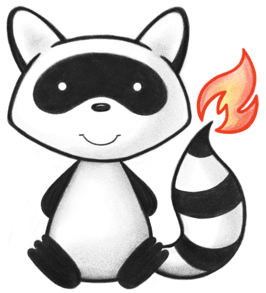
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.IBaseDatatype; 039import org.hl7.fhir.instance.model.api.IBaseExtension; 040import org.hl7.fhir.instance.model.api.IBaseHasExtensions; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.DatatypeDef; 044import ca.uhn.fhir.model.api.annotation.Description; 045/** 046 * Optional Extension Element - found in all resources. 047 */ 048@DatatypeDef(name="Extension") 049public class Extension extends BaseExtension implements IBaseExtension<Extension, Type>, IBaseHasExtensions { 050 051 /** 052 * Source of the definition for the extension code - a logical name or a URL. 053 */ 054 @Child(name = "url", type = {UriType.class}, order=0, min=1, max=1, modifier=false, summary=false) 055 @Description(shortDefinition="identifies the meaning of the extension", formalDefinition="Source of the definition for the extension code - a logical name or a URL." ) 056 protected UriType url; 057 058 /** 059 * Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list). 060 */ 061 @Child(name = "value", type = {}, order=1, min=0, max=1, modifier=false, summary=false) 062 @Description(shortDefinition="Value of extension", formalDefinition="Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list)." ) 063 protected org.hl7.fhir.dstu3.model.Type value; 064 065 private static final long serialVersionUID = 1240831878L; 066 067 /** 068 * Constructor 069 */ 070 public Extension() { 071 super(); 072 } 073 074 /** 075 * Constructor 076 */ 077 public Extension(UriType url) { 078 super(); 079 this.url = url; 080 } 081 082 /** 083 * Constructor 084 */ 085 public Extension(String theUrl) { 086 setUrl(theUrl); 087 } 088 089 /** 090 * Constructor 091 */ 092 public Extension(String theUrl, IBaseDatatype theValue) { 093 setUrl(theUrl); 094 setValue(theValue); 095 } 096 097 /** 098 * @return {@link #url} (Source of the definition for the extension code - a logical name or a URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 099 */ 100 public UriType getUrlElement() { 101 if (this.url == null) 102 if (Configuration.errorOnAutoCreate()) 103 throw new Error("Attempt to auto-create Extension.url"); 104 else if (Configuration.doAutoCreate()) 105 this.url = new UriType(); // bb 106 return this.url; 107 } 108 109 public boolean hasUrlElement() { 110 return this.url != null && !this.url.isEmpty(); 111 } 112 113 public boolean hasUrl() { 114 return this.url != null && !this.url.isEmpty(); 115 } 116 117 /** 118 * @param value {@link #url} (Source of the definition for the extension code - a logical name or a URL.). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 119 */ 120 public Extension setUrlElement(UriType value) { 121 this.url = value; 122 return this; 123 } 124 125 /** 126 * @return Source of the definition for the extension code - a logical name or a URL. 127 */ 128 public String getUrl() { 129 return this.url == null ? null : this.url.getValue(); 130 } 131 132 /** 133 * @param value Source of the definition for the extension code - a logical name or a URL. 134 */ 135 public Extension setUrl(String value) { 136 if (this.url == null) 137 this.url = new UriType(); 138 this.url.setValue(value); 139 return this; 140 } 141 142 /** 143 * @return {@link #value} (Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).) 144 */ 145 public org.hl7.fhir.dstu3.model.Type getValue() { 146 return this.value; 147 } 148 149 public boolean hasValue() { 150 return this.value != null && !this.value.isEmpty(); 151 } 152 153 /** 154 * @param value {@link #value} (Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).) 155 */ 156 public Extension setValue(org.hl7.fhir.dstu3.model.Type value) { 157 this.value = value; 158 return this; 159 } 160 161 protected void listChildren(List<Property> children) { 162 super.listChildren(children); 163 children.add(new Property("url", "uri", "Source of the definition for the extension code - a logical name or a URL.", 0, 1, url)); 164 children.add(new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value)); 165 } 166 167 @Override 168 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 169 switch (_hash) { 170 case 116079: /*url*/ return new Property("url", "uri", "Source of the definition for the extension code - a logical name or a URL.", 0, 1, url); 171 case -1410166417: /*value[x]*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 172 case 111972721: /*value*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 173 case -1535024575: /*valueBase64Binary*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 174 case 733421943: /*valueBoolean*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 175 case -786218365: /*valueCanonical*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 176 case -766209282: /*valueCode*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 177 case -766192449: /*valueDate*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 178 case 1047929900: /*valueDateTime*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 179 case -2083993440: /*valueDecimal*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 180 case 231604844: /*valueId*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 181 case -1668687056: /*valueInstant*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 182 case -1668204915: /*valueInteger*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 183 case -497880704: /*valueMarkdown*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 184 case -1410178407: /*valueOid*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 185 case -1249932027: /*valuePositiveInt*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 186 case -1424603934: /*valueString*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 187 case -765708322: /*valueTime*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 188 case 26529417: /*valueUnsignedInt*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 189 case -1410172357: /*valueUri*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 190 case -1410172354: /*valueUrl*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 191 case -765667124: /*valueUuid*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 192 case -478981821: /*valueAddress*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 193 case -67108992: /*valueAnnotation*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 194 case -475566732: /*valueAttachment*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 195 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 196 case -1887705029: /*valueCoding*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 197 case 944904545: /*valueContactPoint*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 198 case -2026205465: /*valueHumanName*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 199 case -130498310: /*valueIdentifier*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 200 case -1524344174: /*valuePeriod*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 201 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 202 case 2030761548: /*valueRange*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 203 case 2030767386: /*valueRatio*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 204 case 1755241690: /*valueReference*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 205 case -962229101: /*valueSampledData*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 206 case -540985785: /*valueSignature*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 207 case -1406282469: /*valueTiming*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 208 case -1858636920: /*valueDosage*/ return new Property("value[x]", "*", "Value of extension - may be a resource or one of a constrained set of the data types (see Extensibility in the spec for list).", 0, 1, value); 209 default: return super.getNamedProperty(_hash, _name, _checkValid); 210 } 211 212 } 213 214 @Override 215 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 216 switch (hash) { 217 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 218 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // org.hl7.fhir.dstu3.model.Type 219 default: return super.getProperty(hash, name, checkValid); 220 } 221 222 } 223 224 @Override 225 public Base setProperty(int hash, String name, Base value) throws FHIRException { 226 switch (hash) { 227 case 116079: // url 228 this.url = castToUri(value); // UriType 229 return value; 230 case 111972721: // value 231 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 232 return value; 233 default: return super.setProperty(hash, name, value); 234 } 235 236 } 237 238 @Override 239 public Base setProperty(String name, Base value) throws FHIRException { 240 if (name.equals("url")) { 241 this.url = castToUri(value); // UriType 242 } else if (name.equals("value[x]")) { 243 this.value = castToType(value); // org.hl7.fhir.dstu3.model.Type 244 } else 245 return super.setProperty(name, value); 246 return value; 247 } 248 249 @Override 250 public Base makeProperty(int hash, String name) throws FHIRException { 251 switch (hash) { 252 case 116079: return getUrlElement(); 253 case -1410166417: return getValue(); 254 case 111972721: return getValue(); 255 default: return super.makeProperty(hash, name); 256 } 257 258 } 259 260 @Override 261 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 262 switch (hash) { 263 case 116079: /*url*/ return new String[] {"uri"}; 264 case 111972721: /*value*/ return new String[] {"*"}; 265 default: return super.getTypesForProperty(hash, name); 266 } 267 268 } 269 270 @Override 271 public Base addChild(String name) throws FHIRException { 272 if (name.equals("url")) { 273 throw new FHIRException("Cannot call addChild on a singleton property Extension.url"); 274 } 275 else if (name.equals("valueBoolean")) { 276 this.value = new BooleanType(); 277 return this.value; 278 } 279 else if (name.equals("valueInteger")) { 280 this.value = new IntegerType(); 281 return this.value; 282 } 283 else if (name.equals("valueDecimal")) { 284 this.value = new DecimalType(); 285 return this.value; 286 } 287 else if (name.equals("valueBase64Binary")) { 288 this.value = new Base64BinaryType(); 289 return this.value; 290 } 291 else if (name.equals("valueInstant")) { 292 this.value = new InstantType(); 293 return this.value; 294 } 295 else if (name.equals("valueString")) { 296 this.value = new StringType(); 297 return this.value; 298 } 299 else if (name.equals("valueUri")) { 300 this.value = new UriType(); 301 return this.value; 302 } 303 else if (name.equals("valueDate")) { 304 this.value = new DateType(); 305 return this.value; 306 } 307 else if (name.equals("valueDateTime")) { 308 this.value = new DateTimeType(); 309 return this.value; 310 } 311 else if (name.equals("valueTime")) { 312 this.value = new TimeType(); 313 return this.value; 314 } 315 else if (name.equals("valueCode")) { 316 this.value = new CodeType(); 317 return this.value; 318 } 319 else if (name.equals("valueOid")) { 320 this.value = new OidType(); 321 return this.value; 322 } 323 else if (name.equals("valueId")) { 324 this.value = new IdType(); 325 return this.value; 326 } 327 else if (name.equals("valueUnsignedInt")) { 328 this.value = new UnsignedIntType(); 329 return this.value; 330 } 331 else if (name.equals("valuePositiveInt")) { 332 this.value = new PositiveIntType(); 333 return this.value; 334 } 335 else if (name.equals("valueMarkdown")) { 336 this.value = new MarkdownType(); 337 return this.value; 338 } 339 else if (name.equals("valueAnnotation")) { 340 this.value = new Annotation(); 341 return this.value; 342 } 343 else if (name.equals("valueAttachment")) { 344 this.value = new Attachment(); 345 return this.value; 346 } 347 else if (name.equals("valueIdentifier")) { 348 this.value = new Identifier(); 349 return this.value; 350 } 351 else if (name.equals("valueCodeableConcept")) { 352 this.value = new CodeableConcept(); 353 return this.value; 354 } 355 else if (name.equals("valueCoding")) { 356 this.value = new Coding(); 357 return this.value; 358 } 359 else if (name.equals("valueQuantity")) { 360 this.value = new Quantity(); 361 return this.value; 362 } 363 else if (name.equals("valueRange")) { 364 this.value = new Range(); 365 return this.value; 366 } 367 else if (name.equals("valuePeriod")) { 368 this.value = new Period(); 369 return this.value; 370 } 371 else if (name.equals("valueRatio")) { 372 this.value = new Ratio(); 373 return this.value; 374 } 375 else if (name.equals("valueSampledData")) { 376 this.value = new SampledData(); 377 return this.value; 378 } 379 else if (name.equals("valueSignature")) { 380 this.value = new Signature(); 381 return this.value; 382 } 383 else if (name.equals("valueHumanName")) { 384 this.value = new HumanName(); 385 return this.value; 386 } 387 else if (name.equals("valueAddress")) { 388 this.value = new Address(); 389 return this.value; 390 } 391 else if (name.equals("valueContactPoint")) { 392 this.value = new ContactPoint(); 393 return this.value; 394 } 395 else if (name.equals("valueTiming")) { 396 this.value = new Timing(); 397 return this.value; 398 } 399 else if (name.equals("valueReference")) { 400 this.value = new Reference(); 401 return this.value; 402 } 403 else if (name.equals("valueMeta")) { 404 this.value = new Meta(); 405 return this.value; 406 } 407 else 408 return super.addChild(name); 409 } 410 411 public String fhirType() { 412 return "Extension"; 413 414 } 415 416 public Extension copy() { 417 Extension dst = new Extension(); 418 copyValues(dst); 419 dst.url = url == null ? null : url.copy(); 420 dst.value = value == null ? null : value.copy(); 421 return dst; 422 } 423 424 protected Extension typedCopy() { 425 return copy(); 426 } 427 428 @Override 429 public boolean equalsDeep(Base other_) { 430 if (!super.equalsDeep(other_)) 431 return false; 432 if (!(other_ instanceof Extension)) 433 return false; 434 Extension o = (Extension) other_; 435 return compareDeep(url, o.url, true) && compareDeep(value, o.value, true); 436 } 437 438 @Override 439 public boolean equalsShallow(Base other_) { 440 if (!super.equalsShallow(other_)) 441 return false; 442 if (!(other_ instanceof Extension)) 443 return false; 444 Extension o = (Extension) other_; 445 return compareValues(url, o.url, true); 446 } 447 448 public boolean isEmpty() { 449 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(url, value); 450 } 451 452 453}