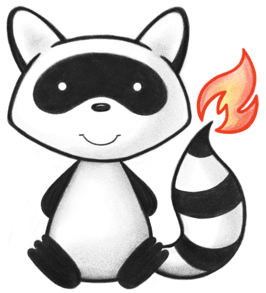
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039 040import ca.uhn.fhir.model.api.annotation.Child; 041import ca.uhn.fhir.model.api.annotation.Description; 042import ca.uhn.fhir.model.api.annotation.ResourceDef; 043import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 044/** 045 * Prospective warnings of potential issues when providing care to the patient. 046 */ 047@ResourceDef(name="Flag", profile="http://hl7.org/fhir/Profile/Flag") 048public class Flag extends DomainResource { 049 050 public enum FlagStatus { 051 /** 052 * A current flag that should be displayed to a user. A system may use the category to determine which roles should view the flag. 053 */ 054 ACTIVE, 055 /** 056 * The flag does not need to be displayed any more. 057 */ 058 INACTIVE, 059 /** 060 * The flag was added in error, and should no longer be displayed. 061 */ 062 ENTEREDINERROR, 063 /** 064 * added to help the parsers with the generic types 065 */ 066 NULL; 067 public static FlagStatus fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("active".equals(codeString)) 071 return ACTIVE; 072 if ("inactive".equals(codeString)) 073 return INACTIVE; 074 if ("entered-in-error".equals(codeString)) 075 return ENTEREDINERROR; 076 if (Configuration.isAcceptInvalidEnums()) 077 return null; 078 else 079 throw new FHIRException("Unknown FlagStatus code '"+codeString+"'"); 080 } 081 public String toCode() { 082 switch (this) { 083 case ACTIVE: return "active"; 084 case INACTIVE: return "inactive"; 085 case ENTEREDINERROR: return "entered-in-error"; 086 case NULL: return null; 087 default: return "?"; 088 } 089 } 090 public String getSystem() { 091 switch (this) { 092 case ACTIVE: return "http://hl7.org/fhir/flag-status"; 093 case INACTIVE: return "http://hl7.org/fhir/flag-status"; 094 case ENTEREDINERROR: return "http://hl7.org/fhir/flag-status"; 095 case NULL: return null; 096 default: return "?"; 097 } 098 } 099 public String getDefinition() { 100 switch (this) { 101 case ACTIVE: return "A current flag that should be displayed to a user. A system may use the category to determine which roles should view the flag."; 102 case INACTIVE: return "The flag does not need to be displayed any more."; 103 case ENTEREDINERROR: return "The flag was added in error, and should no longer be displayed."; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getDisplay() { 109 switch (this) { 110 case ACTIVE: return "Active"; 111 case INACTIVE: return "Inactive"; 112 case ENTEREDINERROR: return "Entered in Error"; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 } 118 119 public static class FlagStatusEnumFactory implements EnumFactory<FlagStatus> { 120 public FlagStatus fromCode(String codeString) throws IllegalArgumentException { 121 if (codeString == null || "".equals(codeString)) 122 if (codeString == null || "".equals(codeString)) 123 return null; 124 if ("active".equals(codeString)) 125 return FlagStatus.ACTIVE; 126 if ("inactive".equals(codeString)) 127 return FlagStatus.INACTIVE; 128 if ("entered-in-error".equals(codeString)) 129 return FlagStatus.ENTEREDINERROR; 130 throw new IllegalArgumentException("Unknown FlagStatus code '"+codeString+"'"); 131 } 132 public Enumeration<FlagStatus> fromType(PrimitiveType<?> code) throws FHIRException { 133 if (code == null) 134 return null; 135 if (code.isEmpty()) 136 return new Enumeration<FlagStatus>(this); 137 String codeString = code.asStringValue(); 138 if (codeString == null || "".equals(codeString)) 139 return null; 140 if ("active".equals(codeString)) 141 return new Enumeration<FlagStatus>(this, FlagStatus.ACTIVE); 142 if ("inactive".equals(codeString)) 143 return new Enumeration<FlagStatus>(this, FlagStatus.INACTIVE); 144 if ("entered-in-error".equals(codeString)) 145 return new Enumeration<FlagStatus>(this, FlagStatus.ENTEREDINERROR); 146 throw new FHIRException("Unknown FlagStatus code '"+codeString+"'"); 147 } 148 public String toCode(FlagStatus code) { 149 if (code == FlagStatus.NULL) 150 return null; 151 if (code == FlagStatus.ACTIVE) 152 return "active"; 153 if (code == FlagStatus.INACTIVE) 154 return "inactive"; 155 if (code == FlagStatus.ENTEREDINERROR) 156 return "entered-in-error"; 157 return "?"; 158 } 159 public String toSystem(FlagStatus code) { 160 return code.getSystem(); 161 } 162 } 163 164 /** 165 * Identifier assigned to the flag for external use (outside the FHIR environment). 166 */ 167 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 168 @Description(shortDefinition="Business identifier", formalDefinition="Identifier assigned to the flag for external use (outside the FHIR environment)." ) 169 protected List<Identifier> identifier; 170 171 /** 172 * Supports basic workflow. 173 */ 174 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 175 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="Supports basic workflow." ) 176 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/flag-status") 177 protected Enumeration<FlagStatus> status; 178 179 /** 180 * Allows an flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context. 181 */ 182 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 183 @Description(shortDefinition="Clinical, administrative, etc.", formalDefinition="Allows an flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context." ) 184 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/flag-category") 185 protected CodeableConcept category; 186 187 /** 188 * The coded value or textual component of the flag to display to the user. 189 */ 190 @Child(name = "code", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=true) 191 @Description(shortDefinition="Coded or textual message to display to user", formalDefinition="The coded value or textual component of the flag to display to the user." ) 192 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/flag-code") 193 protected CodeableConcept code; 194 195 /** 196 * The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with. 197 */ 198 @Child(name = "subject", type = {Patient.class, Location.class, Group.class, Organization.class, Practitioner.class, PlanDefinition.class, Medication.class, Procedure.class}, order=4, min=1, max=1, modifier=false, summary=true) 199 @Description(shortDefinition="Who/What is flag about?", formalDefinition="The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with." ) 200 protected Reference subject; 201 202 /** 203 * The actual object that is the target of the reference (The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with.) 204 */ 205 protected Resource subjectTarget; 206 207 /** 208 * The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified. 209 */ 210 @Child(name = "period", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 211 @Description(shortDefinition="Time period when flag is active", formalDefinition="The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified." ) 212 protected Period period; 213 214 /** 215 * This alert is only relevant during the encounter. 216 */ 217 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=true) 218 @Description(shortDefinition="Alert relevant during encounter", formalDefinition="This alert is only relevant during the encounter." ) 219 protected Reference encounter; 220 221 /** 222 * The actual object that is the target of the reference (This alert is only relevant during the encounter.) 223 */ 224 protected Encounter encounterTarget; 225 226 /** 227 * The person, organization or device that created the flag. 228 */ 229 @Child(name = "author", type = {Device.class, Organization.class, Patient.class, Practitioner.class}, order=7, min=0, max=1, modifier=false, summary=true) 230 @Description(shortDefinition="Flag creator", formalDefinition="The person, organization or device that created the flag." ) 231 protected Reference author; 232 233 /** 234 * The actual object that is the target of the reference (The person, organization or device that created the flag.) 235 */ 236 protected Resource authorTarget; 237 238 private static final long serialVersionUID = -619061399L; 239 240 /** 241 * Constructor 242 */ 243 public Flag() { 244 super(); 245 } 246 247 /** 248 * Constructor 249 */ 250 public Flag(Enumeration<FlagStatus> status, CodeableConcept code, Reference subject) { 251 super(); 252 this.status = status; 253 this.code = code; 254 this.subject = subject; 255 } 256 257 /** 258 * @return {@link #identifier} (Identifier assigned to the flag for external use (outside the FHIR environment).) 259 */ 260 public List<Identifier> getIdentifier() { 261 if (this.identifier == null) 262 this.identifier = new ArrayList<Identifier>(); 263 return this.identifier; 264 } 265 266 /** 267 * @return Returns a reference to <code>this</code> for easy method chaining 268 */ 269 public Flag setIdentifier(List<Identifier> theIdentifier) { 270 this.identifier = theIdentifier; 271 return this; 272 } 273 274 public boolean hasIdentifier() { 275 if (this.identifier == null) 276 return false; 277 for (Identifier item : this.identifier) 278 if (!item.isEmpty()) 279 return true; 280 return false; 281 } 282 283 public Identifier addIdentifier() { //3 284 Identifier t = new Identifier(); 285 if (this.identifier == null) 286 this.identifier = new ArrayList<Identifier>(); 287 this.identifier.add(t); 288 return t; 289 } 290 291 public Flag addIdentifier(Identifier t) { //3 292 if (t == null) 293 return this; 294 if (this.identifier == null) 295 this.identifier = new ArrayList<Identifier>(); 296 this.identifier.add(t); 297 return this; 298 } 299 300 /** 301 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 302 */ 303 public Identifier getIdentifierFirstRep() { 304 if (getIdentifier().isEmpty()) { 305 addIdentifier(); 306 } 307 return getIdentifier().get(0); 308 } 309 310 /** 311 * @return {@link #status} (Supports basic workflow.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 312 */ 313 public Enumeration<FlagStatus> getStatusElement() { 314 if (this.status == null) 315 if (Configuration.errorOnAutoCreate()) 316 throw new Error("Attempt to auto-create Flag.status"); 317 else if (Configuration.doAutoCreate()) 318 this.status = new Enumeration<FlagStatus>(new FlagStatusEnumFactory()); // bb 319 return this.status; 320 } 321 322 public boolean hasStatusElement() { 323 return this.status != null && !this.status.isEmpty(); 324 } 325 326 public boolean hasStatus() { 327 return this.status != null && !this.status.isEmpty(); 328 } 329 330 /** 331 * @param value {@link #status} (Supports basic workflow.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 332 */ 333 public Flag setStatusElement(Enumeration<FlagStatus> value) { 334 this.status = value; 335 return this; 336 } 337 338 /** 339 * @return Supports basic workflow. 340 */ 341 public FlagStatus getStatus() { 342 return this.status == null ? null : this.status.getValue(); 343 } 344 345 /** 346 * @param value Supports basic workflow. 347 */ 348 public Flag setStatus(FlagStatus value) { 349 if (this.status == null) 350 this.status = new Enumeration<FlagStatus>(new FlagStatusEnumFactory()); 351 this.status.setValue(value); 352 return this; 353 } 354 355 /** 356 * @return {@link #category} (Allows an flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.) 357 */ 358 public CodeableConcept getCategory() { 359 if (this.category == null) 360 if (Configuration.errorOnAutoCreate()) 361 throw new Error("Attempt to auto-create Flag.category"); 362 else if (Configuration.doAutoCreate()) 363 this.category = new CodeableConcept(); // cc 364 return this.category; 365 } 366 367 public boolean hasCategory() { 368 return this.category != null && !this.category.isEmpty(); 369 } 370 371 /** 372 * @param value {@link #category} (Allows an flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.) 373 */ 374 public Flag setCategory(CodeableConcept value) { 375 this.category = value; 376 return this; 377 } 378 379 /** 380 * @return {@link #code} (The coded value or textual component of the flag to display to the user.) 381 */ 382 public CodeableConcept getCode() { 383 if (this.code == null) 384 if (Configuration.errorOnAutoCreate()) 385 throw new Error("Attempt to auto-create Flag.code"); 386 else if (Configuration.doAutoCreate()) 387 this.code = new CodeableConcept(); // cc 388 return this.code; 389 } 390 391 public boolean hasCode() { 392 return this.code != null && !this.code.isEmpty(); 393 } 394 395 /** 396 * @param value {@link #code} (The coded value or textual component of the flag to display to the user.) 397 */ 398 public Flag setCode(CodeableConcept value) { 399 this.code = value; 400 return this; 401 } 402 403 /** 404 * @return {@link #subject} (The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with.) 405 */ 406 public Reference getSubject() { 407 if (this.subject == null) 408 if (Configuration.errorOnAutoCreate()) 409 throw new Error("Attempt to auto-create Flag.subject"); 410 else if (Configuration.doAutoCreate()) 411 this.subject = new Reference(); // cc 412 return this.subject; 413 } 414 415 public boolean hasSubject() { 416 return this.subject != null && !this.subject.isEmpty(); 417 } 418 419 /** 420 * @param value {@link #subject} (The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with.) 421 */ 422 public Flag setSubject(Reference value) { 423 this.subject = value; 424 return this; 425 } 426 427 /** 428 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with.) 429 */ 430 public Resource getSubjectTarget() { 431 return this.subjectTarget; 432 } 433 434 /** 435 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with.) 436 */ 437 public Flag setSubjectTarget(Resource value) { 438 this.subjectTarget = value; 439 return this; 440 } 441 442 /** 443 * @return {@link #period} (The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.) 444 */ 445 public Period getPeriod() { 446 if (this.period == null) 447 if (Configuration.errorOnAutoCreate()) 448 throw new Error("Attempt to auto-create Flag.period"); 449 else if (Configuration.doAutoCreate()) 450 this.period = new Period(); // cc 451 return this.period; 452 } 453 454 public boolean hasPeriod() { 455 return this.period != null && !this.period.isEmpty(); 456 } 457 458 /** 459 * @param value {@link #period} (The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.) 460 */ 461 public Flag setPeriod(Period value) { 462 this.period = value; 463 return this; 464 } 465 466 /** 467 * @return {@link #encounter} (This alert is only relevant during the encounter.) 468 */ 469 public Reference getEncounter() { 470 if (this.encounter == null) 471 if (Configuration.errorOnAutoCreate()) 472 throw new Error("Attempt to auto-create Flag.encounter"); 473 else if (Configuration.doAutoCreate()) 474 this.encounter = new Reference(); // cc 475 return this.encounter; 476 } 477 478 public boolean hasEncounter() { 479 return this.encounter != null && !this.encounter.isEmpty(); 480 } 481 482 /** 483 * @param value {@link #encounter} (This alert is only relevant during the encounter.) 484 */ 485 public Flag setEncounter(Reference value) { 486 this.encounter = value; 487 return this; 488 } 489 490 /** 491 * @return {@link #encounter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (This alert is only relevant during the encounter.) 492 */ 493 public Encounter getEncounterTarget() { 494 if (this.encounterTarget == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create Flag.encounter"); 497 else if (Configuration.doAutoCreate()) 498 this.encounterTarget = new Encounter(); // aa 499 return this.encounterTarget; 500 } 501 502 /** 503 * @param value {@link #encounter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (This alert is only relevant during the encounter.) 504 */ 505 public Flag setEncounterTarget(Encounter value) { 506 this.encounterTarget = value; 507 return this; 508 } 509 510 /** 511 * @return {@link #author} (The person, organization or device that created the flag.) 512 */ 513 public Reference getAuthor() { 514 if (this.author == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create Flag.author"); 517 else if (Configuration.doAutoCreate()) 518 this.author = new Reference(); // cc 519 return this.author; 520 } 521 522 public boolean hasAuthor() { 523 return this.author != null && !this.author.isEmpty(); 524 } 525 526 /** 527 * @param value {@link #author} (The person, organization or device that created the flag.) 528 */ 529 public Flag setAuthor(Reference value) { 530 this.author = value; 531 return this; 532 } 533 534 /** 535 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person, organization or device that created the flag.) 536 */ 537 public Resource getAuthorTarget() { 538 return this.authorTarget; 539 } 540 541 /** 542 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person, organization or device that created the flag.) 543 */ 544 public Flag setAuthorTarget(Resource value) { 545 this.authorTarget = value; 546 return this; 547 } 548 549 protected void listChildren(List<Property> children) { 550 super.listChildren(children); 551 children.add(new Property("identifier", "Identifier", "Identifier assigned to the flag for external use (outside the FHIR environment).", 0, java.lang.Integer.MAX_VALUE, identifier)); 552 children.add(new Property("status", "code", "Supports basic workflow.", 0, 1, status)); 553 children.add(new Property("category", "CodeableConcept", "Allows an flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.", 0, 1, category)); 554 children.add(new Property("code", "CodeableConcept", "The coded value or textual component of the flag to display to the user.", 0, 1, code)); 555 children.add(new Property("subject", "Reference(Patient|Location|Group|Organization|Practitioner|PlanDefinition|Medication|Procedure)", "The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with.", 0, 1, subject)); 556 children.add(new Property("period", "Period", "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.", 0, 1, period)); 557 children.add(new Property("encounter", "Reference(Encounter)", "This alert is only relevant during the encounter.", 0, 1, encounter)); 558 children.add(new Property("author", "Reference(Device|Organization|Patient|Practitioner)", "The person, organization or device that created the flag.", 0, 1, author)); 559 } 560 561 @Override 562 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 563 switch (_hash) { 564 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier assigned to the flag for external use (outside the FHIR environment).", 0, java.lang.Integer.MAX_VALUE, identifier); 565 case -892481550: /*status*/ return new Property("status", "code", "Supports basic workflow.", 0, 1, status); 566 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Allows an flag to be divided into different categories like clinical, administrative etc. Intended to be used as a means of filtering which flags are displayed to particular user or in a given context.", 0, 1, category); 567 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The coded value or textual component of the flag to display to the user.", 0, 1, code); 568 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Location|Group|Organization|Practitioner|PlanDefinition|Medication|Procedure)", "The patient, location, group , organization , or practitioner, etc. this is about record this flag is associated with.", 0, 1, subject); 569 case -991726143: /*period*/ return new Property("period", "Period", "The period of time from the activation of the flag to inactivation of the flag. If the flag is active, the end of the period should be unspecified.", 0, 1, period); 570 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "This alert is only relevant during the encounter.", 0, 1, encounter); 571 case -1406328437: /*author*/ return new Property("author", "Reference(Device|Organization|Patient|Practitioner)", "The person, organization or device that created the flag.", 0, 1, author); 572 default: return super.getNamedProperty(_hash, _name, _checkValid); 573 } 574 575 } 576 577 @Override 578 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 579 switch (hash) { 580 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 581 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<FlagStatus> 582 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 583 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 584 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 585 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 586 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 587 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 588 default: return super.getProperty(hash, name, checkValid); 589 } 590 591 } 592 593 @Override 594 public Base setProperty(int hash, String name, Base value) throws FHIRException { 595 switch (hash) { 596 case -1618432855: // identifier 597 this.getIdentifier().add(castToIdentifier(value)); // Identifier 598 return value; 599 case -892481550: // status 600 value = new FlagStatusEnumFactory().fromType(castToCode(value)); 601 this.status = (Enumeration) value; // Enumeration<FlagStatus> 602 return value; 603 case 50511102: // category 604 this.category = castToCodeableConcept(value); // CodeableConcept 605 return value; 606 case 3059181: // code 607 this.code = castToCodeableConcept(value); // CodeableConcept 608 return value; 609 case -1867885268: // subject 610 this.subject = castToReference(value); // Reference 611 return value; 612 case -991726143: // period 613 this.period = castToPeriod(value); // Period 614 return value; 615 case 1524132147: // encounter 616 this.encounter = castToReference(value); // Reference 617 return value; 618 case -1406328437: // author 619 this.author = castToReference(value); // Reference 620 return value; 621 default: return super.setProperty(hash, name, value); 622 } 623 624 } 625 626 @Override 627 public Base setProperty(String name, Base value) throws FHIRException { 628 if (name.equals("identifier")) { 629 this.getIdentifier().add(castToIdentifier(value)); 630 } else if (name.equals("status")) { 631 value = new FlagStatusEnumFactory().fromType(castToCode(value)); 632 this.status = (Enumeration) value; // Enumeration<FlagStatus> 633 } else if (name.equals("category")) { 634 this.category = castToCodeableConcept(value); // CodeableConcept 635 } else if (name.equals("code")) { 636 this.code = castToCodeableConcept(value); // CodeableConcept 637 } else if (name.equals("subject")) { 638 this.subject = castToReference(value); // Reference 639 } else if (name.equals("period")) { 640 this.period = castToPeriod(value); // Period 641 } else if (name.equals("encounter")) { 642 this.encounter = castToReference(value); // Reference 643 } else if (name.equals("author")) { 644 this.author = castToReference(value); // Reference 645 } else 646 return super.setProperty(name, value); 647 return value; 648 } 649 650 @Override 651 public Base makeProperty(int hash, String name) throws FHIRException { 652 switch (hash) { 653 case -1618432855: return addIdentifier(); 654 case -892481550: return getStatusElement(); 655 case 50511102: return getCategory(); 656 case 3059181: return getCode(); 657 case -1867885268: return getSubject(); 658 case -991726143: return getPeriod(); 659 case 1524132147: return getEncounter(); 660 case -1406328437: return getAuthor(); 661 default: return super.makeProperty(hash, name); 662 } 663 664 } 665 666 @Override 667 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 668 switch (hash) { 669 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 670 case -892481550: /*status*/ return new String[] {"code"}; 671 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 672 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 673 case -1867885268: /*subject*/ return new String[] {"Reference"}; 674 case -991726143: /*period*/ return new String[] {"Period"}; 675 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 676 case -1406328437: /*author*/ return new String[] {"Reference"}; 677 default: return super.getTypesForProperty(hash, name); 678 } 679 680 } 681 682 @Override 683 public Base addChild(String name) throws FHIRException { 684 if (name.equals("identifier")) { 685 return addIdentifier(); 686 } 687 else if (name.equals("status")) { 688 throw new FHIRException("Cannot call addChild on a singleton property Flag.status"); 689 } 690 else if (name.equals("category")) { 691 this.category = new CodeableConcept(); 692 return this.category; 693 } 694 else if (name.equals("code")) { 695 this.code = new CodeableConcept(); 696 return this.code; 697 } 698 else if (name.equals("subject")) { 699 this.subject = new Reference(); 700 return this.subject; 701 } 702 else if (name.equals("period")) { 703 this.period = new Period(); 704 return this.period; 705 } 706 else if (name.equals("encounter")) { 707 this.encounter = new Reference(); 708 return this.encounter; 709 } 710 else if (name.equals("author")) { 711 this.author = new Reference(); 712 return this.author; 713 } 714 else 715 return super.addChild(name); 716 } 717 718 public String fhirType() { 719 return "Flag"; 720 721 } 722 723 public Flag copy() { 724 Flag dst = new Flag(); 725 copyValues(dst); 726 if (identifier != null) { 727 dst.identifier = new ArrayList<Identifier>(); 728 for (Identifier i : identifier) 729 dst.identifier.add(i.copy()); 730 }; 731 dst.status = status == null ? null : status.copy(); 732 dst.category = category == null ? null : category.copy(); 733 dst.code = code == null ? null : code.copy(); 734 dst.subject = subject == null ? null : subject.copy(); 735 dst.period = period == null ? null : period.copy(); 736 dst.encounter = encounter == null ? null : encounter.copy(); 737 dst.author = author == null ? null : author.copy(); 738 return dst; 739 } 740 741 protected Flag typedCopy() { 742 return copy(); 743 } 744 745 @Override 746 public boolean equalsDeep(Base other_) { 747 if (!super.equalsDeep(other_)) 748 return false; 749 if (!(other_ instanceof Flag)) 750 return false; 751 Flag o = (Flag) other_; 752 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 753 && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) && compareDeep(period, o.period, true) 754 && compareDeep(encounter, o.encounter, true) && compareDeep(author, o.author, true); 755 } 756 757 @Override 758 public boolean equalsShallow(Base other_) { 759 if (!super.equalsShallow(other_)) 760 return false; 761 if (!(other_ instanceof Flag)) 762 return false; 763 Flag o = (Flag) other_; 764 return compareValues(status, o.status, true); 765 } 766 767 public boolean isEmpty() { 768 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 769 , code, subject, period, encounter, author); 770 } 771 772 @Override 773 public ResourceType getResourceType() { 774 return ResourceType.Flag; 775 } 776 777 /** 778 * Search parameter: <b>date</b> 779 * <p> 780 * Description: <b>Time period when flag is active</b><br> 781 * Type: <b>date</b><br> 782 * Path: <b>Flag.period</b><br> 783 * </p> 784 */ 785 @SearchParamDefinition(name="date", path="Flag.period", description="Time period when flag is active", type="date" ) 786 public static final String SP_DATE = "date"; 787 /** 788 * <b>Fluent Client</b> search parameter constant for <b>date</b> 789 * <p> 790 * Description: <b>Time period when flag is active</b><br> 791 * Type: <b>date</b><br> 792 * Path: <b>Flag.period</b><br> 793 * </p> 794 */ 795 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 796 797 /** 798 * Search parameter: <b>identifier</b> 799 * <p> 800 * Description: <b>Business identifier</b><br> 801 * Type: <b>token</b><br> 802 * Path: <b>Flag.identifier</b><br> 803 * </p> 804 */ 805 @SearchParamDefinition(name="identifier", path="Flag.identifier", description="Business identifier", type="token" ) 806 public static final String SP_IDENTIFIER = "identifier"; 807 /** 808 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 809 * <p> 810 * Description: <b>Business identifier</b><br> 811 * Type: <b>token</b><br> 812 * Path: <b>Flag.identifier</b><br> 813 * </p> 814 */ 815 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 816 817 /** 818 * Search parameter: <b>subject</b> 819 * <p> 820 * Description: <b>The identity of a subject to list flags for</b><br> 821 * Type: <b>reference</b><br> 822 * Path: <b>Flag.subject</b><br> 823 * </p> 824 */ 825 @SearchParamDefinition(name="subject", path="Flag.subject", description="The identity of a subject to list flags for", type="reference", target={Group.class, Location.class, Medication.class, Organization.class, Patient.class, PlanDefinition.class, Practitioner.class, Procedure.class } ) 826 public static final String SP_SUBJECT = "subject"; 827 /** 828 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 829 * <p> 830 * Description: <b>The identity of a subject to list flags for</b><br> 831 * Type: <b>reference</b><br> 832 * Path: <b>Flag.subject</b><br> 833 * </p> 834 */ 835 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 836 837/** 838 * Constant for fluent queries to be used to add include statements. Specifies 839 * the path value of "<b>Flag:subject</b>". 840 */ 841 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Flag:subject").toLocked(); 842 843 /** 844 * Search parameter: <b>patient</b> 845 * <p> 846 * Description: <b>The identity of a subject to list flags for</b><br> 847 * Type: <b>reference</b><br> 848 * Path: <b>Flag.subject</b><br> 849 * </p> 850 */ 851 @SearchParamDefinition(name="patient", path="Flag.subject", description="The identity of a subject to list flags for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 852 public static final String SP_PATIENT = "patient"; 853 /** 854 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 855 * <p> 856 * Description: <b>The identity of a subject to list flags for</b><br> 857 * Type: <b>reference</b><br> 858 * Path: <b>Flag.subject</b><br> 859 * </p> 860 */ 861 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 862 863/** 864 * Constant for fluent queries to be used to add include statements. Specifies 865 * the path value of "<b>Flag:patient</b>". 866 */ 867 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Flag:patient").toLocked(); 868 869 /** 870 * Search parameter: <b>author</b> 871 * <p> 872 * Description: <b>Flag creator</b><br> 873 * Type: <b>reference</b><br> 874 * Path: <b>Flag.author</b><br> 875 * </p> 876 */ 877 @SearchParamDefinition(name="author", path="Flag.author", description="Flag creator", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class } ) 878 public static final String SP_AUTHOR = "author"; 879 /** 880 * <b>Fluent Client</b> search parameter constant for <b>author</b> 881 * <p> 882 * Description: <b>Flag creator</b><br> 883 * Type: <b>reference</b><br> 884 * Path: <b>Flag.author</b><br> 885 * </p> 886 */ 887 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 888 889/** 890 * Constant for fluent queries to be used to add include statements. Specifies 891 * the path value of "<b>Flag:author</b>". 892 */ 893 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Flag:author").toLocked(); 894 895 /** 896 * Search parameter: <b>encounter</b> 897 * <p> 898 * Description: <b>Alert relevant during encounter</b><br> 899 * Type: <b>reference</b><br> 900 * Path: <b>Flag.encounter</b><br> 901 * </p> 902 */ 903 @SearchParamDefinition(name="encounter", path="Flag.encounter", description="Alert relevant during encounter", type="reference", target={Encounter.class } ) 904 public static final String SP_ENCOUNTER = "encounter"; 905 /** 906 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 907 * <p> 908 * Description: <b>Alert relevant during encounter</b><br> 909 * Type: <b>reference</b><br> 910 * Path: <b>Flag.encounter</b><br> 911 * </p> 912 */ 913 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 914 915/** 916 * Constant for fluent queries to be used to add include statements. Specifies 917 * the path value of "<b>Flag:encounter</b>". 918 */ 919 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("Flag:encounter").toLocked(); 920 921 922}