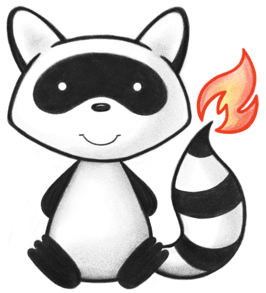
001package org.hl7.fhir.dstu3.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.io.IOException; 035import java.net.URISyntaxException; 036import java.text.ParseException; 037import java.util.UUID; 038 039import org.hl7.fhir.dstu3.model.ContactPoint.ContactPointSystem; 040import org.hl7.fhir.dstu3.model.Narrative.NarrativeStatus; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043import org.hl7.fhir.utilities.xhtml.XhtmlParser; 044 045/* 046Copyright (c) 2011+, HL7, Inc 047All rights reserved. 048 049Redistribution and use in source and binary forms, with or without modification, 050are permitted provided that the following conditions are met: 051 052 * Redistributions of source code must retain the above copyright notice, this 053 list of conditions and the following disclaimer. 054 * Redistributions in binary form must reproduce the above copyright notice, 055 this list of conditions and the following disclaimer in the documentation 056 and/or other materials provided with the distribution. 057 * Neither the name of HL7 nor the names of its contributors may be used to 058 endorse or promote products derived from this software without specific 059 prior written permission. 060 061THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 062ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 063WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 064IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 065INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 066NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 067PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 068WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 069ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 070POSSIBILITY OF SUCH DAMAGE. 071 072*/ 073 074 075 076public class Factory { 077 078 public static IdType newId(String value) { 079 if (value == null) 080 return null; 081 IdType res = new IdType(); 082 res.setValue(value); 083 return res; 084 } 085 086 public static StringType newString_(String value) { 087 if (value == null) 088 return null; 089 StringType res = new StringType(); 090 res.setValue(value); 091 return res; 092 } 093 094 public static UriType newUri(String value) throws URISyntaxException { 095 if (value == null) 096 return null; 097 UriType res = new UriType(); 098 res.setValue(value); 099 return res; 100 } 101 102 public static DateTimeType newDateTime(String value) throws ParseException { 103 if (value == null) 104 return null; 105 return new DateTimeType(value); 106 } 107 108 public static DateType newDate(String value) throws ParseException { 109 if (value == null) 110 return null; 111 return new DateType(value); 112 } 113 114 public static CodeType newCode(String value) { 115 if (value == null) 116 return null; 117 CodeType res = new CodeType(); 118 res.setValue(value); 119 return res; 120 } 121 122 public static IntegerType newInteger(int value) { 123 IntegerType res = new IntegerType(); 124 res.setValue(value); 125 return res; 126 } 127 128 public static IntegerType newInteger(java.lang.Integer value) { 129 if (value == null) 130 return null; 131 IntegerType res = new IntegerType(); 132 res.setValue(value); 133 return res; 134 } 135 136 public static BooleanType newBoolean(boolean value) { 137 BooleanType res = new BooleanType(); 138 res.setValue(value); 139 return res; 140 } 141 142 public static ContactPoint newContactPoint(ContactPointSystem system, String value) { 143 ContactPoint res = new ContactPoint(); 144 res.setSystem(system); 145 res.setValue(value); 146 return res; 147 } 148 149 public static Extension newExtension(String uri, Type value, boolean evenIfNull) { 150 if (!evenIfNull && (value == null || value.isEmpty())) 151 return null; 152 Extension e = new Extension(); 153 e.setUrl(uri); 154 e.setValue(value); 155 return e; 156 } 157 158 public static CodeableConcept newCodeableConcept(String code, String system, String display) { 159 CodeableConcept cc = new CodeableConcept(); 160 Coding c = new Coding(); 161 c.setCode(code); 162 c.setSystem(system); 163 c.setDisplay(display); 164 cc.getCoding().add(c); 165 return cc; 166 } 167 168 public static Reference makeReference(String url) { 169 Reference rr = new Reference(); 170 rr.setReference(url); 171 return rr; 172 } 173 174 public static Narrative newNarrative(NarrativeStatus status, String html) throws IOException, FHIRException { 175 Narrative n = new Narrative(); 176 n.setStatus(status); 177 try { 178 n.setDiv(new XhtmlParser().parseFragment("<div>"+Utilities.escapeXml(html)+"</div>")); 179 } catch (org.hl7.fhir.exceptions.FHIRException e) { 180 throw new FHIRException(e.getMessage(), e); 181 } 182 return n; 183 } 184 185 public static Coding makeCoding(String code) throws FHIRException { 186 String[] parts = code.split("\\|"); 187 Coding c = new Coding(); 188 if (parts.length == 2) { 189 c.setSystem(parts[0]); 190 c.setCode(parts[1]); 191 } else if (parts.length == 3) { 192 c.setSystem(parts[0]); 193 c.setCode(parts[1]); 194 c.setDisplay(parts[2]); 195 } else 196 throw new FHIRException("Unable to understand the code '"+code+"'. Use the format system|code(|display)"); 197 return c; 198 } 199 200 public static Reference makeReference(String url, String text) { 201 Reference rr = new Reference(); 202 rr.setReference(url); 203 if (!Utilities.noString(text)) 204 rr.setDisplay(text); 205 return rr; 206 } 207 208 public static String createUUID() { 209 return "urn:uuid:"+UUID.randomUUID().toString().toLowerCase(); 210 } 211 212 public Type create(String name) throws FHIRException { 213 if (name.equals("boolean")) 214 return new BooleanType(); 215 else if (name.equals("integer")) 216 return new IntegerType(); 217 else if (name.equals("decimal")) 218 return new DecimalType(); 219 else if (name.equals("base64Binary")) 220 return new Base64BinaryType(); 221 else if (name.equals("instant")) 222 return new InstantType(); 223 else if (name.equals("string")) 224 return new StringType(); 225 else if (name.equals("uri")) 226 return new UriType(); 227 else if (name.equals("date")) 228 return new DateType(); 229 else if (name.equals("dateTime")) 230 return new DateTimeType(); 231 else if (name.equals("time")) 232 return new TimeType(); 233 else if (name.equals("code")) 234 return new CodeType(); 235 else if (name.equals("oid")) 236 return new OidType(); 237 else if (name.equals("id")) 238 return new IdType(); 239 else if (name.equals("unsignedInt")) 240 return new UnsignedIntType(); 241 else if (name.equals("positiveInt")) 242 return new PositiveIntType(); 243 else if (name.equals("markdown")) 244 return new MarkdownType(); 245 else if (name.equals("Annotation")) 246 return new Annotation(); 247 else if (name.equals("Attachment")) 248 return new Attachment(); 249 else if (name.equals("Identifier")) 250 return new Identifier(); 251 else if (name.equals("CodeableConcept")) 252 return new CodeableConcept(); 253 else if (name.equals("Coding")) 254 return new Coding(); 255 else if (name.equals("Quantity")) 256 return new Quantity(); 257 else if (name.equals("Range")) 258 return new Range(); 259 else if (name.equals("Period")) 260 return new Period(); 261 else if (name.equals("Ratio")) 262 return new Ratio(); 263 else if (name.equals("SampledData")) 264 return new SampledData(); 265 else if (name.equals("Signature")) 266 return new Signature(); 267 else if (name.equals("HumanName")) 268 return new HumanName(); 269 else if (name.equals("Address")) 270 return new Address(); 271 else if (name.equals("ContactPoint")) 272 return new ContactPoint(); 273 else if (name.equals("Timing")) 274 return new Timing(); 275 else if (name.equals("Reference")) 276 return new Reference(); 277 else if (name.equals("Meta")) 278 return new Meta(); 279 else 280 throw new FHIRException("Unknown data type name "+name); 281 } 282}