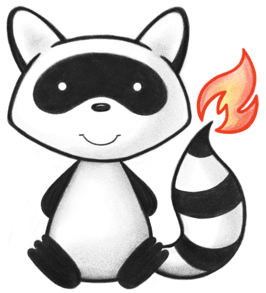
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Representation of the content produced in a DICOM imaging study. A study comprises a set of series, each of which includes a set of Service-Object Pair Instances (SOP Instances - images or other data) acquired or produced in a common context. A series is of only one modality (e.g. X-ray, CT, MR, ultrasound), but a study may have multiple series of different modalities. 050 */ 051@ResourceDef(name="ImagingStudy", profile="http://hl7.org/fhir/Profile/ImagingStudy") 052public class ImagingStudy extends DomainResource { 053 054 public enum InstanceAvailability { 055 /** 056 * null 057 */ 058 ONLINE, 059 /** 060 * null 061 */ 062 OFFLINE, 063 /** 064 * null 065 */ 066 NEARLINE, 067 /** 068 * null 069 */ 070 UNAVAILABLE, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static InstanceAvailability fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("ONLINE".equals(codeString)) 079 return ONLINE; 080 if ("OFFLINE".equals(codeString)) 081 return OFFLINE; 082 if ("NEARLINE".equals(codeString)) 083 return NEARLINE; 084 if ("UNAVAILABLE".equals(codeString)) 085 return UNAVAILABLE; 086 if (Configuration.isAcceptInvalidEnums()) 087 return null; 088 else 089 throw new FHIRException("Unknown InstanceAvailability code '"+codeString+"'"); 090 } 091 public String toCode() { 092 switch (this) { 093 case ONLINE: return "ONLINE"; 094 case OFFLINE: return "OFFLINE"; 095 case NEARLINE: return "NEARLINE"; 096 case UNAVAILABLE: return "UNAVAILABLE"; 097 case NULL: return null; 098 default: return "?"; 099 } 100 } 101 public String getSystem() { 102 switch (this) { 103 case ONLINE: return "http://dicom.nema.org/resources/ontology/DCM"; 104 case OFFLINE: return "http://dicom.nema.org/resources/ontology/DCM"; 105 case NEARLINE: return "http://dicom.nema.org/resources/ontology/DCM"; 106 case UNAVAILABLE: return "http://dicom.nema.org/resources/ontology/DCM"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDefinition() { 112 switch (this) { 113 case ONLINE: return ""; 114 case OFFLINE: return ""; 115 case NEARLINE: return ""; 116 case UNAVAILABLE: return ""; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 public String getDisplay() { 122 switch (this) { 123 case ONLINE: return "ONLINE"; 124 case OFFLINE: return "OFFLINE"; 125 case NEARLINE: return "NEARLINE"; 126 case UNAVAILABLE: return "UNAVAILABLE"; 127 case NULL: return null; 128 default: return "?"; 129 } 130 } 131 } 132 133 public static class InstanceAvailabilityEnumFactory implements EnumFactory<InstanceAvailability> { 134 public InstanceAvailability fromCode(String codeString) throws IllegalArgumentException { 135 if (codeString == null || "".equals(codeString)) 136 if (codeString == null || "".equals(codeString)) 137 return null; 138 if ("ONLINE".equals(codeString)) 139 return InstanceAvailability.ONLINE; 140 if ("OFFLINE".equals(codeString)) 141 return InstanceAvailability.OFFLINE; 142 if ("NEARLINE".equals(codeString)) 143 return InstanceAvailability.NEARLINE; 144 if ("UNAVAILABLE".equals(codeString)) 145 return InstanceAvailability.UNAVAILABLE; 146 throw new IllegalArgumentException("Unknown InstanceAvailability code '"+codeString+"'"); 147 } 148 public Enumeration<InstanceAvailability> fromType(PrimitiveType<?> code) throws FHIRException { 149 if (code == null) 150 return null; 151 if (code.isEmpty()) 152 return new Enumeration<InstanceAvailability>(this); 153 String codeString = code.asStringValue(); 154 if (codeString == null || "".equals(codeString)) 155 return null; 156 if ("ONLINE".equals(codeString)) 157 return new Enumeration<InstanceAvailability>(this, InstanceAvailability.ONLINE); 158 if ("OFFLINE".equals(codeString)) 159 return new Enumeration<InstanceAvailability>(this, InstanceAvailability.OFFLINE); 160 if ("NEARLINE".equals(codeString)) 161 return new Enumeration<InstanceAvailability>(this, InstanceAvailability.NEARLINE); 162 if ("UNAVAILABLE".equals(codeString)) 163 return new Enumeration<InstanceAvailability>(this, InstanceAvailability.UNAVAILABLE); 164 throw new FHIRException("Unknown InstanceAvailability code '"+codeString+"'"); 165 } 166 public String toCode(InstanceAvailability code) { 167 if (code == InstanceAvailability.NULL) 168 return null; 169 if (code == InstanceAvailability.ONLINE) 170 return "ONLINE"; 171 if (code == InstanceAvailability.OFFLINE) 172 return "OFFLINE"; 173 if (code == InstanceAvailability.NEARLINE) 174 return "NEARLINE"; 175 if (code == InstanceAvailability.UNAVAILABLE) 176 return "UNAVAILABLE"; 177 return "?"; 178 } 179 public String toSystem(InstanceAvailability code) { 180 return code.getSystem(); 181 } 182 } 183 184 @Block() 185 public static class ImagingStudySeriesComponent extends BackboneElement implements IBaseBackboneElement { 186 /** 187 * Formal identifier for this series. 188 */ 189 @Child(name = "uid", type = {OidType.class}, order=1, min=1, max=1, modifier=false, summary=true) 190 @Description(shortDefinition="Formal DICOM identifier for this series", formalDefinition="Formal identifier for this series." ) 191 protected OidType uid; 192 193 /** 194 * The numeric identifier of this series in the study. 195 */ 196 @Child(name = "number", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=true) 197 @Description(shortDefinition="Numeric identifier of this series", formalDefinition="The numeric identifier of this series in the study." ) 198 protected UnsignedIntType number; 199 200 /** 201 * The modality of this series sequence. 202 */ 203 @Child(name = "modality", type = {Coding.class}, order=3, min=1, max=1, modifier=false, summary=true) 204 @Description(shortDefinition="The modality of the instances in the series", formalDefinition="The modality of this series sequence." ) 205 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/dicom-cid29") 206 protected Coding modality; 207 208 /** 209 * A description of the series. 210 */ 211 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=true) 212 @Description(shortDefinition="A short human readable summary of the series", formalDefinition="A description of the series." ) 213 protected StringType description; 214 215 /** 216 * Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 217 */ 218 @Child(name = "numberOfInstances", type = {UnsignedIntType.class}, order=5, min=0, max=1, modifier=false, summary=true) 219 @Description(shortDefinition="Number of Series Related Instances", formalDefinition="Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present." ) 220 protected UnsignedIntType numberOfInstances; 221 222 /** 223 * Availability of series (online, offline or nearline). 224 */ 225 @Child(name = "availability", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=true) 226 @Description(shortDefinition="ONLINE | OFFLINE | NEARLINE | UNAVAILABLE", formalDefinition="Availability of series (online, offline or nearline)." ) 227 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/instance-availability") 228 protected Enumeration<InstanceAvailability> availability; 229 230 /** 231 * The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type. 232 */ 233 @Child(name = "endpoint", type = {Endpoint.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 234 @Description(shortDefinition="Series access endpoint", formalDefinition="The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type." ) 235 protected List<Reference> endpoint; 236 /** 237 * The actual objects that are the target of the reference (The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type.) 238 */ 239 protected List<Endpoint> endpointTarget; 240 241 242 /** 243 * The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality. 244 */ 245 @Child(name = "bodySite", type = {Coding.class}, order=8, min=0, max=1, modifier=false, summary=true) 246 @Description(shortDefinition="Body part examined", formalDefinition="The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality." ) 247 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 248 protected Coding bodySite; 249 250 /** 251 * The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite. 252 */ 253 @Child(name = "laterality", type = {Coding.class}, order=9, min=0, max=1, modifier=false, summary=true) 254 @Description(shortDefinition="Body part laterality", formalDefinition="The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite." ) 255 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/bodysite-laterality") 256 protected Coding laterality; 257 258 /** 259 * The date and time the series was started. 260 */ 261 @Child(name = "started", type = {DateTimeType.class}, order=10, min=0, max=1, modifier=false, summary=true) 262 @Description(shortDefinition="When the series started", formalDefinition="The date and time the series was started." ) 263 protected DateTimeType started; 264 265 /** 266 * The physician or operator (often the radiology technician) who performed the series. The performer is recorded at the series level, since each series in a study may be performed by a different practitioner, at different times, and using different devices. A series may be performed by multiple practitioners. 267 */ 268 @Child(name = "performer", type = {Practitioner.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 269 @Description(shortDefinition="Who performed the series", formalDefinition="The physician or operator (often the radiology technician) who performed the series. The performer is recorded at the series level, since each series in a study may be performed by a different practitioner, at different times, and using different devices. A series may be performed by multiple practitioners." ) 270 protected List<Reference> performer; 271 /** 272 * The actual objects that are the target of the reference (The physician or operator (often the radiology technician) who performed the series. The performer is recorded at the series level, since each series in a study may be performed by a different practitioner, at different times, and using different devices. A series may be performed by multiple practitioners.) 273 */ 274 protected List<Practitioner> performerTarget; 275 276 277 /** 278 * A single SOP instance within the series, e.g. an image, or presentation state. 279 */ 280 @Child(name = "instance", type = {}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 281 @Description(shortDefinition="A single SOP instance from the series", formalDefinition="A single SOP instance within the series, e.g. an image, or presentation state." ) 282 protected List<ImagingStudySeriesInstanceComponent> instance; 283 284 private static final long serialVersionUID = -1469376087L; 285 286 /** 287 * Constructor 288 */ 289 public ImagingStudySeriesComponent() { 290 super(); 291 } 292 293 /** 294 * Constructor 295 */ 296 public ImagingStudySeriesComponent(OidType uid, Coding modality) { 297 super(); 298 this.uid = uid; 299 this.modality = modality; 300 } 301 302 /** 303 * @return {@link #uid} (Formal identifier for this series.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 304 */ 305 public OidType getUidElement() { 306 if (this.uid == null) 307 if (Configuration.errorOnAutoCreate()) 308 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.uid"); 309 else if (Configuration.doAutoCreate()) 310 this.uid = new OidType(); // bb 311 return this.uid; 312 } 313 314 public boolean hasUidElement() { 315 return this.uid != null && !this.uid.isEmpty(); 316 } 317 318 public boolean hasUid() { 319 return this.uid != null && !this.uid.isEmpty(); 320 } 321 322 /** 323 * @param value {@link #uid} (Formal identifier for this series.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 324 */ 325 public ImagingStudySeriesComponent setUidElement(OidType value) { 326 this.uid = value; 327 return this; 328 } 329 330 /** 331 * @return Formal identifier for this series. 332 */ 333 public String getUid() { 334 return this.uid == null ? null : this.uid.getValue(); 335 } 336 337 /** 338 * @param value Formal identifier for this series. 339 */ 340 public ImagingStudySeriesComponent setUid(String value) { 341 if (this.uid == null) 342 this.uid = new OidType(); 343 this.uid.setValue(value); 344 return this; 345 } 346 347 /** 348 * @return {@link #number} (The numeric identifier of this series in the study.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 349 */ 350 public UnsignedIntType getNumberElement() { 351 if (this.number == null) 352 if (Configuration.errorOnAutoCreate()) 353 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.number"); 354 else if (Configuration.doAutoCreate()) 355 this.number = new UnsignedIntType(); // bb 356 return this.number; 357 } 358 359 public boolean hasNumberElement() { 360 return this.number != null && !this.number.isEmpty(); 361 } 362 363 public boolean hasNumber() { 364 return this.number != null && !this.number.isEmpty(); 365 } 366 367 /** 368 * @param value {@link #number} (The numeric identifier of this series in the study.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 369 */ 370 public ImagingStudySeriesComponent setNumberElement(UnsignedIntType value) { 371 this.number = value; 372 return this; 373 } 374 375 /** 376 * @return The numeric identifier of this series in the study. 377 */ 378 public int getNumber() { 379 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 380 } 381 382 /** 383 * @param value The numeric identifier of this series in the study. 384 */ 385 public ImagingStudySeriesComponent setNumber(int value) { 386 if (this.number == null) 387 this.number = new UnsignedIntType(); 388 this.number.setValue(value); 389 return this; 390 } 391 392 /** 393 * @return {@link #modality} (The modality of this series sequence.) 394 */ 395 public Coding getModality() { 396 if (this.modality == null) 397 if (Configuration.errorOnAutoCreate()) 398 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.modality"); 399 else if (Configuration.doAutoCreate()) 400 this.modality = new Coding(); // cc 401 return this.modality; 402 } 403 404 public boolean hasModality() { 405 return this.modality != null && !this.modality.isEmpty(); 406 } 407 408 /** 409 * @param value {@link #modality} (The modality of this series sequence.) 410 */ 411 public ImagingStudySeriesComponent setModality(Coding value) { 412 this.modality = value; 413 return this; 414 } 415 416 /** 417 * @return {@link #description} (A description of the series.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 418 */ 419 public StringType getDescriptionElement() { 420 if (this.description == null) 421 if (Configuration.errorOnAutoCreate()) 422 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.description"); 423 else if (Configuration.doAutoCreate()) 424 this.description = new StringType(); // bb 425 return this.description; 426 } 427 428 public boolean hasDescriptionElement() { 429 return this.description != null && !this.description.isEmpty(); 430 } 431 432 public boolean hasDescription() { 433 return this.description != null && !this.description.isEmpty(); 434 } 435 436 /** 437 * @param value {@link #description} (A description of the series.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 438 */ 439 public ImagingStudySeriesComponent setDescriptionElement(StringType value) { 440 this.description = value; 441 return this; 442 } 443 444 /** 445 * @return A description of the series. 446 */ 447 public String getDescription() { 448 return this.description == null ? null : this.description.getValue(); 449 } 450 451 /** 452 * @param value A description of the series. 453 */ 454 public ImagingStudySeriesComponent setDescription(String value) { 455 if (Utilities.noString(value)) 456 this.description = null; 457 else { 458 if (this.description == null) 459 this.description = new StringType(); 460 this.description.setValue(value); 461 } 462 return this; 463 } 464 465 /** 466 * @return {@link #numberOfInstances} (Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfInstances" gives direct access to the value 467 */ 468 public UnsignedIntType getNumberOfInstancesElement() { 469 if (this.numberOfInstances == null) 470 if (Configuration.errorOnAutoCreate()) 471 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.numberOfInstances"); 472 else if (Configuration.doAutoCreate()) 473 this.numberOfInstances = new UnsignedIntType(); // bb 474 return this.numberOfInstances; 475 } 476 477 public boolean hasNumberOfInstancesElement() { 478 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 479 } 480 481 public boolean hasNumberOfInstances() { 482 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 483 } 484 485 /** 486 * @param value {@link #numberOfInstances} (Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfInstances" gives direct access to the value 487 */ 488 public ImagingStudySeriesComponent setNumberOfInstancesElement(UnsignedIntType value) { 489 this.numberOfInstances = value; 490 return this; 491 } 492 493 /** 494 * @return Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 495 */ 496 public int getNumberOfInstances() { 497 return this.numberOfInstances == null || this.numberOfInstances.isEmpty() ? 0 : this.numberOfInstances.getValue(); 498 } 499 500 /** 501 * @param value Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 502 */ 503 public ImagingStudySeriesComponent setNumberOfInstances(int value) { 504 if (this.numberOfInstances == null) 505 this.numberOfInstances = new UnsignedIntType(); 506 this.numberOfInstances.setValue(value); 507 return this; 508 } 509 510 /** 511 * @return {@link #availability} (Availability of series (online, offline or nearline).). This is the underlying object with id, value and extensions. The accessor "getAvailability" gives direct access to the value 512 */ 513 public Enumeration<InstanceAvailability> getAvailabilityElement() { 514 if (this.availability == null) 515 if (Configuration.errorOnAutoCreate()) 516 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.availability"); 517 else if (Configuration.doAutoCreate()) 518 this.availability = new Enumeration<InstanceAvailability>(new InstanceAvailabilityEnumFactory()); // bb 519 return this.availability; 520 } 521 522 public boolean hasAvailabilityElement() { 523 return this.availability != null && !this.availability.isEmpty(); 524 } 525 526 public boolean hasAvailability() { 527 return this.availability != null && !this.availability.isEmpty(); 528 } 529 530 /** 531 * @param value {@link #availability} (Availability of series (online, offline or nearline).). This is the underlying object with id, value and extensions. The accessor "getAvailability" gives direct access to the value 532 */ 533 public ImagingStudySeriesComponent setAvailabilityElement(Enumeration<InstanceAvailability> value) { 534 this.availability = value; 535 return this; 536 } 537 538 /** 539 * @return Availability of series (online, offline or nearline). 540 */ 541 public InstanceAvailability getAvailability() { 542 return this.availability == null ? null : this.availability.getValue(); 543 } 544 545 /** 546 * @param value Availability of series (online, offline or nearline). 547 */ 548 public ImagingStudySeriesComponent setAvailability(InstanceAvailability value) { 549 if (value == null) 550 this.availability = null; 551 else { 552 if (this.availability == null) 553 this.availability = new Enumeration<InstanceAvailability>(new InstanceAvailabilityEnumFactory()); 554 this.availability.setValue(value); 555 } 556 return this; 557 } 558 559 /** 560 * @return {@link #endpoint} (The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type.) 561 */ 562 public List<Reference> getEndpoint() { 563 if (this.endpoint == null) 564 this.endpoint = new ArrayList<Reference>(); 565 return this.endpoint; 566 } 567 568 /** 569 * @return Returns a reference to <code>this</code> for easy method chaining 570 */ 571 public ImagingStudySeriesComponent setEndpoint(List<Reference> theEndpoint) { 572 this.endpoint = theEndpoint; 573 return this; 574 } 575 576 public boolean hasEndpoint() { 577 if (this.endpoint == null) 578 return false; 579 for (Reference item : this.endpoint) 580 if (!item.isEmpty()) 581 return true; 582 return false; 583 } 584 585 public Reference addEndpoint() { //3 586 Reference t = new Reference(); 587 if (this.endpoint == null) 588 this.endpoint = new ArrayList<Reference>(); 589 this.endpoint.add(t); 590 return t; 591 } 592 593 public ImagingStudySeriesComponent addEndpoint(Reference t) { //3 594 if (t == null) 595 return this; 596 if (this.endpoint == null) 597 this.endpoint = new ArrayList<Reference>(); 598 this.endpoint.add(t); 599 return this; 600 } 601 602 /** 603 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 604 */ 605 public Reference getEndpointFirstRep() { 606 if (getEndpoint().isEmpty()) { 607 addEndpoint(); 608 } 609 return getEndpoint().get(0); 610 } 611 612 /** 613 * @deprecated Use Reference#setResource(IBaseResource) instead 614 */ 615 @Deprecated 616 public List<Endpoint> getEndpointTarget() { 617 if (this.endpointTarget == null) 618 this.endpointTarget = new ArrayList<Endpoint>(); 619 return this.endpointTarget; 620 } 621 622 /** 623 * @deprecated Use Reference#setResource(IBaseResource) instead 624 */ 625 @Deprecated 626 public Endpoint addEndpointTarget() { 627 Endpoint r = new Endpoint(); 628 if (this.endpointTarget == null) 629 this.endpointTarget = new ArrayList<Endpoint>(); 630 this.endpointTarget.add(r); 631 return r; 632 } 633 634 /** 635 * @return {@link #bodySite} (The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.) 636 */ 637 public Coding getBodySite() { 638 if (this.bodySite == null) 639 if (Configuration.errorOnAutoCreate()) 640 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.bodySite"); 641 else if (Configuration.doAutoCreate()) 642 this.bodySite = new Coding(); // cc 643 return this.bodySite; 644 } 645 646 public boolean hasBodySite() { 647 return this.bodySite != null && !this.bodySite.isEmpty(); 648 } 649 650 /** 651 * @param value {@link #bodySite} (The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.) 652 */ 653 public ImagingStudySeriesComponent setBodySite(Coding value) { 654 this.bodySite = value; 655 return this; 656 } 657 658 /** 659 * @return {@link #laterality} (The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.) 660 */ 661 public Coding getLaterality() { 662 if (this.laterality == null) 663 if (Configuration.errorOnAutoCreate()) 664 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.laterality"); 665 else if (Configuration.doAutoCreate()) 666 this.laterality = new Coding(); // cc 667 return this.laterality; 668 } 669 670 public boolean hasLaterality() { 671 return this.laterality != null && !this.laterality.isEmpty(); 672 } 673 674 /** 675 * @param value {@link #laterality} (The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.) 676 */ 677 public ImagingStudySeriesComponent setLaterality(Coding value) { 678 this.laterality = value; 679 return this; 680 } 681 682 /** 683 * @return {@link #started} (The date and time the series was started.). This is the underlying object with id, value and extensions. The accessor "getStarted" gives direct access to the value 684 */ 685 public DateTimeType getStartedElement() { 686 if (this.started == null) 687 if (Configuration.errorOnAutoCreate()) 688 throw new Error("Attempt to auto-create ImagingStudySeriesComponent.started"); 689 else if (Configuration.doAutoCreate()) 690 this.started = new DateTimeType(); // bb 691 return this.started; 692 } 693 694 public boolean hasStartedElement() { 695 return this.started != null && !this.started.isEmpty(); 696 } 697 698 public boolean hasStarted() { 699 return this.started != null && !this.started.isEmpty(); 700 } 701 702 /** 703 * @param value {@link #started} (The date and time the series was started.). This is the underlying object with id, value and extensions. The accessor "getStarted" gives direct access to the value 704 */ 705 public ImagingStudySeriesComponent setStartedElement(DateTimeType value) { 706 this.started = value; 707 return this; 708 } 709 710 /** 711 * @return The date and time the series was started. 712 */ 713 public Date getStarted() { 714 return this.started == null ? null : this.started.getValue(); 715 } 716 717 /** 718 * @param value The date and time the series was started. 719 */ 720 public ImagingStudySeriesComponent setStarted(Date value) { 721 if (value == null) 722 this.started = null; 723 else { 724 if (this.started == null) 725 this.started = new DateTimeType(); 726 this.started.setValue(value); 727 } 728 return this; 729 } 730 731 /** 732 * @return {@link #performer} (The physician or operator (often the radiology technician) who performed the series. The performer is recorded at the series level, since each series in a study may be performed by a different practitioner, at different times, and using different devices. A series may be performed by multiple practitioners.) 733 */ 734 public List<Reference> getPerformer() { 735 if (this.performer == null) 736 this.performer = new ArrayList<Reference>(); 737 return this.performer; 738 } 739 740 /** 741 * @return Returns a reference to <code>this</code> for easy method chaining 742 */ 743 public ImagingStudySeriesComponent setPerformer(List<Reference> thePerformer) { 744 this.performer = thePerformer; 745 return this; 746 } 747 748 public boolean hasPerformer() { 749 if (this.performer == null) 750 return false; 751 for (Reference item : this.performer) 752 if (!item.isEmpty()) 753 return true; 754 return false; 755 } 756 757 public Reference addPerformer() { //3 758 Reference t = new Reference(); 759 if (this.performer == null) 760 this.performer = new ArrayList<Reference>(); 761 this.performer.add(t); 762 return t; 763 } 764 765 public ImagingStudySeriesComponent addPerformer(Reference t) { //3 766 if (t == null) 767 return this; 768 if (this.performer == null) 769 this.performer = new ArrayList<Reference>(); 770 this.performer.add(t); 771 return this; 772 } 773 774 /** 775 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 776 */ 777 public Reference getPerformerFirstRep() { 778 if (getPerformer().isEmpty()) { 779 addPerformer(); 780 } 781 return getPerformer().get(0); 782 } 783 784 /** 785 * @deprecated Use Reference#setResource(IBaseResource) instead 786 */ 787 @Deprecated 788 public List<Practitioner> getPerformerTarget() { 789 if (this.performerTarget == null) 790 this.performerTarget = new ArrayList<Practitioner>(); 791 return this.performerTarget; 792 } 793 794 /** 795 * @deprecated Use Reference#setResource(IBaseResource) instead 796 */ 797 @Deprecated 798 public Practitioner addPerformerTarget() { 799 Practitioner r = new Practitioner(); 800 if (this.performerTarget == null) 801 this.performerTarget = new ArrayList<Practitioner>(); 802 this.performerTarget.add(r); 803 return r; 804 } 805 806 /** 807 * @return {@link #instance} (A single SOP instance within the series, e.g. an image, or presentation state.) 808 */ 809 public List<ImagingStudySeriesInstanceComponent> getInstance() { 810 if (this.instance == null) 811 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 812 return this.instance; 813 } 814 815 /** 816 * @return Returns a reference to <code>this</code> for easy method chaining 817 */ 818 public ImagingStudySeriesComponent setInstance(List<ImagingStudySeriesInstanceComponent> theInstance) { 819 this.instance = theInstance; 820 return this; 821 } 822 823 public boolean hasInstance() { 824 if (this.instance == null) 825 return false; 826 for (ImagingStudySeriesInstanceComponent item : this.instance) 827 if (!item.isEmpty()) 828 return true; 829 return false; 830 } 831 832 public ImagingStudySeriesInstanceComponent addInstance() { //3 833 ImagingStudySeriesInstanceComponent t = new ImagingStudySeriesInstanceComponent(); 834 if (this.instance == null) 835 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 836 this.instance.add(t); 837 return t; 838 } 839 840 public ImagingStudySeriesComponent addInstance(ImagingStudySeriesInstanceComponent t) { //3 841 if (t == null) 842 return this; 843 if (this.instance == null) 844 this.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 845 this.instance.add(t); 846 return this; 847 } 848 849 /** 850 * @return The first repetition of repeating field {@link #instance}, creating it if it does not already exist 851 */ 852 public ImagingStudySeriesInstanceComponent getInstanceFirstRep() { 853 if (getInstance().isEmpty()) { 854 addInstance(); 855 } 856 return getInstance().get(0); 857 } 858 859 protected void listChildren(List<Property> children) { 860 super.listChildren(children); 861 children.add(new Property("uid", "oid", "Formal identifier for this series.", 0, 1, uid)); 862 children.add(new Property("number", "unsignedInt", "The numeric identifier of this series in the study.", 0, 1, number)); 863 children.add(new Property("modality", "Coding", "The modality of this series sequence.", 0, 1, modality)); 864 children.add(new Property("description", "string", "A description of the series.", 0, 1, description)); 865 children.add(new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 0, 1, numberOfInstances)); 866 children.add(new Property("availability", "code", "Availability of series (online, offline or nearline).", 0, 1, availability)); 867 children.add(new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 868 children.add(new Property("bodySite", "Coding", "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.", 0, 1, bodySite)); 869 children.add(new Property("laterality", "Coding", "The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.", 0, 1, laterality)); 870 children.add(new Property("started", "dateTime", "The date and time the series was started.", 0, 1, started)); 871 children.add(new Property("performer", "Reference(Practitioner)", "The physician or operator (often the radiology technician) who performed the series. The performer is recorded at the series level, since each series in a study may be performed by a different practitioner, at different times, and using different devices. A series may be performed by multiple practitioners.", 0, java.lang.Integer.MAX_VALUE, performer)); 872 children.add(new Property("instance", "", "A single SOP instance within the series, e.g. an image, or presentation state.", 0, java.lang.Integer.MAX_VALUE, instance)); 873 } 874 875 @Override 876 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 877 switch (_hash) { 878 case 115792: /*uid*/ return new Property("uid", "oid", "Formal identifier for this series.", 0, 1, uid); 879 case -1034364087: /*number*/ return new Property("number", "unsignedInt", "The numeric identifier of this series in the study.", 0, 1, number); 880 case -622722335: /*modality*/ return new Property("modality", "Coding", "The modality of this series sequence.", 0, 1, modality); 881 case -1724546052: /*description*/ return new Property("description", "string", "A description of the series.", 0, 1, description); 882 case -1043544226: /*numberOfInstances*/ return new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in the Study. The value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 0, 1, numberOfInstances); 883 case 1997542747: /*availability*/ return new Property("availability", "code", "Availability of series (online, offline or nearline).", 0, 1, availability); 884 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for this series. See implementation notes for information about using DICOM endpoints. A series-level endpoint, if present, has precedence over a study-level endpoint with the same Endpoint.type.", 0, java.lang.Integer.MAX_VALUE, endpoint); 885 case 1702620169: /*bodySite*/ return new Property("bodySite", "Coding", "The anatomic structures examined. See DICOM Part 16 Annex L (http://dicom.nema.org/medical/dicom/current/output/chtml/part16/chapter_L.html) for DICOM to SNOMED-CT mappings. The bodySite may indicate the laterality of body part imaged; if so, it shall be consistent with any content of ImagingStudy.series.laterality.", 0, 1, bodySite); 886 case -170291817: /*laterality*/ return new Property("laterality", "Coding", "The laterality of the (possibly paired) anatomic structures examined. E.g., the left knee, both lungs, or unpaired abdomen. If present, shall be consistent with any laterality information indicated in ImagingStudy.series.bodySite.", 0, 1, laterality); 887 case -1897185151: /*started*/ return new Property("started", "dateTime", "The date and time the series was started.", 0, 1, started); 888 case 481140686: /*performer*/ return new Property("performer", "Reference(Practitioner)", "The physician or operator (often the radiology technician) who performed the series. The performer is recorded at the series level, since each series in a study may be performed by a different practitioner, at different times, and using different devices. A series may be performed by multiple practitioners.", 0, java.lang.Integer.MAX_VALUE, performer); 889 case 555127957: /*instance*/ return new Property("instance", "", "A single SOP instance within the series, e.g. an image, or presentation state.", 0, java.lang.Integer.MAX_VALUE, instance); 890 default: return super.getNamedProperty(_hash, _name, _checkValid); 891 } 892 893 } 894 895 @Override 896 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 897 switch (hash) { 898 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // OidType 899 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // UnsignedIntType 900 case -622722335: /*modality*/ return this.modality == null ? new Base[0] : new Base[] {this.modality}; // Coding 901 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 902 case -1043544226: /*numberOfInstances*/ return this.numberOfInstances == null ? new Base[0] : new Base[] {this.numberOfInstances}; // UnsignedIntType 903 case 1997542747: /*availability*/ return this.availability == null ? new Base[0] : new Base[] {this.availability}; // Enumeration<InstanceAvailability> 904 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 905 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // Coding 906 case -170291817: /*laterality*/ return this.laterality == null ? new Base[0] : new Base[] {this.laterality}; // Coding 907 case -1897185151: /*started*/ return this.started == null ? new Base[0] : new Base[] {this.started}; // DateTimeType 908 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // Reference 909 case 555127957: /*instance*/ return this.instance == null ? new Base[0] : this.instance.toArray(new Base[this.instance.size()]); // ImagingStudySeriesInstanceComponent 910 default: return super.getProperty(hash, name, checkValid); 911 } 912 913 } 914 915 @Override 916 public Base setProperty(int hash, String name, Base value) throws FHIRException { 917 switch (hash) { 918 case 115792: // uid 919 this.uid = castToOid(value); // OidType 920 return value; 921 case -1034364087: // number 922 this.number = castToUnsignedInt(value); // UnsignedIntType 923 return value; 924 case -622722335: // modality 925 this.modality = castToCoding(value); // Coding 926 return value; 927 case -1724546052: // description 928 this.description = castToString(value); // StringType 929 return value; 930 case -1043544226: // numberOfInstances 931 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 932 return value; 933 case 1997542747: // availability 934 value = new InstanceAvailabilityEnumFactory().fromType(castToCode(value)); 935 this.availability = (Enumeration) value; // Enumeration<InstanceAvailability> 936 return value; 937 case 1741102485: // endpoint 938 this.getEndpoint().add(castToReference(value)); // Reference 939 return value; 940 case 1702620169: // bodySite 941 this.bodySite = castToCoding(value); // Coding 942 return value; 943 case -170291817: // laterality 944 this.laterality = castToCoding(value); // Coding 945 return value; 946 case -1897185151: // started 947 this.started = castToDateTime(value); // DateTimeType 948 return value; 949 case 481140686: // performer 950 this.getPerformer().add(castToReference(value)); // Reference 951 return value; 952 case 555127957: // instance 953 this.getInstance().add((ImagingStudySeriesInstanceComponent) value); // ImagingStudySeriesInstanceComponent 954 return value; 955 default: return super.setProperty(hash, name, value); 956 } 957 958 } 959 960 @Override 961 public Base setProperty(String name, Base value) throws FHIRException { 962 if (name.equals("uid")) { 963 this.uid = castToOid(value); // OidType 964 } else if (name.equals("number")) { 965 this.number = castToUnsignedInt(value); // UnsignedIntType 966 } else if (name.equals("modality")) { 967 this.modality = castToCoding(value); // Coding 968 } else if (name.equals("description")) { 969 this.description = castToString(value); // StringType 970 } else if (name.equals("numberOfInstances")) { 971 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 972 } else if (name.equals("availability")) { 973 value = new InstanceAvailabilityEnumFactory().fromType(castToCode(value)); 974 this.availability = (Enumeration) value; // Enumeration<InstanceAvailability> 975 } else if (name.equals("endpoint")) { 976 this.getEndpoint().add(castToReference(value)); 977 } else if (name.equals("bodySite")) { 978 this.bodySite = castToCoding(value); // Coding 979 } else if (name.equals("laterality")) { 980 this.laterality = castToCoding(value); // Coding 981 } else if (name.equals("started")) { 982 this.started = castToDateTime(value); // DateTimeType 983 } else if (name.equals("performer")) { 984 this.getPerformer().add(castToReference(value)); 985 } else if (name.equals("instance")) { 986 this.getInstance().add((ImagingStudySeriesInstanceComponent) value); 987 } else 988 return super.setProperty(name, value); 989 return value; 990 } 991 992 @Override 993 public Base makeProperty(int hash, String name) throws FHIRException { 994 switch (hash) { 995 case 115792: return getUidElement(); 996 case -1034364087: return getNumberElement(); 997 case -622722335: return getModality(); 998 case -1724546052: return getDescriptionElement(); 999 case -1043544226: return getNumberOfInstancesElement(); 1000 case 1997542747: return getAvailabilityElement(); 1001 case 1741102485: return addEndpoint(); 1002 case 1702620169: return getBodySite(); 1003 case -170291817: return getLaterality(); 1004 case -1897185151: return getStartedElement(); 1005 case 481140686: return addPerformer(); 1006 case 555127957: return addInstance(); 1007 default: return super.makeProperty(hash, name); 1008 } 1009 1010 } 1011 1012 @Override 1013 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1014 switch (hash) { 1015 case 115792: /*uid*/ return new String[] {"oid"}; 1016 case -1034364087: /*number*/ return new String[] {"unsignedInt"}; 1017 case -622722335: /*modality*/ return new String[] {"Coding"}; 1018 case -1724546052: /*description*/ return new String[] {"string"}; 1019 case -1043544226: /*numberOfInstances*/ return new String[] {"unsignedInt"}; 1020 case 1997542747: /*availability*/ return new String[] {"code"}; 1021 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1022 case 1702620169: /*bodySite*/ return new String[] {"Coding"}; 1023 case -170291817: /*laterality*/ return new String[] {"Coding"}; 1024 case -1897185151: /*started*/ return new String[] {"dateTime"}; 1025 case 481140686: /*performer*/ return new String[] {"Reference"}; 1026 case 555127957: /*instance*/ return new String[] {}; 1027 default: return super.getTypesForProperty(hash, name); 1028 } 1029 1030 } 1031 1032 @Override 1033 public Base addChild(String name) throws FHIRException { 1034 if (name.equals("uid")) { 1035 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.uid"); 1036 } 1037 else if (name.equals("number")) { 1038 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.number"); 1039 } 1040 else if (name.equals("modality")) { 1041 this.modality = new Coding(); 1042 return this.modality; 1043 } 1044 else if (name.equals("description")) { 1045 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.description"); 1046 } 1047 else if (name.equals("numberOfInstances")) { 1048 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfInstances"); 1049 } 1050 else if (name.equals("availability")) { 1051 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.availability"); 1052 } 1053 else if (name.equals("endpoint")) { 1054 return addEndpoint(); 1055 } 1056 else if (name.equals("bodySite")) { 1057 this.bodySite = new Coding(); 1058 return this.bodySite; 1059 } 1060 else if (name.equals("laterality")) { 1061 this.laterality = new Coding(); 1062 return this.laterality; 1063 } 1064 else if (name.equals("started")) { 1065 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.started"); 1066 } 1067 else if (name.equals("performer")) { 1068 return addPerformer(); 1069 } 1070 else if (name.equals("instance")) { 1071 return addInstance(); 1072 } 1073 else 1074 return super.addChild(name); 1075 } 1076 1077 public ImagingStudySeriesComponent copy() { 1078 ImagingStudySeriesComponent dst = new ImagingStudySeriesComponent(); 1079 copyValues(dst); 1080 dst.uid = uid == null ? null : uid.copy(); 1081 dst.number = number == null ? null : number.copy(); 1082 dst.modality = modality == null ? null : modality.copy(); 1083 dst.description = description == null ? null : description.copy(); 1084 dst.numberOfInstances = numberOfInstances == null ? null : numberOfInstances.copy(); 1085 dst.availability = availability == null ? null : availability.copy(); 1086 if (endpoint != null) { 1087 dst.endpoint = new ArrayList<Reference>(); 1088 for (Reference i : endpoint) 1089 dst.endpoint.add(i.copy()); 1090 }; 1091 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1092 dst.laterality = laterality == null ? null : laterality.copy(); 1093 dst.started = started == null ? null : started.copy(); 1094 if (performer != null) { 1095 dst.performer = new ArrayList<Reference>(); 1096 for (Reference i : performer) 1097 dst.performer.add(i.copy()); 1098 }; 1099 if (instance != null) { 1100 dst.instance = new ArrayList<ImagingStudySeriesInstanceComponent>(); 1101 for (ImagingStudySeriesInstanceComponent i : instance) 1102 dst.instance.add(i.copy()); 1103 }; 1104 return dst; 1105 } 1106 1107 @Override 1108 public boolean equalsDeep(Base other_) { 1109 if (!super.equalsDeep(other_)) 1110 return false; 1111 if (!(other_ instanceof ImagingStudySeriesComponent)) 1112 return false; 1113 ImagingStudySeriesComponent o = (ImagingStudySeriesComponent) other_; 1114 return compareDeep(uid, o.uid, true) && compareDeep(number, o.number, true) && compareDeep(modality, o.modality, true) 1115 && compareDeep(description, o.description, true) && compareDeep(numberOfInstances, o.numberOfInstances, true) 1116 && compareDeep(availability, o.availability, true) && compareDeep(endpoint, o.endpoint, true) && compareDeep(bodySite, o.bodySite, true) 1117 && compareDeep(laterality, o.laterality, true) && compareDeep(started, o.started, true) && compareDeep(performer, o.performer, true) 1118 && compareDeep(instance, o.instance, true); 1119 } 1120 1121 @Override 1122 public boolean equalsShallow(Base other_) { 1123 if (!super.equalsShallow(other_)) 1124 return false; 1125 if (!(other_ instanceof ImagingStudySeriesComponent)) 1126 return false; 1127 ImagingStudySeriesComponent o = (ImagingStudySeriesComponent) other_; 1128 return compareValues(uid, o.uid, true) && compareValues(number, o.number, true) && compareValues(description, o.description, true) 1129 && compareValues(numberOfInstances, o.numberOfInstances, true) && compareValues(availability, o.availability, true) 1130 && compareValues(started, o.started, true); 1131 } 1132 1133 public boolean isEmpty() { 1134 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, number, modality, description 1135 , numberOfInstances, availability, endpoint, bodySite, laterality, started, performer 1136 , instance); 1137 } 1138 1139 public String fhirType() { 1140 return "ImagingStudy.series"; 1141 1142 } 1143 1144 } 1145 1146 @Block() 1147 public static class ImagingStudySeriesInstanceComponent extends BackboneElement implements IBaseBackboneElement { 1148 /** 1149 * Formal identifier for this image or other content. 1150 */ 1151 @Child(name = "uid", type = {OidType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1152 @Description(shortDefinition="Formal DICOM identifier for this instance", formalDefinition="Formal identifier for this image or other content." ) 1153 protected OidType uid; 1154 1155 /** 1156 * The number of instance in the series. 1157 */ 1158 @Child(name = "number", type = {UnsignedIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1159 @Description(shortDefinition="The number of this instance in the series", formalDefinition="The number of instance in the series." ) 1160 protected UnsignedIntType number; 1161 1162 /** 1163 * DICOM instance type. 1164 */ 1165 @Child(name = "sopClass", type = {OidType.class}, order=3, min=1, max=1, modifier=false, summary=false) 1166 @Description(shortDefinition="DICOM class type", formalDefinition="DICOM instance type." ) 1167 protected OidType sopClass; 1168 1169 /** 1170 * The description of the instance. 1171 */ 1172 @Child(name = "title", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1173 @Description(shortDefinition="Description of instance", formalDefinition="The description of the instance." ) 1174 protected StringType title; 1175 1176 private static final long serialVersionUID = -771526344L; 1177 1178 /** 1179 * Constructor 1180 */ 1181 public ImagingStudySeriesInstanceComponent() { 1182 super(); 1183 } 1184 1185 /** 1186 * Constructor 1187 */ 1188 public ImagingStudySeriesInstanceComponent(OidType uid, OidType sopClass) { 1189 super(); 1190 this.uid = uid; 1191 this.sopClass = sopClass; 1192 } 1193 1194 /** 1195 * @return {@link #uid} (Formal identifier for this image or other content.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 1196 */ 1197 public OidType getUidElement() { 1198 if (this.uid == null) 1199 if (Configuration.errorOnAutoCreate()) 1200 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.uid"); 1201 else if (Configuration.doAutoCreate()) 1202 this.uid = new OidType(); // bb 1203 return this.uid; 1204 } 1205 1206 public boolean hasUidElement() { 1207 return this.uid != null && !this.uid.isEmpty(); 1208 } 1209 1210 public boolean hasUid() { 1211 return this.uid != null && !this.uid.isEmpty(); 1212 } 1213 1214 /** 1215 * @param value {@link #uid} (Formal identifier for this image or other content.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 1216 */ 1217 public ImagingStudySeriesInstanceComponent setUidElement(OidType value) { 1218 this.uid = value; 1219 return this; 1220 } 1221 1222 /** 1223 * @return Formal identifier for this image or other content. 1224 */ 1225 public String getUid() { 1226 return this.uid == null ? null : this.uid.getValue(); 1227 } 1228 1229 /** 1230 * @param value Formal identifier for this image or other content. 1231 */ 1232 public ImagingStudySeriesInstanceComponent setUid(String value) { 1233 if (this.uid == null) 1234 this.uid = new OidType(); 1235 this.uid.setValue(value); 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #number} (The number of instance in the series.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 1241 */ 1242 public UnsignedIntType getNumberElement() { 1243 if (this.number == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.number"); 1246 else if (Configuration.doAutoCreate()) 1247 this.number = new UnsignedIntType(); // bb 1248 return this.number; 1249 } 1250 1251 public boolean hasNumberElement() { 1252 return this.number != null && !this.number.isEmpty(); 1253 } 1254 1255 public boolean hasNumber() { 1256 return this.number != null && !this.number.isEmpty(); 1257 } 1258 1259 /** 1260 * @param value {@link #number} (The number of instance in the series.). This is the underlying object with id, value and extensions. The accessor "getNumber" gives direct access to the value 1261 */ 1262 public ImagingStudySeriesInstanceComponent setNumberElement(UnsignedIntType value) { 1263 this.number = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return The number of instance in the series. 1269 */ 1270 public int getNumber() { 1271 return this.number == null || this.number.isEmpty() ? 0 : this.number.getValue(); 1272 } 1273 1274 /** 1275 * @param value The number of instance in the series. 1276 */ 1277 public ImagingStudySeriesInstanceComponent setNumber(int value) { 1278 if (this.number == null) 1279 this.number = new UnsignedIntType(); 1280 this.number.setValue(value); 1281 return this; 1282 } 1283 1284 /** 1285 * @return {@link #sopClass} (DICOM instance type.). This is the underlying object with id, value and extensions. The accessor "getSopClass" gives direct access to the value 1286 */ 1287 public OidType getSopClassElement() { 1288 if (this.sopClass == null) 1289 if (Configuration.errorOnAutoCreate()) 1290 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.sopClass"); 1291 else if (Configuration.doAutoCreate()) 1292 this.sopClass = new OidType(); // bb 1293 return this.sopClass; 1294 } 1295 1296 public boolean hasSopClassElement() { 1297 return this.sopClass != null && !this.sopClass.isEmpty(); 1298 } 1299 1300 public boolean hasSopClass() { 1301 return this.sopClass != null && !this.sopClass.isEmpty(); 1302 } 1303 1304 /** 1305 * @param value {@link #sopClass} (DICOM instance type.). This is the underlying object with id, value and extensions. The accessor "getSopClass" gives direct access to the value 1306 */ 1307 public ImagingStudySeriesInstanceComponent setSopClassElement(OidType value) { 1308 this.sopClass = value; 1309 return this; 1310 } 1311 1312 /** 1313 * @return DICOM instance type. 1314 */ 1315 public String getSopClass() { 1316 return this.sopClass == null ? null : this.sopClass.getValue(); 1317 } 1318 1319 /** 1320 * @param value DICOM instance type. 1321 */ 1322 public ImagingStudySeriesInstanceComponent setSopClass(String value) { 1323 if (this.sopClass == null) 1324 this.sopClass = new OidType(); 1325 this.sopClass.setValue(value); 1326 return this; 1327 } 1328 1329 /** 1330 * @return {@link #title} (The description of the instance.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1331 */ 1332 public StringType getTitleElement() { 1333 if (this.title == null) 1334 if (Configuration.errorOnAutoCreate()) 1335 throw new Error("Attempt to auto-create ImagingStudySeriesInstanceComponent.title"); 1336 else if (Configuration.doAutoCreate()) 1337 this.title = new StringType(); // bb 1338 return this.title; 1339 } 1340 1341 public boolean hasTitleElement() { 1342 return this.title != null && !this.title.isEmpty(); 1343 } 1344 1345 public boolean hasTitle() { 1346 return this.title != null && !this.title.isEmpty(); 1347 } 1348 1349 /** 1350 * @param value {@link #title} (The description of the instance.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1351 */ 1352 public ImagingStudySeriesInstanceComponent setTitleElement(StringType value) { 1353 this.title = value; 1354 return this; 1355 } 1356 1357 /** 1358 * @return The description of the instance. 1359 */ 1360 public String getTitle() { 1361 return this.title == null ? null : this.title.getValue(); 1362 } 1363 1364 /** 1365 * @param value The description of the instance. 1366 */ 1367 public ImagingStudySeriesInstanceComponent setTitle(String value) { 1368 if (Utilities.noString(value)) 1369 this.title = null; 1370 else { 1371 if (this.title == null) 1372 this.title = new StringType(); 1373 this.title.setValue(value); 1374 } 1375 return this; 1376 } 1377 1378 protected void listChildren(List<Property> children) { 1379 super.listChildren(children); 1380 children.add(new Property("uid", "oid", "Formal identifier for this image or other content.", 0, 1, uid)); 1381 children.add(new Property("number", "unsignedInt", "The number of instance in the series.", 0, 1, number)); 1382 children.add(new Property("sopClass", "oid", "DICOM instance type.", 0, 1, sopClass)); 1383 children.add(new Property("title", "string", "The description of the instance.", 0, 1, title)); 1384 } 1385 1386 @Override 1387 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1388 switch (_hash) { 1389 case 115792: /*uid*/ return new Property("uid", "oid", "Formal identifier for this image or other content.", 0, 1, uid); 1390 case -1034364087: /*number*/ return new Property("number", "unsignedInt", "The number of instance in the series.", 0, 1, number); 1391 case 1560041540: /*sopClass*/ return new Property("sopClass", "oid", "DICOM instance type.", 0, 1, sopClass); 1392 case 110371416: /*title*/ return new Property("title", "string", "The description of the instance.", 0, 1, title); 1393 default: return super.getNamedProperty(_hash, _name, _checkValid); 1394 } 1395 1396 } 1397 1398 @Override 1399 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1400 switch (hash) { 1401 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // OidType 1402 case -1034364087: /*number*/ return this.number == null ? new Base[0] : new Base[] {this.number}; // UnsignedIntType 1403 case 1560041540: /*sopClass*/ return this.sopClass == null ? new Base[0] : new Base[] {this.sopClass}; // OidType 1404 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1405 default: return super.getProperty(hash, name, checkValid); 1406 } 1407 1408 } 1409 1410 @Override 1411 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1412 switch (hash) { 1413 case 115792: // uid 1414 this.uid = castToOid(value); // OidType 1415 return value; 1416 case -1034364087: // number 1417 this.number = castToUnsignedInt(value); // UnsignedIntType 1418 return value; 1419 case 1560041540: // sopClass 1420 this.sopClass = castToOid(value); // OidType 1421 return value; 1422 case 110371416: // title 1423 this.title = castToString(value); // StringType 1424 return value; 1425 default: return super.setProperty(hash, name, value); 1426 } 1427 1428 } 1429 1430 @Override 1431 public Base setProperty(String name, Base value) throws FHIRException { 1432 if (name.equals("uid")) { 1433 this.uid = castToOid(value); // OidType 1434 } else if (name.equals("number")) { 1435 this.number = castToUnsignedInt(value); // UnsignedIntType 1436 } else if (name.equals("sopClass")) { 1437 this.sopClass = castToOid(value); // OidType 1438 } else if (name.equals("title")) { 1439 this.title = castToString(value); // StringType 1440 } else 1441 return super.setProperty(name, value); 1442 return value; 1443 } 1444 1445 @Override 1446 public Base makeProperty(int hash, String name) throws FHIRException { 1447 switch (hash) { 1448 case 115792: return getUidElement(); 1449 case -1034364087: return getNumberElement(); 1450 case 1560041540: return getSopClassElement(); 1451 case 110371416: return getTitleElement(); 1452 default: return super.makeProperty(hash, name); 1453 } 1454 1455 } 1456 1457 @Override 1458 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1459 switch (hash) { 1460 case 115792: /*uid*/ return new String[] {"oid"}; 1461 case -1034364087: /*number*/ return new String[] {"unsignedInt"}; 1462 case 1560041540: /*sopClass*/ return new String[] {"oid"}; 1463 case 110371416: /*title*/ return new String[] {"string"}; 1464 default: return super.getTypesForProperty(hash, name); 1465 } 1466 1467 } 1468 1469 @Override 1470 public Base addChild(String name) throws FHIRException { 1471 if (name.equals("uid")) { 1472 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.uid"); 1473 } 1474 else if (name.equals("number")) { 1475 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.number"); 1476 } 1477 else if (name.equals("sopClass")) { 1478 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.sopClass"); 1479 } 1480 else if (name.equals("title")) { 1481 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.title"); 1482 } 1483 else 1484 return super.addChild(name); 1485 } 1486 1487 public ImagingStudySeriesInstanceComponent copy() { 1488 ImagingStudySeriesInstanceComponent dst = new ImagingStudySeriesInstanceComponent(); 1489 copyValues(dst); 1490 dst.uid = uid == null ? null : uid.copy(); 1491 dst.number = number == null ? null : number.copy(); 1492 dst.sopClass = sopClass == null ? null : sopClass.copy(); 1493 dst.title = title == null ? null : title.copy(); 1494 return dst; 1495 } 1496 1497 @Override 1498 public boolean equalsDeep(Base other_) { 1499 if (!super.equalsDeep(other_)) 1500 return false; 1501 if (!(other_ instanceof ImagingStudySeriesInstanceComponent)) 1502 return false; 1503 ImagingStudySeriesInstanceComponent o = (ImagingStudySeriesInstanceComponent) other_; 1504 return compareDeep(uid, o.uid, true) && compareDeep(number, o.number, true) && compareDeep(sopClass, o.sopClass, true) 1505 && compareDeep(title, o.title, true); 1506 } 1507 1508 @Override 1509 public boolean equalsShallow(Base other_) { 1510 if (!super.equalsShallow(other_)) 1511 return false; 1512 if (!(other_ instanceof ImagingStudySeriesInstanceComponent)) 1513 return false; 1514 ImagingStudySeriesInstanceComponent o = (ImagingStudySeriesInstanceComponent) other_; 1515 return compareValues(uid, o.uid, true) && compareValues(number, o.number, true) && compareValues(sopClass, o.sopClass, true) 1516 && compareValues(title, o.title, true); 1517 } 1518 1519 public boolean isEmpty() { 1520 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, number, sopClass, title 1521 ); 1522 } 1523 1524 public String fhirType() { 1525 return "ImagingStudy.series.instance"; 1526 1527 } 1528 1529 } 1530 1531 /** 1532 * Formal identifier for the study. 1533 */ 1534 @Child(name = "uid", type = {OidType.class}, order=0, min=1, max=1, modifier=false, summary=true) 1535 @Description(shortDefinition="Formal DICOM identifier for the study", formalDefinition="Formal identifier for the study." ) 1536 protected OidType uid; 1537 1538 /** 1539 * Accession Number is an identifier related to some aspect of imaging workflow and data management. Usage may vary across different institutions. See for instance [IHE Radiology Technical Framework Volume 1 Appendix A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf). 1540 */ 1541 @Child(name = "accession", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1542 @Description(shortDefinition="Related workflow identifier (\"Accession Number\")", formalDefinition="Accession Number is an identifier related to some aspect of imaging workflow and data management. Usage may vary across different institutions. See for instance [IHE Radiology Technical Framework Volume 1 Appendix A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf)." ) 1543 protected Identifier accession; 1544 1545 /** 1546 * Other identifiers for the study. 1547 */ 1548 @Child(name = "identifier", type = {Identifier.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1549 @Description(shortDefinition="Other identifiers for the study", formalDefinition="Other identifiers for the study." ) 1550 protected List<Identifier> identifier; 1551 1552 /** 1553 * Availability of study (online, offline, or nearline). 1554 */ 1555 @Child(name = "availability", type = {CodeType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1556 @Description(shortDefinition="ONLINE | OFFLINE | NEARLINE | UNAVAILABLE", formalDefinition="Availability of study (online, offline, or nearline)." ) 1557 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/instance-availability") 1558 protected Enumeration<InstanceAvailability> availability; 1559 1560 /** 1561 * A list of all the Series.ImageModality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19). 1562 */ 1563 @Child(name = "modalityList", type = {Coding.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1564 @Description(shortDefinition="All series modality if actual acquisition modalities", formalDefinition="A list of all the Series.ImageModality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19)." ) 1565 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/dicom-cid29") 1566 protected List<Coding> modalityList; 1567 1568 /** 1569 * The patient imaged in the study. 1570 */ 1571 @Child(name = "patient", type = {Patient.class}, order=5, min=1, max=1, modifier=false, summary=true) 1572 @Description(shortDefinition="Who the images are of", formalDefinition="The patient imaged in the study." ) 1573 protected Reference patient; 1574 1575 /** 1576 * The actual object that is the target of the reference (The patient imaged in the study.) 1577 */ 1578 protected Patient patientTarget; 1579 1580 /** 1581 * The encounter or episode at which the request is initiated. 1582 */ 1583 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=true) 1584 @Description(shortDefinition="Originating context", formalDefinition="The encounter or episode at which the request is initiated." ) 1585 protected Reference context; 1586 1587 /** 1588 * The actual object that is the target of the reference (The encounter or episode at which the request is initiated.) 1589 */ 1590 protected Resource contextTarget; 1591 1592 /** 1593 * Date and time the study started. 1594 */ 1595 @Child(name = "started", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1596 @Description(shortDefinition="When the study was started", formalDefinition="Date and time the study started." ) 1597 protected DateTimeType started; 1598 1599 /** 1600 * A list of the diagnostic requests that resulted in this imaging study being performed. 1601 */ 1602 @Child(name = "basedOn", type = {ReferralRequest.class, CarePlan.class, ProcedureRequest.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1603 @Description(shortDefinition="Request fulfilled", formalDefinition="A list of the diagnostic requests that resulted in this imaging study being performed." ) 1604 protected List<Reference> basedOn; 1605 /** 1606 * The actual objects that are the target of the reference (A list of the diagnostic requests that resulted in this imaging study being performed.) 1607 */ 1608 protected List<Resource> basedOnTarget; 1609 1610 1611 /** 1612 * The requesting/referring physician. 1613 */ 1614 @Child(name = "referrer", type = {Practitioner.class}, order=9, min=0, max=1, modifier=false, summary=true) 1615 @Description(shortDefinition="Referring physician", formalDefinition="The requesting/referring physician." ) 1616 protected Reference referrer; 1617 1618 /** 1619 * The actual object that is the target of the reference (The requesting/referring physician.) 1620 */ 1621 protected Practitioner referrerTarget; 1622 1623 /** 1624 * Who read the study and interpreted the images or other content. 1625 */ 1626 @Child(name = "interpreter", type = {Practitioner.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1627 @Description(shortDefinition="Who interpreted images", formalDefinition="Who read the study and interpreted the images or other content." ) 1628 protected List<Reference> interpreter; 1629 /** 1630 * The actual objects that are the target of the reference (Who read the study and interpreted the images or other content.) 1631 */ 1632 protected List<Practitioner> interpreterTarget; 1633 1634 1635 /** 1636 * The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type. 1637 */ 1638 @Child(name = "endpoint", type = {Endpoint.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1639 @Description(shortDefinition="Study access endpoint", formalDefinition="The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type." ) 1640 protected List<Reference> endpoint; 1641 /** 1642 * The actual objects that are the target of the reference (The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type.) 1643 */ 1644 protected List<Endpoint> endpointTarget; 1645 1646 1647 /** 1648 * Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present. 1649 */ 1650 @Child(name = "numberOfSeries", type = {UnsignedIntType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1651 @Description(shortDefinition="Number of Study Related Series", formalDefinition="Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present." ) 1652 protected UnsignedIntType numberOfSeries; 1653 1654 /** 1655 * Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 1656 */ 1657 @Child(name = "numberOfInstances", type = {UnsignedIntType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1658 @Description(shortDefinition="Number of Study Related Instances", formalDefinition="Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present." ) 1659 protected UnsignedIntType numberOfInstances; 1660 1661 /** 1662 * A reference to the performed Procedure. 1663 */ 1664 @Child(name = "procedureReference", type = {Procedure.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1665 @Description(shortDefinition="The performed Procedure reference", formalDefinition="A reference to the performed Procedure." ) 1666 protected List<Reference> procedureReference; 1667 /** 1668 * The actual objects that are the target of the reference (A reference to the performed Procedure.) 1669 */ 1670 protected List<Procedure> procedureReferenceTarget; 1671 1672 1673 /** 1674 * The code for the performed procedure type. 1675 */ 1676 @Child(name = "procedureCode", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1677 @Description(shortDefinition="The performed procedure code", formalDefinition="The code for the performed procedure type." ) 1678 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-code") 1679 protected List<CodeableConcept> procedureCode; 1680 1681 /** 1682 * Description of clinical condition indicating why the ImagingStudy was requested. 1683 */ 1684 @Child(name = "reason", type = {CodeableConcept.class}, order=16, min=0, max=1, modifier=false, summary=true) 1685 @Description(shortDefinition="Why the study was requested", formalDefinition="Description of clinical condition indicating why the ImagingStudy was requested." ) 1686 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-reason") 1687 protected CodeableConcept reason; 1688 1689 /** 1690 * Institution-generated description or classification of the Study performed. 1691 */ 1692 @Child(name = "description", type = {StringType.class}, order=17, min=0, max=1, modifier=false, summary=true) 1693 @Description(shortDefinition="Institution-generated description", formalDefinition="Institution-generated description or classification of the Study performed." ) 1694 protected StringType description; 1695 1696 /** 1697 * Each study has one or more series of images or other content. 1698 */ 1699 @Child(name = "series", type = {}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1700 @Description(shortDefinition="Each study has one or more series of instances", formalDefinition="Each study has one or more series of images or other content." ) 1701 protected List<ImagingStudySeriesComponent> series; 1702 1703 private static final long serialVersionUID = -1987354693L; 1704 1705 /** 1706 * Constructor 1707 */ 1708 public ImagingStudy() { 1709 super(); 1710 } 1711 1712 /** 1713 * Constructor 1714 */ 1715 public ImagingStudy(OidType uid, Reference patient) { 1716 super(); 1717 this.uid = uid; 1718 this.patient = patient; 1719 } 1720 1721 /** 1722 * @return {@link #uid} (Formal identifier for the study.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 1723 */ 1724 public OidType getUidElement() { 1725 if (this.uid == null) 1726 if (Configuration.errorOnAutoCreate()) 1727 throw new Error("Attempt to auto-create ImagingStudy.uid"); 1728 else if (Configuration.doAutoCreate()) 1729 this.uid = new OidType(); // bb 1730 return this.uid; 1731 } 1732 1733 public boolean hasUidElement() { 1734 return this.uid != null && !this.uid.isEmpty(); 1735 } 1736 1737 public boolean hasUid() { 1738 return this.uid != null && !this.uid.isEmpty(); 1739 } 1740 1741 /** 1742 * @param value {@link #uid} (Formal identifier for the study.). This is the underlying object with id, value and extensions. The accessor "getUid" gives direct access to the value 1743 */ 1744 public ImagingStudy setUidElement(OidType value) { 1745 this.uid = value; 1746 return this; 1747 } 1748 1749 /** 1750 * @return Formal identifier for the study. 1751 */ 1752 public String getUid() { 1753 return this.uid == null ? null : this.uid.getValue(); 1754 } 1755 1756 /** 1757 * @param value Formal identifier for the study. 1758 */ 1759 public ImagingStudy setUid(String value) { 1760 if (this.uid == null) 1761 this.uid = new OidType(); 1762 this.uid.setValue(value); 1763 return this; 1764 } 1765 1766 /** 1767 * @return {@link #accession} (Accession Number is an identifier related to some aspect of imaging workflow and data management. Usage may vary across different institutions. See for instance [IHE Radiology Technical Framework Volume 1 Appendix A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf).) 1768 */ 1769 public Identifier getAccession() { 1770 if (this.accession == null) 1771 if (Configuration.errorOnAutoCreate()) 1772 throw new Error("Attempt to auto-create ImagingStudy.accession"); 1773 else if (Configuration.doAutoCreate()) 1774 this.accession = new Identifier(); // cc 1775 return this.accession; 1776 } 1777 1778 public boolean hasAccession() { 1779 return this.accession != null && !this.accession.isEmpty(); 1780 } 1781 1782 /** 1783 * @param value {@link #accession} (Accession Number is an identifier related to some aspect of imaging workflow and data management. Usage may vary across different institutions. See for instance [IHE Radiology Technical Framework Volume 1 Appendix A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf).) 1784 */ 1785 public ImagingStudy setAccession(Identifier value) { 1786 this.accession = value; 1787 return this; 1788 } 1789 1790 /** 1791 * @return {@link #identifier} (Other identifiers for the study.) 1792 */ 1793 public List<Identifier> getIdentifier() { 1794 if (this.identifier == null) 1795 this.identifier = new ArrayList<Identifier>(); 1796 return this.identifier; 1797 } 1798 1799 /** 1800 * @return Returns a reference to <code>this</code> for easy method chaining 1801 */ 1802 public ImagingStudy setIdentifier(List<Identifier> theIdentifier) { 1803 this.identifier = theIdentifier; 1804 return this; 1805 } 1806 1807 public boolean hasIdentifier() { 1808 if (this.identifier == null) 1809 return false; 1810 for (Identifier item : this.identifier) 1811 if (!item.isEmpty()) 1812 return true; 1813 return false; 1814 } 1815 1816 public Identifier addIdentifier() { //3 1817 Identifier t = new Identifier(); 1818 if (this.identifier == null) 1819 this.identifier = new ArrayList<Identifier>(); 1820 this.identifier.add(t); 1821 return t; 1822 } 1823 1824 public ImagingStudy addIdentifier(Identifier t) { //3 1825 if (t == null) 1826 return this; 1827 if (this.identifier == null) 1828 this.identifier = new ArrayList<Identifier>(); 1829 this.identifier.add(t); 1830 return this; 1831 } 1832 1833 /** 1834 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1835 */ 1836 public Identifier getIdentifierFirstRep() { 1837 if (getIdentifier().isEmpty()) { 1838 addIdentifier(); 1839 } 1840 return getIdentifier().get(0); 1841 } 1842 1843 /** 1844 * @return {@link #availability} (Availability of study (online, offline, or nearline).). This is the underlying object with id, value and extensions. The accessor "getAvailability" gives direct access to the value 1845 */ 1846 public Enumeration<InstanceAvailability> getAvailabilityElement() { 1847 if (this.availability == null) 1848 if (Configuration.errorOnAutoCreate()) 1849 throw new Error("Attempt to auto-create ImagingStudy.availability"); 1850 else if (Configuration.doAutoCreate()) 1851 this.availability = new Enumeration<InstanceAvailability>(new InstanceAvailabilityEnumFactory()); // bb 1852 return this.availability; 1853 } 1854 1855 public boolean hasAvailabilityElement() { 1856 return this.availability != null && !this.availability.isEmpty(); 1857 } 1858 1859 public boolean hasAvailability() { 1860 return this.availability != null && !this.availability.isEmpty(); 1861 } 1862 1863 /** 1864 * @param value {@link #availability} (Availability of study (online, offline, or nearline).). This is the underlying object with id, value and extensions. The accessor "getAvailability" gives direct access to the value 1865 */ 1866 public ImagingStudy setAvailabilityElement(Enumeration<InstanceAvailability> value) { 1867 this.availability = value; 1868 return this; 1869 } 1870 1871 /** 1872 * @return Availability of study (online, offline, or nearline). 1873 */ 1874 public InstanceAvailability getAvailability() { 1875 return this.availability == null ? null : this.availability.getValue(); 1876 } 1877 1878 /** 1879 * @param value Availability of study (online, offline, or nearline). 1880 */ 1881 public ImagingStudy setAvailability(InstanceAvailability value) { 1882 if (value == null) 1883 this.availability = null; 1884 else { 1885 if (this.availability == null) 1886 this.availability = new Enumeration<InstanceAvailability>(new InstanceAvailabilityEnumFactory()); 1887 this.availability.setValue(value); 1888 } 1889 return this; 1890 } 1891 1892 /** 1893 * @return {@link #modalityList} (A list of all the Series.ImageModality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19).) 1894 */ 1895 public List<Coding> getModalityList() { 1896 if (this.modalityList == null) 1897 this.modalityList = new ArrayList<Coding>(); 1898 return this.modalityList; 1899 } 1900 1901 /** 1902 * @return Returns a reference to <code>this</code> for easy method chaining 1903 */ 1904 public ImagingStudy setModalityList(List<Coding> theModalityList) { 1905 this.modalityList = theModalityList; 1906 return this; 1907 } 1908 1909 public boolean hasModalityList() { 1910 if (this.modalityList == null) 1911 return false; 1912 for (Coding item : this.modalityList) 1913 if (!item.isEmpty()) 1914 return true; 1915 return false; 1916 } 1917 1918 public Coding addModalityList() { //3 1919 Coding t = new Coding(); 1920 if (this.modalityList == null) 1921 this.modalityList = new ArrayList<Coding>(); 1922 this.modalityList.add(t); 1923 return t; 1924 } 1925 1926 public ImagingStudy addModalityList(Coding t) { //3 1927 if (t == null) 1928 return this; 1929 if (this.modalityList == null) 1930 this.modalityList = new ArrayList<Coding>(); 1931 this.modalityList.add(t); 1932 return this; 1933 } 1934 1935 /** 1936 * @return The first repetition of repeating field {@link #modalityList}, creating it if it does not already exist 1937 */ 1938 public Coding getModalityListFirstRep() { 1939 if (getModalityList().isEmpty()) { 1940 addModalityList(); 1941 } 1942 return getModalityList().get(0); 1943 } 1944 1945 /** 1946 * @return {@link #patient} (The patient imaged in the study.) 1947 */ 1948 public Reference getPatient() { 1949 if (this.patient == null) 1950 if (Configuration.errorOnAutoCreate()) 1951 throw new Error("Attempt to auto-create ImagingStudy.patient"); 1952 else if (Configuration.doAutoCreate()) 1953 this.patient = new Reference(); // cc 1954 return this.patient; 1955 } 1956 1957 public boolean hasPatient() { 1958 return this.patient != null && !this.patient.isEmpty(); 1959 } 1960 1961 /** 1962 * @param value {@link #patient} (The patient imaged in the study.) 1963 */ 1964 public ImagingStudy setPatient(Reference value) { 1965 this.patient = value; 1966 return this; 1967 } 1968 1969 /** 1970 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient imaged in the study.) 1971 */ 1972 public Patient getPatientTarget() { 1973 if (this.patientTarget == null) 1974 if (Configuration.errorOnAutoCreate()) 1975 throw new Error("Attempt to auto-create ImagingStudy.patient"); 1976 else if (Configuration.doAutoCreate()) 1977 this.patientTarget = new Patient(); // aa 1978 return this.patientTarget; 1979 } 1980 1981 /** 1982 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient imaged in the study.) 1983 */ 1984 public ImagingStudy setPatientTarget(Patient value) { 1985 this.patientTarget = value; 1986 return this; 1987 } 1988 1989 /** 1990 * @return {@link #context} (The encounter or episode at which the request is initiated.) 1991 */ 1992 public Reference getContext() { 1993 if (this.context == null) 1994 if (Configuration.errorOnAutoCreate()) 1995 throw new Error("Attempt to auto-create ImagingStudy.context"); 1996 else if (Configuration.doAutoCreate()) 1997 this.context = new Reference(); // cc 1998 return this.context; 1999 } 2000 2001 public boolean hasContext() { 2002 return this.context != null && !this.context.isEmpty(); 2003 } 2004 2005 /** 2006 * @param value {@link #context} (The encounter or episode at which the request is initiated.) 2007 */ 2008 public ImagingStudy setContext(Reference value) { 2009 this.context = value; 2010 return this; 2011 } 2012 2013 /** 2014 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode at which the request is initiated.) 2015 */ 2016 public Resource getContextTarget() { 2017 return this.contextTarget; 2018 } 2019 2020 /** 2021 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode at which the request is initiated.) 2022 */ 2023 public ImagingStudy setContextTarget(Resource value) { 2024 this.contextTarget = value; 2025 return this; 2026 } 2027 2028 /** 2029 * @return {@link #started} (Date and time the study started.). This is the underlying object with id, value and extensions. The accessor "getStarted" gives direct access to the value 2030 */ 2031 public DateTimeType getStartedElement() { 2032 if (this.started == null) 2033 if (Configuration.errorOnAutoCreate()) 2034 throw new Error("Attempt to auto-create ImagingStudy.started"); 2035 else if (Configuration.doAutoCreate()) 2036 this.started = new DateTimeType(); // bb 2037 return this.started; 2038 } 2039 2040 public boolean hasStartedElement() { 2041 return this.started != null && !this.started.isEmpty(); 2042 } 2043 2044 public boolean hasStarted() { 2045 return this.started != null && !this.started.isEmpty(); 2046 } 2047 2048 /** 2049 * @param value {@link #started} (Date and time the study started.). This is the underlying object with id, value and extensions. The accessor "getStarted" gives direct access to the value 2050 */ 2051 public ImagingStudy setStartedElement(DateTimeType value) { 2052 this.started = value; 2053 return this; 2054 } 2055 2056 /** 2057 * @return Date and time the study started. 2058 */ 2059 public Date getStarted() { 2060 return this.started == null ? null : this.started.getValue(); 2061 } 2062 2063 /** 2064 * @param value Date and time the study started. 2065 */ 2066 public ImagingStudy setStarted(Date value) { 2067 if (value == null) 2068 this.started = null; 2069 else { 2070 if (this.started == null) 2071 this.started = new DateTimeType(); 2072 this.started.setValue(value); 2073 } 2074 return this; 2075 } 2076 2077 /** 2078 * @return {@link #basedOn} (A list of the diagnostic requests that resulted in this imaging study being performed.) 2079 */ 2080 public List<Reference> getBasedOn() { 2081 if (this.basedOn == null) 2082 this.basedOn = new ArrayList<Reference>(); 2083 return this.basedOn; 2084 } 2085 2086 /** 2087 * @return Returns a reference to <code>this</code> for easy method chaining 2088 */ 2089 public ImagingStudy setBasedOn(List<Reference> theBasedOn) { 2090 this.basedOn = theBasedOn; 2091 return this; 2092 } 2093 2094 public boolean hasBasedOn() { 2095 if (this.basedOn == null) 2096 return false; 2097 for (Reference item : this.basedOn) 2098 if (!item.isEmpty()) 2099 return true; 2100 return false; 2101 } 2102 2103 public Reference addBasedOn() { //3 2104 Reference t = new Reference(); 2105 if (this.basedOn == null) 2106 this.basedOn = new ArrayList<Reference>(); 2107 this.basedOn.add(t); 2108 return t; 2109 } 2110 2111 public ImagingStudy addBasedOn(Reference t) { //3 2112 if (t == null) 2113 return this; 2114 if (this.basedOn == null) 2115 this.basedOn = new ArrayList<Reference>(); 2116 this.basedOn.add(t); 2117 return this; 2118 } 2119 2120 /** 2121 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 2122 */ 2123 public Reference getBasedOnFirstRep() { 2124 if (getBasedOn().isEmpty()) { 2125 addBasedOn(); 2126 } 2127 return getBasedOn().get(0); 2128 } 2129 2130 /** 2131 * @deprecated Use Reference#setResource(IBaseResource) instead 2132 */ 2133 @Deprecated 2134 public List<Resource> getBasedOnTarget() { 2135 if (this.basedOnTarget == null) 2136 this.basedOnTarget = new ArrayList<Resource>(); 2137 return this.basedOnTarget; 2138 } 2139 2140 /** 2141 * @return {@link #referrer} (The requesting/referring physician.) 2142 */ 2143 public Reference getReferrer() { 2144 if (this.referrer == null) 2145 if (Configuration.errorOnAutoCreate()) 2146 throw new Error("Attempt to auto-create ImagingStudy.referrer"); 2147 else if (Configuration.doAutoCreate()) 2148 this.referrer = new Reference(); // cc 2149 return this.referrer; 2150 } 2151 2152 public boolean hasReferrer() { 2153 return this.referrer != null && !this.referrer.isEmpty(); 2154 } 2155 2156 /** 2157 * @param value {@link #referrer} (The requesting/referring physician.) 2158 */ 2159 public ImagingStudy setReferrer(Reference value) { 2160 this.referrer = value; 2161 return this; 2162 } 2163 2164 /** 2165 * @return {@link #referrer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The requesting/referring physician.) 2166 */ 2167 public Practitioner getReferrerTarget() { 2168 if (this.referrerTarget == null) 2169 if (Configuration.errorOnAutoCreate()) 2170 throw new Error("Attempt to auto-create ImagingStudy.referrer"); 2171 else if (Configuration.doAutoCreate()) 2172 this.referrerTarget = new Practitioner(); // aa 2173 return this.referrerTarget; 2174 } 2175 2176 /** 2177 * @param value {@link #referrer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The requesting/referring physician.) 2178 */ 2179 public ImagingStudy setReferrerTarget(Practitioner value) { 2180 this.referrerTarget = value; 2181 return this; 2182 } 2183 2184 /** 2185 * @return {@link #interpreter} (Who read the study and interpreted the images or other content.) 2186 */ 2187 public List<Reference> getInterpreter() { 2188 if (this.interpreter == null) 2189 this.interpreter = new ArrayList<Reference>(); 2190 return this.interpreter; 2191 } 2192 2193 /** 2194 * @return Returns a reference to <code>this</code> for easy method chaining 2195 */ 2196 public ImagingStudy setInterpreter(List<Reference> theInterpreter) { 2197 this.interpreter = theInterpreter; 2198 return this; 2199 } 2200 2201 public boolean hasInterpreter() { 2202 if (this.interpreter == null) 2203 return false; 2204 for (Reference item : this.interpreter) 2205 if (!item.isEmpty()) 2206 return true; 2207 return false; 2208 } 2209 2210 public Reference addInterpreter() { //3 2211 Reference t = new Reference(); 2212 if (this.interpreter == null) 2213 this.interpreter = new ArrayList<Reference>(); 2214 this.interpreter.add(t); 2215 return t; 2216 } 2217 2218 public ImagingStudy addInterpreter(Reference t) { //3 2219 if (t == null) 2220 return this; 2221 if (this.interpreter == null) 2222 this.interpreter = new ArrayList<Reference>(); 2223 this.interpreter.add(t); 2224 return this; 2225 } 2226 2227 /** 2228 * @return The first repetition of repeating field {@link #interpreter}, creating it if it does not already exist 2229 */ 2230 public Reference getInterpreterFirstRep() { 2231 if (getInterpreter().isEmpty()) { 2232 addInterpreter(); 2233 } 2234 return getInterpreter().get(0); 2235 } 2236 2237 /** 2238 * @deprecated Use Reference#setResource(IBaseResource) instead 2239 */ 2240 @Deprecated 2241 public List<Practitioner> getInterpreterTarget() { 2242 if (this.interpreterTarget == null) 2243 this.interpreterTarget = new ArrayList<Practitioner>(); 2244 return this.interpreterTarget; 2245 } 2246 2247 /** 2248 * @deprecated Use Reference#setResource(IBaseResource) instead 2249 */ 2250 @Deprecated 2251 public Practitioner addInterpreterTarget() { 2252 Practitioner r = new Practitioner(); 2253 if (this.interpreterTarget == null) 2254 this.interpreterTarget = new ArrayList<Practitioner>(); 2255 this.interpreterTarget.add(r); 2256 return r; 2257 } 2258 2259 /** 2260 * @return {@link #endpoint} (The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type.) 2261 */ 2262 public List<Reference> getEndpoint() { 2263 if (this.endpoint == null) 2264 this.endpoint = new ArrayList<Reference>(); 2265 return this.endpoint; 2266 } 2267 2268 /** 2269 * @return Returns a reference to <code>this</code> for easy method chaining 2270 */ 2271 public ImagingStudy setEndpoint(List<Reference> theEndpoint) { 2272 this.endpoint = theEndpoint; 2273 return this; 2274 } 2275 2276 public boolean hasEndpoint() { 2277 if (this.endpoint == null) 2278 return false; 2279 for (Reference item : this.endpoint) 2280 if (!item.isEmpty()) 2281 return true; 2282 return false; 2283 } 2284 2285 public Reference addEndpoint() { //3 2286 Reference t = new Reference(); 2287 if (this.endpoint == null) 2288 this.endpoint = new ArrayList<Reference>(); 2289 this.endpoint.add(t); 2290 return t; 2291 } 2292 2293 public ImagingStudy addEndpoint(Reference t) { //3 2294 if (t == null) 2295 return this; 2296 if (this.endpoint == null) 2297 this.endpoint = new ArrayList<Reference>(); 2298 this.endpoint.add(t); 2299 return this; 2300 } 2301 2302 /** 2303 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 2304 */ 2305 public Reference getEndpointFirstRep() { 2306 if (getEndpoint().isEmpty()) { 2307 addEndpoint(); 2308 } 2309 return getEndpoint().get(0); 2310 } 2311 2312 /** 2313 * @deprecated Use Reference#setResource(IBaseResource) instead 2314 */ 2315 @Deprecated 2316 public List<Endpoint> getEndpointTarget() { 2317 if (this.endpointTarget == null) 2318 this.endpointTarget = new ArrayList<Endpoint>(); 2319 return this.endpointTarget; 2320 } 2321 2322 /** 2323 * @deprecated Use Reference#setResource(IBaseResource) instead 2324 */ 2325 @Deprecated 2326 public Endpoint addEndpointTarget() { 2327 Endpoint r = new Endpoint(); 2328 if (this.endpointTarget == null) 2329 this.endpointTarget = new ArrayList<Endpoint>(); 2330 this.endpointTarget.add(r); 2331 return r; 2332 } 2333 2334 /** 2335 * @return {@link #numberOfSeries} (Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfSeries" gives direct access to the value 2336 */ 2337 public UnsignedIntType getNumberOfSeriesElement() { 2338 if (this.numberOfSeries == null) 2339 if (Configuration.errorOnAutoCreate()) 2340 throw new Error("Attempt to auto-create ImagingStudy.numberOfSeries"); 2341 else if (Configuration.doAutoCreate()) 2342 this.numberOfSeries = new UnsignedIntType(); // bb 2343 return this.numberOfSeries; 2344 } 2345 2346 public boolean hasNumberOfSeriesElement() { 2347 return this.numberOfSeries != null && !this.numberOfSeries.isEmpty(); 2348 } 2349 2350 public boolean hasNumberOfSeries() { 2351 return this.numberOfSeries != null && !this.numberOfSeries.isEmpty(); 2352 } 2353 2354 /** 2355 * @param value {@link #numberOfSeries} (Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfSeries" gives direct access to the value 2356 */ 2357 public ImagingStudy setNumberOfSeriesElement(UnsignedIntType value) { 2358 this.numberOfSeries = value; 2359 return this; 2360 } 2361 2362 /** 2363 * @return Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present. 2364 */ 2365 public int getNumberOfSeries() { 2366 return this.numberOfSeries == null || this.numberOfSeries.isEmpty() ? 0 : this.numberOfSeries.getValue(); 2367 } 2368 2369 /** 2370 * @param value Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present. 2371 */ 2372 public ImagingStudy setNumberOfSeries(int value) { 2373 if (this.numberOfSeries == null) 2374 this.numberOfSeries = new UnsignedIntType(); 2375 this.numberOfSeries.setValue(value); 2376 return this; 2377 } 2378 2379 /** 2380 * @return {@link #numberOfInstances} (Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfInstances" gives direct access to the value 2381 */ 2382 public UnsignedIntType getNumberOfInstancesElement() { 2383 if (this.numberOfInstances == null) 2384 if (Configuration.errorOnAutoCreate()) 2385 throw new Error("Attempt to auto-create ImagingStudy.numberOfInstances"); 2386 else if (Configuration.doAutoCreate()) 2387 this.numberOfInstances = new UnsignedIntType(); // bb 2388 return this.numberOfInstances; 2389 } 2390 2391 public boolean hasNumberOfInstancesElement() { 2392 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 2393 } 2394 2395 public boolean hasNumberOfInstances() { 2396 return this.numberOfInstances != null && !this.numberOfInstances.isEmpty(); 2397 } 2398 2399 /** 2400 * @param value {@link #numberOfInstances} (Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.). This is the underlying object with id, value and extensions. The accessor "getNumberOfInstances" gives direct access to the value 2401 */ 2402 public ImagingStudy setNumberOfInstancesElement(UnsignedIntType value) { 2403 this.numberOfInstances = value; 2404 return this; 2405 } 2406 2407 /** 2408 * @return Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 2409 */ 2410 public int getNumberOfInstances() { 2411 return this.numberOfInstances == null || this.numberOfInstances.isEmpty() ? 0 : this.numberOfInstances.getValue(); 2412 } 2413 2414 /** 2415 * @param value Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present. 2416 */ 2417 public ImagingStudy setNumberOfInstances(int value) { 2418 if (this.numberOfInstances == null) 2419 this.numberOfInstances = new UnsignedIntType(); 2420 this.numberOfInstances.setValue(value); 2421 return this; 2422 } 2423 2424 /** 2425 * @return {@link #procedureReference} (A reference to the performed Procedure.) 2426 */ 2427 public List<Reference> getProcedureReference() { 2428 if (this.procedureReference == null) 2429 this.procedureReference = new ArrayList<Reference>(); 2430 return this.procedureReference; 2431 } 2432 2433 /** 2434 * @return Returns a reference to <code>this</code> for easy method chaining 2435 */ 2436 public ImagingStudy setProcedureReference(List<Reference> theProcedureReference) { 2437 this.procedureReference = theProcedureReference; 2438 return this; 2439 } 2440 2441 public boolean hasProcedureReference() { 2442 if (this.procedureReference == null) 2443 return false; 2444 for (Reference item : this.procedureReference) 2445 if (!item.isEmpty()) 2446 return true; 2447 return false; 2448 } 2449 2450 public Reference addProcedureReference() { //3 2451 Reference t = new Reference(); 2452 if (this.procedureReference == null) 2453 this.procedureReference = new ArrayList<Reference>(); 2454 this.procedureReference.add(t); 2455 return t; 2456 } 2457 2458 public ImagingStudy addProcedureReference(Reference t) { //3 2459 if (t == null) 2460 return this; 2461 if (this.procedureReference == null) 2462 this.procedureReference = new ArrayList<Reference>(); 2463 this.procedureReference.add(t); 2464 return this; 2465 } 2466 2467 /** 2468 * @return The first repetition of repeating field {@link #procedureReference}, creating it if it does not already exist 2469 */ 2470 public Reference getProcedureReferenceFirstRep() { 2471 if (getProcedureReference().isEmpty()) { 2472 addProcedureReference(); 2473 } 2474 return getProcedureReference().get(0); 2475 } 2476 2477 /** 2478 * @deprecated Use Reference#setResource(IBaseResource) instead 2479 */ 2480 @Deprecated 2481 public List<Procedure> getProcedureReferenceTarget() { 2482 if (this.procedureReferenceTarget == null) 2483 this.procedureReferenceTarget = new ArrayList<Procedure>(); 2484 return this.procedureReferenceTarget; 2485 } 2486 2487 /** 2488 * @deprecated Use Reference#setResource(IBaseResource) instead 2489 */ 2490 @Deprecated 2491 public Procedure addProcedureReferenceTarget() { 2492 Procedure r = new Procedure(); 2493 if (this.procedureReferenceTarget == null) 2494 this.procedureReferenceTarget = new ArrayList<Procedure>(); 2495 this.procedureReferenceTarget.add(r); 2496 return r; 2497 } 2498 2499 /** 2500 * @return {@link #procedureCode} (The code for the performed procedure type.) 2501 */ 2502 public List<CodeableConcept> getProcedureCode() { 2503 if (this.procedureCode == null) 2504 this.procedureCode = new ArrayList<CodeableConcept>(); 2505 return this.procedureCode; 2506 } 2507 2508 /** 2509 * @return Returns a reference to <code>this</code> for easy method chaining 2510 */ 2511 public ImagingStudy setProcedureCode(List<CodeableConcept> theProcedureCode) { 2512 this.procedureCode = theProcedureCode; 2513 return this; 2514 } 2515 2516 public boolean hasProcedureCode() { 2517 if (this.procedureCode == null) 2518 return false; 2519 for (CodeableConcept item : this.procedureCode) 2520 if (!item.isEmpty()) 2521 return true; 2522 return false; 2523 } 2524 2525 public CodeableConcept addProcedureCode() { //3 2526 CodeableConcept t = new CodeableConcept(); 2527 if (this.procedureCode == null) 2528 this.procedureCode = new ArrayList<CodeableConcept>(); 2529 this.procedureCode.add(t); 2530 return t; 2531 } 2532 2533 public ImagingStudy addProcedureCode(CodeableConcept t) { //3 2534 if (t == null) 2535 return this; 2536 if (this.procedureCode == null) 2537 this.procedureCode = new ArrayList<CodeableConcept>(); 2538 this.procedureCode.add(t); 2539 return this; 2540 } 2541 2542 /** 2543 * @return The first repetition of repeating field {@link #procedureCode}, creating it if it does not already exist 2544 */ 2545 public CodeableConcept getProcedureCodeFirstRep() { 2546 if (getProcedureCode().isEmpty()) { 2547 addProcedureCode(); 2548 } 2549 return getProcedureCode().get(0); 2550 } 2551 2552 /** 2553 * @return {@link #reason} (Description of clinical condition indicating why the ImagingStudy was requested.) 2554 */ 2555 public CodeableConcept getReason() { 2556 if (this.reason == null) 2557 if (Configuration.errorOnAutoCreate()) 2558 throw new Error("Attempt to auto-create ImagingStudy.reason"); 2559 else if (Configuration.doAutoCreate()) 2560 this.reason = new CodeableConcept(); // cc 2561 return this.reason; 2562 } 2563 2564 public boolean hasReason() { 2565 return this.reason != null && !this.reason.isEmpty(); 2566 } 2567 2568 /** 2569 * @param value {@link #reason} (Description of clinical condition indicating why the ImagingStudy was requested.) 2570 */ 2571 public ImagingStudy setReason(CodeableConcept value) { 2572 this.reason = value; 2573 return this; 2574 } 2575 2576 /** 2577 * @return {@link #description} (Institution-generated description or classification of the Study performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2578 */ 2579 public StringType getDescriptionElement() { 2580 if (this.description == null) 2581 if (Configuration.errorOnAutoCreate()) 2582 throw new Error("Attempt to auto-create ImagingStudy.description"); 2583 else if (Configuration.doAutoCreate()) 2584 this.description = new StringType(); // bb 2585 return this.description; 2586 } 2587 2588 public boolean hasDescriptionElement() { 2589 return this.description != null && !this.description.isEmpty(); 2590 } 2591 2592 public boolean hasDescription() { 2593 return this.description != null && !this.description.isEmpty(); 2594 } 2595 2596 /** 2597 * @param value {@link #description} (Institution-generated description or classification of the Study performed.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2598 */ 2599 public ImagingStudy setDescriptionElement(StringType value) { 2600 this.description = value; 2601 return this; 2602 } 2603 2604 /** 2605 * @return Institution-generated description or classification of the Study performed. 2606 */ 2607 public String getDescription() { 2608 return this.description == null ? null : this.description.getValue(); 2609 } 2610 2611 /** 2612 * @param value Institution-generated description or classification of the Study performed. 2613 */ 2614 public ImagingStudy setDescription(String value) { 2615 if (Utilities.noString(value)) 2616 this.description = null; 2617 else { 2618 if (this.description == null) 2619 this.description = new StringType(); 2620 this.description.setValue(value); 2621 } 2622 return this; 2623 } 2624 2625 /** 2626 * @return {@link #series} (Each study has one or more series of images or other content.) 2627 */ 2628 public List<ImagingStudySeriesComponent> getSeries() { 2629 if (this.series == null) 2630 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2631 return this.series; 2632 } 2633 2634 /** 2635 * @return Returns a reference to <code>this</code> for easy method chaining 2636 */ 2637 public ImagingStudy setSeries(List<ImagingStudySeriesComponent> theSeries) { 2638 this.series = theSeries; 2639 return this; 2640 } 2641 2642 public boolean hasSeries() { 2643 if (this.series == null) 2644 return false; 2645 for (ImagingStudySeriesComponent item : this.series) 2646 if (!item.isEmpty()) 2647 return true; 2648 return false; 2649 } 2650 2651 public ImagingStudySeriesComponent addSeries() { //3 2652 ImagingStudySeriesComponent t = new ImagingStudySeriesComponent(); 2653 if (this.series == null) 2654 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2655 this.series.add(t); 2656 return t; 2657 } 2658 2659 public ImagingStudy addSeries(ImagingStudySeriesComponent t) { //3 2660 if (t == null) 2661 return this; 2662 if (this.series == null) 2663 this.series = new ArrayList<ImagingStudySeriesComponent>(); 2664 this.series.add(t); 2665 return this; 2666 } 2667 2668 /** 2669 * @return The first repetition of repeating field {@link #series}, creating it if it does not already exist 2670 */ 2671 public ImagingStudySeriesComponent getSeriesFirstRep() { 2672 if (getSeries().isEmpty()) { 2673 addSeries(); 2674 } 2675 return getSeries().get(0); 2676 } 2677 2678 protected void listChildren(List<Property> children) { 2679 super.listChildren(children); 2680 children.add(new Property("uid", "oid", "Formal identifier for the study.", 0, 1, uid)); 2681 children.add(new Property("accession", "Identifier", "Accession Number is an identifier related to some aspect of imaging workflow and data management. Usage may vary across different institutions. See for instance [IHE Radiology Technical Framework Volume 1 Appendix A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf).", 0, 1, accession)); 2682 children.add(new Property("identifier", "Identifier", "Other identifiers for the study.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2683 children.add(new Property("availability", "code", "Availability of study (online, offline, or nearline).", 0, 1, availability)); 2684 children.add(new Property("modalityList", "Coding", "A list of all the Series.ImageModality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19).", 0, java.lang.Integer.MAX_VALUE, modalityList)); 2685 children.add(new Property("patient", "Reference(Patient)", "The patient imaged in the study.", 0, 1, patient)); 2686 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode at which the request is initiated.", 0, 1, context)); 2687 children.add(new Property("started", "dateTime", "Date and time the study started.", 0, 1, started)); 2688 children.add(new Property("basedOn", "Reference(ReferralRequest|CarePlan|ProcedureRequest)", "A list of the diagnostic requests that resulted in this imaging study being performed.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2689 children.add(new Property("referrer", "Reference(Practitioner)", "The requesting/referring physician.", 0, 1, referrer)); 2690 children.add(new Property("interpreter", "Reference(Practitioner)", "Who read the study and interpreted the images or other content.", 0, java.lang.Integer.MAX_VALUE, interpreter)); 2691 children.add(new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 2692 children.add(new Property("numberOfSeries", "unsignedInt", "Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.", 0, 1, numberOfSeries)); 2693 children.add(new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 0, 1, numberOfInstances)); 2694 children.add(new Property("procedureReference", "Reference(Procedure)", "A reference to the performed Procedure.", 0, java.lang.Integer.MAX_VALUE, procedureReference)); 2695 children.add(new Property("procedureCode", "CodeableConcept", "The code for the performed procedure type.", 0, java.lang.Integer.MAX_VALUE, procedureCode)); 2696 children.add(new Property("reason", "CodeableConcept", "Description of clinical condition indicating why the ImagingStudy was requested.", 0, 1, reason)); 2697 children.add(new Property("description", "string", "Institution-generated description or classification of the Study performed.", 0, 1, description)); 2698 children.add(new Property("series", "", "Each study has one or more series of images or other content.", 0, java.lang.Integer.MAX_VALUE, series)); 2699 } 2700 2701 @Override 2702 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2703 switch (_hash) { 2704 case 115792: /*uid*/ return new Property("uid", "oid", "Formal identifier for the study.", 0, 1, uid); 2705 case -2115028956: /*accession*/ return new Property("accession", "Identifier", "Accession Number is an identifier related to some aspect of imaging workflow and data management. Usage may vary across different institutions. See for instance [IHE Radiology Technical Framework Volume 1 Appendix A](http://www.ihe.net/uploadedFiles/Documents/Radiology/IHE_RAD_TF_Rev13.0_Vol1_FT_2014-07-30.pdf).", 0, 1, accession); 2706 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Other identifiers for the study.", 0, java.lang.Integer.MAX_VALUE, identifier); 2707 case 1997542747: /*availability*/ return new Property("availability", "code", "Availability of study (online, offline, or nearline).", 0, 1, availability); 2708 case -1030238433: /*modalityList*/ return new Property("modalityList", "Coding", "A list of all the Series.ImageModality values that are actual acquisition modalities, i.e. those in the DICOM Context Group 29 (value set OID 1.2.840.10008.6.1.19).", 0, java.lang.Integer.MAX_VALUE, modalityList); 2709 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient imaged in the study.", 0, 1, patient); 2710 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode at which the request is initiated.", 0, 1, context); 2711 case -1897185151: /*started*/ return new Property("started", "dateTime", "Date and time the study started.", 0, 1, started); 2712 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(ReferralRequest|CarePlan|ProcedureRequest)", "A list of the diagnostic requests that resulted in this imaging study being performed.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2713 case -722568161: /*referrer*/ return new Property("referrer", "Reference(Practitioner)", "The requesting/referring physician.", 0, 1, referrer); 2714 case -2008009094: /*interpreter*/ return new Property("interpreter", "Reference(Practitioner)", "Who read the study and interpreted the images or other content.", 0, java.lang.Integer.MAX_VALUE, interpreter); 2715 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "The network service providing access (e.g., query, view, or retrieval) for the study. See implementation notes for information about using DICOM endpoints. A study-level endpoint applies to each series in the study, unless overridden by a series-level endpoint with the same Endpoint.type.", 0, java.lang.Integer.MAX_VALUE, endpoint); 2716 case 1920000407: /*numberOfSeries*/ return new Property("numberOfSeries", "unsignedInt", "Number of Series in the Study. This value given may be larger than the number of series elements this Resource contains due to resource availability, security, or other factors. This element should be present if any series elements are present.", 0, 1, numberOfSeries); 2717 case -1043544226: /*numberOfInstances*/ return new Property("numberOfInstances", "unsignedInt", "Number of SOP Instances in Study. This value given may be larger than the number of instance elements this resource contains due to resource availability, security, or other factors. This element should be present if any instance elements are present.", 0, 1, numberOfInstances); 2718 case 881809848: /*procedureReference*/ return new Property("procedureReference", "Reference(Procedure)", "A reference to the performed Procedure.", 0, java.lang.Integer.MAX_VALUE, procedureReference); 2719 case -698023072: /*procedureCode*/ return new Property("procedureCode", "CodeableConcept", "The code for the performed procedure type.", 0, java.lang.Integer.MAX_VALUE, procedureCode); 2720 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Description of clinical condition indicating why the ImagingStudy was requested.", 0, 1, reason); 2721 case -1724546052: /*description*/ return new Property("description", "string", "Institution-generated description or classification of the Study performed.", 0, 1, description); 2722 case -905838985: /*series*/ return new Property("series", "", "Each study has one or more series of images or other content.", 0, java.lang.Integer.MAX_VALUE, series); 2723 default: return super.getNamedProperty(_hash, _name, _checkValid); 2724 } 2725 2726 } 2727 2728 @Override 2729 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2730 switch (hash) { 2731 case 115792: /*uid*/ return this.uid == null ? new Base[0] : new Base[] {this.uid}; // OidType 2732 case -2115028956: /*accession*/ return this.accession == null ? new Base[0] : new Base[] {this.accession}; // Identifier 2733 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2734 case 1997542747: /*availability*/ return this.availability == null ? new Base[0] : new Base[] {this.availability}; // Enumeration<InstanceAvailability> 2735 case -1030238433: /*modalityList*/ return this.modalityList == null ? new Base[0] : this.modalityList.toArray(new Base[this.modalityList.size()]); // Coding 2736 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2737 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2738 case -1897185151: /*started*/ return this.started == null ? new Base[0] : new Base[] {this.started}; // DateTimeType 2739 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2740 case -722568161: /*referrer*/ return this.referrer == null ? new Base[0] : new Base[] {this.referrer}; // Reference 2741 case -2008009094: /*interpreter*/ return this.interpreter == null ? new Base[0] : this.interpreter.toArray(new Base[this.interpreter.size()]); // Reference 2742 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 2743 case 1920000407: /*numberOfSeries*/ return this.numberOfSeries == null ? new Base[0] : new Base[] {this.numberOfSeries}; // UnsignedIntType 2744 case -1043544226: /*numberOfInstances*/ return this.numberOfInstances == null ? new Base[0] : new Base[] {this.numberOfInstances}; // UnsignedIntType 2745 case 881809848: /*procedureReference*/ return this.procedureReference == null ? new Base[0] : this.procedureReference.toArray(new Base[this.procedureReference.size()]); // Reference 2746 case -698023072: /*procedureCode*/ return this.procedureCode == null ? new Base[0] : this.procedureCode.toArray(new Base[this.procedureCode.size()]); // CodeableConcept 2747 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 2748 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 2749 case -905838985: /*series*/ return this.series == null ? new Base[0] : this.series.toArray(new Base[this.series.size()]); // ImagingStudySeriesComponent 2750 default: return super.getProperty(hash, name, checkValid); 2751 } 2752 2753 } 2754 2755 @Override 2756 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2757 switch (hash) { 2758 case 115792: // uid 2759 this.uid = castToOid(value); // OidType 2760 return value; 2761 case -2115028956: // accession 2762 this.accession = castToIdentifier(value); // Identifier 2763 return value; 2764 case -1618432855: // identifier 2765 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2766 return value; 2767 case 1997542747: // availability 2768 value = new InstanceAvailabilityEnumFactory().fromType(castToCode(value)); 2769 this.availability = (Enumeration) value; // Enumeration<InstanceAvailability> 2770 return value; 2771 case -1030238433: // modalityList 2772 this.getModalityList().add(castToCoding(value)); // Coding 2773 return value; 2774 case -791418107: // patient 2775 this.patient = castToReference(value); // Reference 2776 return value; 2777 case 951530927: // context 2778 this.context = castToReference(value); // Reference 2779 return value; 2780 case -1897185151: // started 2781 this.started = castToDateTime(value); // DateTimeType 2782 return value; 2783 case -332612366: // basedOn 2784 this.getBasedOn().add(castToReference(value)); // Reference 2785 return value; 2786 case -722568161: // referrer 2787 this.referrer = castToReference(value); // Reference 2788 return value; 2789 case -2008009094: // interpreter 2790 this.getInterpreter().add(castToReference(value)); // Reference 2791 return value; 2792 case 1741102485: // endpoint 2793 this.getEndpoint().add(castToReference(value)); // Reference 2794 return value; 2795 case 1920000407: // numberOfSeries 2796 this.numberOfSeries = castToUnsignedInt(value); // UnsignedIntType 2797 return value; 2798 case -1043544226: // numberOfInstances 2799 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 2800 return value; 2801 case 881809848: // procedureReference 2802 this.getProcedureReference().add(castToReference(value)); // Reference 2803 return value; 2804 case -698023072: // procedureCode 2805 this.getProcedureCode().add(castToCodeableConcept(value)); // CodeableConcept 2806 return value; 2807 case -934964668: // reason 2808 this.reason = castToCodeableConcept(value); // CodeableConcept 2809 return value; 2810 case -1724546052: // description 2811 this.description = castToString(value); // StringType 2812 return value; 2813 case -905838985: // series 2814 this.getSeries().add((ImagingStudySeriesComponent) value); // ImagingStudySeriesComponent 2815 return value; 2816 default: return super.setProperty(hash, name, value); 2817 } 2818 2819 } 2820 2821 @Override 2822 public Base setProperty(String name, Base value) throws FHIRException { 2823 if (name.equals("uid")) { 2824 this.uid = castToOid(value); // OidType 2825 } else if (name.equals("accession")) { 2826 this.accession = castToIdentifier(value); // Identifier 2827 } else if (name.equals("identifier")) { 2828 this.getIdentifier().add(castToIdentifier(value)); 2829 } else if (name.equals("availability")) { 2830 value = new InstanceAvailabilityEnumFactory().fromType(castToCode(value)); 2831 this.availability = (Enumeration) value; // Enumeration<InstanceAvailability> 2832 } else if (name.equals("modalityList")) { 2833 this.getModalityList().add(castToCoding(value)); 2834 } else if (name.equals("patient")) { 2835 this.patient = castToReference(value); // Reference 2836 } else if (name.equals("context")) { 2837 this.context = castToReference(value); // Reference 2838 } else if (name.equals("started")) { 2839 this.started = castToDateTime(value); // DateTimeType 2840 } else if (name.equals("basedOn")) { 2841 this.getBasedOn().add(castToReference(value)); 2842 } else if (name.equals("referrer")) { 2843 this.referrer = castToReference(value); // Reference 2844 } else if (name.equals("interpreter")) { 2845 this.getInterpreter().add(castToReference(value)); 2846 } else if (name.equals("endpoint")) { 2847 this.getEndpoint().add(castToReference(value)); 2848 } else if (name.equals("numberOfSeries")) { 2849 this.numberOfSeries = castToUnsignedInt(value); // UnsignedIntType 2850 } else if (name.equals("numberOfInstances")) { 2851 this.numberOfInstances = castToUnsignedInt(value); // UnsignedIntType 2852 } else if (name.equals("procedureReference")) { 2853 this.getProcedureReference().add(castToReference(value)); 2854 } else if (name.equals("procedureCode")) { 2855 this.getProcedureCode().add(castToCodeableConcept(value)); 2856 } else if (name.equals("reason")) { 2857 this.reason = castToCodeableConcept(value); // CodeableConcept 2858 } else if (name.equals("description")) { 2859 this.description = castToString(value); // StringType 2860 } else if (name.equals("series")) { 2861 this.getSeries().add((ImagingStudySeriesComponent) value); 2862 } else 2863 return super.setProperty(name, value); 2864 return value; 2865 } 2866 2867 @Override 2868 public Base makeProperty(int hash, String name) throws FHIRException { 2869 switch (hash) { 2870 case 115792: return getUidElement(); 2871 case -2115028956: return getAccession(); 2872 case -1618432855: return addIdentifier(); 2873 case 1997542747: return getAvailabilityElement(); 2874 case -1030238433: return addModalityList(); 2875 case -791418107: return getPatient(); 2876 case 951530927: return getContext(); 2877 case -1897185151: return getStartedElement(); 2878 case -332612366: return addBasedOn(); 2879 case -722568161: return getReferrer(); 2880 case -2008009094: return addInterpreter(); 2881 case 1741102485: return addEndpoint(); 2882 case 1920000407: return getNumberOfSeriesElement(); 2883 case -1043544226: return getNumberOfInstancesElement(); 2884 case 881809848: return addProcedureReference(); 2885 case -698023072: return addProcedureCode(); 2886 case -934964668: return getReason(); 2887 case -1724546052: return getDescriptionElement(); 2888 case -905838985: return addSeries(); 2889 default: return super.makeProperty(hash, name); 2890 } 2891 2892 } 2893 2894 @Override 2895 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2896 switch (hash) { 2897 case 115792: /*uid*/ return new String[] {"oid"}; 2898 case -2115028956: /*accession*/ return new String[] {"Identifier"}; 2899 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2900 case 1997542747: /*availability*/ return new String[] {"code"}; 2901 case -1030238433: /*modalityList*/ return new String[] {"Coding"}; 2902 case -791418107: /*patient*/ return new String[] {"Reference"}; 2903 case 951530927: /*context*/ return new String[] {"Reference"}; 2904 case -1897185151: /*started*/ return new String[] {"dateTime"}; 2905 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 2906 case -722568161: /*referrer*/ return new String[] {"Reference"}; 2907 case -2008009094: /*interpreter*/ return new String[] {"Reference"}; 2908 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 2909 case 1920000407: /*numberOfSeries*/ return new String[] {"unsignedInt"}; 2910 case -1043544226: /*numberOfInstances*/ return new String[] {"unsignedInt"}; 2911 case 881809848: /*procedureReference*/ return new String[] {"Reference"}; 2912 case -698023072: /*procedureCode*/ return new String[] {"CodeableConcept"}; 2913 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 2914 case -1724546052: /*description*/ return new String[] {"string"}; 2915 case -905838985: /*series*/ return new String[] {}; 2916 default: return super.getTypesForProperty(hash, name); 2917 } 2918 2919 } 2920 2921 @Override 2922 public Base addChild(String name) throws FHIRException { 2923 if (name.equals("uid")) { 2924 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.uid"); 2925 } 2926 else if (name.equals("accession")) { 2927 this.accession = new Identifier(); 2928 return this.accession; 2929 } 2930 else if (name.equals("identifier")) { 2931 return addIdentifier(); 2932 } 2933 else if (name.equals("availability")) { 2934 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.availability"); 2935 } 2936 else if (name.equals("modalityList")) { 2937 return addModalityList(); 2938 } 2939 else if (name.equals("patient")) { 2940 this.patient = new Reference(); 2941 return this.patient; 2942 } 2943 else if (name.equals("context")) { 2944 this.context = new Reference(); 2945 return this.context; 2946 } 2947 else if (name.equals("started")) { 2948 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.started"); 2949 } 2950 else if (name.equals("basedOn")) { 2951 return addBasedOn(); 2952 } 2953 else if (name.equals("referrer")) { 2954 this.referrer = new Reference(); 2955 return this.referrer; 2956 } 2957 else if (name.equals("interpreter")) { 2958 return addInterpreter(); 2959 } 2960 else if (name.equals("endpoint")) { 2961 return addEndpoint(); 2962 } 2963 else if (name.equals("numberOfSeries")) { 2964 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfSeries"); 2965 } 2966 else if (name.equals("numberOfInstances")) { 2967 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.numberOfInstances"); 2968 } 2969 else if (name.equals("procedureReference")) { 2970 return addProcedureReference(); 2971 } 2972 else if (name.equals("procedureCode")) { 2973 return addProcedureCode(); 2974 } 2975 else if (name.equals("reason")) { 2976 this.reason = new CodeableConcept(); 2977 return this.reason; 2978 } 2979 else if (name.equals("description")) { 2980 throw new FHIRException("Cannot call addChild on a singleton property ImagingStudy.description"); 2981 } 2982 else if (name.equals("series")) { 2983 return addSeries(); 2984 } 2985 else 2986 return super.addChild(name); 2987 } 2988 2989 public String fhirType() { 2990 return "ImagingStudy"; 2991 2992 } 2993 2994 public ImagingStudy copy() { 2995 ImagingStudy dst = new ImagingStudy(); 2996 copyValues(dst); 2997 dst.uid = uid == null ? null : uid.copy(); 2998 dst.accession = accession == null ? null : accession.copy(); 2999 if (identifier != null) { 3000 dst.identifier = new ArrayList<Identifier>(); 3001 for (Identifier i : identifier) 3002 dst.identifier.add(i.copy()); 3003 }; 3004 dst.availability = availability == null ? null : availability.copy(); 3005 if (modalityList != null) { 3006 dst.modalityList = new ArrayList<Coding>(); 3007 for (Coding i : modalityList) 3008 dst.modalityList.add(i.copy()); 3009 }; 3010 dst.patient = patient == null ? null : patient.copy(); 3011 dst.context = context == null ? null : context.copy(); 3012 dst.started = started == null ? null : started.copy(); 3013 if (basedOn != null) { 3014 dst.basedOn = new ArrayList<Reference>(); 3015 for (Reference i : basedOn) 3016 dst.basedOn.add(i.copy()); 3017 }; 3018 dst.referrer = referrer == null ? null : referrer.copy(); 3019 if (interpreter != null) { 3020 dst.interpreter = new ArrayList<Reference>(); 3021 for (Reference i : interpreter) 3022 dst.interpreter.add(i.copy()); 3023 }; 3024 if (endpoint != null) { 3025 dst.endpoint = new ArrayList<Reference>(); 3026 for (Reference i : endpoint) 3027 dst.endpoint.add(i.copy()); 3028 }; 3029 dst.numberOfSeries = numberOfSeries == null ? null : numberOfSeries.copy(); 3030 dst.numberOfInstances = numberOfInstances == null ? null : numberOfInstances.copy(); 3031 if (procedureReference != null) { 3032 dst.procedureReference = new ArrayList<Reference>(); 3033 for (Reference i : procedureReference) 3034 dst.procedureReference.add(i.copy()); 3035 }; 3036 if (procedureCode != null) { 3037 dst.procedureCode = new ArrayList<CodeableConcept>(); 3038 for (CodeableConcept i : procedureCode) 3039 dst.procedureCode.add(i.copy()); 3040 }; 3041 dst.reason = reason == null ? null : reason.copy(); 3042 dst.description = description == null ? null : description.copy(); 3043 if (series != null) { 3044 dst.series = new ArrayList<ImagingStudySeriesComponent>(); 3045 for (ImagingStudySeriesComponent i : series) 3046 dst.series.add(i.copy()); 3047 }; 3048 return dst; 3049 } 3050 3051 protected ImagingStudy typedCopy() { 3052 return copy(); 3053 } 3054 3055 @Override 3056 public boolean equalsDeep(Base other_) { 3057 if (!super.equalsDeep(other_)) 3058 return false; 3059 if (!(other_ instanceof ImagingStudy)) 3060 return false; 3061 ImagingStudy o = (ImagingStudy) other_; 3062 return compareDeep(uid, o.uid, true) && compareDeep(accession, o.accession, true) && compareDeep(identifier, o.identifier, true) 3063 && compareDeep(availability, o.availability, true) && compareDeep(modalityList, o.modalityList, true) 3064 && compareDeep(patient, o.patient, true) && compareDeep(context, o.context, true) && compareDeep(started, o.started, true) 3065 && compareDeep(basedOn, o.basedOn, true) && compareDeep(referrer, o.referrer, true) && compareDeep(interpreter, o.interpreter, true) 3066 && compareDeep(endpoint, o.endpoint, true) && compareDeep(numberOfSeries, o.numberOfSeries, true) 3067 && compareDeep(numberOfInstances, o.numberOfInstances, true) && compareDeep(procedureReference, o.procedureReference, true) 3068 && compareDeep(procedureCode, o.procedureCode, true) && compareDeep(reason, o.reason, true) && compareDeep(description, o.description, true) 3069 && compareDeep(series, o.series, true); 3070 } 3071 3072 @Override 3073 public boolean equalsShallow(Base other_) { 3074 if (!super.equalsShallow(other_)) 3075 return false; 3076 if (!(other_ instanceof ImagingStudy)) 3077 return false; 3078 ImagingStudy o = (ImagingStudy) other_; 3079 return compareValues(uid, o.uid, true) && compareValues(availability, o.availability, true) && compareValues(started, o.started, true) 3080 && compareValues(numberOfSeries, o.numberOfSeries, true) && compareValues(numberOfInstances, o.numberOfInstances, true) 3081 && compareValues(description, o.description, true); 3082 } 3083 3084 public boolean isEmpty() { 3085 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(uid, accession, identifier 3086 , availability, modalityList, patient, context, started, basedOn, referrer, interpreter 3087 , endpoint, numberOfSeries, numberOfInstances, procedureReference, procedureCode, reason 3088 , description, series); 3089 } 3090 3091 @Override 3092 public ResourceType getResourceType() { 3093 return ResourceType.ImagingStudy; 3094 } 3095 3096 /** 3097 * Search parameter: <b>identifier</b> 3098 * <p> 3099 * Description: <b>Other identifiers for the Study</b><br> 3100 * Type: <b>token</b><br> 3101 * Path: <b>ImagingStudy.identifier</b><br> 3102 * </p> 3103 */ 3104 @SearchParamDefinition(name="identifier", path="ImagingStudy.identifier", description="Other identifiers for the Study", type="token" ) 3105 public static final String SP_IDENTIFIER = "identifier"; 3106 /** 3107 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3108 * <p> 3109 * Description: <b>Other identifiers for the Study</b><br> 3110 * Type: <b>token</b><br> 3111 * Path: <b>ImagingStudy.identifier</b><br> 3112 * </p> 3113 */ 3114 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3115 3116 /** 3117 * Search parameter: <b>reason</b> 3118 * <p> 3119 * Description: <b>The reason for the study</b><br> 3120 * Type: <b>token</b><br> 3121 * Path: <b>ImagingStudy.reason</b><br> 3122 * </p> 3123 */ 3124 @SearchParamDefinition(name="reason", path="ImagingStudy.reason", description="The reason for the study", type="token" ) 3125 public static final String SP_REASON = "reason"; 3126 /** 3127 * <b>Fluent Client</b> search parameter constant for <b>reason</b> 3128 * <p> 3129 * Description: <b>The reason for the study</b><br> 3130 * Type: <b>token</b><br> 3131 * Path: <b>ImagingStudy.reason</b><br> 3132 * </p> 3133 */ 3134 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON); 3135 3136 /** 3137 * Search parameter: <b>study</b> 3138 * <p> 3139 * Description: <b>The study identifier for the image</b><br> 3140 * Type: <b>uri</b><br> 3141 * Path: <b>ImagingStudy.uid</b><br> 3142 * </p> 3143 */ 3144 @SearchParamDefinition(name="study", path="ImagingStudy.uid", description="The study identifier for the image", type="uri" ) 3145 public static final String SP_STUDY = "study"; 3146 /** 3147 * <b>Fluent Client</b> search parameter constant for <b>study</b> 3148 * <p> 3149 * Description: <b>The study identifier for the image</b><br> 3150 * Type: <b>uri</b><br> 3151 * Path: <b>ImagingStudy.uid</b><br> 3152 * </p> 3153 */ 3154 public static final ca.uhn.fhir.rest.gclient.UriClientParam STUDY = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_STUDY); 3155 3156 /** 3157 * Search parameter: <b>dicom-class</b> 3158 * <p> 3159 * Description: <b>The type of the instance</b><br> 3160 * Type: <b>uri</b><br> 3161 * Path: <b>ImagingStudy.series.instance.sopClass</b><br> 3162 * </p> 3163 */ 3164 @SearchParamDefinition(name="dicom-class", path="ImagingStudy.series.instance.sopClass", description="The type of the instance", type="uri" ) 3165 public static final String SP_DICOM_CLASS = "dicom-class"; 3166 /** 3167 * <b>Fluent Client</b> search parameter constant for <b>dicom-class</b> 3168 * <p> 3169 * Description: <b>The type of the instance</b><br> 3170 * Type: <b>uri</b><br> 3171 * Path: <b>ImagingStudy.series.instance.sopClass</b><br> 3172 * </p> 3173 */ 3174 public static final ca.uhn.fhir.rest.gclient.UriClientParam DICOM_CLASS = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DICOM_CLASS); 3175 3176 /** 3177 * Search parameter: <b>modality</b> 3178 * <p> 3179 * Description: <b>The modality of the series</b><br> 3180 * Type: <b>token</b><br> 3181 * Path: <b>ImagingStudy.series.modality</b><br> 3182 * </p> 3183 */ 3184 @SearchParamDefinition(name="modality", path="ImagingStudy.series.modality", description="The modality of the series", type="token" ) 3185 public static final String SP_MODALITY = "modality"; 3186 /** 3187 * <b>Fluent Client</b> search parameter constant for <b>modality</b> 3188 * <p> 3189 * Description: <b>The modality of the series</b><br> 3190 * Type: <b>token</b><br> 3191 * Path: <b>ImagingStudy.series.modality</b><br> 3192 * </p> 3193 */ 3194 public static final ca.uhn.fhir.rest.gclient.TokenClientParam MODALITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_MODALITY); 3195 3196 /** 3197 * Search parameter: <b>bodysite</b> 3198 * <p> 3199 * Description: <b>The body site studied</b><br> 3200 * Type: <b>token</b><br> 3201 * Path: <b>ImagingStudy.series.bodySite</b><br> 3202 * </p> 3203 */ 3204 @SearchParamDefinition(name="bodysite", path="ImagingStudy.series.bodySite", description="The body site studied", type="token" ) 3205 public static final String SP_BODYSITE = "bodysite"; 3206 /** 3207 * <b>Fluent Client</b> search parameter constant for <b>bodysite</b> 3208 * <p> 3209 * Description: <b>The body site studied</b><br> 3210 * Type: <b>token</b><br> 3211 * Path: <b>ImagingStudy.series.bodySite</b><br> 3212 * </p> 3213 */ 3214 public static final ca.uhn.fhir.rest.gclient.TokenClientParam BODYSITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_BODYSITE); 3215 3216 /** 3217 * Search parameter: <b>performer</b> 3218 * <p> 3219 * Description: <b>The person who performed the study</b><br> 3220 * Type: <b>reference</b><br> 3221 * Path: <b>ImagingStudy.series.performer</b><br> 3222 * </p> 3223 */ 3224 @SearchParamDefinition(name="performer", path="ImagingStudy.series.performer", description="The person who performed the study", type="reference", target={Practitioner.class } ) 3225 public static final String SP_PERFORMER = "performer"; 3226 /** 3227 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 3228 * <p> 3229 * Description: <b>The person who performed the study</b><br> 3230 * Type: <b>reference</b><br> 3231 * Path: <b>ImagingStudy.series.performer</b><br> 3232 * </p> 3233 */ 3234 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 3235 3236/** 3237 * Constant for fluent queries to be used to add include statements. Specifies 3238 * the path value of "<b>ImagingStudy:performer</b>". 3239 */ 3240 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("ImagingStudy:performer").toLocked(); 3241 3242 /** 3243 * Search parameter: <b>started</b> 3244 * <p> 3245 * Description: <b>When the study was started</b><br> 3246 * Type: <b>date</b><br> 3247 * Path: <b>ImagingStudy.started</b><br> 3248 * </p> 3249 */ 3250 @SearchParamDefinition(name="started", path="ImagingStudy.started", description="When the study was started", type="date" ) 3251 public static final String SP_STARTED = "started"; 3252 /** 3253 * <b>Fluent Client</b> search parameter constant for <b>started</b> 3254 * <p> 3255 * Description: <b>When the study was started</b><br> 3256 * Type: <b>date</b><br> 3257 * Path: <b>ImagingStudy.started</b><br> 3258 * </p> 3259 */ 3260 public static final ca.uhn.fhir.rest.gclient.DateClientParam STARTED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_STARTED); 3261 3262 /** 3263 * Search parameter: <b>accession</b> 3264 * <p> 3265 * Description: <b>The accession identifier for the study</b><br> 3266 * Type: <b>token</b><br> 3267 * Path: <b>ImagingStudy.accession</b><br> 3268 * </p> 3269 */ 3270 @SearchParamDefinition(name="accession", path="ImagingStudy.accession", description="The accession identifier for the study", type="token" ) 3271 public static final String SP_ACCESSION = "accession"; 3272 /** 3273 * <b>Fluent Client</b> search parameter constant for <b>accession</b> 3274 * <p> 3275 * Description: <b>The accession identifier for the study</b><br> 3276 * Type: <b>token</b><br> 3277 * Path: <b>ImagingStudy.accession</b><br> 3278 * </p> 3279 */ 3280 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ACCESSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ACCESSION); 3281 3282 /** 3283 * Search parameter: <b>uid</b> 3284 * <p> 3285 * Description: <b>The instance unique identifier</b><br> 3286 * Type: <b>uri</b><br> 3287 * Path: <b>ImagingStudy.series.instance.uid</b><br> 3288 * </p> 3289 */ 3290 @SearchParamDefinition(name="uid", path="ImagingStudy.series.instance.uid", description="The instance unique identifier", type="uri" ) 3291 public static final String SP_UID = "uid"; 3292 /** 3293 * <b>Fluent Client</b> search parameter constant for <b>uid</b> 3294 * <p> 3295 * Description: <b>The instance unique identifier</b><br> 3296 * Type: <b>uri</b><br> 3297 * Path: <b>ImagingStudy.series.instance.uid</b><br> 3298 * </p> 3299 */ 3300 public static final ca.uhn.fhir.rest.gclient.UriClientParam UID = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_UID); 3301 3302 /** 3303 * Search parameter: <b>endpoint</b> 3304 * <p> 3305 * Description: <b>The endpoint for te study or series</b><br> 3306 * Type: <b>reference</b><br> 3307 * Path: <b>ImagingStudy.endpoint, ImagingStudy.series.endpoint</b><br> 3308 * </p> 3309 */ 3310 @SearchParamDefinition(name="endpoint", path="ImagingStudy.endpoint | ImagingStudy.series.endpoint", description="The endpoint for te study or series", type="reference", target={Endpoint.class } ) 3311 public static final String SP_ENDPOINT = "endpoint"; 3312 /** 3313 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 3314 * <p> 3315 * Description: <b>The endpoint for te study or series</b><br> 3316 * Type: <b>reference</b><br> 3317 * Path: <b>ImagingStudy.endpoint, ImagingStudy.series.endpoint</b><br> 3318 * </p> 3319 */ 3320 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 3321 3322/** 3323 * Constant for fluent queries to be used to add include statements. Specifies 3324 * the path value of "<b>ImagingStudy:endpoint</b>". 3325 */ 3326 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("ImagingStudy:endpoint").toLocked(); 3327 3328 /** 3329 * Search parameter: <b>patient</b> 3330 * <p> 3331 * Description: <b>Who the study is about</b><br> 3332 * Type: <b>reference</b><br> 3333 * Path: <b>ImagingStudy.patient</b><br> 3334 * </p> 3335 */ 3336 @SearchParamDefinition(name="patient", path="ImagingStudy.patient", description="Who the study is about", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 3337 public static final String SP_PATIENT = "patient"; 3338 /** 3339 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3340 * <p> 3341 * Description: <b>Who the study is about</b><br> 3342 * Type: <b>reference</b><br> 3343 * Path: <b>ImagingStudy.patient</b><br> 3344 * </p> 3345 */ 3346 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3347 3348/** 3349 * Constant for fluent queries to be used to add include statements. Specifies 3350 * the path value of "<b>ImagingStudy:patient</b>". 3351 */ 3352 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ImagingStudy:patient").toLocked(); 3353 3354 /** 3355 * Search parameter: <b>series</b> 3356 * <p> 3357 * Description: <b>The identifier of the series of images</b><br> 3358 * Type: <b>uri</b><br> 3359 * Path: <b>ImagingStudy.series.uid</b><br> 3360 * </p> 3361 */ 3362 @SearchParamDefinition(name="series", path="ImagingStudy.series.uid", description="The identifier of the series of images", type="uri" ) 3363 public static final String SP_SERIES = "series"; 3364 /** 3365 * <b>Fluent Client</b> search parameter constant for <b>series</b> 3366 * <p> 3367 * Description: <b>The identifier of the series of images</b><br> 3368 * Type: <b>uri</b><br> 3369 * Path: <b>ImagingStudy.series.uid</b><br> 3370 * </p> 3371 */ 3372 public static final ca.uhn.fhir.rest.gclient.UriClientParam SERIES = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_SERIES); 3373 3374 /** 3375 * Search parameter: <b>context</b> 3376 * <p> 3377 * Description: <b>The context of the study</b><br> 3378 * Type: <b>reference</b><br> 3379 * Path: <b>ImagingStudy.context</b><br> 3380 * </p> 3381 */ 3382 @SearchParamDefinition(name="context", path="ImagingStudy.context", description="The context of the study", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 3383 public static final String SP_CONTEXT = "context"; 3384 /** 3385 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3386 * <p> 3387 * Description: <b>The context of the study</b><br> 3388 * Type: <b>reference</b><br> 3389 * Path: <b>ImagingStudy.context</b><br> 3390 * </p> 3391 */ 3392 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3393 3394/** 3395 * Constant for fluent queries to be used to add include statements. Specifies 3396 * the path value of "<b>ImagingStudy:context</b>". 3397 */ 3398 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("ImagingStudy:context").toLocked(); 3399 3400 /** 3401 * Search parameter: <b>basedon</b> 3402 * <p> 3403 * Description: <b>The order for the image</b><br> 3404 * Type: <b>reference</b><br> 3405 * Path: <b>ImagingStudy.basedOn</b><br> 3406 * </p> 3407 */ 3408 @SearchParamDefinition(name="basedon", path="ImagingStudy.basedOn", description="The order for the image", type="reference", target={CarePlan.class, ProcedureRequest.class, ReferralRequest.class } ) 3409 public static final String SP_BASEDON = "basedon"; 3410 /** 3411 * <b>Fluent Client</b> search parameter constant for <b>basedon</b> 3412 * <p> 3413 * Description: <b>The order for the image</b><br> 3414 * Type: <b>reference</b><br> 3415 * Path: <b>ImagingStudy.basedOn</b><br> 3416 * </p> 3417 */ 3418 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASEDON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASEDON); 3419 3420/** 3421 * Constant for fluent queries to be used to add include statements. Specifies 3422 * the path value of "<b>ImagingStudy:basedon</b>". 3423 */ 3424 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASEDON = new ca.uhn.fhir.model.api.Include("ImagingStudy:basedon").toLocked(); 3425 3426 3427}