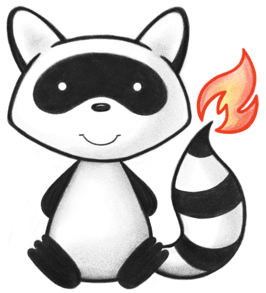
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.List; 036 037import org.hl7.fhir.exceptions.FHIRException; 038import org.hl7.fhir.instance.model.api.ICompositeType; 039import org.hl7.fhir.utilities.Utilities; 040 041import ca.uhn.fhir.model.api.annotation.Child; 042import ca.uhn.fhir.model.api.annotation.DatatypeDef; 043import ca.uhn.fhir.model.api.annotation.Description; 044/** 045 * A technical identifier - identifies some entity uniquely and unambiguously. 046 */ 047@DatatypeDef(name="Identifier") 048public class Identifier extends Type implements ICompositeType { 049 050 public enum IdentifierUse { 051 /** 052 * The identifier recommended for display and use in real-world interactions. 053 */ 054 USUAL, 055 /** 056 * The identifier considered to be most trusted for the identification of this item. 057 */ 058 OFFICIAL, 059 /** 060 * A temporary identifier. 061 */ 062 TEMP, 063 /** 064 * An identifier that was assigned in secondary use - it serves to identify the object in a relative context, but cannot be consistently assigned to the same object again in a different context. 065 */ 066 SECONDARY, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 public static IdentifierUse fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("usual".equals(codeString)) 075 return USUAL; 076 if ("official".equals(codeString)) 077 return OFFICIAL; 078 if ("temp".equals(codeString)) 079 return TEMP; 080 if ("secondary".equals(codeString)) 081 return SECONDARY; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown IdentifierUse code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case USUAL: return "usual"; 090 case OFFICIAL: return "official"; 091 case TEMP: return "temp"; 092 case SECONDARY: return "secondary"; 093 case NULL: return null; 094 default: return "?"; 095 } 096 } 097 public String getSystem() { 098 switch (this) { 099 case USUAL: return "http://hl7.org/fhir/identifier-use"; 100 case OFFICIAL: return "http://hl7.org/fhir/identifier-use"; 101 case TEMP: return "http://hl7.org/fhir/identifier-use"; 102 case SECONDARY: return "http://hl7.org/fhir/identifier-use"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getDefinition() { 108 switch (this) { 109 case USUAL: return "The identifier recommended for display and use in real-world interactions."; 110 case OFFICIAL: return "The identifier considered to be most trusted for the identification of this item."; 111 case TEMP: return "A temporary identifier."; 112 case SECONDARY: return "An identifier that was assigned in secondary use - it serves to identify the object in a relative context, but cannot be consistently assigned to the same object again in a different context."; 113 case NULL: return null; 114 default: return "?"; 115 } 116 } 117 public String getDisplay() { 118 switch (this) { 119 case USUAL: return "Usual"; 120 case OFFICIAL: return "Official"; 121 case TEMP: return "Temp"; 122 case SECONDARY: return "Secondary"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 } 128 129 public static class IdentifierUseEnumFactory implements EnumFactory<IdentifierUse> { 130 public IdentifierUse fromCode(String codeString) throws IllegalArgumentException { 131 if (codeString == null || "".equals(codeString)) 132 if (codeString == null || "".equals(codeString) || "?".equals(codeString)) 133 return null; 134 if ("usual".equals(codeString)) 135 return IdentifierUse.USUAL; 136 if ("official".equals(codeString)) 137 return IdentifierUse.OFFICIAL; 138 if ("temp".equals(codeString)) 139 return IdentifierUse.TEMP; 140 if ("secondary".equals(codeString)) 141 return IdentifierUse.SECONDARY; 142 throw new IllegalArgumentException("Unknown IdentifierUse code '"+codeString+"'"); 143 } 144 public Enumeration<IdentifierUse> fromType(PrimitiveType<?> code) throws FHIRException { 145 if (code == null) 146 return null; 147 if (code.isEmpty()) 148 return new Enumeration<IdentifierUse>(this); 149 String codeString = code.asStringValue(); 150 if (codeString == null || "".equals(codeString)) 151 return null; 152 if ("usual".equals(codeString)) 153 return new Enumeration<IdentifierUse>(this, IdentifierUse.USUAL); 154 if ("official".equals(codeString)) 155 return new Enumeration<IdentifierUse>(this, IdentifierUse.OFFICIAL); 156 if ("temp".equals(codeString)) 157 return new Enumeration<IdentifierUse>(this, IdentifierUse.TEMP); 158 if ("secondary".equals(codeString)) 159 return new Enumeration<IdentifierUse>(this, IdentifierUse.SECONDARY); 160 throw new FHIRException("Unknown IdentifierUse code '"+codeString+"'"); 161 } 162 public String toCode(IdentifierUse code) { 163 if (code == IdentifierUse.NULL) 164 return null; 165 if (code == IdentifierUse.USUAL) 166 return "usual"; 167 if (code == IdentifierUse.OFFICIAL) 168 return "official"; 169 if (code == IdentifierUse.TEMP) 170 return "temp"; 171 if (code == IdentifierUse.SECONDARY) 172 return "secondary"; 173 return "?"; 174 } 175 public String toSystem(IdentifierUse code) { 176 return code.getSystem(); 177 } 178 } 179 180 /** 181 * The purpose of this identifier. 182 */ 183 @Child(name = "use", type = {CodeType.class}, order=0, min=0, max=1, modifier=true, summary=true) 184 @Description(shortDefinition="usual | official | temp | secondary (If known)", formalDefinition="The purpose of this identifier." ) 185 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/identifier-use") 186 protected Enumeration<IdentifierUse> use; 187 188 /** 189 * A coded type for the identifier that can be used to determine which identifier to use for a specific purpose. 190 */ 191 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 192 @Description(shortDefinition="Description of identifier", formalDefinition="A coded type for the identifier that can be used to determine which identifier to use for a specific purpose." ) 193 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/identifier-type") 194 protected CodeableConcept type; 195 196 /** 197 * Establishes the namespace for the value - that is, a URL that describes a set values that are unique. 198 */ 199 @Child(name = "system", type = {UriType.class}, order=2, min=0, max=1, modifier=false, summary=true) 200 @Description(shortDefinition="The namespace for the identifier value", formalDefinition="Establishes the namespace for the value - that is, a URL that describes a set values that are unique." ) 201 protected UriType system; 202 203 /** 204 * The portion of the identifier typically relevant to the user and which is unique within the context of the system. 205 */ 206 @Child(name = "value", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 207 @Description(shortDefinition="The value that is unique", formalDefinition="The portion of the identifier typically relevant to the user and which is unique within the context of the system." ) 208 protected StringType value; 209 210 /** 211 * Time period during which identifier is/was valid for use. 212 */ 213 @Child(name = "period", type = {Period.class}, order=4, min=0, max=1, modifier=false, summary=true) 214 @Description(shortDefinition="Time period when id is/was valid for use", formalDefinition="Time period during which identifier is/was valid for use." ) 215 protected Period period; 216 217 /** 218 * Organization that issued/manages the identifier. 219 */ 220 @Child(name = "assigner", type = {Organization.class}, order=5, min=0, max=1, modifier=false, summary=true) 221 @Description(shortDefinition="Organization that issued id (may be just text)", formalDefinition="Organization that issued/manages the identifier." ) 222 protected Reference assigner; 223 224 /** 225 * The actual object that is the target of the reference (Organization that issued/manages the identifier.) 226 */ 227 protected Organization assignerTarget; 228 229 private static final long serialVersionUID = -478840981L; 230 231 /** 232 * Constructor 233 */ 234 public Identifier() { 235 super(); 236 } 237 238 /** 239 * @return {@link #use} (The purpose of this identifier.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 240 */ 241 public Enumeration<IdentifierUse> getUseElement() { 242 if (this.use == null) 243 if (Configuration.errorOnAutoCreate()) 244 throw new Error("Attempt to auto-create Identifier.use"); 245 else if (Configuration.doAutoCreate()) 246 this.use = new Enumeration<IdentifierUse>(new IdentifierUseEnumFactory()); // bb 247 return this.use; 248 } 249 250 public boolean hasUseElement() { 251 return this.use != null && !this.use.isEmpty(); 252 } 253 254 public boolean hasUse() { 255 return this.use != null && !this.use.isEmpty(); 256 } 257 258 /** 259 * @param value {@link #use} (The purpose of this identifier.). This is the underlying object with id, value and extensions. The accessor "getUse" gives direct access to the value 260 */ 261 public Identifier setUseElement(Enumeration<IdentifierUse> value) { 262 this.use = value; 263 return this; 264 } 265 266 /** 267 * @return The purpose of this identifier. 268 */ 269 public IdentifierUse getUse() { 270 return this.use == null ? null : this.use.getValue(); 271 } 272 273 /** 274 * @param value The purpose of this identifier. 275 */ 276 public Identifier setUse(IdentifierUse value) { 277 if (value == null) 278 this.use = null; 279 else { 280 if (this.use == null) 281 this.use = new Enumeration<IdentifierUse>(new IdentifierUseEnumFactory()); 282 this.use.setValue(value); 283 } 284 return this; 285 } 286 287 /** 288 * @return {@link #type} (A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.) 289 */ 290 public CodeableConcept getType() { 291 if (this.type == null) 292 if (Configuration.errorOnAutoCreate()) 293 throw new Error("Attempt to auto-create Identifier.type"); 294 else if (Configuration.doAutoCreate()) 295 this.type = new CodeableConcept(); // cc 296 return this.type; 297 } 298 299 public boolean hasType() { 300 return this.type != null && !this.type.isEmpty(); 301 } 302 303 /** 304 * @param value {@link #type} (A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.) 305 */ 306 public Identifier setType(CodeableConcept value) { 307 this.type = value; 308 return this; 309 } 310 311 /** 312 * @return {@link #system} (Establishes the namespace for the value - that is, a URL that describes a set values that are unique.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 313 */ 314 public UriType getSystemElement() { 315 if (this.system == null) 316 if (Configuration.errorOnAutoCreate()) 317 throw new Error("Attempt to auto-create Identifier.system"); 318 else if (Configuration.doAutoCreate()) 319 this.system = new UriType(); // bb 320 return this.system; 321 } 322 323 public boolean hasSystemElement() { 324 return this.system != null && !this.system.isEmpty(); 325 } 326 327 public boolean hasSystem() { 328 return this.system != null && !this.system.isEmpty(); 329 } 330 331 /** 332 * @param value {@link #system} (Establishes the namespace for the value - that is, a URL that describes a set values that are unique.). This is the underlying object with id, value and extensions. The accessor "getSystem" gives direct access to the value 333 */ 334 public Identifier setSystemElement(UriType value) { 335 this.system = value; 336 return this; 337 } 338 339 /** 340 * @return Establishes the namespace for the value - that is, a URL that describes a set values that are unique. 341 */ 342 public String getSystem() { 343 return this.system == null ? null : this.system.getValue(); 344 } 345 346 /** 347 * @param value Establishes the namespace for the value - that is, a URL that describes a set values that are unique. 348 */ 349 public Identifier setSystem(String value) { 350 if (Utilities.noString(value)) 351 this.system = null; 352 else { 353 if (this.system == null) 354 this.system = new UriType(); 355 this.system.setValue(value); 356 } 357 return this; 358 } 359 360 /** 361 * @return {@link #value} (The portion of the identifier typically relevant to the user and which is unique within the context of the system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 362 */ 363 public StringType getValueElement() { 364 if (this.value == null) 365 if (Configuration.errorOnAutoCreate()) 366 throw new Error("Attempt to auto-create Identifier.value"); 367 else if (Configuration.doAutoCreate()) 368 this.value = new StringType(); // bb 369 return this.value; 370 } 371 372 public boolean hasValueElement() { 373 return this.value != null && !this.value.isEmpty(); 374 } 375 376 public boolean hasValue() { 377 return this.value != null && !this.value.isEmpty(); 378 } 379 380 /** 381 * @param value {@link #value} (The portion of the identifier typically relevant to the user and which is unique within the context of the system.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 382 */ 383 public Identifier setValueElement(StringType value) { 384 this.value = value; 385 return this; 386 } 387 388 /** 389 * @return The portion of the identifier typically relevant to the user and which is unique within the context of the system. 390 */ 391 public String getValue() { 392 return this.value == null ? null : this.value.getValue(); 393 } 394 395 /** 396 * @param value The portion of the identifier typically relevant to the user and which is unique within the context of the system. 397 */ 398 public Identifier setValue(String value) { 399 if (Utilities.noString(value)) 400 this.value = null; 401 else { 402 if (this.value == null) 403 this.value = new StringType(); 404 this.value.setValue(value); 405 } 406 return this; 407 } 408 409 /** 410 * @return {@link #period} (Time period during which identifier is/was valid for use.) 411 */ 412 public Period getPeriod() { 413 if (this.period == null) 414 if (Configuration.errorOnAutoCreate()) 415 throw new Error("Attempt to auto-create Identifier.period"); 416 else if (Configuration.doAutoCreate()) 417 this.period = new Period(); // cc 418 return this.period; 419 } 420 421 public boolean hasPeriod() { 422 return this.period != null && !this.period.isEmpty(); 423 } 424 425 /** 426 * @param value {@link #period} (Time period during which identifier is/was valid for use.) 427 */ 428 public Identifier setPeriod(Period value) { 429 this.period = value; 430 return this; 431 } 432 433 /** 434 * @return {@link #assigner} (Organization that issued/manages the identifier.) 435 */ 436 public Reference getAssigner() { 437 if (this.assigner == null) 438 if (Configuration.errorOnAutoCreate()) 439 throw new Error("Attempt to auto-create Identifier.assigner"); 440 else if (Configuration.doAutoCreate()) 441 this.assigner = new Reference(); // cc 442 return this.assigner; 443 } 444 445 public boolean hasAssigner() { 446 return this.assigner != null && !this.assigner.isEmpty(); 447 } 448 449 /** 450 * @param value {@link #assigner} (Organization that issued/manages the identifier.) 451 */ 452 public Identifier setAssigner(Reference value) { 453 this.assigner = value; 454 return this; 455 } 456 457 /** 458 * @return {@link #assigner} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Organization that issued/manages the identifier.) 459 */ 460 public Organization getAssignerTarget() { 461 if (this.assignerTarget == null) 462 if (Configuration.errorOnAutoCreate()) 463 throw new Error("Attempt to auto-create Identifier.assigner"); 464 else if (Configuration.doAutoCreate()) 465 this.assignerTarget = new Organization(); // aa 466 return this.assignerTarget; 467 } 468 469 /** 470 * @param value {@link #assigner} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Organization that issued/manages the identifier.) 471 */ 472 public Identifier setAssignerTarget(Organization value) { 473 this.assignerTarget = value; 474 return this; 475 } 476 477 protected void listChildren(List<Property> children) { 478 super.listChildren(children); 479 children.add(new Property("use", "code", "The purpose of this identifier.", 0, 1, use)); 480 children.add(new Property("type", "CodeableConcept", "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.", 0, 1, type)); 481 children.add(new Property("system", "uri", "Establishes the namespace for the value - that is, a URL that describes a set values that are unique.", 0, 1, system)); 482 children.add(new Property("value", "string", "The portion of the identifier typically relevant to the user and which is unique within the context of the system.", 0, 1, value)); 483 children.add(new Property("period", "Period", "Time period during which identifier is/was valid for use.", 0, 1, period)); 484 children.add(new Property("assigner", "Reference(Organization)", "Organization that issued/manages the identifier.", 0, 1, assigner)); 485 } 486 487 @Override 488 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 489 switch (_hash) { 490 case 116103: /*use*/ return new Property("use", "code", "The purpose of this identifier.", 0, 1, use); 491 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A coded type for the identifier that can be used to determine which identifier to use for a specific purpose.", 0, 1, type); 492 case -887328209: /*system*/ return new Property("system", "uri", "Establishes the namespace for the value - that is, a URL that describes a set values that are unique.", 0, 1, system); 493 case 111972721: /*value*/ return new Property("value", "string", "The portion of the identifier typically relevant to the user and which is unique within the context of the system.", 0, 1, value); 494 case -991726143: /*period*/ return new Property("period", "Period", "Time period during which identifier is/was valid for use.", 0, 1, period); 495 case -369881636: /*assigner*/ return new Property("assigner", "Reference(Organization)", "Organization that issued/manages the identifier.", 0, 1, assigner); 496 default: return super.getNamedProperty(_hash, _name, _checkValid); 497 } 498 499 } 500 501 @Override 502 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 503 switch (hash) { 504 case 116103: /*use*/ return this.use == null ? new Base[0] : new Base[] {this.use}; // Enumeration<IdentifierUse> 505 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 506 case -887328209: /*system*/ return this.system == null ? new Base[0] : new Base[] {this.system}; // UriType 507 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 508 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 509 case -369881636: /*assigner*/ return this.assigner == null ? new Base[0] : new Base[] {this.assigner}; // Reference 510 default: return super.getProperty(hash, name, checkValid); 511 } 512 513 } 514 515 @Override 516 public Base setProperty(int hash, String name, Base value) throws FHIRException { 517 switch (hash) { 518 case 116103: // use 519 value = new IdentifierUseEnumFactory().fromType(castToCode(value)); 520 this.use = (Enumeration) value; // Enumeration<IdentifierUse> 521 return value; 522 case 3575610: // type 523 this.type = castToCodeableConcept(value); // CodeableConcept 524 return value; 525 case -887328209: // system 526 this.system = castToUri(value); // UriType 527 return value; 528 case 111972721: // value 529 this.value = castToString(value); // StringType 530 return value; 531 case -991726143: // period 532 this.period = castToPeriod(value); // Period 533 return value; 534 case -369881636: // assigner 535 this.assigner = castToReference(value); // Reference 536 return value; 537 default: return super.setProperty(hash, name, value); 538 } 539 540 } 541 542 @Override 543 public Base setProperty(String name, Base value) throws FHIRException { 544 if (name.equals("use")) { 545 value = new IdentifierUseEnumFactory().fromType(castToCode(value)); 546 this.use = (Enumeration) value; // Enumeration<IdentifierUse> 547 } else if (name.equals("type")) { 548 this.type = castToCodeableConcept(value); // CodeableConcept 549 } else if (name.equals("system")) { 550 this.system = castToUri(value); // UriType 551 } else if (name.equals("value")) { 552 this.value = castToString(value); // StringType 553 } else if (name.equals("period")) { 554 this.period = castToPeriod(value); // Period 555 } else if (name.equals("assigner")) { 556 this.assigner = castToReference(value); // Reference 557 } else 558 return super.setProperty(name, value); 559 return value; 560 } 561 562 @Override 563 public Base makeProperty(int hash, String name) throws FHIRException { 564 switch (hash) { 565 case 116103: return getUseElement(); 566 case 3575610: return getType(); 567 case -887328209: return getSystemElement(); 568 case 111972721: return getValueElement(); 569 case -991726143: return getPeriod(); 570 case -369881636: return getAssigner(); 571 default: return super.makeProperty(hash, name); 572 } 573 574 } 575 576 @Override 577 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 578 switch (hash) { 579 case 116103: /*use*/ return new String[] {"code"}; 580 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 581 case -887328209: /*system*/ return new String[] {"uri"}; 582 case 111972721: /*value*/ return new String[] {"string"}; 583 case -991726143: /*period*/ return new String[] {"Period"}; 584 case -369881636: /*assigner*/ return new String[] {"Reference"}; 585 default: return super.getTypesForProperty(hash, name); 586 } 587 588 } 589 590 @Override 591 public Base addChild(String name) throws FHIRException { 592 if (name.equals("use")) { 593 throw new FHIRException("Cannot call addChild on a singleton property Identifier.use"); 594 } 595 else if (name.equals("type")) { 596 this.type = new CodeableConcept(); 597 return this.type; 598 } 599 else if (name.equals("system")) { 600 throw new FHIRException("Cannot call addChild on a singleton property Identifier.system"); 601 } 602 else if (name.equals("value")) { 603 throw new FHIRException("Cannot call addChild on a singleton property Identifier.value"); 604 } 605 else if (name.equals("period")) { 606 this.period = new Period(); 607 return this.period; 608 } 609 else if (name.equals("assigner")) { 610 this.assigner = new Reference(); 611 return this.assigner; 612 } 613 else 614 return super.addChild(name); 615 } 616 617 public String fhirType() { 618 return "Identifier"; 619 620 } 621 622 public Identifier copy() { 623 Identifier dst = new Identifier(); 624 copyValues(dst); 625 dst.use = use == null ? null : use.copy(); 626 dst.type = type == null ? null : type.copy(); 627 dst.system = system == null ? null : system.copy(); 628 dst.value = value == null ? null : value.copy(); 629 dst.period = period == null ? null : period.copy(); 630 dst.assigner = assigner == null ? null : assigner.copy(); 631 return dst; 632 } 633 634 protected Identifier typedCopy() { 635 return copy(); 636 } 637 638 @Override 639 public boolean equalsDeep(Base other_) { 640 if (!super.equalsDeep(other_)) 641 return false; 642 if (!(other_ instanceof Identifier)) 643 return false; 644 Identifier o = (Identifier) other_; 645 return compareDeep(use, o.use, true) && compareDeep(type, o.type, true) && compareDeep(system, o.system, true) 646 && compareDeep(value, o.value, true) && compareDeep(period, o.period, true) && compareDeep(assigner, o.assigner, true) 647 ; 648 } 649 650 @Override 651 public boolean equalsShallow(Base other_) { 652 if (!super.equalsShallow(other_)) 653 return false; 654 if (!(other_ instanceof Identifier)) 655 return false; 656 Identifier o = (Identifier) other_; 657 return compareValues(use, o.use, true) && compareValues(system, o.system, true) && compareValues(value, o.value, true) 658 ; 659 } 660 661 public boolean isEmpty() { 662 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(use, type, system, value 663 , period, assigner); 664 } 665 666 667}