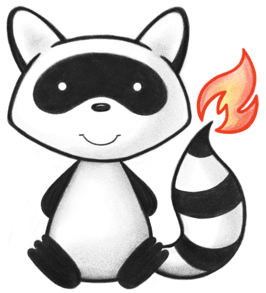
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.exceptions.FHIRFormatError; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Represents a defined collection of entities that may be discussed or acted upon collectively but which are not expected to act collectively and are not formally or legally recognized; i.e. a collection of entities that isn't an Organization. 050 */ 051@ResourceDef(name="Group", profile="http://hl7.org/fhir/Profile/Group") 052public class Group extends DomainResource { 053 054 public enum GroupType { 055 /** 056 * Group contains "person" Patient resources 057 */ 058 PERSON, 059 /** 060 * Group contains "animal" Patient resources 061 */ 062 ANIMAL, 063 /** 064 * Group contains healthcare practitioner resources 065 */ 066 PRACTITIONER, 067 /** 068 * Group contains Device resources 069 */ 070 DEVICE, 071 /** 072 * Group contains Medication resources 073 */ 074 MEDICATION, 075 /** 076 * Group contains Substance resources 077 */ 078 SUBSTANCE, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 public static GroupType fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("person".equals(codeString)) 087 return PERSON; 088 if ("animal".equals(codeString)) 089 return ANIMAL; 090 if ("practitioner".equals(codeString)) 091 return PRACTITIONER; 092 if ("device".equals(codeString)) 093 return DEVICE; 094 if ("medication".equals(codeString)) 095 return MEDICATION; 096 if ("substance".equals(codeString)) 097 return SUBSTANCE; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown GroupType code '"+codeString+"'"); 102 } 103 public String toCode() { 104 switch (this) { 105 case PERSON: return "person"; 106 case ANIMAL: return "animal"; 107 case PRACTITIONER: return "practitioner"; 108 case DEVICE: return "device"; 109 case MEDICATION: return "medication"; 110 case SUBSTANCE: return "substance"; 111 case NULL: return null; 112 default: return "?"; 113 } 114 } 115 public String getSystem() { 116 switch (this) { 117 case PERSON: return "http://hl7.org/fhir/group-type"; 118 case ANIMAL: return "http://hl7.org/fhir/group-type"; 119 case PRACTITIONER: return "http://hl7.org/fhir/group-type"; 120 case DEVICE: return "http://hl7.org/fhir/group-type"; 121 case MEDICATION: return "http://hl7.org/fhir/group-type"; 122 case SUBSTANCE: return "http://hl7.org/fhir/group-type"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 public String getDefinition() { 128 switch (this) { 129 case PERSON: return "Group contains \"person\" Patient resources"; 130 case ANIMAL: return "Group contains \"animal\" Patient resources"; 131 case PRACTITIONER: return "Group contains healthcare practitioner resources"; 132 case DEVICE: return "Group contains Device resources"; 133 case MEDICATION: return "Group contains Medication resources"; 134 case SUBSTANCE: return "Group contains Substance resources"; 135 case NULL: return null; 136 default: return "?"; 137 } 138 } 139 public String getDisplay() { 140 switch (this) { 141 case PERSON: return "Person"; 142 case ANIMAL: return "Animal"; 143 case PRACTITIONER: return "Practitioner"; 144 case DEVICE: return "Device"; 145 case MEDICATION: return "Medication"; 146 case SUBSTANCE: return "Substance"; 147 case NULL: return null; 148 default: return "?"; 149 } 150 } 151 } 152 153 public static class GroupTypeEnumFactory implements EnumFactory<GroupType> { 154 public GroupType fromCode(String codeString) throws IllegalArgumentException { 155 if (codeString == null || "".equals(codeString)) 156 if (codeString == null || "".equals(codeString)) 157 return null; 158 if ("person".equals(codeString)) 159 return GroupType.PERSON; 160 if ("animal".equals(codeString)) 161 return GroupType.ANIMAL; 162 if ("practitioner".equals(codeString)) 163 return GroupType.PRACTITIONER; 164 if ("device".equals(codeString)) 165 return GroupType.DEVICE; 166 if ("medication".equals(codeString)) 167 return GroupType.MEDICATION; 168 if ("substance".equals(codeString)) 169 return GroupType.SUBSTANCE; 170 throw new IllegalArgumentException("Unknown GroupType code '"+codeString+"'"); 171 } 172 public Enumeration<GroupType> fromType(PrimitiveType<?> code) throws FHIRException { 173 if (code == null) 174 return null; 175 if (code.isEmpty()) 176 return new Enumeration<GroupType>(this); 177 String codeString = code.asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("person".equals(codeString)) 181 return new Enumeration<GroupType>(this, GroupType.PERSON); 182 if ("animal".equals(codeString)) 183 return new Enumeration<GroupType>(this, GroupType.ANIMAL); 184 if ("practitioner".equals(codeString)) 185 return new Enumeration<GroupType>(this, GroupType.PRACTITIONER); 186 if ("device".equals(codeString)) 187 return new Enumeration<GroupType>(this, GroupType.DEVICE); 188 if ("medication".equals(codeString)) 189 return new Enumeration<GroupType>(this, GroupType.MEDICATION); 190 if ("substance".equals(codeString)) 191 return new Enumeration<GroupType>(this, GroupType.SUBSTANCE); 192 throw new FHIRException("Unknown GroupType code '"+codeString+"'"); 193 } 194 public String toCode(GroupType code) { 195 if (code == GroupType.NULL) 196 return null; 197 if (code == GroupType.PERSON) 198 return "person"; 199 if (code == GroupType.ANIMAL) 200 return "animal"; 201 if (code == GroupType.PRACTITIONER) 202 return "practitioner"; 203 if (code == GroupType.DEVICE) 204 return "device"; 205 if (code == GroupType.MEDICATION) 206 return "medication"; 207 if (code == GroupType.SUBSTANCE) 208 return "substance"; 209 return "?"; 210 } 211 public String toSystem(GroupType code) { 212 return code.getSystem(); 213 } 214 } 215 216 @Block() 217 public static class GroupCharacteristicComponent extends BackboneElement implements IBaseBackboneElement { 218 /** 219 * A code that identifies the kind of trait being asserted. 220 */ 221 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 222 @Description(shortDefinition="Kind of characteristic", formalDefinition="A code that identifies the kind of trait being asserted." ) 223 protected CodeableConcept code; 224 225 /** 226 * The value of the trait that holds (or does not hold - see 'exclude') for members of the group. 227 */ 228 @Child(name = "value", type = {CodeableConcept.class, BooleanType.class, Quantity.class, Range.class}, order=2, min=1, max=1, modifier=false, summary=false) 229 @Description(shortDefinition="Value held by characteristic", formalDefinition="The value of the trait that holds (or does not hold - see 'exclude') for members of the group." ) 230 protected Type value; 231 232 /** 233 * If true, indicates the characteristic is one that is NOT held by members of the group. 234 */ 235 @Child(name = "exclude", type = {BooleanType.class}, order=3, min=1, max=1, modifier=true, summary=false) 236 @Description(shortDefinition="Group includes or excludes", formalDefinition="If true, indicates the characteristic is one that is NOT held by members of the group." ) 237 protected BooleanType exclude; 238 239 /** 240 * The period over which the characteristic is tested; e.g. the patient had an operation during the month of June. 241 */ 242 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=false) 243 @Description(shortDefinition="Period over which characteristic is tested", formalDefinition="The period over which the characteristic is tested; e.g. the patient had an operation during the month of June." ) 244 protected Period period; 245 246 private static final long serialVersionUID = -1000688967L; 247 248 /** 249 * Constructor 250 */ 251 public GroupCharacteristicComponent() { 252 super(); 253 } 254 255 /** 256 * Constructor 257 */ 258 public GroupCharacteristicComponent(CodeableConcept code, Type value, BooleanType exclude) { 259 super(); 260 this.code = code; 261 this.value = value; 262 this.exclude = exclude; 263 } 264 265 /** 266 * @return {@link #code} (A code that identifies the kind of trait being asserted.) 267 */ 268 public CodeableConcept getCode() { 269 if (this.code == null) 270 if (Configuration.errorOnAutoCreate()) 271 throw new Error("Attempt to auto-create GroupCharacteristicComponent.code"); 272 else if (Configuration.doAutoCreate()) 273 this.code = new CodeableConcept(); // cc 274 return this.code; 275 } 276 277 public boolean hasCode() { 278 return this.code != null && !this.code.isEmpty(); 279 } 280 281 /** 282 * @param value {@link #code} (A code that identifies the kind of trait being asserted.) 283 */ 284 public GroupCharacteristicComponent setCode(CodeableConcept value) { 285 this.code = value; 286 return this; 287 } 288 289 /** 290 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 291 */ 292 public Type getValue() { 293 return this.value; 294 } 295 296 /** 297 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 298 */ 299 public CodeableConcept getValueCodeableConcept() throws FHIRException { 300 if (this.value == null) 301 return null; 302 if (!(this.value instanceof CodeableConcept)) 303 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.value.getClass().getName()+" was encountered"); 304 return (CodeableConcept) this.value; 305 } 306 307 public boolean hasValueCodeableConcept() { 308 return this.value instanceof CodeableConcept; 309 } 310 311 /** 312 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 313 */ 314 public BooleanType getValueBooleanType() throws FHIRException { 315 if (this.value == null) 316 return null; 317 if (!(this.value instanceof BooleanType)) 318 throw new FHIRException("Type mismatch: the type BooleanType was expected, but "+this.value.getClass().getName()+" was encountered"); 319 return (BooleanType) this.value; 320 } 321 322 public boolean hasValueBooleanType() { 323 return this.value instanceof BooleanType; 324 } 325 326 /** 327 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 328 */ 329 public Quantity getValueQuantity() throws FHIRException { 330 if (this.value == null) 331 return null; 332 if (!(this.value instanceof Quantity)) 333 throw new FHIRException("Type mismatch: the type Quantity was expected, but "+this.value.getClass().getName()+" was encountered"); 334 return (Quantity) this.value; 335 } 336 337 public boolean hasValueQuantity() { 338 return this.value instanceof Quantity; 339 } 340 341 /** 342 * @return {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 343 */ 344 public Range getValueRange() throws FHIRException { 345 if (this.value == null) 346 return null; 347 if (!(this.value instanceof Range)) 348 throw new FHIRException("Type mismatch: the type Range was expected, but "+this.value.getClass().getName()+" was encountered"); 349 return (Range) this.value; 350 } 351 352 public boolean hasValueRange() { 353 return this.value instanceof Range; 354 } 355 356 public boolean hasValue() { 357 return this.value != null && !this.value.isEmpty(); 358 } 359 360 /** 361 * @param value {@link #value} (The value of the trait that holds (or does not hold - see 'exclude') for members of the group.) 362 */ 363 public GroupCharacteristicComponent setValue(Type value) throws FHIRFormatError { 364 if (value != null && !(value instanceof CodeableConcept || value instanceof BooleanType || value instanceof Quantity || value instanceof Range)) 365 throw new FHIRFormatError("Not the right type for Group.characteristic.value[x]: "+value.fhirType()); 366 this.value = value; 367 return this; 368 } 369 370 /** 371 * @return {@link #exclude} (If true, indicates the characteristic is one that is NOT held by members of the group.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 372 */ 373 public BooleanType getExcludeElement() { 374 if (this.exclude == null) 375 if (Configuration.errorOnAutoCreate()) 376 throw new Error("Attempt to auto-create GroupCharacteristicComponent.exclude"); 377 else if (Configuration.doAutoCreate()) 378 this.exclude = new BooleanType(); // bb 379 return this.exclude; 380 } 381 382 public boolean hasExcludeElement() { 383 return this.exclude != null && !this.exclude.isEmpty(); 384 } 385 386 public boolean hasExclude() { 387 return this.exclude != null && !this.exclude.isEmpty(); 388 } 389 390 /** 391 * @param value {@link #exclude} (If true, indicates the characteristic is one that is NOT held by members of the group.). This is the underlying object with id, value and extensions. The accessor "getExclude" gives direct access to the value 392 */ 393 public GroupCharacteristicComponent setExcludeElement(BooleanType value) { 394 this.exclude = value; 395 return this; 396 } 397 398 /** 399 * @return If true, indicates the characteristic is one that is NOT held by members of the group. 400 */ 401 public boolean getExclude() { 402 return this.exclude == null || this.exclude.isEmpty() ? false : this.exclude.getValue(); 403 } 404 405 /** 406 * @param value If true, indicates the characteristic is one that is NOT held by members of the group. 407 */ 408 public GroupCharacteristicComponent setExclude(boolean value) { 409 if (this.exclude == null) 410 this.exclude = new BooleanType(); 411 this.exclude.setValue(value); 412 return this; 413 } 414 415 /** 416 * @return {@link #period} (The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.) 417 */ 418 public Period getPeriod() { 419 if (this.period == null) 420 if (Configuration.errorOnAutoCreate()) 421 throw new Error("Attempt to auto-create GroupCharacteristicComponent.period"); 422 else if (Configuration.doAutoCreate()) 423 this.period = new Period(); // cc 424 return this.period; 425 } 426 427 public boolean hasPeriod() { 428 return this.period != null && !this.period.isEmpty(); 429 } 430 431 /** 432 * @param value {@link #period} (The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.) 433 */ 434 public GroupCharacteristicComponent setPeriod(Period value) { 435 this.period = value; 436 return this; 437 } 438 439 protected void listChildren(List<Property> children) { 440 super.listChildren(children); 441 children.add(new Property("code", "CodeableConcept", "A code that identifies the kind of trait being asserted.", 0, 1, code)); 442 children.add(new Property("value[x]", "CodeableConcept|boolean|Quantity|Range", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value)); 443 children.add(new Property("exclude", "boolean", "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude)); 444 children.add(new Property("period", "Period", "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 0, 1, period)); 445 } 446 447 @Override 448 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 449 switch (_hash) { 450 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code that identifies the kind of trait being asserted.", 0, 1, code); 451 case -1410166417: /*value[x]*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 452 case 111972721: /*value*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 453 case 924902896: /*valueCodeableConcept*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 454 case 733421943: /*valueBoolean*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 455 case -2029823716: /*valueQuantity*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 456 case 2030761548: /*valueRange*/ return new Property("value[x]", "CodeableConcept|boolean|Quantity|Range", "The value of the trait that holds (or does not hold - see 'exclude') for members of the group.", 0, 1, value); 457 case -1321148966: /*exclude*/ return new Property("exclude", "boolean", "If true, indicates the characteristic is one that is NOT held by members of the group.", 0, 1, exclude); 458 case -991726143: /*period*/ return new Property("period", "Period", "The period over which the characteristic is tested; e.g. the patient had an operation during the month of June.", 0, 1, period); 459 default: return super.getNamedProperty(_hash, _name, _checkValid); 460 } 461 462 } 463 464 @Override 465 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 466 switch (hash) { 467 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 468 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // Type 469 case -1321148966: /*exclude*/ return this.exclude == null ? new Base[0] : new Base[] {this.exclude}; // BooleanType 470 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 471 default: return super.getProperty(hash, name, checkValid); 472 } 473 474 } 475 476 @Override 477 public Base setProperty(int hash, String name, Base value) throws FHIRException { 478 switch (hash) { 479 case 3059181: // code 480 this.code = castToCodeableConcept(value); // CodeableConcept 481 return value; 482 case 111972721: // value 483 this.value = castToType(value); // Type 484 return value; 485 case -1321148966: // exclude 486 this.exclude = castToBoolean(value); // BooleanType 487 return value; 488 case -991726143: // period 489 this.period = castToPeriod(value); // Period 490 return value; 491 default: return super.setProperty(hash, name, value); 492 } 493 494 } 495 496 @Override 497 public Base setProperty(String name, Base value) throws FHIRException { 498 if (name.equals("code")) { 499 this.code = castToCodeableConcept(value); // CodeableConcept 500 } else if (name.equals("value[x]")) { 501 this.value = castToType(value); // Type 502 } else if (name.equals("exclude")) { 503 this.exclude = castToBoolean(value); // BooleanType 504 } else if (name.equals("period")) { 505 this.period = castToPeriod(value); // Period 506 } else 507 return super.setProperty(name, value); 508 return value; 509 } 510 511 @Override 512 public Base makeProperty(int hash, String name) throws FHIRException { 513 switch (hash) { 514 case 3059181: return getCode(); 515 case -1410166417: return getValue(); 516 case 111972721: return getValue(); 517 case -1321148966: return getExcludeElement(); 518 case -991726143: return getPeriod(); 519 default: return super.makeProperty(hash, name); 520 } 521 522 } 523 524 @Override 525 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 526 switch (hash) { 527 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 528 case 111972721: /*value*/ return new String[] {"CodeableConcept", "boolean", "Quantity", "Range"}; 529 case -1321148966: /*exclude*/ return new String[] {"boolean"}; 530 case -991726143: /*period*/ return new String[] {"Period"}; 531 default: return super.getTypesForProperty(hash, name); 532 } 533 534 } 535 536 @Override 537 public Base addChild(String name) throws FHIRException { 538 if (name.equals("code")) { 539 this.code = new CodeableConcept(); 540 return this.code; 541 } 542 else if (name.equals("valueCodeableConcept")) { 543 this.value = new CodeableConcept(); 544 return this.value; 545 } 546 else if (name.equals("valueBoolean")) { 547 this.value = new BooleanType(); 548 return this.value; 549 } 550 else if (name.equals("valueQuantity")) { 551 this.value = new Quantity(); 552 return this.value; 553 } 554 else if (name.equals("valueRange")) { 555 this.value = new Range(); 556 return this.value; 557 } 558 else if (name.equals("exclude")) { 559 throw new FHIRException("Cannot call addChild on a singleton property Group.exclude"); 560 } 561 else if (name.equals("period")) { 562 this.period = new Period(); 563 return this.period; 564 } 565 else 566 return super.addChild(name); 567 } 568 569 public GroupCharacteristicComponent copy() { 570 GroupCharacteristicComponent dst = new GroupCharacteristicComponent(); 571 copyValues(dst); 572 dst.code = code == null ? null : code.copy(); 573 dst.value = value == null ? null : value.copy(); 574 dst.exclude = exclude == null ? null : exclude.copy(); 575 dst.period = period == null ? null : period.copy(); 576 return dst; 577 } 578 579 @Override 580 public boolean equalsDeep(Base other_) { 581 if (!super.equalsDeep(other_)) 582 return false; 583 if (!(other_ instanceof GroupCharacteristicComponent)) 584 return false; 585 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 586 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true) && compareDeep(exclude, o.exclude, true) 587 && compareDeep(period, o.period, true); 588 } 589 590 @Override 591 public boolean equalsShallow(Base other_) { 592 if (!super.equalsShallow(other_)) 593 return false; 594 if (!(other_ instanceof GroupCharacteristicComponent)) 595 return false; 596 GroupCharacteristicComponent o = (GroupCharacteristicComponent) other_; 597 return compareValues(exclude, o.exclude, true); 598 } 599 600 public boolean isEmpty() { 601 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value, exclude, period 602 ); 603 } 604 605 public String fhirType() { 606 return "Group.characteristic"; 607 608 } 609 610 } 611 612 @Block() 613 public static class GroupMemberComponent extends BackboneElement implements IBaseBackboneElement { 614 /** 615 * A reference to the entity that is a member of the group. Must be consistent with Group.type. 616 */ 617 @Child(name = "entity", type = {Patient.class, Practitioner.class, Device.class, Medication.class, Substance.class}, order=1, min=1, max=1, modifier=false, summary=false) 618 @Description(shortDefinition="Reference to the group member", formalDefinition="A reference to the entity that is a member of the group. Must be consistent with Group.type." ) 619 protected Reference entity; 620 621 /** 622 * The actual object that is the target of the reference (A reference to the entity that is a member of the group. Must be consistent with Group.type.) 623 */ 624 protected Resource entityTarget; 625 626 /** 627 * The period that the member was in the group, if known. 628 */ 629 @Child(name = "period", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 630 @Description(shortDefinition="Period member belonged to the group", formalDefinition="The period that the member was in the group, if known." ) 631 protected Period period; 632 633 /** 634 * A flag to indicate that the member is no longer in the group, but previously may have been a member. 635 */ 636 @Child(name = "inactive", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 637 @Description(shortDefinition="If member is no longer in group", formalDefinition="A flag to indicate that the member is no longer in the group, but previously may have been a member." ) 638 protected BooleanType inactive; 639 640 private static final long serialVersionUID = -333869055L; 641 642 /** 643 * Constructor 644 */ 645 public GroupMemberComponent() { 646 super(); 647 } 648 649 /** 650 * Constructor 651 */ 652 public GroupMemberComponent(Reference entity) { 653 super(); 654 this.entity = entity; 655 } 656 657 /** 658 * @return {@link #entity} (A reference to the entity that is a member of the group. Must be consistent with Group.type.) 659 */ 660 public Reference getEntity() { 661 if (this.entity == null) 662 if (Configuration.errorOnAutoCreate()) 663 throw new Error("Attempt to auto-create GroupMemberComponent.entity"); 664 else if (Configuration.doAutoCreate()) 665 this.entity = new Reference(); // cc 666 return this.entity; 667 } 668 669 public boolean hasEntity() { 670 return this.entity != null && !this.entity.isEmpty(); 671 } 672 673 /** 674 * @param value {@link #entity} (A reference to the entity that is a member of the group. Must be consistent with Group.type.) 675 */ 676 public GroupMemberComponent setEntity(Reference value) { 677 this.entity = value; 678 return this; 679 } 680 681 /** 682 * @return {@link #entity} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the entity that is a member of the group. Must be consistent with Group.type.) 683 */ 684 public Resource getEntityTarget() { 685 return this.entityTarget; 686 } 687 688 /** 689 * @param value {@link #entity} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the entity that is a member of the group. Must be consistent with Group.type.) 690 */ 691 public GroupMemberComponent setEntityTarget(Resource value) { 692 this.entityTarget = value; 693 return this; 694 } 695 696 /** 697 * @return {@link #period} (The period that the member was in the group, if known.) 698 */ 699 public Period getPeriod() { 700 if (this.period == null) 701 if (Configuration.errorOnAutoCreate()) 702 throw new Error("Attempt to auto-create GroupMemberComponent.period"); 703 else if (Configuration.doAutoCreate()) 704 this.period = new Period(); // cc 705 return this.period; 706 } 707 708 public boolean hasPeriod() { 709 return this.period != null && !this.period.isEmpty(); 710 } 711 712 /** 713 * @param value {@link #period} (The period that the member was in the group, if known.) 714 */ 715 public GroupMemberComponent setPeriod(Period value) { 716 this.period = value; 717 return this; 718 } 719 720 /** 721 * @return {@link #inactive} (A flag to indicate that the member is no longer in the group, but previously may have been a member.). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 722 */ 723 public BooleanType getInactiveElement() { 724 if (this.inactive == null) 725 if (Configuration.errorOnAutoCreate()) 726 throw new Error("Attempt to auto-create GroupMemberComponent.inactive"); 727 else if (Configuration.doAutoCreate()) 728 this.inactive = new BooleanType(); // bb 729 return this.inactive; 730 } 731 732 public boolean hasInactiveElement() { 733 return this.inactive != null && !this.inactive.isEmpty(); 734 } 735 736 public boolean hasInactive() { 737 return this.inactive != null && !this.inactive.isEmpty(); 738 } 739 740 /** 741 * @param value {@link #inactive} (A flag to indicate that the member is no longer in the group, but previously may have been a member.). This is the underlying object with id, value and extensions. The accessor "getInactive" gives direct access to the value 742 */ 743 public GroupMemberComponent setInactiveElement(BooleanType value) { 744 this.inactive = value; 745 return this; 746 } 747 748 /** 749 * @return A flag to indicate that the member is no longer in the group, but previously may have been a member. 750 */ 751 public boolean getInactive() { 752 return this.inactive == null || this.inactive.isEmpty() ? false : this.inactive.getValue(); 753 } 754 755 /** 756 * @param value A flag to indicate that the member is no longer in the group, but previously may have been a member. 757 */ 758 public GroupMemberComponent setInactive(boolean value) { 759 if (this.inactive == null) 760 this.inactive = new BooleanType(); 761 this.inactive.setValue(value); 762 return this; 763 } 764 765 protected void listChildren(List<Property> children) { 766 super.listChildren(children); 767 children.add(new Property("entity", "Reference(Patient|Practitioner|Device|Medication|Substance)", "A reference to the entity that is a member of the group. Must be consistent with Group.type.", 0, 1, entity)); 768 children.add(new Property("period", "Period", "The period that the member was in the group, if known.", 0, 1, period)); 769 children.add(new Property("inactive", "boolean", "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 1, inactive)); 770 } 771 772 @Override 773 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 774 switch (_hash) { 775 case -1298275357: /*entity*/ return new Property("entity", "Reference(Patient|Practitioner|Device|Medication|Substance)", "A reference to the entity that is a member of the group. Must be consistent with Group.type.", 0, 1, entity); 776 case -991726143: /*period*/ return new Property("period", "Period", "The period that the member was in the group, if known.", 0, 1, period); 777 case 24665195: /*inactive*/ return new Property("inactive", "boolean", "A flag to indicate that the member is no longer in the group, but previously may have been a member.", 0, 1, inactive); 778 default: return super.getNamedProperty(_hash, _name, _checkValid); 779 } 780 781 } 782 783 @Override 784 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 785 switch (hash) { 786 case -1298275357: /*entity*/ return this.entity == null ? new Base[0] : new Base[] {this.entity}; // Reference 787 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 788 case 24665195: /*inactive*/ return this.inactive == null ? new Base[0] : new Base[] {this.inactive}; // BooleanType 789 default: return super.getProperty(hash, name, checkValid); 790 } 791 792 } 793 794 @Override 795 public Base setProperty(int hash, String name, Base value) throws FHIRException { 796 switch (hash) { 797 case -1298275357: // entity 798 this.entity = castToReference(value); // Reference 799 return value; 800 case -991726143: // period 801 this.period = castToPeriod(value); // Period 802 return value; 803 case 24665195: // inactive 804 this.inactive = castToBoolean(value); // BooleanType 805 return value; 806 default: return super.setProperty(hash, name, value); 807 } 808 809 } 810 811 @Override 812 public Base setProperty(String name, Base value) throws FHIRException { 813 if (name.equals("entity")) { 814 this.entity = castToReference(value); // Reference 815 } else if (name.equals("period")) { 816 this.period = castToPeriod(value); // Period 817 } else if (name.equals("inactive")) { 818 this.inactive = castToBoolean(value); // BooleanType 819 } else 820 return super.setProperty(name, value); 821 return value; 822 } 823 824 @Override 825 public Base makeProperty(int hash, String name) throws FHIRException { 826 switch (hash) { 827 case -1298275357: return getEntity(); 828 case -991726143: return getPeriod(); 829 case 24665195: return getInactiveElement(); 830 default: return super.makeProperty(hash, name); 831 } 832 833 } 834 835 @Override 836 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 837 switch (hash) { 838 case -1298275357: /*entity*/ return new String[] {"Reference"}; 839 case -991726143: /*period*/ return new String[] {"Period"}; 840 case 24665195: /*inactive*/ return new String[] {"boolean"}; 841 default: return super.getTypesForProperty(hash, name); 842 } 843 844 } 845 846 @Override 847 public Base addChild(String name) throws FHIRException { 848 if (name.equals("entity")) { 849 this.entity = new Reference(); 850 return this.entity; 851 } 852 else if (name.equals("period")) { 853 this.period = new Period(); 854 return this.period; 855 } 856 else if (name.equals("inactive")) { 857 throw new FHIRException("Cannot call addChild on a singleton property Group.inactive"); 858 } 859 else 860 return super.addChild(name); 861 } 862 863 public GroupMemberComponent copy() { 864 GroupMemberComponent dst = new GroupMemberComponent(); 865 copyValues(dst); 866 dst.entity = entity == null ? null : entity.copy(); 867 dst.period = period == null ? null : period.copy(); 868 dst.inactive = inactive == null ? null : inactive.copy(); 869 return dst; 870 } 871 872 @Override 873 public boolean equalsDeep(Base other_) { 874 if (!super.equalsDeep(other_)) 875 return false; 876 if (!(other_ instanceof GroupMemberComponent)) 877 return false; 878 GroupMemberComponent o = (GroupMemberComponent) other_; 879 return compareDeep(entity, o.entity, true) && compareDeep(period, o.period, true) && compareDeep(inactive, o.inactive, true) 880 ; 881 } 882 883 @Override 884 public boolean equalsShallow(Base other_) { 885 if (!super.equalsShallow(other_)) 886 return false; 887 if (!(other_ instanceof GroupMemberComponent)) 888 return false; 889 GroupMemberComponent o = (GroupMemberComponent) other_; 890 return compareValues(inactive, o.inactive, true); 891 } 892 893 public boolean isEmpty() { 894 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(entity, period, inactive 895 ); 896 } 897 898 public String fhirType() { 899 return "Group.member"; 900 901 } 902 903 } 904 905 /** 906 * A unique business identifier for this group. 907 */ 908 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 909 @Description(shortDefinition="Unique id", formalDefinition="A unique business identifier for this group." ) 910 protected List<Identifier> identifier; 911 912 /** 913 * Indicates whether the record for the group is available for use or is merely being retained for historical purposes. 914 */ 915 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=false, summary=true) 916 @Description(shortDefinition="Whether this group's record is in active use", formalDefinition="Indicates whether the record for the group is available for use or is merely being retained for historical purposes." ) 917 protected BooleanType active; 918 919 /** 920 * Identifies the broad classification of the kind of resources the group includes. 921 */ 922 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 923 @Description(shortDefinition="person | animal | practitioner | device | medication | substance", formalDefinition="Identifies the broad classification of the kind of resources the group includes." ) 924 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/group-type") 925 protected Enumeration<GroupType> type; 926 927 /** 928 * If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals. 929 */ 930 @Child(name = "actual", type = {BooleanType.class}, order=3, min=1, max=1, modifier=false, summary=true) 931 @Description(shortDefinition="Descriptive or actual", formalDefinition="If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals." ) 932 protected BooleanType actual; 933 934 /** 935 * Provides a specific type of resource the group includes; e.g. "cow", "syringe", etc. 936 */ 937 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 938 @Description(shortDefinition="Kind of Group members", formalDefinition="Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc." ) 939 protected CodeableConcept code; 940 941 /** 942 * A label assigned to the group for human identification and communication. 943 */ 944 @Child(name = "name", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 945 @Description(shortDefinition="Label for Group", formalDefinition="A label assigned to the group for human identification and communication." ) 946 protected StringType name; 947 948 /** 949 * A count of the number of resource instances that are part of the group. 950 */ 951 @Child(name = "quantity", type = {UnsignedIntType.class}, order=6, min=0, max=1, modifier=false, summary=true) 952 @Description(shortDefinition="Number of members", formalDefinition="A count of the number of resource instances that are part of the group." ) 953 protected UnsignedIntType quantity; 954 955 /** 956 * Identifies the traits shared by members of the group. 957 */ 958 @Child(name = "characteristic", type = {}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 959 @Description(shortDefinition="Trait of group members", formalDefinition="Identifies the traits shared by members of the group." ) 960 protected List<GroupCharacteristicComponent> characteristic; 961 962 /** 963 * Identifies the resource instances that are members of the group. 964 */ 965 @Child(name = "member", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 966 @Description(shortDefinition="Who or what is in group", formalDefinition="Identifies the resource instances that are members of the group." ) 967 protected List<GroupMemberComponent> member; 968 969 private static final long serialVersionUID = 659980713L; 970 971 /** 972 * Constructor 973 */ 974 public Group() { 975 super(); 976 } 977 978 /** 979 * Constructor 980 */ 981 public Group(Enumeration<GroupType> type, BooleanType actual) { 982 super(); 983 this.type = type; 984 this.actual = actual; 985 } 986 987 /** 988 * @return {@link #identifier} (A unique business identifier for this group.) 989 */ 990 public List<Identifier> getIdentifier() { 991 if (this.identifier == null) 992 this.identifier = new ArrayList<Identifier>(); 993 return this.identifier; 994 } 995 996 /** 997 * @return Returns a reference to <code>this</code> for easy method chaining 998 */ 999 public Group setIdentifier(List<Identifier> theIdentifier) { 1000 this.identifier = theIdentifier; 1001 return this; 1002 } 1003 1004 public boolean hasIdentifier() { 1005 if (this.identifier == null) 1006 return false; 1007 for (Identifier item : this.identifier) 1008 if (!item.isEmpty()) 1009 return true; 1010 return false; 1011 } 1012 1013 public Identifier addIdentifier() { //3 1014 Identifier t = new Identifier(); 1015 if (this.identifier == null) 1016 this.identifier = new ArrayList<Identifier>(); 1017 this.identifier.add(t); 1018 return t; 1019 } 1020 1021 public Group addIdentifier(Identifier t) { //3 1022 if (t == null) 1023 return this; 1024 if (this.identifier == null) 1025 this.identifier = new ArrayList<Identifier>(); 1026 this.identifier.add(t); 1027 return this; 1028 } 1029 1030 /** 1031 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1032 */ 1033 public Identifier getIdentifierFirstRep() { 1034 if (getIdentifier().isEmpty()) { 1035 addIdentifier(); 1036 } 1037 return getIdentifier().get(0); 1038 } 1039 1040 /** 1041 * @return {@link #active} (Indicates whether the record for the group is available for use or is merely being retained for historical purposes.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1042 */ 1043 public BooleanType getActiveElement() { 1044 if (this.active == null) 1045 if (Configuration.errorOnAutoCreate()) 1046 throw new Error("Attempt to auto-create Group.active"); 1047 else if (Configuration.doAutoCreate()) 1048 this.active = new BooleanType(); // bb 1049 return this.active; 1050 } 1051 1052 public boolean hasActiveElement() { 1053 return this.active != null && !this.active.isEmpty(); 1054 } 1055 1056 public boolean hasActive() { 1057 return this.active != null && !this.active.isEmpty(); 1058 } 1059 1060 /** 1061 * @param value {@link #active} (Indicates whether the record for the group is available for use or is merely being retained for historical purposes.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1062 */ 1063 public Group setActiveElement(BooleanType value) { 1064 this.active = value; 1065 return this; 1066 } 1067 1068 /** 1069 * @return Indicates whether the record for the group is available for use or is merely being retained for historical purposes. 1070 */ 1071 public boolean getActive() { 1072 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1073 } 1074 1075 /** 1076 * @param value Indicates whether the record for the group is available for use or is merely being retained for historical purposes. 1077 */ 1078 public Group setActive(boolean value) { 1079 if (this.active == null) 1080 this.active = new BooleanType(); 1081 this.active.setValue(value); 1082 return this; 1083 } 1084 1085 /** 1086 * @return {@link #type} (Identifies the broad classification of the kind of resources the group includes.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1087 */ 1088 public Enumeration<GroupType> getTypeElement() { 1089 if (this.type == null) 1090 if (Configuration.errorOnAutoCreate()) 1091 throw new Error("Attempt to auto-create Group.type"); 1092 else if (Configuration.doAutoCreate()) 1093 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); // bb 1094 return this.type; 1095 } 1096 1097 public boolean hasTypeElement() { 1098 return this.type != null && !this.type.isEmpty(); 1099 } 1100 1101 public boolean hasType() { 1102 return this.type != null && !this.type.isEmpty(); 1103 } 1104 1105 /** 1106 * @param value {@link #type} (Identifies the broad classification of the kind of resources the group includes.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1107 */ 1108 public Group setTypeElement(Enumeration<GroupType> value) { 1109 this.type = value; 1110 return this; 1111 } 1112 1113 /** 1114 * @return Identifies the broad classification of the kind of resources the group includes. 1115 */ 1116 public GroupType getType() { 1117 return this.type == null ? null : this.type.getValue(); 1118 } 1119 1120 /** 1121 * @param value Identifies the broad classification of the kind of resources the group includes. 1122 */ 1123 public Group setType(GroupType value) { 1124 if (this.type == null) 1125 this.type = new Enumeration<GroupType>(new GroupTypeEnumFactory()); 1126 this.type.setValue(value); 1127 return this; 1128 } 1129 1130 /** 1131 * @return {@link #actual} (If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 1132 */ 1133 public BooleanType getActualElement() { 1134 if (this.actual == null) 1135 if (Configuration.errorOnAutoCreate()) 1136 throw new Error("Attempt to auto-create Group.actual"); 1137 else if (Configuration.doAutoCreate()) 1138 this.actual = new BooleanType(); // bb 1139 return this.actual; 1140 } 1141 1142 public boolean hasActualElement() { 1143 return this.actual != null && !this.actual.isEmpty(); 1144 } 1145 1146 public boolean hasActual() { 1147 return this.actual != null && !this.actual.isEmpty(); 1148 } 1149 1150 /** 1151 * @param value {@link #actual} (If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.). This is the underlying object with id, value and extensions. The accessor "getActual" gives direct access to the value 1152 */ 1153 public Group setActualElement(BooleanType value) { 1154 this.actual = value; 1155 return this; 1156 } 1157 1158 /** 1159 * @return If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals. 1160 */ 1161 public boolean getActual() { 1162 return this.actual == null || this.actual.isEmpty() ? false : this.actual.getValue(); 1163 } 1164 1165 /** 1166 * @param value If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals. 1167 */ 1168 public Group setActual(boolean value) { 1169 if (this.actual == null) 1170 this.actual = new BooleanType(); 1171 this.actual.setValue(value); 1172 return this; 1173 } 1174 1175 /** 1176 * @return {@link #code} (Provides a specific type of resource the group includes; e.g. "cow", "syringe", etc.) 1177 */ 1178 public CodeableConcept getCode() { 1179 if (this.code == null) 1180 if (Configuration.errorOnAutoCreate()) 1181 throw new Error("Attempt to auto-create Group.code"); 1182 else if (Configuration.doAutoCreate()) 1183 this.code = new CodeableConcept(); // cc 1184 return this.code; 1185 } 1186 1187 public boolean hasCode() { 1188 return this.code != null && !this.code.isEmpty(); 1189 } 1190 1191 /** 1192 * @param value {@link #code} (Provides a specific type of resource the group includes; e.g. "cow", "syringe", etc.) 1193 */ 1194 public Group setCode(CodeableConcept value) { 1195 this.code = value; 1196 return this; 1197 } 1198 1199 /** 1200 * @return {@link #name} (A label assigned to the group for human identification and communication.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1201 */ 1202 public StringType getNameElement() { 1203 if (this.name == null) 1204 if (Configuration.errorOnAutoCreate()) 1205 throw new Error("Attempt to auto-create Group.name"); 1206 else if (Configuration.doAutoCreate()) 1207 this.name = new StringType(); // bb 1208 return this.name; 1209 } 1210 1211 public boolean hasNameElement() { 1212 return this.name != null && !this.name.isEmpty(); 1213 } 1214 1215 public boolean hasName() { 1216 return this.name != null && !this.name.isEmpty(); 1217 } 1218 1219 /** 1220 * @param value {@link #name} (A label assigned to the group for human identification and communication.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1221 */ 1222 public Group setNameElement(StringType value) { 1223 this.name = value; 1224 return this; 1225 } 1226 1227 /** 1228 * @return A label assigned to the group for human identification and communication. 1229 */ 1230 public String getName() { 1231 return this.name == null ? null : this.name.getValue(); 1232 } 1233 1234 /** 1235 * @param value A label assigned to the group for human identification and communication. 1236 */ 1237 public Group setName(String value) { 1238 if (Utilities.noString(value)) 1239 this.name = null; 1240 else { 1241 if (this.name == null) 1242 this.name = new StringType(); 1243 this.name.setValue(value); 1244 } 1245 return this; 1246 } 1247 1248 /** 1249 * @return {@link #quantity} (A count of the number of resource instances that are part of the group.). This is the underlying object with id, value and extensions. The accessor "getQuantity" gives direct access to the value 1250 */ 1251 public UnsignedIntType getQuantityElement() { 1252 if (this.quantity == null) 1253 if (Configuration.errorOnAutoCreate()) 1254 throw new Error("Attempt to auto-create Group.quantity"); 1255 else if (Configuration.doAutoCreate()) 1256 this.quantity = new UnsignedIntType(); // bb 1257 return this.quantity; 1258 } 1259 1260 public boolean hasQuantityElement() { 1261 return this.quantity != null && !this.quantity.isEmpty(); 1262 } 1263 1264 public boolean hasQuantity() { 1265 return this.quantity != null && !this.quantity.isEmpty(); 1266 } 1267 1268 /** 1269 * @param value {@link #quantity} (A count of the number of resource instances that are part of the group.). This is the underlying object with id, value and extensions. The accessor "getQuantity" gives direct access to the value 1270 */ 1271 public Group setQuantityElement(UnsignedIntType value) { 1272 this.quantity = value; 1273 return this; 1274 } 1275 1276 /** 1277 * @return A count of the number of resource instances that are part of the group. 1278 */ 1279 public int getQuantity() { 1280 return this.quantity == null || this.quantity.isEmpty() ? 0 : this.quantity.getValue(); 1281 } 1282 1283 /** 1284 * @param value A count of the number of resource instances that are part of the group. 1285 */ 1286 public Group setQuantity(int value) { 1287 if (this.quantity == null) 1288 this.quantity = new UnsignedIntType(); 1289 this.quantity.setValue(value); 1290 return this; 1291 } 1292 1293 /** 1294 * @return {@link #characteristic} (Identifies the traits shared by members of the group.) 1295 */ 1296 public List<GroupCharacteristicComponent> getCharacteristic() { 1297 if (this.characteristic == null) 1298 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1299 return this.characteristic; 1300 } 1301 1302 /** 1303 * @return Returns a reference to <code>this</code> for easy method chaining 1304 */ 1305 public Group setCharacteristic(List<GroupCharacteristicComponent> theCharacteristic) { 1306 this.characteristic = theCharacteristic; 1307 return this; 1308 } 1309 1310 public boolean hasCharacteristic() { 1311 if (this.characteristic == null) 1312 return false; 1313 for (GroupCharacteristicComponent item : this.characteristic) 1314 if (!item.isEmpty()) 1315 return true; 1316 return false; 1317 } 1318 1319 public GroupCharacteristicComponent addCharacteristic() { //3 1320 GroupCharacteristicComponent t = new GroupCharacteristicComponent(); 1321 if (this.characteristic == null) 1322 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1323 this.characteristic.add(t); 1324 return t; 1325 } 1326 1327 public Group addCharacteristic(GroupCharacteristicComponent t) { //3 1328 if (t == null) 1329 return this; 1330 if (this.characteristic == null) 1331 this.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1332 this.characteristic.add(t); 1333 return this; 1334 } 1335 1336 /** 1337 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist 1338 */ 1339 public GroupCharacteristicComponent getCharacteristicFirstRep() { 1340 if (getCharacteristic().isEmpty()) { 1341 addCharacteristic(); 1342 } 1343 return getCharacteristic().get(0); 1344 } 1345 1346 /** 1347 * @return {@link #member} (Identifies the resource instances that are members of the group.) 1348 */ 1349 public List<GroupMemberComponent> getMember() { 1350 if (this.member == null) 1351 this.member = new ArrayList<GroupMemberComponent>(); 1352 return this.member; 1353 } 1354 1355 /** 1356 * @return Returns a reference to <code>this</code> for easy method chaining 1357 */ 1358 public Group setMember(List<GroupMemberComponent> theMember) { 1359 this.member = theMember; 1360 return this; 1361 } 1362 1363 public boolean hasMember() { 1364 if (this.member == null) 1365 return false; 1366 for (GroupMemberComponent item : this.member) 1367 if (!item.isEmpty()) 1368 return true; 1369 return false; 1370 } 1371 1372 public GroupMemberComponent addMember() { //3 1373 GroupMemberComponent t = new GroupMemberComponent(); 1374 if (this.member == null) 1375 this.member = new ArrayList<GroupMemberComponent>(); 1376 this.member.add(t); 1377 return t; 1378 } 1379 1380 public Group addMember(GroupMemberComponent t) { //3 1381 if (t == null) 1382 return this; 1383 if (this.member == null) 1384 this.member = new ArrayList<GroupMemberComponent>(); 1385 this.member.add(t); 1386 return this; 1387 } 1388 1389 /** 1390 * @return The first repetition of repeating field {@link #member}, creating it if it does not already exist 1391 */ 1392 public GroupMemberComponent getMemberFirstRep() { 1393 if (getMember().isEmpty()) { 1394 addMember(); 1395 } 1396 return getMember().get(0); 1397 } 1398 1399 protected void listChildren(List<Property> children) { 1400 super.listChildren(children); 1401 children.add(new Property("identifier", "Identifier", "A unique business identifier for this group.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1402 children.add(new Property("active", "boolean", "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 0, 1, active)); 1403 children.add(new Property("type", "code", "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type)); 1404 children.add(new Property("actual", "boolean", "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 0, 1, actual)); 1405 children.add(new Property("code", "CodeableConcept", "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code)); 1406 children.add(new Property("name", "string", "A label assigned to the group for human identification and communication.", 0, 1, name)); 1407 children.add(new Property("quantity", "unsignedInt", "A count of the number of resource instances that are part of the group.", 0, 1, quantity)); 1408 children.add(new Property("characteristic", "", "Identifies the traits shared by members of the group.", 0, java.lang.Integer.MAX_VALUE, characteristic)); 1409 children.add(new Property("member", "", "Identifies the resource instances that are members of the group.", 0, java.lang.Integer.MAX_VALUE, member)); 1410 } 1411 1412 @Override 1413 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1414 switch (_hash) { 1415 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique business identifier for this group.", 0, java.lang.Integer.MAX_VALUE, identifier); 1416 case -1422950650: /*active*/ return new Property("active", "boolean", "Indicates whether the record for the group is available for use or is merely being retained for historical purposes.", 0, 1, active); 1417 case 3575610: /*type*/ return new Property("type", "code", "Identifies the broad classification of the kind of resources the group includes.", 0, 1, type); 1418 case -1422939762: /*actual*/ return new Property("actual", "boolean", "If true, indicates that the resource refers to a specific group of real individuals. If false, the group defines a set of intended individuals.", 0, 1, actual); 1419 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Provides a specific type of resource the group includes; e.g. \"cow\", \"syringe\", etc.", 0, 1, code); 1420 case 3373707: /*name*/ return new Property("name", "string", "A label assigned to the group for human identification and communication.", 0, 1, name); 1421 case -1285004149: /*quantity*/ return new Property("quantity", "unsignedInt", "A count of the number of resource instances that are part of the group.", 0, 1, quantity); 1422 case 366313883: /*characteristic*/ return new Property("characteristic", "", "Identifies the traits shared by members of the group.", 0, java.lang.Integer.MAX_VALUE, characteristic); 1423 case -1077769574: /*member*/ return new Property("member", "", "Identifies the resource instances that are members of the group.", 0, java.lang.Integer.MAX_VALUE, member); 1424 default: return super.getNamedProperty(_hash, _name, _checkValid); 1425 } 1426 1427 } 1428 1429 @Override 1430 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1431 switch (hash) { 1432 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1433 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 1434 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<GroupType> 1435 case -1422939762: /*actual*/ return this.actual == null ? new Base[0] : new Base[] {this.actual}; // BooleanType 1436 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1437 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1438 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // UnsignedIntType 1439 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // GroupCharacteristicComponent 1440 case -1077769574: /*member*/ return this.member == null ? new Base[0] : this.member.toArray(new Base[this.member.size()]); // GroupMemberComponent 1441 default: return super.getProperty(hash, name, checkValid); 1442 } 1443 1444 } 1445 1446 @Override 1447 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1448 switch (hash) { 1449 case -1618432855: // identifier 1450 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1451 return value; 1452 case -1422950650: // active 1453 this.active = castToBoolean(value); // BooleanType 1454 return value; 1455 case 3575610: // type 1456 value = new GroupTypeEnumFactory().fromType(castToCode(value)); 1457 this.type = (Enumeration) value; // Enumeration<GroupType> 1458 return value; 1459 case -1422939762: // actual 1460 this.actual = castToBoolean(value); // BooleanType 1461 return value; 1462 case 3059181: // code 1463 this.code = castToCodeableConcept(value); // CodeableConcept 1464 return value; 1465 case 3373707: // name 1466 this.name = castToString(value); // StringType 1467 return value; 1468 case -1285004149: // quantity 1469 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1470 return value; 1471 case 366313883: // characteristic 1472 this.getCharacteristic().add((GroupCharacteristicComponent) value); // GroupCharacteristicComponent 1473 return value; 1474 case -1077769574: // member 1475 this.getMember().add((GroupMemberComponent) value); // GroupMemberComponent 1476 return value; 1477 default: return super.setProperty(hash, name, value); 1478 } 1479 1480 } 1481 1482 @Override 1483 public Base setProperty(String name, Base value) throws FHIRException { 1484 if (name.equals("identifier")) { 1485 this.getIdentifier().add(castToIdentifier(value)); 1486 } else if (name.equals("active")) { 1487 this.active = castToBoolean(value); // BooleanType 1488 } else if (name.equals("type")) { 1489 value = new GroupTypeEnumFactory().fromType(castToCode(value)); 1490 this.type = (Enumeration) value; // Enumeration<GroupType> 1491 } else if (name.equals("actual")) { 1492 this.actual = castToBoolean(value); // BooleanType 1493 } else if (name.equals("code")) { 1494 this.code = castToCodeableConcept(value); // CodeableConcept 1495 } else if (name.equals("name")) { 1496 this.name = castToString(value); // StringType 1497 } else if (name.equals("quantity")) { 1498 this.quantity = castToUnsignedInt(value); // UnsignedIntType 1499 } else if (name.equals("characteristic")) { 1500 this.getCharacteristic().add((GroupCharacteristicComponent) value); 1501 } else if (name.equals("member")) { 1502 this.getMember().add((GroupMemberComponent) value); 1503 } else 1504 return super.setProperty(name, value); 1505 return value; 1506 } 1507 1508 @Override 1509 public Base makeProperty(int hash, String name) throws FHIRException { 1510 switch (hash) { 1511 case -1618432855: return addIdentifier(); 1512 case -1422950650: return getActiveElement(); 1513 case 3575610: return getTypeElement(); 1514 case -1422939762: return getActualElement(); 1515 case 3059181: return getCode(); 1516 case 3373707: return getNameElement(); 1517 case -1285004149: return getQuantityElement(); 1518 case 366313883: return addCharacteristic(); 1519 case -1077769574: return addMember(); 1520 default: return super.makeProperty(hash, name); 1521 } 1522 1523 } 1524 1525 @Override 1526 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1527 switch (hash) { 1528 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1529 case -1422950650: /*active*/ return new String[] {"boolean"}; 1530 case 3575610: /*type*/ return new String[] {"code"}; 1531 case -1422939762: /*actual*/ return new String[] {"boolean"}; 1532 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1533 case 3373707: /*name*/ return new String[] {"string"}; 1534 case -1285004149: /*quantity*/ return new String[] {"unsignedInt"}; 1535 case 366313883: /*characteristic*/ return new String[] {}; 1536 case -1077769574: /*member*/ return new String[] {}; 1537 default: return super.getTypesForProperty(hash, name); 1538 } 1539 1540 } 1541 1542 @Override 1543 public Base addChild(String name) throws FHIRException { 1544 if (name.equals("identifier")) { 1545 return addIdentifier(); 1546 } 1547 else if (name.equals("active")) { 1548 throw new FHIRException("Cannot call addChild on a singleton property Group.active"); 1549 } 1550 else if (name.equals("type")) { 1551 throw new FHIRException("Cannot call addChild on a singleton property Group.type"); 1552 } 1553 else if (name.equals("actual")) { 1554 throw new FHIRException("Cannot call addChild on a singleton property Group.actual"); 1555 } 1556 else if (name.equals("code")) { 1557 this.code = new CodeableConcept(); 1558 return this.code; 1559 } 1560 else if (name.equals("name")) { 1561 throw new FHIRException("Cannot call addChild on a singleton property Group.name"); 1562 } 1563 else if (name.equals("quantity")) { 1564 throw new FHIRException("Cannot call addChild on a singleton property Group.quantity"); 1565 } 1566 else if (name.equals("characteristic")) { 1567 return addCharacteristic(); 1568 } 1569 else if (name.equals("member")) { 1570 return addMember(); 1571 } 1572 else 1573 return super.addChild(name); 1574 } 1575 1576 public String fhirType() { 1577 return "Group"; 1578 1579 } 1580 1581 public Group copy() { 1582 Group dst = new Group(); 1583 copyValues(dst); 1584 if (identifier != null) { 1585 dst.identifier = new ArrayList<Identifier>(); 1586 for (Identifier i : identifier) 1587 dst.identifier.add(i.copy()); 1588 }; 1589 dst.active = active == null ? null : active.copy(); 1590 dst.type = type == null ? null : type.copy(); 1591 dst.actual = actual == null ? null : actual.copy(); 1592 dst.code = code == null ? null : code.copy(); 1593 dst.name = name == null ? null : name.copy(); 1594 dst.quantity = quantity == null ? null : quantity.copy(); 1595 if (characteristic != null) { 1596 dst.characteristic = new ArrayList<GroupCharacteristicComponent>(); 1597 for (GroupCharacteristicComponent i : characteristic) 1598 dst.characteristic.add(i.copy()); 1599 }; 1600 if (member != null) { 1601 dst.member = new ArrayList<GroupMemberComponent>(); 1602 for (GroupMemberComponent i : member) 1603 dst.member.add(i.copy()); 1604 }; 1605 return dst; 1606 } 1607 1608 protected Group typedCopy() { 1609 return copy(); 1610 } 1611 1612 @Override 1613 public boolean equalsDeep(Base other_) { 1614 if (!super.equalsDeep(other_)) 1615 return false; 1616 if (!(other_ instanceof Group)) 1617 return false; 1618 Group o = (Group) other_; 1619 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(type, o.type, true) 1620 && compareDeep(actual, o.actual, true) && compareDeep(code, o.code, true) && compareDeep(name, o.name, true) 1621 && compareDeep(quantity, o.quantity, true) && compareDeep(characteristic, o.characteristic, true) 1622 && compareDeep(member, o.member, true); 1623 } 1624 1625 @Override 1626 public boolean equalsShallow(Base other_) { 1627 if (!super.equalsShallow(other_)) 1628 return false; 1629 if (!(other_ instanceof Group)) 1630 return false; 1631 Group o = (Group) other_; 1632 return compareValues(active, o.active, true) && compareValues(type, o.type, true) && compareValues(actual, o.actual, true) 1633 && compareValues(name, o.name, true) && compareValues(quantity, o.quantity, true); 1634 } 1635 1636 public boolean isEmpty() { 1637 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, type 1638 , actual, code, name, quantity, characteristic, member); 1639 } 1640 1641 @Override 1642 public ResourceType getResourceType() { 1643 return ResourceType.Group; 1644 } 1645 1646 /** 1647 * Search parameter: <b>actual</b> 1648 * <p> 1649 * Description: <b>Descriptive or actual</b><br> 1650 * Type: <b>token</b><br> 1651 * Path: <b>Group.actual</b><br> 1652 * </p> 1653 */ 1654 @SearchParamDefinition(name="actual", path="Group.actual", description="Descriptive or actual", type="token" ) 1655 public static final String SP_ACTUAL = "actual"; 1656 /** 1657 * <b>Fluent Client</b> search parameter constant for <b>actual</b> 1658 * <p> 1659 * Description: <b>Descriptive or actual</b><br> 1660 * Type: <b>token</b><br> 1661 * Path: <b>Group.actual</b><br> 1662 * </p> 1663 */ 1664 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTUAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTUAL); 1665 1666 /** 1667 * Search parameter: <b>identifier</b> 1668 * <p> 1669 * Description: <b>Unique id</b><br> 1670 * Type: <b>token</b><br> 1671 * Path: <b>Group.identifier</b><br> 1672 * </p> 1673 */ 1674 @SearchParamDefinition(name="identifier", path="Group.identifier", description="Unique id", type="token" ) 1675 public static final String SP_IDENTIFIER = "identifier"; 1676 /** 1677 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1678 * <p> 1679 * Description: <b>Unique id</b><br> 1680 * Type: <b>token</b><br> 1681 * Path: <b>Group.identifier</b><br> 1682 * </p> 1683 */ 1684 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1685 1686 /** 1687 * Search parameter: <b>characteristic-value</b> 1688 * <p> 1689 * Description: <b>A composite of both characteristic and value</b><br> 1690 * Type: <b>composite</b><br> 1691 * Path: <b></b><br> 1692 * </p> 1693 */ 1694 @SearchParamDefinition(name="characteristic-value", path="Group.characteristic", description="A composite of both characteristic and value", type="composite", compositeOf={"characteristic", "value"} ) 1695 public static final String SP_CHARACTERISTIC_VALUE = "characteristic-value"; 1696 /** 1697 * <b>Fluent Client</b> search parameter constant for <b>characteristic-value</b> 1698 * <p> 1699 * Description: <b>A composite of both characteristic and value</b><br> 1700 * Type: <b>composite</b><br> 1701 * Path: <b></b><br> 1702 * </p> 1703 */ 1704 public static final ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam> CHARACTERISTIC_VALUE = new ca.uhn.fhir.rest.gclient.CompositeClientParam<ca.uhn.fhir.rest.gclient.TokenClientParam, ca.uhn.fhir.rest.gclient.TokenClientParam>(SP_CHARACTERISTIC_VALUE); 1705 1706 /** 1707 * Search parameter: <b>code</b> 1708 * <p> 1709 * Description: <b>The kind of resources contained</b><br> 1710 * Type: <b>token</b><br> 1711 * Path: <b>Group.code</b><br> 1712 * </p> 1713 */ 1714 @SearchParamDefinition(name="code", path="Group.code", description="The kind of resources contained", type="token" ) 1715 public static final String SP_CODE = "code"; 1716 /** 1717 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1718 * <p> 1719 * Description: <b>The kind of resources contained</b><br> 1720 * Type: <b>token</b><br> 1721 * Path: <b>Group.code</b><br> 1722 * </p> 1723 */ 1724 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1725 1726 /** 1727 * Search parameter: <b>member</b> 1728 * <p> 1729 * Description: <b>Reference to the group member</b><br> 1730 * Type: <b>reference</b><br> 1731 * Path: <b>Group.member.entity</b><br> 1732 * </p> 1733 */ 1734 @SearchParamDefinition(name="member", path="Group.member.entity", description="Reference to the group member", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Medication.class, Patient.class, Practitioner.class, Substance.class } ) 1735 public static final String SP_MEMBER = "member"; 1736 /** 1737 * <b>Fluent Client</b> search parameter constant for <b>member</b> 1738 * <p> 1739 * Description: <b>Reference to the group member</b><br> 1740 * Type: <b>reference</b><br> 1741 * Path: <b>Group.member.entity</b><br> 1742 * </p> 1743 */ 1744 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEMBER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEMBER); 1745 1746/** 1747 * Constant for fluent queries to be used to add include statements. Specifies 1748 * the path value of "<b>Group:member</b>". 1749 */ 1750 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEMBER = new ca.uhn.fhir.model.api.Include("Group:member").toLocked(); 1751 1752 /** 1753 * Search parameter: <b>exclude</b> 1754 * <p> 1755 * Description: <b>Group includes or excludes</b><br> 1756 * Type: <b>token</b><br> 1757 * Path: <b>Group.characteristic.exclude</b><br> 1758 * </p> 1759 */ 1760 @SearchParamDefinition(name="exclude", path="Group.characteristic.exclude", description="Group includes or excludes", type="token" ) 1761 public static final String SP_EXCLUDE = "exclude"; 1762 /** 1763 * <b>Fluent Client</b> search parameter constant for <b>exclude</b> 1764 * <p> 1765 * Description: <b>Group includes or excludes</b><br> 1766 * Type: <b>token</b><br> 1767 * Path: <b>Group.characteristic.exclude</b><br> 1768 * </p> 1769 */ 1770 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXCLUDE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXCLUDE); 1771 1772 /** 1773 * Search parameter: <b>type</b> 1774 * <p> 1775 * Description: <b>The type of resources the group contains</b><br> 1776 * Type: <b>token</b><br> 1777 * Path: <b>Group.type</b><br> 1778 * </p> 1779 */ 1780 @SearchParamDefinition(name="type", path="Group.type", description="The type of resources the group contains", type="token" ) 1781 public static final String SP_TYPE = "type"; 1782 /** 1783 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1784 * <p> 1785 * Description: <b>The type of resources the group contains</b><br> 1786 * Type: <b>token</b><br> 1787 * Path: <b>Group.type</b><br> 1788 * </p> 1789 */ 1790 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1791 1792 /** 1793 * Search parameter: <b>value</b> 1794 * <p> 1795 * Description: <b>Value held by characteristic</b><br> 1796 * Type: <b>token</b><br> 1797 * Path: <b>Group.characteristic.value[x]</b><br> 1798 * </p> 1799 */ 1800 @SearchParamDefinition(name="value", path="Group.characteristic.value", description="Value held by characteristic", type="token" ) 1801 public static final String SP_VALUE = "value"; 1802 /** 1803 * <b>Fluent Client</b> search parameter constant for <b>value</b> 1804 * <p> 1805 * Description: <b>Value held by characteristic</b><br> 1806 * Type: <b>token</b><br> 1807 * Path: <b>Group.characteristic.value[x]</b><br> 1808 * </p> 1809 */ 1810 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VALUE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VALUE); 1811 1812 /** 1813 * Search parameter: <b>characteristic</b> 1814 * <p> 1815 * Description: <b>Kind of characteristic</b><br> 1816 * Type: <b>token</b><br> 1817 * Path: <b>Group.characteristic.code</b><br> 1818 * </p> 1819 */ 1820 @SearchParamDefinition(name="characteristic", path="Group.characteristic.code", description="Kind of characteristic", type="token" ) 1821 public static final String SP_CHARACTERISTIC = "characteristic"; 1822 /** 1823 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 1824 * <p> 1825 * Description: <b>Kind of characteristic</b><br> 1826 * Type: <b>token</b><br> 1827 * Path: <b>Group.characteristic.code</b><br> 1828 * </p> 1829 */ 1830 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHARACTERISTIC); 1831 1832 1833}