALUE, encounter); 4320 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 4321 case -231349275: /*adjudication*/ return new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 4322 case -1335224239: /*detail*/ return new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail); 4323 default: return super.getNamedProperty(_hash, _name, _checkValid); 4324 } 4325 4326 } 4327 4328 @Override 4329 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4330 switch (hash) { 4331 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 4332 case -186757789: /*careTeamLinkId*/ return this.careTeamLinkId == null ? new Base[0] : this.careTeamLinkId.toArray(new Base[this.careTeamLinkId.size()]); // PositiveIntType 4333 case -1659207418: /*diagnosisLinkId*/ return this.diagnosisLinkId == null ? new Base[0] : this.diagnosisLinkId.toArray(new Base[this.diagnosisLinkId.size()]); // PositiveIntType 4334 case -532846744: /*procedureLinkId*/ return this.procedureLinkId == null ? new Base[0] : this.procedureLinkId.toArray(new Base[this.procedureLinkId.size()]); // PositiveIntType 4335 case 1965585153: /*informationLinkId*/ return this.informationLinkId == null ? new Base[0] : this.informationLinkId.toArray(new Base[this.informationLinkId.size()]); // PositiveIntType 4336 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 4337 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 4338 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 4339 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 4340 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 4341 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // Type 4342 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Type 4343 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 4344 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 4345 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 4346 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 4347 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 4348 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 4349 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 4350 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 4351 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 4352 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 4353 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 4354 default: return super.getProperty(hash, name, checkValid); 4355 } 4356 4357 } 4358 4359 @Override 4360 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4361 switch (hash) { 4362 case 1349547969: // sequence 4363 this.sequence = castToPositiveInt(value); // PositiveIntType 4364 return value; 4365 case -186757789: // careTeamLinkId 4366 this.getCareTeamLinkId().add(castToPositiveInt(value)); // PositiveIntType 4367 return value; 4368 case -1659207418: // diagnosisLinkId 4369 this.getDiagnosisLinkId().add(castToPositiveInt(value)); // PositiveIntType 4370 return value; 4371 case -532846744: // procedureLinkId 4372 this.getProcedureLinkId().add(castToPositiveInt(value)); // PositiveIntType 4373 return value; 4374 case 1965585153: // informationLinkId 4375 this.getInformationLinkId().add(castToPositiveInt(value)); // PositiveIntType 4376 return value; 4377 case 1099842588: // revenue 4378 this.revenue = castToCodeableConcept(value); // CodeableConcept 4379 return value; 4380 case 50511102: // category 4381 this.category = castToCodeableConcept(value); // CodeableConcept 4382 return value; 4383 case 1984153269: // service 4384 this.service = castToCodeableConcept(value); // CodeableConcept 4385 return value; 4386 case -615513385: // modifier 4387 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 4388 return value; 4389 case 1010065041: // programCode 4390 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 4391 return value; 4392 case 1379209295: // serviced 4393 this.serviced = castToType(value); // Type 4394 return value; 4395 case 1901043637: // location 4396 this.location = castToType(value); // Type 4397 return value; 4398 case -1285004149: // quantity 4399 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4400 return value; 4401 case -486196699: // unitPrice 4402 this.unitPrice = castToMoney(value); // Money 4403 return value; 4404 case -1282148017: // factor 4405 this.factor = castToDecimal(value); // DecimalType 4406 return value; 4407 case 108957: // net 4408 this.net = castToMoney(value); // Money 4409 return value; 4410 case 115642: // udi 4411 this.getUdi().add(castToReference(value)); // Reference 4412 return value; 4413 case 1702620169: // bodySite 4414 this.bodySite = castToCodeableConcept(value); // CodeableConcept 4415 return value; 4416 case -1868566105: // subSite 4417 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 4418 return value; 4419 case 1524132147: // encounter 4420 this.getEncounter().add(castToReference(value)); // Reference 4421 return value; 4422 case -1110033957: // noteNumber 4423 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 4424 return value; 4425 case -231349275: // adjudication 4426 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 4427 return value; 4428 case -1335224239: // detail 4429 this.getDetail().add((DetailComponent) value); // DetailComponent 4430 return value; 4431 default: return super.setProperty(hash, name, value); 4432 } 4433 4434 } 4435 4436 @Override 4437 public Base setProperty(String name, Base value) throws FHIRException { 4438 if (name.equals("sequence")) { 4439 this.sequence = castToPositiveInt(value); // PositiveIntType 4440 } else if (name.equals("careTeamLinkId")) { 4441 this.getCareTeamLinkId().add(castToPositiveInt(value)); 4442 } else if (name.equals("diagnosisLinkId")) { 4443 this.getDiagnosisLinkId().add(castToPositiveInt(value)); 4444 } else if (name.equals("procedureLinkId")) { 4445 this.getProcedureLinkId().add(castToPositiveInt(value)); 4446 } else if (name.equals("informationLinkId")) { 4447 this.getInformationLinkId().add(castToPositiveInt(value)); 4448 } else if (name.equals("revenue")) { 4449 this.revenue = castToCodeableConcept(value); // CodeableConcept 4450 } else if (name.equals("category")) { 4451 this.category = castToCodeableConcept(value); // CodeableConcept 4452 } else if (name.equals("service")) { 4453 this.service = castToCodeableConcept(value); // CodeableConcept 4454 } else if (name.equals("modifier")) { 4455 this.getModifier().add(castToCodeableConcept(value)); 4456 } else if (name.equals("programCode")) { 4457 this.getProgramCode().add(castToCodeableConcept(value)); 4458 } else if (name.equals("serviced[x]")) { 4459 this.serviced = castToType(value); // Type 4460 } else if (name.equals("location[x]")) { 4461 this.location = castToType(value); // Type 4462 } else if (name.equals("quantity")) { 4463 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4464 } else if (name.equals("unitPrice")) { 4465 this.unitPrice = castToMoney(value); // Money 4466 } else if (name.equals("factor")) { 4467 this.factor = castToDecimal(value); // DecimalType 4468 } else if (name.equals("net")) { 4469 this.net = castToMoney(value); // Money 4470 } else if (name.equals("udi")) { 4471 this.getUdi().add(castToReference(value)); 4472 } else if (name.equals("bodySite")) { 4473 this.bodySite = castToCodeableConcept(value); // CodeableConcept 4474 } else if (name.equals("subSite")) { 4475 this.getSubSite().add(castToCodeableConcept(value)); 4476 } else if (name.equals("encounter")) { 4477 this.getEncounter().add(castToReference(value)); 4478 } else if (name.equals("noteNumber")) { 4479 this.getNoteNumber().add(castToPositiveInt(value)); 4480 } else if (name.equals("adjudication")) { 4481 this.getAdjudication().add((AdjudicationComponent) value); 4482 } else if (name.equals("detail")) { 4483 this.getDetail().add((DetailComponent) value); 4484 } else 4485 return super.setProperty(name, value); 4486 return value; 4487 } 4488 4489 @Override 4490 public Base makeProperty(int hash, String name) throws FHIRException { 4491 switch (hash) { 4492 case 1349547969: return getSequenceElement(); 4493 case -186757789: return addCareTeamLinkIdElement(); 4494 case -1659207418: return addDiagnosisLinkIdElement(); 4495 case -532846744: return addProcedureLinkIdElement(); 4496 case 1965585153: return addInformationLinkIdElement(); 4497 case 1099842588: return getRevenue(); 4498 case 50511102: return getCategory(); 4499 case 1984153269: return getService(); 4500 case -615513385: return addModifier(); 4501 case 1010065041: return addProgramCode(); 4502 case -1927922223: return getServiced(); 4503 case 1379209295: return getServiced(); 4504 case 552316075: return getLocation(); 4505 case 1901043637: return getLocation(); 4506 case -1285004149: return getQuantity(); 4507 case -486196699: return getUnitPrice(); 4508 case -1282148017: return getFactorElement(); 4509 case 108957: return getNet(); 4510 case 115642: return addUdi(); 4511 case 1702620169: return getBodySite(); 4512 case -1868566105: return addSubSite(); 4513 case 1524132147: return addEncounter(); 4514 case -1110033957: return addNoteNumberElement(); 4515 case -231349275: return addAdjudication(); 4516 case -1335224239: return addDetail(); 4517 default: return super.makeProperty(hash, name); 4518 } 4519 4520 } 4521 4522 @Override 4523 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4524 switch (hash) { 4525 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 4526 case -186757789: /*careTeamLinkId*/ return new String[] {"positiveInt"}; 4527 case -1659207418: /*diagnosisLinkId*/ return new String[] {"positiveInt"}; 4528 case -532846744: /*procedureLinkId*/ return new String[] {"positiveInt"}; 4529 case 1965585153: /*informationLinkId*/ return new String[] {"positiveInt"}; 4530 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 4531 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 4532 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 4533 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 4534 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 4535 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 4536 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 4537 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 4538 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 4539 case -1282148017: /*factor*/ return new String[] {"decimal"}; 4540 case 108957: /*net*/ return new String[] {"Money"}; 4541 case 115642: /*udi*/ return new String[] {"Reference"}; 4542 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 4543 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 4544 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 4545 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 4546 case -231349275: /*adjudication*/ return new String[] {}; 4547 case -1335224239: /*detail*/ return new String[] {}; 4548 default: return super.getTypesForProperty(hash, name); 4549 } 4550 4551 } 4552 4553 @Override 4554 public Base addChild(String name) throws FHIRException { 4555 if (name.equals("sequence")) { 4556 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 4557 } 4558 else if (name.equals("careTeamLinkId")) { 4559 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.careTeamLinkId"); 4560 } 4561 else if (name.equals("diagnosisLinkId")) { 4562 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.diagnosisLinkId"); 4563 } 4564 else if (name.equals("procedureLinkId")) { 4565 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.procedureLinkId"); 4566 } 4567 else if (name.equals("informationLinkId")) { 4568 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.informationLinkId"); 4569 } 4570 else if (name.equals("revenue")) { 4571 this.revenue = new CodeableConcept(); 4572 return this.revenue; 4573 } 4574 else if (name.equals("category")) { 4575 this.category = new CodeableConcept(); 4576 return this.category; 4577 } 4578 else if (name.equals("service")) { 4579 this.service = new CodeableConcept(); 4580 return this.service; 4581 } 4582 else if (name.equals("modifier")) { 4583 return addModifier(); 4584 } 4585 else if (name.equals("programCode")) { 4586 return addProgramCode(); 4587 } 4588 else if (name.equals("servicedDate")) { 4589 this.serviced = new DateType(); 4590 return this.serviced; 4591 } 4592 else if (name.equals("servicedPeriod")) { 4593 this.serviced = new Period(); 4594 return this.serviced; 4595 } 4596 else if (name.equals("locationCodeableConcept")) { 4597 this.location = new CodeableConcept(); 4598 return this.location; 4599 } 4600 else if (name.equals("locationAddress")) { 4601 this.location = new Address(); 4602 return this.location; 4603 } 4604 else if (name.equals("locationReference")) { 4605 this.location = new Reference(); 4606 return this.location; 4607 } 4608 else if (name.equals("quantity")) { 4609 this.quantity = new SimpleQuantity(); 4610 return this.quantity; 4611 } 4612 else if (name.equals("unitPrice")) { 4613 this.unitPrice = new Money(); 4614 return this.unitPrice; 4615 } 4616 else if (name.equals("factor")) { 4617 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 4618 } 4619 else if (name.equals("net")) { 4620 this.net = new Money(); 4621 return this.net; 4622 } 4623 else if (name.equals("udi")) { 4624 return addUdi(); 4625 } 4626 else if (name.equals("bodySite")) { 4627 this.bodySite = new CodeableConcept(); 4628 return this.bodySite; 4629 } 4630 else if (name.equals("subSite")) { 4631 return addSubSite(); 4632 } 4633 else if (name.equals("encounter")) { 4634 return addEncounter(); 4635 } 4636 else if (name.equals("noteNumber")) { 4637 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 4638 } 4639 else if (name.equals("adjudication")) { 4640 return addAdjudication(); 4641 } 4642 else if (name.equals("detail")) { 4643 return addDetail(); 4644 } 4645 else 4646 return super.addChild(name); 4647 } 4648 4649 public ItemComponent copy() { 4650 ItemComponent dst = new ItemComponent(); 4651 copyValues(dst); 4652 dst.sequence = sequence == null ? null : sequence.copy(); 4653 if (careTeamLinkId != null) { 4654 dst.careTeamLinkId = new ArrayList<PositiveIntType>(); 4655 for (PositiveIntType i : careTeamLinkId) 4656 dst.careTeamLinkId.add(i.copy()); 4657 }; 4658 if (diagnosisLinkId != null) { 4659 dst.diagnosisLinkId = new ArrayList<PositiveIntType>(); 4660 for (PositiveIntType i : diagnosisLinkId) 4661 dst.diagnosisLinkId.add(i.copy()); 4662 }; 4663 if (procedureLinkId != null) { 4664 dst.procedureLinkId = new ArrayList<PositiveIntType>(); 4665 for (PositiveIntType i : procedureLinkId) 4666 dst.procedureLinkId.add(i.copy()); 4667 }; 4668 if (informationLinkId != null) { 4669 dst.informationLinkId = new ArrayList<PositiveIntType>(); 4670 for (PositiveIntType i : informationLinkId) 4671 dst.informationLinkId.add(i.copy()); 4672 }; 4673 dst.revenue = revenue == null ? null : revenue.copy(); 4674 dst.category = category == null ? null : category.copy(); 4675 dst.service = service == null ? null : service.copy(); 4676 if (modifier != null) { 4677 dst.modifier = new ArrayList<CodeableConcept>(); 4678 for (CodeableConcept i : modifier) 4679 dst.modifier.add(i.copy()); 4680 }; 4681 if (programCode != null) { 4682 dst.programCode = new ArrayList<CodeableConcept>(); 4683 for (CodeableConcept i : programCode) 4684 dst.programCode.add(i.copy()); 4685 }; 4686 dst.serviced = serviced == null ? null : serviced.copy(); 4687 dst.location = location == null ? null : location.copy(); 4688 dst.quantity = quantity == null ? null : quantity.copy(); 4689 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4690 dst.factor = factor == null ? null : factor.copy(); 4691 dst.net = net == null ? null : net.copy(); 4692 if (udi != null) { 4693 dst.udi = new ArrayList<Reference>(); 4694 for (Reference i : udi) 4695 dst.udi.add(i.copy()); 4696 }; 4697 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4698 if (subSite != null) { 4699 dst.subSite = new ArrayList<CodeableConcept>(); 4700 for (CodeableConcept i : subSite) 4701 dst.subSite.add(i.copy()); 4702 }; 4703 if (encounter != null) { 4704 dst.encounter = new ArrayList<Reference>(); 4705 for (Reference i : encounter) 4706 dst.encounter.add(i.copy()); 4707 }; 4708 if (noteNumber != null) { 4709 dst.noteNumber = new ArrayList<PositiveIntType>(); 4710 for (PositiveIntType i : noteNumber) 4711 dst.noteNumber.add(i.copy()); 4712 }; 4713 if (adjudication != null) { 4714 dst.adjudication = new ArrayList<AdjudicationComponent>(); 4715 for (AdjudicationComponent i : adjudication) 4716 dst.adjudication.add(i.copy()); 4717 }; 4718 if (detail != null) { 4719 dst.detail = new ArrayList<DetailComponent>(); 4720 for (DetailComponent i : detail) 4721 dst.detail.add(i.copy()); 4722 }; 4723 return dst; 4724 } 4725 4726 @Override 4727 public boolean equalsDeep(Base other_) { 4728 if (!super.equalsDeep(other_)) 4729 return false; 4730 if (!(other_ instanceof ItemComponent)) 4731 return false; 4732 ItemComponent o = (ItemComponent) other_; 4733 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamLinkId, o.careTeamLinkId, true) 4734 && compareDeep(diagnosisLinkId, o.diagnosisLinkId, true) && compareDeep(procedureLinkId, o.procedureLinkId, true) 4735 && compareDeep(informationLinkId, o.informationLinkId, true) && compareDeep(revenue, o.revenue, true) 4736 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 4737 && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) && compareDeep(location, o.location, true) 4738 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 4739 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 4740 && compareDeep(subSite, o.subSite, true) && compareDeep(encounter, o.encounter, true) && compareDeep(noteNumber, o.noteNumber, true) 4741 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 4742 } 4743 4744 @Override 4745 public boolean equalsShallow(Base other_) { 4746 if (!super.equalsShallow(other_)) 4747 return false; 4748 if (!(other_ instanceof ItemComponent)) 4749 return false; 4750 ItemComponent o = (ItemComponent) other_; 4751 return compareValues(sequence, o.sequence, true) && compareValues(careTeamLinkId, o.careTeamLinkId, true) 4752 && compareValues(diagnosisLinkId, o.diagnosisLinkId, true) && compareValues(procedureLinkId, o.procedureLinkId, true) 4753 && compareValues(informationLinkId, o.informationLinkId, true) && compareValues(factor, o.factor, true) 4754 && compareValues(noteNumber, o.noteNumber, true); 4755 } 4756 4757 public boolean isEmpty() { 4758 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamLinkId, diagnosisLinkId 4759 , procedureLinkId, informationLinkId, revenue, category, service, modifier, programCode 4760 , serviced, location, quantity, unitPrice, factor, net, udi, bodySite, subSite 4761 , encounter, noteNumber, adjudication, detail); 4762 } 4763 4764 public String fhirType() { 4765 return "ExplanationOfBenefit.item"; 4766 4767 } 4768 4769 } 4770 4771 @Block() 4772 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 4773 /** 4774 * Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc. 4775 */ 4776 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 4777 @Description(shortDefinition="Adjudication category such as co-pay, eligible, benefit, etc.", formalDefinition="Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc." ) 4778 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 4779 protected CodeableConcept category; 4780 4781 /** 4782 * Adjudication reason such as limit reached. 4783 */ 4784 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 4785 @Description(shortDefinition="Explanation of Adjudication outcome", formalDefinition="Adjudication reason such as limit reached." ) 4786 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-reason") 4787 protected CodeableConcept reason; 4788 4789 /** 4790 * Monitory amount associated with the code. 4791 */ 4792 @Child(name = "amount", type = {Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 4793 @Description(shortDefinition="Monetary amount", formalDefinition="Monitory amount associated with the code." ) 4794 protected Money amount; 4795 4796 /** 4797 * A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4798 */ 4799 @Child(name = "value", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 4800 @Description(shortDefinition="Non-monitory value", formalDefinition="A non-monetary value for example a percentage. Mutually exclusive to the amount element above." ) 4801 protected DecimalType value; 4802 4803 private static final long serialVersionUID = 1559898786L; 4804 4805 /** 4806 * Constructor 4807 */ 4808 public AdjudicationComponent() { 4809 super(); 4810 } 4811 4812 /** 4813 * Constructor 4814 */ 4815 public AdjudicationComponent(CodeableConcept category) { 4816 super(); 4817 this.category = category; 4818 } 4819 4820 /** 4821 * @return {@link #category} (Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc.) 4822 */ 4823 public CodeableConcept getCategory() { 4824 if (this.category == null) 4825 if (Configuration.errorOnAutoCreate()) 4826 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 4827 else if (Configuration.doAutoCreate()) 4828 this.category = new CodeableConcept(); // cc 4829 return this.category; 4830 } 4831 4832 public boolean hasCategory() { 4833 return this.category != null && !this.category.isEmpty(); 4834 } 4835 4836 /** 4837 * @param value {@link #category} (Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc.) 4838 */ 4839 public AdjudicationComponent setCategory(CodeableConcept value) { 4840 this.category = value; 4841 return this; 4842 } 4843 4844 /** 4845 * @return {@link #reason} (Adjudication reason such as limit reached.) 4846 */ 4847 public CodeableConcept getReason() { 4848 if (this.reason == null) 4849 if (Configuration.errorOnAutoCreate()) 4850 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 4851 else if (Configuration.doAutoCreate()) 4852 this.reason = new CodeableConcept(); // cc 4853 return this.reason; 4854 } 4855 4856 public boolean hasReason() { 4857 return this.reason != null && !this.reason.isEmpty(); 4858 } 4859 4860 /** 4861 * @param value {@link #reason} (Adjudication reason such as limit reached.) 4862 */ 4863 public AdjudicationComponent setReason(CodeableConcept value) { 4864 this.reason = value; 4865 return this; 4866 } 4867 4868 /** 4869 * @return {@link #amount} (Monitory amount associated with the code.) 4870 */ 4871 public Money getAmount() { 4872 if (this.amount == null) 4873 if (Configuration.errorOnAutoCreate()) 4874 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 4875 else if (Configuration.doAutoCreate()) 4876 this.amount = new Money(); // cc 4877 return this.amount; 4878 } 4879 4880 public boolean hasAmount() { 4881 return this.amount != null && !this.amount.isEmpty(); 4882 } 4883 4884 /** 4885 * @param value {@link #amount} (Monitory amount associated with the code.) 4886 */ 4887 public AdjudicationComponent setAmount(Money value) { 4888 this.amount = value; 4889 return this; 4890 } 4891 4892 /** 4893 * @return {@link #value} (A non-monetary value for example a percentage. Mutually exclusive to the amount element above.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 4894 */ 4895 public DecimalType getValueElement() { 4896 if (this.value == null) 4897 if (Configuration.errorOnAutoCreate()) 4898 throw new Error("Attempt to auto-create AdjudicationComponent.value"); 4899 else if (Configuration.doAutoCreate()) 4900 this.value = new DecimalType(); // bb 4901 return this.value; 4902 } 4903 4904 public boolean hasValueElement() { 4905 return this.value != null && !this.value.isEmpty(); 4906 } 4907 4908 public boolean hasValue() { 4909 return this.value != null && !this.value.isEmpty(); 4910 } 4911 4912 /** 4913 * @param value {@link #value} (A non-monetary value for example a percentage. Mutually exclusive to the amount element above.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 4914 */ 4915 public AdjudicationComponent setValueElement(DecimalType value) { 4916 this.value = value; 4917 return this; 4918 } 4919 4920 /** 4921 * @return A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4922 */ 4923 public BigDecimal getValue() { 4924 return this.value == null ? null : this.value.getValue(); 4925 } 4926 4927 /** 4928 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4929 */ 4930 public AdjudicationComponent setValue(BigDecimal value) { 4931 if (value == null) 4932 this.value = null; 4933 else { 4934 if (this.value == null) 4935 this.value = new DecimalType(); 4936 this.value.setValue(value); 4937 } 4938 return this; 4939 } 4940 4941 /** 4942 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4943 */ 4944 public AdjudicationComponent setValue(long value) { 4945 this.value = new DecimalType(); 4946 this.value.setValue(value); 4947 return this; 4948 } 4949 4950 /** 4951 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4952 */ 4953 public AdjudicationComponent setValue(double value) { 4954 this.value = new DecimalType(); 4955 this.value.setValue(value); 4956 return this; 4957 } 4958 4959 protected void listChildren(List<Property> children) { 4960 super.listChildren(children); 4961 children.add(new Property("category", "CodeableConcept", "Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc.", 0, 1, category)); 4962 children.add(new Property("reason", "CodeableConcept", "Adjudication reason such as limit reached.", 0, 1, reason)); 4963 children.add(new Property("amount", "Money", "Monitory amount associated with the code.", 0, 1, amount)); 4964 children.add(new Property("value", "decimal", "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 1, value)); 4965 } 4966 4967 @Override 4968 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4969 switch (_hash) { 4970 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc.", 0, 1, category); 4971 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Adjudication reason such as limit reached.", 0, 1, reason); 4972 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monitory amount associated with the code.", 0, 1, amount); 4973 case 111972721: /*value*/ return new Property("value", "decimal", "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 1, value); 4974 default: return super.getNamedProperty(_hash, _name, _checkValid); 4975 } 4976 4977 } 4978 4979 @Override 4980 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4981 switch (hash) { 4982 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 4983 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 4984 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 4985 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DecimalType 4986 default: return super.getProperty(hash, name, checkValid); 4987 } 4988 4989 } 4990 4991 @Override 4992 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4993 switch (hash) { 4994 case 50511102: // category 4995 this.category = castToCodeableConcept(value); // CodeableConcept 4996 return value; 4997 case -934964668: // reason 4998 this.reason = castToCodeableConcept(value); // CodeableConcept 4999 return value; 5000 case -1413853096: // amount 5001 this.amount = castToMoney(value); // Money 5002 return value; 5003 case 111972721: // value 5004 this.value = castToDecimal(value); // DecimalType 5005 return value; 5006 default: return super.setProperty(hash, name, value); 5007 } 5008 5009 } 5010 5011 @Override 5012 public Base setProperty(String name, Base value) throws FHIRException { 5013 if (name.equals("category")) { 5014 this.category = castToCodeableConcept(value); // CodeableConcept 5015 } else if (name.equals("reason")) { 5016 this.reason = castToCodeableConcept(value); // CodeableConcept 5017 } else if (name.equals("amount")) { 5018 this.amount = castToMoney(value); // Money 5019 } else if (name.equals("value")) { 5020 this.value = castToDecimal(value); // DecimalType 5021 } else 5022 return super.setProperty(name, value); 5023 return value; 5024 } 5025 5026 @Override 5027 public Base makeProperty(int hash, String name) throws FHIRException { 5028 switch (hash) { 5029 case 50511102: return getCategory(); 5030 case -934964668: return getReason(); 5031 case -1413853096: return getAmount(); 5032 case 111972721: return getValueElement(); 5033 default: return super.makeProperty(hash, name); 5034 } 5035 5036 } 5037 5038 @Override 5039 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5040 switch (hash) { 5041 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 5042 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 5043 case -1413853096: /*amount*/ return new String[] {"Money"}; 5044 case 111972721: /*value*/ return new String[] {"decimal"}; 5045 default: return super.getTypesForProperty(hash, name); 5046 } 5047 5048 } 5049 5050 @Override 5051 public Base addChild(String name) throws FHIRException { 5052 if (name.equals("category")) { 5053 this.category = new CodeableConcept(); 5054 return this.category; 5055 } 5056 else if (name.equals("reason")) { 5057 this.reason = new CodeableConcept(); 5058 return this.reason; 5059 } 5060 else if (name.equals("amount")) { 5061 this.amount = new Money(); 5062 return this.amount; 5063 } 5064 else if (name.equals("value")) { 5065 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.value"); 5066 } 5067 else 5068 return super.addChild(name); 5069 } 5070 5071 public AdjudicationComponent copy() { 5072 AdjudicationComponent dst = new AdjudicationComponent(); 5073 copyValues(dst); 5074 dst.category = category == null ? null : category.copy(); 5075 dst.reason = reason == null ? null : reason.copy(); 5076 dst.amount = amount == null ? null : amount.copy(); 5077 dst.value = value == null ? null : value.copy(); 5078 return dst; 5079 } 5080 5081 @Override 5082 public boolean equalsDeep(Base other_) { 5083 if (!super.equalsDeep(other_)) 5084 return false; 5085 if (!(other_ instanceof AdjudicationComponent)) 5086 return false; 5087 AdjudicationComponent o = (AdjudicationComponent) other_; 5088 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) && compareDeep(amount, o.amount, true) 5089 && compareDeep(value, o.value, true); 5090 } 5091 5092 @Override 5093 public boolean equalsShallow(Base other_) { 5094 if (!super.equalsShallow(other_)) 5095 return false; 5096 if (!(other_ instanceof AdjudicationComponent)) 5097 return false; 5098 AdjudicationComponent o = (AdjudicationComponent) other_; 5099 return compareValues(value, o.value, true); 5100 } 5101 5102 public boolean isEmpty() { 5103 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount 5104 , value); 5105 } 5106 5107 public String fhirType() { 5108 return "ExplanationOfBenefit.item.adjudication"; 5109 5110 } 5111 5112 } 5113 5114 @Block() 5115 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 5116 /** 5117 * A service line number. 5118 */ 5119 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5120 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 5121 protected PositiveIntType sequence; 5122 5123 /** 5124 * The type of product or service. 5125 */ 5126 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 5127 @Description(shortDefinition="Group or type of product or service", formalDefinition="The type of product or service." ) 5128 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActInvoiceGroupCode") 5129 protected CodeableConcept type; 5130 5131 /** 5132 * The type of reveneu or cost center providing the product and/or service. 5133 */ 5134 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5135 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 5136 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5137 protected CodeableConcept revenue; 5138 5139 /** 5140 * Health Care Service Type Codes to identify the classification of service or benefits. 5141 */ 5142 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5143 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 5144 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 5145 protected CodeableConcept category; 5146 5147 /** 5148 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 5149 */ 5150 @Child(name = "service", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 5151 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 5152 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5153 protected CodeableConcept service; 5154 5155 /** 5156 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 5157 */ 5158 @Child(name = "modifier", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5159 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 5160 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5161 protected List<CodeableConcept> modifier; 5162 5163 /** 5164 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 5165 */ 5166 @Child(name = "programCode", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5167 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 5168 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 5169 protected List<CodeableConcept> programCode; 5170 5171 /** 5172 * The number of repetitions of a service or product. 5173 */ 5174 @Child(name = "quantity", type = {SimpleQuantity.class}, order=8, min=0, max=1, modifier=false, summary=false) 5175 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 5176 protected SimpleQuantity quantity; 5177 5178 /** 5179 * If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group. 5180 */ 5181 @Child(name = "unitPrice", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 5182 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group." ) 5183 protected Money unitPrice; 5184 5185 /** 5186 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5187 */ 5188 @Child(name = "factor", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 5189 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5190 protected DecimalType factor; 5191 5192 /** 5193 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 5194 */ 5195 @Child(name = "net", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 5196 @Description(shortDefinition="Total additional item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 5197 protected Money net; 5198 5199 /** 5200 * List of Unique Device Identifiers associated with this line item. 5201 */ 5202 @Child(name = "udi", type = {Device.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5203 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 5204 protected List<Reference> udi; 5205 /** 5206 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 5207 */ 5208 protected List<Device> udiTarget; 5209 5210 5211 /** 5212 * A list of note references to the notes provided below. 5213 */ 5214 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5215 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 5216 protected List<PositiveIntType> noteNumber; 5217 5218 /** 5219 * The adjudications results. 5220 */ 5221 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5222 @Description(shortDefinition="Detail level adjudication details", formalDefinition="The adjudications results." ) 5223 protected List<AdjudicationComponent> adjudication; 5224 5225 /** 5226 * Third tier of goods and services. 5227 */ 5228 @Child(name = "subDetail", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5229 @Description(shortDefinition="Additional items", formalDefinition="Third tier of goods and services." ) 5230 protected List<SubDetailComponent> subDetail; 5231 5232 private static final long serialVersionUID = -276371489L; 5233 5234 /** 5235 * Constructor 5236 */ 5237 public DetailComponent() { 5238 super(); 5239 } 5240 5241 /** 5242 * Constructor 5243 */ 5244 public DetailComponent(PositiveIntType sequence, CodeableConcept type) { 5245 super(); 5246 this.sequence = sequence; 5247 this.type = type; 5248 } 5249 5250 /** 5251 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5252 */ 5253 public PositiveIntType getSequenceElement() { 5254 if (this.sequence == null) 5255 if (Configuration.errorOnAutoCreate()) 5256 throw new Error("Attempt to auto-create DetailComponent.sequence"); 5257 else if (Configuration.doAutoCreate()) 5258 this.sequence = new PositiveIntType(); // bb 5259 return this.sequence; 5260 } 5261 5262 public boolean hasSequenceElement() { 5263 return this.sequence != null && !this.sequence.isEmpty(); 5264 } 5265 5266 public boolean hasSequence() { 5267 return this.sequence != null && !this.sequence.isEmpty(); 5268 } 5269 5270 /** 5271 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5272 */ 5273 public DetailComponent setSequenceElement(PositiveIntType value) { 5274 this.sequence = value; 5275 return this; 5276 } 5277 5278 /** 5279 * @return A service line number. 5280 */ 5281 public int getSequence() { 5282 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 5283 } 5284 5285 /** 5286 * @param value A service line number. 5287 */ 5288 public DetailComponent setSequence(int value) { 5289 if (this.sequence == null) 5290 this.sequence = new PositiveIntType(); 5291 this.sequence.setValue(value); 5292 return this; 5293 } 5294 5295 /** 5296 * @return {@link #type} (The type of product or service.) 5297 */ 5298 public CodeableConcept getType() { 5299 if (this.type == null) 5300 if (Configuration.errorOnAutoCreate()) 5301 throw new Error("Attempt to auto-create DetailComponent.type"); 5302 else if (Configuration.doAutoCreate()) 5303 this.type = new CodeableConcept(); // cc 5304 return this.type; 5305 } 5306 5307 public boolean hasType() { 5308 return this.type != null && !this.type.isEmpty(); 5309 } 5310 5311 /** 5312 * @param value {@link #type} (The type of product or service.) 5313 */ 5314 public DetailComponent setType(CodeableConcept value) { 5315 this.type = value; 5316 return this; 5317 } 5318 5319 /** 5320 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 5321 */ 5322 public CodeableConcept getRevenue() { 5323 if (this.revenue == null) 5324 if (Configuration.errorOnAutoCreate()) 5325 throw new Error("Attempt to auto-create DetailComponent.revenue"); 5326 else if (Configuration.doAutoCreate()) 5327 this.revenue = new CodeableConcept(); // cc 5328 return this.revenue; 5329 } 5330 5331 public boolean hasRevenue() { 5332 return this.revenue != null && !this.revenue.isEmpty(); 5333 } 5334 5335 /** 5336 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 5337 */ 5338 public DetailComponent setRevenue(CodeableConcept value) { 5339 this.revenue = value; 5340 return this; 5341 } 5342 5343 /** 5344 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 5345 */ 5346 public CodeableConcept getCategory() { 5347 if (this.category == null) 5348 if (Configuration.errorOnAutoCreate()) 5349 throw new Error("Attempt to auto-create DetailComponent.category"); 5350 else if (Configuration.doAutoCreate()) 5351 this.category = new CodeableConcept(); // cc 5352 return this.category; 5353 } 5354 5355 public boolean hasCategory() { 5356 return this.category != null && !this.category.isEmpty(); 5357 } 5358 5359 /** 5360 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 5361 */ 5362 public DetailComponent setCategory(CodeableConcept value) { 5363 this.category = value; 5364 return this; 5365 } 5366 5367 /** 5368 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 5369 */ 5370 public CodeableConcept getService() { 5371 if (this.service == null) 5372 if (Configuration.errorOnAutoCreate()) 5373 throw new Error("Attempt to auto-create DetailComponent.service"); 5374 else if (Configuration.doAutoCreate()) 5375 this.service = new CodeableConcept(); // cc 5376 return this.service; 5377 } 5378 5379 public boolean hasService() { 5380 return this.service != null && !this.service.isEmpty(); 5381 } 5382 5383 /** 5384 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 5385 */ 5386 public DetailComponent setService(CodeableConcept value) { 5387 this.service = value; 5388 return this; 5389 } 5390 5391 /** 5392 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 5393 */ 5394 public List<CodeableConcept> getModifier() { 5395 if (this.modifier == null) 5396 this.modifier = new ArrayList<CodeableConcept>(); 5397 return this.modifier; 5398 } 5399 5400 /** 5401 * @return Returns a reference to <code>this</code> for easy method chaining 5402 */ 5403 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 5404 this.modifier = theModifier; 5405 return this; 5406 } 5407 5408 public boolean hasModifier() { 5409 if (this.modifier == null) 5410 return false; 5411 for (CodeableConcept item : this.modifier) 5412 if (!item.isEmpty()) 5413 return true; 5414 return false; 5415 } 5416 5417 public CodeableConcept addModifier() { //3 5418 CodeableConcept t = new CodeableConcept(); 5419 if (this.modifier == null) 5420 this.modifier = new ArrayList<CodeableConcept>(); 5421 this.modifier.add(t); 5422 return t; 5423 } 5424 5425 public DetailComponent addModifier(CodeableConcept t) { //3 5426 if (t == null) 5427 return this; 5428 if (this.modifier == null) 5429 this.modifier = new ArrayList<CodeableConcept>(); 5430 this.modifier.add(t); 5431 return this; 5432 } 5433 5434 /** 5435 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 5436 */ 5437 public CodeableConcept getModifierFirstRep() { 5438 if (getModifier().isEmpty()) { 5439 addModifier(); 5440 } 5441 return getModifier().get(0); 5442 } 5443 5444 /** 5445 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 5446 */ 5447 public List<CodeableConcept> getProgramCode() { 5448 if (this.programCode == null) 5449 this.programCode = new ArrayList<CodeableConcept>(); 5450 return this.programCode; 5451 } 5452 5453 /** 5454 * @return Returns a reference to <code>this</code> for easy method chaining 5455 */ 5456 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 5457 this.programCode = theProgramCode; 5458 return this; 5459 } 5460 5461 public boolean hasProgramCode() { 5462 if (this.programCode == null) 5463 return false; 5464 for (CodeableConcept item : this.programCode) 5465 if (!item.isEmpty()) 5466 return true; 5467 return false; 5468 } 5469 5470 public CodeableConcept addProgramCode() { //3 5471 CodeableConcept t = new CodeableConcept(); 5472 if (this.programCode == null) 5473 this.programCode = new ArrayList<CodeableConcept>(); 5474 this.programCode.add(t); 5475 return t; 5476 } 5477 5478 public DetailComponent addProgramCode(CodeableConcept t) { //3 5479 if (t == null) 5480 return this; 5481 if (this.programCode == null) 5482 this.programCode = new ArrayList<CodeableConcept>(); 5483 this.programCode.add(t); 5484 return this; 5485 } 5486 5487 /** 5488 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 5489 */ 5490 public CodeableConcept getProgramCodeFirstRep() { 5491 if (getProgramCode().isEmpty()) { 5492 addProgramCode(); 5493 } 5494 return getProgramCode().get(0); 5495 } 5496 5497 /** 5498 * @return {@link #quantity} (The number of repetitions of a service or product.) 5499 */ 5500 public SimpleQuantity getQuantity() { 5501 if (this.quantity == null) 5502 if (Configuration.errorOnAutoCreate()) 5503 throw new Error("Attempt to auto-create DetailComponent.quantity"); 5504 else if (Configuration.doAutoCreate()) 5505 this.quantity = new SimpleQuantity(); // cc 5506 return this.quantity; 5507 } 5508 5509 public boolean hasQuantity() { 5510 return this.quantity != null && !this.quantity.isEmpty(); 5511 } 5512 5513 /** 5514 * @param value {@link #quantity} (The number of repetitions of a service or product.) 5515 */ 5516 public DetailComponent setQuantity(SimpleQuantity value) { 5517 this.quantity = value; 5518 return this; 5519 } 5520 5521 /** 5522 * @return {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 5523 */ 5524 public Money getUnitPrice() { 5525 if (this.unitPrice == null) 5526 if (Configuration.errorOnAutoCreate()) 5527 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 5528 else if (Configuration.doAutoCreate()) 5529 this.unitPrice = new Money(); // cc 5530 return this.unitPrice; 5531 } 5532 5533 public boolean hasUnitPrice() { 5534 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5535 } 5536 5537 /** 5538 * @param value {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 5539 */ 5540 public DetailComponent setUnitPrice(Money value) { 5541 this.unitPrice = value; 5542 return this; 5543 } 5544 5545 /** 5546 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5547 */ 5548 public DecimalType getFactorElement() { 5549 if (this.factor == null) 5550 if (Configuration.errorOnAutoCreate()) 5551 throw new Error("Attempt to auto-create DetailComponent.factor"); 5552 else if (Configuration.doAutoCreate()) 5553 this.factor = new DecimalType(); // bb 5554 return this.factor; 5555 } 5556 5557 public boolean hasFactorElement() { 5558 return this.factor != null && !this.factor.isEmpty(); 5559 } 5560 5561 public boolean hasFactor() { 5562 return this.factor != null && !this.factor.isEmpty(); 5563 } 5564 5565 /** 5566 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5567 */ 5568 public DetailComponent setFactorElement(DecimalType value) { 5569 this.factor = value; 5570 return this; 5571 } 5572 5573 /** 5574 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5575 */ 5576 public BigDecimal getFactor() { 5577 return this.factor == null ? null : this.factor.getValue(); 5578 } 5579 5580 /** 5581 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5582 */ 5583 public DetailComponent setFactor(BigDecimal value) { 5584 if (value == null) 5585 this.factor = null; 5586 else { 5587 if (this.factor == null) 5588 this.factor = new DecimalType(); 5589 this.factor.setValue(value); 5590 } 5591 return this; 5592 } 5593 5594 /** 5595 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5596 */ 5597 public DetailComponent setFactor(long value) { 5598 this.factor = new DecimalType(); 5599 this.factor.setValue(value); 5600 return this; 5601 } 5602 5603 /** 5604 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5605 */ 5606 public DetailComponent setFactor(double value) { 5607 this.factor = new DecimalType(); 5608 this.factor.setValue(value); 5609 return this; 5610 } 5611 5612 /** 5613 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 5614 */ 5615 public Money getNet() { 5616 if (this.net == null) 5617 if (Configuration.errorOnAutoCreate()) 5618 throw new Error("Attempt to auto-create DetailComponent.net"); 5619 else if (Configuration.doAutoCreate()) 5620 this.net = new Money(); // cc 5621 return this.net; 5622 } 5623 5624 public boolean hasNet() { 5625 return this.net != null && !this.net.isEmpty(); 5626 } 5627 5628 /** 5629 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 5630 */ 5631 public DetailComponent setNet(Money value) { 5632 this.net = value; 5633 return this; 5634 } 5635 5636 /** 5637 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 5638 */ 5639 public List<Reference> getUdi() { 5640 if (this.udi == null) 5641 this.udi = new ArrayList<Reference>(); 5642 return this.udi; 5643 } 5644 5645 /** 5646 * @return Returns a reference to <code>this</code> for easy method chaining 5647 */ 5648 public DetailComponent setUdi(List<Reference> theUdi) { 5649 this.udi = theUdi; 5650 return this; 5651 } 5652 5653 public boolean hasUdi() { 5654 if (this.udi == null) 5655 return false; 5656 for (Reference item : this.udi) 5657 if (!item.isEmpty()) 5658 return true; 5659 return false; 5660 } 5661 5662 public Reference addUdi() { //3 5663 Reference t = new Reference(); 5664 if (this.udi == null) 5665 this.udi = new ArrayList<Reference>(); 5666 this.udi.add(t); 5667 return t; 5668 } 5669 5670 public DetailComponent addUdi(Reference t) { //3 5671 if (t == null) 5672 return this; 5673 if (this.udi == null) 5674 this.udi = new ArrayList<Reference>(); 5675 this.udi.add(t); 5676 return this; 5677 } 5678 5679 /** 5680 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 5681 */ 5682 public Reference getUdiFirstRep() { 5683 if (getUdi().isEmpty()) { 5684 addUdi(); 5685 } 5686 return getUdi().get(0); 5687 } 5688 5689 /** 5690 * @deprecated Use Reference#setResource(IBaseResource) instead 5691 */ 5692 @Deprecated 5693 public List<Device> getUdiTarget() { 5694 if (this.udiTarget == null) 5695 this.udiTarget = new ArrayList<Device>(); 5696 return this.udiTarget; 5697 } 5698 5699 /** 5700 * @deprecated Use Reference#setResource(IBaseResource) instead 5701 */ 5702 @Deprecated 5703 public Device addUdiTarget() { 5704 Device r = new Device(); 5705 if (this.udiTarget == null) 5706 this.udiTarget = new ArrayList<Device>(); 5707 this.udiTarget.add(r); 5708 return r; 5709 } 5710 5711 /** 5712 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 5713 */ 5714 public List<PositiveIntType> getNoteNumber() { 5715 if (this.noteNumber == null) 5716 this.noteNumber = new ArrayList<PositiveIntType>(); 5717 return this.noteNumber; 5718 } 5719 5720 /** 5721 * @return Returns a reference to <code>this</code> for easy method chaining 5722 */ 5723 public DetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 5724 this.noteNumber = theNoteNumber; 5725 return this; 5726 } 5727 5728 public boolean hasNoteNumber() { 5729 if (this.noteNumber == null) 5730 return false; 5731 for (PositiveIntType item : this.noteNumber) 5732 if (!item.isEmpty()) 5733 return true; 5734 return false; 5735 } 5736 5737 /** 5738 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 5739 */ 5740 public PositiveIntType addNoteNumberElement() {//2 5741 PositiveIntType t = new PositiveIntType(); 5742 if (this.noteNumber == null) 5743 this.noteNumber = new ArrayList<PositiveIntType>(); 5744 this.noteNumber.add(t); 5745 return t; 5746 } 5747 5748 /** 5749 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 5750 */ 5751 public DetailComponent addNoteNumber(int value) { //1 5752 PositiveIntType t = new PositiveIntType(); 5753 t.setValue(value); 5754 if (this.noteNumber == null) 5755 this.noteNumber = new ArrayList<PositiveIntType>(); 5756 this.noteNumber.add(t); 5757 return this; 5758 } 5759 5760 /** 5761 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 5762 */ 5763 public boolean hasNoteNumber(int value) { 5764 if (this.noteNumber == null) 5765 return false; 5766 for (PositiveIntType v : this.noteNumber) 5767 if (v.getValue().equals(value)) // positiveInt 5768 return true; 5769 return false; 5770 } 5771 5772 /** 5773 * @return {@link #adjudication} (The adjudications results.) 5774 */ 5775 public List<AdjudicationComponent> getAdjudication() { 5776 if (this.adjudication == null) 5777 this.adjudication = new ArrayList<AdjudicationComponent>(); 5778 return this.adjudication; 5779 } 5780 5781 /** 5782 * @return Returns a reference to <code>this</code> for easy method chaining 5783 */ 5784 public DetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 5785 this.adjudication = theAdjudication; 5786 return this; 5787 } 5788 5789 public boolean hasAdjudication() { 5790 if (this.adjudication == null) 5791 return false; 5792 for (AdjudicationComponent item : this.adjudication) 5793 if (!item.isEmpty()) 5794 return true; 5795 return false; 5796 } 5797 5798 public AdjudicationComponent addAdjudication() { //3 5799 AdjudicationComponent t = new AdjudicationComponent(); 5800 if (this.adjudication == null) 5801 this.adjudication = new ArrayList<AdjudicationComponent>(); 5802 this.adjudication.add(t); 5803 return t; 5804 } 5805 5806 public DetailComponent addAdjudication(AdjudicationComponent t) { //3 5807 if (t == null) 5808 return this; 5809 if (this.adjudication == null) 5810 this.adjudication = new ArrayList<AdjudicationComponent>(); 5811 this.adjudication.add(t); 5812 return this; 5813 } 5814 5815 /** 5816 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 5817 */ 5818 public AdjudicationComponent getAdjudicationFirstRep() { 5819 if (getAdjudication().isEmpty()) { 5820 addAdjudication(); 5821 } 5822 return getAdjudication().get(0); 5823 } 5824 5825 /** 5826 * @return {@link #subDetail} (Third tier of goods and services.) 5827 */ 5828 public List<SubDetailComponent> getSubDetail() { 5829 if (this.subDetail == null) 5830 this.subDetail = new ArrayList<SubDetailComponent>(); 5831 return this.subDetail; 5832 } 5833 5834 /** 5835 * @return Returns a reference to <code>this</code> for easy method chaining 5836 */ 5837 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 5838 this.subDetail = theSubDetail; 5839 return this; 5840 } 5841 5842 public boolean hasSubDetail() { 5843 if (this.subDetail == null) 5844 return false; 5845 for (SubDetailComponent item : this.subDetail) 5846 if (!item.isEmpty()) 5847 return true; 5848 return false; 5849 } 5850 5851 public SubDetailComponent addSubDetail() { //3 5852 SubDetailComponent t = new SubDetailComponent(); 5853 if (this.subDetail == null) 5854 this.subDetail = new ArrayList<SubDetailComponent>(); 5855 this.subDetail.add(t); 5856 return t; 5857 } 5858 5859 public DetailComponent addSubDetail(SubDetailComponent t) { //3 5860 if (t == null) 5861 return this; 5862 if (this.subDetail == null) 5863 this.subDetail = new ArrayList<SubDetailComponent>(); 5864 this.subDetail.add(t); 5865 return this; 5866 } 5867 5868 /** 5869 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist 5870 */ 5871 public SubDetailComponent getSubDetailFirstRep() { 5872 if (getSubDetail().isEmpty()) { 5873 addSubDetail(); 5874 } 5875 return getSubDetail().get(0); 5876 } 5877 5878 protected void listChildren(List<Property> children) { 5879 super.listChildren(children); 5880 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 5881 children.add(new Property("type", "CodeableConcept", "The type of product or service.", 0, 1, type)); 5882 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 5883 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 5884 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 5885 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 5886 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 5887 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 5888 children.add(new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice)); 5889 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 5890 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 5891 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 5892 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 5893 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 5894 children.add(new Property("subDetail", "", "Third tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 5895 } 5896 5897 @Override 5898 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5899 switch (_hash) { 5900 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 5901 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of product or service.", 0, 1, type); 5902 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 5903 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 5904 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 5905 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 5906 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 5907 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 5908 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice); 5909 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 5910 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 5911 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 5912 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 5913 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 5914 case -828829007: /*subDetail*/ return new Property("subDetail", "", "Third tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 5915 default: return super.getNamedProperty(_hash, _name, _checkValid); 5916 } 5917 5918 } 5919 5920 @Override 5921 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5922 switch (hash) { 5923 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 5924 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 5925 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 5926 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 5927 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 5928 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5929 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5930 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 5931 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 5932 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 5933 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 5934 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5935 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 5936 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 5937 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 5938 default: return super.getProperty(hash, name, checkValid); 5939 } 5940 5941 } 5942 5943 @Override 5944 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5945 switch (hash) { 5946 case 1349547969: // sequence 5947 this.sequence = castToPositiveInt(value); // PositiveIntType 5948 return value; 5949 case 3575610: // type 5950 this.type = castToCodeableConcept(value); // CodeableConcept 5951 return value; 5952 case 1099842588: // revenue 5953 this.revenue = castToCodeableConcept(value); // CodeableConcept 5954 return value; 5955 case 50511102: // category 5956 this.category = castToCodeableConcept(value); // CodeableConcept 5957 return value; 5958 case 1984153269: // service 5959 this.service = castToCodeableConcept(value); // CodeableConcept 5960 return value; 5961 case -615513385: // modifier 5962 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5963 return value; 5964 case 1010065041: // programCode 5965 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 5966 return value; 5967 case -1285004149: // quantity 5968 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 5969 return value; 5970 case -486196699: // unitPrice 5971 this.unitPrice = castToMoney(value); // Money 5972 return value; 5973 case -1282148017: // factor 5974 this.factor = castToDecimal(value); // DecimalType 5975 return value; 5976 case 108957: // net 5977 this.net = castToMoney(value); // Money 5978 return value; 5979 case 115642: // udi 5980 this.getUdi().add(castToReference(value)); // Reference 5981 return value; 5982 case -1110033957: // noteNumber 5983 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 5984 return value; 5985 case -231349275: // adjudication 5986 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 5987 return value; 5988 case -828829007: // subDetail 5989 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 5990 return value; 5991 default: return super.setProperty(hash, name, value); 5992 } 5993 5994 } 5995 5996 @Override 5997 public Base setProperty(String name, Base value) throws FHIRException { 5998 if (name.equals("sequence")) { 5999 this.sequence = castToPositiveInt(value); // PositiveIntType 6000 } else if (name.equals("type")) { 6001 this.type = castToCodeableConcept(value); // CodeableConcept 6002 } else if (name.equals("revenue")) { 6003 this.revenue = castToCodeableConcept(value); // CodeableConcept 6004 } else if (name.equals("category")) { 6005 this.category = castToCodeableConcept(value); // CodeableConcept 6006 } else if (name.equals("service")) { 6007 this.service = castToCodeableConcept(value); // CodeableConcept 6008 } else if (name.equals("modifier")) { 6009 this.getModifier().add(castToCodeableConcept(value)); 6010 } else if (name.equals("programCode")) { 6011 this.getProgramCode().add(castToCodeableConcept(value)); 6012 } else if (name.equals("quantity")) { 6013 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 6014 } else if (name.equals("unitPrice")) { 6015 this.unitPrice = castToMoney(value); // Money 6016 } else if (name.equals("factor")) { 6017 this.factor = castToDecimal(value); // DecimalType 6018 } else if (name.equals("net")) { 6019 this.net = castToMoney(value); // Money 6020 } else if (name.equals("udi")) { 6021 this.getUdi().add(castToReference(value)); 6022 } else if (name.equals("noteNumber")) { 6023 this.getNoteNumber().add(castToPositiveInt(value)); 6024 } else if (name.equals("adjudication")) { 6025 this.getAdjudication().add((AdjudicationComponent) value); 6026 } else if (name.equals("subDetail")) { 6027 this.getSubDetail().add((SubDetailComponent) value); 6028 } else 6029 return super.setProperty(name, value); 6030 return value; 6031 } 6032 6033 @Override 6034 public Base makeProperty(int hash, String name) throws FHIRException { 6035 switch (hash) { 6036 case 1349547969: return getSequenceElement(); 6037 case 3575610: return getType(); 6038 case 1099842588: return getRevenue(); 6039 case 50511102: return getCategory(); 6040 case 1984153269: return getService(); 6041 case -615513385: return addModifier(); 6042 case 1010065041: return addProgramCode(); 6043 case -1285004149: return getQuantity(); 6044 case -486196699: return getUnitPrice(); 6045 case -1282148017: return getFactorElement(); 6046 case 108957: return getNet(); 6047 case 115642: return addUdi(); 6048 case -1110033957: return addNoteNumberElement(); 6049 case -231349275: return addAdjudication(); 6050 case -828829007: return addSubDetail(); 6051 default: return super.makeProperty(hash, name); 6052 } 6053 6054 } 6055 6056 @Override 6057 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6058 switch (hash) { 6059 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 6060 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 6061 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 6062 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6063 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 6064 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 6065 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 6066 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 6067 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 6068 case -1282148017: /*factor*/ return new String[] {"decimal"}; 6069 case 108957: /*net*/ return new String[] {"Money"}; 6070 case 115642: /*udi*/ return new String[] {"Reference"}; 6071 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 6072 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 6073 case -828829007: /*subDetail*/ return new String[] {}; 6074 default: return super.getTypesForProperty(hash, name); 6075 } 6076 6077 } 6078 6079 @Override 6080 public Base addChild(String name) throws FHIRException { 6081 if (name.equals("sequence")) { 6082 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 6083 } 6084 else if (name.equals("type")) { 6085 this.type = new CodeableConcept(); 6086 return this.type; 6087 } 6088 else if (name.equals("revenue")) { 6089 this.revenue = new CodeableConcept(); 6090 return this.revenue; 6091 } 6092 else if (name.equals("category")) { 6093 this.category = new CodeableConcept(); 6094 return this.category; 6095 } 6096 else if (name.equals("service")) { 6097 this.service = new CodeableConcept(); 6098 return this.service; 6099 } 6100 else if (name.equals("modifier")) { 6101 return addModifier(); 6102 } 6103 else if (name.equals("programCode")) { 6104 return addProgramCode(); 6105 } 6106 else if (name.equals("quantity")) { 6107 this.quantity = new SimpleQuantity(); 6108 return this.quantity; 6109 } 6110 else if (name.equals("unitPrice")) { 6111 this.unitPrice = new Money(); 6112 return this.unitPrice; 6113 } 6114 else if (name.equals("factor")) { 6115 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 6116 } 6117 else if (name.equals("net")) { 6118 this.net = new Money(); 6119 return this.net; 6120 } 6121 else if (name.equals("udi")) { 6122 return addUdi(); 6123 } 6124 else if (name.equals("noteNumber")) { 6125 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 6126 } 6127 else if (name.equals("adjudication")) { 6128 return addAdjudication(); 6129 } 6130 else if (name.equals("subDetail")) { 6131 return addSubDetail(); 6132 } 6133 else 6134 return super.addChild(name); 6135 } 6136 6137 public DetailComponent copy() { 6138 DetailComponent dst = new DetailComponent(); 6139 copyValues(dst); 6140 dst.sequence = sequence == null ? null : sequence.copy(); 6141 dst.type = type == null ? null : type.copy(); 6142 dst.revenue = revenue == null ? null : revenue.copy(); 6143 dst.category = category == null ? null : category.copy(); 6144 dst.service = service == null ? null : service.copy(); 6145 if (modifier != null) { 6146 dst.modifier = new ArrayList<CodeableConcept>(); 6147 for (CodeableConcept i : modifier) 6148 dst.modifier.add(i.copy()); 6149 }; 6150 if (programCode != null) { 6151 dst.programCode = new ArrayList<CodeableConcept>(); 6152 for (CodeableConcept i : programCode) 6153 dst.programCode.add(i.copy()); 6154 }; 6155 dst.quantity = quantity == null ? null : quantity.copy(); 6156 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6157 dst.factor = factor == null ? null : factor.copy(); 6158 dst.net = net == null ? null : net.copy(); 6159 if (udi != null) { 6160 dst.udi = new ArrayList<Reference>(); 6161 for (Reference i : udi) 6162 dst.udi.add(i.copy()); 6163 }; 6164 if (noteNumber != null) { 6165 dst.noteNumber = new ArrayList<PositiveIntType>(); 6166 for (PositiveIntType i : noteNumber) 6167 dst.noteNumber.add(i.copy()); 6168 }; 6169 if (adjudication != null) { 6170 dst.adjudication = new ArrayList<AdjudicationComponent>(); 6171 for (AdjudicationComponent i : adjudication) 6172 dst.adjudication.add(i.copy()); 6173 }; 6174 if (subDetail != null) { 6175 dst.subDetail = new ArrayList<SubDetailComponent>(); 6176 for (SubDetailComponent i : subDetail) 6177 dst.subDetail.add(i.copy()); 6178 }; 6179 return dst; 6180 } 6181 6182 @Override 6183 public boolean equalsDeep(Base other_) { 6184 if (!super.equalsDeep(other_)) 6185 return false; 6186 if (!(other_ instanceof DetailComponent)) 6187 return false; 6188 DetailComponent o = (DetailComponent) other_; 6189 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) && compareDeep(revenue, o.revenue, true) 6190 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 6191 && compareDeep(programCode, o.programCode, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 6192 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 6193 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 6194 && compareDeep(subDetail, o.subDetail, true); 6195 } 6196 6197 @Override 6198 public boolean equalsShallow(Base other_) { 6199 if (!super.equalsShallow(other_)) 6200 return false; 6201 if (!(other_ instanceof DetailComponent)) 6202 return false; 6203 DetailComponent o = (DetailComponent) other_; 6204 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true) 6205 ; 6206 } 6207 6208 public boolean isEmpty() { 6209 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, revenue 6210 , category, service, modifier, programCode, quantity, unitPrice, factor, net 6211 , udi, noteNumber, adjudication, subDetail); 6212 } 6213 6214 public String fhirType() { 6215 return "ExplanationOfBenefit.item.detail"; 6216 6217 } 6218 6219 } 6220 6221 @Block() 6222 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 6223 /** 6224 * A service line number. 6225 */ 6226 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 6227 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 6228 protected PositiveIntType sequence; 6229 6230 /** 6231 * The type of product or service. 6232 */ 6233 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 6234 @Description(shortDefinition="Type of product or service", formalDefinition="The type of product or service." ) 6235 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActInvoiceGroupCode") 6236 protected CodeableConcept type; 6237 6238 /** 6239 * The type of reveneu or cost center providing the product and/or service. 6240 */ 6241 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 6242 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 6243 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 6244 protected CodeableConcept revenue; 6245 6246 /** 6247 * Health Care Service Type Codes to identify the classification of service or benefits. 6248 */ 6249 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 6250 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 6251 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 6252 protected CodeableConcept category; 6253 6254 /** 6255 * A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). 6256 */ 6257 @Child(name = "service", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 6258 @Description(shortDefinition="Billing Code", formalDefinition="A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI)." ) 6259 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6260 protected CodeableConcept service; 6261 6262 /** 6263 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 6264 */ 6265 @Child(name = "modifier", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6266 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 6267 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 6268 protected List<CodeableConcept> modifier; 6269 6270 /** 6271 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 6272 */ 6273 @Child(name = "programCode", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6274 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 6275 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 6276 protected List<CodeableConcept> programCode; 6277 6278 /** 6279 * The number of repetitions of a service or product. 6280 */ 6281 @Child(name = "quantity", type = {SimpleQuantity.class}, order=8, min=0, max=1, modifier=false, summary=false) 6282 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 6283 protected SimpleQuantity quantity; 6284 6285 /** 6286 * The fee for an addittional service or product or charge. 6287 */ 6288 @Child(name = "unitPrice", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 6289 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="The fee for an addittional service or product or charge." ) 6290 protected Money unitPrice; 6291 6292 /** 6293 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6294 */ 6295 @Child(name = "factor", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 6296 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 6297 protected DecimalType factor; 6298 6299 /** 6300 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 6301 */ 6302 @Child(name = "net", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 6303 @Description(shortDefinition="Net additional item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 6304 protected Money net; 6305 6306 /** 6307 * List of Unique Device Identifiers associated with this line item. 6308 */ 6309 @Child(name = "udi", type = {Device.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6310 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 6311 protected List<Reference> udi; 6312 /** 6313 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 6314 */ 6315 protected List<Device> udiTarget; 6316 6317 6318 /** 6319 * A list of note references to the notes provided below. 6320 */ 6321 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6322 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 6323 protected List<PositiveIntType> noteNumber; 6324 6325 /** 6326 * The adjudications results. 6327 */ 6328 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6329 @Description(shortDefinition="Language if different from the resource", formalDefinition="The adjudications results." ) 6330 protected List<AdjudicationComponent> adjudication; 6331 6332 private static final long serialVersionUID = 1621872130L; 6333 6334 /** 6335 * Constructor 6336 */ 6337 public SubDetailComponent() { 6338 super(); 6339 } 6340 6341 /** 6342 * Constructor 6343 */ 6344 public SubDetailComponent(PositiveIntType sequence, CodeableConcept type) { 6345 super(); 6346 this.sequence = sequence; 6347 this.type = type; 6348 } 6349 6350 /** 6351 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6352 */ 6353 public PositiveIntType getSequenceElement() { 6354 if (this.sequence == null) 6355 if (Configuration.errorOnAutoCreate()) 6356 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 6357 else if (Configuration.doAutoCreate()) 6358 this.sequence = new PositiveIntType(); // bb 6359 return this.sequence; 6360 } 6361 6362 public boolean hasSequenceElement() { 6363 return this.sequence != null && !this.sequence.isEmpty(); 6364 } 6365 6366 public boolean hasSequence() { 6367 return this.sequence != null && !this.sequence.isEmpty(); 6368 } 6369 6370 /** 6371 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6372 */ 6373 public SubDetailComponent setSequenceElement(PositiveIntType value) { 6374 this.sequence = value; 6375 return this; 6376 } 6377 6378 /** 6379 * @return A service line number. 6380 */ 6381 public int getSequence() { 6382 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6383 } 6384 6385 /** 6386 * @param value A service line number. 6387 */ 6388 public SubDetailComponent setSequence(int value) { 6389 if (this.sequence == null) 6390 this.sequence = new PositiveIntType(); 6391 this.sequence.setValue(value); 6392 return this; 6393 } 6394 6395 /** 6396 * @return {@link #type} (The type of product or service.) 6397 */ 6398 public CodeableConcept getType() { 6399 if (this.type == null) 6400 if (Configuration.errorOnAutoCreate()) 6401 throw new Error("Attempt to auto-create SubDetailComponent.type"); 6402 else if (Configuration.doAutoCreate()) 6403 this.type = new CodeableConcept(); // cc 6404 return this.type; 6405 } 6406 6407 public boolean hasType() { 6408 return this.type != null && !this.type.isEmpty(); 6409 } 6410 6411 /** 6412 * @param value {@link #type} (The type of product or service.) 6413 */ 6414 public SubDetailComponent setType(CodeableConcept value) { 6415 this.type = value; 6416 return this; 6417 } 6418 6419 /** 6420 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 6421 */ 6422 public CodeableConcept getRevenue() { 6423 if (this.revenue == null) 6424 if (Configuration.errorOnAutoCreate()) 6425 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 6426 else if (Configuration.doAutoCreate()) 6427 this.revenue = new CodeableConcept(); // cc 6428 return this.revenue; 6429 } 6430 6431 public boolean hasRevenue() { 6432 return this.revenue != null && !this.revenue.isEmpty(); 6433 } 6434 6435 /** 6436 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 6437 */ 6438 public SubDetailComponent setRevenue(CodeableConcept value) { 6439 this.revenue = value; 6440 return this; 6441 } 6442 6443 /** 6444 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 6445 */ 6446 public CodeableConcept getCategory() { 6447 if (this.category == null) 6448 if (Configuration.errorOnAutoCreate()) 6449 throw new Error("Attempt to auto-create SubDetailComponent.category"); 6450 else if (Configuration.doAutoCreate()) 6451 this.category = new CodeableConcept(); // cc 6452 return this.category; 6453 } 6454 6455 public boolean hasCategory() { 6456 return this.category != null && !this.category.isEmpty(); 6457 } 6458 6459 /** 6460 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 6461 */ 6462 public SubDetailComponent setCategory(CodeableConcept value) { 6463 this.category = value; 6464 return this; 6465 } 6466 6467 /** 6468 * @return {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 6469 */ 6470 public CodeableConcept getService() { 6471 if (this.service == null) 6472 if (Configuration.errorOnAutoCreate()) 6473 throw new Error("Attempt to auto-create SubDetailComponent.service"); 6474 else if (Configuration.doAutoCreate()) 6475 this.service = new CodeableConcept(); // cc 6476 return this.service; 6477 } 6478 6479 public boolean hasService() { 6480 return this.service != null && !this.service.isEmpty(); 6481 } 6482 6483 /** 6484 * @param value {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 6485 */ 6486 public SubDetailComponent setService(CodeableConcept value) { 6487 this.service = value; 6488 return this; 6489 } 6490 6491 /** 6492 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 6493 */ 6494 public List<CodeableConcept> getModifier() { 6495 if (this.modifier == null) 6496 this.modifier = new ArrayList<CodeableConcept>(); 6497 return this.modifier; 6498 } 6499 6500 /** 6501 * @return Returns a reference to <code>this</code> for easy method chaining 6502 */ 6503 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 6504 this.modifier = theModifier; 6505 return this; 6506 } 6507 6508 public boolean hasModifier() { 6509 if (this.modifier == null) 6510 return false; 6511 for (CodeableConcept item : this.modifier) 6512 if (!item.isEmpty()) 6513 return true; 6514 return false; 6515 } 6516 6517 public CodeableConcept addModifier() { //3 6518 CodeableConcept t = new CodeableConcept(); 6519 if (this.modifier == null) 6520 this.modifier = new ArrayList<CodeableConcept>(); 6521 this.modifier.add(t); 6522 return t; 6523 } 6524 6525 public SubDetailComponent addModifier(CodeableConcept t) { //3 6526 if (t == null) 6527 return this; 6528 if (this.modifier == null) 6529 this.modifier = new ArrayList<CodeableConcept>(); 6530 this.modifier.add(t); 6531 return this; 6532 } 6533 6534 /** 6535 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 6536 */ 6537 public CodeableConcept getModifierFirstRep() { 6538 if (getModifier().isEmpty()) { 6539 addModifier(); 6540 } 6541 return getModifier().get(0); 6542 } 6543 6544 /** 6545 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 6546 */ 6547 public List<CodeableConcept> getProgramCode() { 6548 if (this.programCode == null) 6549 this.programCode = new ArrayList<CodeableConcept>(); 6550 return this.programCode; 6551 } 6552 6553 /** 6554 * @return Returns a reference to <code>this</code> for easy method chaining 6555 */ 6556 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6557 this.programCode = theProgramCode; 6558 return this; 6559 } 6560 6561 public boolean hasProgramCode() { 6562 if (this.programCode == null) 6563 return false; 6564 for (CodeableConcept item : this.programCode) 6565 if (!item.isEmpty()) 6566 return true; 6567 return false; 6568 } 6569 6570 public CodeableConcept addProgramCode() { //3 6571 CodeableConcept t = new CodeableConcept(); 6572 if (this.programCode == null) 6573 this.programCode = new ArrayList<CodeableConcept>(); 6574 this.programCode.add(t); 6575 return t; 6576 } 6577 6578 public SubDetailComponent addProgramCode(CodeableConcept t) { //3 6579 if (t == null) 6580 return this; 6581 if (this.programCode == null) 6582 this.programCode = new ArrayList<CodeableConcept>(); 6583 this.programCode.add(t); 6584 return this; 6585 } 6586 6587 /** 6588 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 6589 */ 6590 public CodeableConcept getProgramCodeFirstRep() { 6591 if (getProgramCode().isEmpty()) { 6592 addProgramCode(); 6593 } 6594 return getProgramCode().get(0); 6595 } 6596 6597 /** 6598 * @return {@link #quantity} (The number of repetitions of a service or product.) 6599 */ 6600 public SimpleQuantity getQuantity() { 6601 if (this.quantity == null) 6602 if (Configuration.errorOnAutoCreate()) 6603 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 6604 else if (Configuration.doAutoCreate()) 6605 this.quantity = new SimpleQuantity(); // cc 6606 return this.quantity; 6607 } 6608 6609 public boolean hasQuantity() { 6610 return this.quantity != null && !this.quantity.isEmpty(); 6611 } 6612 6613 /** 6614 * @param value {@link #quantity} (The number of repetitions of a service or product.) 6615 */ 6616 public SubDetailComponent setQuantity(SimpleQuantity value) { 6617 this.quantity = value; 6618 return this; 6619 } 6620 6621 /** 6622 * @return {@link #unitPrice} (The fee for an addittional service or product or charge.) 6623 */ 6624 public Money getUnitPrice() { 6625 if (this.unitPrice == null) 6626 if (Configuration.errorOnAutoCreate()) 6627 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 6628 else if (Configuration.doAutoCreate()) 6629 this.unitPrice = new Money(); // cc 6630 return this.unitPrice; 6631 } 6632 6633 public boolean hasUnitPrice() { 6634 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6635 } 6636 6637 /** 6638 * @param value {@link #unitPrice} (The fee for an addittional service or product or charge.) 6639 */ 6640 public SubDetailComponent setUnitPrice(Money value) { 6641 this.unitPrice = value; 6642 return this; 6643 } 6644 6645 /** 6646 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6647 */ 6648 public DecimalType getFactorElement() { 6649 if (this.factor == null) 6650 if (Configuration.errorOnAutoCreate()) 6651 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 6652 else if (Configuration.doAutoCreate()) 6653 this.factor = new DecimalType(); // bb 6654 return this.factor; 6655 } 6656 6657 public boolean hasFactorElement() { 6658 return this.factor != null && !this.factor.isEmpty(); 6659 } 6660 6661 public boolean hasFactor() { 6662 return this.factor != null && !this.factor.isEmpty(); 6663 } 6664 6665 /** 6666 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6667 */ 6668 public SubDetailComponent setFactorElement(DecimalType value) { 6669 this.factor = value; 6670 return this; 6671 } 6672 6673 /** 6674 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6675 */ 6676 public BigDecimal getFactor() { 6677 return this.factor == null ? null : this.factor.getValue(); 6678 } 6679 6680 /** 6681 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6682 */ 6683 public SubDetailComponent setFactor(BigDecimal value) { 6684 if (value == null) 6685 this.factor = null; 6686 else { 6687 if (this.factor == null) 6688 this.factor = new DecimalType(); 6689 this.factor.setValue(value); 6690 } 6691 return this; 6692 } 6693 6694 /** 6695 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6696 */ 6697 public SubDetailComponent setFactor(long value) { 6698 this.factor = new DecimalType(); 6699 this.factor.setValue(value); 6700 return this; 6701 } 6702 6703 /** 6704 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6705 */ 6706 public SubDetailComponent setFactor(double value) { 6707 this.factor = new DecimalType(); 6708 this.factor.setValue(value); 6709 return this; 6710 } 6711 6712 /** 6713 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6714 */ 6715 public Money getNet() { 6716 if (this.net == null) 6717 if (Configuration.errorOnAutoCreate()) 6718 throw new Error("Attempt to auto-create SubDetailComponent.net"); 6719 else if (Configuration.doAutoCreate()) 6720 this.net = new Money(); // cc 6721 return this.net; 6722 } 6723 6724 public boolean hasNet() { 6725 return this.net != null && !this.net.isEmpty(); 6726 } 6727 6728 /** 6729 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6730 */ 6731 public SubDetailComponent setNet(Money value) { 6732 this.net = value; 6733 return this; 6734 } 6735 6736 /** 6737 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 6738 */ 6739 public List<Reference> getUdi() { 6740 if (this.udi == null) 6741 this.udi = new ArrayList<Reference>(); 6742 return this.udi; 6743 } 6744 6745 /** 6746 * @return Returns a reference to <code>this</code> for easy method chaining 6747 */ 6748 public SubDetailComponent setUdi(List<Reference> theUdi) { 6749 this.udi = theUdi; 6750 return this; 6751 } 6752 6753 public boolean hasUdi() { 6754 if (this.udi == null) 6755 return false; 6756 for (Reference item : this.udi) 6757 if (!item.isEmpty()) 6758 return true; 6759 return false; 6760 } 6761 6762 public Reference addUdi() { //3 6763 Reference t = new Reference(); 6764 if (this.udi == null) 6765 this.udi = new ArrayList<Reference>(); 6766 this.udi.add(t); 6767 return t; 6768 } 6769 6770 public SubDetailComponent addUdi(Reference t) { //3 6771 if (t == null) 6772 return this; 6773 if (this.udi == null) 6774 this.udi = new ArrayList<Reference>(); 6775 this.udi.add(t); 6776 return this; 6777 } 6778 6779 /** 6780 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 6781 */ 6782 public Reference getUdiFirstRep() { 6783 if (getUdi().isEmpty()) { 6784 addUdi(); 6785 } 6786 return getUdi().get(0); 6787 } 6788 6789 /** 6790 * @deprecated Use Reference#setResource(IBaseResource) instead 6791 */ 6792 @Deprecated 6793 public List<Device> getUdiTarget() { 6794 if (this.udiTarget == null) 6795 this.udiTarget = new ArrayList<Device>(); 6796 return this.udiTarget; 6797 } 6798 6799 /** 6800 * @deprecated Use Reference#setResource(IBaseResource) instead 6801 */ 6802 @Deprecated 6803 public Device addUdiTarget() { 6804 Device r = new Device(); 6805 if (this.udiTarget == null) 6806 this.udiTarget = new ArrayList<Device>(); 6807 this.udiTarget.add(r); 6808 return r; 6809 } 6810 6811 /** 6812 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 6813 */ 6814 public List<PositiveIntType> getNoteNumber() { 6815 if (this.noteNumber == null) 6816 this.noteNumber = new ArrayList<PositiveIntType>(); 6817 return this.noteNumber; 6818 } 6819 6820 /** 6821 * @return Returns a reference to <code>this</code> for easy method chaining 6822 */ 6823 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 6824 this.noteNumber = theNoteNumber; 6825 return this; 6826 } 6827 6828 public boolean hasNoteNumber() { 6829 if (this.noteNumber == null) 6830 return false; 6831 for (PositiveIntType item : this.noteNumber) 6832 if (!item.isEmpty()) 6833 return true; 6834 return false; 6835 } 6836 6837 /** 6838 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 6839 */ 6840 public PositiveIntType addNoteNumberElement() {//2 6841 PositiveIntType t = new PositiveIntType(); 6842 if (this.noteNumber == null) 6843 this.noteNumber = new ArrayList<PositiveIntType>(); 6844 this.noteNumber.add(t); 6845 return t; 6846 } 6847 6848 /** 6849 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 6850 */ 6851 public SubDetailComponent addNoteNumber(int value) { //1 6852 PositiveIntType t = new PositiveIntType(); 6853 t.setValue(value); 6854 if (this.noteNumber == null) 6855 this.noteNumber = new ArrayList<PositiveIntType>(); 6856 this.noteNumber.add(t); 6857 return this; 6858 } 6859 6860 /** 6861 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 6862 */ 6863 public boolean hasNoteNumber(int value) { 6864 if (this.noteNumber == null) 6865 return false; 6866 for (PositiveIntType v : this.noteNumber) 6867 if (v.getValue().equals(value)) // positiveInt 6868 return true; 6869 return false; 6870 } 6871 6872 /** 6873 * @return {@link #adjudication} (The adjudications results.) 6874 */ 6875 public List<AdjudicationComponent> getAdjudication() { 6876 if (this.adjudication == null) 6877 this.adjudication = new ArrayList<AdjudicationComponent>(); 6878 return this.adjudication; 6879 } 6880 6881 /** 6882 * @return Returns a reference to <code>this</code> for easy method chaining 6883 */ 6884 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 6885 this.adjudication = theAdjudication; 6886 return this; 6887 } 6888 6889 public boolean hasAdjudication() { 6890 if (this.adjudication == null) 6891 return false; 6892 for (AdjudicationComponent item : this.adjudication) 6893 if (!item.isEmpty()) 6894 return true; 6895 return false; 6896 } 6897 6898 public AdjudicationComponent addAdjudication() { //3 6899 AdjudicationComponent t = new AdjudicationComponent(); 6900 if (this.adjudication == null) 6901 this.adjudication = new ArrayList<AdjudicationComponent>(); 6902 this.adjudication.add(t); 6903 return t; 6904 } 6905 6906 public SubDetailComponent addAdjudication(AdjudicationComponent t) { //3 6907 if (t == null) 6908 return this; 6909 if (this.adjudication == null) 6910 this.adjudication = new ArrayList<AdjudicationComponent>(); 6911 this.adjudication.add(t); 6912 return this; 6913 } 6914 6915 /** 6916 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 6917 */ 6918 public AdjudicationComponent getAdjudicationFirstRep() { 6919 if (getAdjudication().isEmpty()) { 6920 addAdjudication(); 6921 } 6922 return getAdjudication().get(0); 6923 } 6924 6925 protected void listChildren(List<Property> children) { 6926 super.listChildren(children); 6927 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 6928 children.add(new Property("type", "CodeableConcept", "The type of product or service.", 0, 1, type)); 6929 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 6930 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 6931 children.add(new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service)); 6932 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 6933 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 6934 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 6935 children.add(new Property("unitPrice", "Money", "The fee for an addittional service or product or charge.", 0, 1, unitPrice)); 6936 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 6937 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 6938 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 6939 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 6940 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 6941 } 6942 6943 @Override 6944 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6945 switch (_hash) { 6946 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 6947 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of product or service.", 0, 1, type); 6948 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 6949 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 6950 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service); 6951 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 6952 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 6953 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 6954 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "The fee for an addittional service or product or charge.", 0, 1, unitPrice); 6955 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 6956 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 6957 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 6958 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 6959 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 6960 default: return super.getNamedProperty(_hash, _name, _checkValid); 6961 } 6962 6963 } 6964 6965 @Override 6966 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6967 switch (hash) { 6968 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 6969 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 6970 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 6971 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6972 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 6973 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 6974 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 6975 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 6976 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 6977 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 6978 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 6979 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 6980 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 6981 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 6982 default: return super.getProperty(hash, name, checkValid); 6983 } 6984 6985 } 6986 6987 @Override 6988 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6989 switch (hash) { 6990 case 1349547969: // sequence 6991 this.sequence = castToPositiveInt(value); // PositiveIntType 6992 return value; 6993 case 3575610: // type 6994 this.type = castToCodeableConcept(value); // CodeableConcept 6995 return value; 6996 case 1099842588: // revenue 6997 this.revenue = castToCodeableConcept(value); // CodeableConcept 6998 return value; 6999 case 50511102: // category 7000 this.category = castToCodeableConcept(value); // CodeableConcept 7001 return value; 7002 case 1984153269: // service 7003 this.service = castToCodeableConcept(value); // CodeableConcept 7004 return value; 7005 case -615513385: // modifier 7006 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 7007 return value; 7008 case 1010065041: // programCode 7009 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 7010 return value; 7011 case -1285004149: // quantity 7012 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 7013 return value; 7014 case -486196699: // unitPrice 7015 this.unitPrice = castToMoney(value); // Money 7016 return value; 7017 case -1282148017: // factor 7018 this.factor = castToDecimal(value); // DecimalType 7019 return value; 7020 case 108957: // net 7021 this.net = castToMoney(value); // Money 7022 return value; 7023 case 115642: // udi 7024 this.getUdi().add(castToReference(value)); // Reference 7025 return value; 7026 case -1110033957: // noteNumber 7027 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 7028 return value; 7029 case -231349275: // adjudication 7030 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 7031 return value; 7032 default: return super.setProperty(hash, name, value); 7033 } 7034 7035 } 7036 7037 @Override 7038 public Base setProperty(String name, Base value) throws FHIRException { 7039 if (name.equals("sequence")) { 7040 this.sequence = castToPositiveInt(value); // PositiveIntType 7041 } else if (name.equals("type")) { 7042 this.type = castToCodeableConcept(value); // CodeableConcept 7043 } else if (name.equals("revenue")) { 7044 this.revenue = castToCodeableConcept(value); // CodeableConcept 7045 } else if (name.equals("category")) { 7046 this.category = castToCodeableConcept(value); // CodeableConcept 7047 } else if (name.equals("service")) { 7048 this.service = castToCodeableConcept(value); // CodeableConcept 7049 } else if (name.equals("modifier")) { 7050 this.getModifier().add(castToCodeableConcept(value)); 7051 } else if (name.equals("programCode")) { 7052 this.getProgramCode().add(castToCodeableConcept(value)); 7053 } else if (name.equals("quantity")) { 7054 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 7055 } else if (name.equals("unitPrice")) { 7056 this.unitPrice = castToMoney(value); // Money 7057 } else if (name.equals("factor")) { 7058 this.factor = castToDecimal(value); // DecimalType 7059 } else if (name.equals("net")) { 7060 this.net = castToMoney(value); // Money 7061 } else if (name.equals("udi")) { 7062 this.getUdi().add(castToReference(value)); 7063 } else if (name.equals("noteNumber")) { 7064 this.getNoteNumber().add(castToPositiveInt(value)); 7065 } else if (name.equals("adjudication")) { 7066 this.getAdjudication().add((AdjudicationComponent) value); 7067 } else 7068 return super.setProperty(name, value); 7069 return value; 7070 } 7071 7072 @Override 7073 public Base makeProperty(int hash, String name) throws FHIRException { 7074 switch (hash) { 7075 case 1349547969: return getSequenceElement(); 7076 case 3575610: return getType(); 7077 case 1099842588: return getRevenue(); 7078 case 50511102: return getCategory(); 7079 case 1984153269: return getService(); 7080 case -615513385: return addModifier(); 7081 case 1010065041: return addProgramCode(); 7082 case -1285004149: return getQuantity(); 7083 case -486196699: return getUnitPrice(); 7084 case -1282148017: return getFactorElement(); 7085 case 108957: return getNet(); 7086 case 115642: return addUdi(); 7087 case -1110033957: return addNoteNumberElement(); 7088 case -231349275: return addAdjudication(); 7089 default: return super.makeProperty(hash, name); 7090 } 7091 7092 } 7093 7094 @Override 7095 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7096 switch (hash) { 7097 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 7098 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 7099 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 7100 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 7101 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 7102 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 7103 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 7104 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 7105 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 7106 case -1282148017: /*factor*/ return new String[] {"decimal"}; 7107 case 108957: /*net*/ return new String[] {"Money"}; 7108 case 115642: /*udi*/ return new String[] {"Reference"}; 7109 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 7110 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 7111 default: return super.getTypesForProperty(hash, name); 7112 } 7113 7114 } 7115 7116 @Override 7117 public Base addChild(String name) throws FHIRException { 7118 if (name.equals("sequence")) { 7119 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 7120 } 7121 else if (name.equals("type")) { 7122 this.type = new CodeableConcept(); 7123 return this.type; 7124 } 7125 else if (name.equals("revenue")) { 7126 this.revenue = new CodeableConcept(); 7127 return this.revenue; 7128 } 7129 else if (name.equals("category")) { 7130 this.category = new CodeableConcept(); 7131 return this.category; 7132 } 7133 else if (name.equals("service")) { 7134 this.service = new CodeableConcept(); 7135 return this.service; 7136 } 7137 else if (name.equals("modifier")) { 7138 return addModifier(); 7139 } 7140 else if (name.equals("programCode")) { 7141 return addProgramCode(); 7142 } 7143 else if (name.equals("quantity")) { 7144 this.quantity = new SimpleQuantity(); 7145 return this.quantity; 7146 } 7147 else if (name.equals("unitPrice")) { 7148 this.unitPrice = new Money(); 7149 return this.unitPrice; 7150 } 7151 else if (name.equals("factor")) { 7152 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 7153 } 7154 else if (name.equals("net")) { 7155 this.net = new Money(); 7156 return this.net; 7157 } 7158 else if (name.equals("udi")) { 7159 return addUdi(); 7160 } 7161 else if (name.equals("noteNumber")) { 7162 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 7163 } 7164 else if (name.equals("adjudication")) { 7165 return addAdjudication(); 7166 } 7167 else 7168 return super.addChild(name); 7169 } 7170 7171 public SubDetailComponent copy() { 7172 SubDetailComponent dst = new SubDetailComponent(); 7173 copyValues(dst); 7174 dst.sequence = sequence == null ? null : sequence.copy(); 7175 dst.type = type == null ? null : type.copy(); 7176 dst.revenue = revenue == null ? null : revenue.copy(); 7177 dst.category = category == null ? null : category.copy(); 7178 dst.service = service == null ? null : service.copy(); 7179 if (modifier != null) { 7180 dst.modifier = new ArrayList<CodeableConcept>(); 7181 for (CodeableConcept i : modifier) 7182 dst.modifier.add(i.copy()); 7183 }; 7184 if (programCode != null) { 7185 dst.programCode = new ArrayList<CodeableConcept>(); 7186 for (CodeableConcept i : programCode) 7187 dst.programCode.add(i.copy()); 7188 }; 7189 dst.quantity = quantity == null ? null : quantity.copy(); 7190 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7191 dst.factor = factor == null ? null : factor.copy(); 7192 dst.net = net == null ? null : net.copy(); 7193 if (udi != null) { 7194 dst.udi = new ArrayList<Reference>(); 7195 for (Reference i : udi) 7196 dst.udi.add(i.copy()); 7197 }; 7198 if (noteNumber != null) { 7199 dst.noteNumber = new ArrayList<PositiveIntType>(); 7200 for (PositiveIntType i : noteNumber) 7201 dst.noteNumber.add(i.copy()); 7202 }; 7203 if (adjudication != null) { 7204 dst.adjudication = new ArrayList<AdjudicationComponent>(); 7205 for (AdjudicationComponent i : adjudication) 7206 dst.adjudication.add(i.copy()); 7207 }; 7208 return dst; 7209 } 7210 7211 @Override 7212 public boolean equalsDeep(Base other_) { 7213 if (!super.equalsDeep(other_)) 7214 return false; 7215 if (!(other_ instanceof SubDetailComponent)) 7216 return false; 7217 SubDetailComponent o = (SubDetailComponent) other_; 7218 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) && compareDeep(revenue, o.revenue, true) 7219 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 7220 && compareDeep(programCode, o.programCode, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 7221 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 7222 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 7223 ; 7224 } 7225 7226 @Override 7227 public boolean equalsShallow(Base other_) { 7228 if (!super.equalsShallow(other_)) 7229 return false; 7230 if (!(other_ instanceof SubDetailComponent)) 7231 return false; 7232 SubDetailComponent o = (SubDetailComponent) other_; 7233 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true) 7234 ; 7235 } 7236 7237 public boolean isEmpty() { 7238 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, revenue 7239 , category, service, modifier, programCode, quantity, unitPrice, factor, net 7240 , udi, noteNumber, adjudication); 7241 } 7242 7243 public String fhirType() { 7244 return "ExplanationOfBenefit.item.detail.subDetail"; 7245 7246 } 7247 7248 } 7249 7250 @Block() 7251 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 7252 /** 7253 * List of input service items which this service line is intended to replace. 7254 */ 7255 @Child(name = "sequenceLinkId", type = {PositiveIntType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7256 @Description(shortDefinition="Service instances", formalDefinition="List of input service items which this service line is intended to replace." ) 7257 protected List<PositiveIntType> sequenceLinkId; 7258 7259 /** 7260 * The type of reveneu or cost center providing the product and/or service. 7261 */ 7262 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 7263 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 7264 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 7265 protected CodeableConcept revenue; 7266 7267 /** 7268 * Health Care Service Type Codes to identify the classification of service or benefits. 7269 */ 7270 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 7271 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 7272 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 7273 protected CodeableConcept category; 7274 7275 /** 7276 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 7277 */ 7278 @Child(name = "service", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 7279 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 7280 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 7281 protected CodeableConcept service; 7282 7283 /** 7284 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 7285 */ 7286 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7287 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 7288 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 7289 protected List<CodeableConcept> modifier; 7290 7291 /** 7292 * The fee charged for the professional service or product. 7293 */ 7294 @Child(name = "fee", type = {Money.class}, order=6, min=0, max=1, modifier=false, summary=false) 7295 @Description(shortDefinition="Professional fee or Product charge", formalDefinition="The fee charged for the professional service or product." ) 7296 protected Money fee; 7297 7298 /** 7299 * A list of note references to the notes provided below. 7300 */ 7301 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7302 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 7303 protected List<PositiveIntType> noteNumber; 7304 7305 /** 7306 * The adjudications results. 7307 */ 7308 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7309 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudications results." ) 7310 protected List<AdjudicationComponent> adjudication; 7311 7312 /** 7313 * The second tier service adjudications for payor added services. 7314 */ 7315 @Child(name = "detail", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7316 @Description(shortDefinition="Added items details", formalDefinition="The second tier service adjudications for payor added services." ) 7317 protected List<AddedItemsDetailComponent> detail; 7318 7319 private static final long serialVersionUID = 1969703165L; 7320 7321 /** 7322 * Constructor 7323 */ 7324 public AddedItemComponent() { 7325 super(); 7326 } 7327 7328 /** 7329 * @return {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 7330 */ 7331 public List<PositiveIntType> getSequenceLinkId() { 7332 if (this.sequenceLinkId == null) 7333 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 7334 return this.sequenceLinkId; 7335 } 7336 7337 /** 7338 * @return Returns a reference to <code>this</code> for easy method chaining 7339 */ 7340 public AddedItemComponent setSequenceLinkId(List<PositiveIntType> theSequenceLinkId) { 7341 this.sequenceLinkId = theSequenceLinkId; 7342 return this; 7343 } 7344 7345 public boolean hasSequenceLinkId() { 7346 if (this.sequenceLinkId == null) 7347 return false; 7348 for (PositiveIntType item : this.sequenceLinkId) 7349 if (!item.isEmpty()) 7350 return true; 7351 return false; 7352 } 7353 7354 /** 7355 * @return {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 7356 */ 7357 public PositiveIntType addSequenceLinkIdElement() {//2 7358 PositiveIntType t = new PositiveIntType(); 7359 if (this.sequenceLinkId == null) 7360 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 7361 this.sequenceLinkId.add(t); 7362 return t; 7363 } 7364 7365 /** 7366 * @param value {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 7367 */ 7368 public AddedItemComponent addSequenceLinkId(int value) { //1 7369 PositiveIntType t = new PositiveIntType(); 7370 t.setValue(value); 7371 if (this.sequenceLinkId == null) 7372 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 7373 this.sequenceLinkId.add(t); 7374 return this; 7375 } 7376 7377 /** 7378 * @param value {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 7379 */ 7380 public boolean hasSequenceLinkId(int value) { 7381 if (this.sequenceLinkId == null) 7382 return false; 7383 for (PositiveIntType v : this.sequenceLinkId) 7384 if (v.getValue().equals(value)) // positiveInt 7385 return true; 7386 return false; 7387 } 7388 7389 /** 7390 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 7391 */ 7392 public CodeableConcept getRevenue() { 7393 if (this.revenue == null) 7394 if (Configuration.errorOnAutoCreate()) 7395 throw new Error("Attempt to auto-create AddedItemComponent.revenue"); 7396 else if (Configuration.doAutoCreate()) 7397 this.revenue = new CodeableConcept(); // cc 7398 return this.revenue; 7399 } 7400 7401 public boolean hasRevenue() { 7402 return this.revenue != null && !this.revenue.isEmpty(); 7403 } 7404 7405 /** 7406 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 7407 */ 7408 public AddedItemComponent setRevenue(CodeableConcept value) { 7409 this.revenue = value; 7410 return this; 7411 } 7412 7413 /** 7414 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 7415 */ 7416 public CodeableConcept getCategory() { 7417 if (this.category == null) 7418 if (Configuration.errorOnAutoCreate()) 7419 throw new Error("Attempt to auto-create AddedItemComponent.category"); 7420 else if (Configuration.doAutoCreate()) 7421 this.category = new CodeableConcept(); // cc 7422 return this.category; 7423 } 7424 7425 public boolean hasCategory() { 7426 return this.category != null && !this.category.isEmpty(); 7427 } 7428 7429 /** 7430 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 7431 */ 7432 public AddedItemComponent setCategory(CodeableConcept value) { 7433 this.category = value; 7434 return this; 7435 } 7436 7437 /** 7438 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 7439 */ 7440 public CodeableConcept getService() { 7441 if (this.service == null) 7442 if (Configuration.errorOnAutoCreate()) 7443 throw new Error("Attempt to auto-create AddedItemComponent.service"); 7444 else if (Configuration.doAutoCreate()) 7445 this.service = new CodeableConcept(); // cc 7446 return this.service; 7447 } 7448 7449 public boolean hasService() { 7450 return this.service != null && !this.service.isEmpty(); 7451 } 7452 7453 /** 7454 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 7455 */ 7456 public AddedItemComponent setService(CodeableConcept value) { 7457 this.service = value; 7458 return this; 7459 } 7460 7461 /** 7462 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 7463 */ 7464 public List<CodeableConcept> getModifier() { 7465 if (this.modifier == null) 7466 this.modifier = new ArrayList<CodeableConcept>(); 7467 return this.modifier; 7468 } 7469 7470 /** 7471 * @return Returns a reference to <code>this</code> for easy method chaining 7472 */ 7473 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 7474 this.modifier = theModifier; 7475 return this; 7476 } 7477 7478 public boolean hasModifier() { 7479 if (this.modifier == null) 7480 return false; 7481 for (CodeableConcept item : this.modifier) 7482 if (!item.isEmpty()) 7483 return true; 7484 return false; 7485 } 7486 7487 public CodeableConcept addModifier() { //3 7488 CodeableConcept t = new CodeableConcept(); 7489 if (this.modifier == null) 7490 this.modifier = new ArrayList<CodeableConcept>(); 7491 this.modifier.add(t); 7492 return t; 7493 } 7494 7495 public AddedItemComponent addModifier(CodeableConcept t) { //3 7496 if (t == null) 7497 return this; 7498 if (this.modifier == null) 7499 this.modifier = new ArrayList<CodeableConcept>(); 7500 this.modifier.add(t); 7501 return this; 7502 } 7503 7504 /** 7505 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 7506 */ 7507 public CodeableConcept getModifierFirstRep() { 7508 if (getModifier().isEmpty()) { 7509 addModifier(); 7510 } 7511 return getModifier().get(0); 7512 } 7513 7514 /** 7515 * @return {@link #fee} (The fee charged for the professional service or product.) 7516 */ 7517 public Money getFee() { 7518 if (this.fee == null) 7519 if (Configuration.errorOnAutoCreate()) 7520 throw new Error("Attempt to auto-create AddedItemComponent.fee"); 7521 else if (Configuration.doAutoCreate()) 7522 this.fee = new Money(); // cc 7523 return this.fee; 7524 } 7525 7526 public boolean hasFee() { 7527 return this.fee != null && !this.fee.isEmpty(); 7528 } 7529 7530 /** 7531 * @param value {@link #fee} (The fee charged for the professional service or product.) 7532 */ 7533 public AddedItemComponent setFee(Money value) { 7534 this.fee = value; 7535 return this; 7536 } 7537 7538 /** 7539 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 7540 */ 7541 public List<PositiveIntType> getNoteNumber() { 7542 if (this.noteNumber == null) 7543 this.noteNumber = new ArrayList<PositiveIntType>(); 7544 return this.noteNumber; 7545 } 7546 7547 /** 7548 * @return Returns a reference to <code>this</code> for easy method chaining 7549 */ 7550 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 7551 this.noteNumber = theNoteNumber; 7552 return this; 7553 } 7554 7555 public boolean hasNoteNumber() { 7556 if (this.noteNumber == null) 7557 return false; 7558 for (PositiveIntType item : this.noteNumber) 7559 if (!item.isEmpty()) 7560 return true; 7561 return false; 7562 } 7563 7564 /** 7565 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 7566 */ 7567 public PositiveIntType addNoteNumberElement() {//2 7568 PositiveIntType t = new PositiveIntType(); 7569 if (this.noteNumber == null) 7570 this.noteNumber = new ArrayList<PositiveIntType>(); 7571 this.noteNumber.add(t); 7572 return t; 7573 } 7574 7575 /** 7576 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 7577 */ 7578 public AddedItemComponent addNoteNumber(int value) { //1 7579 PositiveIntType t = new PositiveIntType(); 7580 t.setValue(value); 7581 if (this.noteNumber == null) 7582 this.noteNumber = new ArrayList<PositiveIntType>(); 7583 this.noteNumber.add(t); 7584 return this; 7585 } 7586 7587 /** 7588 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 7589 */ 7590 public boolean hasNoteNumber(int value) { 7591 if (this.noteNumber == null) 7592 return false; 7593 for (PositiveIntType v : this.noteNumber) 7594 if (v.getValue().equals(value)) // positiveInt 7595 return true; 7596 return false; 7597 } 7598 7599 /** 7600 * @return {@link #adjudication} (The adjudications results.) 7601 */ 7602 public List<AdjudicationComponent> getAdjudication() { 7603 if (this.adjudication == null) 7604 this.adjudication = new ArrayList<AdjudicationComponent>(); 7605 return this.adjudication; 7606 } 7607 7608 /** 7609 * @return Returns a reference to <code>this</code> for easy method chaining 7610 */ 7611 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 7612 this.adjudication = theAdjudication; 7613 return this; 7614 } 7615 7616 public boolean hasAdjudication() { 7617 if (this.adjudication == null) 7618 return false; 7619 for (AdjudicationComponent item : this.adjudication) 7620 if (!item.isEmpty()) 7621 return true; 7622 return false; 7623 } 7624 7625 public AdjudicationComponent addAdjudication() { //3 7626 AdjudicationComponent t = new AdjudicationComponent(); 7627 if (this.adjudication == null) 7628 this.adjudication = new ArrayList<AdjudicationComponent>(); 7629 this.adjudication.add(t); 7630 return t; 7631 } 7632 7633 public AddedItemComponent addAdjudication(AdjudicationComponent t) { //3 7634 if (t == null) 7635 return this; 7636 if (this.adjudication == null) 7637 this.adjudication = new ArrayList<AdjudicationComponent>(); 7638 this.adjudication.add(t); 7639 return this; 7640 } 7641 7642 /** 7643 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 7644 */ 7645 public AdjudicationComponent getAdjudicationFirstRep() { 7646 if (getAdjudication().isEmpty()) { 7647 addAdjudication(); 7648 } 7649 return getAdjudication().get(0); 7650 } 7651 7652 /** 7653 * @return {@link #detail} (The second tier service adjudications for payor added services.) 7654 */ 7655 public List<AddedItemsDetailComponent> getDetail() { 7656 if (this.detail == null) 7657 this.detail = new ArrayList<AddedItemsDetailComponent>(); 7658 return this.detail; 7659 } 7660 7661 /** 7662 * @return Returns a reference to <code>this</code> for easy method chaining 7663 */ 7664 public AddedItemComponent setDetail(List<AddedItemsDetailComponent> theDetail) { 7665 this.detail = theDetail; 7666 return this; 7667 } 7668 7669 public boolean hasDetail() { 7670 if (this.detail == null) 7671 return false; 7672 for (AddedItemsDetailComponent item : this.detail) 7673 if (!item.isEmpty()) 7674 return true; 7675 return false; 7676 } 7677 7678 public AddedItemsDetailComponent addDetail() { //3 7679 AddedItemsDetailComponent t = new AddedItemsDetailComponent(); 7680 if (this.detail == null) 7681 this.detail = new ArrayList<AddedItemsDetailComponent>(); 7682 this.detail.add(t); 7683 return t; 7684 } 7685 7686 public AddedItemComponent addDetail(AddedItemsDetailComponent t) { //3 7687 if (t == null) 7688 return this; 7689 if (this.detail == null) 7690 this.detail = new ArrayList<AddedItemsDetailComponent>(); 7691 this.detail.add(t); 7692 return this; 7693 } 7694 7695 /** 7696 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 7697 */ 7698 public AddedItemsDetailComponent getDetailFirstRep() { 7699 if (getDetail().isEmpty()) { 7700 addDetail(); 7701 } 7702 return getDetail().get(0); 7703 } 7704 7705 protected void listChildren(List<Property> children) { 7706 super.listChildren(children); 7707 children.add(new Property("sequenceLinkId", "positiveInt", "List of input service items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, sequenceLinkId)); 7708 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 7709 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 7710 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 7711 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 7712 children.add(new Property("fee", "Money", "The fee charged for the professional service or product.", 0, 1, fee)); 7713 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 7714 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 7715 children.add(new Property("detail", "", "The second tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail)); 7716 } 7717 7718 @Override 7719 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7720 switch (_hash) { 7721 case -1422298666: /*sequenceLinkId*/ return new Property("sequenceLinkId", "positiveInt", "List of input service items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, sequenceLinkId); 7722 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 7723 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 7724 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 7725 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 7726 case 101254: /*fee*/ return new Property("fee", "Money", "The fee charged for the professional service or product.", 0, 1, fee); 7727 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 7728 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 7729 case -1335224239: /*detail*/ return new Property("detail", "", "The second tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 7730 default: return super.getNamedProperty(_hash, _name, _checkValid); 7731 } 7732 7733 } 7734 7735 @Override 7736 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7737 switch (hash) { 7738 case -1422298666: /*sequenceLinkId*/ return this.sequenceLinkId == null ? new Base[0] : this.sequenceLinkId.toArray(new Base[this.sequenceLinkId.size()]); // PositiveIntType 7739 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 7740 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 7741 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 7742 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7743 case 101254: /*fee*/ return this.fee == null ? new Base[0] : new Base[] {this.fee}; // Money 7744 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 7745 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 7746 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemsDetailComponent 7747 default: return super.getProperty(hash, name, checkValid); 7748 } 7749 7750 } 7751 7752 @Override 7753 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7754 switch (hash) { 7755 case -1422298666: // sequenceLinkId 7756 this.getSequenceLinkId().add(castToPositiveInt(value)); // PositiveIntType 7757 return value; 7758 case 1099842588: // revenue 7759 this.revenue = castToCodeableConcept(value); // CodeableConcept 7760 return value; 7761 case 50511102: // category 7762 this.category = castToCodeableConcept(value); // CodeableConcept 7763 return value; 7764 case 1984153269: // service 7765 this.service = castToCodeableConcept(value); // CodeableConcept 7766 return value; 7767 case -615513385: // modifier 7768 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 7769 return value; 7770 case 101254: // fee 7771 this.fee = castToMoney(value); // Money 7772 return value; 7773 case -1110033957: // noteNumber 7774 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 7775 return value; 7776 case -231349275: // adjudication 7777 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 7778 return value; 7779 case -1335224239: // detail 7780 this.getDetail().add((AddedItemsDetailComponent) value); // AddedItemsDetailComponent 7781 return value; 7782 default: return super.setProperty(hash, name, value); 7783 } 7784 7785 } 7786 7787 @Override 7788 public Base setProperty(String name, Base value) throws FHIRException { 7789 if (name.equals("sequenceLinkId")) { 7790 this.getSequenceLinkId().add(castToPositiveInt(value)); 7791 } else if (name.equals("revenue")) { 7792 this.revenue = castToCodeableConcept(value); // CodeableConcept 7793 } else if (name.equals("category")) { 7794 this.category = castToCodeableConcept(value); // CodeableConcept 7795 } else if (name.equals("service")) { 7796 this.service = castToCodeableConcept(value); // CodeableConcept 7797 } else if (name.equals("modifier")) { 7798 this.getModifier().add(castToCodeableConcept(value)); 7799 } else if (name.equals("fee")) { 7800 this.fee = castToMoney(value); // Money 7801 } else if (name.equals("noteNumber")) { 7802 this.getNoteNumber().add(castToPositiveInt(value)); 7803 } else if (name.equals("adjudication")) { 7804 this.getAdjudication().add((AdjudicationComponent) value); 7805 } else if (name.equals("detail")) { 7806 this.getDetail().add((AddedItemsDetailComponent) value); 7807 } else 7808 return super.setProperty(name, value); 7809 return value; 7810 } 7811 7812 @Override 7813 public Base makeProperty(int hash, String name) throws FHIRException { 7814 switch (hash) { 7815 case -1422298666: return addSequenceLinkIdElement(); 7816 case 1099842588: return getRevenue(); 7817 case 50511102: return getCategory(); 7818 case 1984153269: return getService(); 7819 case -615513385: return addModifier(); 7820 case 101254: return getFee(); 7821 case -1110033957: return addNoteNumberElement(); 7822 case -231349275: return addAdjudication(); 7823 case -1335224239: return addDetail(); 7824 default: return super.makeProperty(hash, name); 7825 } 7826 7827 } 7828 7829 @Override 7830 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7831 switch (hash) { 7832 case -1422298666: /*sequenceLinkId*/ return new String[] {"positiveInt"}; 7833 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 7834 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 7835 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 7836 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 7837 case 101254: /*fee*/ return new String[] {"Money"}; 7838 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 7839 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 7840 case -1335224239: /*detail*/ return new String[] {}; 7841 default: return super.getTypesForProperty(hash, name); 7842 } 7843 7844 } 7845 7846 @Override 7847 public Base addChild(String name) throws FHIRException { 7848 if (name.equals("sequenceLinkId")) { 7849 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequenceLinkId"); 7850 } 7851 else if (name.equals("revenue")) { 7852 this.revenue = new CodeableConcept(); 7853 return this.revenue; 7854 } 7855 else if (name.equals("category")) { 7856 this.category = new CodeableConcept(); 7857 return this.category; 7858 } 7859 else if (name.equals("service")) { 7860 this.service = new CodeableConcept(); 7861 return this.service; 7862 } 7863 else if (name.equals("modifier")) { 7864 return addModifier(); 7865 } 7866 else if (name.equals("fee")) { 7867 this.fee = new Money(); 7868 return this.fee; 7869 } 7870 else if (name.equals("noteNumber")) { 7871 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 7872 } 7873 else if (name.equals("adjudication")) { 7874 return addAdjudication(); 7875 } 7876 else if (name.equals("detail")) { 7877 return addDetail(); 7878 } 7879 else 7880 return super.addChild(name); 7881 } 7882 7883 public AddedItemComponent copy() { 7884 AddedItemComponent dst = new AddedItemComponent(); 7885 copyValues(dst); 7886 if (sequenceLinkId != null) { 7887 dst.sequenceLinkId = new ArrayList<PositiveIntType>(); 7888 for (PositiveIntType i : sequenceLinkId) 7889 dst.sequenceLinkId.add(i.copy()); 7890 }; 7891 dst.revenue = revenue == null ? null : revenue.copy(); 7892 dst.category = category == null ? null : category.copy(); 7893 dst.service = service == null ? null : service.copy(); 7894 if (modifier != null) { 7895 dst.modifier = new ArrayList<CodeableConcept>(); 7896 for (CodeableConcept i : modifier) 7897 dst.modifier.add(i.copy()); 7898 }; 7899 dst.fee = fee == null ? null : fee.copy(); 7900 if (noteNumber != null) { 7901 dst.noteNumber = new ArrayList<PositiveIntType>(); 7902 for (PositiveIntType i : noteNumber) 7903 dst.noteNumber.add(i.copy()); 7904 }; 7905 if (adjudication != null) { 7906 dst.adjudication = new ArrayList<AdjudicationComponent>(); 7907 for (AdjudicationComponent i : adjudication) 7908 dst.adjudication.add(i.copy()); 7909 }; 7910 if (detail != null) { 7911 dst.detail = new ArrayList<AddedItemsDetailComponent>(); 7912 for (AddedItemsDetailComponent i : detail) 7913 dst.detail.add(i.copy()); 7914 }; 7915 return dst; 7916 } 7917 7918 @Override 7919 public boolean equalsDeep(Base other_) { 7920 if (!super.equalsDeep(other_)) 7921 return false; 7922 if (!(other_ instanceof AddedItemComponent)) 7923 return false; 7924 AddedItemComponent o = (AddedItemComponent) other_; 7925 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(revenue, o.revenue, true) 7926 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 7927 && compareDeep(fee, o.fee, true) && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 7928 && compareDeep(detail, o.detail, true); 7929 } 7930 7931 @Override 7932 public boolean equalsShallow(Base other_) { 7933 if (!super.equalsShallow(other_)) 7934 return false; 7935 if (!(other_ instanceof AddedItemComponent)) 7936 return false; 7937 AddedItemComponent o = (AddedItemComponent) other_; 7938 return compareValues(sequenceLinkId, o.sequenceLinkId, true) && compareValues(noteNumber, o.noteNumber, true) 7939 ; 7940 } 7941 7942 public boolean isEmpty() { 7943 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceLinkId, revenue, category 7944 , service, modifier, fee, noteNumber, adjudication, detail); 7945 } 7946 7947 public String fhirType() { 7948 return "ExplanationOfBenefit.addItem"; 7949 7950 } 7951 7952 } 7953 7954 @Block() 7955 public static class AddedItemsDetailComponent extends BackboneElement implements IBaseBackboneElement { 7956 /** 7957 * The type of reveneu or cost center providing the product and/or service. 7958 */ 7959 @Child(name = "revenue", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 7960 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 7961 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 7962 protected CodeableConcept revenue; 7963 7964 /** 7965 * Health Care Service Type Codes to identify the classification of service or benefits. 7966 */ 7967 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 7968 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 7969 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 7970 protected CodeableConcept category; 7971 7972 /** 7973 * A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). 7974 */ 7975 @Child(name = "service", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 7976 @Description(shortDefinition="Billing Code", formalDefinition="A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI)." ) 7977 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 7978 protected CodeableConcept service; 7979 7980 /** 7981 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 7982 */ 7983 @Child(name = "modifier", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7984 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 7985 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 7986 protected List<CodeableConcept> modifier; 7987 7988 /** 7989 * The fee charged for the professional service or product. 7990 */ 7991 @Child(name = "fee", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 7992 @Description(shortDefinition="Professional fee or Product charge", formalDefinition="The fee charged for the professional service or product." ) 7993 protected Money fee; 7994 7995 /** 7996 * A list of note references to the notes provided below. 7997 */ 7998 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7999 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 8000 protected List<PositiveIntType> noteNumber; 8001 8002 /** 8003 * The adjudications results. 8004 */ 8005 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8006 @Description(shortDefinition="Added items detail adjudication", formalDefinition="The adjudications results." ) 8007 protected List<AdjudicationComponent> adjudication; 8008 8009 private static final long serialVersionUID = -311484980L; 8010 8011 /** 8012 * Constructor 8013 */ 8014 public AddedItemsDetailComponent() { 8015 super(); 8016 } 8017 8018 /** 8019 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 8020 */ 8021 public CodeableConcept getRevenue() { 8022 if (this.revenue == null) 8023 if (Configuration.errorOnAutoCreate()) 8024 throw new Error("Attempt to auto-create AddedItemsDetailComponent.revenue"); 8025 else if (Configuration.doAutoCreate()) 8026 this.revenue = new CodeableConcept(); // cc 8027 return this.revenue; 8028 } 8029 8030 public boolean hasRevenue() { 8031 return this.revenue != null && !this.revenue.isEmpty(); 8032 } 8033 8034 /** 8035 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 8036 */ 8037 public AddedItemsDetailComponent setRevenue(CodeableConcept value) { 8038 this.revenue = value; 8039 return this; 8040 } 8041 8042 /** 8043 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 8044 */ 8045 public CodeableConcept getCategory() { 8046 if (this.category == null) 8047 if (Configuration.errorOnAutoCreate()) 8048 throw new Error("Attempt to auto-create AddedItemsDetailComponent.category"); 8049 else if (Configuration.doAutoCreate()) 8050 this.category = new CodeableConcept(); // cc 8051 return this.category; 8052 } 8053 8054 public boolean hasCategory() { 8055 return this.category != null && !this.category.isEmpty(); 8056 } 8057 8058 /** 8059 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 8060 */ 8061 public AddedItemsDetailComponent setCategory(CodeableConcept value) { 8062 this.category = value; 8063 return this; 8064 } 8065 8066 /** 8067 * @return {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 8068 */ 8069 public CodeableConcept getService() { 8070 if (this.service == null) 8071 if (Configuration.errorOnAutoCreate()) 8072 throw new Error("Attempt to auto-create AddedItemsDetailComponent.service"); 8073 else if (Configuration.doAutoCreate()) 8074 this.service = new CodeableConcept(); // cc 8075 return this.service; 8076 } 8077 8078 public boolean hasService() { 8079 return this.service != null && !this.service.isEmpty(); 8080 } 8081 8082 /** 8083 * @param value {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 8084 */ 8085 public AddedItemsDetailComponent setService(CodeableConcept value) { 8086 this.service = value; 8087 return this; 8088 } 8089 8090 /** 8091 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 8092 */ 8093 public List<CodeableConcept> getModifier() { 8094 if (this.modifier == null) 8095 this.modifier = new ArrayList<CodeableConcept>(); 8096 return this.modifier; 8097 } 8098 8099 /** 8100 * @return Returns a reference to <code>this</code> for easy method chaining 8101 */ 8102 public AddedItemsDetailComponent setModifier(List<CodeableConcept> theModifier) { 8103 this.modifier = theModifier; 8104 return this; 8105 } 8106 8107 public boolean hasModifier() { 8108 if (this.modifier == null) 8109 return false; 8110 for (CodeableConcept item : this.modifier) 8111 if (!item.isEmpty()) 8112 return true; 8113 return false; 8114 } 8115 8116 public CodeableConcept addModifier() { //3 8117 CodeableConcept t = new CodeableConcept(); 8118 if (this.modifier == null) 8119 this.modifier = new ArrayList<CodeableConcept>(); 8120 this.modifier.add(t); 8121 return t; 8122 } 8123 8124 public AddedItemsDetailComponent addModifier(CodeableConcept t) { //3 8125 if (t == null) 8126 return this; 8127 if (this.modifier == null) 8128 this.modifier = new ArrayList<CodeableConcept>(); 8129 this.modifier.add(t); 8130 return this; 8131 } 8132 8133 /** 8134 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 8135 */ 8136 public CodeableConcept getModifierFirstRep() { 8137 if (getModifier().isEmpty()) { 8138 addModifier(); 8139 } 8140 return getModifier().get(0); 8141 } 8142 8143 /** 8144 * @return {@link #fee} (The fee charged for the professional service or product.) 8145 */ 8146 public Money getFee() { 8147 if (this.fee == null) 8148 if (Configuration.errorOnAutoCreate()) 8149 throw new Error("Attempt to auto-create AddedItemsDetailComponent.fee"); 8150 else if (Configuration.doAutoCreate()) 8151 this.fee = new Money(); // cc 8152 return this.fee; 8153 } 8154 8155 public boolean hasFee() { 8156 return this.fee != null && !this.fee.isEmpty(); 8157 } 8158 8159 /** 8160 * @param value {@link #fee} (The fee charged for the professional service or product.) 8161 */ 8162 public AddedItemsDetailComponent setFee(Money value) { 8163 this.fee = value; 8164 return this; 8165 } 8166 8167 /** 8168 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 8169 */ 8170 public List<PositiveIntType> getNoteNumber() { 8171 if (this.noteNumber == null) 8172 this.noteNumber = new ArrayList<PositiveIntType>(); 8173 return this.noteNumber; 8174 } 8175 8176 /** 8177 * @return Returns a reference to <code>this</code> for easy method chaining 8178 */ 8179 public AddedItemsDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 8180 this.noteNumber = theNoteNumber; 8181 return this; 8182 } 8183 8184 public boolean hasNoteNumber() { 8185 if (this.noteNumber == null) 8186 return false; 8187 for (PositiveIntType item : this.noteNumber) 8188 if (!item.isEmpty()) 8189 return true; 8190 return false; 8191 } 8192 8193 /** 8194 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 8195 */ 8196 public PositiveIntType addNoteNumberElement() {//2 8197 PositiveIntType t = new PositiveIntType(); 8198 if (this.noteNumber == null) 8199 this.noteNumber = new ArrayList<PositiveIntType>(); 8200 this.noteNumber.add(t); 8201 return t; 8202 } 8203 8204 /** 8205 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 8206 */ 8207 public AddedItemsDetailComponent addNoteNumber(int value) { //1 8208 PositiveIntType t = new PositiveIntType(); 8209 t.setValue(value); 8210 if (this.noteNumber == null) 8211 this.noteNumber = new ArrayList<PositiveIntType>(); 8212 this.noteNumber.add(t); 8213 return this; 8214 } 8215 8216 /** 8217 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 8218 */ 8219 public boolean hasNoteNumber(int value) { 8220 if (this.noteNumber == null) 8221 return false; 8222 for (PositiveIntType v : this.noteNumber) 8223 if (v.getValue().equals(value)) // positiveInt 8224 return true; 8225 return false; 8226 } 8227 8228 /** 8229 * @return {@link #adjudication} (The adjudications results.) 8230 */ 8231 public List<AdjudicationComponent> getAdjudication() { 8232 if (this.adjudication == null) 8233 this.adjudication = new ArrayList<AdjudicationComponent>(); 8234 return this.adjudication; 8235 } 8236 8237 /** 8238 * @return Returns a reference to <code>this</code> for easy method chaining 8239 */ 8240 public AddedItemsDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 8241 this.adjudication = theAdjudication; 8242 return this; 8243 } 8244 8245 public boolean hasAdjudication() { 8246 if (this.adjudication == null) 8247 return false; 8248 for (AdjudicationComponent item : this.adjudication) 8249 if (!item.isEmpty()) 8250 return true; 8251 return false; 8252 } 8253 8254 public AdjudicationComponent addAdjudication() { //3 8255 AdjudicationComponent t = new AdjudicationComponent(); 8256 if (this.adjudication == null) 8257 this.adjudication = new ArrayList<AdjudicationComponent>(); 8258 this.adjudication.add(t); 8259 return t; 8260 } 8261 8262 public AddedItemsDetailComponent addAdjudication(AdjudicationComponent t) { //3 8263 if (t == null) 8264 return this; 8265 if (this.adjudication == null) 8266 this.adjudication = new ArrayList<AdjudicationComponent>(); 8267 this.adjudication.add(t); 8268 return this; 8269 } 8270 8271 /** 8272 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 8273 */ 8274 public AdjudicationComponent getAdjudicationFirstRep() { 8275 if (getAdjudication().isEmpty()) { 8276 addAdjudication(); 8277 } 8278 return getAdjudication().get(0); 8279 } 8280 8281 protected void listChildren(List<Property> children) { 8282 super.listChildren(children); 8283 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 8284 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 8285 children.add(new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service)); 8286 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 8287 children.add(new Property("fee", "Money", "The fee charged for the professional service or product.", 0, 1, fee)); 8288 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 8289 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 8290 } 8291 8292 @Override 8293 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8294 switch (_hash) { 8295 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 8296 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 8297 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service); 8298 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 8299 case 101254: /*fee*/ return new Property("fee", "Money", "The fee charged for the professional service or product.", 0, 1, fee); 8300 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 8301 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 8302 default: return super.getNamedProperty(_hash, _name, _checkValid); 8303 } 8304 8305 } 8306 8307 @Override 8308 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8309 switch (hash) { 8310 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 8311 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 8312 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 8313 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 8314 case 101254: /*fee*/ return this.fee == null ? new Base[0] : new Base[] {this.fee}; // Money 8315 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 8316 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 8317 default: return super.getProperty(hash, name, checkValid); 8318 } 8319 8320 } 8321 8322 @Override 8323 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8324 switch (hash) { 8325 case 1099842588: // revenue 8326 this.revenue = castToCodeableConcept(value); // CodeableConcept 8327 return value; 8328 case 50511102: // category 8329 this.category = castToCodeableConcept(value); // CodeableConcept 8330 return value; 8331 case 1984153269: // service 8332 this.service = castToCodeableConcept(value); // CodeableConcept 8333 return value; 8334 case -615513385: // modifier 8335 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 8336 return value; 8337 case 101254: // fee 8338 this.fee = castToMoney(value); // Money 8339 return value; 8340 case -1110033957: // noteNumber 8341 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 8342 return value; 8343 case -231349275: // adjudication 8344 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 8345 return value; 8346 default: return super.setProperty(hash, name, value); 8347 } 8348 8349 } 8350 8351 @Override 8352 public Base setProperty(String name, Base value) throws FHIRException { 8353 if (name.equals("revenue")) { 8354 this.revenue = castToCodeableConcept(value); // CodeableConcept 8355 } else if (name.equals("category")) { 8356 this.category = castToCodeableConcept(value); // CodeableConcept 8357 } else if (name.equals("service")) { 8358 this.service = castToCodeableConcept(value); // CodeableConcept 8359 } else if (name.equals("modifier")) { 8360 this.getModifier().add(castToCodeableConcept(value)); 8361 } else if (name.equals("fee")) { 8362 this.fee = castToMoney(value); // Money 8363 } else if (name.equals("noteNumber")) { 8364 this.getNoteNumber().add(castToPositiveInt(value)); 8365 } else if (name.equals("adjudication")) { 8366 this.getAdjudication().add((AdjudicationComponent) value); 8367 } else 8368 return super.setProperty(name, value); 8369 return value; 8370 } 8371 8372 @Override 8373 public Base makeProperty(int hash, String name) throws FHIRException { 8374 switch (hash) { 8375 case 1099842588: return getRevenue(); 8376 case 50511102: return getCategory(); 8377 case 1984153269: return getService(); 8378 case -615513385: return addModifier(); 8379 case 101254: return getFee(); 8380 case -1110033957: return addNoteNumberElement(); 8381 case -231349275: return addAdjudication(); 8382 default: return super.makeProperty(hash, name); 8383 } 8384 8385 } 8386 8387 @Override 8388 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8389 switch (hash) { 8390 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 8391 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 8392 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 8393 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 8394 case 101254: /*fee*/ return new String[] {"Money"}; 8395 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 8396 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 8397 default: return super.getTypesForProperty(hash, name); 8398 } 8399 8400 } 8401 8402 @Override 8403 public Base addChild(String name) throws FHIRException { 8404 if (name.equals("revenue")) { 8405 this.revenue = new CodeableConcept(); 8406 return this.revenue; 8407 } 8408 else if (name.equals("category")) { 8409 this.category = new CodeableConcept(); 8410 return this.category; 8411 } 8412 else if (name.equals("service")) { 8413 this.service = new CodeableConcept(); 8414 return this.service; 8415 } 8416 else if (name.equals("modifier")) { 8417 return addModifier(); 8418 } 8419 else if (name.equals("fee")) { 8420 this.fee = new Money(); 8421 return this.fee; 8422 } 8423 else if (name.equals("noteNumber")) { 8424 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 8425 } 8426 else if (name.equals("adjudication")) { 8427 return addAdjudication(); 8428 } 8429 else 8430 return super.addChild(name); 8431 } 8432 8433 public AddedItemsDetailComponent copy() { 8434 AddedItemsDetailComponent dst = new AddedItemsDetailComponent(); 8435 copyValues(dst); 8436 dst.revenue = revenue == null ? null : revenue.copy(); 8437 dst.category = category == null ? null : category.copy(); 8438 dst.service = service == null ? null : service.copy(); 8439 if (modifier != null) { 8440 dst.modifier = new ArrayList<CodeableConcept>(); 8441 for (CodeableConcept i : modifier) 8442 dst.modifier.add(i.copy()); 8443 }; 8444 dst.fee = fee == null ? null : fee.copy(); 8445 if (noteNumber != null) { 8446 dst.noteNumber = new ArrayList<PositiveIntType>(); 8447 for (PositiveIntType i : noteNumber) 8448 dst.noteNumber.add(i.copy()); 8449 }; 8450 if (adjudication != null) { 8451 dst.adjudication = new ArrayList<AdjudicationComponent>(); 8452 for (AdjudicationComponent i : adjudication) 8453 dst.adjudication.add(i.copy()); 8454 }; 8455 return dst; 8456 } 8457 8458 @Override 8459 public boolean equalsDeep(Base other_) { 8460 if (!super.equalsDeep(other_)) 8461 return false; 8462 if (!(other_ instanceof AddedItemsDetailComponent)) 8463 return false; 8464 AddedItemsDetailComponent o = (AddedItemsDetailComponent) other_; 8465 return compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) 8466 && compareDeep(modifier, o.modifier, true) && compareDeep(fee, o.fee, true) && compareDeep(noteNumber, o.noteNumber, true) 8467 && compareDeep(adjudication, o.adjudication, true); 8468 } 8469 8470 @Override 8471 public boolean equalsShallow(Base other_) { 8472 if (!super.equalsShallow(other_)) 8473 return false; 8474 if (!(other_ instanceof AddedItemsDetailComponent)) 8475 return false; 8476 AddedItemsDetailComponent o = (AddedItemsDetailComponent) other_; 8477 return compareValues(noteNumber, o.noteNumber, true); 8478 } 8479 8480 public boolean isEmpty() { 8481 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(revenue, category, service 8482 , modifier, fee, noteNumber, adjudication); 8483 } 8484 8485 public String fhirType() { 8486 return "ExplanationOfBenefit.addItem.detail"; 8487 8488 } 8489 8490 } 8491 8492 @Block() 8493 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 8494 /** 8495 * Whether this represents partial or complete payment of the claim. 8496 */ 8497 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 8498 @Description(shortDefinition="Partial or Complete", formalDefinition="Whether this represents partial or complete payment of the claim." ) 8499 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-paymenttype") 8500 protected CodeableConcept type; 8501 8502 /** 8503 * Adjustment to the payment of this transaction which is not related to adjudication of this transaction. 8504 */ 8505 @Child(name = "adjustment", type = {Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 8506 @Description(shortDefinition="Payment adjustment for non-Claim issues", formalDefinition="Adjustment to the payment of this transaction which is not related to adjudication of this transaction." ) 8507 protected Money adjustment; 8508 8509 /** 8510 * Reason for the payment adjustment. 8511 */ 8512 @Child(name = "adjustmentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 8513 @Description(shortDefinition="Explanation for the non-claim adjustment", formalDefinition="Reason for the payment adjustment." ) 8514 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 8515 protected CodeableConcept adjustmentReason; 8516 8517 /** 8518 * Estimated payment date. 8519 */ 8520 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 8521 @Description(shortDefinition="Expected date of Payment", formalDefinition="Estimated payment date." ) 8522 protected DateType date; 8523 8524 /** 8525 * Payable less any payment adjustment. 8526 */ 8527 @Child(name = "amount", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 8528 @Description(shortDefinition="Payable amount after adjustment", formalDefinition="Payable less any payment adjustment." ) 8529 protected Money amount; 8530 8531 /** 8532 * Payment identifer. 8533 */ 8534 @Child(name = "identifier", type = {Identifier.class}, order=6, min=0, max=1, modifier=false, summary=false) 8535 @Description(shortDefinition="Identifier of the payment instrument", formalDefinition="Payment identifer." ) 8536 protected Identifier identifier; 8537 8538 private static final long serialVersionUID = 1539906026L; 8539 8540 /** 8541 * Constructor 8542 */ 8543 public PaymentComponent() { 8544 super(); 8545 } 8546 8547 /** 8548 * @return {@link #type} (Whether this represents partial or complete payment of the claim.) 8549 */ 8550 public CodeableConcept getType() { 8551 if (this.type == null) 8552 if (Configuration.errorOnAutoCreate()) 8553 throw new Error("Attempt to auto-create PaymentComponent.type"); 8554 else if (Configuration.doAutoCreate()) 8555 this.type = new CodeableConcept(); // cc 8556 return this.type; 8557 } 8558 8559 public boolean hasType() { 8560 return this.type != null && !this.type.isEmpty(); 8561 } 8562 8563 /** 8564 * @param value {@link #type} (Whether this represents partial or complete payment of the claim.) 8565 */ 8566 public PaymentComponent setType(CodeableConcept value) { 8567 this.type = value; 8568 return this; 8569 } 8570 8571 /** 8572 * @return {@link #adjustment} (Adjustment to the payment of this transaction which is not related to adjudication of this transaction.) 8573 */ 8574 public Money getAdjustment() { 8575 if (this.adjustment == null) 8576 if (Configuration.errorOnAutoCreate()) 8577 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 8578 else if (Configuration.doAutoCreate()) 8579 this.adjustment = new Money(); // cc 8580 return this.adjustment; 8581 } 8582 8583 public boolean hasAdjustment() { 8584 return this.adjustment != null && !this.adjustment.isEmpty(); 8585 } 8586 8587 /** 8588 * @param value {@link #adjustment} (Adjustment to the payment of this transaction which is not related to adjudication of this transaction.) 8589 */ 8590 public PaymentComponent setAdjustment(Money value) { 8591 this.adjustment = value; 8592 return this; 8593 } 8594 8595 /** 8596 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 8597 */ 8598 public CodeableConcept getAdjustmentReason() { 8599 if (this.adjustmentReason == null) 8600 if (Configuration.errorOnAutoCreate()) 8601 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 8602 else if (Configuration.doAutoCreate()) 8603 this.adjustmentReason = new CodeableConcept(); // cc 8604 return this.adjustmentReason; 8605 } 8606 8607 public boolean hasAdjustmentReason() { 8608 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 8609 } 8610 8611 /** 8612 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 8613 */ 8614 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 8615 this.adjustmentReason = value; 8616 return this; 8617 } 8618 8619 /** 8620 * @return {@link #date} (Estimated payment date.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 8621 */ 8622 public DateType getDateElement() { 8623 if (this.date == null) 8624 if (Configuration.errorOnAutoCreate()) 8625 throw new Error("Attempt to auto-create PaymentComponent.date"); 8626 else if (Configuration.doAutoCreate()) 8627 this.date = new DateType(); // bb 8628 return this.date; 8629 } 8630 8631 public boolean hasDateElement() { 8632 return this.date != null && !this.date.isEmpty(); 8633 } 8634 8635 public boolean hasDate() { 8636 return this.date != null && !this.date.isEmpty(); 8637 } 8638 8639 /** 8640 * @param value {@link #date} (Estimated payment date.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 8641 */ 8642 public PaymentComponent setDateElement(DateType value) { 8643 this.date = value; 8644 return this; 8645 } 8646 8647 /** 8648 * @return Estimated payment date. 8649 */ 8650 public Date getDate() { 8651 return this.date == null ? null : this.date.getValue(); 8652 } 8653 8654 /** 8655 * @param value Estimated payment date. 8656 */ 8657 public PaymentComponent setDate(Date value) { 8658 if (value == null) 8659 this.date = null; 8660 else { 8661 if (this.date == null) 8662 this.date = new DateType(); 8663 this.date.setValue(value); 8664 } 8665 return this; 8666 } 8667 8668 /** 8669 * @return {@link #amount} (Payable less any payment adjustment.) 8670 */ 8671 public Money getAmount() { 8672 if (this.amount == null) 8673 if (Configuration.errorOnAutoCreate()) 8674 throw new Error("Attempt to auto-create PaymentComponent.amount"); 8675 else if (Configuration.doAutoCreate()) 8676 this.amount = new Money(); // cc 8677 return this.amount; 8678 } 8679 8680 public boolean hasAmount() { 8681 return this.amount != null && !this.amount.isEmpty(); 8682 } 8683 8684 /** 8685 * @param value {@link #amount} (Payable less any payment adjustment.) 8686 */ 8687 public PaymentComponent setAmount(Money value) { 8688 this.amount = value; 8689 return this; 8690 } 8691 8692 /** 8693 * @return {@link #identifier} (Payment identifer.) 8694 */ 8695 public Identifier getIdentifier() { 8696 if (this.identifier == null) 8697 if (Configuration.errorOnAutoCreate()) 8698 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 8699 else if (Configuration.doAutoCreate()) 8700 this.identifier = new Identifier(); // cc 8701 return this.identifier; 8702 } 8703 8704 public boolean hasIdentifier() { 8705 return this.identifier != null && !this.identifier.isEmpty(); 8706 } 8707 8708 /** 8709 * @param value {@link #identifier} (Payment identifer.) 8710 */ 8711 public PaymentComponent setIdentifier(Identifier value) { 8712 this.identifier = value; 8713 return this; 8714 } 8715 8716 protected void listChildren(List<Property> children) { 8717 super.listChildren(children); 8718 children.add(new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the claim.", 0, 1, type)); 8719 children.add(new Property("adjustment", "Money", "Adjustment to the payment of this transaction which is not related to adjudication of this transaction.", 0, 1, adjustment)); 8720 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason)); 8721 children.add(new Property("date", "date", "Estimated payment date.", 0, 1, date)); 8722 children.add(new Property("amount", "Money", "Payable less any payment adjustment.", 0, 1, amount)); 8723 children.add(new Property("identifier", "Identifier", "Payment identifer.", 0, 1, identifier)); 8724 } 8725 8726 @Override 8727 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8728 switch (_hash) { 8729 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the claim.", 0, 1, type); 8730 case 1977085293: /*adjustment*/ return new Property("adjustment", "Money", "Adjustment to the payment of this transaction which is not related to adjudication of this transaction.", 0, 1, adjustment); 8731 case -1255938543: /*adjustmentReason*/ return new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason); 8732 case 3076014: /*date*/ return new Property("date", "date", "Estimated payment date.", 0, 1, date); 8733 case -1413853096: /*amount*/ return new Property("amount", "Money", "Payable less any payment adjustment.", 0, 1, amount); 8734 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Payment identifer.", 0, 1, identifier); 8735 default: return super.getNamedProperty(_hash, _name, _checkValid); 8736 } 8737 8738 } 8739 8740 @Override 8741 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8742 switch (hash) { 8743 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 8744 case 1977085293: /*adjustment*/ return this.adjustment == null ? new Base[0] : new Base[] {this.adjustment}; // Money 8745 case -1255938543: /*adjustmentReason*/ return this.adjustmentReason == null ? new Base[0] : new Base[] {this.adjustmentReason}; // CodeableConcept 8746 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 8747 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 8748 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 8749 default: return super.getProperty(hash, name, checkValid); 8750 } 8751 8752 } 8753 8754 @Override 8755 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8756 switch (hash) { 8757 case 3575610: // type 8758 this.type = castToCodeableConcept(value); // CodeableConcept 8759 return value; 8760 case 1977085293: // adjustment 8761 this.adjustment = castToMoney(value); // Money 8762 return value; 8763 case -1255938543: // adjustmentReason 8764 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 8765 return value; 8766 case 3076014: // date 8767 this.date = castToDate(value); // DateType 8768 return value; 8769 case -1413853096: // amount 8770 this.amount = castToMoney(value); // Money 8771 return value; 8772 case -1618432855: // identifier 8773 this.identifier = castToIdentifier(value); // Identifier 8774 return value; 8775 default: return super.setProperty(hash, name, value); 8776 } 8777 8778 } 8779 8780 @Override 8781 public Base setProperty(String name, Base value) throws FHIRException { 8782 if (name.equals("type")) { 8783 this.type = castToCodeableConcept(value); // CodeableConcept 8784 } else if (name.equals("adjustment")) { 8785 this.adjustment = castToMoney(value); // Money 8786 } else if (name.equals("adjustmentReason")) { 8787 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 8788 } else if (name.equals("date")) { 8789 this.date = castToDate(value); // DateType 8790 } else if (name.equals("amount")) { 8791 this.amount = castToMoney(value); // Money 8792 } else if (name.equals("identifier")) { 8793 this.identifier = castToIdentifier(value); // Identifier 8794 } else 8795 return super.setProperty(name, value); 8796 return value; 8797 } 8798 8799 @Override 8800 public Base makeProperty(int hash, String name) throws FHIRException { 8801 switch (hash) { 8802 case 3575610: return getType(); 8803 case 1977085293: return getAdjustment(); 8804 case -1255938543: return getAdjustmentReason(); 8805 case 3076014: return getDateElement(); 8806 case -1413853096: return getAmount(); 8807 case -1618432855: return getIdentifier(); 8808 default: return super.makeProperty(hash, name); 8809 } 8810 8811 } 8812 8813 @Override 8814 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8815 switch (hash) { 8816 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 8817 case 1977085293: /*adjustment*/ return new String[] {"Money"}; 8818 case -1255938543: /*adjustmentReason*/ return new String[] {"CodeableConcept"}; 8819 case 3076014: /*date*/ return new String[] {"date"}; 8820 case -1413853096: /*amount*/ return new String[] {"Money"}; 8821 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 8822 default: return super.getTypesForProperty(hash, name); 8823 } 8824 8825 } 8826 8827 @Override 8828 public Base addChild(String name) throws FHIRException { 8829 if (name.equals("type")) { 8830 this.type = new CodeableConcept(); 8831 return this.type; 8832 } 8833 else if (name.equals("adjustment")) { 8834 this.adjustment = new Money(); 8835 return this.adjustment; 8836 } 8837 else if (name.equals("adjustmentReason")) { 8838 this.adjustmentReason = new CodeableConcept(); 8839 return this.adjustmentReason; 8840 } 8841 else if (name.equals("date")) { 8842 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 8843 } 8844 else if (name.equals("amount")) { 8845 this.amount = new Money(); 8846 return this.amount; 8847 } 8848 else if (name.equals("identifier")) { 8849 this.identifier = new Identifier(); 8850 return this.identifier; 8851 } 8852 else 8853 return super.addChild(name); 8854 } 8855 8856 public PaymentComponent copy() { 8857 PaymentComponent dst = new PaymentComponent(); 8858 copyValues(dst); 8859 dst.type = type == null ? null : type.copy(); 8860 dst.adjustment = adjustment == null ? null : adjustment.copy(); 8861 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 8862 dst.date = date == null ? null : date.copy(); 8863 dst.amount = amount == null ? null : amount.copy(); 8864 dst.identifier = identifier == null ? null : identifier.copy(); 8865 return dst; 8866 } 8867 8868 @Override 8869 public boolean equalsDeep(Base other_) { 8870 if (!super.equalsDeep(other_)) 8871 return false; 8872 if (!(other_ instanceof PaymentComponent)) 8873 return false; 8874 PaymentComponent o = (PaymentComponent) other_; 8875 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) && compareDeep(adjustmentReason, o.adjustmentReason, true) 8876 && compareDeep(date, o.date, true) && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true) 8877 ; 8878 } 8879 8880 @Override 8881 public boolean equalsShallow(Base other_) { 8882 if (!super.equalsShallow(other_)) 8883 return false; 8884 if (!(other_ instanceof PaymentComponent)) 8885 return false; 8886 PaymentComponent o = (PaymentComponent) other_; 8887 return compareValues(date, o.date, true); 8888 } 8889 8890 public boolean isEmpty() { 8891 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason 8892 , date, amount, identifier); 8893 } 8894 8895 public String fhirType() { 8896 return "ExplanationOfBenefit.payment"; 8897 8898 } 8899 8900 } 8901 8902 @Block() 8903 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 8904 /** 8905 * An integer associated with each note which may be referred to from each service line item. 8906 */ 8907 @Child(name = "number", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 8908 @Description(shortDefinition="Sequence number for this note", formalDefinition="An integer associated with each note which may be referred to from each service line item." ) 8909 protected PositiveIntType number; 8910 8911 /** 8912 * The note purpose: Print/Display. 8913 */ 8914 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 8915 @Description(shortDefinition="display | print | printoper", formalDefinition="The note purpose: Print/Display." ) 8916 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 8917 protected CodeableConcept type; 8918 8919 /** 8920 * The note text. 8921 */ 8922 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 8923 @Description(shortDefinition="Note explanitory text", formalDefinition="The note text." ) 8924 protected StringType text; 8925 8926 /** 8927 * The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English. 8928 */ 8929 @Child(name = "language", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 8930 @Description(shortDefinition="Language if different from the resource", formalDefinition="The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English." ) 8931 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 8932 protected CodeableConcept language; 8933 8934 private static final long serialVersionUID = -944255449L; 8935 8936 /** 8937 * Constructor 8938 */ 8939 public NoteComponent() { 8940 super(); 8941 } 8942 8943 /** 8944 * @return {@link #number} (An integer associated with each note which may be referred to from each service line item.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 8945 */ 8946 public PositiveIntType getNumberElement() { 8947 if (this.number == null) 8948 if (Configuration.errorOnAutoCreate()) 8949 throw new Error("Attempt to auto-create NoteComponent.number"); 8950 else if (Configuration.doAutoCreate()) 8951 this.number = new PositiveIntType(); // bb 8952 return this.number; 8953 } 8954 8955 public boolean hasNumberElement() { 8956 return this.number != null && !this.number.isEmpty(); 8957 } 8958 8959 public boolean hasNumber() { 8960 return this.number != null && !this.number.isEmpty(); 8961 } 8962 8963 /** 8964 * @param value {@link #number} (An integer associated with each note which may be referred to from each service line item.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 8965 */ 8966 public NoteComponent setNumberElement(PositiveIntType value) { 8967 this.number = value; 8968 return this; 8969 } 8970 8971 /** 8972 * @return An integer associated with each note which may be referred to from each service line item. 8973 */ 8974 public int getNumber() { 8975 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 8976 } 8977 8978 /** 8979 * @param value An integer associated with each note which may be referred to from each service line item. 8980 */ 8981 public NoteComponent setNumber(int value) { 8982 if (this.number == null) 8983 this.number = new PositiveIntType(); 8984 this.number.setValue(value); 8985 return this; 8986 } 8987 8988 /** 8989 * @return {@link #type} (The note purpose: Print/Display.) 8990 */ 8991 public CodeableConcept getType() { 8992 if (this.type == null) 8993 if (Configuration.errorOnAutoCreate()) 8994 throw new Error("Attempt to auto-create NoteComponent.type"); 8995 else if (Configuration.doAutoCreate()) 8996 this.type = new CodeableConcept(); // cc 8997 return this.type; 8998 } 8999 9000 public boolean hasType() { 9001 return this.type != null && !this.type.isEmpty(); 9002 } 9003 9004 /** 9005 * @param value {@link #type} (The note purpose: Print/Display.) 9006 */ 9007 public NoteComponent setType(CodeableConcept value) { 9008 this.type = value; 9009 return this; 9010 } 9011 9012 /** 9013 * @return {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 9014 */ 9015 public StringType getTextElement() { 9016 if (this.text == null) 9017 if (Configuration.errorOnAutoCreate()) 9018 throw new Error("Attempt to auto-create NoteComponent.text"); 9019 else if (Configuration.doAutoCreate()) 9020 this.text = new StringType(); // bb 9021 return this.text; 9022 } 9023 9024 public boolean hasTextElement() { 9025 return this.text != null && !this.text.isEmpty(); 9026 } 9027 9028 public boolean hasText() { 9029 return this.text != null && !this.text.isEmpty(); 9030 } 9031 9032 /** 9033 * @param value {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 9034 */ 9035 public NoteComponent setTextElement(StringType value) { 9036 this.text = value; 9037 return this; 9038 } 9039 9040 /** 9041 * @return The note text. 9042 */ 9043 public String getText() { 9044 return this.text == null ? null : this.text.getValue(); 9045 } 9046 9047 /** 9048 * @param value The note text. 9049 */ 9050 public NoteComponent setText(String value) { 9051 if (Utilities.noString(value)) 9052 this.text = null; 9053 else { 9054 if (this.text == null) 9055 this.text = new StringType(); 9056 this.text.setValue(value); 9057 } 9058 return this; 9059 } 9060 9061 /** 9062 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English.) 9063 */ 9064 public CodeableConcept getLanguage() { 9065 if (this.language == null) 9066 if (Configuration.errorOnAutoCreate()) 9067 throw new Error("Attempt to auto-create NoteComponent.language"); 9068 else if (Configuration.doAutoCreate()) 9069 this.language = new CodeableConcept(); // cc 9070 return this.language; 9071 } 9072 9073 public boolean hasLanguage() { 9074 return this.language != null && !this.language.isEmpty(); 9075 } 9076 9077 /** 9078 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English.) 9079 */ 9080 public NoteComponent setLanguage(CodeableConcept value) { 9081 this.language = value; 9082 return this; 9083 } 9084 9085 protected void listChildren(List<Property> children) { 9086 super.listChildren(children); 9087 children.add(new Property("number", "positiveInt", "An integer associated with each note which may be referred to from each service line item.", 0, 1, number)); 9088 children.add(new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type)); 9089 children.add(new Property("text", "string", "The note text.", 0, 1, text)); 9090 children.add(new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 0, 1, language)); 9091 } 9092 9093 @Override 9094 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9095 switch (_hash) { 9096 case -1034364087: /*number*/ return new Property("number", "positiveInt", "An integer associated with each note which may be referred to from each service line item.", 0, 1, number); 9097 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type); 9098 case 3556653: /*text*/ return new Property("text", "string", "The note text.", 0, 1, text); 9099 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 0, 1, language); 9100 default: return super.getNamedProperty(_hash, _name, _checkValid); 9101 } 9102 9103 } 9104 9105 @Override 9106 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9107 switch (hash) { 9108 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // PositiveIntType 9109 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 9110 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 9111 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 9112 default: return super.getProperty(hash, name, checkValid); 9113 } 9114 9115 } 9116 9117 @Override 9118 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9119 switch (hash) { 9120 case -1034364087: // number 9121 this.number = castToPositiveInt(value); // PositiveIntType 9122 return value; 9123 case 3575610: // type 9124 this.type = castToCodeableConcept(value); // CodeableConcept 9125 return value; 9126 case 3556653: // text 9127 this.text = castToString(value); // StringType 9128 return value; 9129 case -1613589672: // language 9130 this.language = castToCodeableConcept(value); // CodeableConcept 9131 return value; 9132 default: return super.setProperty(hash, name, value); 9133 } 9134 9135 } 9136 9137 @Override 9138 public Base setProperty(String name, Base value) throws FHIRException { 9139 if (name.equals("number")) { 9140 this.number = castToPositiveInt(value); // PositiveIntType 9141 } else if (name.equals("type")) { 9142 this.type = castToCodeableConcept(value); // CodeableConcept 9143 } else if (name.equals("text")) { 9144 this.text = castToString(value); // StringType 9145 } else if (name.equals("language")) { 9146 this.language = castToCodeableConcept(value); // CodeableConcept 9147 } else 9148 return super.setProperty(name, value); 9149 return value; 9150 } 9151 9152 @Override 9153 public Base makeProperty(int hash, String name) throws FHIRException { 9154 switch (hash) { 9155 case -1034364087: return getNumberElement(); 9156 case 3575610: return getType(); 9157 case 3556653: return getTextElement(); 9158 case -1613589672: return getLanguage(); 9159 default: return super.makeProperty(hash, name); 9160 } 9161 9162 } 9163 9164 @Override 9165 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9166 switch (hash) { 9167 case -1034364087: /*number*/ return new String[] {"positiveInt"}; 9168 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 9169 case 3556653: /*text*/ return new String[] {"string"}; 9170 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 9171 default: return super.getTypesForProperty(hash, name); 9172 } 9173 9174 } 9175 9176 @Override 9177 public Base addChild(String name) throws FHIRException { 9178 if (name.equals("number")) { 9179 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.number"); 9180 } 9181 else if (name.equals("type")) { 9182 this.type = new CodeableConcept(); 9183 return this.type; 9184 } 9185 else if (name.equals("text")) { 9186 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.text"); 9187 } 9188 else if (name.equals("language")) { 9189 this.language = new CodeableConcept(); 9190 return this.language; 9191 } 9192 else 9193 return super.addChild(name); 9194 } 9195 9196 public NoteComponent copy() { 9197 NoteComponent dst = new NoteComponent(); 9198 copyValues(dst); 9199 dst.number = number == null ? null : number.copy(); 9200 dst.type = type == null ? null : type.copy(); 9201 dst.text = text == null ? null : text.copy(); 9202 dst.language = language == null ? null : language.copy(); 9203 return dst; 9204 } 9205 9206 @Override 9207 public boolean equalsDeep(Base other_) { 9208 if (!super.equalsDeep(other_)) 9209 return false; 9210 if (!(other_ instanceof NoteComponent)) 9211 return false; 9212 NoteComponent o = (NoteComponent) other_; 9213 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 9214 && compareDeep(language, o.language, true); 9215 } 9216 9217 @Override 9218 public boolean equalsShallow(Base other_) { 9219 if (!super.equalsShallow(other_)) 9220 return false; 9221 if (!(other_ instanceof NoteComponent)) 9222 return false; 9223 NoteComponent o = (NoteComponent) other_; 9224 return compareValues(number, o.number, true) && compareValues(text, o.text, true); 9225 } 9226 9227 public boolean isEmpty() { 9228 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language 9229 ); 9230 } 9231 9232 public String fhirType() { 9233 return "ExplanationOfBenefit.processNote"; 9234 9235 } 9236 9237 } 9238 9239 @Block() 9240 public static class BenefitBalanceComponent extends BackboneElement implements IBaseBackboneElement { 9241 /** 9242 * Dental, Vision, Medical, Pharmacy, Rehab etc. 9243 */ 9244 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 9245 @Description(shortDefinition="Type of services covered", formalDefinition="Dental, Vision, Medical, Pharmacy, Rehab etc." ) 9246 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-category") 9247 protected CodeableConcept category; 9248 9249 /** 9250 * Dental: basic, major, ortho; Vision exam, glasses, contacts; etc. 9251 */ 9252 @Child(name = "subCategory", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 9253 @Description(shortDefinition="Detailed services covered within the type", formalDefinition="Dental: basic, major, ortho; Vision exam, glasses, contacts; etc." ) 9254 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 9255 protected CodeableConcept subCategory; 9256 9257 /** 9258 * True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 9259 */ 9260 @Child(name = "excluded", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 9261 @Description(shortDefinition="Excluded from the plan", formalDefinition="True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage." ) 9262 protected BooleanType excluded; 9263 9264 /** 9265 * A short name or tag for the benefit, for example MED01, or DENT2. 9266 */ 9267 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 9268 @Description(shortDefinition="Short name for the benefit", formalDefinition="A short name or tag for the benefit, for example MED01, or DENT2." ) 9269 protected StringType name; 9270 9271 /** 9272 * A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 9273 */ 9274 @Child(name = "description", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 9275 @Description(shortDefinition="Description of the benefit or services covered", formalDefinition="A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'." ) 9276 protected StringType description; 9277 9278 /** 9279 * Network designation. 9280 */ 9281 @Child(name = "network", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 9282 @Description(shortDefinition="In or out of network", formalDefinition="Network designation." ) 9283 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-network") 9284 protected CodeableConcept network; 9285 9286 /** 9287 * Unit designation: individual or family. 9288 */ 9289 @Child(name = "unit", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 9290 @Description(shortDefinition="Individual or family", formalDefinition="Unit designation: individual or family." ) 9291 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-unit") 9292 protected CodeableConcept unit; 9293 9294 /** 9295 * The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'. 9296 */ 9297 @Child(name = "term", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 9298 @Description(shortDefinition="Annual or lifetime", formalDefinition="The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'." ) 9299 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-term") 9300 protected CodeableConcept term; 9301 9302 /** 9303 * Benefits Used to date. 9304 */ 9305 @Child(name = "financial", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9306 @Description(shortDefinition="Benefit Summary", formalDefinition="Benefits Used to date." ) 9307 protected List<BenefitComponent> financial; 9308 9309 private static final long serialVersionUID = 833826021L; 9310 9311 /** 9312 * Constructor 9313 */ 9314 public BenefitBalanceComponent() { 9315 super(); 9316 } 9317 9318 /** 9319 * Constructor 9320 */ 9321 public BenefitBalanceComponent(CodeableConcept category) { 9322 super(); 9323 this.category = category; 9324 } 9325 9326 /** 9327 * @return {@link #category} (Dental, Vision, Medical, Pharmacy, Rehab etc.) 9328 */ 9329 public CodeableConcept getCategory() { 9330 if (this.category == null) 9331 if (Configuration.errorOnAutoCreate()) 9332 throw new Error("Attempt to auto-create BenefitBalanceComponent.category"); 9333 else if (Configuration.doAutoCreate()) 9334 this.category = new CodeableConcept(); // cc 9335 return this.category; 9336 } 9337 9338 public boolean hasCategory() { 9339 return this.category != null && !this.category.isEmpty(); 9340 } 9341 9342 /** 9343 * @param value {@link #category} (Dental, Vision, Medical, Pharmacy, Rehab etc.) 9344 */ 9345 public BenefitBalanceComponent setCategory(CodeableConcept value) { 9346 this.category = value; 9347 return this; 9348 } 9349 9350 /** 9351 * @return {@link #subCategory} (Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.) 9352 */ 9353 public CodeableConcept getSubCategory() { 9354 if (this.subCategory == null) 9355 if (Configuration.errorOnAutoCreate()) 9356 throw new Error("Attempt to auto-create BenefitBalanceComponent.subCategory"); 9357 else if (Configuration.doAutoCreate()) 9358 this.subCategory = new CodeableConcept(); // cc 9359 return this.subCategory; 9360 } 9361 9362 public boolean hasSubCategory() { 9363 return this.subCategory != null && !this.subCategory.isEmpty(); 9364 } 9365 9366 /** 9367 * @param value {@link #subCategory} (Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.) 9368 */ 9369 public BenefitBalanceComponent setSubCategory(CodeableConcept value) { 9370 this.subCategory = value; 9371 return this; 9372 } 9373 9374 /** 9375 * @return {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 9376 */ 9377 public BooleanType getExcludedElement() { 9378 if (this.excluded == null) 9379 if (Configuration.errorOnAutoCreate()) 9380 throw new Error("Attempt to auto-create BenefitBalanceComponent.excluded"); 9381 else if (Configuration.doAutoCreate()) 9382 this.excluded = new BooleanType(); // bb 9383 return this.excluded; 9384 } 9385 9386 public boolean hasExcludedElement() { 9387 return this.excluded != null && !this.excluded.isEmpty(); 9388 } 9389 9390 public boolean hasExcluded() { 9391 return this.excluded != null && !this.excluded.isEmpty(); 9392 } 9393 9394 /** 9395 * @param value {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 9396 */ 9397 public BenefitBalanceComponent setExcludedElement(BooleanType value) { 9398 this.excluded = value; 9399 return this; 9400 } 9401 9402 /** 9403 * @return True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 9404 */ 9405 public boolean getExcluded() { 9406 return this.excluded == null || this.excluded.isEmpty() ? false : this.excluded.getValue(); 9407 } 9408 9409 /** 9410 * @param value True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 9411 */ 9412 public BenefitBalanceComponent setExcluded(boolean value) { 9413 if (this.excluded == null) 9414 this.excluded = new BooleanType(); 9415 this.excluded.setValue(value); 9416 return this; 9417 } 9418 9419 /** 9420 * @return {@link #name} (A short name or tag for the benefit, for example MED01, or DENT2.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9421 */ 9422 public StringType getNameElement() { 9423 if (this.name == null) 9424 if (Configuration.errorOnAutoCreate()) 9425 throw new Error("Attempt to auto-create BenefitBalanceComponent.name"); 9426 else if (Configuration.doAutoCreate()) 9427 this.name = new StringType(); // bb 9428 return this.name; 9429 } 9430 9431 public boolean hasNameElement() { 9432 return this.name != null && !this.name.isEmpty(); 9433 } 9434 9435 public boolean hasName() { 9436 return this.name != null && !this.name.isEmpty(); 9437 } 9438 9439 /** 9440 * @param value {@link #name} (A short name or tag for the benefit, for example MED01, or DENT2.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9441 */ 9442 public BenefitBalanceComponent setNameElement(StringType value) { 9443 this.name = value; 9444 return this; 9445 } 9446 9447 /** 9448 * @return A short name or tag for the benefit, for example MED01, or DENT2. 9449 */ 9450 public String getName() { 9451 return this.name == null ? null : this.name.getValue(); 9452 } 9453 9454 /** 9455 * @param value A short name or tag for the benefit, for example MED01, or DENT2. 9456 */ 9457 public BenefitBalanceComponent setName(String value) { 9458 if (Utilities.noString(value)) 9459 this.name = null; 9460 else { 9461 if (this.name == null) 9462 this.name = new StringType(); 9463 this.name.setValue(value); 9464 } 9465 return this; 9466 } 9467 9468 /** 9469 * @return {@link #description} (A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 9470 */ 9471 public StringType getDescriptionElement() { 9472 if (this.description == null) 9473 if (Configuration.errorOnAutoCreate()) 9474 throw new Error("Attempt to auto-create BenefitBalanceComponent.description"); 9475 else if (Configuration.doAutoCreate()) 9476 this.description = new StringType(); // bb 9477 return this.description; 9478 } 9479 9480 public boolean hasDescriptionElement() { 9481 return this.description != null && !this.description.isEmpty(); 9482 } 9483 9484 public boolean hasDescription() { 9485 return this.description != null && !this.description.isEmpty(); 9486 } 9487 9488 /** 9489 * @param value {@link #description} (A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 9490 */ 9491 public BenefitBalanceComponent setDescriptionElement(StringType value) { 9492 this.description = value; 9493 return this; 9494 } 9495 9496 /** 9497 * @return A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 9498 */ 9499 public String getDescription() { 9500 return this.description == null ? null : this.description.getValue(); 9501 } 9502 9503 /** 9504 * @param value A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 9505 */ 9506 public BenefitBalanceComponent setDescription(String value) { 9507 if (Utilities.noString(value)) 9508 this.description = null; 9509 else { 9510 if (this.description == null) 9511 this.description = new StringType(); 9512 this.description.setValue(value); 9513 } 9514 return this; 9515 } 9516 9517 /** 9518 * @return {@link #network} (Network designation.) 9519 */ 9520 public CodeableConcept getNetwork() { 9521 if (this.network == null) 9522 if (Configuration.errorOnAutoCreate()) 9523 throw new Error("Attempt to auto-create BenefitBalanceComponent.network"); 9524 else if (Configuration.doAutoCreate()) 9525 this.network = new CodeableConcept(); // cc 9526 return this.network; 9527 } 9528 9529 public boolean hasNetwork() { 9530 return this.network != null && !this.network.isEmpty(); 9531 } 9532 9533 /** 9534 * @param value {@link #network} (Network designation.) 9535 */ 9536 public BenefitBalanceComponent setNetwork(CodeableConcept value) { 9537 this.network = value; 9538 return this; 9539 } 9540 9541 /** 9542 * @return {@link #unit} (Unit designation: individual or family.) 9543 */ 9544 public CodeableConcept getUnit() { 9545 if (this.unit == null) 9546 if (Configuration.errorOnAutoCreate()) 9547 throw new Error("Attempt to auto-create BenefitBalanceComponent.unit"); 9548 else if (Configuration.doAutoCreate()) 9549 this.unit = new CodeableConcept(); // cc 9550 return this.unit; 9551 } 9552 9553 public boolean hasUnit() { 9554 return this.unit != null && !this.unit.isEmpty(); 9555 } 9556 9557 /** 9558 * @param value {@link #unit} (Unit designation: individual or family.) 9559 */ 9560 public BenefitBalanceComponent setUnit(CodeableConcept value) { 9561 this.unit = value; 9562 return this; 9563 } 9564 9565 /** 9566 * @return {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.) 9567 */ 9568 public CodeableConcept getTerm() { 9569 if (this.term == null) 9570 if (Configuration.errorOnAutoCreate()) 9571 throw new Error("Attempt to auto-create BenefitBalanceComponent.term"); 9572 else if (Configuration.doAutoCreate()) 9573 this.term = new CodeableConcept(); // cc 9574 return this.term; 9575 } 9576 9577 public boolean hasTerm() { 9578 return this.term != null && !this.term.isEmpty(); 9579 } 9580 9581 /** 9582 * @param value {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.) 9583 */ 9584 public BenefitBalanceComponent setTerm(CodeableConcept value) { 9585 this.term = value; 9586 return this; 9587 } 9588 9589 /** 9590 * @return {@link #financial} (Benefits Used to date.) 9591 */ 9592 public List<BenefitComponent> getFinancial() { 9593 if (this.financial == null) 9594 this.financial = new ArrayList<BenefitComponent>(); 9595 return this.financial; 9596 } 9597 9598 /** 9599 * @return Returns a reference to <code>this</code> for easy method chaining 9600 */ 9601 public BenefitBalanceComponent setFinancial(List<BenefitComponent> theFinancial) { 9602 this.financial = theFinancial; 9603 return this; 9604 } 9605 9606 public boolean hasFinancial() { 9607 if (this.financial == null) 9608 return false; 9609 for (BenefitComponent item : this.financial) 9610 if (!item.isEmpty()) 9611 return true; 9612 return false; 9613 } 9614 9615 public BenefitComponent addFinancial() { //3 9616 BenefitComponent t = new BenefitComponent(); 9617 if (this.financial == null) 9618 this.financial = new ArrayList<BenefitComponent>(); 9619 this.financial.add(t); 9620 return t; 9621 } 9622 9623 public BenefitBalanceComponent addFinancial(BenefitComponent t) { //3 9624 if (t == null) 9625 return this; 9626 if (this.financial == null) 9627 this.financial = new ArrayList<BenefitComponent>(); 9628 this.financial.add(t); 9629 return this; 9630 } 9631 9632 /** 9633 * @return The first repetition of repeating field {@link #financial}, creating it if it does not already exist 9634 */ 9635 public BenefitComponent getFinancialFirstRep() { 9636 if (getFinancial().isEmpty()) { 9637 addFinancial(); 9638 } 9639 return getFinancial().get(0); 9640 } 9641 9642 protected void listChildren(List<Property> children) { 9643 super.listChildren(children); 9644 children.add(new Property("category", "CodeableConcept", "Dental, Vision, Medical, Pharmacy, Rehab etc.", 0, 1, category)); 9645 children.add(new Property("subCategory", "CodeableConcept", "Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.", 0, 1, subCategory)); 9646 children.add(new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.", 0, 1, excluded)); 9647 children.add(new Property("name", "string", "A short name or tag for the benefit, for example MED01, or DENT2.", 0, 1, name)); 9648 children.add(new Property("description", "string", "A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.", 0, 1, description)); 9649 children.add(new Property("network", "CodeableConcept", "Network designation.", 0, 1, network)); 9650 children.add(new Property("unit", "CodeableConcept", "Unit designation: individual or family.", 0, 1, unit)); 9651 children.add(new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.", 0, 1, term)); 9652 children.add(new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial)); 9653 } 9654 9655 @Override 9656 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9657 switch (_hash) { 9658 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Dental, Vision, Medical, Pharmacy, Rehab etc.", 0, 1, category); 9659 case 1365024606: /*subCategory*/ return new Property("subCategory", "CodeableConcept", "Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.", 0, 1, subCategory); 9660 case 1994055114: /*excluded*/ return new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.", 0, 1, excluded); 9661 case 3373707: /*name*/ return new Property("name", "string", "A short name or tag for the benefit, for example MED01, or DENT2.", 0, 1, name); 9662 case -1724546052: /*description*/ return new Property("description", "string", "A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.", 0, 1, description); 9663 case 1843485230: /*network*/ return new Property("network", "CodeableConcept", "Network designation.", 0, 1, network); 9664 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Unit designation: individual or family.", 0, 1, unit); 9665 case 3556460: /*term*/ return new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.", 0, 1, term); 9666 case 357555337: /*financial*/ return new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial); 9667 default: return super.getNamedProperty(_hash, _name, _checkValid); 9668 } 9669 9670 } 9671 9672 @Override 9673 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9674 switch (hash) { 9675 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 9676 case 1365024606: /*subCategory*/ return this.subCategory == null ? new Base[0] : new Base[] {this.subCategory}; // CodeableConcept 9677 case 1994055114: /*excluded*/ return this.excluded == null ? new Base[0] : new Base[] {this.excluded}; // BooleanType 9678 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 9679 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 9680 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // CodeableConcept 9681 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 9682 case 3556460: /*term*/ return this.term == null ? new Base[0] : new Base[] {this.term}; // CodeableConcept 9683 case 357555337: /*financial*/ return this.financial == null ? new Base[0] : this.financial.toArray(new Base[this.financial.size()]); // BenefitComponent 9684 default: return super.getProperty(hash, name, checkValid); 9685 } 9686 9687 } 9688 9689 @Override 9690 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9691 switch (hash) { 9692 case 50511102: // category 9693 this.category = castToCodeableConcept(value); // CodeableConcept 9694 return value; 9695 case 1365024606: // subCategory 9696 this.subCategory = castToCodeableConcept(value); // CodeableConcept 9697 return value; 9698 case 1994055114: // excluded 9699 this.excluded = castToBoolean(value); // BooleanType 9700 return value; 9701 case 3373707: // name 9702 this.name = castToString(value); // StringType 9703 return value; 9704 case -1724546052: // description 9705 this.description = castToString(value); // StringType 9706 return value; 9707 case 1843485230: // network 9708 this.network = castToCodeableConcept(value); // CodeableConcept 9709 return value; 9710 case 3594628: // unit 9711 this.unit = castToCodeableConcept(value); // CodeableConcept 9712 return value; 9713 case 3556460: // term 9714 this.term = castToCodeableConcept(value); // CodeableConcept 9715 return value; 9716 case 357555337: // financial 9717 this.getFinancial().add((BenefitComponent) value); // BenefitComponent 9718 return value; 9719 default: return super.setProperty(hash, name, value); 9720 } 9721 9722 } 9723 9724 @Override 9725 public Base setProperty(String name, Base value) throws FHIRException { 9726 if (name.equals("category")) { 9727 this.category = castToCodeableConcept(value); // CodeableConcept 9728 } else if (name.equals("subCategory")) { 9729 this.subCategory = castToCodeableConcept(value); // CodeableConcept 9730 } else if (name.equals("excluded")) { 9731 this.excluded = castToBoolean(value); // BooleanType 9732 } else if (name.equals("name")) { 9733 this.name = castToString(value); // StringType 9734 } else if (name.equals("description")) { 9735 this.description = castToString(value); // StringType 9736 } else if (name.equals("network")) { 9737 this.network = castToCodeableConcept(value); // CodeableConcept 9738 } else if (name.equals("unit")) { 9739 this.unit = castToCodeableConcept(value); // CodeableConcept 9740 } else if (name.equals("term")) { 9741 this.term = castToCodeableConcept(value); // CodeableConcept 9742 } else if (name.equals("financial")) { 9743 this.getFinancial().add((BenefitComponent) value); 9744 } else 9745 return super.setProperty(name, value); 9746 return value; 9747 } 9748 9749 @Override 9750 public Base makeProperty(int hash, String name) throws FHIRException { 9751 switch (hash) { 9752 case 50511102: return getCategory(); 9753 case 1365024606: return getSubCategory(); 9754 case 1994055114: return getExcludedElement(); 9755 case 3373707: return getNameElement(); 9756 case -1724546052: return getDescriptionElement(); 9757 case 1843485230: return getNetwork(); 9758 case 3594628: return getUnit(); 9759 case 3556460: return getTerm(); 9760 case 357555337: return addFinancial(); 9761 default: return super.makeProperty(hash, name); 9762 } 9763 9764 } 9765 9766 @Override 9767 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9768 switch (hash) { 9769 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 9770 case 1365024606: /*subCategory*/ return new String[] {"CodeableConcept"}; 9771 case 1994055114: /*excluded*/ return new String[] {"boolean"}; 9772 case 3373707: /*name*/ return new String[] {"string"}; 9773 case -1724546052: /*description*/ return new String[] {"string"}; 9774 case 1843485230: /*network*/ return new String[] {"CodeableConcept"}; 9775 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 9776 case 3556460: /*term*/ return new String[] {"CodeableConcept"}; 9777 case 357555337: /*financial*/ return new String[] {}; 9778 default: return super.getTypesForProperty(hash, name); 9779 } 9780 9781 } 9782 9783 @Override 9784 public Base addChild(String name) throws FHIRException { 9785 if (name.equals("category")) { 9786 this.category = new CodeableConcept(); 9787 return this.category; 9788 } 9789 else if (name.equals("subCategory")) { 9790 this.subCategory = new CodeableConcept(); 9791 return this.subCategory; 9792 } 9793 else if (name.equals("excluded")) { 9794 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.excluded"); 9795 } 9796 else if (name.equals("name")) { 9797 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.name"); 9798 } 9799 else if (name.equals("description")) { 9800 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.description"); 9801 } 9802 else if (name.equals("network")) { 9803 this.network = new CodeableConcept(); 9804 return this.network; 9805 } 9806 else if (name.equals("unit")) { 9807 this.unit = new CodeableConcept(); 9808 return this.unit; 9809 } 9810 else if (name.equals("term")) { 9811 this.term = new CodeableConcept(); 9812 return this.term; 9813 } 9814 else if (name.equals("financial")) { 9815 return addFinancial(); 9816 } 9817 else 9818 return super.addChild(name); 9819 } 9820 9821 public BenefitBalanceComponent copy() { 9822 BenefitBalanceComponent dst = new BenefitBalanceComponent(); 9823 copyValues(dst); 9824 dst.category = category == null ? null : category.copy(); 9825 dst.subCategory = subCategory == null ? null : subCategory.copy(); 9826 dst.excluded = excluded == null ? null : excluded.copy(); 9827 dst.name = name == null ? null : name.copy(); 9828 dst.description = description == null ? null : description.copy(); 9829 dst.network = network == null ? null : network.copy(); 9830 dst.unit = unit == null ? null : unit.copy(); 9831 dst.term = term == null ? null : term.copy(); 9832 if (financial != null) { 9833 dst.financial = new ArrayList<BenefitComponent>(); 9834 for (BenefitComponent i : financial) 9835 dst.financial.add(i.copy()); 9836 }; 9837 return dst; 9838 } 9839 9840 @Override 9841 public boolean equalsDeep(Base other_) { 9842 if (!super.equalsDeep(other_)) 9843 return false; 9844 if (!(other_ instanceof BenefitBalanceComponent)) 9845 return false; 9846 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 9847 return compareDeep(category, o.category, true) && compareDeep(subCategory, o.subCategory, true) 9848 && compareDeep(excluded, o.excluded, true) && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 9849 && compareDeep(network, o.network, true) && compareDeep(unit, o.unit, true) && compareDeep(term, o.term, true) 9850 && compareDeep(financial, o.financial, true); 9851 } 9852 9853 @Override 9854 public boolean equalsShallow(Base other_) { 9855 if (!super.equalsShallow(other_)) 9856 return false; 9857 if (!(other_ instanceof BenefitBalanceComponent)) 9858 return false; 9859 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 9860 return compareValues(excluded, o.excluded, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 9861 ; 9862 } 9863 9864 public boolean isEmpty() { 9865 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, subCategory, excluded 9866 , name, description, network, unit, term, financial); 9867 } 9868 9869 public String fhirType() { 9870 return "ExplanationOfBenefit.benefitBalance"; 9871 9872 } 9873 9874 } 9875 9876 @Block() 9877 public static class BenefitComponent extends BackboneElement implements IBaseBackboneElement { 9878 /** 9879 * Deductable, visits, benefit amount. 9880 */ 9881 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 9882 @Description(shortDefinition="Deductable, visits, benefit amount", formalDefinition="Deductable, visits, benefit amount." ) 9883 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-type") 9884 protected CodeableConcept type; 9885 9886 /** 9887 * Benefits allowed. 9888 */ 9889 @Child(name = "allowed", type = {UnsignedIntType.class, StringType.class, Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 9890 @Description(shortDefinition="Benefits allowed", formalDefinition="Benefits allowed." ) 9891 protected Type allowed; 9892 9893 /** 9894 * Benefits used. 9895 */ 9896 @Child(name = "used", type = {UnsignedIntType.class, Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 9897 @Description(shortDefinition="Benefits used", formalDefinition="Benefits used." ) 9898 protected Type used; 9899 9900 private static final long serialVersionUID = -1506285314L; 9901 9902 /** 9903 * Constructor 9904 */ 9905 public BenefitComponent() { 9906 super(); 9907 } 9908 9909 /** 9910 * Constructor 9911 */ 9912 public BenefitComponent(CodeableConcept type) { 9913 super(); 9914 this.type = type; 9915 } 9916 9917 /** 9918 * @return {@link #type} (Deductable, visits, benefit amount.) 9919 */ 9920 public CodeableConcept getType() { 9921 if (this.type == null) 9922 if (Configuration.errorOnAutoCreate()) 9923 throw new Error("Attempt to auto-create BenefitComponent.type"); 9924 else if (Configuration.doAutoCreate()) 9925 this.type = new CodeableConcept(); // cc 9926 return this.type; 9927 } 9928 9929 public boolean hasType() { 9930 return this.type != null && !this.type.isEmpty(); 9931 } 9932 9933 /** 9934 * @param value {@link #type} (Deductable, visits, benefit amount.) 9935 */ 9936 public BenefitComponent setType(CodeableConcept value) { 9937 this.type = value; 9938 return this; 9939 } 9940 9941 /** 9942 * @return {@link #allowed} (Benefits allowed.) 9943 */ 9944 public Type getAllowed() { 9945 return this.allowed; 9946 } 9947 9948 /** 9949 * @return {@link #allowed} (Benefits allowed.) 9950 */ 9951 public UnsignedIntType getAllowedUnsignedIntType() throws FHIRException { 9952 if (this.allowed == null) 9953 return null; 9954 if (!(this.allowed instanceof UnsignedIntType)) 9955 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 9956 return (UnsignedIntType) this.allowed; 9957 } 9958 9959 public boolean hasAllowedUnsignedIntType() { 9960 return this != null && this.allowed instanceof UnsignedIntType; 9961 } 9962 9963 /** 9964 * @return {@link #allowed} (Benefits allowed.) 9965 */ 9966 public StringType getAllowedStringType() throws FHIRException { 9967 if (this.allowed == null) 9968 return null; 9969 if (!(this.allowed instanceof StringType)) 9970 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 9971 return (StringType) this.allowed; 9972 } 9973 9974 public boolean hasAllowedStringType() { 9975 return this != null && this.allowed instanceof StringType; 9976 } 9977 9978 /** 9979 * @return {@link #allowed} (Benefits allowed.) 9980 */ 9981 public Money getAllowedMoney() throws FHIRException { 9982 if (this.allowed == null) 9983 return null; 9984 if (!(this.allowed instanceof Money)) 9985 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.allowed.getClass().getName()+" was encountered"); 9986 return (Money) this.allowed; 9987 } 9988 9989 public boolean hasAllowedMoney() { 9990 return this != null && this.allowed instanceof Money; 9991 } 9992 9993 public boolean hasAllowed() { 9994 return this.allowed != null && !this.allowed.isEmpty(); 9995 } 9996 9997 /** 9998 * @param value {@link #allowed} (Benefits allowed.) 9999 */ 10000 public BenefitComponent setAllowed(Type value) throws FHIRFormatError { 10001 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 10002 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.benefitBalance.financial.allowed[x]: "+value.fhirType()); 10003 this.allowed = value; 10004 return this; 10005 } 10006 10007 /** 10008 * @return {@link #used} (Benefits used.) 10009 */ 10010 public Type getUsed() { 10011 return this.used; 10012 } 10013 10014 /** 10015 * @return {@link #used} (Benefits used.) 10016 */ 10017 public UnsignedIntType getUsedUnsignedIntType() throws FHIRException { 10018 if (this.used == null) 10019 return null; 10020 if (!(this.used instanceof UnsignedIntType)) 10021 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.used.getClass().getName()+" was encountered"); 10022 return (UnsignedIntType) this.used; 10023 } 10024 10025 public boolean hasUsedUnsignedIntType() { 10026 return this != null && this.used instanceof UnsignedIntType; 10027 } 10028 10029 /** 10030 * @return {@link #used} (Benefits used.) 10031 */ 10032 public Money getUsedMoney() throws FHIRException { 10033 if (this.used == null) 10034 return null; 10035 if (!(this.used instanceof Money)) 10036 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.used.getClass().getName()+" was encountered"); 10037 return (Money) this.used; 10038 } 10039 10040 public boolean hasUsedMoney() { 10041 return this != null && this.used instanceof Money; 10042 } 10043 10044 public boolean hasUsed() { 10045 return this.used != null && !this.used.isEmpty(); 10046 } 10047 10048 /** 10049 * @param value {@link #used} (Benefits used.) 10050 */ 10051 public BenefitComponent setUsed(Type value) throws FHIRFormatError { 10052 if (value != null && !(value instanceof UnsignedIntType || value instanceof Money)) 10053 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.benefitBalance.financial.used[x]: "+value.fhirType()); 10054 this.used = value; 10055 return this; 10056 } 10057 10058 protected void listChildren(List<Property> children) { 10059 super.listChildren(children); 10060 children.add(new Property("type", "CodeableConcept", "Deductable, visits, benefit amount.", 0, 1, type)); 10061 children.add(new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed)); 10062 children.add(new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used)); 10063 } 10064 10065 @Override 10066 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10067 switch (_hash) { 10068 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Deductable, visits, benefit amount.", 0, 1, type); 10069 case -1336663592: /*allowed[x]*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10070 case -911343192: /*allowed*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10071 case 1668802034: /*allowedUnsignedInt*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10072 case -2135265319: /*allowedString*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10073 case -351668232: /*allowedMoney*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10074 case -147553373: /*used[x]*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 10075 case 3599293: /*used*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 10076 case 1252740285: /*usedUnsignedInt*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 10077 case -78048509: /*usedMoney*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 10078 default: return super.getNamedProperty(_hash, _name, _checkValid); 10079 } 10080 10081 } 10082 10083 @Override 10084 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10085 switch (hash) { 10086 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 10087 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // Type 10088 case 3599293: /*used*/ return this.used == null ? new Base[0] : new Base[] {this.used}; // Type 10089 default: return super.getProperty(hash, name, checkValid); 10090 } 10091 10092 } 10093 10094 @Override 10095 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10096 switch (hash) { 10097 case 3575610: // type 10098 this.type = castToCodeableConcept(value); // CodeableConcept 10099 return value; 10100 case -911343192: // allowed 10101 this.allowed = castToType(value); // Type 10102 return value; 10103 case 3599293: // used 10104 this.used = castToType(value); // Type 10105 return value; 10106 default: return super.setProperty(hash, name, value); 10107 } 10108 10109 } 10110 10111 @Override 10112 public Base setProperty(String name, Base value) throws FHIRException { 10113 if (name.equals("type")) { 10114 this.type = castToCodeableConcept(value); // CodeableConcept 10115 } else if (name.equals("allowed[x]")) { 10116 this.allowed = castToType(value); // Type 10117 } else if (name.equals("used[x]")) { 10118 this.used = castToType(value); // Type 10119 } else 10120 return super.setProperty(name, value); 10121 return value; 10122 } 10123 10124 @Override 10125 public Base makeProperty(int hash, String name) throws FHIRException { 10126 switch (hash) { 10127 case 3575610: return getType(); 10128 case -1336663592: return getAllowed(); 10129 case -911343192: return getAllowed(); 10130 case -147553373: return getUsed(); 10131 case 3599293: return getUsed(); 10132 default: return super.makeProperty(hash, name); 10133 } 10134 10135 } 10136 10137 @Override 10138 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10139 switch (hash) { 10140 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 10141 case -911343192: /*allowed*/ return new String[] {"unsignedInt", "string", "Money"}; 10142 case 3599293: /*used*/ return new String[] {"unsignedInt", "Money"}; 10143 default: return super.getTypesForProperty(hash, name); 10144 } 10145 10146 } 10147 10148 @Override 10149 public Base addChild(String name) throws FHIRException { 10150 if (name.equals("type")) { 10151 this.type = new CodeableConcept(); 10152 return this.type; 10153 } 10154 else if (name.equals("allowedUnsignedInt")) { 10155 this.allowed = new UnsignedIntType(); 10156 return this.allowed; 10157 } 10158 else if (name.equals("allowedString")) { 10159 this.allowed = new StringType(); 10160 return this.allowed; 10161 } 10162 else if (name.equals("allowedMoney")) { 10163 this.allowed = new Money(); 10164 return this.allowed; 10165 } 10166 else if (name.equals("usedUnsignedInt")) { 10167 this.used = new UnsignedIntType(); 10168 return this.used; 10169 } 10170 else if (name.equals("usedMoney")) { 10171 this.used = new Money(); 10172 return this.used; 10173 } 10174 else 10175 return super.addChild(name); 10176 } 10177 10178 public BenefitComponent copy() { 10179 BenefitComponent dst = new BenefitComponent(); 10180 copyValues(dst); 10181 dst.type = type == null ? null : type.copy(); 10182 dst.allowed = allowed == null ? null : allowed.copy(); 10183 dst.used = used == null ? null : used.copy(); 10184 return dst; 10185 } 10186 10187 @Override 10188 public boolean equalsDeep(Base other_) { 10189 if (!super.equalsDeep(other_)) 10190 return false; 10191 if (!(other_ instanceof BenefitComponent)) 10192 return false; 10193 BenefitComponent o = (BenefitComponent) other_; 10194 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true) && compareDeep(used, o.used, true) 10195 ; 10196 } 10197 10198 @Override 10199 public boolean equalsShallow(Base other_) { 10200 if (!super.equalsShallow(other_)) 10201 return false; 10202 if (!(other_ instanceof BenefitComponent)) 10203 return false; 10204 BenefitComponent o = (BenefitComponent) other_; 10205 return true; 10206 } 10207 10208 public boolean isEmpty() { 10209 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed, used); 10210 } 10211 10212 public String fhirType() { 10213 return "ExplanationOfBenefit.benefitBalance.financial"; 10214 10215 } 10216 10217 } 10218 10219 /** 10220 * The EOB Business Identifier. 10221 */ 10222 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10223 @Description(shortDefinition="Business Identifier", formalDefinition="The EOB Business Identifier." ) 10224 protected List<Identifier> identifier; 10225 10226 /** 10227 * The status of the resource instance. 10228 */ 10229 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 10230 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 10231 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/explanationofbenefit-status") 10232 protected Enumeration<ExplanationOfBenefitStatus> status; 10233 10234 /** 10235 * The category of claim, eg, oral, pharmacy, vision, insitutional, professional. 10236 */ 10237 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 10238 @Description(shortDefinition="Type or discipline", formalDefinition="The category of claim, eg, oral, pharmacy, vision, insitutional, professional." ) 10239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 10240 protected CodeableConcept type; 10241 10242 /** 10243 * A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType. 10244 */ 10245 @Child(name = "subType", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10246 @Description(shortDefinition="Finer grained claim type information", formalDefinition="A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType." ) 10247 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 10248 protected List<CodeableConcept> subType; 10249 10250 /** 10251 * Patient Resource. 10252 */ 10253 @Child(name = "patient", type = {Patient.class}, order=4, min=0, max=1, modifier=false, summary=false) 10254 @Description(shortDefinition="The subject of the Products and Services", formalDefinition="Patient Resource." ) 10255 protected Reference patient; 10256 10257 /** 10258 * The actual object that is the target of the reference (Patient Resource.) 10259 */ 10260 protected Patient patientTarget; 10261 10262 /** 10263 * The billable period for which charges are being submitted. 10264 */ 10265 @Child(name = "billablePeriod", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=false) 10266 @Description(shortDefinition="Period for charge submission", formalDefinition="The billable period for which charges are being submitted." ) 10267 protected Period billablePeriod; 10268 10269 /** 10270 * The date when the EOB was created. 10271 */ 10272 @Child(name = "created", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 10273 @Description(shortDefinition="Creation date", formalDefinition="The date when the EOB was created." ) 10274 protected DateTimeType created; 10275 10276 /** 10277 * The person who created the explanation of benefit. 10278 */ 10279 @Child(name = "enterer", type = {Practitioner.class}, order=7, min=0, max=1, modifier=false, summary=false) 10280 @Description(shortDefinition="Author", formalDefinition="The person who created the explanation of benefit." ) 10281 protected Reference enterer; 10282 10283 /** 10284 * The actual object that is the target of the reference (The person who created the explanation of benefit.) 10285 */ 10286 protected Practitioner entererTarget; 10287 10288 /** 10289 * The insurer which is responsible for the explanation of benefit. 10290 */ 10291 @Child(name = "insurer", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 10292 @Description(shortDefinition="Insurer responsible for the EOB", formalDefinition="The insurer which is responsible for the explanation of benefit." ) 10293 protected Reference insurer; 10294 10295 /** 10296 * The actual object that is the target of the reference (The insurer which is responsible for the explanation of benefit.) 10297 */ 10298 protected Organization insurerTarget; 10299 10300 /** 10301 * The provider which is responsible for the claim. 10302 */ 10303 @Child(name = "provider", type = {Practitioner.class}, order=9, min=0, max=1, modifier=false, summary=false) 10304 @Description(shortDefinition="Responsible provider for the claim", formalDefinition="The provider which is responsible for the claim." ) 10305 protected Reference provider; 10306 10307 /** 10308 * The actual object that is the target of the reference (The provider which is responsible for the claim.) 10309 */ 10310 protected Practitioner providerTarget; 10311 10312 /** 10313 * The provider which is responsible for the claim. 10314 */ 10315 @Child(name = "organization", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 10316 @Description(shortDefinition="Responsible organization for the claim", formalDefinition="The provider which is responsible for the claim." ) 10317 protected Reference organization; 10318 10319 /** 10320 * The actual object that is the target of the reference (The provider which is responsible for the claim.) 10321 */ 10322 protected Organization organizationTarget; 10323 10324 /** 10325 * The referral resource which lists the date, practitioner, reason and other supporting information. 10326 */ 10327 @Child(name = "referral", type = {ReferralRequest.class}, order=11, min=0, max=1, modifier=false, summary=false) 10328 @Description(shortDefinition="Treatment Referral", formalDefinition="The referral resource which lists the date, practitioner, reason and other supporting information." ) 10329 protected Reference referral; 10330 10331 /** 10332 * The actual object that is the target of the reference (The referral resource which lists the date, practitioner, reason and other supporting information.) 10333 */ 10334 protected ReferralRequest referralTarget; 10335 10336 /** 10337 * Facility where the services were provided. 10338 */ 10339 @Child(name = "facility", type = {Location.class}, order=12, min=0, max=1, modifier=false, summary=false) 10340 @Description(shortDefinition="Servicing Facility", formalDefinition="Facility where the services were provided." ) 10341 protected Reference facility; 10342 10343 /** 10344 * The actual object that is the target of the reference (Facility where the services were provided.) 10345 */ 10346 protected Location facilityTarget; 10347 10348 /** 10349 * The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number. 10350 */ 10351 @Child(name = "claim", type = {Claim.class}, order=13, min=0, max=1, modifier=false, summary=false) 10352 @Description(shortDefinition="Claim reference", formalDefinition="The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number." ) 10353 protected Reference claim; 10354 10355 /** 10356 * The actual object that is the target of the reference (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 10357 */ 10358 protected Claim claimTarget; 10359 10360 /** 10361 * The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number. 10362 */ 10363 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=14, min=0, max=1, modifier=false, summary=false) 10364 @Description(shortDefinition="Claim response reference", formalDefinition="The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number." ) 10365 protected Reference claimResponse; 10366 10367 /** 10368 * The actual object that is the target of the reference (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 10369 */ 10370 protected ClaimResponse claimResponseTarget; 10371 10372 /** 10373 * Processing outcome errror, partial or complete processing. 10374 */ 10375 @Child(name = "outcome", type = {CodeableConcept.class}, order=15, min=0, max=1, modifier=false, summary=false) 10376 @Description(shortDefinition="complete | error | partial", formalDefinition="Processing outcome errror, partial or complete processing." ) 10377 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/remittance-outcome") 10378 protected CodeableConcept outcome; 10379 10380 /** 10381 * A description of the status of the adjudication. 10382 */ 10383 @Child(name = "disposition", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 10384 @Description(shortDefinition="Disposition Message", formalDefinition="A description of the status of the adjudication." ) 10385 protected StringType disposition; 10386 10387 /** 10388 * Other claims which are related to this claim such as prior claim versions or for related services. 10389 */ 10390 @Child(name = "related", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10391 @Description(shortDefinition="Related Claims which may be revelant to processing this claim", formalDefinition="Other claims which are related to this claim such as prior claim versions or for related services." ) 10392 protected List<RelatedClaimComponent> related; 10393 10394 /** 10395 * Prescription to support the dispensing of Pharmacy or Vision products. 10396 */ 10397 @Child(name = "prescription", type = {MedicationRequest.class, VisionPrescription.class}, order=18, min=0, max=1, modifier=false, summary=false) 10398 @Description(shortDefinition="Prescription authorizing services or products", formalDefinition="Prescription to support the dispensing of Pharmacy or Vision products." ) 10399 protected Reference prescription; 10400 10401 /** 10402 * The actual object that is the target of the reference (Prescription to support the dispensing of Pharmacy or Vision products.) 10403 */ 10404 protected Resource prescriptionTarget; 10405 10406 /** 10407 * Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'. 10408 */ 10409 @Child(name = "originalPrescription", type = {MedicationRequest.class}, order=19, min=0, max=1, modifier=false, summary=false) 10410 @Description(shortDefinition="Original prescription if superceded by fulfiller", formalDefinition="Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'." ) 10411 protected Reference originalPrescription; 10412 10413 /** 10414 * The actual object that is the target of the reference (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 10415 */ 10416 protected MedicationRequest originalPrescriptionTarget; 10417 10418 /** 10419 * The party to be reimbursed for the services. 10420 */ 10421 @Child(name = "payee", type = {}, order=20, min=0, max=1, modifier=false, summary=false) 10422 @Description(shortDefinition="Party to be paid any benefits payable", formalDefinition="The party to be reimbursed for the services." ) 10423 protected PayeeComponent payee; 10424 10425 /** 10426 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required. 10427 */ 10428 @Child(name = "information", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10429 @Description(shortDefinition="Exceptions, special considerations, the condition, situation, prior or concurrent issues", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required." ) 10430 protected List<SupportingInformationComponent> information; 10431 10432 /** 10433 * The members of the team who provided the overall service as well as their role and whether responsible and qualifications. 10434 */ 10435 @Child(name = "careTeam", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10436 @Description(shortDefinition="Care Team members", formalDefinition="The members of the team who provided the overall service as well as their role and whether responsible and qualifications." ) 10437 protected List<CareTeamComponent> careTeam; 10438 10439 /** 10440 * Ordered list of patient diagnosis for which care is sought. 10441 */ 10442 @Child(name = "diagnosis", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10443 @Description(shortDefinition="List of Diagnosis", formalDefinition="Ordered list of patient diagnosis for which care is sought." ) 10444 protected List<DiagnosisComponent> diagnosis; 10445 10446 /** 10447 * Ordered list of patient procedures performed to support the adjudication. 10448 */ 10449 @Child(name = "procedure", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10450 @Description(shortDefinition="Procedures performed", formalDefinition="Ordered list of patient procedures performed to support the adjudication." ) 10451 protected List<ProcedureComponent> procedure; 10452 10453 /** 10454 * Precedence (primary, secondary, etc.). 10455 */ 10456 @Child(name = "precedence", type = {PositiveIntType.class}, order=25, min=0, max=1, modifier=false, summary=false) 10457 @Description(shortDefinition="Precedence (primary, secondary, etc.)", formalDefinition="Precedence (primary, secondary, etc.)." ) 10458 protected PositiveIntType precedence; 10459 10460 /** 10461 * Financial instrument by which payment information for health care. 10462 */ 10463 @Child(name = "insurance", type = {}, order=26, min=0, max=1, modifier=false, summary=false) 10464 @Description(shortDefinition="Insurance or medical plan", formalDefinition="Financial instrument by which payment information for health care." ) 10465 protected InsuranceComponent insurance; 10466 10467 /** 10468 * An accident which resulted in the need for healthcare services. 10469 */ 10470 @Child(name = "accident", type = {}, order=27, min=0, max=1, modifier=false, summary=false) 10471 @Description(shortDefinition="Details of an accident", formalDefinition="An accident which resulted in the need for healthcare services." ) 10472 protected AccidentComponent accident; 10473 10474 /** 10475 * The start and optional end dates of when the patient was precluded from working due to the treatable condition(s). 10476 */ 10477 @Child(name = "employmentImpacted", type = {Period.class}, order=28, min=0, max=1, modifier=false, summary=false) 10478 @Description(shortDefinition="Period unable to work", formalDefinition="The start and optional end dates of when the patient was precluded from working due to the treatable condition(s)." ) 10479 protected Period employmentImpacted; 10480 10481 /** 10482 * The start and optional end dates of when the patient was confined to a treatment center. 10483 */ 10484 @Child(name = "hospitalization", type = {Period.class}, order=29, min=0, max=1, modifier=false, summary=false) 10485 @Description(shortDefinition="Period in hospital", formalDefinition="The start and optional end dates of when the patient was confined to a treatment center." ) 10486 protected Period hospitalization; 10487 10488 /** 10489 * First tier of goods and services. 10490 */ 10491 @Child(name = "item", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10492 @Description(shortDefinition="Goods and Services", formalDefinition="First tier of goods and services." ) 10493 protected List<ItemComponent> item; 10494 10495 /** 10496 * The first tier service adjudications for payor added services. 10497 */ 10498 @Child(name = "addItem", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10499 @Description(shortDefinition="Insurer added line items", formalDefinition="The first tier service adjudications for payor added services." ) 10500 protected List<AddedItemComponent> addItem; 10501 10502 /** 10503 * The total cost of the services reported. 10504 */ 10505 @Child(name = "totalCost", type = {Money.class}, order=32, min=0, max=1, modifier=false, summary=false) 10506 @Description(shortDefinition="Total Cost of service from the Claim", formalDefinition="The total cost of the services reported." ) 10507 protected Money totalCost; 10508 10509 /** 10510 * The amount of deductable applied which was not allocated to any particular service line. 10511 */ 10512 @Child(name = "unallocDeductable", type = {Money.class}, order=33, min=0, max=1, modifier=false, summary=false) 10513 @Description(shortDefinition="Unallocated deductable", formalDefinition="The amount of deductable applied which was not allocated to any particular service line." ) 10514 protected Money unallocDeductable; 10515 10516 /** 10517 * Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable). 10518 */ 10519 @Child(name = "totalBenefit", type = {Money.class}, order=34, min=0, max=1, modifier=false, summary=false) 10520 @Description(shortDefinition="Total benefit payable for the Claim", formalDefinition="Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable)." ) 10521 protected Money totalBenefit; 10522 10523 /** 10524 * Payment details for the claim if the claim has been paid. 10525 */ 10526 @Child(name = "payment", type = {}, order=35, min=0, max=1, modifier=false, summary=false) 10527 @Description(shortDefinition="Payment (if paid)", formalDefinition="Payment details for the claim if the claim has been paid." ) 10528 protected PaymentComponent payment; 10529 10530 /** 10531 * The form to be used for printing the content. 10532 */ 10533 @Child(name = "form", type = {CodeableConcept.class}, order=36, min=0, max=1, modifier=false, summary=false) 10534 @Description(shortDefinition="Printed Form Identifier", formalDefinition="The form to be used for printing the content." ) 10535 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 10536 protected CodeableConcept form; 10537 10538 /** 10539 * Note text. 10540 */ 10541 @Child(name = "processNote", type = {}, order=37, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10542 @Description(shortDefinition="Processing notes", formalDefinition="Note text." ) 10543 protected List<NoteComponent> processNote; 10544 10545 /** 10546 * Balance by Benefit Category. 10547 */ 10548 @Child(name = "benefitBalance", type = {}, order=38, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10549 @Description(shortDefinition="Balance by Benefit Category", formalDefinition="Balance by Benefit Category." ) 10550 protected List<BenefitBalanceComponent> benefitBalance; 10551 10552 private static final long serialVersionUID = -1301056913L; 10553 10554 /** 10555 * Constructor 10556 */ 10557 public ExplanationOfBenefit() { 10558 super(); 10559 } 10560 10561 /** 10562 * @return {@link #identifier} (The EOB Business Identifier.) 10563 */ 10564 public List<Identifier> getIdentifier() { 10565 if (this.identifier == null) 10566 this.identifier = new ArrayList<Identifier>(); 10567 return this.identifier; 10568 } 10569 10570 /** 10571 * @return Returns a reference to <code>this</code> for easy method chaining 10572 */ 10573 public ExplanationOfBenefit setIdentifier(List<Identifier> theIdentifier) { 10574 this.identifier = theIdentifier; 10575 return this; 10576 } 10577 10578 public boolean hasIdentifier() { 10579 if (this.identifier == null) 10580 return false; 10581 for (Identifier item : this.identifier) 10582 if (!item.isEmpty()) 10583 return true; 10584 return false; 10585 } 10586 10587 public Identifier addIdentifier() { //3 10588 Identifier t = new Identifier(); 10589 if (this.identifier == null) 10590 this.identifier = new ArrayList<Identifier>(); 10591 this.identifier.add(t); 10592 return t; 10593 } 10594 10595 public ExplanationOfBenefit addIdentifier(Identifier t) { //3 10596 if (t == null) 10597 return this; 10598 if (this.identifier == null) 10599 this.identifier = new ArrayList<Identifier>(); 10600 this.identifier.add(t); 10601 return this; 10602 } 10603 10604 /** 10605 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 10606 */ 10607 public Identifier getIdentifierFirstRep() { 10608 if (getIdentifier().isEmpty()) { 10609 addIdentifier(); 10610 } 10611 return getIdentifier().get(0); 10612 } 10613 10614 /** 10615 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 10616 */ 10617 public Enumeration<ExplanationOfBenefitStatus> getStatusElement() { 10618 if (this.status == null) 10619 if (Configuration.errorOnAutoCreate()) 10620 throw new Error("Attempt to auto-create ExplanationOfBenefit.status"); 10621 else if (Configuration.doAutoCreate()) 10622 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); // bb 10623 return this.status; 10624 } 10625 10626 public boolean hasStatusElement() { 10627 return this.status != null && !this.status.isEmpty(); 10628 } 10629 10630 public boolean hasStatus() { 10631 return this.status != null && !this.status.isEmpty(); 10632 } 10633 10634 /** 10635 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 10636 */ 10637 public ExplanationOfBenefit setStatusElement(Enumeration<ExplanationOfBenefitStatus> value) { 10638 this.status = value; 10639 return this; 10640 } 10641 10642 /** 10643 * @return The status of the resource instance. 10644 */ 10645 public ExplanationOfBenefitStatus getStatus() { 10646 return this.status == null ? null : this.status.getValue(); 10647 } 10648 10649 /** 10650 * @param value The status of the resource instance. 10651 */ 10652 public ExplanationOfBenefit setStatus(ExplanationOfBenefitStatus value) { 10653 if (value == null) 10654 this.status = null; 10655 else { 10656 if (this.status == null) 10657 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); 10658 this.status.setValue(value); 10659 } 10660 return this; 10661 } 10662 10663 /** 10664 * @return {@link #type} (The category of claim, eg, oral, pharmacy, vision, insitutional, professional.) 10665 */ 10666 public CodeableConcept getType() { 10667 if (this.type == null) 10668 if (Configuration.errorOnAutoCreate()) 10669 throw new Error("Attempt to auto-create ExplanationOfBenefit.type"); 10670 else if (Configuration.doAutoCreate()) 10671 this.type = new CodeableConcept(); // cc 10672 return this.type; 10673 } 10674 10675 public boolean hasType() { 10676 return this.type != null && !this.type.isEmpty(); 10677 } 10678 10679 /** 10680 * @param value {@link #type} (The category of claim, eg, oral, pharmacy, vision, insitutional, professional.) 10681 */ 10682 public ExplanationOfBenefit setType(CodeableConcept value) { 10683 this.type = value; 10684 return this; 10685 } 10686 10687 /** 10688 * @return {@link #subType} (A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.) 10689 */ 10690 public List<CodeableConcept> getSubType() { 10691 if (this.subType == null) 10692 this.subType = new ArrayList<CodeableConcept>(); 10693 return this.subType; 10694 } 10695 10696 /** 10697 * @return Returns a reference to <code>this</code> for easy method chaining 10698 */ 10699 public ExplanationOfBenefit setSubType(List<CodeableConcept> theSubType) { 10700 this.subType = theSubType; 10701 return this; 10702 } 10703 10704 public boolean hasSubType() { 10705 if (this.subType == null) 10706 return false; 10707 for (CodeableConcept item : this.subType) 10708 if (!item.isEmpty()) 10709 return true; 10710 return false; 10711 } 10712 10713 public CodeableConcept addSubType() { //3 10714 CodeableConcept t = new CodeableConcept(); 10715 if (this.subType == null) 10716 this.subType = new ArrayList<CodeableConcept>(); 10717 this.subType.add(t); 10718 return t; 10719 } 10720 10721 public ExplanationOfBenefit addSubType(CodeableConcept t) { //3 10722 if (t == null) 10723 return this; 10724 if (this.subType == null) 10725 this.subType = new ArrayList<CodeableConcept>(); 10726 this.subType.add(t); 10727 return this; 10728 } 10729 10730 /** 10731 * @return The first repetition of repeating field {@link #subType}, creating it if it does not already exist 10732 */ 10733 public CodeableConcept getSubTypeFirstRep() { 10734 if (getSubType().isEmpty()) { 10735 addSubType(); 10736 } 10737 return getSubType().get(0); 10738 } 10739 10740 /** 10741 * @return {@link #patient} (Patient Resource.) 10742 */ 10743 public Reference getPatient() { 10744 if (this.patient == null) 10745 if (Configuration.errorOnAutoCreate()) 10746 throw new Error("Attempt to auto-create ExplanationOfBenefit.patient"); 10747 else if (Configuration.doAutoCreate()) 10748 this.patient = new Reference(); // cc 10749 return this.patient; 10750 } 10751 10752 public boolean hasPatient() { 10753 return this.patient != null && !this.patient.isEmpty(); 10754 } 10755 10756 /** 10757 * @param value {@link #patient} (Patient Resource.) 10758 */ 10759 public ExplanationOfBenefit setPatient(Reference value) { 10760 this.patient = value; 10761 return this; 10762 } 10763 10764 /** 10765 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient Resource.) 10766 */ 10767 public Patient getPatientTarget() { 10768 if (this.patientTarget == null) 10769 if (Configuration.errorOnAutoCreate()) 10770 throw new Error("Attempt to auto-create ExplanationOfBenefit.patient"); 10771 else if (Configuration.doAutoCreate()) 10772 this.patientTarget = new Patient(); // aa 10773 return this.patientTarget; 10774 } 10775 10776 /** 10777 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient Resource.) 10778 */ 10779 public ExplanationOfBenefit setPatientTarget(Patient value) { 10780 this.patientTarget = value; 10781 return this; 10782 } 10783 10784 /** 10785 * @return {@link #billablePeriod} (The billable period for which charges are being submitted.) 10786 */ 10787 public Period getBillablePeriod() { 10788 if (this.billablePeriod == null) 10789 if (Configuration.errorOnAutoCreate()) 10790 throw new Error("Attempt to auto-create ExplanationOfBenefit.billablePeriod"); 10791 else if (Configuration.doAutoCreate()) 10792 this.billablePeriod = new Period(); // cc 10793 return this.billablePeriod; 10794 } 10795 10796 public boolean hasBillablePeriod() { 10797 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 10798 } 10799 10800 /** 10801 * @param value {@link #billablePeriod} (The billable period for which charges are being submitted.) 10802 */ 10803 public ExplanationOfBenefit setBillablePeriod(Period value) { 10804 this.billablePeriod = value; 10805 return this; 10806 } 10807 10808 /** 10809 * @return {@link #created} (The date when the EOB was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 10810 */ 10811 public DateTimeType getCreatedElement() { 10812 if (this.created == null) 10813 if (Configuration.errorOnAutoCreate()) 10814 throw new Error("Attempt to auto-create ExplanationOfBenefit.created"); 10815 else if (Configuration.doAutoCreate()) 10816 this.created = new DateTimeType(); // bb 10817 return this.created; 10818 } 10819 10820 public boolean hasCreatedElement() { 10821 return this.created != null && !this.created.isEmpty(); 10822 } 10823 10824 public boolean hasCreated() { 10825 return this.created != null && !this.created.isEmpty(); 10826 } 10827 10828 /** 10829 * @param value {@link #created} (The date when the EOB was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 10830 */ 10831 public ExplanationOfBenefit setCreatedElement(DateTimeType value) { 10832 this.created = value; 10833 return this; 10834 } 10835 10836 /** 10837 * @return The date when the EOB was created. 10838 */ 10839 public Date getCreated() { 10840 return this.created == null ? null : this.created.getValue(); 10841 } 10842 10843 /** 10844 * @param value The date when the EOB was created. 10845 */ 10846 public ExplanationOfBenefit setCreated(Date value) { 10847 if (value == null) 10848 this.created = null; 10849 else { 10850 if (this.created == null) 10851 this.created = new DateTimeType(); 10852 this.created.setValue(value); 10853 } 10854 return this; 10855 } 10856 10857 /** 10858 * @return {@link #enterer} (The person who created the explanation of benefit.) 10859 */ 10860 public Reference getEnterer() { 10861 if (this.enterer == null) 10862 if (Configuration.errorOnAutoCreate()) 10863 throw new Error("Attempt to auto-create ExplanationOfBenefit.enterer"); 10864 else if (Configuration.doAutoCreate()) 10865 this.enterer = new Reference(); // cc 10866 return this.enterer; 10867 } 10868 10869 public boolean hasEnterer() { 10870 return this.enterer != null && !this.enterer.isEmpty(); 10871 } 10872 10873 /** 10874 * @param value {@link #enterer} (The person who created the explanation of benefit.) 10875 */ 10876 public ExplanationOfBenefit setEnterer(Reference value) { 10877 this.enterer = value; 10878 return this; 10879 } 10880 10881 /** 10882 * @return {@link #enterer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person who created the explanation of benefit.) 10883 */ 10884 public Practitioner getEntererTarget() { 10885 if (this.entererTarget == null) 10886 if (Configuration.errorOnAutoCreate()) 10887 throw new Error("Attempt to auto-create ExplanationOfBenefit.enterer"); 10888 else if (Configuration.doAutoCreate()) 10889 this.entererTarget = new Practitioner(); // aa 10890 return this.entererTarget; 10891 } 10892 10893 /** 10894 * @param value {@link #enterer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person who created the explanation of benefit.) 10895 */ 10896 public ExplanationOfBenefit setEntererTarget(Practitioner value) { 10897 this.entererTarget = value; 10898 return this; 10899 } 10900 10901 /** 10902 * @return {@link #insurer} (The insurer which is responsible for the explanation of benefit.) 10903 */ 10904 public Reference getInsurer() { 10905 if (this.insurer == null) 10906 if (Configuration.errorOnAutoCreate()) 10907 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurer"); 10908 else if (Configuration.doAutoCreate()) 10909 this.insurer = new Reference(); // cc 10910 return this.insurer; 10911 } 10912 10913 public boolean hasInsurer() { 10914 return this.insurer != null && !this.insurer.isEmpty(); 10915 } 10916 10917 /** 10918 * @param value {@link #insurer} (The insurer which is responsible for the explanation of benefit.) 10919 */ 10920 public ExplanationOfBenefit setInsurer(Reference value) { 10921 this.insurer = value; 10922 return this; 10923 } 10924 10925 /** 10926 * @return {@link #insurer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The insurer which is responsible for the explanation of benefit.) 10927 */ 10928 public Organization getInsurerTarget() { 10929 if (this.insurerTarget == null) 10930 if (Configuration.errorOnAutoCreate()) 10931 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurer"); 10932 else if (Configuration.doAutoCreate()) 10933 this.insurerTarget = new Organization(); // aa 10934 return this.insurerTarget; 10935 } 10936 10937 /** 10938 * @param value {@link #insurer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The insurer which is responsible for the explanation of benefit.) 10939 */ 10940 public ExplanationOfBenefit setInsurerTarget(Organization value) { 10941 this.insurerTarget = value; 10942 return this; 10943 } 10944 10945 /** 10946 * @return {@link #provider} (The provider which is responsible for the claim.) 10947 */ 10948 public Reference getProvider() { 10949 if (this.provider == null) 10950 if (Configuration.errorOnAutoCreate()) 10951 throw new Error("Attempt to auto-create ExplanationOfBenefit.provider"); 10952 else if (Configuration.doAutoCreate()) 10953 this.provider = new Reference(); // cc 10954 return this.provider; 10955 } 10956 10957 public boolean hasProvider() { 10958 return this.provider != null && !this.provider.isEmpty(); 10959 } 10960 10961 /** 10962 * @param value {@link #provider} (The provider which is responsible for the claim.) 10963 */ 10964 public ExplanationOfBenefit setProvider(Reference value) { 10965 this.provider = value; 10966 return this; 10967 } 10968 10969 /** 10970 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the claim.) 10971 */ 10972 public Practitioner getProviderTarget() { 10973 if (this.providerTarget == null) 10974 if (Configuration.errorOnAutoCreate()) 10975 throw new Error("Attempt to auto-create ExplanationOfBenefit.provider"); 10976 else if (Configuration.doAutoCreate()) 10977 this.providerTarget = new Practitioner(); // aa 10978 return this.providerTarget; 10979 } 10980 10981 /** 10982 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the claim.) 10983 */ 10984 public ExplanationOfBenefit setProviderTarget(Practitioner value) { 10985 this.providerTarget = value; 10986 return this; 10987 } 10988 10989 /** 10990 * @return {@link #organization} (The provider which is responsible for the claim.) 10991 */ 10992 public Reference getOrganization() { 10993 if (this.organization == null) 10994 if (Configuration.errorOnAutoCreate()) 10995 throw new Error("Attempt to auto-create ExplanationOfBenefit.organization"); 10996 else if (Configuration.doAutoCreate()) 10997 this.organization = new Reference(); // cc 10998 return this.organization; 10999 } 11000 11001 public boolean hasOrganization() { 11002 return this.organization != null && !this.organization.isEmpty(); 11003 } 11004 11005 /** 11006 * @param value {@link #organization} (The provider which is responsible for the claim.) 11007 */ 11008 public ExplanationOfBenefit setOrganization(Reference value) { 11009 this.organization = value; 11010 return this; 11011 } 11012 11013 /** 11014 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the claim.) 11015 */ 11016 public Organization getOrganizationTarget() { 11017 if (this.organizationTarget == null) 11018 if (Configuration.errorOnAutoCreate()) 11019 throw new Error("Attempt to auto-create ExplanationOfBenefit.organization"); 11020 else if (Configuration.doAutoCreate()) 11021 this.organizationTarget = new Organization(); // aa 11022 return this.organizationTarget; 11023 } 11024 11025 /** 11026 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the claim.) 11027 */ 11028 public ExplanationOfBenefit setOrganizationTarget(Organization value) { 11029 this.organizationTarget = value; 11030 return this; 11031 } 11032 11033 /** 11034 * @return {@link #referral} (The referral resource which lists the date, practitioner, reason and other supporting information.) 11035 */ 11036 public Reference getReferral() { 11037 if (this.referral == null) 11038 if (Configuration.errorOnAutoCreate()) 11039 throw new Error("Attempt to auto-create ExplanationOfBenefit.referral"); 11040 else if (Configuration.doAutoCreate()) 11041 this.referral = new Reference(); // cc 11042 return this.referral; 11043 } 11044 11045 public boolean hasReferral() { 11046 return this.referral != null && !this.referral.isEmpty(); 11047 } 11048 11049 /** 11050 * @param value {@link #referral} (The referral resource which lists the date, practitioner, reason and other supporting information.) 11051 */ 11052 public ExplanationOfBenefit setReferral(Reference value) { 11053 this.referral = value; 11054 return this; 11055 } 11056 11057 /** 11058 * @return {@link #referral} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The referral resource which lists the date, practitioner, reason and other supporting information.) 11059 */ 11060 public ReferralRequest getReferralTarget() { 11061 if (this.referralTarget == null) 11062 if (Configuration.errorOnAutoCreate()) 11063 throw new Error("Attempt to auto-create ExplanationOfBenefit.referral"); 11064 else if (Configuration.doAutoCreate()) 11065 this.referralTarget = new ReferralRequest(); // aa 11066 return this.referralTarget; 11067 } 11068 11069 /** 11070 * @param value {@link #referral} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The referral resource which lists the date, practitioner, reason and other supporting information.) 11071 */ 11072 public ExplanationOfBenefit setReferralTarget(ReferralRequest value) { 11073 this.referralTarget = value; 11074 return this; 11075 } 11076 11077 /** 11078 * @return {@link #facility} (Facility where the services were provided.) 11079 */ 11080 public Reference getFacility() { 11081 if (this.facility == null) 11082 if (Configuration.errorOnAutoCreate()) 11083 throw new Error("Attempt to auto-create ExplanationOfBenefit.facility"); 11084 else if (Configuration.doAutoCreate()) 11085 this.facility = new Reference(); // cc 11086 return this.facility; 11087 } 11088 11089 public boolean hasFacility() { 11090 return this.facility != null && !this.facility.isEmpty(); 11091 } 11092 11093 /** 11094 * @param value {@link #facility} (Facility where the services were provided.) 11095 */ 11096 public ExplanationOfBenefit setFacility(Reference value) { 11097 this.facility = value; 11098 return this; 11099 } 11100 11101 /** 11102 * @return {@link #facility} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 11103 */ 11104 public Location getFacilityTarget() { 11105 if (this.facilityTarget == null) 11106 if (Configuration.errorOnAutoCreate()) 11107 throw new Error("Attempt to auto-create ExplanationOfBenefit.facility"); 11108 else if (Configuration.doAutoCreate()) 11109 this.facilityTarget = new Location(); // aa 11110 return this.facilityTarget; 11111 } 11112 11113 /** 11114 * @param value {@link #facility} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 11115 */ 11116 public ExplanationOfBenefit setFacilityTarget(Location value) { 11117 this.facilityTarget = value; 11118 return this; 11119 } 11120 11121 /** 11122 * @return {@link #claim} (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11123 */ 11124 public Reference getClaim() { 11125 if (this.claim == null) 11126 if (Configuration.errorOnAutoCreate()) 11127 throw new Error("Attempt to auto-create ExplanationOfBenefit.claim"); 11128 else if (Configuration.doAutoCreate()) 11129 this.claim = new Reference(); // cc 11130 return this.claim; 11131 } 11132 11133 public boolean hasClaim() { 11134 return this.claim != null && !this.claim.isEmpty(); 11135 } 11136 11137 /** 11138 * @param value {@link #claim} (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11139 */ 11140 public ExplanationOfBenefit setClaim(Reference value) { 11141 this.claim = value; 11142 return this; 11143 } 11144 11145 /** 11146 * @return {@link #claim} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11147 */ 11148 public Claim getClaimTarget() { 11149 if (this.claimTarget == null) 11150 if (Configuration.errorOnAutoCreate()) 11151 throw new Error("Attempt to auto-create ExplanationOfBenefit.claim"); 11152 else if (Configuration.doAutoCreate()) 11153 this.claimTarget = new Claim(); // aa 11154 return this.claimTarget; 11155 } 11156 11157 /** 11158 * @param value {@link #claim} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11159 */ 11160 public ExplanationOfBenefit setClaimTarget(Claim value) { 11161 this.claimTarget = value; 11162 return this; 11163 } 11164 11165 /** 11166 * @return {@link #claimResponse} (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11167 */ 11168 public Reference getClaimResponse() { 11169 if (this.claimResponse == null) 11170 if (Configuration.errorOnAutoCreate()) 11171 throw new Error("Attempt to auto-create ExplanationOfBenefit.claimResponse"); 11172 else if (Configuration.doAutoCreate()) 11173 this.claimResponse = new Reference(); // cc 11174 return this.claimResponse; 11175 } 11176 11177 public boolean hasClaimResponse() { 11178 return this.claimResponse != null && !this.claimResponse.isEmpty(); 11179 } 11180 11181 /** 11182 * @param value {@link #claimResponse} (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11183 */ 11184 public ExplanationOfBenefit setClaimResponse(Reference value) { 11185 this.claimResponse = value; 11186 return this; 11187 } 11188 11189 /** 11190 * @return {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11191 */ 11192 public ClaimResponse getClaimResponseTarget() { 11193 if (this.claimResponseTarget == null) 11194 if (Configuration.errorOnAutoCreate()) 11195 throw new Error("Attempt to auto-create ExplanationOfBenefit.claimResponse"); 11196 else if (Configuration.doAutoCreate()) 11197 this.claimResponseTarget = new ClaimResponse(); // aa 11198 return this.claimResponseTarget; 11199 } 11200 11201 /** 11202 * @param value {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11203 */ 11204 public ExplanationOfBenefit setClaimResponseTarget(ClaimResponse value) { 11205 this.claimResponseTarget = value; 11206 return this; 11207 } 11208 11209 /** 11210 * @return {@link #outcome} (Processing outcome errror, partial or complete processing.) 11211 */ 11212 public CodeableConcept getOutcome() { 11213 if (this.outcome == null) 11214 if (Configuration.errorOnAutoCreate()) 11215 throw new Error("Attempt to auto-create ExplanationOfBenefit.outcome"); 11216 else if (Configuration.doAutoCreate()) 11217 this.outcome = new CodeableConcept(); // cc 11218 return this.outcome; 11219 } 11220 11221 public boolean hasOutcome() { 11222 return this.outcome != null && !this.outcome.isEmpty(); 11223 } 11224 11225 /** 11226 * @param value {@link #outcome} (Processing outcome errror, partial or complete processing.) 11227 */ 11228 public ExplanationOfBenefit setOutcome(CodeableConcept value) { 11229 this.outcome = value; 11230 return this; 11231 } 11232 11233 /** 11234 * @return {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 11235 */ 11236 public StringType getDispositionElement() { 11237 if (this.disposition == null) 11238 if (Configuration.errorOnAutoCreate()) 11239 throw new Error("Attempt to auto-create ExplanationOfBenefit.disposition"); 11240 else if (Configuration.doAutoCreate()) 11241 this.disposition = new StringType(); // bb 11242 return this.disposition; 11243 } 11244 11245 public boolean hasDispositionElement() { 11246 return this.disposition != null && !this.disposition.isEmpty(); 11247 } 11248 11249 public boolean hasDisposition() { 11250 return this.disposition != null && !this.disposition.isEmpty(); 11251 } 11252 11253 /** 11254 * @param value {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 11255 */ 11256 public ExplanationOfBenefit setDispositionElement(StringType value) { 11257 this.disposition = value; 11258 return this; 11259 } 11260 11261 /** 11262 * @return A description of the status of the adjudication. 11263 */ 11264 public String getDisposition() { 11265 return this.disposition == null ? null : this.disposition.getValue(); 11266 } 11267 11268 /** 11269 * @param value A description of the status of the adjudication. 11270 */ 11271 public ExplanationOfBenefit setDisposition(String value) { 11272 if (Utilities.noString(value)) 11273 this.disposition = null; 11274 else { 11275 if (this.disposition == null) 11276 this.disposition = new StringType(); 11277 this.disposition.setValue(value); 11278 } 11279 return this; 11280 } 11281 11282 /** 11283 * @return {@link #related} (Other claims which are related to this claim such as prior claim versions or for related services.) 11284 */ 11285 public List<RelatedClaimComponent> getRelated() { 11286 if (this.related == null) 11287 this.related = new ArrayList<RelatedClaimComponent>(); 11288 return this.related; 11289 } 11290 11291 /** 11292 * @return Returns a reference to <code>this</code> for easy method chaining 11293 */ 11294 public ExplanationOfBenefit setRelated(List<RelatedClaimComponent> theRelated) { 11295 this.related = theRelated; 11296 return this; 11297 } 11298 11299 public boolean hasRelated() { 11300 if (this.related == null) 11301 return false; 11302 for (RelatedClaimComponent item : this.related) 11303 if (!item.isEmpty()) 11304 return true; 11305 return false; 11306 } 11307 11308 public RelatedClaimComponent addRelated() { //3 11309 RelatedClaimComponent t = new RelatedClaimComponent(); 11310 if (this.related == null) 11311 this.related = new ArrayList<RelatedClaimComponent>(); 11312 this.related.add(t); 11313 return t; 11314 } 11315 11316 public ExplanationOfBenefit addRelated(RelatedClaimComponent t) { //3 11317 if (t == null) 11318 return this; 11319 if (this.related == null) 11320 this.related = new ArrayList<RelatedClaimComponent>(); 11321 this.related.add(t); 11322 return this; 11323 } 11324 11325 /** 11326 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist 11327 */ 11328 public RelatedClaimComponent getRelatedFirstRep() { 11329 if (getRelated().isEmpty()) { 11330 addRelated(); 11331 } 11332 return getRelated().get(0); 11333 } 11334 11335 /** 11336 * @return {@link #prescription} (Prescription to support the dispensing of Pharmacy or Vision products.) 11337 */ 11338 public Reference getPrescription() { 11339 if (this.prescription == null) 11340 if (Configuration.errorOnAutoCreate()) 11341 throw new Error("Attempt to auto-create ExplanationOfBenefit.prescription"); 11342 else if (Configuration.doAutoCreate()) 11343 this.prescription = new Reference(); // cc 11344 return this.prescription; 11345 } 11346 11347 public boolean hasPrescription() { 11348 return this.prescription != null && !this.prescription.isEmpty(); 11349 } 11350 11351 /** 11352 * @param value {@link #prescription} (Prescription to support the dispensing of Pharmacy or Vision products.) 11353 */ 11354 public ExplanationOfBenefit setPrescription(Reference value) { 11355 this.prescription = value; 11356 return this; 11357 } 11358 11359 /** 11360 * @return {@link #prescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Prescription to support the dispensing of Pharmacy or Vision products.) 11361 */ 11362 public Resource getPrescriptionTarget() { 11363 return this.prescriptionTarget; 11364 } 11365 11366 /** 11367 * @param value {@link #prescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Prescription to support the dispensing of Pharmacy or Vision products.) 11368 */ 11369 public ExplanationOfBenefit setPrescriptionTarget(Resource value) { 11370 this.prescriptionTarget = value; 11371 return this; 11372 } 11373 11374 /** 11375 * @return {@link #originalPrescription} (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 11376 */ 11377 public Reference getOriginalPrescription() { 11378 if (this.originalPrescription == null) 11379 if (Configuration.errorOnAutoCreate()) 11380 throw new Error("Attempt to auto-create ExplanationOfBenefit.originalPrescription"); 11381 else if (Configuration.doAutoCreate()) 11382 this.originalPrescription = new Reference(); // cc 11383 return this.originalPrescription; 11384 } 11385 11386 public boolean hasOriginalPrescription() { 11387 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 11388 } 11389 11390 /** 11391 * @param value {@link #originalPrescription} (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 11392 */ 11393 public ExplanationOfBenefit setOriginalPrescription(Reference value) { 11394 this.originalPrescription = value; 11395 return this; 11396 } 11397 11398 /** 11399 * @return {@link #originalPrescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 11400 */ 11401 public MedicationRequest getOriginalPrescriptionTarget() { 11402 if (this.originalPrescriptionTarget == null) 11403 if (Configuration.errorOnAutoCreate()) 11404 throw new Error("Attempt to auto-create ExplanationOfBenefit.originalPrescription"); 11405 else if (Configuration.doAutoCreate()) 11406 this.originalPrescriptionTarget = new MedicationRequest(); // aa 11407 return this.originalPrescriptionTarget; 11408 } 11409 11410 /** 11411 * @param value {@link #originalPrescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 11412 */ 11413 public ExplanationOfBenefit setOriginalPrescriptionTarget(MedicationRequest value) { 11414 this.originalPrescriptionTarget = value; 11415 return this; 11416 } 11417 11418 /** 11419 * @return {@link #payee} (The party to be reimbursed for the services.) 11420 */ 11421 public PayeeComponent getPayee() { 11422 if (this.payee == null) 11423 if (Configuration.errorOnAutoCreate()) 11424 throw new Error("Attempt to auto-create ExplanationOfBenefit.payee"); 11425 else if (Configuration.doAutoCreate()) 11426 this.payee = new PayeeComponent(); // cc 11427 return this.payee; 11428 } 11429 11430 public boolean hasPayee() { 11431 return this.payee != null && !this.payee.isEmpty(); 11432 } 11433 11434 /** 11435 * @param value {@link #payee} (The party to be reimbursed for the services.) 11436 */ 11437 public ExplanationOfBenefit setPayee(PayeeComponent value) { 11438 this.payee = value; 11439 return this; 11440 } 11441 11442 /** 11443 * @return {@link #information} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.) 11444 */ 11445 public List<SupportingInformationComponent> getInformation() { 11446 if (this.information == null) 11447 this.information = new ArrayList<SupportingInformationComponent>(); 11448 return this.information; 11449 } 11450 11451 /** 11452 * @return Returns a reference to <code>this</code> for easy method chaining 11453 */ 11454 public ExplanationOfBenefit setInformation(List<SupportingInformationComponent> theInformation) { 11455 this.information = theInformation; 11456 return this; 11457 } 11458 11459 public boolean hasInformation() { 11460 if (this.information == null) 11461 return false; 11462 for (SupportingInformationComponent item : this.information) 11463 if (!item.isEmpty()) 11464 return true; 11465 return false; 11466 } 11467 11468 public SupportingInformationComponent addInformation() { //3 11469 SupportingInformationComponent t = new SupportingInformationComponent(); 11470 if (this.information == null) 11471 this.information = new ArrayList<SupportingInformationComponent>(); 11472 this.information.add(t); 11473 return t; 11474 } 11475 11476 public ExplanationOfBenefit addInformation(SupportingInformationComponent t) { //3 11477 if (t == null) 11478 return this; 11479 if (this.information == null) 11480 this.information = new ArrayList<SupportingInformationComponent>(); 11481 this.information.add(t); 11482 return this; 11483 } 11484 11485 /** 11486 * @return The first repetition of repeating field {@link #information}, creating it if it does not already exist 11487 */ 11488 public SupportingInformationComponent getInformationFirstRep() { 11489 if (getInformation().isEmpty()) { 11490 addInformation(); 11491 } 11492 return getInformation().get(0); 11493 } 11494 11495 /** 11496 * @return {@link #careTeam} (The members of the team who provided the overall service as well as their role and whether responsible and qualifications.) 11497 */ 11498 public List<CareTeamComponent> getCareTeam() { 11499 if (this.careTeam == null) 11500 this.careTeam = new ArrayList<CareTeamComponent>(); 11501 return this.careTeam; 11502 } 11503 11504 /** 11505 * @return Returns a reference to <code>this</code> for easy method chaining 11506 */ 11507 public ExplanationOfBenefit setCareTeam(List<CareTeamComponent> theCareTeam) { 11508 this.careTeam = theCareTeam; 11509 return this; 11510 } 11511 11512 public boolean hasCareTeam() { 11513 if (this.careTeam == null) 11514 return false; 11515 for (CareTeamComponent item : this.careTeam) 11516 if (!item.isEmpty()) 11517 return true; 11518 return false; 11519 } 11520 11521 public CareTeamComponent addCareTeam() { //3 11522 CareTeamComponent t = new CareTeamComponent(); 11523 if (this.careTeam == null) 11524 this.careTeam = new ArrayList<CareTeamComponent>(); 11525 this.careTeam.add(t); 11526 return t; 11527 } 11528 11529 public ExplanationOfBenefit addCareTeam(CareTeamComponent t) { //3 11530 if (t == null) 11531 return this; 11532 if (this.careTeam == null) 11533 this.careTeam = new ArrayList<CareTeamComponent>(); 11534 this.careTeam.add(t); 11535 return this; 11536 } 11537 11538 /** 11539 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist 11540 */ 11541 public CareTeamComponent getCareTeamFirstRep() { 11542 if (getCareTeam().isEmpty()) { 11543 addCareTeam(); 11544 } 11545 return getCareTeam().get(0); 11546 } 11547 11548 /** 11549 * @return {@link #diagnosis} (Ordered list of patient diagnosis for which care is sought.) 11550 */ 11551 public List<DiagnosisComponent> getDiagnosis() { 11552 if (this.diagnosis == null) 11553 this.diagnosis = new ArrayList<DiagnosisComponent>(); 11554 return this.diagnosis; 11555 } 11556 11557 /** 11558 * @return Returns a reference to <code>this</code> for easy method chaining 11559 */ 11560 public ExplanationOfBenefit setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 11561 this.diagnosis = theDiagnosis; 11562 return this; 11563 } 11564 11565 public boolean hasDiagnosis() { 11566 if (this.diagnosis == null) 11567 return false; 11568 for (DiagnosisComponent item : this.diagnosis) 11569 if (!item.isEmpty()) 11570 return true; 11571 return false; 11572 } 11573 11574 public DiagnosisComponent addDiagnosis() { //3 11575 DiagnosisComponent t = new DiagnosisComponent(); 11576 if (this.diagnosis == null) 11577 this.diagnosis = new ArrayList<DiagnosisComponent>(); 11578 this.diagnosis.add(t); 11579 return t; 11580 } 11581 11582 public ExplanationOfBenefit addDiagnosis(DiagnosisComponent t) { //3 11583 if (t == null) 11584 return this; 11585 if (this.diagnosis == null) 11586 this.diagnosis = new ArrayList<DiagnosisComponent>(); 11587 this.diagnosis.add(t); 11588 return this; 11589 } 11590 11591 /** 11592 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist 11593 */ 11594 public DiagnosisComponent getDiagnosisFirstRep() { 11595 if (getDiagnosis().isEmpty()) { 11596 addDiagnosis(); 11597 } 11598 return getDiagnosis().get(0); 11599 } 11600 11601 /** 11602 * @return {@link #procedure} (Ordered list of patient procedures performed to support the adjudication.) 11603 */ 11604 public List<ProcedureComponent> getProcedure() { 11605 if (this.procedure == null) 11606 this.procedure = new ArrayList<ProcedureComponent>(); 11607 return this.procedure; 11608 } 11609 11610 /** 11611 * @return Returns a reference to <code>this</code> for easy method chaining 11612 */ 11613 public ExplanationOfBenefit setProcedure(List<ProcedureComponent> theProcedure) { 11614 this.procedure = theProcedure; 11615 return this; 11616 } 11617 11618 public boolean hasProcedure() { 11619 if (this.procedure == null) 11620 return false; 11621 for (ProcedureComponent item : this.procedure) 11622 if (!item.isEmpty()) 11623 return true; 11624 return false; 11625 } 11626 11627 public ProcedureComponent addProcedure() { //3 11628 ProcedureComponent t = new ProcedureComponent(); 11629 if (this.procedure == null) 11630 this.procedure = new ArrayList<ProcedureComponent>(); 11631 this.procedure.add(t); 11632 return t; 11633 } 11634 11635 public ExplanationOfBenefit addProcedure(ProcedureComponent t) { //3 11636 if (t == null) 11637 return this; 11638 if (this.procedure == null) 11639 this.procedure = new ArrayList<ProcedureComponent>(); 11640 this.procedure.add(t); 11641 return this; 11642 } 11643 11644 /** 11645 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist 11646 */ 11647 public ProcedureComponent getProcedureFirstRep() { 11648 if (getProcedure().isEmpty()) { 11649 addProcedure(); 11650 } 11651 return getProcedure().get(0); 11652 } 11653 11654 /** 11655 * @return {@link #precedence} (Precedence (primary, secondary, etc.).). This is the underlying object with id, value and extensions. The accessor "getPrecedence" gives direct access to the value 11656 */ 11657 public PositiveIntType getPrecedenceElement() { 11658 if (this.precedence == null) 11659 if (Configuration.errorOnAutoCreate()) 11660 throw new Error("Attempt to auto-create ExplanationOfBenefit.precedence"); 11661 else if (Configuration.doAutoCreate()) 11662 this.precedence = new PositiveIntType(); // bb 11663 return this.precedence; 11664 } 11665 11666 public boolean hasPrecedenceElement() { 11667 return this.precedence != null && !this.precedence.isEmpty(); 11668 } 11669 11670 public boolean hasPrecedence() { 11671 return this.precedence != null && !this.precedence.isEmpty(); 11672 } 11673 11674 /** 11675 * @param value {@link #precedence} (Precedence (primary, secondary, etc.).). This is the underlying object with id, value and extensions. The accessor "getPrecedence" gives direct access to the value 11676 */ 11677 public ExplanationOfBenefit setPrecedenceElement(PositiveIntType value) { 11678 this.precedence = value; 11679 return this; 11680 } 11681 11682 /** 11683 * @return Precedence (primary, secondary, etc.). 11684 */ 11685 public int getPrecedence() { 11686 return this.precedence == null || this.precedence.isEmpty() ? 0 : this.precedence.getValue(); 11687 } 11688 11689 /** 11690 * @param value Precedence (primary, secondary, etc.). 11691 */ 11692 public ExplanationOfBenefit setPrecedence(int value) { 11693 if (this.precedence == null) 11694 this.precedence = new PositiveIntType(); 11695 this.precedence.setValue(value); 11696 return this; 11697 } 11698 11699 /** 11700 * @return {@link #insurance} (Financial instrument by which payment information for health care.) 11701 */ 11702 public InsuranceComponent getInsurance() { 11703 if (this.insurance == null) 11704 if (Configuration.errorOnAutoCreate()) 11705 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurance"); 11706 else if (Configuration.doAutoCreate()) 11707 this.insurance = new InsuranceComponent(); // cc 11708 return this.insurance; 11709 } 11710 11711 public boolean hasInsurance() { 11712 return this.insurance != null && !this.insurance.isEmpty(); 11713 } 11714 11715 /** 11716 * @param value {@link #insurance} (Financial instrument by which payment information for health care.) 11717 */ 11718 public ExplanationOfBenefit setInsurance(InsuranceComponent value) { 11719 this.insurance = value; 11720 return this; 11721 } 11722 11723 /** 11724 * @return {@link #accident} (An accident which resulted in the need for healthcare services.) 11725 */ 11726 public AccidentComponent getAccident() { 11727 if (this.accident == null) 11728 if (Configuration.errorOnAutoCreate()) 11729 throw new Error("Attempt to auto-create ExplanationOfBenefit.accident"); 11730 else if (Configuration.doAutoCreate()) 11731 this.accident = new AccidentComponent(); // cc 11732 return this.accident; 11733 } 11734 11735 public boolean hasAccident() { 11736 return this.accident != null && !this.accident.isEmpty(); 11737 } 11738 11739 /** 11740 * @param value {@link #accident} (An accident which resulted in the need for healthcare services.) 11741 */ 11742 public ExplanationOfBenefit setAccident(AccidentComponent value) { 11743 this.accident = value; 11744 return this; 11745 } 11746 11747 /** 11748 * @return {@link #employmentImpacted} (The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).) 11749 */ 11750 public Period getEmploymentImpacted() { 11751 if (this.employmentImpacted == null) 11752 if (Configuration.errorOnAutoCreate()) 11753 throw new Error("Attempt to auto-create ExplanationOfBenefit.employmentImpacted"); 11754 else if (Configuration.doAutoCreate()) 11755 this.employmentImpacted = new Period(); // cc 11756 return this.employmentImpacted; 11757 } 11758 11759 public boolean hasEmploymentImpacted() { 11760 return this.employmentImpacted != null && !this.employmentImpacted.isEmpty(); 11761 } 11762 11763 /** 11764 * @param value {@link #employmentImpacted} (The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).) 11765 */ 11766 public ExplanationOfBenefit setEmploymentImpacted(Period value) { 11767 this.employmentImpacted = value; 11768 return this; 11769 } 11770 11771 /** 11772 * @return {@link #hospitalization} (The start and optional end dates of when the patient was confined to a treatment center.) 11773 */ 11774 public Period getHospitalization() { 11775 if (this.hospitalization == null) 11776 if (Configuration.errorOnAutoCreate()) 11777 throw new Error("Attempt to auto-create ExplanationOfBenefit.hospitalization"); 11778 else if (Configuration.doAutoCreate()) 11779 this.hospitalization = new Period(); // cc 11780 return this.hospitalization; 11781 } 11782 11783 public boolean hasHospitalization() { 11784 return this.hospitalization != null && !this.hospitalization.isEmpty(); 11785 } 11786 11787 /** 11788 * @param value {@link #hospitalization} (The start and optional end dates of when the patient was confined to a treatment center.) 11789 */ 11790 public ExplanationOfBenefit setHospitalization(Period value) { 11791 this.hospitalization = value; 11792 return this; 11793 } 11794 11795 /** 11796 * @return {@link #item} (First tier of goods and services.) 11797 */ 11798 public List<ItemComponent> getItem() { 11799 if (this.item == null) 11800 this.item = new ArrayList<ItemComponent>(); 11801 return this.item; 11802 } 11803 11804 /** 11805 * @return Returns a reference to <code>this</code> for easy method chaining 11806 */ 11807 public ExplanationOfBenefit setItem(List<ItemComponent> theItem) { 11808 this.item = theItem; 11809 return this; 11810 } 11811 11812 public boolean hasItem() { 11813 if (this.item == null) 11814 return false; 11815 for (ItemComponent item : this.item) 11816 if (!item.isEmpty()) 11817 return true; 11818 return false; 11819 } 11820 11821 public ItemComponent addItem() { //3 11822 ItemComponent t = new ItemComponent(); 11823 if (this.item == null) 11824 this.item = new ArrayList<ItemComponent>(); 11825 this.item.add(t); 11826 return t; 11827 } 11828 11829 public ExplanationOfBenefit addItem(ItemComponent t) { //3 11830 if (t == null) 11831 return this; 11832 if (this.item == null) 11833 this.item = new ArrayList<ItemComponent>(); 11834 this.item.add(t); 11835 return this; 11836 } 11837 11838 /** 11839 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 11840 */ 11841 public ItemComponent getItemFirstRep() { 11842 if (getItem().isEmpty()) { 11843 addItem(); 11844 } 11845 return getItem().get(0); 11846 } 11847 11848 /** 11849 * @return {@link #addItem} (The first tier service adjudications for payor added services.) 11850 */ 11851 public List<AddedItemComponent> getAddItem() { 11852 if (this.addItem == null) 11853 this.addItem = new ArrayList<AddedItemComponent>(); 11854 return this.addItem; 11855 } 11856 11857 /** 11858 * @return Returns a reference to <code>this</code> for easy method chaining 11859 */ 11860 public ExplanationOfBenefit setAddItem(List<AddedItemComponent> theAddItem) { 11861 this.addItem = theAddItem; 11862 return this; 11863 } 11864 11865 public boolean hasAddItem() { 11866 if (this.addItem == null) 11867 return false; 11868 for (AddedItemComponent item : this.addItem) 11869 if (!item.isEmpty()) 11870 return true; 11871 return false; 11872 } 11873 11874 public AddedItemComponent addAddItem() { //3 11875 AddedItemComponent t = new AddedItemComponent(); 11876 if (this.addItem == null) 11877 this.addItem = new ArrayList<AddedItemComponent>(); 11878 this.addItem.add(t); 11879 return t; 11880 } 11881 11882 public ExplanationOfBenefit addAddItem(AddedItemComponent t) { //3 11883 if (t == null) 11884 return this; 11885 if (this.addItem == null) 11886 this.addItem = new ArrayList<AddedItemComponent>(); 11887 this.addItem.add(t); 11888 return this; 11889 } 11890 11891 /** 11892 * @return The first repetition of repeating field {@link #addItem}, creating it if it does not already exist 11893 */ 11894 public AddedItemComponent getAddItemFirstRep() { 11895 if (getAddItem().isEmpty()) { 11896 addAddItem(); 11897 } 11898 return getAddItem().get(0); 11899 } 11900 11901 /** 11902 * @return {@link #totalCost} (The total cost of the services reported.) 11903 */ 11904 public Money getTotalCost() { 11905 if (this.totalCost == null) 11906 if (Configuration.errorOnAutoCreate()) 11907 throw new Error("Attempt to auto-create ExplanationOfBenefit.totalCost"); 11908 else if (Configuration.doAutoCreate()) 11909 this.totalCost = new Money(); // cc 11910 return this.totalCost; 11911 } 11912 11913 public boolean hasTotalCost() { 11914 return this.totalCost != null && !this.totalCost.isEmpty(); 11915 } 11916 11917 /** 11918 * @param value {@link #totalCost} (The total cost of the services reported.) 11919 */ 11920 public ExplanationOfBenefit setTotalCost(Money value) { 11921 this.totalCost = value; 11922 return this; 11923 } 11924 11925 /** 11926 * @return {@link #unallocDeductable} (The amount of deductable applied which was not allocated to any particular service line.) 11927 */ 11928 public Money getUnallocDeductable() { 11929 if (this.unallocDeductable == null) 11930 if (Configuration.errorOnAutoCreate()) 11931 throw new Error("Attempt to auto-create ExplanationOfBenefit.unallocDeductable"); 11932 else if (Configuration.doAutoCreate()) 11933 this.unallocDeductable = new Money(); // cc 11934 return this.unallocDeductable; 11935 } 11936 11937 public boolean hasUnallocDeductable() { 11938 return this.unallocDeductable != null && !this.unallocDeductable.isEmpty(); 11939 } 11940 11941 /** 11942 * @param value {@link #unallocDeductable} (The amount of deductable applied which was not allocated to any particular service line.) 11943 */ 11944 public ExplanationOfBenefit setUnallocDeductable(Money value) { 11945 this.unallocDeductable = value; 11946 return this; 11947 } 11948 11949 /** 11950 * @return {@link #totalBenefit} (Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable).) 11951 */ 11952 public Money getTotalBenefit() { 11953 if (this.totalBenefit == null) 11954 if (Configuration.errorOnAutoCreate()) 11955 throw new Error("Attempt to auto-create ExplanationOfBenefit.totalBenefit"); 11956 else if (Configuration.doAutoCreate()) 11957 this.totalBenefit = new Money(); // cc 11958 return this.totalBenefit; 11959 } 11960 11961 public boolean hasTotalBenefit() { 11962 return this.totalBenefit != null && !this.totalBenefit.isEmpty(); 11963 } 11964 11965 /** 11966 * @param value {@link #totalBenefit} (Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable).) 11967 */ 11968 public ExplanationOfBenefit setTotalBenefit(Money value) { 11969 this.totalBenefit = value; 11970 return this; 11971 } 11972 11973 /** 11974 * @return {@link #payment} (Payment details for the claim if the claim has been paid.) 11975 */ 11976 public PaymentComponent getPayment() { 11977 if (this.payment == null) 11978 if (Configuration.errorOnAutoCreate()) 11979 throw new Error("Attempt to auto-create ExplanationOfBenefit.payment"); 11980 else if (Configuration.doAutoCreate()) 11981 this.payment = new PaymentComponent(); // cc 11982 return this.payment; 11983 } 11984 11985 public boolean hasPayment() { 11986 return this.payment != null && !this.payment.isEmpty(); 11987 } 11988 11989 /** 11990 * @param value {@link #payment} (Payment details for the claim if the claim has been paid.) 11991 */ 11992 public ExplanationOfBenefit setPayment(PaymentComponent value) { 11993 this.payment = value; 11994 return this; 11995 } 11996 11997 /** 11998 * @return {@link #form} (The form to be used for printing the content.) 11999 */ 12000 public CodeableConcept getForm() { 12001 if (this.form == null) 12002 if (Configuration.errorOnAutoCreate()) 12003 throw new Error("Attempt to auto-create ExplanationOfBenefit.form"); 12004 else if (Configuration.doAutoCreate()) 12005 this.form = new CodeableConcept(); // cc 12006 return this.form; 12007 } 12008 12009 public boolean hasForm() { 12010 return this.form != null && !this.form.isEmpty(); 12011 } 12012 12013 /** 12014 * @param value {@link #form} (The form to be used for printing the content.) 12015 */ 12016 public ExplanationOfBenefit setForm(CodeableConcept value) { 12017 this.form = value; 12018 return this; 12019 } 12020 12021 /** 12022 * @return {@link #processNote} (Note text.) 12023 */ 12024 public List<NoteComponent> getProcessNote() { 12025 if (this.processNote == null) 12026 this.processNote = new ArrayList<NoteComponent>(); 12027 return this.processNote; 12028 } 12029 12030 /** 12031 * @return Returns a reference to <code>this</code> for easy method chaining 12032 */ 12033 public ExplanationOfBenefit setProcessNote(List<NoteComponent> theProcessNote) { 12034 this.processNote = theProcessNote; 12035 return this; 12036 } 12037 12038 public boolean hasProcessNote() { 12039 if (this.processNote == null) 12040 return false; 12041 for (NoteComponent item : this.processNote) 12042 if (!item.isEmpty()) 12043 return true; 12044 return false; 12045 } 12046 12047 public NoteComponent addProcessNote() { //3 12048 NoteComponent t = new NoteComponent(); 12049 if (this.processNote == null) 12050 this.processNote = new ArrayList<NoteComponent>(); 12051 this.processNote.add(t); 12052 return t; 12053 } 12054 12055 public ExplanationOfBenefit addProcessNote(NoteComponent t) { //3 12056 if (t == null) 12057 return this; 12058 if (this.processNote == null) 12059 this.processNote = new ArrayList<NoteComponent>(); 12060 this.processNote.add(t); 12061 return this; 12062 } 12063 12064 /** 12065 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist 12066 */ 12067 public NoteComponent getProcessNoteFirstRep() { 12068 if (getProcessNote().isEmpty()) { 12069 addProcessNote(); 12070 } 12071 return getProcessNote().get(0); 12072 } 12073 12074 /** 12075 * @return {@link #benefitBalance} (Balance by Benefit Category.) 12076 */ 12077 public List<BenefitBalanceComponent> getBenefitBalance() { 12078 if (this.benefitBalance == null) 12079 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 12080 return this.benefitBalance; 12081 } 12082 12083 /** 12084 * @return Returns a reference to <code>this</code> for easy method chaining 12085 */ 12086 public ExplanationOfBenefit setBenefitBalance(List<BenefitBalanceComponent> theBenefitBalance) { 12087 this.benefitBalance = theBenefitBalance; 12088 return this; 12089 } 12090 12091 public boolean hasBenefitBalance() { 12092 if (this.benefitBalance == null) 12093 return false; 12094 for (BenefitBalanceComponent item : this.benefitBalance) 12095 if (!item.isEmpty()) 12096 return true; 12097 return false; 12098 } 12099 12100 public BenefitBalanceComponent addBenefitBalance() { //3 12101 BenefitBalanceComponent t = new BenefitBalanceComponent(); 12102 if (this.benefitBalance == null) 12103 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 12104 this.benefitBalance.add(t); 12105 return t; 12106 } 12107 12108 public ExplanationOfBenefit addBenefitBalance(BenefitBalanceComponent t) { //3 12109 if (t == null) 12110 return this; 12111 if (this.benefitBalance == null) 12112 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 12113 this.benefitBalance.add(t); 12114 return this; 12115 } 12116 12117 /** 12118 * @return The first repetition of repeating field {@link #benefitBalance}, creating it if it does not already exist 12119 */ 12120 public BenefitBalanceComponent getBenefitBalanceFirstRep() { 12121 if (getBenefitBalance().isEmpty()) { 12122 addBenefitBalance(); 12123 } 12124 return getBenefitBalance().get(0); 12125 } 12126 12127 protected void listChildren(List<Property> children) { 12128 super.listChildren(children); 12129 children.add(new Property("identifier", "Identifier", "The EOB Business Identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 12130 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 12131 children.add(new Property("type", "CodeableConcept", "The category of claim, eg, oral, pharmacy, vision, insitutional, professional.", 0, 1, type)); 12132 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.", 0, java.lang.Integer.MAX_VALUE, subType)); 12133 children.add(new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient)); 12134 children.add(new Property("billablePeriod", "Period", "The billable period for which charges are being submitted.", 0, 1, billablePeriod)); 12135 children.add(new Property("created", "dateTime", "The date when the EOB was created.", 0, 1, created)); 12136 children.add(new Property("enterer", "Reference(Practitioner)", "The person who created the explanation of benefit.", 0, 1, enterer)); 12137 children.add(new Property("insurer", "Reference(Organization)", "The insurer which is responsible for the explanation of benefit.", 0, 1, insurer)); 12138 children.add(new Property("provider", "Reference(Practitioner)", "The provider which is responsible for the claim.", 0, 1, provider)); 12139 children.add(new Property("organization", "Reference(Organization)", "The provider which is responsible for the claim.", 0, 1, organization)); 12140 children.add(new Property("referral", "Reference(ReferralRequest)", "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 1, referral)); 12141 children.add(new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility)); 12142 children.add(new Property("claim", "Reference(Claim)", "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 0, 1, claim)); 12143 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 0, 1, claimResponse)); 12144 children.add(new Property("outcome", "CodeableConcept", "Processing outcome errror, partial or complete processing.", 0, 1, outcome)); 12145 children.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition)); 12146 children.add(new Property("related", "", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, java.lang.Integer.MAX_VALUE, related)); 12147 children.add(new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription to support the dispensing of Pharmacy or Vision products.", 0, 1, prescription)); 12148 children.add(new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.", 0, 1, originalPrescription)); 12149 children.add(new Property("payee", "", "The party to be reimbursed for the services.", 0, 1, payee)); 12150 children.add(new Property("information", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.", 0, java.lang.Integer.MAX_VALUE, information)); 12151 children.add(new Property("careTeam", "", "The members of the team who provided the overall service as well as their role and whether responsible and qualifications.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 12152 children.add(new Property("diagnosis", "", "Ordered list of patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 12153 children.add(new Property("procedure", "", "Ordered list of patient procedures performed to support the adjudication.", 0, java.lang.Integer.MAX_VALUE, procedure)); 12154 children.add(new Property("precedence", "positiveInt", "Precedence (primary, secondary, etc.).", 0, 1, precedence)); 12155 children.add(new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, 1, insurance)); 12156 children.add(new Property("accident", "", "An accident which resulted in the need for healthcare services.", 0, 1, accident)); 12157 children.add(new Property("employmentImpacted", "Period", "The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).", 0, 1, employmentImpacted)); 12158 children.add(new Property("hospitalization", "Period", "The start and optional end dates of when the patient was confined to a treatment center.", 0, 1, hospitalization)); 12159 children.add(new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item)); 12160 children.add(new Property("addItem", "", "The first tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, addItem)); 12161 children.add(new Property("totalCost", "Money", "The total cost of the services reported.", 0, 1, totalCost)); 12162 children.add(new Property("unallocDeductable", "Money", "The amount of deductable applied which was not allocated to any particular service line.", 0, 1, unallocDeductable)); 12163 children.add(new Property("totalBenefit", "Money", "Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable).", 0, 1, totalBenefit)); 12164 children.add(new Property("payment", "", "Payment details for the claim if the claim has been paid.", 0, 1, payment)); 12165 children.add(new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form)); 12166 children.add(new Property("processNote", "", "Note text.", 0, java.lang.Integer.MAX_VALUE, processNote)); 12167 children.add(new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance)); 12168 } 12169 12170 @Override 12171 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12172 switch (_hash) { 12173 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The EOB Business Identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 12174 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 12175 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of claim, eg, oral, pharmacy, vision, insitutional, professional.", 0, 1, type); 12176 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.", 0, java.lang.Integer.MAX_VALUE, subType); 12177 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient); 12178 case -332066046: /*billablePeriod*/ return new Property("billablePeriod", "Period", "The billable period for which charges are being submitted.", 0, 1, billablePeriod); 12179 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the EOB was created.", 0, 1, created); 12180 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner)", "The person who created the explanation of benefit.", 0, 1, enterer); 12181 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The insurer which is responsible for the explanation of benefit.", 0, 1, insurer); 12182 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner)", "The provider which is responsible for the claim.", 0, 1, provider); 12183 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The provider which is responsible for the claim.", 0, 1, organization); 12184 case -722568291: /*referral*/ return new Property("referral", "Reference(ReferralRequest)", "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 1, referral); 12185 case 501116579: /*facility*/ return new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility); 12186 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 0, 1, claim); 12187 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 0, 1, claimResponse); 12188 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Processing outcome errror, partial or complete processing.", 0, 1, outcome); 12189 case 583380919: /*disposition*/ return new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition); 12190 case 1090493483: /*related*/ return new Property("related", "", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, java.lang.Integer.MAX_VALUE, related); 12191 case 460301338: /*prescription*/ return new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription to support the dispensing of Pharmacy or Vision products.", 0, 1, prescription); 12192 case -1814015861: /*originalPrescription*/ return new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.", 0, 1, originalPrescription); 12193 case 106443592: /*payee*/ return new Property("payee", "", "The party to be reimbursed for the services.", 0, 1, payee); 12194 case 1968600364: /*information*/ return new Property("information", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.", 0, java.lang.Integer.MAX_VALUE, information); 12195 case -7323378: /*careTeam*/ return new Property("careTeam", "", "The members of the team who provided the overall service as well as their role and whether responsible and qualifications.", 0, java.lang.Integer.MAX_VALUE, careTeam); 12196 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "Ordered list of patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 12197 case -1095204141: /*procedure*/ return new Property("procedure", "", "Ordered list of patient procedures performed to support the adjudication.", 0, java.lang.Integer.MAX_VALUE, procedure); 12198 case 159695370: /*precedence*/ return new Property("precedence", "positiveInt", "Precedence (primary, secondary, etc.).", 0, 1, precedence); 12199 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, 1, insurance); 12200 case -2143202801: /*accident*/ return new Property("accident", "", "An accident which resulted in the need for healthcare services.", 0, 1, accident); 12201 case 1051487345: /*employmentImpacted*/ return new Property("employmentImpacted", "Period", "The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).", 0, 1, employmentImpacted); 12202 case 1057894634: /*hospitalization*/ return new Property("hospitalization", "Period", "The start and optional end dates of when the patient was confined to a treatment center.", 0, 1, hospitalization); 12203 case 3242771: /*item*/ return new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item); 12204 case -1148899500: /*addItem*/ return new Property("addItem", "", "The first tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, addItem); 12205 case -577782479: /*totalCost*/ return new Property("totalCost", "Money", "The total cost of the services reported.", 0, 1, totalCost); 12206 case 2096309753: /*unallocDeductable*/ return new Property("unallocDeductable", "Money", "The amount of deductable applied which was not allocated to any particular service line.", 0, 1, unallocDeductable); 12207 case 332332211: /*totalBenefit*/ return new Property("totalBenefit", "Money", "Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable).", 0, 1, totalBenefit); 12208 case -786681338: /*payment*/ return new Property("payment", "", "Payment details for the claim if the claim has been paid.", 0, 1, payment); 12209 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form); 12210 case 202339073: /*processNote*/ return new Property("processNote", "", "Note text.", 0, java.lang.Integer.MAX_VALUE, processNote); 12211 case 596003397: /*benefitBalance*/ return new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance); 12212 default: return super.getNamedProperty(_hash, _name, _checkValid); 12213 } 12214 12215 } 12216 12217 @Override 12218 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12219 switch (hash) { 12220 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 12221 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ExplanationOfBenefitStatus> 12222 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 12223 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : this.subType.toArray(new Base[this.subType.size()]); // CodeableConcept 12224 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 12225 case -332066046: /*billablePeriod*/ return this.billablePeriod == null ? new Base[0] : new Base[] {this.billablePeriod}; // Period 12226 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 12227 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 12228 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 12229 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 12230 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 12231 case -722568291: /*referral*/ return this.referral == null ? new Base[0] : new Base[] {this.referral}; // Reference 12232 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 12233 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 12234 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 12235 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 12236 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 12237 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 12238 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 12239 case -1814015861: /*originalPrescription*/ return this.originalPrescription == null ? new Base[0] : new Base[] {this.originalPrescription}; // Reference 12240 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // PayeeComponent 12241 case 1968600364: /*information*/ return this.information == null ? new Base[0] : this.information.toArray(new Base[this.information.size()]); // SupportingInformationComponent 12242 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 12243 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 12244 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 12245 case 159695370: /*precedence*/ return this.precedence == null ? new Base[0] : new Base[] {this.precedence}; // PositiveIntType 12246 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : new Base[] {this.insurance}; // InsuranceComponent 12247 case -2143202801: /*accident*/ return this.accident == null ? new Base[0] : new Base[] {this.accident}; // AccidentComponent 12248 case 1051487345: /*employmentImpacted*/ return this.employmentImpacted == null ? new Base[0] : new Base[] {this.employmentImpacted}; // Period 12249 case 1057894634: /*hospitalization*/ return this.hospitalization == null ? new Base[0] : new Base[] {this.hospitalization}; // Period 12250 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 12251 case -1148899500: /*addItem*/ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 12252 case -577782479: /*totalCost*/ return this.totalCost == null ? new Base[0] : new Base[] {this.totalCost}; // Money 12253 case 2096309753: /*unallocDeductable*/ return this.unallocDeductable == null ? new Base[0] : new Base[] {this.unallocDeductable}; // Money 12254 case 332332211: /*totalBenefit*/ return this.totalBenefit == null ? new Base[0] : new Base[] {this.totalBenefit}; // Money 12255 case -786681338: /*payment*/ return this.payment == null ? new Base[0] : new Base[] {this.payment}; // PaymentComponent 12256 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 12257 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 12258 case 596003397: /*benefitBalance*/ return this.benefitBalance == null ? new Base[0] : this.benefitBalance.toArray(new Base[this.benefitBalance.size()]); // BenefitBalanceComponent 12259 default: return super.getProperty(hash, name, checkValid); 12260 } 12261 12262 } 12263 12264 @Override 12265 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12266 switch (hash) { 12267 case -1618432855: // identifier 12268 this.getIdentifier().add(castToIdentifier(value)); // Identifier 12269 return value; 12270 case -892481550: // status 12271 value = new ExplanationOfBenefitStatusEnumFactory().fromType(castToCode(value)); 12272 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 12273 return value; 12274 case 3575610: // type 12275 this.type = castToCodeableConcept(value); // CodeableConcept 12276 return value; 12277 case -1868521062: // subType 12278 this.getSubType().add(castToCodeableConcept(value)); // CodeableConcept 12279 return value; 12280 case -791418107: // patient 12281 this.patient = castToReference(value); // Reference 12282 return value; 12283 case -332066046: // billablePeriod 12284 this.billablePeriod = castToPeriod(value); // Period 12285 return value; 12286 case 1028554472: // created 12287 this.created = castToDateTime(value); // DateTimeType 12288 return value; 12289 case -1591951995: // enterer 12290 this.enterer = castToReference(value); // Reference 12291 return value; 12292 case 1957615864: // insurer 12293 this.insurer = castToReference(value); // Reference 12294 return value; 12295 case -987494927: // provider 12296 this.provider = castToReference(value); // Reference 12297 return value; 12298 case 1178922291: // organization 12299 this.organization = castToReference(value); // Reference 12300 return value; 12301 case -722568291: // referral 12302 this.referral = castToReference(value); // Reference 12303 return value; 12304 case 501116579: // facility 12305 this.facility = castToReference(value); // Reference 12306 return value; 12307 case 94742588: // claim 12308 this.claim = castToReference(value); // Reference 12309 return value; 12310 case 689513629: // claimResponse 12311 this.claimResponse = castToReference(value); // Reference 12312 return value; 12313 case -1106507950: // outcome 12314 this.outcome = castToCodeableConcept(value); // CodeableConcept 12315 return value; 12316 case 583380919: // disposition 12317 this.disposition = castToString(value); // StringType 12318 return value; 12319 case 1090493483: // related 12320 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 12321 return value; 12322 case 460301338: // prescription 12323 this.prescription = castToReference(value); // Reference 12324 return value; 12325 case -1814015861: // originalPrescription 12326 this.originalPrescription = castToReference(value); // Reference 12327 return value; 12328 case 106443592: // payee 12329 this.payee = (PayeeComponent) value; // PayeeComponent 12330 return value; 12331 case 1968600364: // information 12332 this.getInformation().add((SupportingInformationComponent) value); // SupportingInformationComponent 12333 return value; 12334 case -7323378: // careTeam 12335 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 12336 return value; 12337 case 1196993265: // diagnosis 12338 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 12339 return value; 12340 case -1095204141: // procedure 12341 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 12342 return value; 12343 case 159695370: // precedence 12344 this.precedence = castToPositiveInt(value); // PositiveIntType 12345 return value; 12346 case 73049818: // insurance 12347 this.insurance = (InsuranceComponent) value; // InsuranceComponent 12348 return value; 12349 case -2143202801: // accident 12350 this.accident = (AccidentComponent) value; // AccidentComponent 12351 return value; 12352 case 1051487345: // employmentImpacted 12353 this.employmentImpacted = castToPeriod(value); // Period 12354 return value; 12355 case 1057894634: // hospitalization 12356 this.hospitalization = castToPeriod(value); // Period 12357 return value; 12358 case 3242771: // item 12359 this.getItem().add((ItemComponent) value); // ItemComponent 12360 return value; 12361 case -1148899500: // addItem 12362 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 12363 return value; 12364 case -577782479: // totalCost 12365 this.totalCost = castToMoney(value); // Money 12366 return value; 12367 case 2096309753: // unallocDeductable 12368 this.unallocDeductable = castToMoney(value); // Money 12369 return value; 12370 case 332332211: // totalBenefit 12371 this.totalBenefit = castToMoney(value); // Money 12372 return value; 12373 case -786681338: // payment 12374 this.payment = (PaymentComponent) value; // PaymentComponent 12375 return value; 12376 case 3148996: // form 12377 this.form = castToCodeableConcept(value); // CodeableConcept 12378 return value; 12379 case 202339073: // processNote 12380 this.getProcessNote().add((NoteComponent) value); // NoteComponent 12381 return value; 12382 case 596003397: // benefitBalance 12383 this.getBenefitBalance().add((BenefitBalanceComponent) value); // BenefitBalanceComponent 12384 return value; 12385 default: return super.setProperty(hash, name, value); 12386 } 12387 12388 } 12389 12390 @Override 12391 public Base setProperty(String name, Base value) throws FHIRException { 12392 if (name.equals("identifier")) { 12393 this.getIdentifier().add(castToIdentifier(value)); 12394 } else if (name.equals("status")) { 12395 value = new ExplanationOfBenefitStatusEnumFactory().fromType(castToCode(value)); 12396 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 12397 } else if (name.equals("type")) { 12398 this.type = castToCodeableConcept(value); // CodeableConcept 12399 } else if (name.equals("subType")) { 12400 this.getSubType().add(castToCodeableConcept(value)); 12401 } else if (name.equals("patient")) { 12402 this.patient = castToReference(value); // Reference 12403 } else if (name.equals("billablePeriod")) { 12404 this.billablePeriod = castToPeriod(value); // Period 12405 } else if (name.equals("created")) { 12406 this.created = castToDateTime(value); // DateTimeType 12407 } else if (name.equals("enterer")) { 12408 this.enterer = castToReference(value); // Reference 12409 } else if (name.equals("insurer")) { 12410 this.insurer = castToReference(value); // Reference 12411 } else if (name.equals("provider")) { 12412 this.provider = castToReference(value); // Reference 12413 } else if (name.equals("organization")) { 12414 this.organization = castToReference(value); // Reference 12415 } else if (name.equals("referral")) { 12416 this.referral = castToReference(value); // Reference 12417 } else if (name.equals("facility")) { 12418 this.facility = castToReference(value); // Reference 12419 } else if (name.equals("claim")) { 12420 this.claim = castToReference(value); // Reference 12421 } else if (name.equals("claimResponse")) { 12422 this.claimResponse = castToReference(value); // Reference 12423 } else if (name.equals("outcome")) { 12424 this.outcome = castToCodeableConcept(value); // CodeableConcept 12425 } else if (name.equals("disposition")) { 12426 this.disposition = castToString(value); // StringType 12427 } else if (name.equals("related")) { 12428 this.getRelated().add((RelatedClaimComponent) value); 12429 } else if (name.equals("prescription")) { 12430 this.prescription = castToReference(value); // Reference 12431 } else if (name.equals("originalPrescription")) { 12432 this.originalPrescription = castToReference(value); // Reference 12433 } else if (name.equals("payee")) { 12434 this.payee = (PayeeComponent) value; // PayeeComponent 12435 } else if (name.equals("information")) { 12436 this.getInformation().add((SupportingInformationComponent) value); 12437 } else if (name.equals("careTeam")) { 12438 this.getCareTeam().add((CareTeamComponent) value); 12439 } else if (name.equals("diagnosis")) { 12440 this.getDiagnosis().add((DiagnosisComponent) value); 12441 } else if (name.equals("procedure")) { 12442 this.getProcedure().add((ProcedureComponent) value); 12443 } else if (name.equals("precedence")) { 12444 this.precedence = castToPositiveInt(value); // PositiveIntType 12445 } else if (name.equals("insurance")) { 12446 this.insurance = (InsuranceComponent) value; // InsuranceComponent 12447 } else if (name.equals("accident")) { 12448 this.accident = (AccidentComponent) value; // AccidentComponent 12449 } else if (name.equals("employmentImpacted")) { 12450 this.employmentImpacted = castToPeriod(value); // Period 12451 } else if (name.equals("hospitalization")) { 12452 this.hospitalization = castToPeriod(value); // Period 12453 } else if (name.equals("item")) { 12454 this.getItem().add((ItemComponent) value); 12455 } else if (name.equals("addItem")) { 12456 this.getAddItem().add((AddedItemComponent) value); 12457 } else if (name.equals("totalCost")) { 12458 this.totalCost = castToMoney(value); // Money 12459 } else if (name.equals("unallocDeductable")) { 12460 this.unallocDeductable = castToMoney(value); // Money 12461 } else if (name.equals("totalBenefit")) { 12462 this.totalBenefit = castToMoney(value); // Money 12463 } else if (name.equals("payment")) { 12464 this.payment = (PaymentComponent) value; // PaymentComponent 12465 } else if (name.equals("form")) { 12466 this.form = castToCodeableConcept(value); // CodeableConcept 12467 } else if (name.equals("processNote")) { 12468 this.getProcessNote().add((NoteComponent) value); 12469 } else if (name.equals("benefitBalance")) { 12470 this.getBenefitBalance().add((BenefitBalanceComponent) value); 12471 } else 12472 return super.setProperty(name, value); 12473 return value; 12474 } 12475 12476 @Override 12477 public Base makeProperty(int hash, String name) throws FHIRException { 12478 switch (hash) { 12479 case -1618432855: return addIdentifier(); 12480 case -892481550: return getStatusElement(); 12481 case 3575610: return getType(); 12482 case -1868521062: return addSubType(); 12483 case -791418107: return getPatient(); 12484 case -332066046: return getBillablePeriod(); 12485 case 1028554472: return getCreatedElement(); 12486 case -1591951995: return getEnterer(); 12487 case 1957615864: return getInsurer(); 12488 case -987494927: return getProvider(); 12489 case 1178922291: return getOrganization(); 12490 case -722568291: return getReferral(); 12491 case 501116579: return getFacility(); 12492 case 94742588: return getClaim(); 12493 case 689513629: return getClaimResponse(); 12494 case -1106507950: return getOutcome(); 12495 case 583380919: return getDispositionElement(); 12496 case 1090493483: return addRelated(); 12497 case 460301338: return getPrescription(); 12498 case -1814015861: return getOriginalPrescription(); 12499 case 106443592: return getPayee(); 12500 case 1968600364: return addInformation(); 12501 case -7323378: return addCareTeam(); 12502 case 1196993265: return addDiagnosis(); 12503 case -1095204141: return addProcedure(); 12504 case 159695370: return getPrecedenceElement(); 12505 case 73049818: return getInsurance(); 12506 case -2143202801: return getAccident(); 12507 case 1051487345: return getEmploymentImpacted(); 12508 case 1057894634: return getHospitalization(); 12509 case 3242771: return addItem(); 12510 case -1148899500: return addAddItem(); 12511 case -577782479: return getTotalCost(); 12512 case 2096309753: return getUnallocDeductable(); 12513 case 332332211: return getTotalBenefit(); 12514 case -786681338: return getPayment(); 12515 case 3148996: return getForm(); 12516 case 202339073: return addProcessNote(); 12517 case 596003397: return addBenefitBalance(); 12518 default: return super.makeProperty(hash, name); 12519 } 12520 12521 } 12522 12523 @Override 12524 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12525 switch (hash) { 12526 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 12527 case -892481550: /*status*/ return new String[] {"code"}; 12528 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 12529 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 12530 case -791418107: /*patient*/ return new String[] {"Reference"}; 12531 case -332066046: /*billablePeriod*/ return new String[] {"Period"}; 12532 case 1028554472: /*created*/ return new String[] {"dateTime"}; 12533 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 12534 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 12535 case -987494927: /*provider*/ return new String[] {"Reference"}; 12536 case 1178922291: /*organization*/ return new String[] {"Reference"}; 12537 case -722568291: /*referral*/ return new String[] {"Reference"}; 12538 case 501116579: /*facility*/ return new String[] {"Reference"}; 12539 case 94742588: /*claim*/ return new String[] {"Reference"}; 12540 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 12541 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 12542 case 583380919: /*disposition*/ return new String[] {"string"}; 12543 case 1090493483: /*related*/ return new String[] {}; 12544 case 460301338: /*prescription*/ return new String[] {"Reference"}; 12545 case -1814015861: /*originalPrescription*/ return new String[] {"Reference"}; 12546 case 106443592: /*payee*/ return new String[] {}; 12547 case 1968600364: /*information*/ return new String[] {}; 12548 case -7323378: /*careTeam*/ return new String[] {}; 12549 case 1196993265: /*diagnosis*/ return new String[] {}; 12550 case -1095204141: /*procedure*/ return new String[] {}; 12551 case 159695370: /*precedence*/ return new String[] {"positiveInt"}; 12552 case 73049818: /*insurance*/ return new String[] {}; 12553 case -2143202801: /*accident*/ return new String[] {}; 12554 case 1051487345: /*employmentImpacted*/ return new String[] {"Period"}; 12555 case 1057894634: /*hospitalization*/ return new String[] {"Period"}; 12556 case 3242771: /*item*/ return new String[] {}; 12557 case -1148899500: /*addItem*/ return new String[] {}; 12558 case -577782479: /*totalCost*/ return new String[] {"Money"}; 12559 case 2096309753: /*unallocDeductable*/ return new String[] {"Money"}; 12560 case 332332211: /*totalBenefit*/ return new String[] {"Money"}; 12561 case -786681338: /*payment*/ return new String[] {}; 12562 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 12563 case 202339073: /*processNote*/ return new String[] {}; 12564 case 596003397: /*benefitBalance*/ return new String[] {}; 12565 default: return super.getTypesForProperty(hash, name); 12566 } 12567 12568 } 12569 12570 @Override 12571 public Base addChild(String name) throws FHIRException { 12572 if (name.equals("identifier")) { 12573 return addIdentifier(); 12574 } 12575 else if (name.equals("status")) { 12576 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.status"); 12577 } 12578 else if (name.equals("type")) { 12579 this.type = new CodeableConcept(); 12580 return this.type; 12581 } 12582 else if (name.equals("subType")) { 12583 return addSubType(); 12584 } 12585 else if (name.equals("patient")) { 12586 this.patient = new Reference(); 12587 return this.patient; 12588 } 12589 else if (name.equals("billablePeriod")) { 12590 this.billablePeriod = new Period(); 12591 return this.billablePeriod; 12592 } 12593 else if (name.equals("created")) { 12594 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.created"); 12595 } 12596 else if (name.equals("enterer")) { 12597 this.enterer = new Reference(); 12598 return this.enterer; 12599 } 12600 else if (name.equals("insurer")) { 12601 this.insurer = new Reference(); 12602 return this.insurer; 12603 } 12604 else if (name.equals("provider")) { 12605 this.provider = new Reference(); 12606 return this.provider; 12607 } 12608 else if (name.equals("organization")) { 12609 this.organization = new Reference(); 12610 return this.organization; 12611 } 12612 else if (name.equals("referral")) { 12613 this.referral = new Reference(); 12614 return this.referral; 12615 } 12616 else if (name.equals("facility")) { 12617 this.facility = new Reference(); 12618 return this.facility; 12619 } 12620 else if (name.equals("claim")) { 12621 this.claim = new Reference(); 12622 return this.claim; 12623 } 12624 else if (name.equals("claimResponse")) { 12625 this.claimResponse = new Reference(); 12626 return this.claimResponse; 12627 } 12628 else if (name.equals("outcome")) { 12629 this.outcome = new CodeableConcept(); 12630 return this.outcome; 12631 } 12632 else if (name.equals("disposition")) { 12633 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.disposition"); 12634 } 12635 else if (name.equals("related")) { 12636 return addRelated(); 12637 } 12638 else if (name.equals("prescription")) { 12639 this.prescription = new Reference(); 12640 return this.prescription; 12641 } 12642 else if (name.equals("originalPrescription")) { 12643 this.originalPrescription = new Reference(); 12644 return this.originalPrescription; 12645 } 12646 else if (name.equals("payee")) { 12647 this.payee = new PayeeComponent(); 12648 return this.payee; 12649 } 12650 else if (name.equals("information")) { 12651 return addInformation(); 12652 } 12653 else if (name.equals("careTeam")) { 12654 return addCareTeam(); 12655 } 12656 else if (name.equals("diagnosis")) { 12657 return addDiagnosis(); 12658 } 12659 else if (name.equals("procedure")) { 12660 return addProcedure(); 12661 } 12662 else if (name.equals("precedence")) { 12663 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.precedence"); 12664 } 12665 else if (name.equals("insurance")) { 12666 this.insurance = new InsuranceComponent(); 12667 return this.insurance; 12668 } 12669 else if (name.equals("accident")) { 12670 this.accident = new AccidentComponent(); 12671 return this.accident; 12672 } 12673 else if (name.equals("employmentImpacted")) { 12674 this.employmentImpacted = new Period(); 12675 return this.employmentImpacted; 12676 } 12677 else if (name.equals("hospitalization")) { 12678 this.hospitalization = new Period(); 12679 return this.hospitalization; 12680 } 12681 else if (name.equals("item")) { 12682 return addItem(); 12683 } 12684 else if (name.equals("addItem")) { 12685 return addAddItem(); 12686 } 12687 else if (name.equals("totalCost")) { 12688 this.totalCost = new Money(); 12689 return this.totalCost; 12690 } 12691 else if (name.equals("unallocDeductable")) { 12692 this.unallocDeductable = new Money(); 12693 return this.unallocDeductable; 12694 } 12695 else if (name.equals("totalBenefit")) { 12696 this.totalBenefit = new Money(); 12697 return this.totalBenefit; 12698 } 12699 else if (name.equals("payment")) { 12700 this.payment = new PaymentComponent(); 12701 return this.payment; 12702 } 12703 else if (name.equals("form")) { 12704 this.form = new CodeableConcept(); 12705 return this.form; 12706 } 12707 else if (name.equals("processNote")) { 12708 return addProcessNote(); 12709 } 12710 else if (name.equals("benefitBalance")) { 12711 return addBenefitBalance(); 12712 } 12713 else 12714 return super.addChild(name); 12715 } 12716 12717 public String fhirType() { 12718 return "ExplanationOfBenefit"; 12719 12720 } 12721 12722 public ExplanationOfBenefit copy() { 12723 ExplanationOfBenefit dst = new ExplanationOfBenefit(); 12724 copyValues(dst); 12725 if (identifier != null) { 12726 dst.identifier = new ArrayList<Identifier>(); 12727 for (Identifier i : identifier) 12728 dst.identifier.add(i.copy()); 12729 }; 12730 dst.status = status == null ? null : status.copy(); 12731 dst.type = type == null ? null : type.copy(); 12732 if (subType != null) { 12733 dst.subType = new ArrayList<CodeableConcept>(); 12734 for (CodeableConcept i : subType) 12735 dst.subType.add(i.copy()); 12736 }; 12737 dst.patient = patient == null ? null : patient.copy(); 12738 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 12739 dst.created = created == null ? null : created.copy(); 12740 dst.enterer = enterer == null ? null : enterer.copy(); 12741 dst.insurer = insurer == null ? null : insurer.copy(); 12742 dst.provider = provider == null ? null : provider.copy(); 12743 dst.organization = organization == null ? null : organization.copy(); 12744 dst.referral = referral == null ? null : referral.copy(); 12745 dst.facility = facility == null ? null : facility.copy(); 12746 dst.claim = claim == null ? null : claim.copy(); 12747 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 12748 dst.outcome = outcome == null ? null : outcome.copy(); 12749 dst.disposition = disposition == null ? null : disposition.copy(); 12750 if (related != null) { 12751 dst.related = new ArrayList<RelatedClaimComponent>(); 12752 for (RelatedClaimComponent i : related) 12753 dst.related.add(i.copy()); 12754 }; 12755 dst.prescription = prescription == null ? null : prescription.copy(); 12756 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 12757 dst.payee = payee == null ? null : payee.copy(); 12758 if (information != null) { 12759 dst.information = new ArrayList<SupportingInformationComponent>(); 12760 for (SupportingInformationComponent i : information) 12761 dst.information.add(i.copy()); 12762 }; 12763 if (careTeam != null) { 12764 dst.careTeam = new ArrayList<CareTeamComponent>(); 12765 for (CareTeamComponent i : careTeam) 12766 dst.careTeam.add(i.copy()); 12767 }; 12768 if (diagnosis != null) { 12769 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 12770 for (DiagnosisComponent i : diagnosis) 12771 dst.diagnosis.add(i.copy()); 12772 }; 12773 if (procedure != null) { 12774 dst.procedure = new ArrayList<ProcedureComponent>(); 12775 for (ProcedureComponent i : procedure) 12776 dst.procedure.add(i.copy()); 12777 }; 12778 dst.precedence = precedence == null ? null : precedence.copy(); 12779 dst.insurance = insurance == null ? null : insurance.copy(); 12780 dst.accident = accident == null ? null : accident.copy(); 12781 dst.employmentImpacted = employmentImpacted == null ? null : employmentImpacted.copy(); 12782 dst.hospitalization = hospitalization == null ? null : hospitalization.copy(); 12783 if (item != null) { 12784 dst.item = new ArrayList<ItemComponent>(); 12785 for (ItemComponent i : item) 12786 dst.item.add(i.copy()); 12787 }; 12788 if (addItem != null) { 12789 dst.addItem = new ArrayList<AddedItemComponent>(); 12790 for (AddedItemComponent i : addItem) 12791 dst.addItem.add(i.copy()); 12792 }; 12793 dst.totalCost = totalCost == null ? null : totalCost.copy(); 12794 dst.unallocDeductable = unallocDeductable == null ? null : unallocDeductable.copy(); 12795 dst.totalBenefit = totalBenefit == null ? null : totalBenefit.copy(); 12796 dst.payment = payment == null ? null : payment.copy(); 12797 dst.form = form == null ? null : form.copy(); 12798 if (processNote != null) { 12799 dst.processNote = new ArrayList<NoteComponent>(); 12800 for (NoteComponent i : processNote) 12801 dst.processNote.add(i.copy()); 12802 }; 12803 if (benefitBalance != null) { 12804 dst.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 12805 for (BenefitBalanceComponent i : benefitBalance) 12806 dst.benefitBalance.add(i.copy()); 12807 }; 12808 return dst; 12809 } 12810 12811 protected ExplanationOfBenefit typedCopy() { 12812 return copy(); 12813 } 12814 12815 @Override 12816 public boolean equalsDeep(Base other_) { 12817 if (!super.equalsDeep(other_)) 12818 return false; 12819 if (!(other_ instanceof ExplanationOfBenefit)) 12820 return false; 12821 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 12822 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 12823 && compareDeep(subType, o.subType, true) && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 12824 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) && compareDeep(insurer, o.insurer, true) 12825 && compareDeep(provider, o.provider, true) && compareDeep(organization, o.organization, true) && compareDeep(referral, o.referral, true) 12826 && compareDeep(facility, o.facility, true) && compareDeep(claim, o.claim, true) && compareDeep(claimResponse, o.claimResponse, true) 12827 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) && compareDeep(related, o.related, true) 12828 && compareDeep(prescription, o.prescription, true) && compareDeep(originalPrescription, o.originalPrescription, true) 12829 && compareDeep(payee, o.payee, true) && compareDeep(information, o.information, true) && compareDeep(careTeam, o.careTeam, true) 12830 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(procedure, o.procedure, true) && compareDeep(precedence, o.precedence, true) 12831 && compareDeep(insurance, o.insurance, true) && compareDeep(accident, o.accident, true) && compareDeep(employmentImpacted, o.employmentImpacted, true) 12832 && compareDeep(hospitalization, o.hospitalization, true) && compareDeep(item, o.item, true) && compareDeep(addItem, o.addItem, true) 12833 && compareDeep(totalCost, o.totalCost, true) && compareDeep(unallocDeductable, o.unallocDeductable, true) 12834 && compareDeep(totalBenefit, o.totalBenefit, true) && compareDeep(payment, o.payment, true) && compareDeep(form, o.form, true) 12835 && compareDeep(processNote, o.processNote, true) && compareDeep(benefitBalance, o.benefitBalance, true) 12836 ; 12837 } 12838 12839 @Override 12840 public boolean equalsShallow(Base other_) { 12841 if (!super.equalsShallow(other_)) 12842 return false; 12843 if (!(other_ instanceof ExplanationOfBenefit)) 12844 return false; 12845 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 12846 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(disposition, o.disposition, true) 12847 && compareValues(precedence, o.precedence, true); 12848 } 12849 12850 public boolean isEmpty() { 12851 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 12852 , subType, patient, billablePeriod, created, enterer, insurer, provider, organization 12853 , referral, facility, claim, claimResponse, outcome, disposition, related, prescription 12854 , originalPrescription, payee, information, careTeam, diagnosis, procedure, precedence 12855 , insurance, accident, employmentImpacted, hospitalization, item, addItem, totalCost 12856 , unallocDeductable, totalBenefit, payment, form, processNote, benefitBalance); 12857 } 12858 12859 @Override 12860 public ResourceType getResourceType() { 12861 return ResourceType.ExplanationOfBenefit; 12862 } 12863 12864 /** 12865 * Search parameter: <b>coverage</b> 12866 * <p> 12867 * Description: <b>The plan under which the claim was adjudicated</b><br> 12868 * Type: <b>reference</b><br> 12869 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 12870 * </p> 12871 */ 12872 @SearchParamDefinition(name="coverage", path="ExplanationOfBenefit.insurance.coverage", description="The plan under which the claim was adjudicated", type="reference", target={Coverage.class } ) 12873 public static final String SP_COVERAGE = "coverage"; 12874 /** 12875 * <b>Fluent Client</b> search parameter constant for <b>coverage</b> 12876 * <p> 12877 * Description: <b>The plan under which the claim was adjudicated</b><br> 12878 * Type: <b>reference</b><br> 12879 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 12880 * </p> 12881 */ 12882 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COVERAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COVERAGE); 12883 12884/** 12885 * Constant for fluent queries to be used to add include statements. Specifies 12886 * the path value of "<b>ExplanationOfBenefit:coverage</b>". 12887 */ 12888 public static final ca.uhn.fhir.model.api.Include INCLUDE_COVERAGE = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:coverage").toLocked(); 12889 12890 /** 12891 * Search parameter: <b>care-team</b> 12892 * <p> 12893 * Description: <b>Member of the CareTeam</b><br> 12894 * Type: <b>reference</b><br> 12895 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 12896 * </p> 12897 */ 12898 @SearchParamDefinition(name="care-team", path="ExplanationOfBenefit.careTeam.provider", description="Member of the CareTeam", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 12899 public static final String SP_CARE_TEAM = "care-team"; 12900 /** 12901 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 12902 * <p> 12903 * Description: <b>Member of the CareTeam</b><br> 12904 * Type: <b>reference</b><br> 12905 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 12906 * </p> 12907 */ 12908 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 12909 12910/** 12911 * Constant for fluent queries to be used to add include statements. Specifies 12912 * the path value of "<b>ExplanationOfBenefit:care-team</b>". 12913 */ 12914 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:care-team").toLocked(); 12915 12916 /** 12917 * Search parameter: <b>identifier</b> 12918 * <p> 12919 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 12920 * Type: <b>token</b><br> 12921 * Path: <b>ExplanationOfBenefit.identifier</b><br> 12922 * </p> 12923 */ 12924 @SearchParamDefinition(name="identifier", path="ExplanationOfBenefit.identifier", description="The business identifier of the Explanation of Benefit", type="token" ) 12925 public static final String SP_IDENTIFIER = "identifier"; 12926 /** 12927 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 12928 * <p> 12929 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 12930 * Type: <b>token</b><br> 12931 * Path: <b>ExplanationOfBenefit.identifier</b><br> 12932 * </p> 12933 */ 12934 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 12935 12936 /** 12937 * Search parameter: <b>created</b> 12938 * <p> 12939 * Description: <b>The creation date for the EOB</b><br> 12940 * Type: <b>date</b><br> 12941 * Path: <b>ExplanationOfBenefit.created</b><br> 12942 * </p> 12943 */ 12944 @SearchParamDefinition(name="created", path="ExplanationOfBenefit.created", description="The creation date for the EOB", type="date" ) 12945 public static final String SP_CREATED = "created"; 12946 /** 12947 * <b>Fluent Client</b> search parameter constant for <b>created</b> 12948 * <p> 12949 * Description: <b>The creation date for the EOB</b><br> 12950 * Type: <b>date</b><br> 12951 * Path: <b>ExplanationOfBenefit.created</b><br> 12952 * </p> 12953 */ 12954 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 12955 12956 /** 12957 * Search parameter: <b>encounter</b> 12958 * <p> 12959 * Description: <b>Encounters associated with a billed line item</b><br> 12960 * Type: <b>reference</b><br> 12961 * Path: <b>ExplanationOfBenefit.item.encounter</b><br> 12962 * </p> 12963 */ 12964 @SearchParamDefinition(name="encounter", path="ExplanationOfBenefit.item.encounter", description="Encounters associated with a billed line item", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 12965 public static final String SP_ENCOUNTER = "encounter"; 12966 /** 12967 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 12968 * <p> 12969 * Description: <b>Encounters associated with a billed line item</b><br> 12970 * Type: <b>reference</b><br> 12971 * Path: <b>ExplanationOfBenefit.item.encounter</b><br> 12972 * </p> 12973 */ 12974 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 12975 12976/** 12977 * Constant for fluent queries to be used to add include statements. Specifies 12978 * the path value of "<b>ExplanationOfBenefit:encounter</b>". 12979 */ 12980 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:encounter").toLocked(); 12981 12982 /** 12983 * Search parameter: <b>payee</b> 12984 * <p> 12985 * Description: <b>The party receiving any payment for the Claim</b><br> 12986 * Type: <b>reference</b><br> 12987 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 12988 * </p> 12989 */ 12990 @SearchParamDefinition(name="payee", path="ExplanationOfBenefit.payee.party", description="The party receiving any payment for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 12991 public static final String SP_PAYEE = "payee"; 12992 /** 12993 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 12994 * <p> 12995 * Description: <b>The party receiving any payment for the Claim</b><br> 12996 * Type: <b>reference</b><br> 12997 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 12998 * </p> 12999 */ 13000 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYEE); 13001 13002/** 13003 * Constant for fluent queries to be used to add include statements. Specifies 13004 * the path value of "<b>ExplanationOfBenefit:payee</b>". 13005 */ 13006 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:payee").toLocked(); 13007 13008 /** 13009 * Search parameter: <b>disposition</b> 13010 * <p> 13011 * Description: <b>The contents of the disposition message</b><br> 13012 * Type: <b>string</b><br> 13013 * Path: <b>ExplanationOfBenefit.disposition</b><br> 13014 * </p> 13015 */ 13016 @SearchParamDefinition(name="disposition", path="ExplanationOfBenefit.disposition", description="The contents of the disposition message", type="string" ) 13017 public static final String SP_DISPOSITION = "disposition"; 13018 /** 13019 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 13020 * <p> 13021 * Description: <b>The contents of the disposition message</b><br> 13022 * Type: <b>string</b><br> 13023 * Path: <b>ExplanationOfBenefit.disposition</b><br> 13024 * </p> 13025 */ 13026 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 13027 13028 /** 13029 * Search parameter: <b>provider</b> 13030 * <p> 13031 * Description: <b>The reference to the provider</b><br> 13032 * Type: <b>reference</b><br> 13033 * Path: <b>ExplanationOfBenefit.provider</b><br> 13034 * </p> 13035 */ 13036 @SearchParamDefinition(name="provider", path="ExplanationOfBenefit.provider", description="The reference to the provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 13037 public static final String SP_PROVIDER = "provider"; 13038 /** 13039 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 13040 * <p> 13041 * Description: <b>The reference to the provider</b><br> 13042 * Type: <b>reference</b><br> 13043 * Path: <b>ExplanationOfBenefit.provider</b><br> 13044 * </p> 13045 */ 13046 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 13047 13048/** 13049 * Constant for fluent queries to be used to add include statements. Specifies 13050 * the path value of "<b>ExplanationOfBenefit:provider</b>". 13051 */ 13052 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:provider").toLocked(); 13053 13054 /** 13055 * Search parameter: <b>patient</b> 13056 * <p> 13057 * Description: <b>The reference to the patient</b><br> 13058 * Type: <b>reference</b><br> 13059 * Path: <b>ExplanationOfBenefit.patient</b><br> 13060 * </p> 13061 */ 13062 @SearchParamDefinition(name="patient", path="ExplanationOfBenefit.patient", description="The reference to the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 13063 public static final String SP_PATIENT = "patient"; 13064 /** 13065 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 13066 * <p> 13067 * Description: <b>The reference to the patient</b><br> 13068 * Type: <b>reference</b><br> 13069 * Path: <b>ExplanationOfBenefit.patient</b><br> 13070 * </p> 13071 */ 13072 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 13073 13074/** 13075 * Constant for fluent queries to be used to add include statements. Specifies 13076 * the path value of "<b>ExplanationOfBenefit:patient</b>". 13077 */ 13078 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:patient").toLocked(); 13079 13080 /** 13081 * Search parameter: <b>organization</b> 13082 * <p> 13083 * Description: <b>The reference to the providing organization</b><br> 13084 * Type: <b>reference</b><br> 13085 * Path: <b>ExplanationOfBenefit.organization</b><br> 13086 * </p> 13087 */ 13088 @SearchParamDefinition(name="organization", path="ExplanationOfBenefit.organization", description="The reference to the providing organization", type="reference", target={Organization.class } ) 13089 public static final String SP_ORGANIZATION = "organization"; 13090 /** 13091 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 13092 * <p> 13093 * Description: <b>The reference to the providing organization</b><br> 13094 * Type: <b>reference</b><br> 13095 * Path: <b>ExplanationOfBenefit.organization</b><br> 13096 * </p> 13097 */ 13098 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 13099 13100/** 13101 * Constant for fluent queries to be used to add include statements. Specifies 13102 * the path value of "<b>ExplanationOfBenefit:organization</b>". 13103 */ 13104 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:organization").toLocked(); 13105 13106 /** 13107 * Search parameter: <b>claim</b> 13108 * <p> 13109 * Description: <b>The reference to the claim</b><br> 13110 * Type: <b>reference</b><br> 13111 * Path: <b>ExplanationOfBenefit.claim</b><br> 13112 * </p> 13113 */ 13114 @SearchParamDefinition(name="claim", path="ExplanationOfBenefit.claim", description="The reference to the claim", type="reference", target={Claim.class } ) 13115 public static final String SP_CLAIM = "claim"; 13116 /** 13117 * <b>Fluent Client</b> search parameter constant for <b>claim</b> 13118 * <p> 13119 * Description: <b>The reference to the claim</b><br> 13120 * Type: <b>reference</b><br> 13121 * Path: <b>ExplanationOfBenefit.claim</b><br> 13122 * </p> 13123 */ 13124 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CLAIM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CLAIM); 13125 13126/** 13127 * Constant for fluent queries to be used to add include statements. Specifies 13128 * the path value of "<b>ExplanationOfBenefit:claim</b>". 13129 */ 13130 public static final ca.uhn.fhir.model.api.Include INCLUDE_CLAIM = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:claim").toLocked(); 13131 13132 /** 13133 * Search parameter: <b>enterer</b> 13134 * <p> 13135 * Description: <b>The party responsible for the entry of the Claim</b><br> 13136 * Type: <b>reference</b><br> 13137 * Path: <b>ExplanationOfBenefit.enterer</b><br> 13138 * </p> 13139 */ 13140 @SearchParamDefinition(name="enterer", path="ExplanationOfBenefit.enterer", description="The party responsible for the entry of the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 13141 public static final String SP_ENTERER = "enterer"; 13142 /** 13143 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 13144 * <p> 13145 * Description: <b>The party responsible for the entry of the Claim</b><br> 13146 * Type: <b>reference</b><br> 13147 * Path: <b>ExplanationOfBenefit.enterer</b><br> 13148 * </p> 13149 */ 13150 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 13151 13152/** 13153 * Constant for fluent queries to be used to add include statements. Specifies 13154 * the path value of "<b>ExplanationOfBenefit:enterer</b>". 13155 */ 13156 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:enterer").toLocked(); 13157 13158 /** 13159 * Search parameter: <b>facility</b> 13160 * <p> 13161 * Description: <b>Facility responsible for the goods and services</b><br> 13162 * Type: <b>reference</b><br> 13163 * Path: <b>ExplanationOfBenefit.facility</b><br> 13164 * </p> 13165 */ 13166 @SearchParamDefinition(name="facility", path="ExplanationOfBenefit.facility", description="Facility responsible for the goods and services", type="reference", target={Location.class } ) 13167 public static final String SP_FACILITY = "facility"; 13168 /** 13169 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 13170 * <p> 13171 * Description: <b>Facility responsible for the goods and services</b><br> 13172 * Type: <b>reference</b><br> 13173 * Path: <b>ExplanationOfBenefit.facility</b><br> 13174 * </p> 13175 */ 13176 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 13177 13178/** 13179 * Constant for fluent queries to be used to add include statements. Specifies 13180 * the path value of "<b>ExplanationOfBenefit:facility</b>". 13181 */ 13182 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:facility").toLocked(); 13183 13184 13185}