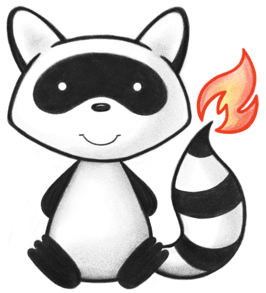
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * A text description of the DICOM SOP instances selected in the ImagingManifest; or the reason for, or significance of, the selection. 051 */ 052@ResourceDef(name="ImagingManifest", profile="http://hl7.org/fhir/Profile/ImagingManifest") 053public class ImagingManifest extends DomainResource { 054 055 @Block() 056 public static class StudyComponent extends BackboneElement implements IBaseBackboneElement { 057 /** 058 * Study instance UID of the SOP instances in the selection. 059 */ 060 @Child(name = "uid", type = {OidType.class}, order=1, min=1, max=1, modifier=false, summary=true) 061 @Description(shortDefinition="Study instance UID", formalDefinition="Study instance UID of the SOP instances in the selection." ) 062 protected OidType uid; 063 064 /** 065 * Reference to the Imaging Study in FHIR form. 066 */ 067 @Child(name = "imagingStudy", type = {ImagingStudy.class}, order=2, min=0, max=1, modifier=false, summary=true) 068 @Description(shortDefinition="Reference to ImagingStudy", formalDefinition="Reference to the Imaging Study in FHIR form." ) 069 protected Reference imagingStudy; 070 071 /** 072 * The actual object that is the target of the reference (Reference to the Imaging Study in FHIR form.) 073 */ 074 protected ImagingStudy imagingStudyTarget; 075 076 /** 077 * The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type. 078 */ 079 @Child(name = "endpoint", type = {Endpoint.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 080 @Description(shortDefinition="Study access service endpoint", formalDefinition="The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type." ) 081 protected List<Reference> endpoint; 082 /** 083 * The actual objects that are the target of the reference (The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type.) 084 */ 085 protected List<Endpoint> endpointTarget; 086 087 088 /** 089 * Series identity and locating information of the DICOM SOP instances in the selection. 090 */ 091 @Child(name = "series", type = {}, order=4, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 092 @Description(shortDefinition="Series identity of the selected instances", formalDefinition="Series identity and locating information of the DICOM SOP instances in the selection." ) 093 protected List<SeriesComponent> series; 094 095 private static final long serialVersionUID = -538170921L; 096 097 /** 098 * Constructor 099 */ 100 public StudyComponent() { 101 super(); 102 } 103 104 /** 105 * Constructor 106 */ 107 public StudyComponent(OidType uid) { 108 super(); 109 this.uid = uid; 110 } 111 112 /** 113 * @return {@link #uid} (Study instance UID of the SOP instances in the selection.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 114 */ 115 public OidType getUidElement() { 116 if (this.uid == null) 117 if (Configuration.errorOnAutoCreate()) 118 throw new Error("Attempt to auto-create StudyComponent.uid"); 119 else if (Configuration.doAutoCreate()) 120 this.uid = new OidType(); // bb 121 return this.uid; 122 } 123 124 public boolean hasUidElement() { 125 return this.uid != null && !this.uid.isEmpty(); 126 } 127 128 public boolean hasUid() { 129 return this.uid != null && !this.uid.isEmpty(); 130 } 131 132 /** 133 * @param value {@link #uid} (Study instance UID of the SOP instances in the selection.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 134 */ 135 public StudyComponent setUidElement(OidType value) { 136 this.uid = value; 137 return this; 138 } 139 140 /** 141 * @return Study instance UID of the SOP instances in the selection. 142 */ 143 public String getUid() { 144 return this.uid == null ? null : this.uid.getValue(); 145 } 146 147 /** 148 * @param value Study instance UID of the SOP instances in the selection. 149 */ 150 public StudyComponent setUid(String value) { 151 if (this.uid == null) 152 this.uid = new OidType(); 153 this.uid.setValue(value); 154 return this; 155 } 156 157 /** 158 * @return {@link #imagingStudy} (Reference to the Imaging Study in FHIR form.) 159 */ 160 public Reference getImagingStudy() { 161 if (this.imagingStudy == null) 162 if (Configuration.errorOnAutoCreate()) 163 throw new Error("Attempt to auto-create StudyComponent.imagingStudy"); 164 else if (Configuration.doAutoCreate()) 165 this.imagingStudy = new Reference(); // cc 166 return this.imagingStudy; 167 } 168 169 public boolean hasImagingStudy() { 170 return this.imagingStudy != null && !this.imagingStudy.isEmpty(); 171 } 172 173 /** 174 * @param value {@link #imagingStudy} (Reference to the Imaging Study in FHIR form.) 175 */ 176 public StudyComponent setImagingStudy(Reference value) { 177 this.imagingStudy = value; 178 return this; 179 } 180 181 /** 182 * @return {@link #imagingStudy} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reference to the Imaging Study in FHIR form.) 183 */ 184 public ImagingStudy getImagingStudyTarget() { 185 if (this.imagingStudyTarget == null) 186 if (Configuration.errorOnAutoCreate()) 187 throw new Error("Attempt to auto-create StudyComponent.imagingStudy"); 188 else if (Configuration.doAutoCreate()) 189 this.imagingStudyTarget = new ImagingStudy(); // aa 190 return this.imagingStudyTarget; 191 } 192 193 /** 194 * @param value {@link #imagingStudy} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reference to the Imaging Study in FHIR form.) 195 */ 196 public StudyComponent setImagingStudyTarget(ImagingStudy value) { 197 this.imagingStudyTarget = value; 198 return this; 199 } 200 201 /** 202 * @return {@link #endpoint} (The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type.) 203 */ 204 public List<Reference> getEndpoint() { 205 if (this.endpoint == null) 206 this.endpoint = new ArrayList<Reference>(); 207 return this.endpoint; 208 } 209 210 /** 211 * @return Returns a reference to <code>this</code> for easy method chaining 212 */ 213 public StudyComponent setEndpoint(List<Reference> theEndpoint) { 214 this.endpoint = theEndpoint; 215 return this; 216 } 217 218 public boolean hasEndpoint() { 219 if (this.endpoint == null) 220 return false; 221 for (Reference item : this.endpoint) 222 if (!item.isEmpty()) 223 return true; 224 return false; 225 } 226 227 public Reference addEndpoint() { //3 228 Reference t = new Reference(); 229 if (this.endpoint == null) 230 this.endpoint = new ArrayList<Reference>(); 231 this.endpoint.add(t); 232 return t; 233 } 234 235 public StudyComponent addEndpoint(Reference t) { //3 236 if (t == null) 237 return this; 238 if (this.endpoint == null) 239 this.endpoint = new ArrayList<Reference>(); 240 this.endpoint.add(t); 241 return this; 242 } 243 244 /** 245 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 246 */ 247 public Reference getEndpointFirstRep() { 248 if (getEndpoint().isEmpty()) { 249 addEndpoint(); 250 } 251 return getEndpoint().get(0); 252 } 253 254 /** 255 * @deprecated Use Reference#setResource(IBaseResource) instead 256 */ 257 @Deprecated 258 public List<Endpoint> getEndpointTarget() { 259 if (this.endpointTarget == null) 260 this.endpointTarget = new ArrayList<Endpoint>(); 261 return this.endpointTarget; 262 } 263 264 /** 265 * @deprecated Use Reference#setResource(IBaseResource) instead 266 */ 267 @Deprecated 268 public Endpoint addEndpointTarget() { 269 Endpoint r = new Endpoint(); 270 if (this.endpointTarget == null) 271 this.endpointTarget = new ArrayList<Endpoint>(); 272 this.endpointTarget.add(r); 273 return r; 274 } 275 276 /** 277 * @return {@link #series} (Series identity and locating information of the DICOM SOP instances in the selection.) 278 */ 279 public List<SeriesComponent> getSeries() { 280 if (this.series == null) 281 this.series = new ArrayList<SeriesComponent>(); 282 return this.series; 283 } 284 285 /** 286 * @return Returns a reference to <code>this</code> for easy method chaining 287 */ 288 public StudyComponent setSeries(List<SeriesComponent> theSeries) { 289 this.series = theSeries; 290 return this; 291 } 292 293 public boolean hasSeries() { 294 if (this.series == null) 295 return false; 296 for (SeriesComponent item : this.series) 297 if (!item.isEmpty()) 298 return true; 299 return false; 300 } 301 302 public SeriesComponent addSeries() { //3 303 SeriesComponent t = new SeriesComponent(); 304 if (this.series == null) 305 this.series = new ArrayList<SeriesComponent>(); 306 this.series.add(t); 307 return t; 308 } 309 310 public StudyComponent addSeries(SeriesComponent t) { //3 311 if (t == null) 312 return this; 313 if (this.series == null) 314 this.series = new ArrayList<SeriesComponent>(); 315 this.series.add(t); 316 return this; 317 } 318 319 /** 320 * @return The first repetition of repeating field {@link #series}, creating it if it does not already exist 321 */ 322 public SeriesComponent getSeriesFirstRep() { 323 if (getSeries().isEmpty()) { 324 addSeries(); 325 } 326 return getSeries().get(0); 327 } 328 329 protected void listChildren(List<Property> children) { 330 super.listChildren(children); 331 children.add(new Property("uid", "oid", "Study instance UID of the SOP instances in the selection.", 0, 1, uid)); 332 children.add(new Property("imagingStudy", "Reference(ImagingStudy)", "Reference to the Imaging Study in FHIR form.", 0, 1, imagingStudy)); 333 children.add(new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 334 children.add(new Property("series", "", "Series identity and locating information of the DICOM SOP instances in the selection.", 0, java.lang.Integer.MAX_VALUE, series)); 335 } 336 337 @Override 338 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 339 switch (_hash) { 340 case 115792: /*uid*/ return new Property("uid", "oid", "Study instance UID of the SOP instances in the selection.", 0, 1, uid); 341 case -814900911: /*imagingStudy*/ return new Property("imagingStudy", "Reference(ImagingStudy)", "Reference to the Imaging Study in FHIR form.", 0, 1, imagingStudy); 342 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type.", 0, java.lang.Integer.MAX_VALUE, endpoint); 343 case -905838985: /*series*/ return new Property("series", "", "Series identity and locating information of the DICOM SOP instances in the selection.", 0, java.lang.Integer.MAX_VALUE, series); 344 default: return super.getNamedProperty(_hash, _name, _checkValid); 345 } 346 347 } 348 349 @Override 350 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 351 switch (hash) { 352 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // OidType 353 case -814900911: /*imagingStudy*/ return this.imagingStudy == null ? new Base[0] : new Base[] {this.imagingStudy}; // Reference 354 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 355 case -905838985: /*series*/ return this.series == null ? new Base[0] : this.series.toArray(new Base[this.series.size()]); // SeriesComponent 356 default: return super.getProperty(hash, name, checkValid); 357 } 358 359 } 360 361 @Override 362 public Base setProperty(int hash, String name, Base value) throws FHIRException { 363 switch (hash) { 364 case 115792: // uid 365 this.uid = castToOid(value); // OidType 366 return value; 367 case -814900911: // imagingStudy 368 this.imagingStudy = castToReference(value); // Reference 369 return value; 370 case 1741102485: // endpoint 371 this.getEndpoint().add(castToReference(value)); // Reference 372 return value; 373 case -905838985: // series 374 this.getSeries().add((SeriesComponent) value); // SeriesComponent 375 return value; 376 default: return super.setProperty(hash, name, value); 377 } 378 379 } 380 381 @Override 382 public Base setProperty(String name, Base value) throws FHIRException { 383 if (name.equals("uid")) { 384 this.uid = castToOid(value); // OidType 385 } else if (name.equals("imagingStudy")) { 386 this.imagingStudy = castToReference(value); // Reference 387 } else if (name.equals("endpoint")) { 388 this.getEndpoint().add(castToReference(value)); 389 } else if (name.equals("series")) { 390 this.getSeries().add((SeriesComponent) value); 391 } else 392 return super.setProperty(name, value); 393 return value; 394 } 395 396 @Override 397 public Base makeProperty(int hash, String name) throws FHIRException { 398 switch (hash) { 399 case 115792: return getUidElement(); 400 case -814900911: return getImagingStudy(); 401 case 1741102485: return addEndpoint(); 402 case -905838985: return addSeries(); 403 default: return super.makeProperty(hash, name); 404 } 405 406 } 407 408 @Override 409 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 410 switch (hash) { 411 case 115792: /*uid*/ return new String[] {"oid"}; 412 case -814900911: /*imagingStudy*/ return new String[] {"Reference"}; 413 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 414 case -905838985: /*series*/ return new String[] {}; 415 default: return super.getTypesForProperty(hash, name); 416 } 417 418 } 419 420 @Override 421 public Base addChild(String name) throws FHIRException { 422 if (name.equals("uid")) { 423 throw new FHIRException("Cannot call addChild on a singleton property ImagingManifest.uid"); 424 } 425 else if (name.equals("imagingStudy")) { 426 this.imagingStudy = new Reference(); 427 return this.imagingStudy; 428 } 429 else if (name.equals("endpoint")) { 430 return addEndpoint(); 431 } 432 else if (name.equals("series")) { 433 return addSeries(); 434 } 435 else 436 return super.addChild(name); 437 } 438 439 public StudyComponent copy() { 440 StudyComponent dst = new StudyComponent(); 441 copyValues(dst); 442 dst.uid = uid == null ? null : uid.copy(); 443 dst.imagingStudy = imagingStudy == null ? null : imagingStudy.copy(); 444 if (endpoint != null) { 445 dst.endpoint = new ArrayList<Reference>(); 446 for (Reference i : endpoint) 447 dst.endpoint.add(i.copy()); 448 }; 449 if (series != null) { 450 dst.series = new ArrayList<SeriesComponent>(); 451 for (SeriesComponent i : series) 452 dst.series.add(i.copy()); 453 }; 454 return dst; 455 } 456 457 @Override 458 public boolean equalsDeep(Base other_) { 459 if (!super.equalsDeep(other_)) 460 return false; 461 if (!(other_ instanceof StudyComponent)) 462 return false; 463 StudyComponent o = (StudyComponent) other_; 464 return compareDeep(uid, o.uid, true) && compareDeep(imagingStudy, o.imagingStudy, true) && compareDeep(endpoint, o.endpoint, true) 465 && compareDeep(series, o.series, true); 466 } 467 468 @Override 469 public boolean equalsShallow(Base other_) { 470 if (!super.equalsShallow(other_)) 471 return false; 472 if (!(other_ instanceof StudyComponent)) 473 return false; 474 StudyComponent o = (StudyComponent) other_; 475 return compareValues(uid, o.uid, true); 476 } 477 478 public boolean isEmpty() { 479 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, imagingStudy, endpoint 480 , series); 481 } 482 483 public String fhirType() { 484 return "ImagingManifest.study"; 485 486 } 487 488 } 489 490 @Block() 491 public static class SeriesComponent extends BackboneElement implements IBaseBackboneElement { 492 /** 493 * Series instance UID of the SOP instances in the selection. 494 */ 495 @Child(name = "uid", type = {OidType.class}, order=1, min=1, max=1, modifier=false, summary=true) 496 @Description(shortDefinition="Series instance UID", formalDefinition="Series instance UID of the SOP instances in the selection." ) 497 protected OidType uid; 498 499 /** 500 * The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type. 501 */ 502 @Child(name = "endpoint", type = {Endpoint.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 503 @Description(shortDefinition="Series access endpoint", formalDefinition="The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type." ) 504 protected List<Reference> endpoint; 505 /** 506 * The actual objects that are the target of the reference (The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type.) 507 */ 508 protected List<Endpoint> endpointTarget; 509 510 511 /** 512 * Identity and locating information of the selected DICOM SOP instances. 513 */ 514 @Child(name = "instance", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 515 @Description(shortDefinition="The selected instance", formalDefinition="Identity and locating information of the selected DICOM SOP instances." ) 516 protected List<InstanceComponent> instance; 517 518 private static final long serialVersionUID = -1682136598L; 519 520 /** 521 * Constructor 522 */ 523 public SeriesComponent() { 524 super(); 525 } 526 527 /** 528 * Constructor 529 */ 530 public SeriesComponent(OidType uid) { 531 super(); 532 this.uid = uid; 533 } 534 535 /** 536 * @return {@link #uid} (Series instance UID of the SOP instances in the selection.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 537 */ 538 public OidType getUidElement() { 539 if (this.uid == null) 540 if (Configuration.errorOnAutoCreate()) 541 throw new Error("Attempt to auto-create SeriesComponent.uid"); 542 else if (Configuration.doAutoCreate()) 543 this.uid = new OidType(); // bb 544 return this.uid; 545 } 546 547 public boolean hasUidElement() { 548 return this.uid != null && !this.uid.isEmpty(); 549 } 550 551 public boolean hasUid() { 552 return this.uid != null && !this.uid.isEmpty(); 553 } 554 555 /** 556 * @param value {@link #uid} (Series instance UID of the SOP instances in the selection.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 557 */ 558 public SeriesComponent setUidElement(OidType value) { 559 this.uid = value; 560 return this; 561 } 562 563 /** 564 * @return Series instance UID of the SOP instances in the selection. 565 */ 566 public String getUid() { 567 return this.uid == null ? null : this.uid.getValue(); 568 } 569 570 /** 571 * @param value Series instance UID of the SOP instances in the selection. 572 */ 573 public SeriesComponent setUid(String value) { 574 if (this.uid == null) 575 this.uid = new OidType(); 576 this.uid.setValue(value); 577 return this; 578 } 579 580 /** 581 * @return {@link #endpoint} (The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type.) 582 */ 583 public List<Reference> getEndpoint() { 584 if (this.endpoint == null) 585 this.endpoint = new ArrayList<Reference>(); 586 return this.endpoint; 587 } 588 589 /** 590 * @return Returns a reference to <code>this</code> for easy method chaining 591 */ 592 public SeriesComponent setEndpoint(List<Reference> theEndpoint) { 593 this.endpoint = theEndpoint; 594 return this; 595 } 596 597 public boolean hasEndpoint() { 598 if (this.endpoint == null) 599 return false; 600 for (Reference item : this.endpoint) 601 if (!item.isEmpty()) 602 return true; 603 return false; 604 } 605 606 public Reference addEndpoint() { //3 607 Reference t = new Reference(); 608 if (this.endpoint == null) 609 this.endpoint = new ArrayList<Reference>(); 610 this.endpoint.add(t); 611 return t; 612 } 613 614 public SeriesComponent addEndpoint(Reference t) { //3 615 if (t == null) 616 return this; 617 if (this.endpoint == null) 618 this.endpoint = new ArrayList<Reference>(); 619 this.endpoint.add(t); 620 return this; 621 } 622 623 /** 624 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 625 */ 626 public Reference getEndpointFirstRep() { 627 if (getEndpoint().isEmpty()) { 628 addEndpoint(); 629 } 630 return getEndpoint().get(0); 631 } 632 633 /** 634 * @deprecated Use Reference#setResource(IBaseResource) instead 635 */ 636 @Deprecated 637 public List<Endpoint> getEndpointTarget() { 638 if (this.endpointTarget == null) 639 this.endpointTarget = new ArrayList<Endpoint>(); 640 return this.endpointTarget; 641 } 642 643 /** 644 * @deprecated Use Reference#setResource(IBaseResource) instead 645 */ 646 @Deprecated 647 public Endpoint addEndpointTarget() { 648 Endpoint r = new Endpoint(); 649 if (this.endpointTarget == null) 650 this.endpointTarget = new ArrayList<Endpoint>(); 651 this.endpointTarget.add(r); 652 return r; 653 } 654 655 /** 656 * @return {@link #instance} (Identity and locating information of the selected DICOM SOP instances.) 657 */ 658 public List<InstanceComponent> getInstance() { 659 if (this.instance == null) 660 this.instance = new ArrayList<InstanceComponent>(); 661 return this.instance; 662 } 663 664 /** 665 * @return Returns a reference to <code>this</code> for easy method chaining 666 */ 667 public SeriesComponent setInstance(List<InstanceComponent> theInstance) { 668 this.instance = theInstance; 669 return this; 670 } 671 672 public boolean hasInstance() { 673 if (this.instance == null) 674 return false; 675 for (InstanceComponent item : this.instance) 676 if (!item.isEmpty()) 677 return true; 678 return false; 679 } 680 681 public InstanceComponent addInstance() { //3 682 InstanceComponent t = new InstanceComponent(); 683 if (this.instance == null) 684 this.instance = new ArrayList<InstanceComponent>(); 685 this.instance.add(t); 686 return t; 687 } 688 689 public SeriesComponent addInstance(InstanceComponent t) { //3 690 if (t == null) 691 return this; 692 if (this.instance == null) 693 this.instance = new ArrayList<InstanceComponent>(); 694 this.instance.add(t); 695 return this; 696 } 697 698 /** 699 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist 700 */ 701 public InstanceComponent getInstanceFirstRep() { 702 if (getInstance().isEmpty()) { 703 addInstance(); 704 } 705 return getInstance().get(0); 706 } 707 708 protected void listChildren(List<Property> children) { 709 super.listChildren(children); 710 children.add(new Property("uid", "oid", "Series instance UID of the SOP instances in the selection.", 0, 1, uid)); 711 children.add(new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 712 children.add(new Property("instance", "", "Identity and locating information of the selected DICOM SOP instances.", 0, java.lang.Integer.MAX_VALUE, instance)); 713 } 714 715 @Override 716 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 717 switch (_hash) { 718 case 115792: /*uid*/ return new Property("uid", "oid", "Series instance UID of the SOP instances in the selection.", 0, 1, uid); 719 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type.", 0, java.lang.Integer.MAX_VALUE, endpoint); 720 case 555127957: /*instance*/ return new Property("instance", "", "Identity and locating information of the selected DICOM SOP instances.", 0, java.lang.Integer.MAX_VALUE, instance); 721 default: return super.getNamedProperty(_hash, _name, _checkValid); 722 } 723 724 } 725 726 @Override 727 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 728 switch (hash) { 729 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // OidType 730 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 731 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // InstanceComponent 732 default: return super.getProperty(hash, name, checkValid); 733 } 734 735 } 736 737 @Override 738 public Base setProperty(int hash, String name, Base value) throws FHIRException { 739 switch (hash) { 740 case 115792: // uid 741 this.uid = castToOid(value); // OidType 742 return value; 743 case 1741102485: // endpoint 744 this.getEndpoint().add(castToReference(value)); // Reference 745 return value; 746 case 555127957: // instance 747 this.getInstance().add((InstanceComponent) value); // InstanceComponent 748 return value; 749 default: return super.setProperty(hash, name, value); 750 } 751 752 } 753 754 @Override 755 public Base setProperty(String name, Base value) throws FHIRException { 756 if (name.equals("uid")) { 757 this.uid = castToOid(value); // OidType 758 } else if (name.equals("endpoint")) { 759 this.getEndpoint().add(castToReference(value)); 760 } else if (name.equals("instance")) { 761 this.getInstance().add((InstanceComponent) value); 762 } else 763 return super.setProperty(name, value); 764 return value; 765 } 766 767 @Override 768 public Base makeProperty(int hash, String name) throws FHIRException { 769 switch (hash) { 770 case 115792: return getUidElement(); 771 case 1741102485: return addEndpoint(); 772 case 555127957: return addInstance(); 773 default: return super.makeProperty(hash, name); 774 } 775 776 } 777 778 @Override 779 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 780 switch (hash) { 781 case 115792: /*uid*/ return new String[] {"oid"}; 782 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 783 case 555127957: /*instance*/ return new String[] {}; 784 default: return super.getTypesForProperty(hash, name); 785 } 786 787 } 788 789 @Override 790 public Base addChild(String name) throws FHIRException { 791 if (name.equals("uid")) { 792 throw new FHIRException("Cannot call addChild on a singleton property ImagingManifest.uid"); 793 } 794 else if (name.equals("endpoint")) { 795 return addEndpoint(); 796 } 797 else if (name.equals("instance")) { 798 return addInstance(); 799 } 800 else 801 return super.addChild(name); 802 } 803 804 public SeriesComponent copy() { 805 SeriesComponent dst = new SeriesComponent(); 806 copyValues(dst); 807 dst.uid = uid == null ? null : uid.copy(); 808 if (endpoint != null) { 809 dst.endpoint = new ArrayList<Reference>(); 810 for (Reference i : endpoint) 811 dst.endpoint.add(i.copy()); 812 }; 813 if (instance != null) { 814 dst.instance = new ArrayList<InstanceComponent>(); 815 for (InstanceComponent i : instance) 816 dst.instance.add(i.copy()); 817 }; 818 return dst; 819 } 820 821 @Override 822 public boolean equalsDeep(Base other_) { 823 if (!super.equalsDeep(other_)) 824 return false; 825 if (!(other_ instanceof SeriesComponent)) 826 return false; 827 SeriesComponent o = (SeriesComponent) other_; 828 return compareDeep(uid, o.uid, true) && compareDeep(endpoint, o.endpoint, true) && compareDeep(instance, o.instance, true) 829 ; 830 } 831 832 @Override 833 public boolean equalsShallow(Base other_) { 834 if (!super.equalsShallow(other_)) 835 return false; 836 if (!(other_ instanceof SeriesComponent)) 837 return false; 838 SeriesComponent o = (SeriesComponent) other_; 839 return compareValues(uid, o.uid, true); 840 } 841 842 public boolean isEmpty() { 843 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, endpoint, instance 844 ); 845 } 846 847 public String fhirType() { 848 return "ImagingManifest.study.series"; 849 850 } 851 852 } 853 854 @Block() 855 public static class InstanceComponent extends BackboneElement implements IBaseBackboneElement { 856 /** 857 * SOP class UID of the selected instance. 858 */ 859 @Child(name = "sopClass", type = {OidType.class}, order=1, min=1, max=1, modifier=false, summary=true) 860 @Description(shortDefinition="SOP class UID of instance", formalDefinition="SOP class UID of the selected instance." ) 861 protected OidType sopClass; 862 863 /** 864 * SOP Instance UID of the selected instance. 865 */ 866 @Child(name = "uid", type = {OidType.class}, order=2, min=1, max=1, modifier=false, summary=true) 867 @Description(shortDefinition="Selected instance UID", formalDefinition="SOP Instance UID of the selected instance." ) 868 protected OidType uid; 869 870 private static final long serialVersionUID = -885780004L; 871 872 /** 873 * Constructor 874 */ 875 public InstanceComponent() { 876 super(); 877 } 878 879 /** 880 * Constructor 881 */ 882 public InstanceComponent(OidType sopClass, OidType uid) { 883 super(); 884 this.sopClass = sopClass; 885 this.uid = uid; 886 } 887 888 /** 889 * @return {@link #sopClass} (SOP class UID of the selected instance.). This is the underlying object with id, value and extensions. The accessor "getSopClass" gives direct access to the value 890 */ 891 public OidType getSopClassElement() { 892 if (this.sopClass == null) 893 if (Configuration.errorOnAutoCreate()) 894 throw new Error("Attempt to auto-create InstanceComponent.sopClass"); 895 else if (Configuration.doAutoCreate()) 896 this.sopClass = new OidType(); // bb 897 return this.sopClass; 898 } 899 900 public boolean hasSopClassElement() { 901 return this.sopClass != null && !this.sopClass.isEmpty(); 902 } 903 904 public boolean hasSopClass() { 905 return this.sopClass != null && !this.sopClass.isEmpty(); 906 } 907 908 /** 909 * @param value {@link #sopClass} (SOP class UID of the selected instance.). This is the underlying object with id, value and extensions. The accessor "getSopClass" gives direct access to the value 910 */ 911 public InstanceComponent setSopClassElement(OidType value) { 912 this.sopClass = value; 913 return this; 914 } 915 916 /** 917 * @return SOP class UID of the selected instance. 918 */ 919 public String getSopClass() { 920 return this.sopClass == null ? null : this.sopClass.getValue(); 921 } 922 923 /** 924 * @param value SOP class UID of the selected instance. 925 */ 926 public InstanceComponent setSopClass(String value) { 927 if (this.sopClass == null) 928 this.sopClass = new OidType(); 929 this.sopClass.setValue(value); 930 return this; 931 } 932 933 /** 934 * @return {@link #uid} (SOP Instance UID of the selected instance.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 935 */ 936 public OidType getUidElement() { 937 if (this.uid == null) 938 if (Configuration.errorOnAutoCreate()) 939 throw new Error("Attempt to auto-create InstanceComponent.uid"); 940 else if (Configuration.doAutoCreate()) 941 this.uid = new OidType(); // bb 942 return this.uid; 943 } 944 945 public boolean hasUidElement() { 946 return this.uid != null && !this.uid.isEmpty(); 947 } 948 949 public boolean hasUid() { 950 return this.uid != null && !this.uid.isEmpty(); 951 } 952 953 /** 954 * @param value {@link #uid} (SOP Instance UID of the selected instance.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 955 */ 956 public InstanceComponent setUidElement(OidType value) { 957 this.uid = value; 958 return this; 959 } 960 961 /** 962 * @return SOP Instance UID of the selected instance. 963 */ 964 public String getUid() { 965 return this.uid == null ? null : this.uid.getValue(); 966 } 967 968 /** 969 * @param value SOP Instance UID of the selected instance. 970 */ 971 public InstanceComponent setUid(String value) { 972 if (this.uid == null) 973 this.uid = new OidType(); 974 this.uid.setValue(value); 975 return this; 976 } 977 978 protected void listChildren(List<Property> children) { 979 super.listChildren(children); 980 children.add(new Property("sopClass", "oid", "SOP class UID of the selected instance.", 0, 1, sopClass)); 981 children.add(new Property("uid", "oid", "SOP Instance UID of the selected instance.", 0, 1, uid)); 982 } 983 984 @Override 985 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 986 switch (_hash) { 987 case 1560041540: /*sopClass*/ return new Property("sopClass", "oid", "SOP class UID of the selected instance.", 0, 1, sopClass); 988 case 115792: /*uid*/ return new Property("uid", "oid", "SOP Instance UID of the selected instance.", 0, 1, uid); 989 default: return super.getNamedProperty(_hash, _name, _checkValid); 990 } 991 992 } 993 994 @Override 995 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 996 switch (hash) { 997 case 1560041540: /*sopClass*/ return this.sopClass == null ? new Base[0] : new Base[] {this.sopClass}; // OidType 998 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // OidType 999 default: return super.getProperty(hash, name, checkValid); 1000 } 1001 1002 } 1003 1004 @Override 1005 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1006 switch (hash) { 1007 case 1560041540: // sopClass 1008 this.sopClass = castToOid(value); // OidType 1009 return value; 1010 case 115792: // uid 1011 this.uid = castToOid(value); // OidType 1012 return value; 1013 default: return super.setProperty(hash, name, value); 1014 } 1015 1016 } 1017 1018 @Override 1019 public Base setProperty(String name, Base value) throws FHIRException { 1020 if (name.equals("sopClass")) { 1021 this.sopClass = castToOid(value); // OidType 1022 } else if (name.equals("uid")) { 1023 this.uid = castToOid(value); // OidType 1024 } else 1025 return super.setProperty(name, value); 1026 return value; 1027 } 1028 1029 @Override 1030 public Base makeProperty(int hash, String name) throws FHIRException { 1031 switch (hash) { 1032 case 1560041540: return getSopClassElement(); 1033 case 115792: return getUidElement(); 1034 default: return super.makeProperty(hash, name); 1035 } 1036 1037 } 1038 1039 @Override 1040 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1041 switch (hash) { 1042 case 1560041540: /*sopClass*/ return new String[] {"oid"}; 1043 case 115792: /*uid*/ return new String[] {"oid"}; 1044 default: return super.getTypesForProperty(hash, name); 1045 } 1046 1047 } 1048 1049 @Override 1050 public Base addChild(String name) throws FHIRException { 1051 if (name.equals("sopClass")) { 1052 throw new FHIRException("Cannot call addChild on a singleton property ImagingManifest.sopClass"); 1053 } 1054 else if (name.equals("uid")) { 1055 throw new FHIRException("Cannot call addChild on a singleton property ImagingManifest.uid"); 1056 } 1057 else 1058 return super.addChild(name); 1059 } 1060 1061 public InstanceComponent copy() { 1062 InstanceComponent dst = new InstanceComponent(); 1063 copyValues(dst); 1064 dst.sopClass = sopClass == null ? null : sopClass.copy(); 1065 dst.uid = uid == null ? null : uid.copy(); 1066 return dst; 1067 } 1068 1069 @Override 1070 public boolean equalsDeep(Base other_) { 1071 if (!super.equalsDeep(other_)) 1072 return false; 1073 if (!(other_ instanceof InstanceComponent)) 1074 return false; 1075 InstanceComponent o = (InstanceComponent) other_; 1076 return compareDeep(sopClass, o.sopClass, true) && compareDeep(uid, o.uid, true); 1077 } 1078 1079 @Override 1080 public boolean equalsShallow(Base other_) { 1081 if (!super.equalsShallow(other_)) 1082 return false; 1083 if (!(other_ instanceof InstanceComponent)) 1084 return false; 1085 InstanceComponent o = (InstanceComponent) other_; 1086 return compareValues(sopClass, o.sopClass, true) && compareValues(uid, o.uid, true); 1087 } 1088 1089 public boolean isEmpty() { 1090 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(sopClass, uid); 1091 } 1092 1093 public String fhirType() { 1094 return "ImagingManifest.study.series.instance"; 1095 1096 } 1097 1098 } 1099 1100 /** 1101 * Unique identifier of the DICOM Key Object Selection (KOS) that this resource represents. 1102 */ 1103 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 1104 @Description(shortDefinition="SOP Instance UID", formalDefinition="Unique identifier of the DICOM Key Object Selection (KOS) that this resource represents." ) 1105 protected Identifier identifier; 1106 1107 /** 1108 * A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest. 1109 */ 1110 @Child(name = "patient", type = {Patient.class}, order=1, min=1, max=1, modifier=false, summary=true) 1111 @Description(shortDefinition="Patient of the selected objects", formalDefinition="A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest." ) 1112 protected Reference patient; 1113 1114 /** 1115 * The actual object that is the target of the reference (A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest.) 1116 */ 1117 protected Patient patientTarget; 1118 1119 /** 1120 * Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image). 1121 */ 1122 @Child(name = "authoringTime", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1123 @Description(shortDefinition="Time when the selection of instances was made", formalDefinition="Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image)." ) 1124 protected DateTimeType authoringTime; 1125 1126 /** 1127 * Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion. 1128 */ 1129 @Child(name = "author", type = {Practitioner.class, Device.class, Organization.class, Patient.class, RelatedPerson.class}, order=3, min=0, max=1, modifier=false, summary=true) 1130 @Description(shortDefinition="Author (human or machine)", formalDefinition="Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion." ) 1131 protected Reference author; 1132 1133 /** 1134 * The actual object that is the target of the reference (Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.) 1135 */ 1136 protected Resource authorTarget; 1137 1138 /** 1139 * Free text narrative description of the ImagingManifest. 1140The value may be derived from the DICOM Standard Part 16, CID-7010 descriptions (e.g. Best in Set, Complete Study Content). Note that those values cover the wide range of uses of the DICOM Key Object Selection object, several of which are not supported by ImagingManifest. Specifically, there is no expected behavior associated with descriptions that suggest referenced images be removed or not used. 1141 */ 1142 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 1143 @Description(shortDefinition="Description text", formalDefinition="Free text narrative description of the ImagingManifest. \nThe value may be derived from the DICOM Standard Part 16, CID-7010 descriptions (e.g. Best in Set, Complete Study Content). Note that those values cover the wide range of uses of the DICOM Key Object Selection object, several of which are not supported by ImagingManifest. Specifically, there is no expected behavior associated with descriptions that suggest referenced images be removed or not used." ) 1144 protected StringType description; 1145 1146 /** 1147 * Study identity and locating information of the DICOM SOP instances in the selection. 1148 */ 1149 @Child(name = "study", type = {}, order=5, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1150 @Description(shortDefinition="Study identity of the selected instances", formalDefinition="Study identity and locating information of the DICOM SOP instances in the selection." ) 1151 protected List<StudyComponent> study; 1152 1153 private static final long serialVersionUID = 245941978L; 1154 1155 /** 1156 * Constructor 1157 */ 1158 public ImagingManifest() { 1159 super(); 1160 } 1161 1162 /** 1163 * Constructor 1164 */ 1165 public ImagingManifest(Reference patient) { 1166 super(); 1167 this.patient = patient; 1168 } 1169 1170 /** 1171 * @return {@link #identifier} (Unique identifier of the DICOM Key Object Selection (KOS) that this resource represents.) 1172 */ 1173 public Identifier getIdentifier() { 1174 if (this.identifier == null) 1175 if (Configuration.errorOnAutoCreate()) 1176 throw new Error("Attempt to auto-create ImagingManifest.identifier"); 1177 else if (Configuration.doAutoCreate()) 1178 this.identifier = new Identifier(); // cc 1179 return this.identifier; 1180 } 1181 1182 public boolean hasIdentifier() { 1183 return this.identifier != null && !this.identifier.isEmpty(); 1184 } 1185 1186 /** 1187 * @param value {@link #identifier} (Unique identifier of the DICOM Key Object Selection (KOS) that this resource represents.) 1188 */ 1189 public ImagingManifest setIdentifier(Identifier value) { 1190 this.identifier = value; 1191 return this; 1192 } 1193 1194 /** 1195 * @return {@link #patient} (A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest.) 1196 */ 1197 public Reference getPatient() { 1198 if (this.patient == null) 1199 if (Configuration.errorOnAutoCreate()) 1200 throw new Error("Attempt to auto-create ImagingManifest.patient"); 1201 else if (Configuration.doAutoCreate()) 1202 this.patient = new Reference(); // cc 1203 return this.patient; 1204 } 1205 1206 public boolean hasPatient() { 1207 return this.patient != null && !this.patient.isEmpty(); 1208 } 1209 1210 /** 1211 * @param value {@link #patient} (A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest.) 1212 */ 1213 public ImagingManifest setPatient(Reference value) { 1214 this.patient = value; 1215 return this; 1216 } 1217 1218 /** 1219 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest.) 1220 */ 1221 public Patient getPatientTarget() { 1222 if (this.patientTarget == null) 1223 if (Configuration.errorOnAutoCreate()) 1224 throw new Error("Attempt to auto-create ImagingManifest.patient"); 1225 else if (Configuration.doAutoCreate()) 1226 this.patientTarget = new Patient(); // aa 1227 return this.patientTarget; 1228 } 1229 1230 /** 1231 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest.) 1232 */ 1233 public ImagingManifest setPatientTarget(Patient value) { 1234 this.patientTarget = value; 1235 return this; 1236 } 1237 1238 /** 1239 * @return {@link #authoringTime} (Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image).). This is the underlying object with id, value and extensions. The accessor "getAuthoringTime" gives direct access to the value 1240 */ 1241 public DateTimeType getAuthoringTimeElement() { 1242 if (this.authoringTime == null) 1243 if (Configuration.errorOnAutoCreate()) 1244 throw new Error("Attempt to auto-create ImagingManifest.authoringTime"); 1245 else if (Configuration.doAutoCreate()) 1246 this.authoringTime = new DateTimeType(); // bb 1247 return this.authoringTime; 1248 } 1249 1250 public boolean hasAuthoringTimeElement() { 1251 return this.authoringTime != null && !this.authoringTime.isEmpty(); 1252 } 1253 1254 public boolean hasAuthoringTime() { 1255 return this.authoringTime != null && !this.authoringTime.isEmpty(); 1256 } 1257 1258 /** 1259 * @param value {@link #authoringTime} (Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image).). This is the underlying object with id, value and extensions. The accessor "getAuthoringTime" gives direct access to the value 1260 */ 1261 public ImagingManifest setAuthoringTimeElement(DateTimeType value) { 1262 this.authoringTime = value; 1263 return this; 1264 } 1265 1266 /** 1267 * @return Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image). 1268 */ 1269 public Date getAuthoringTime() { 1270 return this.authoringTime == null ? null : this.authoringTime.getValue(); 1271 } 1272 1273 /** 1274 * @param value Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image). 1275 */ 1276 public ImagingManifest setAuthoringTime(Date value) { 1277 if (value == null) 1278 this.authoringTime = null; 1279 else { 1280 if (this.authoringTime == null) 1281 this.authoringTime = new DateTimeType(); 1282 this.authoringTime.setValue(value); 1283 } 1284 return this; 1285 } 1286 1287 /** 1288 * @return {@link #author} (Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.) 1289 */ 1290 public Reference getAuthor() { 1291 if (this.author == null) 1292 if (Configuration.errorOnAutoCreate()) 1293 throw new Error("Attempt to auto-create ImagingManifest.author"); 1294 else if (Configuration.doAutoCreate()) 1295 this.author = new Reference(); // cc 1296 return this.author; 1297 } 1298 1299 public boolean hasAuthor() { 1300 return this.author != null && !this.author.isEmpty(); 1301 } 1302 1303 /** 1304 * @param value {@link #author} (Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.) 1305 */ 1306 public ImagingManifest setAuthor(Reference value) { 1307 this.author = value; 1308 return this; 1309 } 1310 1311 /** 1312 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.) 1313 */ 1314 public Resource getAuthorTarget() { 1315 return this.authorTarget; 1316 } 1317 1318 /** 1319 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.) 1320 */ 1321 public ImagingManifest setAuthorTarget(Resource value) { 1322 this.authorTarget = value; 1323 return this; 1324 } 1325 1326 /** 1327 * @return {@link #description} (Free text narrative description of the ImagingManifest. 1328The value may be derived from the DICOM Standard Part 16, CID-7010 descriptions (e.g. Best in Set, Complete Study Content). Note that those values cover the wide range of uses of the DICOM Key Object Selection object, several of which are not supported by ImagingManifest. Specifically, there is no expected behavior associated with descriptions that suggest referenced images be removed or not used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1329 */ 1330 public StringType getDescriptionElement() { 1331 if (this.description == null) 1332 if (Configuration.errorOnAutoCreate()) 1333 throw new Error("Attempt to auto-create ImagingManifest.description"); 1334 else if (Configuration.doAutoCreate()) 1335 this.description = new StringType(); // bb 1336 return this.description; 1337 } 1338 1339 public boolean hasDescriptionElement() { 1340 return this.description != null && !this.description.isEmpty(); 1341 } 1342 1343 public boolean hasDescription() { 1344 return this.description != null && !this.description.isEmpty(); 1345 } 1346 1347 /** 1348 * @param value {@link #description} (Free text narrative description of the ImagingManifest. 1349The value may be derived from the DICOM Standard Part 16, CID-7010 descriptions (e.g. Best in Set, Complete Study Content). Note that those values cover the wide range of uses of the DICOM Key Object Selection object, several of which are not supported by ImagingManifest. Specifically, there is no expected behavior associated with descriptions that suggest referenced images be removed or not used.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1350 */ 1351 public ImagingManifest setDescriptionElement(StringType value) { 1352 this.description = value; 1353 return this; 1354 } 1355 1356 /** 1357 * @return Free text narrative description of the ImagingManifest. 1358The value may be derived from the DICOM Standard Part 16, CID-7010 descriptions (e.g. Best in Set, Complete Study Content). Note that those values cover the wide range of uses of the DICOM Key Object Selection object, several of which are not supported by ImagingManifest. Specifically, there is no expected behavior associated with descriptions that suggest referenced images be removed or not used. 1359 */ 1360 public String getDescription() { 1361 return this.description == null ? null : this.description.getValue(); 1362 } 1363 1364 /** 1365 * @param value Free text narrative description of the ImagingManifest. 1366The value may be derived from the DICOM Standard Part 16, CID-7010 descriptions (e.g. Best in Set, Complete Study Content). Note that those values cover the wide range of uses of the DICOM Key Object Selection object, several of which are not supported by ImagingManifest. Specifically, there is no expected behavior associated with descriptions that suggest referenced images be removed or not used. 1367 */ 1368 public ImagingManifest setDescription(String value) { 1369 if (Utilities.noString(value)) 1370 this.description = null; 1371 else { 1372 if (this.description == null) 1373 this.description = new StringType(); 1374 this.description.setValue(value); 1375 } 1376 return this; 1377 } 1378 1379 /** 1380 * @return {@link #study} (Study identity and locating information of the DICOM SOP instances in the selection.) 1381 */ 1382 public List<StudyComponent> getStudy() { 1383 if (this.study == null) 1384 this.study = new ArrayList<StudyComponent>(); 1385 return this.study; 1386 } 1387 1388 /** 1389 * @return Returns a reference to <code>this</code> for easy method chaining 1390 */ 1391 public ImagingManifest setStudy(List<StudyComponent> theStudy) { 1392 this.study = theStudy; 1393 return this; 1394 } 1395 1396 public boolean hasStudy() { 1397 if (this.study == null) 1398 return false; 1399 for (StudyComponent item : this.study) 1400 if (!item.isEmpty()) 1401 return true; 1402 return false; 1403 } 1404 1405 public StudyComponent addStudy() { //3 1406 StudyComponent t = new StudyComponent(); 1407 if (this.study == null) 1408 this.study = new ArrayList<StudyComponent>(); 1409 this.study.add(t); 1410 return t; 1411 } 1412 1413 public ImagingManifest addStudy(StudyComponent t) { //3 1414 if (t == null) 1415 return this; 1416 if (this.study == null) 1417 this.study = new ArrayList<StudyComponent>(); 1418 this.study.add(t); 1419 return this; 1420 } 1421 1422 /** 1423 * @return The first repetition of repeating field {@link #study}, creating it if it does not already exist 1424 */ 1425 public StudyComponent getStudyFirstRep() { 1426 if (getStudy().isEmpty()) { 1427 addStudy(); 1428 } 1429 return getStudy().get(0); 1430 } 1431 1432 protected void listChildren(List<Property> children) { 1433 super.listChildren(children); 1434 children.add(new Property("identifier", "Identifier", "Unique identifier of the DICOM Key Object Selection (KOS) that this resource represents.", 0, 1, identifier)); 1435 children.add(new Property("patient", "Reference(Patient)", "A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest.", 0, 1, patient)); 1436 children.add(new Property("authoringTime", "dateTime", "Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image).", 0, 1, authoringTime)); 1437 children.add(new Property("author", "Reference(Practitioner|Device|Organization|Patient|RelatedPerson)", "Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.", 0, 1, author)); 1438 children.add(new Property("description", "string", "Free text narrative description of the ImagingManifest. \nThe value may be derived from the DICOM Standard Part 16, CID-7010 descriptions (e.g. Best in Set, Complete Study Content). Note that those values cover the wide range of uses of the DICOM Key Object Selection object, several of which are not supported by ImagingManifest. Specifically, there is no expected behavior associated with descriptions that suggest referenced images be removed or not used.", 0, 1, description)); 1439 children.add(new Property("study", "", "Study identity and locating information of the DICOM SOP instances in the selection.", 0, java.lang.Integer.MAX_VALUE, study)); 1440 } 1441 1442 @Override 1443 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1444 switch (_hash) { 1445 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique identifier of the DICOM Key Object Selection (KOS) that this resource represents.", 0, 1, identifier); 1446 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "A patient resource reference which is the patient subject of all DICOM SOP Instances in this ImagingManifest.", 0, 1, patient); 1447 case -1724532252: /*authoringTime*/ return new Property("authoringTime", "dateTime", "Date and time when the selection of the referenced instances were made. It is (typically) different from the creation date of the selection resource, and from dates associated with the referenced instances (e.g. capture time of the referenced image).", 0, 1, authoringTime); 1448 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|Device|Organization|Patient|RelatedPerson)", "Author of ImagingManifest. It can be a human author or a device which made the decision of the SOP instances selected. For example, a radiologist selected a set of imaging SOP instances to attach in a diagnostic report, and a CAD application may author a selection to describe SOP instances it used to generate a detection conclusion.", 0, 1, author); 1449 case -1724546052: /*description*/ return new Property("description", "string", "Free text narrative description of the ImagingManifest. \nThe value may be derived from the DICOM Standard Part 16, CID-7010 descriptions (e.g. Best in Set, Complete Study Content). Note that those values cover the wide range of uses of the DICOM Key Object Selection object, several of which are not supported by ImagingManifest. Specifically, there is no expected behavior associated with descriptions that suggest referenced images be removed or not used.", 0, 1, description); 1450 case 109776329: /*study*/ return new Property("study", "", "Study identity and locating information of the DICOM SOP instances in the selection.", 0, java.lang.Integer.MAX_VALUE, study); 1451 default: return super.getNamedProperty(_hash, _name, _checkValid); 1452 } 1453 1454 } 1455 1456 @Override 1457 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1458 switch (hash) { 1459 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1460 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1461 case -1724532252: /*authoringTime*/ return this.authoringTime == null ? new Base[0] : new Base[] {this.authoringTime}; // DateTimeType 1462 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 1463 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1464 case 109776329: /*study*/ return this.study == null ? new Base[0] : this.study.toArray(new Base[this.study.size()]); // StudyComponent 1465 default: return super.getProperty(hash, name, checkValid); 1466 } 1467 1468 } 1469 1470 @Override 1471 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1472 switch (hash) { 1473 case -1618432855: // identifier 1474 this.identifier = castToIdentifier(value); // Identifier 1475 return value; 1476 case -791418107: // patient 1477 this.patient = castToReference(value); // Reference 1478 return value; 1479 case -1724532252: // authoringTime 1480 this.authoringTime = castToDateTime(value); // DateTimeType 1481 return value; 1482 case -1406328437: // author 1483 this.author = castToReference(value); // Reference 1484 return value; 1485 case -1724546052: // description 1486 this.description = castToString(value); // StringType 1487 return value; 1488 case 109776329: // study 1489 this.getStudy().add((StudyComponent) value); // StudyComponent 1490 return value; 1491 default: return super.setProperty(hash, name, value); 1492 } 1493 1494 } 1495 1496 @Override 1497 public Base setProperty(String name, Base value) throws FHIRException { 1498 if (name.equals("identifier")) { 1499 this.identifier = castToIdentifier(value); // Identifier 1500 } else if (name.equals("patient")) { 1501 this.patient = castToReference(value); // Reference 1502 } else if (name.equals("authoringTime")) { 1503 this.authoringTime = castToDateTime(value); // DateTimeType 1504 } else if (name.equals("author")) { 1505 this.author = castToReference(value); // Reference 1506 } else if (name.equals("description")) { 1507 this.description = castToString(value); // StringType 1508 } else if (name.equals("study")) { 1509 this.getStudy().add((StudyComponent) value); 1510 } else 1511 return super.setProperty(name, value); 1512 return value; 1513 } 1514 1515 @Override 1516 public Base makeProperty(int hash, String name) throws FHIRException { 1517 switch (hash) { 1518 case -1618432855: return getIdentifier(); 1519 case -791418107: return getPatient(); 1520 case -1724532252: return getAuthoringTimeElement(); 1521 case -1406328437: return getAuthor(); 1522 case -1724546052: return getDescriptionElement(); 1523 case 109776329: return addStudy(); 1524 default: return super.makeProperty(hash, name); 1525 } 1526 1527 } 1528 1529 @Override 1530 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1531 switch (hash) { 1532 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1533 case -791418107: /*patient*/ return new String[] {"Reference"}; 1534 case -1724532252: /*authoringTime*/ return new String[] {"dateTime"}; 1535 case -1406328437: /*author*/ return new String[] {"Reference"}; 1536 case -1724546052: /*description*/ return new String[] {"string"}; 1537 case 109776329: /*study*/ return new String[] {}; 1538 default: return super.getTypesForProperty(hash, name); 1539 } 1540 1541 } 1542 1543 @Override 1544 public Base addChild(String name) throws FHIRException { 1545 if (name.equals("identifier")) { 1546 this.identifier = new Identifier(); 1547 return this.identifier; 1548 } 1549 else if (name.equals("patient")) { 1550 this.patient = new Reference(); 1551 return this.patient; 1552 } 1553 else if (name.equals("authoringTime")) { 1554 throw new FHIRException("Cannot call addChild on a singleton property ImagingManifest.authoringTime"); 1555 } 1556 else if (name.equals("author")) { 1557 this.author = new Reference(); 1558 return this.author; 1559 } 1560 else if (name.equals("description")) { 1561 throw new FHIRException("Cannot call addChild on a singleton property ImagingManifest.description"); 1562 } 1563 else if (name.equals("study")) { 1564 return addStudy(); 1565 } 1566 else 1567 return super.addChild(name); 1568 } 1569 1570 public String fhirType() { 1571 return "ImagingManifest"; 1572 1573 } 1574 1575 public ImagingManifest copy() { 1576 ImagingManifest dst = new ImagingManifest(); 1577 copyValues(dst); 1578 dst.identifier = identifier == null ? null : identifier.copy(); 1579 dst.patient = patient == null ? null : patient.copy(); 1580 dst.authoringTime = authoringTime == null ? null : authoringTime.copy(); 1581 dst.author = author == null ? null : author.copy(); 1582 dst.description = description == null ? null : description.copy(); 1583 if (study != null) { 1584 dst.study = new ArrayList<StudyComponent>(); 1585 for (StudyComponent i : study) 1586 dst.study.add(i.copy()); 1587 }; 1588 return dst; 1589 } 1590 1591 protected ImagingManifest typedCopy() { 1592 return copy(); 1593 } 1594 1595 @Override 1596 public boolean equalsDeep(Base other_) { 1597 if (!super.equalsDeep(other_)) 1598 return false; 1599 if (!(other_ instanceof ImagingManifest)) 1600 return false; 1601 ImagingManifest o = (ImagingManifest) other_; 1602 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) && compareDeep(authoringTime, o.authoringTime, true) 1603 && compareDeep(author, o.author, true) && compareDeep(description, o.description, true) && compareDeep(study, o.study, true) 1604 ; 1605 } 1606 1607 @Override 1608 public boolean equalsShallow(Base other_) { 1609 if (!super.equalsShallow(other_)) 1610 return false; 1611 if (!(other_ instanceof ImagingManifest)) 1612 return false; 1613 ImagingManifest o = (ImagingManifest) other_; 1614 return compareValues(authoringTime, o.authoringTime, true) && compareValues(description, o.description, true) 1615 ; 1616 } 1617 1618 public boolean isEmpty() { 1619 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, patient, authoringTime 1620 , author, description, study); 1621 } 1622 1623 @Override 1624 public ResourceType getResourceType() { 1625 return ResourceType.ImagingManifest; 1626 } 1627 1628 /** 1629 * Search parameter: <b>identifier</b> 1630 * <p> 1631 * Description: <b>UID of the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1632 * Type: <b>token</b><br> 1633 * Path: <b>ImagingManifest.identifier</b><br> 1634 * </p> 1635 */ 1636 @SearchParamDefinition(name="identifier", path="ImagingManifest.identifier", description="UID of the ImagingManifest (or a DICOM Key Object Selection which it represents)", type="token" ) 1637 public static final String SP_IDENTIFIER = "identifier"; 1638 /** 1639 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1640 * <p> 1641 * Description: <b>UID of the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1642 * Type: <b>token</b><br> 1643 * Path: <b>ImagingManifest.identifier</b><br> 1644 * </p> 1645 */ 1646 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1647 1648 /** 1649 * Search parameter: <b>endpoint</b> 1650 * <p> 1651 * Description: <b>The endpoint for the study or series</b><br> 1652 * Type: <b>reference</b><br> 1653 * Path: <b>ImagingManifest.study.endpoint, ImagingManifest.study.series.endpoint</b><br> 1654 * </p> 1655 */ 1656 @SearchParamDefinition(name="endpoint", path="ImagingManifest.study.endpoint | ImagingManifest.study.series.endpoint", description="The endpoint for the study or series", type="reference", target={Endpoint.class } ) 1657 public static final String SP_ENDPOINT = "endpoint"; 1658 /** 1659 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 1660 * <p> 1661 * Description: <b>The endpoint for the study or series</b><br> 1662 * Type: <b>reference</b><br> 1663 * Path: <b>ImagingManifest.study.endpoint, ImagingManifest.study.series.endpoint</b><br> 1664 * </p> 1665 */ 1666 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 1667 1668/** 1669 * Constant for fluent queries to be used to add include statements. Specifies 1670 * the path value of "<b>ImagingManifest:endpoint</b>". 1671 */ 1672 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("ImagingManifest:endpoint").toLocked(); 1673 1674 /** 1675 * Search parameter: <b>authoring-time</b> 1676 * <p> 1677 * Description: <b>Time of the ImagingManifest (or a DICOM Key Object Selection which it represents) authoring</b><br> 1678 * Type: <b>date</b><br> 1679 * Path: <b>ImagingManifest.authoringTime</b><br> 1680 * </p> 1681 */ 1682 @SearchParamDefinition(name="authoring-time", path="ImagingManifest.authoringTime", description="Time of the ImagingManifest (or a DICOM Key Object Selection which it represents) authoring", type="date" ) 1683 public static final String SP_AUTHORING_TIME = "authoring-time"; 1684 /** 1685 * <b>Fluent Client</b> search parameter constant for <b>authoring-time</b> 1686 * <p> 1687 * Description: <b>Time of the ImagingManifest (or a DICOM Key Object Selection which it represents) authoring</b><br> 1688 * Type: <b>date</b><br> 1689 * Path: <b>ImagingManifest.authoringTime</b><br> 1690 * </p> 1691 */ 1692 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHORING_TIME = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHORING_TIME); 1693 1694 /** 1695 * Search parameter: <b>selected-study</b> 1696 * <p> 1697 * Description: <b>Study selected in the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1698 * Type: <b>uri</b><br> 1699 * Path: <b>ImagingManifest.study.uid</b><br> 1700 * </p> 1701 */ 1702 @SearchParamDefinition(name="selected-study", path="ImagingManifest.study.uid", description="Study selected in the ImagingManifest (or a DICOM Key Object Selection which it represents)", type="uri" ) 1703 public static final String SP_SELECTED_STUDY = "selected-study"; 1704 /** 1705 * <b>Fluent Client</b> search parameter constant for <b>selected-study</b> 1706 * <p> 1707 * Description: <b>Study selected in the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1708 * Type: <b>uri</b><br> 1709 * Path: <b>ImagingManifest.study.uid</b><br> 1710 * </p> 1711 */ 1712 public static final ca.uhn.fhir.rest.gclient.UriClientParam SELECTED_STUDY = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_SELECTED_STUDY); 1713 1714 /** 1715 * Search parameter: <b>author</b> 1716 * <p> 1717 * Description: <b>Author of the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1718 * Type: <b>reference</b><br> 1719 * Path: <b>ImagingManifest.author</b><br> 1720 * </p> 1721 */ 1722 @SearchParamDefinition(name="author", path="ImagingManifest.author", description="Author of the ImagingManifest (or a DICOM Key Object Selection which it represents)", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 1723 public static final String SP_AUTHOR = "author"; 1724 /** 1725 * <b>Fluent Client</b> search parameter constant for <b>author</b> 1726 * <p> 1727 * Description: <b>Author of the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1728 * Type: <b>reference</b><br> 1729 * Path: <b>ImagingManifest.author</b><br> 1730 * </p> 1731 */ 1732 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 1733 1734/** 1735 * Constant for fluent queries to be used to add include statements. Specifies 1736 * the path value of "<b>ImagingManifest:author</b>". 1737 */ 1738 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("ImagingManifest:author").toLocked(); 1739 1740 /** 1741 * Search parameter: <b>patient</b> 1742 * <p> 1743 * Description: <b>Subject of the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1744 * Type: <b>reference</b><br> 1745 * Path: <b>ImagingManifest.patient</b><br> 1746 * </p> 1747 */ 1748 @SearchParamDefinition(name="patient", path="ImagingManifest.patient", description="Subject of the ImagingManifest (or a DICOM Key Object Selection which it represents)", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1749 public static final String SP_PATIENT = "patient"; 1750 /** 1751 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1752 * <p> 1753 * Description: <b>Subject of the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1754 * Type: <b>reference</b><br> 1755 * Path: <b>ImagingManifest.patient</b><br> 1756 * </p> 1757 */ 1758 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1759 1760/** 1761 * Constant for fluent queries to be used to add include statements. Specifies 1762 * the path value of "<b>ImagingManifest:patient</b>". 1763 */ 1764 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ImagingManifest:patient").toLocked(); 1765 1766 /** 1767 * Search parameter: <b>imaging-study</b> 1768 * <p> 1769 * Description: <b>ImagingStudy resource selected in the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1770 * Type: <b>reference</b><br> 1771 * Path: <b>ImagingManifest.study.imagingStudy</b><br> 1772 * </p> 1773 */ 1774 @SearchParamDefinition(name="imaging-study", path="ImagingManifest.study.imagingStudy", description="ImagingStudy resource selected in the ImagingManifest (or a DICOM Key Object Selection which it represents)", type="reference", target={ImagingStudy.class } ) 1775 public static final String SP_IMAGING_STUDY = "imaging-study"; 1776 /** 1777 * <b>Fluent Client</b> search parameter constant for <b>imaging-study</b> 1778 * <p> 1779 * Description: <b>ImagingStudy resource selected in the ImagingManifest (or a DICOM Key Object Selection which it represents)</b><br> 1780 * Type: <b>reference</b><br> 1781 * Path: <b>ImagingManifest.study.imagingStudy</b><br> 1782 * </p> 1783 */ 1784 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam IMAGING_STUDY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_IMAGING_STUDY); 1785 1786/** 1787 * Constant for fluent queries to be used to add include statements. Specifies 1788 * the path value of "<b>ImagingManifest:imaging-study</b>". 1789 */ 1790 public static final ca.uhn.fhir.model.api.Include INCLUDE_IMAGING_STUDY = new ca.uhn.fhir.model.api.Include("ImagingManifest:imaging-study").toLocked(); 1791 1792 1793}