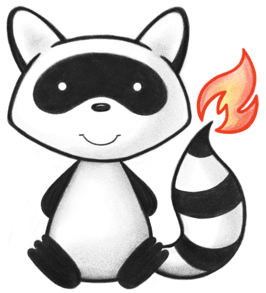
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * Describes the intended objective(s) for a patient, group or organization care, for example, weight loss, restoring an activity of daily living, obtaining herd immunity via immunization, meeting a process improvement objective, etc. 051 */ 052@ResourceDef(name="Goal", profile="http://hl7.org/fhir/Profile/Goal") 053public class Goal extends DomainResource { 054 055 public enum GoalStatus { 056 /** 057 * A goal is proposed for this patient 058 */ 059 PROPOSED, 060 /** 061 * A proposed goal was accepted or acknowledged 062 */ 063 ACCEPTED, 064 /** 065 * A goal is planned for this patient 066 */ 067 PLANNED, 068 /** 069 * The goal is being sought but has not yet been reached. (Also applies if goal was reached in the past but there has been regression and goal is being sought again) 070 */ 071 INPROGRESS, 072 /** 073 * The goal is on schedule for the planned timelines 074 */ 075 ONTARGET, 076 /** 077 * The goal is ahead of the planned timelines 078 */ 079 AHEADOFTARGET, 080 /** 081 * The goal is behind the planned timelines 082 */ 083 BEHINDTARGET, 084 /** 085 * The goal has been met, but ongoing activity is needed to sustain the goal objective 086 */ 087 SUSTAINING, 088 /** 089 * The goal has been met and no further action is needed 090 */ 091 ACHIEVED, 092 /** 093 * The goal remains a long term objective but is no longer being actively pursued for a temporary period of time. 094 */ 095 ONHOLD, 096 /** 097 * The previously accepted goal is no longer being sought 098 */ 099 CANCELLED, 100 /** 101 * The goal was entered in error and voided. 102 */ 103 ENTEREDINERROR, 104 /** 105 * A proposed goal was rejected 106 */ 107 REJECTED, 108 /** 109 * added to help the parsers with the generic types 110 */ 111 NULL; 112 public static GoalStatus fromCode(String codeString) throws FHIRException { 113 if (codeString == null || "".equals(codeString)) 114 return null; 115 if ("proposed".equals(codeString)) 116 return PROPOSED; 117 if ("accepted".equals(codeString)) 118 return ACCEPTED; 119 if ("planned".equals(codeString)) 120 return PLANNED; 121 if ("in-progress".equals(codeString)) 122 return INPROGRESS; 123 if ("on-target".equals(codeString)) 124 return ONTARGET; 125 if ("ahead-of-target".equals(codeString)) 126 return AHEADOFTARGET; 127 if ("behind-target".equals(codeString)) 128 return BEHINDTARGET; 129 if ("sustaining".equals(codeString)) 130 return SUSTAINING; 131 if ("achieved".equals(codeString)) 132 return ACHIEVED; 133 if ("on-hold".equals(codeString)) 134 return ONHOLD; 135 if ("cancelled".equals(codeString)) 136 return CANCELLED; 137 if ("entered-in-error".equals(codeString)) 138 return ENTEREDINERROR; 139 if ("rejected".equals(codeString)) 140 return REJECTED; 141 if (Configuration.isAcceptInvalidEnums()) 142 return null; 143 else 144 throw new FHIRException("Unknown GoalStatus code '"+codeString+"'"); 145 } 146 public String toCode() { 147 switch (this) { 148 case PROPOSED: return "proposed"; 149 case ACCEPTED: return "accepted"; 150 case PLANNED: return "planned"; 151 case INPROGRESS: return "in-progress"; 152 case ONTARGET: return "on-target"; 153 case AHEADOFTARGET: return "ahead-of-target"; 154 case BEHINDTARGET: return "behind-target"; 155 case SUSTAINING: return "sustaining"; 156 case ACHIEVED: return "achieved"; 157 case ONHOLD: return "on-hold"; 158 case CANCELLED: return "cancelled"; 159 case ENTEREDINERROR: return "entered-in-error"; 160 case REJECTED: return "rejected"; 161 case NULL: return null; 162 default: return "?"; 163 } 164 } 165 public String getSystem() { 166 switch (this) { 167 case PROPOSED: return "http://hl7.org/fhir/goal-status"; 168 case ACCEPTED: return "http://hl7.org/fhir/goal-status"; 169 case PLANNED: return "http://hl7.org/fhir/goal-status"; 170 case INPROGRESS: return "http://hl7.org/fhir/goal-status"; 171 case ONTARGET: return "http://hl7.org/fhir/goal-status"; 172 case AHEADOFTARGET: return "http://hl7.org/fhir/goal-status"; 173 case BEHINDTARGET: return "http://hl7.org/fhir/goal-status"; 174 case SUSTAINING: return "http://hl7.org/fhir/goal-status"; 175 case ACHIEVED: return "http://hl7.org/fhir/goal-status"; 176 case ONHOLD: return "http://hl7.org/fhir/goal-status"; 177 case CANCELLED: return "http://hl7.org/fhir/goal-status"; 178 case ENTEREDINERROR: return "http://hl7.org/fhir/goal-status"; 179 case REJECTED: return "http://hl7.org/fhir/goal-status"; 180 case NULL: return null; 181 default: return "?"; 182 } 183 } 184 public String getDefinition() { 185 switch (this) { 186 case PROPOSED: return "A goal is proposed for this patient"; 187 case ACCEPTED: return "A proposed goal was accepted or acknowledged"; 188 case PLANNED: return "A goal is planned for this patient"; 189 case INPROGRESS: return "The goal is being sought but has not yet been reached. (Also applies if goal was reached in the past but there has been regression and goal is being sought again)"; 190 case ONTARGET: return "The goal is on schedule for the planned timelines"; 191 case AHEADOFTARGET: return "The goal is ahead of the planned timelines"; 192 case BEHINDTARGET: return "The goal is behind the planned timelines"; 193 case SUSTAINING: return "The goal has been met, but ongoing activity is needed to sustain the goal objective"; 194 case ACHIEVED: return "The goal has been met and no further action is needed"; 195 case ONHOLD: return "The goal remains a long term objective but is no longer being actively pursued for a temporary period of time."; 196 case CANCELLED: return "The previously accepted goal is no longer being sought"; 197 case ENTEREDINERROR: return "The goal was entered in error and voided."; 198 case REJECTED: return "A proposed goal was rejected"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 public String getDisplay() { 204 switch (this) { 205 case PROPOSED: return "Proposed"; 206 case ACCEPTED: return "Accepted"; 207 case PLANNED: return "Planned"; 208 case INPROGRESS: return "In Progress"; 209 case ONTARGET: return "On Target"; 210 case AHEADOFTARGET: return "Ahead of Target"; 211 case BEHINDTARGET: return "Behind Target"; 212 case SUSTAINING: return "Sustaining"; 213 case ACHIEVED: return "Achieved"; 214 case ONHOLD: return "On Hold"; 215 case CANCELLED: return "Cancelled"; 216 case ENTEREDINERROR: return "Entered In Error"; 217 case REJECTED: return "Rejected"; 218 case NULL: return null; 219 default: return "?"; 220 } 221 } 222 } 223 224 public static class GoalStatusEnumFactory implements EnumFactory<GoalStatus> { 225 public GoalStatus fromCode(String codeString) throws IllegalArgumentException { 226 if (codeString == null || "".equals(codeString)) 227 if (codeString == null || "".equals(codeString)) 228 return null; 229 if ("proposed".equals(codeString)) 230 return GoalStatus.PROPOSED; 231 if ("accepted".equals(codeString)) 232 return GoalStatus.ACCEPTED; 233 if ("planned".equals(codeString)) 234 return GoalStatus.PLANNED; 235 if ("in-progress".equals(codeString)) 236 return GoalStatus.INPROGRESS; 237 if ("on-target".equals(codeString)) 238 return GoalStatus.ONTARGET; 239 if ("ahead-of-target".equals(codeString)) 240 return GoalStatus.AHEADOFTARGET; 241 if ("behind-target".equals(codeString)) 242 return GoalStatus.BEHINDTARGET; 243 if ("sustaining".equals(codeString)) 244 return GoalStatus.SUSTAINING; 245 if ("achieved".equals(codeString)) 246 return GoalStatus.ACHIEVED; 247 if ("on-hold".equals(codeString)) 248 return GoalStatus.ONHOLD; 249 if ("cancelled".equals(codeString)) 250 return GoalStatus.CANCELLED; 251 if ("entered-in-error".equals(codeString)) 252 return GoalStatus.ENTEREDINERROR; 253 if ("rejected".equals(codeString)) 254 return GoalStatus.REJECTED; 255 throw new IllegalArgumentException("Unknown GoalStatus code '"+codeString+"'"); 256 } 257 public Enumeration<GoalStatus> fromType(PrimitiveType<?> code) throws FHIRException { 258 if (code == null) 259 return null; 260 if (code.isEmpty()) 261 return new Enumeration<GoalStatus>(this); 262 String codeString = code.asStringValue(); 263 if (codeString == null || "".equals(codeString)) 264 return null; 265 if ("proposed".equals(codeString)) 266 return new Enumeration<GoalStatus>(this, GoalStatus.PROPOSED); 267 if ("accepted".equals(codeString)) 268 return new Enumeration<GoalStatus>(this, GoalStatus.ACCEPTED); 269 if ("planned".equals(codeString)) 270 return new Enumeration<GoalStatus>(this, GoalStatus.PLANNED); 271 if ("in-progress".equals(codeString)) 272 return new Enumeration<GoalStatus>(this, GoalStatus.INPROGRESS); 273 if ("on-target".equals(codeString)) 274 return new Enumeration<GoalStatus>(this, GoalStatus.ONTARGET); 275 if ("ahead-of-target".equals(codeString)) 276 return new Enumeration<GoalStatus>(this, GoalStatus.AHEADOFTARGET); 277 if ("behind-target".equals(codeString)) 278 return new Enumeration<GoalStatus>(this, GoalStatus.BEHINDTARGET); 279 if ("sustaining".equals(codeString)) 280 return new Enumeration<GoalStatus>(this, GoalStatus.SUSTAINING); 281 if ("achieved".equals(codeString)) 282 return new Enumeration<GoalStatus>(this, GoalStatus.ACHIEVED); 283 if ("on-hold".equals(codeString)) 284 return new Enumeration<GoalStatus>(this, GoalStatus.ONHOLD); 285 if ("cancelled".equals(codeString)) 286 return new Enumeration<GoalStatus>(this, GoalStatus.CANCELLED); 287 if ("entered-in-error".equals(codeString)) 288 return new Enumeration<GoalStatus>(this, GoalStatus.ENTEREDINERROR); 289 if ("rejected".equals(codeString)) 290 return new Enumeration<GoalStatus>(this, GoalStatus.REJECTED); 291 throw new FHIRException("Unknown GoalStatus code '"+codeString+"'"); 292 } 293 public String toCode(GoalStatus code) { 294 if (code == GoalStatus.NULL) 295 return null; 296 if (code == GoalStatus.PROPOSED) 297 return "proposed"; 298 if (code == GoalStatus.ACCEPTED) 299 return "accepted"; 300 if (code == GoalStatus.PLANNED) 301 return "planned"; 302 if (code == GoalStatus.INPROGRESS) 303 return "in-progress"; 304 if (code == GoalStatus.ONTARGET) 305 return "on-target"; 306 if (code == GoalStatus.AHEADOFTARGET) 307 return "ahead-of-target"; 308 if (code == GoalStatus.BEHINDTARGET) 309 return "behind-target"; 310 if (code == GoalStatus.SUSTAINING) 311 return "sustaining"; 312 if (code == GoalStatus.ACHIEVED) 313 return "achieved"; 314 if (code == GoalStatus.ONHOLD) 315 return "on-hold"; 316 if (code == GoalStatus.CANCELLED) 317 return "cancelled"; 318 if (code == GoalStatus.ENTEREDINERROR) 319 return "entered-in-error"; 320 if (code == GoalStatus.REJECTED) 321 return "rejected"; 322 return "?"; 323 } 324 public String toSystem(GoalStatus code) { 325 return code.getSystem(); 326 } 327 } 328 329 @Block() 330 public static class GoalTargetComponent extends BackboneElement implements IBaseBackboneElement { 331 /** 332 * The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level. 333 */ 334 @Child(name = "measure", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 335 @Description(shortDefinition="The parameter whose value is being tracked", formalDefinition="The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level." ) 336 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/observation-codes") 337 protected CodeableConcept measure; 338 339 /** 340 * The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value. 341 */ 342 @Child(name = "detail", type = {Quantity.class, Range.class, CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 343 @Description(shortDefinition="The target value to be achieved", formalDefinition="The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value." ) 344 protected Type detail; 345 346 /** 347 * Indicates either the date or the duration after start by which the goal should be met. 348 */ 349 @Child(name = "due", type = {DateType.class, Duration.class}, order=3, min=0, max=1, modifier=false, summary=true) 350 @Description(shortDefinition="Reach goal on or before", formalDefinition="Indicates either the date or the duration after start by which the goal should be met." ) 351 protected Type due; 352 353 private static final long serialVersionUID = -585108934L; 354 355 /** 356 * Constructor 357 */ 358 public GoalTargetComponent() { 359 super(); 360 } 361 362 /** 363 * @return {@link #measure} (The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.) 364 */ 365 public CodeableConcept getMeasure() { 366 if (this.measure == null) 367 if (Configuration.errorOnAutoCreate()) 368 throw new Error("Attempt to auto-create GoalTargetComponent.measure"); 369 else if (Configuration.doAutoCreate()) 370 this.measure = new CodeableConcept(); // cc 371 return this.measure; 372 } 373 374 public boolean hasMeasure() { 375 return this.measure != null && !this.measure.isEmpty(); 376 } 377 378 /** 379 * @param value {@link #measure} (The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.) 380 */ 381 public GoalTargetComponent setMeasure(CodeableConcept value) { 382 this.measure = value; 383 return this; 384 } 385 386 /** 387 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 388 */ 389 public Type getDetail() { 390 return this.detail; 391 } 392 393 /** 394 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 395 */ 396 public Quantity getDetailQuantity() throws FHIRException { 397 if (this.detail == null) 398 return null; 399 if (!(this.detail instanceof Quantity)) 400 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.detail.getClass().getName()+" was encountered"); 401 return (Quantity) this.detail; 402 } 403 404 public boolean hasDetailQuantity() { 405 return this != null && this.detail instanceof Quantity; 406 } 407 408 /** 409 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 410 */ 411 public Range getDetailRange() throws FHIRException { 412 if (this.detail == null) 413 return null; 414 if (!(this.detail instanceof Range)) 415 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.detail.getClass().getName()+" was encountered"); 416 return (Range) this.detail; 417 } 418 419 public boolean hasDetailRange() { 420 return this != null && this.detail instanceof Range; 421 } 422 423 /** 424 * @return {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 425 */ 426 public CodeableConcept getDetailCodeableConcept() throws FHIRException { 427 if (this.detail == null) 428 return null; 429 if (!(this.detail instanceof CodeableConcept)) 430 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.detail.getClass().getName()+" was encountered"); 431 return (CodeableConcept) this.detail; 432 } 433 434 public boolean hasDetailCodeableConcept() { 435 return this != null && this.detail instanceof CodeableConcept; 436 } 437 438 public boolean hasDetail() { 439 return this.detail != null && !this.detail.isEmpty(); 440 } 441 442 /** 443 * @param value {@link #detail} (The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.) 444 */ 445 public GoalTargetComponent setDetail(Type value) throws FHIRFormatError { 446 if (value != null && !(value instanceof Quantity || value instanceof Range || value instanceof CodeableConcept)) 447 throw new FHIRFormatError("Not the right type for Goal.target.detail[x]: "+value.fhirType()); 448 this.detail = value; 449 return this; 450 } 451 452 /** 453 * @return {@link #due} (Indicates either the date or the duration after start by which the goal should be met.) 454 */ 455 public Type getDue() { 456 return this.due; 457 } 458 459 /** 460 * @return {@link #due} (Indicates either the date or the duration after start by which the goal should be met.) 461 */ 462 public DateType getDueDateType() throws FHIRException { 463 if (this.due == null) 464 return null; 465 if (!(this.due instanceof DateType)) 466 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.due.getClass().getName()+" was encountered"); 467 return (DateType) this.due; 468 } 469 470 public boolean hasDueDateType() { 471 return this != null && this.due instanceof DateType; 472 } 473 474 /** 475 * @return {@link #due} (Indicates either the date or the duration after start by which the goal should be met.) 476 */ 477 public Duration getDueDuration() throws FHIRException { 478 if (this.due == null) 479 return null; 480 if (!(this.due instanceof Duration)) 481 throw new FHIRException("Type mismatch: the type Duration was expected, but "+this.due.getClass().getName()+" was encountered"); 482 return (Duration) this.due; 483 } 484 485 public boolean hasDueDuration() { 486 return this != null && this.due instanceof Duration; 487 } 488 489 public boolean hasDue() { 490 return this.due != null && !this.due.isEmpty(); 491 } 492 493 /** 494 * @param value {@link #due} (Indicates either the date or the duration after start by which the goal should be met.) 495 */ 496 public GoalTargetComponent setDue(Type value) throws FHIRFormatError { 497 if (value != null && !(value instanceof DateType || value instanceof Duration)) 498 throw new FHIRFormatError("Not the right type for Goal.target.due[x]: "+value.fhirType()); 499 this.due = value; 500 return this; 501 } 502 503 protected void listChildren(List<Property> children) { 504 super.listChildren(children); 505 children.add(new Property("measure", "CodeableConcept", "The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 1, measure)); 506 children.add(new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail)); 507 children.add(new Property("due[x]", "date|Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due)); 508 } 509 510 @Override 511 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 512 switch (_hash) { 513 case 938321246: /*measure*/ return new Property("measure", "CodeableConcept", "The parameter whose value is being tracked, e.g. body weight, blood pressure, or hemoglobin A1c level.", 0, 1, measure); 514 case -1973084529: /*detail[x]*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 515 case -1335224239: /*detail*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 516 case -1313079300: /*detailQuantity*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 517 case -2062632084: /*detailRange*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 518 case -175586544: /*detailCodeableConcept*/ return new Property("detail[x]", "Quantity|Range|CodeableConcept", "The target value of the focus to be achieved to signify the fulfillment of the goal, e.g. 150 pounds, 7.0%. Either the high or low or both values of the range can be specified. When a low value is missing, it indicates that the goal is achieved at any focus value at or below the high value. Similarly, if the high value is missing, it indicates that the goal is achieved at any focus value at or above the low value.", 0, 1, detail); 519 case -1320900084: /*due[x]*/ return new Property("due[x]", "date|Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 520 case 99828: /*due*/ return new Property("due[x]", "date|Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 521 case 2001063874: /*dueDate*/ return new Property("due[x]", "date|Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 522 case -620428376: /*dueDuration*/ return new Property("due[x]", "date|Duration", "Indicates either the date or the duration after start by which the goal should be met.", 0, 1, due); 523 default: return super.getNamedProperty(_hash, _name, _checkValid); 524 } 525 526 } 527 528 @Override 529 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 530 switch (hash) { 531 case 938321246: /*measure*/ return this.measure == null ? new Base[0] : new Base[] {this.measure}; // CodeableConcept 532 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // Type 533 case 99828: /*due*/ return this.due == null ? new Base[0] : new Base[] {this.due}; // Type 534 default: return super.getProperty(hash, name, checkValid); 535 } 536 537 } 538 539 @Override 540 public Base setProperty(int hash, String name, Base value) throws FHIRException { 541 switch (hash) { 542 case 938321246: // measure 543 this.measure = castToCodeableConcept(value); // CodeableConcept 544 return value; 545 case -1335224239: // detail 546 this.detail = castToType(value); // Type 547 return value; 548 case 99828: // due 549 this.due = castToType(value); // Type 550 return value; 551 default: return super.setProperty(hash, name, value); 552 } 553 554 } 555 556 @Override 557 public Base setProperty(String name, Base value) throws FHIRException { 558 if (name.equals("measure")) { 559 this.measure = castToCodeableConcept(value); // CodeableConcept 560 } else if (name.equals("detail[x]")) { 561 this.detail = castToType(value); // Type 562 } else if (name.equals("due[x]")) { 563 this.due = castToType(value); // Type 564 } else 565 return super.setProperty(name, value); 566 return value; 567 } 568 569 @Override 570 public Base makeProperty(int hash, String name) throws FHIRException { 571 switch (hash) { 572 case 938321246: return getMeasure(); 573 case -1973084529: return getDetail(); 574 case -1335224239: return getDetail(); 575 case -1320900084: return getDue(); 576 case 99828: return getDue(); 577 default: return super.makeProperty(hash, name); 578 } 579 580 } 581 582 @Override 583 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 584 switch (hash) { 585 case 938321246: /*measure*/ return new String[] {"CodeableConcept"}; 586 case -1335224239: /*detail*/ return new String[] {"Quantity", "Range", "CodeableConcept"}; 587 case 99828: /*due*/ return new String[] {"date", "Duration"}; 588 default: return super.getTypesForProperty(hash, name); 589 } 590 591 } 592 593 @Override 594 public Base addChild(String name) throws FHIRException { 595 if (name.equals("measure")) { 596 this.measure = new CodeableConcept(); 597 return this.measure; 598 } 599 else if (name.equals("detailQuantity")) { 600 this.detail = new Quantity(); 601 return this.detail; 602 } 603 else if (name.equals("detailRange")) { 604 this.detail = new Range(); 605 return this.detail; 606 } 607 else if (name.equals("detailCodeableConcept")) { 608 this.detail = new CodeableConcept(); 609 return this.detail; 610 } 611 else if (name.equals("dueDate")) { 612 this.due = new DateType(); 613 return this.due; 614 } 615 else if (name.equals("dueDuration")) { 616 this.due = new Duration(); 617 return this.due; 618 } 619 else 620 return super.addChild(name); 621 } 622 623 public GoalTargetComponent copy() { 624 GoalTargetComponent dst = new GoalTargetComponent(); 625 copyValues(dst); 626 dst.measure = measure == null ? null : measure.copy(); 627 dst.detail = detail == null ? null : detail.copy(); 628 dst.due = due == null ? null : due.copy(); 629 return dst; 630 } 631 632 @Override 633 public boolean equalsDeep(Base other_) { 634 if (!super.equalsDeep(other_)) 635 return false; 636 if (!(other_ instanceof GoalTargetComponent)) 637 return false; 638 GoalTargetComponent o = (GoalTargetComponent) other_; 639 return compareDeep(measure, o.measure, true) && compareDeep(detail, o.detail, true) && compareDeep(due, o.due, true) 640 ; 641 } 642 643 @Override 644 public boolean equalsShallow(Base other_) { 645 if (!super.equalsShallow(other_)) 646 return false; 647 if (!(other_ instanceof GoalTargetComponent)) 648 return false; 649 GoalTargetComponent o = (GoalTargetComponent) other_; 650 return true; 651 } 652 653 public boolean isEmpty() { 654 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(measure, detail, due); 655 } 656 657 public String fhirType() { 658 return "Goal.target"; 659 660 } 661 662 } 663 664 /** 665 * This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation). 666 */ 667 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 668 @Description(shortDefinition="External Ids for this goal", formalDefinition="This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation)." ) 669 protected List<Identifier> identifier; 670 671 /** 672 * Indicates whether the goal has been reached and is still considered relevant. 673 */ 674 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 675 @Description(shortDefinition="proposed | accepted | planned | in-progress | on-target | ahead-of-target | behind-target | sustaining | achieved | on-hold | cancelled | entered-in-error | rejected", formalDefinition="Indicates whether the goal has been reached and is still considered relevant." ) 676 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-status") 677 protected Enumeration<GoalStatus> status; 678 679 /** 680 * Indicates a category the goal falls within. 681 */ 682 @Child(name = "category", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 683 @Description(shortDefinition="E.g. Treatment, dietary, behavioral, etc.", formalDefinition="Indicates a category the goal falls within." ) 684 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-category") 685 protected List<CodeableConcept> category; 686 687 /** 688 * Identifies the mutually agreed level of importance associated with reaching/sustaining the goal. 689 */ 690 @Child(name = "priority", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 691 @Description(shortDefinition="high-priority | medium-priority | low-priority", formalDefinition="Identifies the mutually agreed level of importance associated with reaching/sustaining the goal." ) 692 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-priority") 693 protected CodeableConcept priority; 694 695 /** 696 * Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding". 697 */ 698 @Child(name = "description", type = {CodeableConcept.class}, order=4, min=1, max=1, modifier=false, summary=true) 699 @Description(shortDefinition="Code or text describing goal", formalDefinition="Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\"." ) 700 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 701 protected CodeableConcept description; 702 703 /** 704 * Identifies the patient, group or organization for whom the goal is being established. 705 */ 706 @Child(name = "subject", type = {Patient.class, Group.class, Organization.class}, order=5, min=0, max=1, modifier=false, summary=true) 707 @Description(shortDefinition="Who this goal is intended for", formalDefinition="Identifies the patient, group or organization for whom the goal is being established." ) 708 protected Reference subject; 709 710 /** 711 * The actual object that is the target of the reference (Identifies the patient, group or organization for whom the goal is being established.) 712 */ 713 protected Resource subjectTarget; 714 715 /** 716 * The date or event after which the goal should begin being pursued. 717 */ 718 @Child(name = "start", type = {DateType.class, CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=true) 719 @Description(shortDefinition="When goal pursuit begins", formalDefinition="The date or event after which the goal should begin being pursued." ) 720 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/goal-start-event") 721 protected Type start; 722 723 /** 724 * Indicates what should be done by when. 725 */ 726 @Child(name = "target", type = {}, order=7, min=0, max=1, modifier=false, summary=false) 727 @Description(shortDefinition="Target outcome for the goal", formalDefinition="Indicates what should be done by when." ) 728 protected GoalTargetComponent target; 729 730 /** 731 * Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc. 732 */ 733 @Child(name = "statusDate", type = {DateType.class}, order=8, min=0, max=1, modifier=false, summary=true) 734 @Description(shortDefinition="When goal status took effect", formalDefinition="Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc." ) 735 protected DateType statusDate; 736 737 /** 738 * Captures the reason for the current status. 739 */ 740 @Child(name = "statusReason", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 741 @Description(shortDefinition="Reason for current status", formalDefinition="Captures the reason for the current status." ) 742 protected StringType statusReason; 743 744 /** 745 * Indicates whose goal this is - patient goal, practitioner goal, etc. 746 */ 747 @Child(name = "expressedBy", type = {Patient.class, Practitioner.class, RelatedPerson.class}, order=10, min=0, max=1, modifier=false, summary=true) 748 @Description(shortDefinition="Who's responsible for creating Goal?", formalDefinition="Indicates whose goal this is - patient goal, practitioner goal, etc." ) 749 protected Reference expressedBy; 750 751 /** 752 * The actual object that is the target of the reference (Indicates whose goal this is - patient goal, practitioner goal, etc.) 753 */ 754 protected Resource expressedByTarget; 755 756 /** 757 * The identified conditions and other health record elements that are intended to be addressed by the goal. 758 */ 759 @Child(name = "addresses", type = {Condition.class, Observation.class, MedicationStatement.class, NutritionOrder.class, ProcedureRequest.class, RiskAssessment.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 760 @Description(shortDefinition="Issues addressed by this goal", formalDefinition="The identified conditions and other health record elements that are intended to be addressed by the goal." ) 761 protected List<Reference> addresses; 762 /** 763 * The actual objects that are the target of the reference (The identified conditions and other health record elements that are intended to be addressed by the goal.) 764 */ 765 protected List<Resource> addressesTarget; 766 767 768 /** 769 * Any comments related to the goal. 770 */ 771 @Child(name = "note", type = {Annotation.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 772 @Description(shortDefinition="Comments about the goal", formalDefinition="Any comments related to the goal." ) 773 protected List<Annotation> note; 774 775 /** 776 * Identifies the change (or lack of change) at the point when the status of the goal is assessed. 777 */ 778 @Child(name = "outcomeCode", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 779 @Description(shortDefinition="What result was achieved regarding the goal?", formalDefinition="Identifies the change (or lack of change) at the point when the status of the goal is assessed." ) 780 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/clinical-findings") 781 protected List<CodeableConcept> outcomeCode; 782 783 /** 784 * Details of what's changed (or not changed). 785 */ 786 @Child(name = "outcomeReference", type = {Observation.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 787 @Description(shortDefinition="Observation that resulted from goal", formalDefinition="Details of what's changed (or not changed)." ) 788 protected List<Reference> outcomeReference; 789 /** 790 * The actual objects that are the target of the reference (Details of what's changed (or not changed).) 791 */ 792 protected List<Observation> outcomeReferenceTarget; 793 794 795 private static final long serialVersionUID = -1045412647L; 796 797 /** 798 * Constructor 799 */ 800 public Goal() { 801 super(); 802 } 803 804 /** 805 * Constructor 806 */ 807 public Goal(Enumeration<GoalStatus> status, CodeableConcept description) { 808 super(); 809 this.status = status; 810 this.description = description; 811 } 812 813 /** 814 * @return {@link #identifier} (This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).) 815 */ 816 public List<Identifier> getIdentifier() { 817 if (this.identifier == null) 818 this.identifier = new ArrayList<Identifier>(); 819 return this.identifier; 820 } 821 822 /** 823 * @return Returns a reference to <code>this</code> for easy method chaining 824 */ 825 public Goal setIdentifier(List<Identifier> theIdentifier) { 826 this.identifier = theIdentifier; 827 return this; 828 } 829 830 public boolean hasIdentifier() { 831 if (this.identifier == null) 832 return false; 833 for (Identifier item : this.identifier) 834 if (!item.isEmpty()) 835 return true; 836 return false; 837 } 838 839 public Identifier addIdentifier() { //3 840 Identifier t = new Identifier(); 841 if (this.identifier == null) 842 this.identifier = new ArrayList<Identifier>(); 843 this.identifier.add(t); 844 return t; 845 } 846 847 public Goal addIdentifier(Identifier t) { //3 848 if (t == null) 849 return this; 850 if (this.identifier == null) 851 this.identifier = new ArrayList<Identifier>(); 852 this.identifier.add(t); 853 return this; 854 } 855 856 /** 857 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 858 */ 859 public Identifier getIdentifierFirstRep() { 860 if (getIdentifier().isEmpty()) { 861 addIdentifier(); 862 } 863 return getIdentifier().get(0); 864 } 865 866 /** 867 * @return {@link #status} (Indicates whether the goal has been reached and is still considered relevant.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 868 */ 869 public Enumeration<GoalStatus> getStatusElement() { 870 if (this.status == null) 871 if (Configuration.errorOnAutoCreate()) 872 throw new Error("Attempt to auto-create Goal.status"); 873 else if (Configuration.doAutoCreate()) 874 this.status = new Enumeration<GoalStatus>(new GoalStatusEnumFactory()); // bb 875 return this.status; 876 } 877 878 public boolean hasStatusElement() { 879 return this.status != null && !this.status.isEmpty(); 880 } 881 882 public boolean hasStatus() { 883 return this.status != null && !this.status.isEmpty(); 884 } 885 886 /** 887 * @param value {@link #status} (Indicates whether the goal has been reached and is still considered relevant.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 888 */ 889 public Goal setStatusElement(Enumeration<GoalStatus> value) { 890 this.status = value; 891 return this; 892 } 893 894 /** 895 * @return Indicates whether the goal has been reached and is still considered relevant. 896 */ 897 public GoalStatus getStatus() { 898 return this.status == null ? null : this.status.getValue(); 899 } 900 901 /** 902 * @param value Indicates whether the goal has been reached and is still considered relevant. 903 */ 904 public Goal setStatus(GoalStatus value) { 905 if (this.status == null) 906 this.status = new Enumeration<GoalStatus>(new GoalStatusEnumFactory()); 907 this.status.setValue(value); 908 return this; 909 } 910 911 /** 912 * @return {@link #category} (Indicates a category the goal falls within.) 913 */ 914 public List<CodeableConcept> getCategory() { 915 if (this.category == null) 916 this.category = new ArrayList<CodeableConcept>(); 917 return this.category; 918 } 919 920 /** 921 * @return Returns a reference to <code>this</code> for easy method chaining 922 */ 923 public Goal setCategory(List<CodeableConcept> theCategory) { 924 this.category = theCategory; 925 return this; 926 } 927 928 public boolean hasCategory() { 929 if (this.category == null) 930 return false; 931 for (CodeableConcept item : this.category) 932 if (!item.isEmpty()) 933 return true; 934 return false; 935 } 936 937 public CodeableConcept addCategory() { //3 938 CodeableConcept t = new CodeableConcept(); 939 if (this.category == null) 940 this.category = new ArrayList<CodeableConcept>(); 941 this.category.add(t); 942 return t; 943 } 944 945 public Goal addCategory(CodeableConcept t) { //3 946 if (t == null) 947 return this; 948 if (this.category == null) 949 this.category = new ArrayList<CodeableConcept>(); 950 this.category.add(t); 951 return this; 952 } 953 954 /** 955 * @return The first repetition of repeating field {@link #category}, creating it if it does not already exist 956 */ 957 public CodeableConcept getCategoryFirstRep() { 958 if (getCategory().isEmpty()) { 959 addCategory(); 960 } 961 return getCategory().get(0); 962 } 963 964 /** 965 * @return {@link #priority} (Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.) 966 */ 967 public CodeableConcept getPriority() { 968 if (this.priority == null) 969 if (Configuration.errorOnAutoCreate()) 970 throw new Error("Attempt to auto-create Goal.priority"); 971 else if (Configuration.doAutoCreate()) 972 this.priority = new CodeableConcept(); // cc 973 return this.priority; 974 } 975 976 public boolean hasPriority() { 977 return this.priority != null && !this.priority.isEmpty(); 978 } 979 980 /** 981 * @param value {@link #priority} (Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.) 982 */ 983 public Goal setPriority(CodeableConcept value) { 984 this.priority = value; 985 return this; 986 } 987 988 /** 989 * @return {@link #description} (Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding".) 990 */ 991 public CodeableConcept getDescription() { 992 if (this.description == null) 993 if (Configuration.errorOnAutoCreate()) 994 throw new Error("Attempt to auto-create Goal.description"); 995 else if (Configuration.doAutoCreate()) 996 this.description = new CodeableConcept(); // cc 997 return this.description; 998 } 999 1000 public boolean hasDescription() { 1001 return this.description != null && !this.description.isEmpty(); 1002 } 1003 1004 /** 1005 * @param value {@link #description} (Human-readable and/or coded description of a specific desired objective of care, such as "control blood pressure" or "negotiate an obstacle course" or "dance with child at wedding".) 1006 */ 1007 public Goal setDescription(CodeableConcept value) { 1008 this.description = value; 1009 return this; 1010 } 1011 1012 /** 1013 * @return {@link #subject} (Identifies the patient, group or organization for whom the goal is being established.) 1014 */ 1015 public Reference getSubject() { 1016 if (this.subject == null) 1017 if (Configuration.errorOnAutoCreate()) 1018 throw new Error("Attempt to auto-create Goal.subject"); 1019 else if (Configuration.doAutoCreate()) 1020 this.subject = new Reference(); // cc 1021 return this.subject; 1022 } 1023 1024 public boolean hasSubject() { 1025 return this.subject != null && !this.subject.isEmpty(); 1026 } 1027 1028 /** 1029 * @param value {@link #subject} (Identifies the patient, group or organization for whom the goal is being established.) 1030 */ 1031 public Goal setSubject(Reference value) { 1032 this.subject = value; 1033 return this; 1034 } 1035 1036 /** 1037 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the patient, group or organization for whom the goal is being established.) 1038 */ 1039 public Resource getSubjectTarget() { 1040 return this.subjectTarget; 1041 } 1042 1043 /** 1044 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the patient, group or organization for whom the goal is being established.) 1045 */ 1046 public Goal setSubjectTarget(Resource value) { 1047 this.subjectTarget = value; 1048 return this; 1049 } 1050 1051 /** 1052 * @return {@link #start} (The date or event after which the goal should begin being pursued.) 1053 */ 1054 public Type getStart() { 1055 return this.start; 1056 } 1057 1058 /** 1059 * @return {@link #start} (The date or event after which the goal should begin being pursued.) 1060 */ 1061 public DateType getStartDateType() throws FHIRException { 1062 if (this.start == null) 1063 return null; 1064 if (!(this.start instanceof DateType)) 1065 throw new FHIRException("Type mismatch: the type DateType was expected, but "+this.start.getClass().getName()+" was encountered"); 1066 return (DateType) this.start; 1067 } 1068 1069 public boolean hasStartDateType() { 1070 return this != null && this.start instanceof DateType; 1071 } 1072 1073 /** 1074 * @return {@link #start} (The date or event after which the goal should begin being pursued.) 1075 */ 1076 public CodeableConcept getStartCodeableConcept() throws FHIRException { 1077 if (this.start == null) 1078 return null; 1079 if (!(this.start instanceof CodeableConcept)) 1080 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.start.getClass().getName()+" was encountered"); 1081 return (CodeableConcept) this.start; 1082 } 1083 1084 public boolean hasStartCodeableConcept() { 1085 return this != null && this.start instanceof CodeableConcept; 1086 } 1087 1088 public boolean hasStart() { 1089 return this.start != null && !this.start.isEmpty(); 1090 } 1091 1092 /** 1093 * @param value {@link #start} (The date or event after which the goal should begin being pursued.) 1094 */ 1095 public Goal setStart(Type value) throws FHIRFormatError { 1096 if (value != null && !(value instanceof DateType || value instanceof CodeableConcept)) 1097 throw new FHIRFormatError("Not the right type for Goal.start[x]: "+value.fhirType()); 1098 this.start = value; 1099 return this; 1100 } 1101 1102 /** 1103 * @return {@link #target} (Indicates what should be done by when.) 1104 */ 1105 public GoalTargetComponent getTarget() { 1106 if (this.target == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create Goal.target"); 1109 else if (Configuration.doAutoCreate()) 1110 this.target = new GoalTargetComponent(); // cc 1111 return this.target; 1112 } 1113 1114 public boolean hasTarget() { 1115 return this.target != null && !this.target.isEmpty(); 1116 } 1117 1118 /** 1119 * @param value {@link #target} (Indicates what should be done by when.) 1120 */ 1121 public Goal setTarget(GoalTargetComponent value) { 1122 this.target = value; 1123 return this; 1124 } 1125 1126 /** 1127 * @return {@link #statusDate} (Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 1128 */ 1129 public DateType getStatusDateElement() { 1130 if (this.statusDate == null) 1131 if (Configuration.errorOnAutoCreate()) 1132 throw new Error("Attempt to auto-create Goal.statusDate"); 1133 else if (Configuration.doAutoCreate()) 1134 this.statusDate = new DateType(); // bb 1135 return this.statusDate; 1136 } 1137 1138 public boolean hasStatusDateElement() { 1139 return this.statusDate != null && !this.statusDate.isEmpty(); 1140 } 1141 1142 public boolean hasStatusDate() { 1143 return this.statusDate != null && !this.statusDate.isEmpty(); 1144 } 1145 1146 /** 1147 * @param value {@link #statusDate} (Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.). This is the underlying object with id, value and extensions. The accessor "getStatusDate" gives direct access to the value 1148 */ 1149 public Goal setStatusDateElement(DateType value) { 1150 this.statusDate = value; 1151 return this; 1152 } 1153 1154 /** 1155 * @return Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc. 1156 */ 1157 public Date getStatusDate() { 1158 return this.statusDate == null ? null : this.statusDate.getValue(); 1159 } 1160 1161 /** 1162 * @param value Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc. 1163 */ 1164 public Goal setStatusDate(Date value) { 1165 if (value == null) 1166 this.statusDate = null; 1167 else { 1168 if (this.statusDate == null) 1169 this.statusDate = new DateType(); 1170 this.statusDate.setValue(value); 1171 } 1172 return this; 1173 } 1174 1175 /** 1176 * @return {@link #statusReason} (Captures the reason for the current status.). This is the underlying object with id, value and extensions. The accessor "getStatusReason" gives direct access to the value 1177 */ 1178 public StringType getStatusReasonElement() { 1179 if (this.statusReason == null) 1180 if (Configuration.errorOnAutoCreate()) 1181 throw new Error("Attempt to auto-create Goal.statusReason"); 1182 else if (Configuration.doAutoCreate()) 1183 this.statusReason = new StringType(); // bb 1184 return this.statusReason; 1185 } 1186 1187 public boolean hasStatusReasonElement() { 1188 return this.statusReason != null && !this.statusReason.isEmpty(); 1189 } 1190 1191 public boolean hasStatusReason() { 1192 return this.statusReason != null && !this.statusReason.isEmpty(); 1193 } 1194 1195 /** 1196 * @param value {@link #statusReason} (Captures the reason for the current status.). This is the underlying object with id, value and extensions. The accessor "getStatusReason" gives direct access to the value 1197 */ 1198 public Goal setStatusReasonElement(StringType value) { 1199 this.statusReason = value; 1200 return this; 1201 } 1202 1203 /** 1204 * @return Captures the reason for the current status. 1205 */ 1206 public String getStatusReason() { 1207 return this.statusReason == null ? null : this.statusReason.getValue(); 1208 } 1209 1210 /** 1211 * @param value Captures the reason for the current status. 1212 */ 1213 public Goal setStatusReason(String value) { 1214 if (Utilities.noString(value)) 1215 this.statusReason = null; 1216 else { 1217 if (this.statusReason == null) 1218 this.statusReason = new StringType(); 1219 this.statusReason.setValue(value); 1220 } 1221 return this; 1222 } 1223 1224 /** 1225 * @return {@link #expressedBy} (Indicates whose goal this is - patient goal, practitioner goal, etc.) 1226 */ 1227 public Reference getExpressedBy() { 1228 if (this.expressedBy == null) 1229 if (Configuration.errorOnAutoCreate()) 1230 throw new Error("Attempt to auto-create Goal.expressedBy"); 1231 else if (Configuration.doAutoCreate()) 1232 this.expressedBy = new Reference(); // cc 1233 return this.expressedBy; 1234 } 1235 1236 public boolean hasExpressedBy() { 1237 return this.expressedBy != null && !this.expressedBy.isEmpty(); 1238 } 1239 1240 /** 1241 * @param value {@link #expressedBy} (Indicates whose goal this is - patient goal, practitioner goal, etc.) 1242 */ 1243 public Goal setExpressedBy(Reference value) { 1244 this.expressedBy = value; 1245 return this; 1246 } 1247 1248 /** 1249 * @return {@link #expressedBy} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates whose goal this is - patient goal, practitioner goal, etc.) 1250 */ 1251 public Resource getExpressedByTarget() { 1252 return this.expressedByTarget; 1253 } 1254 1255 /** 1256 * @param value {@link #expressedBy} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates whose goal this is - patient goal, practitioner goal, etc.) 1257 */ 1258 public Goal setExpressedByTarget(Resource value) { 1259 this.expressedByTarget = value; 1260 return this; 1261 } 1262 1263 /** 1264 * @return {@link #addresses} (The identified conditions and other health record elements that are intended to be addressed by the goal.) 1265 */ 1266 public List<Reference> getAddresses() { 1267 if (this.addresses == null) 1268 this.addresses = new ArrayList<Reference>(); 1269 return this.addresses; 1270 } 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public Goal setAddresses(List<Reference> theAddresses) { 1276 this.addresses = theAddresses; 1277 return this; 1278 } 1279 1280 public boolean hasAddresses() { 1281 if (this.addresses == null) 1282 return false; 1283 for (Reference item : this.addresses) 1284 if (!item.isEmpty()) 1285 return true; 1286 return false; 1287 } 1288 1289 public Reference addAddresses() { //3 1290 Reference t = new Reference(); 1291 if (this.addresses == null) 1292 this.addresses = new ArrayList<Reference>(); 1293 this.addresses.add(t); 1294 return t; 1295 } 1296 1297 public Goal addAddresses(Reference t) { //3 1298 if (t == null) 1299 return this; 1300 if (this.addresses == null) 1301 this.addresses = new ArrayList<Reference>(); 1302 this.addresses.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field {@link #addresses}, creating it if it does not already exist 1308 */ 1309 public Reference getAddressesFirstRep() { 1310 if (getAddresses().isEmpty()) { 1311 addAddresses(); 1312 } 1313 return getAddresses().get(0); 1314 } 1315 1316 /** 1317 * @deprecated Use Reference#setResource(IBaseResource) instead 1318 */ 1319 @Deprecated 1320 public List<Resource> getAddressesTarget() { 1321 if (this.addressesTarget == null) 1322 this.addressesTarget = new ArrayList<Resource>(); 1323 return this.addressesTarget; 1324 } 1325 1326 /** 1327 * @return {@link #note} (Any comments related to the goal.) 1328 */ 1329 public List<Annotation> getNote() { 1330 if (this.note == null) 1331 this.note = new ArrayList<Annotation>(); 1332 return this.note; 1333 } 1334 1335 /** 1336 * @return Returns a reference to <code>this</code> for easy method chaining 1337 */ 1338 public Goal setNote(List<Annotation> theNote) { 1339 this.note = theNote; 1340 return this; 1341 } 1342 1343 public boolean hasNote() { 1344 if (this.note == null) 1345 return false; 1346 for (Annotation item : this.note) 1347 if (!item.isEmpty()) 1348 return true; 1349 return false; 1350 } 1351 1352 public Annotation addNote() { //3 1353 Annotation t = new Annotation(); 1354 if (this.note == null) 1355 this.note = new ArrayList<Annotation>(); 1356 this.note.add(t); 1357 return t; 1358 } 1359 1360 public Goal addNote(Annotation t) { //3 1361 if (t == null) 1362 return this; 1363 if (this.note == null) 1364 this.note = new ArrayList<Annotation>(); 1365 this.note.add(t); 1366 return this; 1367 } 1368 1369 /** 1370 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1371 */ 1372 public Annotation getNoteFirstRep() { 1373 if (getNote().isEmpty()) { 1374 addNote(); 1375 } 1376 return getNote().get(0); 1377 } 1378 1379 /** 1380 * @return {@link #outcomeCode} (Identifies the change (or lack of change) at the point when the status of the goal is assessed.) 1381 */ 1382 public List<CodeableConcept> getOutcomeCode() { 1383 if (this.outcomeCode == null) 1384 this.outcomeCode = new ArrayList<CodeableConcept>(); 1385 return this.outcomeCode; 1386 } 1387 1388 /** 1389 * @return Returns a reference to <code>this</code> for easy method chaining 1390 */ 1391 public Goal setOutcomeCode(List<CodeableConcept> theOutcomeCode) { 1392 this.outcomeCode = theOutcomeCode; 1393 return this; 1394 } 1395 1396 public boolean hasOutcomeCode() { 1397 if (this.outcomeCode == null) 1398 return false; 1399 for (CodeableConcept item : this.outcomeCode) 1400 if (!item.isEmpty()) 1401 return true; 1402 return false; 1403 } 1404 1405 public CodeableConcept addOutcomeCode() { //3 1406 CodeableConcept t = new CodeableConcept(); 1407 if (this.outcomeCode == null) 1408 this.outcomeCode = new ArrayList<CodeableConcept>(); 1409 this.outcomeCode.add(t); 1410 return t; 1411 } 1412 1413 public Goal addOutcomeCode(CodeableConcept t) { //3 1414 if (t == null) 1415 return this; 1416 if (this.outcomeCode == null) 1417 this.outcomeCode = new ArrayList<CodeableConcept>(); 1418 this.outcomeCode.add(t); 1419 return this; 1420 } 1421 1422 /** 1423 * @return The first repetition of repeating field {@link #outcomeCode}, creating it if it does not already exist 1424 */ 1425 public CodeableConcept getOutcomeCodeFirstRep() { 1426 if (getOutcomeCode().isEmpty()) { 1427 addOutcomeCode(); 1428 } 1429 return getOutcomeCode().get(0); 1430 } 1431 1432 /** 1433 * @return {@link #outcomeReference} (Details of what's changed (or not changed).) 1434 */ 1435 public List<Reference> getOutcomeReference() { 1436 if (this.outcomeReference == null) 1437 this.outcomeReference = new ArrayList<Reference>(); 1438 return this.outcomeReference; 1439 } 1440 1441 /** 1442 * @return Returns a reference to <code>this</code> for easy method chaining 1443 */ 1444 public Goal setOutcomeReference(List<Reference> theOutcomeReference) { 1445 this.outcomeReference = theOutcomeReference; 1446 return this; 1447 } 1448 1449 public boolean hasOutcomeReference() { 1450 if (this.outcomeReference == null) 1451 return false; 1452 for (Reference item : this.outcomeReference) 1453 if (!item.isEmpty()) 1454 return true; 1455 return false; 1456 } 1457 1458 public Reference addOutcomeReference() { //3 1459 Reference t = new Reference(); 1460 if (this.outcomeReference == null) 1461 this.outcomeReference = new ArrayList<Reference>(); 1462 this.outcomeReference.add(t); 1463 return t; 1464 } 1465 1466 public Goal addOutcomeReference(Reference t) { //3 1467 if (t == null) 1468 return this; 1469 if (this.outcomeReference == null) 1470 this.outcomeReference = new ArrayList<Reference>(); 1471 this.outcomeReference.add(t); 1472 return this; 1473 } 1474 1475 /** 1476 * @return The first repetition of repeating field {@link #outcomeReference}, creating it if it does not already exist 1477 */ 1478 public Reference getOutcomeReferenceFirstRep() { 1479 if (getOutcomeReference().isEmpty()) { 1480 addOutcomeReference(); 1481 } 1482 return getOutcomeReference().get(0); 1483 } 1484 1485 /** 1486 * @deprecated Use Reference#setResource(IBaseResource) instead 1487 */ 1488 @Deprecated 1489 public List<Observation> getOutcomeReferenceTarget() { 1490 if (this.outcomeReferenceTarget == null) 1491 this.outcomeReferenceTarget = new ArrayList<Observation>(); 1492 return this.outcomeReferenceTarget; 1493 } 1494 1495 /** 1496 * @deprecated Use Reference#setResource(IBaseResource) instead 1497 */ 1498 @Deprecated 1499 public Observation addOutcomeReferenceTarget() { 1500 Observation r = new Observation(); 1501 if (this.outcomeReferenceTarget == null) 1502 this.outcomeReferenceTarget = new ArrayList<Observation>(); 1503 this.outcomeReferenceTarget.add(r); 1504 return r; 1505 } 1506 1507 protected void listChildren(List<Property> children) { 1508 super.listChildren(children); 1509 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier)); 1510 children.add(new Property("status", "code", "Indicates whether the goal has been reached and is still considered relevant.", 0, 1, status)); 1511 children.add(new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, java.lang.Integer.MAX_VALUE, category)); 1512 children.add(new Property("priority", "CodeableConcept", "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.", 0, 1, priority)); 1513 children.add(new Property("description", "CodeableConcept", "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 0, 1, description)); 1514 children.add(new Property("subject", "Reference(Patient|Group|Organization)", "Identifies the patient, group or organization for whom the goal is being established.", 0, 1, subject)); 1515 children.add(new Property("start[x]", "date|CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start)); 1516 children.add(new Property("target", "", "Indicates what should be done by when.", 0, 1, target)); 1517 children.add(new Property("statusDate", "date", "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.", 0, 1, statusDate)); 1518 children.add(new Property("statusReason", "string", "Captures the reason for the current status.", 0, 1, statusReason)); 1519 children.add(new Property("expressedBy", "Reference(Patient|Practitioner|RelatedPerson)", "Indicates whose goal this is - patient goal, practitioner goal, etc.", 0, 1, expressedBy)); 1520 children.add(new Property("addresses", "Reference(Condition|Observation|MedicationStatement|NutritionOrder|ProcedureRequest|RiskAssessment)", "The identified conditions and other health record elements that are intended to be addressed by the goal.", 0, java.lang.Integer.MAX_VALUE, addresses)); 1521 children.add(new Property("note", "Annotation", "Any comments related to the goal.", 0, java.lang.Integer.MAX_VALUE, note)); 1522 children.add(new Property("outcomeCode", "CodeableConcept", "Identifies the change (or lack of change) at the point when the status of the goal is assessed.", 0, java.lang.Integer.MAX_VALUE, outcomeCode)); 1523 children.add(new Property("outcomeReference", "Reference(Observation)", "Details of what's changed (or not changed).", 0, java.lang.Integer.MAX_VALUE, outcomeReference)); 1524 } 1525 1526 @Override 1527 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1528 switch (_hash) { 1529 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this care plan that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate (e.g. in CDA documents, or in written / printed documentation).", 0, java.lang.Integer.MAX_VALUE, identifier); 1530 case -892481550: /*status*/ return new Property("status", "code", "Indicates whether the goal has been reached and is still considered relevant.", 0, 1, status); 1531 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates a category the goal falls within.", 0, java.lang.Integer.MAX_VALUE, category); 1532 case -1165461084: /*priority*/ return new Property("priority", "CodeableConcept", "Identifies the mutually agreed level of importance associated with reaching/sustaining the goal.", 0, 1, priority); 1533 case -1724546052: /*description*/ return new Property("description", "CodeableConcept", "Human-readable and/or coded description of a specific desired objective of care, such as \"control blood pressure\" or \"negotiate an obstacle course\" or \"dance with child at wedding\".", 0, 1, description); 1534 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Organization)", "Identifies the patient, group or organization for whom the goal is being established.", 0, 1, subject); 1535 case 1316793566: /*start[x]*/ return new Property("start[x]", "date|CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start); 1536 case 109757538: /*start*/ return new Property("start[x]", "date|CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start); 1537 case -2129778896: /*startDate*/ return new Property("start[x]", "date|CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start); 1538 case -1758833953: /*startCodeableConcept*/ return new Property("start[x]", "date|CodeableConcept", "The date or event after which the goal should begin being pursued.", 0, 1, start); 1539 case -880905839: /*target*/ return new Property("target", "", "Indicates what should be done by when.", 0, 1, target); 1540 case 247524032: /*statusDate*/ return new Property("statusDate", "date", "Identifies when the current status. I.e. When initially created, when achieved, when cancelled, etc.", 0, 1, statusDate); 1541 case 2051346646: /*statusReason*/ return new Property("statusReason", "string", "Captures the reason for the current status.", 0, 1, statusReason); 1542 case 175423686: /*expressedBy*/ return new Property("expressedBy", "Reference(Patient|Practitioner|RelatedPerson)", "Indicates whose goal this is - patient goal, practitioner goal, etc.", 0, 1, expressedBy); 1543 case 874544034: /*addresses*/ return new Property("addresses", "Reference(Condition|Observation|MedicationStatement|NutritionOrder|ProcedureRequest|RiskAssessment)", "The identified conditions and other health record elements that are intended to be addressed by the goal.", 0, java.lang.Integer.MAX_VALUE, addresses); 1544 case 3387378: /*note*/ return new Property("note", "Annotation", "Any comments related to the goal.", 0, java.lang.Integer.MAX_VALUE, note); 1545 case 1062482015: /*outcomeCode*/ return new Property("outcomeCode", "CodeableConcept", "Identifies the change (or lack of change) at the point when the status of the goal is assessed.", 0, java.lang.Integer.MAX_VALUE, outcomeCode); 1546 case -782273511: /*outcomeReference*/ return new Property("outcomeReference", "Reference(Observation)", "Details of what's changed (or not changed).", 0, java.lang.Integer.MAX_VALUE, outcomeReference); 1547 default: return super.getNamedProperty(_hash, _name, _checkValid); 1548 } 1549 1550 } 1551 1552 @Override 1553 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1554 switch (hash) { 1555 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1556 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<GoalStatus> 1557 case 50511102: /*category*/ return this.category == null ? new Base[0] : this.category.toArray(new Base[this.category.size()]); // CodeableConcept 1558 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // CodeableConcept 1559 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // CodeableConcept 1560 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1561 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // Type 1562 case -880905839: /*target*/ return this.target == null ? new Base[0] : new Base[] {this.target}; // GoalTargetComponent 1563 case 247524032: /*statusDate*/ return this.statusDate == null ? new Base[0] : new Base[] {this.statusDate}; // DateType 1564 case 2051346646: /*statusReason*/ return this.statusReason == null ? new Base[0] : new Base[] {this.statusReason}; // StringType 1565 case 175423686: /*expressedBy*/ return this.expressedBy == null ? new Base[0] : new Base[] {this.expressedBy}; // Reference 1566 case 874544034: /*addresses*/ return this.addresses == null ? new Base[0] : this.addresses.toArray(new Base[this.addresses.size()]); // Reference 1567 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1568 case 1062482015: /*outcomeCode*/ return this.outcomeCode == null ? new Base[0] : this.outcomeCode.toArray(new Base[this.outcomeCode.size()]); // CodeableConcept 1569 case -782273511: /*outcomeReference*/ return this.outcomeReference == null ? new Base[0] : this.outcomeReference.toArray(new Base[this.outcomeReference.size()]); // Reference 1570 default: return super.getProperty(hash, name, checkValid); 1571 } 1572 1573 } 1574 1575 @Override 1576 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1577 switch (hash) { 1578 case -1618432855: // identifier 1579 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1580 return value; 1581 case -892481550: // status 1582 value = new GoalStatusEnumFactory().fromType(castToCode(value)); 1583 this.status = (Enumeration) value; // Enumeration<GoalStatus> 1584 return value; 1585 case 50511102: // category 1586 this.getCategory().add(castToCodeableConcept(value)); // CodeableConcept 1587 return value; 1588 case -1165461084: // priority 1589 this.priority = castToCodeableConcept(value); // CodeableConcept 1590 return value; 1591 case -1724546052: // description 1592 this.description = castToCodeableConcept(value); // CodeableConcept 1593 return value; 1594 case -1867885268: // subject 1595 this.subject = castToReference(value); // Reference 1596 return value; 1597 case 109757538: // start 1598 this.start = castToType(value); // Type 1599 return value; 1600 case -880905839: // target 1601 this.target = (GoalTargetComponent) value; // GoalTargetComponent 1602 return value; 1603 case 247524032: // statusDate 1604 this.statusDate = castToDate(value); // DateType 1605 return value; 1606 case 2051346646: // statusReason 1607 this.statusReason = castToString(value); // StringType 1608 return value; 1609 case 175423686: // expressedBy 1610 this.expressedBy = castToReference(value); // Reference 1611 return value; 1612 case 874544034: // addresses 1613 this.getAddresses().add(castToReference(value)); // Reference 1614 return value; 1615 case 3387378: // note 1616 this.getNote().add(castToAnnotation(value)); // Annotation 1617 return value; 1618 case 1062482015: // outcomeCode 1619 this.getOutcomeCode().add(castToCodeableConcept(value)); // CodeableConcept 1620 return value; 1621 case -782273511: // outcomeReference 1622 this.getOutcomeReference().add(castToReference(value)); // Reference 1623 return value; 1624 default: return super.setProperty(hash, name, value); 1625 } 1626 1627 } 1628 1629 @Override 1630 public Base setProperty(String name, Base value) throws FHIRException { 1631 if (name.equals("identifier")) { 1632 this.getIdentifier().add(castToIdentifier(value)); 1633 } else if (name.equals("status")) { 1634 value = new GoalStatusEnumFactory().fromType(castToCode(value)); 1635 this.status = (Enumeration) value; // Enumeration<GoalStatus> 1636 } else if (name.equals("category")) { 1637 this.getCategory().add(castToCodeableConcept(value)); 1638 } else if (name.equals("priority")) { 1639 this.priority = castToCodeableConcept(value); // CodeableConcept 1640 } else if (name.equals("description")) { 1641 this.description = castToCodeableConcept(value); // CodeableConcept 1642 } else if (name.equals("subject")) { 1643 this.subject = castToReference(value); // Reference 1644 } else if (name.equals("start[x]")) { 1645 this.start = castToType(value); // Type 1646 } else if (name.equals("target")) { 1647 this.target = (GoalTargetComponent) value; // GoalTargetComponent 1648 } else if (name.equals("statusDate")) { 1649 this.statusDate = castToDate(value); // DateType 1650 } else if (name.equals("statusReason")) { 1651 this.statusReason = castToString(value); // StringType 1652 } else if (name.equals("expressedBy")) { 1653 this.expressedBy = castToReference(value); // Reference 1654 } else if (name.equals("addresses")) { 1655 this.getAddresses().add(castToReference(value)); 1656 } else if (name.equals("note")) { 1657 this.getNote().add(castToAnnotation(value)); 1658 } else if (name.equals("outcomeCode")) { 1659 this.getOutcomeCode().add(castToCodeableConcept(value)); 1660 } else if (name.equals("outcomeReference")) { 1661 this.getOutcomeReference().add(castToReference(value)); 1662 } else 1663 return super.setProperty(name, value); 1664 return value; 1665 } 1666 1667 @Override 1668 public Base makeProperty(int hash, String name) throws FHIRException { 1669 switch (hash) { 1670 case -1618432855: return addIdentifier(); 1671 case -892481550: return getStatusElement(); 1672 case 50511102: return addCategory(); 1673 case -1165461084: return getPriority(); 1674 case -1724546052: return getDescription(); 1675 case -1867885268: return getSubject(); 1676 case 1316793566: return getStart(); 1677 case 109757538: return getStart(); 1678 case -880905839: return getTarget(); 1679 case 247524032: return getStatusDateElement(); 1680 case 2051346646: return getStatusReasonElement(); 1681 case 175423686: return getExpressedBy(); 1682 case 874544034: return addAddresses(); 1683 case 3387378: return addNote(); 1684 case 1062482015: return addOutcomeCode(); 1685 case -782273511: return addOutcomeReference(); 1686 default: return super.makeProperty(hash, name); 1687 } 1688 1689 } 1690 1691 @Override 1692 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1693 switch (hash) { 1694 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1695 case -892481550: /*status*/ return new String[] {"code"}; 1696 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1697 case -1165461084: /*priority*/ return new String[] {"CodeableConcept"}; 1698 case -1724546052: /*description*/ return new String[] {"CodeableConcept"}; 1699 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1700 case 109757538: /*start*/ return new String[] {"date", "CodeableConcept"}; 1701 case -880905839: /*target*/ return new String[] {}; 1702 case 247524032: /*statusDate*/ return new String[] {"date"}; 1703 case 2051346646: /*statusReason*/ return new String[] {"string"}; 1704 case 175423686: /*expressedBy*/ return new String[] {"Reference"}; 1705 case 874544034: /*addresses*/ return new String[] {"Reference"}; 1706 case 3387378: /*note*/ return new String[] {"Annotation"}; 1707 case 1062482015: /*outcomeCode*/ return new String[] {"CodeableConcept"}; 1708 case -782273511: /*outcomeReference*/ return new String[] {"Reference"}; 1709 default: return super.getTypesForProperty(hash, name); 1710 } 1711 1712 } 1713 1714 @Override 1715 public Base addChild(String name) throws FHIRException { 1716 if (name.equals("identifier")) { 1717 return addIdentifier(); 1718 } 1719 else if (name.equals("status")) { 1720 throw new FHIRException("Cannot call addChild on a singleton property Goal.status"); 1721 } 1722 else if (name.equals("category")) { 1723 return addCategory(); 1724 } 1725 else if (name.equals("priority")) { 1726 this.priority = new CodeableConcept(); 1727 return this.priority; 1728 } 1729 else if (name.equals("description")) { 1730 this.description = new CodeableConcept(); 1731 return this.description; 1732 } 1733 else if (name.equals("subject")) { 1734 this.subject = new Reference(); 1735 return this.subject; 1736 } 1737 else if (name.equals("startDate")) { 1738 this.start = new DateType(); 1739 return this.start; 1740 } 1741 else if (name.equals("startCodeableConcept")) { 1742 this.start = new CodeableConcept(); 1743 return this.start; 1744 } 1745 else if (name.equals("target")) { 1746 this.target = new GoalTargetComponent(); 1747 return this.target; 1748 } 1749 else if (name.equals("statusDate")) { 1750 throw new FHIRException("Cannot call addChild on a singleton property Goal.statusDate"); 1751 } 1752 else if (name.equals("statusReason")) { 1753 throw new FHIRException("Cannot call addChild on a singleton property Goal.statusReason"); 1754 } 1755 else if (name.equals("expressedBy")) { 1756 this.expressedBy = new Reference(); 1757 return this.expressedBy; 1758 } 1759 else if (name.equals("addresses")) { 1760 return addAddresses(); 1761 } 1762 else if (name.equals("note")) { 1763 return addNote(); 1764 } 1765 else if (name.equals("outcomeCode")) { 1766 return addOutcomeCode(); 1767 } 1768 else if (name.equals("outcomeReference")) { 1769 return addOutcomeReference(); 1770 } 1771 else 1772 return super.addChild(name); 1773 } 1774 1775 public String fhirType() { 1776 return "Goal"; 1777 1778 } 1779 1780 public Goal copy() { 1781 Goal dst = new Goal(); 1782 copyValues(dst); 1783 if (identifier != null) { 1784 dst.identifier = new ArrayList<Identifier>(); 1785 for (Identifier i : identifier) 1786 dst.identifier.add(i.copy()); 1787 }; 1788 dst.status = status == null ? null : status.copy(); 1789 if (category != null) { 1790 dst.category = new ArrayList<CodeableConcept>(); 1791 for (CodeableConcept i : category) 1792 dst.category.add(i.copy()); 1793 }; 1794 dst.priority = priority == null ? null : priority.copy(); 1795 dst.description = description == null ? null : description.copy(); 1796 dst.subject = subject == null ? null : subject.copy(); 1797 dst.start = start == null ? null : start.copy(); 1798 dst.target = target == null ? null : target.copy(); 1799 dst.statusDate = statusDate == null ? null : statusDate.copy(); 1800 dst.statusReason = statusReason == null ? null : statusReason.copy(); 1801 dst.expressedBy = expressedBy == null ? null : expressedBy.copy(); 1802 if (addresses != null) { 1803 dst.addresses = new ArrayList<Reference>(); 1804 for (Reference i : addresses) 1805 dst.addresses.add(i.copy()); 1806 }; 1807 if (note != null) { 1808 dst.note = new ArrayList<Annotation>(); 1809 for (Annotation i : note) 1810 dst.note.add(i.copy()); 1811 }; 1812 if (outcomeCode != null) { 1813 dst.outcomeCode = new ArrayList<CodeableConcept>(); 1814 for (CodeableConcept i : outcomeCode) 1815 dst.outcomeCode.add(i.copy()); 1816 }; 1817 if (outcomeReference != null) { 1818 dst.outcomeReference = new ArrayList<Reference>(); 1819 for (Reference i : outcomeReference) 1820 dst.outcomeReference.add(i.copy()); 1821 }; 1822 return dst; 1823 } 1824 1825 protected Goal typedCopy() { 1826 return copy(); 1827 } 1828 1829 @Override 1830 public boolean equalsDeep(Base other_) { 1831 if (!super.equalsDeep(other_)) 1832 return false; 1833 if (!(other_ instanceof Goal)) 1834 return false; 1835 Goal o = (Goal) other_; 1836 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1837 && compareDeep(priority, o.priority, true) && compareDeep(description, o.description, true) && compareDeep(subject, o.subject, true) 1838 && compareDeep(start, o.start, true) && compareDeep(target, o.target, true) && compareDeep(statusDate, o.statusDate, true) 1839 && compareDeep(statusReason, o.statusReason, true) && compareDeep(expressedBy, o.expressedBy, true) 1840 && compareDeep(addresses, o.addresses, true) && compareDeep(note, o.note, true) && compareDeep(outcomeCode, o.outcomeCode, true) 1841 && compareDeep(outcomeReference, o.outcomeReference, true); 1842 } 1843 1844 @Override 1845 public boolean equalsShallow(Base other_) { 1846 if (!super.equalsShallow(other_)) 1847 return false; 1848 if (!(other_ instanceof Goal)) 1849 return false; 1850 Goal o = (Goal) other_; 1851 return compareValues(status, o.status, true) && compareValues(statusDate, o.statusDate, true) && compareValues(statusReason, o.statusReason, true) 1852 ; 1853 } 1854 1855 public boolean isEmpty() { 1856 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, category 1857 , priority, description, subject, start, target, statusDate, statusReason, expressedBy 1858 , addresses, note, outcomeCode, outcomeReference); 1859 } 1860 1861 @Override 1862 public ResourceType getResourceType() { 1863 return ResourceType.Goal; 1864 } 1865 1866 /** 1867 * Search parameter: <b>identifier</b> 1868 * <p> 1869 * Description: <b>External Ids for this goal</b><br> 1870 * Type: <b>token</b><br> 1871 * Path: <b>Goal.identifier</b><br> 1872 * </p> 1873 */ 1874 @SearchParamDefinition(name="identifier", path="Goal.identifier", description="External Ids for this goal", type="token" ) 1875 public static final String SP_IDENTIFIER = "identifier"; 1876 /** 1877 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1878 * <p> 1879 * Description: <b>External Ids for this goal</b><br> 1880 * Type: <b>token</b><br> 1881 * Path: <b>Goal.identifier</b><br> 1882 * </p> 1883 */ 1884 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1885 1886 /** 1887 * Search parameter: <b>patient</b> 1888 * <p> 1889 * Description: <b>Who this goal is intended for</b><br> 1890 * Type: <b>reference</b><br> 1891 * Path: <b>Goal.subject</b><br> 1892 * </p> 1893 */ 1894 @SearchParamDefinition(name="patient", path="Goal.subject", description="Who this goal is intended for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1895 public static final String SP_PATIENT = "patient"; 1896 /** 1897 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1898 * <p> 1899 * Description: <b>Who this goal is intended for</b><br> 1900 * Type: <b>reference</b><br> 1901 * Path: <b>Goal.subject</b><br> 1902 * </p> 1903 */ 1904 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1905 1906/** 1907 * Constant for fluent queries to be used to add include statements. Specifies 1908 * the path value of "<b>Goal:patient</b>". 1909 */ 1910 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Goal:patient").toLocked(); 1911 1912 /** 1913 * Search parameter: <b>subject</b> 1914 * <p> 1915 * Description: <b>Who this goal is intended for</b><br> 1916 * Type: <b>reference</b><br> 1917 * Path: <b>Goal.subject</b><br> 1918 * </p> 1919 */ 1920 @SearchParamDefinition(name="subject", path="Goal.subject", description="Who this goal is intended for", type="reference", target={Group.class, Organization.class, Patient.class } ) 1921 public static final String SP_SUBJECT = "subject"; 1922 /** 1923 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1924 * <p> 1925 * Description: <b>Who this goal is intended for</b><br> 1926 * Type: <b>reference</b><br> 1927 * Path: <b>Goal.subject</b><br> 1928 * </p> 1929 */ 1930 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1931 1932/** 1933 * Constant for fluent queries to be used to add include statements. Specifies 1934 * the path value of "<b>Goal:subject</b>". 1935 */ 1936 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Goal:subject").toLocked(); 1937 1938 /** 1939 * Search parameter: <b>start-date</b> 1940 * <p> 1941 * Description: <b>When goal pursuit begins</b><br> 1942 * Type: <b>date</b><br> 1943 * Path: <b>Goal.startDate</b><br> 1944 * </p> 1945 */ 1946 @SearchParamDefinition(name="start-date", path="Goal.start.as(Date)", description="When goal pursuit begins", type="date" ) 1947 public static final String SP_START_DATE = "start-date"; 1948 /** 1949 * <b>Fluent Client</b> search parameter constant for <b>start-date</b> 1950 * <p> 1951 * Description: <b>When goal pursuit begins</b><br> 1952 * Type: <b>date</b><br> 1953 * Path: <b>Goal.startDate</b><br> 1954 * </p> 1955 */ 1956 public static final ca.uhn.fhir.rest.gclient.DateClientParam START_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_START_DATE); 1957 1958 /** 1959 * Search parameter: <b>category</b> 1960 * <p> 1961 * Description: <b>E.g. Treatment, dietary, behavioral, etc.</b><br> 1962 * Type: <b>token</b><br> 1963 * Path: <b>Goal.category</b><br> 1964 * </p> 1965 */ 1966 @SearchParamDefinition(name="category", path="Goal.category", description="E.g. Treatment, dietary, behavioral, etc.", type="token" ) 1967 public static final String SP_CATEGORY = "category"; 1968 /** 1969 * <b>Fluent Client</b> search parameter constant for <b>category</b> 1970 * <p> 1971 * Description: <b>E.g. Treatment, dietary, behavioral, etc.</b><br> 1972 * Type: <b>token</b><br> 1973 * Path: <b>Goal.category</b><br> 1974 * </p> 1975 */ 1976 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 1977 1978 /** 1979 * Search parameter: <b>target-date</b> 1980 * <p> 1981 * Description: <b>Reach goal on or before</b><br> 1982 * Type: <b>date</b><br> 1983 * Path: <b>Goal.target.dueDate</b><br> 1984 * </p> 1985 */ 1986 @SearchParamDefinition(name="target-date", path="Goal.target.due.as(Date)", description="Reach goal on or before", type="date" ) 1987 public static final String SP_TARGET_DATE = "target-date"; 1988 /** 1989 * <b>Fluent Client</b> search parameter constant for <b>target-date</b> 1990 * <p> 1991 * Description: <b>Reach goal on or before</b><br> 1992 * Type: <b>date</b><br> 1993 * Path: <b>Goal.target.dueDate</b><br> 1994 * </p> 1995 */ 1996 public static final ca.uhn.fhir.rest.gclient.DateClientParam TARGET_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_TARGET_DATE); 1997 1998 /** 1999 * Search parameter: <b>status</b> 2000 * <p> 2001 * Description: <b>proposed | accepted | planned | in-progress | on-target | ahead-of-target | behind-target | sustaining | achieved | on-hold | cancelled | entered-in-error | rejected</b><br> 2002 * Type: <b>token</b><br> 2003 * Path: <b>Goal.status</b><br> 2004 * </p> 2005 */ 2006 @SearchParamDefinition(name="status", path="Goal.status", description="proposed | accepted | planned | in-progress | on-target | ahead-of-target | behind-target | sustaining | achieved | on-hold | cancelled | entered-in-error | rejected", type="token" ) 2007 public static final String SP_STATUS = "status"; 2008 /** 2009 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2010 * <p> 2011 * Description: <b>proposed | accepted | planned | in-progress | on-target | ahead-of-target | behind-target | sustaining | achieved | on-hold | cancelled | entered-in-error | rejected</b><br> 2012 * Type: <b>token</b><br> 2013 * Path: <b>Goal.status</b><br> 2014 * </p> 2015 */ 2016 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2017 2018 2019}