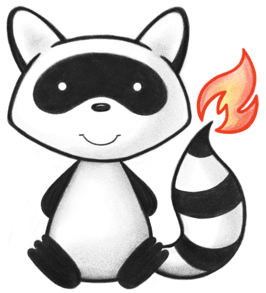
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * The details of a healthcare service available at a location. 050 */ 051@ResourceDef(name="HealthcareService", profile="http://hl7.org/fhir/Profile/HealthcareService") 052public class HealthcareService extends DomainResource { 053 054 public enum DaysOfWeek { 055 /** 056 * Monday 057 */ 058 MON, 059 /** 060 * Tuesday 061 */ 062 TUE, 063 /** 064 * Wednesday 065 */ 066 WED, 067 /** 068 * Thursday 069 */ 070 THU, 071 /** 072 * Friday 073 */ 074 FRI, 075 /** 076 * Saturday 077 */ 078 SAT, 079 /** 080 * Sunday 081 */ 082 SUN, 083 /** 084 * added to help the parsers with the generic types 085 */ 086 NULL; 087 public static DaysOfWeek fromCode(String codeString) throws FHIRException { 088 if (codeString == null || "".equals(codeString)) 089 return null; 090 if ("mon".equals(codeString)) 091 return MON; 092 if ("tue".equals(codeString)) 093 return TUE; 094 if ("wed".equals(codeString)) 095 return WED; 096 if ("thu".equals(codeString)) 097 return THU; 098 if ("fri".equals(codeString)) 099 return FRI; 100 if ("sat".equals(codeString)) 101 return SAT; 102 if ("sun".equals(codeString)) 103 return SUN; 104 if (Configuration.isAcceptInvalidEnums()) 105 return null; 106 else 107 throw new FHIRException("Unknown DaysOfWeek code '"+codeString+"'"); 108 } 109 public String toCode() { 110 switch (this) { 111 case MON: return "mon"; 112 case TUE: return "tue"; 113 case WED: return "wed"; 114 case THU: return "thu"; 115 case FRI: return "fri"; 116 case SAT: return "sat"; 117 case SUN: return "sun"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 public String getSystem() { 123 switch (this) { 124 case MON: return "http://hl7.org/fhir/days-of-week"; 125 case TUE: return "http://hl7.org/fhir/days-of-week"; 126 case WED: return "http://hl7.org/fhir/days-of-week"; 127 case THU: return "http://hl7.org/fhir/days-of-week"; 128 case FRI: return "http://hl7.org/fhir/days-of-week"; 129 case SAT: return "http://hl7.org/fhir/days-of-week"; 130 case SUN: return "http://hl7.org/fhir/days-of-week"; 131 case NULL: return null; 132 default: return "?"; 133 } 134 } 135 public String getDefinition() { 136 switch (this) { 137 case MON: return "Monday"; 138 case TUE: return "Tuesday"; 139 case WED: return "Wednesday"; 140 case THU: return "Thursday"; 141 case FRI: return "Friday"; 142 case SAT: return "Saturday"; 143 case SUN: return "Sunday"; 144 case NULL: return null; 145 default: return "?"; 146 } 147 } 148 public String getDisplay() { 149 switch (this) { 150 case MON: return "Monday"; 151 case TUE: return "Tuesday"; 152 case WED: return "Wednesday"; 153 case THU: return "Thursday"; 154 case FRI: return "Friday"; 155 case SAT: return "Saturday"; 156 case SUN: return "Sunday"; 157 case NULL: return null; 158 default: return "?"; 159 } 160 } 161 } 162 163 public static class DaysOfWeekEnumFactory implements EnumFactory<DaysOfWeek> { 164 public DaysOfWeek fromCode(String codeString) throws IllegalArgumentException { 165 if (codeString == null || "".equals(codeString)) 166 if (codeString == null || "".equals(codeString)) 167 return null; 168 if ("mon".equals(codeString)) 169 return DaysOfWeek.MON; 170 if ("tue".equals(codeString)) 171 return DaysOfWeek.TUE; 172 if ("wed".equals(codeString)) 173 return DaysOfWeek.WED; 174 if ("thu".equals(codeString)) 175 return DaysOfWeek.THU; 176 if ("fri".equals(codeString)) 177 return DaysOfWeek.FRI; 178 if ("sat".equals(codeString)) 179 return DaysOfWeek.SAT; 180 if ("sun".equals(codeString)) 181 return DaysOfWeek.SUN; 182 throw new IllegalArgumentException("Unknown DaysOfWeek code '"+codeString+"'"); 183 } 184 public Enumeration<DaysOfWeek> fromType(PrimitiveType<?> code) throws FHIRException { 185 if (code == null) 186 return null; 187 if (code.isEmpty()) 188 return new Enumeration<DaysOfWeek>(this); 189 String codeString = code.asStringValue(); 190 if (codeString == null || "".equals(codeString)) 191 return null; 192 if ("mon".equals(codeString)) 193 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.MON); 194 if ("tue".equals(codeString)) 195 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.TUE); 196 if ("wed".equals(codeString)) 197 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.WED); 198 if ("thu".equals(codeString)) 199 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.THU); 200 if ("fri".equals(codeString)) 201 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.FRI); 202 if ("sat".equals(codeString)) 203 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SAT); 204 if ("sun".equals(codeString)) 205 return new Enumeration<DaysOfWeek>(this, DaysOfWeek.SUN); 206 throw new FHIRException("Unknown DaysOfWeek code '"+codeString+"'"); 207 } 208 public String toCode(DaysOfWeek code) { 209 if (code == DaysOfWeek.NULL) 210 return null; 211 if (code == DaysOfWeek.MON) 212 return "mon"; 213 if (code == DaysOfWeek.TUE) 214 return "tue"; 215 if (code == DaysOfWeek.WED) 216 return "wed"; 217 if (code == DaysOfWeek.THU) 218 return "thu"; 219 if (code == DaysOfWeek.FRI) 220 return "fri"; 221 if (code == DaysOfWeek.SAT) 222 return "sat"; 223 if (code == DaysOfWeek.SUN) 224 return "sun"; 225 return "?"; 226 } 227 public String toSystem(DaysOfWeek code) { 228 return code.getSystem(); 229 } 230 } 231 232 @Block() 233 public static class HealthcareServiceAvailableTimeComponent extends BackboneElement implements IBaseBackboneElement { 234 /** 235 * Indicates which days of the week are available between the start and end Times. 236 */ 237 @Child(name = "daysOfWeek", type = {CodeType.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 238 @Description(shortDefinition="mon | tue | wed | thu | fri | sat | sun", formalDefinition="Indicates which days of the week are available between the start and end Times." ) 239 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/days-of-week") 240 protected List<Enumeration<DaysOfWeek>> daysOfWeek; 241 242 /** 243 * Is this always available? (hence times are irrelevant) e.g. 24 hour service. 244 */ 245 @Child(name = "allDay", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 246 @Description(shortDefinition="Always available? e.g. 24 hour service", formalDefinition="Is this always available? (hence times are irrelevant) e.g. 24 hour service." ) 247 protected BooleanType allDay; 248 249 /** 250 * The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 251 */ 252 @Child(name = "availableStartTime", type = {TimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 253 @Description(shortDefinition="Opening time of day (ignored if allDay = true)", formalDefinition="The opening time of day. Note: If the AllDay flag is set, then this time is ignored." ) 254 protected TimeType availableStartTime; 255 256 /** 257 * The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 258 */ 259 @Child(name = "availableEndTime", type = {TimeType.class}, order=4, min=0, max=1, modifier=false, summary=false) 260 @Description(shortDefinition="Closing time of day (ignored if allDay = true)", formalDefinition="The closing time of day. Note: If the AllDay flag is set, then this time is ignored." ) 261 protected TimeType availableEndTime; 262 263 private static final long serialVersionUID = -2139510127L; 264 265 /** 266 * Constructor 267 */ 268 public HealthcareServiceAvailableTimeComponent() { 269 super(); 270 } 271 272 /** 273 * @return {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 274 */ 275 public List<Enumeration<DaysOfWeek>> getDaysOfWeek() { 276 if (this.daysOfWeek == null) 277 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 278 return this.daysOfWeek; 279 } 280 281 /** 282 * @return Returns a reference to <code>this</code> for easy method chaining 283 */ 284 public HealthcareServiceAvailableTimeComponent setDaysOfWeek(List<Enumeration<DaysOfWeek>> theDaysOfWeek) { 285 this.daysOfWeek = theDaysOfWeek; 286 return this; 287 } 288 289 public boolean hasDaysOfWeek() { 290 if (this.daysOfWeek == null) 291 return false; 292 for (Enumeration<DaysOfWeek> item : this.daysOfWeek) 293 if (!item.isEmpty()) 294 return true; 295 return false; 296 } 297 298 /** 299 * @return {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 300 */ 301 public Enumeration<DaysOfWeek> addDaysOfWeekElement() {//2 302 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 303 if (this.daysOfWeek == null) 304 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 305 this.daysOfWeek.add(t); 306 return t; 307 } 308 309 /** 310 * @param value {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 311 */ 312 public HealthcareServiceAvailableTimeComponent addDaysOfWeek(DaysOfWeek value) { //1 313 Enumeration<DaysOfWeek> t = new Enumeration<DaysOfWeek>(new DaysOfWeekEnumFactory()); 314 t.setValue(value); 315 if (this.daysOfWeek == null) 316 this.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 317 this.daysOfWeek.add(t); 318 return this; 319 } 320 321 /** 322 * @param value {@link #daysOfWeek} (Indicates which days of the week are available between the start and end Times.) 323 */ 324 public boolean hasDaysOfWeek(DaysOfWeek value) { 325 if (this.daysOfWeek == null) 326 return false; 327 for (Enumeration<DaysOfWeek> v : this.daysOfWeek) 328 if (v.getValue().equals(value)) // code 329 return true; 330 return false; 331 } 332 333 /** 334 * @return {@link #allDay} (Is this always available? (hence times are irrelevant) e.g. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 335 */ 336 public BooleanType getAllDayElement() { 337 if (this.allDay == null) 338 if (Configuration.errorOnAutoCreate()) 339 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.allDay"); 340 else if (Configuration.doAutoCreate()) 341 this.allDay = new BooleanType(); // bb 342 return this.allDay; 343 } 344 345 public boolean hasAllDayElement() { 346 return this.allDay != null && !this.allDay.isEmpty(); 347 } 348 349 public boolean hasAllDay() { 350 return this.allDay != null && !this.allDay.isEmpty(); 351 } 352 353 /** 354 * @param value {@link #allDay} (Is this always available? (hence times are irrelevant) e.g. 24 hour service.). This is the underlying object with id, value and extensions. The accessor "getAllDay" gives direct access to the value 355 */ 356 public HealthcareServiceAvailableTimeComponent setAllDayElement(BooleanType value) { 357 this.allDay = value; 358 return this; 359 } 360 361 /** 362 * @return Is this always available? (hence times are irrelevant) e.g. 24 hour service. 363 */ 364 public boolean getAllDay() { 365 return this.allDay == null || this.allDay.isEmpty() ? false : this.allDay.getValue(); 366 } 367 368 /** 369 * @param value Is this always available? (hence times are irrelevant) e.g. 24 hour service. 370 */ 371 public HealthcareServiceAvailableTimeComponent setAllDay(boolean value) { 372 if (this.allDay == null) 373 this.allDay = new BooleanType(); 374 this.allDay.setValue(value); 375 return this; 376 } 377 378 /** 379 * @return {@link #availableStartTime} (The opening time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 380 */ 381 public TimeType getAvailableStartTimeElement() { 382 if (this.availableStartTime == null) 383 if (Configuration.errorOnAutoCreate()) 384 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.availableStartTime"); 385 else if (Configuration.doAutoCreate()) 386 this.availableStartTime = new TimeType(); // bb 387 return this.availableStartTime; 388 } 389 390 public boolean hasAvailableStartTimeElement() { 391 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 392 } 393 394 public boolean hasAvailableStartTime() { 395 return this.availableStartTime != null && !this.availableStartTime.isEmpty(); 396 } 397 398 /** 399 * @param value {@link #availableStartTime} (The opening time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableStartTime" gives direct access to the value 400 */ 401 public HealthcareServiceAvailableTimeComponent setAvailableStartTimeElement(TimeType value) { 402 this.availableStartTime = value; 403 return this; 404 } 405 406 /** 407 * @return The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 408 */ 409 public String getAvailableStartTime() { 410 return this.availableStartTime == null ? null : this.availableStartTime.getValue(); 411 } 412 413 /** 414 * @param value The opening time of day. Note: If the AllDay flag is set, then this time is ignored. 415 */ 416 public HealthcareServiceAvailableTimeComponent setAvailableStartTime(String value) { 417 if (value == null) 418 this.availableStartTime = null; 419 else { 420 if (this.availableStartTime == null) 421 this.availableStartTime = new TimeType(); 422 this.availableStartTime.setValue(value); 423 } 424 return this; 425 } 426 427 /** 428 * @return {@link #availableEndTime} (The closing time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 429 */ 430 public TimeType getAvailableEndTimeElement() { 431 if (this.availableEndTime == null) 432 if (Configuration.errorOnAutoCreate()) 433 throw new Error("Attempt to auto-create HealthcareServiceAvailableTimeComponent.availableEndTime"); 434 else if (Configuration.doAutoCreate()) 435 this.availableEndTime = new TimeType(); // bb 436 return this.availableEndTime; 437 } 438 439 public boolean hasAvailableEndTimeElement() { 440 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 441 } 442 443 public boolean hasAvailableEndTime() { 444 return this.availableEndTime != null && !this.availableEndTime.isEmpty(); 445 } 446 447 /** 448 * @param value {@link #availableEndTime} (The closing time of day. Note: If the AllDay flag is set, then this time is ignored.). This is the underlying object with id, value and extensions. The accessor "getAvailableEndTime" gives direct access to the value 449 */ 450 public HealthcareServiceAvailableTimeComponent setAvailableEndTimeElement(TimeType value) { 451 this.availableEndTime = value; 452 return this; 453 } 454 455 /** 456 * @return The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 457 */ 458 public String getAvailableEndTime() { 459 return this.availableEndTime == null ? null : this.availableEndTime.getValue(); 460 } 461 462 /** 463 * @param value The closing time of day. Note: If the AllDay flag is set, then this time is ignored. 464 */ 465 public HealthcareServiceAvailableTimeComponent setAvailableEndTime(String value) { 466 if (value == null) 467 this.availableEndTime = null; 468 else { 469 if (this.availableEndTime == null) 470 this.availableEndTime = new TimeType(); 471 this.availableEndTime.setValue(value); 472 } 473 return this; 474 } 475 476 protected void listChildren(List<Property> children) { 477 super.listChildren(children); 478 children.add(new Property("daysOfWeek", "code", "Indicates which days of the week are available between the start and end Times.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek)); 479 children.add(new Property("allDay", "boolean", "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay)); 480 children.add(new Property("availableStartTime", "time", "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableStartTime)); 481 children.add(new Property("availableEndTime", "time", "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableEndTime)); 482 } 483 484 @Override 485 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 486 switch (_hash) { 487 case 68050338: /*daysOfWeek*/ return new Property("daysOfWeek", "code", "Indicates which days of the week are available between the start and end Times.", 0, java.lang.Integer.MAX_VALUE, daysOfWeek); 488 case -1414913477: /*allDay*/ return new Property("allDay", "boolean", "Is this always available? (hence times are irrelevant) e.g. 24 hour service.", 0, 1, allDay); 489 case -1039453818: /*availableStartTime*/ return new Property("availableStartTime", "time", "The opening time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableStartTime); 490 case 101151551: /*availableEndTime*/ return new Property("availableEndTime", "time", "The closing time of day. Note: If the AllDay flag is set, then this time is ignored.", 0, 1, availableEndTime); 491 default: return super.getNamedProperty(_hash, _name, _checkValid); 492 } 493 494 } 495 496 @Override 497 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 498 switch (hash) { 499 case 68050338: /*daysOfWeek*/ return this.daysOfWeek == null ? new Base[0] : this.daysOfWeek.toArray(new Base[this.daysOfWeek.size()]); // Enumeration<DaysOfWeek> 500 case -1414913477: /*allDay*/ return this.allDay == null ? new Base[0] : new Base[] {this.allDay}; // BooleanType 501 case -1039453818: /*availableStartTime*/ return this.availableStartTime == null ? new Base[0] : new Base[] {this.availableStartTime}; // TimeType 502 case 101151551: /*availableEndTime*/ return this.availableEndTime == null ? new Base[0] : new Base[] {this.availableEndTime}; // TimeType 503 default: return super.getProperty(hash, name, checkValid); 504 } 505 506 } 507 508 @Override 509 public Base setProperty(int hash, String name, Base value) throws FHIRException { 510 switch (hash) { 511 case 68050338: // daysOfWeek 512 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 513 this.getDaysOfWeek().add((Enumeration) value); // Enumeration<DaysOfWeek> 514 return value; 515 case -1414913477: // allDay 516 this.allDay = castToBoolean(value); // BooleanType 517 return value; 518 case -1039453818: // availableStartTime 519 this.availableStartTime = castToTime(value); // TimeType 520 return value; 521 case 101151551: // availableEndTime 522 this.availableEndTime = castToTime(value); // TimeType 523 return value; 524 default: return super.setProperty(hash, name, value); 525 } 526 527 } 528 529 @Override 530 public Base setProperty(String name, Base value) throws FHIRException { 531 if (name.equals("daysOfWeek")) { 532 value = new DaysOfWeekEnumFactory().fromType(castToCode(value)); 533 this.getDaysOfWeek().add((Enumeration) value); 534 } else if (name.equals("allDay")) { 535 this.allDay = castToBoolean(value); // BooleanType 536 } else if (name.equals("availableStartTime")) { 537 this.availableStartTime = castToTime(value); // TimeType 538 } else if (name.equals("availableEndTime")) { 539 this.availableEndTime = castToTime(value); // TimeType 540 } else 541 return super.setProperty(name, value); 542 return value; 543 } 544 545 @Override 546 public Base makeProperty(int hash, String name) throws FHIRException { 547 switch (hash) { 548 case 68050338: return addDaysOfWeekElement(); 549 case -1414913477: return getAllDayElement(); 550 case -1039453818: return getAvailableStartTimeElement(); 551 case 101151551: return getAvailableEndTimeElement(); 552 default: return super.makeProperty(hash, name); 553 } 554 555 } 556 557 @Override 558 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 559 switch (hash) { 560 case 68050338: /*daysOfWeek*/ return new String[] {"code"}; 561 case -1414913477: /*allDay*/ return new String[] {"boolean"}; 562 case -1039453818: /*availableStartTime*/ return new String[] {"time"}; 563 case 101151551: /*availableEndTime*/ return new String[] {"time"}; 564 default: return super.getTypesForProperty(hash, name); 565 } 566 567 } 568 569 @Override 570 public Base addChild(String name) throws FHIRException { 571 if (name.equals("daysOfWeek")) { 572 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.daysOfWeek"); 573 } 574 else if (name.equals("allDay")) { 575 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.allDay"); 576 } 577 else if (name.equals("availableStartTime")) { 578 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availableStartTime"); 579 } 580 else if (name.equals("availableEndTime")) { 581 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availableEndTime"); 582 } 583 else 584 return super.addChild(name); 585 } 586 587 public HealthcareServiceAvailableTimeComponent copy() { 588 HealthcareServiceAvailableTimeComponent dst = new HealthcareServiceAvailableTimeComponent(); 589 copyValues(dst); 590 if (daysOfWeek != null) { 591 dst.daysOfWeek = new ArrayList<Enumeration<DaysOfWeek>>(); 592 for (Enumeration<DaysOfWeek> i : daysOfWeek) 593 dst.daysOfWeek.add(i.copy()); 594 }; 595 dst.allDay = allDay == null ? null : allDay.copy(); 596 dst.availableStartTime = availableStartTime == null ? null : availableStartTime.copy(); 597 dst.availableEndTime = availableEndTime == null ? null : availableEndTime.copy(); 598 return dst; 599 } 600 601 @Override 602 public boolean equalsDeep(Base other_) { 603 if (!super.equalsDeep(other_)) 604 return false; 605 if (!(other_ instanceof HealthcareServiceAvailableTimeComponent)) 606 return false; 607 HealthcareServiceAvailableTimeComponent o = (HealthcareServiceAvailableTimeComponent) other_; 608 return compareDeep(daysOfWeek, o.daysOfWeek, true) && compareDeep(allDay, o.allDay, true) && compareDeep(availableStartTime, o.availableStartTime, true) 609 && compareDeep(availableEndTime, o.availableEndTime, true); 610 } 611 612 @Override 613 public boolean equalsShallow(Base other_) { 614 if (!super.equalsShallow(other_)) 615 return false; 616 if (!(other_ instanceof HealthcareServiceAvailableTimeComponent)) 617 return false; 618 HealthcareServiceAvailableTimeComponent o = (HealthcareServiceAvailableTimeComponent) other_; 619 return compareValues(daysOfWeek, o.daysOfWeek, true) && compareValues(allDay, o.allDay, true) && compareValues(availableStartTime, o.availableStartTime, true) 620 && compareValues(availableEndTime, o.availableEndTime, true); 621 } 622 623 public boolean isEmpty() { 624 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(daysOfWeek, allDay, availableStartTime 625 , availableEndTime); 626 } 627 628 public String fhirType() { 629 return "HealthcareService.availableTime"; 630 631 } 632 633 } 634 635 @Block() 636 public static class HealthcareServiceNotAvailableComponent extends BackboneElement implements IBaseBackboneElement { 637 /** 638 * The reason that can be presented to the user as to why this time is not available. 639 */ 640 @Child(name = "description", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 641 @Description(shortDefinition="Reason presented to the user explaining why time not available", formalDefinition="The reason that can be presented to the user as to why this time is not available." ) 642 protected StringType description; 643 644 /** 645 * Service is not available (seasonally or for a public holiday) from this date. 646 */ 647 @Child(name = "during", type = {Period.class}, order=2, min=0, max=1, modifier=false, summary=false) 648 @Description(shortDefinition="Service not availablefrom this date", formalDefinition="Service is not available (seasonally or for a public holiday) from this date." ) 649 protected Period during; 650 651 private static final long serialVersionUID = 310849929L; 652 653 /** 654 * Constructor 655 */ 656 public HealthcareServiceNotAvailableComponent() { 657 super(); 658 } 659 660 /** 661 * Constructor 662 */ 663 public HealthcareServiceNotAvailableComponent(StringType description) { 664 super(); 665 this.description = description; 666 } 667 668 /** 669 * @return {@link #description} (The reason that can be presented to the user as to why this time is not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 670 */ 671 public StringType getDescriptionElement() { 672 if (this.description == null) 673 if (Configuration.errorOnAutoCreate()) 674 throw new Error("Attempt to auto-create HealthcareServiceNotAvailableComponent.description"); 675 else if (Configuration.doAutoCreate()) 676 this.description = new StringType(); // bb 677 return this.description; 678 } 679 680 public boolean hasDescriptionElement() { 681 return this.description != null && !this.description.isEmpty(); 682 } 683 684 public boolean hasDescription() { 685 return this.description != null && !this.description.isEmpty(); 686 } 687 688 /** 689 * @param value {@link #description} (The reason that can be presented to the user as to why this time is not available.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 690 */ 691 public HealthcareServiceNotAvailableComponent setDescriptionElement(StringType value) { 692 this.description = value; 693 return this; 694 } 695 696 /** 697 * @return The reason that can be presented to the user as to why this time is not available. 698 */ 699 public String getDescription() { 700 return this.description == null ? null : this.description.getValue(); 701 } 702 703 /** 704 * @param value The reason that can be presented to the user as to why this time is not available. 705 */ 706 public HealthcareServiceNotAvailableComponent setDescription(String value) { 707 if (this.description == null) 708 this.description = new StringType(); 709 this.description.setValue(value); 710 return this; 711 } 712 713 /** 714 * @return {@link #during} (Service is not available (seasonally or for a public holiday) from this date.) 715 */ 716 public Period getDuring() { 717 if (this.during == null) 718 if (Configuration.errorOnAutoCreate()) 719 throw new Error("Attempt to auto-create HealthcareServiceNotAvailableComponent.during"); 720 else if (Configuration.doAutoCreate()) 721 this.during = new Period(); // cc 722 return this.during; 723 } 724 725 public boolean hasDuring() { 726 return this.during != null && !this.during.isEmpty(); 727 } 728 729 /** 730 * @param value {@link #during} (Service is not available (seasonally or for a public holiday) from this date.) 731 */ 732 public HealthcareServiceNotAvailableComponent setDuring(Period value) { 733 this.during = value; 734 return this; 735 } 736 737 protected void listChildren(List<Property> children) { 738 super.listChildren(children); 739 children.add(new Property("description", "string", "The reason that can be presented to the user as to why this time is not available.", 0, 1, description)); 740 children.add(new Property("during", "Period", "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during)); 741 } 742 743 @Override 744 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 745 switch (_hash) { 746 case -1724546052: /*description*/ return new Property("description", "string", "The reason that can be presented to the user as to why this time is not available.", 0, 1, description); 747 case -1320499647: /*during*/ return new Property("during", "Period", "Service is not available (seasonally or for a public holiday) from this date.", 0, 1, during); 748 default: return super.getNamedProperty(_hash, _name, _checkValid); 749 } 750 751 } 752 753 @Override 754 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 755 switch (hash) { 756 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 757 case -1320499647: /*during*/ return this.during == null ? new Base[0] : new Base[] {this.during}; // Period 758 default: return super.getProperty(hash, name, checkValid); 759 } 760 761 } 762 763 @Override 764 public Base setProperty(int hash, String name, Base value) throws FHIRException { 765 switch (hash) { 766 case -1724546052: // description 767 this.description = castToString(value); // StringType 768 return value; 769 case -1320499647: // during 770 this.during = castToPeriod(value); // Period 771 return value; 772 default: return super.setProperty(hash, name, value); 773 } 774 775 } 776 777 @Override 778 public Base setProperty(String name, Base value) throws FHIRException { 779 if (name.equals("description")) { 780 this.description = castToString(value); // StringType 781 } else if (name.equals("during")) { 782 this.during = castToPeriod(value); // Period 783 } else 784 return super.setProperty(name, value); 785 return value; 786 } 787 788 @Override 789 public Base makeProperty(int hash, String name) throws FHIRException { 790 switch (hash) { 791 case -1724546052: return getDescriptionElement(); 792 case -1320499647: return getDuring(); 793 default: return super.makeProperty(hash, name); 794 } 795 796 } 797 798 @Override 799 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 800 switch (hash) { 801 case -1724546052: /*description*/ return new String[] {"string"}; 802 case -1320499647: /*during*/ return new String[] {"Period"}; 803 default: return super.getTypesForProperty(hash, name); 804 } 805 806 } 807 808 @Override 809 public Base addChild(String name) throws FHIRException { 810 if (name.equals("description")) { 811 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.description"); 812 } 813 else if (name.equals("during")) { 814 this.during = new Period(); 815 return this.during; 816 } 817 else 818 return super.addChild(name); 819 } 820 821 public HealthcareServiceNotAvailableComponent copy() { 822 HealthcareServiceNotAvailableComponent dst = new HealthcareServiceNotAvailableComponent(); 823 copyValues(dst); 824 dst.description = description == null ? null : description.copy(); 825 dst.during = during == null ? null : during.copy(); 826 return dst; 827 } 828 829 @Override 830 public boolean equalsDeep(Base other_) { 831 if (!super.equalsDeep(other_)) 832 return false; 833 if (!(other_ instanceof HealthcareServiceNotAvailableComponent)) 834 return false; 835 HealthcareServiceNotAvailableComponent o = (HealthcareServiceNotAvailableComponent) other_; 836 return compareDeep(description, o.description, true) && compareDeep(during, o.during, true); 837 } 838 839 @Override 840 public boolean equalsShallow(Base other_) { 841 if (!super.equalsShallow(other_)) 842 return false; 843 if (!(other_ instanceof HealthcareServiceNotAvailableComponent)) 844 return false; 845 HealthcareServiceNotAvailableComponent o = (HealthcareServiceNotAvailableComponent) other_; 846 return compareValues(description, o.description, true); 847 } 848 849 public boolean isEmpty() { 850 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(description, during); 851 } 852 853 public String fhirType() { 854 return "HealthcareService.notAvailable"; 855 856 } 857 858 } 859 860 /** 861 * External identifiers for this item. 862 */ 863 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 864 @Description(shortDefinition="External identifiers for this item", formalDefinition="External identifiers for this item." ) 865 protected List<Identifier> identifier; 866 867 /** 868 * Whether this healthcareservice record is in active use. 869 */ 870 @Child(name = "active", type = {BooleanType.class}, order=1, min=0, max=1, modifier=true, summary=true) 871 @Description(shortDefinition="Whether this healthcareservice is in active use", formalDefinition="Whether this healthcareservice record is in active use." ) 872 protected BooleanType active; 873 874 /** 875 * The organization that provides this healthcare service. 876 */ 877 @Child(name = "providedBy", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 878 @Description(shortDefinition="Organization that provides this service", formalDefinition="The organization that provides this healthcare service." ) 879 protected Reference providedBy; 880 881 /** 882 * The actual object that is the target of the reference (The organization that provides this healthcare service.) 883 */ 884 protected Organization providedByTarget; 885 886 /** 887 * Identifies the broad category of service being performed or delivered. 888 */ 889 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 890 @Description(shortDefinition="Broad category of service being performed or delivered", formalDefinition="Identifies the broad category of service being performed or delivered." ) 891 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-category") 892 protected CodeableConcept category; 893 894 /** 895 * The specific type of service that may be delivered or performed. 896 */ 897 @Child(name = "type", type = {CodeableConcept.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 898 @Description(shortDefinition="Type of service that may be delivered or performed", formalDefinition="The specific type of service that may be delivered or performed." ) 899 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-type") 900 protected List<CodeableConcept> type; 901 902 /** 903 * Collection of specialties handled by the service site. This is more of a medical term. 904 */ 905 @Child(name = "specialty", type = {CodeableConcept.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 906 @Description(shortDefinition="Specialties handled by the HealthcareService", formalDefinition="Collection of specialties handled by the service site. This is more of a medical term." ) 907 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/c80-practice-codes") 908 protected List<CodeableConcept> specialty; 909 910 /** 911 * The location(s) where this healthcare service may be provided. 912 */ 913 @Child(name = "location", type = {Location.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 914 @Description(shortDefinition="Location(s) where service may be provided", formalDefinition="The location(s) where this healthcare service may be provided." ) 915 protected List<Reference> location; 916 /** 917 * The actual objects that are the target of the reference (The location(s) where this healthcare service may be provided.) 918 */ 919 protected List<Location> locationTarget; 920 921 922 /** 923 * Further description of the service as it would be presented to a consumer while searching. 924 */ 925 @Child(name = "name", type = {StringType.class}, order=7, min=0, max=1, modifier=false, summary=true) 926 @Description(shortDefinition="Description of service as presented to a consumer while searching", formalDefinition="Further description of the service as it would be presented to a consumer while searching." ) 927 protected StringType name; 928 929 /** 930 * Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName. 931 */ 932 @Child(name = "comment", type = {StringType.class}, order=8, min=0, max=1, modifier=false, summary=true) 933 @Description(shortDefinition="Additional description and/or any specific issues not covered elsewhere", formalDefinition="Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName." ) 934 protected StringType comment; 935 936 /** 937 * Extra details about the service that can't be placed in the other fields. 938 */ 939 @Child(name = "extraDetails", type = {StringType.class}, order=9, min=0, max=1, modifier=false, summary=false) 940 @Description(shortDefinition="Extra details about the service that can't be placed in the other fields", formalDefinition="Extra details about the service that can't be placed in the other fields." ) 941 protected StringType extraDetails; 942 943 /** 944 * If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list. 945 */ 946 @Child(name = "photo", type = {Attachment.class}, order=10, min=0, max=1, modifier=false, summary=true) 947 @Description(shortDefinition="Facilitates quick identification of the service", formalDefinition="If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list." ) 948 protected Attachment photo; 949 950 /** 951 * List of contacts related to this specific healthcare service. 952 */ 953 @Child(name = "telecom", type = {ContactPoint.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 954 @Description(shortDefinition="Contacts related to the healthcare service", formalDefinition="List of contacts related to this specific healthcare service." ) 955 protected List<ContactPoint> telecom; 956 957 /** 958 * The location(s) that this service is available to (not where the service is provided). 959 */ 960 @Child(name = "coverageArea", type = {Location.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 961 @Description(shortDefinition="Location(s) service is inteded for/available to", formalDefinition="The location(s) that this service is available to (not where the service is provided)." ) 962 protected List<Reference> coverageArea; 963 /** 964 * The actual objects that are the target of the reference (The location(s) that this service is available to (not where the service is provided).) 965 */ 966 protected List<Location> coverageAreaTarget; 967 968 969 /** 970 * The code(s) that detail the conditions under which the healthcare service is available/offered. 971 */ 972 @Child(name = "serviceProvisionCode", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 973 @Description(shortDefinition="Conditions under which service is available/offered", formalDefinition="The code(s) that detail the conditions under which the healthcare service is available/offered." ) 974 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-provision-conditions") 975 protected List<CodeableConcept> serviceProvisionCode; 976 977 /** 978 * Does this service have specific eligibility requirements that need to be met in order to use the service? 979 */ 980 @Child(name = "eligibility", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 981 @Description(shortDefinition="Specific eligibility requirements required to use the service", formalDefinition="Does this service have specific eligibility requirements that need to be met in order to use the service?" ) 982 protected CodeableConcept eligibility; 983 984 /** 985 * Describes the eligibility conditions for the service. 986 */ 987 @Child(name = "eligibilityNote", type = {StringType.class}, order=15, min=0, max=1, modifier=false, summary=false) 988 @Description(shortDefinition="Describes the eligibility conditions for the service", formalDefinition="Describes the eligibility conditions for the service." ) 989 protected StringType eligibilityNote; 990 991 /** 992 * Program Names that can be used to categorize the service. 993 */ 994 @Child(name = "programName", type = {StringType.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 995 @Description(shortDefinition="Program Names that categorize the service", formalDefinition="Program Names that can be used to categorize the service." ) 996 protected List<StringType> programName; 997 998 /** 999 * Collection of characteristics (attributes). 1000 */ 1001 @Child(name = "characteristic", type = {CodeableConcept.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1002 @Description(shortDefinition="Collection of characteristics (attributes)", formalDefinition="Collection of characteristics (attributes)." ) 1003 protected List<CodeableConcept> characteristic; 1004 1005 /** 1006 * Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required. 1007 */ 1008 @Child(name = "referralMethod", type = {CodeableConcept.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1009 @Description(shortDefinition="Ways that the service accepts referrals", formalDefinition="Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required." ) 1010 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/service-referral-method") 1011 protected List<CodeableConcept> referralMethod; 1012 1013 /** 1014 * Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service. 1015 */ 1016 @Child(name = "appointmentRequired", type = {BooleanType.class}, order=19, min=0, max=1, modifier=false, summary=false) 1017 @Description(shortDefinition="If an appointment is required for access to this service", formalDefinition="Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service." ) 1018 protected BooleanType appointmentRequired; 1019 1020 /** 1021 * A collection of times that the Service Site is available. 1022 */ 1023 @Child(name = "availableTime", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1024 @Description(shortDefinition="Times the Service Site is available", formalDefinition="A collection of times that the Service Site is available." ) 1025 protected List<HealthcareServiceAvailableTimeComponent> availableTime; 1026 1027 /** 1028 * The HealthcareService is not available during this period of time due to the provided reason. 1029 */ 1030 @Child(name = "notAvailable", type = {}, order=21, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1031 @Description(shortDefinition="Not available during this time due to provided reason", formalDefinition="The HealthcareService is not available during this period of time due to the provided reason." ) 1032 protected List<HealthcareServiceNotAvailableComponent> notAvailable; 1033 1034 /** 1035 * A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 1036 */ 1037 @Child(name = "availabilityExceptions", type = {StringType.class}, order=22, min=0, max=1, modifier=false, summary=false) 1038 @Description(shortDefinition="Description of availability exceptions", formalDefinition="A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times." ) 1039 protected StringType availabilityExceptions; 1040 1041 /** 1042 * Technical endpoints providing access to services operated for the specific healthcare services defined at this resource. 1043 */ 1044 @Child(name = "endpoint", type = {Endpoint.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1045 @Description(shortDefinition="Technical endpoints providing access to services operated for the location", formalDefinition="Technical endpoints providing access to services operated for the specific healthcare services defined at this resource." ) 1046 protected List<Reference> endpoint; 1047 /** 1048 * The actual objects that are the target of the reference (Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.) 1049 */ 1050 protected List<Endpoint> endpointTarget; 1051 1052 1053 private static final long serialVersionUID = -202805485L; 1054 1055 /** 1056 * Constructor 1057 */ 1058 public HealthcareService() { 1059 super(); 1060 } 1061 1062 /** 1063 * @return {@link #identifier} (External identifiers for this item.) 1064 */ 1065 public List<Identifier> getIdentifier() { 1066 if (this.identifier == null) 1067 this.identifier = new ArrayList<Identifier>(); 1068 return this.identifier; 1069 } 1070 1071 /** 1072 * @return Returns a reference to <code>this</code> for easy method chaining 1073 */ 1074 public HealthcareService setIdentifier(List<Identifier> theIdentifier) { 1075 this.identifier = theIdentifier; 1076 return this; 1077 } 1078 1079 public boolean hasIdentifier() { 1080 if (this.identifier == null) 1081 return false; 1082 for (Identifier item : this.identifier) 1083 if (!item.isEmpty()) 1084 return true; 1085 return false; 1086 } 1087 1088 public Identifier addIdentifier() { //3 1089 Identifier t = new Identifier(); 1090 if (this.identifier == null) 1091 this.identifier = new ArrayList<Identifier>(); 1092 this.identifier.add(t); 1093 return t; 1094 } 1095 1096 public HealthcareService addIdentifier(Identifier t) { //3 1097 if (t == null) 1098 return this; 1099 if (this.identifier == null) 1100 this.identifier = new ArrayList<Identifier>(); 1101 this.identifier.add(t); 1102 return this; 1103 } 1104 1105 /** 1106 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1107 */ 1108 public Identifier getIdentifierFirstRep() { 1109 if (getIdentifier().isEmpty()) { 1110 addIdentifier(); 1111 } 1112 return getIdentifier().get(0); 1113 } 1114 1115 /** 1116 * @return {@link #active} (Whether this healthcareservice record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1117 */ 1118 public BooleanType getActiveElement() { 1119 if (this.active == null) 1120 if (Configuration.errorOnAutoCreate()) 1121 throw new Error("Attempt to auto-create HealthcareService.active"); 1122 else if (Configuration.doAutoCreate()) 1123 this.active = new BooleanType(); // bb 1124 return this.active; 1125 } 1126 1127 public boolean hasActiveElement() { 1128 return this.active != null && !this.active.isEmpty(); 1129 } 1130 1131 public boolean hasActive() { 1132 return this.active != null && !this.active.isEmpty(); 1133 } 1134 1135 /** 1136 * @param value {@link #active} (Whether this healthcareservice record is in active use.). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 1137 */ 1138 public HealthcareService setActiveElement(BooleanType value) { 1139 this.active = value; 1140 return this; 1141 } 1142 1143 /** 1144 * @return Whether this healthcareservice record is in active use. 1145 */ 1146 public boolean getActive() { 1147 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 1148 } 1149 1150 /** 1151 * @param value Whether this healthcareservice record is in active use. 1152 */ 1153 public HealthcareService setActive(boolean value) { 1154 if (this.active == null) 1155 this.active = new BooleanType(); 1156 this.active.setValue(value); 1157 return this; 1158 } 1159 1160 /** 1161 * @return {@link #providedBy} (The organization that provides this healthcare service.) 1162 */ 1163 public Reference getProvidedBy() { 1164 if (this.providedBy == null) 1165 if (Configuration.errorOnAutoCreate()) 1166 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 1167 else if (Configuration.doAutoCreate()) 1168 this.providedBy = new Reference(); // cc 1169 return this.providedBy; 1170 } 1171 1172 public boolean hasProvidedBy() { 1173 return this.providedBy != null && !this.providedBy.isEmpty(); 1174 } 1175 1176 /** 1177 * @param value {@link #providedBy} (The organization that provides this healthcare service.) 1178 */ 1179 public HealthcareService setProvidedBy(Reference value) { 1180 this.providedBy = value; 1181 return this; 1182 } 1183 1184 /** 1185 * @return {@link #providedBy} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization that provides this healthcare service.) 1186 */ 1187 public Organization getProvidedByTarget() { 1188 if (this.providedByTarget == null) 1189 if (Configuration.errorOnAutoCreate()) 1190 throw new Error("Attempt to auto-create HealthcareService.providedBy"); 1191 else if (Configuration.doAutoCreate()) 1192 this.providedByTarget = new Organization(); // aa 1193 return this.providedByTarget; 1194 } 1195 1196 /** 1197 * @param value {@link #providedBy} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization that provides this healthcare service.) 1198 */ 1199 public HealthcareService setProvidedByTarget(Organization value) { 1200 this.providedByTarget = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return {@link #category} (Identifies the broad category of service being performed or delivered.) 1206 */ 1207 public CodeableConcept getCategory() { 1208 if (this.category == null) 1209 if (Configuration.errorOnAutoCreate()) 1210 throw new Error("Attempt to auto-create HealthcareService.category"); 1211 else if (Configuration.doAutoCreate()) 1212 this.category = new CodeableConcept(); // cc 1213 return this.category; 1214 } 1215 1216 public boolean hasCategory() { 1217 return this.category != null && !this.category.isEmpty(); 1218 } 1219 1220 /** 1221 * @param value {@link #category} (Identifies the broad category of service being performed or delivered.) 1222 */ 1223 public HealthcareService setCategory(CodeableConcept value) { 1224 this.category = value; 1225 return this; 1226 } 1227 1228 /** 1229 * @return {@link #type} (The specific type of service that may be delivered or performed.) 1230 */ 1231 public List<CodeableConcept> getType() { 1232 if (this.type == null) 1233 this.type = new ArrayList<CodeableConcept>(); 1234 return this.type; 1235 } 1236 1237 /** 1238 * @return Returns a reference to <code>this</code> for easy method chaining 1239 */ 1240 public HealthcareService setType(List<CodeableConcept> theType) { 1241 this.type = theType; 1242 return this; 1243 } 1244 1245 public boolean hasType() { 1246 if (this.type == null) 1247 return false; 1248 for (CodeableConcept item : this.type) 1249 if (!item.isEmpty()) 1250 return true; 1251 return false; 1252 } 1253 1254 public CodeableConcept addType() { //3 1255 CodeableConcept t = new CodeableConcept(); 1256 if (this.type == null) 1257 this.type = new ArrayList<CodeableConcept>(); 1258 this.type.add(t); 1259 return t; 1260 } 1261 1262 public HealthcareService addType(CodeableConcept t) { //3 1263 if (t == null) 1264 return this; 1265 if (this.type == null) 1266 this.type = new ArrayList<CodeableConcept>(); 1267 this.type.add(t); 1268 return this; 1269 } 1270 1271 /** 1272 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 1273 */ 1274 public CodeableConcept getTypeFirstRep() { 1275 if (getType().isEmpty()) { 1276 addType(); 1277 } 1278 return getType().get(0); 1279 } 1280 1281 /** 1282 * @return {@link #specialty} (Collection of specialties handled by the service site. This is more of a medical term.) 1283 */ 1284 public List<CodeableConcept> getSpecialty() { 1285 if (this.specialty == null) 1286 this.specialty = new ArrayList<CodeableConcept>(); 1287 return this.specialty; 1288 } 1289 1290 /** 1291 * @return Returns a reference to <code>this</code> for easy method chaining 1292 */ 1293 public HealthcareService setSpecialty(List<CodeableConcept> theSpecialty) { 1294 this.specialty = theSpecialty; 1295 return this; 1296 } 1297 1298 public boolean hasSpecialty() { 1299 if (this.specialty == null) 1300 return false; 1301 for (CodeableConcept item : this.specialty) 1302 if (!item.isEmpty()) 1303 return true; 1304 return false; 1305 } 1306 1307 public CodeableConcept addSpecialty() { //3 1308 CodeableConcept t = new CodeableConcept(); 1309 if (this.specialty == null) 1310 this.specialty = new ArrayList<CodeableConcept>(); 1311 this.specialty.add(t); 1312 return t; 1313 } 1314 1315 public HealthcareService addSpecialty(CodeableConcept t) { //3 1316 if (t == null) 1317 return this; 1318 if (this.specialty == null) 1319 this.specialty = new ArrayList<CodeableConcept>(); 1320 this.specialty.add(t); 1321 return this; 1322 } 1323 1324 /** 1325 * @return The first repetition of repeating field {@link #specialty}, creating it if it does not already exist 1326 */ 1327 public CodeableConcept getSpecialtyFirstRep() { 1328 if (getSpecialty().isEmpty()) { 1329 addSpecialty(); 1330 } 1331 return getSpecialty().get(0); 1332 } 1333 1334 /** 1335 * @return {@link #location} (The location(s) where this healthcare service may be provided.) 1336 */ 1337 public List<Reference> getLocation() { 1338 if (this.location == null) 1339 this.location = new ArrayList<Reference>(); 1340 return this.location; 1341 } 1342 1343 /** 1344 * @return Returns a reference to <code>this</code> for easy method chaining 1345 */ 1346 public HealthcareService setLocation(List<Reference> theLocation) { 1347 this.location = theLocation; 1348 return this; 1349 } 1350 1351 public boolean hasLocation() { 1352 if (this.location == null) 1353 return false; 1354 for (Reference item : this.location) 1355 if (!item.isEmpty()) 1356 return true; 1357 return false; 1358 } 1359 1360 public Reference addLocation() { //3 1361 Reference t = new Reference(); 1362 if (this.location == null) 1363 this.location = new ArrayList<Reference>(); 1364 this.location.add(t); 1365 return t; 1366 } 1367 1368 public HealthcareService addLocation(Reference t) { //3 1369 if (t == null) 1370 return this; 1371 if (this.location == null) 1372 this.location = new ArrayList<Reference>(); 1373 this.location.add(t); 1374 return this; 1375 } 1376 1377 /** 1378 * @return The first repetition of repeating field {@link #location}, creating it if it does not already exist 1379 */ 1380 public Reference getLocationFirstRep() { 1381 if (getLocation().isEmpty()) { 1382 addLocation(); 1383 } 1384 return getLocation().get(0); 1385 } 1386 1387 /** 1388 * @deprecated Use Reference#setResource(IBaseResource) instead 1389 */ 1390 @Deprecated 1391 public List<Location> getLocationTarget() { 1392 if (this.locationTarget == null) 1393 this.locationTarget = new ArrayList<Location>(); 1394 return this.locationTarget; 1395 } 1396 1397 /** 1398 * @deprecated Use Reference#setResource(IBaseResource) instead 1399 */ 1400 @Deprecated 1401 public Location addLocationTarget() { 1402 Location r = new Location(); 1403 if (this.locationTarget == null) 1404 this.locationTarget = new ArrayList<Location>(); 1405 this.locationTarget.add(r); 1406 return r; 1407 } 1408 1409 /** 1410 * @return {@link #name} (Further description of the service as it would be presented to a consumer while searching.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1411 */ 1412 public StringType getNameElement() { 1413 if (this.name == null) 1414 if (Configuration.errorOnAutoCreate()) 1415 throw new Error("Attempt to auto-create HealthcareService.name"); 1416 else if (Configuration.doAutoCreate()) 1417 this.name = new StringType(); // bb 1418 return this.name; 1419 } 1420 1421 public boolean hasNameElement() { 1422 return this.name != null && !this.name.isEmpty(); 1423 } 1424 1425 public boolean hasName() { 1426 return this.name != null && !this.name.isEmpty(); 1427 } 1428 1429 /** 1430 * @param value {@link #name} (Further description of the service as it would be presented to a consumer while searching.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1431 */ 1432 public HealthcareService setNameElement(StringType value) { 1433 this.name = value; 1434 return this; 1435 } 1436 1437 /** 1438 * @return Further description of the service as it would be presented to a consumer while searching. 1439 */ 1440 public String getName() { 1441 return this.name == null ? null : this.name.getValue(); 1442 } 1443 1444 /** 1445 * @param value Further description of the service as it would be presented to a consumer while searching. 1446 */ 1447 public HealthcareService setName(String value) { 1448 if (Utilities.noString(value)) 1449 this.name = null; 1450 else { 1451 if (this.name == null) 1452 this.name = new StringType(); 1453 this.name.setValue(value); 1454 } 1455 return this; 1456 } 1457 1458 /** 1459 * @return {@link #comment} (Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1460 */ 1461 public StringType getCommentElement() { 1462 if (this.comment == null) 1463 if (Configuration.errorOnAutoCreate()) 1464 throw new Error("Attempt to auto-create HealthcareService.comment"); 1465 else if (Configuration.doAutoCreate()) 1466 this.comment = new StringType(); // bb 1467 return this.comment; 1468 } 1469 1470 public boolean hasCommentElement() { 1471 return this.comment != null && !this.comment.isEmpty(); 1472 } 1473 1474 public boolean hasComment() { 1475 return this.comment != null && !this.comment.isEmpty(); 1476 } 1477 1478 /** 1479 * @param value {@link #comment} (Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.). This is the underlying object with id, value and extensions. The accessor "getComment" gives direct access to the value 1480 */ 1481 public HealthcareService setCommentElement(StringType value) { 1482 this.comment = value; 1483 return this; 1484 } 1485 1486 /** 1487 * @return Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName. 1488 */ 1489 public String getComment() { 1490 return this.comment == null ? null : this.comment.getValue(); 1491 } 1492 1493 /** 1494 * @param value Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName. 1495 */ 1496 public HealthcareService setComment(String value) { 1497 if (Utilities.noString(value)) 1498 this.comment = null; 1499 else { 1500 if (this.comment == null) 1501 this.comment = new StringType(); 1502 this.comment.setValue(value); 1503 } 1504 return this; 1505 } 1506 1507 /** 1508 * @return {@link #extraDetails} (Extra details about the service that can't be placed in the other fields.). This is the underlying object with id, value and extensions. The accessor "getExtraDetails" gives direct access to the value 1509 */ 1510 public StringType getExtraDetailsElement() { 1511 if (this.extraDetails == null) 1512 if (Configuration.errorOnAutoCreate()) 1513 throw new Error("Attempt to auto-create HealthcareService.extraDetails"); 1514 else if (Configuration.doAutoCreate()) 1515 this.extraDetails = new StringType(); // bb 1516 return this.extraDetails; 1517 } 1518 1519 public boolean hasExtraDetailsElement() { 1520 return this.extraDetails != null && !this.extraDetails.isEmpty(); 1521 } 1522 1523 public boolean hasExtraDetails() { 1524 return this.extraDetails != null && !this.extraDetails.isEmpty(); 1525 } 1526 1527 /** 1528 * @param value {@link #extraDetails} (Extra details about the service that can't be placed in the other fields.). This is the underlying object with id, value and extensions. The accessor "getExtraDetails" gives direct access to the value 1529 */ 1530 public HealthcareService setExtraDetailsElement(StringType value) { 1531 this.extraDetails = value; 1532 return this; 1533 } 1534 1535 /** 1536 * @return Extra details about the service that can't be placed in the other fields. 1537 */ 1538 public String getExtraDetails() { 1539 return this.extraDetails == null ? null : this.extraDetails.getValue(); 1540 } 1541 1542 /** 1543 * @param value Extra details about the service that can't be placed in the other fields. 1544 */ 1545 public HealthcareService setExtraDetails(String value) { 1546 if (Utilities.noString(value)) 1547 this.extraDetails = null; 1548 else { 1549 if (this.extraDetails == null) 1550 this.extraDetails = new StringType(); 1551 this.extraDetails.setValue(value); 1552 } 1553 return this; 1554 } 1555 1556 /** 1557 * @return {@link #photo} (If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.) 1558 */ 1559 public Attachment getPhoto() { 1560 if (this.photo == null) 1561 if (Configuration.errorOnAutoCreate()) 1562 throw new Error("Attempt to auto-create HealthcareService.photo"); 1563 else if (Configuration.doAutoCreate()) 1564 this.photo = new Attachment(); // cc 1565 return this.photo; 1566 } 1567 1568 public boolean hasPhoto() { 1569 return this.photo != null && !this.photo.isEmpty(); 1570 } 1571 1572 /** 1573 * @param value {@link #photo} (If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.) 1574 */ 1575 public HealthcareService setPhoto(Attachment value) { 1576 this.photo = value; 1577 return this; 1578 } 1579 1580 /** 1581 * @return {@link #telecom} (List of contacts related to this specific healthcare service.) 1582 */ 1583 public List<ContactPoint> getTelecom() { 1584 if (this.telecom == null) 1585 this.telecom = new ArrayList<ContactPoint>(); 1586 return this.telecom; 1587 } 1588 1589 /** 1590 * @return Returns a reference to <code>this</code> for easy method chaining 1591 */ 1592 public HealthcareService setTelecom(List<ContactPoint> theTelecom) { 1593 this.telecom = theTelecom; 1594 return this; 1595 } 1596 1597 public boolean hasTelecom() { 1598 if (this.telecom == null) 1599 return false; 1600 for (ContactPoint item : this.telecom) 1601 if (!item.isEmpty()) 1602 return true; 1603 return false; 1604 } 1605 1606 public ContactPoint addTelecom() { //3 1607 ContactPoint t = new ContactPoint(); 1608 if (this.telecom == null) 1609 this.telecom = new ArrayList<ContactPoint>(); 1610 this.telecom.add(t); 1611 return t; 1612 } 1613 1614 public HealthcareService addTelecom(ContactPoint t) { //3 1615 if (t == null) 1616 return this; 1617 if (this.telecom == null) 1618 this.telecom = new ArrayList<ContactPoint>(); 1619 this.telecom.add(t); 1620 return this; 1621 } 1622 1623 /** 1624 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 1625 */ 1626 public ContactPoint getTelecomFirstRep() { 1627 if (getTelecom().isEmpty()) { 1628 addTelecom(); 1629 } 1630 return getTelecom().get(0); 1631 } 1632 1633 /** 1634 * @return {@link #coverageArea} (The location(s) that this service is available to (not where the service is provided).) 1635 */ 1636 public List<Reference> getCoverageArea() { 1637 if (this.coverageArea == null) 1638 this.coverageArea = new ArrayList<Reference>(); 1639 return this.coverageArea; 1640 } 1641 1642 /** 1643 * @return Returns a reference to <code>this</code> for easy method chaining 1644 */ 1645 public HealthcareService setCoverageArea(List<Reference> theCoverageArea) { 1646 this.coverageArea = theCoverageArea; 1647 return this; 1648 } 1649 1650 public boolean hasCoverageArea() { 1651 if (this.coverageArea == null) 1652 return false; 1653 for (Reference item : this.coverageArea) 1654 if (!item.isEmpty()) 1655 return true; 1656 return false; 1657 } 1658 1659 public Reference addCoverageArea() { //3 1660 Reference t = new Reference(); 1661 if (this.coverageArea == null) 1662 this.coverageArea = new ArrayList<Reference>(); 1663 this.coverageArea.add(t); 1664 return t; 1665 } 1666 1667 public HealthcareService addCoverageArea(Reference t) { //3 1668 if (t == null) 1669 return this; 1670 if (this.coverageArea == null) 1671 this.coverageArea = new ArrayList<Reference>(); 1672 this.coverageArea.add(t); 1673 return this; 1674 } 1675 1676 /** 1677 * @return The first repetition of repeating field {@link #coverageArea}, creating it if it does not already exist 1678 */ 1679 public Reference getCoverageAreaFirstRep() { 1680 if (getCoverageArea().isEmpty()) { 1681 addCoverageArea(); 1682 } 1683 return getCoverageArea().get(0); 1684 } 1685 1686 /** 1687 * @deprecated Use Reference#setResource(IBaseResource) instead 1688 */ 1689 @Deprecated 1690 public List<Location> getCoverageAreaTarget() { 1691 if (this.coverageAreaTarget == null) 1692 this.coverageAreaTarget = new ArrayList<Location>(); 1693 return this.coverageAreaTarget; 1694 } 1695 1696 /** 1697 * @deprecated Use Reference#setResource(IBaseResource) instead 1698 */ 1699 @Deprecated 1700 public Location addCoverageAreaTarget() { 1701 Location r = new Location(); 1702 if (this.coverageAreaTarget == null) 1703 this.coverageAreaTarget = new ArrayList<Location>(); 1704 this.coverageAreaTarget.add(r); 1705 return r; 1706 } 1707 1708 /** 1709 * @return {@link #serviceProvisionCode} (The code(s) that detail the conditions under which the healthcare service is available/offered.) 1710 */ 1711 public List<CodeableConcept> getServiceProvisionCode() { 1712 if (this.serviceProvisionCode == null) 1713 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1714 return this.serviceProvisionCode; 1715 } 1716 1717 /** 1718 * @return Returns a reference to <code>this</code> for easy method chaining 1719 */ 1720 public HealthcareService setServiceProvisionCode(List<CodeableConcept> theServiceProvisionCode) { 1721 this.serviceProvisionCode = theServiceProvisionCode; 1722 return this; 1723 } 1724 1725 public boolean hasServiceProvisionCode() { 1726 if (this.serviceProvisionCode == null) 1727 return false; 1728 for (CodeableConcept item : this.serviceProvisionCode) 1729 if (!item.isEmpty()) 1730 return true; 1731 return false; 1732 } 1733 1734 public CodeableConcept addServiceProvisionCode() { //3 1735 CodeableConcept t = new CodeableConcept(); 1736 if (this.serviceProvisionCode == null) 1737 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1738 this.serviceProvisionCode.add(t); 1739 return t; 1740 } 1741 1742 public HealthcareService addServiceProvisionCode(CodeableConcept t) { //3 1743 if (t == null) 1744 return this; 1745 if (this.serviceProvisionCode == null) 1746 this.serviceProvisionCode = new ArrayList<CodeableConcept>(); 1747 this.serviceProvisionCode.add(t); 1748 return this; 1749 } 1750 1751 /** 1752 * @return The first repetition of repeating field {@link #serviceProvisionCode}, creating it if it does not already exist 1753 */ 1754 public CodeableConcept getServiceProvisionCodeFirstRep() { 1755 if (getServiceProvisionCode().isEmpty()) { 1756 addServiceProvisionCode(); 1757 } 1758 return getServiceProvisionCode().get(0); 1759 } 1760 1761 /** 1762 * @return {@link #eligibility} (Does this service have specific eligibility requirements that need to be met in order to use the service?) 1763 */ 1764 public CodeableConcept getEligibility() { 1765 if (this.eligibility == null) 1766 if (Configuration.errorOnAutoCreate()) 1767 throw new Error("Attempt to auto-create HealthcareService.eligibility"); 1768 else if (Configuration.doAutoCreate()) 1769 this.eligibility = new CodeableConcept(); // cc 1770 return this.eligibility; 1771 } 1772 1773 public boolean hasEligibility() { 1774 return this.eligibility != null && !this.eligibility.isEmpty(); 1775 } 1776 1777 /** 1778 * @param value {@link #eligibility} (Does this service have specific eligibility requirements that need to be met in order to use the service?) 1779 */ 1780 public HealthcareService setEligibility(CodeableConcept value) { 1781 this.eligibility = value; 1782 return this; 1783 } 1784 1785 /** 1786 * @return {@link #eligibilityNote} (Describes the eligibility conditions for the service.). This is the underlying object with id, value and extensions. The accessor "getEligibilityNote" gives direct access to the value 1787 */ 1788 public StringType getEligibilityNoteElement() { 1789 if (this.eligibilityNote == null) 1790 if (Configuration.errorOnAutoCreate()) 1791 throw new Error("Attempt to auto-create HealthcareService.eligibilityNote"); 1792 else if (Configuration.doAutoCreate()) 1793 this.eligibilityNote = new StringType(); // bb 1794 return this.eligibilityNote; 1795 } 1796 1797 public boolean hasEligibilityNoteElement() { 1798 return this.eligibilityNote != null && !this.eligibilityNote.isEmpty(); 1799 } 1800 1801 public boolean hasEligibilityNote() { 1802 return this.eligibilityNote != null && !this.eligibilityNote.isEmpty(); 1803 } 1804 1805 /** 1806 * @param value {@link #eligibilityNote} (Describes the eligibility conditions for the service.). This is the underlying object with id, value and extensions. The accessor "getEligibilityNote" gives direct access to the value 1807 */ 1808 public HealthcareService setEligibilityNoteElement(StringType value) { 1809 this.eligibilityNote = value; 1810 return this; 1811 } 1812 1813 /** 1814 * @return Describes the eligibility conditions for the service. 1815 */ 1816 public String getEligibilityNote() { 1817 return this.eligibilityNote == null ? null : this.eligibilityNote.getValue(); 1818 } 1819 1820 /** 1821 * @param value Describes the eligibility conditions for the service. 1822 */ 1823 public HealthcareService setEligibilityNote(String value) { 1824 if (Utilities.noString(value)) 1825 this.eligibilityNote = null; 1826 else { 1827 if (this.eligibilityNote == null) 1828 this.eligibilityNote = new StringType(); 1829 this.eligibilityNote.setValue(value); 1830 } 1831 return this; 1832 } 1833 1834 /** 1835 * @return {@link #programName} (Program Names that can be used to categorize the service.) 1836 */ 1837 public List<StringType> getProgramName() { 1838 if (this.programName == null) 1839 this.programName = new ArrayList<StringType>(); 1840 return this.programName; 1841 } 1842 1843 /** 1844 * @return Returns a reference to <code>this</code> for easy method chaining 1845 */ 1846 public HealthcareService setProgramName(List<StringType> theProgramName) { 1847 this.programName = theProgramName; 1848 return this; 1849 } 1850 1851 public boolean hasProgramName() { 1852 if (this.programName == null) 1853 return false; 1854 for (StringType item : this.programName) 1855 if (!item.isEmpty()) 1856 return true; 1857 return false; 1858 } 1859 1860 /** 1861 * @return {@link #programName} (Program Names that can be used to categorize the service.) 1862 */ 1863 public StringType addProgramNameElement() {//2 1864 StringType t = new StringType(); 1865 if (this.programName == null) 1866 this.programName = new ArrayList<StringType>(); 1867 this.programName.add(t); 1868 return t; 1869 } 1870 1871 /** 1872 * @param value {@link #programName} (Program Names that can be used to categorize the service.) 1873 */ 1874 public HealthcareService addProgramName(String value) { //1 1875 StringType t = new StringType(); 1876 t.setValue(value); 1877 if (this.programName == null) 1878 this.programName = new ArrayList<StringType>(); 1879 this.programName.add(t); 1880 return this; 1881 } 1882 1883 /** 1884 * @param value {@link #programName} (Program Names that can be used to categorize the service.) 1885 */ 1886 public boolean hasProgramName(String value) { 1887 if (this.programName == null) 1888 return false; 1889 for (StringType v : this.programName) 1890 if (v.getValue().equals(value)) // string 1891 return true; 1892 return false; 1893 } 1894 1895 /** 1896 * @return {@link #characteristic} (Collection of characteristics (attributes).) 1897 */ 1898 public List<CodeableConcept> getCharacteristic() { 1899 if (this.characteristic == null) 1900 this.characteristic = new ArrayList<CodeableConcept>(); 1901 return this.characteristic; 1902 } 1903 1904 /** 1905 * @return Returns a reference to <code>this</code> for easy method chaining 1906 */ 1907 public HealthcareService setCharacteristic(List<CodeableConcept> theCharacteristic) { 1908 this.characteristic = theCharacteristic; 1909 return this; 1910 } 1911 1912 public boolean hasCharacteristic() { 1913 if (this.characteristic == null) 1914 return false; 1915 for (CodeableConcept item : this.characteristic) 1916 if (!item.isEmpty()) 1917 return true; 1918 return false; 1919 } 1920 1921 public CodeableConcept addCharacteristic() { //3 1922 CodeableConcept t = new CodeableConcept(); 1923 if (this.characteristic == null) 1924 this.characteristic = new ArrayList<CodeableConcept>(); 1925 this.characteristic.add(t); 1926 return t; 1927 } 1928 1929 public HealthcareService addCharacteristic(CodeableConcept t) { //3 1930 if (t == null) 1931 return this; 1932 if (this.characteristic == null) 1933 this.characteristic = new ArrayList<CodeableConcept>(); 1934 this.characteristic.add(t); 1935 return this; 1936 } 1937 1938 /** 1939 * @return The first repetition of repeating field {@link #characteristic}, creating it if it does not already exist 1940 */ 1941 public CodeableConcept getCharacteristicFirstRep() { 1942 if (getCharacteristic().isEmpty()) { 1943 addCharacteristic(); 1944 } 1945 return getCharacteristic().get(0); 1946 } 1947 1948 /** 1949 * @return {@link #referralMethod} (Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.) 1950 */ 1951 public List<CodeableConcept> getReferralMethod() { 1952 if (this.referralMethod == null) 1953 this.referralMethod = new ArrayList<CodeableConcept>(); 1954 return this.referralMethod; 1955 } 1956 1957 /** 1958 * @return Returns a reference to <code>this</code> for easy method chaining 1959 */ 1960 public HealthcareService setReferralMethod(List<CodeableConcept> theReferralMethod) { 1961 this.referralMethod = theReferralMethod; 1962 return this; 1963 } 1964 1965 public boolean hasReferralMethod() { 1966 if (this.referralMethod == null) 1967 return false; 1968 for (CodeableConcept item : this.referralMethod) 1969 if (!item.isEmpty()) 1970 return true; 1971 return false; 1972 } 1973 1974 public CodeableConcept addReferralMethod() { //3 1975 CodeableConcept t = new CodeableConcept(); 1976 if (this.referralMethod == null) 1977 this.referralMethod = new ArrayList<CodeableConcept>(); 1978 this.referralMethod.add(t); 1979 return t; 1980 } 1981 1982 public HealthcareService addReferralMethod(CodeableConcept t) { //3 1983 if (t == null) 1984 return this; 1985 if (this.referralMethod == null) 1986 this.referralMethod = new ArrayList<CodeableConcept>(); 1987 this.referralMethod.add(t); 1988 return this; 1989 } 1990 1991 /** 1992 * @return The first repetition of repeating field {@link #referralMethod}, creating it if it does not already exist 1993 */ 1994 public CodeableConcept getReferralMethodFirstRep() { 1995 if (getReferralMethod().isEmpty()) { 1996 addReferralMethod(); 1997 } 1998 return getReferralMethod().get(0); 1999 } 2000 2001 /** 2002 * @return {@link #appointmentRequired} (Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.). This is the underlying object with id, value and extensions. The accessor "getAppointmentRequired" gives direct access to the value 2003 */ 2004 public BooleanType getAppointmentRequiredElement() { 2005 if (this.appointmentRequired == null) 2006 if (Configuration.errorOnAutoCreate()) 2007 throw new Error("Attempt to auto-create HealthcareService.appointmentRequired"); 2008 else if (Configuration.doAutoCreate()) 2009 this.appointmentRequired = new BooleanType(); // bb 2010 return this.appointmentRequired; 2011 } 2012 2013 public boolean hasAppointmentRequiredElement() { 2014 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 2015 } 2016 2017 public boolean hasAppointmentRequired() { 2018 return this.appointmentRequired != null && !this.appointmentRequired.isEmpty(); 2019 } 2020 2021 /** 2022 * @param value {@link #appointmentRequired} (Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.). This is the underlying object with id, value and extensions. The accessor "getAppointmentRequired" gives direct access to the value 2023 */ 2024 public HealthcareService setAppointmentRequiredElement(BooleanType value) { 2025 this.appointmentRequired = value; 2026 return this; 2027 } 2028 2029 /** 2030 * @return Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service. 2031 */ 2032 public boolean getAppointmentRequired() { 2033 return this.appointmentRequired == null || this.appointmentRequired.isEmpty() ? false : this.appointmentRequired.getValue(); 2034 } 2035 2036 /** 2037 * @param value Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service. 2038 */ 2039 public HealthcareService setAppointmentRequired(boolean value) { 2040 if (this.appointmentRequired == null) 2041 this.appointmentRequired = new BooleanType(); 2042 this.appointmentRequired.setValue(value); 2043 return this; 2044 } 2045 2046 /** 2047 * @return {@link #availableTime} (A collection of times that the Service Site is available.) 2048 */ 2049 public List<HealthcareServiceAvailableTimeComponent> getAvailableTime() { 2050 if (this.availableTime == null) 2051 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2052 return this.availableTime; 2053 } 2054 2055 /** 2056 * @return Returns a reference to <code>this</code> for easy method chaining 2057 */ 2058 public HealthcareService setAvailableTime(List<HealthcareServiceAvailableTimeComponent> theAvailableTime) { 2059 this.availableTime = theAvailableTime; 2060 return this; 2061 } 2062 2063 public boolean hasAvailableTime() { 2064 if (this.availableTime == null) 2065 return false; 2066 for (HealthcareServiceAvailableTimeComponent item : this.availableTime) 2067 if (!item.isEmpty()) 2068 return true; 2069 return false; 2070 } 2071 2072 public HealthcareServiceAvailableTimeComponent addAvailableTime() { //3 2073 HealthcareServiceAvailableTimeComponent t = new HealthcareServiceAvailableTimeComponent(); 2074 if (this.availableTime == null) 2075 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2076 this.availableTime.add(t); 2077 return t; 2078 } 2079 2080 public HealthcareService addAvailableTime(HealthcareServiceAvailableTimeComponent t) { //3 2081 if (t == null) 2082 return this; 2083 if (this.availableTime == null) 2084 this.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2085 this.availableTime.add(t); 2086 return this; 2087 } 2088 2089 /** 2090 * @return The first repetition of repeating field {@link #availableTime}, creating it if it does not already exist 2091 */ 2092 public HealthcareServiceAvailableTimeComponent getAvailableTimeFirstRep() { 2093 if (getAvailableTime().isEmpty()) { 2094 addAvailableTime(); 2095 } 2096 return getAvailableTime().get(0); 2097 } 2098 2099 /** 2100 * @return {@link #notAvailable} (The HealthcareService is not available during this period of time due to the provided reason.) 2101 */ 2102 public List<HealthcareServiceNotAvailableComponent> getNotAvailable() { 2103 if (this.notAvailable == null) 2104 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2105 return this.notAvailable; 2106 } 2107 2108 /** 2109 * @return Returns a reference to <code>this</code> for easy method chaining 2110 */ 2111 public HealthcareService setNotAvailable(List<HealthcareServiceNotAvailableComponent> theNotAvailable) { 2112 this.notAvailable = theNotAvailable; 2113 return this; 2114 } 2115 2116 public boolean hasNotAvailable() { 2117 if (this.notAvailable == null) 2118 return false; 2119 for (HealthcareServiceNotAvailableComponent item : this.notAvailable) 2120 if (!item.isEmpty()) 2121 return true; 2122 return false; 2123 } 2124 2125 public HealthcareServiceNotAvailableComponent addNotAvailable() { //3 2126 HealthcareServiceNotAvailableComponent t = new HealthcareServiceNotAvailableComponent(); 2127 if (this.notAvailable == null) 2128 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2129 this.notAvailable.add(t); 2130 return t; 2131 } 2132 2133 public HealthcareService addNotAvailable(HealthcareServiceNotAvailableComponent t) { //3 2134 if (t == null) 2135 return this; 2136 if (this.notAvailable == null) 2137 this.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2138 this.notAvailable.add(t); 2139 return this; 2140 } 2141 2142 /** 2143 * @return The first repetition of repeating field {@link #notAvailable}, creating it if it does not already exist 2144 */ 2145 public HealthcareServiceNotAvailableComponent getNotAvailableFirstRep() { 2146 if (getNotAvailable().isEmpty()) { 2147 addNotAvailable(); 2148 } 2149 return getNotAvailable().get(0); 2150 } 2151 2152 /** 2153 * @return {@link #availabilityExceptions} (A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.). This is the underlying object with id, value and extensions. The accessor "getAvailabilityExceptions" gives direct access to the value 2154 */ 2155 public StringType getAvailabilityExceptionsElement() { 2156 if (this.availabilityExceptions == null) 2157 if (Configuration.errorOnAutoCreate()) 2158 throw new Error("Attempt to auto-create HealthcareService.availabilityExceptions"); 2159 else if (Configuration.doAutoCreate()) 2160 this.availabilityExceptions = new StringType(); // bb 2161 return this.availabilityExceptions; 2162 } 2163 2164 public boolean hasAvailabilityExceptionsElement() { 2165 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2166 } 2167 2168 public boolean hasAvailabilityExceptions() { 2169 return this.availabilityExceptions != null && !this.availabilityExceptions.isEmpty(); 2170 } 2171 2172 /** 2173 * @param value {@link #availabilityExceptions} (A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.). This is the underlying object with id, value and extensions. The accessor "getAvailabilityExceptions" gives direct access to the value 2174 */ 2175 public HealthcareService setAvailabilityExceptionsElement(StringType value) { 2176 this.availabilityExceptions = value; 2177 return this; 2178 } 2179 2180 /** 2181 * @return A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 2182 */ 2183 public String getAvailabilityExceptions() { 2184 return this.availabilityExceptions == null ? null : this.availabilityExceptions.getValue(); 2185 } 2186 2187 /** 2188 * @param value A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times. 2189 */ 2190 public HealthcareService setAvailabilityExceptions(String value) { 2191 if (Utilities.noString(value)) 2192 this.availabilityExceptions = null; 2193 else { 2194 if (this.availabilityExceptions == null) 2195 this.availabilityExceptions = new StringType(); 2196 this.availabilityExceptions.setValue(value); 2197 } 2198 return this; 2199 } 2200 2201 /** 2202 * @return {@link #endpoint} (Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.) 2203 */ 2204 public List<Reference> getEndpoint() { 2205 if (this.endpoint == null) 2206 this.endpoint = new ArrayList<Reference>(); 2207 return this.endpoint; 2208 } 2209 2210 /** 2211 * @return Returns a reference to <code>this</code> for easy method chaining 2212 */ 2213 public HealthcareService setEndpoint(List<Reference> theEndpoint) { 2214 this.endpoint = theEndpoint; 2215 return this; 2216 } 2217 2218 public boolean hasEndpoint() { 2219 if (this.endpoint == null) 2220 return false; 2221 for (Reference item : this.endpoint) 2222 if (!item.isEmpty()) 2223 return true; 2224 return false; 2225 } 2226 2227 public Reference addEndpoint() { //3 2228 Reference t = new Reference(); 2229 if (this.endpoint == null) 2230 this.endpoint = new ArrayList<Reference>(); 2231 this.endpoint.add(t); 2232 return t; 2233 } 2234 2235 public HealthcareService addEndpoint(Reference t) { //3 2236 if (t == null) 2237 return this; 2238 if (this.endpoint == null) 2239 this.endpoint = new ArrayList<Reference>(); 2240 this.endpoint.add(t); 2241 return this; 2242 } 2243 2244 /** 2245 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 2246 */ 2247 public Reference getEndpointFirstRep() { 2248 if (getEndpoint().isEmpty()) { 2249 addEndpoint(); 2250 } 2251 return getEndpoint().get(0); 2252 } 2253 2254 /** 2255 * @deprecated Use Reference#setResource(IBaseResource) instead 2256 */ 2257 @Deprecated 2258 public List<Endpoint> getEndpointTarget() { 2259 if (this.endpointTarget == null) 2260 this.endpointTarget = new ArrayList<Endpoint>(); 2261 return this.endpointTarget; 2262 } 2263 2264 /** 2265 * @deprecated Use Reference#setResource(IBaseResource) instead 2266 */ 2267 @Deprecated 2268 public Endpoint addEndpointTarget() { 2269 Endpoint r = new Endpoint(); 2270 if (this.endpointTarget == null) 2271 this.endpointTarget = new ArrayList<Endpoint>(); 2272 this.endpointTarget.add(r); 2273 return r; 2274 } 2275 2276 protected void listChildren(List<Property> children) { 2277 super.listChildren(children); 2278 children.add(new Property("identifier", "Identifier", "External identifiers for this item.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2279 children.add(new Property("active", "boolean", "Whether this healthcareservice record is in active use.", 0, 1, active)); 2280 children.add(new Property("providedBy", "Reference(Organization)", "The organization that provides this healthcare service.", 0, 1, providedBy)); 2281 children.add(new Property("category", "CodeableConcept", "Identifies the broad category of service being performed or delivered.", 0, 1, category)); 2282 children.add(new Property("type", "CodeableConcept", "The specific type of service that may be delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type)); 2283 children.add(new Property("specialty", "CodeableConcept", "Collection of specialties handled by the service site. This is more of a medical term.", 0, java.lang.Integer.MAX_VALUE, specialty)); 2284 children.add(new Property("location", "Reference(Location)", "The location(s) where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location)); 2285 children.add(new Property("name", "string", "Further description of the service as it would be presented to a consumer while searching.", 0, 1, name)); 2286 children.add(new Property("comment", "string", "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 0, 1, comment)); 2287 children.add(new Property("extraDetails", "string", "Extra details about the service that can't be placed in the other fields.", 0, 1, extraDetails)); 2288 children.add(new Property("photo", "Attachment", "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 0, 1, photo)); 2289 children.add(new Property("telecom", "ContactPoint", "List of contacts related to this specific healthcare service.", 0, java.lang.Integer.MAX_VALUE, telecom)); 2290 children.add(new Property("coverageArea", "Reference(Location)", "The location(s) that this service is available to (not where the service is provided).", 0, java.lang.Integer.MAX_VALUE, coverageArea)); 2291 children.add(new Property("serviceProvisionCode", "CodeableConcept", "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, java.lang.Integer.MAX_VALUE, serviceProvisionCode)); 2292 children.add(new Property("eligibility", "CodeableConcept", "Does this service have specific eligibility requirements that need to be met in order to use the service?", 0, 1, eligibility)); 2293 children.add(new Property("eligibilityNote", "string", "Describes the eligibility conditions for the service.", 0, 1, eligibilityNote)); 2294 children.add(new Property("programName", "string", "Program Names that can be used to categorize the service.", 0, java.lang.Integer.MAX_VALUE, programName)); 2295 children.add(new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic)); 2296 children.add(new Property("referralMethod", "CodeableConcept", "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 0, java.lang.Integer.MAX_VALUE, referralMethod)); 2297 children.add(new Property("appointmentRequired", "boolean", "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 0, 1, appointmentRequired)); 2298 children.add(new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, java.lang.Integer.MAX_VALUE, availableTime)); 2299 children.add(new Property("notAvailable", "", "The HealthcareService is not available during this period of time due to the provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailable)); 2300 children.add(new Property("availabilityExceptions", "string", "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 0, 1, availabilityExceptions)); 2301 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 2302 } 2303 2304 @Override 2305 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2306 switch (_hash) { 2307 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External identifiers for this item.", 0, java.lang.Integer.MAX_VALUE, identifier); 2308 case -1422950650: /*active*/ return new Property("active", "boolean", "Whether this healthcareservice record is in active use.", 0, 1, active); 2309 case 205136282: /*providedBy*/ return new Property("providedBy", "Reference(Organization)", "The organization that provides this healthcare service.", 0, 1, providedBy); 2310 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Identifies the broad category of service being performed or delivered.", 0, 1, category); 2311 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "The specific type of service that may be delivered or performed.", 0, java.lang.Integer.MAX_VALUE, type); 2312 case -1694759682: /*specialty*/ return new Property("specialty", "CodeableConcept", "Collection of specialties handled by the service site. This is more of a medical term.", 0, java.lang.Integer.MAX_VALUE, specialty); 2313 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The location(s) where this healthcare service may be provided.", 0, java.lang.Integer.MAX_VALUE, location); 2314 case 3373707: /*name*/ return new Property("name", "string", "Further description of the service as it would be presented to a consumer while searching.", 0, 1, name); 2315 case 950398559: /*comment*/ return new Property("comment", "string", "Any additional description of the service and/or any specific issues not covered by the other attributes, which can be displayed as further detail under the serviceName.", 0, 1, comment); 2316 case -1469168622: /*extraDetails*/ return new Property("extraDetails", "string", "Extra details about the service that can't be placed in the other fields.", 0, 1, extraDetails); 2317 case 106642994: /*photo*/ return new Property("photo", "Attachment", "If there is a photo/symbol associated with this HealthcareService, it may be included here to facilitate quick identification of the service in a list.", 0, 1, photo); 2318 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "List of contacts related to this specific healthcare service.", 0, java.lang.Integer.MAX_VALUE, telecom); 2319 case -1532328299: /*coverageArea*/ return new Property("coverageArea", "Reference(Location)", "The location(s) that this service is available to (not where the service is provided).", 0, java.lang.Integer.MAX_VALUE, coverageArea); 2320 case 1504575405: /*serviceProvisionCode*/ return new Property("serviceProvisionCode", "CodeableConcept", "The code(s) that detail the conditions under which the healthcare service is available/offered.", 0, java.lang.Integer.MAX_VALUE, serviceProvisionCode); 2321 case -930847859: /*eligibility*/ return new Property("eligibility", "CodeableConcept", "Does this service have specific eligibility requirements that need to be met in order to use the service?", 0, 1, eligibility); 2322 case 1635973407: /*eligibilityNote*/ return new Property("eligibilityNote", "string", "Describes the eligibility conditions for the service.", 0, 1, eligibilityNote); 2323 case 1010379567: /*programName*/ return new Property("programName", "string", "Program Names that can be used to categorize the service.", 0, java.lang.Integer.MAX_VALUE, programName); 2324 case 366313883: /*characteristic*/ return new Property("characteristic", "CodeableConcept", "Collection of characteristics (attributes).", 0, java.lang.Integer.MAX_VALUE, characteristic); 2325 case -2092740898: /*referralMethod*/ return new Property("referralMethod", "CodeableConcept", "Ways that the service accepts referrals, if this is not provided then it is implied that no referral is required.", 0, java.lang.Integer.MAX_VALUE, referralMethod); 2326 case 427220062: /*appointmentRequired*/ return new Property("appointmentRequired", "boolean", "Indicates whether or not a prospective consumer will require an appointment for a particular service at a site to be provided by the Organization. Indicates if an appointment is required for access to this service.", 0, 1, appointmentRequired); 2327 case 1873069366: /*availableTime*/ return new Property("availableTime", "", "A collection of times that the Service Site is available.", 0, java.lang.Integer.MAX_VALUE, availableTime); 2328 case -629572298: /*notAvailable*/ return new Property("notAvailable", "", "The HealthcareService is not available during this period of time due to the provided reason.", 0, java.lang.Integer.MAX_VALUE, notAvailable); 2329 case -1149143617: /*availabilityExceptions*/ return new Property("availabilityExceptions", "string", "A description of site availability exceptions, e.g. public holiday availability. Succinctly describing all possible exceptions to normal site availability as details in the available Times and not available Times.", 0, 1, availabilityExceptions); 2330 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the specific healthcare services defined at this resource.", 0, java.lang.Integer.MAX_VALUE, endpoint); 2331 default: return super.getNamedProperty(_hash, _name, _checkValid); 2332 } 2333 2334 } 2335 2336 @Override 2337 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2338 switch (hash) { 2339 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2340 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 2341 case 205136282: /*providedBy*/ return this.providedBy == null ? new Base[0] : new Base[] {this.providedBy}; // Reference 2342 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2343 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 2344 case -1694759682: /*specialty*/ return this.specialty == null ? new Base[0] : this.specialty.toArray(new Base[this.specialty.size()]); // CodeableConcept 2345 case 1901043637: /*location*/ return this.location == null ? new Base[0] : this.location.toArray(new Base[this.location.size()]); // Reference 2346 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2347 case 950398559: /*comment*/ return this.comment == null ? new Base[0] : new Base[] {this.comment}; // StringType 2348 case -1469168622: /*extraDetails*/ return this.extraDetails == null ? new Base[0] : new Base[] {this.extraDetails}; // StringType 2349 case 106642994: /*photo*/ return this.photo == null ? new Base[0] : new Base[] {this.photo}; // Attachment 2350 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 2351 case -1532328299: /*coverageArea*/ return this.coverageArea == null ? new Base[0] : this.coverageArea.toArray(new Base[this.coverageArea.size()]); // Reference 2352 case 1504575405: /*serviceProvisionCode*/ return this.serviceProvisionCode == null ? new Base[0] : this.serviceProvisionCode.toArray(new Base[this.serviceProvisionCode.size()]); // CodeableConcept 2353 case -930847859: /*eligibility*/ return this.eligibility == null ? new Base[0] : new Base[] {this.eligibility}; // CodeableConcept 2354 case 1635973407: /*eligibilityNote*/ return this.eligibilityNote == null ? new Base[0] : new Base[] {this.eligibilityNote}; // StringType 2355 case 1010379567: /*programName*/ return this.programName == null ? new Base[0] : this.programName.toArray(new Base[this.programName.size()]); // StringType 2356 case 366313883: /*characteristic*/ return this.characteristic == null ? new Base[0] : this.characteristic.toArray(new Base[this.characteristic.size()]); // CodeableConcept 2357 case -2092740898: /*referralMethod*/ return this.referralMethod == null ? new Base[0] : this.referralMethod.toArray(new Base[this.referralMethod.size()]); // CodeableConcept 2358 case 427220062: /*appointmentRequired*/ return this.appointmentRequired == null ? new Base[0] : new Base[] {this.appointmentRequired}; // BooleanType 2359 case 1873069366: /*availableTime*/ return this.availableTime == null ? new Base[0] : this.availableTime.toArray(new Base[this.availableTime.size()]); // HealthcareServiceAvailableTimeComponent 2360 case -629572298: /*notAvailable*/ return this.notAvailable == null ? new Base[0] : this.notAvailable.toArray(new Base[this.notAvailable.size()]); // HealthcareServiceNotAvailableComponent 2361 case -1149143617: /*availabilityExceptions*/ return this.availabilityExceptions == null ? new Base[0] : new Base[] {this.availabilityExceptions}; // StringType 2362 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2363 default: return super.getProperty(hash, name, checkValid); 2364 } 2365 2366 } 2367 2368 @Override 2369 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2370 switch (hash) { 2371 case -1618432855: // identifier 2372 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2373 return value; 2374 case -1422950650: // active 2375 this.active = castToBoolean(value); // BooleanType 2376 return value; 2377 case 205136282: // providedBy 2378 this.providedBy = castToReference(value); // Reference 2379 return value; 2380 case 50511102: // category 2381 this.category = castToCodeableConcept(value); // CodeableConcept 2382 return value; 2383 case 3575610: // type 2384 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 2385 return value; 2386 case -1694759682: // specialty 2387 this.getSpecialty().add(castToCodeableConcept(value)); // CodeableConcept 2388 return value; 2389 case 1901043637: // location 2390 this.getLocation().add(castToReference(value)); // Reference 2391 return value; 2392 case 3373707: // name 2393 this.name = castToString(value); // StringType 2394 return value; 2395 case 950398559: // comment 2396 this.comment = castToString(value); // StringType 2397 return value; 2398 case -1469168622: // extraDetails 2399 this.extraDetails = castToString(value); // StringType 2400 return value; 2401 case 106642994: // photo 2402 this.photo = castToAttachment(value); // Attachment 2403 return value; 2404 case -1429363305: // telecom 2405 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 2406 return value; 2407 case -1532328299: // coverageArea 2408 this.getCoverageArea().add(castToReference(value)); // Reference 2409 return value; 2410 case 1504575405: // serviceProvisionCode 2411 this.getServiceProvisionCode().add(castToCodeableConcept(value)); // CodeableConcept 2412 return value; 2413 case -930847859: // eligibility 2414 this.eligibility = castToCodeableConcept(value); // CodeableConcept 2415 return value; 2416 case 1635973407: // eligibilityNote 2417 this.eligibilityNote = castToString(value); // StringType 2418 return value; 2419 case 1010379567: // programName 2420 this.getProgramName().add(castToString(value)); // StringType 2421 return value; 2422 case 366313883: // characteristic 2423 this.getCharacteristic().add(castToCodeableConcept(value)); // CodeableConcept 2424 return value; 2425 case -2092740898: // referralMethod 2426 this.getReferralMethod().add(castToCodeableConcept(value)); // CodeableConcept 2427 return value; 2428 case 427220062: // appointmentRequired 2429 this.appointmentRequired = castToBoolean(value); // BooleanType 2430 return value; 2431 case 1873069366: // availableTime 2432 this.getAvailableTime().add((HealthcareServiceAvailableTimeComponent) value); // HealthcareServiceAvailableTimeComponent 2433 return value; 2434 case -629572298: // notAvailable 2435 this.getNotAvailable().add((HealthcareServiceNotAvailableComponent) value); // HealthcareServiceNotAvailableComponent 2436 return value; 2437 case -1149143617: // availabilityExceptions 2438 this.availabilityExceptions = castToString(value); // StringType 2439 return value; 2440 case 1741102485: // endpoint 2441 this.getEndpoint().add(castToReference(value)); // Reference 2442 return value; 2443 default: return super.setProperty(hash, name, value); 2444 } 2445 2446 } 2447 2448 @Override 2449 public Base setProperty(String name, Base value) throws FHIRException { 2450 if (name.equals("identifier")) { 2451 this.getIdentifier().add(castToIdentifier(value)); 2452 } else if (name.equals("active")) { 2453 this.active = castToBoolean(value); // BooleanType 2454 } else if (name.equals("providedBy")) { 2455 this.providedBy = castToReference(value); // Reference 2456 } else if (name.equals("category")) { 2457 this.category = castToCodeableConcept(value); // CodeableConcept 2458 } else if (name.equals("type")) { 2459 this.getType().add(castToCodeableConcept(value)); 2460 } else if (name.equals("specialty")) { 2461 this.getSpecialty().add(castToCodeableConcept(value)); 2462 } else if (name.equals("location")) { 2463 this.getLocation().add(castToReference(value)); 2464 } else if (name.equals("name")) { 2465 this.name = castToString(value); // StringType 2466 } else if (name.equals("comment")) { 2467 this.comment = castToString(value); // StringType 2468 } else if (name.equals("extraDetails")) { 2469 this.extraDetails = castToString(value); // StringType 2470 } else if (name.equals("photo")) { 2471 this.photo = castToAttachment(value); // Attachment 2472 } else if (name.equals("telecom")) { 2473 this.getTelecom().add(castToContactPoint(value)); 2474 } else if (name.equals("coverageArea")) { 2475 this.getCoverageArea().add(castToReference(value)); 2476 } else if (name.equals("serviceProvisionCode")) { 2477 this.getServiceProvisionCode().add(castToCodeableConcept(value)); 2478 } else if (name.equals("eligibility")) { 2479 this.eligibility = castToCodeableConcept(value); // CodeableConcept 2480 } else if (name.equals("eligibilityNote")) { 2481 this.eligibilityNote = castToString(value); // StringType 2482 } else if (name.equals("programName")) { 2483 this.getProgramName().add(castToString(value)); 2484 } else if (name.equals("characteristic")) { 2485 this.getCharacteristic().add(castToCodeableConcept(value)); 2486 } else if (name.equals("referralMethod")) { 2487 this.getReferralMethod().add(castToCodeableConcept(value)); 2488 } else if (name.equals("appointmentRequired")) { 2489 this.appointmentRequired = castToBoolean(value); // BooleanType 2490 } else if (name.equals("availableTime")) { 2491 this.getAvailableTime().add((HealthcareServiceAvailableTimeComponent) value); 2492 } else if (name.equals("notAvailable")) { 2493 this.getNotAvailable().add((HealthcareServiceNotAvailableComponent) value); 2494 } else if (name.equals("availabilityExceptions")) { 2495 this.availabilityExceptions = castToString(value); // StringType 2496 } else if (name.equals("endpoint")) { 2497 this.getEndpoint().add(castToReference(value)); 2498 } else 2499 return super.setProperty(name, value); 2500 return value; 2501 } 2502 2503 @Override 2504 public Base makeProperty(int hash, String name) throws FHIRException { 2505 switch (hash) { 2506 case -1618432855: return addIdentifier(); 2507 case -1422950650: return getActiveElement(); 2508 case 205136282: return getProvidedBy(); 2509 case 50511102: return getCategory(); 2510 case 3575610: return addType(); 2511 case -1694759682: return addSpecialty(); 2512 case 1901043637: return addLocation(); 2513 case 3373707: return getNameElement(); 2514 case 950398559: return getCommentElement(); 2515 case -1469168622: return getExtraDetailsElement(); 2516 case 106642994: return getPhoto(); 2517 case -1429363305: return addTelecom(); 2518 case -1532328299: return addCoverageArea(); 2519 case 1504575405: return addServiceProvisionCode(); 2520 case -930847859: return getEligibility(); 2521 case 1635973407: return getEligibilityNoteElement(); 2522 case 1010379567: return addProgramNameElement(); 2523 case 366313883: return addCharacteristic(); 2524 case -2092740898: return addReferralMethod(); 2525 case 427220062: return getAppointmentRequiredElement(); 2526 case 1873069366: return addAvailableTime(); 2527 case -629572298: return addNotAvailable(); 2528 case -1149143617: return getAvailabilityExceptionsElement(); 2529 case 1741102485: return addEndpoint(); 2530 default: return super.makeProperty(hash, name); 2531 } 2532 2533 } 2534 2535 @Override 2536 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2537 switch (hash) { 2538 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2539 case -1422950650: /*active*/ return new String[] {"boolean"}; 2540 case 205136282: /*providedBy*/ return new String[] {"Reference"}; 2541 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2542 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2543 case -1694759682: /*specialty*/ return new String[] {"CodeableConcept"}; 2544 case 1901043637: /*location*/ return new String[] {"Reference"}; 2545 case 3373707: /*name*/ return new String[] {"string"}; 2546 case 950398559: /*comment*/ return new String[] {"string"}; 2547 case -1469168622: /*extraDetails*/ return new String[] {"string"}; 2548 case 106642994: /*photo*/ return new String[] {"Attachment"}; 2549 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 2550 case -1532328299: /*coverageArea*/ return new String[] {"Reference"}; 2551 case 1504575405: /*serviceProvisionCode*/ return new String[] {"CodeableConcept"}; 2552 case -930847859: /*eligibility*/ return new String[] {"CodeableConcept"}; 2553 case 1635973407: /*eligibilityNote*/ return new String[] {"string"}; 2554 case 1010379567: /*programName*/ return new String[] {"string"}; 2555 case 366313883: /*characteristic*/ return new String[] {"CodeableConcept"}; 2556 case -2092740898: /*referralMethod*/ return new String[] {"CodeableConcept"}; 2557 case 427220062: /*appointmentRequired*/ return new String[] {"boolean"}; 2558 case 1873069366: /*availableTime*/ return new String[] {}; 2559 case -629572298: /*notAvailable*/ return new String[] {}; 2560 case -1149143617: /*availabilityExceptions*/ return new String[] {"string"}; 2561 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 2562 default: return super.getTypesForProperty(hash, name); 2563 } 2564 2565 } 2566 2567 @Override 2568 public Base addChild(String name) throws FHIRException { 2569 if (name.equals("identifier")) { 2570 return addIdentifier(); 2571 } 2572 else if (name.equals("active")) { 2573 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.active"); 2574 } 2575 else if (name.equals("providedBy")) { 2576 this.providedBy = new Reference(); 2577 return this.providedBy; 2578 } 2579 else if (name.equals("category")) { 2580 this.category = new CodeableConcept(); 2581 return this.category; 2582 } 2583 else if (name.equals("type")) { 2584 return addType(); 2585 } 2586 else if (name.equals("specialty")) { 2587 return addSpecialty(); 2588 } 2589 else if (name.equals("location")) { 2590 return addLocation(); 2591 } 2592 else if (name.equals("name")) { 2593 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.name"); 2594 } 2595 else if (name.equals("comment")) { 2596 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.comment"); 2597 } 2598 else if (name.equals("extraDetails")) { 2599 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.extraDetails"); 2600 } 2601 else if (name.equals("photo")) { 2602 this.photo = new Attachment(); 2603 return this.photo; 2604 } 2605 else if (name.equals("telecom")) { 2606 return addTelecom(); 2607 } 2608 else if (name.equals("coverageArea")) { 2609 return addCoverageArea(); 2610 } 2611 else if (name.equals("serviceProvisionCode")) { 2612 return addServiceProvisionCode(); 2613 } 2614 else if (name.equals("eligibility")) { 2615 this.eligibility = new CodeableConcept(); 2616 return this.eligibility; 2617 } 2618 else if (name.equals("eligibilityNote")) { 2619 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.eligibilityNote"); 2620 } 2621 else if (name.equals("programName")) { 2622 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.programName"); 2623 } 2624 else if (name.equals("characteristic")) { 2625 return addCharacteristic(); 2626 } 2627 else if (name.equals("referralMethod")) { 2628 return addReferralMethod(); 2629 } 2630 else if (name.equals("appointmentRequired")) { 2631 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.appointmentRequired"); 2632 } 2633 else if (name.equals("availableTime")) { 2634 return addAvailableTime(); 2635 } 2636 else if (name.equals("notAvailable")) { 2637 return addNotAvailable(); 2638 } 2639 else if (name.equals("availabilityExceptions")) { 2640 throw new FHIRException("Cannot call addChild on a singleton property HealthcareService.availabilityExceptions"); 2641 } 2642 else if (name.equals("endpoint")) { 2643 return addEndpoint(); 2644 } 2645 else 2646 return super.addChild(name); 2647 } 2648 2649 public String fhirType() { 2650 return "HealthcareService"; 2651 2652 } 2653 2654 public HealthcareService copy() { 2655 HealthcareService dst = new HealthcareService(); 2656 copyValues(dst); 2657 if (identifier != null) { 2658 dst.identifier = new ArrayList<Identifier>(); 2659 for (Identifier i : identifier) 2660 dst.identifier.add(i.copy()); 2661 }; 2662 dst.active = active == null ? null : active.copy(); 2663 dst.providedBy = providedBy == null ? null : providedBy.copy(); 2664 dst.category = category == null ? null : category.copy(); 2665 if (type != null) { 2666 dst.type = new ArrayList<CodeableConcept>(); 2667 for (CodeableConcept i : type) 2668 dst.type.add(i.copy()); 2669 }; 2670 if (specialty != null) { 2671 dst.specialty = new ArrayList<CodeableConcept>(); 2672 for (CodeableConcept i : specialty) 2673 dst.specialty.add(i.copy()); 2674 }; 2675 if (location != null) { 2676 dst.location = new ArrayList<Reference>(); 2677 for (Reference i : location) 2678 dst.location.add(i.copy()); 2679 }; 2680 dst.name = name == null ? null : name.copy(); 2681 dst.comment = comment == null ? null : comment.copy(); 2682 dst.extraDetails = extraDetails == null ? null : extraDetails.copy(); 2683 dst.photo = photo == null ? null : photo.copy(); 2684 if (telecom != null) { 2685 dst.telecom = new ArrayList<ContactPoint>(); 2686 for (ContactPoint i : telecom) 2687 dst.telecom.add(i.copy()); 2688 }; 2689 if (coverageArea != null) { 2690 dst.coverageArea = new ArrayList<Reference>(); 2691 for (Reference i : coverageArea) 2692 dst.coverageArea.add(i.copy()); 2693 }; 2694 if (serviceProvisionCode != null) { 2695 dst.serviceProvisionCode = new ArrayList<CodeableConcept>(); 2696 for (CodeableConcept i : serviceProvisionCode) 2697 dst.serviceProvisionCode.add(i.copy()); 2698 }; 2699 dst.eligibility = eligibility == null ? null : eligibility.copy(); 2700 dst.eligibilityNote = eligibilityNote == null ? null : eligibilityNote.copy(); 2701 if (programName != null) { 2702 dst.programName = new ArrayList<StringType>(); 2703 for (StringType i : programName) 2704 dst.programName.add(i.copy()); 2705 }; 2706 if (characteristic != null) { 2707 dst.characteristic = new ArrayList<CodeableConcept>(); 2708 for (CodeableConcept i : characteristic) 2709 dst.characteristic.add(i.copy()); 2710 }; 2711 if (referralMethod != null) { 2712 dst.referralMethod = new ArrayList<CodeableConcept>(); 2713 for (CodeableConcept i : referralMethod) 2714 dst.referralMethod.add(i.copy()); 2715 }; 2716 dst.appointmentRequired = appointmentRequired == null ? null : appointmentRequired.copy(); 2717 if (availableTime != null) { 2718 dst.availableTime = new ArrayList<HealthcareServiceAvailableTimeComponent>(); 2719 for (HealthcareServiceAvailableTimeComponent i : availableTime) 2720 dst.availableTime.add(i.copy()); 2721 }; 2722 if (notAvailable != null) { 2723 dst.notAvailable = new ArrayList<HealthcareServiceNotAvailableComponent>(); 2724 for (HealthcareServiceNotAvailableComponent i : notAvailable) 2725 dst.notAvailable.add(i.copy()); 2726 }; 2727 dst.availabilityExceptions = availabilityExceptions == null ? null : availabilityExceptions.copy(); 2728 if (endpoint != null) { 2729 dst.endpoint = new ArrayList<Reference>(); 2730 for (Reference i : endpoint) 2731 dst.endpoint.add(i.copy()); 2732 }; 2733 return dst; 2734 } 2735 2736 protected HealthcareService typedCopy() { 2737 return copy(); 2738 } 2739 2740 @Override 2741 public boolean equalsDeep(Base other_) { 2742 if (!super.equalsDeep(other_)) 2743 return false; 2744 if (!(other_ instanceof HealthcareService)) 2745 return false; 2746 HealthcareService o = (HealthcareService) other_; 2747 return compareDeep(identifier, o.identifier, true) && compareDeep(active, o.active, true) && compareDeep(providedBy, o.providedBy, true) 2748 && compareDeep(category, o.category, true) && compareDeep(type, o.type, true) && compareDeep(specialty, o.specialty, true) 2749 && compareDeep(location, o.location, true) && compareDeep(name, o.name, true) && compareDeep(comment, o.comment, true) 2750 && compareDeep(extraDetails, o.extraDetails, true) && compareDeep(photo, o.photo, true) && compareDeep(telecom, o.telecom, true) 2751 && compareDeep(coverageArea, o.coverageArea, true) && compareDeep(serviceProvisionCode, o.serviceProvisionCode, true) 2752 && compareDeep(eligibility, o.eligibility, true) && compareDeep(eligibilityNote, o.eligibilityNote, true) 2753 && compareDeep(programName, o.programName, true) && compareDeep(characteristic, o.characteristic, true) 2754 && compareDeep(referralMethod, o.referralMethod, true) && compareDeep(appointmentRequired, o.appointmentRequired, true) 2755 && compareDeep(availableTime, o.availableTime, true) && compareDeep(notAvailable, o.notAvailable, true) 2756 && compareDeep(availabilityExceptions, o.availabilityExceptions, true) && compareDeep(endpoint, o.endpoint, true) 2757 ; 2758 } 2759 2760 @Override 2761 public boolean equalsShallow(Base other_) { 2762 if (!super.equalsShallow(other_)) 2763 return false; 2764 if (!(other_ instanceof HealthcareService)) 2765 return false; 2766 HealthcareService o = (HealthcareService) other_; 2767 return compareValues(active, o.active, true) && compareValues(name, o.name, true) && compareValues(comment, o.comment, true) 2768 && compareValues(extraDetails, o.extraDetails, true) && compareValues(eligibilityNote, o.eligibilityNote, true) 2769 && compareValues(programName, o.programName, true) && compareValues(appointmentRequired, o.appointmentRequired, true) 2770 && compareValues(availabilityExceptions, o.availabilityExceptions, true); 2771 } 2772 2773 public boolean isEmpty() { 2774 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, active, providedBy 2775 , category, type, specialty, location, name, comment, extraDetails, photo, telecom 2776 , coverageArea, serviceProvisionCode, eligibility, eligibilityNote, programName, characteristic 2777 , referralMethod, appointmentRequired, availableTime, notAvailable, availabilityExceptions 2778 , endpoint); 2779 } 2780 2781 @Override 2782 public ResourceType getResourceType() { 2783 return ResourceType.HealthcareService; 2784 } 2785 2786 /** 2787 * Search parameter: <b>identifier</b> 2788 * <p> 2789 * Description: <b>External identifiers for this item</b><br> 2790 * Type: <b>token</b><br> 2791 * Path: <b>HealthcareService.identifier</b><br> 2792 * </p> 2793 */ 2794 @SearchParamDefinition(name="identifier", path="HealthcareService.identifier", description="External identifiers for this item", type="token" ) 2795 public static final String SP_IDENTIFIER = "identifier"; 2796 /** 2797 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2798 * <p> 2799 * Description: <b>External identifiers for this item</b><br> 2800 * Type: <b>token</b><br> 2801 * Path: <b>HealthcareService.identifier</b><br> 2802 * </p> 2803 */ 2804 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2805 2806 /** 2807 * Search parameter: <b>endpoint</b> 2808 * <p> 2809 * Description: <b>Technical endpoints providing access to services operated for the location</b><br> 2810 * Type: <b>reference</b><br> 2811 * Path: <b>HealthcareService.endpoint</b><br> 2812 * </p> 2813 */ 2814 @SearchParamDefinition(name="endpoint", path="HealthcareService.endpoint", description="Technical endpoints providing access to services operated for the location", type="reference", target={Endpoint.class } ) 2815 public static final String SP_ENDPOINT = "endpoint"; 2816 /** 2817 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 2818 * <p> 2819 * Description: <b>Technical endpoints providing access to services operated for the location</b><br> 2820 * Type: <b>reference</b><br> 2821 * Path: <b>HealthcareService.endpoint</b><br> 2822 * </p> 2823 */ 2824 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 2825 2826/** 2827 * Constant for fluent queries to be used to add include statements. Specifies 2828 * the path value of "<b>HealthcareService:endpoint</b>". 2829 */ 2830 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("HealthcareService:endpoint").toLocked(); 2831 2832 /** 2833 * Search parameter: <b>organization</b> 2834 * <p> 2835 * Description: <b>The organization that provides this Healthcare Service</b><br> 2836 * Type: <b>reference</b><br> 2837 * Path: <b>HealthcareService.providedBy</b><br> 2838 * </p> 2839 */ 2840 @SearchParamDefinition(name="organization", path="HealthcareService.providedBy", description="The organization that provides this Healthcare Service", type="reference", target={Organization.class } ) 2841 public static final String SP_ORGANIZATION = "organization"; 2842 /** 2843 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 2844 * <p> 2845 * Description: <b>The organization that provides this Healthcare Service</b><br> 2846 * Type: <b>reference</b><br> 2847 * Path: <b>HealthcareService.providedBy</b><br> 2848 * </p> 2849 */ 2850 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2851 2852/** 2853 * Constant for fluent queries to be used to add include statements. Specifies 2854 * the path value of "<b>HealthcareService:organization</b>". 2855 */ 2856 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("HealthcareService:organization").toLocked(); 2857 2858 /** 2859 * Search parameter: <b>name</b> 2860 * <p> 2861 * Description: <b>A portion of the Healthcare service name</b><br> 2862 * Type: <b>string</b><br> 2863 * Path: <b>HealthcareService.name</b><br> 2864 * </p> 2865 */ 2866 @SearchParamDefinition(name="name", path="HealthcareService.name", description="A portion of the Healthcare service name", type="string" ) 2867 public static final String SP_NAME = "name"; 2868 /** 2869 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2870 * <p> 2871 * Description: <b>A portion of the Healthcare service name</b><br> 2872 * Type: <b>string</b><br> 2873 * Path: <b>HealthcareService.name</b><br> 2874 * </p> 2875 */ 2876 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2877 2878 /** 2879 * Search parameter: <b>programname</b> 2880 * <p> 2881 * Description: <b>One of the Program Names serviced by this HealthcareService</b><br> 2882 * Type: <b>string</b><br> 2883 * Path: <b>HealthcareService.programName</b><br> 2884 * </p> 2885 */ 2886 @SearchParamDefinition(name="programname", path="HealthcareService.programName", description="One of the Program Names serviced by this HealthcareService", type="string" ) 2887 public static final String SP_PROGRAMNAME = "programname"; 2888 /** 2889 * <b>Fluent Client</b> search parameter constant for <b>programname</b> 2890 * <p> 2891 * Description: <b>One of the Program Names serviced by this HealthcareService</b><br> 2892 * Type: <b>string</b><br> 2893 * Path: <b>HealthcareService.programName</b><br> 2894 * </p> 2895 */ 2896 public static final ca.uhn.fhir.rest.gclient.StringClientParam PROGRAMNAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PROGRAMNAME); 2897 2898 /** 2899 * Search parameter: <b>active</b> 2900 * <p> 2901 * Description: <b>The Healthcare Service is currently marked as active</b><br> 2902 * Type: <b>token</b><br> 2903 * Path: <b>HealthcareService.active</b><br> 2904 * </p> 2905 */ 2906 @SearchParamDefinition(name="active", path="HealthcareService.active", description="The Healthcare Service is currently marked as active", type="token" ) 2907 public static final String SP_ACTIVE = "active"; 2908 /** 2909 * <b>Fluent Client</b> search parameter constant for <b>active</b> 2910 * <p> 2911 * Description: <b>The Healthcare Service is currently marked as active</b><br> 2912 * Type: <b>token</b><br> 2913 * Path: <b>HealthcareService.active</b><br> 2914 * </p> 2915 */ 2916 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACTIVE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACTIVE); 2917 2918 /** 2919 * Search parameter: <b>location</b> 2920 * <p> 2921 * Description: <b>The location of the Healthcare Service</b><br> 2922 * Type: <b>reference</b><br> 2923 * Path: <b>HealthcareService.location</b><br> 2924 * </p> 2925 */ 2926 @SearchParamDefinition(name="location", path="HealthcareService.location", description="The location of the Healthcare Service", type="reference", target={Location.class } ) 2927 public static final String SP_LOCATION = "location"; 2928 /** 2929 * <b>Fluent Client</b> search parameter constant for <b>location</b> 2930 * <p> 2931 * Description: <b>The location of the Healthcare Service</b><br> 2932 * Type: <b>reference</b><br> 2933 * Path: <b>HealthcareService.location</b><br> 2934 * </p> 2935 */ 2936 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 2937 2938/** 2939 * Constant for fluent queries to be used to add include statements. Specifies 2940 * the path value of "<b>HealthcareService:location</b>". 2941 */ 2942 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("HealthcareService:location").toLocked(); 2943 2944 /** 2945 * Search parameter: <b>category</b> 2946 * <p> 2947 * Description: <b>Service Category of the Healthcare Service</b><br> 2948 * Type: <b>token</b><br> 2949 * Path: <b>HealthcareService.category</b><br> 2950 * </p> 2951 */ 2952 @SearchParamDefinition(name="category", path="HealthcareService.category", description="Service Category of the Healthcare Service", type="token" ) 2953 public static final String SP_CATEGORY = "category"; 2954 /** 2955 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2956 * <p> 2957 * Description: <b>Service Category of the Healthcare Service</b><br> 2958 * Type: <b>token</b><br> 2959 * Path: <b>HealthcareService.category</b><br> 2960 * </p> 2961 */ 2962 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2963 2964 /** 2965 * Search parameter: <b>type</b> 2966 * <p> 2967 * Description: <b>The type of service provided by this healthcare service</b><br> 2968 * Type: <b>token</b><br> 2969 * Path: <b>HealthcareService.type</b><br> 2970 * </p> 2971 */ 2972 @SearchParamDefinition(name="type", path="HealthcareService.type", description="The type of service provided by this healthcare service", type="token" ) 2973 public static final String SP_TYPE = "type"; 2974 /** 2975 * <b>Fluent Client</b> search parameter constant for <b>type</b> 2976 * <p> 2977 * Description: <b>The type of service provided by this healthcare service</b><br> 2978 * Type: <b>token</b><br> 2979 * Path: <b>HealthcareService.type</b><br> 2980 * </p> 2981 */ 2982 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 2983 2984 /** 2985 * Search parameter: <b>characteristic</b> 2986 * <p> 2987 * Description: <b>One of the HealthcareService's characteristics</b><br> 2988 * Type: <b>token</b><br> 2989 * Path: <b>HealthcareService.characteristic</b><br> 2990 * </p> 2991 */ 2992 @SearchParamDefinition(name="characteristic", path="HealthcareService.characteristic", description="One of the HealthcareService's characteristics", type="token" ) 2993 public static final String SP_CHARACTERISTIC = "characteristic"; 2994 /** 2995 * <b>Fluent Client</b> search parameter constant for <b>characteristic</b> 2996 * <p> 2997 * Description: <b>One of the HealthcareService's characteristics</b><br> 2998 * Type: <b>token</b><br> 2999 * Path: <b>HealthcareService.characteristic</b><br> 3000 * </p> 3001 */ 3002 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CHARACTERISTIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CHARACTERISTIC); 3003 3004 3005}