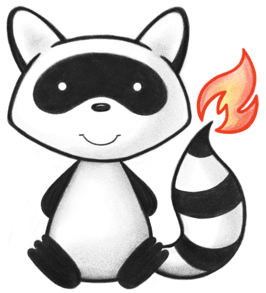
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.List; 037 038import org.hl7.fhir.exceptions.FHIRException; 039import org.hl7.fhir.instance.model.api.ICompositeType; 040import org.hl7.fhir.instance.model.api.IPrimitiveType; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.DatatypeDef; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.util.DatatypeUtil; 047/** 048 * A human's name with the ability to identify parts and usage. 049 */ 050@DatatypeDef(name="HumanName") 051public class HumanName extends Type implements ICompositeType { 052 053 public enum NameUse { 054 /** 055 * Known as/conventional/the one you normally use 056 */ 057 USUAL, 058 /** 059 * The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called "legal name". 060 */ 061 OFFICIAL, 062 /** 063 * A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations. 064 */ 065 TEMP, 066 /** 067 * A name that is used to address the person in an informal manner, but is not part of their formal or usual name 068 */ 069 NICKNAME, 070 /** 071 * Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons) 072 */ 073 ANONYMOUS, 074 /** 075 * This name is no longer in use (or was never correct, but retained for records) 076 */ 077 OLD, 078 /** 079 * A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name 080 */ 081 MAIDEN, 082 /** 083 * added to help the parsers with the generic types 084 */ 085 NULL; 086 public static NameUse fromCode(String codeString) throws FHIRException { 087 if (codeString == null || "".equals(codeString)) 088 return null; 089 if ("usual".equals(codeString)) 090 return USUAL; 091 if ("official".equals(codeString)) 092 return OFFICIAL; 093 if ("temp".equals(codeString)) 094 return TEMP; 095 if ("nickname".equals(codeString)) 096 return NICKNAME; 097 if ("anonymous".equals(codeString)) 098 return ANONYMOUS; 099 if ("old".equals(codeString)) 100 return OLD; 101 if ("maiden".equals(codeString)) 102 return MAIDEN; 103 if (Configuration.isAcceptInvalidEnums()) 104 return null; 105 else 106 throw new FHIRException("Unknown NameUse code '"+codeString+"'"); 107 } 108 public String toCode() { 109 switch (this) { 110 case USUAL: return "usual"; 111 case OFFICIAL: return "official"; 112 case TEMP: return "temp"; 113 case NICKNAME: return "nickname"; 114 case ANONYMOUS: return "anonymous"; 115 case OLD: return "old"; 116 case MAIDEN: return "maiden"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getSystem() { 122 switch (this) { 123 case USUAL: return "http://hl7.org/fhir/name-use"; 124 case OFFICIAL: return "http://hl7.org/fhir/name-use"; 125 case TEMP: return "http://hl7.org/fhir/name-use"; 126 case NICKNAME: return "http://hl7.org/fhir/name-use"; 127 case ANONYMOUS: return "http://hl7.org/fhir/name-use"; 128 case OLD: return "http://hl7.org/fhir/name-use"; 129 case MAIDEN: return "http://hl7.org/fhir/name-use"; 130 case NULL: return null; 131 default: return "?"; 132 } 133 } 134 public String getDefinition() { 135 switch (this) { 136 case USUAL: return "Known as/conventional/the one you normally use"; 137 case OFFICIAL: return "The formal name as registered in an official (government) registry, but which name might not be commonly used. May be called \"legal name\"."; 138 case TEMP: return "A temporary name. Name.period can provide more detailed information. This may also be used for temporary names assigned at birth or in emergency situations."; 139 case NICKNAME: return "A name that is used to address the person in an informal manner, but is not part of their formal or usual name"; 140 case ANONYMOUS: return "Anonymous assigned name, alias, or pseudonym (used to protect a person's identity for privacy reasons)"; 141 case OLD: return "This name is no longer in use (or was never correct, but retained for records)"; 142 case MAIDEN: return "A name used prior to changing name because of marriage. This name use is for use by applications that collect and store names that were used prior to a marriage. Marriage naming customs vary greatly around the world, and are constantly changing. This term is not gender specific. The use of this term does not imply any particular history for a person's name"; 143 case NULL: return null; 144 default: return "?"; 145 } 146 } 147 public String getDisplay() { 148 switch (this) { 149 case USUAL: return "Usual"; 150 case OFFICIAL: return "Official"; 151 case TEMP: return "Temp"; 152 case NICKNAME: return "Nickname"; 153 case ANONYMOUS: return "Anonymous"; 154 case OLD: return "Old"; 155 case MAIDEN: return "Name changed for Marriage"; 156 case NULL: return null; 157 default: return "?"; 158 } 159 } 160 } 161 162 public static class NameUseEnumFactory implements EnumFactory<NameUse> { 163 public NameUse fromCode(String codeString) throws IllegalArgumentException { 164 if (codeString == null || "".equals(codeString)) 165 if (codeString == null || "".equals(codeString)) 166 return null; 167 if ("usual".equals(codeString)) 168 return NameUse.USUAL; 169 if ("official".equals(codeString)) 170 return NameUse.OFFICIAL; 171 if ("temp".equals(codeString)) 172 return NameUse.TEMP; 173 if ("nickname".equals(codeString)) 174 return NameUse.NICKNAME; 175 if ("anonymous".equals(codeString)) 176 return NameUse.ANONYMOUS; 177 if ("old".equals(codeString)) 178 return NameUse.OLD; 179 if ("maiden".equals(codeString)) 180 return NameUse.MAIDEN; 181 throw new IllegalArgumentException("Unknown NameUse code '"+codeString+"'"); 182 } 183 public Enumeration<NameUse> fromType(PrimitiveType<?> code) throws FHIRException { 184 if (code == null) 185 return null; 186 if (code.isEmpty()) 187 return new Enumeration<NameUse>(this); 188 String codeString = code.asStringValue(); 189 if (codeString == null || "".equals(codeString)) 190 return null; 191 if ("usual".equals(codeString)) 192 return new Enumeration<NameUse>(this, NameUse.USUAL); 193 if ("official".equals(codeString)) 194 return new Enumeration<NameUse>(this, NameUse.OFFICIAL); 195 if ("temp".equals(codeString)) 196 return new Enumeration<NameUse>(this, NameUse.TEMP); 197 if ("nickname".equals(codeString)) 198 return new Enumeration<NameUse>(this, NameUse.NICKNAME); 199 if ("anonymous".equals(codeString)) 200 return new Enumeration<NameUse>(this, NameUse.ANONYMOUS); 201 if ("old".equals(codeString)) 202 return new Enumeration<NameUse>(this, NameUse.OLD); 203 if ("maiden".equals(codeString)) 204 return new Enumeration<NameUse>(this, NameUse.MAIDEN); 205 throw new FHIRException("Unknown NameUse code '"+codeString+"'"); 206 } 207 public String toCode(NameUse code) { 208 if (code == NameUse.NULL) 209 return null; 210 if (code == NameUse.USUAL) 211 return "usual"; 212 if (code == NameUse.OFFICIAL) 213 return "official"; 214 if (code == NameUse.TEMP) 215 return "temp"; 216 if (code == NameUse.NICKNAME) 217 return "nickname"; 218 if (code == NameUse.ANONYMOUS) 219 return "anonymous"; 220 if (code == NameUse.OLD) 221 return "old"; 222 if (code == NameUse.MAIDEN) 223 return "maiden"; 224 return "?"; 225 } 226 public String toSystem(NameUse code) { 227 return code.getSystem(); 228 } 229 } 230 231 /** 232 * Identifies the purpose for this name. 233 */ 234 @Child(name = "use", type = {CodeType.class}, order=0, min=0, max=1, modifier=true, summary=true) 235 @Description(shortDefinition="usual | official | temp | nickname | anonymous | old | maiden", formalDefinition="Identifies the purpose for this name." ) 236 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/name-use") 237 protected Enumeration<NameUse> use; 238 239 /** 240 * A full text representation of the name. 241 */ 242 @Child(name = "text", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=true) 243 @Description(shortDefinition="Text representation of the full name", formalDefinition="A full text representation of the name." ) 244 protected StringType text; 245 246 /** 247 * The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 248 */ 249 @Child(name = "family", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 250 @Description(shortDefinition="Family name (often called 'Surname')", formalDefinition="The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father." ) 251 protected StringType family; 252 253 /** 254 * Given name. 255 */ 256 @Child(name = "given", type = {StringType.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 257 @Description(shortDefinition="Given names (not always 'first'). Includes middle names", formalDefinition="Given name." ) 258 protected List<StringType> given; 259 260 /** 261 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name. 262 */ 263 @Child(name = "prefix", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 264 @Description(shortDefinition="Parts that come before the name", formalDefinition="Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name." ) 265 protected List<StringType> prefix; 266 267 /** 268 * Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name. 269 */ 270 @Child(name = "suffix", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 271 @Description(shortDefinition="Parts that come after the name", formalDefinition="Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name." ) 272 protected List<StringType> suffix; 273 274 /** 275 * Indicates the period of time when this name was valid for the named person. 276 */ 277 @Child(name = "period", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 278 @Description(shortDefinition="Time period when name was/is in use", formalDefinition="Indicates the period of time when this name was valid for the named person." ) 279 protected Period period; 280 281 private static final long serialVersionUID = -507469160L; 282 283 /** 284 * Constructor 285 */ 286 public HumanName() { 287 super(); 288 } 289 290 /** 291 * @return {@link #use} (Identifies the purpose for this name.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 292 */ 293 public Enumeration<NameUse> getUseElement() { 294 if (this.use == null) 295 if (Configuration.errorOnAutoCreate()) 296 throw new Error("Attempt to auto-create HumanName.use"); 297 else if (Configuration.doAutoCreate()) 298 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); // bb 299 return this.use; 300 } 301 302 public boolean hasUseElement() { 303 return this.use != null && !this.use.isEmpty(); 304 } 305 306 public boolean hasUse() { 307 return this.use != null && !this.use.isEmpty(); 308 } 309 310 /** 311 * @param value {@link #use} (Identifies the purpose for this name.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 312 */ 313 public HumanName setUseElement(Enumeration<NameUse> value) { 314 this.use = value; 315 return this; 316 } 317 318 /** 319 * @return Identifies the purpose for this name. 320 */ 321 public NameUse getUse() { 322 return this.use == null ? null : this.use.getValue(); 323 } 324 325 /** 326 * @param value Identifies the purpose for this name. 327 */ 328 public HumanName setUse(NameUse value) { 329 if (value == null) 330 this.use = null; 331 else { 332 if (this.use == null) 333 this.use = new Enumeration<NameUse>(new NameUseEnumFactory()); 334 this.use.setValue(value); 335 } 336 return this; 337 } 338 339 /** 340 * @return {@link #text} (A full text representation of the name.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 341 */ 342 public StringType getTextElement() { 343 if (this.text == null) 344 if (Configuration.errorOnAutoCreate()) 345 throw new Error("Attempt to auto-create HumanName.text"); 346 else if (Configuration.doAutoCreate()) 347 this.text = new StringType(); // bb 348 return this.text; 349 } 350 351 public boolean hasTextElement() { 352 return this.text != null && !this.text.isEmpty(); 353 } 354 355 public boolean hasText() { 356 return this.text != null && !this.text.isEmpty(); 357 } 358 359 /** 360 * @param value {@link #text} (A full text representation of the name.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 361 */ 362 public HumanName setTextElement(StringType value) { 363 this.text = value; 364 return this; 365 } 366 367 /** 368 * @return A full text representation of the name. 369 */ 370 public String getText() { 371 return this.text == null ? null : this.text.getValue(); 372 } 373 374 /** 375 * @param value A full text representation of the name. 376 */ 377 public HumanName setText(String value) { 378 if (Utilities.noString(value)) 379 this.text = null; 380 else { 381 if (this.text == null) 382 this.text = new StringType(); 383 this.text.setValue(value); 384 } 385 return this; 386 } 387 388 /** 389 * @return {@link #family} (The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.). This is the underlying object with id, value and extensions. The accessor "getFamily" gives direct access to the value 390 */ 391 public StringType getFamilyElement() { 392 if (this.family == null) 393 if (Configuration.errorOnAutoCreate()) 394 throw new Error("Attempt to auto-create HumanName.family"); 395 else if (Configuration.doAutoCreate()) 396 this.family = new StringType(); // bb 397 return this.family; 398 } 399 400 public boolean hasFamilyElement() { 401 return this.family != null && !this.family.isEmpty(); 402 } 403 404 public boolean hasFamily() { 405 return this.family != null && !this.family.isEmpty(); 406 } 407 408 /** 409 * @param value {@link #family} (The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.). This is the underlying object with id, value and extensions. The accessor "getFamily" gives direct access to the value 410 */ 411 public HumanName setFamilyElement(StringType value) { 412 this.family = value; 413 return this; 414 } 415 416 /** 417 * @return The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 418 */ 419 public String getFamily() { 420 return this.family == null ? null : this.family.getValue(); 421 } 422 423 /** 424 * @param value The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father. 425 */ 426 public HumanName setFamily(String value) { 427 if (Utilities.noString(value)) 428 this.family = null; 429 else { 430 if (this.family == null) 431 this.family = new StringType(); 432 this.family.setValue(value); 433 } 434 return this; 435 } 436 437 /** 438 * @return {@link #given} (Given name.) 439 */ 440 public List<StringType> getGiven() { 441 if (this.given == null) 442 this.given = new ArrayList<StringType>(); 443 return this.given; 444 } 445 446 /** 447 * @return Returns a reference to <code>this</code> for easy method chaining 448 */ 449 public HumanName setGiven(List<StringType> theGiven) { 450 this.given = theGiven; 451 return this; 452 } 453 454 public boolean hasGiven() { 455 if (this.given == null) 456 return false; 457 for (StringType item : this.given) 458 if (!item.isEmpty()) 459 return true; 460 return false; 461 } 462 463 /** 464 * @return {@link #given} (Given name.) 465 */ 466 public StringType addGivenElement() {//2 467 StringType t = new StringType(); 468 if (this.given == null) 469 this.given = new ArrayList<StringType>(); 470 this.given.add(t); 471 return t; 472 } 473 474 /** 475 * @param value {@link #given} (Given name.) 476 */ 477 public HumanName addGiven(String value) { //1 478 StringType t = new StringType(); 479 t.setValue(value); 480 if (this.given == null) 481 this.given = new ArrayList<StringType>(); 482 this.given.add(t); 483 return this; 484 } 485 486 /** 487 * @param value {@link #given} (Given name.) 488 */ 489 public boolean hasGiven(String value) { 490 if (this.given == null) 491 return false; 492 for (StringType v : this.given) 493 if (v.getValue().equals(value)) // string 494 return true; 495 return false; 496 } 497 498 /** 499 * @return {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 500 */ 501 public List<StringType> getPrefix() { 502 if (this.prefix == null) 503 this.prefix = new ArrayList<StringType>(); 504 return this.prefix; 505 } 506 507 /** 508 * @return Returns a reference to <code>this</code> for easy method chaining 509 */ 510 public HumanName setPrefix(List<StringType> thePrefix) { 511 this.prefix = thePrefix; 512 return this; 513 } 514 515 public boolean hasPrefix() { 516 if (this.prefix == null) 517 return false; 518 for (StringType item : this.prefix) 519 if (!item.isEmpty()) 520 return true; 521 return false; 522 } 523 524 /** 525 * @return {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 526 */ 527 public StringType addPrefixElement() {//2 528 StringType t = new StringType(); 529 if (this.prefix == null) 530 this.prefix = new ArrayList<StringType>(); 531 this.prefix.add(t); 532 return t; 533 } 534 535 /** 536 * @param value {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 537 */ 538 public HumanName addPrefix(String value) { //1 539 StringType t = new StringType(); 540 t.setValue(value); 541 if (this.prefix == null) 542 this.prefix = new ArrayList<StringType>(); 543 this.prefix.add(t); 544 return this; 545 } 546 547 /** 548 * @param value {@link #prefix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.) 549 */ 550 public boolean hasPrefix(String value) { 551 if (this.prefix == null) 552 return false; 553 for (StringType v : this.prefix) 554 if (v.getValue().equals(value)) // string 555 return true; 556 return false; 557 } 558 559 /** 560 * @return {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 561 */ 562 public List<StringType> getSuffix() { 563 if (this.suffix == null) 564 this.suffix = new ArrayList<StringType>(); 565 return this.suffix; 566 } 567 568 /** 569 * @return Returns a reference to <code>this</code> for easy method chaining 570 */ 571 public HumanName setSuffix(List<StringType> theSuffix) { 572 this.suffix = theSuffix; 573 return this; 574 } 575 576 public boolean hasSuffix() { 577 if (this.suffix == null) 578 return false; 579 for (StringType item : this.suffix) 580 if (!item.isEmpty()) 581 return true; 582 return false; 583 } 584 585 /** 586 * @return {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 587 */ 588 public StringType addSuffixElement() {//2 589 StringType t = new StringType(); 590 if (this.suffix == null) 591 this.suffix = new ArrayList<StringType>(); 592 this.suffix.add(t); 593 return t; 594 } 595 596 /** 597 * @param value {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 598 */ 599 public HumanName addSuffix(String value) { //1 600 StringType t = new StringType(); 601 t.setValue(value); 602 if (this.suffix == null) 603 this.suffix = new ArrayList<StringType>(); 604 this.suffix.add(t); 605 return this; 606 } 607 608 /** 609 * @param value {@link #suffix} (Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.) 610 */ 611 public boolean hasSuffix(String value) { 612 if (this.suffix == null) 613 return false; 614 for (StringType v : this.suffix) 615 if (v.getValue().equals(value)) // string 616 return true; 617 return false; 618 } 619 620 /** 621 * @return {@link #period} (Indicates the period of time when this name was valid for the named person.) 622 */ 623 public Period getPeriod() { 624 if (this.period == null) 625 if (Configuration.errorOnAutoCreate()) 626 throw new Error("Attempt to auto-create HumanName.period"); 627 else if (Configuration.doAutoCreate()) 628 this.period = new Period(); // cc 629 return this.period; 630 } 631 632 public boolean hasPeriod() { 633 return this.period != null && !this.period.isEmpty(); 634 } 635 636 /** 637 * @param value {@link #period} (Indicates the period of time when this name was valid for the named person.) 638 */ 639 public HumanName setPeriod(Period value) { 640 this.period = value; 641 return this; 642 } 643 644 /** 645 /** 646 * Returns all repetitions of {@link #getGiven() given name} as a space separated string 647 * 648 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 649 */ 650 public String getGivenAsSingleString() { 651 return joinStringsSpaceSeparated(getGiven()); 652 } 653 654 /** 655 * Returns all repetitions of {@link #getPrefix() prefix name} as a space separated string 656 * 657 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 658 */ 659 public String getPrefixAsSingleString() { 660 return joinStringsSpaceSeparated(getPrefix()); 661 } 662 663 /** 664 * Returns all repetitions of {@link #getSuffix() suffix} as a space separated string 665 * 666 * @see DatatypeUtil#joinStringsSpaceSeparated(List) 667 */ 668 public String getSuffixAsSingleString() { 669 return joinStringsSpaceSeparated(getSuffix()); 670 } 671 672 /** 673 * <p>Returns the {@link #getTextElement() text} element value if it is not null.</p> 674 675 * <p>If the {@link #getTextElement() text} element value is null, returns all the components of the name (prefix, 676 * given, family, suffix) as a single string with a single spaced string separating each part. </p> 677 * 678 * @return the human name as a single string 679 */ 680 public String getNameAsSingleString() { 681 if (hasText()) { 682 return getText().toString(); 683 } 684 685 List<StringType> nameParts = new ArrayList<StringType>(); 686 nameParts.addAll(getPrefix()); 687 nameParts.addAll(getGiven()); 688 if (hasFamilyElement()) { 689 nameParts.add(getFamilyElement()); 690 } 691 nameParts.addAll(getSuffix()); 692 if (nameParts.size() > 0) { 693 return joinStringsSpaceSeparated(nameParts); 694 } else { 695 return getTextElement().getValue(); 696 } 697 } 698 699 /** 700 * Joins a list of strings with a single space (' ') between each string 701 * 702 * TODO: replace with call to ca.uhn.fhir.util.DatatypeUtil.joinStringsSpaceSeparated when HAPI upgrades to 1.4 703 */ 704 private static String joinStringsSpaceSeparated(List<? extends IPrimitiveType<String>> theStrings) { 705 StringBuilder stringBuilder = new StringBuilder(); 706 for (IPrimitiveType<String> string : theStrings) { 707 if (string.isEmpty()) { 708 continue; 709 } 710 if (stringBuilder.length() > 0) { 711 stringBuilder.append(' '); 712 } 713 stringBuilder.append(string.getValue()); 714 } 715 return stringBuilder.toString(); 716 } 717 protected void listChildren(List<Property> children) { 718 super.listChildren(children); 719 children.add(new Property("use", "code", "Identifies the purpose for this name.", 0, 1, use)); 720 children.add(new Property("text", "string", "A full text representation of the name.", 0, 1, text)); 721 children.add(new Property("family", "string", "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 0, 1, family)); 722 children.add(new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given)); 723 children.add(new Property("prefix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 0, java.lang.Integer.MAX_VALUE, prefix)); 724 children.add(new Property("suffix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 0, java.lang.Integer.MAX_VALUE, suffix)); 725 children.add(new Property("period", "Period", "Indicates the period of time when this name was valid for the named person.", 0, 1, period)); 726 } 727 728 @Override 729 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 730 switch (_hash) { 731 case 116103: /*use*/ return new Property("use", "code", "Identifies the purpose for this name.", 0, 1, use); 732 case 3556653: /*text*/ return new Property("text", "string", "A full text representation of the name.", 0, 1, text); 733 case -1281860764: /*family*/ return new Property("family", "string", "The part of a name that links to the genealogy. In some cultures (e.g. Eritrea) the family name of a son is the first name of his father.", 0, 1, family); 734 case 98367357: /*given*/ return new Property("given", "string", "Given name.", 0, java.lang.Integer.MAX_VALUE, given); 735 case -980110702: /*prefix*/ return new Property("prefix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the start of the name.", 0, java.lang.Integer.MAX_VALUE, prefix); 736 case -891422895: /*suffix*/ return new Property("suffix", "string", "Part of the name that is acquired as a title due to academic, legal, employment or nobility status, etc. and that appears at the end of the name.", 0, java.lang.Integer.MAX_VALUE, suffix); 737 case -991726143: /*period*/ return new Property("period", "Period", "Indicates the period of time when this name was valid for the named person.", 0, 1, period); 738 default: return super.getNamedProperty(_hash, _name, _checkValid); 739 } 740 741 } 742 743 @Override 744 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 745 switch (hash) { 746 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<NameUse> 747 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 748 case -1281860764: /*family*/ return this.family == null ? new Base[0] : new Base[] {this.family}; // StringType 749 case 98367357: /*given*/ return this.given == null ? new Base[0] : this.given.toArray(new Base[this.given.size()]); // StringType 750 case -980110702: /*prefix*/ return this.prefix == null ? new Base[0] : this.prefix.toArray(new Base[this.prefix.size()]); // StringType 751 case -891422895: /*suffix*/ return this.suffix == null ? new Base[0] : this.suffix.toArray(new Base[this.suffix.size()]); // StringType 752 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 753 default: return super.getProperty(hash, name, checkValid); 754 } 755 756 } 757 758 @Override 759 public Base setProperty(int hash, String name, Base value) throws FHIRException { 760 switch (hash) { 761 case 116103: // use 762 value = new NameUseEnumFactory().fromType(castToCode(value)); 763 this.use = (Enumeration) value; // Enumeration<NameUse> 764 return value; 765 case 3556653: // text 766 this.text = castToString(value); // StringType 767 return value; 768 case -1281860764: // family 769 this.family = castToString(value); // StringType 770 return value; 771 case 98367357: // given 772 this.getGiven().add(castToString(value)); // StringType 773 return value; 774 case -980110702: // prefix 775 this.getPrefix().add(castToString(value)); // StringType 776 return value; 777 case -891422895: // suffix 778 this.getSuffix().add(castToString(value)); // StringType 779 return value; 780 case -991726143: // period 781 this.period = castToPeriod(value); // Period 782 return value; 783 default: return super.setProperty(hash, name, value); 784 } 785 786 } 787 788 @Override 789 public Base setProperty(String name, Base value) throws FHIRException { 790 if (name.equals("use")) { 791 value = new NameUseEnumFactory().fromType(castToCode(value)); 792 this.use = (Enumeration) value; // Enumeration<NameUse> 793 } else if (name.equals("text")) { 794 this.text = castToString(value); // StringType 795 } else if (name.equals("family")) { 796 this.family = castToString(value); // StringType 797 } else if (name.equals("given")) { 798 this.getGiven().add(castToString(value)); 799 } else if (name.equals("prefix")) { 800 this.getPrefix().add(castToString(value)); 801 } else if (name.equals("suffix")) { 802 this.getSuffix().add(castToString(value)); 803 } else if (name.equals("period")) { 804 this.period = castToPeriod(value); // Period 805 } else 806 return super.setProperty(name, value); 807 return value; 808 } 809 810 @Override 811 public Base makeProperty(int hash, String name) throws FHIRException { 812 switch (hash) { 813 case 116103: return getUseElement(); 814 case 3556653: return getTextElement(); 815 case -1281860764: return getFamilyElement(); 816 case 98367357: return addGivenElement(); 817 case -980110702: return addPrefixElement(); 818 case -891422895: return addSuffixElement(); 819 case -991726143: return getPeriod(); 820 default: return super.makeProperty(hash, name); 821 } 822 823 } 824 825 @Override 826 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 827 switch (hash) { 828 case 116103: /*use*/ return new String[] {"code"}; 829 case 3556653: /*text*/ return new String[] {"string"}; 830 case -1281860764: /*family*/ return new String[] {"string"}; 831 case 98367357: /*given*/ return new String[] {"string"}; 832 case -980110702: /*prefix*/ return new String[] {"string"}; 833 case -891422895: /*suffix*/ return new String[] {"string"}; 834 case -991726143: /*period*/ return new String[] {"Period"}; 835 default: return super.getTypesForProperty(hash, name); 836 } 837 838 } 839 840 @Override 841 public Base addChild(String name) throws FHIRException { 842 if (name.equals("use")) { 843 throw new FHIRException("Cannot call addChild on a singleton property HumanName.use"); 844 } 845 else if (name.equals("text")) { 846 throw new FHIRException("Cannot call addChild on a singleton property HumanName.text"); 847 } 848 else if (name.equals("family")) { 849 throw new FHIRException("Cannot call addChild on a singleton property HumanName.family"); 850 } 851 else if (name.equals("given")) { 852 throw new FHIRException("Cannot call addChild on a singleton property HumanName.given"); 853 } 854 else if (name.equals("prefix")) { 855 throw new FHIRException("Cannot call addChild on a singleton property HumanName.prefix"); 856 } 857 else if (name.equals("suffix")) { 858 throw new FHIRException("Cannot call addChild on a singleton property HumanName.suffix"); 859 } 860 else if (name.equals("period")) { 861 this.period = new Period(); 862 return this.period; 863 } 864 else 865 return super.addChild(name); 866 } 867 868 public String fhirType() { 869 return "HumanName"; 870 871 } 872 873 public HumanName copy() { 874 HumanName dst = new HumanName(); 875 copyValues(dst); 876 dst.use = use == null ? null : use.copy(); 877 dst.text = text == null ? null : text.copy(); 878 dst.family = family == null ? null : family.copy(); 879 if (given != null) { 880 dst.given = new ArrayList<StringType>(); 881 for (StringType i : given) 882 dst.given.add(i.copy()); 883 }; 884 if (prefix != null) { 885 dst.prefix = new ArrayList<StringType>(); 886 for (StringType i : prefix) 887 dst.prefix.add(i.copy()); 888 }; 889 if (suffix != null) { 890 dst.suffix = new ArrayList<StringType>(); 891 for (StringType i : suffix) 892 dst.suffix.add(i.copy()); 893 }; 894 dst.period = period == null ? null : period.copy(); 895 return dst; 896 } 897 898 protected HumanName typedCopy() { 899 return copy(); 900 } 901 902 @Override 903 public boolean equalsDeep(Base other_) { 904 if (!super.equalsDeep(other_)) 905 return false; 906 if (!(other_ instanceof HumanName)) 907 return false; 908 HumanName o = (HumanName) other_; 909 return compareDeep(use, o.use, true) && compareDeep(text, o.text, true) && compareDeep(family, o.family, true) 910 && compareDeep(given, o.given, true) && compareDeep(prefix, o.prefix, true) && compareDeep(suffix, o.suffix, true) 911 && compareDeep(period, o.period, true); 912 } 913 914 @Override 915 public boolean equalsShallow(Base other_) { 916 if (!super.equalsShallow(other_)) 917 return false; 918 if (!(other_ instanceof HumanName)) 919 return false; 920 HumanName o = (HumanName) other_; 921 return compareValues(use, o.use, true) && compareValues(text, o.text, true) && compareValues(family, o.family, true) 922 && compareValues(given, o.given, true) && compareValues(prefix, o.prefix, true) && compareValues(suffix, o.suffix, true) 923 ; 924 } 925 926 public boolean isEmpty() { 927 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, text, family, given 928 , prefix, suffix, period); 929 } 930 931 932}