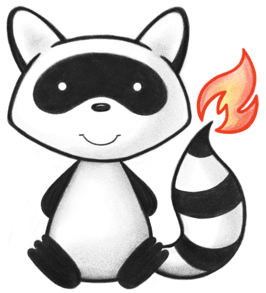
001package org.hl7.fhir.dstu3.model; 002 003import java.math.BigDecimal; 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * This resource provides: the claim details; adjudication details from the processing of a Claim; and optionally account balance information, for informing the subscriber of the benefits provided. 051 */ 052@ResourceDef(name="ExplanationOfBenefit", profile="http://hl7.org/fhir/Profile/ExplanationOfBenefit") 053public class ExplanationOfBenefit extends DomainResource { 054 055 public enum ExplanationOfBenefitStatus { 056 /** 057 * The resource instance is currently in-force. 058 */ 059 ACTIVE, 060 /** 061 * The resource instance is withdrawn, rescinded or reversed. 062 */ 063 CANCELLED, 064 /** 065 * A new resource instance the contents of which is not complete. 066 */ 067 DRAFT, 068 /** 069 * The resource instance was entered in error. 070 */ 071 ENTEREDINERROR, 072 /** 073 * added to help the parsers with the generic types 074 */ 075 NULL; 076 public static ExplanationOfBenefitStatus fromCode(String codeString) throws FHIRException { 077 if (codeString == null || "".equals(codeString)) 078 return null; 079 if ("active".equals(codeString)) 080 return ACTIVE; 081 if ("cancelled".equals(codeString)) 082 return CANCELLED; 083 if ("draft".equals(codeString)) 084 return DRAFT; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if (Configuration.isAcceptInvalidEnums()) 088 return null; 089 else 090 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 091 } 092 public String toCode() { 093 switch (this) { 094 case ACTIVE: return "active"; 095 case CANCELLED: return "cancelled"; 096 case DRAFT: return "draft"; 097 case ENTEREDINERROR: return "entered-in-error"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getSystem() { 103 switch (this) { 104 case ACTIVE: return "http://hl7.org/fhir/explanationofbenefit-status"; 105 case CANCELLED: return "http://hl7.org/fhir/explanationofbenefit-status"; 106 case DRAFT: return "http://hl7.org/fhir/explanationofbenefit-status"; 107 case ENTEREDINERROR: return "http://hl7.org/fhir/explanationofbenefit-status"; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDefinition() { 113 switch (this) { 114 case ACTIVE: return "The resource instance is currently in-force."; 115 case CANCELLED: return "The resource instance is withdrawn, rescinded or reversed."; 116 case DRAFT: return "A new resource instance the contents of which is not complete."; 117 case ENTEREDINERROR: return "The resource instance was entered in error."; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getDisplay() { 123 switch (this) { 124 case ACTIVE: return "Active"; 125 case CANCELLED: return "Cancelled"; 126 case DRAFT: return "Draft"; 127 case ENTEREDINERROR: return "Entered In Error"; 128 case NULL: return null; 129 default: return "?"; 130 } 131 } 132 } 133 134 public static class ExplanationOfBenefitStatusEnumFactory implements EnumFactory<ExplanationOfBenefitStatus> { 135 public ExplanationOfBenefitStatus fromCode(String codeString) throws IllegalArgumentException { 136 if (codeString == null || "".equals(codeString)) 137 if (codeString == null || "".equals(codeString)) 138 return null; 139 if ("active".equals(codeString)) 140 return ExplanationOfBenefitStatus.ACTIVE; 141 if ("cancelled".equals(codeString)) 142 return ExplanationOfBenefitStatus.CANCELLED; 143 if ("draft".equals(codeString)) 144 return ExplanationOfBenefitStatus.DRAFT; 145 if ("entered-in-error".equals(codeString)) 146 return ExplanationOfBenefitStatus.ENTEREDINERROR; 147 throw new IllegalArgumentException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 148 } 149 public Enumeration<ExplanationOfBenefitStatus> fromType(PrimitiveType<?> code) throws FHIRException { 150 if (code == null) 151 return null; 152 if (code.isEmpty()) 153 return new Enumeration<ExplanationOfBenefitStatus>(this); 154 String codeString = code.asStringValue(); 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("active".equals(codeString)) 158 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ACTIVE); 159 if ("cancelled".equals(codeString)) 160 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.CANCELLED); 161 if ("draft".equals(codeString)) 162 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.DRAFT); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<ExplanationOfBenefitStatus>(this, ExplanationOfBenefitStatus.ENTEREDINERROR); 165 throw new FHIRException("Unknown ExplanationOfBenefitStatus code '"+codeString+"'"); 166 } 167 public String toCode(ExplanationOfBenefitStatus code) { 168 if (code == ExplanationOfBenefitStatus.ACTIVE) 169 return "active"; 170 if (code == ExplanationOfBenefitStatus.CANCELLED) 171 return "cancelled"; 172 if (code == ExplanationOfBenefitStatus.DRAFT) 173 return "draft"; 174 if (code == ExplanationOfBenefitStatus.ENTEREDINERROR) 175 return "entered-in-error"; 176 return "?"; 177 } 178 public String toSystem(ExplanationOfBenefitStatus code) { 179 return code.getSystem(); 180 } 181 } 182 183 @Block() 184 public static class RelatedClaimComponent extends BackboneElement implements IBaseBackboneElement { 185 /** 186 * Other claims which are related to this claim such as prior claim versions or for related services. 187 */ 188 @Child(name = "claim", type = {Claim.class}, order=1, min=0, max=1, modifier=false, summary=false) 189 @Description(shortDefinition="Reference to the related claim", formalDefinition="Other claims which are related to this claim such as prior claim versions or for related services." ) 190 protected Reference claim; 191 192 /** 193 * The actual object that is the target of the reference (Other claims which are related to this claim such as prior claim versions or for related services.) 194 */ 195 protected Claim claimTarget; 196 197 /** 198 * For example prior or umbrella. 199 */ 200 @Child(name = "relationship", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 201 @Description(shortDefinition="How the reference claim is related", formalDefinition="For example prior or umbrella." ) 202 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/related-claim-relationship") 203 protected CodeableConcept relationship; 204 205 /** 206 * An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # . 207 */ 208 @Child(name = "reference", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=false) 209 @Description(shortDefinition="Related file or case reference", formalDefinition="An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # ." ) 210 protected Identifier reference; 211 212 private static final long serialVersionUID = -379338905L; 213 214 /** 215 * Constructor 216 */ 217 public RelatedClaimComponent() { 218 super(); 219 } 220 221 /** 222 * @return {@link #claim} (Other claims which are related to this claim such as prior claim versions or for related services.) 223 */ 224 public Reference getClaim() { 225 if (this.claim == null) 226 if (Configuration.errorOnAutoCreate()) 227 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 228 else if (Configuration.doAutoCreate()) 229 this.claim = new Reference(); // cc 230 return this.claim; 231 } 232 233 public boolean hasClaim() { 234 return this.claim != null && !this.claim.isEmpty(); 235 } 236 237 /** 238 * @param value {@link #claim} (Other claims which are related to this claim such as prior claim versions or for related services.) 239 */ 240 public RelatedClaimComponent setClaim(Reference value) { 241 this.claim = value; 242 return this; 243 } 244 245 /** 246 * @return {@link #claim} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Other claims which are related to this claim such as prior claim versions or for related services.) 247 */ 248 public Claim getClaimTarget() { 249 if (this.claimTarget == null) 250 if (Configuration.errorOnAutoCreate()) 251 throw new Error("Attempt to auto-create RelatedClaimComponent.claim"); 252 else if (Configuration.doAutoCreate()) 253 this.claimTarget = new Claim(); // aa 254 return this.claimTarget; 255 } 256 257 /** 258 * @param value {@link #claim} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Other claims which are related to this claim such as prior claim versions or for related services.) 259 */ 260 public RelatedClaimComponent setClaimTarget(Claim value) { 261 this.claimTarget = value; 262 return this; 263 } 264 265 /** 266 * @return {@link #relationship} (For example prior or umbrella.) 267 */ 268 public CodeableConcept getRelationship() { 269 if (this.relationship == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create RelatedClaimComponent.relationship"); 272 else if (Configuration.doAutoCreate()) 273 this.relationship = new CodeableConcept(); // cc 274 return this.relationship; 275 } 276 277 public boolean hasRelationship() { 278 return this.relationship != null && !this.relationship.isEmpty(); 279 } 280 281 /** 282 * @param value {@link #relationship} (For example prior or umbrella.) 283 */ 284 public RelatedClaimComponent setRelationship(CodeableConcept value) { 285 this.relationship = value; 286 return this; 287 } 288 289 /** 290 * @return {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .) 291 */ 292 public Identifier getReference() { 293 if (this.reference == null) 294 if (Configuration.errorOnAutoCreate()) 295 throw new Error("Attempt to auto-create RelatedClaimComponent.reference"); 296 else if (Configuration.doAutoCreate()) 297 this.reference = new Identifier(); // cc 298 return this.reference; 299 } 300 301 public boolean hasReference() { 302 return this.reference != null && !this.reference.isEmpty(); 303 } 304 305 /** 306 * @param value {@link #reference} (An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .) 307 */ 308 public RelatedClaimComponent setReference(Identifier value) { 309 this.reference = value; 310 return this; 311 } 312 313 protected void listChildren(List<Property> children) { 314 super.listChildren(children); 315 children.add(new Property("claim", "Reference(Claim)", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, 1, claim)); 316 children.add(new Property("relationship", "CodeableConcept", "For example prior or umbrella.", 0, 1, relationship)); 317 children.add(new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .", 0, 1, reference)); 318 } 319 320 @Override 321 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 322 switch (_hash) { 323 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, 1, claim); 324 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "For example prior or umbrella.", 0, 1, relationship); 325 case -925155509: /*reference*/ return new Property("reference", "Identifier", "An alternate organizational reference to the case or file to which this particular claim pertains - eg Property/Casualy insurer claim # or Workers Compensation case # .", 0, 1, reference); 326 default: return super.getNamedProperty(_hash, _name, _checkValid); 327 } 328 329 } 330 331 @Override 332 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 333 switch (hash) { 334 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 335 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 336 case -925155509: /*reference*/ return this.reference == null ? new Base[0] : new Base[] {this.reference}; // Identifier 337 default: return super.getProperty(hash, name, checkValid); 338 } 339 340 } 341 342 @Override 343 public Base setProperty(int hash, String name, Base value) throws FHIRException { 344 switch (hash) { 345 case 94742588: // claim 346 this.claim = castToReference(value); // Reference 347 return value; 348 case -261851592: // relationship 349 this.relationship = castToCodeableConcept(value); // CodeableConcept 350 return value; 351 case -925155509: // reference 352 this.reference = castToIdentifier(value); // Identifier 353 return value; 354 default: return super.setProperty(hash, name, value); 355 } 356 357 } 358 359 @Override 360 public Base setProperty(String name, Base value) throws FHIRException { 361 if (name.equals("claim")) { 362 this.claim = castToReference(value); // Reference 363 } else if (name.equals("relationship")) { 364 this.relationship = castToCodeableConcept(value); // CodeableConcept 365 } else if (name.equals("reference")) { 366 this.reference = castToIdentifier(value); // Identifier 367 } else 368 return super.setProperty(name, value); 369 return value; 370 } 371 372 @Override 373 public Base makeProperty(int hash, String name) throws FHIRException { 374 switch (hash) { 375 case 94742588: return getClaim(); 376 case -261851592: return getRelationship(); 377 case -925155509: return getReference(); 378 default: return super.makeProperty(hash, name); 379 } 380 381 } 382 383 @Override 384 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 385 switch (hash) { 386 case 94742588: /*claim*/ return new String[] {"Reference"}; 387 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 388 case -925155509: /*reference*/ return new String[] {"Identifier"}; 389 default: return super.getTypesForProperty(hash, name); 390 } 391 392 } 393 394 @Override 395 public Base addChild(String name) throws FHIRException { 396 if (name.equals("claim")) { 397 this.claim = new Reference(); 398 return this.claim; 399 } 400 else if (name.equals("relationship")) { 401 this.relationship = new CodeableConcept(); 402 return this.relationship; 403 } 404 else if (name.equals("reference")) { 405 this.reference = new Identifier(); 406 return this.reference; 407 } 408 else 409 return super.addChild(name); 410 } 411 412 public RelatedClaimComponent copy() { 413 RelatedClaimComponent dst = new RelatedClaimComponent(); 414 copyValues(dst); 415 dst.claim = claim == null ? null : claim.copy(); 416 dst.relationship = relationship == null ? null : relationship.copy(); 417 dst.reference = reference == null ? null : reference.copy(); 418 return dst; 419 } 420 421 @Override 422 public boolean equalsDeep(Base other_) { 423 if (!super.equalsDeep(other_)) 424 return false; 425 if (!(other_ instanceof RelatedClaimComponent)) 426 return false; 427 RelatedClaimComponent o = (RelatedClaimComponent) other_; 428 return compareDeep(claim, o.claim, true) && compareDeep(relationship, o.relationship, true) && compareDeep(reference, o.reference, true) 429 ; 430 } 431 432 @Override 433 public boolean equalsShallow(Base other_) { 434 if (!super.equalsShallow(other_)) 435 return false; 436 if (!(other_ instanceof RelatedClaimComponent)) 437 return false; 438 RelatedClaimComponent o = (RelatedClaimComponent) other_; 439 return true; 440 } 441 442 public boolean isEmpty() { 443 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(claim, relationship, reference 444 ); 445 } 446 447 public String fhirType() { 448 return "ExplanationOfBenefit.related"; 449 450 } 451 452 } 453 454 @Block() 455 public static class PayeeComponent extends BackboneElement implements IBaseBackboneElement { 456 /** 457 * Type of Party to be reimbursed: Subscriber, provider, other. 458 */ 459 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 460 @Description(shortDefinition="Type of party: Subscriber, Provider, other", formalDefinition="Type of Party to be reimbursed: Subscriber, provider, other." ) 461 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payeetype") 462 protected CodeableConcept type; 463 464 /** 465 * organization | patient | practitioner | relatedperson. 466 */ 467 @Child(name = "resourceType", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 468 @Description(shortDefinition="organization | patient | practitioner | relatedperson", formalDefinition="organization | patient | practitioner | relatedperson." ) 469 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-type-link") 470 protected CodeableConcept resourceType; 471 472 /** 473 * Party to be reimbursed: Subscriber, provider, other. 474 */ 475 @Child(name = "party", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=false) 476 @Description(shortDefinition="Party to receive the payable", formalDefinition="Party to be reimbursed: Subscriber, provider, other." ) 477 protected Reference party; 478 479 /** 480 * The actual object that is the target of the reference (Party to be reimbursed: Subscriber, provider, other.) 481 */ 482 protected Resource partyTarget; 483 484 private static final long serialVersionUID = 1146157718L; 485 486 /** 487 * Constructor 488 */ 489 public PayeeComponent() { 490 super(); 491 } 492 493 /** 494 * @return {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 495 */ 496 public CodeableConcept getType() { 497 if (this.type == null) 498 if (Configuration.errorOnAutoCreate()) 499 throw new Error("Attempt to auto-create PayeeComponent.type"); 500 else if (Configuration.doAutoCreate()) 501 this.type = new CodeableConcept(); // cc 502 return this.type; 503 } 504 505 public boolean hasType() { 506 return this.type != null && !this.type.isEmpty(); 507 } 508 509 /** 510 * @param value {@link #type} (Type of Party to be reimbursed: Subscriber, provider, other.) 511 */ 512 public PayeeComponent setType(CodeableConcept value) { 513 this.type = value; 514 return this; 515 } 516 517 /** 518 * @return {@link #resourceType} (organization | patient | practitioner | relatedperson.) 519 */ 520 public CodeableConcept getResourceType() { 521 if (this.resourceType == null) 522 if (Configuration.errorOnAutoCreate()) 523 throw new Error("Attempt to auto-create PayeeComponent.resourceType"); 524 else if (Configuration.doAutoCreate()) 525 this.resourceType = new CodeableConcept(); // cc 526 return this.resourceType; 527 } 528 529 public boolean hasResourceType() { 530 return this.resourceType != null && !this.resourceType.isEmpty(); 531 } 532 533 /** 534 * @param value {@link #resourceType} (organization | patient | practitioner | relatedperson.) 535 */ 536 public PayeeComponent setResourceType(CodeableConcept value) { 537 this.resourceType = value; 538 return this; 539 } 540 541 /** 542 * @return {@link #party} (Party to be reimbursed: Subscriber, provider, other.) 543 */ 544 public Reference getParty() { 545 if (this.party == null) 546 if (Configuration.errorOnAutoCreate()) 547 throw new Error("Attempt to auto-create PayeeComponent.party"); 548 else if (Configuration.doAutoCreate()) 549 this.party = new Reference(); // cc 550 return this.party; 551 } 552 553 public boolean hasParty() { 554 return this.party != null && !this.party.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #party} (Party to be reimbursed: Subscriber, provider, other.) 559 */ 560 public PayeeComponent setParty(Reference value) { 561 this.party = value; 562 return this; 563 } 564 565 /** 566 * @return {@link #party} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Party to be reimbursed: Subscriber, provider, other.) 567 */ 568 public Resource getPartyTarget() { 569 return this.partyTarget; 570 } 571 572 /** 573 * @param value {@link #party} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Party to be reimbursed: Subscriber, provider, other.) 574 */ 575 public PayeeComponent setPartyTarget(Resource value) { 576 this.partyTarget = value; 577 return this; 578 } 579 580 protected void listChildren(List<Property> children) { 581 super.listChildren(children); 582 children.add(new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type)); 583 children.add(new Property("resourceType", "CodeableConcept", "organization | patient | practitioner | relatedperson.", 0, 1, resourceType)); 584 children.add(new Property("party", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, party)); 585 } 586 587 @Override 588 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 589 switch (_hash) { 590 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of Party to be reimbursed: Subscriber, provider, other.", 0, 1, type); 591 case -384364440: /*resourceType*/ return new Property("resourceType", "CodeableConcept", "organization | patient | practitioner | relatedperson.", 0, 1, resourceType); 592 case 106437350: /*party*/ return new Property("party", "Reference(Practitioner|Organization|Patient|RelatedPerson)", "Party to be reimbursed: Subscriber, provider, other.", 0, 1, party); 593 default: return super.getNamedProperty(_hash, _name, _checkValid); 594 } 595 596 } 597 598 @Override 599 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 600 switch (hash) { 601 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 602 case -384364440: /*resourceType*/ return this.resourceType == null ? new Base[0] : new Base[] {this.resourceType}; // CodeableConcept 603 case 106437350: /*party*/ return this.party == null ? new Base[0] : new Base[] {this.party}; // Reference 604 default: return super.getProperty(hash, name, checkValid); 605 } 606 607 } 608 609 @Override 610 public Base setProperty(int hash, String name, Base value) throws FHIRException { 611 switch (hash) { 612 case 3575610: // type 613 this.type = castToCodeableConcept(value); // CodeableConcept 614 return value; 615 case -384364440: // resourceType 616 this.resourceType = castToCodeableConcept(value); // CodeableConcept 617 return value; 618 case 106437350: // party 619 this.party = castToReference(value); // Reference 620 return value; 621 default: return super.setProperty(hash, name, value); 622 } 623 624 } 625 626 @Override 627 public Base setProperty(String name, Base value) throws FHIRException { 628 if (name.equals("type")) { 629 this.type = castToCodeableConcept(value); // CodeableConcept 630 } else if (name.equals("resourceType")) { 631 this.resourceType = castToCodeableConcept(value); // CodeableConcept 632 } else if (name.equals("party")) { 633 this.party = castToReference(value); // Reference 634 } else 635 return super.setProperty(name, value); 636 return value; 637 } 638 639 @Override 640 public Base makeProperty(int hash, String name) throws FHIRException { 641 switch (hash) { 642 case 3575610: return getType(); 643 case -384364440: return getResourceType(); 644 case 106437350: return getParty(); 645 default: return super.makeProperty(hash, name); 646 } 647 648 } 649 650 @Override 651 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 652 switch (hash) { 653 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 654 case -384364440: /*resourceType*/ return new String[] {"CodeableConcept"}; 655 case 106437350: /*party*/ return new String[] {"Reference"}; 656 default: return super.getTypesForProperty(hash, name); 657 } 658 659 } 660 661 @Override 662 public Base addChild(String name) throws FHIRException { 663 if (name.equals("type")) { 664 this.type = new CodeableConcept(); 665 return this.type; 666 } 667 else if (name.equals("resourceType")) { 668 this.resourceType = new CodeableConcept(); 669 return this.resourceType; 670 } 671 else if (name.equals("party")) { 672 this.party = new Reference(); 673 return this.party; 674 } 675 else 676 return super.addChild(name); 677 } 678 679 public PayeeComponent copy() { 680 PayeeComponent dst = new PayeeComponent(); 681 copyValues(dst); 682 dst.type = type == null ? null : type.copy(); 683 dst.resourceType = resourceType == null ? null : resourceType.copy(); 684 dst.party = party == null ? null : party.copy(); 685 return dst; 686 } 687 688 @Override 689 public boolean equalsDeep(Base other_) { 690 if (!super.equalsDeep(other_)) 691 return false; 692 if (!(other_ instanceof PayeeComponent)) 693 return false; 694 PayeeComponent o = (PayeeComponent) other_; 695 return compareDeep(type, o.type, true) && compareDeep(resourceType, o.resourceType, true) && compareDeep(party, o.party, true) 696 ; 697 } 698 699 @Override 700 public boolean equalsShallow(Base other_) { 701 if (!super.equalsShallow(other_)) 702 return false; 703 if (!(other_ instanceof PayeeComponent)) 704 return false; 705 PayeeComponent o = (PayeeComponent) other_; 706 return true; 707 } 708 709 public boolean isEmpty() { 710 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resourceType, party 711 ); 712 } 713 714 public String fhirType() { 715 return "ExplanationOfBenefit.payee"; 716 717 } 718 719 } 720 721 @Block() 722 public static class SupportingInformationComponent extends BackboneElement implements IBaseBackboneElement { 723 /** 724 * Sequence of the information element which serves to provide a link. 725 */ 726 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 727 @Description(shortDefinition="Information instance identifier", formalDefinition="Sequence of the information element which serves to provide a link." ) 728 protected PositiveIntType sequence; 729 730 /** 731 * The general class of the information supplied: information; exception; accident, employment; onset, etc. 732 */ 733 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 734 @Description(shortDefinition="General class of information", formalDefinition="The general class of the information supplied: information; exception; accident, employment; onset, etc." ) 735 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-informationcategory") 736 protected CodeableConcept category; 737 738 /** 739 * System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication. 740 */ 741 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 742 @Description(shortDefinition="Type of information", formalDefinition="System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication." ) 743 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-exception") 744 protected CodeableConcept code; 745 746 /** 747 * The date when or period to which this information refers. 748 */ 749 @Child(name = "timing", type = {DateType.class, Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 750 @Description(shortDefinition="When it occurred", formalDefinition="The date when or period to which this information refers." ) 751 protected Type timing; 752 753 /** 754 * Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data. 755 */ 756 @Child(name = "value", type = {StringType.class, Quantity.class, Attachment.class, Reference.class}, order=5, min=0, max=1, modifier=false, summary=false) 757 @Description(shortDefinition="Additional Data or supporting information", formalDefinition="Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data." ) 758 protected Type value; 759 760 /** 761 * For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content. 762 */ 763 @Child(name = "reason", type = {Coding.class}, order=6, min=0, max=1, modifier=false, summary=false) 764 @Description(shortDefinition="Reason associated with the information", formalDefinition="For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content." ) 765 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/missing-tooth-reason") 766 protected Coding reason; 767 768 private static final long serialVersionUID = -410136661L; 769 770 /** 771 * Constructor 772 */ 773 public SupportingInformationComponent() { 774 super(); 775 } 776 777 /** 778 * Constructor 779 */ 780 public SupportingInformationComponent(PositiveIntType sequence, CodeableConcept category) { 781 super(); 782 this.sequence = sequence; 783 this.category = category; 784 } 785 786 /** 787 * @return {@link #sequence} (Sequence of the information element which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 788 */ 789 public PositiveIntType getSequenceElement() { 790 if (this.sequence == null) 791 if (Configuration.errorOnAutoCreate()) 792 throw new Error("Attempt to auto-create SupportingInformationComponent.sequence"); 793 else if (Configuration.doAutoCreate()) 794 this.sequence = new PositiveIntType(); // bb 795 return this.sequence; 796 } 797 798 public boolean hasSequenceElement() { 799 return this.sequence != null && !this.sequence.isEmpty(); 800 } 801 802 public boolean hasSequence() { 803 return this.sequence != null && !this.sequence.isEmpty(); 804 } 805 806 /** 807 * @param value {@link #sequence} (Sequence of the information element which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 808 */ 809 public SupportingInformationComponent setSequenceElement(PositiveIntType value) { 810 this.sequence = value; 811 return this; 812 } 813 814 /** 815 * @return Sequence of the information element which serves to provide a link. 816 */ 817 public int getSequence() { 818 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 819 } 820 821 /** 822 * @param value Sequence of the information element which serves to provide a link. 823 */ 824 public SupportingInformationComponent setSequence(int value) { 825 if (this.sequence == null) 826 this.sequence = new PositiveIntType(); 827 this.sequence.setValue(value); 828 return this; 829 } 830 831 /** 832 * @return {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 833 */ 834 public CodeableConcept getCategory() { 835 if (this.category == null) 836 if (Configuration.errorOnAutoCreate()) 837 throw new Error("Attempt to auto-create SupportingInformationComponent.category"); 838 else if (Configuration.doAutoCreate()) 839 this.category = new CodeableConcept(); // cc 840 return this.category; 841 } 842 843 public boolean hasCategory() { 844 return this.category != null && !this.category.isEmpty(); 845 } 846 847 /** 848 * @param value {@link #category} (The general class of the information supplied: information; exception; accident, employment; onset, etc.) 849 */ 850 public SupportingInformationComponent setCategory(CodeableConcept value) { 851 this.category = value; 852 return this; 853 } 854 855 /** 856 * @return {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.) 857 */ 858 public CodeableConcept getCode() { 859 if (this.code == null) 860 if (Configuration.errorOnAutoCreate()) 861 throw new Error("Attempt to auto-create SupportingInformationComponent.code"); 862 else if (Configuration.doAutoCreate()) 863 this.code = new CodeableConcept(); // cc 864 return this.code; 865 } 866 867 public boolean hasCode() { 868 return this.code != null && !this.code.isEmpty(); 869 } 870 871 /** 872 * @param value {@link #code} (System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.) 873 */ 874 public SupportingInformationComponent setCode(CodeableConcept value) { 875 this.code = value; 876 return this; 877 } 878 879 /** 880 * @return {@link #timing} (The date when or period to which this information refers.) 881 */ 882 public Type getTiming() { 883 return this.timing; 884 } 885 886 /** 887 * @return {@link #timing} (The date when or period to which this information refers.) 888 */ 889 public DateType getTimingDateType() throws FHIRException { 890 if (this.timing == null) 891 return null; 892 if (!(this.timing instanceof DateType)) 893 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.timing.getClass().getName()+" was encountered"); 894 return (DateType) this.timing; 895 } 896 897 public boolean hasTimingDateType() { 898 return this != null && this.timing instanceof DateType; 899 } 900 901 /** 902 * @return {@link #timing} (The date when or period to which this information refers.) 903 */ 904 public Period getTimingPeriod() throws FHIRException { 905 if (this.timing == null) 906 return null; 907 if (!(this.timing instanceof Period)) 908 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.timing.getClass().getName()+" was encountered"); 909 return (Period) this.timing; 910 } 911 912 public boolean hasTimingPeriod() { 913 return this != null && this.timing instanceof Period; 914 } 915 916 public boolean hasTiming() { 917 return this.timing != null && !this.timing.isEmpty(); 918 } 919 920 /** 921 * @param value {@link #timing} (The date when or period to which this information refers.) 922 */ 923 public SupportingInformationComponent setTiming(Type value) throws FHIRFormatError { 924 if (value != null && !(value instanceof DateType || value instanceof Period)) 925 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.information.timing[x]: "+value.fhirType()); 926 this.timing = value; 927 return this; 928 } 929 930 /** 931 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 932 */ 933 public Type getValue() { 934 return this.value; 935 } 936 937 /** 938 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 939 */ 940 public StringType getValueStringType() throws FHIRException { 941 if (this.value == null) 942 return null; 943 if (!(this.value instanceof StringType)) 944 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.value.getClass().getName()+" was encountered"); 945 return (StringType) this.value; 946 } 947 948 public boolean hasValueStringType() { 949 return this != null && this.value instanceof StringType; 950 } 951 952 /** 953 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 954 */ 955 public Quantity getValueQuantity() throws FHIRException { 956 if (this.value == null) 957 return null; 958 if (!(this.value instanceof Quantity)) 959 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 960 return (Quantity) this.value; 961 } 962 963 public boolean hasValueQuantity() { 964 return this != null && this.value instanceof Quantity; 965 } 966 967 /** 968 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 969 */ 970 public Attachment getValueAttachment() throws FHIRException { 971 if (this.value == null) 972 return null; 973 if (!(this.value instanceof Attachment)) 974 throw new FHIRException("Type mismatch: the type Attachment was expected, but "+this.value.getClass().getName()+" was encountered"); 975 return (Attachment) this.value; 976 } 977 978 public boolean hasValueAttachment() { 979 return this != null && this.value instanceof Attachment; 980 } 981 982 /** 983 * @return {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 984 */ 985 public Reference getValueReference() throws FHIRException { 986 if (this.value == null) 987 return null; 988 if (!(this.value instanceof Reference)) 989 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.value.getClass().getName()+" was encountered"); 990 return (Reference) this.value; 991 } 992 993 public boolean hasValueReference() { 994 return this != null && this.value instanceof Reference; 995 } 996 997 public boolean hasValue() { 998 return this.value != null && !this.value.isEmpty(); 999 } 1000 1001 /** 1002 * @param value {@link #value} (Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.) 1003 */ 1004 public SupportingInformationComponent setValue(Type value) throws FHIRFormatError { 1005 if (value != null && !(value instanceof StringType || value instanceof Quantity || value instanceof Attachment || value instanceof Reference)) 1006 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.information.value[x]: "+value.fhirType()); 1007 this.value = value; 1008 return this; 1009 } 1010 1011 /** 1012 * @return {@link #reason} (For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.) 1013 */ 1014 public Coding getReason() { 1015 if (this.reason == null) 1016 if (Configuration.errorOnAutoCreate()) 1017 throw new Error("Attempt to auto-create SupportingInformationComponent.reason"); 1018 else if (Configuration.doAutoCreate()) 1019 this.reason = new Coding(); // cc 1020 return this.reason; 1021 } 1022 1023 public boolean hasReason() { 1024 return this.reason != null && !this.reason.isEmpty(); 1025 } 1026 1027 /** 1028 * @param value {@link #reason} (For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.) 1029 */ 1030 public SupportingInformationComponent setReason(Coding value) { 1031 this.reason = value; 1032 return this; 1033 } 1034 1035 protected void listChildren(List<Property> children) { 1036 super.listChildren(children); 1037 children.add(new Property("sequence", "positiveInt", "Sequence of the information element which serves to provide a link.", 0, 1, sequence)); 1038 children.add(new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category)); 1039 children.add(new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.", 0, 1, code)); 1040 children.add(new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing)); 1041 children.add(new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value)); 1042 children.add(new Property("reason", "Coding", "For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.", 0, 1, reason)); 1043 } 1044 1045 @Override 1046 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1047 switch (_hash) { 1048 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of the information element which serves to provide a link.", 0, 1, sequence); 1049 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "The general class of the information supplied: information; exception; accident, employment; onset, etc.", 0, 1, category); 1050 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "System and code pertaining to the specific information regarding special conditions relating to the setting, treatment or patient for which care is sought which may influence the adjudication.", 0, 1, code); 1051 case 164632566: /*timing[x]*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1052 case -873664438: /*timing*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1053 case 807935768: /*timingDate*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1054 case -615615829: /*timingPeriod*/ return new Property("timing[x]", "date|Period", "The date when or period to which this information refers.", 0, 1, timing); 1055 case -1410166417: /*value[x]*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1056 case 111972721: /*value*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1057 case -1424603934: /*valueString*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1058 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1059 case -475566732: /*valueAttachment*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1060 case 1755241690: /*valueReference*/ return new Property("value[x]", "string|Quantity|Attachment|Reference(Any)", "Additional data or information such as resources, documents, images etc. including references to the data or the actual inclusion of the data.", 0, 1, value); 1061 case -934964668: /*reason*/ return new Property("reason", "Coding", "For example, provides the reason for: the additional stay, or missing tooth or any other situation where a reason code is required in addition to the content.", 0, 1, reason); 1062 default: return super.getNamedProperty(_hash, _name, _checkValid); 1063 } 1064 1065 } 1066 1067 @Override 1068 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1069 switch (hash) { 1070 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1071 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1072 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1073 case -873664438: /*timing*/ return this.timing == null ? new Base[0] : new Base[] {this.timing}; // Type 1074 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 1075 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Coding 1076 default: return super.getProperty(hash, name, checkValid); 1077 } 1078 1079 } 1080 1081 @Override 1082 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1083 switch (hash) { 1084 case 1349547969: // sequence 1085 this.sequence = castToPositiveInt(value); // PositiveIntType 1086 return value; 1087 case 50511102: // category 1088 this.category = castToCodeableConcept(value); // CodeableConcept 1089 return value; 1090 case 3059181: // code 1091 this.code = castToCodeableConcept(value); // CodeableConcept 1092 return value; 1093 case -873664438: // timing 1094 this.timing = castToType(value); // Type 1095 return value; 1096 case 111972721: // value 1097 this.value = castToType(value); // Type 1098 return value; 1099 case -934964668: // reason 1100 this.reason = castToCoding(value); // Coding 1101 return value; 1102 default: return super.setProperty(hash, name, value); 1103 } 1104 1105 } 1106 1107 @Override 1108 public Base setProperty(String name, Base value) throws FHIRException { 1109 if (name.equals("sequence")) { 1110 this.sequence = castToPositiveInt(value); // PositiveIntType 1111 } else if (name.equals("category")) { 1112 this.category = castToCodeableConcept(value); // CodeableConcept 1113 } else if (name.equals("code")) { 1114 this.code = castToCodeableConcept(value); // CodeableConcept 1115 } else if (name.equals("timing[x]")) { 1116 this.timing = castToType(value); // Type 1117 } else if (name.equals("value[x]")) { 1118 this.value = castToType(value); // Type 1119 } else if (name.equals("reason")) { 1120 this.reason = castToCoding(value); // Coding 1121 } else 1122 return super.setProperty(name, value); 1123 return value; 1124 } 1125 1126 @Override 1127 public Base makeProperty(int hash, String name) throws FHIRException { 1128 switch (hash) { 1129 case 1349547969: return getSequenceElement(); 1130 case 50511102: return getCategory(); 1131 case 3059181: return getCode(); 1132 case 164632566: return getTiming(); 1133 case -873664438: return getTiming(); 1134 case -1410166417: return getValue(); 1135 case 111972721: return getValue(); 1136 case -934964668: return getReason(); 1137 default: return super.makeProperty(hash, name); 1138 } 1139 1140 } 1141 1142 @Override 1143 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1144 switch (hash) { 1145 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1146 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1147 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1148 case -873664438: /*timing*/ return new String[] {"date", "Period"}; 1149 case 111972721: /*value*/ return new String[] {"string", "Quantity", "Attachment", "Reference"}; 1150 case -934964668: /*reason*/ return new String[] {"Coding"}; 1151 default: return super.getTypesForProperty(hash, name); 1152 } 1153 1154 } 1155 1156 @Override 1157 public Base addChild(String name) throws FHIRException { 1158 if (name.equals("sequence")) { 1159 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 1160 } 1161 else if (name.equals("category")) { 1162 this.category = new CodeableConcept(); 1163 return this.category; 1164 } 1165 else if (name.equals("code")) { 1166 this.code = new CodeableConcept(); 1167 return this.code; 1168 } 1169 else if (name.equals("timingDate")) { 1170 this.timing = new DateType(); 1171 return this.timing; 1172 } 1173 else if (name.equals("timingPeriod")) { 1174 this.timing = new Period(); 1175 return this.timing; 1176 } 1177 else if (name.equals("valueString")) { 1178 this.value = new StringType(); 1179 return this.value; 1180 } 1181 else if (name.equals("valueQuantity")) { 1182 this.value = new Quantity(); 1183 return this.value; 1184 } 1185 else if (name.equals("valueAttachment")) { 1186 this.value = new Attachment(); 1187 return this.value; 1188 } 1189 else if (name.equals("valueReference")) { 1190 this.value = new Reference(); 1191 return this.value; 1192 } 1193 else if (name.equals("reason")) { 1194 this.reason = new Coding(); 1195 return this.reason; 1196 } 1197 else 1198 return super.addChild(name); 1199 } 1200 1201 public SupportingInformationComponent copy() { 1202 SupportingInformationComponent dst = new SupportingInformationComponent(); 1203 copyValues(dst); 1204 dst.sequence = sequence == null ? null : sequence.copy(); 1205 dst.category = category == null ? null : category.copy(); 1206 dst.code = code == null ? null : code.copy(); 1207 dst.timing = timing == null ? null : timing.copy(); 1208 dst.value = value == null ? null : value.copy(); 1209 dst.reason = reason == null ? null : reason.copy(); 1210 return dst; 1211 } 1212 1213 @Override 1214 public boolean equalsDeep(Base other_) { 1215 if (!super.equalsDeep(other_)) 1216 return false; 1217 if (!(other_ instanceof SupportingInformationComponent)) 1218 return false; 1219 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1220 return compareDeep(sequence, o.sequence, true) && compareDeep(category, o.category, true) && compareDeep(code, o.code, true) 1221 && compareDeep(timing, o.timing, true) && compareDeep(value, o.value, true) && compareDeep(reason, o.reason, true) 1222 ; 1223 } 1224 1225 @Override 1226 public boolean equalsShallow(Base other_) { 1227 if (!super.equalsShallow(other_)) 1228 return false; 1229 if (!(other_ instanceof SupportingInformationComponent)) 1230 return false; 1231 SupportingInformationComponent o = (SupportingInformationComponent) other_; 1232 return compareValues(sequence, o.sequence, true); 1233 } 1234 1235 public boolean isEmpty() { 1236 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, category, code 1237 , timing, value, reason); 1238 } 1239 1240 public String fhirType() { 1241 return "ExplanationOfBenefit.information"; 1242 1243 } 1244 1245 } 1246 1247 @Block() 1248 public static class CareTeamComponent extends BackboneElement implements IBaseBackboneElement { 1249 /** 1250 * Sequence of careteam which serves to order and provide a link. 1251 */ 1252 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1253 @Description(shortDefinition="Number to covey order of careteam", formalDefinition="Sequence of careteam which serves to order and provide a link." ) 1254 protected PositiveIntType sequence; 1255 1256 /** 1257 * The members of the team who provided the overall service. 1258 */ 1259 @Child(name = "provider", type = {Practitioner.class, Organization.class}, order=2, min=1, max=1, modifier=false, summary=false) 1260 @Description(shortDefinition="Member of the Care Team", formalDefinition="The members of the team who provided the overall service." ) 1261 protected Reference provider; 1262 1263 /** 1264 * The actual object that is the target of the reference (The members of the team who provided the overall service.) 1265 */ 1266 protected Resource providerTarget; 1267 1268 /** 1269 * The practitioner who is billing and responsible for the claimed services rendered to the patient. 1270 */ 1271 @Child(name = "responsible", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1272 @Description(shortDefinition="Billing practitioner", formalDefinition="The practitioner who is billing and responsible for the claimed services rendered to the patient." ) 1273 protected BooleanType responsible; 1274 1275 /** 1276 * The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team. 1277 */ 1278 @Child(name = "role", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1279 @Description(shortDefinition="Role on the team", formalDefinition="The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team." ) 1280 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-careteamrole") 1281 protected CodeableConcept role; 1282 1283 /** 1284 * The qualification which is applicable for this service. 1285 */ 1286 @Child(name = "qualification", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1287 @Description(shortDefinition="Type, classification or Specialization", formalDefinition="The qualification which is applicable for this service." ) 1288 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/provider-qualification") 1289 protected CodeableConcept qualification; 1290 1291 private static final long serialVersionUID = 1758966968L; 1292 1293 /** 1294 * Constructor 1295 */ 1296 public CareTeamComponent() { 1297 super(); 1298 } 1299 1300 /** 1301 * Constructor 1302 */ 1303 public CareTeamComponent(PositiveIntType sequence, Reference provider) { 1304 super(); 1305 this.sequence = sequence; 1306 this.provider = provider; 1307 } 1308 1309 /** 1310 * @return {@link #sequence} (Sequence of careteam which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1311 */ 1312 public PositiveIntType getSequenceElement() { 1313 if (this.sequence == null) 1314 if (Configuration.errorOnAutoCreate()) 1315 throw new Error("Attempt to auto-create CareTeamComponent.sequence"); 1316 else if (Configuration.doAutoCreate()) 1317 this.sequence = new PositiveIntType(); // bb 1318 return this.sequence; 1319 } 1320 1321 public boolean hasSequenceElement() { 1322 return this.sequence != null && !this.sequence.isEmpty(); 1323 } 1324 1325 public boolean hasSequence() { 1326 return this.sequence != null && !this.sequence.isEmpty(); 1327 } 1328 1329 /** 1330 * @param value {@link #sequence} (Sequence of careteam which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1331 */ 1332 public CareTeamComponent setSequenceElement(PositiveIntType value) { 1333 this.sequence = value; 1334 return this; 1335 } 1336 1337 /** 1338 * @return Sequence of careteam which serves to order and provide a link. 1339 */ 1340 public int getSequence() { 1341 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1342 } 1343 1344 /** 1345 * @param value Sequence of careteam which serves to order and provide a link. 1346 */ 1347 public CareTeamComponent setSequence(int value) { 1348 if (this.sequence == null) 1349 this.sequence = new PositiveIntType(); 1350 this.sequence.setValue(value); 1351 return this; 1352 } 1353 1354 /** 1355 * @return {@link #provider} (The members of the team who provided the overall service.) 1356 */ 1357 public Reference getProvider() { 1358 if (this.provider == null) 1359 if (Configuration.errorOnAutoCreate()) 1360 throw new Error("Attempt to auto-create CareTeamComponent.provider"); 1361 else if (Configuration.doAutoCreate()) 1362 this.provider = new Reference(); // cc 1363 return this.provider; 1364 } 1365 1366 public boolean hasProvider() { 1367 return this.provider != null && !this.provider.isEmpty(); 1368 } 1369 1370 /** 1371 * @param value {@link #provider} (The members of the team who provided the overall service.) 1372 */ 1373 public CareTeamComponent setProvider(Reference value) { 1374 this.provider = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The members of the team who provided the overall service.) 1380 */ 1381 public Resource getProviderTarget() { 1382 return this.providerTarget; 1383 } 1384 1385 /** 1386 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The members of the team who provided the overall service.) 1387 */ 1388 public CareTeamComponent setProviderTarget(Resource value) { 1389 this.providerTarget = value; 1390 return this; 1391 } 1392 1393 /** 1394 * @return {@link #responsible} (The practitioner who is billing and responsible for the claimed services rendered to the patient.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1395 */ 1396 public BooleanType getResponsibleElement() { 1397 if (this.responsible == null) 1398 if (Configuration.errorOnAutoCreate()) 1399 throw new Error("Attempt to auto-create CareTeamComponent.responsible"); 1400 else if (Configuration.doAutoCreate()) 1401 this.responsible = new BooleanType(); // bb 1402 return this.responsible; 1403 } 1404 1405 public boolean hasResponsibleElement() { 1406 return this.responsible != null && !this.responsible.isEmpty(); 1407 } 1408 1409 public boolean hasResponsible() { 1410 return this.responsible != null && !this.responsible.isEmpty(); 1411 } 1412 1413 /** 1414 * @param value {@link #responsible} (The practitioner who is billing and responsible for the claimed services rendered to the patient.). This is the underlying object with id, value and extensions. The accessor "getResponsible" gives direct access to the value 1415 */ 1416 public CareTeamComponent setResponsibleElement(BooleanType value) { 1417 this.responsible = value; 1418 return this; 1419 } 1420 1421 /** 1422 * @return The practitioner who is billing and responsible for the claimed services rendered to the patient. 1423 */ 1424 public boolean getResponsible() { 1425 return this.responsible == null || this.responsible.isEmpty() ? false : this.responsible.getValue(); 1426 } 1427 1428 /** 1429 * @param value The practitioner who is billing and responsible for the claimed services rendered to the patient. 1430 */ 1431 public CareTeamComponent setResponsible(boolean value) { 1432 if (this.responsible == null) 1433 this.responsible = new BooleanType(); 1434 this.responsible.setValue(value); 1435 return this; 1436 } 1437 1438 /** 1439 * @return {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.) 1440 */ 1441 public CodeableConcept getRole() { 1442 if (this.role == null) 1443 if (Configuration.errorOnAutoCreate()) 1444 throw new Error("Attempt to auto-create CareTeamComponent.role"); 1445 else if (Configuration.doAutoCreate()) 1446 this.role = new CodeableConcept(); // cc 1447 return this.role; 1448 } 1449 1450 public boolean hasRole() { 1451 return this.role != null && !this.role.isEmpty(); 1452 } 1453 1454 /** 1455 * @param value {@link #role} (The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.) 1456 */ 1457 public CareTeamComponent setRole(CodeableConcept value) { 1458 this.role = value; 1459 return this; 1460 } 1461 1462 /** 1463 * @return {@link #qualification} (The qualification which is applicable for this service.) 1464 */ 1465 public CodeableConcept getQualification() { 1466 if (this.qualification == null) 1467 if (Configuration.errorOnAutoCreate()) 1468 throw new Error("Attempt to auto-create CareTeamComponent.qualification"); 1469 else if (Configuration.doAutoCreate()) 1470 this.qualification = new CodeableConcept(); // cc 1471 return this.qualification; 1472 } 1473 1474 public boolean hasQualification() { 1475 return this.qualification != null && !this.qualification.isEmpty(); 1476 } 1477 1478 /** 1479 * @param value {@link #qualification} (The qualification which is applicable for this service.) 1480 */ 1481 public CareTeamComponent setQualification(CodeableConcept value) { 1482 this.qualification = value; 1483 return this; 1484 } 1485 1486 protected void listChildren(List<Property> children) { 1487 super.listChildren(children); 1488 children.add(new Property("sequence", "positiveInt", "Sequence of careteam which serves to order and provide a link.", 0, 1, sequence)); 1489 children.add(new Property("provider", "Reference(Practitioner|Organization)", "The members of the team who provided the overall service.", 0, 1, provider)); 1490 children.add(new Property("responsible", "boolean", "The practitioner who is billing and responsible for the claimed services rendered to the patient.", 0, 1, responsible)); 1491 children.add(new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.", 0, 1, role)); 1492 children.add(new Property("qualification", "CodeableConcept", "The qualification which is applicable for this service.", 0, 1, qualification)); 1493 } 1494 1495 @Override 1496 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1497 switch (_hash) { 1498 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of careteam which serves to order and provide a link.", 0, 1, sequence); 1499 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner|Organization)", "The members of the team who provided the overall service.", 0, 1, provider); 1500 case 1847674614: /*responsible*/ return new Property("responsible", "boolean", "The practitioner who is billing and responsible for the claimed services rendered to the patient.", 0, 1, responsible); 1501 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "The lead, assisting or supervising practitioner and their discipline if a multidisiplinary team.", 0, 1, role); 1502 case -631333393: /*qualification*/ return new Property("qualification", "CodeableConcept", "The qualification which is applicable for this service.", 0, 1, qualification); 1503 default: return super.getNamedProperty(_hash, _name, _checkValid); 1504 } 1505 1506 } 1507 1508 @Override 1509 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1510 switch (hash) { 1511 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1512 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 1513 case 1847674614: /*responsible*/ return this.responsible == null ? new Base[0] : new Base[] {this.responsible}; // BooleanType 1514 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 1515 case -631333393: /*qualification*/ return this.qualification == null ? new Base[0] : new Base[] {this.qualification}; // CodeableConcept 1516 default: return super.getProperty(hash, name, checkValid); 1517 } 1518 1519 } 1520 1521 @Override 1522 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1523 switch (hash) { 1524 case 1349547969: // sequence 1525 this.sequence = castToPositiveInt(value); // PositiveIntType 1526 return value; 1527 case -987494927: // provider 1528 this.provider = castToReference(value); // Reference 1529 return value; 1530 case 1847674614: // responsible 1531 this.responsible = castToBoolean(value); // BooleanType 1532 return value; 1533 case 3506294: // role 1534 this.role = castToCodeableConcept(value); // CodeableConcept 1535 return value; 1536 case -631333393: // qualification 1537 this.qualification = castToCodeableConcept(value); // CodeableConcept 1538 return value; 1539 default: return super.setProperty(hash, name, value); 1540 } 1541 1542 } 1543 1544 @Override 1545 public Base setProperty(String name, Base value) throws FHIRException { 1546 if (name.equals("sequence")) { 1547 this.sequence = castToPositiveInt(value); // PositiveIntType 1548 } else if (name.equals("provider")) { 1549 this.provider = castToReference(value); // Reference 1550 } else if (name.equals("responsible")) { 1551 this.responsible = castToBoolean(value); // BooleanType 1552 } else if (name.equals("role")) { 1553 this.role = castToCodeableConcept(value); // CodeableConcept 1554 } else if (name.equals("qualification")) { 1555 this.qualification = castToCodeableConcept(value); // CodeableConcept 1556 } else 1557 return super.setProperty(name, value); 1558 return value; 1559 } 1560 1561 @Override 1562 public Base makeProperty(int hash, String name) throws FHIRException { 1563 switch (hash) { 1564 case 1349547969: return getSequenceElement(); 1565 case -987494927: return getProvider(); 1566 case 1847674614: return getResponsibleElement(); 1567 case 3506294: return getRole(); 1568 case -631333393: return getQualification(); 1569 default: return super.makeProperty(hash, name); 1570 } 1571 1572 } 1573 1574 @Override 1575 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1576 switch (hash) { 1577 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1578 case -987494927: /*provider*/ return new String[] {"Reference"}; 1579 case 1847674614: /*responsible*/ return new String[] {"boolean"}; 1580 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 1581 case -631333393: /*qualification*/ return new String[] {"CodeableConcept"}; 1582 default: return super.getTypesForProperty(hash, name); 1583 } 1584 1585 } 1586 1587 @Override 1588 public Base addChild(String name) throws FHIRException { 1589 if (name.equals("sequence")) { 1590 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 1591 } 1592 else if (name.equals("provider")) { 1593 this.provider = new Reference(); 1594 return this.provider; 1595 } 1596 else if (name.equals("responsible")) { 1597 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.responsible"); 1598 } 1599 else if (name.equals("role")) { 1600 this.role = new CodeableConcept(); 1601 return this.role; 1602 } 1603 else if (name.equals("qualification")) { 1604 this.qualification = new CodeableConcept(); 1605 return this.qualification; 1606 } 1607 else 1608 return super.addChild(name); 1609 } 1610 1611 public CareTeamComponent copy() { 1612 CareTeamComponent dst = new CareTeamComponent(); 1613 copyValues(dst); 1614 dst.sequence = sequence == null ? null : sequence.copy(); 1615 dst.provider = provider == null ? null : provider.copy(); 1616 dst.responsible = responsible == null ? null : responsible.copy(); 1617 dst.role = role == null ? null : role.copy(); 1618 dst.qualification = qualification == null ? null : qualification.copy(); 1619 return dst; 1620 } 1621 1622 @Override 1623 public boolean equalsDeep(Base other_) { 1624 if (!super.equalsDeep(other_)) 1625 return false; 1626 if (!(other_ instanceof CareTeamComponent)) 1627 return false; 1628 CareTeamComponent o = (CareTeamComponent) other_; 1629 return compareDeep(sequence, o.sequence, true) && compareDeep(provider, o.provider, true) && compareDeep(responsible, o.responsible, true) 1630 && compareDeep(role, o.role, true) && compareDeep(qualification, o.qualification, true); 1631 } 1632 1633 @Override 1634 public boolean equalsShallow(Base other_) { 1635 if (!super.equalsShallow(other_)) 1636 return false; 1637 if (!(other_ instanceof CareTeamComponent)) 1638 return false; 1639 CareTeamComponent o = (CareTeamComponent) other_; 1640 return compareValues(sequence, o.sequence, true) && compareValues(responsible, o.responsible, true) 1641 ; 1642 } 1643 1644 public boolean isEmpty() { 1645 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, provider, responsible 1646 , role, qualification); 1647 } 1648 1649 public String fhirType() { 1650 return "ExplanationOfBenefit.careTeam"; 1651 1652 } 1653 1654 } 1655 1656 @Block() 1657 public static class DiagnosisComponent extends BackboneElement implements IBaseBackboneElement { 1658 /** 1659 * Sequence of diagnosis which serves to provide a link. 1660 */ 1661 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1662 @Description(shortDefinition="Number to covey order of diagnosis", formalDefinition="Sequence of diagnosis which serves to provide a link." ) 1663 protected PositiveIntType sequence; 1664 1665 /** 1666 * The diagnosis. 1667 */ 1668 @Child(name = "diagnosis", type = {CodeableConcept.class, Condition.class}, order=2, min=1, max=1, modifier=false, summary=false) 1669 @Description(shortDefinition="Patient's diagnosis", formalDefinition="The diagnosis." ) 1670 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10") 1671 protected Type diagnosis; 1672 1673 /** 1674 * The type of the Diagnosis, for example: admitting, primary, secondary, discharge. 1675 */ 1676 @Child(name = "type", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1677 @Description(shortDefinition="Timing or nature of the diagnosis", formalDefinition="The type of the Diagnosis, for example: admitting, primary, secondary, discharge." ) 1678 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosistype") 1679 protected List<CodeableConcept> type; 1680 1681 /** 1682 * The package billing code, for example DRG, based on the assigned grouping code system. 1683 */ 1684 @Child(name = "packageCode", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 1685 @Description(shortDefinition="Package billing code", formalDefinition="The package billing code, for example DRG, based on the assigned grouping code system." ) 1686 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-diagnosisrelatedgroup") 1687 protected CodeableConcept packageCode; 1688 1689 private static final long serialVersionUID = -350960873L; 1690 1691 /** 1692 * Constructor 1693 */ 1694 public DiagnosisComponent() { 1695 super(); 1696 } 1697 1698 /** 1699 * Constructor 1700 */ 1701 public DiagnosisComponent(PositiveIntType sequence, Type diagnosis) { 1702 super(); 1703 this.sequence = sequence; 1704 this.diagnosis = diagnosis; 1705 } 1706 1707 /** 1708 * @return {@link #sequence} (Sequence of diagnosis which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1709 */ 1710 public PositiveIntType getSequenceElement() { 1711 if (this.sequence == null) 1712 if (Configuration.errorOnAutoCreate()) 1713 throw new Error("Attempt to auto-create DiagnosisComponent.sequence"); 1714 else if (Configuration.doAutoCreate()) 1715 this.sequence = new PositiveIntType(); // bb 1716 return this.sequence; 1717 } 1718 1719 public boolean hasSequenceElement() { 1720 return this.sequence != null && !this.sequence.isEmpty(); 1721 } 1722 1723 public boolean hasSequence() { 1724 return this.sequence != null && !this.sequence.isEmpty(); 1725 } 1726 1727 /** 1728 * @param value {@link #sequence} (Sequence of diagnosis which serves to provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 1729 */ 1730 public DiagnosisComponent setSequenceElement(PositiveIntType value) { 1731 this.sequence = value; 1732 return this; 1733 } 1734 1735 /** 1736 * @return Sequence of diagnosis which serves to provide a link. 1737 */ 1738 public int getSequence() { 1739 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 1740 } 1741 1742 /** 1743 * @param value Sequence of diagnosis which serves to provide a link. 1744 */ 1745 public DiagnosisComponent setSequence(int value) { 1746 if (this.sequence == null) 1747 this.sequence = new PositiveIntType(); 1748 this.sequence.setValue(value); 1749 return this; 1750 } 1751 1752 /** 1753 * @return {@link #diagnosis} (The diagnosis.) 1754 */ 1755 public Type getDiagnosis() { 1756 return this.diagnosis; 1757 } 1758 1759 /** 1760 * @return {@link #diagnosis} (The diagnosis.) 1761 */ 1762 public CodeableConcept getDiagnosisCodeableConcept() throws FHIRException { 1763 if (this.diagnosis == null) 1764 return null; 1765 if (!(this.diagnosis instanceof CodeableConcept)) 1766 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1767 return (CodeableConcept) this.diagnosis; 1768 } 1769 1770 public boolean hasDiagnosisCodeableConcept() { 1771 return this != null && this.diagnosis instanceof CodeableConcept; 1772 } 1773 1774 /** 1775 * @return {@link #diagnosis} (The diagnosis.) 1776 */ 1777 public Reference getDiagnosisReference() throws FHIRException { 1778 if (this.diagnosis == null) 1779 return null; 1780 if (!(this.diagnosis instanceof Reference)) 1781 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.diagnosis.getClass().getName()+" was encountered"); 1782 return (Reference) this.diagnosis; 1783 } 1784 1785 public boolean hasDiagnosisReference() { 1786 return this != null && this.diagnosis instanceof Reference; 1787 } 1788 1789 public boolean hasDiagnosis() { 1790 return this.diagnosis != null && !this.diagnosis.isEmpty(); 1791 } 1792 1793 /** 1794 * @param value {@link #diagnosis} (The diagnosis.) 1795 */ 1796 public DiagnosisComponent setDiagnosis(Type value) throws FHIRFormatError { 1797 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1798 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.diagnosis.diagnosis[x]: "+value.fhirType()); 1799 this.diagnosis = value; 1800 return this; 1801 } 1802 1803 /** 1804 * @return {@link #type} (The type of the Diagnosis, for example: admitting, primary, secondary, discharge.) 1805 */ 1806 public List<CodeableConcept> getType() { 1807 if (this.type == null) 1808 this.type = new ArrayList<CodeableConcept>(); 1809 return this.type; 1810 } 1811 1812 /** 1813 * @return Returns a reference to <code>this</code> for easy method chaining 1814 */ 1815 public DiagnosisComponent setType(List<CodeableConcept> theType) { 1816 this.type = theType; 1817 return this; 1818 } 1819 1820 public boolean hasType() { 1821 if (this.type == null) 1822 return false; 1823 for (CodeableConcept item : this.type) 1824 if (!item.isEmpty()) 1825 return true; 1826 return false; 1827 } 1828 1829 public CodeableConcept addType() { //3 1830 CodeableConcept t = new CodeableConcept(); 1831 if (this.type == null) 1832 this.type = new ArrayList<CodeableConcept>(); 1833 this.type.add(t); 1834 return t; 1835 } 1836 1837 public DiagnosisComponent addType(CodeableConcept t) { //3 1838 if (t == null) 1839 return this; 1840 if (this.type == null) 1841 this.type = new ArrayList<CodeableConcept>(); 1842 this.type.add(t); 1843 return this; 1844 } 1845 1846 /** 1847 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 1848 */ 1849 public CodeableConcept getTypeFirstRep() { 1850 if (getType().isEmpty()) { 1851 addType(); 1852 } 1853 return getType().get(0); 1854 } 1855 1856 /** 1857 * @return {@link #packageCode} (The package billing code, for example DRG, based on the assigned grouping code system.) 1858 */ 1859 public CodeableConcept getPackageCode() { 1860 if (this.packageCode == null) 1861 if (Configuration.errorOnAutoCreate()) 1862 throw new Error("Attempt to auto-create DiagnosisComponent.packageCode"); 1863 else if (Configuration.doAutoCreate()) 1864 this.packageCode = new CodeableConcept(); // cc 1865 return this.packageCode; 1866 } 1867 1868 public boolean hasPackageCode() { 1869 return this.packageCode != null && !this.packageCode.isEmpty(); 1870 } 1871 1872 /** 1873 * @param value {@link #packageCode} (The package billing code, for example DRG, based on the assigned grouping code system.) 1874 */ 1875 public DiagnosisComponent setPackageCode(CodeableConcept value) { 1876 this.packageCode = value; 1877 return this; 1878 } 1879 1880 protected void listChildren(List<Property> children) { 1881 super.listChildren(children); 1882 children.add(new Property("sequence", "positiveInt", "Sequence of diagnosis which serves to provide a link.", 0, 1, sequence)); 1883 children.add(new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis)); 1884 children.add(new Property("type", "CodeableConcept", "The type of the Diagnosis, for example: admitting, primary, secondary, discharge.", 0, java.lang.Integer.MAX_VALUE, type)); 1885 children.add(new Property("packageCode", "CodeableConcept", "The package billing code, for example DRG, based on the assigned grouping code system.", 0, 1, packageCode)); 1886 } 1887 1888 @Override 1889 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1890 switch (_hash) { 1891 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of diagnosis which serves to provide a link.", 0, 1, sequence); 1892 case -1487009809: /*diagnosis[x]*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 1893 case 1196993265: /*diagnosis*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 1894 case 277781616: /*diagnosisCodeableConcept*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 1895 case 2050454362: /*diagnosisReference*/ return new Property("diagnosis[x]", "CodeableConcept|Reference(Condition)", "The diagnosis.", 0, 1, diagnosis); 1896 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of the Diagnosis, for example: admitting, primary, secondary, discharge.", 0, java.lang.Integer.MAX_VALUE, type); 1897 case 908444499: /*packageCode*/ return new Property("packageCode", "CodeableConcept", "The package billing code, for example DRG, based on the assigned grouping code system.", 0, 1, packageCode); 1898 default: return super.getNamedProperty(_hash, _name, _checkValid); 1899 } 1900 1901 } 1902 1903 @Override 1904 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1905 switch (hash) { 1906 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 1907 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : new Base[] {this.diagnosis}; // Type 1908 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 1909 case 908444499: /*packageCode*/ return this.packageCode == null ? new Base[0] : new Base[] {this.packageCode}; // CodeableConcept 1910 default: return super.getProperty(hash, name, checkValid); 1911 } 1912 1913 } 1914 1915 @Override 1916 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1917 switch (hash) { 1918 case 1349547969: // sequence 1919 this.sequence = castToPositiveInt(value); // PositiveIntType 1920 return value; 1921 case 1196993265: // diagnosis 1922 this.diagnosis = castToType(value); // Type 1923 return value; 1924 case 3575610: // type 1925 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 1926 return value; 1927 case 908444499: // packageCode 1928 this.packageCode = castToCodeableConcept(value); // CodeableConcept 1929 return value; 1930 default: return super.setProperty(hash, name, value); 1931 } 1932 1933 } 1934 1935 @Override 1936 public Base setProperty(String name, Base value) throws FHIRException { 1937 if (name.equals("sequence")) { 1938 this.sequence = castToPositiveInt(value); // PositiveIntType 1939 } else if (name.equals("diagnosis[x]")) { 1940 this.diagnosis = castToType(value); // Type 1941 } else if (name.equals("type")) { 1942 this.getType().add(castToCodeableConcept(value)); 1943 } else if (name.equals("packageCode")) { 1944 this.packageCode = castToCodeableConcept(value); // CodeableConcept 1945 } else 1946 return super.setProperty(name, value); 1947 return value; 1948 } 1949 1950 @Override 1951 public Base makeProperty(int hash, String name) throws FHIRException { 1952 switch (hash) { 1953 case 1349547969: return getSequenceElement(); 1954 case -1487009809: return getDiagnosis(); 1955 case 1196993265: return getDiagnosis(); 1956 case 3575610: return addType(); 1957 case 908444499: return getPackageCode(); 1958 default: return super.makeProperty(hash, name); 1959 } 1960 1961 } 1962 1963 @Override 1964 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1965 switch (hash) { 1966 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 1967 case 1196993265: /*diagnosis*/ return new String[] {"CodeableConcept", "Reference"}; 1968 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1969 case 908444499: /*packageCode*/ return new String[] {"CodeableConcept"}; 1970 default: return super.getTypesForProperty(hash, name); 1971 } 1972 1973 } 1974 1975 @Override 1976 public Base addChild(String name) throws FHIRException { 1977 if (name.equals("sequence")) { 1978 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 1979 } 1980 else if (name.equals("diagnosisCodeableConcept")) { 1981 this.diagnosis = new CodeableConcept(); 1982 return this.diagnosis; 1983 } 1984 else if (name.equals("diagnosisReference")) { 1985 this.diagnosis = new Reference(); 1986 return this.diagnosis; 1987 } 1988 else if (name.equals("type")) { 1989 return addType(); 1990 } 1991 else if (name.equals("packageCode")) { 1992 this.packageCode = new CodeableConcept(); 1993 return this.packageCode; 1994 } 1995 else 1996 return super.addChild(name); 1997 } 1998 1999 public DiagnosisComponent copy() { 2000 DiagnosisComponent dst = new DiagnosisComponent(); 2001 copyValues(dst); 2002 dst.sequence = sequence == null ? null : sequence.copy(); 2003 dst.diagnosis = diagnosis == null ? null : diagnosis.copy(); 2004 if (type != null) { 2005 dst.type = new ArrayList<CodeableConcept>(); 2006 for (CodeableConcept i : type) 2007 dst.type.add(i.copy()); 2008 }; 2009 dst.packageCode = packageCode == null ? null : packageCode.copy(); 2010 return dst; 2011 } 2012 2013 @Override 2014 public boolean equalsDeep(Base other_) { 2015 if (!super.equalsDeep(other_)) 2016 return false; 2017 if (!(other_ instanceof DiagnosisComponent)) 2018 return false; 2019 DiagnosisComponent o = (DiagnosisComponent) other_; 2020 return compareDeep(sequence, o.sequence, true) && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(type, o.type, true) 2021 && compareDeep(packageCode, o.packageCode, true); 2022 } 2023 2024 @Override 2025 public boolean equalsShallow(Base other_) { 2026 if (!super.equalsShallow(other_)) 2027 return false; 2028 if (!(other_ instanceof DiagnosisComponent)) 2029 return false; 2030 DiagnosisComponent o = (DiagnosisComponent) other_; 2031 return compareValues(sequence, o.sequence, true); 2032 } 2033 2034 public boolean isEmpty() { 2035 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, diagnosis, type 2036 , packageCode); 2037 } 2038 2039 public String fhirType() { 2040 return "ExplanationOfBenefit.diagnosis"; 2041 2042 } 2043 2044 } 2045 2046 @Block() 2047 public static class ProcedureComponent extends BackboneElement implements IBaseBackboneElement { 2048 /** 2049 * Sequence of procedures which serves to order and provide a link. 2050 */ 2051 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2052 @Description(shortDefinition="Procedure sequence for reference", formalDefinition="Sequence of procedures which serves to order and provide a link." ) 2053 protected PositiveIntType sequence; 2054 2055 /** 2056 * Date and optionally time the procedure was performed . 2057 */ 2058 @Child(name = "date", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 2059 @Description(shortDefinition="When the procedure was performed", formalDefinition="Date and optionally time the procedure was performed ." ) 2060 protected DateTimeType date; 2061 2062 /** 2063 * The procedure code. 2064 */ 2065 @Child(name = "procedure", type = {CodeableConcept.class, Procedure.class}, order=3, min=1, max=1, modifier=false, summary=false) 2066 @Description(shortDefinition="Patient's list of procedures performed", formalDefinition="The procedure code." ) 2067 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/icd-10-procedures") 2068 protected Type procedure; 2069 2070 private static final long serialVersionUID = 864307347L; 2071 2072 /** 2073 * Constructor 2074 */ 2075 public ProcedureComponent() { 2076 super(); 2077 } 2078 2079 /** 2080 * Constructor 2081 */ 2082 public ProcedureComponent(PositiveIntType sequence, Type procedure) { 2083 super(); 2084 this.sequence = sequence; 2085 this.procedure = procedure; 2086 } 2087 2088 /** 2089 * @return {@link #sequence} (Sequence of procedures which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2090 */ 2091 public PositiveIntType getSequenceElement() { 2092 if (this.sequence == null) 2093 if (Configuration.errorOnAutoCreate()) 2094 throw new Error("Attempt to auto-create ProcedureComponent.sequence"); 2095 else if (Configuration.doAutoCreate()) 2096 this.sequence = new PositiveIntType(); // bb 2097 return this.sequence; 2098 } 2099 2100 public boolean hasSequenceElement() { 2101 return this.sequence != null && !this.sequence.isEmpty(); 2102 } 2103 2104 public boolean hasSequence() { 2105 return this.sequence != null && !this.sequence.isEmpty(); 2106 } 2107 2108 /** 2109 * @param value {@link #sequence} (Sequence of procedures which serves to order and provide a link.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 2110 */ 2111 public ProcedureComponent setSequenceElement(PositiveIntType value) { 2112 this.sequence = value; 2113 return this; 2114 } 2115 2116 /** 2117 * @return Sequence of procedures which serves to order and provide a link. 2118 */ 2119 public int getSequence() { 2120 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 2121 } 2122 2123 /** 2124 * @param value Sequence of procedures which serves to order and provide a link. 2125 */ 2126 public ProcedureComponent setSequence(int value) { 2127 if (this.sequence == null) 2128 this.sequence = new PositiveIntType(); 2129 this.sequence.setValue(value); 2130 return this; 2131 } 2132 2133 /** 2134 * @return {@link #date} (Date and optionally time the procedure was performed .). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2135 */ 2136 public DateTimeType getDateElement() { 2137 if (this.date == null) 2138 if (Configuration.errorOnAutoCreate()) 2139 throw new Error("Attempt to auto-create ProcedureComponent.date"); 2140 else if (Configuration.doAutoCreate()) 2141 this.date = new DateTimeType(); // bb 2142 return this.date; 2143 } 2144 2145 public boolean hasDateElement() { 2146 return this.date != null && !this.date.isEmpty(); 2147 } 2148 2149 public boolean hasDate() { 2150 return this.date != null && !this.date.isEmpty(); 2151 } 2152 2153 /** 2154 * @param value {@link #date} (Date and optionally time the procedure was performed .). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2155 */ 2156 public ProcedureComponent setDateElement(DateTimeType value) { 2157 this.date = value; 2158 return this; 2159 } 2160 2161 /** 2162 * @return Date and optionally time the procedure was performed . 2163 */ 2164 public Date getDate() { 2165 return this.date == null ? null : this.date.getValue(); 2166 } 2167 2168 /** 2169 * @param value Date and optionally time the procedure was performed . 2170 */ 2171 public ProcedureComponent setDate(Date value) { 2172 if (value == null) 2173 this.date = null; 2174 else { 2175 if (this.date == null) 2176 this.date = new DateTimeType(); 2177 this.date.setValue(value); 2178 } 2179 return this; 2180 } 2181 2182 /** 2183 * @return {@link #procedure} (The procedure code.) 2184 */ 2185 public Type getProcedure() { 2186 return this.procedure; 2187 } 2188 2189 /** 2190 * @return {@link #procedure} (The procedure code.) 2191 */ 2192 public CodeableConcept getProcedureCodeableConcept() throws FHIRException { 2193 if (this.procedure == null) 2194 return null; 2195 if (!(this.procedure instanceof CodeableConcept)) 2196 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2197 return (CodeableConcept) this.procedure; 2198 } 2199 2200 public boolean hasProcedureCodeableConcept() { 2201 return this != null && this.procedure instanceof CodeableConcept; 2202 } 2203 2204 /** 2205 * @return {@link #procedure} (The procedure code.) 2206 */ 2207 public Reference getProcedureReference() throws FHIRException { 2208 if (this.procedure == null) 2209 return null; 2210 if (!(this.procedure instanceof Reference)) 2211 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.procedure.getClass().getName()+" was encountered"); 2212 return (Reference) this.procedure; 2213 } 2214 2215 public boolean hasProcedureReference() { 2216 return this != null && this.procedure instanceof Reference; 2217 } 2218 2219 public boolean hasProcedure() { 2220 return this.procedure != null && !this.procedure.isEmpty(); 2221 } 2222 2223 /** 2224 * @param value {@link #procedure} (The procedure code.) 2225 */ 2226 public ProcedureComponent setProcedure(Type value) throws FHIRFormatError { 2227 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2228 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.procedure.procedure[x]: "+value.fhirType()); 2229 this.procedure = value; 2230 return this; 2231 } 2232 2233 protected void listChildren(List<Property> children) { 2234 super.listChildren(children); 2235 children.add(new Property("sequence", "positiveInt", "Sequence of procedures which serves to order and provide a link.", 0, 1, sequence)); 2236 children.add(new Property("date", "dateTime", "Date and optionally time the procedure was performed .", 0, 1, date)); 2237 children.add(new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure)); 2238 } 2239 2240 @Override 2241 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2242 switch (_hash) { 2243 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "Sequence of procedures which serves to order and provide a link.", 0, 1, sequence); 2244 case 3076014: /*date*/ return new Property("date", "dateTime", "Date and optionally time the procedure was performed .", 0, 1, date); 2245 case 1640074445: /*procedure[x]*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2246 case -1095204141: /*procedure*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2247 case -1284783026: /*procedureCodeableConcept*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2248 case 881809848: /*procedureReference*/ return new Property("procedure[x]", "CodeableConcept|Reference(Procedure)", "The procedure code.", 0, 1, procedure); 2249 default: return super.getNamedProperty(_hash, _name, _checkValid); 2250 } 2251 2252 } 2253 2254 @Override 2255 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2256 switch (hash) { 2257 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 2258 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2259 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : new Base[] {this.procedure}; // Type 2260 default: return super.getProperty(hash, name, checkValid); 2261 } 2262 2263 } 2264 2265 @Override 2266 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2267 switch (hash) { 2268 case 1349547969: // sequence 2269 this.sequence = castToPositiveInt(value); // PositiveIntType 2270 return value; 2271 case 3076014: // date 2272 this.date = castToDateTime(value); // DateTimeType 2273 return value; 2274 case -1095204141: // procedure 2275 this.procedure = castToType(value); // Type 2276 return value; 2277 default: return super.setProperty(hash, name, value); 2278 } 2279 2280 } 2281 2282 @Override 2283 public Base setProperty(String name, Base value) throws FHIRException { 2284 if (name.equals("sequence")) { 2285 this.sequence = castToPositiveInt(value); // PositiveIntType 2286 } else if (name.equals("date")) { 2287 this.date = castToDateTime(value); // DateTimeType 2288 } else if (name.equals("procedure[x]")) { 2289 this.procedure = castToType(value); // Type 2290 } else 2291 return super.setProperty(name, value); 2292 return value; 2293 } 2294 2295 @Override 2296 public Base makeProperty(int hash, String name) throws FHIRException { 2297 switch (hash) { 2298 case 1349547969: return getSequenceElement(); 2299 case 3076014: return getDateElement(); 2300 case 1640074445: return getProcedure(); 2301 case -1095204141: return getProcedure(); 2302 default: return super.makeProperty(hash, name); 2303 } 2304 2305 } 2306 2307 @Override 2308 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2309 switch (hash) { 2310 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 2311 case 3076014: /*date*/ return new String[] {"dateTime"}; 2312 case -1095204141: /*procedure*/ return new String[] {"CodeableConcept", "Reference"}; 2313 default: return super.getTypesForProperty(hash, name); 2314 } 2315 2316 } 2317 2318 @Override 2319 public Base addChild(String name) throws FHIRException { 2320 if (name.equals("sequence")) { 2321 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 2322 } 2323 else if (name.equals("date")) { 2324 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 2325 } 2326 else if (name.equals("procedureCodeableConcept")) { 2327 this.procedure = new CodeableConcept(); 2328 return this.procedure; 2329 } 2330 else if (name.equals("procedureReference")) { 2331 this.procedure = new Reference(); 2332 return this.procedure; 2333 } 2334 else 2335 return super.addChild(name); 2336 } 2337 2338 public ProcedureComponent copy() { 2339 ProcedureComponent dst = new ProcedureComponent(); 2340 copyValues(dst); 2341 dst.sequence = sequence == null ? null : sequence.copy(); 2342 dst.date = date == null ? null : date.copy(); 2343 dst.procedure = procedure == null ? null : procedure.copy(); 2344 return dst; 2345 } 2346 2347 @Override 2348 public boolean equalsDeep(Base other_) { 2349 if (!super.equalsDeep(other_)) 2350 return false; 2351 if (!(other_ instanceof ProcedureComponent)) 2352 return false; 2353 ProcedureComponent o = (ProcedureComponent) other_; 2354 return compareDeep(sequence, o.sequence, true) && compareDeep(date, o.date, true) && compareDeep(procedure, o.procedure, true) 2355 ; 2356 } 2357 2358 @Override 2359 public boolean equalsShallow(Base other_) { 2360 if (!super.equalsShallow(other_)) 2361 return false; 2362 if (!(other_ instanceof ProcedureComponent)) 2363 return false; 2364 ProcedureComponent o = (ProcedureComponent) other_; 2365 return compareValues(sequence, o.sequence, true) && compareValues(date, o.date, true); 2366 } 2367 2368 public boolean isEmpty() { 2369 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, date, procedure 2370 ); 2371 } 2372 2373 public String fhirType() { 2374 return "ExplanationOfBenefit.procedure"; 2375 2376 } 2377 2378 } 2379 2380 @Block() 2381 public static class InsuranceComponent extends BackboneElement implements IBaseBackboneElement { 2382 /** 2383 * Reference to the program or plan identification, underwriter or payor. 2384 */ 2385 @Child(name = "coverage", type = {Coverage.class}, order=1, min=0, max=1, modifier=false, summary=false) 2386 @Description(shortDefinition="Insurance information", formalDefinition="Reference to the program or plan identification, underwriter or payor." ) 2387 protected Reference coverage; 2388 2389 /** 2390 * The actual object that is the target of the reference (Reference to the program or plan identification, underwriter or payor.) 2391 */ 2392 protected Coverage coverageTarget; 2393 2394 /** 2395 * A list of references from the Insurer to which these services pertain. 2396 */ 2397 @Child(name = "preAuthRef", type = {StringType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2398 @Description(shortDefinition="Pre-Authorization/Determination Reference", formalDefinition="A list of references from the Insurer to which these services pertain." ) 2399 protected List<StringType> preAuthRef; 2400 2401 private static final long serialVersionUID = -870298727L; 2402 2403 /** 2404 * Constructor 2405 */ 2406 public InsuranceComponent() { 2407 super(); 2408 } 2409 2410 /** 2411 * @return {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 2412 */ 2413 public Reference getCoverage() { 2414 if (this.coverage == null) 2415 if (Configuration.errorOnAutoCreate()) 2416 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2417 else if (Configuration.doAutoCreate()) 2418 this.coverage = new Reference(); // cc 2419 return this.coverage; 2420 } 2421 2422 public boolean hasCoverage() { 2423 return this.coverage != null && !this.coverage.isEmpty(); 2424 } 2425 2426 /** 2427 * @param value {@link #coverage} (Reference to the program or plan identification, underwriter or payor.) 2428 */ 2429 public InsuranceComponent setCoverage(Reference value) { 2430 this.coverage = value; 2431 return this; 2432 } 2433 2434 /** 2435 * @return {@link #coverage} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 2436 */ 2437 public Coverage getCoverageTarget() { 2438 if (this.coverageTarget == null) 2439 if (Configuration.errorOnAutoCreate()) 2440 throw new Error("Attempt to auto-create InsuranceComponent.coverage"); 2441 else if (Configuration.doAutoCreate()) 2442 this.coverageTarget = new Coverage(); // aa 2443 return this.coverageTarget; 2444 } 2445 2446 /** 2447 * @param value {@link #coverage} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the program or plan identification, underwriter or payor.) 2448 */ 2449 public InsuranceComponent setCoverageTarget(Coverage value) { 2450 this.coverageTarget = value; 2451 return this; 2452 } 2453 2454 /** 2455 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2456 */ 2457 public List<StringType> getPreAuthRef() { 2458 if (this.preAuthRef == null) 2459 this.preAuthRef = new ArrayList<StringType>(); 2460 return this.preAuthRef; 2461 } 2462 2463 /** 2464 * @return Returns a reference to <code>this</code> for easy method chaining 2465 */ 2466 public InsuranceComponent setPreAuthRef(List<StringType> thePreAuthRef) { 2467 this.preAuthRef = thePreAuthRef; 2468 return this; 2469 } 2470 2471 public boolean hasPreAuthRef() { 2472 if (this.preAuthRef == null) 2473 return false; 2474 for (StringType item : this.preAuthRef) 2475 if (!item.isEmpty()) 2476 return true; 2477 return false; 2478 } 2479 2480 /** 2481 * @return {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2482 */ 2483 public StringType addPreAuthRefElement() {//2 2484 StringType t = new StringType(); 2485 if (this.preAuthRef == null) 2486 this.preAuthRef = new ArrayList<StringType>(); 2487 this.preAuthRef.add(t); 2488 return t; 2489 } 2490 2491 /** 2492 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2493 */ 2494 public InsuranceComponent addPreAuthRef(String value) { //1 2495 StringType t = new StringType(); 2496 t.setValue(value); 2497 if (this.preAuthRef == null) 2498 this.preAuthRef = new ArrayList<StringType>(); 2499 this.preAuthRef.add(t); 2500 return this; 2501 } 2502 2503 /** 2504 * @param value {@link #preAuthRef} (A list of references from the Insurer to which these services pertain.) 2505 */ 2506 public boolean hasPreAuthRef(String value) { 2507 if (this.preAuthRef == null) 2508 return false; 2509 for (StringType v : this.preAuthRef) 2510 if (v.getValue().equals(value)) // string 2511 return true; 2512 return false; 2513 } 2514 2515 protected void listChildren(List<Property> children) { 2516 super.listChildren(children); 2517 children.add(new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage)); 2518 children.add(new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef)); 2519 } 2520 2521 @Override 2522 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2523 switch (_hash) { 2524 case -351767064: /*coverage*/ return new Property("coverage", "Reference(Coverage)", "Reference to the program or plan identification, underwriter or payor.", 0, 1, coverage); 2525 case 522246568: /*preAuthRef*/ return new Property("preAuthRef", "string", "A list of references from the Insurer to which these services pertain.", 0, java.lang.Integer.MAX_VALUE, preAuthRef); 2526 default: return super.getNamedProperty(_hash, _name, _checkValid); 2527 } 2528 2529 } 2530 2531 @Override 2532 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2533 switch (hash) { 2534 case -351767064: /*coverage*/ return this.coverage == null ? new Base[0] : new Base[] {this.coverage}; // Reference 2535 case 522246568: /*preAuthRef*/ return this.preAuthRef == null ? new Base[0] : this.preAuthRef.toArray(new Base[this.preAuthRef.size()]); // StringType 2536 default: return super.getProperty(hash, name, checkValid); 2537 } 2538 2539 } 2540 2541 @Override 2542 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2543 switch (hash) { 2544 case -351767064: // coverage 2545 this.coverage = castToReference(value); // Reference 2546 return value; 2547 case 522246568: // preAuthRef 2548 this.getPreAuthRef().add(castToString(value)); // StringType 2549 return value; 2550 default: return super.setProperty(hash, name, value); 2551 } 2552 2553 } 2554 2555 @Override 2556 public Base setProperty(String name, Base value) throws FHIRException { 2557 if (name.equals("coverage")) { 2558 this.coverage = castToReference(value); // Reference 2559 } else if (name.equals("preAuthRef")) { 2560 this.getPreAuthRef().add(castToString(value)); 2561 } else 2562 return super.setProperty(name, value); 2563 return value; 2564 } 2565 2566 @Override 2567 public Base makeProperty(int hash, String name) throws FHIRException { 2568 switch (hash) { 2569 case -351767064: return getCoverage(); 2570 case 522246568: return addPreAuthRefElement(); 2571 default: return super.makeProperty(hash, name); 2572 } 2573 2574 } 2575 2576 @Override 2577 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2578 switch (hash) { 2579 case -351767064: /*coverage*/ return new String[] {"Reference"}; 2580 case 522246568: /*preAuthRef*/ return new String[] {"string"}; 2581 default: return super.getTypesForProperty(hash, name); 2582 } 2583 2584 } 2585 2586 @Override 2587 public Base addChild(String name) throws FHIRException { 2588 if (name.equals("coverage")) { 2589 this.coverage = new Reference(); 2590 return this.coverage; 2591 } 2592 else if (name.equals("preAuthRef")) { 2593 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.preAuthRef"); 2594 } 2595 else 2596 return super.addChild(name); 2597 } 2598 2599 public InsuranceComponent copy() { 2600 InsuranceComponent dst = new InsuranceComponent(); 2601 copyValues(dst); 2602 dst.coverage = coverage == null ? null : coverage.copy(); 2603 if (preAuthRef != null) { 2604 dst.preAuthRef = new ArrayList<StringType>(); 2605 for (StringType i : preAuthRef) 2606 dst.preAuthRef.add(i.copy()); 2607 }; 2608 return dst; 2609 } 2610 2611 @Override 2612 public boolean equalsDeep(Base other_) { 2613 if (!super.equalsDeep(other_)) 2614 return false; 2615 if (!(other_ instanceof InsuranceComponent)) 2616 return false; 2617 InsuranceComponent o = (InsuranceComponent) other_; 2618 return compareDeep(coverage, o.coverage, true) && compareDeep(preAuthRef, o.preAuthRef, true); 2619 } 2620 2621 @Override 2622 public boolean equalsShallow(Base other_) { 2623 if (!super.equalsShallow(other_)) 2624 return false; 2625 if (!(other_ instanceof InsuranceComponent)) 2626 return false; 2627 InsuranceComponent o = (InsuranceComponent) other_; 2628 return compareValues(preAuthRef, o.preAuthRef, true); 2629 } 2630 2631 public boolean isEmpty() { 2632 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(coverage, preAuthRef); 2633 } 2634 2635 public String fhirType() { 2636 return "ExplanationOfBenefit.insurance"; 2637 2638 } 2639 2640 } 2641 2642 @Block() 2643 public static class AccidentComponent extends BackboneElement implements IBaseBackboneElement { 2644 /** 2645 * Date of an accident which these services are addressing. 2646 */ 2647 @Child(name = "date", type = {DateType.class}, order=1, min=0, max=1, modifier=false, summary=false) 2648 @Description(shortDefinition="When the accident occurred", formalDefinition="Date of an accident which these services are addressing." ) 2649 protected DateType date; 2650 2651 /** 2652 * Type of accident: work, auto, etc. 2653 */ 2654 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 2655 @Description(shortDefinition="The nature of the accident", formalDefinition="Type of accident: work, auto, etc." ) 2656 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActIncidentCode") 2657 protected CodeableConcept type; 2658 2659 /** 2660 * Where the accident occurred. 2661 */ 2662 @Child(name = "location", type = {Address.class, Location.class}, order=3, min=0, max=1, modifier=false, summary=false) 2663 @Description(shortDefinition="Accident Place", formalDefinition="Where the accident occurred." ) 2664 protected Type location; 2665 2666 private static final long serialVersionUID = 622904984L; 2667 2668 /** 2669 * Constructor 2670 */ 2671 public AccidentComponent() { 2672 super(); 2673 } 2674 2675 /** 2676 * @return {@link #date} (Date of an accident which these services are addressing.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2677 */ 2678 public DateType getDateElement() { 2679 if (this.date == null) 2680 if (Configuration.errorOnAutoCreate()) 2681 throw new Error("Attempt to auto-create AccidentComponent.date"); 2682 else if (Configuration.doAutoCreate()) 2683 this.date = new DateType(); // bb 2684 return this.date; 2685 } 2686 2687 public boolean hasDateElement() { 2688 return this.date != null && !this.date.isEmpty(); 2689 } 2690 2691 public boolean hasDate() { 2692 return this.date != null && !this.date.isEmpty(); 2693 } 2694 2695 /** 2696 * @param value {@link #date} (Date of an accident which these services are addressing.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2697 */ 2698 public AccidentComponent setDateElement(DateType value) { 2699 this.date = value; 2700 return this; 2701 } 2702 2703 /** 2704 * @return Date of an accident which these services are addressing. 2705 */ 2706 public Date getDate() { 2707 return this.date == null ? null : this.date.getValue(); 2708 } 2709 2710 /** 2711 * @param value Date of an accident which these services are addressing. 2712 */ 2713 public AccidentComponent setDate(Date value) { 2714 if (value == null) 2715 this.date = null; 2716 else { 2717 if (this.date == null) 2718 this.date = new DateType(); 2719 this.date.setValue(value); 2720 } 2721 return this; 2722 } 2723 2724 /** 2725 * @return {@link #type} (Type of accident: work, auto, etc.) 2726 */ 2727 public CodeableConcept getType() { 2728 if (this.type == null) 2729 if (Configuration.errorOnAutoCreate()) 2730 throw new Error("Attempt to auto-create AccidentComponent.type"); 2731 else if (Configuration.doAutoCreate()) 2732 this.type = new CodeableConcept(); // cc 2733 return this.type; 2734 } 2735 2736 public boolean hasType() { 2737 return this.type != null && !this.type.isEmpty(); 2738 } 2739 2740 /** 2741 * @param value {@link #type} (Type of accident: work, auto, etc.) 2742 */ 2743 public AccidentComponent setType(CodeableConcept value) { 2744 this.type = value; 2745 return this; 2746 } 2747 2748 /** 2749 * @return {@link #location} (Where the accident occurred.) 2750 */ 2751 public Type getLocation() { 2752 return this.location; 2753 } 2754 2755 /** 2756 * @return {@link #location} (Where the accident occurred.) 2757 */ 2758 public Address getLocationAddress() throws FHIRException { 2759 if (this.location == null) 2760 return null; 2761 if (!(this.location instanceof Address)) 2762 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 2763 return (Address) this.location; 2764 } 2765 2766 public boolean hasLocationAddress() { 2767 return this != null && this.location instanceof Address; 2768 } 2769 2770 /** 2771 * @return {@link #location} (Where the accident occurred.) 2772 */ 2773 public Reference getLocationReference() throws FHIRException { 2774 if (this.location == null) 2775 return null; 2776 if (!(this.location instanceof Reference)) 2777 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 2778 return (Reference) this.location; 2779 } 2780 2781 public boolean hasLocationReference() { 2782 return this != null && this.location instanceof Reference; 2783 } 2784 2785 public boolean hasLocation() { 2786 return this.location != null && !this.location.isEmpty(); 2787 } 2788 2789 /** 2790 * @param value {@link #location} (Where the accident occurred.) 2791 */ 2792 public AccidentComponent setLocation(Type value) throws FHIRFormatError { 2793 if (value != null && !(value instanceof Address || value instanceof Reference)) 2794 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.accident.location[x]: "+value.fhirType()); 2795 this.location = value; 2796 return this; 2797 } 2798 2799 protected void listChildren(List<Property> children) { 2800 super.listChildren(children); 2801 children.add(new Property("date", "date", "Date of an accident which these services are addressing.", 0, 1, date)); 2802 children.add(new Property("type", "CodeableConcept", "Type of accident: work, auto, etc.", 0, 1, type)); 2803 children.add(new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location)); 2804 } 2805 2806 @Override 2807 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2808 switch (_hash) { 2809 case 3076014: /*date*/ return new Property("date", "date", "Date of an accident which these services are addressing.", 0, 1, date); 2810 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Type of accident: work, auto, etc.", 0, 1, type); 2811 case 552316075: /*location[x]*/ return new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location); 2812 case 1901043637: /*location*/ return new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location); 2813 case -1280020865: /*locationAddress*/ return new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location); 2814 case 755866390: /*locationReference*/ return new Property("location[x]", "Address|Reference(Location)", "Where the accident occurred.", 0, 1, location); 2815 default: return super.getNamedProperty(_hash, _name, _checkValid); 2816 } 2817 2818 } 2819 2820 @Override 2821 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2822 switch (hash) { 2823 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 2824 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2825 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Type 2826 default: return super.getProperty(hash, name, checkValid); 2827 } 2828 2829 } 2830 2831 @Override 2832 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2833 switch (hash) { 2834 case 3076014: // date 2835 this.date = castToDate(value); // DateType 2836 return value; 2837 case 3575610: // type 2838 this.type = castToCodeableConcept(value); // CodeableConcept 2839 return value; 2840 case 1901043637: // location 2841 this.location = castToType(value); // Type 2842 return value; 2843 default: return super.setProperty(hash, name, value); 2844 } 2845 2846 } 2847 2848 @Override 2849 public Base setProperty(String name, Base value) throws FHIRException { 2850 if (name.equals("date")) { 2851 this.date = castToDate(value); // DateType 2852 } else if (name.equals("type")) { 2853 this.type = castToCodeableConcept(value); // CodeableConcept 2854 } else if (name.equals("location[x]")) { 2855 this.location = castToType(value); // Type 2856 } else 2857 return super.setProperty(name, value); 2858 return value; 2859 } 2860 2861 @Override 2862 public Base makeProperty(int hash, String name) throws FHIRException { 2863 switch (hash) { 2864 case 3076014: return getDateElement(); 2865 case 3575610: return getType(); 2866 case 552316075: return getLocation(); 2867 case 1901043637: return getLocation(); 2868 default: return super.makeProperty(hash, name); 2869 } 2870 2871 } 2872 2873 @Override 2874 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2875 switch (hash) { 2876 case 3076014: /*date*/ return new String[] {"date"}; 2877 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2878 case 1901043637: /*location*/ return new String[] {"Address", "Reference"}; 2879 default: return super.getTypesForProperty(hash, name); 2880 } 2881 2882 } 2883 2884 @Override 2885 public Base addChild(String name) throws FHIRException { 2886 if (name.equals("date")) { 2887 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 2888 } 2889 else if (name.equals("type")) { 2890 this.type = new CodeableConcept(); 2891 return this.type; 2892 } 2893 else if (name.equals("locationAddress")) { 2894 this.location = new Address(); 2895 return this.location; 2896 } 2897 else if (name.equals("locationReference")) { 2898 this.location = new Reference(); 2899 return this.location; 2900 } 2901 else 2902 return super.addChild(name); 2903 } 2904 2905 public AccidentComponent copy() { 2906 AccidentComponent dst = new AccidentComponent(); 2907 copyValues(dst); 2908 dst.date = date == null ? null : date.copy(); 2909 dst.type = type == null ? null : type.copy(); 2910 dst.location = location == null ? null : location.copy(); 2911 return dst; 2912 } 2913 2914 @Override 2915 public boolean equalsDeep(Base other_) { 2916 if (!super.equalsDeep(other_)) 2917 return false; 2918 if (!(other_ instanceof AccidentComponent)) 2919 return false; 2920 AccidentComponent o = (AccidentComponent) other_; 2921 return compareDeep(date, o.date, true) && compareDeep(type, o.type, true) && compareDeep(location, o.location, true) 2922 ; 2923 } 2924 2925 @Override 2926 public boolean equalsShallow(Base other_) { 2927 if (!super.equalsShallow(other_)) 2928 return false; 2929 if (!(other_ instanceof AccidentComponent)) 2930 return false; 2931 AccidentComponent o = (AccidentComponent) other_; 2932 return compareValues(date, o.date, true); 2933 } 2934 2935 public boolean isEmpty() { 2936 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, type, location); 2937 } 2938 2939 public String fhirType() { 2940 return "ExplanationOfBenefit.accident"; 2941 2942 } 2943 2944 } 2945 2946 @Block() 2947 public static class ItemComponent extends BackboneElement implements IBaseBackboneElement { 2948 /** 2949 * A service line number. 2950 */ 2951 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 2952 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 2953 protected PositiveIntType sequence; 2954 2955 /** 2956 * Careteam applicable for this service or product line. 2957 */ 2958 @Child(name = "careTeamLinkId", type = {PositiveIntType.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2959 @Description(shortDefinition="Applicable careteam members", formalDefinition="Careteam applicable for this service or product line." ) 2960 protected List<PositiveIntType> careTeamLinkId; 2961 2962 /** 2963 * Diagnosis applicable for this service or product line. 2964 */ 2965 @Child(name = "diagnosisLinkId", type = {PositiveIntType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2966 @Description(shortDefinition="Applicable diagnoses", formalDefinition="Diagnosis applicable for this service or product line." ) 2967 protected List<PositiveIntType> diagnosisLinkId; 2968 2969 /** 2970 * Procedures applicable for this service or product line. 2971 */ 2972 @Child(name = "procedureLinkId", type = {PositiveIntType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2973 @Description(shortDefinition="Applicable procedures", formalDefinition="Procedures applicable for this service or product line." ) 2974 protected List<PositiveIntType> procedureLinkId; 2975 2976 /** 2977 * Exceptions, special conditions and supporting information pplicable for this service or product line. 2978 */ 2979 @Child(name = "informationLinkId", type = {PositiveIntType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2980 @Description(shortDefinition="Applicable exception and supporting information", formalDefinition="Exceptions, special conditions and supporting information pplicable for this service or product line." ) 2981 protected List<PositiveIntType> informationLinkId; 2982 2983 /** 2984 * The type of reveneu or cost center providing the product and/or service. 2985 */ 2986 @Child(name = "revenue", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 2987 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 2988 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 2989 protected CodeableConcept revenue; 2990 2991 /** 2992 * Health Care Service Type Codes to identify the classification of service or benefits. 2993 */ 2994 @Child(name = "category", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 2995 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 2996 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 2997 protected CodeableConcept category; 2998 2999 /** 3000 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 3001 */ 3002 @Child(name = "service", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 3003 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 3004 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 3005 protected CodeableConcept service; 3006 3007 /** 3008 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 3009 */ 3010 @Child(name = "modifier", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3011 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 3012 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 3013 protected List<CodeableConcept> modifier; 3014 3015 /** 3016 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 3017 */ 3018 @Child(name = "programCode", type = {CodeableConcept.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3019 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 3020 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 3021 protected List<CodeableConcept> programCode; 3022 3023 /** 3024 * The date or dates when the enclosed suite of services were performed or completed. 3025 */ 3026 @Child(name = "serviced", type = {DateType.class, Period.class}, order=11, min=0, max=1, modifier=false, summary=false) 3027 @Description(shortDefinition="Date or dates of Service", formalDefinition="The date or dates when the enclosed suite of services were performed or completed." ) 3028 protected Type serviced; 3029 3030 /** 3031 * Where the service was provided. 3032 */ 3033 @Child(name = "location", type = {CodeableConcept.class, Address.class, Location.class}, order=12, min=0, max=1, modifier=false, summary=false) 3034 @Description(shortDefinition="Place of service", formalDefinition="Where the service was provided." ) 3035 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-place") 3036 protected Type location; 3037 3038 /** 3039 * The number of repetitions of a service or product. 3040 */ 3041 @Child(name = "quantity", type = {SimpleQuantity.class}, order=13, min=0, max=1, modifier=false, summary=false) 3042 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 3043 protected SimpleQuantity quantity; 3044 3045 /** 3046 * If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group. 3047 */ 3048 @Child(name = "unitPrice", type = {Money.class}, order=14, min=0, max=1, modifier=false, summary=false) 3049 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group." ) 3050 protected Money unitPrice; 3051 3052 /** 3053 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3054 */ 3055 @Child(name = "factor", type = {DecimalType.class}, order=15, min=0, max=1, modifier=false, summary=false) 3056 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 3057 protected DecimalType factor; 3058 3059 /** 3060 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 3061 */ 3062 @Child(name = "net", type = {Money.class}, order=16, min=0, max=1, modifier=false, summary=false) 3063 @Description(shortDefinition="Total item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 3064 protected Money net; 3065 3066 /** 3067 * List of Unique Device Identifiers associated with this line item. 3068 */ 3069 @Child(name = "udi", type = {Device.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3070 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 3071 protected List<Reference> udi; 3072 /** 3073 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 3074 */ 3075 protected List<Device> udiTarget; 3076 3077 3078 /** 3079 * Physical service site on the patient (limb, tooth, etc). 3080 */ 3081 @Child(name = "bodySite", type = {CodeableConcept.class}, order=18, min=0, max=1, modifier=false, summary=false) 3082 @Description(shortDefinition="Service Location", formalDefinition="Physical service site on the patient (limb, tooth, etc)." ) 3083 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/tooth") 3084 protected CodeableConcept bodySite; 3085 3086 /** 3087 * A region or surface of the site, eg. limb region or tooth surface(s). 3088 */ 3089 @Child(name = "subSite", type = {CodeableConcept.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3090 @Description(shortDefinition="Service Sub-location", formalDefinition="A region or surface of the site, eg. limb region or tooth surface(s)." ) 3091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/surface") 3092 protected List<CodeableConcept> subSite; 3093 3094 /** 3095 * A billed item may include goods or services provided in multiple encounters. 3096 */ 3097 @Child(name = "encounter", type = {Encounter.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3098 @Description(shortDefinition="Encounters related to this billed item", formalDefinition="A billed item may include goods or services provided in multiple encounters." ) 3099 protected List<Reference> encounter; 3100 /** 3101 * The actual objects that are the target of the reference (A billed item may include goods or services provided in multiple encounters.) 3102 */ 3103 protected List<Encounter> encounterTarget; 3104 3105 3106 /** 3107 * A list of note references to the notes provided below. 3108 */ 3109 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3110 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 3111 protected List<PositiveIntType> noteNumber; 3112 3113 /** 3114 * The adjudications results. 3115 */ 3116 @Child(name = "adjudication", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3117 @Description(shortDefinition="Adjudication details", formalDefinition="The adjudications results." ) 3118 protected List<AdjudicationComponent> adjudication; 3119 3120 /** 3121 * Second tier of goods and services. 3122 */ 3123 @Child(name = "detail", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 3124 @Description(shortDefinition="Additional items", formalDefinition="Second tier of goods and services." ) 3125 protected List<DetailComponent> detail; 3126 3127 private static final long serialVersionUID = -1567825229L; 3128 3129 /** 3130 * Constructor 3131 */ 3132 public ItemComponent() { 3133 super(); 3134 } 3135 3136 /** 3137 * Constructor 3138 */ 3139 public ItemComponent(PositiveIntType sequence) { 3140 super(); 3141 this.sequence = sequence; 3142 } 3143 3144 /** 3145 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3146 */ 3147 public PositiveIntType getSequenceElement() { 3148 if (this.sequence == null) 3149 if (Configuration.errorOnAutoCreate()) 3150 throw new Error("Attempt to auto-create ItemComponent.sequence"); 3151 else if (Configuration.doAutoCreate()) 3152 this.sequence = new PositiveIntType(); // bb 3153 return this.sequence; 3154 } 3155 3156 public boolean hasSequenceElement() { 3157 return this.sequence != null && !this.sequence.isEmpty(); 3158 } 3159 3160 public boolean hasSequence() { 3161 return this.sequence != null && !this.sequence.isEmpty(); 3162 } 3163 3164 /** 3165 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 3166 */ 3167 public ItemComponent setSequenceElement(PositiveIntType value) { 3168 this.sequence = value; 3169 return this; 3170 } 3171 3172 /** 3173 * @return A service line number. 3174 */ 3175 public int getSequence() { 3176 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 3177 } 3178 3179 /** 3180 * @param value A service line number. 3181 */ 3182 public ItemComponent setSequence(int value) { 3183 if (this.sequence == null) 3184 this.sequence = new PositiveIntType(); 3185 this.sequence.setValue(value); 3186 return this; 3187 } 3188 3189 /** 3190 * @return {@link #careTeamLinkId} (Careteam applicable for this service or product line.) 3191 */ 3192 public List<PositiveIntType> getCareTeamLinkId() { 3193 if (this.careTeamLinkId == null) 3194 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3195 return this.careTeamLinkId; 3196 } 3197 3198 /** 3199 * @return Returns a reference to <code>this</code> for easy method chaining 3200 */ 3201 public ItemComponent setCareTeamLinkId(List<PositiveIntType> theCareTeamLinkId) { 3202 this.careTeamLinkId = theCareTeamLinkId; 3203 return this; 3204 } 3205 3206 public boolean hasCareTeamLinkId() { 3207 if (this.careTeamLinkId == null) 3208 return false; 3209 for (PositiveIntType item : this.careTeamLinkId) 3210 if (!item.isEmpty()) 3211 return true; 3212 return false; 3213 } 3214 3215 /** 3216 * @return {@link #careTeamLinkId} (Careteam applicable for this service or product line.) 3217 */ 3218 public PositiveIntType addCareTeamLinkIdElement() {//2 3219 PositiveIntType t = new PositiveIntType(); 3220 if (this.careTeamLinkId == null) 3221 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3222 this.careTeamLinkId.add(t); 3223 return t; 3224 } 3225 3226 /** 3227 * @param value {@link #careTeamLinkId} (Careteam applicable for this service or product line.) 3228 */ 3229 public ItemComponent addCareTeamLinkId(int value) { //1 3230 PositiveIntType t = new PositiveIntType(); 3231 t.setValue(value); 3232 if (this.careTeamLinkId == null) 3233 this.careTeamLinkId = new ArrayList<PositiveIntType>(); 3234 this.careTeamLinkId.add(t); 3235 return this; 3236 } 3237 3238 /** 3239 * @param value {@link #careTeamLinkId} (Careteam applicable for this service or product line.) 3240 */ 3241 public boolean hasCareTeamLinkId(int value) { 3242 if (this.careTeamLinkId == null) 3243 return false; 3244 for (PositiveIntType v : this.careTeamLinkId) 3245 if (v.getValue().equals(value)) // positiveInt 3246 return true; 3247 return false; 3248 } 3249 3250 /** 3251 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3252 */ 3253 public List<PositiveIntType> getDiagnosisLinkId() { 3254 if (this.diagnosisLinkId == null) 3255 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3256 return this.diagnosisLinkId; 3257 } 3258 3259 /** 3260 * @return Returns a reference to <code>this</code> for easy method chaining 3261 */ 3262 public ItemComponent setDiagnosisLinkId(List<PositiveIntType> theDiagnosisLinkId) { 3263 this.diagnosisLinkId = theDiagnosisLinkId; 3264 return this; 3265 } 3266 3267 public boolean hasDiagnosisLinkId() { 3268 if (this.diagnosisLinkId == null) 3269 return false; 3270 for (PositiveIntType item : this.diagnosisLinkId) 3271 if (!item.isEmpty()) 3272 return true; 3273 return false; 3274 } 3275 3276 /** 3277 * @return {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3278 */ 3279 public PositiveIntType addDiagnosisLinkIdElement() {//2 3280 PositiveIntType t = new PositiveIntType(); 3281 if (this.diagnosisLinkId == null) 3282 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3283 this.diagnosisLinkId.add(t); 3284 return t; 3285 } 3286 3287 /** 3288 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3289 */ 3290 public ItemComponent addDiagnosisLinkId(int value) { //1 3291 PositiveIntType t = new PositiveIntType(); 3292 t.setValue(value); 3293 if (this.diagnosisLinkId == null) 3294 this.diagnosisLinkId = new ArrayList<PositiveIntType>(); 3295 this.diagnosisLinkId.add(t); 3296 return this; 3297 } 3298 3299 /** 3300 * @param value {@link #diagnosisLinkId} (Diagnosis applicable for this service or product line.) 3301 */ 3302 public boolean hasDiagnosisLinkId(int value) { 3303 if (this.diagnosisLinkId == null) 3304 return false; 3305 for (PositiveIntType v : this.diagnosisLinkId) 3306 if (v.getValue().equals(value)) // positiveInt 3307 return true; 3308 return false; 3309 } 3310 3311 /** 3312 * @return {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3313 */ 3314 public List<PositiveIntType> getProcedureLinkId() { 3315 if (this.procedureLinkId == null) 3316 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3317 return this.procedureLinkId; 3318 } 3319 3320 /** 3321 * @return Returns a reference to <code>this</code> for easy method chaining 3322 */ 3323 public ItemComponent setProcedureLinkId(List<PositiveIntType> theProcedureLinkId) { 3324 this.procedureLinkId = theProcedureLinkId; 3325 return this; 3326 } 3327 3328 public boolean hasProcedureLinkId() { 3329 if (this.procedureLinkId == null) 3330 return false; 3331 for (PositiveIntType item : this.procedureLinkId) 3332 if (!item.isEmpty()) 3333 return true; 3334 return false; 3335 } 3336 3337 /** 3338 * @return {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3339 */ 3340 public PositiveIntType addProcedureLinkIdElement() {//2 3341 PositiveIntType t = new PositiveIntType(); 3342 if (this.procedureLinkId == null) 3343 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3344 this.procedureLinkId.add(t); 3345 return t; 3346 } 3347 3348 /** 3349 * @param value {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3350 */ 3351 public ItemComponent addProcedureLinkId(int value) { //1 3352 PositiveIntType t = new PositiveIntType(); 3353 t.setValue(value); 3354 if (this.procedureLinkId == null) 3355 this.procedureLinkId = new ArrayList<PositiveIntType>(); 3356 this.procedureLinkId.add(t); 3357 return this; 3358 } 3359 3360 /** 3361 * @param value {@link #procedureLinkId} (Procedures applicable for this service or product line.) 3362 */ 3363 public boolean hasProcedureLinkId(int value) { 3364 if (this.procedureLinkId == null) 3365 return false; 3366 for (PositiveIntType v : this.procedureLinkId) 3367 if (v.getValue().equals(value)) // positiveInt 3368 return true; 3369 return false; 3370 } 3371 3372 /** 3373 * @return {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3374 */ 3375 public List<PositiveIntType> getInformationLinkId() { 3376 if (this.informationLinkId == null) 3377 this.informationLinkId = new ArrayList<PositiveIntType>(); 3378 return this.informationLinkId; 3379 } 3380 3381 /** 3382 * @return Returns a reference to <code>this</code> for easy method chaining 3383 */ 3384 public ItemComponent setInformationLinkId(List<PositiveIntType> theInformationLinkId) { 3385 this.informationLinkId = theInformationLinkId; 3386 return this; 3387 } 3388 3389 public boolean hasInformationLinkId() { 3390 if (this.informationLinkId == null) 3391 return false; 3392 for (PositiveIntType item : this.informationLinkId) 3393 if (!item.isEmpty()) 3394 return true; 3395 return false; 3396 } 3397 3398 /** 3399 * @return {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3400 */ 3401 public PositiveIntType addInformationLinkIdElement() {//2 3402 PositiveIntType t = new PositiveIntType(); 3403 if (this.informationLinkId == null) 3404 this.informationLinkId = new ArrayList<PositiveIntType>(); 3405 this.informationLinkId.add(t); 3406 return t; 3407 } 3408 3409 /** 3410 * @param value {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3411 */ 3412 public ItemComponent addInformationLinkId(int value) { //1 3413 PositiveIntType t = new PositiveIntType(); 3414 t.setValue(value); 3415 if (this.informationLinkId == null) 3416 this.informationLinkId = new ArrayList<PositiveIntType>(); 3417 this.informationLinkId.add(t); 3418 return this; 3419 } 3420 3421 /** 3422 * @param value {@link #informationLinkId} (Exceptions, special conditions and supporting information pplicable for this service or product line.) 3423 */ 3424 public boolean hasInformationLinkId(int value) { 3425 if (this.informationLinkId == null) 3426 return false; 3427 for (PositiveIntType v : this.informationLinkId) 3428 if (v.getValue().equals(value)) // positiveInt 3429 return true; 3430 return false; 3431 } 3432 3433 /** 3434 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 3435 */ 3436 public CodeableConcept getRevenue() { 3437 if (this.revenue == null) 3438 if (Configuration.errorOnAutoCreate()) 3439 throw new Error("Attempt to auto-create ItemComponent.revenue"); 3440 else if (Configuration.doAutoCreate()) 3441 this.revenue = new CodeableConcept(); // cc 3442 return this.revenue; 3443 } 3444 3445 public boolean hasRevenue() { 3446 return this.revenue != null && !this.revenue.isEmpty(); 3447 } 3448 3449 /** 3450 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 3451 */ 3452 public ItemComponent setRevenue(CodeableConcept value) { 3453 this.revenue = value; 3454 return this; 3455 } 3456 3457 /** 3458 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 3459 */ 3460 public CodeableConcept getCategory() { 3461 if (this.category == null) 3462 if (Configuration.errorOnAutoCreate()) 3463 throw new Error("Attempt to auto-create ItemComponent.category"); 3464 else if (Configuration.doAutoCreate()) 3465 this.category = new CodeableConcept(); // cc 3466 return this.category; 3467 } 3468 3469 public boolean hasCategory() { 3470 return this.category != null && !this.category.isEmpty(); 3471 } 3472 3473 /** 3474 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 3475 */ 3476 public ItemComponent setCategory(CodeableConcept value) { 3477 this.category = value; 3478 return this; 3479 } 3480 3481 /** 3482 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 3483 */ 3484 public CodeableConcept getService() { 3485 if (this.service == null) 3486 if (Configuration.errorOnAutoCreate()) 3487 throw new Error("Attempt to auto-create ItemComponent.service"); 3488 else if (Configuration.doAutoCreate()) 3489 this.service = new CodeableConcept(); // cc 3490 return this.service; 3491 } 3492 3493 public boolean hasService() { 3494 return this.service != null && !this.service.isEmpty(); 3495 } 3496 3497 /** 3498 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 3499 */ 3500 public ItemComponent setService(CodeableConcept value) { 3501 this.service = value; 3502 return this; 3503 } 3504 3505 /** 3506 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 3507 */ 3508 public List<CodeableConcept> getModifier() { 3509 if (this.modifier == null) 3510 this.modifier = new ArrayList<CodeableConcept>(); 3511 return this.modifier; 3512 } 3513 3514 /** 3515 * @return Returns a reference to <code>this</code> for easy method chaining 3516 */ 3517 public ItemComponent setModifier(List<CodeableConcept> theModifier) { 3518 this.modifier = theModifier; 3519 return this; 3520 } 3521 3522 public boolean hasModifier() { 3523 if (this.modifier == null) 3524 return false; 3525 for (CodeableConcept item : this.modifier) 3526 if (!item.isEmpty()) 3527 return true; 3528 return false; 3529 } 3530 3531 public CodeableConcept addModifier() { //3 3532 CodeableConcept t = new CodeableConcept(); 3533 if (this.modifier == null) 3534 this.modifier = new ArrayList<CodeableConcept>(); 3535 this.modifier.add(t); 3536 return t; 3537 } 3538 3539 public ItemComponent addModifier(CodeableConcept t) { //3 3540 if (t == null) 3541 return this; 3542 if (this.modifier == null) 3543 this.modifier = new ArrayList<CodeableConcept>(); 3544 this.modifier.add(t); 3545 return this; 3546 } 3547 3548 /** 3549 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 3550 */ 3551 public CodeableConcept getModifierFirstRep() { 3552 if (getModifier().isEmpty()) { 3553 addModifier(); 3554 } 3555 return getModifier().get(0); 3556 } 3557 3558 /** 3559 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 3560 */ 3561 public List<CodeableConcept> getProgramCode() { 3562 if (this.programCode == null) 3563 this.programCode = new ArrayList<CodeableConcept>(); 3564 return this.programCode; 3565 } 3566 3567 /** 3568 * @return Returns a reference to <code>this</code> for easy method chaining 3569 */ 3570 public ItemComponent setProgramCode(List<CodeableConcept> theProgramCode) { 3571 this.programCode = theProgramCode; 3572 return this; 3573 } 3574 3575 public boolean hasProgramCode() { 3576 if (this.programCode == null) 3577 return false; 3578 for (CodeableConcept item : this.programCode) 3579 if (!item.isEmpty()) 3580 return true; 3581 return false; 3582 } 3583 3584 public CodeableConcept addProgramCode() { //3 3585 CodeableConcept t = new CodeableConcept(); 3586 if (this.programCode == null) 3587 this.programCode = new ArrayList<CodeableConcept>(); 3588 this.programCode.add(t); 3589 return t; 3590 } 3591 3592 public ItemComponent addProgramCode(CodeableConcept t) { //3 3593 if (t == null) 3594 return this; 3595 if (this.programCode == null) 3596 this.programCode = new ArrayList<CodeableConcept>(); 3597 this.programCode.add(t); 3598 return this; 3599 } 3600 3601 /** 3602 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 3603 */ 3604 public CodeableConcept getProgramCodeFirstRep() { 3605 if (getProgramCode().isEmpty()) { 3606 addProgramCode(); 3607 } 3608 return getProgramCode().get(0); 3609 } 3610 3611 /** 3612 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3613 */ 3614 public Type getServiced() { 3615 return this.serviced; 3616 } 3617 3618 /** 3619 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3620 */ 3621 public DateType getServicedDateType() throws FHIRException { 3622 if (this.serviced == null) 3623 return null; 3624 if (!(this.serviced instanceof DateType)) 3625 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.serviced.getClass().getName()+" was encountered"); 3626 return (DateType) this.serviced; 3627 } 3628 3629 public boolean hasServicedDateType() { 3630 return this != null && this.serviced instanceof DateType; 3631 } 3632 3633 /** 3634 * @return {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3635 */ 3636 public Period getServicedPeriod() throws FHIRException { 3637 if (this.serviced == null) 3638 return null; 3639 if (!(this.serviced instanceof Period)) 3640 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.serviced.getClass().getName()+" was encountered"); 3641 return (Period) this.serviced; 3642 } 3643 3644 public boolean hasServicedPeriod() { 3645 return this != null && this.serviced instanceof Period; 3646 } 3647 3648 public boolean hasServiced() { 3649 return this.serviced != null && !this.serviced.isEmpty(); 3650 } 3651 3652 /** 3653 * @param value {@link #serviced} (The date or dates when the enclosed suite of services were performed or completed.) 3654 */ 3655 public ItemComponent setServiced(Type value) throws FHIRFormatError { 3656 if (value != null && !(value instanceof DateType || value instanceof Period)) 3657 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.item.serviced[x]: "+value.fhirType()); 3658 this.serviced = value; 3659 return this; 3660 } 3661 3662 /** 3663 * @return {@link #location} (Where the service was provided.) 3664 */ 3665 public Type getLocation() { 3666 return this.location; 3667 } 3668 3669 /** 3670 * @return {@link #location} (Where the service was provided.) 3671 */ 3672 public CodeableConcept getLocationCodeableConcept() throws FHIRException { 3673 if (this.location == null) 3674 return null; 3675 if (!(this.location instanceof CodeableConcept)) 3676 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.location.getClass().getName()+" was encountered"); 3677 return (CodeableConcept) this.location; 3678 } 3679 3680 public boolean hasLocationCodeableConcept() { 3681 return this != null && this.location instanceof CodeableConcept; 3682 } 3683 3684 /** 3685 * @return {@link #location} (Where the service was provided.) 3686 */ 3687 public Address getLocationAddress() throws FHIRException { 3688 if (this.location == null) 3689 return null; 3690 if (!(this.location instanceof Address)) 3691 throw new FHIRException("Type mismatch: the type Address was expected, but "+this.location.getClass().getName()+" was encountered"); 3692 return (Address) this.location; 3693 } 3694 3695 public boolean hasLocationAddress() { 3696 return this != null && this.location instanceof Address; 3697 } 3698 3699 /** 3700 * @return {@link #location} (Where the service was provided.) 3701 */ 3702 public Reference getLocationReference() throws FHIRException { 3703 if (this.location == null) 3704 return null; 3705 if (!(this.location instanceof Reference)) 3706 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.location.getClass().getName()+" was encountered"); 3707 return (Reference) this.location; 3708 } 3709 3710 public boolean hasLocationReference() { 3711 return this != null && this.location instanceof Reference; 3712 } 3713 3714 public boolean hasLocation() { 3715 return this.location != null && !this.location.isEmpty(); 3716 } 3717 3718 /** 3719 * @param value {@link #location} (Where the service was provided.) 3720 */ 3721 public ItemComponent setLocation(Type value) throws FHIRFormatError { 3722 if (value != null && !(value instanceof CodeableConcept || value instanceof Address || value instanceof Reference)) 3723 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.item.location[x]: "+value.fhirType()); 3724 this.location = value; 3725 return this; 3726 } 3727 3728 /** 3729 * @return {@link #quantity} (The number of repetitions of a service or product.) 3730 */ 3731 public SimpleQuantity getQuantity() { 3732 if (this.quantity == null) 3733 if (Configuration.errorOnAutoCreate()) 3734 throw new Error("Attempt to auto-create ItemComponent.quantity"); 3735 else if (Configuration.doAutoCreate()) 3736 this.quantity = new SimpleQuantity(); // cc 3737 return this.quantity; 3738 } 3739 3740 public boolean hasQuantity() { 3741 return this.quantity != null && !this.quantity.isEmpty(); 3742 } 3743 3744 /** 3745 * @param value {@link #quantity} (The number of repetitions of a service or product.) 3746 */ 3747 public ItemComponent setQuantity(SimpleQuantity value) { 3748 this.quantity = value; 3749 return this; 3750 } 3751 3752 /** 3753 * @return {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 3754 */ 3755 public Money getUnitPrice() { 3756 if (this.unitPrice == null) 3757 if (Configuration.errorOnAutoCreate()) 3758 throw new Error("Attempt to auto-create ItemComponent.unitPrice"); 3759 else if (Configuration.doAutoCreate()) 3760 this.unitPrice = new Money(); // cc 3761 return this.unitPrice; 3762 } 3763 3764 public boolean hasUnitPrice() { 3765 return this.unitPrice != null && !this.unitPrice.isEmpty(); 3766 } 3767 3768 /** 3769 * @param value {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 3770 */ 3771 public ItemComponent setUnitPrice(Money value) { 3772 this.unitPrice = value; 3773 return this; 3774 } 3775 3776 /** 3777 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 3778 */ 3779 public DecimalType getFactorElement() { 3780 if (this.factor == null) 3781 if (Configuration.errorOnAutoCreate()) 3782 throw new Error("Attempt to auto-create ItemComponent.factor"); 3783 else if (Configuration.doAutoCreate()) 3784 this.factor = new DecimalType(); // bb 3785 return this.factor; 3786 } 3787 3788 public boolean hasFactorElement() { 3789 return this.factor != null && !this.factor.isEmpty(); 3790 } 3791 3792 public boolean hasFactor() { 3793 return this.factor != null && !this.factor.isEmpty(); 3794 } 3795 3796 /** 3797 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 3798 */ 3799 public ItemComponent setFactorElement(DecimalType value) { 3800 this.factor = value; 3801 return this; 3802 } 3803 3804 /** 3805 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3806 */ 3807 public BigDecimal getFactor() { 3808 return this.factor == null ? null : this.factor.getValue(); 3809 } 3810 3811 /** 3812 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3813 */ 3814 public ItemComponent setFactor(BigDecimal value) { 3815 if (value == null) 3816 this.factor = null; 3817 else { 3818 if (this.factor == null) 3819 this.factor = new DecimalType(); 3820 this.factor.setValue(value); 3821 } 3822 return this; 3823 } 3824 3825 /** 3826 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3827 */ 3828 public ItemComponent setFactor(long value) { 3829 this.factor = new DecimalType(); 3830 this.factor.setValue(value); 3831 return this; 3832 } 3833 3834 /** 3835 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 3836 */ 3837 public ItemComponent setFactor(double value) { 3838 this.factor = new DecimalType(); 3839 this.factor.setValue(value); 3840 return this; 3841 } 3842 3843 /** 3844 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 3845 */ 3846 public Money getNet() { 3847 if (this.net == null) 3848 if (Configuration.errorOnAutoCreate()) 3849 throw new Error("Attempt to auto-create ItemComponent.net"); 3850 else if (Configuration.doAutoCreate()) 3851 this.net = new Money(); // cc 3852 return this.net; 3853 } 3854 3855 public boolean hasNet() { 3856 return this.net != null && !this.net.isEmpty(); 3857 } 3858 3859 /** 3860 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 3861 */ 3862 public ItemComponent setNet(Money value) { 3863 this.net = value; 3864 return this; 3865 } 3866 3867 /** 3868 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 3869 */ 3870 public List<Reference> getUdi() { 3871 if (this.udi == null) 3872 this.udi = new ArrayList<Reference>(); 3873 return this.udi; 3874 } 3875 3876 /** 3877 * @return Returns a reference to <code>this</code> for easy method chaining 3878 */ 3879 public ItemComponent setUdi(List<Reference> theUdi) { 3880 this.udi = theUdi; 3881 return this; 3882 } 3883 3884 public boolean hasUdi() { 3885 if (this.udi == null) 3886 return false; 3887 for (Reference item : this.udi) 3888 if (!item.isEmpty()) 3889 return true; 3890 return false; 3891 } 3892 3893 public Reference addUdi() { //3 3894 Reference t = new Reference(); 3895 if (this.udi == null) 3896 this.udi = new ArrayList<Reference>(); 3897 this.udi.add(t); 3898 return t; 3899 } 3900 3901 public ItemComponent addUdi(Reference t) { //3 3902 if (t == null) 3903 return this; 3904 if (this.udi == null) 3905 this.udi = new ArrayList<Reference>(); 3906 this.udi.add(t); 3907 return this; 3908 } 3909 3910 /** 3911 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 3912 */ 3913 public Reference getUdiFirstRep() { 3914 if (getUdi().isEmpty()) { 3915 addUdi(); 3916 } 3917 return getUdi().get(0); 3918 } 3919 3920 /** 3921 * @deprecated Use Reference#setResource(IBaseResource) instead 3922 */ 3923 @Deprecated 3924 public List<Device> getUdiTarget() { 3925 if (this.udiTarget == null) 3926 this.udiTarget = new ArrayList<Device>(); 3927 return this.udiTarget; 3928 } 3929 3930 /** 3931 * @deprecated Use Reference#setResource(IBaseResource) instead 3932 */ 3933 @Deprecated 3934 public Device addUdiTarget() { 3935 Device r = new Device(); 3936 if (this.udiTarget == null) 3937 this.udiTarget = new ArrayList<Device>(); 3938 this.udiTarget.add(r); 3939 return r; 3940 } 3941 3942 /** 3943 * @return {@link #bodySite} (Physical service site on the patient (limb, tooth, etc).) 3944 */ 3945 public CodeableConcept getBodySite() { 3946 if (this.bodySite == null) 3947 if (Configuration.errorOnAutoCreate()) 3948 throw new Error("Attempt to auto-create ItemComponent.bodySite"); 3949 else if (Configuration.doAutoCreate()) 3950 this.bodySite = new CodeableConcept(); // cc 3951 return this.bodySite; 3952 } 3953 3954 public boolean hasBodySite() { 3955 return this.bodySite != null && !this.bodySite.isEmpty(); 3956 } 3957 3958 /** 3959 * @param value {@link #bodySite} (Physical service site on the patient (limb, tooth, etc).) 3960 */ 3961 public ItemComponent setBodySite(CodeableConcept value) { 3962 this.bodySite = value; 3963 return this; 3964 } 3965 3966 /** 3967 * @return {@link #subSite} (A region or surface of the site, eg. limb region or tooth surface(s).) 3968 */ 3969 public List<CodeableConcept> getSubSite() { 3970 if (this.subSite == null) 3971 this.subSite = new ArrayList<CodeableConcept>(); 3972 return this.subSite; 3973 } 3974 3975 /** 3976 * @return Returns a reference to <code>this</code> for easy method chaining 3977 */ 3978 public ItemComponent setSubSite(List<CodeableConcept> theSubSite) { 3979 this.subSite = theSubSite; 3980 return this; 3981 } 3982 3983 public boolean hasSubSite() { 3984 if (this.subSite == null) 3985 return false; 3986 for (CodeableConcept item : this.subSite) 3987 if (!item.isEmpty()) 3988 return true; 3989 return false; 3990 } 3991 3992 public CodeableConcept addSubSite() { //3 3993 CodeableConcept t = new CodeableConcept(); 3994 if (this.subSite == null) 3995 this.subSite = new ArrayList<CodeableConcept>(); 3996 this.subSite.add(t); 3997 return t; 3998 } 3999 4000 public ItemComponent addSubSite(CodeableConcept t) { //3 4001 if (t == null) 4002 return this; 4003 if (this.subSite == null) 4004 this.subSite = new ArrayList<CodeableConcept>(); 4005 this.subSite.add(t); 4006 return this; 4007 } 4008 4009 /** 4010 * @return The first repetition of repeating field {@link #subSite}, creating it if it does not already exist 4011 */ 4012 public CodeableConcept getSubSiteFirstRep() { 4013 if (getSubSite().isEmpty()) { 4014 addSubSite(); 4015 } 4016 return getSubSite().get(0); 4017 } 4018 4019 /** 4020 * @return {@link #encounter} (A billed item may include goods or services provided in multiple encounters.) 4021 */ 4022 public List<Reference> getEncounter() { 4023 if (this.encounter == null) 4024 this.encounter = new ArrayList<Reference>(); 4025 return this.encounter; 4026 } 4027 4028 /** 4029 * @return Returns a reference to <code>this</code> for easy method chaining 4030 */ 4031 public ItemComponent setEncounter(List<Reference> theEncounter) { 4032 this.encounter = theEncounter; 4033 return this; 4034 } 4035 4036 public boolean hasEncounter() { 4037 if (this.encounter == null) 4038 return false; 4039 for (Reference item : this.encounter) 4040 if (!item.isEmpty()) 4041 return true; 4042 return false; 4043 } 4044 4045 public Reference addEncounter() { //3 4046 Reference t = new Reference(); 4047 if (this.encounter == null) 4048 this.encounter = new ArrayList<Reference>(); 4049 this.encounter.add(t); 4050 return t; 4051 } 4052 4053 public ItemComponent addEncounter(Reference t) { //3 4054 if (t == null) 4055 return this; 4056 if (this.encounter == null) 4057 this.encounter = new ArrayList<Reference>(); 4058 this.encounter.add(t); 4059 return this; 4060 } 4061 4062 /** 4063 * @return The first repetition of repeating field {@link #encounter}, creating it if it does not already exist 4064 */ 4065 public Reference getEncounterFirstRep() { 4066 if (getEncounter().isEmpty()) { 4067 addEncounter(); 4068 } 4069 return getEncounter().get(0); 4070 } 4071 4072 /** 4073 * @deprecated Use Reference#setResource(IBaseResource) instead 4074 */ 4075 @Deprecated 4076 public List<Encounter> getEncounterTarget() { 4077 if (this.encounterTarget == null) 4078 this.encounterTarget = new ArrayList<Encounter>(); 4079 return this.encounterTarget; 4080 } 4081 4082 /** 4083 * @deprecated Use Reference#setResource(IBaseResource) instead 4084 */ 4085 @Deprecated 4086 public Encounter addEncounterTarget() { 4087 Encounter r = new Encounter(); 4088 if (this.encounterTarget == null) 4089 this.encounterTarget = new ArrayList<Encounter>(); 4090 this.encounterTarget.add(r); 4091 return r; 4092 } 4093 4094 /** 4095 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 4096 */ 4097 public List<PositiveIntType> getNoteNumber() { 4098 if (this.noteNumber == null) 4099 this.noteNumber = new ArrayList<PositiveIntType>(); 4100 return this.noteNumber; 4101 } 4102 4103 /** 4104 * @return Returns a reference to <code>this</code> for easy method chaining 4105 */ 4106 public ItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 4107 this.noteNumber = theNoteNumber; 4108 return this; 4109 } 4110 4111 public boolean hasNoteNumber() { 4112 if (this.noteNumber == null) 4113 return false; 4114 for (PositiveIntType item : this.noteNumber) 4115 if (!item.isEmpty()) 4116 return true; 4117 return false; 4118 } 4119 4120 /** 4121 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 4122 */ 4123 public PositiveIntType addNoteNumberElement() {//2 4124 PositiveIntType t = new PositiveIntType(); 4125 if (this.noteNumber == null) 4126 this.noteNumber = new ArrayList<PositiveIntType>(); 4127 this.noteNumber.add(t); 4128 return t; 4129 } 4130 4131 /** 4132 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 4133 */ 4134 public ItemComponent addNoteNumber(int value) { //1 4135 PositiveIntType t = new PositiveIntType(); 4136 t.setValue(value); 4137 if (this.noteNumber == null) 4138 this.noteNumber = new ArrayList<PositiveIntType>(); 4139 this.noteNumber.add(t); 4140 return this; 4141 } 4142 4143 /** 4144 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 4145 */ 4146 public boolean hasNoteNumber(int value) { 4147 if (this.noteNumber == null) 4148 return false; 4149 for (PositiveIntType v : this.noteNumber) 4150 if (v.getValue().equals(value)) // positiveInt 4151 return true; 4152 return false; 4153 } 4154 4155 /** 4156 * @return {@link #adjudication} (The adjudications results.) 4157 */ 4158 public List<AdjudicationComponent> getAdjudication() { 4159 if (this.adjudication == null) 4160 this.adjudication = new ArrayList<AdjudicationComponent>(); 4161 return this.adjudication; 4162 } 4163 4164 /** 4165 * @return Returns a reference to <code>this</code> for easy method chaining 4166 */ 4167 public ItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 4168 this.adjudication = theAdjudication; 4169 return this; 4170 } 4171 4172 public boolean hasAdjudication() { 4173 if (this.adjudication == null) 4174 return false; 4175 for (AdjudicationComponent item : this.adjudication) 4176 if (!item.isEmpty()) 4177 return true; 4178 return false; 4179 } 4180 4181 public AdjudicationComponent addAdjudication() { //3 4182 AdjudicationComponent t = new AdjudicationComponent(); 4183 if (this.adjudication == null) 4184 this.adjudication = new ArrayList<AdjudicationComponent>(); 4185 this.adjudication.add(t); 4186 return t; 4187 } 4188 4189 public ItemComponent addAdjudication(AdjudicationComponent t) { //3 4190 if (t == null) 4191 return this; 4192 if (this.adjudication == null) 4193 this.adjudication = new ArrayList<AdjudicationComponent>(); 4194 this.adjudication.add(t); 4195 return this; 4196 } 4197 4198 /** 4199 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 4200 */ 4201 public AdjudicationComponent getAdjudicationFirstRep() { 4202 if (getAdjudication().isEmpty()) { 4203 addAdjudication(); 4204 } 4205 return getAdjudication().get(0); 4206 } 4207 4208 /** 4209 * @return {@link #detail} (Second tier of goods and services.) 4210 */ 4211 public List<DetailComponent> getDetail() { 4212 if (this.detail == null) 4213 this.detail = new ArrayList<DetailComponent>(); 4214 return this.detail; 4215 } 4216 4217 /** 4218 * @return Returns a reference to <code>this</code> for easy method chaining 4219 */ 4220 public ItemComponent setDetail(List<DetailComponent> theDetail) { 4221 this.detail = theDetail; 4222 return this; 4223 } 4224 4225 public boolean hasDetail() { 4226 if (this.detail == null) 4227 return false; 4228 for (DetailComponent item : this.detail) 4229 if (!item.isEmpty()) 4230 return true; 4231 return false; 4232 } 4233 4234 public DetailComponent addDetail() { //3 4235 DetailComponent t = new DetailComponent(); 4236 if (this.detail == null) 4237 this.detail = new ArrayList<DetailComponent>(); 4238 this.detail.add(t); 4239 return t; 4240 } 4241 4242 public ItemComponent addDetail(DetailComponent t) { //3 4243 if (t == null) 4244 return this; 4245 if (this.detail == null) 4246 this.detail = new ArrayList<DetailComponent>(); 4247 this.detail.add(t); 4248 return this; 4249 } 4250 4251 /** 4252 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 4253 */ 4254 public DetailComponent getDetailFirstRep() { 4255 if (getDetail().isEmpty()) { 4256 addDetail(); 4257 } 4258 return getDetail().get(0); 4259 } 4260 4261 protected void listChildren(List<Property> children) { 4262 super.listChildren(children); 4263 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 4264 children.add(new Property("careTeamLinkId", "positiveInt", "Careteam applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, careTeamLinkId)); 4265 children.add(new Property("diagnosisLinkId", "positiveInt", "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId)); 4266 children.add(new Property("procedureLinkId", "positiveInt", "Procedures applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, procedureLinkId)); 4267 children.add(new Property("informationLinkId", "positiveInt", "Exceptions, special conditions and supporting information pplicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, informationLinkId)); 4268 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 4269 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 4270 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 4271 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 4272 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 4273 children.add(new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced)); 4274 children.add(new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location)); 4275 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 4276 children.add(new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice)); 4277 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 4278 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 4279 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 4280 children.add(new Property("bodySite", "CodeableConcept", "Physical service site on the patient (limb, tooth, etc).", 0, 1, bodySite)); 4281 children.add(new Property("subSite", "CodeableConcept", "A region or surface of the site, eg. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite)); 4282 children.add(new Property("encounter", "Reference(Encounter)", "A billed item may include goods or services provided in multiple encounters.", 0, java.lang.Integer.MAX_VALUE, encounter)); 4283 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 4284 children.add(new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 4285 children.add(new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail)); 4286 } 4287 4288 @Override 4289 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4290 switch (_hash) { 4291 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 4292 case -186757789: /*careTeamLinkId*/ return new Property("careTeamLinkId", "positiveInt", "Careteam applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, careTeamLinkId); 4293 case -1659207418: /*diagnosisLinkId*/ return new Property("diagnosisLinkId", "positiveInt", "Diagnosis applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, diagnosisLinkId); 4294 case -532846744: /*procedureLinkId*/ return new Property("procedureLinkId", "positiveInt", "Procedures applicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, procedureLinkId); 4295 case 1965585153: /*informationLinkId*/ return new Property("informationLinkId", "positiveInt", "Exceptions, special conditions and supporting information pplicable for this service or product line.", 0, java.lang.Integer.MAX_VALUE, informationLinkId); 4296 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 4297 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 4298 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 4299 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 4300 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 4301 case -1927922223: /*serviced[x]*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4302 case 1379209295: /*serviced*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4303 case 363246749: /*servicedDate*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4304 case 1534966512: /*servicedPeriod*/ return new Property("serviced[x]", "date|Period", "The date or dates when the enclosed suite of services were performed or completed.", 0, 1, serviced); 4305 case 552316075: /*location[x]*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4306 case 1901043637: /*location*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4307 case -1224800468: /*locationCodeableConcept*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4308 case -1280020865: /*locationAddress*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4309 case 755866390: /*locationReference*/ return new Property("location[x]", "CodeableConcept|Address|Reference(Location)", "Where the service was provided.", 0, 1, location); 4310 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 4311 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice); 4312 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 4313 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 4314 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 4315 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Physical service site on the patient (limb, tooth, etc).", 0, 1, bodySite); 4316 case -1868566105: /*subSite*/ return new Property("subSite", "CodeableConcept", "A region or surface of the site, eg. limb region or tooth surface(s).", 0, java.lang.Integer.MAX_VALUE, subSite); 4317 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "A billed item may include goods or services provided in multiple encounters.", 0, java.lang.Integer.MAX_VALUE, encounter); 4318 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 4319 case -231349275: /*adjudication*/ return new Property("adjudication", "", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 4320 case -1335224239: /*detail*/ return new Property("detail", "", "Second tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, detail); 4321 default: return super.getNamedProperty(_hash, _name, _checkValid); 4322 } 4323 4324 } 4325 4326 @Override 4327 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4328 switch (hash) { 4329 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 4330 case -186757789: /*careTeamLinkId*/ return this.careTeamLinkId == null ? new Base[0] : this.careTeamLinkId.toArray(new Base[this.careTeamLinkId.size()]); // PositiveIntType 4331 case -1659207418: /*diagnosisLinkId*/ return this.diagnosisLinkId == null ? new Base[0] : this.diagnosisLinkId.toArray(new Base[this.diagnosisLinkId.size()]); // PositiveIntType 4332 case -532846744: /*procedureLinkId*/ return this.procedureLinkId == null ? new Base[0] : this.procedureLinkId.toArray(new Base[this.procedureLinkId.size()]); // PositiveIntType 4333 case 1965585153: /*informationLinkId*/ return this.informationLinkId == null ? new Base[0] : this.informationLinkId.toArray(new Base[this.informationLinkId.size()]); // PositiveIntType 4334 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 4335 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 4336 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 4337 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 4338 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 4339 case 1379209295: /*serviced*/ return this.serviced == null ? new Base[0] : new Base[] {this.serviced}; // Type 4340 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Type 4341 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 4342 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 4343 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 4344 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 4345 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 4346 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 4347 case -1868566105: /*subSite*/ return this.subSite == null ? new Base[0] : this.subSite.toArray(new Base[this.subSite.size()]); // CodeableConcept 4348 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : this.encounter.toArray(new Base[this.encounter.size()]); // Reference 4349 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 4350 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 4351 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // DetailComponent 4352 default: return super.getProperty(hash, name, checkValid); 4353 } 4354 4355 } 4356 4357 @Override 4358 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4359 switch (hash) { 4360 case 1349547969: // sequence 4361 this.sequence = castToPositiveInt(value); // PositiveIntType 4362 return value; 4363 case -186757789: // careTeamLinkId 4364 this.getCareTeamLinkId().add(castToPositiveInt(value)); // PositiveIntType 4365 return value; 4366 case -1659207418: // diagnosisLinkId 4367 this.getDiagnosisLinkId().add(castToPositiveInt(value)); // PositiveIntType 4368 return value; 4369 case -532846744: // procedureLinkId 4370 this.getProcedureLinkId().add(castToPositiveInt(value)); // PositiveIntType 4371 return value; 4372 case 1965585153: // informationLinkId 4373 this.getInformationLinkId().add(castToPositiveInt(value)); // PositiveIntType 4374 return value; 4375 case 1099842588: // revenue 4376 this.revenue = castToCodeableConcept(value); // CodeableConcept 4377 return value; 4378 case 50511102: // category 4379 this.category = castToCodeableConcept(value); // CodeableConcept 4380 return value; 4381 case 1984153269: // service 4382 this.service = castToCodeableConcept(value); // CodeableConcept 4383 return value; 4384 case -615513385: // modifier 4385 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 4386 return value; 4387 case 1010065041: // programCode 4388 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 4389 return value; 4390 case 1379209295: // serviced 4391 this.serviced = castToType(value); // Type 4392 return value; 4393 case 1901043637: // location 4394 this.location = castToType(value); // Type 4395 return value; 4396 case -1285004149: // quantity 4397 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4398 return value; 4399 case -486196699: // unitPrice 4400 this.unitPrice = castToMoney(value); // Money 4401 return value; 4402 case -1282148017: // factor 4403 this.factor = castToDecimal(value); // DecimalType 4404 return value; 4405 case 108957: // net 4406 this.net = castToMoney(value); // Money 4407 return value; 4408 case 115642: // udi 4409 this.getUdi().add(castToReference(value)); // Reference 4410 return value; 4411 case 1702620169: // bodySite 4412 this.bodySite = castToCodeableConcept(value); // CodeableConcept 4413 return value; 4414 case -1868566105: // subSite 4415 this.getSubSite().add(castToCodeableConcept(value)); // CodeableConcept 4416 return value; 4417 case 1524132147: // encounter 4418 this.getEncounter().add(castToReference(value)); // Reference 4419 return value; 4420 case -1110033957: // noteNumber 4421 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 4422 return value; 4423 case -231349275: // adjudication 4424 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 4425 return value; 4426 case -1335224239: // detail 4427 this.getDetail().add((DetailComponent) value); // DetailComponent 4428 return value; 4429 default: return super.setProperty(hash, name, value); 4430 } 4431 4432 } 4433 4434 @Override 4435 public Base setProperty(String name, Base value) throws FHIRException { 4436 if (name.equals("sequence")) { 4437 this.sequence = castToPositiveInt(value); // PositiveIntType 4438 } else if (name.equals("careTeamLinkId")) { 4439 this.getCareTeamLinkId().add(castToPositiveInt(value)); 4440 } else if (name.equals("diagnosisLinkId")) { 4441 this.getDiagnosisLinkId().add(castToPositiveInt(value)); 4442 } else if (name.equals("procedureLinkId")) { 4443 this.getProcedureLinkId().add(castToPositiveInt(value)); 4444 } else if (name.equals("informationLinkId")) { 4445 this.getInformationLinkId().add(castToPositiveInt(value)); 4446 } else if (name.equals("revenue")) { 4447 this.revenue = castToCodeableConcept(value); // CodeableConcept 4448 } else if (name.equals("category")) { 4449 this.category = castToCodeableConcept(value); // CodeableConcept 4450 } else if (name.equals("service")) { 4451 this.service = castToCodeableConcept(value); // CodeableConcept 4452 } else if (name.equals("modifier")) { 4453 this.getModifier().add(castToCodeableConcept(value)); 4454 } else if (name.equals("programCode")) { 4455 this.getProgramCode().add(castToCodeableConcept(value)); 4456 } else if (name.equals("serviced[x]")) { 4457 this.serviced = castToType(value); // Type 4458 } else if (name.equals("location[x]")) { 4459 this.location = castToType(value); // Type 4460 } else if (name.equals("quantity")) { 4461 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 4462 } else if (name.equals("unitPrice")) { 4463 this.unitPrice = castToMoney(value); // Money 4464 } else if (name.equals("factor")) { 4465 this.factor = castToDecimal(value); // DecimalType 4466 } else if (name.equals("net")) { 4467 this.net = castToMoney(value); // Money 4468 } else if (name.equals("udi")) { 4469 this.getUdi().add(castToReference(value)); 4470 } else if (name.equals("bodySite")) { 4471 this.bodySite = castToCodeableConcept(value); // CodeableConcept 4472 } else if (name.equals("subSite")) { 4473 this.getSubSite().add(castToCodeableConcept(value)); 4474 } else if (name.equals("encounter")) { 4475 this.getEncounter().add(castToReference(value)); 4476 } else if (name.equals("noteNumber")) { 4477 this.getNoteNumber().add(castToPositiveInt(value)); 4478 } else if (name.equals("adjudication")) { 4479 this.getAdjudication().add((AdjudicationComponent) value); 4480 } else if (name.equals("detail")) { 4481 this.getDetail().add((DetailComponent) value); 4482 } else 4483 return super.setProperty(name, value); 4484 return value; 4485 } 4486 4487 @Override 4488 public Base makeProperty(int hash, String name) throws FHIRException { 4489 switch (hash) { 4490 case 1349547969: return getSequenceElement(); 4491 case -186757789: return addCareTeamLinkIdElement(); 4492 case -1659207418: return addDiagnosisLinkIdElement(); 4493 case -532846744: return addProcedureLinkIdElement(); 4494 case 1965585153: return addInformationLinkIdElement(); 4495 case 1099842588: return getRevenue(); 4496 case 50511102: return getCategory(); 4497 case 1984153269: return getService(); 4498 case -615513385: return addModifier(); 4499 case 1010065041: return addProgramCode(); 4500 case -1927922223: return getServiced(); 4501 case 1379209295: return getServiced(); 4502 case 552316075: return getLocation(); 4503 case 1901043637: return getLocation(); 4504 case -1285004149: return getQuantity(); 4505 case -486196699: return getUnitPrice(); 4506 case -1282148017: return getFactorElement(); 4507 case 108957: return getNet(); 4508 case 115642: return addUdi(); 4509 case 1702620169: return getBodySite(); 4510 case -1868566105: return addSubSite(); 4511 case 1524132147: return addEncounter(); 4512 case -1110033957: return addNoteNumberElement(); 4513 case -231349275: return addAdjudication(); 4514 case -1335224239: return addDetail(); 4515 default: return super.makeProperty(hash, name); 4516 } 4517 4518 } 4519 4520 @Override 4521 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 4522 switch (hash) { 4523 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 4524 case -186757789: /*careTeamLinkId*/ return new String[] {"positiveInt"}; 4525 case -1659207418: /*diagnosisLinkId*/ return new String[] {"positiveInt"}; 4526 case -532846744: /*procedureLinkId*/ return new String[] {"positiveInt"}; 4527 case 1965585153: /*informationLinkId*/ return new String[] {"positiveInt"}; 4528 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 4529 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 4530 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 4531 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 4532 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 4533 case 1379209295: /*serviced*/ return new String[] {"date", "Period"}; 4534 case 1901043637: /*location*/ return new String[] {"CodeableConcept", "Address", "Reference"}; 4535 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 4536 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 4537 case -1282148017: /*factor*/ return new String[] {"decimal"}; 4538 case 108957: /*net*/ return new String[] {"Money"}; 4539 case 115642: /*udi*/ return new String[] {"Reference"}; 4540 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 4541 case -1868566105: /*subSite*/ return new String[] {"CodeableConcept"}; 4542 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 4543 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 4544 case -231349275: /*adjudication*/ return new String[] {}; 4545 case -1335224239: /*detail*/ return new String[] {}; 4546 default: return super.getTypesForProperty(hash, name); 4547 } 4548 4549 } 4550 4551 @Override 4552 public Base addChild(String name) throws FHIRException { 4553 if (name.equals("sequence")) { 4554 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 4555 } 4556 else if (name.equals("careTeamLinkId")) { 4557 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.careTeamLinkId"); 4558 } 4559 else if (name.equals("diagnosisLinkId")) { 4560 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.diagnosisLinkId"); 4561 } 4562 else if (name.equals("procedureLinkId")) { 4563 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.procedureLinkId"); 4564 } 4565 else if (name.equals("informationLinkId")) { 4566 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.informationLinkId"); 4567 } 4568 else if (name.equals("revenue")) { 4569 this.revenue = new CodeableConcept(); 4570 return this.revenue; 4571 } 4572 else if (name.equals("category")) { 4573 this.category = new CodeableConcept(); 4574 return this.category; 4575 } 4576 else if (name.equals("service")) { 4577 this.service = new CodeableConcept(); 4578 return this.service; 4579 } 4580 else if (name.equals("modifier")) { 4581 return addModifier(); 4582 } 4583 else if (name.equals("programCode")) { 4584 return addProgramCode(); 4585 } 4586 else if (name.equals("servicedDate")) { 4587 this.serviced = new DateType(); 4588 return this.serviced; 4589 } 4590 else if (name.equals("servicedPeriod")) { 4591 this.serviced = new Period(); 4592 return this.serviced; 4593 } 4594 else if (name.equals("locationCodeableConcept")) { 4595 this.location = new CodeableConcept(); 4596 return this.location; 4597 } 4598 else if (name.equals("locationAddress")) { 4599 this.location = new Address(); 4600 return this.location; 4601 } 4602 else if (name.equals("locationReference")) { 4603 this.location = new Reference(); 4604 return this.location; 4605 } 4606 else if (name.equals("quantity")) { 4607 this.quantity = new SimpleQuantity(); 4608 return this.quantity; 4609 } 4610 else if (name.equals("unitPrice")) { 4611 this.unitPrice = new Money(); 4612 return this.unitPrice; 4613 } 4614 else if (name.equals("factor")) { 4615 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 4616 } 4617 else if (name.equals("net")) { 4618 this.net = new Money(); 4619 return this.net; 4620 } 4621 else if (name.equals("udi")) { 4622 return addUdi(); 4623 } 4624 else if (name.equals("bodySite")) { 4625 this.bodySite = new CodeableConcept(); 4626 return this.bodySite; 4627 } 4628 else if (name.equals("subSite")) { 4629 return addSubSite(); 4630 } 4631 else if (name.equals("encounter")) { 4632 return addEncounter(); 4633 } 4634 else if (name.equals("noteNumber")) { 4635 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 4636 } 4637 else if (name.equals("adjudication")) { 4638 return addAdjudication(); 4639 } 4640 else if (name.equals("detail")) { 4641 return addDetail(); 4642 } 4643 else 4644 return super.addChild(name); 4645 } 4646 4647 public ItemComponent copy() { 4648 ItemComponent dst = new ItemComponent(); 4649 copyValues(dst); 4650 dst.sequence = sequence == null ? null : sequence.copy(); 4651 if (careTeamLinkId != null) { 4652 dst.careTeamLinkId = new ArrayList<PositiveIntType>(); 4653 for (PositiveIntType i : careTeamLinkId) 4654 dst.careTeamLinkId.add(i.copy()); 4655 }; 4656 if (diagnosisLinkId != null) { 4657 dst.diagnosisLinkId = new ArrayList<PositiveIntType>(); 4658 for (PositiveIntType i : diagnosisLinkId) 4659 dst.diagnosisLinkId.add(i.copy()); 4660 }; 4661 if (procedureLinkId != null) { 4662 dst.procedureLinkId = new ArrayList<PositiveIntType>(); 4663 for (PositiveIntType i : procedureLinkId) 4664 dst.procedureLinkId.add(i.copy()); 4665 }; 4666 if (informationLinkId != null) { 4667 dst.informationLinkId = new ArrayList<PositiveIntType>(); 4668 for (PositiveIntType i : informationLinkId) 4669 dst.informationLinkId.add(i.copy()); 4670 }; 4671 dst.revenue = revenue == null ? null : revenue.copy(); 4672 dst.category = category == null ? null : category.copy(); 4673 dst.service = service == null ? null : service.copy(); 4674 if (modifier != null) { 4675 dst.modifier = new ArrayList<CodeableConcept>(); 4676 for (CodeableConcept i : modifier) 4677 dst.modifier.add(i.copy()); 4678 }; 4679 if (programCode != null) { 4680 dst.programCode = new ArrayList<CodeableConcept>(); 4681 for (CodeableConcept i : programCode) 4682 dst.programCode.add(i.copy()); 4683 }; 4684 dst.serviced = serviced == null ? null : serviced.copy(); 4685 dst.location = location == null ? null : location.copy(); 4686 dst.quantity = quantity == null ? null : quantity.copy(); 4687 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 4688 dst.factor = factor == null ? null : factor.copy(); 4689 dst.net = net == null ? null : net.copy(); 4690 if (udi != null) { 4691 dst.udi = new ArrayList<Reference>(); 4692 for (Reference i : udi) 4693 dst.udi.add(i.copy()); 4694 }; 4695 dst.bodySite = bodySite == null ? null : bodySite.copy(); 4696 if (subSite != null) { 4697 dst.subSite = new ArrayList<CodeableConcept>(); 4698 for (CodeableConcept i : subSite) 4699 dst.subSite.add(i.copy()); 4700 }; 4701 if (encounter != null) { 4702 dst.encounter = new ArrayList<Reference>(); 4703 for (Reference i : encounter) 4704 dst.encounter.add(i.copy()); 4705 }; 4706 if (noteNumber != null) { 4707 dst.noteNumber = new ArrayList<PositiveIntType>(); 4708 for (PositiveIntType i : noteNumber) 4709 dst.noteNumber.add(i.copy()); 4710 }; 4711 if (adjudication != null) { 4712 dst.adjudication = new ArrayList<AdjudicationComponent>(); 4713 for (AdjudicationComponent i : adjudication) 4714 dst.adjudication.add(i.copy()); 4715 }; 4716 if (detail != null) { 4717 dst.detail = new ArrayList<DetailComponent>(); 4718 for (DetailComponent i : detail) 4719 dst.detail.add(i.copy()); 4720 }; 4721 return dst; 4722 } 4723 4724 @Override 4725 public boolean equalsDeep(Base other_) { 4726 if (!super.equalsDeep(other_)) 4727 return false; 4728 if (!(other_ instanceof ItemComponent)) 4729 return false; 4730 ItemComponent o = (ItemComponent) other_; 4731 return compareDeep(sequence, o.sequence, true) && compareDeep(careTeamLinkId, o.careTeamLinkId, true) 4732 && compareDeep(diagnosisLinkId, o.diagnosisLinkId, true) && compareDeep(procedureLinkId, o.procedureLinkId, true) 4733 && compareDeep(informationLinkId, o.informationLinkId, true) && compareDeep(revenue, o.revenue, true) 4734 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 4735 && compareDeep(programCode, o.programCode, true) && compareDeep(serviced, o.serviced, true) && compareDeep(location, o.location, true) 4736 && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) && compareDeep(factor, o.factor, true) 4737 && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) && compareDeep(bodySite, o.bodySite, true) 4738 && compareDeep(subSite, o.subSite, true) && compareDeep(encounter, o.encounter, true) && compareDeep(noteNumber, o.noteNumber, true) 4739 && compareDeep(adjudication, o.adjudication, true) && compareDeep(detail, o.detail, true); 4740 } 4741 4742 @Override 4743 public boolean equalsShallow(Base other_) { 4744 if (!super.equalsShallow(other_)) 4745 return false; 4746 if (!(other_ instanceof ItemComponent)) 4747 return false; 4748 ItemComponent o = (ItemComponent) other_; 4749 return compareValues(sequence, o.sequence, true) && compareValues(careTeamLinkId, o.careTeamLinkId, true) 4750 && compareValues(diagnosisLinkId, o.diagnosisLinkId, true) && compareValues(procedureLinkId, o.procedureLinkId, true) 4751 && compareValues(informationLinkId, o.informationLinkId, true) && compareValues(factor, o.factor, true) 4752 && compareValues(noteNumber, o.noteNumber, true); 4753 } 4754 4755 public boolean isEmpty() { 4756 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, careTeamLinkId, diagnosisLinkId 4757 , procedureLinkId, informationLinkId, revenue, category, service, modifier, programCode 4758 , serviced, location, quantity, unitPrice, factor, net, udi, bodySite, subSite 4759 , encounter, noteNumber, adjudication, detail); 4760 } 4761 4762 public String fhirType() { 4763 return "ExplanationOfBenefit.item"; 4764 4765 } 4766 4767 } 4768 4769 @Block() 4770 public static class AdjudicationComponent extends BackboneElement implements IBaseBackboneElement { 4771 /** 4772 * Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc. 4773 */ 4774 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 4775 @Description(shortDefinition="Adjudication category such as co-pay, eligible, benefit, etc.", formalDefinition="Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc." ) 4776 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication") 4777 protected CodeableConcept category; 4778 4779 /** 4780 * Adjudication reason such as limit reached. 4781 */ 4782 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 4783 @Description(shortDefinition="Explanation of Adjudication outcome", formalDefinition="Adjudication reason such as limit reached." ) 4784 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/adjudication-reason") 4785 protected CodeableConcept reason; 4786 4787 /** 4788 * Monitory amount associated with the code. 4789 */ 4790 @Child(name = "amount", type = {Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 4791 @Description(shortDefinition="Monetary amount", formalDefinition="Monitory amount associated with the code." ) 4792 protected Money amount; 4793 4794 /** 4795 * A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4796 */ 4797 @Child(name = "value", type = {DecimalType.class}, order=4, min=0, max=1, modifier=false, summary=false) 4798 @Description(shortDefinition="Non-monitory value", formalDefinition="A non-monetary value for example a percentage. Mutually exclusive to the amount element above." ) 4799 protected DecimalType value; 4800 4801 private static final long serialVersionUID = 1559898786L; 4802 4803 /** 4804 * Constructor 4805 */ 4806 public AdjudicationComponent() { 4807 super(); 4808 } 4809 4810 /** 4811 * Constructor 4812 */ 4813 public AdjudicationComponent(CodeableConcept category) { 4814 super(); 4815 this.category = category; 4816 } 4817 4818 /** 4819 * @return {@link #category} (Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc.) 4820 */ 4821 public CodeableConcept getCategory() { 4822 if (this.category == null) 4823 if (Configuration.errorOnAutoCreate()) 4824 throw new Error("Attempt to auto-create AdjudicationComponent.category"); 4825 else if (Configuration.doAutoCreate()) 4826 this.category = new CodeableConcept(); // cc 4827 return this.category; 4828 } 4829 4830 public boolean hasCategory() { 4831 return this.category != null && !this.category.isEmpty(); 4832 } 4833 4834 /** 4835 * @param value {@link #category} (Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc.) 4836 */ 4837 public AdjudicationComponent setCategory(CodeableConcept value) { 4838 this.category = value; 4839 return this; 4840 } 4841 4842 /** 4843 * @return {@link #reason} (Adjudication reason such as limit reached.) 4844 */ 4845 public CodeableConcept getReason() { 4846 if (this.reason == null) 4847 if (Configuration.errorOnAutoCreate()) 4848 throw new Error("Attempt to auto-create AdjudicationComponent.reason"); 4849 else if (Configuration.doAutoCreate()) 4850 this.reason = new CodeableConcept(); // cc 4851 return this.reason; 4852 } 4853 4854 public boolean hasReason() { 4855 return this.reason != null && !this.reason.isEmpty(); 4856 } 4857 4858 /** 4859 * @param value {@link #reason} (Adjudication reason such as limit reached.) 4860 */ 4861 public AdjudicationComponent setReason(CodeableConcept value) { 4862 this.reason = value; 4863 return this; 4864 } 4865 4866 /** 4867 * @return {@link #amount} (Monitory amount associated with the code.) 4868 */ 4869 public Money getAmount() { 4870 if (this.amount == null) 4871 if (Configuration.errorOnAutoCreate()) 4872 throw new Error("Attempt to auto-create AdjudicationComponent.amount"); 4873 else if (Configuration.doAutoCreate()) 4874 this.amount = new Money(); // cc 4875 return this.amount; 4876 } 4877 4878 public boolean hasAmount() { 4879 return this.amount != null && !this.amount.isEmpty(); 4880 } 4881 4882 /** 4883 * @param value {@link #amount} (Monitory amount associated with the code.) 4884 */ 4885 public AdjudicationComponent setAmount(Money value) { 4886 this.amount = value; 4887 return this; 4888 } 4889 4890 /** 4891 * @return {@link #value} (A non-monetary value for example a percentage. Mutually exclusive to the amount element above.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 4892 */ 4893 public DecimalType getValueElement() { 4894 if (this.value == null) 4895 if (Configuration.errorOnAutoCreate()) 4896 throw new Error("Attempt to auto-create AdjudicationComponent.value"); 4897 else if (Configuration.doAutoCreate()) 4898 this.value = new DecimalType(); // bb 4899 return this.value; 4900 } 4901 4902 public boolean hasValueElement() { 4903 return this.value != null && !this.value.isEmpty(); 4904 } 4905 4906 public boolean hasValue() { 4907 return this.value != null && !this.value.isEmpty(); 4908 } 4909 4910 /** 4911 * @param value {@link #value} (A non-monetary value for example a percentage. Mutually exclusive to the amount element above.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 4912 */ 4913 public AdjudicationComponent setValueElement(DecimalType value) { 4914 this.value = value; 4915 return this; 4916 } 4917 4918 /** 4919 * @return A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4920 */ 4921 public BigDecimal getValue() { 4922 return this.value == null ? null : this.value.getValue(); 4923 } 4924 4925 /** 4926 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4927 */ 4928 public AdjudicationComponent setValue(BigDecimal value) { 4929 if (value == null) 4930 this.value = null; 4931 else { 4932 if (this.value == null) 4933 this.value = new DecimalType(); 4934 this.value.setValue(value); 4935 } 4936 return this; 4937 } 4938 4939 /** 4940 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4941 */ 4942 public AdjudicationComponent setValue(long value) { 4943 this.value = new DecimalType(); 4944 this.value.setValue(value); 4945 return this; 4946 } 4947 4948 /** 4949 * @param value A non-monetary value for example a percentage. Mutually exclusive to the amount element above. 4950 */ 4951 public AdjudicationComponent setValue(double value) { 4952 this.value = new DecimalType(); 4953 this.value.setValue(value); 4954 return this; 4955 } 4956 4957 protected void listChildren(List<Property> children) { 4958 super.listChildren(children); 4959 children.add(new Property("category", "CodeableConcept", "Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc.", 0, 1, category)); 4960 children.add(new Property("reason", "CodeableConcept", "Adjudication reason such as limit reached.", 0, 1, reason)); 4961 children.add(new Property("amount", "Money", "Monitory amount associated with the code.", 0, 1, amount)); 4962 children.add(new Property("value", "decimal", "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 1, value)); 4963 } 4964 4965 @Override 4966 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 4967 switch (_hash) { 4968 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Code indicating: Co-Pay, deductable, elegible, benefit, tax, etc.", 0, 1, category); 4969 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Adjudication reason such as limit reached.", 0, 1, reason); 4970 case -1413853096: /*amount*/ return new Property("amount", "Money", "Monitory amount associated with the code.", 0, 1, amount); 4971 case 111972721: /*value*/ return new Property("value", "decimal", "A non-monetary value for example a percentage. Mutually exclusive to the amount element above.", 0, 1, value); 4972 default: return super.getNamedProperty(_hash, _name, _checkValid); 4973 } 4974 4975 } 4976 4977 @Override 4978 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 4979 switch (hash) { 4980 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 4981 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 4982 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 4983 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DecimalType 4984 default: return super.getProperty(hash, name, checkValid); 4985 } 4986 4987 } 4988 4989 @Override 4990 public Base setProperty(int hash, String name, Base value) throws FHIRException { 4991 switch (hash) { 4992 case 50511102: // category 4993 this.category = castToCodeableConcept(value); // CodeableConcept 4994 return value; 4995 case -934964668: // reason 4996 this.reason = castToCodeableConcept(value); // CodeableConcept 4997 return value; 4998 case -1413853096: // amount 4999 this.amount = castToMoney(value); // Money 5000 return value; 5001 case 111972721: // value 5002 this.value = castToDecimal(value); // DecimalType 5003 return value; 5004 default: return super.setProperty(hash, name, value); 5005 } 5006 5007 } 5008 5009 @Override 5010 public Base setProperty(String name, Base value) throws FHIRException { 5011 if (name.equals("category")) { 5012 this.category = castToCodeableConcept(value); // CodeableConcept 5013 } else if (name.equals("reason")) { 5014 this.reason = castToCodeableConcept(value); // CodeableConcept 5015 } else if (name.equals("amount")) { 5016 this.amount = castToMoney(value); // Money 5017 } else if (name.equals("value")) { 5018 this.value = castToDecimal(value); // DecimalType 5019 } else 5020 return super.setProperty(name, value); 5021 return value; 5022 } 5023 5024 @Override 5025 public Base makeProperty(int hash, String name) throws FHIRException { 5026 switch (hash) { 5027 case 50511102: return getCategory(); 5028 case -934964668: return getReason(); 5029 case -1413853096: return getAmount(); 5030 case 111972721: return getValueElement(); 5031 default: return super.makeProperty(hash, name); 5032 } 5033 5034 } 5035 5036 @Override 5037 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 5038 switch (hash) { 5039 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 5040 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 5041 case -1413853096: /*amount*/ return new String[] {"Money"}; 5042 case 111972721: /*value*/ return new String[] {"decimal"}; 5043 default: return super.getTypesForProperty(hash, name); 5044 } 5045 5046 } 5047 5048 @Override 5049 public Base addChild(String name) throws FHIRException { 5050 if (name.equals("category")) { 5051 this.category = new CodeableConcept(); 5052 return this.category; 5053 } 5054 else if (name.equals("reason")) { 5055 this.reason = new CodeableConcept(); 5056 return this.reason; 5057 } 5058 else if (name.equals("amount")) { 5059 this.amount = new Money(); 5060 return this.amount; 5061 } 5062 else if (name.equals("value")) { 5063 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.value"); 5064 } 5065 else 5066 return super.addChild(name); 5067 } 5068 5069 public AdjudicationComponent copy() { 5070 AdjudicationComponent dst = new AdjudicationComponent(); 5071 copyValues(dst); 5072 dst.category = category == null ? null : category.copy(); 5073 dst.reason = reason == null ? null : reason.copy(); 5074 dst.amount = amount == null ? null : amount.copy(); 5075 dst.value = value == null ? null : value.copy(); 5076 return dst; 5077 } 5078 5079 @Override 5080 public boolean equalsDeep(Base other_) { 5081 if (!super.equalsDeep(other_)) 5082 return false; 5083 if (!(other_ instanceof AdjudicationComponent)) 5084 return false; 5085 AdjudicationComponent o = (AdjudicationComponent) other_; 5086 return compareDeep(category, o.category, true) && compareDeep(reason, o.reason, true) && compareDeep(amount, o.amount, true) 5087 && compareDeep(value, o.value, true); 5088 } 5089 5090 @Override 5091 public boolean equalsShallow(Base other_) { 5092 if (!super.equalsShallow(other_)) 5093 return false; 5094 if (!(other_ instanceof AdjudicationComponent)) 5095 return false; 5096 AdjudicationComponent o = (AdjudicationComponent) other_; 5097 return compareValues(value, o.value, true); 5098 } 5099 5100 public boolean isEmpty() { 5101 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, reason, amount 5102 , value); 5103 } 5104 5105 public String fhirType() { 5106 return "ExplanationOfBenefit.item.adjudication"; 5107 5108 } 5109 5110 } 5111 5112 @Block() 5113 public static class DetailComponent extends BackboneElement implements IBaseBackboneElement { 5114 /** 5115 * A service line number. 5116 */ 5117 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 5118 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 5119 protected PositiveIntType sequence; 5120 5121 /** 5122 * The type of product or service. 5123 */ 5124 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 5125 @Description(shortDefinition="Group or type of product or service", formalDefinition="The type of product or service." ) 5126 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActInvoiceGroupCode") 5127 protected CodeableConcept type; 5128 5129 /** 5130 * The type of reveneu or cost center providing the product and/or service. 5131 */ 5132 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 5133 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 5134 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 5135 protected CodeableConcept revenue; 5136 5137 /** 5138 * Health Care Service Type Codes to identify the classification of service or benefits. 5139 */ 5140 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 5141 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 5142 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 5143 protected CodeableConcept category; 5144 5145 /** 5146 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 5147 */ 5148 @Child(name = "service", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 5149 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 5150 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 5151 protected CodeableConcept service; 5152 5153 /** 5154 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 5155 */ 5156 @Child(name = "modifier", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5157 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 5158 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 5159 protected List<CodeableConcept> modifier; 5160 5161 /** 5162 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 5163 */ 5164 @Child(name = "programCode", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5165 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 5166 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 5167 protected List<CodeableConcept> programCode; 5168 5169 /** 5170 * The number of repetitions of a service or product. 5171 */ 5172 @Child(name = "quantity", type = {SimpleQuantity.class}, order=8, min=0, max=1, modifier=false, summary=false) 5173 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 5174 protected SimpleQuantity quantity; 5175 5176 /** 5177 * If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group. 5178 */ 5179 @Child(name = "unitPrice", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 5180 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group." ) 5181 protected Money unitPrice; 5182 5183 /** 5184 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5185 */ 5186 @Child(name = "factor", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 5187 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 5188 protected DecimalType factor; 5189 5190 /** 5191 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 5192 */ 5193 @Child(name = "net", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 5194 @Description(shortDefinition="Total additional item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 5195 protected Money net; 5196 5197 /** 5198 * List of Unique Device Identifiers associated with this line item. 5199 */ 5200 @Child(name = "udi", type = {Device.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5201 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 5202 protected List<Reference> udi; 5203 /** 5204 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 5205 */ 5206 protected List<Device> udiTarget; 5207 5208 5209 /** 5210 * A list of note references to the notes provided below. 5211 */ 5212 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5213 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 5214 protected List<PositiveIntType> noteNumber; 5215 5216 /** 5217 * The adjudications results. 5218 */ 5219 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5220 @Description(shortDefinition="Detail level adjudication details", formalDefinition="The adjudications results." ) 5221 protected List<AdjudicationComponent> adjudication; 5222 5223 /** 5224 * Third tier of goods and services. 5225 */ 5226 @Child(name = "subDetail", type = {}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 5227 @Description(shortDefinition="Additional items", formalDefinition="Third tier of goods and services." ) 5228 protected List<SubDetailComponent> subDetail; 5229 5230 private static final long serialVersionUID = -276371489L; 5231 5232 /** 5233 * Constructor 5234 */ 5235 public DetailComponent() { 5236 super(); 5237 } 5238 5239 /** 5240 * Constructor 5241 */ 5242 public DetailComponent(PositiveIntType sequence, CodeableConcept type) { 5243 super(); 5244 this.sequence = sequence; 5245 this.type = type; 5246 } 5247 5248 /** 5249 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5250 */ 5251 public PositiveIntType getSequenceElement() { 5252 if (this.sequence == null) 5253 if (Configuration.errorOnAutoCreate()) 5254 throw new Error("Attempt to auto-create DetailComponent.sequence"); 5255 else if (Configuration.doAutoCreate()) 5256 this.sequence = new PositiveIntType(); // bb 5257 return this.sequence; 5258 } 5259 5260 public boolean hasSequenceElement() { 5261 return this.sequence != null && !this.sequence.isEmpty(); 5262 } 5263 5264 public boolean hasSequence() { 5265 return this.sequence != null && !this.sequence.isEmpty(); 5266 } 5267 5268 /** 5269 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 5270 */ 5271 public DetailComponent setSequenceElement(PositiveIntType value) { 5272 this.sequence = value; 5273 return this; 5274 } 5275 5276 /** 5277 * @return A service line number. 5278 */ 5279 public int getSequence() { 5280 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 5281 } 5282 5283 /** 5284 * @param value A service line number. 5285 */ 5286 public DetailComponent setSequence(int value) { 5287 if (this.sequence == null) 5288 this.sequence = new PositiveIntType(); 5289 this.sequence.setValue(value); 5290 return this; 5291 } 5292 5293 /** 5294 * @return {@link #type} (The type of product or service.) 5295 */ 5296 public CodeableConcept getType() { 5297 if (this.type == null) 5298 if (Configuration.errorOnAutoCreate()) 5299 throw new Error("Attempt to auto-create DetailComponent.type"); 5300 else if (Configuration.doAutoCreate()) 5301 this.type = new CodeableConcept(); // cc 5302 return this.type; 5303 } 5304 5305 public boolean hasType() { 5306 return this.type != null && !this.type.isEmpty(); 5307 } 5308 5309 /** 5310 * @param value {@link #type} (The type of product or service.) 5311 */ 5312 public DetailComponent setType(CodeableConcept value) { 5313 this.type = value; 5314 return this; 5315 } 5316 5317 /** 5318 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 5319 */ 5320 public CodeableConcept getRevenue() { 5321 if (this.revenue == null) 5322 if (Configuration.errorOnAutoCreate()) 5323 throw new Error("Attempt to auto-create DetailComponent.revenue"); 5324 else if (Configuration.doAutoCreate()) 5325 this.revenue = new CodeableConcept(); // cc 5326 return this.revenue; 5327 } 5328 5329 public boolean hasRevenue() { 5330 return this.revenue != null && !this.revenue.isEmpty(); 5331 } 5332 5333 /** 5334 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 5335 */ 5336 public DetailComponent setRevenue(CodeableConcept value) { 5337 this.revenue = value; 5338 return this; 5339 } 5340 5341 /** 5342 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 5343 */ 5344 public CodeableConcept getCategory() { 5345 if (this.category == null) 5346 if (Configuration.errorOnAutoCreate()) 5347 throw new Error("Attempt to auto-create DetailComponent.category"); 5348 else if (Configuration.doAutoCreate()) 5349 this.category = new CodeableConcept(); // cc 5350 return this.category; 5351 } 5352 5353 public boolean hasCategory() { 5354 return this.category != null && !this.category.isEmpty(); 5355 } 5356 5357 /** 5358 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 5359 */ 5360 public DetailComponent setCategory(CodeableConcept value) { 5361 this.category = value; 5362 return this; 5363 } 5364 5365 /** 5366 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 5367 */ 5368 public CodeableConcept getService() { 5369 if (this.service == null) 5370 if (Configuration.errorOnAutoCreate()) 5371 throw new Error("Attempt to auto-create DetailComponent.service"); 5372 else if (Configuration.doAutoCreate()) 5373 this.service = new CodeableConcept(); // cc 5374 return this.service; 5375 } 5376 5377 public boolean hasService() { 5378 return this.service != null && !this.service.isEmpty(); 5379 } 5380 5381 /** 5382 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 5383 */ 5384 public DetailComponent setService(CodeableConcept value) { 5385 this.service = value; 5386 return this; 5387 } 5388 5389 /** 5390 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 5391 */ 5392 public List<CodeableConcept> getModifier() { 5393 if (this.modifier == null) 5394 this.modifier = new ArrayList<CodeableConcept>(); 5395 return this.modifier; 5396 } 5397 5398 /** 5399 * @return Returns a reference to <code>this</code> for easy method chaining 5400 */ 5401 public DetailComponent setModifier(List<CodeableConcept> theModifier) { 5402 this.modifier = theModifier; 5403 return this; 5404 } 5405 5406 public boolean hasModifier() { 5407 if (this.modifier == null) 5408 return false; 5409 for (CodeableConcept item : this.modifier) 5410 if (!item.isEmpty()) 5411 return true; 5412 return false; 5413 } 5414 5415 public CodeableConcept addModifier() { //3 5416 CodeableConcept t = new CodeableConcept(); 5417 if (this.modifier == null) 5418 this.modifier = new ArrayList<CodeableConcept>(); 5419 this.modifier.add(t); 5420 return t; 5421 } 5422 5423 public DetailComponent addModifier(CodeableConcept t) { //3 5424 if (t == null) 5425 return this; 5426 if (this.modifier == null) 5427 this.modifier = new ArrayList<CodeableConcept>(); 5428 this.modifier.add(t); 5429 return this; 5430 } 5431 5432 /** 5433 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 5434 */ 5435 public CodeableConcept getModifierFirstRep() { 5436 if (getModifier().isEmpty()) { 5437 addModifier(); 5438 } 5439 return getModifier().get(0); 5440 } 5441 5442 /** 5443 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 5444 */ 5445 public List<CodeableConcept> getProgramCode() { 5446 if (this.programCode == null) 5447 this.programCode = new ArrayList<CodeableConcept>(); 5448 return this.programCode; 5449 } 5450 5451 /** 5452 * @return Returns a reference to <code>this</code> for easy method chaining 5453 */ 5454 public DetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 5455 this.programCode = theProgramCode; 5456 return this; 5457 } 5458 5459 public boolean hasProgramCode() { 5460 if (this.programCode == null) 5461 return false; 5462 for (CodeableConcept item : this.programCode) 5463 if (!item.isEmpty()) 5464 return true; 5465 return false; 5466 } 5467 5468 public CodeableConcept addProgramCode() { //3 5469 CodeableConcept t = new CodeableConcept(); 5470 if (this.programCode == null) 5471 this.programCode = new ArrayList<CodeableConcept>(); 5472 this.programCode.add(t); 5473 return t; 5474 } 5475 5476 public DetailComponent addProgramCode(CodeableConcept t) { //3 5477 if (t == null) 5478 return this; 5479 if (this.programCode == null) 5480 this.programCode = new ArrayList<CodeableConcept>(); 5481 this.programCode.add(t); 5482 return this; 5483 } 5484 5485 /** 5486 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 5487 */ 5488 public CodeableConcept getProgramCodeFirstRep() { 5489 if (getProgramCode().isEmpty()) { 5490 addProgramCode(); 5491 } 5492 return getProgramCode().get(0); 5493 } 5494 5495 /** 5496 * @return {@link #quantity} (The number of repetitions of a service or product.) 5497 */ 5498 public SimpleQuantity getQuantity() { 5499 if (this.quantity == null) 5500 if (Configuration.errorOnAutoCreate()) 5501 throw new Error("Attempt to auto-create DetailComponent.quantity"); 5502 else if (Configuration.doAutoCreate()) 5503 this.quantity = new SimpleQuantity(); // cc 5504 return this.quantity; 5505 } 5506 5507 public boolean hasQuantity() { 5508 return this.quantity != null && !this.quantity.isEmpty(); 5509 } 5510 5511 /** 5512 * @param value {@link #quantity} (The number of repetitions of a service or product.) 5513 */ 5514 public DetailComponent setQuantity(SimpleQuantity value) { 5515 this.quantity = value; 5516 return this; 5517 } 5518 5519 /** 5520 * @return {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 5521 */ 5522 public Money getUnitPrice() { 5523 if (this.unitPrice == null) 5524 if (Configuration.errorOnAutoCreate()) 5525 throw new Error("Attempt to auto-create DetailComponent.unitPrice"); 5526 else if (Configuration.doAutoCreate()) 5527 this.unitPrice = new Money(); // cc 5528 return this.unitPrice; 5529 } 5530 5531 public boolean hasUnitPrice() { 5532 return this.unitPrice != null && !this.unitPrice.isEmpty(); 5533 } 5534 5535 /** 5536 * @param value {@link #unitPrice} (If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.) 5537 */ 5538 public DetailComponent setUnitPrice(Money value) { 5539 this.unitPrice = value; 5540 return this; 5541 } 5542 5543 /** 5544 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5545 */ 5546 public DecimalType getFactorElement() { 5547 if (this.factor == null) 5548 if (Configuration.errorOnAutoCreate()) 5549 throw new Error("Attempt to auto-create DetailComponent.factor"); 5550 else if (Configuration.doAutoCreate()) 5551 this.factor = new DecimalType(); // bb 5552 return this.factor; 5553 } 5554 5555 public boolean hasFactorElement() { 5556 return this.factor != null && !this.factor.isEmpty(); 5557 } 5558 5559 public boolean hasFactor() { 5560 return this.factor != null && !this.factor.isEmpty(); 5561 } 5562 5563 /** 5564 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 5565 */ 5566 public DetailComponent setFactorElement(DecimalType value) { 5567 this.factor = value; 5568 return this; 5569 } 5570 5571 /** 5572 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5573 */ 5574 public BigDecimal getFactor() { 5575 return this.factor == null ? null : this.factor.getValue(); 5576 } 5577 5578 /** 5579 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5580 */ 5581 public DetailComponent setFactor(BigDecimal value) { 5582 if (value == null) 5583 this.factor = null; 5584 else { 5585 if (this.factor == null) 5586 this.factor = new DecimalType(); 5587 this.factor.setValue(value); 5588 } 5589 return this; 5590 } 5591 5592 /** 5593 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5594 */ 5595 public DetailComponent setFactor(long value) { 5596 this.factor = new DecimalType(); 5597 this.factor.setValue(value); 5598 return this; 5599 } 5600 5601 /** 5602 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 5603 */ 5604 public DetailComponent setFactor(double value) { 5605 this.factor = new DecimalType(); 5606 this.factor.setValue(value); 5607 return this; 5608 } 5609 5610 /** 5611 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 5612 */ 5613 public Money getNet() { 5614 if (this.net == null) 5615 if (Configuration.errorOnAutoCreate()) 5616 throw new Error("Attempt to auto-create DetailComponent.net"); 5617 else if (Configuration.doAutoCreate()) 5618 this.net = new Money(); // cc 5619 return this.net; 5620 } 5621 5622 public boolean hasNet() { 5623 return this.net != null && !this.net.isEmpty(); 5624 } 5625 5626 /** 5627 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 5628 */ 5629 public DetailComponent setNet(Money value) { 5630 this.net = value; 5631 return this; 5632 } 5633 5634 /** 5635 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 5636 */ 5637 public List<Reference> getUdi() { 5638 if (this.udi == null) 5639 this.udi = new ArrayList<Reference>(); 5640 return this.udi; 5641 } 5642 5643 /** 5644 * @return Returns a reference to <code>this</code> for easy method chaining 5645 */ 5646 public DetailComponent setUdi(List<Reference> theUdi) { 5647 this.udi = theUdi; 5648 return this; 5649 } 5650 5651 public boolean hasUdi() { 5652 if (this.udi == null) 5653 return false; 5654 for (Reference item : this.udi) 5655 if (!item.isEmpty()) 5656 return true; 5657 return false; 5658 } 5659 5660 public Reference addUdi() { //3 5661 Reference t = new Reference(); 5662 if (this.udi == null) 5663 this.udi = new ArrayList<Reference>(); 5664 this.udi.add(t); 5665 return t; 5666 } 5667 5668 public DetailComponent addUdi(Reference t) { //3 5669 if (t == null) 5670 return this; 5671 if (this.udi == null) 5672 this.udi = new ArrayList<Reference>(); 5673 this.udi.add(t); 5674 return this; 5675 } 5676 5677 /** 5678 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 5679 */ 5680 public Reference getUdiFirstRep() { 5681 if (getUdi().isEmpty()) { 5682 addUdi(); 5683 } 5684 return getUdi().get(0); 5685 } 5686 5687 /** 5688 * @deprecated Use Reference#setResource(IBaseResource) instead 5689 */ 5690 @Deprecated 5691 public List<Device> getUdiTarget() { 5692 if (this.udiTarget == null) 5693 this.udiTarget = new ArrayList<Device>(); 5694 return this.udiTarget; 5695 } 5696 5697 /** 5698 * @deprecated Use Reference#setResource(IBaseResource) instead 5699 */ 5700 @Deprecated 5701 public Device addUdiTarget() { 5702 Device r = new Device(); 5703 if (this.udiTarget == null) 5704 this.udiTarget = new ArrayList<Device>(); 5705 this.udiTarget.add(r); 5706 return r; 5707 } 5708 5709 /** 5710 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 5711 */ 5712 public List<PositiveIntType> getNoteNumber() { 5713 if (this.noteNumber == null) 5714 this.noteNumber = new ArrayList<PositiveIntType>(); 5715 return this.noteNumber; 5716 } 5717 5718 /** 5719 * @return Returns a reference to <code>this</code> for easy method chaining 5720 */ 5721 public DetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 5722 this.noteNumber = theNoteNumber; 5723 return this; 5724 } 5725 5726 public boolean hasNoteNumber() { 5727 if (this.noteNumber == null) 5728 return false; 5729 for (PositiveIntType item : this.noteNumber) 5730 if (!item.isEmpty()) 5731 return true; 5732 return false; 5733 } 5734 5735 /** 5736 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 5737 */ 5738 public PositiveIntType addNoteNumberElement() {//2 5739 PositiveIntType t = new PositiveIntType(); 5740 if (this.noteNumber == null) 5741 this.noteNumber = new ArrayList<PositiveIntType>(); 5742 this.noteNumber.add(t); 5743 return t; 5744 } 5745 5746 /** 5747 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 5748 */ 5749 public DetailComponent addNoteNumber(int value) { //1 5750 PositiveIntType t = new PositiveIntType(); 5751 t.setValue(value); 5752 if (this.noteNumber == null) 5753 this.noteNumber = new ArrayList<PositiveIntType>(); 5754 this.noteNumber.add(t); 5755 return this; 5756 } 5757 5758 /** 5759 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 5760 */ 5761 public boolean hasNoteNumber(int value) { 5762 if (this.noteNumber == null) 5763 return false; 5764 for (PositiveIntType v : this.noteNumber) 5765 if (v.getValue().equals(value)) // positiveInt 5766 return true; 5767 return false; 5768 } 5769 5770 /** 5771 * @return {@link #adjudication} (The adjudications results.) 5772 */ 5773 public List<AdjudicationComponent> getAdjudication() { 5774 if (this.adjudication == null) 5775 this.adjudication = new ArrayList<AdjudicationComponent>(); 5776 return this.adjudication; 5777 } 5778 5779 /** 5780 * @return Returns a reference to <code>this</code> for easy method chaining 5781 */ 5782 public DetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 5783 this.adjudication = theAdjudication; 5784 return this; 5785 } 5786 5787 public boolean hasAdjudication() { 5788 if (this.adjudication == null) 5789 return false; 5790 for (AdjudicationComponent item : this.adjudication) 5791 if (!item.isEmpty()) 5792 return true; 5793 return false; 5794 } 5795 5796 public AdjudicationComponent addAdjudication() { //3 5797 AdjudicationComponent t = new AdjudicationComponent(); 5798 if (this.adjudication == null) 5799 this.adjudication = new ArrayList<AdjudicationComponent>(); 5800 this.adjudication.add(t); 5801 return t; 5802 } 5803 5804 public DetailComponent addAdjudication(AdjudicationComponent t) { //3 5805 if (t == null) 5806 return this; 5807 if (this.adjudication == null) 5808 this.adjudication = new ArrayList<AdjudicationComponent>(); 5809 this.adjudication.add(t); 5810 return this; 5811 } 5812 5813 /** 5814 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 5815 */ 5816 public AdjudicationComponent getAdjudicationFirstRep() { 5817 if (getAdjudication().isEmpty()) { 5818 addAdjudication(); 5819 } 5820 return getAdjudication().get(0); 5821 } 5822 5823 /** 5824 * @return {@link #subDetail} (Third tier of goods and services.) 5825 */ 5826 public List<SubDetailComponent> getSubDetail() { 5827 if (this.subDetail == null) 5828 this.subDetail = new ArrayList<SubDetailComponent>(); 5829 return this.subDetail; 5830 } 5831 5832 /** 5833 * @return Returns a reference to <code>this</code> for easy method chaining 5834 */ 5835 public DetailComponent setSubDetail(List<SubDetailComponent> theSubDetail) { 5836 this.subDetail = theSubDetail; 5837 return this; 5838 } 5839 5840 public boolean hasSubDetail() { 5841 if (this.subDetail == null) 5842 return false; 5843 for (SubDetailComponent item : this.subDetail) 5844 if (!item.isEmpty()) 5845 return true; 5846 return false; 5847 } 5848 5849 public SubDetailComponent addSubDetail() { //3 5850 SubDetailComponent t = new SubDetailComponent(); 5851 if (this.subDetail == null) 5852 this.subDetail = new ArrayList<SubDetailComponent>(); 5853 this.subDetail.add(t); 5854 return t; 5855 } 5856 5857 public DetailComponent addSubDetail(SubDetailComponent t) { //3 5858 if (t == null) 5859 return this; 5860 if (this.subDetail == null) 5861 this.subDetail = new ArrayList<SubDetailComponent>(); 5862 this.subDetail.add(t); 5863 return this; 5864 } 5865 5866 /** 5867 * @return The first repetition of repeating field {@link #subDetail}, creating it if it does not already exist 5868 */ 5869 public SubDetailComponent getSubDetailFirstRep() { 5870 if (getSubDetail().isEmpty()) { 5871 addSubDetail(); 5872 } 5873 return getSubDetail().get(0); 5874 } 5875 5876 protected void listChildren(List<Property> children) { 5877 super.listChildren(children); 5878 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 5879 children.add(new Property("type", "CodeableConcept", "The type of product or service.", 0, 1, type)); 5880 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 5881 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 5882 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 5883 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 5884 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 5885 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 5886 children.add(new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice)); 5887 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 5888 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 5889 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 5890 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 5891 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 5892 children.add(new Property("subDetail", "", "Third tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail)); 5893 } 5894 5895 @Override 5896 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 5897 switch (_hash) { 5898 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 5899 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of product or service.", 0, 1, type); 5900 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 5901 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 5902 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 5903 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 5904 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 5905 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 5906 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "If the item is a node then this is the fee for the product or service, otherwise this is the total of the fees for the children of the group.", 0, 1, unitPrice); 5907 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 5908 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 5909 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 5910 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 5911 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 5912 case -828829007: /*subDetail*/ return new Property("subDetail", "", "Third tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, subDetail); 5913 default: return super.getNamedProperty(_hash, _name, _checkValid); 5914 } 5915 5916 } 5917 5918 @Override 5919 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 5920 switch (hash) { 5921 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 5922 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 5923 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 5924 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 5925 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 5926 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 5927 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 5928 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 5929 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 5930 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 5931 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 5932 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 5933 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 5934 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 5935 case -828829007: /*subDetail*/ return this.subDetail == null ? new Base[0] : this.subDetail.toArray(new Base[this.subDetail.size()]); // SubDetailComponent 5936 default: return super.getProperty(hash, name, checkValid); 5937 } 5938 5939 } 5940 5941 @Override 5942 public Base setProperty(int hash, String name, Base value) throws FHIRException { 5943 switch (hash) { 5944 case 1349547969: // sequence 5945 this.sequence = castToPositiveInt(value); // PositiveIntType 5946 return value; 5947 case 3575610: // type 5948 this.type = castToCodeableConcept(value); // CodeableConcept 5949 return value; 5950 case 1099842588: // revenue 5951 this.revenue = castToCodeableConcept(value); // CodeableConcept 5952 return value; 5953 case 50511102: // category 5954 this.category = castToCodeableConcept(value); // CodeableConcept 5955 return value; 5956 case 1984153269: // service 5957 this.service = castToCodeableConcept(value); // CodeableConcept 5958 return value; 5959 case -615513385: // modifier 5960 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 5961 return value; 5962 case 1010065041: // programCode 5963 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 5964 return value; 5965 case -1285004149: // quantity 5966 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 5967 return value; 5968 case -486196699: // unitPrice 5969 this.unitPrice = castToMoney(value); // Money 5970 return value; 5971 case -1282148017: // factor 5972 this.factor = castToDecimal(value); // DecimalType 5973 return value; 5974 case 108957: // net 5975 this.net = castToMoney(value); // Money 5976 return value; 5977 case 115642: // udi 5978 this.getUdi().add(castToReference(value)); // Reference 5979 return value; 5980 case -1110033957: // noteNumber 5981 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 5982 return value; 5983 case -231349275: // adjudication 5984 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 5985 return value; 5986 case -828829007: // subDetail 5987 this.getSubDetail().add((SubDetailComponent) value); // SubDetailComponent 5988 return value; 5989 default: return super.setProperty(hash, name, value); 5990 } 5991 5992 } 5993 5994 @Override 5995 public Base setProperty(String name, Base value) throws FHIRException { 5996 if (name.equals("sequence")) { 5997 this.sequence = castToPositiveInt(value); // PositiveIntType 5998 } else if (name.equals("type")) { 5999 this.type = castToCodeableConcept(value); // CodeableConcept 6000 } else if (name.equals("revenue")) { 6001 this.revenue = castToCodeableConcept(value); // CodeableConcept 6002 } else if (name.equals("category")) { 6003 this.category = castToCodeableConcept(value); // CodeableConcept 6004 } else if (name.equals("service")) { 6005 this.service = castToCodeableConcept(value); // CodeableConcept 6006 } else if (name.equals("modifier")) { 6007 this.getModifier().add(castToCodeableConcept(value)); 6008 } else if (name.equals("programCode")) { 6009 this.getProgramCode().add(castToCodeableConcept(value)); 6010 } else if (name.equals("quantity")) { 6011 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 6012 } else if (name.equals("unitPrice")) { 6013 this.unitPrice = castToMoney(value); // Money 6014 } else if (name.equals("factor")) { 6015 this.factor = castToDecimal(value); // DecimalType 6016 } else if (name.equals("net")) { 6017 this.net = castToMoney(value); // Money 6018 } else if (name.equals("udi")) { 6019 this.getUdi().add(castToReference(value)); 6020 } else if (name.equals("noteNumber")) { 6021 this.getNoteNumber().add(castToPositiveInt(value)); 6022 } else if (name.equals("adjudication")) { 6023 this.getAdjudication().add((AdjudicationComponent) value); 6024 } else if (name.equals("subDetail")) { 6025 this.getSubDetail().add((SubDetailComponent) value); 6026 } else 6027 return super.setProperty(name, value); 6028 return value; 6029 } 6030 6031 @Override 6032 public Base makeProperty(int hash, String name) throws FHIRException { 6033 switch (hash) { 6034 case 1349547969: return getSequenceElement(); 6035 case 3575610: return getType(); 6036 case 1099842588: return getRevenue(); 6037 case 50511102: return getCategory(); 6038 case 1984153269: return getService(); 6039 case -615513385: return addModifier(); 6040 case 1010065041: return addProgramCode(); 6041 case -1285004149: return getQuantity(); 6042 case -486196699: return getUnitPrice(); 6043 case -1282148017: return getFactorElement(); 6044 case 108957: return getNet(); 6045 case 115642: return addUdi(); 6046 case -1110033957: return addNoteNumberElement(); 6047 case -231349275: return addAdjudication(); 6048 case -828829007: return addSubDetail(); 6049 default: return super.makeProperty(hash, name); 6050 } 6051 6052 } 6053 6054 @Override 6055 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 6056 switch (hash) { 6057 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 6058 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 6059 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 6060 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 6061 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 6062 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 6063 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 6064 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 6065 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 6066 case -1282148017: /*factor*/ return new String[] {"decimal"}; 6067 case 108957: /*net*/ return new String[] {"Money"}; 6068 case 115642: /*udi*/ return new String[] {"Reference"}; 6069 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 6070 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 6071 case -828829007: /*subDetail*/ return new String[] {}; 6072 default: return super.getTypesForProperty(hash, name); 6073 } 6074 6075 } 6076 6077 @Override 6078 public Base addChild(String name) throws FHIRException { 6079 if (name.equals("sequence")) { 6080 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 6081 } 6082 else if (name.equals("type")) { 6083 this.type = new CodeableConcept(); 6084 return this.type; 6085 } 6086 else if (name.equals("revenue")) { 6087 this.revenue = new CodeableConcept(); 6088 return this.revenue; 6089 } 6090 else if (name.equals("category")) { 6091 this.category = new CodeableConcept(); 6092 return this.category; 6093 } 6094 else if (name.equals("service")) { 6095 this.service = new CodeableConcept(); 6096 return this.service; 6097 } 6098 else if (name.equals("modifier")) { 6099 return addModifier(); 6100 } 6101 else if (name.equals("programCode")) { 6102 return addProgramCode(); 6103 } 6104 else if (name.equals("quantity")) { 6105 this.quantity = new SimpleQuantity(); 6106 return this.quantity; 6107 } 6108 else if (name.equals("unitPrice")) { 6109 this.unitPrice = new Money(); 6110 return this.unitPrice; 6111 } 6112 else if (name.equals("factor")) { 6113 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 6114 } 6115 else if (name.equals("net")) { 6116 this.net = new Money(); 6117 return this.net; 6118 } 6119 else if (name.equals("udi")) { 6120 return addUdi(); 6121 } 6122 else if (name.equals("noteNumber")) { 6123 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 6124 } 6125 else if (name.equals("adjudication")) { 6126 return addAdjudication(); 6127 } 6128 else if (name.equals("subDetail")) { 6129 return addSubDetail(); 6130 } 6131 else 6132 return super.addChild(name); 6133 } 6134 6135 public DetailComponent copy() { 6136 DetailComponent dst = new DetailComponent(); 6137 copyValues(dst); 6138 dst.sequence = sequence == null ? null : sequence.copy(); 6139 dst.type = type == null ? null : type.copy(); 6140 dst.revenue = revenue == null ? null : revenue.copy(); 6141 dst.category = category == null ? null : category.copy(); 6142 dst.service = service == null ? null : service.copy(); 6143 if (modifier != null) { 6144 dst.modifier = new ArrayList<CodeableConcept>(); 6145 for (CodeableConcept i : modifier) 6146 dst.modifier.add(i.copy()); 6147 }; 6148 if (programCode != null) { 6149 dst.programCode = new ArrayList<CodeableConcept>(); 6150 for (CodeableConcept i : programCode) 6151 dst.programCode.add(i.copy()); 6152 }; 6153 dst.quantity = quantity == null ? null : quantity.copy(); 6154 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 6155 dst.factor = factor == null ? null : factor.copy(); 6156 dst.net = net == null ? null : net.copy(); 6157 if (udi != null) { 6158 dst.udi = new ArrayList<Reference>(); 6159 for (Reference i : udi) 6160 dst.udi.add(i.copy()); 6161 }; 6162 if (noteNumber != null) { 6163 dst.noteNumber = new ArrayList<PositiveIntType>(); 6164 for (PositiveIntType i : noteNumber) 6165 dst.noteNumber.add(i.copy()); 6166 }; 6167 if (adjudication != null) { 6168 dst.adjudication = new ArrayList<AdjudicationComponent>(); 6169 for (AdjudicationComponent i : adjudication) 6170 dst.adjudication.add(i.copy()); 6171 }; 6172 if (subDetail != null) { 6173 dst.subDetail = new ArrayList<SubDetailComponent>(); 6174 for (SubDetailComponent i : subDetail) 6175 dst.subDetail.add(i.copy()); 6176 }; 6177 return dst; 6178 } 6179 6180 @Override 6181 public boolean equalsDeep(Base other_) { 6182 if (!super.equalsDeep(other_)) 6183 return false; 6184 if (!(other_ instanceof DetailComponent)) 6185 return false; 6186 DetailComponent o = (DetailComponent) other_; 6187 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) && compareDeep(revenue, o.revenue, true) 6188 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 6189 && compareDeep(programCode, o.programCode, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 6190 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 6191 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 6192 && compareDeep(subDetail, o.subDetail, true); 6193 } 6194 6195 @Override 6196 public boolean equalsShallow(Base other_) { 6197 if (!super.equalsShallow(other_)) 6198 return false; 6199 if (!(other_ instanceof DetailComponent)) 6200 return false; 6201 DetailComponent o = (DetailComponent) other_; 6202 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true) 6203 ; 6204 } 6205 6206 public boolean isEmpty() { 6207 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, revenue 6208 , category, service, modifier, programCode, quantity, unitPrice, factor, net 6209 , udi, noteNumber, adjudication, subDetail); 6210 } 6211 6212 public String fhirType() { 6213 return "ExplanationOfBenefit.item.detail"; 6214 6215 } 6216 6217 } 6218 6219 @Block() 6220 public static class SubDetailComponent extends BackboneElement implements IBaseBackboneElement { 6221 /** 6222 * A service line number. 6223 */ 6224 @Child(name = "sequence", type = {PositiveIntType.class}, order=1, min=1, max=1, modifier=false, summary=false) 6225 @Description(shortDefinition="Service instance", formalDefinition="A service line number." ) 6226 protected PositiveIntType sequence; 6227 6228 /** 6229 * The type of product or service. 6230 */ 6231 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=1, max=1, modifier=false, summary=false) 6232 @Description(shortDefinition="Type of product or service", formalDefinition="The type of product or service." ) 6233 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActInvoiceGroupCode") 6234 protected CodeableConcept type; 6235 6236 /** 6237 * The type of reveneu or cost center providing the product and/or service. 6238 */ 6239 @Child(name = "revenue", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 6240 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 6241 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 6242 protected CodeableConcept revenue; 6243 6244 /** 6245 * Health Care Service Type Codes to identify the classification of service or benefits. 6246 */ 6247 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 6248 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 6249 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 6250 protected CodeableConcept category; 6251 6252 /** 6253 * A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). 6254 */ 6255 @Child(name = "service", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 6256 @Description(shortDefinition="Billing Code", formalDefinition="A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI)." ) 6257 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 6258 protected CodeableConcept service; 6259 6260 /** 6261 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 6262 */ 6263 @Child(name = "modifier", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6264 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 6265 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 6266 protected List<CodeableConcept> modifier; 6267 6268 /** 6269 * For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program. 6270 */ 6271 @Child(name = "programCode", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6272 @Description(shortDefinition="Program specific reason for item inclusion", formalDefinition="For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program." ) 6273 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-program-code") 6274 protected List<CodeableConcept> programCode; 6275 6276 /** 6277 * The number of repetitions of a service or product. 6278 */ 6279 @Child(name = "quantity", type = {SimpleQuantity.class}, order=8, min=0, max=1, modifier=false, summary=false) 6280 @Description(shortDefinition="Count of Products or Services", formalDefinition="The number of repetitions of a service or product." ) 6281 protected SimpleQuantity quantity; 6282 6283 /** 6284 * The fee for an addittional service or product or charge. 6285 */ 6286 @Child(name = "unitPrice", type = {Money.class}, order=9, min=0, max=1, modifier=false, summary=false) 6287 @Description(shortDefinition="Fee, charge or cost per point", formalDefinition="The fee for an addittional service or product or charge." ) 6288 protected Money unitPrice; 6289 6290 /** 6291 * A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6292 */ 6293 @Child(name = "factor", type = {DecimalType.class}, order=10, min=0, max=1, modifier=false, summary=false) 6294 @Description(shortDefinition="Price scaling factor", formalDefinition="A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount." ) 6295 protected DecimalType factor; 6296 6297 /** 6298 * The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied. 6299 */ 6300 @Child(name = "net", type = {Money.class}, order=11, min=0, max=1, modifier=false, summary=false) 6301 @Description(shortDefinition="Net additional item cost", formalDefinition="The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied." ) 6302 protected Money net; 6303 6304 /** 6305 * List of Unique Device Identifiers associated with this line item. 6306 */ 6307 @Child(name = "udi", type = {Device.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6308 @Description(shortDefinition="Unique Device Identifier", formalDefinition="List of Unique Device Identifiers associated with this line item." ) 6309 protected List<Reference> udi; 6310 /** 6311 * The actual objects that are the target of the reference (List of Unique Device Identifiers associated with this line item.) 6312 */ 6313 protected List<Device> udiTarget; 6314 6315 6316 /** 6317 * A list of note references to the notes provided below. 6318 */ 6319 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6320 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 6321 protected List<PositiveIntType> noteNumber; 6322 6323 /** 6324 * The adjudications results. 6325 */ 6326 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 6327 @Description(shortDefinition="Language if different from the resource", formalDefinition="The adjudications results." ) 6328 protected List<AdjudicationComponent> adjudication; 6329 6330 private static final long serialVersionUID = 1621872130L; 6331 6332 /** 6333 * Constructor 6334 */ 6335 public SubDetailComponent() { 6336 super(); 6337 } 6338 6339 /** 6340 * Constructor 6341 */ 6342 public SubDetailComponent(PositiveIntType sequence, CodeableConcept type) { 6343 super(); 6344 this.sequence = sequence; 6345 this.type = type; 6346 } 6347 6348 /** 6349 * @return {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6350 */ 6351 public PositiveIntType getSequenceElement() { 6352 if (this.sequence == null) 6353 if (Configuration.errorOnAutoCreate()) 6354 throw new Error("Attempt to auto-create SubDetailComponent.sequence"); 6355 else if (Configuration.doAutoCreate()) 6356 this.sequence = new PositiveIntType(); // bb 6357 return this.sequence; 6358 } 6359 6360 public boolean hasSequenceElement() { 6361 return this.sequence != null && !this.sequence.isEmpty(); 6362 } 6363 6364 public boolean hasSequence() { 6365 return this.sequence != null && !this.sequence.isEmpty(); 6366 } 6367 6368 /** 6369 * @param value {@link #sequence} (A service line number.). This is the underlying object with id, value and extensions. The accessor "getSequence" gives direct access to the value 6370 */ 6371 public SubDetailComponent setSequenceElement(PositiveIntType value) { 6372 this.sequence = value; 6373 return this; 6374 } 6375 6376 /** 6377 * @return A service line number. 6378 */ 6379 public int getSequence() { 6380 return this.sequence == null || this.sequence.isEmpty() ? 0 : this.sequence.getValue(); 6381 } 6382 6383 /** 6384 * @param value A service line number. 6385 */ 6386 public SubDetailComponent setSequence(int value) { 6387 if (this.sequence == null) 6388 this.sequence = new PositiveIntType(); 6389 this.sequence.setValue(value); 6390 return this; 6391 } 6392 6393 /** 6394 * @return {@link #type} (The type of product or service.) 6395 */ 6396 public CodeableConcept getType() { 6397 if (this.type == null) 6398 if (Configuration.errorOnAutoCreate()) 6399 throw new Error("Attempt to auto-create SubDetailComponent.type"); 6400 else if (Configuration.doAutoCreate()) 6401 this.type = new CodeableConcept(); // cc 6402 return this.type; 6403 } 6404 6405 public boolean hasType() { 6406 return this.type != null && !this.type.isEmpty(); 6407 } 6408 6409 /** 6410 * @param value {@link #type} (The type of product or service.) 6411 */ 6412 public SubDetailComponent setType(CodeableConcept value) { 6413 this.type = value; 6414 return this; 6415 } 6416 6417 /** 6418 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 6419 */ 6420 public CodeableConcept getRevenue() { 6421 if (this.revenue == null) 6422 if (Configuration.errorOnAutoCreate()) 6423 throw new Error("Attempt to auto-create SubDetailComponent.revenue"); 6424 else if (Configuration.doAutoCreate()) 6425 this.revenue = new CodeableConcept(); // cc 6426 return this.revenue; 6427 } 6428 6429 public boolean hasRevenue() { 6430 return this.revenue != null && !this.revenue.isEmpty(); 6431 } 6432 6433 /** 6434 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 6435 */ 6436 public SubDetailComponent setRevenue(CodeableConcept value) { 6437 this.revenue = value; 6438 return this; 6439 } 6440 6441 /** 6442 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 6443 */ 6444 public CodeableConcept getCategory() { 6445 if (this.category == null) 6446 if (Configuration.errorOnAutoCreate()) 6447 throw new Error("Attempt to auto-create SubDetailComponent.category"); 6448 else if (Configuration.doAutoCreate()) 6449 this.category = new CodeableConcept(); // cc 6450 return this.category; 6451 } 6452 6453 public boolean hasCategory() { 6454 return this.category != null && !this.category.isEmpty(); 6455 } 6456 6457 /** 6458 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 6459 */ 6460 public SubDetailComponent setCategory(CodeableConcept value) { 6461 this.category = value; 6462 return this; 6463 } 6464 6465 /** 6466 * @return {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 6467 */ 6468 public CodeableConcept getService() { 6469 if (this.service == null) 6470 if (Configuration.errorOnAutoCreate()) 6471 throw new Error("Attempt to auto-create SubDetailComponent.service"); 6472 else if (Configuration.doAutoCreate()) 6473 this.service = new CodeableConcept(); // cc 6474 return this.service; 6475 } 6476 6477 public boolean hasService() { 6478 return this.service != null && !this.service.isEmpty(); 6479 } 6480 6481 /** 6482 * @param value {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 6483 */ 6484 public SubDetailComponent setService(CodeableConcept value) { 6485 this.service = value; 6486 return this; 6487 } 6488 6489 /** 6490 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 6491 */ 6492 public List<CodeableConcept> getModifier() { 6493 if (this.modifier == null) 6494 this.modifier = new ArrayList<CodeableConcept>(); 6495 return this.modifier; 6496 } 6497 6498 /** 6499 * @return Returns a reference to <code>this</code> for easy method chaining 6500 */ 6501 public SubDetailComponent setModifier(List<CodeableConcept> theModifier) { 6502 this.modifier = theModifier; 6503 return this; 6504 } 6505 6506 public boolean hasModifier() { 6507 if (this.modifier == null) 6508 return false; 6509 for (CodeableConcept item : this.modifier) 6510 if (!item.isEmpty()) 6511 return true; 6512 return false; 6513 } 6514 6515 public CodeableConcept addModifier() { //3 6516 CodeableConcept t = new CodeableConcept(); 6517 if (this.modifier == null) 6518 this.modifier = new ArrayList<CodeableConcept>(); 6519 this.modifier.add(t); 6520 return t; 6521 } 6522 6523 public SubDetailComponent addModifier(CodeableConcept t) { //3 6524 if (t == null) 6525 return this; 6526 if (this.modifier == null) 6527 this.modifier = new ArrayList<CodeableConcept>(); 6528 this.modifier.add(t); 6529 return this; 6530 } 6531 6532 /** 6533 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 6534 */ 6535 public CodeableConcept getModifierFirstRep() { 6536 if (getModifier().isEmpty()) { 6537 addModifier(); 6538 } 6539 return getModifier().get(0); 6540 } 6541 6542 /** 6543 * @return {@link #programCode} (For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.) 6544 */ 6545 public List<CodeableConcept> getProgramCode() { 6546 if (this.programCode == null) 6547 this.programCode = new ArrayList<CodeableConcept>(); 6548 return this.programCode; 6549 } 6550 6551 /** 6552 * @return Returns a reference to <code>this</code> for easy method chaining 6553 */ 6554 public SubDetailComponent setProgramCode(List<CodeableConcept> theProgramCode) { 6555 this.programCode = theProgramCode; 6556 return this; 6557 } 6558 6559 public boolean hasProgramCode() { 6560 if (this.programCode == null) 6561 return false; 6562 for (CodeableConcept item : this.programCode) 6563 if (!item.isEmpty()) 6564 return true; 6565 return false; 6566 } 6567 6568 public CodeableConcept addProgramCode() { //3 6569 CodeableConcept t = new CodeableConcept(); 6570 if (this.programCode == null) 6571 this.programCode = new ArrayList<CodeableConcept>(); 6572 this.programCode.add(t); 6573 return t; 6574 } 6575 6576 public SubDetailComponent addProgramCode(CodeableConcept t) { //3 6577 if (t == null) 6578 return this; 6579 if (this.programCode == null) 6580 this.programCode = new ArrayList<CodeableConcept>(); 6581 this.programCode.add(t); 6582 return this; 6583 } 6584 6585 /** 6586 * @return The first repetition of repeating field {@link #programCode}, creating it if it does not already exist 6587 */ 6588 public CodeableConcept getProgramCodeFirstRep() { 6589 if (getProgramCode().isEmpty()) { 6590 addProgramCode(); 6591 } 6592 return getProgramCode().get(0); 6593 } 6594 6595 /** 6596 * @return {@link #quantity} (The number of repetitions of a service or product.) 6597 */ 6598 public SimpleQuantity getQuantity() { 6599 if (this.quantity == null) 6600 if (Configuration.errorOnAutoCreate()) 6601 throw new Error("Attempt to auto-create SubDetailComponent.quantity"); 6602 else if (Configuration.doAutoCreate()) 6603 this.quantity = new SimpleQuantity(); // cc 6604 return this.quantity; 6605 } 6606 6607 public boolean hasQuantity() { 6608 return this.quantity != null && !this.quantity.isEmpty(); 6609 } 6610 6611 /** 6612 * @param value {@link #quantity} (The number of repetitions of a service or product.) 6613 */ 6614 public SubDetailComponent setQuantity(SimpleQuantity value) { 6615 this.quantity = value; 6616 return this; 6617 } 6618 6619 /** 6620 * @return {@link #unitPrice} (The fee for an addittional service or product or charge.) 6621 */ 6622 public Money getUnitPrice() { 6623 if (this.unitPrice == null) 6624 if (Configuration.errorOnAutoCreate()) 6625 throw new Error("Attempt to auto-create SubDetailComponent.unitPrice"); 6626 else if (Configuration.doAutoCreate()) 6627 this.unitPrice = new Money(); // cc 6628 return this.unitPrice; 6629 } 6630 6631 public boolean hasUnitPrice() { 6632 return this.unitPrice != null && !this.unitPrice.isEmpty(); 6633 } 6634 6635 /** 6636 * @param value {@link #unitPrice} (The fee for an addittional service or product or charge.) 6637 */ 6638 public SubDetailComponent setUnitPrice(Money value) { 6639 this.unitPrice = value; 6640 return this; 6641 } 6642 6643 /** 6644 * @return {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6645 */ 6646 public DecimalType getFactorElement() { 6647 if (this.factor == null) 6648 if (Configuration.errorOnAutoCreate()) 6649 throw new Error("Attempt to auto-create SubDetailComponent.factor"); 6650 else if (Configuration.doAutoCreate()) 6651 this.factor = new DecimalType(); // bb 6652 return this.factor; 6653 } 6654 6655 public boolean hasFactorElement() { 6656 return this.factor != null && !this.factor.isEmpty(); 6657 } 6658 6659 public boolean hasFactor() { 6660 return this.factor != null && !this.factor.isEmpty(); 6661 } 6662 6663 /** 6664 * @param value {@link #factor} (A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.). This is the underlying object with id, value and extensions. The accessor "getFactor" gives direct access to the value 6665 */ 6666 public SubDetailComponent setFactorElement(DecimalType value) { 6667 this.factor = value; 6668 return this; 6669 } 6670 6671 /** 6672 * @return A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6673 */ 6674 public BigDecimal getFactor() { 6675 return this.factor == null ? null : this.factor.getValue(); 6676 } 6677 6678 /** 6679 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6680 */ 6681 public SubDetailComponent setFactor(BigDecimal value) { 6682 if (value == null) 6683 this.factor = null; 6684 else { 6685 if (this.factor == null) 6686 this.factor = new DecimalType(); 6687 this.factor.setValue(value); 6688 } 6689 return this; 6690 } 6691 6692 /** 6693 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6694 */ 6695 public SubDetailComponent setFactor(long value) { 6696 this.factor = new DecimalType(); 6697 this.factor.setValue(value); 6698 return this; 6699 } 6700 6701 /** 6702 * @param value A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount. 6703 */ 6704 public SubDetailComponent setFactor(double value) { 6705 this.factor = new DecimalType(); 6706 this.factor.setValue(value); 6707 return this; 6708 } 6709 6710 /** 6711 * @return {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6712 */ 6713 public Money getNet() { 6714 if (this.net == null) 6715 if (Configuration.errorOnAutoCreate()) 6716 throw new Error("Attempt to auto-create SubDetailComponent.net"); 6717 else if (Configuration.doAutoCreate()) 6718 this.net = new Money(); // cc 6719 return this.net; 6720 } 6721 6722 public boolean hasNet() { 6723 return this.net != null && !this.net.isEmpty(); 6724 } 6725 6726 /** 6727 * @param value {@link #net} (The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.) 6728 */ 6729 public SubDetailComponent setNet(Money value) { 6730 this.net = value; 6731 return this; 6732 } 6733 6734 /** 6735 * @return {@link #udi} (List of Unique Device Identifiers associated with this line item.) 6736 */ 6737 public List<Reference> getUdi() { 6738 if (this.udi == null) 6739 this.udi = new ArrayList<Reference>(); 6740 return this.udi; 6741 } 6742 6743 /** 6744 * @return Returns a reference to <code>this</code> for easy method chaining 6745 */ 6746 public SubDetailComponent setUdi(List<Reference> theUdi) { 6747 this.udi = theUdi; 6748 return this; 6749 } 6750 6751 public boolean hasUdi() { 6752 if (this.udi == null) 6753 return false; 6754 for (Reference item : this.udi) 6755 if (!item.isEmpty()) 6756 return true; 6757 return false; 6758 } 6759 6760 public Reference addUdi() { //3 6761 Reference t = new Reference(); 6762 if (this.udi == null) 6763 this.udi = new ArrayList<Reference>(); 6764 this.udi.add(t); 6765 return t; 6766 } 6767 6768 public SubDetailComponent addUdi(Reference t) { //3 6769 if (t == null) 6770 return this; 6771 if (this.udi == null) 6772 this.udi = new ArrayList<Reference>(); 6773 this.udi.add(t); 6774 return this; 6775 } 6776 6777 /** 6778 * @return The first repetition of repeating field {@link #udi}, creating it if it does not already exist 6779 */ 6780 public Reference getUdiFirstRep() { 6781 if (getUdi().isEmpty()) { 6782 addUdi(); 6783 } 6784 return getUdi().get(0); 6785 } 6786 6787 /** 6788 * @deprecated Use Reference#setResource(IBaseResource) instead 6789 */ 6790 @Deprecated 6791 public List<Device> getUdiTarget() { 6792 if (this.udiTarget == null) 6793 this.udiTarget = new ArrayList<Device>(); 6794 return this.udiTarget; 6795 } 6796 6797 /** 6798 * @deprecated Use Reference#setResource(IBaseResource) instead 6799 */ 6800 @Deprecated 6801 public Device addUdiTarget() { 6802 Device r = new Device(); 6803 if (this.udiTarget == null) 6804 this.udiTarget = new ArrayList<Device>(); 6805 this.udiTarget.add(r); 6806 return r; 6807 } 6808 6809 /** 6810 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 6811 */ 6812 public List<PositiveIntType> getNoteNumber() { 6813 if (this.noteNumber == null) 6814 this.noteNumber = new ArrayList<PositiveIntType>(); 6815 return this.noteNumber; 6816 } 6817 6818 /** 6819 * @return Returns a reference to <code>this</code> for easy method chaining 6820 */ 6821 public SubDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 6822 this.noteNumber = theNoteNumber; 6823 return this; 6824 } 6825 6826 public boolean hasNoteNumber() { 6827 if (this.noteNumber == null) 6828 return false; 6829 for (PositiveIntType item : this.noteNumber) 6830 if (!item.isEmpty()) 6831 return true; 6832 return false; 6833 } 6834 6835 /** 6836 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 6837 */ 6838 public PositiveIntType addNoteNumberElement() {//2 6839 PositiveIntType t = new PositiveIntType(); 6840 if (this.noteNumber == null) 6841 this.noteNumber = new ArrayList<PositiveIntType>(); 6842 this.noteNumber.add(t); 6843 return t; 6844 } 6845 6846 /** 6847 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 6848 */ 6849 public SubDetailComponent addNoteNumber(int value) { //1 6850 PositiveIntType t = new PositiveIntType(); 6851 t.setValue(value); 6852 if (this.noteNumber == null) 6853 this.noteNumber = new ArrayList<PositiveIntType>(); 6854 this.noteNumber.add(t); 6855 return this; 6856 } 6857 6858 /** 6859 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 6860 */ 6861 public boolean hasNoteNumber(int value) { 6862 if (this.noteNumber == null) 6863 return false; 6864 for (PositiveIntType v : this.noteNumber) 6865 if (v.getValue().equals(value)) // positiveInt 6866 return true; 6867 return false; 6868 } 6869 6870 /** 6871 * @return {@link #adjudication} (The adjudications results.) 6872 */ 6873 public List<AdjudicationComponent> getAdjudication() { 6874 if (this.adjudication == null) 6875 this.adjudication = new ArrayList<AdjudicationComponent>(); 6876 return this.adjudication; 6877 } 6878 6879 /** 6880 * @return Returns a reference to <code>this</code> for easy method chaining 6881 */ 6882 public SubDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 6883 this.adjudication = theAdjudication; 6884 return this; 6885 } 6886 6887 public boolean hasAdjudication() { 6888 if (this.adjudication == null) 6889 return false; 6890 for (AdjudicationComponent item : this.adjudication) 6891 if (!item.isEmpty()) 6892 return true; 6893 return false; 6894 } 6895 6896 public AdjudicationComponent addAdjudication() { //3 6897 AdjudicationComponent t = new AdjudicationComponent(); 6898 if (this.adjudication == null) 6899 this.adjudication = new ArrayList<AdjudicationComponent>(); 6900 this.adjudication.add(t); 6901 return t; 6902 } 6903 6904 public SubDetailComponent addAdjudication(AdjudicationComponent t) { //3 6905 if (t == null) 6906 return this; 6907 if (this.adjudication == null) 6908 this.adjudication = new ArrayList<AdjudicationComponent>(); 6909 this.adjudication.add(t); 6910 return this; 6911 } 6912 6913 /** 6914 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 6915 */ 6916 public AdjudicationComponent getAdjudicationFirstRep() { 6917 if (getAdjudication().isEmpty()) { 6918 addAdjudication(); 6919 } 6920 return getAdjudication().get(0); 6921 } 6922 6923 protected void listChildren(List<Property> children) { 6924 super.listChildren(children); 6925 children.add(new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence)); 6926 children.add(new Property("type", "CodeableConcept", "The type of product or service.", 0, 1, type)); 6927 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 6928 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 6929 children.add(new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service)); 6930 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 6931 children.add(new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode)); 6932 children.add(new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity)); 6933 children.add(new Property("unitPrice", "Money", "The fee for an addittional service or product or charge.", 0, 1, unitPrice)); 6934 children.add(new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor)); 6935 children.add(new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net)); 6936 children.add(new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi)); 6937 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 6938 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 6939 } 6940 6941 @Override 6942 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 6943 switch (_hash) { 6944 case 1349547969: /*sequence*/ return new Property("sequence", "positiveInt", "A service line number.", 0, 1, sequence); 6945 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The type of product or service.", 0, 1, type); 6946 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 6947 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 6948 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service); 6949 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 6950 case 1010065041: /*programCode*/ return new Property("programCode", "CodeableConcept", "For programs which require reson codes for the inclusion, covering, of this billed item under the program or sub-program.", 0, java.lang.Integer.MAX_VALUE, programCode); 6951 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The number of repetitions of a service or product.", 0, 1, quantity); 6952 case -486196699: /*unitPrice*/ return new Property("unitPrice", "Money", "The fee for an addittional service or product or charge.", 0, 1, unitPrice); 6953 case -1282148017: /*factor*/ return new Property("factor", "decimal", "A real number that represents a multiplier used in determining the overall value of services delivered and/or goods received. The concept of a Factor allows for a discount or surcharge multiplier to be applied to a monetary amount.", 0, 1, factor); 6954 case 108957: /*net*/ return new Property("net", "Money", "The quantity times the unit price for an addittional service or product or charge. For example, the formula: unit Quantity * unit Price (Cost per Point) * factor Number * points = net Amount. Quantity, factor and points are assumed to be 1 if not supplied.", 0, 1, net); 6955 case 115642: /*udi*/ return new Property("udi", "Reference(Device)", "List of Unique Device Identifiers associated with this line item.", 0, java.lang.Integer.MAX_VALUE, udi); 6956 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 6957 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 6958 default: return super.getNamedProperty(_hash, _name, _checkValid); 6959 } 6960 6961 } 6962 6963 @Override 6964 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 6965 switch (hash) { 6966 case 1349547969: /*sequence*/ return this.sequence == null ? new Base[0] : new Base[] {this.sequence}; // PositiveIntType 6967 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 6968 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 6969 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 6970 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 6971 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 6972 case 1010065041: /*programCode*/ return this.programCode == null ? new Base[0] : this.programCode.toArray(new Base[this.programCode.size()]); // CodeableConcept 6973 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 6974 case -486196699: /*unitPrice*/ return this.unitPrice == null ? new Base[0] : new Base[] {this.unitPrice}; // Money 6975 case -1282148017: /*factor*/ return this.factor == null ? new Base[0] : new Base[] {this.factor}; // DecimalType 6976 case 108957: /*net*/ return this.net == null ? new Base[0] : new Base[] {this.net}; // Money 6977 case 115642: /*udi*/ return this.udi == null ? new Base[0] : this.udi.toArray(new Base[this.udi.size()]); // Reference 6978 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 6979 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 6980 default: return super.getProperty(hash, name, checkValid); 6981 } 6982 6983 } 6984 6985 @Override 6986 public Base setProperty(int hash, String name, Base value) throws FHIRException { 6987 switch (hash) { 6988 case 1349547969: // sequence 6989 this.sequence = castToPositiveInt(value); // PositiveIntType 6990 return value; 6991 case 3575610: // type 6992 this.type = castToCodeableConcept(value); // CodeableConcept 6993 return value; 6994 case 1099842588: // revenue 6995 this.revenue = castToCodeableConcept(value); // CodeableConcept 6996 return value; 6997 case 50511102: // category 6998 this.category = castToCodeableConcept(value); // CodeableConcept 6999 return value; 7000 case 1984153269: // service 7001 this.service = castToCodeableConcept(value); // CodeableConcept 7002 return value; 7003 case -615513385: // modifier 7004 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 7005 return value; 7006 case 1010065041: // programCode 7007 this.getProgramCode().add(castToCodeableConcept(value)); // CodeableConcept 7008 return value; 7009 case -1285004149: // quantity 7010 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 7011 return value; 7012 case -486196699: // unitPrice 7013 this.unitPrice = castToMoney(value); // Money 7014 return value; 7015 case -1282148017: // factor 7016 this.factor = castToDecimal(value); // DecimalType 7017 return value; 7018 case 108957: // net 7019 this.net = castToMoney(value); // Money 7020 return value; 7021 case 115642: // udi 7022 this.getUdi().add(castToReference(value)); // Reference 7023 return value; 7024 case -1110033957: // noteNumber 7025 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 7026 return value; 7027 case -231349275: // adjudication 7028 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 7029 return value; 7030 default: return super.setProperty(hash, name, value); 7031 } 7032 7033 } 7034 7035 @Override 7036 public Base setProperty(String name, Base value) throws FHIRException { 7037 if (name.equals("sequence")) { 7038 this.sequence = castToPositiveInt(value); // PositiveIntType 7039 } else if (name.equals("type")) { 7040 this.type = castToCodeableConcept(value); // CodeableConcept 7041 } else if (name.equals("revenue")) { 7042 this.revenue = castToCodeableConcept(value); // CodeableConcept 7043 } else if (name.equals("category")) { 7044 this.category = castToCodeableConcept(value); // CodeableConcept 7045 } else if (name.equals("service")) { 7046 this.service = castToCodeableConcept(value); // CodeableConcept 7047 } else if (name.equals("modifier")) { 7048 this.getModifier().add(castToCodeableConcept(value)); 7049 } else if (name.equals("programCode")) { 7050 this.getProgramCode().add(castToCodeableConcept(value)); 7051 } else if (name.equals("quantity")) { 7052 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 7053 } else if (name.equals("unitPrice")) { 7054 this.unitPrice = castToMoney(value); // Money 7055 } else if (name.equals("factor")) { 7056 this.factor = castToDecimal(value); // DecimalType 7057 } else if (name.equals("net")) { 7058 this.net = castToMoney(value); // Money 7059 } else if (name.equals("udi")) { 7060 this.getUdi().add(castToReference(value)); 7061 } else if (name.equals("noteNumber")) { 7062 this.getNoteNumber().add(castToPositiveInt(value)); 7063 } else if (name.equals("adjudication")) { 7064 this.getAdjudication().add((AdjudicationComponent) value); 7065 } else 7066 return super.setProperty(name, value); 7067 return value; 7068 } 7069 7070 @Override 7071 public Base makeProperty(int hash, String name) throws FHIRException { 7072 switch (hash) { 7073 case 1349547969: return getSequenceElement(); 7074 case 3575610: return getType(); 7075 case 1099842588: return getRevenue(); 7076 case 50511102: return getCategory(); 7077 case 1984153269: return getService(); 7078 case -615513385: return addModifier(); 7079 case 1010065041: return addProgramCode(); 7080 case -1285004149: return getQuantity(); 7081 case -486196699: return getUnitPrice(); 7082 case -1282148017: return getFactorElement(); 7083 case 108957: return getNet(); 7084 case 115642: return addUdi(); 7085 case -1110033957: return addNoteNumberElement(); 7086 case -231349275: return addAdjudication(); 7087 default: return super.makeProperty(hash, name); 7088 } 7089 7090 } 7091 7092 @Override 7093 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7094 switch (hash) { 7095 case 1349547969: /*sequence*/ return new String[] {"positiveInt"}; 7096 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 7097 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 7098 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 7099 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 7100 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 7101 case 1010065041: /*programCode*/ return new String[] {"CodeableConcept"}; 7102 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 7103 case -486196699: /*unitPrice*/ return new String[] {"Money"}; 7104 case -1282148017: /*factor*/ return new String[] {"decimal"}; 7105 case 108957: /*net*/ return new String[] {"Money"}; 7106 case 115642: /*udi*/ return new String[] {"Reference"}; 7107 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 7108 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 7109 default: return super.getTypesForProperty(hash, name); 7110 } 7111 7112 } 7113 7114 @Override 7115 public Base addChild(String name) throws FHIRException { 7116 if (name.equals("sequence")) { 7117 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequence"); 7118 } 7119 else if (name.equals("type")) { 7120 this.type = new CodeableConcept(); 7121 return this.type; 7122 } 7123 else if (name.equals("revenue")) { 7124 this.revenue = new CodeableConcept(); 7125 return this.revenue; 7126 } 7127 else if (name.equals("category")) { 7128 this.category = new CodeableConcept(); 7129 return this.category; 7130 } 7131 else if (name.equals("service")) { 7132 this.service = new CodeableConcept(); 7133 return this.service; 7134 } 7135 else if (name.equals("modifier")) { 7136 return addModifier(); 7137 } 7138 else if (name.equals("programCode")) { 7139 return addProgramCode(); 7140 } 7141 else if (name.equals("quantity")) { 7142 this.quantity = new SimpleQuantity(); 7143 return this.quantity; 7144 } 7145 else if (name.equals("unitPrice")) { 7146 this.unitPrice = new Money(); 7147 return this.unitPrice; 7148 } 7149 else if (name.equals("factor")) { 7150 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.factor"); 7151 } 7152 else if (name.equals("net")) { 7153 this.net = new Money(); 7154 return this.net; 7155 } 7156 else if (name.equals("udi")) { 7157 return addUdi(); 7158 } 7159 else if (name.equals("noteNumber")) { 7160 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 7161 } 7162 else if (name.equals("adjudication")) { 7163 return addAdjudication(); 7164 } 7165 else 7166 return super.addChild(name); 7167 } 7168 7169 public SubDetailComponent copy() { 7170 SubDetailComponent dst = new SubDetailComponent(); 7171 copyValues(dst); 7172 dst.sequence = sequence == null ? null : sequence.copy(); 7173 dst.type = type == null ? null : type.copy(); 7174 dst.revenue = revenue == null ? null : revenue.copy(); 7175 dst.category = category == null ? null : category.copy(); 7176 dst.service = service == null ? null : service.copy(); 7177 if (modifier != null) { 7178 dst.modifier = new ArrayList<CodeableConcept>(); 7179 for (CodeableConcept i : modifier) 7180 dst.modifier.add(i.copy()); 7181 }; 7182 if (programCode != null) { 7183 dst.programCode = new ArrayList<CodeableConcept>(); 7184 for (CodeableConcept i : programCode) 7185 dst.programCode.add(i.copy()); 7186 }; 7187 dst.quantity = quantity == null ? null : quantity.copy(); 7188 dst.unitPrice = unitPrice == null ? null : unitPrice.copy(); 7189 dst.factor = factor == null ? null : factor.copy(); 7190 dst.net = net == null ? null : net.copy(); 7191 if (udi != null) { 7192 dst.udi = new ArrayList<Reference>(); 7193 for (Reference i : udi) 7194 dst.udi.add(i.copy()); 7195 }; 7196 if (noteNumber != null) { 7197 dst.noteNumber = new ArrayList<PositiveIntType>(); 7198 for (PositiveIntType i : noteNumber) 7199 dst.noteNumber.add(i.copy()); 7200 }; 7201 if (adjudication != null) { 7202 dst.adjudication = new ArrayList<AdjudicationComponent>(); 7203 for (AdjudicationComponent i : adjudication) 7204 dst.adjudication.add(i.copy()); 7205 }; 7206 return dst; 7207 } 7208 7209 @Override 7210 public boolean equalsDeep(Base other_) { 7211 if (!super.equalsDeep(other_)) 7212 return false; 7213 if (!(other_ instanceof SubDetailComponent)) 7214 return false; 7215 SubDetailComponent o = (SubDetailComponent) other_; 7216 return compareDeep(sequence, o.sequence, true) && compareDeep(type, o.type, true) && compareDeep(revenue, o.revenue, true) 7217 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 7218 && compareDeep(programCode, o.programCode, true) && compareDeep(quantity, o.quantity, true) && compareDeep(unitPrice, o.unitPrice, true) 7219 && compareDeep(factor, o.factor, true) && compareDeep(net, o.net, true) && compareDeep(udi, o.udi, true) 7220 && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 7221 ; 7222 } 7223 7224 @Override 7225 public boolean equalsShallow(Base other_) { 7226 if (!super.equalsShallow(other_)) 7227 return false; 7228 if (!(other_ instanceof SubDetailComponent)) 7229 return false; 7230 SubDetailComponent o = (SubDetailComponent) other_; 7231 return compareValues(sequence, o.sequence, true) && compareValues(factor, o.factor, true) && compareValues(noteNumber, o.noteNumber, true) 7232 ; 7233 } 7234 7235 public boolean isEmpty() { 7236 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequence, type, revenue 7237 , category, service, modifier, programCode, quantity, unitPrice, factor, net 7238 , udi, noteNumber, adjudication); 7239 } 7240 7241 public String fhirType() { 7242 return "ExplanationOfBenefit.item.detail.subDetail"; 7243 7244 } 7245 7246 } 7247 7248 @Block() 7249 public static class AddedItemComponent extends BackboneElement implements IBaseBackboneElement { 7250 /** 7251 * List of input service items which this service line is intended to replace. 7252 */ 7253 @Child(name = "sequenceLinkId", type = {PositiveIntType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7254 @Description(shortDefinition="Service instances", formalDefinition="List of input service items which this service line is intended to replace." ) 7255 protected List<PositiveIntType> sequenceLinkId; 7256 7257 /** 7258 * The type of reveneu or cost center providing the product and/or service. 7259 */ 7260 @Child(name = "revenue", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 7261 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 7262 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 7263 protected CodeableConcept revenue; 7264 7265 /** 7266 * Health Care Service Type Codes to identify the classification of service or benefits. 7267 */ 7268 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 7269 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 7270 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 7271 protected CodeableConcept category; 7272 7273 /** 7274 * If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'. 7275 */ 7276 @Child(name = "service", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 7277 @Description(shortDefinition="Billing Code", formalDefinition="If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'." ) 7278 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 7279 protected CodeableConcept service; 7280 7281 /** 7282 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 7283 */ 7284 @Child(name = "modifier", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7285 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 7286 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 7287 protected List<CodeableConcept> modifier; 7288 7289 /** 7290 * The fee charged for the professional service or product. 7291 */ 7292 @Child(name = "fee", type = {Money.class}, order=6, min=0, max=1, modifier=false, summary=false) 7293 @Description(shortDefinition="Professional fee or Product charge", formalDefinition="The fee charged for the professional service or product." ) 7294 protected Money fee; 7295 7296 /** 7297 * A list of note references to the notes provided below. 7298 */ 7299 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7300 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 7301 protected List<PositiveIntType> noteNumber; 7302 7303 /** 7304 * The adjudications results. 7305 */ 7306 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7307 @Description(shortDefinition="Added items adjudication", formalDefinition="The adjudications results." ) 7308 protected List<AdjudicationComponent> adjudication; 7309 7310 /** 7311 * The second tier service adjudications for payor added services. 7312 */ 7313 @Child(name = "detail", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7314 @Description(shortDefinition="Added items details", formalDefinition="The second tier service adjudications for payor added services." ) 7315 protected List<AddedItemsDetailComponent> detail; 7316 7317 private static final long serialVersionUID = 1969703165L; 7318 7319 /** 7320 * Constructor 7321 */ 7322 public AddedItemComponent() { 7323 super(); 7324 } 7325 7326 /** 7327 * @return {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 7328 */ 7329 public List<PositiveIntType> getSequenceLinkId() { 7330 if (this.sequenceLinkId == null) 7331 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 7332 return this.sequenceLinkId; 7333 } 7334 7335 /** 7336 * @return Returns a reference to <code>this</code> for easy method chaining 7337 */ 7338 public AddedItemComponent setSequenceLinkId(List<PositiveIntType> theSequenceLinkId) { 7339 this.sequenceLinkId = theSequenceLinkId; 7340 return this; 7341 } 7342 7343 public boolean hasSequenceLinkId() { 7344 if (this.sequenceLinkId == null) 7345 return false; 7346 for (PositiveIntType item : this.sequenceLinkId) 7347 if (!item.isEmpty()) 7348 return true; 7349 return false; 7350 } 7351 7352 /** 7353 * @return {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 7354 */ 7355 public PositiveIntType addSequenceLinkIdElement() {//2 7356 PositiveIntType t = new PositiveIntType(); 7357 if (this.sequenceLinkId == null) 7358 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 7359 this.sequenceLinkId.add(t); 7360 return t; 7361 } 7362 7363 /** 7364 * @param value {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 7365 */ 7366 public AddedItemComponent addSequenceLinkId(int value) { //1 7367 PositiveIntType t = new PositiveIntType(); 7368 t.setValue(value); 7369 if (this.sequenceLinkId == null) 7370 this.sequenceLinkId = new ArrayList<PositiveIntType>(); 7371 this.sequenceLinkId.add(t); 7372 return this; 7373 } 7374 7375 /** 7376 * @param value {@link #sequenceLinkId} (List of input service items which this service line is intended to replace.) 7377 */ 7378 public boolean hasSequenceLinkId(int value) { 7379 if (this.sequenceLinkId == null) 7380 return false; 7381 for (PositiveIntType v : this.sequenceLinkId) 7382 if (v.getValue().equals(value)) // positiveInt 7383 return true; 7384 return false; 7385 } 7386 7387 /** 7388 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 7389 */ 7390 public CodeableConcept getRevenue() { 7391 if (this.revenue == null) 7392 if (Configuration.errorOnAutoCreate()) 7393 throw new Error("Attempt to auto-create AddedItemComponent.revenue"); 7394 else if (Configuration.doAutoCreate()) 7395 this.revenue = new CodeableConcept(); // cc 7396 return this.revenue; 7397 } 7398 7399 public boolean hasRevenue() { 7400 return this.revenue != null && !this.revenue.isEmpty(); 7401 } 7402 7403 /** 7404 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 7405 */ 7406 public AddedItemComponent setRevenue(CodeableConcept value) { 7407 this.revenue = value; 7408 return this; 7409 } 7410 7411 /** 7412 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 7413 */ 7414 public CodeableConcept getCategory() { 7415 if (this.category == null) 7416 if (Configuration.errorOnAutoCreate()) 7417 throw new Error("Attempt to auto-create AddedItemComponent.category"); 7418 else if (Configuration.doAutoCreate()) 7419 this.category = new CodeableConcept(); // cc 7420 return this.category; 7421 } 7422 7423 public boolean hasCategory() { 7424 return this.category != null && !this.category.isEmpty(); 7425 } 7426 7427 /** 7428 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 7429 */ 7430 public AddedItemComponent setCategory(CodeableConcept value) { 7431 this.category = value; 7432 return this; 7433 } 7434 7435 /** 7436 * @return {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 7437 */ 7438 public CodeableConcept getService() { 7439 if (this.service == null) 7440 if (Configuration.errorOnAutoCreate()) 7441 throw new Error("Attempt to auto-create AddedItemComponent.service"); 7442 else if (Configuration.doAutoCreate()) 7443 this.service = new CodeableConcept(); // cc 7444 return this.service; 7445 } 7446 7447 public boolean hasService() { 7448 return this.service != null && !this.service.isEmpty(); 7449 } 7450 7451 /** 7452 * @param value {@link #service} (If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.) 7453 */ 7454 public AddedItemComponent setService(CodeableConcept value) { 7455 this.service = value; 7456 return this; 7457 } 7458 7459 /** 7460 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 7461 */ 7462 public List<CodeableConcept> getModifier() { 7463 if (this.modifier == null) 7464 this.modifier = new ArrayList<CodeableConcept>(); 7465 return this.modifier; 7466 } 7467 7468 /** 7469 * @return Returns a reference to <code>this</code> for easy method chaining 7470 */ 7471 public AddedItemComponent setModifier(List<CodeableConcept> theModifier) { 7472 this.modifier = theModifier; 7473 return this; 7474 } 7475 7476 public boolean hasModifier() { 7477 if (this.modifier == null) 7478 return false; 7479 for (CodeableConcept item : this.modifier) 7480 if (!item.isEmpty()) 7481 return true; 7482 return false; 7483 } 7484 7485 public CodeableConcept addModifier() { //3 7486 CodeableConcept t = new CodeableConcept(); 7487 if (this.modifier == null) 7488 this.modifier = new ArrayList<CodeableConcept>(); 7489 this.modifier.add(t); 7490 return t; 7491 } 7492 7493 public AddedItemComponent addModifier(CodeableConcept t) { //3 7494 if (t == null) 7495 return this; 7496 if (this.modifier == null) 7497 this.modifier = new ArrayList<CodeableConcept>(); 7498 this.modifier.add(t); 7499 return this; 7500 } 7501 7502 /** 7503 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 7504 */ 7505 public CodeableConcept getModifierFirstRep() { 7506 if (getModifier().isEmpty()) { 7507 addModifier(); 7508 } 7509 return getModifier().get(0); 7510 } 7511 7512 /** 7513 * @return {@link #fee} (The fee charged for the professional service or product.) 7514 */ 7515 public Money getFee() { 7516 if (this.fee == null) 7517 if (Configuration.errorOnAutoCreate()) 7518 throw new Error("Attempt to auto-create AddedItemComponent.fee"); 7519 else if (Configuration.doAutoCreate()) 7520 this.fee = new Money(); // cc 7521 return this.fee; 7522 } 7523 7524 public boolean hasFee() { 7525 return this.fee != null && !this.fee.isEmpty(); 7526 } 7527 7528 /** 7529 * @param value {@link #fee} (The fee charged for the professional service or product.) 7530 */ 7531 public AddedItemComponent setFee(Money value) { 7532 this.fee = value; 7533 return this; 7534 } 7535 7536 /** 7537 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 7538 */ 7539 public List<PositiveIntType> getNoteNumber() { 7540 if (this.noteNumber == null) 7541 this.noteNumber = new ArrayList<PositiveIntType>(); 7542 return this.noteNumber; 7543 } 7544 7545 /** 7546 * @return Returns a reference to <code>this</code> for easy method chaining 7547 */ 7548 public AddedItemComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 7549 this.noteNumber = theNoteNumber; 7550 return this; 7551 } 7552 7553 public boolean hasNoteNumber() { 7554 if (this.noteNumber == null) 7555 return false; 7556 for (PositiveIntType item : this.noteNumber) 7557 if (!item.isEmpty()) 7558 return true; 7559 return false; 7560 } 7561 7562 /** 7563 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 7564 */ 7565 public PositiveIntType addNoteNumberElement() {//2 7566 PositiveIntType t = new PositiveIntType(); 7567 if (this.noteNumber == null) 7568 this.noteNumber = new ArrayList<PositiveIntType>(); 7569 this.noteNumber.add(t); 7570 return t; 7571 } 7572 7573 /** 7574 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 7575 */ 7576 public AddedItemComponent addNoteNumber(int value) { //1 7577 PositiveIntType t = new PositiveIntType(); 7578 t.setValue(value); 7579 if (this.noteNumber == null) 7580 this.noteNumber = new ArrayList<PositiveIntType>(); 7581 this.noteNumber.add(t); 7582 return this; 7583 } 7584 7585 /** 7586 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 7587 */ 7588 public boolean hasNoteNumber(int value) { 7589 if (this.noteNumber == null) 7590 return false; 7591 for (PositiveIntType v : this.noteNumber) 7592 if (v.getValue().equals(value)) // positiveInt 7593 return true; 7594 return false; 7595 } 7596 7597 /** 7598 * @return {@link #adjudication} (The adjudications results.) 7599 */ 7600 public List<AdjudicationComponent> getAdjudication() { 7601 if (this.adjudication == null) 7602 this.adjudication = new ArrayList<AdjudicationComponent>(); 7603 return this.adjudication; 7604 } 7605 7606 /** 7607 * @return Returns a reference to <code>this</code> for easy method chaining 7608 */ 7609 public AddedItemComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 7610 this.adjudication = theAdjudication; 7611 return this; 7612 } 7613 7614 public boolean hasAdjudication() { 7615 if (this.adjudication == null) 7616 return false; 7617 for (AdjudicationComponent item : this.adjudication) 7618 if (!item.isEmpty()) 7619 return true; 7620 return false; 7621 } 7622 7623 public AdjudicationComponent addAdjudication() { //3 7624 AdjudicationComponent t = new AdjudicationComponent(); 7625 if (this.adjudication == null) 7626 this.adjudication = new ArrayList<AdjudicationComponent>(); 7627 this.adjudication.add(t); 7628 return t; 7629 } 7630 7631 public AddedItemComponent addAdjudication(AdjudicationComponent t) { //3 7632 if (t == null) 7633 return this; 7634 if (this.adjudication == null) 7635 this.adjudication = new ArrayList<AdjudicationComponent>(); 7636 this.adjudication.add(t); 7637 return this; 7638 } 7639 7640 /** 7641 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 7642 */ 7643 public AdjudicationComponent getAdjudicationFirstRep() { 7644 if (getAdjudication().isEmpty()) { 7645 addAdjudication(); 7646 } 7647 return getAdjudication().get(0); 7648 } 7649 7650 /** 7651 * @return {@link #detail} (The second tier service adjudications for payor added services.) 7652 */ 7653 public List<AddedItemsDetailComponent> getDetail() { 7654 if (this.detail == null) 7655 this.detail = new ArrayList<AddedItemsDetailComponent>(); 7656 return this.detail; 7657 } 7658 7659 /** 7660 * @return Returns a reference to <code>this</code> for easy method chaining 7661 */ 7662 public AddedItemComponent setDetail(List<AddedItemsDetailComponent> theDetail) { 7663 this.detail = theDetail; 7664 return this; 7665 } 7666 7667 public boolean hasDetail() { 7668 if (this.detail == null) 7669 return false; 7670 for (AddedItemsDetailComponent item : this.detail) 7671 if (!item.isEmpty()) 7672 return true; 7673 return false; 7674 } 7675 7676 public AddedItemsDetailComponent addDetail() { //3 7677 AddedItemsDetailComponent t = new AddedItemsDetailComponent(); 7678 if (this.detail == null) 7679 this.detail = new ArrayList<AddedItemsDetailComponent>(); 7680 this.detail.add(t); 7681 return t; 7682 } 7683 7684 public AddedItemComponent addDetail(AddedItemsDetailComponent t) { //3 7685 if (t == null) 7686 return this; 7687 if (this.detail == null) 7688 this.detail = new ArrayList<AddedItemsDetailComponent>(); 7689 this.detail.add(t); 7690 return this; 7691 } 7692 7693 /** 7694 * @return The first repetition of repeating field {@link #detail}, creating it if it does not already exist 7695 */ 7696 public AddedItemsDetailComponent getDetailFirstRep() { 7697 if (getDetail().isEmpty()) { 7698 addDetail(); 7699 } 7700 return getDetail().get(0); 7701 } 7702 7703 protected void listChildren(List<Property> children) { 7704 super.listChildren(children); 7705 children.add(new Property("sequenceLinkId", "positiveInt", "List of input service items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, sequenceLinkId)); 7706 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 7707 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 7708 children.add(new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service)); 7709 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 7710 children.add(new Property("fee", "Money", "The fee charged for the professional service or product.", 0, 1, fee)); 7711 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 7712 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 7713 children.add(new Property("detail", "", "The second tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail)); 7714 } 7715 7716 @Override 7717 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 7718 switch (_hash) { 7719 case -1422298666: /*sequenceLinkId*/ return new Property("sequenceLinkId", "positiveInt", "List of input service items which this service line is intended to replace.", 0, java.lang.Integer.MAX_VALUE, sequenceLinkId); 7720 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 7721 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 7722 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "If this is an actual service or product line, ie. not a Group, then use code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). If a grouping item then use a group code to indicate the type of thing being grouped eg. 'glasses' or 'compound'.", 0, 1, service); 7723 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 7724 case 101254: /*fee*/ return new Property("fee", "Money", "The fee charged for the professional service or product.", 0, 1, fee); 7725 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 7726 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 7727 case -1335224239: /*detail*/ return new Property("detail", "", "The second tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, detail); 7728 default: return super.getNamedProperty(_hash, _name, _checkValid); 7729 } 7730 7731 } 7732 7733 @Override 7734 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 7735 switch (hash) { 7736 case -1422298666: /*sequenceLinkId*/ return this.sequenceLinkId == null ? new Base[0] : this.sequenceLinkId.toArray(new Base[this.sequenceLinkId.size()]); // PositiveIntType 7737 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 7738 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 7739 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 7740 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 7741 case 101254: /*fee*/ return this.fee == null ? new Base[0] : new Base[] {this.fee}; // Money 7742 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 7743 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 7744 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : this.detail.toArray(new Base[this.detail.size()]); // AddedItemsDetailComponent 7745 default: return super.getProperty(hash, name, checkValid); 7746 } 7747 7748 } 7749 7750 @Override 7751 public Base setProperty(int hash, String name, Base value) throws FHIRException { 7752 switch (hash) { 7753 case -1422298666: // sequenceLinkId 7754 this.getSequenceLinkId().add(castToPositiveInt(value)); // PositiveIntType 7755 return value; 7756 case 1099842588: // revenue 7757 this.revenue = castToCodeableConcept(value); // CodeableConcept 7758 return value; 7759 case 50511102: // category 7760 this.category = castToCodeableConcept(value); // CodeableConcept 7761 return value; 7762 case 1984153269: // service 7763 this.service = castToCodeableConcept(value); // CodeableConcept 7764 return value; 7765 case -615513385: // modifier 7766 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 7767 return value; 7768 case 101254: // fee 7769 this.fee = castToMoney(value); // Money 7770 return value; 7771 case -1110033957: // noteNumber 7772 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 7773 return value; 7774 case -231349275: // adjudication 7775 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 7776 return value; 7777 case -1335224239: // detail 7778 this.getDetail().add((AddedItemsDetailComponent) value); // AddedItemsDetailComponent 7779 return value; 7780 default: return super.setProperty(hash, name, value); 7781 } 7782 7783 } 7784 7785 @Override 7786 public Base setProperty(String name, Base value) throws FHIRException { 7787 if (name.equals("sequenceLinkId")) { 7788 this.getSequenceLinkId().add(castToPositiveInt(value)); 7789 } else if (name.equals("revenue")) { 7790 this.revenue = castToCodeableConcept(value); // CodeableConcept 7791 } else if (name.equals("category")) { 7792 this.category = castToCodeableConcept(value); // CodeableConcept 7793 } else if (name.equals("service")) { 7794 this.service = castToCodeableConcept(value); // CodeableConcept 7795 } else if (name.equals("modifier")) { 7796 this.getModifier().add(castToCodeableConcept(value)); 7797 } else if (name.equals("fee")) { 7798 this.fee = castToMoney(value); // Money 7799 } else if (name.equals("noteNumber")) { 7800 this.getNoteNumber().add(castToPositiveInt(value)); 7801 } else if (name.equals("adjudication")) { 7802 this.getAdjudication().add((AdjudicationComponent) value); 7803 } else if (name.equals("detail")) { 7804 this.getDetail().add((AddedItemsDetailComponent) value); 7805 } else 7806 return super.setProperty(name, value); 7807 return value; 7808 } 7809 7810 @Override 7811 public Base makeProperty(int hash, String name) throws FHIRException { 7812 switch (hash) { 7813 case -1422298666: return addSequenceLinkIdElement(); 7814 case 1099842588: return getRevenue(); 7815 case 50511102: return getCategory(); 7816 case 1984153269: return getService(); 7817 case -615513385: return addModifier(); 7818 case 101254: return getFee(); 7819 case -1110033957: return addNoteNumberElement(); 7820 case -231349275: return addAdjudication(); 7821 case -1335224239: return addDetail(); 7822 default: return super.makeProperty(hash, name); 7823 } 7824 7825 } 7826 7827 @Override 7828 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 7829 switch (hash) { 7830 case -1422298666: /*sequenceLinkId*/ return new String[] {"positiveInt"}; 7831 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 7832 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 7833 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 7834 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 7835 case 101254: /*fee*/ return new String[] {"Money"}; 7836 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 7837 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 7838 case -1335224239: /*detail*/ return new String[] {}; 7839 default: return super.getTypesForProperty(hash, name); 7840 } 7841 7842 } 7843 7844 @Override 7845 public Base addChild(String name) throws FHIRException { 7846 if (name.equals("sequenceLinkId")) { 7847 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.sequenceLinkId"); 7848 } 7849 else if (name.equals("revenue")) { 7850 this.revenue = new CodeableConcept(); 7851 return this.revenue; 7852 } 7853 else if (name.equals("category")) { 7854 this.category = new CodeableConcept(); 7855 return this.category; 7856 } 7857 else if (name.equals("service")) { 7858 this.service = new CodeableConcept(); 7859 return this.service; 7860 } 7861 else if (name.equals("modifier")) { 7862 return addModifier(); 7863 } 7864 else if (name.equals("fee")) { 7865 this.fee = new Money(); 7866 return this.fee; 7867 } 7868 else if (name.equals("noteNumber")) { 7869 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 7870 } 7871 else if (name.equals("adjudication")) { 7872 return addAdjudication(); 7873 } 7874 else if (name.equals("detail")) { 7875 return addDetail(); 7876 } 7877 else 7878 return super.addChild(name); 7879 } 7880 7881 public AddedItemComponent copy() { 7882 AddedItemComponent dst = new AddedItemComponent(); 7883 copyValues(dst); 7884 if (sequenceLinkId != null) { 7885 dst.sequenceLinkId = new ArrayList<PositiveIntType>(); 7886 for (PositiveIntType i : sequenceLinkId) 7887 dst.sequenceLinkId.add(i.copy()); 7888 }; 7889 dst.revenue = revenue == null ? null : revenue.copy(); 7890 dst.category = category == null ? null : category.copy(); 7891 dst.service = service == null ? null : service.copy(); 7892 if (modifier != null) { 7893 dst.modifier = new ArrayList<CodeableConcept>(); 7894 for (CodeableConcept i : modifier) 7895 dst.modifier.add(i.copy()); 7896 }; 7897 dst.fee = fee == null ? null : fee.copy(); 7898 if (noteNumber != null) { 7899 dst.noteNumber = new ArrayList<PositiveIntType>(); 7900 for (PositiveIntType i : noteNumber) 7901 dst.noteNumber.add(i.copy()); 7902 }; 7903 if (adjudication != null) { 7904 dst.adjudication = new ArrayList<AdjudicationComponent>(); 7905 for (AdjudicationComponent i : adjudication) 7906 dst.adjudication.add(i.copy()); 7907 }; 7908 if (detail != null) { 7909 dst.detail = new ArrayList<AddedItemsDetailComponent>(); 7910 for (AddedItemsDetailComponent i : detail) 7911 dst.detail.add(i.copy()); 7912 }; 7913 return dst; 7914 } 7915 7916 @Override 7917 public boolean equalsDeep(Base other_) { 7918 if (!super.equalsDeep(other_)) 7919 return false; 7920 if (!(other_ instanceof AddedItemComponent)) 7921 return false; 7922 AddedItemComponent o = (AddedItemComponent) other_; 7923 return compareDeep(sequenceLinkId, o.sequenceLinkId, true) && compareDeep(revenue, o.revenue, true) 7924 && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) && compareDeep(modifier, o.modifier, true) 7925 && compareDeep(fee, o.fee, true) && compareDeep(noteNumber, o.noteNumber, true) && compareDeep(adjudication, o.adjudication, true) 7926 && compareDeep(detail, o.detail, true); 7927 } 7928 7929 @Override 7930 public boolean equalsShallow(Base other_) { 7931 if (!super.equalsShallow(other_)) 7932 return false; 7933 if (!(other_ instanceof AddedItemComponent)) 7934 return false; 7935 AddedItemComponent o = (AddedItemComponent) other_; 7936 return compareValues(sequenceLinkId, o.sequenceLinkId, true) && compareValues(noteNumber, o.noteNumber, true) 7937 ; 7938 } 7939 7940 public boolean isEmpty() { 7941 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sequenceLinkId, revenue, category 7942 , service, modifier, fee, noteNumber, adjudication, detail); 7943 } 7944 7945 public String fhirType() { 7946 return "ExplanationOfBenefit.addItem"; 7947 7948 } 7949 7950 } 7951 7952 @Block() 7953 public static class AddedItemsDetailComponent extends BackboneElement implements IBaseBackboneElement { 7954 /** 7955 * The type of reveneu or cost center providing the product and/or service. 7956 */ 7957 @Child(name = "revenue", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 7958 @Description(shortDefinition="Revenue or cost center code", formalDefinition="The type of reveneu or cost center providing the product and/or service." ) 7959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-revenue-center") 7960 protected CodeableConcept revenue; 7961 7962 /** 7963 * Health Care Service Type Codes to identify the classification of service or benefits. 7964 */ 7965 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 7966 @Description(shortDefinition="Type of service or product", formalDefinition="Health Care Service Type Codes to identify the classification of service or benefits." ) 7967 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 7968 protected CodeableConcept category; 7969 7970 /** 7971 * A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI). 7972 */ 7973 @Child(name = "service", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 7974 @Description(shortDefinition="Billing Code", formalDefinition="A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI)." ) 7975 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-uscls") 7976 protected CodeableConcept service; 7977 7978 /** 7979 * Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours. 7980 */ 7981 @Child(name = "modifier", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7982 @Description(shortDefinition="Service/Product billing modifiers", formalDefinition="Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours." ) 7983 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-modifiers") 7984 protected List<CodeableConcept> modifier; 7985 7986 /** 7987 * The fee charged for the professional service or product. 7988 */ 7989 @Child(name = "fee", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 7990 @Description(shortDefinition="Professional fee or Product charge", formalDefinition="The fee charged for the professional service or product." ) 7991 protected Money fee; 7992 7993 /** 7994 * A list of note references to the notes provided below. 7995 */ 7996 @Child(name = "noteNumber", type = {PositiveIntType.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 7997 @Description(shortDefinition="List of note numbers which apply", formalDefinition="A list of note references to the notes provided below." ) 7998 protected List<PositiveIntType> noteNumber; 7999 8000 /** 8001 * The adjudications results. 8002 */ 8003 @Child(name = "adjudication", type = {AdjudicationComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 8004 @Description(shortDefinition="Added items detail adjudication", formalDefinition="The adjudications results." ) 8005 protected List<AdjudicationComponent> adjudication; 8006 8007 private static final long serialVersionUID = -311484980L; 8008 8009 /** 8010 * Constructor 8011 */ 8012 public AddedItemsDetailComponent() { 8013 super(); 8014 } 8015 8016 /** 8017 * @return {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 8018 */ 8019 public CodeableConcept getRevenue() { 8020 if (this.revenue == null) 8021 if (Configuration.errorOnAutoCreate()) 8022 throw new Error("Attempt to auto-create AddedItemsDetailComponent.revenue"); 8023 else if (Configuration.doAutoCreate()) 8024 this.revenue = new CodeableConcept(); // cc 8025 return this.revenue; 8026 } 8027 8028 public boolean hasRevenue() { 8029 return this.revenue != null && !this.revenue.isEmpty(); 8030 } 8031 8032 /** 8033 * @param value {@link #revenue} (The type of reveneu or cost center providing the product and/or service.) 8034 */ 8035 public AddedItemsDetailComponent setRevenue(CodeableConcept value) { 8036 this.revenue = value; 8037 return this; 8038 } 8039 8040 /** 8041 * @return {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 8042 */ 8043 public CodeableConcept getCategory() { 8044 if (this.category == null) 8045 if (Configuration.errorOnAutoCreate()) 8046 throw new Error("Attempt to auto-create AddedItemsDetailComponent.category"); 8047 else if (Configuration.doAutoCreate()) 8048 this.category = new CodeableConcept(); // cc 8049 return this.category; 8050 } 8051 8052 public boolean hasCategory() { 8053 return this.category != null && !this.category.isEmpty(); 8054 } 8055 8056 /** 8057 * @param value {@link #category} (Health Care Service Type Codes to identify the classification of service or benefits.) 8058 */ 8059 public AddedItemsDetailComponent setCategory(CodeableConcept value) { 8060 this.category = value; 8061 return this; 8062 } 8063 8064 /** 8065 * @return {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 8066 */ 8067 public CodeableConcept getService() { 8068 if (this.service == null) 8069 if (Configuration.errorOnAutoCreate()) 8070 throw new Error("Attempt to auto-create AddedItemsDetailComponent.service"); 8071 else if (Configuration.doAutoCreate()) 8072 this.service = new CodeableConcept(); // cc 8073 return this.service; 8074 } 8075 8076 public boolean hasService() { 8077 return this.service != null && !this.service.isEmpty(); 8078 } 8079 8080 /** 8081 * @param value {@link #service} (A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).) 8082 */ 8083 public AddedItemsDetailComponent setService(CodeableConcept value) { 8084 this.service = value; 8085 return this; 8086 } 8087 8088 /** 8089 * @return {@link #modifier} (Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.) 8090 */ 8091 public List<CodeableConcept> getModifier() { 8092 if (this.modifier == null) 8093 this.modifier = new ArrayList<CodeableConcept>(); 8094 return this.modifier; 8095 } 8096 8097 /** 8098 * @return Returns a reference to <code>this</code> for easy method chaining 8099 */ 8100 public AddedItemsDetailComponent setModifier(List<CodeableConcept> theModifier) { 8101 this.modifier = theModifier; 8102 return this; 8103 } 8104 8105 public boolean hasModifier() { 8106 if (this.modifier == null) 8107 return false; 8108 for (CodeableConcept item : this.modifier) 8109 if (!item.isEmpty()) 8110 return true; 8111 return false; 8112 } 8113 8114 public CodeableConcept addModifier() { //3 8115 CodeableConcept t = new CodeableConcept(); 8116 if (this.modifier == null) 8117 this.modifier = new ArrayList<CodeableConcept>(); 8118 this.modifier.add(t); 8119 return t; 8120 } 8121 8122 public AddedItemsDetailComponent addModifier(CodeableConcept t) { //3 8123 if (t == null) 8124 return this; 8125 if (this.modifier == null) 8126 this.modifier = new ArrayList<CodeableConcept>(); 8127 this.modifier.add(t); 8128 return this; 8129 } 8130 8131 /** 8132 * @return The first repetition of repeating field {@link #modifier}, creating it if it does not already exist 8133 */ 8134 public CodeableConcept getModifierFirstRep() { 8135 if (getModifier().isEmpty()) { 8136 addModifier(); 8137 } 8138 return getModifier().get(0); 8139 } 8140 8141 /** 8142 * @return {@link #fee} (The fee charged for the professional service or product.) 8143 */ 8144 public Money getFee() { 8145 if (this.fee == null) 8146 if (Configuration.errorOnAutoCreate()) 8147 throw new Error("Attempt to auto-create AddedItemsDetailComponent.fee"); 8148 else if (Configuration.doAutoCreate()) 8149 this.fee = new Money(); // cc 8150 return this.fee; 8151 } 8152 8153 public boolean hasFee() { 8154 return this.fee != null && !this.fee.isEmpty(); 8155 } 8156 8157 /** 8158 * @param value {@link #fee} (The fee charged for the professional service or product.) 8159 */ 8160 public AddedItemsDetailComponent setFee(Money value) { 8161 this.fee = value; 8162 return this; 8163 } 8164 8165 /** 8166 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 8167 */ 8168 public List<PositiveIntType> getNoteNumber() { 8169 if (this.noteNumber == null) 8170 this.noteNumber = new ArrayList<PositiveIntType>(); 8171 return this.noteNumber; 8172 } 8173 8174 /** 8175 * @return Returns a reference to <code>this</code> for easy method chaining 8176 */ 8177 public AddedItemsDetailComponent setNoteNumber(List<PositiveIntType> theNoteNumber) { 8178 this.noteNumber = theNoteNumber; 8179 return this; 8180 } 8181 8182 public boolean hasNoteNumber() { 8183 if (this.noteNumber == null) 8184 return false; 8185 for (PositiveIntType item : this.noteNumber) 8186 if (!item.isEmpty()) 8187 return true; 8188 return false; 8189 } 8190 8191 /** 8192 * @return {@link #noteNumber} (A list of note references to the notes provided below.) 8193 */ 8194 public PositiveIntType addNoteNumberElement() {//2 8195 PositiveIntType t = new PositiveIntType(); 8196 if (this.noteNumber == null) 8197 this.noteNumber = new ArrayList<PositiveIntType>(); 8198 this.noteNumber.add(t); 8199 return t; 8200 } 8201 8202 /** 8203 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 8204 */ 8205 public AddedItemsDetailComponent addNoteNumber(int value) { //1 8206 PositiveIntType t = new PositiveIntType(); 8207 t.setValue(value); 8208 if (this.noteNumber == null) 8209 this.noteNumber = new ArrayList<PositiveIntType>(); 8210 this.noteNumber.add(t); 8211 return this; 8212 } 8213 8214 /** 8215 * @param value {@link #noteNumber} (A list of note references to the notes provided below.) 8216 */ 8217 public boolean hasNoteNumber(int value) { 8218 if (this.noteNumber == null) 8219 return false; 8220 for (PositiveIntType v : this.noteNumber) 8221 if (v.getValue().equals(value)) // positiveInt 8222 return true; 8223 return false; 8224 } 8225 8226 /** 8227 * @return {@link #adjudication} (The adjudications results.) 8228 */ 8229 public List<AdjudicationComponent> getAdjudication() { 8230 if (this.adjudication == null) 8231 this.adjudication = new ArrayList<AdjudicationComponent>(); 8232 return this.adjudication; 8233 } 8234 8235 /** 8236 * @return Returns a reference to <code>this</code> for easy method chaining 8237 */ 8238 public AddedItemsDetailComponent setAdjudication(List<AdjudicationComponent> theAdjudication) { 8239 this.adjudication = theAdjudication; 8240 return this; 8241 } 8242 8243 public boolean hasAdjudication() { 8244 if (this.adjudication == null) 8245 return false; 8246 for (AdjudicationComponent item : this.adjudication) 8247 if (!item.isEmpty()) 8248 return true; 8249 return false; 8250 } 8251 8252 public AdjudicationComponent addAdjudication() { //3 8253 AdjudicationComponent t = new AdjudicationComponent(); 8254 if (this.adjudication == null) 8255 this.adjudication = new ArrayList<AdjudicationComponent>(); 8256 this.adjudication.add(t); 8257 return t; 8258 } 8259 8260 public AddedItemsDetailComponent addAdjudication(AdjudicationComponent t) { //3 8261 if (t == null) 8262 return this; 8263 if (this.adjudication == null) 8264 this.adjudication = new ArrayList<AdjudicationComponent>(); 8265 this.adjudication.add(t); 8266 return this; 8267 } 8268 8269 /** 8270 * @return The first repetition of repeating field {@link #adjudication}, creating it if it does not already exist 8271 */ 8272 public AdjudicationComponent getAdjudicationFirstRep() { 8273 if (getAdjudication().isEmpty()) { 8274 addAdjudication(); 8275 } 8276 return getAdjudication().get(0); 8277 } 8278 8279 protected void listChildren(List<Property> children) { 8280 super.listChildren(children); 8281 children.add(new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue)); 8282 children.add(new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category)); 8283 children.add(new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service)); 8284 children.add(new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier)); 8285 children.add(new Property("fee", "Money", "The fee charged for the professional service or product.", 0, 1, fee)); 8286 children.add(new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber)); 8287 children.add(new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication)); 8288 } 8289 8290 @Override 8291 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8292 switch (_hash) { 8293 case 1099842588: /*revenue*/ return new Property("revenue", "CodeableConcept", "The type of reveneu or cost center providing the product and/or service.", 0, 1, revenue); 8294 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Health Care Service Type Codes to identify the classification of service or benefits.", 0, 1, category); 8295 case 1984153269: /*service*/ return new Property("service", "CodeableConcept", "A code to indicate the Professional Service or Product supplied (eg. CTP, HCPCS,USCLS,ICD10, NCPDP,DIN,ACHI,CCI).", 0, 1, service); 8296 case -615513385: /*modifier*/ return new Property("modifier", "CodeableConcept", "Item typification or modifiers codes, eg for Oral whether the treatment is cosmetic or associated with TMJ, or for medical whether the treatment was outside the clinic or out of office hours.", 0, java.lang.Integer.MAX_VALUE, modifier); 8297 case 101254: /*fee*/ return new Property("fee", "Money", "The fee charged for the professional service or product.", 0, 1, fee); 8298 case -1110033957: /*noteNumber*/ return new Property("noteNumber", "positiveInt", "A list of note references to the notes provided below.", 0, java.lang.Integer.MAX_VALUE, noteNumber); 8299 case -231349275: /*adjudication*/ return new Property("adjudication", "@ExplanationOfBenefit.item.adjudication", "The adjudications results.", 0, java.lang.Integer.MAX_VALUE, adjudication); 8300 default: return super.getNamedProperty(_hash, _name, _checkValid); 8301 } 8302 8303 } 8304 8305 @Override 8306 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8307 switch (hash) { 8308 case 1099842588: /*revenue*/ return this.revenue == null ? new Base[0] : new Base[] {this.revenue}; // CodeableConcept 8309 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 8310 case 1984153269: /*service*/ return this.service == null ? new Base[0] : new Base[] {this.service}; // CodeableConcept 8311 case -615513385: /*modifier*/ return this.modifier == null ? new Base[0] : this.modifier.toArray(new Base[this.modifier.size()]); // CodeableConcept 8312 case 101254: /*fee*/ return this.fee == null ? new Base[0] : new Base[] {this.fee}; // Money 8313 case -1110033957: /*noteNumber*/ return this.noteNumber == null ? new Base[0] : this.noteNumber.toArray(new Base[this.noteNumber.size()]); // PositiveIntType 8314 case -231349275: /*adjudication*/ return this.adjudication == null ? new Base[0] : this.adjudication.toArray(new Base[this.adjudication.size()]); // AdjudicationComponent 8315 default: return super.getProperty(hash, name, checkValid); 8316 } 8317 8318 } 8319 8320 @Override 8321 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8322 switch (hash) { 8323 case 1099842588: // revenue 8324 this.revenue = castToCodeableConcept(value); // CodeableConcept 8325 return value; 8326 case 50511102: // category 8327 this.category = castToCodeableConcept(value); // CodeableConcept 8328 return value; 8329 case 1984153269: // service 8330 this.service = castToCodeableConcept(value); // CodeableConcept 8331 return value; 8332 case -615513385: // modifier 8333 this.getModifier().add(castToCodeableConcept(value)); // CodeableConcept 8334 return value; 8335 case 101254: // fee 8336 this.fee = castToMoney(value); // Money 8337 return value; 8338 case -1110033957: // noteNumber 8339 this.getNoteNumber().add(castToPositiveInt(value)); // PositiveIntType 8340 return value; 8341 case -231349275: // adjudication 8342 this.getAdjudication().add((AdjudicationComponent) value); // AdjudicationComponent 8343 return value; 8344 default: return super.setProperty(hash, name, value); 8345 } 8346 8347 } 8348 8349 @Override 8350 public Base setProperty(String name, Base value) throws FHIRException { 8351 if (name.equals("revenue")) { 8352 this.revenue = castToCodeableConcept(value); // CodeableConcept 8353 } else if (name.equals("category")) { 8354 this.category = castToCodeableConcept(value); // CodeableConcept 8355 } else if (name.equals("service")) { 8356 this.service = castToCodeableConcept(value); // CodeableConcept 8357 } else if (name.equals("modifier")) { 8358 this.getModifier().add(castToCodeableConcept(value)); 8359 } else if (name.equals("fee")) { 8360 this.fee = castToMoney(value); // Money 8361 } else if (name.equals("noteNumber")) { 8362 this.getNoteNumber().add(castToPositiveInt(value)); 8363 } else if (name.equals("adjudication")) { 8364 this.getAdjudication().add((AdjudicationComponent) value); 8365 } else 8366 return super.setProperty(name, value); 8367 return value; 8368 } 8369 8370 @Override 8371 public Base makeProperty(int hash, String name) throws FHIRException { 8372 switch (hash) { 8373 case 1099842588: return getRevenue(); 8374 case 50511102: return getCategory(); 8375 case 1984153269: return getService(); 8376 case -615513385: return addModifier(); 8377 case 101254: return getFee(); 8378 case -1110033957: return addNoteNumberElement(); 8379 case -231349275: return addAdjudication(); 8380 default: return super.makeProperty(hash, name); 8381 } 8382 8383 } 8384 8385 @Override 8386 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8387 switch (hash) { 8388 case 1099842588: /*revenue*/ return new String[] {"CodeableConcept"}; 8389 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 8390 case 1984153269: /*service*/ return new String[] {"CodeableConcept"}; 8391 case -615513385: /*modifier*/ return new String[] {"CodeableConcept"}; 8392 case 101254: /*fee*/ return new String[] {"Money"}; 8393 case -1110033957: /*noteNumber*/ return new String[] {"positiveInt"}; 8394 case -231349275: /*adjudication*/ return new String[] {"@ExplanationOfBenefit.item.adjudication"}; 8395 default: return super.getTypesForProperty(hash, name); 8396 } 8397 8398 } 8399 8400 @Override 8401 public Base addChild(String name) throws FHIRException { 8402 if (name.equals("revenue")) { 8403 this.revenue = new CodeableConcept(); 8404 return this.revenue; 8405 } 8406 else if (name.equals("category")) { 8407 this.category = new CodeableConcept(); 8408 return this.category; 8409 } 8410 else if (name.equals("service")) { 8411 this.service = new CodeableConcept(); 8412 return this.service; 8413 } 8414 else if (name.equals("modifier")) { 8415 return addModifier(); 8416 } 8417 else if (name.equals("fee")) { 8418 this.fee = new Money(); 8419 return this.fee; 8420 } 8421 else if (name.equals("noteNumber")) { 8422 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.noteNumber"); 8423 } 8424 else if (name.equals("adjudication")) { 8425 return addAdjudication(); 8426 } 8427 else 8428 return super.addChild(name); 8429 } 8430 8431 public AddedItemsDetailComponent copy() { 8432 AddedItemsDetailComponent dst = new AddedItemsDetailComponent(); 8433 copyValues(dst); 8434 dst.revenue = revenue == null ? null : revenue.copy(); 8435 dst.category = category == null ? null : category.copy(); 8436 dst.service = service == null ? null : service.copy(); 8437 if (modifier != null) { 8438 dst.modifier = new ArrayList<CodeableConcept>(); 8439 for (CodeableConcept i : modifier) 8440 dst.modifier.add(i.copy()); 8441 }; 8442 dst.fee = fee == null ? null : fee.copy(); 8443 if (noteNumber != null) { 8444 dst.noteNumber = new ArrayList<PositiveIntType>(); 8445 for (PositiveIntType i : noteNumber) 8446 dst.noteNumber.add(i.copy()); 8447 }; 8448 if (adjudication != null) { 8449 dst.adjudication = new ArrayList<AdjudicationComponent>(); 8450 for (AdjudicationComponent i : adjudication) 8451 dst.adjudication.add(i.copy()); 8452 }; 8453 return dst; 8454 } 8455 8456 @Override 8457 public boolean equalsDeep(Base other_) { 8458 if (!super.equalsDeep(other_)) 8459 return false; 8460 if (!(other_ instanceof AddedItemsDetailComponent)) 8461 return false; 8462 AddedItemsDetailComponent o = (AddedItemsDetailComponent) other_; 8463 return compareDeep(revenue, o.revenue, true) && compareDeep(category, o.category, true) && compareDeep(service, o.service, true) 8464 && compareDeep(modifier, o.modifier, true) && compareDeep(fee, o.fee, true) && compareDeep(noteNumber, o.noteNumber, true) 8465 && compareDeep(adjudication, o.adjudication, true); 8466 } 8467 8468 @Override 8469 public boolean equalsShallow(Base other_) { 8470 if (!super.equalsShallow(other_)) 8471 return false; 8472 if (!(other_ instanceof AddedItemsDetailComponent)) 8473 return false; 8474 AddedItemsDetailComponent o = (AddedItemsDetailComponent) other_; 8475 return compareValues(noteNumber, o.noteNumber, true); 8476 } 8477 8478 public boolean isEmpty() { 8479 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(revenue, category, service 8480 , modifier, fee, noteNumber, adjudication); 8481 } 8482 8483 public String fhirType() { 8484 return "ExplanationOfBenefit.addItem.detail"; 8485 8486 } 8487 8488 } 8489 8490 @Block() 8491 public static class PaymentComponent extends BackboneElement implements IBaseBackboneElement { 8492 /** 8493 * Whether this represents partial or complete payment of the claim. 8494 */ 8495 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 8496 @Description(shortDefinition="Partial or Complete", formalDefinition="Whether this represents partial or complete payment of the claim." ) 8497 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/ex-paymenttype") 8498 protected CodeableConcept type; 8499 8500 /** 8501 * Adjustment to the payment of this transaction which is not related to adjudication of this transaction. 8502 */ 8503 @Child(name = "adjustment", type = {Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 8504 @Description(shortDefinition="Payment adjustment for non-Claim issues", formalDefinition="Adjustment to the payment of this transaction which is not related to adjudication of this transaction." ) 8505 protected Money adjustment; 8506 8507 /** 8508 * Reason for the payment adjustment. 8509 */ 8510 @Child(name = "adjustmentReason", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 8511 @Description(shortDefinition="Explanation for the non-claim adjustment", formalDefinition="Reason for the payment adjustment." ) 8512 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/payment-adjustment-reason") 8513 protected CodeableConcept adjustmentReason; 8514 8515 /** 8516 * Estimated payment date. 8517 */ 8518 @Child(name = "date", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 8519 @Description(shortDefinition="Expected date of Payment", formalDefinition="Estimated payment date." ) 8520 protected DateType date; 8521 8522 /** 8523 * Payable less any payment adjustment. 8524 */ 8525 @Child(name = "amount", type = {Money.class}, order=5, min=0, max=1, modifier=false, summary=false) 8526 @Description(shortDefinition="Payable amount after adjustment", formalDefinition="Payable less any payment adjustment." ) 8527 protected Money amount; 8528 8529 /** 8530 * Payment identifer. 8531 */ 8532 @Child(name = "identifier", type = {Identifier.class}, order=6, min=0, max=1, modifier=false, summary=false) 8533 @Description(shortDefinition="Identifier of the payment instrument", formalDefinition="Payment identifer." ) 8534 protected Identifier identifier; 8535 8536 private static final long serialVersionUID = 1539906026L; 8537 8538 /** 8539 * Constructor 8540 */ 8541 public PaymentComponent() { 8542 super(); 8543 } 8544 8545 /** 8546 * @return {@link #type} (Whether this represents partial or complete payment of the claim.) 8547 */ 8548 public CodeableConcept getType() { 8549 if (this.type == null) 8550 if (Configuration.errorOnAutoCreate()) 8551 throw new Error("Attempt to auto-create PaymentComponent.type"); 8552 else if (Configuration.doAutoCreate()) 8553 this.type = new CodeableConcept(); // cc 8554 return this.type; 8555 } 8556 8557 public boolean hasType() { 8558 return this.type != null && !this.type.isEmpty(); 8559 } 8560 8561 /** 8562 * @param value {@link #type} (Whether this represents partial or complete payment of the claim.) 8563 */ 8564 public PaymentComponent setType(CodeableConcept value) { 8565 this.type = value; 8566 return this; 8567 } 8568 8569 /** 8570 * @return {@link #adjustment} (Adjustment to the payment of this transaction which is not related to adjudication of this transaction.) 8571 */ 8572 public Money getAdjustment() { 8573 if (this.adjustment == null) 8574 if (Configuration.errorOnAutoCreate()) 8575 throw new Error("Attempt to auto-create PaymentComponent.adjustment"); 8576 else if (Configuration.doAutoCreate()) 8577 this.adjustment = new Money(); // cc 8578 return this.adjustment; 8579 } 8580 8581 public boolean hasAdjustment() { 8582 return this.adjustment != null && !this.adjustment.isEmpty(); 8583 } 8584 8585 /** 8586 * @param value {@link #adjustment} (Adjustment to the payment of this transaction which is not related to adjudication of this transaction.) 8587 */ 8588 public PaymentComponent setAdjustment(Money value) { 8589 this.adjustment = value; 8590 return this; 8591 } 8592 8593 /** 8594 * @return {@link #adjustmentReason} (Reason for the payment adjustment.) 8595 */ 8596 public CodeableConcept getAdjustmentReason() { 8597 if (this.adjustmentReason == null) 8598 if (Configuration.errorOnAutoCreate()) 8599 throw new Error("Attempt to auto-create PaymentComponent.adjustmentReason"); 8600 else if (Configuration.doAutoCreate()) 8601 this.adjustmentReason = new CodeableConcept(); // cc 8602 return this.adjustmentReason; 8603 } 8604 8605 public boolean hasAdjustmentReason() { 8606 return this.adjustmentReason != null && !this.adjustmentReason.isEmpty(); 8607 } 8608 8609 /** 8610 * @param value {@link #adjustmentReason} (Reason for the payment adjustment.) 8611 */ 8612 public PaymentComponent setAdjustmentReason(CodeableConcept value) { 8613 this.adjustmentReason = value; 8614 return this; 8615 } 8616 8617 /** 8618 * @return {@link #date} (Estimated payment date.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 8619 */ 8620 public DateType getDateElement() { 8621 if (this.date == null) 8622 if (Configuration.errorOnAutoCreate()) 8623 throw new Error("Attempt to auto-create PaymentComponent.date"); 8624 else if (Configuration.doAutoCreate()) 8625 this.date = new DateType(); // bb 8626 return this.date; 8627 } 8628 8629 public boolean hasDateElement() { 8630 return this.date != null && !this.date.isEmpty(); 8631 } 8632 8633 public boolean hasDate() { 8634 return this.date != null && !this.date.isEmpty(); 8635 } 8636 8637 /** 8638 * @param value {@link #date} (Estimated payment date.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 8639 */ 8640 public PaymentComponent setDateElement(DateType value) { 8641 this.date = value; 8642 return this; 8643 } 8644 8645 /** 8646 * @return Estimated payment date. 8647 */ 8648 public Date getDate() { 8649 return this.date == null ? null : this.date.getValue(); 8650 } 8651 8652 /** 8653 * @param value Estimated payment date. 8654 */ 8655 public PaymentComponent setDate(Date value) { 8656 if (value == null) 8657 this.date = null; 8658 else { 8659 if (this.date == null) 8660 this.date = new DateType(); 8661 this.date.setValue(value); 8662 } 8663 return this; 8664 } 8665 8666 /** 8667 * @return {@link #amount} (Payable less any payment adjustment.) 8668 */ 8669 public Money getAmount() { 8670 if (this.amount == null) 8671 if (Configuration.errorOnAutoCreate()) 8672 throw new Error("Attempt to auto-create PaymentComponent.amount"); 8673 else if (Configuration.doAutoCreate()) 8674 this.amount = new Money(); // cc 8675 return this.amount; 8676 } 8677 8678 public boolean hasAmount() { 8679 return this.amount != null && !this.amount.isEmpty(); 8680 } 8681 8682 /** 8683 * @param value {@link #amount} (Payable less any payment adjustment.) 8684 */ 8685 public PaymentComponent setAmount(Money value) { 8686 this.amount = value; 8687 return this; 8688 } 8689 8690 /** 8691 * @return {@link #identifier} (Payment identifer.) 8692 */ 8693 public Identifier getIdentifier() { 8694 if (this.identifier == null) 8695 if (Configuration.errorOnAutoCreate()) 8696 throw new Error("Attempt to auto-create PaymentComponent.identifier"); 8697 else if (Configuration.doAutoCreate()) 8698 this.identifier = new Identifier(); // cc 8699 return this.identifier; 8700 } 8701 8702 public boolean hasIdentifier() { 8703 return this.identifier != null && !this.identifier.isEmpty(); 8704 } 8705 8706 /** 8707 * @param value {@link #identifier} (Payment identifer.) 8708 */ 8709 public PaymentComponent setIdentifier(Identifier value) { 8710 this.identifier = value; 8711 return this; 8712 } 8713 8714 protected void listChildren(List<Property> children) { 8715 super.listChildren(children); 8716 children.add(new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the claim.", 0, 1, type)); 8717 children.add(new Property("adjustment", "Money", "Adjustment to the payment of this transaction which is not related to adjudication of this transaction.", 0, 1, adjustment)); 8718 children.add(new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason)); 8719 children.add(new Property("date", "date", "Estimated payment date.", 0, 1, date)); 8720 children.add(new Property("amount", "Money", "Payable less any payment adjustment.", 0, 1, amount)); 8721 children.add(new Property("identifier", "Identifier", "Payment identifer.", 0, 1, identifier)); 8722 } 8723 8724 @Override 8725 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 8726 switch (_hash) { 8727 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Whether this represents partial or complete payment of the claim.", 0, 1, type); 8728 case 1977085293: /*adjustment*/ return new Property("adjustment", "Money", "Adjustment to the payment of this transaction which is not related to adjudication of this transaction.", 0, 1, adjustment); 8729 case -1255938543: /*adjustmentReason*/ return new Property("adjustmentReason", "CodeableConcept", "Reason for the payment adjustment.", 0, 1, adjustmentReason); 8730 case 3076014: /*date*/ return new Property("date", "date", "Estimated payment date.", 0, 1, date); 8731 case -1413853096: /*amount*/ return new Property("amount", "Money", "Payable less any payment adjustment.", 0, 1, amount); 8732 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Payment identifer.", 0, 1, identifier); 8733 default: return super.getNamedProperty(_hash, _name, _checkValid); 8734 } 8735 8736 } 8737 8738 @Override 8739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 8740 switch (hash) { 8741 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 8742 case 1977085293: /*adjustment*/ return this.adjustment == null ? new Base[0] : new Base[] {this.adjustment}; // Money 8743 case -1255938543: /*adjustmentReason*/ return this.adjustmentReason == null ? new Base[0] : new Base[] {this.adjustmentReason}; // CodeableConcept 8744 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateType 8745 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Money 8746 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 8747 default: return super.getProperty(hash, name, checkValid); 8748 } 8749 8750 } 8751 8752 @Override 8753 public Base setProperty(int hash, String name, Base value) throws FHIRException { 8754 switch (hash) { 8755 case 3575610: // type 8756 this.type = castToCodeableConcept(value); // CodeableConcept 8757 return value; 8758 case 1977085293: // adjustment 8759 this.adjustment = castToMoney(value); // Money 8760 return value; 8761 case -1255938543: // adjustmentReason 8762 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 8763 return value; 8764 case 3076014: // date 8765 this.date = castToDate(value); // DateType 8766 return value; 8767 case -1413853096: // amount 8768 this.amount = castToMoney(value); // Money 8769 return value; 8770 case -1618432855: // identifier 8771 this.identifier = castToIdentifier(value); // Identifier 8772 return value; 8773 default: return super.setProperty(hash, name, value); 8774 } 8775 8776 } 8777 8778 @Override 8779 public Base setProperty(String name, Base value) throws FHIRException { 8780 if (name.equals("type")) { 8781 this.type = castToCodeableConcept(value); // CodeableConcept 8782 } else if (name.equals("adjustment")) { 8783 this.adjustment = castToMoney(value); // Money 8784 } else if (name.equals("adjustmentReason")) { 8785 this.adjustmentReason = castToCodeableConcept(value); // CodeableConcept 8786 } else if (name.equals("date")) { 8787 this.date = castToDate(value); // DateType 8788 } else if (name.equals("amount")) { 8789 this.amount = castToMoney(value); // Money 8790 } else if (name.equals("identifier")) { 8791 this.identifier = castToIdentifier(value); // Identifier 8792 } else 8793 return super.setProperty(name, value); 8794 return value; 8795 } 8796 8797 @Override 8798 public Base makeProperty(int hash, String name) throws FHIRException { 8799 switch (hash) { 8800 case 3575610: return getType(); 8801 case 1977085293: return getAdjustment(); 8802 case -1255938543: return getAdjustmentReason(); 8803 case 3076014: return getDateElement(); 8804 case -1413853096: return getAmount(); 8805 case -1618432855: return getIdentifier(); 8806 default: return super.makeProperty(hash, name); 8807 } 8808 8809 } 8810 8811 @Override 8812 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 8813 switch (hash) { 8814 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 8815 case 1977085293: /*adjustment*/ return new String[] {"Money"}; 8816 case -1255938543: /*adjustmentReason*/ return new String[] {"CodeableConcept"}; 8817 case 3076014: /*date*/ return new String[] {"date"}; 8818 case -1413853096: /*amount*/ return new String[] {"Money"}; 8819 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 8820 default: return super.getTypesForProperty(hash, name); 8821 } 8822 8823 } 8824 8825 @Override 8826 public Base addChild(String name) throws FHIRException { 8827 if (name.equals("type")) { 8828 this.type = new CodeableConcept(); 8829 return this.type; 8830 } 8831 else if (name.equals("adjustment")) { 8832 this.adjustment = new Money(); 8833 return this.adjustment; 8834 } 8835 else if (name.equals("adjustmentReason")) { 8836 this.adjustmentReason = new CodeableConcept(); 8837 return this.adjustmentReason; 8838 } 8839 else if (name.equals("date")) { 8840 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.date"); 8841 } 8842 else if (name.equals("amount")) { 8843 this.amount = new Money(); 8844 return this.amount; 8845 } 8846 else if (name.equals("identifier")) { 8847 this.identifier = new Identifier(); 8848 return this.identifier; 8849 } 8850 else 8851 return super.addChild(name); 8852 } 8853 8854 public PaymentComponent copy() { 8855 PaymentComponent dst = new PaymentComponent(); 8856 copyValues(dst); 8857 dst.type = type == null ? null : type.copy(); 8858 dst.adjustment = adjustment == null ? null : adjustment.copy(); 8859 dst.adjustmentReason = adjustmentReason == null ? null : adjustmentReason.copy(); 8860 dst.date = date == null ? null : date.copy(); 8861 dst.amount = amount == null ? null : amount.copy(); 8862 dst.identifier = identifier == null ? null : identifier.copy(); 8863 return dst; 8864 } 8865 8866 @Override 8867 public boolean equalsDeep(Base other_) { 8868 if (!super.equalsDeep(other_)) 8869 return false; 8870 if (!(other_ instanceof PaymentComponent)) 8871 return false; 8872 PaymentComponent o = (PaymentComponent) other_; 8873 return compareDeep(type, o.type, true) && compareDeep(adjustment, o.adjustment, true) && compareDeep(adjustmentReason, o.adjustmentReason, true) 8874 && compareDeep(date, o.date, true) && compareDeep(amount, o.amount, true) && compareDeep(identifier, o.identifier, true) 8875 ; 8876 } 8877 8878 @Override 8879 public boolean equalsShallow(Base other_) { 8880 if (!super.equalsShallow(other_)) 8881 return false; 8882 if (!(other_ instanceof PaymentComponent)) 8883 return false; 8884 PaymentComponent o = (PaymentComponent) other_; 8885 return compareValues(date, o.date, true); 8886 } 8887 8888 public boolean isEmpty() { 8889 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, adjustment, adjustmentReason 8890 , date, amount, identifier); 8891 } 8892 8893 public String fhirType() { 8894 return "ExplanationOfBenefit.payment"; 8895 8896 } 8897 8898 } 8899 8900 @Block() 8901 public static class NoteComponent extends BackboneElement implements IBaseBackboneElement { 8902 /** 8903 * An integer associated with each note which may be referred to from each service line item. 8904 */ 8905 @Child(name = "number", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 8906 @Description(shortDefinition="Sequence number for this note", formalDefinition="An integer associated with each note which may be referred to from each service line item." ) 8907 protected PositiveIntType number; 8908 8909 /** 8910 * The note purpose: Print/Display. 8911 */ 8912 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 8913 @Description(shortDefinition="display | print | printoper", formalDefinition="The note purpose: Print/Display." ) 8914 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/note-type") 8915 protected CodeableConcept type; 8916 8917 /** 8918 * The note text. 8919 */ 8920 @Child(name = "text", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 8921 @Description(shortDefinition="Note explanitory text", formalDefinition="The note text." ) 8922 protected StringType text; 8923 8924 /** 8925 * The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English. 8926 */ 8927 @Child(name = "language", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 8928 @Description(shortDefinition="Language if different from the resource", formalDefinition="The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English." ) 8929 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/languages") 8930 protected CodeableConcept language; 8931 8932 private static final long serialVersionUID = -944255449L; 8933 8934 /** 8935 * Constructor 8936 */ 8937 public NoteComponent() { 8938 super(); 8939 } 8940 8941 /** 8942 * @return {@link #number} (An integer associated with each note which may be referred to from each service line item.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 8943 */ 8944 public PositiveIntType getNumberElement() { 8945 if (this.number == null) 8946 if (Configuration.errorOnAutoCreate()) 8947 throw new Error("Attempt to auto-create NoteComponent.number"); 8948 else if (Configuration.doAutoCreate()) 8949 this.number = new PositiveIntType(); // bb 8950 return this.number; 8951 } 8952 8953 public boolean hasNumberElement() { 8954 return this.number != null && !this.number.isEmpty(); 8955 } 8956 8957 public boolean hasNumber() { 8958 return this.number != null && !this.number.isEmpty(); 8959 } 8960 8961 /** 8962 * @param value {@link #number} (An integer associated with each note which may be referred to from each service line item.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 8963 */ 8964 public NoteComponent setNumberElement(PositiveIntType value) { 8965 this.number = value; 8966 return this; 8967 } 8968 8969 /** 8970 * @return An integer associated with each note which may be referred to from each service line item. 8971 */ 8972 public int getNumber() { 8973 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 8974 } 8975 8976 /** 8977 * @param value An integer associated with each note which may be referred to from each service line item. 8978 */ 8979 public NoteComponent setNumber(int value) { 8980 if (this.number == null) 8981 this.number = new PositiveIntType(); 8982 this.number.setValue(value); 8983 return this; 8984 } 8985 8986 /** 8987 * @return {@link #type} (The note purpose: Print/Display.) 8988 */ 8989 public CodeableConcept getType() { 8990 if (this.type == null) 8991 if (Configuration.errorOnAutoCreate()) 8992 throw new Error("Attempt to auto-create NoteComponent.type"); 8993 else if (Configuration.doAutoCreate()) 8994 this.type = new CodeableConcept(); // cc 8995 return this.type; 8996 } 8997 8998 public boolean hasType() { 8999 return this.type != null && !this.type.isEmpty(); 9000 } 9001 9002 /** 9003 * @param value {@link #type} (The note purpose: Print/Display.) 9004 */ 9005 public NoteComponent setType(CodeableConcept value) { 9006 this.type = value; 9007 return this; 9008 } 9009 9010 /** 9011 * @return {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 9012 */ 9013 public StringType getTextElement() { 9014 if (this.text == null) 9015 if (Configuration.errorOnAutoCreate()) 9016 throw new Error("Attempt to auto-create NoteComponent.text"); 9017 else if (Configuration.doAutoCreate()) 9018 this.text = new StringType(); // bb 9019 return this.text; 9020 } 9021 9022 public boolean hasTextElement() { 9023 return this.text != null && !this.text.isEmpty(); 9024 } 9025 9026 public boolean hasText() { 9027 return this.text != null && !this.text.isEmpty(); 9028 } 9029 9030 /** 9031 * @param value {@link #text} (The note text.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 9032 */ 9033 public NoteComponent setTextElement(StringType value) { 9034 this.text = value; 9035 return this; 9036 } 9037 9038 /** 9039 * @return The note text. 9040 */ 9041 public String getText() { 9042 return this.text == null ? null : this.text.getValue(); 9043 } 9044 9045 /** 9046 * @param value The note text. 9047 */ 9048 public NoteComponent setText(String value) { 9049 if (Utilities.noString(value)) 9050 this.text = null; 9051 else { 9052 if (this.text == null) 9053 this.text = new StringType(); 9054 this.text.setValue(value); 9055 } 9056 return this; 9057 } 9058 9059 /** 9060 * @return {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English.) 9061 */ 9062 public CodeableConcept getLanguage() { 9063 if (this.language == null) 9064 if (Configuration.errorOnAutoCreate()) 9065 throw new Error("Attempt to auto-create NoteComponent.language"); 9066 else if (Configuration.doAutoCreate()) 9067 this.language = new CodeableConcept(); // cc 9068 return this.language; 9069 } 9070 9071 public boolean hasLanguage() { 9072 return this.language != null && !this.language.isEmpty(); 9073 } 9074 9075 /** 9076 * @param value {@link #language} (The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. "en" for English, or "en-US" for American English versus "en-EN" for England English.) 9077 */ 9078 public NoteComponent setLanguage(CodeableConcept value) { 9079 this.language = value; 9080 return this; 9081 } 9082 9083 protected void listChildren(List<Property> children) { 9084 super.listChildren(children); 9085 children.add(new Property("number", "positiveInt", "An integer associated with each note which may be referred to from each service line item.", 0, 1, number)); 9086 children.add(new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type)); 9087 children.add(new Property("text", "string", "The note text.", 0, 1, text)); 9088 children.add(new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 0, 1, language)); 9089 } 9090 9091 @Override 9092 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9093 switch (_hash) { 9094 case -1034364087: /*number*/ return new Property("number", "positiveInt", "An integer associated with each note which may be referred to from each service line item.", 0, 1, number); 9095 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The note purpose: Print/Display.", 0, 1, type); 9096 case 3556653: /*text*/ return new Property("text", "string", "The note text.", 0, 1, text); 9097 case -1613589672: /*language*/ return new Property("language", "CodeableConcept", "The ISO-639-1 alpha 2 code in lower case for the language, optionally followed by a hyphen and the ISO-3166-1 alpha 2 code for the region in upper case; e.g. \"en\" for English, or \"en-US\" for American English versus \"en-EN\" for England English.", 0, 1, language); 9098 default: return super.getNamedProperty(_hash, _name, _checkValid); 9099 } 9100 9101 } 9102 9103 @Override 9104 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9105 switch (hash) { 9106 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // PositiveIntType 9107 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 9108 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 9109 case -1613589672: /*language*/ return this.language == null ? new Base[0] : new Base[] {this.language}; // CodeableConcept 9110 default: return super.getProperty(hash, name, checkValid); 9111 } 9112 9113 } 9114 9115 @Override 9116 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9117 switch (hash) { 9118 case -1034364087: // number 9119 this.number = castToPositiveInt(value); // PositiveIntType 9120 return value; 9121 case 3575610: // type 9122 this.type = castToCodeableConcept(value); // CodeableConcept 9123 return value; 9124 case 3556653: // text 9125 this.text = castToString(value); // StringType 9126 return value; 9127 case -1613589672: // language 9128 this.language = castToCodeableConcept(value); // CodeableConcept 9129 return value; 9130 default: return super.setProperty(hash, name, value); 9131 } 9132 9133 } 9134 9135 @Override 9136 public Base setProperty(String name, Base value) throws FHIRException { 9137 if (name.equals("number")) { 9138 this.number = castToPositiveInt(value); // PositiveIntType 9139 } else if (name.equals("type")) { 9140 this.type = castToCodeableConcept(value); // CodeableConcept 9141 } else if (name.equals("text")) { 9142 this.text = castToString(value); // StringType 9143 } else if (name.equals("language")) { 9144 this.language = castToCodeableConcept(value); // CodeableConcept 9145 } else 9146 return super.setProperty(name, value); 9147 return value; 9148 } 9149 9150 @Override 9151 public Base makeProperty(int hash, String name) throws FHIRException { 9152 switch (hash) { 9153 case -1034364087: return getNumberElement(); 9154 case 3575610: return getType(); 9155 case 3556653: return getTextElement(); 9156 case -1613589672: return getLanguage(); 9157 default: return super.makeProperty(hash, name); 9158 } 9159 9160 } 9161 9162 @Override 9163 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9164 switch (hash) { 9165 case -1034364087: /*number*/ return new String[] {"positiveInt"}; 9166 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 9167 case 3556653: /*text*/ return new String[] {"string"}; 9168 case -1613589672: /*language*/ return new String[] {"CodeableConcept"}; 9169 default: return super.getTypesForProperty(hash, name); 9170 } 9171 9172 } 9173 9174 @Override 9175 public Base addChild(String name) throws FHIRException { 9176 if (name.equals("number")) { 9177 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.number"); 9178 } 9179 else if (name.equals("type")) { 9180 this.type = new CodeableConcept(); 9181 return this.type; 9182 } 9183 else if (name.equals("text")) { 9184 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.text"); 9185 } 9186 else if (name.equals("language")) { 9187 this.language = new CodeableConcept(); 9188 return this.language; 9189 } 9190 else 9191 return super.addChild(name); 9192 } 9193 9194 public NoteComponent copy() { 9195 NoteComponent dst = new NoteComponent(); 9196 copyValues(dst); 9197 dst.number = number == null ? null : number.copy(); 9198 dst.type = type == null ? null : type.copy(); 9199 dst.text = text == null ? null : text.copy(); 9200 dst.language = language == null ? null : language.copy(); 9201 return dst; 9202 } 9203 9204 @Override 9205 public boolean equalsDeep(Base other_) { 9206 if (!super.equalsDeep(other_)) 9207 return false; 9208 if (!(other_ instanceof NoteComponent)) 9209 return false; 9210 NoteComponent o = (NoteComponent) other_; 9211 return compareDeep(number, o.number, true) && compareDeep(type, o.type, true) && compareDeep(text, o.text, true) 9212 && compareDeep(language, o.language, true); 9213 } 9214 9215 @Override 9216 public boolean equalsShallow(Base other_) { 9217 if (!super.equalsShallow(other_)) 9218 return false; 9219 if (!(other_ instanceof NoteComponent)) 9220 return false; 9221 NoteComponent o = (NoteComponent) other_; 9222 return compareValues(number, o.number, true) && compareValues(text, o.text, true); 9223 } 9224 9225 public boolean isEmpty() { 9226 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(number, type, text, language 9227 ); 9228 } 9229 9230 public String fhirType() { 9231 return "ExplanationOfBenefit.processNote"; 9232 9233 } 9234 9235 } 9236 9237 @Block() 9238 public static class BenefitBalanceComponent extends BackboneElement implements IBaseBackboneElement { 9239 /** 9240 * Dental, Vision, Medical, Pharmacy, Rehab etc. 9241 */ 9242 @Child(name = "category", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 9243 @Description(shortDefinition="Type of services covered", formalDefinition="Dental, Vision, Medical, Pharmacy, Rehab etc." ) 9244 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-category") 9245 protected CodeableConcept category; 9246 9247 /** 9248 * Dental: basic, major, ortho; Vision exam, glasses, contacts; etc. 9249 */ 9250 @Child(name = "subCategory", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 9251 @Description(shortDefinition="Detailed services covered within the type", formalDefinition="Dental: basic, major, ortho; Vision exam, glasses, contacts; etc." ) 9252 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-subcategory") 9253 protected CodeableConcept subCategory; 9254 9255 /** 9256 * True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 9257 */ 9258 @Child(name = "excluded", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 9259 @Description(shortDefinition="Excluded from the plan", formalDefinition="True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage." ) 9260 protected BooleanType excluded; 9261 9262 /** 9263 * A short name or tag for the benefit, for example MED01, or DENT2. 9264 */ 9265 @Child(name = "name", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 9266 @Description(shortDefinition="Short name for the benefit", formalDefinition="A short name or tag for the benefit, for example MED01, or DENT2." ) 9267 protected StringType name; 9268 9269 /** 9270 * A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 9271 */ 9272 @Child(name = "description", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 9273 @Description(shortDefinition="Description of the benefit or services covered", formalDefinition="A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'." ) 9274 protected StringType description; 9275 9276 /** 9277 * Network designation. 9278 */ 9279 @Child(name = "network", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 9280 @Description(shortDefinition="In or out of network", formalDefinition="Network designation." ) 9281 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-network") 9282 protected CodeableConcept network; 9283 9284 /** 9285 * Unit designation: individual or family. 9286 */ 9287 @Child(name = "unit", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=false) 9288 @Description(shortDefinition="Individual or family", formalDefinition="Unit designation: individual or family." ) 9289 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-unit") 9290 protected CodeableConcept unit; 9291 9292 /** 9293 * The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'. 9294 */ 9295 @Child(name = "term", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 9296 @Description(shortDefinition="Annual or lifetime", formalDefinition="The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'." ) 9297 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-term") 9298 protected CodeableConcept term; 9299 9300 /** 9301 * Benefits Used to date. 9302 */ 9303 @Child(name = "financial", type = {}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 9304 @Description(shortDefinition="Benefit Summary", formalDefinition="Benefits Used to date." ) 9305 protected List<BenefitComponent> financial; 9306 9307 private static final long serialVersionUID = 833826021L; 9308 9309 /** 9310 * Constructor 9311 */ 9312 public BenefitBalanceComponent() { 9313 super(); 9314 } 9315 9316 /** 9317 * Constructor 9318 */ 9319 public BenefitBalanceComponent(CodeableConcept category) { 9320 super(); 9321 this.category = category; 9322 } 9323 9324 /** 9325 * @return {@link #category} (Dental, Vision, Medical, Pharmacy, Rehab etc.) 9326 */ 9327 public CodeableConcept getCategory() { 9328 if (this.category == null) 9329 if (Configuration.errorOnAutoCreate()) 9330 throw new Error("Attempt to auto-create BenefitBalanceComponent.category"); 9331 else if (Configuration.doAutoCreate()) 9332 this.category = new CodeableConcept(); // cc 9333 return this.category; 9334 } 9335 9336 public boolean hasCategory() { 9337 return this.category != null && !this.category.isEmpty(); 9338 } 9339 9340 /** 9341 * @param value {@link #category} (Dental, Vision, Medical, Pharmacy, Rehab etc.) 9342 */ 9343 public BenefitBalanceComponent setCategory(CodeableConcept value) { 9344 this.category = value; 9345 return this; 9346 } 9347 9348 /** 9349 * @return {@link #subCategory} (Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.) 9350 */ 9351 public CodeableConcept getSubCategory() { 9352 if (this.subCategory == null) 9353 if (Configuration.errorOnAutoCreate()) 9354 throw new Error("Attempt to auto-create BenefitBalanceComponent.subCategory"); 9355 else if (Configuration.doAutoCreate()) 9356 this.subCategory = new CodeableConcept(); // cc 9357 return this.subCategory; 9358 } 9359 9360 public boolean hasSubCategory() { 9361 return this.subCategory != null && !this.subCategory.isEmpty(); 9362 } 9363 9364 /** 9365 * @param value {@link #subCategory} (Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.) 9366 */ 9367 public BenefitBalanceComponent setSubCategory(CodeableConcept value) { 9368 this.subCategory = value; 9369 return this; 9370 } 9371 9372 /** 9373 * @return {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 9374 */ 9375 public BooleanType getExcludedElement() { 9376 if (this.excluded == null) 9377 if (Configuration.errorOnAutoCreate()) 9378 throw new Error("Attempt to auto-create BenefitBalanceComponent.excluded"); 9379 else if (Configuration.doAutoCreate()) 9380 this.excluded = new BooleanType(); // bb 9381 return this.excluded; 9382 } 9383 9384 public boolean hasExcludedElement() { 9385 return this.excluded != null && !this.excluded.isEmpty(); 9386 } 9387 9388 public boolean hasExcluded() { 9389 return this.excluded != null && !this.excluded.isEmpty(); 9390 } 9391 9392 /** 9393 * @param value {@link #excluded} (True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.). This is the underlying object with id, value and extensions. The accessor "getExcluded" gives direct access to the value 9394 */ 9395 public BenefitBalanceComponent setExcludedElement(BooleanType value) { 9396 this.excluded = value; 9397 return this; 9398 } 9399 9400 /** 9401 * @return True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 9402 */ 9403 public boolean getExcluded() { 9404 return this.excluded == null || this.excluded.isEmpty() ? false : this.excluded.getValue(); 9405 } 9406 9407 /** 9408 * @param value True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage. 9409 */ 9410 public BenefitBalanceComponent setExcluded(boolean value) { 9411 if (this.excluded == null) 9412 this.excluded = new BooleanType(); 9413 this.excluded.setValue(value); 9414 return this; 9415 } 9416 9417 /** 9418 * @return {@link #name} (A short name or tag for the benefit, for example MED01, or DENT2.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9419 */ 9420 public StringType getNameElement() { 9421 if (this.name == null) 9422 if (Configuration.errorOnAutoCreate()) 9423 throw new Error("Attempt to auto-create BenefitBalanceComponent.name"); 9424 else if (Configuration.doAutoCreate()) 9425 this.name = new StringType(); // bb 9426 return this.name; 9427 } 9428 9429 public boolean hasNameElement() { 9430 return this.name != null && !this.name.isEmpty(); 9431 } 9432 9433 public boolean hasName() { 9434 return this.name != null && !this.name.isEmpty(); 9435 } 9436 9437 /** 9438 * @param value {@link #name} (A short name or tag for the benefit, for example MED01, or DENT2.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 9439 */ 9440 public BenefitBalanceComponent setNameElement(StringType value) { 9441 this.name = value; 9442 return this; 9443 } 9444 9445 /** 9446 * @return A short name or tag for the benefit, for example MED01, or DENT2. 9447 */ 9448 public String getName() { 9449 return this.name == null ? null : this.name.getValue(); 9450 } 9451 9452 /** 9453 * @param value A short name or tag for the benefit, for example MED01, or DENT2. 9454 */ 9455 public BenefitBalanceComponent setName(String value) { 9456 if (Utilities.noString(value)) 9457 this.name = null; 9458 else { 9459 if (this.name == null) 9460 this.name = new StringType(); 9461 this.name.setValue(value); 9462 } 9463 return this; 9464 } 9465 9466 /** 9467 * @return {@link #description} (A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 9468 */ 9469 public StringType getDescriptionElement() { 9470 if (this.description == null) 9471 if (Configuration.errorOnAutoCreate()) 9472 throw new Error("Attempt to auto-create BenefitBalanceComponent.description"); 9473 else if (Configuration.doAutoCreate()) 9474 this.description = new StringType(); // bb 9475 return this.description; 9476 } 9477 9478 public boolean hasDescriptionElement() { 9479 return this.description != null && !this.description.isEmpty(); 9480 } 9481 9482 public boolean hasDescription() { 9483 return this.description != null && !this.description.isEmpty(); 9484 } 9485 9486 /** 9487 * @param value {@link #description} (A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 9488 */ 9489 public BenefitBalanceComponent setDescriptionElement(StringType value) { 9490 this.description = value; 9491 return this; 9492 } 9493 9494 /** 9495 * @return A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 9496 */ 9497 public String getDescription() { 9498 return this.description == null ? null : this.description.getValue(); 9499 } 9500 9501 /** 9502 * @param value A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'. 9503 */ 9504 public BenefitBalanceComponent setDescription(String value) { 9505 if (Utilities.noString(value)) 9506 this.description = null; 9507 else { 9508 if (this.description == null) 9509 this.description = new StringType(); 9510 this.description.setValue(value); 9511 } 9512 return this; 9513 } 9514 9515 /** 9516 * @return {@link #network} (Network designation.) 9517 */ 9518 public CodeableConcept getNetwork() { 9519 if (this.network == null) 9520 if (Configuration.errorOnAutoCreate()) 9521 throw new Error("Attempt to auto-create BenefitBalanceComponent.network"); 9522 else if (Configuration.doAutoCreate()) 9523 this.network = new CodeableConcept(); // cc 9524 return this.network; 9525 } 9526 9527 public boolean hasNetwork() { 9528 return this.network != null && !this.network.isEmpty(); 9529 } 9530 9531 /** 9532 * @param value {@link #network} (Network designation.) 9533 */ 9534 public BenefitBalanceComponent setNetwork(CodeableConcept value) { 9535 this.network = value; 9536 return this; 9537 } 9538 9539 /** 9540 * @return {@link #unit} (Unit designation: individual or family.) 9541 */ 9542 public CodeableConcept getUnit() { 9543 if (this.unit == null) 9544 if (Configuration.errorOnAutoCreate()) 9545 throw new Error("Attempt to auto-create BenefitBalanceComponent.unit"); 9546 else if (Configuration.doAutoCreate()) 9547 this.unit = new CodeableConcept(); // cc 9548 return this.unit; 9549 } 9550 9551 public boolean hasUnit() { 9552 return this.unit != null && !this.unit.isEmpty(); 9553 } 9554 9555 /** 9556 * @param value {@link #unit} (Unit designation: individual or family.) 9557 */ 9558 public BenefitBalanceComponent setUnit(CodeableConcept value) { 9559 this.unit = value; 9560 return this; 9561 } 9562 9563 /** 9564 * @return {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.) 9565 */ 9566 public CodeableConcept getTerm() { 9567 if (this.term == null) 9568 if (Configuration.errorOnAutoCreate()) 9569 throw new Error("Attempt to auto-create BenefitBalanceComponent.term"); 9570 else if (Configuration.doAutoCreate()) 9571 this.term = new CodeableConcept(); // cc 9572 return this.term; 9573 } 9574 9575 public boolean hasTerm() { 9576 return this.term != null && !this.term.isEmpty(); 9577 } 9578 9579 /** 9580 * @param value {@link #term} (The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.) 9581 */ 9582 public BenefitBalanceComponent setTerm(CodeableConcept value) { 9583 this.term = value; 9584 return this; 9585 } 9586 9587 /** 9588 * @return {@link #financial} (Benefits Used to date.) 9589 */ 9590 public List<BenefitComponent> getFinancial() { 9591 if (this.financial == null) 9592 this.financial = new ArrayList<BenefitComponent>(); 9593 return this.financial; 9594 } 9595 9596 /** 9597 * @return Returns a reference to <code>this</code> for easy method chaining 9598 */ 9599 public BenefitBalanceComponent setFinancial(List<BenefitComponent> theFinancial) { 9600 this.financial = theFinancial; 9601 return this; 9602 } 9603 9604 public boolean hasFinancial() { 9605 if (this.financial == null) 9606 return false; 9607 for (BenefitComponent item : this.financial) 9608 if (!item.isEmpty()) 9609 return true; 9610 return false; 9611 } 9612 9613 public BenefitComponent addFinancial() { //3 9614 BenefitComponent t = new BenefitComponent(); 9615 if (this.financial == null) 9616 this.financial = new ArrayList<BenefitComponent>(); 9617 this.financial.add(t); 9618 return t; 9619 } 9620 9621 public BenefitBalanceComponent addFinancial(BenefitComponent t) { //3 9622 if (t == null) 9623 return this; 9624 if (this.financial == null) 9625 this.financial = new ArrayList<BenefitComponent>(); 9626 this.financial.add(t); 9627 return this; 9628 } 9629 9630 /** 9631 * @return The first repetition of repeating field {@link #financial}, creating it if it does not already exist 9632 */ 9633 public BenefitComponent getFinancialFirstRep() { 9634 if (getFinancial().isEmpty()) { 9635 addFinancial(); 9636 } 9637 return getFinancial().get(0); 9638 } 9639 9640 protected void listChildren(List<Property> children) { 9641 super.listChildren(children); 9642 children.add(new Property("category", "CodeableConcept", "Dental, Vision, Medical, Pharmacy, Rehab etc.", 0, 1, category)); 9643 children.add(new Property("subCategory", "CodeableConcept", "Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.", 0, 1, subCategory)); 9644 children.add(new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.", 0, 1, excluded)); 9645 children.add(new Property("name", "string", "A short name or tag for the benefit, for example MED01, or DENT2.", 0, 1, name)); 9646 children.add(new Property("description", "string", "A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.", 0, 1, description)); 9647 children.add(new Property("network", "CodeableConcept", "Network designation.", 0, 1, network)); 9648 children.add(new Property("unit", "CodeableConcept", "Unit designation: individual or family.", 0, 1, unit)); 9649 children.add(new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.", 0, 1, term)); 9650 children.add(new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial)); 9651 } 9652 9653 @Override 9654 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 9655 switch (_hash) { 9656 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Dental, Vision, Medical, Pharmacy, Rehab etc.", 0, 1, category); 9657 case 1365024606: /*subCategory*/ return new Property("subCategory", "CodeableConcept", "Dental: basic, major, ortho; Vision exam, glasses, contacts; etc.", 0, 1, subCategory); 9658 case 1994055114: /*excluded*/ return new Property("excluded", "boolean", "True if the indicated class of service is excluded from the plan, missing or False indicated the service is included in the coverage.", 0, 1, excluded); 9659 case 3373707: /*name*/ return new Property("name", "string", "A short name or tag for the benefit, for example MED01, or DENT2.", 0, 1, name); 9660 case -1724546052: /*description*/ return new Property("description", "string", "A richer description of the benefit, for example 'DENT2 covers 100% of basic, 50% of major but exclused Ortho, Implants and Costmetic services'.", 0, 1, description); 9661 case 1843485230: /*network*/ return new Property("network", "CodeableConcept", "Network designation.", 0, 1, network); 9662 case 3594628: /*unit*/ return new Property("unit", "CodeableConcept", "Unit designation: individual or family.", 0, 1, unit); 9663 case 3556460: /*term*/ return new Property("term", "CodeableConcept", "The term or period of the values such as 'maximum lifetime benefit' or 'maximum annual vistis'.", 0, 1, term); 9664 case 357555337: /*financial*/ return new Property("financial", "", "Benefits Used to date.", 0, java.lang.Integer.MAX_VALUE, financial); 9665 default: return super.getNamedProperty(_hash, _name, _checkValid); 9666 } 9667 9668 } 9669 9670 @Override 9671 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 9672 switch (hash) { 9673 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 9674 case 1365024606: /*subCategory*/ return this.subCategory == null ? new Base[0] : new Base[] {this.subCategory}; // CodeableConcept 9675 case 1994055114: /*excluded*/ return this.excluded == null ? new Base[0] : new Base[] {this.excluded}; // BooleanType 9676 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 9677 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 9678 case 1843485230: /*network*/ return this.network == null ? new Base[0] : new Base[] {this.network}; // CodeableConcept 9679 case 3594628: /*unit*/ return this.unit == null ? new Base[0] : new Base[] {this.unit}; // CodeableConcept 9680 case 3556460: /*term*/ return this.term == null ? new Base[0] : new Base[] {this.term}; // CodeableConcept 9681 case 357555337: /*financial*/ return this.financial == null ? new Base[0] : this.financial.toArray(new Base[this.financial.size()]); // BenefitComponent 9682 default: return super.getProperty(hash, name, checkValid); 9683 } 9684 9685 } 9686 9687 @Override 9688 public Base setProperty(int hash, String name, Base value) throws FHIRException { 9689 switch (hash) { 9690 case 50511102: // category 9691 this.category = castToCodeableConcept(value); // CodeableConcept 9692 return value; 9693 case 1365024606: // subCategory 9694 this.subCategory = castToCodeableConcept(value); // CodeableConcept 9695 return value; 9696 case 1994055114: // excluded 9697 this.excluded = castToBoolean(value); // BooleanType 9698 return value; 9699 case 3373707: // name 9700 this.name = castToString(value); // StringType 9701 return value; 9702 case -1724546052: // description 9703 this.description = castToString(value); // StringType 9704 return value; 9705 case 1843485230: // network 9706 this.network = castToCodeableConcept(value); // CodeableConcept 9707 return value; 9708 case 3594628: // unit 9709 this.unit = castToCodeableConcept(value); // CodeableConcept 9710 return value; 9711 case 3556460: // term 9712 this.term = castToCodeableConcept(value); // CodeableConcept 9713 return value; 9714 case 357555337: // financial 9715 this.getFinancial().add((BenefitComponent) value); // BenefitComponent 9716 return value; 9717 default: return super.setProperty(hash, name, value); 9718 } 9719 9720 } 9721 9722 @Override 9723 public Base setProperty(String name, Base value) throws FHIRException { 9724 if (name.equals("category")) { 9725 this.category = castToCodeableConcept(value); // CodeableConcept 9726 } else if (name.equals("subCategory")) { 9727 this.subCategory = castToCodeableConcept(value); // CodeableConcept 9728 } else if (name.equals("excluded")) { 9729 this.excluded = castToBoolean(value); // BooleanType 9730 } else if (name.equals("name")) { 9731 this.name = castToString(value); // StringType 9732 } else if (name.equals("description")) { 9733 this.description = castToString(value); // StringType 9734 } else if (name.equals("network")) { 9735 this.network = castToCodeableConcept(value); // CodeableConcept 9736 } else if (name.equals("unit")) { 9737 this.unit = castToCodeableConcept(value); // CodeableConcept 9738 } else if (name.equals("term")) { 9739 this.term = castToCodeableConcept(value); // CodeableConcept 9740 } else if (name.equals("financial")) { 9741 this.getFinancial().add((BenefitComponent) value); 9742 } else 9743 return super.setProperty(name, value); 9744 return value; 9745 } 9746 9747 @Override 9748 public Base makeProperty(int hash, String name) throws FHIRException { 9749 switch (hash) { 9750 case 50511102: return getCategory(); 9751 case 1365024606: return getSubCategory(); 9752 case 1994055114: return getExcludedElement(); 9753 case 3373707: return getNameElement(); 9754 case -1724546052: return getDescriptionElement(); 9755 case 1843485230: return getNetwork(); 9756 case 3594628: return getUnit(); 9757 case 3556460: return getTerm(); 9758 case 357555337: return addFinancial(); 9759 default: return super.makeProperty(hash, name); 9760 } 9761 9762 } 9763 9764 @Override 9765 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 9766 switch (hash) { 9767 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 9768 case 1365024606: /*subCategory*/ return new String[] {"CodeableConcept"}; 9769 case 1994055114: /*excluded*/ return new String[] {"boolean"}; 9770 case 3373707: /*name*/ return new String[] {"string"}; 9771 case -1724546052: /*description*/ return new String[] {"string"}; 9772 case 1843485230: /*network*/ return new String[] {"CodeableConcept"}; 9773 case 3594628: /*unit*/ return new String[] {"CodeableConcept"}; 9774 case 3556460: /*term*/ return new String[] {"CodeableConcept"}; 9775 case 357555337: /*financial*/ return new String[] {}; 9776 default: return super.getTypesForProperty(hash, name); 9777 } 9778 9779 } 9780 9781 @Override 9782 public Base addChild(String name) throws FHIRException { 9783 if (name.equals("category")) { 9784 this.category = new CodeableConcept(); 9785 return this.category; 9786 } 9787 else if (name.equals("subCategory")) { 9788 this.subCategory = new CodeableConcept(); 9789 return this.subCategory; 9790 } 9791 else if (name.equals("excluded")) { 9792 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.excluded"); 9793 } 9794 else if (name.equals("name")) { 9795 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.name"); 9796 } 9797 else if (name.equals("description")) { 9798 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.description"); 9799 } 9800 else if (name.equals("network")) { 9801 this.network = new CodeableConcept(); 9802 return this.network; 9803 } 9804 else if (name.equals("unit")) { 9805 this.unit = new CodeableConcept(); 9806 return this.unit; 9807 } 9808 else if (name.equals("term")) { 9809 this.term = new CodeableConcept(); 9810 return this.term; 9811 } 9812 else if (name.equals("financial")) { 9813 return addFinancial(); 9814 } 9815 else 9816 return super.addChild(name); 9817 } 9818 9819 public BenefitBalanceComponent copy() { 9820 BenefitBalanceComponent dst = new BenefitBalanceComponent(); 9821 copyValues(dst); 9822 dst.category = category == null ? null : category.copy(); 9823 dst.subCategory = subCategory == null ? null : subCategory.copy(); 9824 dst.excluded = excluded == null ? null : excluded.copy(); 9825 dst.name = name == null ? null : name.copy(); 9826 dst.description = description == null ? null : description.copy(); 9827 dst.network = network == null ? null : network.copy(); 9828 dst.unit = unit == null ? null : unit.copy(); 9829 dst.term = term == null ? null : term.copy(); 9830 if (financial != null) { 9831 dst.financial = new ArrayList<BenefitComponent>(); 9832 for (BenefitComponent i : financial) 9833 dst.financial.add(i.copy()); 9834 }; 9835 return dst; 9836 } 9837 9838 @Override 9839 public boolean equalsDeep(Base other_) { 9840 if (!super.equalsDeep(other_)) 9841 return false; 9842 if (!(other_ instanceof BenefitBalanceComponent)) 9843 return false; 9844 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 9845 return compareDeep(category, o.category, true) && compareDeep(subCategory, o.subCategory, true) 9846 && compareDeep(excluded, o.excluded, true) && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 9847 && compareDeep(network, o.network, true) && compareDeep(unit, o.unit, true) && compareDeep(term, o.term, true) 9848 && compareDeep(financial, o.financial, true); 9849 } 9850 9851 @Override 9852 public boolean equalsShallow(Base other_) { 9853 if (!super.equalsShallow(other_)) 9854 return false; 9855 if (!(other_ instanceof BenefitBalanceComponent)) 9856 return false; 9857 BenefitBalanceComponent o = (BenefitBalanceComponent) other_; 9858 return compareValues(excluded, o.excluded, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 9859 ; 9860 } 9861 9862 public boolean isEmpty() { 9863 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(category, subCategory, excluded 9864 , name, description, network, unit, term, financial); 9865 } 9866 9867 public String fhirType() { 9868 return "ExplanationOfBenefit.benefitBalance"; 9869 9870 } 9871 9872 } 9873 9874 @Block() 9875 public static class BenefitComponent extends BackboneElement implements IBaseBackboneElement { 9876 /** 9877 * Deductable, visits, benefit amount. 9878 */ 9879 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 9880 @Description(shortDefinition="Deductable, visits, benefit amount", formalDefinition="Deductable, visits, benefit amount." ) 9881 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/benefit-type") 9882 protected CodeableConcept type; 9883 9884 /** 9885 * Benefits allowed. 9886 */ 9887 @Child(name = "allowed", type = {UnsignedIntType.class, StringType.class, Money.class}, order=2, min=0, max=1, modifier=false, summary=false) 9888 @Description(shortDefinition="Benefits allowed", formalDefinition="Benefits allowed." ) 9889 protected Type allowed; 9890 9891 /** 9892 * Benefits used. 9893 */ 9894 @Child(name = "used", type = {UnsignedIntType.class, Money.class}, order=3, min=0, max=1, modifier=false, summary=false) 9895 @Description(shortDefinition="Benefits used", formalDefinition="Benefits used." ) 9896 protected Type used; 9897 9898 private static final long serialVersionUID = -1506285314L; 9899 9900 /** 9901 * Constructor 9902 */ 9903 public BenefitComponent() { 9904 super(); 9905 } 9906 9907 /** 9908 * Constructor 9909 */ 9910 public BenefitComponent(CodeableConcept type) { 9911 super(); 9912 this.type = type; 9913 } 9914 9915 /** 9916 * @return {@link #type} (Deductable, visits, benefit amount.) 9917 */ 9918 public CodeableConcept getType() { 9919 if (this.type == null) 9920 if (Configuration.errorOnAutoCreate()) 9921 throw new Error("Attempt to auto-create BenefitComponent.type"); 9922 else if (Configuration.doAutoCreate()) 9923 this.type = new CodeableConcept(); // cc 9924 return this.type; 9925 } 9926 9927 public boolean hasType() { 9928 return this.type != null && !this.type.isEmpty(); 9929 } 9930 9931 /** 9932 * @param value {@link #type} (Deductable, visits, benefit amount.) 9933 */ 9934 public BenefitComponent setType(CodeableConcept value) { 9935 this.type = value; 9936 return this; 9937 } 9938 9939 /** 9940 * @return {@link #allowed} (Benefits allowed.) 9941 */ 9942 public Type getAllowed() { 9943 return this.allowed; 9944 } 9945 9946 /** 9947 * @return {@link #allowed} (Benefits allowed.) 9948 */ 9949 public UnsignedIntType getAllowedUnsignedIntType() throws FHIRException { 9950 if (this.allowed == null) 9951 return null; 9952 if (!(this.allowed instanceof UnsignedIntType)) 9953 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 9954 return (UnsignedIntType) this.allowed; 9955 } 9956 9957 public boolean hasAllowedUnsignedIntType() { 9958 return this != null && this.allowed instanceof UnsignedIntType; 9959 } 9960 9961 /** 9962 * @return {@link #allowed} (Benefits allowed.) 9963 */ 9964 public StringType getAllowedStringType() throws FHIRException { 9965 if (this.allowed == null) 9966 return null; 9967 if (!(this.allowed instanceof StringType)) 9968 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.allowed.getClass().getName()+" was encountered"); 9969 return (StringType) this.allowed; 9970 } 9971 9972 public boolean hasAllowedStringType() { 9973 return this != null && this.allowed instanceof StringType; 9974 } 9975 9976 /** 9977 * @return {@link #allowed} (Benefits allowed.) 9978 */ 9979 public Money getAllowedMoney() throws FHIRException { 9980 if (this.allowed == null) 9981 return null; 9982 if (!(this.allowed instanceof Money)) 9983 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.allowed.getClass().getName()+" was encountered"); 9984 return (Money) this.allowed; 9985 } 9986 9987 public boolean hasAllowedMoney() { 9988 return this != null && this.allowed instanceof Money; 9989 } 9990 9991 public boolean hasAllowed() { 9992 return this.allowed != null && !this.allowed.isEmpty(); 9993 } 9994 9995 /** 9996 * @param value {@link #allowed} (Benefits allowed.) 9997 */ 9998 public BenefitComponent setAllowed(Type value) throws FHIRFormatError { 9999 if (value != null && !(value instanceof UnsignedIntType || value instanceof StringType || value instanceof Money)) 10000 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.benefitBalance.financial.allowed[x]: "+value.fhirType()); 10001 this.allowed = value; 10002 return this; 10003 } 10004 10005 /** 10006 * @return {@link #used} (Benefits used.) 10007 */ 10008 public Type getUsed() { 10009 return this.used; 10010 } 10011 10012 /** 10013 * @return {@link #used} (Benefits used.) 10014 */ 10015 public UnsignedIntType getUsedUnsignedIntType() throws FHIRException { 10016 if (this.used == null) 10017 return null; 10018 if (!(this.used instanceof UnsignedIntType)) 10019 throw new FHIRException("Type mismatch: the type UnsignedIntType was expected, but "+this.used.getClass().getName()+" was encountered"); 10020 return (UnsignedIntType) this.used; 10021 } 10022 10023 public boolean hasUsedUnsignedIntType() { 10024 return this != null && this.used instanceof UnsignedIntType; 10025 } 10026 10027 /** 10028 * @return {@link #used} (Benefits used.) 10029 */ 10030 public Money getUsedMoney() throws FHIRException { 10031 if (this.used == null) 10032 return null; 10033 if (!(this.used instanceof Money)) 10034 throw new FHIRException("Type mismatch: the type Money was expected, but "+this.used.getClass().getName()+" was encountered"); 10035 return (Money) this.used; 10036 } 10037 10038 public boolean hasUsedMoney() { 10039 return this != null && this.used instanceof Money; 10040 } 10041 10042 public boolean hasUsed() { 10043 return this.used != null && !this.used.isEmpty(); 10044 } 10045 10046 /** 10047 * @param value {@link #used} (Benefits used.) 10048 */ 10049 public BenefitComponent setUsed(Type value) throws FHIRFormatError { 10050 if (value != null && !(value instanceof UnsignedIntType || value instanceof Money)) 10051 throw new FHIRFormatError("Not the right type for ExplanationOfBenefit.benefitBalance.financial.used[x]: "+value.fhirType()); 10052 this.used = value; 10053 return this; 10054 } 10055 10056 protected void listChildren(List<Property> children) { 10057 super.listChildren(children); 10058 children.add(new Property("type", "CodeableConcept", "Deductable, visits, benefit amount.", 0, 1, type)); 10059 children.add(new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed)); 10060 children.add(new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used)); 10061 } 10062 10063 @Override 10064 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 10065 switch (_hash) { 10066 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Deductable, visits, benefit amount.", 0, 1, type); 10067 case -1336663592: /*allowed[x]*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10068 case -911343192: /*allowed*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10069 case 1668802034: /*allowedUnsignedInt*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10070 case -2135265319: /*allowedString*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10071 case -351668232: /*allowedMoney*/ return new Property("allowed[x]", "unsignedInt|string|Money", "Benefits allowed.", 0, 1, allowed); 10072 case -147553373: /*used[x]*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 10073 case 3599293: /*used*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 10074 case 1252740285: /*usedUnsignedInt*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 10075 case -78048509: /*usedMoney*/ return new Property("used[x]", "unsignedInt|Money", "Benefits used.", 0, 1, used); 10076 default: return super.getNamedProperty(_hash, _name, _checkValid); 10077 } 10078 10079 } 10080 10081 @Override 10082 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 10083 switch (hash) { 10084 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 10085 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // Type 10086 case 3599293: /*used*/ return this.used == null ? new Base[0] : new Base[] {this.used}; // Type 10087 default: return super.getProperty(hash, name, checkValid); 10088 } 10089 10090 } 10091 10092 @Override 10093 public Base setProperty(int hash, String name, Base value) throws FHIRException { 10094 switch (hash) { 10095 case 3575610: // type 10096 this.type = castToCodeableConcept(value); // CodeableConcept 10097 return value; 10098 case -911343192: // allowed 10099 this.allowed = castToType(value); // Type 10100 return value; 10101 case 3599293: // used 10102 this.used = castToType(value); // Type 10103 return value; 10104 default: return super.setProperty(hash, name, value); 10105 } 10106 10107 } 10108 10109 @Override 10110 public Base setProperty(String name, Base value) throws FHIRException { 10111 if (name.equals("type")) { 10112 this.type = castToCodeableConcept(value); // CodeableConcept 10113 } else if (name.equals("allowed[x]")) { 10114 this.allowed = castToType(value); // Type 10115 } else if (name.equals("used[x]")) { 10116 this.used = castToType(value); // Type 10117 } else 10118 return super.setProperty(name, value); 10119 return value; 10120 } 10121 10122 @Override 10123 public Base makeProperty(int hash, String name) throws FHIRException { 10124 switch (hash) { 10125 case 3575610: return getType(); 10126 case -1336663592: return getAllowed(); 10127 case -911343192: return getAllowed(); 10128 case -147553373: return getUsed(); 10129 case 3599293: return getUsed(); 10130 default: return super.makeProperty(hash, name); 10131 } 10132 10133 } 10134 10135 @Override 10136 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 10137 switch (hash) { 10138 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 10139 case -911343192: /*allowed*/ return new String[] {"unsignedInt", "string", "Money"}; 10140 case 3599293: /*used*/ return new String[] {"unsignedInt", "Money"}; 10141 default: return super.getTypesForProperty(hash, name); 10142 } 10143 10144 } 10145 10146 @Override 10147 public Base addChild(String name) throws FHIRException { 10148 if (name.equals("type")) { 10149 this.type = new CodeableConcept(); 10150 return this.type; 10151 } 10152 else if (name.equals("allowedUnsignedInt")) { 10153 this.allowed = new UnsignedIntType(); 10154 return this.allowed; 10155 } 10156 else if (name.equals("allowedString")) { 10157 this.allowed = new StringType(); 10158 return this.allowed; 10159 } 10160 else if (name.equals("allowedMoney")) { 10161 this.allowed = new Money(); 10162 return this.allowed; 10163 } 10164 else if (name.equals("usedUnsignedInt")) { 10165 this.used = new UnsignedIntType(); 10166 return this.used; 10167 } 10168 else if (name.equals("usedMoney")) { 10169 this.used = new Money(); 10170 return this.used; 10171 } 10172 else 10173 return super.addChild(name); 10174 } 10175 10176 public BenefitComponent copy() { 10177 BenefitComponent dst = new BenefitComponent(); 10178 copyValues(dst); 10179 dst.type = type == null ? null : type.copy(); 10180 dst.allowed = allowed == null ? null : allowed.copy(); 10181 dst.used = used == null ? null : used.copy(); 10182 return dst; 10183 } 10184 10185 @Override 10186 public boolean equalsDeep(Base other_) { 10187 if (!super.equalsDeep(other_)) 10188 return false; 10189 if (!(other_ instanceof BenefitComponent)) 10190 return false; 10191 BenefitComponent o = (BenefitComponent) other_; 10192 return compareDeep(type, o.type, true) && compareDeep(allowed, o.allowed, true) && compareDeep(used, o.used, true) 10193 ; 10194 } 10195 10196 @Override 10197 public boolean equalsShallow(Base other_) { 10198 if (!super.equalsShallow(other_)) 10199 return false; 10200 if (!(other_ instanceof BenefitComponent)) 10201 return false; 10202 BenefitComponent o = (BenefitComponent) other_; 10203 return true; 10204 } 10205 10206 public boolean isEmpty() { 10207 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, allowed, used); 10208 } 10209 10210 public String fhirType() { 10211 return "ExplanationOfBenefit.benefitBalance.financial"; 10212 10213 } 10214 10215 } 10216 10217 /** 10218 * The EOB Business Identifier. 10219 */ 10220 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10221 @Description(shortDefinition="Business Identifier", formalDefinition="The EOB Business Identifier." ) 10222 protected List<Identifier> identifier; 10223 10224 /** 10225 * The status of the resource instance. 10226 */ 10227 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 10228 @Description(shortDefinition="active | cancelled | draft | entered-in-error", formalDefinition="The status of the resource instance." ) 10229 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/explanationofbenefit-status") 10230 protected Enumeration<ExplanationOfBenefitStatus> status; 10231 10232 /** 10233 * The category of claim, eg, oral, pharmacy, vision, insitutional, professional. 10234 */ 10235 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 10236 @Description(shortDefinition="Type or discipline", formalDefinition="The category of claim, eg, oral, pharmacy, vision, insitutional, professional." ) 10237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-type") 10238 protected CodeableConcept type; 10239 10240 /** 10241 * A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType. 10242 */ 10243 @Child(name = "subType", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10244 @Description(shortDefinition="Finer grained claim type information", formalDefinition="A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType." ) 10245 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/claim-subtype") 10246 protected List<CodeableConcept> subType; 10247 10248 /** 10249 * Patient Resource. 10250 */ 10251 @Child(name = "patient", type = {Patient.class}, order=4, min=0, max=1, modifier=false, summary=false) 10252 @Description(shortDefinition="The subject of the Products and Services", formalDefinition="Patient Resource." ) 10253 protected Reference patient; 10254 10255 /** 10256 * The actual object that is the target of the reference (Patient Resource.) 10257 */ 10258 protected Patient patientTarget; 10259 10260 /** 10261 * The billable period for which charges are being submitted. 10262 */ 10263 @Child(name = "billablePeriod", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=false) 10264 @Description(shortDefinition="Period for charge submission", formalDefinition="The billable period for which charges are being submitted." ) 10265 protected Period billablePeriod; 10266 10267 /** 10268 * The date when the EOB was created. 10269 */ 10270 @Child(name = "created", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 10271 @Description(shortDefinition="Creation date", formalDefinition="The date when the EOB was created." ) 10272 protected DateTimeType created; 10273 10274 /** 10275 * The person who created the explanation of benefit. 10276 */ 10277 @Child(name = "enterer", type = {Practitioner.class}, order=7, min=0, max=1, modifier=false, summary=false) 10278 @Description(shortDefinition="Author", formalDefinition="The person who created the explanation of benefit." ) 10279 protected Reference enterer; 10280 10281 /** 10282 * The actual object that is the target of the reference (The person who created the explanation of benefit.) 10283 */ 10284 protected Practitioner entererTarget; 10285 10286 /** 10287 * The insurer which is responsible for the explanation of benefit. 10288 */ 10289 @Child(name = "insurer", type = {Organization.class}, order=8, min=0, max=1, modifier=false, summary=false) 10290 @Description(shortDefinition="Insurer responsible for the EOB", formalDefinition="The insurer which is responsible for the explanation of benefit." ) 10291 protected Reference insurer; 10292 10293 /** 10294 * The actual object that is the target of the reference (The insurer which is responsible for the explanation of benefit.) 10295 */ 10296 protected Organization insurerTarget; 10297 10298 /** 10299 * The provider which is responsible for the claim. 10300 */ 10301 @Child(name = "provider", type = {Practitioner.class}, order=9, min=0, max=1, modifier=false, summary=false) 10302 @Description(shortDefinition="Responsible provider for the claim", formalDefinition="The provider which is responsible for the claim." ) 10303 protected Reference provider; 10304 10305 /** 10306 * The actual object that is the target of the reference (The provider which is responsible for the claim.) 10307 */ 10308 protected Practitioner providerTarget; 10309 10310 /** 10311 * The provider which is responsible for the claim. 10312 */ 10313 @Child(name = "organization", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 10314 @Description(shortDefinition="Responsible organization for the claim", formalDefinition="The provider which is responsible for the claim." ) 10315 protected Reference organization; 10316 10317 /** 10318 * The actual object that is the target of the reference (The provider which is responsible for the claim.) 10319 */ 10320 protected Organization organizationTarget; 10321 10322 /** 10323 * The referral resource which lists the date, practitioner, reason and other supporting information. 10324 */ 10325 @Child(name = "referral", type = {ReferralRequest.class}, order=11, min=0, max=1, modifier=false, summary=false) 10326 @Description(shortDefinition="Treatment Referral", formalDefinition="The referral resource which lists the date, practitioner, reason and other supporting information." ) 10327 protected Reference referral; 10328 10329 /** 10330 * The actual object that is the target of the reference (The referral resource which lists the date, practitioner, reason and other supporting information.) 10331 */ 10332 protected ReferralRequest referralTarget; 10333 10334 /** 10335 * Facility where the services were provided. 10336 */ 10337 @Child(name = "facility", type = {Location.class}, order=12, min=0, max=1, modifier=false, summary=false) 10338 @Description(shortDefinition="Servicing Facility", formalDefinition="Facility where the services were provided." ) 10339 protected Reference facility; 10340 10341 /** 10342 * The actual object that is the target of the reference (Facility where the services were provided.) 10343 */ 10344 protected Location facilityTarget; 10345 10346 /** 10347 * The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number. 10348 */ 10349 @Child(name = "claim", type = {Claim.class}, order=13, min=0, max=1, modifier=false, summary=false) 10350 @Description(shortDefinition="Claim reference", formalDefinition="The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number." ) 10351 protected Reference claim; 10352 10353 /** 10354 * The actual object that is the target of the reference (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 10355 */ 10356 protected Claim claimTarget; 10357 10358 /** 10359 * The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number. 10360 */ 10361 @Child(name = "claimResponse", type = {ClaimResponse.class}, order=14, min=0, max=1, modifier=false, summary=false) 10362 @Description(shortDefinition="Claim response reference", formalDefinition="The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number." ) 10363 protected Reference claimResponse; 10364 10365 /** 10366 * The actual object that is the target of the reference (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 10367 */ 10368 protected ClaimResponse claimResponseTarget; 10369 10370 /** 10371 * Processing outcome errror, partial or complete processing. 10372 */ 10373 @Child(name = "outcome", type = {CodeableConcept.class}, order=15, min=0, max=1, modifier=false, summary=false) 10374 @Description(shortDefinition="complete | error | partial", formalDefinition="Processing outcome errror, partial or complete processing." ) 10375 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/remittance-outcome") 10376 protected CodeableConcept outcome; 10377 10378 /** 10379 * A description of the status of the adjudication. 10380 */ 10381 @Child(name = "disposition", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=false) 10382 @Description(shortDefinition="Disposition Message", formalDefinition="A description of the status of the adjudication." ) 10383 protected StringType disposition; 10384 10385 /** 10386 * Other claims which are related to this claim such as prior claim versions or for related services. 10387 */ 10388 @Child(name = "related", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10389 @Description(shortDefinition="Related Claims which may be revelant to processing this claim", formalDefinition="Other claims which are related to this claim such as prior claim versions or for related services." ) 10390 protected List<RelatedClaimComponent> related; 10391 10392 /** 10393 * Prescription to support the dispensing of Pharmacy or Vision products. 10394 */ 10395 @Child(name = "prescription", type = {MedicationRequest.class, VisionPrescription.class}, order=18, min=0, max=1, modifier=false, summary=false) 10396 @Description(shortDefinition="Prescription authorizing services or products", formalDefinition="Prescription to support the dispensing of Pharmacy or Vision products." ) 10397 protected Reference prescription; 10398 10399 /** 10400 * The actual object that is the target of the reference (Prescription to support the dispensing of Pharmacy or Vision products.) 10401 */ 10402 protected Resource prescriptionTarget; 10403 10404 /** 10405 * Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'. 10406 */ 10407 @Child(name = "originalPrescription", type = {MedicationRequest.class}, order=19, min=0, max=1, modifier=false, summary=false) 10408 @Description(shortDefinition="Original prescription if superceded by fulfiller", formalDefinition="Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'." ) 10409 protected Reference originalPrescription; 10410 10411 /** 10412 * The actual object that is the target of the reference (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 10413 */ 10414 protected MedicationRequest originalPrescriptionTarget; 10415 10416 /** 10417 * The party to be reimbursed for the services. 10418 */ 10419 @Child(name = "payee", type = {}, order=20, min=0, max=1, modifier=false, summary=false) 10420 @Description(shortDefinition="Party to be paid any benefits payable", formalDefinition="The party to be reimbursed for the services." ) 10421 protected PayeeComponent payee; 10422 10423 /** 10424 * Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required. 10425 */ 10426 @Child(name = "information", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10427 @Description(shortDefinition="Exceptions, special considerations, the condition, situation, prior or concurrent issues", formalDefinition="Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required." ) 10428 protected List<SupportingInformationComponent> information; 10429 10430 /** 10431 * The members of the team who provided the overall service as well as their role and whether responsible and qualifications. 10432 */ 10433 @Child(name = "careTeam", type = {}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10434 @Description(shortDefinition="Care Team members", formalDefinition="The members of the team who provided the overall service as well as their role and whether responsible and qualifications." ) 10435 protected List<CareTeamComponent> careTeam; 10436 10437 /** 10438 * Ordered list of patient diagnosis for which care is sought. 10439 */ 10440 @Child(name = "diagnosis", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10441 @Description(shortDefinition="List of Diagnosis", formalDefinition="Ordered list of patient diagnosis for which care is sought." ) 10442 protected List<DiagnosisComponent> diagnosis; 10443 10444 /** 10445 * Ordered list of patient procedures performed to support the adjudication. 10446 */ 10447 @Child(name = "procedure", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10448 @Description(shortDefinition="Procedures performed", formalDefinition="Ordered list of patient procedures performed to support the adjudication." ) 10449 protected List<ProcedureComponent> procedure; 10450 10451 /** 10452 * Precedence (primary, secondary, etc.). 10453 */ 10454 @Child(name = "precedence", type = {PositiveIntType.class}, order=25, min=0, max=1, modifier=false, summary=false) 10455 @Description(shortDefinition="Precedence (primary, secondary, etc.)", formalDefinition="Precedence (primary, secondary, etc.)." ) 10456 protected PositiveIntType precedence; 10457 10458 /** 10459 * Financial instrument by which payment information for health care. 10460 */ 10461 @Child(name = "insurance", type = {}, order=26, min=0, max=1, modifier=false, summary=false) 10462 @Description(shortDefinition="Insurance or medical plan", formalDefinition="Financial instrument by which payment information for health care." ) 10463 protected InsuranceComponent insurance; 10464 10465 /** 10466 * An accident which resulted in the need for healthcare services. 10467 */ 10468 @Child(name = "accident", type = {}, order=27, min=0, max=1, modifier=false, summary=false) 10469 @Description(shortDefinition="Details of an accident", formalDefinition="An accident which resulted in the need for healthcare services." ) 10470 protected AccidentComponent accident; 10471 10472 /** 10473 * The start and optional end dates of when the patient was precluded from working due to the treatable condition(s). 10474 */ 10475 @Child(name = "employmentImpacted", type = {Period.class}, order=28, min=0, max=1, modifier=false, summary=false) 10476 @Description(shortDefinition="Period unable to work", formalDefinition="The start and optional end dates of when the patient was precluded from working due to the treatable condition(s)." ) 10477 protected Period employmentImpacted; 10478 10479 /** 10480 * The start and optional end dates of when the patient was confined to a treatment center. 10481 */ 10482 @Child(name = "hospitalization", type = {Period.class}, order=29, min=0, max=1, modifier=false, summary=false) 10483 @Description(shortDefinition="Period in hospital", formalDefinition="The start and optional end dates of when the patient was confined to a treatment center." ) 10484 protected Period hospitalization; 10485 10486 /** 10487 * First tier of goods and services. 10488 */ 10489 @Child(name = "item", type = {}, order=30, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10490 @Description(shortDefinition="Goods and Services", formalDefinition="First tier of goods and services." ) 10491 protected List<ItemComponent> item; 10492 10493 /** 10494 * The first tier service adjudications for payor added services. 10495 */ 10496 @Child(name = "addItem", type = {}, order=31, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10497 @Description(shortDefinition="Insurer added line items", formalDefinition="The first tier service adjudications for payor added services." ) 10498 protected List<AddedItemComponent> addItem; 10499 10500 /** 10501 * The total cost of the services reported. 10502 */ 10503 @Child(name = "totalCost", type = {Money.class}, order=32, min=0, max=1, modifier=false, summary=false) 10504 @Description(shortDefinition="Total Cost of service from the Claim", formalDefinition="The total cost of the services reported." ) 10505 protected Money totalCost; 10506 10507 /** 10508 * The amount of deductable applied which was not allocated to any particular service line. 10509 */ 10510 @Child(name = "unallocDeductable", type = {Money.class}, order=33, min=0, max=1, modifier=false, summary=false) 10511 @Description(shortDefinition="Unallocated deductable", formalDefinition="The amount of deductable applied which was not allocated to any particular service line." ) 10512 protected Money unallocDeductable; 10513 10514 /** 10515 * Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable). 10516 */ 10517 @Child(name = "totalBenefit", type = {Money.class}, order=34, min=0, max=1, modifier=false, summary=false) 10518 @Description(shortDefinition="Total benefit payable for the Claim", formalDefinition="Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable)." ) 10519 protected Money totalBenefit; 10520 10521 /** 10522 * Payment details for the claim if the claim has been paid. 10523 */ 10524 @Child(name = "payment", type = {}, order=35, min=0, max=1, modifier=false, summary=false) 10525 @Description(shortDefinition="Payment (if paid)", formalDefinition="Payment details for the claim if the claim has been paid." ) 10526 protected PaymentComponent payment; 10527 10528 /** 10529 * The form to be used for printing the content. 10530 */ 10531 @Child(name = "form", type = {CodeableConcept.class}, order=36, min=0, max=1, modifier=false, summary=false) 10532 @Description(shortDefinition="Printed Form Identifier", formalDefinition="The form to be used for printing the content." ) 10533 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/forms") 10534 protected CodeableConcept form; 10535 10536 /** 10537 * Note text. 10538 */ 10539 @Child(name = "processNote", type = {}, order=37, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10540 @Description(shortDefinition="Processing notes", formalDefinition="Note text." ) 10541 protected List<NoteComponent> processNote; 10542 10543 /** 10544 * Balance by Benefit Category. 10545 */ 10546 @Child(name = "benefitBalance", type = {}, order=38, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 10547 @Description(shortDefinition="Balance by Benefit Category", formalDefinition="Balance by Benefit Category." ) 10548 protected List<BenefitBalanceComponent> benefitBalance; 10549 10550 private static final long serialVersionUID = -1301056913L; 10551 10552 /** 10553 * Constructor 10554 */ 10555 public ExplanationOfBenefit() { 10556 super(); 10557 } 10558 10559 /** 10560 * @return {@link #identifier} (The EOB Business Identifier.) 10561 */ 10562 public List<Identifier> getIdentifier() { 10563 if (this.identifier == null) 10564 this.identifier = new ArrayList<Identifier>(); 10565 return this.identifier; 10566 } 10567 10568 /** 10569 * @return Returns a reference to <code>this</code> for easy method chaining 10570 */ 10571 public ExplanationOfBenefit setIdentifier(List<Identifier> theIdentifier) { 10572 this.identifier = theIdentifier; 10573 return this; 10574 } 10575 10576 public boolean hasIdentifier() { 10577 if (this.identifier == null) 10578 return false; 10579 for (Identifier item : this.identifier) 10580 if (!item.isEmpty()) 10581 return true; 10582 return false; 10583 } 10584 10585 public Identifier addIdentifier() { //3 10586 Identifier t = new Identifier(); 10587 if (this.identifier == null) 10588 this.identifier = new ArrayList<Identifier>(); 10589 this.identifier.add(t); 10590 return t; 10591 } 10592 10593 public ExplanationOfBenefit addIdentifier(Identifier t) { //3 10594 if (t == null) 10595 return this; 10596 if (this.identifier == null) 10597 this.identifier = new ArrayList<Identifier>(); 10598 this.identifier.add(t); 10599 return this; 10600 } 10601 10602 /** 10603 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 10604 */ 10605 public Identifier getIdentifierFirstRep() { 10606 if (getIdentifier().isEmpty()) { 10607 addIdentifier(); 10608 } 10609 return getIdentifier().get(0); 10610 } 10611 10612 /** 10613 * @return {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 10614 */ 10615 public Enumeration<ExplanationOfBenefitStatus> getStatusElement() { 10616 if (this.status == null) 10617 if (Configuration.errorOnAutoCreate()) 10618 throw new Error("Attempt to auto-create ExplanationOfBenefit.status"); 10619 else if (Configuration.doAutoCreate()) 10620 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); // bb 10621 return this.status; 10622 } 10623 10624 public boolean hasStatusElement() { 10625 return this.status != null && !this.status.isEmpty(); 10626 } 10627 10628 public boolean hasStatus() { 10629 return this.status != null && !this.status.isEmpty(); 10630 } 10631 10632 /** 10633 * @param value {@link #status} (The status of the resource instance.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 10634 */ 10635 public ExplanationOfBenefit setStatusElement(Enumeration<ExplanationOfBenefitStatus> value) { 10636 this.status = value; 10637 return this; 10638 } 10639 10640 /** 10641 * @return The status of the resource instance. 10642 */ 10643 public ExplanationOfBenefitStatus getStatus() { 10644 return this.status == null ? null : this.status.getValue(); 10645 } 10646 10647 /** 10648 * @param value The status of the resource instance. 10649 */ 10650 public ExplanationOfBenefit setStatus(ExplanationOfBenefitStatus value) { 10651 if (value == null) 10652 this.status = null; 10653 else { 10654 if (this.status == null) 10655 this.status = new Enumeration<ExplanationOfBenefitStatus>(new ExplanationOfBenefitStatusEnumFactory()); 10656 this.status.setValue(value); 10657 } 10658 return this; 10659 } 10660 10661 /** 10662 * @return {@link #type} (The category of claim, eg, oral, pharmacy, vision, insitutional, professional.) 10663 */ 10664 public CodeableConcept getType() { 10665 if (this.type == null) 10666 if (Configuration.errorOnAutoCreate()) 10667 throw new Error("Attempt to auto-create ExplanationOfBenefit.type"); 10668 else if (Configuration.doAutoCreate()) 10669 this.type = new CodeableConcept(); // cc 10670 return this.type; 10671 } 10672 10673 public boolean hasType() { 10674 return this.type != null && !this.type.isEmpty(); 10675 } 10676 10677 /** 10678 * @param value {@link #type} (The category of claim, eg, oral, pharmacy, vision, insitutional, professional.) 10679 */ 10680 public ExplanationOfBenefit setType(CodeableConcept value) { 10681 this.type = value; 10682 return this; 10683 } 10684 10685 /** 10686 * @return {@link #subType} (A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.) 10687 */ 10688 public List<CodeableConcept> getSubType() { 10689 if (this.subType == null) 10690 this.subType = new ArrayList<CodeableConcept>(); 10691 return this.subType; 10692 } 10693 10694 /** 10695 * @return Returns a reference to <code>this</code> for easy method chaining 10696 */ 10697 public ExplanationOfBenefit setSubType(List<CodeableConcept> theSubType) { 10698 this.subType = theSubType; 10699 return this; 10700 } 10701 10702 public boolean hasSubType() { 10703 if (this.subType == null) 10704 return false; 10705 for (CodeableConcept item : this.subType) 10706 if (!item.isEmpty()) 10707 return true; 10708 return false; 10709 } 10710 10711 public CodeableConcept addSubType() { //3 10712 CodeableConcept t = new CodeableConcept(); 10713 if (this.subType == null) 10714 this.subType = new ArrayList<CodeableConcept>(); 10715 this.subType.add(t); 10716 return t; 10717 } 10718 10719 public ExplanationOfBenefit addSubType(CodeableConcept t) { //3 10720 if (t == null) 10721 return this; 10722 if (this.subType == null) 10723 this.subType = new ArrayList<CodeableConcept>(); 10724 this.subType.add(t); 10725 return this; 10726 } 10727 10728 /** 10729 * @return The first repetition of repeating field {@link #subType}, creating it if it does not already exist 10730 */ 10731 public CodeableConcept getSubTypeFirstRep() { 10732 if (getSubType().isEmpty()) { 10733 addSubType(); 10734 } 10735 return getSubType().get(0); 10736 } 10737 10738 /** 10739 * @return {@link #patient} (Patient Resource.) 10740 */ 10741 public Reference getPatient() { 10742 if (this.patient == null) 10743 if (Configuration.errorOnAutoCreate()) 10744 throw new Error("Attempt to auto-create ExplanationOfBenefit.patient"); 10745 else if (Configuration.doAutoCreate()) 10746 this.patient = new Reference(); // cc 10747 return this.patient; 10748 } 10749 10750 public boolean hasPatient() { 10751 return this.patient != null && !this.patient.isEmpty(); 10752 } 10753 10754 /** 10755 * @param value {@link #patient} (Patient Resource.) 10756 */ 10757 public ExplanationOfBenefit setPatient(Reference value) { 10758 this.patient = value; 10759 return this; 10760 } 10761 10762 /** 10763 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Patient Resource.) 10764 */ 10765 public Patient getPatientTarget() { 10766 if (this.patientTarget == null) 10767 if (Configuration.errorOnAutoCreate()) 10768 throw new Error("Attempt to auto-create ExplanationOfBenefit.patient"); 10769 else if (Configuration.doAutoCreate()) 10770 this.patientTarget = new Patient(); // aa 10771 return this.patientTarget; 10772 } 10773 10774 /** 10775 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Patient Resource.) 10776 */ 10777 public ExplanationOfBenefit setPatientTarget(Patient value) { 10778 this.patientTarget = value; 10779 return this; 10780 } 10781 10782 /** 10783 * @return {@link #billablePeriod} (The billable period for which charges are being submitted.) 10784 */ 10785 public Period getBillablePeriod() { 10786 if (this.billablePeriod == null) 10787 if (Configuration.errorOnAutoCreate()) 10788 throw new Error("Attempt to auto-create ExplanationOfBenefit.billablePeriod"); 10789 else if (Configuration.doAutoCreate()) 10790 this.billablePeriod = new Period(); // cc 10791 return this.billablePeriod; 10792 } 10793 10794 public boolean hasBillablePeriod() { 10795 return this.billablePeriod != null && !this.billablePeriod.isEmpty(); 10796 } 10797 10798 /** 10799 * @param value {@link #billablePeriod} (The billable period for which charges are being submitted.) 10800 */ 10801 public ExplanationOfBenefit setBillablePeriod(Period value) { 10802 this.billablePeriod = value; 10803 return this; 10804 } 10805 10806 /** 10807 * @return {@link #created} (The date when the EOB was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 10808 */ 10809 public DateTimeType getCreatedElement() { 10810 if (this.created == null) 10811 if (Configuration.errorOnAutoCreate()) 10812 throw new Error("Attempt to auto-create ExplanationOfBenefit.created"); 10813 else if (Configuration.doAutoCreate()) 10814 this.created = new DateTimeType(); // bb 10815 return this.created; 10816 } 10817 10818 public boolean hasCreatedElement() { 10819 return this.created != null && !this.created.isEmpty(); 10820 } 10821 10822 public boolean hasCreated() { 10823 return this.created != null && !this.created.isEmpty(); 10824 } 10825 10826 /** 10827 * @param value {@link #created} (The date when the EOB was created.). This is the underlying object with id, value and extensions. The accessor "getCreated" gives direct access to the value 10828 */ 10829 public ExplanationOfBenefit setCreatedElement(DateTimeType value) { 10830 this.created = value; 10831 return this; 10832 } 10833 10834 /** 10835 * @return The date when the EOB was created. 10836 */ 10837 public Date getCreated() { 10838 return this.created == null ? null : this.created.getValue(); 10839 } 10840 10841 /** 10842 * @param value The date when the EOB was created. 10843 */ 10844 public ExplanationOfBenefit setCreated(Date value) { 10845 if (value == null) 10846 this.created = null; 10847 else { 10848 if (this.created == null) 10849 this.created = new DateTimeType(); 10850 this.created.setValue(value); 10851 } 10852 return this; 10853 } 10854 10855 /** 10856 * @return {@link #enterer} (The person who created the explanation of benefit.) 10857 */ 10858 public Reference getEnterer() { 10859 if (this.enterer == null) 10860 if (Configuration.errorOnAutoCreate()) 10861 throw new Error("Attempt to auto-create ExplanationOfBenefit.enterer"); 10862 else if (Configuration.doAutoCreate()) 10863 this.enterer = new Reference(); // cc 10864 return this.enterer; 10865 } 10866 10867 public boolean hasEnterer() { 10868 return this.enterer != null && !this.enterer.isEmpty(); 10869 } 10870 10871 /** 10872 * @param value {@link #enterer} (The person who created the explanation of benefit.) 10873 */ 10874 public ExplanationOfBenefit setEnterer(Reference value) { 10875 this.enterer = value; 10876 return this; 10877 } 10878 10879 /** 10880 * @return {@link #enterer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person who created the explanation of benefit.) 10881 */ 10882 public Practitioner getEntererTarget() { 10883 if (this.entererTarget == null) 10884 if (Configuration.errorOnAutoCreate()) 10885 throw new Error("Attempt to auto-create ExplanationOfBenefit.enterer"); 10886 else if (Configuration.doAutoCreate()) 10887 this.entererTarget = new Practitioner(); // aa 10888 return this.entererTarget; 10889 } 10890 10891 /** 10892 * @param value {@link #enterer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person who created the explanation of benefit.) 10893 */ 10894 public ExplanationOfBenefit setEntererTarget(Practitioner value) { 10895 this.entererTarget = value; 10896 return this; 10897 } 10898 10899 /** 10900 * @return {@link #insurer} (The insurer which is responsible for the explanation of benefit.) 10901 */ 10902 public Reference getInsurer() { 10903 if (this.insurer == null) 10904 if (Configuration.errorOnAutoCreate()) 10905 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurer"); 10906 else if (Configuration.doAutoCreate()) 10907 this.insurer = new Reference(); // cc 10908 return this.insurer; 10909 } 10910 10911 public boolean hasInsurer() { 10912 return this.insurer != null && !this.insurer.isEmpty(); 10913 } 10914 10915 /** 10916 * @param value {@link #insurer} (The insurer which is responsible for the explanation of benefit.) 10917 */ 10918 public ExplanationOfBenefit setInsurer(Reference value) { 10919 this.insurer = value; 10920 return this; 10921 } 10922 10923 /** 10924 * @return {@link #insurer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The insurer which is responsible for the explanation of benefit.) 10925 */ 10926 public Organization getInsurerTarget() { 10927 if (this.insurerTarget == null) 10928 if (Configuration.errorOnAutoCreate()) 10929 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurer"); 10930 else if (Configuration.doAutoCreate()) 10931 this.insurerTarget = new Organization(); // aa 10932 return this.insurerTarget; 10933 } 10934 10935 /** 10936 * @param value {@link #insurer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The insurer which is responsible for the explanation of benefit.) 10937 */ 10938 public ExplanationOfBenefit setInsurerTarget(Organization value) { 10939 this.insurerTarget = value; 10940 return this; 10941 } 10942 10943 /** 10944 * @return {@link #provider} (The provider which is responsible for the claim.) 10945 */ 10946 public Reference getProvider() { 10947 if (this.provider == null) 10948 if (Configuration.errorOnAutoCreate()) 10949 throw new Error("Attempt to auto-create ExplanationOfBenefit.provider"); 10950 else if (Configuration.doAutoCreate()) 10951 this.provider = new Reference(); // cc 10952 return this.provider; 10953 } 10954 10955 public boolean hasProvider() { 10956 return this.provider != null && !this.provider.isEmpty(); 10957 } 10958 10959 /** 10960 * @param value {@link #provider} (The provider which is responsible for the claim.) 10961 */ 10962 public ExplanationOfBenefit setProvider(Reference value) { 10963 this.provider = value; 10964 return this; 10965 } 10966 10967 /** 10968 * @return {@link #provider} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the claim.) 10969 */ 10970 public Practitioner getProviderTarget() { 10971 if (this.providerTarget == null) 10972 if (Configuration.errorOnAutoCreate()) 10973 throw new Error("Attempt to auto-create ExplanationOfBenefit.provider"); 10974 else if (Configuration.doAutoCreate()) 10975 this.providerTarget = new Practitioner(); // aa 10976 return this.providerTarget; 10977 } 10978 10979 /** 10980 * @param value {@link #provider} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the claim.) 10981 */ 10982 public ExplanationOfBenefit setProviderTarget(Practitioner value) { 10983 this.providerTarget = value; 10984 return this; 10985 } 10986 10987 /** 10988 * @return {@link #organization} (The provider which is responsible for the claim.) 10989 */ 10990 public Reference getOrganization() { 10991 if (this.organization == null) 10992 if (Configuration.errorOnAutoCreate()) 10993 throw new Error("Attempt to auto-create ExplanationOfBenefit.organization"); 10994 else if (Configuration.doAutoCreate()) 10995 this.organization = new Reference(); // cc 10996 return this.organization; 10997 } 10998 10999 public boolean hasOrganization() { 11000 return this.organization != null && !this.organization.isEmpty(); 11001 } 11002 11003 /** 11004 * @param value {@link #organization} (The provider which is responsible for the claim.) 11005 */ 11006 public ExplanationOfBenefit setOrganization(Reference value) { 11007 this.organization = value; 11008 return this; 11009 } 11010 11011 /** 11012 * @return {@link #organization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the claim.) 11013 */ 11014 public Organization getOrganizationTarget() { 11015 if (this.organizationTarget == null) 11016 if (Configuration.errorOnAutoCreate()) 11017 throw new Error("Attempt to auto-create ExplanationOfBenefit.organization"); 11018 else if (Configuration.doAutoCreate()) 11019 this.organizationTarget = new Organization(); // aa 11020 return this.organizationTarget; 11021 } 11022 11023 /** 11024 * @param value {@link #organization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The provider which is responsible for the claim.) 11025 */ 11026 public ExplanationOfBenefit setOrganizationTarget(Organization value) { 11027 this.organizationTarget = value; 11028 return this; 11029 } 11030 11031 /** 11032 * @return {@link #referral} (The referral resource which lists the date, practitioner, reason and other supporting information.) 11033 */ 11034 public Reference getReferral() { 11035 if (this.referral == null) 11036 if (Configuration.errorOnAutoCreate()) 11037 throw new Error("Attempt to auto-create ExplanationOfBenefit.referral"); 11038 else if (Configuration.doAutoCreate()) 11039 this.referral = new Reference(); // cc 11040 return this.referral; 11041 } 11042 11043 public boolean hasReferral() { 11044 return this.referral != null && !this.referral.isEmpty(); 11045 } 11046 11047 /** 11048 * @param value {@link #referral} (The referral resource which lists the date, practitioner, reason and other supporting information.) 11049 */ 11050 public ExplanationOfBenefit setReferral(Reference value) { 11051 this.referral = value; 11052 return this; 11053 } 11054 11055 /** 11056 * @return {@link #referral} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The referral resource which lists the date, practitioner, reason and other supporting information.) 11057 */ 11058 public ReferralRequest getReferralTarget() { 11059 if (this.referralTarget == null) 11060 if (Configuration.errorOnAutoCreate()) 11061 throw new Error("Attempt to auto-create ExplanationOfBenefit.referral"); 11062 else if (Configuration.doAutoCreate()) 11063 this.referralTarget = new ReferralRequest(); // aa 11064 return this.referralTarget; 11065 } 11066 11067 /** 11068 * @param value {@link #referral} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The referral resource which lists the date, practitioner, reason and other supporting information.) 11069 */ 11070 public ExplanationOfBenefit setReferralTarget(ReferralRequest value) { 11071 this.referralTarget = value; 11072 return this; 11073 } 11074 11075 /** 11076 * @return {@link #facility} (Facility where the services were provided.) 11077 */ 11078 public Reference getFacility() { 11079 if (this.facility == null) 11080 if (Configuration.errorOnAutoCreate()) 11081 throw new Error("Attempt to auto-create ExplanationOfBenefit.facility"); 11082 else if (Configuration.doAutoCreate()) 11083 this.facility = new Reference(); // cc 11084 return this.facility; 11085 } 11086 11087 public boolean hasFacility() { 11088 return this.facility != null && !this.facility.isEmpty(); 11089 } 11090 11091 /** 11092 * @param value {@link #facility} (Facility where the services were provided.) 11093 */ 11094 public ExplanationOfBenefit setFacility(Reference value) { 11095 this.facility = value; 11096 return this; 11097 } 11098 11099 /** 11100 * @return {@link #facility} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 11101 */ 11102 public Location getFacilityTarget() { 11103 if (this.facilityTarget == null) 11104 if (Configuration.errorOnAutoCreate()) 11105 throw new Error("Attempt to auto-create ExplanationOfBenefit.facility"); 11106 else if (Configuration.doAutoCreate()) 11107 this.facilityTarget = new Location(); // aa 11108 return this.facilityTarget; 11109 } 11110 11111 /** 11112 * @param value {@link #facility} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Facility where the services were provided.) 11113 */ 11114 public ExplanationOfBenefit setFacilityTarget(Location value) { 11115 this.facilityTarget = value; 11116 return this; 11117 } 11118 11119 /** 11120 * @return {@link #claim} (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11121 */ 11122 public Reference getClaim() { 11123 if (this.claim == null) 11124 if (Configuration.errorOnAutoCreate()) 11125 throw new Error("Attempt to auto-create ExplanationOfBenefit.claim"); 11126 else if (Configuration.doAutoCreate()) 11127 this.claim = new Reference(); // cc 11128 return this.claim; 11129 } 11130 11131 public boolean hasClaim() { 11132 return this.claim != null && !this.claim.isEmpty(); 11133 } 11134 11135 /** 11136 * @param value {@link #claim} (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11137 */ 11138 public ExplanationOfBenefit setClaim(Reference value) { 11139 this.claim = value; 11140 return this; 11141 } 11142 11143 /** 11144 * @return {@link #claim} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11145 */ 11146 public Claim getClaimTarget() { 11147 if (this.claimTarget == null) 11148 if (Configuration.errorOnAutoCreate()) 11149 throw new Error("Attempt to auto-create ExplanationOfBenefit.claim"); 11150 else if (Configuration.doAutoCreate()) 11151 this.claimTarget = new Claim(); // aa 11152 return this.claimTarget; 11153 } 11154 11155 /** 11156 * @param value {@link #claim} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11157 */ 11158 public ExplanationOfBenefit setClaimTarget(Claim value) { 11159 this.claimTarget = value; 11160 return this; 11161 } 11162 11163 /** 11164 * @return {@link #claimResponse} (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11165 */ 11166 public Reference getClaimResponse() { 11167 if (this.claimResponse == null) 11168 if (Configuration.errorOnAutoCreate()) 11169 throw new Error("Attempt to auto-create ExplanationOfBenefit.claimResponse"); 11170 else if (Configuration.doAutoCreate()) 11171 this.claimResponse = new Reference(); // cc 11172 return this.claimResponse; 11173 } 11174 11175 public boolean hasClaimResponse() { 11176 return this.claimResponse != null && !this.claimResponse.isEmpty(); 11177 } 11178 11179 /** 11180 * @param value {@link #claimResponse} (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11181 */ 11182 public ExplanationOfBenefit setClaimResponse(Reference value) { 11183 this.claimResponse = value; 11184 return this; 11185 } 11186 11187 /** 11188 * @return {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11189 */ 11190 public ClaimResponse getClaimResponseTarget() { 11191 if (this.claimResponseTarget == null) 11192 if (Configuration.errorOnAutoCreate()) 11193 throw new Error("Attempt to auto-create ExplanationOfBenefit.claimResponse"); 11194 else if (Configuration.doAutoCreate()) 11195 this.claimResponseTarget = new ClaimResponse(); // aa 11196 return this.claimResponseTarget; 11197 } 11198 11199 /** 11200 * @param value {@link #claimResponse} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.) 11201 */ 11202 public ExplanationOfBenefit setClaimResponseTarget(ClaimResponse value) { 11203 this.claimResponseTarget = value; 11204 return this; 11205 } 11206 11207 /** 11208 * @return {@link #outcome} (Processing outcome errror, partial or complete processing.) 11209 */ 11210 public CodeableConcept getOutcome() { 11211 if (this.outcome == null) 11212 if (Configuration.errorOnAutoCreate()) 11213 throw new Error("Attempt to auto-create ExplanationOfBenefit.outcome"); 11214 else if (Configuration.doAutoCreate()) 11215 this.outcome = new CodeableConcept(); // cc 11216 return this.outcome; 11217 } 11218 11219 public boolean hasOutcome() { 11220 return this.outcome != null && !this.outcome.isEmpty(); 11221 } 11222 11223 /** 11224 * @param value {@link #outcome} (Processing outcome errror, partial or complete processing.) 11225 */ 11226 public ExplanationOfBenefit setOutcome(CodeableConcept value) { 11227 this.outcome = value; 11228 return this; 11229 } 11230 11231 /** 11232 * @return {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 11233 */ 11234 public StringType getDispositionElement() { 11235 if (this.disposition == null) 11236 if (Configuration.errorOnAutoCreate()) 11237 throw new Error("Attempt to auto-create ExplanationOfBenefit.disposition"); 11238 else if (Configuration.doAutoCreate()) 11239 this.disposition = new StringType(); // bb 11240 return this.disposition; 11241 } 11242 11243 public boolean hasDispositionElement() { 11244 return this.disposition != null && !this.disposition.isEmpty(); 11245 } 11246 11247 public boolean hasDisposition() { 11248 return this.disposition != null && !this.disposition.isEmpty(); 11249 } 11250 11251 /** 11252 * @param value {@link #disposition} (A description of the status of the adjudication.). This is the underlying object with id, value and extensions. The accessor "getDisposition" gives direct access to the value 11253 */ 11254 public ExplanationOfBenefit setDispositionElement(StringType value) { 11255 this.disposition = value; 11256 return this; 11257 } 11258 11259 /** 11260 * @return A description of the status of the adjudication. 11261 */ 11262 public String getDisposition() { 11263 return this.disposition == null ? null : this.disposition.getValue(); 11264 } 11265 11266 /** 11267 * @param value A description of the status of the adjudication. 11268 */ 11269 public ExplanationOfBenefit setDisposition(String value) { 11270 if (Utilities.noString(value)) 11271 this.disposition = null; 11272 else { 11273 if (this.disposition == null) 11274 this.disposition = new StringType(); 11275 this.disposition.setValue(value); 11276 } 11277 return this; 11278 } 11279 11280 /** 11281 * @return {@link #related} (Other claims which are related to this claim such as prior claim versions or for related services.) 11282 */ 11283 public List<RelatedClaimComponent> getRelated() { 11284 if (this.related == null) 11285 this.related = new ArrayList<RelatedClaimComponent>(); 11286 return this.related; 11287 } 11288 11289 /** 11290 * @return Returns a reference to <code>this</code> for easy method chaining 11291 */ 11292 public ExplanationOfBenefit setRelated(List<RelatedClaimComponent> theRelated) { 11293 this.related = theRelated; 11294 return this; 11295 } 11296 11297 public boolean hasRelated() { 11298 if (this.related == null) 11299 return false; 11300 for (RelatedClaimComponent item : this.related) 11301 if (!item.isEmpty()) 11302 return true; 11303 return false; 11304 } 11305 11306 public RelatedClaimComponent addRelated() { //3 11307 RelatedClaimComponent t = new RelatedClaimComponent(); 11308 if (this.related == null) 11309 this.related = new ArrayList<RelatedClaimComponent>(); 11310 this.related.add(t); 11311 return t; 11312 } 11313 11314 public ExplanationOfBenefit addRelated(RelatedClaimComponent t) { //3 11315 if (t == null) 11316 return this; 11317 if (this.related == null) 11318 this.related = new ArrayList<RelatedClaimComponent>(); 11319 this.related.add(t); 11320 return this; 11321 } 11322 11323 /** 11324 * @return The first repetition of repeating field {@link #related}, creating it if it does not already exist 11325 */ 11326 public RelatedClaimComponent getRelatedFirstRep() { 11327 if (getRelated().isEmpty()) { 11328 addRelated(); 11329 } 11330 return getRelated().get(0); 11331 } 11332 11333 /** 11334 * @return {@link #prescription} (Prescription to support the dispensing of Pharmacy or Vision products.) 11335 */ 11336 public Reference getPrescription() { 11337 if (this.prescription == null) 11338 if (Configuration.errorOnAutoCreate()) 11339 throw new Error("Attempt to auto-create ExplanationOfBenefit.prescription"); 11340 else if (Configuration.doAutoCreate()) 11341 this.prescription = new Reference(); // cc 11342 return this.prescription; 11343 } 11344 11345 public boolean hasPrescription() { 11346 return this.prescription != null && !this.prescription.isEmpty(); 11347 } 11348 11349 /** 11350 * @param value {@link #prescription} (Prescription to support the dispensing of Pharmacy or Vision products.) 11351 */ 11352 public ExplanationOfBenefit setPrescription(Reference value) { 11353 this.prescription = value; 11354 return this; 11355 } 11356 11357 /** 11358 * @return {@link #prescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Prescription to support the dispensing of Pharmacy or Vision products.) 11359 */ 11360 public Resource getPrescriptionTarget() { 11361 return this.prescriptionTarget; 11362 } 11363 11364 /** 11365 * @param value {@link #prescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Prescription to support the dispensing of Pharmacy or Vision products.) 11366 */ 11367 public ExplanationOfBenefit setPrescriptionTarget(Resource value) { 11368 this.prescriptionTarget = value; 11369 return this; 11370 } 11371 11372 /** 11373 * @return {@link #originalPrescription} (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 11374 */ 11375 public Reference getOriginalPrescription() { 11376 if (this.originalPrescription == null) 11377 if (Configuration.errorOnAutoCreate()) 11378 throw new Error("Attempt to auto-create ExplanationOfBenefit.originalPrescription"); 11379 else if (Configuration.doAutoCreate()) 11380 this.originalPrescription = new Reference(); // cc 11381 return this.originalPrescription; 11382 } 11383 11384 public boolean hasOriginalPrescription() { 11385 return this.originalPrescription != null && !this.originalPrescription.isEmpty(); 11386 } 11387 11388 /** 11389 * @param value {@link #originalPrescription} (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 11390 */ 11391 public ExplanationOfBenefit setOriginalPrescription(Reference value) { 11392 this.originalPrescription = value; 11393 return this; 11394 } 11395 11396 /** 11397 * @return {@link #originalPrescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 11398 */ 11399 public MedicationRequest getOriginalPrescriptionTarget() { 11400 if (this.originalPrescriptionTarget == null) 11401 if (Configuration.errorOnAutoCreate()) 11402 throw new Error("Attempt to auto-create ExplanationOfBenefit.originalPrescription"); 11403 else if (Configuration.doAutoCreate()) 11404 this.originalPrescriptionTarget = new MedicationRequest(); // aa 11405 return this.originalPrescriptionTarget; 11406 } 11407 11408 /** 11409 * @param value {@link #originalPrescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.) 11410 */ 11411 public ExplanationOfBenefit setOriginalPrescriptionTarget(MedicationRequest value) { 11412 this.originalPrescriptionTarget = value; 11413 return this; 11414 } 11415 11416 /** 11417 * @return {@link #payee} (The party to be reimbursed for the services.) 11418 */ 11419 public PayeeComponent getPayee() { 11420 if (this.payee == null) 11421 if (Configuration.errorOnAutoCreate()) 11422 throw new Error("Attempt to auto-create ExplanationOfBenefit.payee"); 11423 else if (Configuration.doAutoCreate()) 11424 this.payee = new PayeeComponent(); // cc 11425 return this.payee; 11426 } 11427 11428 public boolean hasPayee() { 11429 return this.payee != null && !this.payee.isEmpty(); 11430 } 11431 11432 /** 11433 * @param value {@link #payee} (The party to be reimbursed for the services.) 11434 */ 11435 public ExplanationOfBenefit setPayee(PayeeComponent value) { 11436 this.payee = value; 11437 return this; 11438 } 11439 11440 /** 11441 * @return {@link #information} (Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.) 11442 */ 11443 public List<SupportingInformationComponent> getInformation() { 11444 if (this.information == null) 11445 this.information = new ArrayList<SupportingInformationComponent>(); 11446 return this.information; 11447 } 11448 11449 /** 11450 * @return Returns a reference to <code>this</code> for easy method chaining 11451 */ 11452 public ExplanationOfBenefit setInformation(List<SupportingInformationComponent> theInformation) { 11453 this.information = theInformation; 11454 return this; 11455 } 11456 11457 public boolean hasInformation() { 11458 if (this.information == null) 11459 return false; 11460 for (SupportingInformationComponent item : this.information) 11461 if (!item.isEmpty()) 11462 return true; 11463 return false; 11464 } 11465 11466 public SupportingInformationComponent addInformation() { //3 11467 SupportingInformationComponent t = new SupportingInformationComponent(); 11468 if (this.information == null) 11469 this.information = new ArrayList<SupportingInformationComponent>(); 11470 this.information.add(t); 11471 return t; 11472 } 11473 11474 public ExplanationOfBenefit addInformation(SupportingInformationComponent t) { //3 11475 if (t == null) 11476 return this; 11477 if (this.information == null) 11478 this.information = new ArrayList<SupportingInformationComponent>(); 11479 this.information.add(t); 11480 return this; 11481 } 11482 11483 /** 11484 * @return The first repetition of repeating field {@link #information}, creating it if it does not already exist 11485 */ 11486 public SupportingInformationComponent getInformationFirstRep() { 11487 if (getInformation().isEmpty()) { 11488 addInformation(); 11489 } 11490 return getInformation().get(0); 11491 } 11492 11493 /** 11494 * @return {@link #careTeam} (The members of the team who provided the overall service as well as their role and whether responsible and qualifications.) 11495 */ 11496 public List<CareTeamComponent> getCareTeam() { 11497 if (this.careTeam == null) 11498 this.careTeam = new ArrayList<CareTeamComponent>(); 11499 return this.careTeam; 11500 } 11501 11502 /** 11503 * @return Returns a reference to <code>this</code> for easy method chaining 11504 */ 11505 public ExplanationOfBenefit setCareTeam(List<CareTeamComponent> theCareTeam) { 11506 this.careTeam = theCareTeam; 11507 return this; 11508 } 11509 11510 public boolean hasCareTeam() { 11511 if (this.careTeam == null) 11512 return false; 11513 for (CareTeamComponent item : this.careTeam) 11514 if (!item.isEmpty()) 11515 return true; 11516 return false; 11517 } 11518 11519 public CareTeamComponent addCareTeam() { //3 11520 CareTeamComponent t = new CareTeamComponent(); 11521 if (this.careTeam == null) 11522 this.careTeam = new ArrayList<CareTeamComponent>(); 11523 this.careTeam.add(t); 11524 return t; 11525 } 11526 11527 public ExplanationOfBenefit addCareTeam(CareTeamComponent t) { //3 11528 if (t == null) 11529 return this; 11530 if (this.careTeam == null) 11531 this.careTeam = new ArrayList<CareTeamComponent>(); 11532 this.careTeam.add(t); 11533 return this; 11534 } 11535 11536 /** 11537 * @return The first repetition of repeating field {@link #careTeam}, creating it if it does not already exist 11538 */ 11539 public CareTeamComponent getCareTeamFirstRep() { 11540 if (getCareTeam().isEmpty()) { 11541 addCareTeam(); 11542 } 11543 return getCareTeam().get(0); 11544 } 11545 11546 /** 11547 * @return {@link #diagnosis} (Ordered list of patient diagnosis for which care is sought.) 11548 */ 11549 public List<DiagnosisComponent> getDiagnosis() { 11550 if (this.diagnosis == null) 11551 this.diagnosis = new ArrayList<DiagnosisComponent>(); 11552 return this.diagnosis; 11553 } 11554 11555 /** 11556 * @return Returns a reference to <code>this</code> for easy method chaining 11557 */ 11558 public ExplanationOfBenefit setDiagnosis(List<DiagnosisComponent> theDiagnosis) { 11559 this.diagnosis = theDiagnosis; 11560 return this; 11561 } 11562 11563 public boolean hasDiagnosis() { 11564 if (this.diagnosis == null) 11565 return false; 11566 for (DiagnosisComponent item : this.diagnosis) 11567 if (!item.isEmpty()) 11568 return true; 11569 return false; 11570 } 11571 11572 public DiagnosisComponent addDiagnosis() { //3 11573 DiagnosisComponent t = new DiagnosisComponent(); 11574 if (this.diagnosis == null) 11575 this.diagnosis = new ArrayList<DiagnosisComponent>(); 11576 this.diagnosis.add(t); 11577 return t; 11578 } 11579 11580 public ExplanationOfBenefit addDiagnosis(DiagnosisComponent t) { //3 11581 if (t == null) 11582 return this; 11583 if (this.diagnosis == null) 11584 this.diagnosis = new ArrayList<DiagnosisComponent>(); 11585 this.diagnosis.add(t); 11586 return this; 11587 } 11588 11589 /** 11590 * @return The first repetition of repeating field {@link #diagnosis}, creating it if it does not already exist 11591 */ 11592 public DiagnosisComponent getDiagnosisFirstRep() { 11593 if (getDiagnosis().isEmpty()) { 11594 addDiagnosis(); 11595 } 11596 return getDiagnosis().get(0); 11597 } 11598 11599 /** 11600 * @return {@link #procedure} (Ordered list of patient procedures performed to support the adjudication.) 11601 */ 11602 public List<ProcedureComponent> getProcedure() { 11603 if (this.procedure == null) 11604 this.procedure = new ArrayList<ProcedureComponent>(); 11605 return this.procedure; 11606 } 11607 11608 /** 11609 * @return Returns a reference to <code>this</code> for easy method chaining 11610 */ 11611 public ExplanationOfBenefit setProcedure(List<ProcedureComponent> theProcedure) { 11612 this.procedure = theProcedure; 11613 return this; 11614 } 11615 11616 public boolean hasProcedure() { 11617 if (this.procedure == null) 11618 return false; 11619 for (ProcedureComponent item : this.procedure) 11620 if (!item.isEmpty()) 11621 return true; 11622 return false; 11623 } 11624 11625 public ProcedureComponent addProcedure() { //3 11626 ProcedureComponent t = new ProcedureComponent(); 11627 if (this.procedure == null) 11628 this.procedure = new ArrayList<ProcedureComponent>(); 11629 this.procedure.add(t); 11630 return t; 11631 } 11632 11633 public ExplanationOfBenefit addProcedure(ProcedureComponent t) { //3 11634 if (t == null) 11635 return this; 11636 if (this.procedure == null) 11637 this.procedure = new ArrayList<ProcedureComponent>(); 11638 this.procedure.add(t); 11639 return this; 11640 } 11641 11642 /** 11643 * @return The first repetition of repeating field {@link #procedure}, creating it if it does not already exist 11644 */ 11645 public ProcedureComponent getProcedureFirstRep() { 11646 if (getProcedure().isEmpty()) { 11647 addProcedure(); 11648 } 11649 return getProcedure().get(0); 11650 } 11651 11652 /** 11653 * @return {@link #precedence} (Precedence (primary, secondary, etc.).). This is the underlying object with id, value and extensions. The accessor "getPrecedence" gives direct access to the value 11654 */ 11655 public PositiveIntType getPrecedenceElement() { 11656 if (this.precedence == null) 11657 if (Configuration.errorOnAutoCreate()) 11658 throw new Error("Attempt to auto-create ExplanationOfBenefit.precedence"); 11659 else if (Configuration.doAutoCreate()) 11660 this.precedence = new PositiveIntType(); // bb 11661 return this.precedence; 11662 } 11663 11664 public boolean hasPrecedenceElement() { 11665 return this.precedence != null && !this.precedence.isEmpty(); 11666 } 11667 11668 public boolean hasPrecedence() { 11669 return this.precedence != null && !this.precedence.isEmpty(); 11670 } 11671 11672 /** 11673 * @param value {@link #precedence} (Precedence (primary, secondary, etc.).). This is the underlying object with id, value and extensions. The accessor "getPrecedence" gives direct access to the value 11674 */ 11675 public ExplanationOfBenefit setPrecedenceElement(PositiveIntType value) { 11676 this.precedence = value; 11677 return this; 11678 } 11679 11680 /** 11681 * @return Precedence (primary, secondary, etc.). 11682 */ 11683 public int getPrecedence() { 11684 return this.precedence == null || this.precedence.isEmpty() ? 0 : this.precedence.getValue(); 11685 } 11686 11687 /** 11688 * @param value Precedence (primary, secondary, etc.). 11689 */ 11690 public ExplanationOfBenefit setPrecedence(int value) { 11691 if (this.precedence == null) 11692 this.precedence = new PositiveIntType(); 11693 this.precedence.setValue(value); 11694 return this; 11695 } 11696 11697 /** 11698 * @return {@link #insurance} (Financial instrument by which payment information for health care.) 11699 */ 11700 public InsuranceComponent getInsurance() { 11701 if (this.insurance == null) 11702 if (Configuration.errorOnAutoCreate()) 11703 throw new Error("Attempt to auto-create ExplanationOfBenefit.insurance"); 11704 else if (Configuration.doAutoCreate()) 11705 this.insurance = new InsuranceComponent(); // cc 11706 return this.insurance; 11707 } 11708 11709 public boolean hasInsurance() { 11710 return this.insurance != null && !this.insurance.isEmpty(); 11711 } 11712 11713 /** 11714 * @param value {@link #insurance} (Financial instrument by which payment information for health care.) 11715 */ 11716 public ExplanationOfBenefit setInsurance(InsuranceComponent value) { 11717 this.insurance = value; 11718 return this; 11719 } 11720 11721 /** 11722 * @return {@link #accident} (An accident which resulted in the need for healthcare services.) 11723 */ 11724 public AccidentComponent getAccident() { 11725 if (this.accident == null) 11726 if (Configuration.errorOnAutoCreate()) 11727 throw new Error("Attempt to auto-create ExplanationOfBenefit.accident"); 11728 else if (Configuration.doAutoCreate()) 11729 this.accident = new AccidentComponent(); // cc 11730 return this.accident; 11731 } 11732 11733 public boolean hasAccident() { 11734 return this.accident != null && !this.accident.isEmpty(); 11735 } 11736 11737 /** 11738 * @param value {@link #accident} (An accident which resulted in the need for healthcare services.) 11739 */ 11740 public ExplanationOfBenefit setAccident(AccidentComponent value) { 11741 this.accident = value; 11742 return this; 11743 } 11744 11745 /** 11746 * @return {@link #employmentImpacted} (The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).) 11747 */ 11748 public Period getEmploymentImpacted() { 11749 if (this.employmentImpacted == null) 11750 if (Configuration.errorOnAutoCreate()) 11751 throw new Error("Attempt to auto-create ExplanationOfBenefit.employmentImpacted"); 11752 else if (Configuration.doAutoCreate()) 11753 this.employmentImpacted = new Period(); // cc 11754 return this.employmentImpacted; 11755 } 11756 11757 public boolean hasEmploymentImpacted() { 11758 return this.employmentImpacted != null && !this.employmentImpacted.isEmpty(); 11759 } 11760 11761 /** 11762 * @param value {@link #employmentImpacted} (The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).) 11763 */ 11764 public ExplanationOfBenefit setEmploymentImpacted(Period value) { 11765 this.employmentImpacted = value; 11766 return this; 11767 } 11768 11769 /** 11770 * @return {@link #hospitalization} (The start and optional end dates of when the patient was confined to a treatment center.) 11771 */ 11772 public Period getHospitalization() { 11773 if (this.hospitalization == null) 11774 if (Configuration.errorOnAutoCreate()) 11775 throw new Error("Attempt to auto-create ExplanationOfBenefit.hospitalization"); 11776 else if (Configuration.doAutoCreate()) 11777 this.hospitalization = new Period(); // cc 11778 return this.hospitalization; 11779 } 11780 11781 public boolean hasHospitalization() { 11782 return this.hospitalization != null && !this.hospitalization.isEmpty(); 11783 } 11784 11785 /** 11786 * @param value {@link #hospitalization} (The start and optional end dates of when the patient was confined to a treatment center.) 11787 */ 11788 public ExplanationOfBenefit setHospitalization(Period value) { 11789 this.hospitalization = value; 11790 return this; 11791 } 11792 11793 /** 11794 * @return {@link #item} (First tier of goods and services.) 11795 */ 11796 public List<ItemComponent> getItem() { 11797 if (this.item == null) 11798 this.item = new ArrayList<ItemComponent>(); 11799 return this.item; 11800 } 11801 11802 /** 11803 * @return Returns a reference to <code>this</code> for easy method chaining 11804 */ 11805 public ExplanationOfBenefit setItem(List<ItemComponent> theItem) { 11806 this.item = theItem; 11807 return this; 11808 } 11809 11810 public boolean hasItem() { 11811 if (this.item == null) 11812 return false; 11813 for (ItemComponent item : this.item) 11814 if (!item.isEmpty()) 11815 return true; 11816 return false; 11817 } 11818 11819 public ItemComponent addItem() { //3 11820 ItemComponent t = new ItemComponent(); 11821 if (this.item == null) 11822 this.item = new ArrayList<ItemComponent>(); 11823 this.item.add(t); 11824 return t; 11825 } 11826 11827 public ExplanationOfBenefit addItem(ItemComponent t) { //3 11828 if (t == null) 11829 return this; 11830 if (this.item == null) 11831 this.item = new ArrayList<ItemComponent>(); 11832 this.item.add(t); 11833 return this; 11834 } 11835 11836 /** 11837 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 11838 */ 11839 public ItemComponent getItemFirstRep() { 11840 if (getItem().isEmpty()) { 11841 addItem(); 11842 } 11843 return getItem().get(0); 11844 } 11845 11846 /** 11847 * @return {@link #addItem} (The first tier service adjudications for payor added services.) 11848 */ 11849 public List<AddedItemComponent> getAddItem() { 11850 if (this.addItem == null) 11851 this.addItem = new ArrayList<AddedItemComponent>(); 11852 return this.addItem; 11853 } 11854 11855 /** 11856 * @return Returns a reference to <code>this</code> for easy method chaining 11857 */ 11858 public ExplanationOfBenefit setAddItem(List<AddedItemComponent> theAddItem) { 11859 this.addItem = theAddItem; 11860 return this; 11861 } 11862 11863 public boolean hasAddItem() { 11864 if (this.addItem == null) 11865 return false; 11866 for (AddedItemComponent item : this.addItem) 11867 if (!item.isEmpty()) 11868 return true; 11869 return false; 11870 } 11871 11872 public AddedItemComponent addAddItem() { //3 11873 AddedItemComponent t = new AddedItemComponent(); 11874 if (this.addItem == null) 11875 this.addItem = new ArrayList<AddedItemComponent>(); 11876 this.addItem.add(t); 11877 return t; 11878 } 11879 11880 public ExplanationOfBenefit addAddItem(AddedItemComponent t) { //3 11881 if (t == null) 11882 return this; 11883 if (this.addItem == null) 11884 this.addItem = new ArrayList<AddedItemComponent>(); 11885 this.addItem.add(t); 11886 return this; 11887 } 11888 11889 /** 11890 * @return The first repetition of repeating field {@link #addItem}, creating it if it does not already exist 11891 */ 11892 public AddedItemComponent getAddItemFirstRep() { 11893 if (getAddItem().isEmpty()) { 11894 addAddItem(); 11895 } 11896 return getAddItem().get(0); 11897 } 11898 11899 /** 11900 * @return {@link #totalCost} (The total cost of the services reported.) 11901 */ 11902 public Money getTotalCost() { 11903 if (this.totalCost == null) 11904 if (Configuration.errorOnAutoCreate()) 11905 throw new Error("Attempt to auto-create ExplanationOfBenefit.totalCost"); 11906 else if (Configuration.doAutoCreate()) 11907 this.totalCost = new Money(); // cc 11908 return this.totalCost; 11909 } 11910 11911 public boolean hasTotalCost() { 11912 return this.totalCost != null && !this.totalCost.isEmpty(); 11913 } 11914 11915 /** 11916 * @param value {@link #totalCost} (The total cost of the services reported.) 11917 */ 11918 public ExplanationOfBenefit setTotalCost(Money value) { 11919 this.totalCost = value; 11920 return this; 11921 } 11922 11923 /** 11924 * @return {@link #unallocDeductable} (The amount of deductable applied which was not allocated to any particular service line.) 11925 */ 11926 public Money getUnallocDeductable() { 11927 if (this.unallocDeductable == null) 11928 if (Configuration.errorOnAutoCreate()) 11929 throw new Error("Attempt to auto-create ExplanationOfBenefit.unallocDeductable"); 11930 else if (Configuration.doAutoCreate()) 11931 this.unallocDeductable = new Money(); // cc 11932 return this.unallocDeductable; 11933 } 11934 11935 public boolean hasUnallocDeductable() { 11936 return this.unallocDeductable != null && !this.unallocDeductable.isEmpty(); 11937 } 11938 11939 /** 11940 * @param value {@link #unallocDeductable} (The amount of deductable applied which was not allocated to any particular service line.) 11941 */ 11942 public ExplanationOfBenefit setUnallocDeductable(Money value) { 11943 this.unallocDeductable = value; 11944 return this; 11945 } 11946 11947 /** 11948 * @return {@link #totalBenefit} (Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable).) 11949 */ 11950 public Money getTotalBenefit() { 11951 if (this.totalBenefit == null) 11952 if (Configuration.errorOnAutoCreate()) 11953 throw new Error("Attempt to auto-create ExplanationOfBenefit.totalBenefit"); 11954 else if (Configuration.doAutoCreate()) 11955 this.totalBenefit = new Money(); // cc 11956 return this.totalBenefit; 11957 } 11958 11959 public boolean hasTotalBenefit() { 11960 return this.totalBenefit != null && !this.totalBenefit.isEmpty(); 11961 } 11962 11963 /** 11964 * @param value {@link #totalBenefit} (Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable).) 11965 */ 11966 public ExplanationOfBenefit setTotalBenefit(Money value) { 11967 this.totalBenefit = value; 11968 return this; 11969 } 11970 11971 /** 11972 * @return {@link #payment} (Payment details for the claim if the claim has been paid.) 11973 */ 11974 public PaymentComponent getPayment() { 11975 if (this.payment == null) 11976 if (Configuration.errorOnAutoCreate()) 11977 throw new Error("Attempt to auto-create ExplanationOfBenefit.payment"); 11978 else if (Configuration.doAutoCreate()) 11979 this.payment = new PaymentComponent(); // cc 11980 return this.payment; 11981 } 11982 11983 public boolean hasPayment() { 11984 return this.payment != null && !this.payment.isEmpty(); 11985 } 11986 11987 /** 11988 * @param value {@link #payment} (Payment details for the claim if the claim has been paid.) 11989 */ 11990 public ExplanationOfBenefit setPayment(PaymentComponent value) { 11991 this.payment = value; 11992 return this; 11993 } 11994 11995 /** 11996 * @return {@link #form} (The form to be used for printing the content.) 11997 */ 11998 public CodeableConcept getForm() { 11999 if (this.form == null) 12000 if (Configuration.errorOnAutoCreate()) 12001 throw new Error("Attempt to auto-create ExplanationOfBenefit.form"); 12002 else if (Configuration.doAutoCreate()) 12003 this.form = new CodeableConcept(); // cc 12004 return this.form; 12005 } 12006 12007 public boolean hasForm() { 12008 return this.form != null && !this.form.isEmpty(); 12009 } 12010 12011 /** 12012 * @param value {@link #form} (The form to be used for printing the content.) 12013 */ 12014 public ExplanationOfBenefit setForm(CodeableConcept value) { 12015 this.form = value; 12016 return this; 12017 } 12018 12019 /** 12020 * @return {@link #processNote} (Note text.) 12021 */ 12022 public List<NoteComponent> getProcessNote() { 12023 if (this.processNote == null) 12024 this.processNote = new ArrayList<NoteComponent>(); 12025 return this.processNote; 12026 } 12027 12028 /** 12029 * @return Returns a reference to <code>this</code> for easy method chaining 12030 */ 12031 public ExplanationOfBenefit setProcessNote(List<NoteComponent> theProcessNote) { 12032 this.processNote = theProcessNote; 12033 return this; 12034 } 12035 12036 public boolean hasProcessNote() { 12037 if (this.processNote == null) 12038 return false; 12039 for (NoteComponent item : this.processNote) 12040 if (!item.isEmpty()) 12041 return true; 12042 return false; 12043 } 12044 12045 public NoteComponent addProcessNote() { //3 12046 NoteComponent t = new NoteComponent(); 12047 if (this.processNote == null) 12048 this.processNote = new ArrayList<NoteComponent>(); 12049 this.processNote.add(t); 12050 return t; 12051 } 12052 12053 public ExplanationOfBenefit addProcessNote(NoteComponent t) { //3 12054 if (t == null) 12055 return this; 12056 if (this.processNote == null) 12057 this.processNote = new ArrayList<NoteComponent>(); 12058 this.processNote.add(t); 12059 return this; 12060 } 12061 12062 /** 12063 * @return The first repetition of repeating field {@link #processNote}, creating it if it does not already exist 12064 */ 12065 public NoteComponent getProcessNoteFirstRep() { 12066 if (getProcessNote().isEmpty()) { 12067 addProcessNote(); 12068 } 12069 return getProcessNote().get(0); 12070 } 12071 12072 /** 12073 * @return {@link #benefitBalance} (Balance by Benefit Category.) 12074 */ 12075 public List<BenefitBalanceComponent> getBenefitBalance() { 12076 if (this.benefitBalance == null) 12077 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 12078 return this.benefitBalance; 12079 } 12080 12081 /** 12082 * @return Returns a reference to <code>this</code> for easy method chaining 12083 */ 12084 public ExplanationOfBenefit setBenefitBalance(List<BenefitBalanceComponent> theBenefitBalance) { 12085 this.benefitBalance = theBenefitBalance; 12086 return this; 12087 } 12088 12089 public boolean hasBenefitBalance() { 12090 if (this.benefitBalance == null) 12091 return false; 12092 for (BenefitBalanceComponent item : this.benefitBalance) 12093 if (!item.isEmpty()) 12094 return true; 12095 return false; 12096 } 12097 12098 public BenefitBalanceComponent addBenefitBalance() { //3 12099 BenefitBalanceComponent t = new BenefitBalanceComponent(); 12100 if (this.benefitBalance == null) 12101 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 12102 this.benefitBalance.add(t); 12103 return t; 12104 } 12105 12106 public ExplanationOfBenefit addBenefitBalance(BenefitBalanceComponent t) { //3 12107 if (t == null) 12108 return this; 12109 if (this.benefitBalance == null) 12110 this.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 12111 this.benefitBalance.add(t); 12112 return this; 12113 } 12114 12115 /** 12116 * @return The first repetition of repeating field {@link #benefitBalance}, creating it if it does not already exist 12117 */ 12118 public BenefitBalanceComponent getBenefitBalanceFirstRep() { 12119 if (getBenefitBalance().isEmpty()) { 12120 addBenefitBalance(); 12121 } 12122 return getBenefitBalance().get(0); 12123 } 12124 12125 protected void listChildren(List<Property> children) { 12126 super.listChildren(children); 12127 children.add(new Property("identifier", "Identifier", "The EOB Business Identifier.", 0, java.lang.Integer.MAX_VALUE, identifier)); 12128 children.add(new Property("status", "code", "The status of the resource instance.", 0, 1, status)); 12129 children.add(new Property("type", "CodeableConcept", "The category of claim, eg, oral, pharmacy, vision, insitutional, professional.", 0, 1, type)); 12130 children.add(new Property("subType", "CodeableConcept", "A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.", 0, java.lang.Integer.MAX_VALUE, subType)); 12131 children.add(new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient)); 12132 children.add(new Property("billablePeriod", "Period", "The billable period for which charges are being submitted.", 0, 1, billablePeriod)); 12133 children.add(new Property("created", "dateTime", "The date when the EOB was created.", 0, 1, created)); 12134 children.add(new Property("enterer", "Reference(Practitioner)", "The person who created the explanation of benefit.", 0, 1, enterer)); 12135 children.add(new Property("insurer", "Reference(Organization)", "The insurer which is responsible for the explanation of benefit.", 0, 1, insurer)); 12136 children.add(new Property("provider", "Reference(Practitioner)", "The provider which is responsible for the claim.", 0, 1, provider)); 12137 children.add(new Property("organization", "Reference(Organization)", "The provider which is responsible for the claim.", 0, 1, organization)); 12138 children.add(new Property("referral", "Reference(ReferralRequest)", "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 1, referral)); 12139 children.add(new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility)); 12140 children.add(new Property("claim", "Reference(Claim)", "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 0, 1, claim)); 12141 children.add(new Property("claimResponse", "Reference(ClaimResponse)", "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 0, 1, claimResponse)); 12142 children.add(new Property("outcome", "CodeableConcept", "Processing outcome errror, partial or complete processing.", 0, 1, outcome)); 12143 children.add(new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition)); 12144 children.add(new Property("related", "", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, java.lang.Integer.MAX_VALUE, related)); 12145 children.add(new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription to support the dispensing of Pharmacy or Vision products.", 0, 1, prescription)); 12146 children.add(new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.", 0, 1, originalPrescription)); 12147 children.add(new Property("payee", "", "The party to be reimbursed for the services.", 0, 1, payee)); 12148 children.add(new Property("information", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.", 0, java.lang.Integer.MAX_VALUE, information)); 12149 children.add(new Property("careTeam", "", "The members of the team who provided the overall service as well as their role and whether responsible and qualifications.", 0, java.lang.Integer.MAX_VALUE, careTeam)); 12150 children.add(new Property("diagnosis", "", "Ordered list of patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis)); 12151 children.add(new Property("procedure", "", "Ordered list of patient procedures performed to support the adjudication.", 0, java.lang.Integer.MAX_VALUE, procedure)); 12152 children.add(new Property("precedence", "positiveInt", "Precedence (primary, secondary, etc.).", 0, 1, precedence)); 12153 children.add(new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, 1, insurance)); 12154 children.add(new Property("accident", "", "An accident which resulted in the need for healthcare services.", 0, 1, accident)); 12155 children.add(new Property("employmentImpacted", "Period", "The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).", 0, 1, employmentImpacted)); 12156 children.add(new Property("hospitalization", "Period", "The start and optional end dates of when the patient was confined to a treatment center.", 0, 1, hospitalization)); 12157 children.add(new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item)); 12158 children.add(new Property("addItem", "", "The first tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, addItem)); 12159 children.add(new Property("totalCost", "Money", "The total cost of the services reported.", 0, 1, totalCost)); 12160 children.add(new Property("unallocDeductable", "Money", "The amount of deductable applied which was not allocated to any particular service line.", 0, 1, unallocDeductable)); 12161 children.add(new Property("totalBenefit", "Money", "Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable).", 0, 1, totalBenefit)); 12162 children.add(new Property("payment", "", "Payment details for the claim if the claim has been paid.", 0, 1, payment)); 12163 children.add(new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form)); 12164 children.add(new Property("processNote", "", "Note text.", 0, java.lang.Integer.MAX_VALUE, processNote)); 12165 children.add(new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance)); 12166 } 12167 12168 @Override 12169 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 12170 switch (_hash) { 12171 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The EOB Business Identifier.", 0, java.lang.Integer.MAX_VALUE, identifier); 12172 case -892481550: /*status*/ return new Property("status", "code", "The status of the resource instance.", 0, 1, status); 12173 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The category of claim, eg, oral, pharmacy, vision, insitutional, professional.", 0, 1, type); 12174 case -1868521062: /*subType*/ return new Property("subType", "CodeableConcept", "A finer grained suite of claim subtype codes which may convey Inpatient vs Outpatient and/or a specialty service. In the US the BillType.", 0, java.lang.Integer.MAX_VALUE, subType); 12175 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Patient Resource.", 0, 1, patient); 12176 case -332066046: /*billablePeriod*/ return new Property("billablePeriod", "Period", "The billable period for which charges are being submitted.", 0, 1, billablePeriod); 12177 case 1028554472: /*created*/ return new Property("created", "dateTime", "The date when the EOB was created.", 0, 1, created); 12178 case -1591951995: /*enterer*/ return new Property("enterer", "Reference(Practitioner)", "The person who created the explanation of benefit.", 0, 1, enterer); 12179 case 1957615864: /*insurer*/ return new Property("insurer", "Reference(Organization)", "The insurer which is responsible for the explanation of benefit.", 0, 1, insurer); 12180 case -987494927: /*provider*/ return new Property("provider", "Reference(Practitioner)", "The provider which is responsible for the claim.", 0, 1, provider); 12181 case 1178922291: /*organization*/ return new Property("organization", "Reference(Organization)", "The provider which is responsible for the claim.", 0, 1, organization); 12182 case -722568291: /*referral*/ return new Property("referral", "Reference(ReferralRequest)", "The referral resource which lists the date, practitioner, reason and other supporting information.", 0, 1, referral); 12183 case 501116579: /*facility*/ return new Property("facility", "Reference(Location)", "Facility where the services were provided.", 0, 1, facility); 12184 case 94742588: /*claim*/ return new Property("claim", "Reference(Claim)", "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 0, 1, claim); 12185 case 689513629: /*claimResponse*/ return new Property("claimResponse", "Reference(ClaimResponse)", "The business identifier for the instance: invoice number, claim number, pre-determination or pre-authorization number.", 0, 1, claimResponse); 12186 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Processing outcome errror, partial or complete processing.", 0, 1, outcome); 12187 case 583380919: /*disposition*/ return new Property("disposition", "string", "A description of the status of the adjudication.", 0, 1, disposition); 12188 case 1090493483: /*related*/ return new Property("related", "", "Other claims which are related to this claim such as prior claim versions or for related services.", 0, java.lang.Integer.MAX_VALUE, related); 12189 case 460301338: /*prescription*/ return new Property("prescription", "Reference(MedicationRequest|VisionPrescription)", "Prescription to support the dispensing of Pharmacy or Vision products.", 0, 1, prescription); 12190 case -1814015861: /*originalPrescription*/ return new Property("originalPrescription", "Reference(MedicationRequest)", "Original prescription which has been superceded by this prescription to support the dispensing of pharmacy services, medications or products. For example, a physician may prescribe a medication which the pharmacy determines is contraindicated, or for which the patient has an intolerance, and therefor issues a new precription for an alternate medication which has the same theraputic intent. The prescription from the pharmacy becomes the 'prescription' and that from the physician becomes the 'original prescription'.", 0, 1, originalPrescription); 12191 case 106443592: /*payee*/ return new Property("payee", "", "The party to be reimbursed for the services.", 0, 1, payee); 12192 case 1968600364: /*information*/ return new Property("information", "", "Additional information codes regarding exceptions, special considerations, the condition, situation, prior or concurrent issues. Often there are mutiple jurisdiction specific valuesets which are required.", 0, java.lang.Integer.MAX_VALUE, information); 12193 case -7323378: /*careTeam*/ return new Property("careTeam", "", "The members of the team who provided the overall service as well as their role and whether responsible and qualifications.", 0, java.lang.Integer.MAX_VALUE, careTeam); 12194 case 1196993265: /*diagnosis*/ return new Property("diagnosis", "", "Ordered list of patient diagnosis for which care is sought.", 0, java.lang.Integer.MAX_VALUE, diagnosis); 12195 case -1095204141: /*procedure*/ return new Property("procedure", "", "Ordered list of patient procedures performed to support the adjudication.", 0, java.lang.Integer.MAX_VALUE, procedure); 12196 case 159695370: /*precedence*/ return new Property("precedence", "positiveInt", "Precedence (primary, secondary, etc.).", 0, 1, precedence); 12197 case 73049818: /*insurance*/ return new Property("insurance", "", "Financial instrument by which payment information for health care.", 0, 1, insurance); 12198 case -2143202801: /*accident*/ return new Property("accident", "", "An accident which resulted in the need for healthcare services.", 0, 1, accident); 12199 case 1051487345: /*employmentImpacted*/ return new Property("employmentImpacted", "Period", "The start and optional end dates of when the patient was precluded from working due to the treatable condition(s).", 0, 1, employmentImpacted); 12200 case 1057894634: /*hospitalization*/ return new Property("hospitalization", "Period", "The start and optional end dates of when the patient was confined to a treatment center.", 0, 1, hospitalization); 12201 case 3242771: /*item*/ return new Property("item", "", "First tier of goods and services.", 0, java.lang.Integer.MAX_VALUE, item); 12202 case -1148899500: /*addItem*/ return new Property("addItem", "", "The first tier service adjudications for payor added services.", 0, java.lang.Integer.MAX_VALUE, addItem); 12203 case -577782479: /*totalCost*/ return new Property("totalCost", "Money", "The total cost of the services reported.", 0, 1, totalCost); 12204 case 2096309753: /*unallocDeductable*/ return new Property("unallocDeductable", "Money", "The amount of deductable applied which was not allocated to any particular service line.", 0, 1, unallocDeductable); 12205 case 332332211: /*totalBenefit*/ return new Property("totalBenefit", "Money", "Total amount of benefit payable (Equal to sum of the Benefit amounts from all detail lines and additions less the Unallocated Deductable).", 0, 1, totalBenefit); 12206 case -786681338: /*payment*/ return new Property("payment", "", "Payment details for the claim if the claim has been paid.", 0, 1, payment); 12207 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "The form to be used for printing the content.", 0, 1, form); 12208 case 202339073: /*processNote*/ return new Property("processNote", "", "Note text.", 0, java.lang.Integer.MAX_VALUE, processNote); 12209 case 596003397: /*benefitBalance*/ return new Property("benefitBalance", "", "Balance by Benefit Category.", 0, java.lang.Integer.MAX_VALUE, benefitBalance); 12210 default: return super.getNamedProperty(_hash, _name, _checkValid); 12211 } 12212 12213 } 12214 12215 @Override 12216 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 12217 switch (hash) { 12218 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 12219 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ExplanationOfBenefitStatus> 12220 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 12221 case -1868521062: /*subType*/ return this.subType == null ? new Base[0] : this.subType.toArray(new Base[this.subType.size()]); // CodeableConcept 12222 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 12223 case -332066046: /*billablePeriod*/ return this.billablePeriod == null ? new Base[0] : new Base[] {this.billablePeriod}; // Period 12224 case 1028554472: /*created*/ return this.created == null ? new Base[0] : new Base[] {this.created}; // DateTimeType 12225 case -1591951995: /*enterer*/ return this.enterer == null ? new Base[0] : new Base[] {this.enterer}; // Reference 12226 case 1957615864: /*insurer*/ return this.insurer == null ? new Base[0] : new Base[] {this.insurer}; // Reference 12227 case -987494927: /*provider*/ return this.provider == null ? new Base[0] : new Base[] {this.provider}; // Reference 12228 case 1178922291: /*organization*/ return this.organization == null ? new Base[0] : new Base[] {this.organization}; // Reference 12229 case -722568291: /*referral*/ return this.referral == null ? new Base[0] : new Base[] {this.referral}; // Reference 12230 case 501116579: /*facility*/ return this.facility == null ? new Base[0] : new Base[] {this.facility}; // Reference 12231 case 94742588: /*claim*/ return this.claim == null ? new Base[0] : new Base[] {this.claim}; // Reference 12232 case 689513629: /*claimResponse*/ return this.claimResponse == null ? new Base[0] : new Base[] {this.claimResponse}; // Reference 12233 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 12234 case 583380919: /*disposition*/ return this.disposition == null ? new Base[0] : new Base[] {this.disposition}; // StringType 12235 case 1090493483: /*related*/ return this.related == null ? new Base[0] : this.related.toArray(new Base[this.related.size()]); // RelatedClaimComponent 12236 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 12237 case -1814015861: /*originalPrescription*/ return this.originalPrescription == null ? new Base[0] : new Base[] {this.originalPrescription}; // Reference 12238 case 106443592: /*payee*/ return this.payee == null ? new Base[0] : new Base[] {this.payee}; // PayeeComponent 12239 case 1968600364: /*information*/ return this.information == null ? new Base[0] : this.information.toArray(new Base[this.information.size()]); // SupportingInformationComponent 12240 case -7323378: /*careTeam*/ return this.careTeam == null ? new Base[0] : this.careTeam.toArray(new Base[this.careTeam.size()]); // CareTeamComponent 12241 case 1196993265: /*diagnosis*/ return this.diagnosis == null ? new Base[0] : this.diagnosis.toArray(new Base[this.diagnosis.size()]); // DiagnosisComponent 12242 case -1095204141: /*procedure*/ return this.procedure == null ? new Base[0] : this.procedure.toArray(new Base[this.procedure.size()]); // ProcedureComponent 12243 case 159695370: /*precedence*/ return this.precedence == null ? new Base[0] : new Base[] {this.precedence}; // PositiveIntType 12244 case 73049818: /*insurance*/ return this.insurance == null ? new Base[0] : new Base[] {this.insurance}; // InsuranceComponent 12245 case -2143202801: /*accident*/ return this.accident == null ? new Base[0] : new Base[] {this.accident}; // AccidentComponent 12246 case 1051487345: /*employmentImpacted*/ return this.employmentImpacted == null ? new Base[0] : new Base[] {this.employmentImpacted}; // Period 12247 case 1057894634: /*hospitalization*/ return this.hospitalization == null ? new Base[0] : new Base[] {this.hospitalization}; // Period 12248 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // ItemComponent 12249 case -1148899500: /*addItem*/ return this.addItem == null ? new Base[0] : this.addItem.toArray(new Base[this.addItem.size()]); // AddedItemComponent 12250 case -577782479: /*totalCost*/ return this.totalCost == null ? new Base[0] : new Base[] {this.totalCost}; // Money 12251 case 2096309753: /*unallocDeductable*/ return this.unallocDeductable == null ? new Base[0] : new Base[] {this.unallocDeductable}; // Money 12252 case 332332211: /*totalBenefit*/ return this.totalBenefit == null ? new Base[0] : new Base[] {this.totalBenefit}; // Money 12253 case -786681338: /*payment*/ return this.payment == null ? new Base[0] : new Base[] {this.payment}; // PaymentComponent 12254 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 12255 case 202339073: /*processNote*/ return this.processNote == null ? new Base[0] : this.processNote.toArray(new Base[this.processNote.size()]); // NoteComponent 12256 case 596003397: /*benefitBalance*/ return this.benefitBalance == null ? new Base[0] : this.benefitBalance.toArray(new Base[this.benefitBalance.size()]); // BenefitBalanceComponent 12257 default: return super.getProperty(hash, name, checkValid); 12258 } 12259 12260 } 12261 12262 @Override 12263 public Base setProperty(int hash, String name, Base value) throws FHIRException { 12264 switch (hash) { 12265 case -1618432855: // identifier 12266 this.getIdentifier().add(castToIdentifier(value)); // Identifier 12267 return value; 12268 case -892481550: // status 12269 value = new ExplanationOfBenefitStatusEnumFactory().fromType(castToCode(value)); 12270 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 12271 return value; 12272 case 3575610: // type 12273 this.type = castToCodeableConcept(value); // CodeableConcept 12274 return value; 12275 case -1868521062: // subType 12276 this.getSubType().add(castToCodeableConcept(value)); // CodeableConcept 12277 return value; 12278 case -791418107: // patient 12279 this.patient = castToReference(value); // Reference 12280 return value; 12281 case -332066046: // billablePeriod 12282 this.billablePeriod = castToPeriod(value); // Period 12283 return value; 12284 case 1028554472: // created 12285 this.created = castToDateTime(value); // DateTimeType 12286 return value; 12287 case -1591951995: // enterer 12288 this.enterer = castToReference(value); // Reference 12289 return value; 12290 case 1957615864: // insurer 12291 this.insurer = castToReference(value); // Reference 12292 return value; 12293 case -987494927: // provider 12294 this.provider = castToReference(value); // Reference 12295 return value; 12296 case 1178922291: // organization 12297 this.organization = castToReference(value); // Reference 12298 return value; 12299 case -722568291: // referral 12300 this.referral = castToReference(value); // Reference 12301 return value; 12302 case 501116579: // facility 12303 this.facility = castToReference(value); // Reference 12304 return value; 12305 case 94742588: // claim 12306 this.claim = castToReference(value); // Reference 12307 return value; 12308 case 689513629: // claimResponse 12309 this.claimResponse = castToReference(value); // Reference 12310 return value; 12311 case -1106507950: // outcome 12312 this.outcome = castToCodeableConcept(value); // CodeableConcept 12313 return value; 12314 case 583380919: // disposition 12315 this.disposition = castToString(value); // StringType 12316 return value; 12317 case 1090493483: // related 12318 this.getRelated().add((RelatedClaimComponent) value); // RelatedClaimComponent 12319 return value; 12320 case 460301338: // prescription 12321 this.prescription = castToReference(value); // Reference 12322 return value; 12323 case -1814015861: // originalPrescription 12324 this.originalPrescription = castToReference(value); // Reference 12325 return value; 12326 case 106443592: // payee 12327 this.payee = (PayeeComponent) value; // PayeeComponent 12328 return value; 12329 case 1968600364: // information 12330 this.getInformation().add((SupportingInformationComponent) value); // SupportingInformationComponent 12331 return value; 12332 case -7323378: // careTeam 12333 this.getCareTeam().add((CareTeamComponent) value); // CareTeamComponent 12334 return value; 12335 case 1196993265: // diagnosis 12336 this.getDiagnosis().add((DiagnosisComponent) value); // DiagnosisComponent 12337 return value; 12338 case -1095204141: // procedure 12339 this.getProcedure().add((ProcedureComponent) value); // ProcedureComponent 12340 return value; 12341 case 159695370: // precedence 12342 this.precedence = castToPositiveInt(value); // PositiveIntType 12343 return value; 12344 case 73049818: // insurance 12345 this.insurance = (InsuranceComponent) value; // InsuranceComponent 12346 return value; 12347 case -2143202801: // accident 12348 this.accident = (AccidentComponent) value; // AccidentComponent 12349 return value; 12350 case 1051487345: // employmentImpacted 12351 this.employmentImpacted = castToPeriod(value); // Period 12352 return value; 12353 case 1057894634: // hospitalization 12354 this.hospitalization = castToPeriod(value); // Period 12355 return value; 12356 case 3242771: // item 12357 this.getItem().add((ItemComponent) value); // ItemComponent 12358 return value; 12359 case -1148899500: // addItem 12360 this.getAddItem().add((AddedItemComponent) value); // AddedItemComponent 12361 return value; 12362 case -577782479: // totalCost 12363 this.totalCost = castToMoney(value); // Money 12364 return value; 12365 case 2096309753: // unallocDeductable 12366 this.unallocDeductable = castToMoney(value); // Money 12367 return value; 12368 case 332332211: // totalBenefit 12369 this.totalBenefit = castToMoney(value); // Money 12370 return value; 12371 case -786681338: // payment 12372 this.payment = (PaymentComponent) value; // PaymentComponent 12373 return value; 12374 case 3148996: // form 12375 this.form = castToCodeableConcept(value); // CodeableConcept 12376 return value; 12377 case 202339073: // processNote 12378 this.getProcessNote().add((NoteComponent) value); // NoteComponent 12379 return value; 12380 case 596003397: // benefitBalance 12381 this.getBenefitBalance().add((BenefitBalanceComponent) value); // BenefitBalanceComponent 12382 return value; 12383 default: return super.setProperty(hash, name, value); 12384 } 12385 12386 } 12387 12388 @Override 12389 public Base setProperty(String name, Base value) throws FHIRException { 12390 if (name.equals("identifier")) { 12391 this.getIdentifier().add(castToIdentifier(value)); 12392 } else if (name.equals("status")) { 12393 value = new ExplanationOfBenefitStatusEnumFactory().fromType(castToCode(value)); 12394 this.status = (Enumeration) value; // Enumeration<ExplanationOfBenefitStatus> 12395 } else if (name.equals("type")) { 12396 this.type = castToCodeableConcept(value); // CodeableConcept 12397 } else if (name.equals("subType")) { 12398 this.getSubType().add(castToCodeableConcept(value)); 12399 } else if (name.equals("patient")) { 12400 this.patient = castToReference(value); // Reference 12401 } else if (name.equals("billablePeriod")) { 12402 this.billablePeriod = castToPeriod(value); // Period 12403 } else if (name.equals("created")) { 12404 this.created = castToDateTime(value); // DateTimeType 12405 } else if (name.equals("enterer")) { 12406 this.enterer = castToReference(value); // Reference 12407 } else if (name.equals("insurer")) { 12408 this.insurer = castToReference(value); // Reference 12409 } else if (name.equals("provider")) { 12410 this.provider = castToReference(value); // Reference 12411 } else if (name.equals("organization")) { 12412 this.organization = castToReference(value); // Reference 12413 } else if (name.equals("referral")) { 12414 this.referral = castToReference(value); // Reference 12415 } else if (name.equals("facility")) { 12416 this.facility = castToReference(value); // Reference 12417 } else if (name.equals("claim")) { 12418 this.claim = castToReference(value); // Reference 12419 } else if (name.equals("claimResponse")) { 12420 this.claimResponse = castToReference(value); // Reference 12421 } else if (name.equals("outcome")) { 12422 this.outcome = castToCodeableConcept(value); // CodeableConcept 12423 } else if (name.equals("disposition")) { 12424 this.disposition = castToString(value); // StringType 12425 } else if (name.equals("related")) { 12426 this.getRelated().add((RelatedClaimComponent) value); 12427 } else if (name.equals("prescription")) { 12428 this.prescription = castToReference(value); // Reference 12429 } else if (name.equals("originalPrescription")) { 12430 this.originalPrescription = castToReference(value); // Reference 12431 } else if (name.equals("payee")) { 12432 this.payee = (PayeeComponent) value; // PayeeComponent 12433 } else if (name.equals("information")) { 12434 this.getInformation().add((SupportingInformationComponent) value); 12435 } else if (name.equals("careTeam")) { 12436 this.getCareTeam().add((CareTeamComponent) value); 12437 } else if (name.equals("diagnosis")) { 12438 this.getDiagnosis().add((DiagnosisComponent) value); 12439 } else if (name.equals("procedure")) { 12440 this.getProcedure().add((ProcedureComponent) value); 12441 } else if (name.equals("precedence")) { 12442 this.precedence = castToPositiveInt(value); // PositiveIntType 12443 } else if (name.equals("insurance")) { 12444 this.insurance = (InsuranceComponent) value; // InsuranceComponent 12445 } else if (name.equals("accident")) { 12446 this.accident = (AccidentComponent) value; // AccidentComponent 12447 } else if (name.equals("employmentImpacted")) { 12448 this.employmentImpacted = castToPeriod(value); // Period 12449 } else if (name.equals("hospitalization")) { 12450 this.hospitalization = castToPeriod(value); // Period 12451 } else if (name.equals("item")) { 12452 this.getItem().add((ItemComponent) value); 12453 } else if (name.equals("addItem")) { 12454 this.getAddItem().add((AddedItemComponent) value); 12455 } else if (name.equals("totalCost")) { 12456 this.totalCost = castToMoney(value); // Money 12457 } else if (name.equals("unallocDeductable")) { 12458 this.unallocDeductable = castToMoney(value); // Money 12459 } else if (name.equals("totalBenefit")) { 12460 this.totalBenefit = castToMoney(value); // Money 12461 } else if (name.equals("payment")) { 12462 this.payment = (PaymentComponent) value; // PaymentComponent 12463 } else if (name.equals("form")) { 12464 this.form = castToCodeableConcept(value); // CodeableConcept 12465 } else if (name.equals("processNote")) { 12466 this.getProcessNote().add((NoteComponent) value); 12467 } else if (name.equals("benefitBalance")) { 12468 this.getBenefitBalance().add((BenefitBalanceComponent) value); 12469 } else 12470 return super.setProperty(name, value); 12471 return value; 12472 } 12473 12474 @Override 12475 public Base makeProperty(int hash, String name) throws FHIRException { 12476 switch (hash) { 12477 case -1618432855: return addIdentifier(); 12478 case -892481550: return getStatusElement(); 12479 case 3575610: return getType(); 12480 case -1868521062: return addSubType(); 12481 case -791418107: return getPatient(); 12482 case -332066046: return getBillablePeriod(); 12483 case 1028554472: return getCreatedElement(); 12484 case -1591951995: return getEnterer(); 12485 case 1957615864: return getInsurer(); 12486 case -987494927: return getProvider(); 12487 case 1178922291: return getOrganization(); 12488 case -722568291: return getReferral(); 12489 case 501116579: return getFacility(); 12490 case 94742588: return getClaim(); 12491 case 689513629: return getClaimResponse(); 12492 case -1106507950: return getOutcome(); 12493 case 583380919: return getDispositionElement(); 12494 case 1090493483: return addRelated(); 12495 case 460301338: return getPrescription(); 12496 case -1814015861: return getOriginalPrescription(); 12497 case 106443592: return getPayee(); 12498 case 1968600364: return addInformation(); 12499 case -7323378: return addCareTeam(); 12500 case 1196993265: return addDiagnosis(); 12501 case -1095204141: return addProcedure(); 12502 case 159695370: return getPrecedenceElement(); 12503 case 73049818: return getInsurance(); 12504 case -2143202801: return getAccident(); 12505 case 1051487345: return getEmploymentImpacted(); 12506 case 1057894634: return getHospitalization(); 12507 case 3242771: return addItem(); 12508 case -1148899500: return addAddItem(); 12509 case -577782479: return getTotalCost(); 12510 case 2096309753: return getUnallocDeductable(); 12511 case 332332211: return getTotalBenefit(); 12512 case -786681338: return getPayment(); 12513 case 3148996: return getForm(); 12514 case 202339073: return addProcessNote(); 12515 case 596003397: return addBenefitBalance(); 12516 default: return super.makeProperty(hash, name); 12517 } 12518 12519 } 12520 12521 @Override 12522 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 12523 switch (hash) { 12524 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 12525 case -892481550: /*status*/ return new String[] {"code"}; 12526 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 12527 case -1868521062: /*subType*/ return new String[] {"CodeableConcept"}; 12528 case -791418107: /*patient*/ return new String[] {"Reference"}; 12529 case -332066046: /*billablePeriod*/ return new String[] {"Period"}; 12530 case 1028554472: /*created*/ return new String[] {"dateTime"}; 12531 case -1591951995: /*enterer*/ return new String[] {"Reference"}; 12532 case 1957615864: /*insurer*/ return new String[] {"Reference"}; 12533 case -987494927: /*provider*/ return new String[] {"Reference"}; 12534 case 1178922291: /*organization*/ return new String[] {"Reference"}; 12535 case -722568291: /*referral*/ return new String[] {"Reference"}; 12536 case 501116579: /*facility*/ return new String[] {"Reference"}; 12537 case 94742588: /*claim*/ return new String[] {"Reference"}; 12538 case 689513629: /*claimResponse*/ return new String[] {"Reference"}; 12539 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 12540 case 583380919: /*disposition*/ return new String[] {"string"}; 12541 case 1090493483: /*related*/ return new String[] {}; 12542 case 460301338: /*prescription*/ return new String[] {"Reference"}; 12543 case -1814015861: /*originalPrescription*/ return new String[] {"Reference"}; 12544 case 106443592: /*payee*/ return new String[] {}; 12545 case 1968600364: /*information*/ return new String[] {}; 12546 case -7323378: /*careTeam*/ return new String[] {}; 12547 case 1196993265: /*diagnosis*/ return new String[] {}; 12548 case -1095204141: /*procedure*/ return new String[] {}; 12549 case 159695370: /*precedence*/ return new String[] {"positiveInt"}; 12550 case 73049818: /*insurance*/ return new String[] {}; 12551 case -2143202801: /*accident*/ return new String[] {}; 12552 case 1051487345: /*employmentImpacted*/ return new String[] {"Period"}; 12553 case 1057894634: /*hospitalization*/ return new String[] {"Period"}; 12554 case 3242771: /*item*/ return new String[] {}; 12555 case -1148899500: /*addItem*/ return new String[] {}; 12556 case -577782479: /*totalCost*/ return new String[] {"Money"}; 12557 case 2096309753: /*unallocDeductable*/ return new String[] {"Money"}; 12558 case 332332211: /*totalBenefit*/ return new String[] {"Money"}; 12559 case -786681338: /*payment*/ return new String[] {}; 12560 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 12561 case 202339073: /*processNote*/ return new String[] {}; 12562 case 596003397: /*benefitBalance*/ return new String[] {}; 12563 default: return super.getTypesForProperty(hash, name); 12564 } 12565 12566 } 12567 12568 @Override 12569 public Base addChild(String name) throws FHIRException { 12570 if (name.equals("identifier")) { 12571 return addIdentifier(); 12572 } 12573 else if (name.equals("status")) { 12574 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.status"); 12575 } 12576 else if (name.equals("type")) { 12577 this.type = new CodeableConcept(); 12578 return this.type; 12579 } 12580 else if (name.equals("subType")) { 12581 return addSubType(); 12582 } 12583 else if (name.equals("patient")) { 12584 this.patient = new Reference(); 12585 return this.patient; 12586 } 12587 else if (name.equals("billablePeriod")) { 12588 this.billablePeriod = new Period(); 12589 return this.billablePeriod; 12590 } 12591 else if (name.equals("created")) { 12592 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.created"); 12593 } 12594 else if (name.equals("enterer")) { 12595 this.enterer = new Reference(); 12596 return this.enterer; 12597 } 12598 else if (name.equals("insurer")) { 12599 this.insurer = new Reference(); 12600 return this.insurer; 12601 } 12602 else if (name.equals("provider")) { 12603 this.provider = new Reference(); 12604 return this.provider; 12605 } 12606 else if (name.equals("organization")) { 12607 this.organization = new Reference(); 12608 return this.organization; 12609 } 12610 else if (name.equals("referral")) { 12611 this.referral = new Reference(); 12612 return this.referral; 12613 } 12614 else if (name.equals("facility")) { 12615 this.facility = new Reference(); 12616 return this.facility; 12617 } 12618 else if (name.equals("claim")) { 12619 this.claim = new Reference(); 12620 return this.claim; 12621 } 12622 else if (name.equals("claimResponse")) { 12623 this.claimResponse = new Reference(); 12624 return this.claimResponse; 12625 } 12626 else if (name.equals("outcome")) { 12627 this.outcome = new CodeableConcept(); 12628 return this.outcome; 12629 } 12630 else if (name.equals("disposition")) { 12631 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.disposition"); 12632 } 12633 else if (name.equals("related")) { 12634 return addRelated(); 12635 } 12636 else if (name.equals("prescription")) { 12637 this.prescription = new Reference(); 12638 return this.prescription; 12639 } 12640 else if (name.equals("originalPrescription")) { 12641 this.originalPrescription = new Reference(); 12642 return this.originalPrescription; 12643 } 12644 else if (name.equals("payee")) { 12645 this.payee = new PayeeComponent(); 12646 return this.payee; 12647 } 12648 else if (name.equals("information")) { 12649 return addInformation(); 12650 } 12651 else if (name.equals("careTeam")) { 12652 return addCareTeam(); 12653 } 12654 else if (name.equals("diagnosis")) { 12655 return addDiagnosis(); 12656 } 12657 else if (name.equals("procedure")) { 12658 return addProcedure(); 12659 } 12660 else if (name.equals("precedence")) { 12661 throw new FHIRException("Cannot call addChild on a singleton property ExplanationOfBenefit.precedence"); 12662 } 12663 else if (name.equals("insurance")) { 12664 this.insurance = new InsuranceComponent(); 12665 return this.insurance; 12666 } 12667 else if (name.equals("accident")) { 12668 this.accident = new AccidentComponent(); 12669 return this.accident; 12670 } 12671 else if (name.equals("employmentImpacted")) { 12672 this.employmentImpacted = new Period(); 12673 return this.employmentImpacted; 12674 } 12675 else if (name.equals("hospitalization")) { 12676 this.hospitalization = new Period(); 12677 return this.hospitalization; 12678 } 12679 else if (name.equals("item")) { 12680 return addItem(); 12681 } 12682 else if (name.equals("addItem")) { 12683 return addAddItem(); 12684 } 12685 else if (name.equals("totalCost")) { 12686 this.totalCost = new Money(); 12687 return this.totalCost; 12688 } 12689 else if (name.equals("unallocDeductable")) { 12690 this.unallocDeductable = new Money(); 12691 return this.unallocDeductable; 12692 } 12693 else if (name.equals("totalBenefit")) { 12694 this.totalBenefit = new Money(); 12695 return this.totalBenefit; 12696 } 12697 else if (name.equals("payment")) { 12698 this.payment = new PaymentComponent(); 12699 return this.payment; 12700 } 12701 else if (name.equals("form")) { 12702 this.form = new CodeableConcept(); 12703 return this.form; 12704 } 12705 else if (name.equals("processNote")) { 12706 return addProcessNote(); 12707 } 12708 else if (name.equals("benefitBalance")) { 12709 return addBenefitBalance(); 12710 } 12711 else 12712 return super.addChild(name); 12713 } 12714 12715 public String fhirType() { 12716 return "ExplanationOfBenefit"; 12717 12718 } 12719 12720 public ExplanationOfBenefit copy() { 12721 ExplanationOfBenefit dst = new ExplanationOfBenefit(); 12722 copyValues(dst); 12723 if (identifier != null) { 12724 dst.identifier = new ArrayList<Identifier>(); 12725 for (Identifier i : identifier) 12726 dst.identifier.add(i.copy()); 12727 }; 12728 dst.status = status == null ? null : status.copy(); 12729 dst.type = type == null ? null : type.copy(); 12730 if (subType != null) { 12731 dst.subType = new ArrayList<CodeableConcept>(); 12732 for (CodeableConcept i : subType) 12733 dst.subType.add(i.copy()); 12734 }; 12735 dst.patient = patient == null ? null : patient.copy(); 12736 dst.billablePeriod = billablePeriod == null ? null : billablePeriod.copy(); 12737 dst.created = created == null ? null : created.copy(); 12738 dst.enterer = enterer == null ? null : enterer.copy(); 12739 dst.insurer = insurer == null ? null : insurer.copy(); 12740 dst.provider = provider == null ? null : provider.copy(); 12741 dst.organization = organization == null ? null : organization.copy(); 12742 dst.referral = referral == null ? null : referral.copy(); 12743 dst.facility = facility == null ? null : facility.copy(); 12744 dst.claim = claim == null ? null : claim.copy(); 12745 dst.claimResponse = claimResponse == null ? null : claimResponse.copy(); 12746 dst.outcome = outcome == null ? null : outcome.copy(); 12747 dst.disposition = disposition == null ? null : disposition.copy(); 12748 if (related != null) { 12749 dst.related = new ArrayList<RelatedClaimComponent>(); 12750 for (RelatedClaimComponent i : related) 12751 dst.related.add(i.copy()); 12752 }; 12753 dst.prescription = prescription == null ? null : prescription.copy(); 12754 dst.originalPrescription = originalPrescription == null ? null : originalPrescription.copy(); 12755 dst.payee = payee == null ? null : payee.copy(); 12756 if (information != null) { 12757 dst.information = new ArrayList<SupportingInformationComponent>(); 12758 for (SupportingInformationComponent i : information) 12759 dst.information.add(i.copy()); 12760 }; 12761 if (careTeam != null) { 12762 dst.careTeam = new ArrayList<CareTeamComponent>(); 12763 for (CareTeamComponent i : careTeam) 12764 dst.careTeam.add(i.copy()); 12765 }; 12766 if (diagnosis != null) { 12767 dst.diagnosis = new ArrayList<DiagnosisComponent>(); 12768 for (DiagnosisComponent i : diagnosis) 12769 dst.diagnosis.add(i.copy()); 12770 }; 12771 if (procedure != null) { 12772 dst.procedure = new ArrayList<ProcedureComponent>(); 12773 for (ProcedureComponent i : procedure) 12774 dst.procedure.add(i.copy()); 12775 }; 12776 dst.precedence = precedence == null ? null : precedence.copy(); 12777 dst.insurance = insurance == null ? null : insurance.copy(); 12778 dst.accident = accident == null ? null : accident.copy(); 12779 dst.employmentImpacted = employmentImpacted == null ? null : employmentImpacted.copy(); 12780 dst.hospitalization = hospitalization == null ? null : hospitalization.copy(); 12781 if (item != null) { 12782 dst.item = new ArrayList<ItemComponent>(); 12783 for (ItemComponent i : item) 12784 dst.item.add(i.copy()); 12785 }; 12786 if (addItem != null) { 12787 dst.addItem = new ArrayList<AddedItemComponent>(); 12788 for (AddedItemComponent i : addItem) 12789 dst.addItem.add(i.copy()); 12790 }; 12791 dst.totalCost = totalCost == null ? null : totalCost.copy(); 12792 dst.unallocDeductable = unallocDeductable == null ? null : unallocDeductable.copy(); 12793 dst.totalBenefit = totalBenefit == null ? null : totalBenefit.copy(); 12794 dst.payment = payment == null ? null : payment.copy(); 12795 dst.form = form == null ? null : form.copy(); 12796 if (processNote != null) { 12797 dst.processNote = new ArrayList<NoteComponent>(); 12798 for (NoteComponent i : processNote) 12799 dst.processNote.add(i.copy()); 12800 }; 12801 if (benefitBalance != null) { 12802 dst.benefitBalance = new ArrayList<BenefitBalanceComponent>(); 12803 for (BenefitBalanceComponent i : benefitBalance) 12804 dst.benefitBalance.add(i.copy()); 12805 }; 12806 return dst; 12807 } 12808 12809 protected ExplanationOfBenefit typedCopy() { 12810 return copy(); 12811 } 12812 12813 @Override 12814 public boolean equalsDeep(Base other_) { 12815 if (!super.equalsDeep(other_)) 12816 return false; 12817 if (!(other_ instanceof ExplanationOfBenefit)) 12818 return false; 12819 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 12820 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 12821 && compareDeep(subType, o.subType, true) && compareDeep(patient, o.patient, true) && compareDeep(billablePeriod, o.billablePeriod, true) 12822 && compareDeep(created, o.created, true) && compareDeep(enterer, o.enterer, true) && compareDeep(insurer, o.insurer, true) 12823 && compareDeep(provider, o.provider, true) && compareDeep(organization, o.organization, true) && compareDeep(referral, o.referral, true) 12824 && compareDeep(facility, o.facility, true) && compareDeep(claim, o.claim, true) && compareDeep(claimResponse, o.claimResponse, true) 12825 && compareDeep(outcome, o.outcome, true) && compareDeep(disposition, o.disposition, true) && compareDeep(related, o.related, true) 12826 && compareDeep(prescription, o.prescription, true) && compareDeep(originalPrescription, o.originalPrescription, true) 12827 && compareDeep(payee, o.payee, true) && compareDeep(information, o.information, true) && compareDeep(careTeam, o.careTeam, true) 12828 && compareDeep(diagnosis, o.diagnosis, true) && compareDeep(procedure, o.procedure, true) && compareDeep(precedence, o.precedence, true) 12829 && compareDeep(insurance, o.insurance, true) && compareDeep(accident, o.accident, true) && compareDeep(employmentImpacted, o.employmentImpacted, true) 12830 && compareDeep(hospitalization, o.hospitalization, true) && compareDeep(item, o.item, true) && compareDeep(addItem, o.addItem, true) 12831 && compareDeep(totalCost, o.totalCost, true) && compareDeep(unallocDeductable, o.unallocDeductable, true) 12832 && compareDeep(totalBenefit, o.totalBenefit, true) && compareDeep(payment, o.payment, true) && compareDeep(form, o.form, true) 12833 && compareDeep(processNote, o.processNote, true) && compareDeep(benefitBalance, o.benefitBalance, true) 12834 ; 12835 } 12836 12837 @Override 12838 public boolean equalsShallow(Base other_) { 12839 if (!super.equalsShallow(other_)) 12840 return false; 12841 if (!(other_ instanceof ExplanationOfBenefit)) 12842 return false; 12843 ExplanationOfBenefit o = (ExplanationOfBenefit) other_; 12844 return compareValues(status, o.status, true) && compareValues(created, o.created, true) && compareValues(disposition, o.disposition, true) 12845 && compareValues(precedence, o.precedence, true); 12846 } 12847 12848 public boolean isEmpty() { 12849 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 12850 , subType, patient, billablePeriod, created, enterer, insurer, provider, organization 12851 , referral, facility, claim, claimResponse, outcome, disposition, related, prescription 12852 , originalPrescription, payee, information, careTeam, diagnosis, procedure, precedence 12853 , insurance, accident, employmentImpacted, hospitalization, item, addItem, totalCost 12854 , unallocDeductable, totalBenefit, payment, form, processNote, benefitBalance); 12855 } 12856 12857 @Override 12858 public ResourceType getResourceType() { 12859 return ResourceType.ExplanationOfBenefit; 12860 } 12861 12862 /** 12863 * Search parameter: <b>coverage</b> 12864 * <p> 12865 * Description: <b>The plan under which the claim was adjudicated</b><br> 12866 * Type: <b>reference</b><br> 12867 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 12868 * </p> 12869 */ 12870 @SearchParamDefinition(name="coverage", path="ExplanationOfBenefit.insurance.coverage", description="The plan under which the claim was adjudicated", type="reference", target={Coverage.class } ) 12871 public static final String SP_COVERAGE = "coverage"; 12872 /** 12873 * <b>Fluent Client</b> search parameter constant for <b>coverage</b> 12874 * <p> 12875 * Description: <b>The plan under which the claim was adjudicated</b><br> 12876 * Type: <b>reference</b><br> 12877 * Path: <b>ExplanationOfBenefit.insurance.coverage</b><br> 12878 * </p> 12879 */ 12880 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COVERAGE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COVERAGE); 12881 12882/** 12883 * Constant for fluent queries to be used to add include statements. Specifies 12884 * the path value of "<b>ExplanationOfBenefit:coverage</b>". 12885 */ 12886 public static final ca.uhn.fhir.model.api.Include INCLUDE_COVERAGE = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:coverage").toLocked(); 12887 12888 /** 12889 * Search parameter: <b>care-team</b> 12890 * <p> 12891 * Description: <b>Member of the CareTeam</b><br> 12892 * Type: <b>reference</b><br> 12893 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 12894 * </p> 12895 */ 12896 @SearchParamDefinition(name="care-team", path="ExplanationOfBenefit.careTeam.provider", description="Member of the CareTeam", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 12897 public static final String SP_CARE_TEAM = "care-team"; 12898 /** 12899 * <b>Fluent Client</b> search parameter constant for <b>care-team</b> 12900 * <p> 12901 * Description: <b>Member of the CareTeam</b><br> 12902 * Type: <b>reference</b><br> 12903 * Path: <b>ExplanationOfBenefit.careTeam.provider</b><br> 12904 * </p> 12905 */ 12906 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CARE_TEAM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CARE_TEAM); 12907 12908/** 12909 * Constant for fluent queries to be used to add include statements. Specifies 12910 * the path value of "<b>ExplanationOfBenefit:care-team</b>". 12911 */ 12912 public static final ca.uhn.fhir.model.api.Include INCLUDE_CARE_TEAM = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:care-team").toLocked(); 12913 12914 /** 12915 * Search parameter: <b>identifier</b> 12916 * <p> 12917 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 12918 * Type: <b>token</b><br> 12919 * Path: <b>ExplanationOfBenefit.identifier</b><br> 12920 * </p> 12921 */ 12922 @SearchParamDefinition(name="identifier", path="ExplanationOfBenefit.identifier", description="The business identifier of the Explanation of Benefit", type="token" ) 12923 public static final String SP_IDENTIFIER = "identifier"; 12924 /** 12925 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 12926 * <p> 12927 * Description: <b>The business identifier of the Explanation of Benefit</b><br> 12928 * Type: <b>token</b><br> 12929 * Path: <b>ExplanationOfBenefit.identifier</b><br> 12930 * </p> 12931 */ 12932 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 12933 12934 /** 12935 * Search parameter: <b>created</b> 12936 * <p> 12937 * Description: <b>The creation date for the EOB</b><br> 12938 * Type: <b>date</b><br> 12939 * Path: <b>ExplanationOfBenefit.created</b><br> 12940 * </p> 12941 */ 12942 @SearchParamDefinition(name="created", path="ExplanationOfBenefit.created", description="The creation date for the EOB", type="date" ) 12943 public static final String SP_CREATED = "created"; 12944 /** 12945 * <b>Fluent Client</b> search parameter constant for <b>created</b> 12946 * <p> 12947 * Description: <b>The creation date for the EOB</b><br> 12948 * Type: <b>date</b><br> 12949 * Path: <b>ExplanationOfBenefit.created</b><br> 12950 * </p> 12951 */ 12952 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 12953 12954 /** 12955 * Search parameter: <b>encounter</b> 12956 * <p> 12957 * Description: <b>Encounters associated with a billed line item</b><br> 12958 * Type: <b>reference</b><br> 12959 * Path: <b>ExplanationOfBenefit.item.encounter</b><br> 12960 * </p> 12961 */ 12962 @SearchParamDefinition(name="encounter", path="ExplanationOfBenefit.item.encounter", description="Encounters associated with a billed line item", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class } ) 12963 public static final String SP_ENCOUNTER = "encounter"; 12964 /** 12965 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 12966 * <p> 12967 * Description: <b>Encounters associated with a billed line item</b><br> 12968 * Type: <b>reference</b><br> 12969 * Path: <b>ExplanationOfBenefit.item.encounter</b><br> 12970 * </p> 12971 */ 12972 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 12973 12974/** 12975 * Constant for fluent queries to be used to add include statements. Specifies 12976 * the path value of "<b>ExplanationOfBenefit:encounter</b>". 12977 */ 12978 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:encounter").toLocked(); 12979 12980 /** 12981 * Search parameter: <b>payee</b> 12982 * <p> 12983 * Description: <b>The party receiving any payment for the Claim</b><br> 12984 * Type: <b>reference</b><br> 12985 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 12986 * </p> 12987 */ 12988 @SearchParamDefinition(name="payee", path="ExplanationOfBenefit.payee.party", description="The party receiving any payment for the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 12989 public static final String SP_PAYEE = "payee"; 12990 /** 12991 * <b>Fluent Client</b> search parameter constant for <b>payee</b> 12992 * <p> 12993 * Description: <b>The party receiving any payment for the Claim</b><br> 12994 * Type: <b>reference</b><br> 12995 * Path: <b>ExplanationOfBenefit.payee.party</b><br> 12996 * </p> 12997 */ 12998 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PAYEE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PAYEE); 12999 13000/** 13001 * Constant for fluent queries to be used to add include statements. Specifies 13002 * the path value of "<b>ExplanationOfBenefit:payee</b>". 13003 */ 13004 public static final ca.uhn.fhir.model.api.Include INCLUDE_PAYEE = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:payee").toLocked(); 13005 13006 /** 13007 * Search parameter: <b>disposition</b> 13008 * <p> 13009 * Description: <b>The contents of the disposition message</b><br> 13010 * Type: <b>string</b><br> 13011 * Path: <b>ExplanationOfBenefit.disposition</b><br> 13012 * </p> 13013 */ 13014 @SearchParamDefinition(name="disposition", path="ExplanationOfBenefit.disposition", description="The contents of the disposition message", type="string" ) 13015 public static final String SP_DISPOSITION = "disposition"; 13016 /** 13017 * <b>Fluent Client</b> search parameter constant for <b>disposition</b> 13018 * <p> 13019 * Description: <b>The contents of the disposition message</b><br> 13020 * Type: <b>string</b><br> 13021 * Path: <b>ExplanationOfBenefit.disposition</b><br> 13022 * </p> 13023 */ 13024 public static final ca.uhn.fhir.rest.gclient.StringClientParam DISPOSITION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DISPOSITION); 13025 13026 /** 13027 * Search parameter: <b>provider</b> 13028 * <p> 13029 * Description: <b>The reference to the provider</b><br> 13030 * Type: <b>reference</b><br> 13031 * Path: <b>ExplanationOfBenefit.provider</b><br> 13032 * </p> 13033 */ 13034 @SearchParamDefinition(name="provider", path="ExplanationOfBenefit.provider", description="The reference to the provider", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 13035 public static final String SP_PROVIDER = "provider"; 13036 /** 13037 * <b>Fluent Client</b> search parameter constant for <b>provider</b> 13038 * <p> 13039 * Description: <b>The reference to the provider</b><br> 13040 * Type: <b>reference</b><br> 13041 * Path: <b>ExplanationOfBenefit.provider</b><br> 13042 * </p> 13043 */ 13044 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PROVIDER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PROVIDER); 13045 13046/** 13047 * Constant for fluent queries to be used to add include statements. Specifies 13048 * the path value of "<b>ExplanationOfBenefit:provider</b>". 13049 */ 13050 public static final ca.uhn.fhir.model.api.Include INCLUDE_PROVIDER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:provider").toLocked(); 13051 13052 /** 13053 * Search parameter: <b>patient</b> 13054 * <p> 13055 * Description: <b>The reference to the patient</b><br> 13056 * Type: <b>reference</b><br> 13057 * Path: <b>ExplanationOfBenefit.patient</b><br> 13058 * </p> 13059 */ 13060 @SearchParamDefinition(name="patient", path="ExplanationOfBenefit.patient", description="The reference to the patient", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 13061 public static final String SP_PATIENT = "patient"; 13062 /** 13063 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 13064 * <p> 13065 * Description: <b>The reference to the patient</b><br> 13066 * Type: <b>reference</b><br> 13067 * Path: <b>ExplanationOfBenefit.patient</b><br> 13068 * </p> 13069 */ 13070 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 13071 13072/** 13073 * Constant for fluent queries to be used to add include statements. Specifies 13074 * the path value of "<b>ExplanationOfBenefit:patient</b>". 13075 */ 13076 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:patient").toLocked(); 13077 13078 /** 13079 * Search parameter: <b>organization</b> 13080 * <p> 13081 * Description: <b>The reference to the providing organization</b><br> 13082 * Type: <b>reference</b><br> 13083 * Path: <b>ExplanationOfBenefit.organization</b><br> 13084 * </p> 13085 */ 13086 @SearchParamDefinition(name="organization", path="ExplanationOfBenefit.organization", description="The reference to the providing organization", type="reference", target={Organization.class } ) 13087 public static final String SP_ORGANIZATION = "organization"; 13088 /** 13089 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 13090 * <p> 13091 * Description: <b>The reference to the providing organization</b><br> 13092 * Type: <b>reference</b><br> 13093 * Path: <b>ExplanationOfBenefit.organization</b><br> 13094 * </p> 13095 */ 13096 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 13097 13098/** 13099 * Constant for fluent queries to be used to add include statements. Specifies 13100 * the path value of "<b>ExplanationOfBenefit:organization</b>". 13101 */ 13102 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:organization").toLocked(); 13103 13104 /** 13105 * Search parameter: <b>claim</b> 13106 * <p> 13107 * Description: <b>The reference to the claim</b><br> 13108 * Type: <b>reference</b><br> 13109 * Path: <b>ExplanationOfBenefit.claim</b><br> 13110 * </p> 13111 */ 13112 @SearchParamDefinition(name="claim", path="ExplanationOfBenefit.claim", description="The reference to the claim", type="reference", target={Claim.class } ) 13113 public static final String SP_CLAIM = "claim"; 13114 /** 13115 * <b>Fluent Client</b> search parameter constant for <b>claim</b> 13116 * <p> 13117 * Description: <b>The reference to the claim</b><br> 13118 * Type: <b>reference</b><br> 13119 * Path: <b>ExplanationOfBenefit.claim</b><br> 13120 * </p> 13121 */ 13122 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CLAIM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CLAIM); 13123 13124/** 13125 * Constant for fluent queries to be used to add include statements. Specifies 13126 * the path value of "<b>ExplanationOfBenefit:claim</b>". 13127 */ 13128 public static final ca.uhn.fhir.model.api.Include INCLUDE_CLAIM = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:claim").toLocked(); 13129 13130 /** 13131 * Search parameter: <b>enterer</b> 13132 * <p> 13133 * Description: <b>The party responsible for the entry of the Claim</b><br> 13134 * Type: <b>reference</b><br> 13135 * Path: <b>ExplanationOfBenefit.enterer</b><br> 13136 * </p> 13137 */ 13138 @SearchParamDefinition(name="enterer", path="ExplanationOfBenefit.enterer", description="The party responsible for the entry of the Claim", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 13139 public static final String SP_ENTERER = "enterer"; 13140 /** 13141 * <b>Fluent Client</b> search parameter constant for <b>enterer</b> 13142 * <p> 13143 * Description: <b>The party responsible for the entry of the Claim</b><br> 13144 * Type: <b>reference</b><br> 13145 * Path: <b>ExplanationOfBenefit.enterer</b><br> 13146 * </p> 13147 */ 13148 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENTERER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENTERER); 13149 13150/** 13151 * Constant for fluent queries to be used to add include statements. Specifies 13152 * the path value of "<b>ExplanationOfBenefit:enterer</b>". 13153 */ 13154 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENTERER = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:enterer").toLocked(); 13155 13156 /** 13157 * Search parameter: <b>facility</b> 13158 * <p> 13159 * Description: <b>Facility responsible for the goods and services</b><br> 13160 * Type: <b>reference</b><br> 13161 * Path: <b>ExplanationOfBenefit.facility</b><br> 13162 * </p> 13163 */ 13164 @SearchParamDefinition(name="facility", path="ExplanationOfBenefit.facility", description="Facility responsible for the goods and services", type="reference", target={Location.class } ) 13165 public static final String SP_FACILITY = "facility"; 13166 /** 13167 * <b>Fluent Client</b> search parameter constant for <b>facility</b> 13168 * <p> 13169 * Description: <b>Facility responsible for the goods and services</b><br> 13170 * Type: <b>reference</b><br> 13171 * Path: <b>ExplanationOfBenefit.facility</b><br> 13172 * </p> 13173 */ 13174 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam FACILITY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_FACILITY); 13175 13176/** 13177 * Constant for fluent queries to be used to add include statements. Specifies 13178 * the path value of "<b>ExplanationOfBenefit:facility</b>". 13179 */ 13180 public static final ca.uhn.fhir.model.api.Include INCLUDE_FACILITY = new ca.uhn.fhir.model.api.Include("ExplanationOfBenefit:facility").toLocked(); 13181 13182 13183}