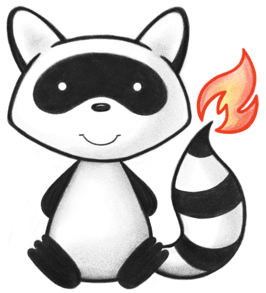
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGender; 040import org.hl7.fhir.dstu3.model.Enumerations.AdministrativeGenderEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.exceptions.FHIRFormatError; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * Significant health events and conditions for a person related to the patient relevant in the context of care for the patient. 053 */ 054@ResourceDef(name="FamilyMemberHistory", profile="http://hl7.org/fhir/Profile/FamilyMemberHistory") 055public class FamilyMemberHistory extends DomainResource { 056 057 public enum FamilyHistoryStatus { 058 /** 059 * Some health information is known and captured, but not complete - see notes for details. 060 */ 061 PARTIAL, 062 /** 063 * All available related health information is captured as of the date (and possibly time) when the family member history was taken. 064 */ 065 COMPLETED, 066 /** 067 * This instance should not have been part of this patient's medical record. 068 */ 069 ENTEREDINERROR, 070 /** 071 * Health information for this individual is unavailable/unknown. 072 */ 073 HEALTHUNKNOWN, 074 /** 075 * added to help the parsers with the generic types 076 */ 077 NULL; 078 public static FamilyHistoryStatus fromCode(String codeString) throws FHIRException { 079 if (codeString == null || "".equals(codeString)) 080 return null; 081 if ("partial".equals(codeString)) 082 return PARTIAL; 083 if ("completed".equals(codeString)) 084 return COMPLETED; 085 if ("entered-in-error".equals(codeString)) 086 return ENTEREDINERROR; 087 if ("health-unknown".equals(codeString)) 088 return HEALTHUNKNOWN; 089 if (Configuration.isAcceptInvalidEnums()) 090 return null; 091 else 092 throw new FHIRException("Unknown FamilyHistoryStatus code '"+codeString+"'"); 093 } 094 public String toCode() { 095 switch (this) { 096 case PARTIAL: return "partial"; 097 case COMPLETED: return "completed"; 098 case ENTEREDINERROR: return "entered-in-error"; 099 case HEALTHUNKNOWN: return "health-unknown"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getSystem() { 105 switch (this) { 106 case PARTIAL: return "http://hl7.org/fhir/history-status"; 107 case COMPLETED: return "http://hl7.org/fhir/history-status"; 108 case ENTEREDINERROR: return "http://hl7.org/fhir/history-status"; 109 case HEALTHUNKNOWN: return "http://hl7.org/fhir/history-status"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDefinition() { 115 switch (this) { 116 case PARTIAL: return "Some health information is known and captured, but not complete - see notes for details."; 117 case COMPLETED: return "All available related health information is captured as of the date (and possibly time) when the family member history was taken."; 118 case ENTEREDINERROR: return "This instance should not have been part of this patient's medical record."; 119 case HEALTHUNKNOWN: return "Health information for this individual is unavailable/unknown."; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getDisplay() { 125 switch (this) { 126 case PARTIAL: return "Partial"; 127 case COMPLETED: return "Completed"; 128 case ENTEREDINERROR: return "Entered in error"; 129 case HEALTHUNKNOWN: return "Health unknown"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 } 135 136 public static class FamilyHistoryStatusEnumFactory implements EnumFactory<FamilyHistoryStatus> { 137 public FamilyHistoryStatus fromCode(String codeString) throws IllegalArgumentException { 138 if (codeString == null || "".equals(codeString)) 139 if (codeString == null || "".equals(codeString)) 140 return null; 141 if ("partial".equals(codeString)) 142 return FamilyHistoryStatus.PARTIAL; 143 if ("completed".equals(codeString)) 144 return FamilyHistoryStatus.COMPLETED; 145 if ("entered-in-error".equals(codeString)) 146 return FamilyHistoryStatus.ENTEREDINERROR; 147 if ("health-unknown".equals(codeString)) 148 return FamilyHistoryStatus.HEALTHUNKNOWN; 149 throw new IllegalArgumentException("Unknown FamilyHistoryStatus code '"+codeString+"'"); 150 } 151 public Enumeration<FamilyHistoryStatus> fromType(PrimitiveType<?> code) throws FHIRException { 152 if (code == null) 153 return null; 154 if (code.isEmpty()) 155 return new Enumeration<FamilyHistoryStatus>(this); 156 String codeString = code.asStringValue(); 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("partial".equals(codeString)) 160 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.PARTIAL); 161 if ("completed".equals(codeString)) 162 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.COMPLETED); 163 if ("entered-in-error".equals(codeString)) 164 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.ENTEREDINERROR); 165 if ("health-unknown".equals(codeString)) 166 return new Enumeration<FamilyHistoryStatus>(this, FamilyHistoryStatus.HEALTHUNKNOWN); 167 throw new FHIRException("Unknown FamilyHistoryStatus code '"+codeString+"'"); 168 } 169 public String toCode(FamilyHistoryStatus code) { 170 if (code == FamilyHistoryStatus.NULL) 171 return null; 172 if (code == FamilyHistoryStatus.PARTIAL) 173 return "partial"; 174 if (code == FamilyHistoryStatus.COMPLETED) 175 return "completed"; 176 if (code == FamilyHistoryStatus.ENTEREDINERROR) 177 return "entered-in-error"; 178 if (code == FamilyHistoryStatus.HEALTHUNKNOWN) 179 return "health-unknown"; 180 return "?"; 181 } 182 public String toSystem(FamilyHistoryStatus code) { 183 return code.getSystem(); 184 } 185 } 186 187 @Block() 188 public static class FamilyMemberHistoryConditionComponent extends BackboneElement implements IBaseBackboneElement { 189 /** 190 * The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system. 191 */ 192 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 193 @Description(shortDefinition="Condition suffered by relation", formalDefinition="The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system." ) 194 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 195 protected CodeableConcept code; 196 197 /** 198 * Indicates what happened as a result of this condition. If the condition resulted in death, deceased date is captured on the relation. 199 */ 200 @Child(name = "outcome", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 201 @Description(shortDefinition="deceased | permanent disability | etc.", formalDefinition="Indicates what happened as a result of this condition. If the condition resulted in death, deceased date is captured on the relation." ) 202 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-outcome") 203 protected CodeableConcept outcome; 204 205 /** 206 * Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence. 207 */ 208 @Child(name = "onset", type = {Age.class, Range.class, Period.class, StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 209 @Description(shortDefinition="When condition first manifested", formalDefinition="Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence." ) 210 protected Type onset; 211 212 /** 213 * An area where general notes can be placed about this specific condition. 214 */ 215 @Child(name = "note", type = {Annotation.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 216 @Description(shortDefinition="Extra information about condition", formalDefinition="An area where general notes can be placed about this specific condition." ) 217 protected List<Annotation> note; 218 219 private static final long serialVersionUID = 598309281L; 220 221 /** 222 * Constructor 223 */ 224 public FamilyMemberHistoryConditionComponent() { 225 super(); 226 } 227 228 /** 229 * Constructor 230 */ 231 public FamilyMemberHistoryConditionComponent(CodeableConcept code) { 232 super(); 233 this.code = code; 234 } 235 236 /** 237 * @return {@link #code} (The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.) 238 */ 239 public CodeableConcept getCode() { 240 if (this.code == null) 241 if (Configuration.errorOnAutoCreate()) 242 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.code"); 243 else if (Configuration.doAutoCreate()) 244 this.code = new CodeableConcept(); // cc 245 return this.code; 246 } 247 248 public boolean hasCode() { 249 return this.code != null && !this.code.isEmpty(); 250 } 251 252 /** 253 * @param value {@link #code} (The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.) 254 */ 255 public FamilyMemberHistoryConditionComponent setCode(CodeableConcept value) { 256 this.code = value; 257 return this; 258 } 259 260 /** 261 * @return {@link #outcome} (Indicates what happened as a result of this condition. If the condition resulted in death, deceased date is captured on the relation.) 262 */ 263 public CodeableConcept getOutcome() { 264 if (this.outcome == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create FamilyMemberHistoryConditionComponent.outcome"); 267 else if (Configuration.doAutoCreate()) 268 this.outcome = new CodeableConcept(); // cc 269 return this.outcome; 270 } 271 272 public boolean hasOutcome() { 273 return this.outcome != null && !this.outcome.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #outcome} (Indicates what happened as a result of this condition. If the condition resulted in death, deceased date is captured on the relation.) 278 */ 279 public FamilyMemberHistoryConditionComponent setOutcome(CodeableConcept value) { 280 this.outcome = value; 281 return this; 282 } 283 284 /** 285 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 286 */ 287 public Type getOnset() { 288 return this.onset; 289 } 290 291 /** 292 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 293 */ 294 public Age getOnsetAge() throws FHIRException { 295 if (this.onset == null) 296 return null; 297 if (!(this.onset instanceof Age)) 298 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.onset.getClass().getName()+" was encountered"); 299 return (Age) this.onset; 300 } 301 302 public boolean hasOnsetAge() { 303 return this != null && this.onset instanceof Age; 304 } 305 306 /** 307 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 308 */ 309 public Range getOnsetRange() throws FHIRException { 310 if (this.onset == null) 311 return null; 312 if (!(this.onset instanceof Range)) 313 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.onset.getClass().getName()+" was encountered"); 314 return (Range) this.onset; 315 } 316 317 public boolean hasOnsetRange() { 318 return this != null && this.onset instanceof Range; 319 } 320 321 /** 322 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 323 */ 324 public Period getOnsetPeriod() throws FHIRException { 325 if (this.onset == null) 326 return null; 327 if (!(this.onset instanceof Period)) 328 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.onset.getClass().getName()+" was encountered"); 329 return (Period) this.onset; 330 } 331 332 public boolean hasOnsetPeriod() { 333 return this != null && this.onset instanceof Period; 334 } 335 336 /** 337 * @return {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 338 */ 339 public StringType getOnsetStringType() throws FHIRException { 340 if (this.onset == null) 341 return null; 342 if (!(this.onset instanceof StringType)) 343 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.onset.getClass().getName()+" was encountered"); 344 return (StringType) this.onset; 345 } 346 347 public boolean hasOnsetStringType() { 348 return this != null && this.onset instanceof StringType; 349 } 350 351 public boolean hasOnset() { 352 return this.onset != null && !this.onset.isEmpty(); 353 } 354 355 /** 356 * @param value {@link #onset} (Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.) 357 */ 358 public FamilyMemberHistoryConditionComponent setOnset(Type value) throws FHIRFormatError { 359 if (value != null && !(value instanceof Age || value instanceof Range || value instanceof Period || value instanceof StringType)) 360 throw new FHIRFormatError("Not the right type for FamilyMemberHistory.condition.onset[x]: "+value.fhirType()); 361 this.onset = value; 362 return this; 363 } 364 365 /** 366 * @return {@link #note} (An area where general notes can be placed about this specific condition.) 367 */ 368 public List<Annotation> getNote() { 369 if (this.note == null) 370 this.note = new ArrayList<Annotation>(); 371 return this.note; 372 } 373 374 /** 375 * @return Returns a reference to <code>this</code> for easy method chaining 376 */ 377 public FamilyMemberHistoryConditionComponent setNote(List<Annotation> theNote) { 378 this.note = theNote; 379 return this; 380 } 381 382 public boolean hasNote() { 383 if (this.note == null) 384 return false; 385 for (Annotation item : this.note) 386 if (!item.isEmpty()) 387 return true; 388 return false; 389 } 390 391 public Annotation addNote() { //3 392 Annotation t = new Annotation(); 393 if (this.note == null) 394 this.note = new ArrayList<Annotation>(); 395 this.note.add(t); 396 return t; 397 } 398 399 public FamilyMemberHistoryConditionComponent addNote(Annotation t) { //3 400 if (t == null) 401 return this; 402 if (this.note == null) 403 this.note = new ArrayList<Annotation>(); 404 this.note.add(t); 405 return this; 406 } 407 408 /** 409 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 410 */ 411 public Annotation getNoteFirstRep() { 412 if (getNote().isEmpty()) { 413 addNote(); 414 } 415 return getNote().get(0); 416 } 417 418 protected void listChildren(List<Property> children) { 419 super.listChildren(children); 420 children.add(new Property("code", "CodeableConcept", "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.", 0, 1, code)); 421 children.add(new Property("outcome", "CodeableConcept", "Indicates what happened as a result of this condition. If the condition resulted in death, deceased date is captured on the relation.", 0, 1, outcome)); 422 children.add(new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset)); 423 children.add(new Property("note", "Annotation", "An area where general notes can be placed about this specific condition.", 0, java.lang.Integer.MAX_VALUE, note)); 424 } 425 426 @Override 427 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 428 switch (_hash) { 429 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The actual condition specified. Could be a coded condition (like MI or Diabetes) or a less specific string like 'cancer' depending on how much is known about the condition and the capabilities of the creating system.", 0, 1, code); 430 case -1106507950: /*outcome*/ return new Property("outcome", "CodeableConcept", "Indicates what happened as a result of this condition. If the condition resulted in death, deceased date is captured on the relation.", 0, 1, outcome); 431 case -1886216323: /*onset[x]*/ return new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 432 case 105901603: /*onset*/ return new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 433 case -1886241828: /*onsetAge*/ return new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 434 case -186664742: /*onsetRange*/ return new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 435 case -1545082428: /*onsetPeriod*/ return new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 436 case -1445342188: /*onsetString*/ return new Property("onset[x]", "Age|Range|Period|string", "Either the age of onset, range of approximate age or descriptive string can be recorded. For conditions with multiple occurrences, this describes the first known occurrence.", 0, 1, onset); 437 case 3387378: /*note*/ return new Property("note", "Annotation", "An area where general notes can be placed about this specific condition.", 0, java.lang.Integer.MAX_VALUE, note); 438 default: return super.getNamedProperty(_hash, _name, _checkValid); 439 } 440 441 } 442 443 @Override 444 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 445 switch (hash) { 446 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 447 case -1106507950: /*outcome*/ return this.outcome == null ? new Base[0] : new Base[] {this.outcome}; // CodeableConcept 448 case 105901603: /*onset*/ return this.onset == null ? new Base[0] : new Base[] {this.onset}; // Type 449 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 450 default: return super.getProperty(hash, name, checkValid); 451 } 452 453 } 454 455 @Override 456 public Base setProperty(int hash, String name, Base value) throws FHIRException { 457 switch (hash) { 458 case 3059181: // code 459 this.code = castToCodeableConcept(value); // CodeableConcept 460 return value; 461 case -1106507950: // outcome 462 this.outcome = castToCodeableConcept(value); // CodeableConcept 463 return value; 464 case 105901603: // onset 465 this.onset = castToType(value); // Type 466 return value; 467 case 3387378: // note 468 this.getNote().add(castToAnnotation(value)); // Annotation 469 return value; 470 default: return super.setProperty(hash, name, value); 471 } 472 473 } 474 475 @Override 476 public Base setProperty(String name, Base value) throws FHIRException { 477 if (name.equals("code")) { 478 this.code = castToCodeableConcept(value); // CodeableConcept 479 } else if (name.equals("outcome")) { 480 this.outcome = castToCodeableConcept(value); // CodeableConcept 481 } else if (name.equals("onset[x]")) { 482 this.onset = castToType(value); // Type 483 } else if (name.equals("note")) { 484 this.getNote().add(castToAnnotation(value)); 485 } else 486 return super.setProperty(name, value); 487 return value; 488 } 489 490 @Override 491 public Base makeProperty(int hash, String name) throws FHIRException { 492 switch (hash) { 493 case 3059181: return getCode(); 494 case -1106507950: return getOutcome(); 495 case -1886216323: return getOnset(); 496 case 105901603: return getOnset(); 497 case 3387378: return addNote(); 498 default: return super.makeProperty(hash, name); 499 } 500 501 } 502 503 @Override 504 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 505 switch (hash) { 506 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 507 case -1106507950: /*outcome*/ return new String[] {"CodeableConcept"}; 508 case 105901603: /*onset*/ return new String[] {"Age", "Range", "Period", "string"}; 509 case 3387378: /*note*/ return new String[] {"Annotation"}; 510 default: return super.getTypesForProperty(hash, name); 511 } 512 513 } 514 515 @Override 516 public Base addChild(String name) throws FHIRException { 517 if (name.equals("code")) { 518 this.code = new CodeableConcept(); 519 return this.code; 520 } 521 else if (name.equals("outcome")) { 522 this.outcome = new CodeableConcept(); 523 return this.outcome; 524 } 525 else if (name.equals("onsetAge")) { 526 this.onset = new Age(); 527 return this.onset; 528 } 529 else if (name.equals("onsetRange")) { 530 this.onset = new Range(); 531 return this.onset; 532 } 533 else if (name.equals("onsetPeriod")) { 534 this.onset = new Period(); 535 return this.onset; 536 } 537 else if (name.equals("onsetString")) { 538 this.onset = new StringType(); 539 return this.onset; 540 } 541 else if (name.equals("note")) { 542 return addNote(); 543 } 544 else 545 return super.addChild(name); 546 } 547 548 public FamilyMemberHistoryConditionComponent copy() { 549 FamilyMemberHistoryConditionComponent dst = new FamilyMemberHistoryConditionComponent(); 550 copyValues(dst); 551 dst.code = code == null ? null : code.copy(); 552 dst.outcome = outcome == null ? null : outcome.copy(); 553 dst.onset = onset == null ? null : onset.copy(); 554 if (note != null) { 555 dst.note = new ArrayList<Annotation>(); 556 for (Annotation i : note) 557 dst.note.add(i.copy()); 558 }; 559 return dst; 560 } 561 562 @Override 563 public boolean equalsDeep(Base other_) { 564 if (!super.equalsDeep(other_)) 565 return false; 566 if (!(other_ instanceof FamilyMemberHistoryConditionComponent)) 567 return false; 568 FamilyMemberHistoryConditionComponent o = (FamilyMemberHistoryConditionComponent) other_; 569 return compareDeep(code, o.code, true) && compareDeep(outcome, o.outcome, true) && compareDeep(onset, o.onset, true) 570 && compareDeep(note, o.note, true); 571 } 572 573 @Override 574 public boolean equalsShallow(Base other_) { 575 if (!super.equalsShallow(other_)) 576 return false; 577 if (!(other_ instanceof FamilyMemberHistoryConditionComponent)) 578 return false; 579 FamilyMemberHistoryConditionComponent o = (FamilyMemberHistoryConditionComponent) other_; 580 return true; 581 } 582 583 public boolean isEmpty() { 584 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, outcome, onset, note 585 ); 586 } 587 588 public String fhirType() { 589 return "FamilyMemberHistory.condition"; 590 591 } 592 593 } 594 595 /** 596 * This records identifiers associated with this family member history record that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 597 */ 598 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 599 @Description(shortDefinition="External Id(s) for this record", formalDefinition="This records identifiers associated with this family member history record that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 600 protected List<Identifier> identifier; 601 602 /** 603 * A protocol or questionnaire that was adhered to in whole or in part by this event. 604 */ 605 @Child(name = "definition", type = {PlanDefinition.class, Questionnaire.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 606 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="A protocol or questionnaire that was adhered to in whole or in part by this event." ) 607 protected List<Reference> definition; 608 /** 609 * The actual objects that are the target of the reference (A protocol or questionnaire that was adhered to in whole or in part by this event.) 610 */ 611 protected List<Resource> definitionTarget; 612 613 614 /** 615 * A code specifying the status of the record of the family history of a specific family member. 616 */ 617 @Child(name = "status", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 618 @Description(shortDefinition="partial | completed | entered-in-error | health-unknown", formalDefinition="A code specifying the status of the record of the family history of a specific family member." ) 619 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/history-status") 620 protected Enumeration<FamilyHistoryStatus> status; 621 622 /** 623 * If true, indicates the taking of an individual family member's history did not occur. The notDone element should not be used to document negated conditions, such as a family member that did not have a condition. 624 */ 625 @Child(name = "notDone", type = {BooleanType.class}, order=3, min=0, max=1, modifier=true, summary=true) 626 @Description(shortDefinition="The taking of a family member's history did not occur", formalDefinition="If true, indicates the taking of an individual family member's history did not occur. The notDone element should not be used to document negated conditions, such as a family member that did not have a condition." ) 627 protected BooleanType notDone; 628 629 /** 630 * Describes why the family member's history is absent. 631 */ 632 @Child(name = "notDoneReason", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 633 @Description(shortDefinition="subject-unknown | withheld | unable-to-obtain | deferred", formalDefinition="Describes why the family member's history is absent." ) 634 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/history-not-done-reason") 635 protected CodeableConcept notDoneReason; 636 637 /** 638 * The person who this history concerns. 639 */ 640 @Child(name = "patient", type = {Patient.class}, order=5, min=1, max=1, modifier=false, summary=true) 641 @Description(shortDefinition="Patient history is about", formalDefinition="The person who this history concerns." ) 642 protected Reference patient; 643 644 /** 645 * The actual object that is the target of the reference (The person who this history concerns.) 646 */ 647 protected Patient patientTarget; 648 649 /** 650 * The date (and possibly time) when the family member history was taken. 651 */ 652 @Child(name = "date", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 653 @Description(shortDefinition="When history was captured/updated", formalDefinition="The date (and possibly time) when the family member history was taken." ) 654 protected DateTimeType date; 655 656 /** 657 * This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair". 658 */ 659 @Child(name = "name", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 660 @Description(shortDefinition="The family member described", formalDefinition="This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\"." ) 661 protected StringType name; 662 663 /** 664 * The type of relationship this person has to the patient (father, mother, brother etc.). 665 */ 666 @Child(name = "relationship", type = {CodeableConcept.class}, order=8, min=1, max=1, modifier=false, summary=true) 667 @Description(shortDefinition="Relationship to the subject", formalDefinition="The type of relationship this person has to the patient (father, mother, brother etc.)." ) 668 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-FamilyMember") 669 protected CodeableConcept relationship; 670 671 /** 672 * Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes. 673 */ 674 @Child(name = "gender", type = {CodeType.class}, order=9, min=0, max=1, modifier=false, summary=true) 675 @Description(shortDefinition="male | female | other | unknown", formalDefinition="Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes." ) 676 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administrative-gender") 677 protected Enumeration<AdministrativeGender> gender; 678 679 /** 680 * The actual or approximate date of birth of the relative. 681 */ 682 @Child(name = "born", type = {Period.class, DateType.class, StringType.class}, order=10, min=0, max=1, modifier=false, summary=false) 683 @Description(shortDefinition="(approximate) date of birth", formalDefinition="The actual or approximate date of birth of the relative." ) 684 protected Type born; 685 686 /** 687 * The age of the relative at the time the family member history is recorded. 688 */ 689 @Child(name = "age", type = {Age.class, Range.class, StringType.class}, order=11, min=0, max=1, modifier=false, summary=true) 690 @Description(shortDefinition="(approximate) age", formalDefinition="The age of the relative at the time the family member history is recorded." ) 691 protected Type age; 692 693 /** 694 * If true, indicates that the age value specified is an estimated value. 695 */ 696 @Child(name = "estimatedAge", type = {BooleanType.class}, order=12, min=0, max=1, modifier=true, summary=true) 697 @Description(shortDefinition="Age is estimated?", formalDefinition="If true, indicates that the age value specified is an estimated value." ) 698 protected BooleanType estimatedAge; 699 700 /** 701 * Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record. 702 */ 703 @Child(name = "deceased", type = {BooleanType.class, Age.class, Range.class, DateType.class, StringType.class}, order=13, min=0, max=1, modifier=false, summary=true) 704 @Description(shortDefinition="Dead? How old/when?", formalDefinition="Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record." ) 705 protected Type deceased; 706 707 /** 708 * Describes why the family member history occurred in coded or textual form. 709 */ 710 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 711 @Description(shortDefinition="Why was family member history performed?", formalDefinition="Describes why the family member history occurred in coded or textual form." ) 712 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 713 protected List<CodeableConcept> reasonCode; 714 715 /** 716 * Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event. 717 */ 718 @Child(name = "reasonReference", type = {Condition.class, Observation.class, AllergyIntolerance.class, QuestionnaireResponse.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 719 @Description(shortDefinition="Why was family member history performed?", formalDefinition="Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event." ) 720 protected List<Reference> reasonReference; 721 /** 722 * The actual objects that are the target of the reference (Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.) 723 */ 724 protected List<Resource> reasonReferenceTarget; 725 726 727 /** 728 * This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible. 729 */ 730 @Child(name = "note", type = {Annotation.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 731 @Description(shortDefinition="General note about related person", formalDefinition="This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible." ) 732 protected List<Annotation> note; 733 734 /** 735 * The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition. 736 */ 737 @Child(name = "condition", type = {}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 738 @Description(shortDefinition="Condition that the related person had", formalDefinition="The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition." ) 739 protected List<FamilyMemberHistoryConditionComponent> condition; 740 741 private static final long serialVersionUID = 388742645L; 742 743 /** 744 * Constructor 745 */ 746 public FamilyMemberHistory() { 747 super(); 748 } 749 750 /** 751 * Constructor 752 */ 753 public FamilyMemberHistory(Enumeration<FamilyHistoryStatus> status, Reference patient, CodeableConcept relationship) { 754 super(); 755 this.status = status; 756 this.patient = patient; 757 this.relationship = relationship; 758 } 759 760 /** 761 * @return {@link #identifier} (This records identifiers associated with this family member history record that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 762 */ 763 public List<Identifier> getIdentifier() { 764 if (this.identifier == null) 765 this.identifier = new ArrayList<Identifier>(); 766 return this.identifier; 767 } 768 769 /** 770 * @return Returns a reference to <code>this</code> for easy method chaining 771 */ 772 public FamilyMemberHistory setIdentifier(List<Identifier> theIdentifier) { 773 this.identifier = theIdentifier; 774 return this; 775 } 776 777 public boolean hasIdentifier() { 778 if (this.identifier == null) 779 return false; 780 for (Identifier item : this.identifier) 781 if (!item.isEmpty()) 782 return true; 783 return false; 784 } 785 786 public Identifier addIdentifier() { //3 787 Identifier t = new Identifier(); 788 if (this.identifier == null) 789 this.identifier = new ArrayList<Identifier>(); 790 this.identifier.add(t); 791 return t; 792 } 793 794 public FamilyMemberHistory addIdentifier(Identifier t) { //3 795 if (t == null) 796 return this; 797 if (this.identifier == null) 798 this.identifier = new ArrayList<Identifier>(); 799 this.identifier.add(t); 800 return this; 801 } 802 803 /** 804 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 805 */ 806 public Identifier getIdentifierFirstRep() { 807 if (getIdentifier().isEmpty()) { 808 addIdentifier(); 809 } 810 return getIdentifier().get(0); 811 } 812 813 /** 814 * @return {@link #definition} (A protocol or questionnaire that was adhered to in whole or in part by this event.) 815 */ 816 public List<Reference> getDefinition() { 817 if (this.definition == null) 818 this.definition = new ArrayList<Reference>(); 819 return this.definition; 820 } 821 822 /** 823 * @return Returns a reference to <code>this</code> for easy method chaining 824 */ 825 public FamilyMemberHistory setDefinition(List<Reference> theDefinition) { 826 this.definition = theDefinition; 827 return this; 828 } 829 830 public boolean hasDefinition() { 831 if (this.definition == null) 832 return false; 833 for (Reference item : this.definition) 834 if (!item.isEmpty()) 835 return true; 836 return false; 837 } 838 839 public Reference addDefinition() { //3 840 Reference t = new Reference(); 841 if (this.definition == null) 842 this.definition = new ArrayList<Reference>(); 843 this.definition.add(t); 844 return t; 845 } 846 847 public FamilyMemberHistory addDefinition(Reference t) { //3 848 if (t == null) 849 return this; 850 if (this.definition == null) 851 this.definition = new ArrayList<Reference>(); 852 this.definition.add(t); 853 return this; 854 } 855 856 /** 857 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 858 */ 859 public Reference getDefinitionFirstRep() { 860 if (getDefinition().isEmpty()) { 861 addDefinition(); 862 } 863 return getDefinition().get(0); 864 } 865 866 /** 867 * @deprecated Use Reference#setResource(IBaseResource) instead 868 */ 869 @Deprecated 870 public List<Resource> getDefinitionTarget() { 871 if (this.definitionTarget == null) 872 this.definitionTarget = new ArrayList<Resource>(); 873 return this.definitionTarget; 874 } 875 876 /** 877 * @return {@link #status} (A code specifying the status of the record of the family history of a specific family member.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 878 */ 879 public Enumeration<FamilyHistoryStatus> getStatusElement() { 880 if (this.status == null) 881 if (Configuration.errorOnAutoCreate()) 882 throw new Error("Attempt to auto-create FamilyMemberHistory.status"); 883 else if (Configuration.doAutoCreate()) 884 this.status = new Enumeration<FamilyHistoryStatus>(new FamilyHistoryStatusEnumFactory()); // bb 885 return this.status; 886 } 887 888 public boolean hasStatusElement() { 889 return this.status != null && !this.status.isEmpty(); 890 } 891 892 public boolean hasStatus() { 893 return this.status != null && !this.status.isEmpty(); 894 } 895 896 /** 897 * @param value {@link #status} (A code specifying the status of the record of the family history of a specific family member.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 898 */ 899 public FamilyMemberHistory setStatusElement(Enumeration<FamilyHistoryStatus> value) { 900 this.status = value; 901 return this; 902 } 903 904 /** 905 * @return A code specifying the status of the record of the family history of a specific family member. 906 */ 907 public FamilyHistoryStatus getStatus() { 908 return this.status == null ? null : this.status.getValue(); 909 } 910 911 /** 912 * @param value A code specifying the status of the record of the family history of a specific family member. 913 */ 914 public FamilyMemberHistory setStatus(FamilyHistoryStatus value) { 915 if (this.status == null) 916 this.status = new Enumeration<FamilyHistoryStatus>(new FamilyHistoryStatusEnumFactory()); 917 this.status.setValue(value); 918 return this; 919 } 920 921 /** 922 * @return {@link #notDone} (If true, indicates the taking of an individual family member's history did not occur. The notDone element should not be used to document negated conditions, such as a family member that did not have a condition.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 923 */ 924 public BooleanType getNotDoneElement() { 925 if (this.notDone == null) 926 if (Configuration.errorOnAutoCreate()) 927 throw new Error("Attempt to auto-create FamilyMemberHistory.notDone"); 928 else if (Configuration.doAutoCreate()) 929 this.notDone = new BooleanType(); // bb 930 return this.notDone; 931 } 932 933 public boolean hasNotDoneElement() { 934 return this.notDone != null && !this.notDone.isEmpty(); 935 } 936 937 public boolean hasNotDone() { 938 return this.notDone != null && !this.notDone.isEmpty(); 939 } 940 941 /** 942 * @param value {@link #notDone} (If true, indicates the taking of an individual family member's history did not occur. The notDone element should not be used to document negated conditions, such as a family member that did not have a condition.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 943 */ 944 public FamilyMemberHistory setNotDoneElement(BooleanType value) { 945 this.notDone = value; 946 return this; 947 } 948 949 /** 950 * @return If true, indicates the taking of an individual family member's history did not occur. The notDone element should not be used to document negated conditions, such as a family member that did not have a condition. 951 */ 952 public boolean getNotDone() { 953 return this.notDone == null || this.notDone.isEmpty() ? false : this.notDone.getValue(); 954 } 955 956 /** 957 * @param value If true, indicates the taking of an individual family member's history did not occur. The notDone element should not be used to document negated conditions, such as a family member that did not have a condition. 958 */ 959 public FamilyMemberHistory setNotDone(boolean value) { 960 if (this.notDone == null) 961 this.notDone = new BooleanType(); 962 this.notDone.setValue(value); 963 return this; 964 } 965 966 /** 967 * @return {@link #notDoneReason} (Describes why the family member's history is absent.) 968 */ 969 public CodeableConcept getNotDoneReason() { 970 if (this.notDoneReason == null) 971 if (Configuration.errorOnAutoCreate()) 972 throw new Error("Attempt to auto-create FamilyMemberHistory.notDoneReason"); 973 else if (Configuration.doAutoCreate()) 974 this.notDoneReason = new CodeableConcept(); // cc 975 return this.notDoneReason; 976 } 977 978 public boolean hasNotDoneReason() { 979 return this.notDoneReason != null && !this.notDoneReason.isEmpty(); 980 } 981 982 /** 983 * @param value {@link #notDoneReason} (Describes why the family member's history is absent.) 984 */ 985 public FamilyMemberHistory setNotDoneReason(CodeableConcept value) { 986 this.notDoneReason = value; 987 return this; 988 } 989 990 /** 991 * @return {@link #patient} (The person who this history concerns.) 992 */ 993 public Reference getPatient() { 994 if (this.patient == null) 995 if (Configuration.errorOnAutoCreate()) 996 throw new Error("Attempt to auto-create FamilyMemberHistory.patient"); 997 else if (Configuration.doAutoCreate()) 998 this.patient = new Reference(); // cc 999 return this.patient; 1000 } 1001 1002 public boolean hasPatient() { 1003 return this.patient != null && !this.patient.isEmpty(); 1004 } 1005 1006 /** 1007 * @param value {@link #patient} (The person who this history concerns.) 1008 */ 1009 public FamilyMemberHistory setPatient(Reference value) { 1010 this.patient = value; 1011 return this; 1012 } 1013 1014 /** 1015 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person who this history concerns.) 1016 */ 1017 public Patient getPatientTarget() { 1018 if (this.patientTarget == null) 1019 if (Configuration.errorOnAutoCreate()) 1020 throw new Error("Attempt to auto-create FamilyMemberHistory.patient"); 1021 else if (Configuration.doAutoCreate()) 1022 this.patientTarget = new Patient(); // aa 1023 return this.patientTarget; 1024 } 1025 1026 /** 1027 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person who this history concerns.) 1028 */ 1029 public FamilyMemberHistory setPatientTarget(Patient value) { 1030 this.patientTarget = value; 1031 return this; 1032 } 1033 1034 /** 1035 * @return {@link #date} (The date (and possibly time) when the family member history was taken.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1036 */ 1037 public DateTimeType getDateElement() { 1038 if (this.date == null) 1039 if (Configuration.errorOnAutoCreate()) 1040 throw new Error("Attempt to auto-create FamilyMemberHistory.date"); 1041 else if (Configuration.doAutoCreate()) 1042 this.date = new DateTimeType(); // bb 1043 return this.date; 1044 } 1045 1046 public boolean hasDateElement() { 1047 return this.date != null && !this.date.isEmpty(); 1048 } 1049 1050 public boolean hasDate() { 1051 return this.date != null && !this.date.isEmpty(); 1052 } 1053 1054 /** 1055 * @param value {@link #date} (The date (and possibly time) when the family member history was taken.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1056 */ 1057 public FamilyMemberHistory setDateElement(DateTimeType value) { 1058 this.date = value; 1059 return this; 1060 } 1061 1062 /** 1063 * @return The date (and possibly time) when the family member history was taken. 1064 */ 1065 public Date getDate() { 1066 return this.date == null ? null : this.date.getValue(); 1067 } 1068 1069 /** 1070 * @param value The date (and possibly time) when the family member history was taken. 1071 */ 1072 public FamilyMemberHistory setDate(Date value) { 1073 if (value == null) 1074 this.date = null; 1075 else { 1076 if (this.date == null) 1077 this.date = new DateTimeType(); 1078 this.date.setValue(value); 1079 } 1080 return this; 1081 } 1082 1083 /** 1084 * @return {@link #name} (This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair".). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1085 */ 1086 public StringType getNameElement() { 1087 if (this.name == null) 1088 if (Configuration.errorOnAutoCreate()) 1089 throw new Error("Attempt to auto-create FamilyMemberHistory.name"); 1090 else if (Configuration.doAutoCreate()) 1091 this.name = new StringType(); // bb 1092 return this.name; 1093 } 1094 1095 public boolean hasNameElement() { 1096 return this.name != null && !this.name.isEmpty(); 1097 } 1098 1099 public boolean hasName() { 1100 return this.name != null && !this.name.isEmpty(); 1101 } 1102 1103 /** 1104 * @param value {@link #name} (This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair".). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1105 */ 1106 public FamilyMemberHistory setNameElement(StringType value) { 1107 this.name = value; 1108 return this; 1109 } 1110 1111 /** 1112 * @return This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair". 1113 */ 1114 public String getName() { 1115 return this.name == null ? null : this.name.getValue(); 1116 } 1117 1118 /** 1119 * @param value This will either be a name or a description; e.g. "Aunt Susan", "my cousin with the red hair". 1120 */ 1121 public FamilyMemberHistory setName(String value) { 1122 if (Utilities.noString(value)) 1123 this.name = null; 1124 else { 1125 if (this.name == null) 1126 this.name = new StringType(); 1127 this.name.setValue(value); 1128 } 1129 return this; 1130 } 1131 1132 /** 1133 * @return {@link #relationship} (The type of relationship this person has to the patient (father, mother, brother etc.).) 1134 */ 1135 public CodeableConcept getRelationship() { 1136 if (this.relationship == null) 1137 if (Configuration.errorOnAutoCreate()) 1138 throw new Error("Attempt to auto-create FamilyMemberHistory.relationship"); 1139 else if (Configuration.doAutoCreate()) 1140 this.relationship = new CodeableConcept(); // cc 1141 return this.relationship; 1142 } 1143 1144 public boolean hasRelationship() { 1145 return this.relationship != null && !this.relationship.isEmpty(); 1146 } 1147 1148 /** 1149 * @param value {@link #relationship} (The type of relationship this person has to the patient (father, mother, brother etc.).) 1150 */ 1151 public FamilyMemberHistory setRelationship(CodeableConcept value) { 1152 this.relationship = value; 1153 return this; 1154 } 1155 1156 /** 1157 * @return {@link #gender} (Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 1158 */ 1159 public Enumeration<AdministrativeGender> getGenderElement() { 1160 if (this.gender == null) 1161 if (Configuration.errorOnAutoCreate()) 1162 throw new Error("Attempt to auto-create FamilyMemberHistory.gender"); 1163 else if (Configuration.doAutoCreate()) 1164 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); // bb 1165 return this.gender; 1166 } 1167 1168 public boolean hasGenderElement() { 1169 return this.gender != null && !this.gender.isEmpty(); 1170 } 1171 1172 public boolean hasGender() { 1173 return this.gender != null && !this.gender.isEmpty(); 1174 } 1175 1176 /** 1177 * @param value {@link #gender} (Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes.). This is the underlying object with id, value and extensions. The accessor "getGender" gives direct access to the value 1178 */ 1179 public FamilyMemberHistory setGenderElement(Enumeration<AdministrativeGender> value) { 1180 this.gender = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes. 1186 */ 1187 public AdministrativeGender getGender() { 1188 return this.gender == null ? null : this.gender.getValue(); 1189 } 1190 1191 /** 1192 * @param value Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes. 1193 */ 1194 public FamilyMemberHistory setGender(AdministrativeGender value) { 1195 if (value == null) 1196 this.gender = null; 1197 else { 1198 if (this.gender == null) 1199 this.gender = new Enumeration<AdministrativeGender>(new AdministrativeGenderEnumFactory()); 1200 this.gender.setValue(value); 1201 } 1202 return this; 1203 } 1204 1205 /** 1206 * @return {@link #born} (The actual or approximate date of birth of the relative.) 1207 */ 1208 public Type getBorn() { 1209 return this.born; 1210 } 1211 1212 /** 1213 * @return {@link #born} (The actual or approximate date of birth of the relative.) 1214 */ 1215 public Period getBornPeriod() throws FHIRException { 1216 if (this.born == null) 1217 return null; 1218 if (!(this.born instanceof Period)) 1219 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.born.getClass().getName()+" was encountered"); 1220 return (Period) this.born; 1221 } 1222 1223 public boolean hasBornPeriod() { 1224 return this != null && this.born instanceof Period; 1225 } 1226 1227 /** 1228 * @return {@link #born} (The actual or approximate date of birth of the relative.) 1229 */ 1230 public DateType getBornDateType() throws FHIRException { 1231 if (this.born == null) 1232 return null; 1233 if (!(this.born instanceof DateType)) 1234 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.born.getClass().getName()+" was encountered"); 1235 return (DateType) this.born; 1236 } 1237 1238 public boolean hasBornDateType() { 1239 return this != null && this.born instanceof DateType; 1240 } 1241 1242 /** 1243 * @return {@link #born} (The actual or approximate date of birth of the relative.) 1244 */ 1245 public StringType getBornStringType() throws FHIRException { 1246 if (this.born == null) 1247 return null; 1248 if (!(this.born instanceof StringType)) 1249 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.born.getClass().getName()+" was encountered"); 1250 return (StringType) this.born; 1251 } 1252 1253 public boolean hasBornStringType() { 1254 return this != null && this.born instanceof StringType; 1255 } 1256 1257 public boolean hasBorn() { 1258 return this.born != null && !this.born.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #born} (The actual or approximate date of birth of the relative.) 1263 */ 1264 public FamilyMemberHistory setBorn(Type value) throws FHIRFormatError { 1265 if (value != null && !(value instanceof Period || value instanceof DateType || value instanceof StringType)) 1266 throw new FHIRFormatError("Not the right type for FamilyMemberHistory.born[x]: "+value.fhirType()); 1267 this.born = value; 1268 return this; 1269 } 1270 1271 /** 1272 * @return {@link #age} (The age of the relative at the time the family member history is recorded.) 1273 */ 1274 public Type getAge() { 1275 return this.age; 1276 } 1277 1278 /** 1279 * @return {@link #age} (The age of the relative at the time the family member history is recorded.) 1280 */ 1281 public Age getAgeAge() throws FHIRException { 1282 if (this.age == null) 1283 return null; 1284 if (!(this.age instanceof Age)) 1285 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.age.getClass().getName()+" was encountered"); 1286 return (Age) this.age; 1287 } 1288 1289 public boolean hasAgeAge() { 1290 return this != null && this.age instanceof Age; 1291 } 1292 1293 /** 1294 * @return {@link #age} (The age of the relative at the time the family member history is recorded.) 1295 */ 1296 public Range getAgeRange() throws FHIRException { 1297 if (this.age == null) 1298 return null; 1299 if (!(this.age instanceof Range)) 1300 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.age.getClass().getName()+" was encountered"); 1301 return (Range) this.age; 1302 } 1303 1304 public boolean hasAgeRange() { 1305 return this != null && this.age instanceof Range; 1306 } 1307 1308 /** 1309 * @return {@link #age} (The age of the relative at the time the family member history is recorded.) 1310 */ 1311 public StringType getAgeStringType() throws FHIRException { 1312 if (this.age == null) 1313 return null; 1314 if (!(this.age instanceof StringType)) 1315 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.age.getClass().getName()+" was encountered"); 1316 return (StringType) this.age; 1317 } 1318 1319 public boolean hasAgeStringType() { 1320 return this != null && this.age instanceof StringType; 1321 } 1322 1323 public boolean hasAge() { 1324 return this.age != null && !this.age.isEmpty(); 1325 } 1326 1327 /** 1328 * @param value {@link #age} (The age of the relative at the time the family member history is recorded.) 1329 */ 1330 public FamilyMemberHistory setAge(Type value) throws FHIRFormatError { 1331 if (value != null && !(value instanceof Age || value instanceof Range || value instanceof StringType)) 1332 throw new FHIRFormatError("Not the right type for FamilyMemberHistory.age[x]: "+value.fhirType()); 1333 this.age = value; 1334 return this; 1335 } 1336 1337 /** 1338 * @return {@link #estimatedAge} (If true, indicates that the age value specified is an estimated value.). This is the underlying object with id, value and extensions. The accessor "getEstimatedAge" gives direct access to the value 1339 */ 1340 public BooleanType getEstimatedAgeElement() { 1341 if (this.estimatedAge == null) 1342 if (Configuration.errorOnAutoCreate()) 1343 throw new Error("Attempt to auto-create FamilyMemberHistory.estimatedAge"); 1344 else if (Configuration.doAutoCreate()) 1345 this.estimatedAge = new BooleanType(); // bb 1346 return this.estimatedAge; 1347 } 1348 1349 public boolean hasEstimatedAgeElement() { 1350 return this.estimatedAge != null && !this.estimatedAge.isEmpty(); 1351 } 1352 1353 public boolean hasEstimatedAge() { 1354 return this.estimatedAge != null && !this.estimatedAge.isEmpty(); 1355 } 1356 1357 /** 1358 * @param value {@link #estimatedAge} (If true, indicates that the age value specified is an estimated value.). This is the underlying object with id, value and extensions. The accessor "getEstimatedAge" gives direct access to the value 1359 */ 1360 public FamilyMemberHistory setEstimatedAgeElement(BooleanType value) { 1361 this.estimatedAge = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return If true, indicates that the age value specified is an estimated value. 1367 */ 1368 public boolean getEstimatedAge() { 1369 return this.estimatedAge == null || this.estimatedAge.isEmpty() ? false : this.estimatedAge.getValue(); 1370 } 1371 1372 /** 1373 * @param value If true, indicates that the age value specified is an estimated value. 1374 */ 1375 public FamilyMemberHistory setEstimatedAge(boolean value) { 1376 if (this.estimatedAge == null) 1377 this.estimatedAge = new BooleanType(); 1378 this.estimatedAge.setValue(value); 1379 return this; 1380 } 1381 1382 /** 1383 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 1384 */ 1385 public Type getDeceased() { 1386 return this.deceased; 1387 } 1388 1389 /** 1390 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 1391 */ 1392 public BooleanType getDeceasedBooleanType() throws FHIRException { 1393 if (this.deceased == null) 1394 return null; 1395 if (!(this.deceased instanceof BooleanType)) 1396 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1397 return (BooleanType) this.deceased; 1398 } 1399 1400 public boolean hasDeceasedBooleanType() { 1401 return this != null && this.deceased instanceof BooleanType; 1402 } 1403 1404 /** 1405 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 1406 */ 1407 public Age getDeceasedAge() throws FHIRException { 1408 if (this.deceased == null) 1409 return null; 1410 if (!(this.deceased instanceof Age)) 1411 throw new FHIRException("Type mismatch: the type Age was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1412 return (Age) this.deceased; 1413 } 1414 1415 public boolean hasDeceasedAge() { 1416 return this != null && this.deceased instanceof Age; 1417 } 1418 1419 /** 1420 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 1421 */ 1422 public Range getDeceasedRange() throws FHIRException { 1423 if (this.deceased == null) 1424 return null; 1425 if (!(this.deceased instanceof Range)) 1426 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1427 return (Range) this.deceased; 1428 } 1429 1430 public boolean hasDeceasedRange() { 1431 return this != null && this.deceased instanceof Range; 1432 } 1433 1434 /** 1435 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 1436 */ 1437 public DateType getDeceasedDateType() throws FHIRException { 1438 if (this.deceased == null) 1439 return null; 1440 if (!(this.deceased instanceof DateType)) 1441 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1442 return (DateType) this.deceased; 1443 } 1444 1445 public boolean hasDeceasedDateType() { 1446 return this != null && this.deceased instanceof DateType; 1447 } 1448 1449 /** 1450 * @return {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 1451 */ 1452 public StringType getDeceasedStringType() throws FHIRException { 1453 if (this.deceased == null) 1454 return null; 1455 if (!(this.deceased instanceof StringType)) 1456 throw new FHIRException("Type mismatch: the type StringType was expected, but "+this.deceased.getClass().getName()+" was encountered"); 1457 return (StringType) this.deceased; 1458 } 1459 1460 public boolean hasDeceasedStringType() { 1461 return this != null && this.deceased instanceof StringType; 1462 } 1463 1464 public boolean hasDeceased() { 1465 return this.deceased != null && !this.deceased.isEmpty(); 1466 } 1467 1468 /** 1469 * @param value {@link #deceased} (Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.) 1470 */ 1471 public FamilyMemberHistory setDeceased(Type value) throws FHIRFormatError { 1472 if (value != null && !(value instanceof BooleanType || value instanceof Age || value instanceof Range || value instanceof DateType || value instanceof StringType)) 1473 throw new FHIRFormatError("Not the right type for FamilyMemberHistory.deceased[x]: "+value.fhirType()); 1474 this.deceased = value; 1475 return this; 1476 } 1477 1478 /** 1479 * @return {@link #reasonCode} (Describes why the family member history occurred in coded or textual form.) 1480 */ 1481 public List<CodeableConcept> getReasonCode() { 1482 if (this.reasonCode == null) 1483 this.reasonCode = new ArrayList<CodeableConcept>(); 1484 return this.reasonCode; 1485 } 1486 1487 /** 1488 * @return Returns a reference to <code>this</code> for easy method chaining 1489 */ 1490 public FamilyMemberHistory setReasonCode(List<CodeableConcept> theReasonCode) { 1491 this.reasonCode = theReasonCode; 1492 return this; 1493 } 1494 1495 public boolean hasReasonCode() { 1496 if (this.reasonCode == null) 1497 return false; 1498 for (CodeableConcept item : this.reasonCode) 1499 if (!item.isEmpty()) 1500 return true; 1501 return false; 1502 } 1503 1504 public CodeableConcept addReasonCode() { //3 1505 CodeableConcept t = new CodeableConcept(); 1506 if (this.reasonCode == null) 1507 this.reasonCode = new ArrayList<CodeableConcept>(); 1508 this.reasonCode.add(t); 1509 return t; 1510 } 1511 1512 public FamilyMemberHistory addReasonCode(CodeableConcept t) { //3 1513 if (t == null) 1514 return this; 1515 if (this.reasonCode == null) 1516 this.reasonCode = new ArrayList<CodeableConcept>(); 1517 this.reasonCode.add(t); 1518 return this; 1519 } 1520 1521 /** 1522 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1523 */ 1524 public CodeableConcept getReasonCodeFirstRep() { 1525 if (getReasonCode().isEmpty()) { 1526 addReasonCode(); 1527 } 1528 return getReasonCode().get(0); 1529 } 1530 1531 /** 1532 * @return {@link #reasonReference} (Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.) 1533 */ 1534 public List<Reference> getReasonReference() { 1535 if (this.reasonReference == null) 1536 this.reasonReference = new ArrayList<Reference>(); 1537 return this.reasonReference; 1538 } 1539 1540 /** 1541 * @return Returns a reference to <code>this</code> for easy method chaining 1542 */ 1543 public FamilyMemberHistory setReasonReference(List<Reference> theReasonReference) { 1544 this.reasonReference = theReasonReference; 1545 return this; 1546 } 1547 1548 public boolean hasReasonReference() { 1549 if (this.reasonReference == null) 1550 return false; 1551 for (Reference item : this.reasonReference) 1552 if (!item.isEmpty()) 1553 return true; 1554 return false; 1555 } 1556 1557 public Reference addReasonReference() { //3 1558 Reference t = new Reference(); 1559 if (this.reasonReference == null) 1560 this.reasonReference = new ArrayList<Reference>(); 1561 this.reasonReference.add(t); 1562 return t; 1563 } 1564 1565 public FamilyMemberHistory addReasonReference(Reference t) { //3 1566 if (t == null) 1567 return this; 1568 if (this.reasonReference == null) 1569 this.reasonReference = new ArrayList<Reference>(); 1570 this.reasonReference.add(t); 1571 return this; 1572 } 1573 1574 /** 1575 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1576 */ 1577 public Reference getReasonReferenceFirstRep() { 1578 if (getReasonReference().isEmpty()) { 1579 addReasonReference(); 1580 } 1581 return getReasonReference().get(0); 1582 } 1583 1584 /** 1585 * @deprecated Use Reference#setResource(IBaseResource) instead 1586 */ 1587 @Deprecated 1588 public List<Resource> getReasonReferenceTarget() { 1589 if (this.reasonReferenceTarget == null) 1590 this.reasonReferenceTarget = new ArrayList<Resource>(); 1591 return this.reasonReferenceTarget; 1592 } 1593 1594 /** 1595 * @return {@link #note} (This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.) 1596 */ 1597 public List<Annotation> getNote() { 1598 if (this.note == null) 1599 this.note = new ArrayList<Annotation>(); 1600 return this.note; 1601 } 1602 1603 /** 1604 * @return Returns a reference to <code>this</code> for easy method chaining 1605 */ 1606 public FamilyMemberHistory setNote(List<Annotation> theNote) { 1607 this.note = theNote; 1608 return this; 1609 } 1610 1611 public boolean hasNote() { 1612 if (this.note == null) 1613 return false; 1614 for (Annotation item : this.note) 1615 if (!item.isEmpty()) 1616 return true; 1617 return false; 1618 } 1619 1620 public Annotation addNote() { //3 1621 Annotation t = new Annotation(); 1622 if (this.note == null) 1623 this.note = new ArrayList<Annotation>(); 1624 this.note.add(t); 1625 return t; 1626 } 1627 1628 public FamilyMemberHistory addNote(Annotation t) { //3 1629 if (t == null) 1630 return this; 1631 if (this.note == null) 1632 this.note = new ArrayList<Annotation>(); 1633 this.note.add(t); 1634 return this; 1635 } 1636 1637 /** 1638 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1639 */ 1640 public Annotation getNoteFirstRep() { 1641 if (getNote().isEmpty()) { 1642 addNote(); 1643 } 1644 return getNote().get(0); 1645 } 1646 1647 /** 1648 * @return {@link #condition} (The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.) 1649 */ 1650 public List<FamilyMemberHistoryConditionComponent> getCondition() { 1651 if (this.condition == null) 1652 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 1653 return this.condition; 1654 } 1655 1656 /** 1657 * @return Returns a reference to <code>this</code> for easy method chaining 1658 */ 1659 public FamilyMemberHistory setCondition(List<FamilyMemberHistoryConditionComponent> theCondition) { 1660 this.condition = theCondition; 1661 return this; 1662 } 1663 1664 public boolean hasCondition() { 1665 if (this.condition == null) 1666 return false; 1667 for (FamilyMemberHistoryConditionComponent item : this.condition) 1668 if (!item.isEmpty()) 1669 return true; 1670 return false; 1671 } 1672 1673 public FamilyMemberHistoryConditionComponent addCondition() { //3 1674 FamilyMemberHistoryConditionComponent t = new FamilyMemberHistoryConditionComponent(); 1675 if (this.condition == null) 1676 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 1677 this.condition.add(t); 1678 return t; 1679 } 1680 1681 public FamilyMemberHistory addCondition(FamilyMemberHistoryConditionComponent t) { //3 1682 if (t == null) 1683 return this; 1684 if (this.condition == null) 1685 this.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 1686 this.condition.add(t); 1687 return this; 1688 } 1689 1690 /** 1691 * @return The first repetition of repeating field {@link #condition}, creating it if it does not already exist 1692 */ 1693 public FamilyMemberHistoryConditionComponent getConditionFirstRep() { 1694 if (getCondition().isEmpty()) { 1695 addCondition(); 1696 } 1697 return getCondition().get(0); 1698 } 1699 1700 protected void listChildren(List<Property> children) { 1701 super.listChildren(children); 1702 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this family member history record that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 1703 children.add(new Property("definition", "Reference(PlanDefinition|Questionnaire)", "A protocol or questionnaire that was adhered to in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, definition)); 1704 children.add(new Property("status", "code", "A code specifying the status of the record of the family history of a specific family member.", 0, 1, status)); 1705 children.add(new Property("notDone", "boolean", "If true, indicates the taking of an individual family member's history did not occur. The notDone element should not be used to document negated conditions, such as a family member that did not have a condition.", 0, 1, notDone)); 1706 children.add(new Property("notDoneReason", "CodeableConcept", "Describes why the family member's history is absent.", 0, 1, notDoneReason)); 1707 children.add(new Property("patient", "Reference(Patient)", "The person who this history concerns.", 0, 1, patient)); 1708 children.add(new Property("date", "dateTime", "The date (and possibly time) when the family member history was taken.", 0, 1, date)); 1709 children.add(new Property("name", "string", "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".", 0, 1, name)); 1710 children.add(new Property("relationship", "CodeableConcept", "The type of relationship this person has to the patient (father, mother, brother etc.).", 0, 1, relationship)); 1711 children.add(new Property("gender", "code", "Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes.", 0, 1, gender)); 1712 children.add(new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born)); 1713 children.add(new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age)); 1714 children.add(new Property("estimatedAge", "boolean", "If true, indicates that the age value specified is an estimated value.", 0, 1, estimatedAge)); 1715 children.add(new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased)); 1716 children.add(new Property("reasonCode", "CodeableConcept", "Describes why the family member history occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1717 children.add(new Property("reasonReference", "Reference(Condition|Observation|AllergyIntolerance|QuestionnaireResponse)", "Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1718 children.add(new Property("note", "Annotation", "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.", 0, java.lang.Integer.MAX_VALUE, note)); 1719 children.add(new Property("condition", "", "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.", 0, java.lang.Integer.MAX_VALUE, condition)); 1720 } 1721 1722 @Override 1723 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1724 switch (_hash) { 1725 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this family member history record that are defined by business processes and/ or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 1726 case -1014418093: /*definition*/ return new Property("definition", "Reference(PlanDefinition|Questionnaire)", "A protocol or questionnaire that was adhered to in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, definition); 1727 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the status of the record of the family history of a specific family member.", 0, 1, status); 1728 case 2128257269: /*notDone*/ return new Property("notDone", "boolean", "If true, indicates the taking of an individual family member's history did not occur. The notDone element should not be used to document negated conditions, such as a family member that did not have a condition.", 0, 1, notDone); 1729 case -1973169255: /*notDoneReason*/ return new Property("notDoneReason", "CodeableConcept", "Describes why the family member's history is absent.", 0, 1, notDoneReason); 1730 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The person who this history concerns.", 0, 1, patient); 1731 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and possibly time) when the family member history was taken.", 0, 1, date); 1732 case 3373707: /*name*/ return new Property("name", "string", "This will either be a name or a description; e.g. \"Aunt Susan\", \"my cousin with the red hair\".", 0, 1, name); 1733 case -261851592: /*relationship*/ return new Property("relationship", "CodeableConcept", "The type of relationship this person has to the patient (father, mother, brother etc.).", 0, 1, relationship); 1734 case -1249512767: /*gender*/ return new Property("gender", "code", "Administrative Gender - the gender that the relative is considered to have for administration and record keeping purposes.", 0, 1, gender); 1735 case 67532951: /*born[x]*/ return new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born); 1736 case 3029833: /*born*/ return new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born); 1737 case 1497711210: /*bornPeriod*/ return new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born); 1738 case 2092814999: /*bornDate*/ return new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born); 1739 case 1597451450: /*bornString*/ return new Property("born[x]", "Period|date|string", "The actual or approximate date of birth of the relative.", 0, 1, born); 1740 case -1419716831: /*age[x]*/ return new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 1741 case 96511: /*age*/ return new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 1742 case -1419742336: /*ageAge*/ return new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 1743 case 1442748286: /*ageRange*/ return new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 1744 case 1821821424: /*ageString*/ return new Property("age[x]", "Age|Range|string", "The age of the relative at the time the family member history is recorded.", 0, 1, age); 1745 case 2130167587: /*estimatedAge*/ return new Property("estimatedAge", "boolean", "If true, indicates that the age value specified is an estimated value.", 0, 1, estimatedAge); 1746 case -1311442804: /*deceased[x]*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 1747 case 561497972: /*deceased*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 1748 case 497463828: /*deceasedBoolean*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 1749 case -1311468309: /*deceasedAge*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 1750 case -1880094167: /*deceasedRange*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 1751 case -2000727742: /*deceasedDate*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 1752 case 1892920485: /*deceasedString*/ return new Property("deceased[x]", "boolean|Age|Range|date|string", "Deceased flag or the actual or approximate age of the relative at the time of death for the family member history record.", 0, 1, deceased); 1753 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Describes why the family member history occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1754 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation|AllergyIntolerance|QuestionnaireResponse)", "Indicates a Condition, Observation, AllergyIntolerance, or QuestionnaireResponse that justifies this family member history event.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1755 case 3387378: /*note*/ return new Property("note", "Annotation", "This property allows a non condition-specific note to the made about the related person. Ideally, the note would be in the condition property, but this is not always possible.", 0, java.lang.Integer.MAX_VALUE, note); 1756 case -861311717: /*condition*/ return new Property("condition", "", "The significant Conditions (or condition) that the family member had. This is a repeating section to allow a system to represent more than one condition per resource, though there is nothing stopping multiple resources - one per condition.", 0, java.lang.Integer.MAX_VALUE, condition); 1757 default: return super.getNamedProperty(_hash, _name, _checkValid); 1758 } 1759 1760 } 1761 1762 @Override 1763 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1764 switch (hash) { 1765 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1766 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 1767 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FamilyHistoryStatus> 1768 case 2128257269: /*notDone*/ return this.notDone == null ? new Base[0] : new Base[] {this.notDone}; // BooleanType 1769 case -1973169255: /*notDoneReason*/ return this.notDoneReason == null ? new Base[0] : new Base[] {this.notDoneReason}; // CodeableConcept 1770 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1771 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1772 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1773 case -261851592: /*relationship*/ return this.relationship == null ? new Base[0] : new Base[] {this.relationship}; // CodeableConcept 1774 case -1249512767: /*gender*/ return this.gender == null ? new Base[0] : new Base[] {this.gender}; // Enumeration<AdministrativeGender> 1775 case 3029833: /*born*/ return this.born == null ? new Base[0] : new Base[] {this.born}; // Type 1776 case 96511: /*age*/ return this.age == null ? new Base[0] : new Base[] {this.age}; // Type 1777 case 2130167587: /*estimatedAge*/ return this.estimatedAge == null ? new Base[0] : new Base[] {this.estimatedAge}; // BooleanType 1778 case 561497972: /*deceased*/ return this.deceased == null ? new Base[0] : new Base[] {this.deceased}; // Type 1779 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1780 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1781 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1782 case -861311717: /*condition*/ return this.condition == null ? new Base[0] : this.condition.toArray(new Base[this.condition.size()]); // FamilyMemberHistoryConditionComponent 1783 default: return super.getProperty(hash, name, checkValid); 1784 } 1785 1786 } 1787 1788 @Override 1789 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1790 switch (hash) { 1791 case -1618432855: // identifier 1792 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1793 return value; 1794 case -1014418093: // definition 1795 this.getDefinition().add(castToReference(value)); // Reference 1796 return value; 1797 case -892481550: // status 1798 value = new FamilyHistoryStatusEnumFactory().fromType(castToCode(value)); 1799 this.status = (Enumeration) value; // Enumeration<FamilyHistoryStatus> 1800 return value; 1801 case 2128257269: // notDone 1802 this.notDone = castToBoolean(value); // BooleanType 1803 return value; 1804 case -1973169255: // notDoneReason 1805 this.notDoneReason = castToCodeableConcept(value); // CodeableConcept 1806 return value; 1807 case -791418107: // patient 1808 this.patient = castToReference(value); // Reference 1809 return value; 1810 case 3076014: // date 1811 this.date = castToDateTime(value); // DateTimeType 1812 return value; 1813 case 3373707: // name 1814 this.name = castToString(value); // StringType 1815 return value; 1816 case -261851592: // relationship 1817 this.relationship = castToCodeableConcept(value); // CodeableConcept 1818 return value; 1819 case -1249512767: // gender 1820 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1821 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1822 return value; 1823 case 3029833: // born 1824 this.born = castToType(value); // Type 1825 return value; 1826 case 96511: // age 1827 this.age = castToType(value); // Type 1828 return value; 1829 case 2130167587: // estimatedAge 1830 this.estimatedAge = castToBoolean(value); // BooleanType 1831 return value; 1832 case 561497972: // deceased 1833 this.deceased = castToType(value); // Type 1834 return value; 1835 case 722137681: // reasonCode 1836 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1837 return value; 1838 case -1146218137: // reasonReference 1839 this.getReasonReference().add(castToReference(value)); // Reference 1840 return value; 1841 case 3387378: // note 1842 this.getNote().add(castToAnnotation(value)); // Annotation 1843 return value; 1844 case -861311717: // condition 1845 this.getCondition().add((FamilyMemberHistoryConditionComponent) value); // FamilyMemberHistoryConditionComponent 1846 return value; 1847 default: return super.setProperty(hash, name, value); 1848 } 1849 1850 } 1851 1852 @Override 1853 public Base setProperty(String name, Base value) throws FHIRException { 1854 if (name.equals("identifier")) { 1855 this.getIdentifier().add(castToIdentifier(value)); 1856 } else if (name.equals("definition")) { 1857 this.getDefinition().add(castToReference(value)); 1858 } else if (name.equals("status")) { 1859 value = new FamilyHistoryStatusEnumFactory().fromType(castToCode(value)); 1860 this.status = (Enumeration) value; // Enumeration<FamilyHistoryStatus> 1861 } else if (name.equals("notDone")) { 1862 this.notDone = castToBoolean(value); // BooleanType 1863 } else if (name.equals("notDoneReason")) { 1864 this.notDoneReason = castToCodeableConcept(value); // CodeableConcept 1865 } else if (name.equals("patient")) { 1866 this.patient = castToReference(value); // Reference 1867 } else if (name.equals("date")) { 1868 this.date = castToDateTime(value); // DateTimeType 1869 } else if (name.equals("name")) { 1870 this.name = castToString(value); // StringType 1871 } else if (name.equals("relationship")) { 1872 this.relationship = castToCodeableConcept(value); // CodeableConcept 1873 } else if (name.equals("gender")) { 1874 value = new AdministrativeGenderEnumFactory().fromType(castToCode(value)); 1875 this.gender = (Enumeration) value; // Enumeration<AdministrativeGender> 1876 } else if (name.equals("born[x]")) { 1877 this.born = castToType(value); // Type 1878 } else if (name.equals("age[x]")) { 1879 this.age = castToType(value); // Type 1880 } else if (name.equals("estimatedAge")) { 1881 this.estimatedAge = castToBoolean(value); // BooleanType 1882 } else if (name.equals("deceased[x]")) { 1883 this.deceased = castToType(value); // Type 1884 } else if (name.equals("reasonCode")) { 1885 this.getReasonCode().add(castToCodeableConcept(value)); 1886 } else if (name.equals("reasonReference")) { 1887 this.getReasonReference().add(castToReference(value)); 1888 } else if (name.equals("note")) { 1889 this.getNote().add(castToAnnotation(value)); 1890 } else if (name.equals("condition")) { 1891 this.getCondition().add((FamilyMemberHistoryConditionComponent) value); 1892 } else 1893 return super.setProperty(name, value); 1894 return value; 1895 } 1896 1897 @Override 1898 public Base makeProperty(int hash, String name) throws FHIRException { 1899 switch (hash) { 1900 case -1618432855: return addIdentifier(); 1901 case -1014418093: return addDefinition(); 1902 case -892481550: return getStatusElement(); 1903 case 2128257269: return getNotDoneElement(); 1904 case -1973169255: return getNotDoneReason(); 1905 case -791418107: return getPatient(); 1906 case 3076014: return getDateElement(); 1907 case 3373707: return getNameElement(); 1908 case -261851592: return getRelationship(); 1909 case -1249512767: return getGenderElement(); 1910 case 67532951: return getBorn(); 1911 case 3029833: return getBorn(); 1912 case -1419716831: return getAge(); 1913 case 96511: return getAge(); 1914 case 2130167587: return getEstimatedAgeElement(); 1915 case -1311442804: return getDeceased(); 1916 case 561497972: return getDeceased(); 1917 case 722137681: return addReasonCode(); 1918 case -1146218137: return addReasonReference(); 1919 case 3387378: return addNote(); 1920 case -861311717: return addCondition(); 1921 default: return super.makeProperty(hash, name); 1922 } 1923 1924 } 1925 1926 @Override 1927 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1928 switch (hash) { 1929 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1930 case -1014418093: /*definition*/ return new String[] {"Reference"}; 1931 case -892481550: /*status*/ return new String[] {"code"}; 1932 case 2128257269: /*notDone*/ return new String[] {"boolean"}; 1933 case -1973169255: /*notDoneReason*/ return new String[] {"CodeableConcept"}; 1934 case -791418107: /*patient*/ return new String[] {"Reference"}; 1935 case 3076014: /*date*/ return new String[] {"dateTime"}; 1936 case 3373707: /*name*/ return new String[] {"string"}; 1937 case -261851592: /*relationship*/ return new String[] {"CodeableConcept"}; 1938 case -1249512767: /*gender*/ return new String[] {"code"}; 1939 case 3029833: /*born*/ return new String[] {"Period", "date", "string"}; 1940 case 96511: /*age*/ return new String[] {"Age", "Range", "string"}; 1941 case 2130167587: /*estimatedAge*/ return new String[] {"boolean"}; 1942 case 561497972: /*deceased*/ return new String[] {"boolean", "Age", "Range", "date", "string"}; 1943 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 1944 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 1945 case 3387378: /*note*/ return new String[] {"Annotation"}; 1946 case -861311717: /*condition*/ return new String[] {}; 1947 default: return super.getTypesForProperty(hash, name); 1948 } 1949 1950 } 1951 1952 @Override 1953 public Base addChild(String name) throws FHIRException { 1954 if (name.equals("identifier")) { 1955 return addIdentifier(); 1956 } 1957 else if (name.equals("definition")) { 1958 return addDefinition(); 1959 } 1960 else if (name.equals("status")) { 1961 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.status"); 1962 } 1963 else if (name.equals("notDone")) { 1964 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.notDone"); 1965 } 1966 else if (name.equals("notDoneReason")) { 1967 this.notDoneReason = new CodeableConcept(); 1968 return this.notDoneReason; 1969 } 1970 else if (name.equals("patient")) { 1971 this.patient = new Reference(); 1972 return this.patient; 1973 } 1974 else if (name.equals("date")) { 1975 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.date"); 1976 } 1977 else if (name.equals("name")) { 1978 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.name"); 1979 } 1980 else if (name.equals("relationship")) { 1981 this.relationship = new CodeableConcept(); 1982 return this.relationship; 1983 } 1984 else if (name.equals("gender")) { 1985 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.gender"); 1986 } 1987 else if (name.equals("bornPeriod")) { 1988 this.born = new Period(); 1989 return this.born; 1990 } 1991 else if (name.equals("bornDate")) { 1992 this.born = new DateType(); 1993 return this.born; 1994 } 1995 else if (name.equals("bornString")) { 1996 this.born = new StringType(); 1997 return this.born; 1998 } 1999 else if (name.equals("ageAge")) { 2000 this.age = new Age(); 2001 return this.age; 2002 } 2003 else if (name.equals("ageRange")) { 2004 this.age = new Range(); 2005 return this.age; 2006 } 2007 else if (name.equals("ageString")) { 2008 this.age = new StringType(); 2009 return this.age; 2010 } 2011 else if (name.equals("estimatedAge")) { 2012 throw new FHIRException("Cannot call addChild on a singleton property FamilyMemberHistory.estimatedAge"); 2013 } 2014 else if (name.equals("deceasedBoolean")) { 2015 this.deceased = new BooleanType(); 2016 return this.deceased; 2017 } 2018 else if (name.equals("deceasedAge")) { 2019 this.deceased = new Age(); 2020 return this.deceased; 2021 } 2022 else if (name.equals("deceasedRange")) { 2023 this.deceased = new Range(); 2024 return this.deceased; 2025 } 2026 else if (name.equals("deceasedDate")) { 2027 this.deceased = new DateType(); 2028 return this.deceased; 2029 } 2030 else if (name.equals("deceasedString")) { 2031 this.deceased = new StringType(); 2032 return this.deceased; 2033 } 2034 else if (name.equals("reasonCode")) { 2035 return addReasonCode(); 2036 } 2037 else if (name.equals("reasonReference")) { 2038 return addReasonReference(); 2039 } 2040 else if (name.equals("note")) { 2041 return addNote(); 2042 } 2043 else if (name.equals("condition")) { 2044 return addCondition(); 2045 } 2046 else 2047 return super.addChild(name); 2048 } 2049 2050 public String fhirType() { 2051 return "FamilyMemberHistory"; 2052 2053 } 2054 2055 public FamilyMemberHistory copy() { 2056 FamilyMemberHistory dst = new FamilyMemberHistory(); 2057 copyValues(dst); 2058 if (identifier != null) { 2059 dst.identifier = new ArrayList<Identifier>(); 2060 for (Identifier i : identifier) 2061 dst.identifier.add(i.copy()); 2062 }; 2063 if (definition != null) { 2064 dst.definition = new ArrayList<Reference>(); 2065 for (Reference i : definition) 2066 dst.definition.add(i.copy()); 2067 }; 2068 dst.status = status == null ? null : status.copy(); 2069 dst.notDone = notDone == null ? null : notDone.copy(); 2070 dst.notDoneReason = notDoneReason == null ? null : notDoneReason.copy(); 2071 dst.patient = patient == null ? null : patient.copy(); 2072 dst.date = date == null ? null : date.copy(); 2073 dst.name = name == null ? null : name.copy(); 2074 dst.relationship = relationship == null ? null : relationship.copy(); 2075 dst.gender = gender == null ? null : gender.copy(); 2076 dst.born = born == null ? null : born.copy(); 2077 dst.age = age == null ? null : age.copy(); 2078 dst.estimatedAge = estimatedAge == null ? null : estimatedAge.copy(); 2079 dst.deceased = deceased == null ? null : deceased.copy(); 2080 if (reasonCode != null) { 2081 dst.reasonCode = new ArrayList<CodeableConcept>(); 2082 for (CodeableConcept i : reasonCode) 2083 dst.reasonCode.add(i.copy()); 2084 }; 2085 if (reasonReference != null) { 2086 dst.reasonReference = new ArrayList<Reference>(); 2087 for (Reference i : reasonReference) 2088 dst.reasonReference.add(i.copy()); 2089 }; 2090 if (note != null) { 2091 dst.note = new ArrayList<Annotation>(); 2092 for (Annotation i : note) 2093 dst.note.add(i.copy()); 2094 }; 2095 if (condition != null) { 2096 dst.condition = new ArrayList<FamilyMemberHistoryConditionComponent>(); 2097 for (FamilyMemberHistoryConditionComponent i : condition) 2098 dst.condition.add(i.copy()); 2099 }; 2100 return dst; 2101 } 2102 2103 protected FamilyMemberHistory typedCopy() { 2104 return copy(); 2105 } 2106 2107 @Override 2108 public boolean equalsDeep(Base other_) { 2109 if (!super.equalsDeep(other_)) 2110 return false; 2111 if (!(other_ instanceof FamilyMemberHistory)) 2112 return false; 2113 FamilyMemberHistory o = (FamilyMemberHistory) other_; 2114 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2115 && compareDeep(status, o.status, true) && compareDeep(notDone, o.notDone, true) && compareDeep(notDoneReason, o.notDoneReason, true) 2116 && compareDeep(patient, o.patient, true) && compareDeep(date, o.date, true) && compareDeep(name, o.name, true) 2117 && compareDeep(relationship, o.relationship, true) && compareDeep(gender, o.gender, true) && compareDeep(born, o.born, true) 2118 && compareDeep(age, o.age, true) && compareDeep(estimatedAge, o.estimatedAge, true) && compareDeep(deceased, o.deceased, true) 2119 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2120 && compareDeep(note, o.note, true) && compareDeep(condition, o.condition, true); 2121 } 2122 2123 @Override 2124 public boolean equalsShallow(Base other_) { 2125 if (!super.equalsShallow(other_)) 2126 return false; 2127 if (!(other_ instanceof FamilyMemberHistory)) 2128 return false; 2129 FamilyMemberHistory o = (FamilyMemberHistory) other_; 2130 return compareValues(status, o.status, true) && compareValues(notDone, o.notDone, true) && compareValues(date, o.date, true) 2131 && compareValues(name, o.name, true) && compareValues(gender, o.gender, true) && compareValues(estimatedAge, o.estimatedAge, true) 2132 ; 2133 } 2134 2135 public boolean isEmpty() { 2136 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, status 2137 , notDone, notDoneReason, patient, date, name, relationship, gender, born, age 2138 , estimatedAge, deceased, reasonCode, reasonReference, note, condition); 2139 } 2140 2141 @Override 2142 public ResourceType getResourceType() { 2143 return ResourceType.FamilyMemberHistory; 2144 } 2145 2146 /** 2147 * Search parameter: <b>date</b> 2148 * <p> 2149 * Description: <b>When history was captured/updated</b><br> 2150 * Type: <b>date</b><br> 2151 * Path: <b>FamilyMemberHistory.date</b><br> 2152 * </p> 2153 */ 2154 @SearchParamDefinition(name="date", path="FamilyMemberHistory.date", description="When history was captured/updated", type="date" ) 2155 public static final String SP_DATE = "date"; 2156 /** 2157 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2158 * <p> 2159 * Description: <b>When history was captured/updated</b><br> 2160 * Type: <b>date</b><br> 2161 * Path: <b>FamilyMemberHistory.date</b><br> 2162 * </p> 2163 */ 2164 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2165 2166 /** 2167 * Search parameter: <b>identifier</b> 2168 * <p> 2169 * Description: <b>A search by a record identifier</b><br> 2170 * Type: <b>token</b><br> 2171 * Path: <b>FamilyMemberHistory.identifier</b><br> 2172 * </p> 2173 */ 2174 @SearchParamDefinition(name="identifier", path="FamilyMemberHistory.identifier", description="A search by a record identifier", type="token" ) 2175 public static final String SP_IDENTIFIER = "identifier"; 2176 /** 2177 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2178 * <p> 2179 * Description: <b>A search by a record identifier</b><br> 2180 * Type: <b>token</b><br> 2181 * Path: <b>FamilyMemberHistory.identifier</b><br> 2182 * </p> 2183 */ 2184 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2185 2186 /** 2187 * Search parameter: <b>code</b> 2188 * <p> 2189 * Description: <b>A search by a condition code</b><br> 2190 * Type: <b>token</b><br> 2191 * Path: <b>FamilyMemberHistory.condition.code</b><br> 2192 * </p> 2193 */ 2194 @SearchParamDefinition(name="code", path="FamilyMemberHistory.condition.code", description="A search by a condition code", type="token" ) 2195 public static final String SP_CODE = "code"; 2196 /** 2197 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2198 * <p> 2199 * Description: <b>A search by a condition code</b><br> 2200 * Type: <b>token</b><br> 2201 * Path: <b>FamilyMemberHistory.condition.code</b><br> 2202 * </p> 2203 */ 2204 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2205 2206 /** 2207 * Search parameter: <b>gender</b> 2208 * <p> 2209 * Description: <b>A search by a gender code of a family member</b><br> 2210 * Type: <b>token</b><br> 2211 * Path: <b>FamilyMemberHistory.gender</b><br> 2212 * </p> 2213 */ 2214 @SearchParamDefinition(name="gender", path="FamilyMemberHistory.gender", description="A search by a gender code of a family member", type="token" ) 2215 public static final String SP_GENDER = "gender"; 2216 /** 2217 * <b>Fluent Client</b> search parameter constant for <b>gender</b> 2218 * <p> 2219 * Description: <b>A search by a gender code of a family member</b><br> 2220 * Type: <b>token</b><br> 2221 * Path: <b>FamilyMemberHistory.gender</b><br> 2222 * </p> 2223 */ 2224 public static final ca.uhn.fhir.rest.gclient.TokenClientParam GENDER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_GENDER); 2225 2226 /** 2227 * Search parameter: <b>patient</b> 2228 * <p> 2229 * Description: <b>The identity of a subject to list family member history items for</b><br> 2230 * Type: <b>reference</b><br> 2231 * Path: <b>FamilyMemberHistory.patient</b><br> 2232 * </p> 2233 */ 2234 @SearchParamDefinition(name="patient", path="FamilyMemberHistory.patient", description="The identity of a subject to list family member history items for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2235 public static final String SP_PATIENT = "patient"; 2236 /** 2237 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2238 * <p> 2239 * Description: <b>The identity of a subject to list family member history items for</b><br> 2240 * Type: <b>reference</b><br> 2241 * Path: <b>FamilyMemberHistory.patient</b><br> 2242 * </p> 2243 */ 2244 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2245 2246/** 2247 * Constant for fluent queries to be used to add include statements. Specifies 2248 * the path value of "<b>FamilyMemberHistory:patient</b>". 2249 */ 2250 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("FamilyMemberHistory:patient").toLocked(); 2251 2252 /** 2253 * Search parameter: <b>definition</b> 2254 * <p> 2255 * Description: <b>Instantiates protocol or definition</b><br> 2256 * Type: <b>reference</b><br> 2257 * Path: <b>FamilyMemberHistory.definition</b><br> 2258 * </p> 2259 */ 2260 @SearchParamDefinition(name="definition", path="FamilyMemberHistory.definition", description="Instantiates protocol or definition", type="reference", target={PlanDefinition.class, Questionnaire.class } ) 2261 public static final String SP_DEFINITION = "definition"; 2262 /** 2263 * <b>Fluent Client</b> search parameter constant for <b>definition</b> 2264 * <p> 2265 * Description: <b>Instantiates protocol or definition</b><br> 2266 * Type: <b>reference</b><br> 2267 * Path: <b>FamilyMemberHistory.definition</b><br> 2268 * </p> 2269 */ 2270 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEFINITION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEFINITION); 2271 2272/** 2273 * Constant for fluent queries to be used to add include statements. Specifies 2274 * the path value of "<b>FamilyMemberHistory:definition</b>". 2275 */ 2276 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEFINITION = new ca.uhn.fhir.model.api.Include("FamilyMemberHistory:definition").toLocked(); 2277 2278 /** 2279 * Search parameter: <b>relationship</b> 2280 * <p> 2281 * Description: <b>A search by a relationship type</b><br> 2282 * Type: <b>token</b><br> 2283 * Path: <b>FamilyMemberHistory.relationship</b><br> 2284 * </p> 2285 */ 2286 @SearchParamDefinition(name="relationship", path="FamilyMemberHistory.relationship", description="A search by a relationship type", type="token" ) 2287 public static final String SP_RELATIONSHIP = "relationship"; 2288 /** 2289 * <b>Fluent Client</b> search parameter constant for <b>relationship</b> 2290 * <p> 2291 * Description: <b>A search by a relationship type</b><br> 2292 * Type: <b>token</b><br> 2293 * Path: <b>FamilyMemberHistory.relationship</b><br> 2294 * </p> 2295 */ 2296 public static final ca.uhn.fhir.rest.gclient.TokenClientParam RELATIONSHIP = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_RELATIONSHIP); 2297 2298 /** 2299 * Search parameter: <b>status</b> 2300 * <p> 2301 * Description: <b>partial | completed | entered-in-error | health-unknown</b><br> 2302 * Type: <b>token</b><br> 2303 * Path: <b>FamilyMemberHistory.status</b><br> 2304 * </p> 2305 */ 2306 @SearchParamDefinition(name="status", path="FamilyMemberHistory.status", description="partial | completed | entered-in-error | health-unknown", type="token" ) 2307 public static final String SP_STATUS = "status"; 2308 /** 2309 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2310 * <p> 2311 * Description: <b>partial | completed | entered-in-error | health-unknown</b><br> 2312 * Type: <b>token</b><br> 2313 * Path: <b>FamilyMemberHistory.status</b><br> 2314 * </p> 2315 */ 2316 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2317 2318 2319}