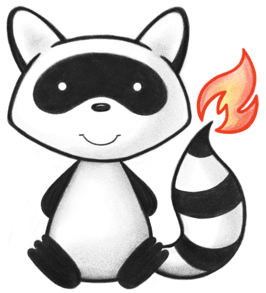
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * A guidance response is the formal response to a guidance request, including any output parameters returned by the evaluation, as well as the description of any proposed actions to be taken. 049 */ 050@ResourceDef(name="GuidanceResponse", profile="http://hl7.org/fhir/Profile/GuidanceResponse") 051public class GuidanceResponse extends DomainResource { 052 053 public enum GuidanceResponseStatus { 054 /** 055 * The request was processed successfully 056 */ 057 SUCCESS, 058 /** 059 * The request was processed successfully, but more data may result in a more complete evaluation 060 */ 061 DATAREQUESTED, 062 /** 063 * The request was processed, but more data is required to complete the evaluation 064 */ 065 DATAREQUIRED, 066 /** 067 * The request is currently being processed 068 */ 069 INPROGRESS, 070 /** 071 * The request was not processed successfully 072 */ 073 FAILURE, 074 /** 075 * The response was entered in error 076 */ 077 ENTEREDINERROR, 078 /** 079 * added to help the parsers with the generic types 080 */ 081 NULL; 082 public static GuidanceResponseStatus fromCode(String codeString) throws FHIRException { 083 if (codeString == null || "".equals(codeString)) 084 return null; 085 if ("success".equals(codeString)) 086 return SUCCESS; 087 if ("data-requested".equals(codeString)) 088 return DATAREQUESTED; 089 if ("data-required".equals(codeString)) 090 return DATAREQUIRED; 091 if ("in-progress".equals(codeString)) 092 return INPROGRESS; 093 if ("failure".equals(codeString)) 094 return FAILURE; 095 if ("entered-in-error".equals(codeString)) 096 return ENTEREDINERROR; 097 if (Configuration.isAcceptInvalidEnums()) 098 return null; 099 else 100 throw new FHIRException("Unknown GuidanceResponseStatus code '"+codeString+"'"); 101 } 102 public String toCode() { 103 switch (this) { 104 case SUCCESS: return "success"; 105 case DATAREQUESTED: return "data-requested"; 106 case DATAREQUIRED: return "data-required"; 107 case INPROGRESS: return "in-progress"; 108 case FAILURE: return "failure"; 109 case ENTEREDINERROR: return "entered-in-error"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getSystem() { 115 switch (this) { 116 case SUCCESS: return "http://hl7.org/fhir/guidance-response-status"; 117 case DATAREQUESTED: return "http://hl7.org/fhir/guidance-response-status"; 118 case DATAREQUIRED: return "http://hl7.org/fhir/guidance-response-status"; 119 case INPROGRESS: return "http://hl7.org/fhir/guidance-response-status"; 120 case FAILURE: return "http://hl7.org/fhir/guidance-response-status"; 121 case ENTEREDINERROR: return "http://hl7.org/fhir/guidance-response-status"; 122 case NULL: return null; 123 default: return "?"; 124 } 125 } 126 public String getDefinition() { 127 switch (this) { 128 case SUCCESS: return "The request was processed successfully"; 129 case DATAREQUESTED: return "The request was processed successfully, but more data may result in a more complete evaluation"; 130 case DATAREQUIRED: return "The request was processed, but more data is required to complete the evaluation"; 131 case INPROGRESS: return "The request is currently being processed"; 132 case FAILURE: return "The request was not processed successfully"; 133 case ENTEREDINERROR: return "The response was entered in error"; 134 case NULL: return null; 135 default: return "?"; 136 } 137 } 138 public String getDisplay() { 139 switch (this) { 140 case SUCCESS: return "Success"; 141 case DATAREQUESTED: return "Data Requested"; 142 case DATAREQUIRED: return "Data Required"; 143 case INPROGRESS: return "In Progress"; 144 case FAILURE: return "Failure"; 145 case ENTEREDINERROR: return "Entered In Error"; 146 case NULL: return null; 147 default: return "?"; 148 } 149 } 150 } 151 152 public static class GuidanceResponseStatusEnumFactory implements EnumFactory<GuidanceResponseStatus> { 153 public GuidanceResponseStatus fromCode(String codeString) throws IllegalArgumentException { 154 if (codeString == null || "".equals(codeString)) 155 if (codeString == null || "".equals(codeString)) 156 return null; 157 if ("success".equals(codeString)) 158 return GuidanceResponseStatus.SUCCESS; 159 if ("data-requested".equals(codeString)) 160 return GuidanceResponseStatus.DATAREQUESTED; 161 if ("data-required".equals(codeString)) 162 return GuidanceResponseStatus.DATAREQUIRED; 163 if ("in-progress".equals(codeString)) 164 return GuidanceResponseStatus.INPROGRESS; 165 if ("failure".equals(codeString)) 166 return GuidanceResponseStatus.FAILURE; 167 if ("entered-in-error".equals(codeString)) 168 return GuidanceResponseStatus.ENTEREDINERROR; 169 throw new IllegalArgumentException("Unknown GuidanceResponseStatus code '"+codeString+"'"); 170 } 171 public Enumeration<GuidanceResponseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 172 if (code == null) 173 return null; 174 if (code.isEmpty()) 175 return new Enumeration<GuidanceResponseStatus>(this); 176 String codeString = code.asStringValue(); 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("success".equals(codeString)) 180 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.SUCCESS); 181 if ("data-requested".equals(codeString)) 182 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.DATAREQUESTED); 183 if ("data-required".equals(codeString)) 184 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.DATAREQUIRED); 185 if ("in-progress".equals(codeString)) 186 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.INPROGRESS); 187 if ("failure".equals(codeString)) 188 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.FAILURE); 189 if ("entered-in-error".equals(codeString)) 190 return new Enumeration<GuidanceResponseStatus>(this, GuidanceResponseStatus.ENTEREDINERROR); 191 throw new FHIRException("Unknown GuidanceResponseStatus code '"+codeString+"'"); 192 } 193 public String toCode(GuidanceResponseStatus code) { 194 if (code == GuidanceResponseStatus.NULL) 195 return null; 196 if (code == GuidanceResponseStatus.SUCCESS) 197 return "success"; 198 if (code == GuidanceResponseStatus.DATAREQUESTED) 199 return "data-requested"; 200 if (code == GuidanceResponseStatus.DATAREQUIRED) 201 return "data-required"; 202 if (code == GuidanceResponseStatus.INPROGRESS) 203 return "in-progress"; 204 if (code == GuidanceResponseStatus.FAILURE) 205 return "failure"; 206 if (code == GuidanceResponseStatus.ENTEREDINERROR) 207 return "entered-in-error"; 208 return "?"; 209 } 210 public String toSystem(GuidanceResponseStatus code) { 211 return code.getSystem(); 212 } 213 } 214 215 /** 216 * The id of the request associated with this response. If an id was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario. 217 */ 218 @Child(name = "requestId", type = {IdType.class}, order=0, min=0, max=1, modifier=false, summary=true) 219 @Description(shortDefinition="The id of the request associated with this response, if any", formalDefinition="The id of the request associated with this response. If an id was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario." ) 220 protected IdType requestId; 221 222 /** 223 * Allows a service to provide a unique, business identifier for the response. 224 */ 225 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 226 @Description(shortDefinition="Business identifier", formalDefinition="Allows a service to provide a unique, business identifier for the response." ) 227 protected Identifier identifier; 228 229 /** 230 * A reference to the knowledge module that was invoked. 231 */ 232 @Child(name = "module", type = {ServiceDefinition.class}, order=2, min=1, max=1, modifier=false, summary=true) 233 @Description(shortDefinition="A reference to a knowledge module", formalDefinition="A reference to the knowledge module that was invoked." ) 234 protected Reference module; 235 236 /** 237 * The actual object that is the target of the reference (A reference to the knowledge module that was invoked.) 238 */ 239 protected ServiceDefinition moduleTarget; 240 241 /** 242 * The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information. 243 */ 244 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 245 @Description(shortDefinition="success | data-requested | data-required | in-progress | failure | entered-in-error", formalDefinition="The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information." ) 246 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/guidance-response-status") 247 protected Enumeration<GuidanceResponseStatus> status; 248 249 /** 250 * The patient for which the request was processed. 251 */ 252 @Child(name = "subject", type = {Patient.class, Group.class}, order=4, min=0, max=1, modifier=false, summary=false) 253 @Description(shortDefinition="Patient the request was performed for", formalDefinition="The patient for which the request was processed." ) 254 protected Reference subject; 255 256 /** 257 * The actual object that is the target of the reference (The patient for which the request was processed.) 258 */ 259 protected Resource subjectTarget; 260 261 /** 262 * Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable. 263 */ 264 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=5, min=0, max=1, modifier=false, summary=false) 265 @Description(shortDefinition="Encounter or Episode during which the response was returned", formalDefinition="Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable." ) 266 protected Reference context; 267 268 /** 269 * The actual object that is the target of the reference (Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable.) 270 */ 271 protected Resource contextTarget; 272 273 /** 274 * Indicates when the guidance response was processed. 275 */ 276 @Child(name = "occurrenceDateTime", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 277 @Description(shortDefinition="When the guidance response was processed", formalDefinition="Indicates when the guidance response was processed." ) 278 protected DateTimeType occurrenceDateTime; 279 280 /** 281 * Provides a reference to the device that performed the guidance. 282 */ 283 @Child(name = "performer", type = {Device.class}, order=7, min=0, max=1, modifier=false, summary=false) 284 @Description(shortDefinition="Device returning the guidance", formalDefinition="Provides a reference to the device that performed the guidance." ) 285 protected Reference performer; 286 287 /** 288 * The actual object that is the target of the reference (Provides a reference to the device that performed the guidance.) 289 */ 290 protected Device performerTarget; 291 292 /** 293 * Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response. 294 */ 295 @Child(name = "reason", type = {CodeableConcept.class, Reference.class}, order=8, min=0, max=1, modifier=false, summary=false) 296 @Description(shortDefinition="Reason for the response", formalDefinition="Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response." ) 297 protected Type reason; 298 299 /** 300 * Provides a mechanism to communicate additional information about the response. 301 */ 302 @Child(name = "note", type = {Annotation.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 303 @Description(shortDefinition="Additional notes about the response", formalDefinition="Provides a mechanism to communicate additional information about the response." ) 304 protected List<Annotation> note; 305 306 /** 307 * Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element. 308 */ 309 @Child(name = "evaluationMessage", type = {OperationOutcome.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 310 @Description(shortDefinition="Messages resulting from the evaluation of the artifact or artifacts", formalDefinition="Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element." ) 311 protected List<Reference> evaluationMessage; 312 /** 313 * The actual objects that are the target of the reference (Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.) 314 */ 315 protected List<OperationOutcome> evaluationMessageTarget; 316 317 318 /** 319 * The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element. 320 */ 321 @Child(name = "outputParameters", type = {Parameters.class}, order=11, min=0, max=1, modifier=false, summary=false) 322 @Description(shortDefinition="The output parameters of the evaluation, if any", formalDefinition="The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element." ) 323 protected Reference outputParameters; 324 325 /** 326 * The actual object that is the target of the reference (The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.) 327 */ 328 protected Parameters outputParametersTarget; 329 330 /** 331 * The actions, if any, produced by the evaluation of the artifact. 332 */ 333 @Child(name = "result", type = {CarePlan.class, RequestGroup.class}, order=12, min=0, max=1, modifier=false, summary=false) 334 @Description(shortDefinition="Proposed actions, if any", formalDefinition="The actions, if any, produced by the evaluation of the artifact." ) 335 protected Reference result; 336 337 /** 338 * The actual object that is the target of the reference (The actions, if any, produced by the evaluation of the artifact.) 339 */ 340 protected Resource resultTarget; 341 342 /** 343 * If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data. 344 */ 345 @Child(name = "dataRequirement", type = {DataRequirement.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 346 @Description(shortDefinition="Additional required data", formalDefinition="If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data." ) 347 protected List<DataRequirement> dataRequirement; 348 349 private static final long serialVersionUID = -2107029772L; 350 351 /** 352 * Constructor 353 */ 354 public GuidanceResponse() { 355 super(); 356 } 357 358 /** 359 * Constructor 360 */ 361 public GuidanceResponse(Reference module, Enumeration<GuidanceResponseStatus> status) { 362 super(); 363 this.module = module; 364 this.status = status; 365 } 366 367 /** 368 * @return {@link #requestId} (The id of the request associated with this response. If an id was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.). This is the underlying object with id, value and extensions. The accessor "getRequestId" gives direct access to the value 369 */ 370 public IdType getRequestIdElement() { 371 if (this.requestId == null) 372 if (Configuration.errorOnAutoCreate()) 373 throw new Error("Attempt to auto-create GuidanceResponse.requestId"); 374 else if (Configuration.doAutoCreate()) 375 this.requestId = new IdType(); // bb 376 return this.requestId; 377 } 378 379 public boolean hasRequestIdElement() { 380 return this.requestId != null && !this.requestId.isEmpty(); 381 } 382 383 public boolean hasRequestId() { 384 return this.requestId != null && !this.requestId.isEmpty(); 385 } 386 387 /** 388 * @param value {@link #requestId} (The id of the request associated with this response. If an id was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.). This is the underlying object with id, value and extensions. The accessor "getRequestId" gives direct access to the value 389 */ 390 public GuidanceResponse setRequestIdElement(IdType value) { 391 this.requestId = value; 392 return this; 393 } 394 395 /** 396 * @return The id of the request associated with this response. If an id was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario. 397 */ 398 public String getRequestId() { 399 return this.requestId == null ? null : this.requestId.getValue(); 400 } 401 402 /** 403 * @param value The id of the request associated with this response. If an id was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario. 404 */ 405 public GuidanceResponse setRequestId(String value) { 406 if (Utilities.noString(value)) 407 this.requestId = null; 408 else { 409 if (this.requestId == null) 410 this.requestId = new IdType(); 411 this.requestId.setValue(value); 412 } 413 return this; 414 } 415 416 /** 417 * @return {@link #identifier} (Allows a service to provide a unique, business identifier for the response.) 418 */ 419 public Identifier getIdentifier() { 420 if (this.identifier == null) 421 if (Configuration.errorOnAutoCreate()) 422 throw new Error("Attempt to auto-create GuidanceResponse.identifier"); 423 else if (Configuration.doAutoCreate()) 424 this.identifier = new Identifier(); // cc 425 return this.identifier; 426 } 427 428 public boolean hasIdentifier() { 429 return this.identifier != null && !this.identifier.isEmpty(); 430 } 431 432 /** 433 * @param value {@link #identifier} (Allows a service to provide a unique, business identifier for the response.) 434 */ 435 public GuidanceResponse setIdentifier(Identifier value) { 436 this.identifier = value; 437 return this; 438 } 439 440 /** 441 * @return {@link #module} (A reference to the knowledge module that was invoked.) 442 */ 443 public Reference getModule() { 444 if (this.module == null) 445 if (Configuration.errorOnAutoCreate()) 446 throw new Error("Attempt to auto-create GuidanceResponse.module"); 447 else if (Configuration.doAutoCreate()) 448 this.module = new Reference(); // cc 449 return this.module; 450 } 451 452 public boolean hasModule() { 453 return this.module != null && !this.module.isEmpty(); 454 } 455 456 /** 457 * @param value {@link #module} (A reference to the knowledge module that was invoked.) 458 */ 459 public GuidanceResponse setModule(Reference value) { 460 this.module = value; 461 return this; 462 } 463 464 /** 465 * @return {@link #module} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the knowledge module that was invoked.) 466 */ 467 public ServiceDefinition getModuleTarget() { 468 if (this.moduleTarget == null) 469 if (Configuration.errorOnAutoCreate()) 470 throw new Error("Attempt to auto-create GuidanceResponse.module"); 471 else if (Configuration.doAutoCreate()) 472 this.moduleTarget = new ServiceDefinition(); // aa 473 return this.moduleTarget; 474 } 475 476 /** 477 * @param value {@link #module} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the knowledge module that was invoked.) 478 */ 479 public GuidanceResponse setModuleTarget(ServiceDefinition value) { 480 this.moduleTarget = value; 481 return this; 482 } 483 484 /** 485 * @return {@link #status} (The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 486 */ 487 public Enumeration<GuidanceResponseStatus> getStatusElement() { 488 if (this.status == null) 489 if (Configuration.errorOnAutoCreate()) 490 throw new Error("Attempt to auto-create GuidanceResponse.status"); 491 else if (Configuration.doAutoCreate()) 492 this.status = new Enumeration<GuidanceResponseStatus>(new GuidanceResponseStatusEnumFactory()); // bb 493 return this.status; 494 } 495 496 public boolean hasStatusElement() { 497 return this.status != null && !this.status.isEmpty(); 498 } 499 500 public boolean hasStatus() { 501 return this.status != null && !this.status.isEmpty(); 502 } 503 504 /** 505 * @param value {@link #status} (The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 506 */ 507 public GuidanceResponse setStatusElement(Enumeration<GuidanceResponseStatus> value) { 508 this.status = value; 509 return this; 510 } 511 512 /** 513 * @return The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information. 514 */ 515 public GuidanceResponseStatus getStatus() { 516 return this.status == null ? null : this.status.getValue(); 517 } 518 519 /** 520 * @param value The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information. 521 */ 522 public GuidanceResponse setStatus(GuidanceResponseStatus value) { 523 if (this.status == null) 524 this.status = new Enumeration<GuidanceResponseStatus>(new GuidanceResponseStatusEnumFactory()); 525 this.status.setValue(value); 526 return this; 527 } 528 529 /** 530 * @return {@link #subject} (The patient for which the request was processed.) 531 */ 532 public Reference getSubject() { 533 if (this.subject == null) 534 if (Configuration.errorOnAutoCreate()) 535 throw new Error("Attempt to auto-create GuidanceResponse.subject"); 536 else if (Configuration.doAutoCreate()) 537 this.subject = new Reference(); // cc 538 return this.subject; 539 } 540 541 public boolean hasSubject() { 542 return this.subject != null && !this.subject.isEmpty(); 543 } 544 545 /** 546 * @param value {@link #subject} (The patient for which the request was processed.) 547 */ 548 public GuidanceResponse setSubject(Reference value) { 549 this.subject = value; 550 return this; 551 } 552 553 /** 554 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient for which the request was processed.) 555 */ 556 public Resource getSubjectTarget() { 557 return this.subjectTarget; 558 } 559 560 /** 561 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient for which the request was processed.) 562 */ 563 public GuidanceResponse setSubjectTarget(Resource value) { 564 this.subjectTarget = value; 565 return this; 566 } 567 568 /** 569 * @return {@link #context} (Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable.) 570 */ 571 public Reference getContext() { 572 if (this.context == null) 573 if (Configuration.errorOnAutoCreate()) 574 throw new Error("Attempt to auto-create GuidanceResponse.context"); 575 else if (Configuration.doAutoCreate()) 576 this.context = new Reference(); // cc 577 return this.context; 578 } 579 580 public boolean hasContext() { 581 return this.context != null && !this.context.isEmpty(); 582 } 583 584 /** 585 * @param value {@link #context} (Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable.) 586 */ 587 public GuidanceResponse setContext(Reference value) { 588 this.context = value; 589 return this; 590 } 591 592 /** 593 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable.) 594 */ 595 public Resource getContextTarget() { 596 return this.contextTarget; 597 } 598 599 /** 600 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable.) 601 */ 602 public GuidanceResponse setContextTarget(Resource value) { 603 this.contextTarget = value; 604 return this; 605 } 606 607 /** 608 * @return {@link #occurrenceDateTime} (Indicates when the guidance response was processed.). This is the underlying object with id, value and extensions. The accessor "getOccurrenceDateTime" gives direct access to the value 609 */ 610 public DateTimeType getOccurrenceDateTimeElement() { 611 if (this.occurrenceDateTime == null) 612 if (Configuration.errorOnAutoCreate()) 613 throw new Error("Attempt to auto-create GuidanceResponse.occurrenceDateTime"); 614 else if (Configuration.doAutoCreate()) 615 this.occurrenceDateTime = new DateTimeType(); // bb 616 return this.occurrenceDateTime; 617 } 618 619 public boolean hasOccurrenceDateTimeElement() { 620 return this.occurrenceDateTime != null && !this.occurrenceDateTime.isEmpty(); 621 } 622 623 public boolean hasOccurrenceDateTime() { 624 return this.occurrenceDateTime != null && !this.occurrenceDateTime.isEmpty(); 625 } 626 627 /** 628 * @param value {@link #occurrenceDateTime} (Indicates when the guidance response was processed.). This is the underlying object with id, value and extensions. The accessor "getOccurrenceDateTime" gives direct access to the value 629 */ 630 public GuidanceResponse setOccurrenceDateTimeElement(DateTimeType value) { 631 this.occurrenceDateTime = value; 632 return this; 633 } 634 635 /** 636 * @return Indicates when the guidance response was processed. 637 */ 638 public Date getOccurrenceDateTime() { 639 return this.occurrenceDateTime == null ? null : this.occurrenceDateTime.getValue(); 640 } 641 642 /** 643 * @param value Indicates when the guidance response was processed. 644 */ 645 public GuidanceResponse setOccurrenceDateTime(Date value) { 646 if (value == null) 647 this.occurrenceDateTime = null; 648 else { 649 if (this.occurrenceDateTime == null) 650 this.occurrenceDateTime = new DateTimeType(); 651 this.occurrenceDateTime.setValue(value); 652 } 653 return this; 654 } 655 656 /** 657 * @return {@link #performer} (Provides a reference to the device that performed the guidance.) 658 */ 659 public Reference getPerformer() { 660 if (this.performer == null) 661 if (Configuration.errorOnAutoCreate()) 662 throw new Error("Attempt to auto-create GuidanceResponse.performer"); 663 else if (Configuration.doAutoCreate()) 664 this.performer = new Reference(); // cc 665 return this.performer; 666 } 667 668 public boolean hasPerformer() { 669 return this.performer != null && !this.performer.isEmpty(); 670 } 671 672 /** 673 * @param value {@link #performer} (Provides a reference to the device that performed the guidance.) 674 */ 675 public GuidanceResponse setPerformer(Reference value) { 676 this.performer = value; 677 return this; 678 } 679 680 /** 681 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Provides a reference to the device that performed the guidance.) 682 */ 683 public Device getPerformerTarget() { 684 if (this.performerTarget == null) 685 if (Configuration.errorOnAutoCreate()) 686 throw new Error("Attempt to auto-create GuidanceResponse.performer"); 687 else if (Configuration.doAutoCreate()) 688 this.performerTarget = new Device(); // aa 689 return this.performerTarget; 690 } 691 692 /** 693 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Provides a reference to the device that performed the guidance.) 694 */ 695 public GuidanceResponse setPerformerTarget(Device value) { 696 this.performerTarget = value; 697 return this; 698 } 699 700 /** 701 * @return {@link #reason} (Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 702 */ 703 public Type getReason() { 704 return this.reason; 705 } 706 707 /** 708 * @return {@link #reason} (Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 709 */ 710 public CodeableConcept getReasonCodeableConcept() throws FHIRException { 711 if (this.reason == null) 712 return null; 713 if (!(this.reason instanceof CodeableConcept)) 714 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.reason.getClass().getName()+" was encountered"); 715 return (CodeableConcept) this.reason; 716 } 717 718 public boolean hasReasonCodeableConcept() { 719 return this != null && this.reason instanceof CodeableConcept; 720 } 721 722 /** 723 * @return {@link #reason} (Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 724 */ 725 public Reference getReasonReference() throws FHIRException { 726 if (this.reason == null) 727 return null; 728 if (!(this.reason instanceof Reference)) 729 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.reason.getClass().getName()+" was encountered"); 730 return (Reference) this.reason; 731 } 732 733 public boolean hasReasonReference() { 734 return this != null && this.reason instanceof Reference; 735 } 736 737 public boolean hasReason() { 738 return this.reason != null && !this.reason.isEmpty(); 739 } 740 741 /** 742 * @param value {@link #reason} (Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.) 743 */ 744 public GuidanceResponse setReason(Type value) throws FHIRFormatError { 745 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 746 throw new FHIRFormatError("Not the right type for GuidanceResponse.reason[x]: "+value.fhirType()); 747 this.reason = value; 748 return this; 749 } 750 751 /** 752 * @return {@link #note} (Provides a mechanism to communicate additional information about the response.) 753 */ 754 public List<Annotation> getNote() { 755 if (this.note == null) 756 this.note = new ArrayList<Annotation>(); 757 return this.note; 758 } 759 760 /** 761 * @return Returns a reference to <code>this</code> for easy method chaining 762 */ 763 public GuidanceResponse setNote(List<Annotation> theNote) { 764 this.note = theNote; 765 return this; 766 } 767 768 public boolean hasNote() { 769 if (this.note == null) 770 return false; 771 for (Annotation item : this.note) 772 if (!item.isEmpty()) 773 return true; 774 return false; 775 } 776 777 public Annotation addNote() { //3 778 Annotation t = new Annotation(); 779 if (this.note == null) 780 this.note = new ArrayList<Annotation>(); 781 this.note.add(t); 782 return t; 783 } 784 785 public GuidanceResponse addNote(Annotation t) { //3 786 if (t == null) 787 return this; 788 if (this.note == null) 789 this.note = new ArrayList<Annotation>(); 790 this.note.add(t); 791 return this; 792 } 793 794 /** 795 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 796 */ 797 public Annotation getNoteFirstRep() { 798 if (getNote().isEmpty()) { 799 addNote(); 800 } 801 return getNote().get(0); 802 } 803 804 /** 805 * @return {@link #evaluationMessage} (Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.) 806 */ 807 public List<Reference> getEvaluationMessage() { 808 if (this.evaluationMessage == null) 809 this.evaluationMessage = new ArrayList<Reference>(); 810 return this.evaluationMessage; 811 } 812 813 /** 814 * @return Returns a reference to <code>this</code> for easy method chaining 815 */ 816 public GuidanceResponse setEvaluationMessage(List<Reference> theEvaluationMessage) { 817 this.evaluationMessage = theEvaluationMessage; 818 return this; 819 } 820 821 public boolean hasEvaluationMessage() { 822 if (this.evaluationMessage == null) 823 return false; 824 for (Reference item : this.evaluationMessage) 825 if (!item.isEmpty()) 826 return true; 827 return false; 828 } 829 830 public Reference addEvaluationMessage() { //3 831 Reference t = new Reference(); 832 if (this.evaluationMessage == null) 833 this.evaluationMessage = new ArrayList<Reference>(); 834 this.evaluationMessage.add(t); 835 return t; 836 } 837 838 public GuidanceResponse addEvaluationMessage(Reference t) { //3 839 if (t == null) 840 return this; 841 if (this.evaluationMessage == null) 842 this.evaluationMessage = new ArrayList<Reference>(); 843 this.evaluationMessage.add(t); 844 return this; 845 } 846 847 /** 848 * @return The first repetition of repeating field {@link #evaluationMessage}, creating it if it does not already exist 849 */ 850 public Reference getEvaluationMessageFirstRep() { 851 if (getEvaluationMessage().isEmpty()) { 852 addEvaluationMessage(); 853 } 854 return getEvaluationMessage().get(0); 855 } 856 857 /** 858 * @deprecated Use Reference#setResource(IBaseResource) instead 859 */ 860 @Deprecated 861 public List<OperationOutcome> getEvaluationMessageTarget() { 862 if (this.evaluationMessageTarget == null) 863 this.evaluationMessageTarget = new ArrayList<OperationOutcome>(); 864 return this.evaluationMessageTarget; 865 } 866 867 /** 868 * @deprecated Use Reference#setResource(IBaseResource) instead 869 */ 870 @Deprecated 871 public OperationOutcome addEvaluationMessageTarget() { 872 OperationOutcome r = new OperationOutcome(); 873 if (this.evaluationMessageTarget == null) 874 this.evaluationMessageTarget = new ArrayList<OperationOutcome>(); 875 this.evaluationMessageTarget.add(r); 876 return r; 877 } 878 879 /** 880 * @return {@link #outputParameters} (The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.) 881 */ 882 public Reference getOutputParameters() { 883 if (this.outputParameters == null) 884 if (Configuration.errorOnAutoCreate()) 885 throw new Error("Attempt to auto-create GuidanceResponse.outputParameters"); 886 else if (Configuration.doAutoCreate()) 887 this.outputParameters = new Reference(); // cc 888 return this.outputParameters; 889 } 890 891 public boolean hasOutputParameters() { 892 return this.outputParameters != null && !this.outputParameters.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #outputParameters} (The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.) 897 */ 898 public GuidanceResponse setOutputParameters(Reference value) { 899 this.outputParameters = value; 900 return this; 901 } 902 903 /** 904 * @return {@link #outputParameters} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.) 905 */ 906 public Parameters getOutputParametersTarget() { 907 if (this.outputParametersTarget == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create GuidanceResponse.outputParameters"); 910 else if (Configuration.doAutoCreate()) 911 this.outputParametersTarget = new Parameters(); // aa 912 return this.outputParametersTarget; 913 } 914 915 /** 916 * @param value {@link #outputParameters} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.) 917 */ 918 public GuidanceResponse setOutputParametersTarget(Parameters value) { 919 this.outputParametersTarget = value; 920 return this; 921 } 922 923 /** 924 * @return {@link #result} (The actions, if any, produced by the evaluation of the artifact.) 925 */ 926 public Reference getResult() { 927 if (this.result == null) 928 if (Configuration.errorOnAutoCreate()) 929 throw new Error("Attempt to auto-create GuidanceResponse.result"); 930 else if (Configuration.doAutoCreate()) 931 this.result = new Reference(); // cc 932 return this.result; 933 } 934 935 public boolean hasResult() { 936 return this.result != null && !this.result.isEmpty(); 937 } 938 939 /** 940 * @param value {@link #result} (The actions, if any, produced by the evaluation of the artifact.) 941 */ 942 public GuidanceResponse setResult(Reference value) { 943 this.result = value; 944 return this; 945 } 946 947 /** 948 * @return {@link #result} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The actions, if any, produced by the evaluation of the artifact.) 949 */ 950 public Resource getResultTarget() { 951 return this.resultTarget; 952 } 953 954 /** 955 * @param value {@link #result} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The actions, if any, produced by the evaluation of the artifact.) 956 */ 957 public GuidanceResponse setResultTarget(Resource value) { 958 this.resultTarget = value; 959 return this; 960 } 961 962 /** 963 * @return {@link #dataRequirement} (If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.) 964 */ 965 public List<DataRequirement> getDataRequirement() { 966 if (this.dataRequirement == null) 967 this.dataRequirement = new ArrayList<DataRequirement>(); 968 return this.dataRequirement; 969 } 970 971 /** 972 * @return Returns a reference to <code>this</code> for easy method chaining 973 */ 974 public GuidanceResponse setDataRequirement(List<DataRequirement> theDataRequirement) { 975 this.dataRequirement = theDataRequirement; 976 return this; 977 } 978 979 public boolean hasDataRequirement() { 980 if (this.dataRequirement == null) 981 return false; 982 for (DataRequirement item : this.dataRequirement) 983 if (!item.isEmpty()) 984 return true; 985 return false; 986 } 987 988 public DataRequirement addDataRequirement() { //3 989 DataRequirement t = new DataRequirement(); 990 if (this.dataRequirement == null) 991 this.dataRequirement = new ArrayList<DataRequirement>(); 992 this.dataRequirement.add(t); 993 return t; 994 } 995 996 public GuidanceResponse addDataRequirement(DataRequirement t) { //3 997 if (t == null) 998 return this; 999 if (this.dataRequirement == null) 1000 this.dataRequirement = new ArrayList<DataRequirement>(); 1001 this.dataRequirement.add(t); 1002 return this; 1003 } 1004 1005 /** 1006 * @return The first repetition of repeating field {@link #dataRequirement}, creating it if it does not already exist 1007 */ 1008 public DataRequirement getDataRequirementFirstRep() { 1009 if (getDataRequirement().isEmpty()) { 1010 addDataRequirement(); 1011 } 1012 return getDataRequirement().get(0); 1013 } 1014 1015 protected void listChildren(List<Property> children) { 1016 super.listChildren(children); 1017 children.add(new Property("requestId", "id", "The id of the request associated with this response. If an id was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.", 0, 1, requestId)); 1018 children.add(new Property("identifier", "Identifier", "Allows a service to provide a unique, business identifier for the response.", 0, 1, identifier)); 1019 children.add(new Property("module", "Reference(ServiceDefinition)", "A reference to the knowledge module that was invoked.", 0, 1, module)); 1020 children.add(new Property("status", "code", "The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.", 0, 1, status)); 1021 children.add(new Property("subject", "Reference(Patient|Group)", "The patient for which the request was processed.", 0, 1, subject)); 1022 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable.", 0, 1, context)); 1023 children.add(new Property("occurrenceDateTime", "dateTime", "Indicates when the guidance response was processed.", 0, 1, occurrenceDateTime)); 1024 children.add(new Property("performer", "Reference(Device)", "Provides a reference to the device that performed the guidance.", 0, 1, performer)); 1025 children.add(new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason)); 1026 children.add(new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note)); 1027 children.add(new Property("evaluationMessage", "Reference(OperationOutcome)", "Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.", 0, java.lang.Integer.MAX_VALUE, evaluationMessage)); 1028 children.add(new Property("outputParameters", "Reference(Parameters)", "The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.", 0, 1, outputParameters)); 1029 children.add(new Property("result", "Reference(CarePlan|RequestGroup)", "The actions, if any, produced by the evaluation of the artifact.", 0, 1, result)); 1030 children.add(new Property("dataRequirement", "DataRequirement", "If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.", 0, java.lang.Integer.MAX_VALUE, dataRequirement)); 1031 } 1032 1033 @Override 1034 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1035 switch (_hash) { 1036 case 693933066: /*requestId*/ return new Property("requestId", "id", "The id of the request associated with this response. If an id was given as part of the request, it will be reproduced here to enable the requester to more easily identify the response in a multi-request scenario.", 0, 1, requestId); 1037 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Allows a service to provide a unique, business identifier for the response.", 0, 1, identifier); 1038 case -1068784020: /*module*/ return new Property("module", "Reference(ServiceDefinition)", "A reference to the knowledge module that was invoked.", 0, 1, module); 1039 case -892481550: /*status*/ return new Property("status", "code", "The status of the response. If the evaluation is completed successfully, the status will indicate success. However, in order to complete the evaluation, the engine may require more information. In this case, the status will be data-required, and the response will contain a description of the additional required information. If the evaluation completed successfully, but the engine determines that a potentially more accurate response could be provided if more data was available, the status will be data-requested, and the response will contain a description of the additional requested information.", 0, 1, status); 1040 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The patient for which the request was processed.", 0, 1, subject); 1041 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "Allows the context of the guidance response to be provided if available. In a service context, this would likely be unavailable.", 0, 1, context); 1042 case -298443636: /*occurrenceDateTime*/ return new Property("occurrenceDateTime", "dateTime", "Indicates when the guidance response was processed.", 0, 1, occurrenceDateTime); 1043 case 481140686: /*performer*/ return new Property("performer", "Reference(Device)", "Provides a reference to the device that performed the guidance.", 0, 1, performer); 1044 case -669418564: /*reason[x]*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 1045 case -934964668: /*reason*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 1046 case -610155331: /*reasonCodeableConcept*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 1047 case -1146218137: /*reasonReference*/ return new Property("reason[x]", "CodeableConcept|Reference(Any)", "Indicates the reason the request was initiated. This is typically provided as a parameter to the evaluation and echoed by the service, although for some use cases, such as subscription- or event-based scenarios, it may provide an indication of the cause for the response.", 0, 1, reason); 1048 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides a mechanism to communicate additional information about the response.", 0, java.lang.Integer.MAX_VALUE, note); 1049 case 1081619755: /*evaluationMessage*/ return new Property("evaluationMessage", "Reference(OperationOutcome)", "Messages resulting from the evaluation of the artifact or artifacts. As part of evaluating the request, the engine may produce informational or warning messages. These messages will be provided by this element.", 0, java.lang.Integer.MAX_VALUE, evaluationMessage); 1050 case 525609419: /*outputParameters*/ return new Property("outputParameters", "Reference(Parameters)", "The output parameters of the evaluation, if any. Many modules will result in the return of specific resources such as procedure or communication requests that are returned as part of the operation result. However, modules may define specific outputs that would be returned as the result of the evaluation, and these would be returned in this element.", 0, 1, outputParameters); 1051 case -934426595: /*result*/ return new Property("result", "Reference(CarePlan|RequestGroup)", "The actions, if any, produced by the evaluation of the artifact.", 0, 1, result); 1052 case 629147193: /*dataRequirement*/ return new Property("dataRequirement", "DataRequirement", "If the evaluation could not be completed due to lack of information, or additional information would potentially result in a more accurate response, this element will a description of the data required in order to proceed with the evaluation. A subsequent request to the service should include this data.", 0, java.lang.Integer.MAX_VALUE, dataRequirement); 1053 default: return super.getNamedProperty(_hash, _name, _checkValid); 1054 } 1055 1056 } 1057 1058 @Override 1059 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1060 switch (hash) { 1061 case 693933066: /*requestId*/ return this.requestId == null ? new Base[0] : new Base[] {this.requestId}; // IdType 1062 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1063 case -1068784020: /*module*/ return this.module == null ? new Base[0] : new Base[] {this.module}; // Reference 1064 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<GuidanceResponseStatus> 1065 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1066 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1067 case -298443636: /*occurrenceDateTime*/ return this.occurrenceDateTime == null ? new Base[0] : new Base[] {this.occurrenceDateTime}; // DateTimeType 1068 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 1069 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // Type 1070 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1071 case 1081619755: /*evaluationMessage*/ return this.evaluationMessage == null ? new Base[0] : this.evaluationMessage.toArray(new Base[this.evaluationMessage.size()]); // Reference 1072 case 525609419: /*outputParameters*/ return this.outputParameters == null ? new Base[0] : new Base[] {this.outputParameters}; // Reference 1073 case -934426595: /*result*/ return this.result == null ? new Base[0] : new Base[] {this.result}; // Reference 1074 case 629147193: /*dataRequirement*/ return this.dataRequirement == null ? new Base[0] : this.dataRequirement.toArray(new Base[this.dataRequirement.size()]); // DataRequirement 1075 default: return super.getProperty(hash, name, checkValid); 1076 } 1077 1078 } 1079 1080 @Override 1081 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1082 switch (hash) { 1083 case 693933066: // requestId 1084 this.requestId = castToId(value); // IdType 1085 return value; 1086 case -1618432855: // identifier 1087 this.identifier = castToIdentifier(value); // Identifier 1088 return value; 1089 case -1068784020: // module 1090 this.module = castToReference(value); // Reference 1091 return value; 1092 case -892481550: // status 1093 value = new GuidanceResponseStatusEnumFactory().fromType(castToCode(value)); 1094 this.status = (Enumeration) value; // Enumeration<GuidanceResponseStatus> 1095 return value; 1096 case -1867885268: // subject 1097 this.subject = castToReference(value); // Reference 1098 return value; 1099 case 951530927: // context 1100 this.context = castToReference(value); // Reference 1101 return value; 1102 case -298443636: // occurrenceDateTime 1103 this.occurrenceDateTime = castToDateTime(value); // DateTimeType 1104 return value; 1105 case 481140686: // performer 1106 this.performer = castToReference(value); // Reference 1107 return value; 1108 case -934964668: // reason 1109 this.reason = castToType(value); // Type 1110 return value; 1111 case 3387378: // note 1112 this.getNote().add(castToAnnotation(value)); // Annotation 1113 return value; 1114 case 1081619755: // evaluationMessage 1115 this.getEvaluationMessage().add(castToReference(value)); // Reference 1116 return value; 1117 case 525609419: // outputParameters 1118 this.outputParameters = castToReference(value); // Reference 1119 return value; 1120 case -934426595: // result 1121 this.result = castToReference(value); // Reference 1122 return value; 1123 case 629147193: // dataRequirement 1124 this.getDataRequirement().add(castToDataRequirement(value)); // DataRequirement 1125 return value; 1126 default: return super.setProperty(hash, name, value); 1127 } 1128 1129 } 1130 1131 @Override 1132 public Base setProperty(String name, Base value) throws FHIRException { 1133 if (name.equals("requestId")) { 1134 this.requestId = castToId(value); // IdType 1135 } else if (name.equals("identifier")) { 1136 this.identifier = castToIdentifier(value); // Identifier 1137 } else if (name.equals("module")) { 1138 this.module = castToReference(value); // Reference 1139 } else if (name.equals("status")) { 1140 value = new GuidanceResponseStatusEnumFactory().fromType(castToCode(value)); 1141 this.status = (Enumeration) value; // Enumeration<GuidanceResponseStatus> 1142 } else if (name.equals("subject")) { 1143 this.subject = castToReference(value); // Reference 1144 } else if (name.equals("context")) { 1145 this.context = castToReference(value); // Reference 1146 } else if (name.equals("occurrenceDateTime")) { 1147 this.occurrenceDateTime = castToDateTime(value); // DateTimeType 1148 } else if (name.equals("performer")) { 1149 this.performer = castToReference(value); // Reference 1150 } else if (name.equals("reason[x]")) { 1151 this.reason = castToType(value); // Type 1152 } else if (name.equals("note")) { 1153 this.getNote().add(castToAnnotation(value)); 1154 } else if (name.equals("evaluationMessage")) { 1155 this.getEvaluationMessage().add(castToReference(value)); 1156 } else if (name.equals("outputParameters")) { 1157 this.outputParameters = castToReference(value); // Reference 1158 } else if (name.equals("result")) { 1159 this.result = castToReference(value); // Reference 1160 } else if (name.equals("dataRequirement")) { 1161 this.getDataRequirement().add(castToDataRequirement(value)); 1162 } else 1163 return super.setProperty(name, value); 1164 return value; 1165 } 1166 1167 @Override 1168 public Base makeProperty(int hash, String name) throws FHIRException { 1169 switch (hash) { 1170 case 693933066: return getRequestIdElement(); 1171 case -1618432855: return getIdentifier(); 1172 case -1068784020: return getModule(); 1173 case -892481550: return getStatusElement(); 1174 case -1867885268: return getSubject(); 1175 case 951530927: return getContext(); 1176 case -298443636: return getOccurrenceDateTimeElement(); 1177 case 481140686: return getPerformer(); 1178 case -669418564: return getReason(); 1179 case -934964668: return getReason(); 1180 case 3387378: return addNote(); 1181 case 1081619755: return addEvaluationMessage(); 1182 case 525609419: return getOutputParameters(); 1183 case -934426595: return getResult(); 1184 case 629147193: return addDataRequirement(); 1185 default: return super.makeProperty(hash, name); 1186 } 1187 1188 } 1189 1190 @Override 1191 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1192 switch (hash) { 1193 case 693933066: /*requestId*/ return new String[] {"id"}; 1194 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1195 case -1068784020: /*module*/ return new String[] {"Reference"}; 1196 case -892481550: /*status*/ return new String[] {"code"}; 1197 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1198 case 951530927: /*context*/ return new String[] {"Reference"}; 1199 case -298443636: /*occurrenceDateTime*/ return new String[] {"dateTime"}; 1200 case 481140686: /*performer*/ return new String[] {"Reference"}; 1201 case -934964668: /*reason*/ return new String[] {"CodeableConcept", "Reference"}; 1202 case 3387378: /*note*/ return new String[] {"Annotation"}; 1203 case 1081619755: /*evaluationMessage*/ return new String[] {"Reference"}; 1204 case 525609419: /*outputParameters*/ return new String[] {"Reference"}; 1205 case -934426595: /*result*/ return new String[] {"Reference"}; 1206 case 629147193: /*dataRequirement*/ return new String[] {"DataRequirement"}; 1207 default: return super.getTypesForProperty(hash, name); 1208 } 1209 1210 } 1211 1212 @Override 1213 public Base addChild(String name) throws FHIRException { 1214 if (name.equals("requestId")) { 1215 throw new FHIRException("Cannot call addChild on a singleton property GuidanceResponse.requestId"); 1216 } 1217 else if (name.equals("identifier")) { 1218 this.identifier = new Identifier(); 1219 return this.identifier; 1220 } 1221 else if (name.equals("module")) { 1222 this.module = new Reference(); 1223 return this.module; 1224 } 1225 else if (name.equals("status")) { 1226 throw new FHIRException("Cannot call addChild on a singleton property GuidanceResponse.status"); 1227 } 1228 else if (name.equals("subject")) { 1229 this.subject = new Reference(); 1230 return this.subject; 1231 } 1232 else if (name.equals("context")) { 1233 this.context = new Reference(); 1234 return this.context; 1235 } 1236 else if (name.equals("occurrenceDateTime")) { 1237 throw new FHIRException("Cannot call addChild on a singleton property GuidanceResponse.occurrenceDateTime"); 1238 } 1239 else if (name.equals("performer")) { 1240 this.performer = new Reference(); 1241 return this.performer; 1242 } 1243 else if (name.equals("reasonCodeableConcept")) { 1244 this.reason = new CodeableConcept(); 1245 return this.reason; 1246 } 1247 else if (name.equals("reasonReference")) { 1248 this.reason = new Reference(); 1249 return this.reason; 1250 } 1251 else if (name.equals("note")) { 1252 return addNote(); 1253 } 1254 else if (name.equals("evaluationMessage")) { 1255 return addEvaluationMessage(); 1256 } 1257 else if (name.equals("outputParameters")) { 1258 this.outputParameters = new Reference(); 1259 return this.outputParameters; 1260 } 1261 else if (name.equals("result")) { 1262 this.result = new Reference(); 1263 return this.result; 1264 } 1265 else if (name.equals("dataRequirement")) { 1266 return addDataRequirement(); 1267 } 1268 else 1269 return super.addChild(name); 1270 } 1271 1272 public String fhirType() { 1273 return "GuidanceResponse"; 1274 1275 } 1276 1277 public GuidanceResponse copy() { 1278 GuidanceResponse dst = new GuidanceResponse(); 1279 copyValues(dst); 1280 dst.requestId = requestId == null ? null : requestId.copy(); 1281 dst.identifier = identifier == null ? null : identifier.copy(); 1282 dst.module = module == null ? null : module.copy(); 1283 dst.status = status == null ? null : status.copy(); 1284 dst.subject = subject == null ? null : subject.copy(); 1285 dst.context = context == null ? null : context.copy(); 1286 dst.occurrenceDateTime = occurrenceDateTime == null ? null : occurrenceDateTime.copy(); 1287 dst.performer = performer == null ? null : performer.copy(); 1288 dst.reason = reason == null ? null : reason.copy(); 1289 if (note != null) { 1290 dst.note = new ArrayList<Annotation>(); 1291 for (Annotation i : note) 1292 dst.note.add(i.copy()); 1293 }; 1294 if (evaluationMessage != null) { 1295 dst.evaluationMessage = new ArrayList<Reference>(); 1296 for (Reference i : evaluationMessage) 1297 dst.evaluationMessage.add(i.copy()); 1298 }; 1299 dst.outputParameters = outputParameters == null ? null : outputParameters.copy(); 1300 dst.result = result == null ? null : result.copy(); 1301 if (dataRequirement != null) { 1302 dst.dataRequirement = new ArrayList<DataRequirement>(); 1303 for (DataRequirement i : dataRequirement) 1304 dst.dataRequirement.add(i.copy()); 1305 }; 1306 return dst; 1307 } 1308 1309 protected GuidanceResponse typedCopy() { 1310 return copy(); 1311 } 1312 1313 @Override 1314 public boolean equalsDeep(Base other_) { 1315 if (!super.equalsDeep(other_)) 1316 return false; 1317 if (!(other_ instanceof GuidanceResponse)) 1318 return false; 1319 GuidanceResponse o = (GuidanceResponse) other_; 1320 return compareDeep(requestId, o.requestId, true) && compareDeep(identifier, o.identifier, true) 1321 && compareDeep(module, o.module, true) && compareDeep(status, o.status, true) && compareDeep(subject, o.subject, true) 1322 && compareDeep(context, o.context, true) && compareDeep(occurrenceDateTime, o.occurrenceDateTime, true) 1323 && compareDeep(performer, o.performer, true) && compareDeep(reason, o.reason, true) && compareDeep(note, o.note, true) 1324 && compareDeep(evaluationMessage, o.evaluationMessage, true) && compareDeep(outputParameters, o.outputParameters, true) 1325 && compareDeep(result, o.result, true) && compareDeep(dataRequirement, o.dataRequirement, true) 1326 ; 1327 } 1328 1329 @Override 1330 public boolean equalsShallow(Base other_) { 1331 if (!super.equalsShallow(other_)) 1332 return false; 1333 if (!(other_ instanceof GuidanceResponse)) 1334 return false; 1335 GuidanceResponse o = (GuidanceResponse) other_; 1336 return compareValues(requestId, o.requestId, true) && compareValues(status, o.status, true) && compareValues(occurrenceDateTime, o.occurrenceDateTime, true) 1337 ; 1338 } 1339 1340 public boolean isEmpty() { 1341 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(requestId, identifier, module 1342 , status, subject, context, occurrenceDateTime, performer, reason, note, evaluationMessage 1343 , outputParameters, result, dataRequirement); 1344 } 1345 1346 @Override 1347 public ResourceType getResourceType() { 1348 return ResourceType.GuidanceResponse; 1349 } 1350 1351 /** 1352 * Search parameter: <b>request</b> 1353 * <p> 1354 * Description: <b>The identifier of the request associated with the response</b><br> 1355 * Type: <b>token</b><br> 1356 * Path: <b>GuidanceResponse.requestId</b><br> 1357 * </p> 1358 */ 1359 @SearchParamDefinition(name="request", path="GuidanceResponse.requestId", description="The identifier of the request associated with the response", type="token" ) 1360 public static final String SP_REQUEST = "request"; 1361 /** 1362 * <b>Fluent Client</b> search parameter constant for <b>request</b> 1363 * <p> 1364 * Description: <b>The identifier of the request associated with the response</b><br> 1365 * Type: <b>token</b><br> 1366 * Path: <b>GuidanceResponse.requestId</b><br> 1367 * </p> 1368 */ 1369 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REQUEST = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REQUEST); 1370 1371 /** 1372 * Search parameter: <b>identifier</b> 1373 * <p> 1374 * Description: <b>The identifier of the guidance response</b><br> 1375 * Type: <b>token</b><br> 1376 * Path: <b>GuidanceResponse.identifier</b><br> 1377 * </p> 1378 */ 1379 @SearchParamDefinition(name="identifier", path="GuidanceResponse.identifier", description="The identifier of the guidance response", type="token" ) 1380 public static final String SP_IDENTIFIER = "identifier"; 1381 /** 1382 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1383 * <p> 1384 * Description: <b>The identifier of the guidance response</b><br> 1385 * Type: <b>token</b><br> 1386 * Path: <b>GuidanceResponse.identifier</b><br> 1387 * </p> 1388 */ 1389 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1390 1391 /** 1392 * Search parameter: <b>patient</b> 1393 * <p> 1394 * Description: <b>The identity of a patient to search for guidance response results</b><br> 1395 * Type: <b>reference</b><br> 1396 * Path: <b>GuidanceResponse.subject</b><br> 1397 * </p> 1398 */ 1399 @SearchParamDefinition(name="patient", path="GuidanceResponse.subject", description="The identity of a patient to search for guidance response results", type="reference", target={Patient.class } ) 1400 public static final String SP_PATIENT = "patient"; 1401 /** 1402 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1403 * <p> 1404 * Description: <b>The identity of a patient to search for guidance response results</b><br> 1405 * Type: <b>reference</b><br> 1406 * Path: <b>GuidanceResponse.subject</b><br> 1407 * </p> 1408 */ 1409 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1410 1411/** 1412 * Constant for fluent queries to be used to add include statements. Specifies 1413 * the path value of "<b>GuidanceResponse:patient</b>". 1414 */ 1415 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("GuidanceResponse:patient").toLocked(); 1416 1417 /** 1418 * Search parameter: <b>subject</b> 1419 * <p> 1420 * Description: <b>The subject that the guidance response is about</b><br> 1421 * Type: <b>reference</b><br> 1422 * Path: <b>GuidanceResponse.subject</b><br> 1423 * </p> 1424 */ 1425 @SearchParamDefinition(name="subject", path="GuidanceResponse.subject", description="The subject that the guidance response is about", type="reference", target={Group.class, Patient.class } ) 1426 public static final String SP_SUBJECT = "subject"; 1427 /** 1428 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1429 * <p> 1430 * Description: <b>The subject that the guidance response is about</b><br> 1431 * Type: <b>reference</b><br> 1432 * Path: <b>GuidanceResponse.subject</b><br> 1433 * </p> 1434 */ 1435 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1436 1437/** 1438 * Constant for fluent queries to be used to add include statements. Specifies 1439 * the path value of "<b>GuidanceResponse:subject</b>". 1440 */ 1441 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("GuidanceResponse:subject").toLocked(); 1442 1443 1444}