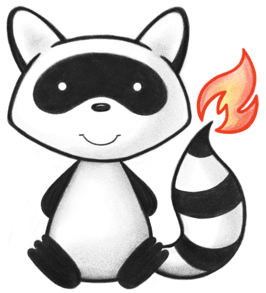
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 041import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.ChildOrder; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.ResourceDef; 051import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 052/** 053 * A formal computable definition of a graph of resources - that is, a coherent set of resources that form a graph by following references. The Graph Definition resource defines a set and makes rules about the set. 054 */ 055@ResourceDef(name="GraphDefinition", profile="http://hl7.org/fhir/Profile/GraphDefinition") 056@ChildOrder(names={"url", "version", "name", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "start", "profile", "link"}) 057public class GraphDefinition extends MetadataResource { 058 059 public enum CompartmentCode { 060 /** 061 * The compartment definition is for the patient compartment 062 */ 063 PATIENT, 064 /** 065 * The compartment definition is for the encounter compartment 066 */ 067 ENCOUNTER, 068 /** 069 * The compartment definition is for the related-person compartment 070 */ 071 RELATEDPERSON, 072 /** 073 * The compartment definition is for the practitioner compartment 074 */ 075 PRACTITIONER, 076 /** 077 * The compartment definition is for the device compartment 078 */ 079 DEVICE, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 public static CompartmentCode fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("Patient".equals(codeString)) 088 return PATIENT; 089 if ("Encounter".equals(codeString)) 090 return ENCOUNTER; 091 if ("RelatedPerson".equals(codeString)) 092 return RELATEDPERSON; 093 if ("Practitioner".equals(codeString)) 094 return PRACTITIONER; 095 if ("Device".equals(codeString)) 096 return DEVICE; 097 if (Configuration.isAcceptInvalidEnums()) 098 return null; 099 else 100 throw new FHIRException("Unknown CompartmentCode code '"+codeString+"'"); 101 } 102 public String toCode() { 103 switch (this) { 104 case PATIENT: return "Patient"; 105 case ENCOUNTER: return "Encounter"; 106 case RELATEDPERSON: return "RelatedPerson"; 107 case PRACTITIONER: return "Practitioner"; 108 case DEVICE: return "Device"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getSystem() { 114 switch (this) { 115 case PATIENT: return "http://hl7.org/fhir/compartment-type"; 116 case ENCOUNTER: return "http://hl7.org/fhir/compartment-type"; 117 case RELATEDPERSON: return "http://hl7.org/fhir/compartment-type"; 118 case PRACTITIONER: return "http://hl7.org/fhir/compartment-type"; 119 case DEVICE: return "http://hl7.org/fhir/compartment-type"; 120 case NULL: return null; 121 default: return "?"; 122 } 123 } 124 public String getDefinition() { 125 switch (this) { 126 case PATIENT: return "The compartment definition is for the patient compartment"; 127 case ENCOUNTER: return "The compartment definition is for the encounter compartment"; 128 case RELATEDPERSON: return "The compartment definition is for the related-person compartment"; 129 case PRACTITIONER: return "The compartment definition is for the practitioner compartment"; 130 case DEVICE: return "The compartment definition is for the device compartment"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDisplay() { 136 switch (this) { 137 case PATIENT: return "Patient"; 138 case ENCOUNTER: return "Encounter"; 139 case RELATEDPERSON: return "RelatedPerson"; 140 case PRACTITIONER: return "Practitioner"; 141 case DEVICE: return "Device"; 142 case NULL: return null; 143 default: return "?"; 144 } 145 } 146 } 147 148 public static class CompartmentCodeEnumFactory implements EnumFactory<CompartmentCode> { 149 public CompartmentCode fromCode(String codeString) throws IllegalArgumentException { 150 if (codeString == null || "".equals(codeString)) 151 if (codeString == null || "".equals(codeString)) 152 return null; 153 if ("Patient".equals(codeString)) 154 return CompartmentCode.PATIENT; 155 if ("Encounter".equals(codeString)) 156 return CompartmentCode.ENCOUNTER; 157 if ("RelatedPerson".equals(codeString)) 158 return CompartmentCode.RELATEDPERSON; 159 if ("Practitioner".equals(codeString)) 160 return CompartmentCode.PRACTITIONER; 161 if ("Device".equals(codeString)) 162 return CompartmentCode.DEVICE; 163 throw new IllegalArgumentException("Unknown CompartmentCode code '"+codeString+"'"); 164 } 165 public Enumeration<CompartmentCode> fromType(PrimitiveType<?> code) throws FHIRException { 166 if (code == null) 167 return null; 168 if (code.isEmpty()) 169 return new Enumeration<CompartmentCode>(this); 170 String codeString = code.asStringValue(); 171 if (codeString == null || "".equals(codeString)) 172 return null; 173 if ("Patient".equals(codeString)) 174 return new Enumeration<CompartmentCode>(this, CompartmentCode.PATIENT); 175 if ("Encounter".equals(codeString)) 176 return new Enumeration<CompartmentCode>(this, CompartmentCode.ENCOUNTER); 177 if ("RelatedPerson".equals(codeString)) 178 return new Enumeration<CompartmentCode>(this, CompartmentCode.RELATEDPERSON); 179 if ("Practitioner".equals(codeString)) 180 return new Enumeration<CompartmentCode>(this, CompartmentCode.PRACTITIONER); 181 if ("Device".equals(codeString)) 182 return new Enumeration<CompartmentCode>(this, CompartmentCode.DEVICE); 183 throw new FHIRException("Unknown CompartmentCode code '"+codeString+"'"); 184 } 185 public String toCode(CompartmentCode code) { 186 if (code == CompartmentCode.NULL) 187 return null; 188 if (code == CompartmentCode.PATIENT) 189 return "Patient"; 190 if (code == CompartmentCode.ENCOUNTER) 191 return "Encounter"; 192 if (code == CompartmentCode.RELATEDPERSON) 193 return "RelatedPerson"; 194 if (code == CompartmentCode.PRACTITIONER) 195 return "Practitioner"; 196 if (code == CompartmentCode.DEVICE) 197 return "Device"; 198 return "?"; 199 } 200 public String toSystem(CompartmentCode code) { 201 return code.getSystem(); 202 } 203 } 204 205 public enum GraphCompartmentRule { 206 /** 207 * The compartment must be identical (the same literal reference) 208 */ 209 IDENTICAL, 210 /** 211 * The compartment must be the same - the record must be about the same patient, but the reference may be different 212 */ 213 MATCHING, 214 /** 215 * The compartment must be different 216 */ 217 DIFFERENT, 218 /** 219 * The compartment rule is defined in the accompanying FHIRPath expression 220 */ 221 CUSTOM, 222 /** 223 * added to help the parsers with the generic types 224 */ 225 NULL; 226 public static GraphCompartmentRule fromCode(String codeString) throws FHIRException { 227 if (codeString == null || "".equals(codeString)) 228 return null; 229 if ("identical".equals(codeString)) 230 return IDENTICAL; 231 if ("matching".equals(codeString)) 232 return MATCHING; 233 if ("different".equals(codeString)) 234 return DIFFERENT; 235 if ("custom".equals(codeString)) 236 return CUSTOM; 237 if (Configuration.isAcceptInvalidEnums()) 238 return null; 239 else 240 throw new FHIRException("Unknown GraphCompartmentRule code '"+codeString+"'"); 241 } 242 public String toCode() { 243 switch (this) { 244 case IDENTICAL: return "identical"; 245 case MATCHING: return "matching"; 246 case DIFFERENT: return "different"; 247 case CUSTOM: return "custom"; 248 case NULL: return null; 249 default: return "?"; 250 } 251 } 252 public String getSystem() { 253 switch (this) { 254 case IDENTICAL: return "http://hl7.org/fhir/graph-compartment-rule"; 255 case MATCHING: return "http://hl7.org/fhir/graph-compartment-rule"; 256 case DIFFERENT: return "http://hl7.org/fhir/graph-compartment-rule"; 257 case CUSTOM: return "http://hl7.org/fhir/graph-compartment-rule"; 258 case NULL: return null; 259 default: return "?"; 260 } 261 } 262 public String getDefinition() { 263 switch (this) { 264 case IDENTICAL: return "The compartment must be identical (the same literal reference)"; 265 case MATCHING: return "The compartment must be the same - the record must be about the same patient, but the reference may be different"; 266 case DIFFERENT: return "The compartment must be different"; 267 case CUSTOM: return "The compartment rule is defined in the accompanying FHIRPath expression"; 268 case NULL: return null; 269 default: return "?"; 270 } 271 } 272 public String getDisplay() { 273 switch (this) { 274 case IDENTICAL: return "Identical"; 275 case MATCHING: return "Matching"; 276 case DIFFERENT: return "Different"; 277 case CUSTOM: return "Custom"; 278 case NULL: return null; 279 default: return "?"; 280 } 281 } 282 } 283 284 public static class GraphCompartmentRuleEnumFactory implements EnumFactory<GraphCompartmentRule> { 285 public GraphCompartmentRule fromCode(String codeString) throws IllegalArgumentException { 286 if (codeString == null || "".equals(codeString)) 287 if (codeString == null || "".equals(codeString)) 288 return null; 289 if ("identical".equals(codeString)) 290 return GraphCompartmentRule.IDENTICAL; 291 if ("matching".equals(codeString)) 292 return GraphCompartmentRule.MATCHING; 293 if ("different".equals(codeString)) 294 return GraphCompartmentRule.DIFFERENT; 295 if ("custom".equals(codeString)) 296 return GraphCompartmentRule.CUSTOM; 297 throw new IllegalArgumentException("Unknown GraphCompartmentRule code '"+codeString+"'"); 298 } 299 public Enumeration<GraphCompartmentRule> fromType(PrimitiveType<?> code) throws FHIRException { 300 if (code == null) 301 return null; 302 if (code.isEmpty()) 303 return new Enumeration<GraphCompartmentRule>(this); 304 String codeString = code.asStringValue(); 305 if (codeString == null || "".equals(codeString)) 306 return null; 307 if ("identical".equals(codeString)) 308 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.IDENTICAL); 309 if ("matching".equals(codeString)) 310 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.MATCHING); 311 if ("different".equals(codeString)) 312 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.DIFFERENT); 313 if ("custom".equals(codeString)) 314 return new Enumeration<GraphCompartmentRule>(this, GraphCompartmentRule.CUSTOM); 315 throw new FHIRException("Unknown GraphCompartmentRule code '"+codeString+"'"); 316 } 317 public String toCode(GraphCompartmentRule code) { 318 if (code == GraphCompartmentRule.NULL) 319 return null; 320 if (code == GraphCompartmentRule.IDENTICAL) 321 return "identical"; 322 if (code == GraphCompartmentRule.MATCHING) 323 return "matching"; 324 if (code == GraphCompartmentRule.DIFFERENT) 325 return "different"; 326 if (code == GraphCompartmentRule.CUSTOM) 327 return "custom"; 328 return "?"; 329 } 330 public String toSystem(GraphCompartmentRule code) { 331 return code.getSystem(); 332 } 333 } 334 335 @Block() 336 public static class GraphDefinitionLinkComponent extends BackboneElement implements IBaseBackboneElement { 337 /** 338 * Path in the resource that contains the link. 339 */ 340 @Child(name = "path", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 341 @Description(shortDefinition="Path in the resource that contains the link", formalDefinition="Path in the resource that contains the link." ) 342 protected StringType path; 343 344 /** 345 * Which slice (if profiled). 346 */ 347 @Child(name = "sliceName", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 348 @Description(shortDefinition="Which slice (if profiled)", formalDefinition="Which slice (if profiled)." ) 349 protected StringType sliceName; 350 351 /** 352 * Minimum occurrences for this link. 353 */ 354 @Child(name = "min", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=false) 355 @Description(shortDefinition="Minimum occurrences for this link", formalDefinition="Minimum occurrences for this link." ) 356 protected IntegerType min; 357 358 /** 359 * Maximum occurrences for this link. 360 */ 361 @Child(name = "max", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 362 @Description(shortDefinition="Maximum occurrences for this link", formalDefinition="Maximum occurrences for this link." ) 363 protected StringType max; 364 365 /** 366 * Information about why this link is of interest in this graph definition. 367 */ 368 @Child(name = "description", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=false) 369 @Description(shortDefinition="Why this link is specified", formalDefinition="Information about why this link is of interest in this graph definition." ) 370 protected StringType description; 371 372 /** 373 * Potential target for the link. 374 */ 375 @Child(name = "target", type = {}, order=6, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 376 @Description(shortDefinition="Potential target for the link", formalDefinition="Potential target for the link." ) 377 protected List<GraphDefinitionLinkTargetComponent> target; 378 379 private static final long serialVersionUID = -593733346L; 380 381 /** 382 * Constructor 383 */ 384 public GraphDefinitionLinkComponent() { 385 super(); 386 } 387 388 /** 389 * Constructor 390 */ 391 public GraphDefinitionLinkComponent(StringType path) { 392 super(); 393 this.path = path; 394 } 395 396 /** 397 * @return {@link #path} (Path in the resource that contains the link.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 398 */ 399 public StringType getPathElement() { 400 if (this.path == null) 401 if (Configuration.errorOnAutoCreate()) 402 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.path"); 403 else if (Configuration.doAutoCreate()) 404 this.path = new StringType(); // bb 405 return this.path; 406 } 407 408 public boolean hasPathElement() { 409 return this.path != null && !this.path.isEmpty(); 410 } 411 412 public boolean hasPath() { 413 return this.path != null && !this.path.isEmpty(); 414 } 415 416 /** 417 * @param value {@link #path} (Path in the resource that contains the link.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 418 */ 419 public GraphDefinitionLinkComponent setPathElement(StringType value) { 420 this.path = value; 421 return this; 422 } 423 424 /** 425 * @return Path in the resource that contains the link. 426 */ 427 public String getPath() { 428 return this.path == null ? null : this.path.getValue(); 429 } 430 431 /** 432 * @param value Path in the resource that contains the link. 433 */ 434 public GraphDefinitionLinkComponent setPath(String value) { 435 if (this.path == null) 436 this.path = new StringType(); 437 this.path.setValue(value); 438 return this; 439 } 440 441 /** 442 * @return {@link #sliceName} (Which slice (if profiled).). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 443 */ 444 public StringType getSliceNameElement() { 445 if (this.sliceName == null) 446 if (Configuration.errorOnAutoCreate()) 447 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.sliceName"); 448 else if (Configuration.doAutoCreate()) 449 this.sliceName = new StringType(); // bb 450 return this.sliceName; 451 } 452 453 public boolean hasSliceNameElement() { 454 return this.sliceName != null && !this.sliceName.isEmpty(); 455 } 456 457 public boolean hasSliceName() { 458 return this.sliceName != null && !this.sliceName.isEmpty(); 459 } 460 461 /** 462 * @param value {@link #sliceName} (Which slice (if profiled).). This is the underlying object with id, value and extensions. The accessor "getSliceName" gives direct access to the value 463 */ 464 public GraphDefinitionLinkComponent setSliceNameElement(StringType value) { 465 this.sliceName = value; 466 return this; 467 } 468 469 /** 470 * @return Which slice (if profiled). 471 */ 472 public String getSliceName() { 473 return this.sliceName == null ? null : this.sliceName.getValue(); 474 } 475 476 /** 477 * @param value Which slice (if profiled). 478 */ 479 public GraphDefinitionLinkComponent setSliceName(String value) { 480 if (Utilities.noString(value)) 481 this.sliceName = null; 482 else { 483 if (this.sliceName == null) 484 this.sliceName = new StringType(); 485 this.sliceName.setValue(value); 486 } 487 return this; 488 } 489 490 /** 491 * @return {@link #min} (Minimum occurrences for this link.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 492 */ 493 public IntegerType getMinElement() { 494 if (this.min == null) 495 if (Configuration.errorOnAutoCreate()) 496 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.min"); 497 else if (Configuration.doAutoCreate()) 498 this.min = new IntegerType(); // bb 499 return this.min; 500 } 501 502 public boolean hasMinElement() { 503 return this.min != null && !this.min.isEmpty(); 504 } 505 506 public boolean hasMin() { 507 return this.min != null && !this.min.isEmpty(); 508 } 509 510 /** 511 * @param value {@link #min} (Minimum occurrences for this link.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 512 */ 513 public GraphDefinitionLinkComponent setMinElement(IntegerType value) { 514 this.min = value; 515 return this; 516 } 517 518 /** 519 * @return Minimum occurrences for this link. 520 */ 521 public int getMin() { 522 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 523 } 524 525 /** 526 * @param value Minimum occurrences for this link. 527 */ 528 public GraphDefinitionLinkComponent setMin(int value) { 529 if (this.min == null) 530 this.min = new IntegerType(); 531 this.min.setValue(value); 532 return this; 533 } 534 535 /** 536 * @return {@link #max} (Maximum occurrences for this link.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 537 */ 538 public StringType getMaxElement() { 539 if (this.max == null) 540 if (Configuration.errorOnAutoCreate()) 541 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.max"); 542 else if (Configuration.doAutoCreate()) 543 this.max = new StringType(); // bb 544 return this.max; 545 } 546 547 public boolean hasMaxElement() { 548 return this.max != null && !this.max.isEmpty(); 549 } 550 551 public boolean hasMax() { 552 return this.max != null && !this.max.isEmpty(); 553 } 554 555 /** 556 * @param value {@link #max} (Maximum occurrences for this link.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 557 */ 558 public GraphDefinitionLinkComponent setMaxElement(StringType value) { 559 this.max = value; 560 return this; 561 } 562 563 /** 564 * @return Maximum occurrences for this link. 565 */ 566 public String getMax() { 567 return this.max == null ? null : this.max.getValue(); 568 } 569 570 /** 571 * @param value Maximum occurrences for this link. 572 */ 573 public GraphDefinitionLinkComponent setMax(String value) { 574 if (Utilities.noString(value)) 575 this.max = null; 576 else { 577 if (this.max == null) 578 this.max = new StringType(); 579 this.max.setValue(value); 580 } 581 return this; 582 } 583 584 /** 585 * @return {@link #description} (Information about why this link is of interest in this graph definition.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 586 */ 587 public StringType getDescriptionElement() { 588 if (this.description == null) 589 if (Configuration.errorOnAutoCreate()) 590 throw new Error("Attempt to auto-create GraphDefinitionLinkComponent.description"); 591 else if (Configuration.doAutoCreate()) 592 this.description = new StringType(); // bb 593 return this.description; 594 } 595 596 public boolean hasDescriptionElement() { 597 return this.description != null && !this.description.isEmpty(); 598 } 599 600 public boolean hasDescription() { 601 return this.description != null && !this.description.isEmpty(); 602 } 603 604 /** 605 * @param value {@link #description} (Information about why this link is of interest in this graph definition.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 606 */ 607 public GraphDefinitionLinkComponent setDescriptionElement(StringType value) { 608 this.description = value; 609 return this; 610 } 611 612 /** 613 * @return Information about why this link is of interest in this graph definition. 614 */ 615 public String getDescription() { 616 return this.description == null ? null : this.description.getValue(); 617 } 618 619 /** 620 * @param value Information about why this link is of interest in this graph definition. 621 */ 622 public GraphDefinitionLinkComponent setDescription(String value) { 623 if (Utilities.noString(value)) 624 this.description = null; 625 else { 626 if (this.description == null) 627 this.description = new StringType(); 628 this.description.setValue(value); 629 } 630 return this; 631 } 632 633 /** 634 * @return {@link #target} (Potential target for the link.) 635 */ 636 public List<GraphDefinitionLinkTargetComponent> getTarget() { 637 if (this.target == null) 638 this.target = new ArrayList<GraphDefinitionLinkTargetComponent>(); 639 return this.target; 640 } 641 642 /** 643 * @return Returns a reference to <code>this</code> for easy method chaining 644 */ 645 public GraphDefinitionLinkComponent setTarget(List<GraphDefinitionLinkTargetComponent> theTarget) { 646 this.target = theTarget; 647 return this; 648 } 649 650 public boolean hasTarget() { 651 if (this.target == null) 652 return false; 653 for (GraphDefinitionLinkTargetComponent item : this.target) 654 if (!item.isEmpty()) 655 return true; 656 return false; 657 } 658 659 public GraphDefinitionLinkTargetComponent addTarget() { //3 660 GraphDefinitionLinkTargetComponent t = new GraphDefinitionLinkTargetComponent(); 661 if (this.target == null) 662 this.target = new ArrayList<GraphDefinitionLinkTargetComponent>(); 663 this.target.add(t); 664 return t; 665 } 666 667 public GraphDefinitionLinkComponent addTarget(GraphDefinitionLinkTargetComponent t) { //3 668 if (t == null) 669 return this; 670 if (this.target == null) 671 this.target = new ArrayList<GraphDefinitionLinkTargetComponent>(); 672 this.target.add(t); 673 return this; 674 } 675 676 /** 677 * @return The first repetition of repeating field {@link #target}, creating it if it does not already exist 678 */ 679 public GraphDefinitionLinkTargetComponent getTargetFirstRep() { 680 if (getTarget().isEmpty()) { 681 addTarget(); 682 } 683 return getTarget().get(0); 684 } 685 686 protected void listChildren(List<Property> children) { 687 super.listChildren(children); 688 children.add(new Property("path", "string", "Path in the resource that contains the link.", 0, 1, path)); 689 children.add(new Property("sliceName", "string", "Which slice (if profiled).", 0, 1, sliceName)); 690 children.add(new Property("min", "integer", "Minimum occurrences for this link.", 0, 1, min)); 691 children.add(new Property("max", "string", "Maximum occurrences for this link.", 0, 1, max)); 692 children.add(new Property("description", "string", "Information about why this link is of interest in this graph definition.", 0, 1, description)); 693 children.add(new Property("target", "", "Potential target for the link.", 0, java.lang.Integer.MAX_VALUE, target)); 694 } 695 696 @Override 697 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 698 switch (_hash) { 699 case 3433509: /*path*/ return new Property("path", "string", "Path in the resource that contains the link.", 0, 1, path); 700 case -825289923: /*sliceName*/ return new Property("sliceName", "string", "Which slice (if profiled).", 0, 1, sliceName); 701 case 108114: /*min*/ return new Property("min", "integer", "Minimum occurrences for this link.", 0, 1, min); 702 case 107876: /*max*/ return new Property("max", "string", "Maximum occurrences for this link.", 0, 1, max); 703 case -1724546052: /*description*/ return new Property("description", "string", "Information about why this link is of interest in this graph definition.", 0, 1, description); 704 case -880905839: /*target*/ return new Property("target", "", "Potential target for the link.", 0, java.lang.Integer.MAX_VALUE, target); 705 default: return super.getNamedProperty(_hash, _name, _checkValid); 706 } 707 708 } 709 710 @Override 711 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 712 switch (hash) { 713 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 714 case -825289923: /*sliceName*/ return this.sliceName == null ? new Base[0] : new Base[] {this.sliceName}; // StringType 715 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // IntegerType 716 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 717 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 718 case -880905839: /*target*/ return this.target == null ? new Base[0] : this.target.toArray(new Base[this.target.size()]); // GraphDefinitionLinkTargetComponent 719 default: return super.getProperty(hash, name, checkValid); 720 } 721 722 } 723 724 @Override 725 public Base setProperty(int hash, String name, Base value) throws FHIRException { 726 switch (hash) { 727 case 3433509: // path 728 this.path = castToString(value); // StringType 729 return value; 730 case -825289923: // sliceName 731 this.sliceName = castToString(value); // StringType 732 return value; 733 case 108114: // min 734 this.min = castToInteger(value); // IntegerType 735 return value; 736 case 107876: // max 737 this.max = castToString(value); // StringType 738 return value; 739 case -1724546052: // description 740 this.description = castToString(value); // StringType 741 return value; 742 case -880905839: // target 743 this.getTarget().add((GraphDefinitionLinkTargetComponent) value); // GraphDefinitionLinkTargetComponent 744 return value; 745 default: return super.setProperty(hash, name, value); 746 } 747 748 } 749 750 @Override 751 public Base setProperty(String name, Base value) throws FHIRException { 752 if (name.equals("path")) { 753 this.path = castToString(value); // StringType 754 } else if (name.equals("sliceName")) { 755 this.sliceName = castToString(value); // StringType 756 } else if (name.equals("min")) { 757 this.min = castToInteger(value); // IntegerType 758 } else if (name.equals("max")) { 759 this.max = castToString(value); // StringType 760 } else if (name.equals("description")) { 761 this.description = castToString(value); // StringType 762 } else if (name.equals("target")) { 763 this.getTarget().add((GraphDefinitionLinkTargetComponent) value); 764 } else 765 return super.setProperty(name, value); 766 return value; 767 } 768 769 @Override 770 public Base makeProperty(int hash, String name) throws FHIRException { 771 switch (hash) { 772 case 3433509: return getPathElement(); 773 case -825289923: return getSliceNameElement(); 774 case 108114: return getMinElement(); 775 case 107876: return getMaxElement(); 776 case -1724546052: return getDescriptionElement(); 777 case -880905839: return addTarget(); 778 default: return super.makeProperty(hash, name); 779 } 780 781 } 782 783 @Override 784 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 785 switch (hash) { 786 case 3433509: /*path*/ return new String[] {"string"}; 787 case -825289923: /*sliceName*/ return new String[] {"string"}; 788 case 108114: /*min*/ return new String[] {"integer"}; 789 case 107876: /*max*/ return new String[] {"string"}; 790 case -1724546052: /*description*/ return new String[] {"string"}; 791 case -880905839: /*target*/ return new String[] {}; 792 default: return super.getTypesForProperty(hash, name); 793 } 794 795 } 796 797 @Override 798 public Base addChild(String name) throws FHIRException { 799 if (name.equals("path")) { 800 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.path"); 801 } 802 else if (name.equals("sliceName")) { 803 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.sliceName"); 804 } 805 else if (name.equals("min")) { 806 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.min"); 807 } 808 else if (name.equals("max")) { 809 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.max"); 810 } 811 else if (name.equals("description")) { 812 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.description"); 813 } 814 else if (name.equals("target")) { 815 return addTarget(); 816 } 817 else 818 return super.addChild(name); 819 } 820 821 public GraphDefinitionLinkComponent copy() { 822 GraphDefinitionLinkComponent dst = new GraphDefinitionLinkComponent(); 823 copyValues(dst); 824 dst.path = path == null ? null : path.copy(); 825 dst.sliceName = sliceName == null ? null : sliceName.copy(); 826 dst.min = min == null ? null : min.copy(); 827 dst.max = max == null ? null : max.copy(); 828 dst.description = description == null ? null : description.copy(); 829 if (target != null) { 830 dst.target = new ArrayList<GraphDefinitionLinkTargetComponent>(); 831 for (GraphDefinitionLinkTargetComponent i : target) 832 dst.target.add(i.copy()); 833 }; 834 return dst; 835 } 836 837 @Override 838 public boolean equalsDeep(Base other_) { 839 if (!super.equalsDeep(other_)) 840 return false; 841 if (!(other_ instanceof GraphDefinitionLinkComponent)) 842 return false; 843 GraphDefinitionLinkComponent o = (GraphDefinitionLinkComponent) other_; 844 return compareDeep(path, o.path, true) && compareDeep(sliceName, o.sliceName, true) && compareDeep(min, o.min, true) 845 && compareDeep(max, o.max, true) && compareDeep(description, o.description, true) && compareDeep(target, o.target, true) 846 ; 847 } 848 849 @Override 850 public boolean equalsShallow(Base other_) { 851 if (!super.equalsShallow(other_)) 852 return false; 853 if (!(other_ instanceof GraphDefinitionLinkComponent)) 854 return false; 855 GraphDefinitionLinkComponent o = (GraphDefinitionLinkComponent) other_; 856 return compareValues(path, o.path, true) && compareValues(sliceName, o.sliceName, true) && compareValues(min, o.min, true) 857 && compareValues(max, o.max, true) && compareValues(description, o.description, true); 858 } 859 860 public boolean isEmpty() { 861 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(path, sliceName, min, max 862 , description, target); 863 } 864 865 public String fhirType() { 866 return "GraphDefinition.link"; 867 868 } 869 870 } 871 872 @Block() 873 public static class GraphDefinitionLinkTargetComponent extends BackboneElement implements IBaseBackboneElement { 874 /** 875 * Type of resource this link refers to. 876 */ 877 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 878 @Description(shortDefinition="Type of resource this link refers to", formalDefinition="Type of resource this link refers to." ) 879 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 880 protected CodeType type; 881 882 /** 883 * Profile for the target resource. 884 */ 885 @Child(name = "profile", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 886 @Description(shortDefinition="Profile for the target resource", formalDefinition="Profile for the target resource." ) 887 protected UriType profile; 888 889 /** 890 * Compartment Consistency Rules. 891 */ 892 @Child(name = "compartment", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 893 @Description(shortDefinition="Compartment Consistency Rules", formalDefinition="Compartment Consistency Rules." ) 894 protected List<GraphDefinitionLinkTargetCompartmentComponent> compartment; 895 896 /** 897 * Additional links from target resource. 898 */ 899 @Child(name = "link", type = {GraphDefinitionLinkComponent.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 900 @Description(shortDefinition="Additional links from target resource", formalDefinition="Additional links from target resource." ) 901 protected List<GraphDefinitionLinkComponent> link; 902 903 private static final long serialVersionUID = -1862411341L; 904 905 /** 906 * Constructor 907 */ 908 public GraphDefinitionLinkTargetComponent() { 909 super(); 910 } 911 912 /** 913 * Constructor 914 */ 915 public GraphDefinitionLinkTargetComponent(CodeType type) { 916 super(); 917 this.type = type; 918 } 919 920 /** 921 * @return {@link #type} (Type of resource this link refers to.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 922 */ 923 public CodeType getTypeElement() { 924 if (this.type == null) 925 if (Configuration.errorOnAutoCreate()) 926 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetComponent.type"); 927 else if (Configuration.doAutoCreate()) 928 this.type = new CodeType(); // bb 929 return this.type; 930 } 931 932 public boolean hasTypeElement() { 933 return this.type != null && !this.type.isEmpty(); 934 } 935 936 public boolean hasType() { 937 return this.type != null && !this.type.isEmpty(); 938 } 939 940 /** 941 * @param value {@link #type} (Type of resource this link refers to.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 942 */ 943 public GraphDefinitionLinkTargetComponent setTypeElement(CodeType value) { 944 this.type = value; 945 return this; 946 } 947 948 /** 949 * @return Type of resource this link refers to. 950 */ 951 public String getType() { 952 return this.type == null ? null : this.type.getValue(); 953 } 954 955 /** 956 * @param value Type of resource this link refers to. 957 */ 958 public GraphDefinitionLinkTargetComponent setType(String value) { 959 if (this.type == null) 960 this.type = new CodeType(); 961 this.type.setValue(value); 962 return this; 963 } 964 965 /** 966 * @return {@link #profile} (Profile for the target resource.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 967 */ 968 public UriType getProfileElement() { 969 if (this.profile == null) 970 if (Configuration.errorOnAutoCreate()) 971 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetComponent.profile"); 972 else if (Configuration.doAutoCreate()) 973 this.profile = new UriType(); // bb 974 return this.profile; 975 } 976 977 public boolean hasProfileElement() { 978 return this.profile != null && !this.profile.isEmpty(); 979 } 980 981 public boolean hasProfile() { 982 return this.profile != null && !this.profile.isEmpty(); 983 } 984 985 /** 986 * @param value {@link #profile} (Profile for the target resource.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 987 */ 988 public GraphDefinitionLinkTargetComponent setProfileElement(UriType value) { 989 this.profile = value; 990 return this; 991 } 992 993 /** 994 * @return Profile for the target resource. 995 */ 996 public String getProfile() { 997 return this.profile == null ? null : this.profile.getValue(); 998 } 999 1000 /** 1001 * @param value Profile for the target resource. 1002 */ 1003 public GraphDefinitionLinkTargetComponent setProfile(String value) { 1004 if (Utilities.noString(value)) 1005 this.profile = null; 1006 else { 1007 if (this.profile == null) 1008 this.profile = new UriType(); 1009 this.profile.setValue(value); 1010 } 1011 return this; 1012 } 1013 1014 /** 1015 * @return {@link #compartment} (Compartment Consistency Rules.) 1016 */ 1017 public List<GraphDefinitionLinkTargetCompartmentComponent> getCompartment() { 1018 if (this.compartment == null) 1019 this.compartment = new ArrayList<GraphDefinitionLinkTargetCompartmentComponent>(); 1020 return this.compartment; 1021 } 1022 1023 /** 1024 * @return Returns a reference to <code>this</code> for easy method chaining 1025 */ 1026 public GraphDefinitionLinkTargetComponent setCompartment(List<GraphDefinitionLinkTargetCompartmentComponent> theCompartment) { 1027 this.compartment = theCompartment; 1028 return this; 1029 } 1030 1031 public boolean hasCompartment() { 1032 if (this.compartment == null) 1033 return false; 1034 for (GraphDefinitionLinkTargetCompartmentComponent item : this.compartment) 1035 if (!item.isEmpty()) 1036 return true; 1037 return false; 1038 } 1039 1040 public GraphDefinitionLinkTargetCompartmentComponent addCompartment() { //3 1041 GraphDefinitionLinkTargetCompartmentComponent t = new GraphDefinitionLinkTargetCompartmentComponent(); 1042 if (this.compartment == null) 1043 this.compartment = new ArrayList<GraphDefinitionLinkTargetCompartmentComponent>(); 1044 this.compartment.add(t); 1045 return t; 1046 } 1047 1048 public GraphDefinitionLinkTargetComponent addCompartment(GraphDefinitionLinkTargetCompartmentComponent t) { //3 1049 if (t == null) 1050 return this; 1051 if (this.compartment == null) 1052 this.compartment = new ArrayList<GraphDefinitionLinkTargetCompartmentComponent>(); 1053 this.compartment.add(t); 1054 return this; 1055 } 1056 1057 /** 1058 * @return The first repetition of repeating field {@link #compartment}, creating it if it does not already exist 1059 */ 1060 public GraphDefinitionLinkTargetCompartmentComponent getCompartmentFirstRep() { 1061 if (getCompartment().isEmpty()) { 1062 addCompartment(); 1063 } 1064 return getCompartment().get(0); 1065 } 1066 1067 /** 1068 * @return {@link #link} (Additional links from target resource.) 1069 */ 1070 public List<GraphDefinitionLinkComponent> getLink() { 1071 if (this.link == null) 1072 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 1073 return this.link; 1074 } 1075 1076 /** 1077 * @return Returns a reference to <code>this</code> for easy method chaining 1078 */ 1079 public GraphDefinitionLinkTargetComponent setLink(List<GraphDefinitionLinkComponent> theLink) { 1080 this.link = theLink; 1081 return this; 1082 } 1083 1084 public boolean hasLink() { 1085 if (this.link == null) 1086 return false; 1087 for (GraphDefinitionLinkComponent item : this.link) 1088 if (!item.isEmpty()) 1089 return true; 1090 return false; 1091 } 1092 1093 public GraphDefinitionLinkComponent addLink() { //3 1094 GraphDefinitionLinkComponent t = new GraphDefinitionLinkComponent(); 1095 if (this.link == null) 1096 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 1097 this.link.add(t); 1098 return t; 1099 } 1100 1101 public GraphDefinitionLinkTargetComponent addLink(GraphDefinitionLinkComponent t) { //3 1102 if (t == null) 1103 return this; 1104 if (this.link == null) 1105 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 1106 this.link.add(t); 1107 return this; 1108 } 1109 1110 /** 1111 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist 1112 */ 1113 public GraphDefinitionLinkComponent getLinkFirstRep() { 1114 if (getLink().isEmpty()) { 1115 addLink(); 1116 } 1117 return getLink().get(0); 1118 } 1119 1120 protected void listChildren(List<Property> children) { 1121 super.listChildren(children); 1122 children.add(new Property("type", "code", "Type of resource this link refers to.", 0, 1, type)); 1123 children.add(new Property("profile", "uri", "Profile for the target resource.", 0, 1, profile)); 1124 children.add(new Property("compartment", "", "Compartment Consistency Rules.", 0, java.lang.Integer.MAX_VALUE, compartment)); 1125 children.add(new Property("link", "@GraphDefinition.link", "Additional links from target resource.", 0, java.lang.Integer.MAX_VALUE, link)); 1126 } 1127 1128 @Override 1129 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1130 switch (_hash) { 1131 case 3575610: /*type*/ return new Property("type", "code", "Type of resource this link refers to.", 0, 1, type); 1132 case -309425751: /*profile*/ return new Property("profile", "uri", "Profile for the target resource.", 0, 1, profile); 1133 case -397756334: /*compartment*/ return new Property("compartment", "", "Compartment Consistency Rules.", 0, java.lang.Integer.MAX_VALUE, compartment); 1134 case 3321850: /*link*/ return new Property("link", "@GraphDefinition.link", "Additional links from target resource.", 0, java.lang.Integer.MAX_VALUE, link); 1135 default: return super.getNamedProperty(_hash, _name, _checkValid); 1136 } 1137 1138 } 1139 1140 @Override 1141 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1142 switch (hash) { 1143 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 1144 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // UriType 1145 case -397756334: /*compartment*/ return this.compartment == null ? new Base[0] : this.compartment.toArray(new Base[this.compartment.size()]); // GraphDefinitionLinkTargetCompartmentComponent 1146 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // GraphDefinitionLinkComponent 1147 default: return super.getProperty(hash, name, checkValid); 1148 } 1149 1150 } 1151 1152 @Override 1153 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1154 switch (hash) { 1155 case 3575610: // type 1156 this.type = castToCode(value); // CodeType 1157 return value; 1158 case -309425751: // profile 1159 this.profile = castToUri(value); // UriType 1160 return value; 1161 case -397756334: // compartment 1162 this.getCompartment().add((GraphDefinitionLinkTargetCompartmentComponent) value); // GraphDefinitionLinkTargetCompartmentComponent 1163 return value; 1164 case 3321850: // link 1165 this.getLink().add((GraphDefinitionLinkComponent) value); // GraphDefinitionLinkComponent 1166 return value; 1167 default: return super.setProperty(hash, name, value); 1168 } 1169 1170 } 1171 1172 @Override 1173 public Base setProperty(String name, Base value) throws FHIRException { 1174 if (name.equals("type")) { 1175 this.type = castToCode(value); // CodeType 1176 } else if (name.equals("profile")) { 1177 this.profile = castToUri(value); // UriType 1178 } else if (name.equals("compartment")) { 1179 this.getCompartment().add((GraphDefinitionLinkTargetCompartmentComponent) value); 1180 } else if (name.equals("link")) { 1181 this.getLink().add((GraphDefinitionLinkComponent) value); 1182 } else 1183 return super.setProperty(name, value); 1184 return value; 1185 } 1186 1187 @Override 1188 public Base makeProperty(int hash, String name) throws FHIRException { 1189 switch (hash) { 1190 case 3575610: return getTypeElement(); 1191 case -309425751: return getProfileElement(); 1192 case -397756334: return addCompartment(); 1193 case 3321850: return addLink(); 1194 default: return super.makeProperty(hash, name); 1195 } 1196 1197 } 1198 1199 @Override 1200 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1201 switch (hash) { 1202 case 3575610: /*type*/ return new String[] {"code"}; 1203 case -309425751: /*profile*/ return new String[] {"uri"}; 1204 case -397756334: /*compartment*/ return new String[] {}; 1205 case 3321850: /*link*/ return new String[] {"@GraphDefinition.link"}; 1206 default: return super.getTypesForProperty(hash, name); 1207 } 1208 1209 } 1210 1211 @Override 1212 public Base addChild(String name) throws FHIRException { 1213 if (name.equals("type")) { 1214 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.type"); 1215 } 1216 else if (name.equals("profile")) { 1217 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.profile"); 1218 } 1219 else if (name.equals("compartment")) { 1220 return addCompartment(); 1221 } 1222 else if (name.equals("link")) { 1223 return addLink(); 1224 } 1225 else 1226 return super.addChild(name); 1227 } 1228 1229 public GraphDefinitionLinkTargetComponent copy() { 1230 GraphDefinitionLinkTargetComponent dst = new GraphDefinitionLinkTargetComponent(); 1231 copyValues(dst); 1232 dst.type = type == null ? null : type.copy(); 1233 dst.profile = profile == null ? null : profile.copy(); 1234 if (compartment != null) { 1235 dst.compartment = new ArrayList<GraphDefinitionLinkTargetCompartmentComponent>(); 1236 for (GraphDefinitionLinkTargetCompartmentComponent i : compartment) 1237 dst.compartment.add(i.copy()); 1238 }; 1239 if (link != null) { 1240 dst.link = new ArrayList<GraphDefinitionLinkComponent>(); 1241 for (GraphDefinitionLinkComponent i : link) 1242 dst.link.add(i.copy()); 1243 }; 1244 return dst; 1245 } 1246 1247 @Override 1248 public boolean equalsDeep(Base other_) { 1249 if (!super.equalsDeep(other_)) 1250 return false; 1251 if (!(other_ instanceof GraphDefinitionLinkTargetComponent)) 1252 return false; 1253 GraphDefinitionLinkTargetComponent o = (GraphDefinitionLinkTargetComponent) other_; 1254 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true) && compareDeep(compartment, o.compartment, true) 1255 && compareDeep(link, o.link, true); 1256 } 1257 1258 @Override 1259 public boolean equalsShallow(Base other_) { 1260 if (!super.equalsShallow(other_)) 1261 return false; 1262 if (!(other_ instanceof GraphDefinitionLinkTargetComponent)) 1263 return false; 1264 GraphDefinitionLinkTargetComponent o = (GraphDefinitionLinkTargetComponent) other_; 1265 return compareValues(type, o.type, true) && compareValues(profile, o.profile, true); 1266 } 1267 1268 public boolean isEmpty() { 1269 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile, compartment 1270 , link); 1271 } 1272 1273 public String fhirType() { 1274 return "GraphDefinition.link.target"; 1275 1276 } 1277 1278 } 1279 1280 @Block() 1281 public static class GraphDefinitionLinkTargetCompartmentComponent extends BackboneElement implements IBaseBackboneElement { 1282 /** 1283 * Identifies the compartment. 1284 */ 1285 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1286 @Description(shortDefinition="Identifies the compartment", formalDefinition="Identifies the compartment." ) 1287 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/compartment-type") 1288 protected Enumeration<CompartmentCode> code; 1289 1290 /** 1291 * identical | matching | different | no-rule | custom. 1292 */ 1293 @Child(name = "rule", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 1294 @Description(shortDefinition="identical | matching | different | custom", formalDefinition="identical | matching | different | no-rule | custom." ) 1295 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/graph-compartment-rule") 1296 protected Enumeration<GraphCompartmentRule> rule; 1297 1298 /** 1299 * Custom rule, as a FHIRPath expression. 1300 */ 1301 @Child(name = "expression", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1302 @Description(shortDefinition="Custom rule, as a FHIRPath expression", formalDefinition="Custom rule, as a FHIRPath expression." ) 1303 protected StringType expression; 1304 1305 /** 1306 * Documentation for FHIRPath expression. 1307 */ 1308 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1309 @Description(shortDefinition="Documentation for FHIRPath expression", formalDefinition="Documentation for FHIRPath expression." ) 1310 protected StringType description; 1311 1312 private static final long serialVersionUID = -1046660576L; 1313 1314 /** 1315 * Constructor 1316 */ 1317 public GraphDefinitionLinkTargetCompartmentComponent() { 1318 super(); 1319 } 1320 1321 /** 1322 * Constructor 1323 */ 1324 public GraphDefinitionLinkTargetCompartmentComponent(Enumeration<CompartmentCode> code, Enumeration<GraphCompartmentRule> rule) { 1325 super(); 1326 this.code = code; 1327 this.rule = rule; 1328 } 1329 1330 /** 1331 * @return {@link #code} (Identifies the compartment.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1332 */ 1333 public Enumeration<CompartmentCode> getCodeElement() { 1334 if (this.code == null) 1335 if (Configuration.errorOnAutoCreate()) 1336 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.code"); 1337 else if (Configuration.doAutoCreate()) 1338 this.code = new Enumeration<CompartmentCode>(new CompartmentCodeEnumFactory()); // bb 1339 return this.code; 1340 } 1341 1342 public boolean hasCodeElement() { 1343 return this.code != null && !this.code.isEmpty(); 1344 } 1345 1346 public boolean hasCode() { 1347 return this.code != null && !this.code.isEmpty(); 1348 } 1349 1350 /** 1351 * @param value {@link #code} (Identifies the compartment.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 1352 */ 1353 public GraphDefinitionLinkTargetCompartmentComponent setCodeElement(Enumeration<CompartmentCode> value) { 1354 this.code = value; 1355 return this; 1356 } 1357 1358 /** 1359 * @return Identifies the compartment. 1360 */ 1361 public CompartmentCode getCode() { 1362 return this.code == null ? null : this.code.getValue(); 1363 } 1364 1365 /** 1366 * @param value Identifies the compartment. 1367 */ 1368 public GraphDefinitionLinkTargetCompartmentComponent setCode(CompartmentCode value) { 1369 if (this.code == null) 1370 this.code = new Enumeration<CompartmentCode>(new CompartmentCodeEnumFactory()); 1371 this.code.setValue(value); 1372 return this; 1373 } 1374 1375 /** 1376 * @return {@link #rule} (identical | matching | different | no-rule | custom.). This is the underlying object with id, value and extensions. The accessor "getRule" gives direct access to the value 1377 */ 1378 public Enumeration<GraphCompartmentRule> getRuleElement() { 1379 if (this.rule == null) 1380 if (Configuration.errorOnAutoCreate()) 1381 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.rule"); 1382 else if (Configuration.doAutoCreate()) 1383 this.rule = new Enumeration<GraphCompartmentRule>(new GraphCompartmentRuleEnumFactory()); // bb 1384 return this.rule; 1385 } 1386 1387 public boolean hasRuleElement() { 1388 return this.rule != null && !this.rule.isEmpty(); 1389 } 1390 1391 public boolean hasRule() { 1392 return this.rule != null && !this.rule.isEmpty(); 1393 } 1394 1395 /** 1396 * @param value {@link #rule} (identical | matching | different | no-rule | custom.). This is the underlying object with id, value and extensions. The accessor "getRule" gives direct access to the value 1397 */ 1398 public GraphDefinitionLinkTargetCompartmentComponent setRuleElement(Enumeration<GraphCompartmentRule> value) { 1399 this.rule = value; 1400 return this; 1401 } 1402 1403 /** 1404 * @return identical | matching | different | no-rule | custom. 1405 */ 1406 public GraphCompartmentRule getRule() { 1407 return this.rule == null ? null : this.rule.getValue(); 1408 } 1409 1410 /** 1411 * @param value identical | matching | different | no-rule | custom. 1412 */ 1413 public GraphDefinitionLinkTargetCompartmentComponent setRule(GraphCompartmentRule value) { 1414 if (this.rule == null) 1415 this.rule = new Enumeration<GraphCompartmentRule>(new GraphCompartmentRuleEnumFactory()); 1416 this.rule.setValue(value); 1417 return this; 1418 } 1419 1420 /** 1421 * @return {@link #expression} (Custom rule, as a FHIRPath expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1422 */ 1423 public StringType getExpressionElement() { 1424 if (this.expression == null) 1425 if (Configuration.errorOnAutoCreate()) 1426 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.expression"); 1427 else if (Configuration.doAutoCreate()) 1428 this.expression = new StringType(); // bb 1429 return this.expression; 1430 } 1431 1432 public boolean hasExpressionElement() { 1433 return this.expression != null && !this.expression.isEmpty(); 1434 } 1435 1436 public boolean hasExpression() { 1437 return this.expression != null && !this.expression.isEmpty(); 1438 } 1439 1440 /** 1441 * @param value {@link #expression} (Custom rule, as a FHIRPath expression.). This is the underlying object with id, value and extensions. The accessor "getExpression" gives direct access to the value 1442 */ 1443 public GraphDefinitionLinkTargetCompartmentComponent setExpressionElement(StringType value) { 1444 this.expression = value; 1445 return this; 1446 } 1447 1448 /** 1449 * @return Custom rule, as a FHIRPath expression. 1450 */ 1451 public String getExpression() { 1452 return this.expression == null ? null : this.expression.getValue(); 1453 } 1454 1455 /** 1456 * @param value Custom rule, as a FHIRPath expression. 1457 */ 1458 public GraphDefinitionLinkTargetCompartmentComponent setExpression(String value) { 1459 if (Utilities.noString(value)) 1460 this.expression = null; 1461 else { 1462 if (this.expression == null) 1463 this.expression = new StringType(); 1464 this.expression.setValue(value); 1465 } 1466 return this; 1467 } 1468 1469 /** 1470 * @return {@link #description} (Documentation for FHIRPath expression.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1471 */ 1472 public StringType getDescriptionElement() { 1473 if (this.description == null) 1474 if (Configuration.errorOnAutoCreate()) 1475 throw new Error("Attempt to auto-create GraphDefinitionLinkTargetCompartmentComponent.description"); 1476 else if (Configuration.doAutoCreate()) 1477 this.description = new StringType(); // bb 1478 return this.description; 1479 } 1480 1481 public boolean hasDescriptionElement() { 1482 return this.description != null && !this.description.isEmpty(); 1483 } 1484 1485 public boolean hasDescription() { 1486 return this.description != null && !this.description.isEmpty(); 1487 } 1488 1489 /** 1490 * @param value {@link #description} (Documentation for FHIRPath expression.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1491 */ 1492 public GraphDefinitionLinkTargetCompartmentComponent setDescriptionElement(StringType value) { 1493 this.description = value; 1494 return this; 1495 } 1496 1497 /** 1498 * @return Documentation for FHIRPath expression. 1499 */ 1500 public String getDescription() { 1501 return this.description == null ? null : this.description.getValue(); 1502 } 1503 1504 /** 1505 * @param value Documentation for FHIRPath expression. 1506 */ 1507 public GraphDefinitionLinkTargetCompartmentComponent setDescription(String value) { 1508 if (Utilities.noString(value)) 1509 this.description = null; 1510 else { 1511 if (this.description == null) 1512 this.description = new StringType(); 1513 this.description.setValue(value); 1514 } 1515 return this; 1516 } 1517 1518 protected void listChildren(List<Property> children) { 1519 super.listChildren(children); 1520 children.add(new Property("code", "code", "Identifies the compartment.", 0, 1, code)); 1521 children.add(new Property("rule", "code", "identical | matching | different | no-rule | custom.", 0, 1, rule)); 1522 children.add(new Property("expression", "string", "Custom rule, as a FHIRPath expression.", 0, 1, expression)); 1523 children.add(new Property("description", "string", "Documentation for FHIRPath expression.", 0, 1, description)); 1524 } 1525 1526 @Override 1527 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1528 switch (_hash) { 1529 case 3059181: /*code*/ return new Property("code", "code", "Identifies the compartment.", 0, 1, code); 1530 case 3512060: /*rule*/ return new Property("rule", "code", "identical | matching | different | no-rule | custom.", 0, 1, rule); 1531 case -1795452264: /*expression*/ return new Property("expression", "string", "Custom rule, as a FHIRPath expression.", 0, 1, expression); 1532 case -1724546052: /*description*/ return new Property("description", "string", "Documentation for FHIRPath expression.", 0, 1, description); 1533 default: return super.getNamedProperty(_hash, _name, _checkValid); 1534 } 1535 1536 } 1537 1538 @Override 1539 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1540 switch (hash) { 1541 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // Enumeration<CompartmentCode> 1542 case 3512060: /*rule*/ return this.rule == null ? new Base[0] : new Base[] {this.rule}; // Enumeration<GraphCompartmentRule> 1543 case -1795452264: /*expression*/ return this.expression == null ? new Base[0] : new Base[] {this.expression}; // StringType 1544 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1545 default: return super.getProperty(hash, name, checkValid); 1546 } 1547 1548 } 1549 1550 @Override 1551 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1552 switch (hash) { 1553 case 3059181: // code 1554 value = new CompartmentCodeEnumFactory().fromType(castToCode(value)); 1555 this.code = (Enumeration) value; // Enumeration<CompartmentCode> 1556 return value; 1557 case 3512060: // rule 1558 value = new GraphCompartmentRuleEnumFactory().fromType(castToCode(value)); 1559 this.rule = (Enumeration) value; // Enumeration<GraphCompartmentRule> 1560 return value; 1561 case -1795452264: // expression 1562 this.expression = castToString(value); // StringType 1563 return value; 1564 case -1724546052: // description 1565 this.description = castToString(value); // StringType 1566 return value; 1567 default: return super.setProperty(hash, name, value); 1568 } 1569 1570 } 1571 1572 @Override 1573 public Base setProperty(String name, Base value) throws FHIRException { 1574 if (name.equals("code")) { 1575 value = new CompartmentCodeEnumFactory().fromType(castToCode(value)); 1576 this.code = (Enumeration) value; // Enumeration<CompartmentCode> 1577 } else if (name.equals("rule")) { 1578 value = new GraphCompartmentRuleEnumFactory().fromType(castToCode(value)); 1579 this.rule = (Enumeration) value; // Enumeration<GraphCompartmentRule> 1580 } else if (name.equals("expression")) { 1581 this.expression = castToString(value); // StringType 1582 } else if (name.equals("description")) { 1583 this.description = castToString(value); // StringType 1584 } else 1585 return super.setProperty(name, value); 1586 return value; 1587 } 1588 1589 @Override 1590 public Base makeProperty(int hash, String name) throws FHIRException { 1591 switch (hash) { 1592 case 3059181: return getCodeElement(); 1593 case 3512060: return getRuleElement(); 1594 case -1795452264: return getExpressionElement(); 1595 case -1724546052: return getDescriptionElement(); 1596 default: return super.makeProperty(hash, name); 1597 } 1598 1599 } 1600 1601 @Override 1602 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1603 switch (hash) { 1604 case 3059181: /*code*/ return new String[] {"code"}; 1605 case 3512060: /*rule*/ return new String[] {"code"}; 1606 case -1795452264: /*expression*/ return new String[] {"string"}; 1607 case -1724546052: /*description*/ return new String[] {"string"}; 1608 default: return super.getTypesForProperty(hash, name); 1609 } 1610 1611 } 1612 1613 @Override 1614 public Base addChild(String name) throws FHIRException { 1615 if (name.equals("code")) { 1616 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.code"); 1617 } 1618 else if (name.equals("rule")) { 1619 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.rule"); 1620 } 1621 else if (name.equals("expression")) { 1622 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.expression"); 1623 } 1624 else if (name.equals("description")) { 1625 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.description"); 1626 } 1627 else 1628 return super.addChild(name); 1629 } 1630 1631 public GraphDefinitionLinkTargetCompartmentComponent copy() { 1632 GraphDefinitionLinkTargetCompartmentComponent dst = new GraphDefinitionLinkTargetCompartmentComponent(); 1633 copyValues(dst); 1634 dst.code = code == null ? null : code.copy(); 1635 dst.rule = rule == null ? null : rule.copy(); 1636 dst.expression = expression == null ? null : expression.copy(); 1637 dst.description = description == null ? null : description.copy(); 1638 return dst; 1639 } 1640 1641 @Override 1642 public boolean equalsDeep(Base other_) { 1643 if (!super.equalsDeep(other_)) 1644 return false; 1645 if (!(other_ instanceof GraphDefinitionLinkTargetCompartmentComponent)) 1646 return false; 1647 GraphDefinitionLinkTargetCompartmentComponent o = (GraphDefinitionLinkTargetCompartmentComponent) other_; 1648 return compareDeep(code, o.code, true) && compareDeep(rule, o.rule, true) && compareDeep(expression, o.expression, true) 1649 && compareDeep(description, o.description, true); 1650 } 1651 1652 @Override 1653 public boolean equalsShallow(Base other_) { 1654 if (!super.equalsShallow(other_)) 1655 return false; 1656 if (!(other_ instanceof GraphDefinitionLinkTargetCompartmentComponent)) 1657 return false; 1658 GraphDefinitionLinkTargetCompartmentComponent o = (GraphDefinitionLinkTargetCompartmentComponent) other_; 1659 return compareValues(code, o.code, true) && compareValues(rule, o.rule, true) && compareValues(expression, o.expression, true) 1660 && compareValues(description, o.description, true); 1661 } 1662 1663 public boolean isEmpty() { 1664 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, rule, expression, description 1665 ); 1666 } 1667 1668 public String fhirType() { 1669 return "GraphDefinition.link.target.compartment"; 1670 1671 } 1672 1673 } 1674 1675 /** 1676 * Explaination of why this graph definition is needed and why it has been designed as it has. 1677 */ 1678 @Child(name = "purpose", type = {MarkdownType.class}, order=0, min=0, max=1, modifier=false, summary=false) 1679 @Description(shortDefinition="Why this graph definition is defined", formalDefinition="Explaination of why this graph definition is needed and why it has been designed as it has." ) 1680 protected MarkdownType purpose; 1681 1682 /** 1683 * The type of FHIR resource at which instances of this graph start. 1684 */ 1685 @Child(name = "start", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1686 @Description(shortDefinition="Type of resource at which the graph starts", formalDefinition="The type of FHIR resource at which instances of this graph start." ) 1687 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 1688 protected CodeType start; 1689 1690 /** 1691 * The profile that describes the use of the base resource. 1692 */ 1693 @Child(name = "profile", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1694 @Description(shortDefinition="Profile on base resource", formalDefinition="The profile that describes the use of the base resource." ) 1695 protected UriType profile; 1696 1697 /** 1698 * Links this graph makes rules about. 1699 */ 1700 @Child(name = "link", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1701 @Description(shortDefinition="Links this graph makes rules about", formalDefinition="Links this graph makes rules about." ) 1702 protected List<GraphDefinitionLinkComponent> link; 1703 1704 private static final long serialVersionUID = 86877575L; 1705 1706 /** 1707 * Constructor 1708 */ 1709 public GraphDefinition() { 1710 super(); 1711 } 1712 1713 /** 1714 * Constructor 1715 */ 1716 public GraphDefinition(StringType name, Enumeration<PublicationStatus> status, CodeType start) { 1717 super(); 1718 this.name = name; 1719 this.status = status; 1720 this.start = start; 1721 } 1722 1723 /** 1724 * @return {@link #url} (An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this graph definition is (or will be) published. The URL SHOULD include the major version of the graph definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1725 */ 1726 public UriType getUrlElement() { 1727 if (this.url == null) 1728 if (Configuration.errorOnAutoCreate()) 1729 throw new Error("Attempt to auto-create GraphDefinition.url"); 1730 else if (Configuration.doAutoCreate()) 1731 this.url = new UriType(); // bb 1732 return this.url; 1733 } 1734 1735 public boolean hasUrlElement() { 1736 return this.url != null && !this.url.isEmpty(); 1737 } 1738 1739 public boolean hasUrl() { 1740 return this.url != null && !this.url.isEmpty(); 1741 } 1742 1743 /** 1744 * @param value {@link #url} (An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this graph definition is (or will be) published. The URL SHOULD include the major version of the graph definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1745 */ 1746 public GraphDefinition setUrlElement(UriType value) { 1747 this.url = value; 1748 return this; 1749 } 1750 1751 /** 1752 * @return An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this graph definition is (or will be) published. The URL SHOULD include the major version of the graph definition. For more information see [Technical and Business Versions](resource.html#versions). 1753 */ 1754 public String getUrl() { 1755 return this.url == null ? null : this.url.getValue(); 1756 } 1757 1758 /** 1759 * @param value An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this graph definition is (or will be) published. The URL SHOULD include the major version of the graph definition. For more information see [Technical and Business Versions](resource.html#versions). 1760 */ 1761 public GraphDefinition setUrl(String value) { 1762 if (Utilities.noString(value)) 1763 this.url = null; 1764 else { 1765 if (this.url == null) 1766 this.url = new UriType(); 1767 this.url.setValue(value); 1768 } 1769 return this; 1770 } 1771 1772 /** 1773 * @return {@link #version} (The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1774 */ 1775 public StringType getVersionElement() { 1776 if (this.version == null) 1777 if (Configuration.errorOnAutoCreate()) 1778 throw new Error("Attempt to auto-create GraphDefinition.version"); 1779 else if (Configuration.doAutoCreate()) 1780 this.version = new StringType(); // bb 1781 return this.version; 1782 } 1783 1784 public boolean hasVersionElement() { 1785 return this.version != null && !this.version.isEmpty(); 1786 } 1787 1788 public boolean hasVersion() { 1789 return this.version != null && !this.version.isEmpty(); 1790 } 1791 1792 /** 1793 * @param value {@link #version} (The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1794 */ 1795 public GraphDefinition setVersionElement(StringType value) { 1796 this.version = value; 1797 return this; 1798 } 1799 1800 /** 1801 * @return The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1802 */ 1803 public String getVersion() { 1804 return this.version == null ? null : this.version.getValue(); 1805 } 1806 1807 /** 1808 * @param value The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1809 */ 1810 public GraphDefinition setVersion(String value) { 1811 if (Utilities.noString(value)) 1812 this.version = null; 1813 else { 1814 if (this.version == null) 1815 this.version = new StringType(); 1816 this.version.setValue(value); 1817 } 1818 return this; 1819 } 1820 1821 /** 1822 * @return {@link #name} (A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1823 */ 1824 public StringType getNameElement() { 1825 if (this.name == null) 1826 if (Configuration.errorOnAutoCreate()) 1827 throw new Error("Attempt to auto-create GraphDefinition.name"); 1828 else if (Configuration.doAutoCreate()) 1829 this.name = new StringType(); // bb 1830 return this.name; 1831 } 1832 1833 public boolean hasNameElement() { 1834 return this.name != null && !this.name.isEmpty(); 1835 } 1836 1837 public boolean hasName() { 1838 return this.name != null && !this.name.isEmpty(); 1839 } 1840 1841 /** 1842 * @param value {@link #name} (A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1843 */ 1844 public GraphDefinition setNameElement(StringType value) { 1845 this.name = value; 1846 return this; 1847 } 1848 1849 /** 1850 * @return A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1851 */ 1852 public String getName() { 1853 return this.name == null ? null : this.name.getValue(); 1854 } 1855 1856 /** 1857 * @param value A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1858 */ 1859 public GraphDefinition setName(String value) { 1860 if (this.name == null) 1861 this.name = new StringType(); 1862 this.name.setValue(value); 1863 return this; 1864 } 1865 1866 /** 1867 * @return {@link #status} (The status of this graph definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1868 */ 1869 public Enumeration<PublicationStatus> getStatusElement() { 1870 if (this.status == null) 1871 if (Configuration.errorOnAutoCreate()) 1872 throw new Error("Attempt to auto-create GraphDefinition.status"); 1873 else if (Configuration.doAutoCreate()) 1874 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1875 return this.status; 1876 } 1877 1878 public boolean hasStatusElement() { 1879 return this.status != null && !this.status.isEmpty(); 1880 } 1881 1882 public boolean hasStatus() { 1883 return this.status != null && !this.status.isEmpty(); 1884 } 1885 1886 /** 1887 * @param value {@link #status} (The status of this graph definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1888 */ 1889 public GraphDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1890 this.status = value; 1891 return this; 1892 } 1893 1894 /** 1895 * @return The status of this graph definition. Enables tracking the life-cycle of the content. 1896 */ 1897 public PublicationStatus getStatus() { 1898 return this.status == null ? null : this.status.getValue(); 1899 } 1900 1901 /** 1902 * @param value The status of this graph definition. Enables tracking the life-cycle of the content. 1903 */ 1904 public GraphDefinition setStatus(PublicationStatus value) { 1905 if (this.status == null) 1906 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1907 this.status.setValue(value); 1908 return this; 1909 } 1910 1911 /** 1912 * @return {@link #experimental} (A boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1913 */ 1914 public BooleanType getExperimentalElement() { 1915 if (this.experimental == null) 1916 if (Configuration.errorOnAutoCreate()) 1917 throw new Error("Attempt to auto-create GraphDefinition.experimental"); 1918 else if (Configuration.doAutoCreate()) 1919 this.experimental = new BooleanType(); // bb 1920 return this.experimental; 1921 } 1922 1923 public boolean hasExperimentalElement() { 1924 return this.experimental != null && !this.experimental.isEmpty(); 1925 } 1926 1927 public boolean hasExperimental() { 1928 return this.experimental != null && !this.experimental.isEmpty(); 1929 } 1930 1931 /** 1932 * @param value {@link #experimental} (A boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1933 */ 1934 public GraphDefinition setExperimentalElement(BooleanType value) { 1935 this.experimental = value; 1936 return this; 1937 } 1938 1939 /** 1940 * @return A boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1941 */ 1942 public boolean getExperimental() { 1943 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1944 } 1945 1946 /** 1947 * @param value A boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1948 */ 1949 public GraphDefinition setExperimental(boolean value) { 1950 if (this.experimental == null) 1951 this.experimental = new BooleanType(); 1952 this.experimental.setValue(value); 1953 return this; 1954 } 1955 1956 /** 1957 * @return {@link #date} (The date (and optionally time) when the graph definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1958 */ 1959 public DateTimeType getDateElement() { 1960 if (this.date == null) 1961 if (Configuration.errorOnAutoCreate()) 1962 throw new Error("Attempt to auto-create GraphDefinition.date"); 1963 else if (Configuration.doAutoCreate()) 1964 this.date = new DateTimeType(); // bb 1965 return this.date; 1966 } 1967 1968 public boolean hasDateElement() { 1969 return this.date != null && !this.date.isEmpty(); 1970 } 1971 1972 public boolean hasDate() { 1973 return this.date != null && !this.date.isEmpty(); 1974 } 1975 1976 /** 1977 * @param value {@link #date} (The date (and optionally time) when the graph definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1978 */ 1979 public GraphDefinition setDateElement(DateTimeType value) { 1980 this.date = value; 1981 return this; 1982 } 1983 1984 /** 1985 * @return The date (and optionally time) when the graph definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes. 1986 */ 1987 public Date getDate() { 1988 return this.date == null ? null : this.date.getValue(); 1989 } 1990 1991 /** 1992 * @param value The date (and optionally time) when the graph definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes. 1993 */ 1994 public GraphDefinition setDate(Date value) { 1995 if (value == null) 1996 this.date = null; 1997 else { 1998 if (this.date == null) 1999 this.date = new DateTimeType(); 2000 this.date.setValue(value); 2001 } 2002 return this; 2003 } 2004 2005 /** 2006 * @return {@link #publisher} (The name of the individual or organization that published the graph definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2007 */ 2008 public StringType getPublisherElement() { 2009 if (this.publisher == null) 2010 if (Configuration.errorOnAutoCreate()) 2011 throw new Error("Attempt to auto-create GraphDefinition.publisher"); 2012 else if (Configuration.doAutoCreate()) 2013 this.publisher = new StringType(); // bb 2014 return this.publisher; 2015 } 2016 2017 public boolean hasPublisherElement() { 2018 return this.publisher != null && !this.publisher.isEmpty(); 2019 } 2020 2021 public boolean hasPublisher() { 2022 return this.publisher != null && !this.publisher.isEmpty(); 2023 } 2024 2025 /** 2026 * @param value {@link #publisher} (The name of the individual or organization that published the graph definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2027 */ 2028 public GraphDefinition setPublisherElement(StringType value) { 2029 this.publisher = value; 2030 return this; 2031 } 2032 2033 /** 2034 * @return The name of the individual or organization that published the graph definition. 2035 */ 2036 public String getPublisher() { 2037 return this.publisher == null ? null : this.publisher.getValue(); 2038 } 2039 2040 /** 2041 * @param value The name of the individual or organization that published the graph definition. 2042 */ 2043 public GraphDefinition setPublisher(String value) { 2044 if (Utilities.noString(value)) 2045 this.publisher = null; 2046 else { 2047 if (this.publisher == null) 2048 this.publisher = new StringType(); 2049 this.publisher.setValue(value); 2050 } 2051 return this; 2052 } 2053 2054 /** 2055 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2056 */ 2057 public List<ContactDetail> getContact() { 2058 if (this.contact == null) 2059 this.contact = new ArrayList<ContactDetail>(); 2060 return this.contact; 2061 } 2062 2063 /** 2064 * @return Returns a reference to <code>this</code> for easy method chaining 2065 */ 2066 public GraphDefinition setContact(List<ContactDetail> theContact) { 2067 this.contact = theContact; 2068 return this; 2069 } 2070 2071 public boolean hasContact() { 2072 if (this.contact == null) 2073 return false; 2074 for (ContactDetail item : this.contact) 2075 if (!item.isEmpty()) 2076 return true; 2077 return false; 2078 } 2079 2080 public ContactDetail addContact() { //3 2081 ContactDetail t = new ContactDetail(); 2082 if (this.contact == null) 2083 this.contact = new ArrayList<ContactDetail>(); 2084 this.contact.add(t); 2085 return t; 2086 } 2087 2088 public GraphDefinition addContact(ContactDetail t) { //3 2089 if (t == null) 2090 return this; 2091 if (this.contact == null) 2092 this.contact = new ArrayList<ContactDetail>(); 2093 this.contact.add(t); 2094 return this; 2095 } 2096 2097 /** 2098 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 2099 */ 2100 public ContactDetail getContactFirstRep() { 2101 if (getContact().isEmpty()) { 2102 addContact(); 2103 } 2104 return getContact().get(0); 2105 } 2106 2107 /** 2108 * @return {@link #description} (A free text natural language description of the graph definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2109 */ 2110 public MarkdownType getDescriptionElement() { 2111 if (this.description == null) 2112 if (Configuration.errorOnAutoCreate()) 2113 throw new Error("Attempt to auto-create GraphDefinition.description"); 2114 else if (Configuration.doAutoCreate()) 2115 this.description = new MarkdownType(); // bb 2116 return this.description; 2117 } 2118 2119 public boolean hasDescriptionElement() { 2120 return this.description != null && !this.description.isEmpty(); 2121 } 2122 2123 public boolean hasDescription() { 2124 return this.description != null && !this.description.isEmpty(); 2125 } 2126 2127 /** 2128 * @param value {@link #description} (A free text natural language description of the graph definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2129 */ 2130 public GraphDefinition setDescriptionElement(MarkdownType value) { 2131 this.description = value; 2132 return this; 2133 } 2134 2135 /** 2136 * @return A free text natural language description of the graph definition from a consumer's perspective. 2137 */ 2138 public String getDescription() { 2139 return this.description == null ? null : this.description.getValue(); 2140 } 2141 2142 /** 2143 * @param value A free text natural language description of the graph definition from a consumer's perspective. 2144 */ 2145 public GraphDefinition setDescription(String value) { 2146 if (value == null) 2147 this.description = null; 2148 else { 2149 if (this.description == null) 2150 this.description = new MarkdownType(); 2151 this.description.setValue(value); 2152 } 2153 return this; 2154 } 2155 2156 /** 2157 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate graph definition instances.) 2158 */ 2159 public List<UsageContext> getUseContext() { 2160 if (this.useContext == null) 2161 this.useContext = new ArrayList<UsageContext>(); 2162 return this.useContext; 2163 } 2164 2165 /** 2166 * @return Returns a reference to <code>this</code> for easy method chaining 2167 */ 2168 public GraphDefinition setUseContext(List<UsageContext> theUseContext) { 2169 this.useContext = theUseContext; 2170 return this; 2171 } 2172 2173 public boolean hasUseContext() { 2174 if (this.useContext == null) 2175 return false; 2176 for (UsageContext item : this.useContext) 2177 if (!item.isEmpty()) 2178 return true; 2179 return false; 2180 } 2181 2182 public UsageContext addUseContext() { //3 2183 UsageContext t = new UsageContext(); 2184 if (this.useContext == null) 2185 this.useContext = new ArrayList<UsageContext>(); 2186 this.useContext.add(t); 2187 return t; 2188 } 2189 2190 public GraphDefinition addUseContext(UsageContext t) { //3 2191 if (t == null) 2192 return this; 2193 if (this.useContext == null) 2194 this.useContext = new ArrayList<UsageContext>(); 2195 this.useContext.add(t); 2196 return this; 2197 } 2198 2199 /** 2200 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 2201 */ 2202 public UsageContext getUseContextFirstRep() { 2203 if (getUseContext().isEmpty()) { 2204 addUseContext(); 2205 } 2206 return getUseContext().get(0); 2207 } 2208 2209 /** 2210 * @return {@link #jurisdiction} (A legal or geographic region in which the graph definition is intended to be used.) 2211 */ 2212 public List<CodeableConcept> getJurisdiction() { 2213 if (this.jurisdiction == null) 2214 this.jurisdiction = new ArrayList<CodeableConcept>(); 2215 return this.jurisdiction; 2216 } 2217 2218 /** 2219 * @return Returns a reference to <code>this</code> for easy method chaining 2220 */ 2221 public GraphDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 2222 this.jurisdiction = theJurisdiction; 2223 return this; 2224 } 2225 2226 public boolean hasJurisdiction() { 2227 if (this.jurisdiction == null) 2228 return false; 2229 for (CodeableConcept item : this.jurisdiction) 2230 if (!item.isEmpty()) 2231 return true; 2232 return false; 2233 } 2234 2235 public CodeableConcept addJurisdiction() { //3 2236 CodeableConcept t = new CodeableConcept(); 2237 if (this.jurisdiction == null) 2238 this.jurisdiction = new ArrayList<CodeableConcept>(); 2239 this.jurisdiction.add(t); 2240 return t; 2241 } 2242 2243 public GraphDefinition addJurisdiction(CodeableConcept t) { //3 2244 if (t == null) 2245 return this; 2246 if (this.jurisdiction == null) 2247 this.jurisdiction = new ArrayList<CodeableConcept>(); 2248 this.jurisdiction.add(t); 2249 return this; 2250 } 2251 2252 /** 2253 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 2254 */ 2255 public CodeableConcept getJurisdictionFirstRep() { 2256 if (getJurisdiction().isEmpty()) { 2257 addJurisdiction(); 2258 } 2259 return getJurisdiction().get(0); 2260 } 2261 2262 /** 2263 * @return {@link #purpose} (Explaination of why this graph definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2264 */ 2265 public MarkdownType getPurposeElement() { 2266 if (this.purpose == null) 2267 if (Configuration.errorOnAutoCreate()) 2268 throw new Error("Attempt to auto-create GraphDefinition.purpose"); 2269 else if (Configuration.doAutoCreate()) 2270 this.purpose = new MarkdownType(); // bb 2271 return this.purpose; 2272 } 2273 2274 public boolean hasPurposeElement() { 2275 return this.purpose != null && !this.purpose.isEmpty(); 2276 } 2277 2278 public boolean hasPurpose() { 2279 return this.purpose != null && !this.purpose.isEmpty(); 2280 } 2281 2282 /** 2283 * @param value {@link #purpose} (Explaination of why this graph definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2284 */ 2285 public GraphDefinition setPurposeElement(MarkdownType value) { 2286 this.purpose = value; 2287 return this; 2288 } 2289 2290 /** 2291 * @return Explaination of why this graph definition is needed and why it has been designed as it has. 2292 */ 2293 public String getPurpose() { 2294 return this.purpose == null ? null : this.purpose.getValue(); 2295 } 2296 2297 /** 2298 * @param value Explaination of why this graph definition is needed and why it has been designed as it has. 2299 */ 2300 public GraphDefinition setPurpose(String value) { 2301 if (value == null) 2302 this.purpose = null; 2303 else { 2304 if (this.purpose == null) 2305 this.purpose = new MarkdownType(); 2306 this.purpose.setValue(value); 2307 } 2308 return this; 2309 } 2310 2311 /** 2312 * @return {@link #start} (The type of FHIR resource at which instances of this graph start.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 2313 */ 2314 public CodeType getStartElement() { 2315 if (this.start == null) 2316 if (Configuration.errorOnAutoCreate()) 2317 throw new Error("Attempt to auto-create GraphDefinition.start"); 2318 else if (Configuration.doAutoCreate()) 2319 this.start = new CodeType(); // bb 2320 return this.start; 2321 } 2322 2323 public boolean hasStartElement() { 2324 return this.start != null && !this.start.isEmpty(); 2325 } 2326 2327 public boolean hasStart() { 2328 return this.start != null && !this.start.isEmpty(); 2329 } 2330 2331 /** 2332 * @param value {@link #start} (The type of FHIR resource at which instances of this graph start.). This is the underlying object with id, value and extensions. The accessor "getStart" gives direct access to the value 2333 */ 2334 public GraphDefinition setStartElement(CodeType value) { 2335 this.start = value; 2336 return this; 2337 } 2338 2339 /** 2340 * @return The type of FHIR resource at which instances of this graph start. 2341 */ 2342 public String getStart() { 2343 return this.start == null ? null : this.start.getValue(); 2344 } 2345 2346 /** 2347 * @param value The type of FHIR resource at which instances of this graph start. 2348 */ 2349 public GraphDefinition setStart(String value) { 2350 if (this.start == null) 2351 this.start = new CodeType(); 2352 this.start.setValue(value); 2353 return this; 2354 } 2355 2356 /** 2357 * @return {@link #profile} (The profile that describes the use of the base resource.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 2358 */ 2359 public UriType getProfileElement() { 2360 if (this.profile == null) 2361 if (Configuration.errorOnAutoCreate()) 2362 throw new Error("Attempt to auto-create GraphDefinition.profile"); 2363 else if (Configuration.doAutoCreate()) 2364 this.profile = new UriType(); // bb 2365 return this.profile; 2366 } 2367 2368 public boolean hasProfileElement() { 2369 return this.profile != null && !this.profile.isEmpty(); 2370 } 2371 2372 public boolean hasProfile() { 2373 return this.profile != null && !this.profile.isEmpty(); 2374 } 2375 2376 /** 2377 * @param value {@link #profile} (The profile that describes the use of the base resource.). This is the underlying object with id, value and extensions. The accessor "getProfile" gives direct access to the value 2378 */ 2379 public GraphDefinition setProfileElement(UriType value) { 2380 this.profile = value; 2381 return this; 2382 } 2383 2384 /** 2385 * @return The profile that describes the use of the base resource. 2386 */ 2387 public String getProfile() { 2388 return this.profile == null ? null : this.profile.getValue(); 2389 } 2390 2391 /** 2392 * @param value The profile that describes the use of the base resource. 2393 */ 2394 public GraphDefinition setProfile(String value) { 2395 if (Utilities.noString(value)) 2396 this.profile = null; 2397 else { 2398 if (this.profile == null) 2399 this.profile = new UriType(); 2400 this.profile.setValue(value); 2401 } 2402 return this; 2403 } 2404 2405 /** 2406 * @return {@link #link} (Links this graph makes rules about.) 2407 */ 2408 public List<GraphDefinitionLinkComponent> getLink() { 2409 if (this.link == null) 2410 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 2411 return this.link; 2412 } 2413 2414 /** 2415 * @return Returns a reference to <code>this</code> for easy method chaining 2416 */ 2417 public GraphDefinition setLink(List<GraphDefinitionLinkComponent> theLink) { 2418 this.link = theLink; 2419 return this; 2420 } 2421 2422 public boolean hasLink() { 2423 if (this.link == null) 2424 return false; 2425 for (GraphDefinitionLinkComponent item : this.link) 2426 if (!item.isEmpty()) 2427 return true; 2428 return false; 2429 } 2430 2431 public GraphDefinitionLinkComponent addLink() { //3 2432 GraphDefinitionLinkComponent t = new GraphDefinitionLinkComponent(); 2433 if (this.link == null) 2434 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 2435 this.link.add(t); 2436 return t; 2437 } 2438 2439 public GraphDefinition addLink(GraphDefinitionLinkComponent t) { //3 2440 if (t == null) 2441 return this; 2442 if (this.link == null) 2443 this.link = new ArrayList<GraphDefinitionLinkComponent>(); 2444 this.link.add(t); 2445 return this; 2446 } 2447 2448 /** 2449 * @return The first repetition of repeating field {@link #link}, creating it if it does not already exist 2450 */ 2451 public GraphDefinitionLinkComponent getLinkFirstRep() { 2452 if (getLink().isEmpty()) { 2453 addLink(); 2454 } 2455 return getLink().get(0); 2456 } 2457 2458 protected void listChildren(List<Property> children) { 2459 super.listChildren(children); 2460 children.add(new Property("url", "uri", "An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this graph definition is (or will be) published. The URL SHOULD include the major version of the graph definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 2461 children.add(new Property("version", "string", "The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2462 children.add(new Property("name", "string", "A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2463 children.add(new Property("status", "code", "The status of this graph definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2464 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 2465 children.add(new Property("date", "dateTime", "The date (and optionally time) when the graph definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.", 0, 1, date)); 2466 children.add(new Property("publisher", "string", "The name of the individual or organization that published the graph definition.", 0, 1, publisher)); 2467 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2468 children.add(new Property("description", "markdown", "A free text natural language description of the graph definition from a consumer's perspective.", 0, 1, description)); 2469 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate graph definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2470 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the graph definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2471 children.add(new Property("purpose", "markdown", "Explaination of why this graph definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2472 children.add(new Property("start", "code", "The type of FHIR resource at which instances of this graph start.", 0, 1, start)); 2473 children.add(new Property("profile", "uri", "The profile that describes the use of the base resource.", 0, 1, profile)); 2474 children.add(new Property("link", "", "Links this graph makes rules about.", 0, java.lang.Integer.MAX_VALUE, link)); 2475 } 2476 2477 @Override 2478 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2479 switch (_hash) { 2480 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this graph definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this graph definition is (or will be) published. The URL SHOULD include the major version of the graph definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 2481 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the graph definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the graph definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2482 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the graph definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2483 case -892481550: /*status*/ return new Property("status", "code", "The status of this graph definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2484 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this graph definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 2485 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the graph definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the graph definition changes.", 0, 1, date); 2486 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the graph definition.", 0, 1, publisher); 2487 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2488 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the graph definition from a consumer's perspective.", 0, 1, description); 2489 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate graph definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2490 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the graph definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2491 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this graph definition is needed and why it has been designed as it has.", 0, 1, purpose); 2492 case 109757538: /*start*/ return new Property("start", "code", "The type of FHIR resource at which instances of this graph start.", 0, 1, start); 2493 case -309425751: /*profile*/ return new Property("profile", "uri", "The profile that describes the use of the base resource.", 0, 1, profile); 2494 case 3321850: /*link*/ return new Property("link", "", "Links this graph makes rules about.", 0, java.lang.Integer.MAX_VALUE, link); 2495 default: return super.getNamedProperty(_hash, _name, _checkValid); 2496 } 2497 2498 } 2499 2500 @Override 2501 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2502 switch (hash) { 2503 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2504 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2505 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2506 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2507 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2508 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2509 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2510 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2511 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2512 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2513 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2514 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2515 case 109757538: /*start*/ return this.start == null ? new Base[0] : new Base[] {this.start}; // CodeType 2516 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // UriType 2517 case 3321850: /*link*/ return this.link == null ? new Base[0] : this.link.toArray(new Base[this.link.size()]); // GraphDefinitionLinkComponent 2518 default: return super.getProperty(hash, name, checkValid); 2519 } 2520 2521 } 2522 2523 @Override 2524 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2525 switch (hash) { 2526 case 116079: // url 2527 this.url = castToUri(value); // UriType 2528 return value; 2529 case 351608024: // version 2530 this.version = castToString(value); // StringType 2531 return value; 2532 case 3373707: // name 2533 this.name = castToString(value); // StringType 2534 return value; 2535 case -892481550: // status 2536 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2537 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2538 return value; 2539 case -404562712: // experimental 2540 this.experimental = castToBoolean(value); // BooleanType 2541 return value; 2542 case 3076014: // date 2543 this.date = castToDateTime(value); // DateTimeType 2544 return value; 2545 case 1447404028: // publisher 2546 this.publisher = castToString(value); // StringType 2547 return value; 2548 case 951526432: // contact 2549 this.getContact().add(castToContactDetail(value)); // ContactDetail 2550 return value; 2551 case -1724546052: // description 2552 this.description = castToMarkdown(value); // MarkdownType 2553 return value; 2554 case -669707736: // useContext 2555 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2556 return value; 2557 case -507075711: // jurisdiction 2558 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2559 return value; 2560 case -220463842: // purpose 2561 this.purpose = castToMarkdown(value); // MarkdownType 2562 return value; 2563 case 109757538: // start 2564 this.start = castToCode(value); // CodeType 2565 return value; 2566 case -309425751: // profile 2567 this.profile = castToUri(value); // UriType 2568 return value; 2569 case 3321850: // link 2570 this.getLink().add((GraphDefinitionLinkComponent) value); // GraphDefinitionLinkComponent 2571 return value; 2572 default: return super.setProperty(hash, name, value); 2573 } 2574 2575 } 2576 2577 @Override 2578 public Base setProperty(String name, Base value) throws FHIRException { 2579 if (name.equals("url")) { 2580 this.url = castToUri(value); // UriType 2581 } else if (name.equals("version")) { 2582 this.version = castToString(value); // StringType 2583 } else if (name.equals("name")) { 2584 this.name = castToString(value); // StringType 2585 } else if (name.equals("status")) { 2586 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2587 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2588 } else if (name.equals("experimental")) { 2589 this.experimental = castToBoolean(value); // BooleanType 2590 } else if (name.equals("date")) { 2591 this.date = castToDateTime(value); // DateTimeType 2592 } else if (name.equals("publisher")) { 2593 this.publisher = castToString(value); // StringType 2594 } else if (name.equals("contact")) { 2595 this.getContact().add(castToContactDetail(value)); 2596 } else if (name.equals("description")) { 2597 this.description = castToMarkdown(value); // MarkdownType 2598 } else if (name.equals("useContext")) { 2599 this.getUseContext().add(castToUsageContext(value)); 2600 } else if (name.equals("jurisdiction")) { 2601 this.getJurisdiction().add(castToCodeableConcept(value)); 2602 } else if (name.equals("purpose")) { 2603 this.purpose = castToMarkdown(value); // MarkdownType 2604 } else if (name.equals("start")) { 2605 this.start = castToCode(value); // CodeType 2606 } else if (name.equals("profile")) { 2607 this.profile = castToUri(value); // UriType 2608 } else if (name.equals("link")) { 2609 this.getLink().add((GraphDefinitionLinkComponent) value); 2610 } else 2611 return super.setProperty(name, value); 2612 return value; 2613 } 2614 2615 @Override 2616 public Base makeProperty(int hash, String name) throws FHIRException { 2617 switch (hash) { 2618 case 116079: return getUrlElement(); 2619 case 351608024: return getVersionElement(); 2620 case 3373707: return getNameElement(); 2621 case -892481550: return getStatusElement(); 2622 case -404562712: return getExperimentalElement(); 2623 case 3076014: return getDateElement(); 2624 case 1447404028: return getPublisherElement(); 2625 case 951526432: return addContact(); 2626 case -1724546052: return getDescriptionElement(); 2627 case -669707736: return addUseContext(); 2628 case -507075711: return addJurisdiction(); 2629 case -220463842: return getPurposeElement(); 2630 case 109757538: return getStartElement(); 2631 case -309425751: return getProfileElement(); 2632 case 3321850: return addLink(); 2633 default: return super.makeProperty(hash, name); 2634 } 2635 2636 } 2637 2638 @Override 2639 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2640 switch (hash) { 2641 case 116079: /*url*/ return new String[] {"uri"}; 2642 case 351608024: /*version*/ return new String[] {"string"}; 2643 case 3373707: /*name*/ return new String[] {"string"}; 2644 case -892481550: /*status*/ return new String[] {"code"}; 2645 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2646 case 3076014: /*date*/ return new String[] {"dateTime"}; 2647 case 1447404028: /*publisher*/ return new String[] {"string"}; 2648 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2649 case -1724546052: /*description*/ return new String[] {"markdown"}; 2650 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2651 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2652 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2653 case 109757538: /*start*/ return new String[] {"code"}; 2654 case -309425751: /*profile*/ return new String[] {"uri"}; 2655 case 3321850: /*link*/ return new String[] {}; 2656 default: return super.getTypesForProperty(hash, name); 2657 } 2658 2659 } 2660 2661 @Override 2662 public Base addChild(String name) throws FHIRException { 2663 if (name.equals("url")) { 2664 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.url"); 2665 } 2666 else if (name.equals("version")) { 2667 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.version"); 2668 } 2669 else if (name.equals("name")) { 2670 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.name"); 2671 } 2672 else if (name.equals("status")) { 2673 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.status"); 2674 } 2675 else if (name.equals("experimental")) { 2676 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.experimental"); 2677 } 2678 else if (name.equals("date")) { 2679 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.date"); 2680 } 2681 else if (name.equals("publisher")) { 2682 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.publisher"); 2683 } 2684 else if (name.equals("contact")) { 2685 return addContact(); 2686 } 2687 else if (name.equals("description")) { 2688 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.description"); 2689 } 2690 else if (name.equals("useContext")) { 2691 return addUseContext(); 2692 } 2693 else if (name.equals("jurisdiction")) { 2694 return addJurisdiction(); 2695 } 2696 else if (name.equals("purpose")) { 2697 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.purpose"); 2698 } 2699 else if (name.equals("start")) { 2700 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.start"); 2701 } 2702 else if (name.equals("profile")) { 2703 throw new FHIRException("Cannot call addChild on a singleton property GraphDefinition.profile"); 2704 } 2705 else if (name.equals("link")) { 2706 return addLink(); 2707 } 2708 else 2709 return super.addChild(name); 2710 } 2711 2712 public String fhirType() { 2713 return "GraphDefinition"; 2714 2715 } 2716 2717 public GraphDefinition copy() { 2718 GraphDefinition dst = new GraphDefinition(); 2719 copyValues(dst); 2720 dst.url = url == null ? null : url.copy(); 2721 dst.version = version == null ? null : version.copy(); 2722 dst.name = name == null ? null : name.copy(); 2723 dst.status = status == null ? null : status.copy(); 2724 dst.experimental = experimental == null ? null : experimental.copy(); 2725 dst.date = date == null ? null : date.copy(); 2726 dst.publisher = publisher == null ? null : publisher.copy(); 2727 if (contact != null) { 2728 dst.contact = new ArrayList<ContactDetail>(); 2729 for (ContactDetail i : contact) 2730 dst.contact.add(i.copy()); 2731 }; 2732 dst.description = description == null ? null : description.copy(); 2733 if (useContext != null) { 2734 dst.useContext = new ArrayList<UsageContext>(); 2735 for (UsageContext i : useContext) 2736 dst.useContext.add(i.copy()); 2737 }; 2738 if (jurisdiction != null) { 2739 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2740 for (CodeableConcept i : jurisdiction) 2741 dst.jurisdiction.add(i.copy()); 2742 }; 2743 dst.purpose = purpose == null ? null : purpose.copy(); 2744 dst.start = start == null ? null : start.copy(); 2745 dst.profile = profile == null ? null : profile.copy(); 2746 if (link != null) { 2747 dst.link = new ArrayList<GraphDefinitionLinkComponent>(); 2748 for (GraphDefinitionLinkComponent i : link) 2749 dst.link.add(i.copy()); 2750 }; 2751 return dst; 2752 } 2753 2754 protected GraphDefinition typedCopy() { 2755 return copy(); 2756 } 2757 2758 @Override 2759 public boolean equalsDeep(Base other_) { 2760 if (!super.equalsDeep(other_)) 2761 return false; 2762 if (!(other_ instanceof GraphDefinition)) 2763 return false; 2764 GraphDefinition o = (GraphDefinition) other_; 2765 return compareDeep(purpose, o.purpose, true) && compareDeep(start, o.start, true) && compareDeep(profile, o.profile, true) 2766 && compareDeep(link, o.link, true); 2767 } 2768 2769 @Override 2770 public boolean equalsShallow(Base other_) { 2771 if (!super.equalsShallow(other_)) 2772 return false; 2773 if (!(other_ instanceof GraphDefinition)) 2774 return false; 2775 GraphDefinition o = (GraphDefinition) other_; 2776 return compareValues(purpose, o.purpose, true) && compareValues(start, o.start, true) && compareValues(profile, o.profile, true) 2777 ; 2778 } 2779 2780 public boolean isEmpty() { 2781 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(purpose, start, profile 2782 , link); 2783 } 2784 2785 @Override 2786 public ResourceType getResourceType() { 2787 return ResourceType.GraphDefinition; 2788 } 2789 2790 /** 2791 * Search parameter: <b>date</b> 2792 * <p> 2793 * Description: <b>The graph definition publication date</b><br> 2794 * Type: <b>date</b><br> 2795 * Path: <b>GraphDefinition.date</b><br> 2796 * </p> 2797 */ 2798 @SearchParamDefinition(name="date", path="GraphDefinition.date", description="The graph definition publication date", type="date" ) 2799 public static final String SP_DATE = "date"; 2800 /** 2801 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2802 * <p> 2803 * Description: <b>The graph definition publication date</b><br> 2804 * Type: <b>date</b><br> 2805 * Path: <b>GraphDefinition.date</b><br> 2806 * </p> 2807 */ 2808 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2809 2810 /** 2811 * Search parameter: <b>jurisdiction</b> 2812 * <p> 2813 * Description: <b>Intended jurisdiction for the graph definition</b><br> 2814 * Type: <b>token</b><br> 2815 * Path: <b>GraphDefinition.jurisdiction</b><br> 2816 * </p> 2817 */ 2818 @SearchParamDefinition(name="jurisdiction", path="GraphDefinition.jurisdiction", description="Intended jurisdiction for the graph definition", type="token" ) 2819 public static final String SP_JURISDICTION = "jurisdiction"; 2820 /** 2821 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2822 * <p> 2823 * Description: <b>Intended jurisdiction for the graph definition</b><br> 2824 * Type: <b>token</b><br> 2825 * Path: <b>GraphDefinition.jurisdiction</b><br> 2826 * </p> 2827 */ 2828 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 2829 2830 /** 2831 * Search parameter: <b>name</b> 2832 * <p> 2833 * Description: <b>Computationally friendly name of the graph definition</b><br> 2834 * Type: <b>string</b><br> 2835 * Path: <b>GraphDefinition.name</b><br> 2836 * </p> 2837 */ 2838 @SearchParamDefinition(name="name", path="GraphDefinition.name", description="Computationally friendly name of the graph definition", type="string" ) 2839 public static final String SP_NAME = "name"; 2840 /** 2841 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2842 * <p> 2843 * Description: <b>Computationally friendly name of the graph definition</b><br> 2844 * Type: <b>string</b><br> 2845 * Path: <b>GraphDefinition.name</b><br> 2846 * </p> 2847 */ 2848 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2849 2850 /** 2851 * Search parameter: <b>start</b> 2852 * <p> 2853 * Description: <b>Type of resource at which the graph starts</b><br> 2854 * Type: <b>token</b><br> 2855 * Path: <b>GraphDefinition.start</b><br> 2856 * </p> 2857 */ 2858 @SearchParamDefinition(name="start", path="GraphDefinition.start", description="Type of resource at which the graph starts", type="token" ) 2859 public static final String SP_START = "start"; 2860 /** 2861 * <b>Fluent Client</b> search parameter constant for <b>start</b> 2862 * <p> 2863 * Description: <b>Type of resource at which the graph starts</b><br> 2864 * Type: <b>token</b><br> 2865 * Path: <b>GraphDefinition.start</b><br> 2866 * </p> 2867 */ 2868 public static final ca.uhn.fhir.rest.gclient.TokenClientParam START = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_START); 2869 2870 /** 2871 * Search parameter: <b>description</b> 2872 * <p> 2873 * Description: <b>The description of the graph definition</b><br> 2874 * Type: <b>string</b><br> 2875 * Path: <b>GraphDefinition.description</b><br> 2876 * </p> 2877 */ 2878 @SearchParamDefinition(name="description", path="GraphDefinition.description", description="The description of the graph definition", type="string" ) 2879 public static final String SP_DESCRIPTION = "description"; 2880 /** 2881 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2882 * <p> 2883 * Description: <b>The description of the graph definition</b><br> 2884 * Type: <b>string</b><br> 2885 * Path: <b>GraphDefinition.description</b><br> 2886 * </p> 2887 */ 2888 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 2889 2890 /** 2891 * Search parameter: <b>publisher</b> 2892 * <p> 2893 * Description: <b>Name of the publisher of the graph definition</b><br> 2894 * Type: <b>string</b><br> 2895 * Path: <b>GraphDefinition.publisher</b><br> 2896 * </p> 2897 */ 2898 @SearchParamDefinition(name="publisher", path="GraphDefinition.publisher", description="Name of the publisher of the graph definition", type="string" ) 2899 public static final String SP_PUBLISHER = "publisher"; 2900 /** 2901 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2902 * <p> 2903 * Description: <b>Name of the publisher of the graph definition</b><br> 2904 * Type: <b>string</b><br> 2905 * Path: <b>GraphDefinition.publisher</b><br> 2906 * </p> 2907 */ 2908 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2909 2910 /** 2911 * Search parameter: <b>version</b> 2912 * <p> 2913 * Description: <b>The business version of the graph definition</b><br> 2914 * Type: <b>token</b><br> 2915 * Path: <b>GraphDefinition.version</b><br> 2916 * </p> 2917 */ 2918 @SearchParamDefinition(name="version", path="GraphDefinition.version", description="The business version of the graph definition", type="token" ) 2919 public static final String SP_VERSION = "version"; 2920 /** 2921 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2922 * <p> 2923 * Description: <b>The business version of the graph definition</b><br> 2924 * Type: <b>token</b><br> 2925 * Path: <b>GraphDefinition.version</b><br> 2926 * </p> 2927 */ 2928 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 2929 2930 /** 2931 * Search parameter: <b>url</b> 2932 * <p> 2933 * Description: <b>The uri that identifies the graph definition</b><br> 2934 * Type: <b>uri</b><br> 2935 * Path: <b>GraphDefinition.url</b><br> 2936 * </p> 2937 */ 2938 @SearchParamDefinition(name="url", path="GraphDefinition.url", description="The uri that identifies the graph definition", type="uri" ) 2939 public static final String SP_URL = "url"; 2940 /** 2941 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2942 * <p> 2943 * Description: <b>The uri that identifies the graph definition</b><br> 2944 * Type: <b>uri</b><br> 2945 * Path: <b>GraphDefinition.url</b><br> 2946 * </p> 2947 */ 2948 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2949 2950 /** 2951 * Search parameter: <b>status</b> 2952 * <p> 2953 * Description: <b>The current status of the graph definition</b><br> 2954 * Type: <b>token</b><br> 2955 * Path: <b>GraphDefinition.status</b><br> 2956 * </p> 2957 */ 2958 @SearchParamDefinition(name="status", path="GraphDefinition.status", description="The current status of the graph definition", type="token" ) 2959 public static final String SP_STATUS = "status"; 2960 /** 2961 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2962 * <p> 2963 * Description: <b>The current status of the graph definition</b><br> 2964 * Type: <b>token</b><br> 2965 * Path: <b>GraphDefinition.status</b><br> 2966 * </p> 2967 */ 2968 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2969 2970 2971}