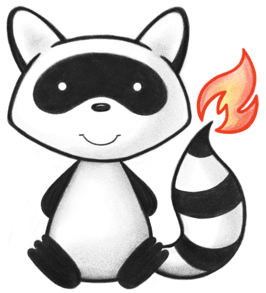
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 050 */ 051@ResourceDef(name="Immunization", profile="http://hl7.org/fhir/Profile/Immunization") 052public class Immunization extends DomainResource { 053 054 public enum ImmunizationStatus { 055 /** 056 * null 057 */ 058 COMPLETED, 059 /** 060 * null 061 */ 062 ENTEREDINERROR, 063 /** 064 * added to help the parsers with the generic types 065 */ 066 NULL; 067 public static ImmunizationStatus fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("completed".equals(codeString)) 071 return COMPLETED; 072 if ("entered-in-error".equals(codeString)) 073 return ENTEREDINERROR; 074 if (Configuration.isAcceptInvalidEnums()) 075 return null; 076 else 077 throw new FHIRException("Unknown ImmunizationStatus code '"+codeString+"'"); 078 } 079 public String toCode() { 080 switch (this) { 081 case COMPLETED: return "completed"; 082 case ENTEREDINERROR: return "entered-in-error"; 083 case NULL: return null; 084 default: return "?"; 085 } 086 } 087 public String getSystem() { 088 switch (this) { 089 case COMPLETED: return "http://hl7.org/fhir/medication-admin-status"; 090 case ENTEREDINERROR: return "http://hl7.org/fhir/medication-admin-status"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getDefinition() { 096 switch (this) { 097 case COMPLETED: return ""; 098 case ENTEREDINERROR: return ""; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDisplay() { 104 switch (this) { 105 case COMPLETED: return "completed"; 106 case ENTEREDINERROR: return "entered-in-error"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 } 112 113 public static class ImmunizationStatusEnumFactory implements EnumFactory<ImmunizationStatus> { 114 public ImmunizationStatus fromCode(String codeString) throws IllegalArgumentException { 115 if (codeString == null || "".equals(codeString)) 116 if (codeString == null || "".equals(codeString)) 117 return null; 118 if ("completed".equals(codeString)) 119 return ImmunizationStatus.COMPLETED; 120 if ("entered-in-error".equals(codeString)) 121 return ImmunizationStatus.ENTEREDINERROR; 122 throw new IllegalArgumentException("Unknown ImmunizationStatus code '"+codeString+"'"); 123 } 124 public Enumeration<ImmunizationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 125 if (code == null) 126 return null; 127 if (code.isEmpty()) 128 return new Enumeration<ImmunizationStatus>(this); 129 String codeString = code.asStringValue(); 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("completed".equals(codeString)) 133 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.COMPLETED); 134 if ("entered-in-error".equals(codeString)) 135 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.ENTEREDINERROR); 136 throw new FHIRException("Unknown ImmunizationStatus code '"+codeString+"'"); 137 } 138 public String toCode(ImmunizationStatus code) { 139 if (code == ImmunizationStatus.NULL) 140 return null; 141 if (code == ImmunizationStatus.COMPLETED) 142 return "completed"; 143 if (code == ImmunizationStatus.ENTEREDINERROR) 144 return "entered-in-error"; 145 return "?"; 146 } 147 public String toSystem(ImmunizationStatus code) { 148 return code.getSystem(); 149 } 150 } 151 152 @Block() 153 public static class ImmunizationPractitionerComponent extends BackboneElement implements IBaseBackboneElement { 154 /** 155 * Describes the type of performance (e.g. ordering provider, administering provider, etc.). 156 */ 157 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 158 @Description(shortDefinition="What type of performance was done", formalDefinition="Describes the type of performance (e.g. ordering provider, administering provider, etc.)." ) 159 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-role") 160 protected CodeableConcept role; 161 162 /** 163 * The device, practitioner, etc. who performed the action. 164 */ 165 @Child(name = "actor", type = {Practitioner.class}, order=2, min=1, max=1, modifier=false, summary=true) 166 @Description(shortDefinition="Individual who was performing", formalDefinition="The device, practitioner, etc. who performed the action." ) 167 protected Reference actor; 168 169 /** 170 * The actual object that is the target of the reference (The device, practitioner, etc. who performed the action.) 171 */ 172 protected Practitioner actorTarget; 173 174 private static final long serialVersionUID = -922003669L; 175 176 /** 177 * Constructor 178 */ 179 public ImmunizationPractitionerComponent() { 180 super(); 181 } 182 183 /** 184 * Constructor 185 */ 186 public ImmunizationPractitionerComponent(Reference actor) { 187 super(); 188 this.actor = actor; 189 } 190 191 /** 192 * @return {@link #role} (Describes the type of performance (e.g. ordering provider, administering provider, etc.).) 193 */ 194 public CodeableConcept getRole() { 195 if (this.role == null) 196 if (Configuration.errorOnAutoCreate()) 197 throw new Error("Attempt to auto-create ImmunizationPractitionerComponent.role"); 198 else if (Configuration.doAutoCreate()) 199 this.role = new CodeableConcept(); // cc 200 return this.role; 201 } 202 203 public boolean hasRole() { 204 return this.role != null && !this.role.isEmpty(); 205 } 206 207 /** 208 * @param value {@link #role} (Describes the type of performance (e.g. ordering provider, administering provider, etc.).) 209 */ 210 public ImmunizationPractitionerComponent setRole(CodeableConcept value) { 211 this.role = value; 212 return this; 213 } 214 215 /** 216 * @return {@link #actor} (The device, practitioner, etc. who performed the action.) 217 */ 218 public Reference getActor() { 219 if (this.actor == null) 220 if (Configuration.errorOnAutoCreate()) 221 throw new Error("Attempt to auto-create ImmunizationPractitionerComponent.actor"); 222 else if (Configuration.doAutoCreate()) 223 this.actor = new Reference(); // cc 224 return this.actor; 225 } 226 227 public boolean hasActor() { 228 return this.actor != null && !this.actor.isEmpty(); 229 } 230 231 /** 232 * @param value {@link #actor} (The device, practitioner, etc. who performed the action.) 233 */ 234 public ImmunizationPractitionerComponent setActor(Reference value) { 235 this.actor = value; 236 return this; 237 } 238 239 /** 240 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed the action.) 241 */ 242 public Practitioner getActorTarget() { 243 if (this.actorTarget == null) 244 if (Configuration.errorOnAutoCreate()) 245 throw new Error("Attempt to auto-create ImmunizationPractitionerComponent.actor"); 246 else if (Configuration.doAutoCreate()) 247 this.actorTarget = new Practitioner(); // aa 248 return this.actorTarget; 249 } 250 251 /** 252 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed the action.) 253 */ 254 public ImmunizationPractitionerComponent setActorTarget(Practitioner value) { 255 this.actorTarget = value; 256 return this; 257 } 258 259 protected void listChildren(List<Property> children) { 260 super.listChildren(children); 261 children.add(new Property("role", "CodeableConcept", "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, role)); 262 children.add(new Property("actor", "Reference(Practitioner)", "The device, practitioner, etc. who performed the action.", 0, 1, actor)); 263 } 264 265 @Override 266 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 267 switch (_hash) { 268 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, role); 269 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner)", "The device, practitioner, etc. who performed the action.", 0, 1, actor); 270 default: return super.getNamedProperty(_hash, _name, _checkValid); 271 } 272 273 } 274 275 @Override 276 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 277 switch (hash) { 278 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 279 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 280 default: return super.getProperty(hash, name, checkValid); 281 } 282 283 } 284 285 @Override 286 public Base setProperty(int hash, String name, Base value) throws FHIRException { 287 switch (hash) { 288 case 3506294: // role 289 this.role = castToCodeableConcept(value); // CodeableConcept 290 return value; 291 case 92645877: // actor 292 this.actor = castToReference(value); // Reference 293 return value; 294 default: return super.setProperty(hash, name, value); 295 } 296 297 } 298 299 @Override 300 public Base setProperty(String name, Base value) throws FHIRException { 301 if (name.equals("role")) { 302 this.role = castToCodeableConcept(value); // CodeableConcept 303 } else if (name.equals("actor")) { 304 this.actor = castToReference(value); // Reference 305 } else 306 return super.setProperty(name, value); 307 return value; 308 } 309 310 @Override 311 public Base makeProperty(int hash, String name) throws FHIRException { 312 switch (hash) { 313 case 3506294: return getRole(); 314 case 92645877: return getActor(); 315 default: return super.makeProperty(hash, name); 316 } 317 318 } 319 320 @Override 321 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 322 switch (hash) { 323 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 324 case 92645877: /*actor*/ return new String[] {"Reference"}; 325 default: return super.getTypesForProperty(hash, name); 326 } 327 328 } 329 330 @Override 331 public Base addChild(String name) throws FHIRException { 332 if (name.equals("role")) { 333 this.role = new CodeableConcept(); 334 return this.role; 335 } 336 else if (name.equals("actor")) { 337 this.actor = new Reference(); 338 return this.actor; 339 } 340 else 341 return super.addChild(name); 342 } 343 344 public ImmunizationPractitionerComponent copy() { 345 ImmunizationPractitionerComponent dst = new ImmunizationPractitionerComponent(); 346 copyValues(dst); 347 dst.role = role == null ? null : role.copy(); 348 dst.actor = actor == null ? null : actor.copy(); 349 return dst; 350 } 351 352 @Override 353 public boolean equalsDeep(Base other_) { 354 if (!super.equalsDeep(other_)) 355 return false; 356 if (!(other_ instanceof ImmunizationPractitionerComponent)) 357 return false; 358 ImmunizationPractitionerComponent o = (ImmunizationPractitionerComponent) other_; 359 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true); 360 } 361 362 @Override 363 public boolean equalsShallow(Base other_) { 364 if (!super.equalsShallow(other_)) 365 return false; 366 if (!(other_ instanceof ImmunizationPractitionerComponent)) 367 return false; 368 ImmunizationPractitionerComponent o = (ImmunizationPractitionerComponent) other_; 369 return true; 370 } 371 372 public boolean isEmpty() { 373 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor); 374 } 375 376 public String fhirType() { 377 return "Immunization.practitioner"; 378 379 } 380 381 } 382 383 @Block() 384 public static class ImmunizationExplanationComponent extends BackboneElement implements IBaseBackboneElement { 385 /** 386 * Reasons why a vaccine was administered. 387 */ 388 @Child(name = "reason", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 389 @Description(shortDefinition="Why immunization occurred", formalDefinition="Reasons why a vaccine was administered." ) 390 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-reason") 391 protected List<CodeableConcept> reason; 392 393 /** 394 * Reason why a vaccine was not administered. 395 */ 396 @Child(name = "reasonNotGiven", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 397 @Description(shortDefinition="Why immunization did not occur", formalDefinition="Reason why a vaccine was not administered." ) 398 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/no-immunization-reason") 399 protected List<CodeableConcept> reasonNotGiven; 400 401 private static final long serialVersionUID = -539821866L; 402 403 /** 404 * Constructor 405 */ 406 public ImmunizationExplanationComponent() { 407 super(); 408 } 409 410 /** 411 * @return {@link #reason} (Reasons why a vaccine was administered.) 412 */ 413 public List<CodeableConcept> getReason() { 414 if (this.reason == null) 415 this.reason = new ArrayList<CodeableConcept>(); 416 return this.reason; 417 } 418 419 /** 420 * @return Returns a reference to <code>this</code> for easy method chaining 421 */ 422 public ImmunizationExplanationComponent setReason(List<CodeableConcept> theReason) { 423 this.reason = theReason; 424 return this; 425 } 426 427 public boolean hasReason() { 428 if (this.reason == null) 429 return false; 430 for (CodeableConcept item : this.reason) 431 if (!item.isEmpty()) 432 return true; 433 return false; 434 } 435 436 public CodeableConcept addReason() { //3 437 CodeableConcept t = new CodeableConcept(); 438 if (this.reason == null) 439 this.reason = new ArrayList<CodeableConcept>(); 440 this.reason.add(t); 441 return t; 442 } 443 444 public ImmunizationExplanationComponent addReason(CodeableConcept t) { //3 445 if (t == null) 446 return this; 447 if (this.reason == null) 448 this.reason = new ArrayList<CodeableConcept>(); 449 this.reason.add(t); 450 return this; 451 } 452 453 /** 454 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist 455 */ 456 public CodeableConcept getReasonFirstRep() { 457 if (getReason().isEmpty()) { 458 addReason(); 459 } 460 return getReason().get(0); 461 } 462 463 /** 464 * @return {@link #reasonNotGiven} (Reason why a vaccine was not administered.) 465 */ 466 public List<CodeableConcept> getReasonNotGiven() { 467 if (this.reasonNotGiven == null) 468 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 469 return this.reasonNotGiven; 470 } 471 472 /** 473 * @return Returns a reference to <code>this</code> for easy method chaining 474 */ 475 public ImmunizationExplanationComponent setReasonNotGiven(List<CodeableConcept> theReasonNotGiven) { 476 this.reasonNotGiven = theReasonNotGiven; 477 return this; 478 } 479 480 public boolean hasReasonNotGiven() { 481 if (this.reasonNotGiven == null) 482 return false; 483 for (CodeableConcept item : this.reasonNotGiven) 484 if (!item.isEmpty()) 485 return true; 486 return false; 487 } 488 489 public CodeableConcept addReasonNotGiven() { //3 490 CodeableConcept t = new CodeableConcept(); 491 if (this.reasonNotGiven == null) 492 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 493 this.reasonNotGiven.add(t); 494 return t; 495 } 496 497 public ImmunizationExplanationComponent addReasonNotGiven(CodeableConcept t) { //3 498 if (t == null) 499 return this; 500 if (this.reasonNotGiven == null) 501 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 502 this.reasonNotGiven.add(t); 503 return this; 504 } 505 506 /** 507 * @return The first repetition of repeating field {@link #reasonNotGiven}, creating it if it does not already exist 508 */ 509 public CodeableConcept getReasonNotGivenFirstRep() { 510 if (getReasonNotGiven().isEmpty()) { 511 addReasonNotGiven(); 512 } 513 return getReasonNotGiven().get(0); 514 } 515 516 protected void listChildren(List<Property> children) { 517 super.listChildren(children); 518 children.add(new Property("reason", "CodeableConcept", "Reasons why a vaccine was administered.", 0, java.lang.Integer.MAX_VALUE, reason)); 519 children.add(new Property("reasonNotGiven", "CodeableConcept", "Reason why a vaccine was not administered.", 0, java.lang.Integer.MAX_VALUE, reasonNotGiven)); 520 } 521 522 @Override 523 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 524 switch (_hash) { 525 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Reasons why a vaccine was administered.", 0, java.lang.Integer.MAX_VALUE, reason); 526 case 2101123790: /*reasonNotGiven*/ return new Property("reasonNotGiven", "CodeableConcept", "Reason why a vaccine was not administered.", 0, java.lang.Integer.MAX_VALUE, reasonNotGiven); 527 default: return super.getNamedProperty(_hash, _name, _checkValid); 528 } 529 530 } 531 532 @Override 533 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 534 switch (hash) { 535 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 536 case 2101123790: /*reasonNotGiven*/ return this.reasonNotGiven == null ? new Base[0] : this.reasonNotGiven.toArray(new Base[this.reasonNotGiven.size()]); // CodeableConcept 537 default: return super.getProperty(hash, name, checkValid); 538 } 539 540 } 541 542 @Override 543 public Base setProperty(int hash, String name, Base value) throws FHIRException { 544 switch (hash) { 545 case -934964668: // reason 546 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 547 return value; 548 case 2101123790: // reasonNotGiven 549 this.getReasonNotGiven().add(castToCodeableConcept(value)); // CodeableConcept 550 return value; 551 default: return super.setProperty(hash, name, value); 552 } 553 554 } 555 556 @Override 557 public Base setProperty(String name, Base value) throws FHIRException { 558 if (name.equals("reason")) { 559 this.getReason().add(castToCodeableConcept(value)); 560 } else if (name.equals("reasonNotGiven")) { 561 this.getReasonNotGiven().add(castToCodeableConcept(value)); 562 } else 563 return super.setProperty(name, value); 564 return value; 565 } 566 567 @Override 568 public Base makeProperty(int hash, String name) throws FHIRException { 569 switch (hash) { 570 case -934964668: return addReason(); 571 case 2101123790: return addReasonNotGiven(); 572 default: return super.makeProperty(hash, name); 573 } 574 575 } 576 577 @Override 578 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 579 switch (hash) { 580 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 581 case 2101123790: /*reasonNotGiven*/ return new String[] {"CodeableConcept"}; 582 default: return super.getTypesForProperty(hash, name); 583 } 584 585 } 586 587 @Override 588 public Base addChild(String name) throws FHIRException { 589 if (name.equals("reason")) { 590 return addReason(); 591 } 592 else if (name.equals("reasonNotGiven")) { 593 return addReasonNotGiven(); 594 } 595 else 596 return super.addChild(name); 597 } 598 599 public ImmunizationExplanationComponent copy() { 600 ImmunizationExplanationComponent dst = new ImmunizationExplanationComponent(); 601 copyValues(dst); 602 if (reason != null) { 603 dst.reason = new ArrayList<CodeableConcept>(); 604 for (CodeableConcept i : reason) 605 dst.reason.add(i.copy()); 606 }; 607 if (reasonNotGiven != null) { 608 dst.reasonNotGiven = new ArrayList<CodeableConcept>(); 609 for (CodeableConcept i : reasonNotGiven) 610 dst.reasonNotGiven.add(i.copy()); 611 }; 612 return dst; 613 } 614 615 @Override 616 public boolean equalsDeep(Base other_) { 617 if (!super.equalsDeep(other_)) 618 return false; 619 if (!(other_ instanceof ImmunizationExplanationComponent)) 620 return false; 621 ImmunizationExplanationComponent o = (ImmunizationExplanationComponent) other_; 622 return compareDeep(reason, o.reason, true) && compareDeep(reasonNotGiven, o.reasonNotGiven, true) 623 ; 624 } 625 626 @Override 627 public boolean equalsShallow(Base other_) { 628 if (!super.equalsShallow(other_)) 629 return false; 630 if (!(other_ instanceof ImmunizationExplanationComponent)) 631 return false; 632 ImmunizationExplanationComponent o = (ImmunizationExplanationComponent) other_; 633 return true; 634 } 635 636 public boolean isEmpty() { 637 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reason, reasonNotGiven); 638 } 639 640 public String fhirType() { 641 return "Immunization.explanation"; 642 643 } 644 645 } 646 647 @Block() 648 public static class ImmunizationReactionComponent extends BackboneElement implements IBaseBackboneElement { 649 /** 650 * Date of reaction to the immunization. 651 */ 652 @Child(name = "date", type = {DateTimeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 653 @Description(shortDefinition="When reaction started", formalDefinition="Date of reaction to the immunization." ) 654 protected DateTimeType date; 655 656 /** 657 * Details of the reaction. 658 */ 659 @Child(name = "detail", type = {Observation.class}, order=2, min=0, max=1, modifier=false, summary=false) 660 @Description(shortDefinition="Additional information on reaction", formalDefinition="Details of the reaction." ) 661 protected Reference detail; 662 663 /** 664 * The actual object that is the target of the reference (Details of the reaction.) 665 */ 666 protected Observation detailTarget; 667 668 /** 669 * Self-reported indicator. 670 */ 671 @Child(name = "reported", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 672 @Description(shortDefinition="Indicates self-reported reaction", formalDefinition="Self-reported indicator." ) 673 protected BooleanType reported; 674 675 private static final long serialVersionUID = -1297668556L; 676 677 /** 678 * Constructor 679 */ 680 public ImmunizationReactionComponent() { 681 super(); 682 } 683 684 /** 685 * @return {@link #date} (Date of reaction to the immunization.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 686 */ 687 public DateTimeType getDateElement() { 688 if (this.date == null) 689 if (Configuration.errorOnAutoCreate()) 690 throw new Error("Attempt to auto-create ImmunizationReactionComponent.date"); 691 else if (Configuration.doAutoCreate()) 692 this.date = new DateTimeType(); // bb 693 return this.date; 694 } 695 696 public boolean hasDateElement() { 697 return this.date != null && !this.date.isEmpty(); 698 } 699 700 public boolean hasDate() { 701 return this.date != null && !this.date.isEmpty(); 702 } 703 704 /** 705 * @param value {@link #date} (Date of reaction to the immunization.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 706 */ 707 public ImmunizationReactionComponent setDateElement(DateTimeType value) { 708 this.date = value; 709 return this; 710 } 711 712 /** 713 * @return Date of reaction to the immunization. 714 */ 715 public Date getDate() { 716 return this.date == null ? null : this.date.getValue(); 717 } 718 719 /** 720 * @param value Date of reaction to the immunization. 721 */ 722 public ImmunizationReactionComponent setDate(Date value) { 723 if (value == null) 724 this.date = null; 725 else { 726 if (this.date == null) 727 this.date = new DateTimeType(); 728 this.date.setValue(value); 729 } 730 return this; 731 } 732 733 /** 734 * @return {@link #detail} (Details of the reaction.) 735 */ 736 public Reference getDetail() { 737 if (this.detail == null) 738 if (Configuration.errorOnAutoCreate()) 739 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 740 else if (Configuration.doAutoCreate()) 741 this.detail = new Reference(); // cc 742 return this.detail; 743 } 744 745 public boolean hasDetail() { 746 return this.detail != null && !this.detail.isEmpty(); 747 } 748 749 /** 750 * @param value {@link #detail} (Details of the reaction.) 751 */ 752 public ImmunizationReactionComponent setDetail(Reference value) { 753 this.detail = value; 754 return this; 755 } 756 757 /** 758 * @return {@link #detail} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Details of the reaction.) 759 */ 760 public Observation getDetailTarget() { 761 if (this.detailTarget == null) 762 if (Configuration.errorOnAutoCreate()) 763 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 764 else if (Configuration.doAutoCreate()) 765 this.detailTarget = new Observation(); // aa 766 return this.detailTarget; 767 } 768 769 /** 770 * @param value {@link #detail} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Details of the reaction.) 771 */ 772 public ImmunizationReactionComponent setDetailTarget(Observation value) { 773 this.detailTarget = value; 774 return this; 775 } 776 777 /** 778 * @return {@link #reported} (Self-reported indicator.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 779 */ 780 public BooleanType getReportedElement() { 781 if (this.reported == null) 782 if (Configuration.errorOnAutoCreate()) 783 throw new Error("Attempt to auto-create ImmunizationReactionComponent.reported"); 784 else if (Configuration.doAutoCreate()) 785 this.reported = new BooleanType(); // bb 786 return this.reported; 787 } 788 789 public boolean hasReportedElement() { 790 return this.reported != null && !this.reported.isEmpty(); 791 } 792 793 public boolean hasReported() { 794 return this.reported != null && !this.reported.isEmpty(); 795 } 796 797 /** 798 * @param value {@link #reported} (Self-reported indicator.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 799 */ 800 public ImmunizationReactionComponent setReportedElement(BooleanType value) { 801 this.reported = value; 802 return this; 803 } 804 805 /** 806 * @return Self-reported indicator. 807 */ 808 public boolean getReported() { 809 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 810 } 811 812 /** 813 * @param value Self-reported indicator. 814 */ 815 public ImmunizationReactionComponent setReported(boolean value) { 816 if (this.reported == null) 817 this.reported = new BooleanType(); 818 this.reported.setValue(value); 819 return this; 820 } 821 822 protected void listChildren(List<Property> children) { 823 super.listChildren(children); 824 children.add(new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date)); 825 children.add(new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 1, detail)); 826 children.add(new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported)); 827 } 828 829 @Override 830 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 831 switch (_hash) { 832 case 3076014: /*date*/ return new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date); 833 case -1335224239: /*detail*/ return new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 1, detail); 834 case -427039533: /*reported*/ return new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported); 835 default: return super.getNamedProperty(_hash, _name, _checkValid); 836 } 837 838 } 839 840 @Override 841 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 842 switch (hash) { 843 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 844 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // Reference 845 case -427039533: /*reported*/ return this.reported == null ? new Base[0] : new Base[] {this.reported}; // BooleanType 846 default: return super.getProperty(hash, name, checkValid); 847 } 848 849 } 850 851 @Override 852 public Base setProperty(int hash, String name, Base value) throws FHIRException { 853 switch (hash) { 854 case 3076014: // date 855 this.date = castToDateTime(value); // DateTimeType 856 return value; 857 case -1335224239: // detail 858 this.detail = castToReference(value); // Reference 859 return value; 860 case -427039533: // reported 861 this.reported = castToBoolean(value); // BooleanType 862 return value; 863 default: return super.setProperty(hash, name, value); 864 } 865 866 } 867 868 @Override 869 public Base setProperty(String name, Base value) throws FHIRException { 870 if (name.equals("date")) { 871 this.date = castToDateTime(value); // DateTimeType 872 } else if (name.equals("detail")) { 873 this.detail = castToReference(value); // Reference 874 } else if (name.equals("reported")) { 875 this.reported = castToBoolean(value); // BooleanType 876 } else 877 return super.setProperty(name, value); 878 return value; 879 } 880 881 @Override 882 public Base makeProperty(int hash, String name) throws FHIRException { 883 switch (hash) { 884 case 3076014: return getDateElement(); 885 case -1335224239: return getDetail(); 886 case -427039533: return getReportedElement(); 887 default: return super.makeProperty(hash, name); 888 } 889 890 } 891 892 @Override 893 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 894 switch (hash) { 895 case 3076014: /*date*/ return new String[] {"dateTime"}; 896 case -1335224239: /*detail*/ return new String[] {"Reference"}; 897 case -427039533: /*reported*/ return new String[] {"boolean"}; 898 default: return super.getTypesForProperty(hash, name); 899 } 900 901 } 902 903 @Override 904 public Base addChild(String name) throws FHIRException { 905 if (name.equals("date")) { 906 throw new FHIRException("Cannot call addChild on a singleton property Immunization.date"); 907 } 908 else if (name.equals("detail")) { 909 this.detail = new Reference(); 910 return this.detail; 911 } 912 else if (name.equals("reported")) { 913 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reported"); 914 } 915 else 916 return super.addChild(name); 917 } 918 919 public ImmunizationReactionComponent copy() { 920 ImmunizationReactionComponent dst = new ImmunizationReactionComponent(); 921 copyValues(dst); 922 dst.date = date == null ? null : date.copy(); 923 dst.detail = detail == null ? null : detail.copy(); 924 dst.reported = reported == null ? null : reported.copy(); 925 return dst; 926 } 927 928 @Override 929 public boolean equalsDeep(Base other_) { 930 if (!super.equalsDeep(other_)) 931 return false; 932 if (!(other_ instanceof ImmunizationReactionComponent)) 933 return false; 934 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 935 return compareDeep(date, o.date, true) && compareDeep(detail, o.detail, true) && compareDeep(reported, o.reported, true) 936 ; 937 } 938 939 @Override 940 public boolean equalsShallow(Base other_) { 941 if (!super.equalsShallow(other_)) 942 return false; 943 if (!(other_ instanceof ImmunizationReactionComponent)) 944 return false; 945 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 946 return compareValues(date, o.date, true) && compareValues(reported, o.reported, true); 947 } 948 949 public boolean isEmpty() { 950 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, detail, reported); 951 } 952 953 public String fhirType() { 954 return "Immunization.reaction"; 955 956 } 957 958 } 959 960 @Block() 961 public static class ImmunizationVaccinationProtocolComponent extends BackboneElement implements IBaseBackboneElement { 962 /** 963 * Nominal position in a series. 964 */ 965 @Child(name = "doseSequence", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 966 @Description(shortDefinition="Dose number within series", formalDefinition="Nominal position in a series." ) 967 protected PositiveIntType doseSequence; 968 969 /** 970 * Contains the description about the protocol under which the vaccine was administered. 971 */ 972 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 973 @Description(shortDefinition="Details of vaccine protocol", formalDefinition="Contains the description about the protocol under which the vaccine was administered." ) 974 protected StringType description; 975 976 /** 977 * Indicates the authority who published the protocol. E.g. ACIP. 978 */ 979 @Child(name = "authority", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 980 @Description(shortDefinition="Who is responsible for protocol", formalDefinition="Indicates the authority who published the protocol. E.g. ACIP." ) 981 protected Reference authority; 982 983 /** 984 * The actual object that is the target of the reference (Indicates the authority who published the protocol. E.g. ACIP.) 985 */ 986 protected Organization authorityTarget; 987 988 /** 989 * One possible path to achieve presumed immunity against a disease - within the context of an authority. 990 */ 991 @Child(name = "series", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 992 @Description(shortDefinition="Name of vaccine series", formalDefinition="One possible path to achieve presumed immunity against a disease - within the context of an authority." ) 993 protected StringType series; 994 995 /** 996 * The recommended number of doses to achieve immunity. 997 */ 998 @Child(name = "seriesDoses", type = {PositiveIntType.class}, order=5, min=0, max=1, modifier=false, summary=false) 999 @Description(shortDefinition="Recommended number of doses for immunity", formalDefinition="The recommended number of doses to achieve immunity." ) 1000 protected PositiveIntType seriesDoses; 1001 1002 /** 1003 * The targeted disease. 1004 */ 1005 @Child(name = "targetDisease", type = {CodeableConcept.class}, order=6, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1006 @Description(shortDefinition="Disease immunized against", formalDefinition="The targeted disease." ) 1007 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccination-protocol-dose-target") 1008 protected List<CodeableConcept> targetDisease; 1009 1010 /** 1011 * Indicates if the immunization event should "count" against the protocol. 1012 */ 1013 @Child(name = "doseStatus", type = {CodeableConcept.class}, order=7, min=1, max=1, modifier=false, summary=false) 1014 @Description(shortDefinition="Indicates if dose counts towards immunity", formalDefinition="Indicates if the immunization event should \"count\" against the protocol." ) 1015 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccination-protocol-dose-status") 1016 protected CodeableConcept doseStatus; 1017 1018 /** 1019 * Provides an explanation as to why an immunization event should or should not count against the protocol. 1020 */ 1021 @Child(name = "doseStatusReason", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 1022 @Description(shortDefinition="Why dose does (not) count", formalDefinition="Provides an explanation as to why an immunization event should or should not count against the protocol." ) 1023 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccination-protocol-dose-status-reason") 1024 protected CodeableConcept doseStatusReason; 1025 1026 private static final long serialVersionUID = 386814037L; 1027 1028 /** 1029 * Constructor 1030 */ 1031 public ImmunizationVaccinationProtocolComponent() { 1032 super(); 1033 } 1034 1035 /** 1036 * Constructor 1037 */ 1038 public ImmunizationVaccinationProtocolComponent(CodeableConcept doseStatus) { 1039 super(); 1040 this.doseStatus = doseStatus; 1041 } 1042 1043 /** 1044 * @return {@link #doseSequence} (Nominal position in a series.). This is the underlying object with id, value and extensions. The accessor "getDoseSequence" gives direct access to the value 1045 */ 1046 public PositiveIntType getDoseSequenceElement() { 1047 if (this.doseSequence == null) 1048 if (Configuration.errorOnAutoCreate()) 1049 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseSequence"); 1050 else if (Configuration.doAutoCreate()) 1051 this.doseSequence = new PositiveIntType(); // bb 1052 return this.doseSequence; 1053 } 1054 1055 public boolean hasDoseSequenceElement() { 1056 return this.doseSequence != null && !this.doseSequence.isEmpty(); 1057 } 1058 1059 public boolean hasDoseSequence() { 1060 return this.doseSequence != null && !this.doseSequence.isEmpty(); 1061 } 1062 1063 /** 1064 * @param value {@link #doseSequence} (Nominal position in a series.). This is the underlying object with id, value and extensions. The accessor "getDoseSequence" gives direct access to the value 1065 */ 1066 public ImmunizationVaccinationProtocolComponent setDoseSequenceElement(PositiveIntType value) { 1067 this.doseSequence = value; 1068 return this; 1069 } 1070 1071 /** 1072 * @return Nominal position in a series. 1073 */ 1074 public int getDoseSequence() { 1075 return this.doseSequence == null || this.doseSequence.isEmpty() ? 0 : this.doseSequence.getValue(); 1076 } 1077 1078 /** 1079 * @param value Nominal position in a series. 1080 */ 1081 public ImmunizationVaccinationProtocolComponent setDoseSequence(int value) { 1082 if (this.doseSequence == null) 1083 this.doseSequence = new PositiveIntType(); 1084 this.doseSequence.setValue(value); 1085 return this; 1086 } 1087 1088 /** 1089 * @return {@link #description} (Contains the description about the protocol under which the vaccine was administered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1090 */ 1091 public StringType getDescriptionElement() { 1092 if (this.description == null) 1093 if (Configuration.errorOnAutoCreate()) 1094 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.description"); 1095 else if (Configuration.doAutoCreate()) 1096 this.description = new StringType(); // bb 1097 return this.description; 1098 } 1099 1100 public boolean hasDescriptionElement() { 1101 return this.description != null && !this.description.isEmpty(); 1102 } 1103 1104 public boolean hasDescription() { 1105 return this.description != null && !this.description.isEmpty(); 1106 } 1107 1108 /** 1109 * @param value {@link #description} (Contains the description about the protocol under which the vaccine was administered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1110 */ 1111 public ImmunizationVaccinationProtocolComponent setDescriptionElement(StringType value) { 1112 this.description = value; 1113 return this; 1114 } 1115 1116 /** 1117 * @return Contains the description about the protocol under which the vaccine was administered. 1118 */ 1119 public String getDescription() { 1120 return this.description == null ? null : this.description.getValue(); 1121 } 1122 1123 /** 1124 * @param value Contains the description about the protocol under which the vaccine was administered. 1125 */ 1126 public ImmunizationVaccinationProtocolComponent setDescription(String value) { 1127 if (Utilities.noString(value)) 1128 this.description = null; 1129 else { 1130 if (this.description == null) 1131 this.description = new StringType(); 1132 this.description.setValue(value); 1133 } 1134 return this; 1135 } 1136 1137 /** 1138 * @return {@link #authority} (Indicates the authority who published the protocol. E.g. ACIP.) 1139 */ 1140 public Reference getAuthority() { 1141 if (this.authority == null) 1142 if (Configuration.errorOnAutoCreate()) 1143 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.authority"); 1144 else if (Configuration.doAutoCreate()) 1145 this.authority = new Reference(); // cc 1146 return this.authority; 1147 } 1148 1149 public boolean hasAuthority() { 1150 return this.authority != null && !this.authority.isEmpty(); 1151 } 1152 1153 /** 1154 * @param value {@link #authority} (Indicates the authority who published the protocol. E.g. ACIP.) 1155 */ 1156 public ImmunizationVaccinationProtocolComponent setAuthority(Reference value) { 1157 this.authority = value; 1158 return this; 1159 } 1160 1161 /** 1162 * @return {@link #authority} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the authority who published the protocol. E.g. ACIP.) 1163 */ 1164 public Organization getAuthorityTarget() { 1165 if (this.authorityTarget == null) 1166 if (Configuration.errorOnAutoCreate()) 1167 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.authority"); 1168 else if (Configuration.doAutoCreate()) 1169 this.authorityTarget = new Organization(); // aa 1170 return this.authorityTarget; 1171 } 1172 1173 /** 1174 * @param value {@link #authority} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the authority who published the protocol. E.g. ACIP.) 1175 */ 1176 public ImmunizationVaccinationProtocolComponent setAuthorityTarget(Organization value) { 1177 this.authorityTarget = value; 1178 return this; 1179 } 1180 1181 /** 1182 * @return {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 1183 */ 1184 public StringType getSeriesElement() { 1185 if (this.series == null) 1186 if (Configuration.errorOnAutoCreate()) 1187 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.series"); 1188 else if (Configuration.doAutoCreate()) 1189 this.series = new StringType(); // bb 1190 return this.series; 1191 } 1192 1193 public boolean hasSeriesElement() { 1194 return this.series != null && !this.series.isEmpty(); 1195 } 1196 1197 public boolean hasSeries() { 1198 return this.series != null && !this.series.isEmpty(); 1199 } 1200 1201 /** 1202 * @param value {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 1203 */ 1204 public ImmunizationVaccinationProtocolComponent setSeriesElement(StringType value) { 1205 this.series = value; 1206 return this; 1207 } 1208 1209 /** 1210 * @return One possible path to achieve presumed immunity against a disease - within the context of an authority. 1211 */ 1212 public String getSeries() { 1213 return this.series == null ? null : this.series.getValue(); 1214 } 1215 1216 /** 1217 * @param value One possible path to achieve presumed immunity against a disease - within the context of an authority. 1218 */ 1219 public ImmunizationVaccinationProtocolComponent setSeries(String value) { 1220 if (Utilities.noString(value)) 1221 this.series = null; 1222 else { 1223 if (this.series == null) 1224 this.series = new StringType(); 1225 this.series.setValue(value); 1226 } 1227 return this; 1228 } 1229 1230 /** 1231 * @return {@link #seriesDoses} (The recommended number of doses to achieve immunity.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 1232 */ 1233 public PositiveIntType getSeriesDosesElement() { 1234 if (this.seriesDoses == null) 1235 if (Configuration.errorOnAutoCreate()) 1236 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.seriesDoses"); 1237 else if (Configuration.doAutoCreate()) 1238 this.seriesDoses = new PositiveIntType(); // bb 1239 return this.seriesDoses; 1240 } 1241 1242 public boolean hasSeriesDosesElement() { 1243 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 1244 } 1245 1246 public boolean hasSeriesDoses() { 1247 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 1248 } 1249 1250 /** 1251 * @param value {@link #seriesDoses} (The recommended number of doses to achieve immunity.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 1252 */ 1253 public ImmunizationVaccinationProtocolComponent setSeriesDosesElement(PositiveIntType value) { 1254 this.seriesDoses = value; 1255 return this; 1256 } 1257 1258 /** 1259 * @return The recommended number of doses to achieve immunity. 1260 */ 1261 public int getSeriesDoses() { 1262 return this.seriesDoses == null || this.seriesDoses.isEmpty() ? 0 : this.seriesDoses.getValue(); 1263 } 1264 1265 /** 1266 * @param value The recommended number of doses to achieve immunity. 1267 */ 1268 public ImmunizationVaccinationProtocolComponent setSeriesDoses(int value) { 1269 if (this.seriesDoses == null) 1270 this.seriesDoses = new PositiveIntType(); 1271 this.seriesDoses.setValue(value); 1272 return this; 1273 } 1274 1275 /** 1276 * @return {@link #targetDisease} (The targeted disease.) 1277 */ 1278 public List<CodeableConcept> getTargetDisease() { 1279 if (this.targetDisease == null) 1280 this.targetDisease = new ArrayList<CodeableConcept>(); 1281 return this.targetDisease; 1282 } 1283 1284 /** 1285 * @return Returns a reference to <code>this</code> for easy method chaining 1286 */ 1287 public ImmunizationVaccinationProtocolComponent setTargetDisease(List<CodeableConcept> theTargetDisease) { 1288 this.targetDisease = theTargetDisease; 1289 return this; 1290 } 1291 1292 public boolean hasTargetDisease() { 1293 if (this.targetDisease == null) 1294 return false; 1295 for (CodeableConcept item : this.targetDisease) 1296 if (!item.isEmpty()) 1297 return true; 1298 return false; 1299 } 1300 1301 public CodeableConcept addTargetDisease() { //3 1302 CodeableConcept t = new CodeableConcept(); 1303 if (this.targetDisease == null) 1304 this.targetDisease = new ArrayList<CodeableConcept>(); 1305 this.targetDisease.add(t); 1306 return t; 1307 } 1308 1309 public ImmunizationVaccinationProtocolComponent addTargetDisease(CodeableConcept t) { //3 1310 if (t == null) 1311 return this; 1312 if (this.targetDisease == null) 1313 this.targetDisease = new ArrayList<CodeableConcept>(); 1314 this.targetDisease.add(t); 1315 return this; 1316 } 1317 1318 /** 1319 * @return The first repetition of repeating field {@link #targetDisease}, creating it if it does not already exist 1320 */ 1321 public CodeableConcept getTargetDiseaseFirstRep() { 1322 if (getTargetDisease().isEmpty()) { 1323 addTargetDisease(); 1324 } 1325 return getTargetDisease().get(0); 1326 } 1327 1328 /** 1329 * @return {@link #doseStatus} (Indicates if the immunization event should "count" against the protocol.) 1330 */ 1331 public CodeableConcept getDoseStatus() { 1332 if (this.doseStatus == null) 1333 if (Configuration.errorOnAutoCreate()) 1334 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseStatus"); 1335 else if (Configuration.doAutoCreate()) 1336 this.doseStatus = new CodeableConcept(); // cc 1337 return this.doseStatus; 1338 } 1339 1340 public boolean hasDoseStatus() { 1341 return this.doseStatus != null && !this.doseStatus.isEmpty(); 1342 } 1343 1344 /** 1345 * @param value {@link #doseStatus} (Indicates if the immunization event should "count" against the protocol.) 1346 */ 1347 public ImmunizationVaccinationProtocolComponent setDoseStatus(CodeableConcept value) { 1348 this.doseStatus = value; 1349 return this; 1350 } 1351 1352 /** 1353 * @return {@link #doseStatusReason} (Provides an explanation as to why an immunization event should or should not count against the protocol.) 1354 */ 1355 public CodeableConcept getDoseStatusReason() { 1356 if (this.doseStatusReason == null) 1357 if (Configuration.errorOnAutoCreate()) 1358 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseStatusReason"); 1359 else if (Configuration.doAutoCreate()) 1360 this.doseStatusReason = new CodeableConcept(); // cc 1361 return this.doseStatusReason; 1362 } 1363 1364 public boolean hasDoseStatusReason() { 1365 return this.doseStatusReason != null && !this.doseStatusReason.isEmpty(); 1366 } 1367 1368 /** 1369 * @param value {@link #doseStatusReason} (Provides an explanation as to why an immunization event should or should not count against the protocol.) 1370 */ 1371 public ImmunizationVaccinationProtocolComponent setDoseStatusReason(CodeableConcept value) { 1372 this.doseStatusReason = value; 1373 return this; 1374 } 1375 1376 protected void listChildren(List<Property> children) { 1377 super.listChildren(children); 1378 children.add(new Property("doseSequence", "positiveInt", "Nominal position in a series.", 0, 1, doseSequence)); 1379 children.add(new Property("description", "string", "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description)); 1380 children.add(new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol. E.g. ACIP.", 0, 1, authority)); 1381 children.add(new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series)); 1382 children.add(new Property("seriesDoses", "positiveInt", "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses)); 1383 children.add(new Property("targetDisease", "CodeableConcept", "The targeted disease.", 0, java.lang.Integer.MAX_VALUE, targetDisease)); 1384 children.add(new Property("doseStatus", "CodeableConcept", "Indicates if the immunization event should \"count\" against the protocol.", 0, 1, doseStatus)); 1385 children.add(new Property("doseStatusReason", "CodeableConcept", "Provides an explanation as to why an immunization event should or should not count against the protocol.", 0, 1, doseStatusReason)); 1386 } 1387 1388 @Override 1389 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1390 switch (_hash) { 1391 case 550933246: /*doseSequence*/ return new Property("doseSequence", "positiveInt", "Nominal position in a series.", 0, 1, doseSequence); 1392 case -1724546052: /*description*/ return new Property("description", "string", "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description); 1393 case 1475610435: /*authority*/ return new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol. E.g. ACIP.", 0, 1, authority); 1394 case -905838985: /*series*/ return new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series); 1395 case -1936727105: /*seriesDoses*/ return new Property("seriesDoses", "positiveInt", "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1396 case -319593813: /*targetDisease*/ return new Property("targetDisease", "CodeableConcept", "The targeted disease.", 0, java.lang.Integer.MAX_VALUE, targetDisease); 1397 case -745826705: /*doseStatus*/ return new Property("doseStatus", "CodeableConcept", "Indicates if the immunization event should \"count\" against the protocol.", 0, 1, doseStatus); 1398 case 662783379: /*doseStatusReason*/ return new Property("doseStatusReason", "CodeableConcept", "Provides an explanation as to why an immunization event should or should not count against the protocol.", 0, 1, doseStatusReason); 1399 default: return super.getNamedProperty(_hash, _name, _checkValid); 1400 } 1401 1402 } 1403 1404 @Override 1405 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1406 switch (hash) { 1407 case 550933246: /*doseSequence*/ return this.doseSequence == null ? new Base[0] : new Base[] {this.doseSequence}; // PositiveIntType 1408 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1409 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // Reference 1410 case -905838985: /*series*/ return this.series == null ? new Base[0] : new Base[] {this.series}; // StringType 1411 case -1936727105: /*seriesDoses*/ return this.seriesDoses == null ? new Base[0] : new Base[] {this.seriesDoses}; // PositiveIntType 1412 case -319593813: /*targetDisease*/ return this.targetDisease == null ? new Base[0] : this.targetDisease.toArray(new Base[this.targetDisease.size()]); // CodeableConcept 1413 case -745826705: /*doseStatus*/ return this.doseStatus == null ? new Base[0] : new Base[] {this.doseStatus}; // CodeableConcept 1414 case 662783379: /*doseStatusReason*/ return this.doseStatusReason == null ? new Base[0] : new Base[] {this.doseStatusReason}; // CodeableConcept 1415 default: return super.getProperty(hash, name, checkValid); 1416 } 1417 1418 } 1419 1420 @Override 1421 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1422 switch (hash) { 1423 case 550933246: // doseSequence 1424 this.doseSequence = castToPositiveInt(value); // PositiveIntType 1425 return value; 1426 case -1724546052: // description 1427 this.description = castToString(value); // StringType 1428 return value; 1429 case 1475610435: // authority 1430 this.authority = castToReference(value); // Reference 1431 return value; 1432 case -905838985: // series 1433 this.series = castToString(value); // StringType 1434 return value; 1435 case -1936727105: // seriesDoses 1436 this.seriesDoses = castToPositiveInt(value); // PositiveIntType 1437 return value; 1438 case -319593813: // targetDisease 1439 this.getTargetDisease().add(castToCodeableConcept(value)); // CodeableConcept 1440 return value; 1441 case -745826705: // doseStatus 1442 this.doseStatus = castToCodeableConcept(value); // CodeableConcept 1443 return value; 1444 case 662783379: // doseStatusReason 1445 this.doseStatusReason = castToCodeableConcept(value); // CodeableConcept 1446 return value; 1447 default: return super.setProperty(hash, name, value); 1448 } 1449 1450 } 1451 1452 @Override 1453 public Base setProperty(String name, Base value) throws FHIRException { 1454 if (name.equals("doseSequence")) { 1455 this.doseSequence = castToPositiveInt(value); // PositiveIntType 1456 } else if (name.equals("description")) { 1457 this.description = castToString(value); // StringType 1458 } else if (name.equals("authority")) { 1459 this.authority = castToReference(value); // Reference 1460 } else if (name.equals("series")) { 1461 this.series = castToString(value); // StringType 1462 } else if (name.equals("seriesDoses")) { 1463 this.seriesDoses = castToPositiveInt(value); // PositiveIntType 1464 } else if (name.equals("targetDisease")) { 1465 this.getTargetDisease().add(castToCodeableConcept(value)); 1466 } else if (name.equals("doseStatus")) { 1467 this.doseStatus = castToCodeableConcept(value); // CodeableConcept 1468 } else if (name.equals("doseStatusReason")) { 1469 this.doseStatusReason = castToCodeableConcept(value); // CodeableConcept 1470 } else 1471 return super.setProperty(name, value); 1472 return value; 1473 } 1474 1475 @Override 1476 public Base makeProperty(int hash, String name) throws FHIRException { 1477 switch (hash) { 1478 case 550933246: return getDoseSequenceElement(); 1479 case -1724546052: return getDescriptionElement(); 1480 case 1475610435: return getAuthority(); 1481 case -905838985: return getSeriesElement(); 1482 case -1936727105: return getSeriesDosesElement(); 1483 case -319593813: return addTargetDisease(); 1484 case -745826705: return getDoseStatus(); 1485 case 662783379: return getDoseStatusReason(); 1486 default: return super.makeProperty(hash, name); 1487 } 1488 1489 } 1490 1491 @Override 1492 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1493 switch (hash) { 1494 case 550933246: /*doseSequence*/ return new String[] {"positiveInt"}; 1495 case -1724546052: /*description*/ return new String[] {"string"}; 1496 case 1475610435: /*authority*/ return new String[] {"Reference"}; 1497 case -905838985: /*series*/ return new String[] {"string"}; 1498 case -1936727105: /*seriesDoses*/ return new String[] {"positiveInt"}; 1499 case -319593813: /*targetDisease*/ return new String[] {"CodeableConcept"}; 1500 case -745826705: /*doseStatus*/ return new String[] {"CodeableConcept"}; 1501 case 662783379: /*doseStatusReason*/ return new String[] {"CodeableConcept"}; 1502 default: return super.getTypesForProperty(hash, name); 1503 } 1504 1505 } 1506 1507 @Override 1508 public Base addChild(String name) throws FHIRException { 1509 if (name.equals("doseSequence")) { 1510 throw new FHIRException("Cannot call addChild on a singleton property Immunization.doseSequence"); 1511 } 1512 else if (name.equals("description")) { 1513 throw new FHIRException("Cannot call addChild on a singleton property Immunization.description"); 1514 } 1515 else if (name.equals("authority")) { 1516 this.authority = new Reference(); 1517 return this.authority; 1518 } 1519 else if (name.equals("series")) { 1520 throw new FHIRException("Cannot call addChild on a singleton property Immunization.series"); 1521 } 1522 else if (name.equals("seriesDoses")) { 1523 throw new FHIRException("Cannot call addChild on a singleton property Immunization.seriesDoses"); 1524 } 1525 else if (name.equals("targetDisease")) { 1526 return addTargetDisease(); 1527 } 1528 else if (name.equals("doseStatus")) { 1529 this.doseStatus = new CodeableConcept(); 1530 return this.doseStatus; 1531 } 1532 else if (name.equals("doseStatusReason")) { 1533 this.doseStatusReason = new CodeableConcept(); 1534 return this.doseStatusReason; 1535 } 1536 else 1537 return super.addChild(name); 1538 } 1539 1540 public ImmunizationVaccinationProtocolComponent copy() { 1541 ImmunizationVaccinationProtocolComponent dst = new ImmunizationVaccinationProtocolComponent(); 1542 copyValues(dst); 1543 dst.doseSequence = doseSequence == null ? null : doseSequence.copy(); 1544 dst.description = description == null ? null : description.copy(); 1545 dst.authority = authority == null ? null : authority.copy(); 1546 dst.series = series == null ? null : series.copy(); 1547 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1548 if (targetDisease != null) { 1549 dst.targetDisease = new ArrayList<CodeableConcept>(); 1550 for (CodeableConcept i : targetDisease) 1551 dst.targetDisease.add(i.copy()); 1552 }; 1553 dst.doseStatus = doseStatus == null ? null : doseStatus.copy(); 1554 dst.doseStatusReason = doseStatusReason == null ? null : doseStatusReason.copy(); 1555 return dst; 1556 } 1557 1558 @Override 1559 public boolean equalsDeep(Base other_) { 1560 if (!super.equalsDeep(other_)) 1561 return false; 1562 if (!(other_ instanceof ImmunizationVaccinationProtocolComponent)) 1563 return false; 1564 ImmunizationVaccinationProtocolComponent o = (ImmunizationVaccinationProtocolComponent) other_; 1565 return compareDeep(doseSequence, o.doseSequence, true) && compareDeep(description, o.description, true) 1566 && compareDeep(authority, o.authority, true) && compareDeep(series, o.series, true) && compareDeep(seriesDoses, o.seriesDoses, true) 1567 && compareDeep(targetDisease, o.targetDisease, true) && compareDeep(doseStatus, o.doseStatus, true) 1568 && compareDeep(doseStatusReason, o.doseStatusReason, true); 1569 } 1570 1571 @Override 1572 public boolean equalsShallow(Base other_) { 1573 if (!super.equalsShallow(other_)) 1574 return false; 1575 if (!(other_ instanceof ImmunizationVaccinationProtocolComponent)) 1576 return false; 1577 ImmunizationVaccinationProtocolComponent o = (ImmunizationVaccinationProtocolComponent) other_; 1578 return compareValues(doseSequence, o.doseSequence, true) && compareValues(description, o.description, true) 1579 && compareValues(series, o.series, true) && compareValues(seriesDoses, o.seriesDoses, true); 1580 } 1581 1582 public boolean isEmpty() { 1583 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(doseSequence, description 1584 , authority, series, seriesDoses, targetDisease, doseStatus, doseStatusReason); 1585 } 1586 1587 public String fhirType() { 1588 return "Immunization.vaccinationProtocol"; 1589 1590 } 1591 1592 } 1593 1594 /** 1595 * A unique identifier assigned to this immunization record. 1596 */ 1597 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1598 @Description(shortDefinition="Business identifier", formalDefinition="A unique identifier assigned to this immunization record." ) 1599 protected List<Identifier> identifier; 1600 1601 /** 1602 * Indicates the current status of the vaccination event. 1603 */ 1604 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1605 @Description(shortDefinition="completed | entered-in-error", formalDefinition="Indicates the current status of the vaccination event." ) 1606 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-status") 1607 protected Enumeration<ImmunizationStatus> status; 1608 1609 /** 1610 * Indicates if the vaccination was or was not given. 1611 */ 1612 @Child(name = "notGiven", type = {BooleanType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1613 @Description(shortDefinition="Flag for whether immunization was given", formalDefinition="Indicates if the vaccination was or was not given." ) 1614 protected BooleanType notGiven; 1615 1616 /** 1617 * Vaccine that was administered or was to be administered. 1618 */ 1619 @Child(name = "vaccineCode", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=false) 1620 @Description(shortDefinition="Vaccine product administered", formalDefinition="Vaccine that was administered or was to be administered." ) 1621 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccine-code") 1622 protected CodeableConcept vaccineCode; 1623 1624 /** 1625 * The patient who either received or did not receive the immunization. 1626 */ 1627 @Child(name = "patient", type = {Patient.class}, order=4, min=1, max=1, modifier=false, summary=false) 1628 @Description(shortDefinition="Who was immunized", formalDefinition="The patient who either received or did not receive the immunization." ) 1629 protected Reference patient; 1630 1631 /** 1632 * The actual object that is the target of the reference (The patient who either received or did not receive the immunization.) 1633 */ 1634 protected Patient patientTarget; 1635 1636 /** 1637 * The visit or admission or other contact between patient and health care provider the immunization was performed as part of. 1638 */ 1639 @Child(name = "encounter", type = {Encounter.class}, order=5, min=0, max=1, modifier=false, summary=false) 1640 @Description(shortDefinition="Encounter administered as part of", formalDefinition="The visit or admission or other contact between patient and health care provider the immunization was performed as part of." ) 1641 protected Reference encounter; 1642 1643 /** 1644 * The actual object that is the target of the reference (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 1645 */ 1646 protected Encounter encounterTarget; 1647 1648 /** 1649 * Date vaccine administered or was to be administered. 1650 */ 1651 @Child(name = "date", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1652 @Description(shortDefinition="Vaccination administration date", formalDefinition="Date vaccine administered or was to be administered." ) 1653 protected DateTimeType date; 1654 1655 /** 1656 * An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded. 1657 */ 1658 @Child(name = "primarySource", type = {BooleanType.class}, order=7, min=1, max=1, modifier=false, summary=false) 1659 @Description(shortDefinition="Indicates context the data was recorded in", formalDefinition="An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded." ) 1660 protected BooleanType primarySource; 1661 1662 /** 1663 * The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine. 1664 */ 1665 @Child(name = "reportOrigin", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 1666 @Description(shortDefinition="Indicates the source of a secondarily reported record", formalDefinition="The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine." ) 1667 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-origin") 1668 protected CodeableConcept reportOrigin; 1669 1670 /** 1671 * The service delivery location where the vaccine administration occurred. 1672 */ 1673 @Child(name = "location", type = {Location.class}, order=9, min=0, max=1, modifier=false, summary=false) 1674 @Description(shortDefinition="Where vaccination occurred", formalDefinition="The service delivery location where the vaccine administration occurred." ) 1675 protected Reference location; 1676 1677 /** 1678 * The actual object that is the target of the reference (The service delivery location where the vaccine administration occurred.) 1679 */ 1680 protected Location locationTarget; 1681 1682 /** 1683 * Name of vaccine manufacturer. 1684 */ 1685 @Child(name = "manufacturer", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 1686 @Description(shortDefinition="Vaccine manufacturer", formalDefinition="Name of vaccine manufacturer." ) 1687 protected Reference manufacturer; 1688 1689 /** 1690 * The actual object that is the target of the reference (Name of vaccine manufacturer.) 1691 */ 1692 protected Organization manufacturerTarget; 1693 1694 /** 1695 * Lot number of the vaccine product. 1696 */ 1697 @Child(name = "lotNumber", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1698 @Description(shortDefinition="Vaccine lot number", formalDefinition="Lot number of the vaccine product." ) 1699 protected StringType lotNumber; 1700 1701 /** 1702 * Date vaccine batch expires. 1703 */ 1704 @Child(name = "expirationDate", type = {DateType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1705 @Description(shortDefinition="Vaccine expiration date", formalDefinition="Date vaccine batch expires." ) 1706 protected DateType expirationDate; 1707 1708 /** 1709 * Body site where vaccine was administered. 1710 */ 1711 @Child(name = "site", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 1712 @Description(shortDefinition="Body site vaccine was administered", formalDefinition="Body site where vaccine was administered." ) 1713 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-site") 1714 protected CodeableConcept site; 1715 1716 /** 1717 * The path by which the vaccine product is taken into the body. 1718 */ 1719 @Child(name = "route", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 1720 @Description(shortDefinition="How vaccine entered body", formalDefinition="The path by which the vaccine product is taken into the body." ) 1721 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-route") 1722 protected CodeableConcept route; 1723 1724 /** 1725 * The quantity of vaccine product that was administered. 1726 */ 1727 @Child(name = "doseQuantity", type = {SimpleQuantity.class}, order=15, min=0, max=1, modifier=false, summary=false) 1728 @Description(shortDefinition="Amount of vaccine administered", formalDefinition="The quantity of vaccine product that was administered." ) 1729 protected SimpleQuantity doseQuantity; 1730 1731 /** 1732 * Indicates who or what performed the event. 1733 */ 1734 @Child(name = "practitioner", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1735 @Description(shortDefinition="Who performed event", formalDefinition="Indicates who or what performed the event." ) 1736 protected List<ImmunizationPractitionerComponent> practitioner; 1737 1738 /** 1739 * Extra information about the immunization that is not conveyed by the other attributes. 1740 */ 1741 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1742 @Description(shortDefinition="Vaccination notes", formalDefinition="Extra information about the immunization that is not conveyed by the other attributes." ) 1743 protected List<Annotation> note; 1744 1745 /** 1746 * Reasons why a vaccine was or was not administered. 1747 */ 1748 @Child(name = "explanation", type = {}, order=18, min=0, max=1, modifier=false, summary=false) 1749 @Description(shortDefinition="Administration/non-administration reasons", formalDefinition="Reasons why a vaccine was or was not administered." ) 1750 protected ImmunizationExplanationComponent explanation; 1751 1752 /** 1753 * Categorical data indicating that an adverse event is associated in time to an immunization. 1754 */ 1755 @Child(name = "reaction", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1756 @Description(shortDefinition="Details of a reaction that follows immunization", formalDefinition="Categorical data indicating that an adverse event is associated in time to an immunization." ) 1757 protected List<ImmunizationReactionComponent> reaction; 1758 1759 /** 1760 * Contains information about the protocol(s) under which the vaccine was administered. 1761 */ 1762 @Child(name = "vaccinationProtocol", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1763 @Description(shortDefinition="What protocol was followed", formalDefinition="Contains information about the protocol(s) under which the vaccine was administered." ) 1764 protected List<ImmunizationVaccinationProtocolComponent> vaccinationProtocol; 1765 1766 private static final long serialVersionUID = 658058655L; 1767 1768 /** 1769 * Constructor 1770 */ 1771 public Immunization() { 1772 super(); 1773 } 1774 1775 /** 1776 * Constructor 1777 */ 1778 public Immunization(Enumeration<ImmunizationStatus> status, BooleanType notGiven, CodeableConcept vaccineCode, Reference patient, BooleanType primarySource) { 1779 super(); 1780 this.status = status; 1781 this.notGiven = notGiven; 1782 this.vaccineCode = vaccineCode; 1783 this.patient = patient; 1784 this.primarySource = primarySource; 1785 } 1786 1787 /** 1788 * @return {@link #identifier} (A unique identifier assigned to this immunization record.) 1789 */ 1790 public List<Identifier> getIdentifier() { 1791 if (this.identifier == null) 1792 this.identifier = new ArrayList<Identifier>(); 1793 return this.identifier; 1794 } 1795 1796 /** 1797 * @return Returns a reference to <code>this</code> for easy method chaining 1798 */ 1799 public Immunization setIdentifier(List<Identifier> theIdentifier) { 1800 this.identifier = theIdentifier; 1801 return this; 1802 } 1803 1804 public boolean hasIdentifier() { 1805 if (this.identifier == null) 1806 return false; 1807 for (Identifier item : this.identifier) 1808 if (!item.isEmpty()) 1809 return true; 1810 return false; 1811 } 1812 1813 public Identifier addIdentifier() { //3 1814 Identifier t = new Identifier(); 1815 if (this.identifier == null) 1816 this.identifier = new ArrayList<Identifier>(); 1817 this.identifier.add(t); 1818 return t; 1819 } 1820 1821 public Immunization addIdentifier(Identifier t) { //3 1822 if (t == null) 1823 return this; 1824 if (this.identifier == null) 1825 this.identifier = new ArrayList<Identifier>(); 1826 this.identifier.add(t); 1827 return this; 1828 } 1829 1830 /** 1831 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1832 */ 1833 public Identifier getIdentifierFirstRep() { 1834 if (getIdentifier().isEmpty()) { 1835 addIdentifier(); 1836 } 1837 return getIdentifier().get(0); 1838 } 1839 1840 /** 1841 * @return {@link #status} (Indicates the current status of the vaccination event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1842 */ 1843 public Enumeration<ImmunizationStatus> getStatusElement() { 1844 if (this.status == null) 1845 if (Configuration.errorOnAutoCreate()) 1846 throw new Error("Attempt to auto-create Immunization.status"); 1847 else if (Configuration.doAutoCreate()) 1848 this.status = new Enumeration<ImmunizationStatus>(new ImmunizationStatusEnumFactory()); // bb 1849 return this.status; 1850 } 1851 1852 public boolean hasStatusElement() { 1853 return this.status != null && !this.status.isEmpty(); 1854 } 1855 1856 public boolean hasStatus() { 1857 return this.status != null && !this.status.isEmpty(); 1858 } 1859 1860 /** 1861 * @param value {@link #status} (Indicates the current status of the vaccination event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1862 */ 1863 public Immunization setStatusElement(Enumeration<ImmunizationStatus> value) { 1864 this.status = value; 1865 return this; 1866 } 1867 1868 /** 1869 * @return Indicates the current status of the vaccination event. 1870 */ 1871 public ImmunizationStatus getStatus() { 1872 return this.status == null ? null : this.status.getValue(); 1873 } 1874 1875 /** 1876 * @param value Indicates the current status of the vaccination event. 1877 */ 1878 public Immunization setStatus(ImmunizationStatus value) { 1879 if (this.status == null) 1880 this.status = new Enumeration<ImmunizationStatus>(new ImmunizationStatusEnumFactory()); 1881 this.status.setValue(value); 1882 return this; 1883 } 1884 1885 /** 1886 * @return {@link #notGiven} (Indicates if the vaccination was or was not given.). This is the underlying object with id, value and extensions. The accessor "getNotGiven" gives direct access to the value 1887 */ 1888 public BooleanType getNotGivenElement() { 1889 if (this.notGiven == null) 1890 if (Configuration.errorOnAutoCreate()) 1891 throw new Error("Attempt to auto-create Immunization.notGiven"); 1892 else if (Configuration.doAutoCreate()) 1893 this.notGiven = new BooleanType(); // bb 1894 return this.notGiven; 1895 } 1896 1897 public boolean hasNotGivenElement() { 1898 return this.notGiven != null && !this.notGiven.isEmpty(); 1899 } 1900 1901 public boolean hasNotGiven() { 1902 return this.notGiven != null && !this.notGiven.isEmpty(); 1903 } 1904 1905 /** 1906 * @param value {@link #notGiven} (Indicates if the vaccination was or was not given.). This is the underlying object with id, value and extensions. The accessor "getNotGiven" gives direct access to the value 1907 */ 1908 public Immunization setNotGivenElement(BooleanType value) { 1909 this.notGiven = value; 1910 return this; 1911 } 1912 1913 /** 1914 * @return Indicates if the vaccination was or was not given. 1915 */ 1916 public boolean getNotGiven() { 1917 return this.notGiven == null || this.notGiven.isEmpty() ? false : this.notGiven.getValue(); 1918 } 1919 1920 /** 1921 * @param value Indicates if the vaccination was or was not given. 1922 */ 1923 public Immunization setNotGiven(boolean value) { 1924 if (this.notGiven == null) 1925 this.notGiven = new BooleanType(); 1926 this.notGiven.setValue(value); 1927 return this; 1928 } 1929 1930 /** 1931 * @return {@link #vaccineCode} (Vaccine that was administered or was to be administered.) 1932 */ 1933 public CodeableConcept getVaccineCode() { 1934 if (this.vaccineCode == null) 1935 if (Configuration.errorOnAutoCreate()) 1936 throw new Error("Attempt to auto-create Immunization.vaccineCode"); 1937 else if (Configuration.doAutoCreate()) 1938 this.vaccineCode = new CodeableConcept(); // cc 1939 return this.vaccineCode; 1940 } 1941 1942 public boolean hasVaccineCode() { 1943 return this.vaccineCode != null && !this.vaccineCode.isEmpty(); 1944 } 1945 1946 /** 1947 * @param value {@link #vaccineCode} (Vaccine that was administered or was to be administered.) 1948 */ 1949 public Immunization setVaccineCode(CodeableConcept value) { 1950 this.vaccineCode = value; 1951 return this; 1952 } 1953 1954 /** 1955 * @return {@link #patient} (The patient who either received or did not receive the immunization.) 1956 */ 1957 public Reference getPatient() { 1958 if (this.patient == null) 1959 if (Configuration.errorOnAutoCreate()) 1960 throw new Error("Attempt to auto-create Immunization.patient"); 1961 else if (Configuration.doAutoCreate()) 1962 this.patient = new Reference(); // cc 1963 return this.patient; 1964 } 1965 1966 public boolean hasPatient() { 1967 return this.patient != null && !this.patient.isEmpty(); 1968 } 1969 1970 /** 1971 * @param value {@link #patient} (The patient who either received or did not receive the immunization.) 1972 */ 1973 public Immunization setPatient(Reference value) { 1974 this.patient = value; 1975 return this; 1976 } 1977 1978 /** 1979 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient who either received or did not receive the immunization.) 1980 */ 1981 public Patient getPatientTarget() { 1982 if (this.patientTarget == null) 1983 if (Configuration.errorOnAutoCreate()) 1984 throw new Error("Attempt to auto-create Immunization.patient"); 1985 else if (Configuration.doAutoCreate()) 1986 this.patientTarget = new Patient(); // aa 1987 return this.patientTarget; 1988 } 1989 1990 /** 1991 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient who either received or did not receive the immunization.) 1992 */ 1993 public Immunization setPatientTarget(Patient value) { 1994 this.patientTarget = value; 1995 return this; 1996 } 1997 1998 /** 1999 * @return {@link #encounter} (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 2000 */ 2001 public Reference getEncounter() { 2002 if (this.encounter == null) 2003 if (Configuration.errorOnAutoCreate()) 2004 throw new Error("Attempt to auto-create Immunization.encounter"); 2005 else if (Configuration.doAutoCreate()) 2006 this.encounter = new Reference(); // cc 2007 return this.encounter; 2008 } 2009 2010 public boolean hasEncounter() { 2011 return this.encounter != null && !this.encounter.isEmpty(); 2012 } 2013 2014 /** 2015 * @param value {@link #encounter} (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 2016 */ 2017 public Immunization setEncounter(Reference value) { 2018 this.encounter = value; 2019 return this; 2020 } 2021 2022 /** 2023 * @return {@link #encounter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 2024 */ 2025 public Encounter getEncounterTarget() { 2026 if (this.encounterTarget == null) 2027 if (Configuration.errorOnAutoCreate()) 2028 throw new Error("Attempt to auto-create Immunization.encounter"); 2029 else if (Configuration.doAutoCreate()) 2030 this.encounterTarget = new Encounter(); // aa 2031 return this.encounterTarget; 2032 } 2033 2034 /** 2035 * @param value {@link #encounter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 2036 */ 2037 public Immunization setEncounterTarget(Encounter value) { 2038 this.encounterTarget = value; 2039 return this; 2040 } 2041 2042 /** 2043 * @return {@link #date} (Date vaccine administered or was to be administered.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2044 */ 2045 public DateTimeType getDateElement() { 2046 if (this.date == null) 2047 if (Configuration.errorOnAutoCreate()) 2048 throw new Error("Attempt to auto-create Immunization.date"); 2049 else if (Configuration.doAutoCreate()) 2050 this.date = new DateTimeType(); // bb 2051 return this.date; 2052 } 2053 2054 public boolean hasDateElement() { 2055 return this.date != null && !this.date.isEmpty(); 2056 } 2057 2058 public boolean hasDate() { 2059 return this.date != null && !this.date.isEmpty(); 2060 } 2061 2062 /** 2063 * @param value {@link #date} (Date vaccine administered or was to be administered.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2064 */ 2065 public Immunization setDateElement(DateTimeType value) { 2066 this.date = value; 2067 return this; 2068 } 2069 2070 /** 2071 * @return Date vaccine administered or was to be administered. 2072 */ 2073 public Date getDate() { 2074 return this.date == null ? null : this.date.getValue(); 2075 } 2076 2077 /** 2078 * @param value Date vaccine administered or was to be administered. 2079 */ 2080 public Immunization setDate(Date value) { 2081 if (value == null) 2082 this.date = null; 2083 else { 2084 if (this.date == null) 2085 this.date = new DateTimeType(); 2086 this.date.setValue(value); 2087 } 2088 return this; 2089 } 2090 2091 /** 2092 * @return {@link #primarySource} (An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.). This is the underlying object with id, value and extensions. The accessor "getPrimarySource" gives direct access to the value 2093 */ 2094 public BooleanType getPrimarySourceElement() { 2095 if (this.primarySource == null) 2096 if (Configuration.errorOnAutoCreate()) 2097 throw new Error("Attempt to auto-create Immunization.primarySource"); 2098 else if (Configuration.doAutoCreate()) 2099 this.primarySource = new BooleanType(); // bb 2100 return this.primarySource; 2101 } 2102 2103 public boolean hasPrimarySourceElement() { 2104 return this.primarySource != null && !this.primarySource.isEmpty(); 2105 } 2106 2107 public boolean hasPrimarySource() { 2108 return this.primarySource != null && !this.primarySource.isEmpty(); 2109 } 2110 2111 /** 2112 * @param value {@link #primarySource} (An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.). This is the underlying object with id, value and extensions. The accessor "getPrimarySource" gives direct access to the value 2113 */ 2114 public Immunization setPrimarySourceElement(BooleanType value) { 2115 this.primarySource = value; 2116 return this; 2117 } 2118 2119 /** 2120 * @return An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded. 2121 */ 2122 public boolean getPrimarySource() { 2123 return this.primarySource == null || this.primarySource.isEmpty() ? false : this.primarySource.getValue(); 2124 } 2125 2126 /** 2127 * @param value An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded. 2128 */ 2129 public Immunization setPrimarySource(boolean value) { 2130 if (this.primarySource == null) 2131 this.primarySource = new BooleanType(); 2132 this.primarySource.setValue(value); 2133 return this; 2134 } 2135 2136 /** 2137 * @return {@link #reportOrigin} (The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.) 2138 */ 2139 public CodeableConcept getReportOrigin() { 2140 if (this.reportOrigin == null) 2141 if (Configuration.errorOnAutoCreate()) 2142 throw new Error("Attempt to auto-create Immunization.reportOrigin"); 2143 else if (Configuration.doAutoCreate()) 2144 this.reportOrigin = new CodeableConcept(); // cc 2145 return this.reportOrigin; 2146 } 2147 2148 public boolean hasReportOrigin() { 2149 return this.reportOrigin != null && !this.reportOrigin.isEmpty(); 2150 } 2151 2152 /** 2153 * @param value {@link #reportOrigin} (The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.) 2154 */ 2155 public Immunization setReportOrigin(CodeableConcept value) { 2156 this.reportOrigin = value; 2157 return this; 2158 } 2159 2160 /** 2161 * @return {@link #location} (The service delivery location where the vaccine administration occurred.) 2162 */ 2163 public Reference getLocation() { 2164 if (this.location == null) 2165 if (Configuration.errorOnAutoCreate()) 2166 throw new Error("Attempt to auto-create Immunization.location"); 2167 else if (Configuration.doAutoCreate()) 2168 this.location = new Reference(); // cc 2169 return this.location; 2170 } 2171 2172 public boolean hasLocation() { 2173 return this.location != null && !this.location.isEmpty(); 2174 } 2175 2176 /** 2177 * @param value {@link #location} (The service delivery location where the vaccine administration occurred.) 2178 */ 2179 public Immunization setLocation(Reference value) { 2180 this.location = value; 2181 return this; 2182 } 2183 2184 /** 2185 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The service delivery location where the vaccine administration occurred.) 2186 */ 2187 public Location getLocationTarget() { 2188 if (this.locationTarget == null) 2189 if (Configuration.errorOnAutoCreate()) 2190 throw new Error("Attempt to auto-create Immunization.location"); 2191 else if (Configuration.doAutoCreate()) 2192 this.locationTarget = new Location(); // aa 2193 return this.locationTarget; 2194 } 2195 2196 /** 2197 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The service delivery location where the vaccine administration occurred.) 2198 */ 2199 public Immunization setLocationTarget(Location value) { 2200 this.locationTarget = value; 2201 return this; 2202 } 2203 2204 /** 2205 * @return {@link #manufacturer} (Name of vaccine manufacturer.) 2206 */ 2207 public Reference getManufacturer() { 2208 if (this.manufacturer == null) 2209 if (Configuration.errorOnAutoCreate()) 2210 throw new Error("Attempt to auto-create Immunization.manufacturer"); 2211 else if (Configuration.doAutoCreate()) 2212 this.manufacturer = new Reference(); // cc 2213 return this.manufacturer; 2214 } 2215 2216 public boolean hasManufacturer() { 2217 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2218 } 2219 2220 /** 2221 * @param value {@link #manufacturer} (Name of vaccine manufacturer.) 2222 */ 2223 public Immunization setManufacturer(Reference value) { 2224 this.manufacturer = value; 2225 return this; 2226 } 2227 2228 /** 2229 * @return {@link #manufacturer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Name of vaccine manufacturer.) 2230 */ 2231 public Organization getManufacturerTarget() { 2232 if (this.manufacturerTarget == null) 2233 if (Configuration.errorOnAutoCreate()) 2234 throw new Error("Attempt to auto-create Immunization.manufacturer"); 2235 else if (Configuration.doAutoCreate()) 2236 this.manufacturerTarget = new Organization(); // aa 2237 return this.manufacturerTarget; 2238 } 2239 2240 /** 2241 * @param value {@link #manufacturer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Name of vaccine manufacturer.) 2242 */ 2243 public Immunization setManufacturerTarget(Organization value) { 2244 this.manufacturerTarget = value; 2245 return this; 2246 } 2247 2248 /** 2249 * @return {@link #lotNumber} (Lot number of the vaccine product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 2250 */ 2251 public StringType getLotNumberElement() { 2252 if (this.lotNumber == null) 2253 if (Configuration.errorOnAutoCreate()) 2254 throw new Error("Attempt to auto-create Immunization.lotNumber"); 2255 else if (Configuration.doAutoCreate()) 2256 this.lotNumber = new StringType(); // bb 2257 return this.lotNumber; 2258 } 2259 2260 public boolean hasLotNumberElement() { 2261 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2262 } 2263 2264 public boolean hasLotNumber() { 2265 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2266 } 2267 2268 /** 2269 * @param value {@link #lotNumber} (Lot number of the vaccine product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 2270 */ 2271 public Immunization setLotNumberElement(StringType value) { 2272 this.lotNumber = value; 2273 return this; 2274 } 2275 2276 /** 2277 * @return Lot number of the vaccine product. 2278 */ 2279 public String getLotNumber() { 2280 return this.lotNumber == null ? null : this.lotNumber.getValue(); 2281 } 2282 2283 /** 2284 * @param value Lot number of the vaccine product. 2285 */ 2286 public Immunization setLotNumber(String value) { 2287 if (Utilities.noString(value)) 2288 this.lotNumber = null; 2289 else { 2290 if (this.lotNumber == null) 2291 this.lotNumber = new StringType(); 2292 this.lotNumber.setValue(value); 2293 } 2294 return this; 2295 } 2296 2297 /** 2298 * @return {@link #expirationDate} (Date vaccine batch expires.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2299 */ 2300 public DateType getExpirationDateElement() { 2301 if (this.expirationDate == null) 2302 if (Configuration.errorOnAutoCreate()) 2303 throw new Error("Attempt to auto-create Immunization.expirationDate"); 2304 else if (Configuration.doAutoCreate()) 2305 this.expirationDate = new DateType(); // bb 2306 return this.expirationDate; 2307 } 2308 2309 public boolean hasExpirationDateElement() { 2310 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2311 } 2312 2313 public boolean hasExpirationDate() { 2314 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2315 } 2316 2317 /** 2318 * @param value {@link #expirationDate} (Date vaccine batch expires.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2319 */ 2320 public Immunization setExpirationDateElement(DateType value) { 2321 this.expirationDate = value; 2322 return this; 2323 } 2324 2325 /** 2326 * @return Date vaccine batch expires. 2327 */ 2328 public Date getExpirationDate() { 2329 return this.expirationDate == null ? null : this.expirationDate.getValue(); 2330 } 2331 2332 /** 2333 * @param value Date vaccine batch expires. 2334 */ 2335 public Immunization setExpirationDate(Date value) { 2336 if (value == null) 2337 this.expirationDate = null; 2338 else { 2339 if (this.expirationDate == null) 2340 this.expirationDate = new DateType(); 2341 this.expirationDate.setValue(value); 2342 } 2343 return this; 2344 } 2345 2346 /** 2347 * @return {@link #site} (Body site where vaccine was administered.) 2348 */ 2349 public CodeableConcept getSite() { 2350 if (this.site == null) 2351 if (Configuration.errorOnAutoCreate()) 2352 throw new Error("Attempt to auto-create Immunization.site"); 2353 else if (Configuration.doAutoCreate()) 2354 this.site = new CodeableConcept(); // cc 2355 return this.site; 2356 } 2357 2358 public boolean hasSite() { 2359 return this.site != null && !this.site.isEmpty(); 2360 } 2361 2362 /** 2363 * @param value {@link #site} (Body site where vaccine was administered.) 2364 */ 2365 public Immunization setSite(CodeableConcept value) { 2366 this.site = value; 2367 return this; 2368 } 2369 2370 /** 2371 * @return {@link #route} (The path by which the vaccine product is taken into the body.) 2372 */ 2373 public CodeableConcept getRoute() { 2374 if (this.route == null) 2375 if (Configuration.errorOnAutoCreate()) 2376 throw new Error("Attempt to auto-create Immunization.route"); 2377 else if (Configuration.doAutoCreate()) 2378 this.route = new CodeableConcept(); // cc 2379 return this.route; 2380 } 2381 2382 public boolean hasRoute() { 2383 return this.route != null && !this.route.isEmpty(); 2384 } 2385 2386 /** 2387 * @param value {@link #route} (The path by which the vaccine product is taken into the body.) 2388 */ 2389 public Immunization setRoute(CodeableConcept value) { 2390 this.route = value; 2391 return this; 2392 } 2393 2394 /** 2395 * @return {@link #doseQuantity} (The quantity of vaccine product that was administered.) 2396 */ 2397 public SimpleQuantity getDoseQuantity() { 2398 if (this.doseQuantity == null) 2399 if (Configuration.errorOnAutoCreate()) 2400 throw new Error("Attempt to auto-create Immunization.doseQuantity"); 2401 else if (Configuration.doAutoCreate()) 2402 this.doseQuantity = new SimpleQuantity(); // cc 2403 return this.doseQuantity; 2404 } 2405 2406 public boolean hasDoseQuantity() { 2407 return this.doseQuantity != null && !this.doseQuantity.isEmpty(); 2408 } 2409 2410 /** 2411 * @param value {@link #doseQuantity} (The quantity of vaccine product that was administered.) 2412 */ 2413 public Immunization setDoseQuantity(SimpleQuantity value) { 2414 this.doseQuantity = value; 2415 return this; 2416 } 2417 2418 /** 2419 * @return {@link #practitioner} (Indicates who or what performed the event.) 2420 */ 2421 public List<ImmunizationPractitionerComponent> getPractitioner() { 2422 if (this.practitioner == null) 2423 this.practitioner = new ArrayList<ImmunizationPractitionerComponent>(); 2424 return this.practitioner; 2425 } 2426 2427 /** 2428 * @return Returns a reference to <code>this</code> for easy method chaining 2429 */ 2430 public Immunization setPractitioner(List<ImmunizationPractitionerComponent> thePractitioner) { 2431 this.practitioner = thePractitioner; 2432 return this; 2433 } 2434 2435 public boolean hasPractitioner() { 2436 if (this.practitioner == null) 2437 return false; 2438 for (ImmunizationPractitionerComponent item : this.practitioner) 2439 if (!item.isEmpty()) 2440 return true; 2441 return false; 2442 } 2443 2444 public ImmunizationPractitionerComponent addPractitioner() { //3 2445 ImmunizationPractitionerComponent t = new ImmunizationPractitionerComponent(); 2446 if (this.practitioner == null) 2447 this.practitioner = new ArrayList<ImmunizationPractitionerComponent>(); 2448 this.practitioner.add(t); 2449 return t; 2450 } 2451 2452 public Immunization addPractitioner(ImmunizationPractitionerComponent t) { //3 2453 if (t == null) 2454 return this; 2455 if (this.practitioner == null) 2456 this.practitioner = new ArrayList<ImmunizationPractitionerComponent>(); 2457 this.practitioner.add(t); 2458 return this; 2459 } 2460 2461 /** 2462 * @return The first repetition of repeating field {@link #practitioner}, creating it if it does not already exist 2463 */ 2464 public ImmunizationPractitionerComponent getPractitionerFirstRep() { 2465 if (getPractitioner().isEmpty()) { 2466 addPractitioner(); 2467 } 2468 return getPractitioner().get(0); 2469 } 2470 2471 /** 2472 * @return {@link #note} (Extra information about the immunization that is not conveyed by the other attributes.) 2473 */ 2474 public List<Annotation> getNote() { 2475 if (this.note == null) 2476 this.note = new ArrayList<Annotation>(); 2477 return this.note; 2478 } 2479 2480 /** 2481 * @return Returns a reference to <code>this</code> for easy method chaining 2482 */ 2483 public Immunization setNote(List<Annotation> theNote) { 2484 this.note = theNote; 2485 return this; 2486 } 2487 2488 public boolean hasNote() { 2489 if (this.note == null) 2490 return false; 2491 for (Annotation item : this.note) 2492 if (!item.isEmpty()) 2493 return true; 2494 return false; 2495 } 2496 2497 public Annotation addNote() { //3 2498 Annotation t = new Annotation(); 2499 if (this.note == null) 2500 this.note = new ArrayList<Annotation>(); 2501 this.note.add(t); 2502 return t; 2503 } 2504 2505 public Immunization addNote(Annotation t) { //3 2506 if (t == null) 2507 return this; 2508 if (this.note == null) 2509 this.note = new ArrayList<Annotation>(); 2510 this.note.add(t); 2511 return this; 2512 } 2513 2514 /** 2515 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2516 */ 2517 public Annotation getNoteFirstRep() { 2518 if (getNote().isEmpty()) { 2519 addNote(); 2520 } 2521 return getNote().get(0); 2522 } 2523 2524 /** 2525 * @return {@link #explanation} (Reasons why a vaccine was or was not administered.) 2526 */ 2527 public ImmunizationExplanationComponent getExplanation() { 2528 if (this.explanation == null) 2529 if (Configuration.errorOnAutoCreate()) 2530 throw new Error("Attempt to auto-create Immunization.explanation"); 2531 else if (Configuration.doAutoCreate()) 2532 this.explanation = new ImmunizationExplanationComponent(); // cc 2533 return this.explanation; 2534 } 2535 2536 public boolean hasExplanation() { 2537 return this.explanation != null && !this.explanation.isEmpty(); 2538 } 2539 2540 /** 2541 * @param value {@link #explanation} (Reasons why a vaccine was or was not administered.) 2542 */ 2543 public Immunization setExplanation(ImmunizationExplanationComponent value) { 2544 this.explanation = value; 2545 return this; 2546 } 2547 2548 /** 2549 * @return {@link #reaction} (Categorical data indicating that an adverse event is associated in time to an immunization.) 2550 */ 2551 public List<ImmunizationReactionComponent> getReaction() { 2552 if (this.reaction == null) 2553 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2554 return this.reaction; 2555 } 2556 2557 /** 2558 * @return Returns a reference to <code>this</code> for easy method chaining 2559 */ 2560 public Immunization setReaction(List<ImmunizationReactionComponent> theReaction) { 2561 this.reaction = theReaction; 2562 return this; 2563 } 2564 2565 public boolean hasReaction() { 2566 if (this.reaction == null) 2567 return false; 2568 for (ImmunizationReactionComponent item : this.reaction) 2569 if (!item.isEmpty()) 2570 return true; 2571 return false; 2572 } 2573 2574 public ImmunizationReactionComponent addReaction() { //3 2575 ImmunizationReactionComponent t = new ImmunizationReactionComponent(); 2576 if (this.reaction == null) 2577 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2578 this.reaction.add(t); 2579 return t; 2580 } 2581 2582 public Immunization addReaction(ImmunizationReactionComponent t) { //3 2583 if (t == null) 2584 return this; 2585 if (this.reaction == null) 2586 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2587 this.reaction.add(t); 2588 return this; 2589 } 2590 2591 /** 2592 * @return The first repetition of repeating field {@link #reaction}, creating it if it does not already exist 2593 */ 2594 public ImmunizationReactionComponent getReactionFirstRep() { 2595 if (getReaction().isEmpty()) { 2596 addReaction(); 2597 } 2598 return getReaction().get(0); 2599 } 2600 2601 /** 2602 * @return {@link #vaccinationProtocol} (Contains information about the protocol(s) under which the vaccine was administered.) 2603 */ 2604 public List<ImmunizationVaccinationProtocolComponent> getVaccinationProtocol() { 2605 if (this.vaccinationProtocol == null) 2606 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2607 return this.vaccinationProtocol; 2608 } 2609 2610 /** 2611 * @return Returns a reference to <code>this</code> for easy method chaining 2612 */ 2613 public Immunization setVaccinationProtocol(List<ImmunizationVaccinationProtocolComponent> theVaccinationProtocol) { 2614 this.vaccinationProtocol = theVaccinationProtocol; 2615 return this; 2616 } 2617 2618 public boolean hasVaccinationProtocol() { 2619 if (this.vaccinationProtocol == null) 2620 return false; 2621 for (ImmunizationVaccinationProtocolComponent item : this.vaccinationProtocol) 2622 if (!item.isEmpty()) 2623 return true; 2624 return false; 2625 } 2626 2627 public ImmunizationVaccinationProtocolComponent addVaccinationProtocol() { //3 2628 ImmunizationVaccinationProtocolComponent t = new ImmunizationVaccinationProtocolComponent(); 2629 if (this.vaccinationProtocol == null) 2630 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2631 this.vaccinationProtocol.add(t); 2632 return t; 2633 } 2634 2635 public Immunization addVaccinationProtocol(ImmunizationVaccinationProtocolComponent t) { //3 2636 if (t == null) 2637 return this; 2638 if (this.vaccinationProtocol == null) 2639 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2640 this.vaccinationProtocol.add(t); 2641 return this; 2642 } 2643 2644 /** 2645 * @return The first repetition of repeating field {@link #vaccinationProtocol}, creating it if it does not already exist 2646 */ 2647 public ImmunizationVaccinationProtocolComponent getVaccinationProtocolFirstRep() { 2648 if (getVaccinationProtocol().isEmpty()) { 2649 addVaccinationProtocol(); 2650 } 2651 return getVaccinationProtocol().get(0); 2652 } 2653 2654 protected void listChildren(List<Property> children) { 2655 super.listChildren(children); 2656 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2657 children.add(new Property("status", "code", "Indicates the current status of the vaccination event.", 0, 1, status)); 2658 children.add(new Property("notGiven", "boolean", "Indicates if the vaccination was or was not given.", 0, 1, notGiven)); 2659 children.add(new Property("vaccineCode", "CodeableConcept", "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode)); 2660 children.add(new Property("patient", "Reference(Patient)", "The patient who either received or did not receive the immunization.", 0, 1, patient)); 2661 children.add(new Property("encounter", "Reference(Encounter)", "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 0, 1, encounter)); 2662 children.add(new Property("date", "dateTime", "Date vaccine administered or was to be administered.", 0, 1, date)); 2663 children.add(new Property("primarySource", "boolean", "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.", 0, 1, primarySource)); 2664 children.add(new Property("reportOrigin", "CodeableConcept", "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 0, 1, reportOrigin)); 2665 children.add(new Property("location", "Reference(Location)", "The service delivery location where the vaccine administration occurred.", 0, 1, location)); 2666 children.add(new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 0, 1, manufacturer)); 2667 children.add(new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, lotNumber)); 2668 children.add(new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, expirationDate)); 2669 children.add(new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, site)); 2670 children.add(new Property("route", "CodeableConcept", "The path by which the vaccine product is taken into the body.", 0, 1, route)); 2671 children.add(new Property("doseQuantity", "SimpleQuantity", "The quantity of vaccine product that was administered.", 0, 1, doseQuantity)); 2672 children.add(new Property("practitioner", "", "Indicates who or what performed the event.", 0, java.lang.Integer.MAX_VALUE, practitioner)); 2673 children.add(new Property("note", "Annotation", "Extra information about the immunization that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2674 children.add(new Property("explanation", "", "Reasons why a vaccine was or was not administered.", 0, 1, explanation)); 2675 children.add(new Property("reaction", "", "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, java.lang.Integer.MAX_VALUE, reaction)); 2676 children.add(new Property("vaccinationProtocol", "", "Contains information about the protocol(s) under which the vaccine was administered.", 0, java.lang.Integer.MAX_VALUE, vaccinationProtocol)); 2677 } 2678 2679 @Override 2680 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2681 switch (_hash) { 2682 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier); 2683 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current status of the vaccination event.", 0, 1, status); 2684 case 1554065514: /*notGiven*/ return new Property("notGiven", "boolean", "Indicates if the vaccination was or was not given.", 0, 1, notGiven); 2685 case 664556354: /*vaccineCode*/ return new Property("vaccineCode", "CodeableConcept", "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode); 2686 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient who either received or did not receive the immunization.", 0, 1, patient); 2687 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 0, 1, encounter); 2688 case 3076014: /*date*/ return new Property("date", "dateTime", "Date vaccine administered or was to be administered.", 0, 1, date); 2689 case -528721731: /*primarySource*/ return new Property("primarySource", "boolean", "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.", 0, 1, primarySource); 2690 case 486750586: /*reportOrigin*/ return new Property("reportOrigin", "CodeableConcept", "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 0, 1, reportOrigin); 2691 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The service delivery location where the vaccine administration occurred.", 0, 1, location); 2692 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 0, 1, manufacturer); 2693 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, lotNumber); 2694 case -668811523: /*expirationDate*/ return new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, expirationDate); 2695 case 3530567: /*site*/ return new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, site); 2696 case 108704329: /*route*/ return new Property("route", "CodeableConcept", "The path by which the vaccine product is taken into the body.", 0, 1, route); 2697 case -2083618872: /*doseQuantity*/ return new Property("doseQuantity", "SimpleQuantity", "The quantity of vaccine product that was administered.", 0, 1, doseQuantity); 2698 case 574573338: /*practitioner*/ return new Property("practitioner", "", "Indicates who or what performed the event.", 0, java.lang.Integer.MAX_VALUE, practitioner); 2699 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the immunization that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2700 case -1105867239: /*explanation*/ return new Property("explanation", "", "Reasons why a vaccine was or was not administered.", 0, 1, explanation); 2701 case -867509719: /*reaction*/ return new Property("reaction", "", "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, java.lang.Integer.MAX_VALUE, reaction); 2702 case -179633155: /*vaccinationProtocol*/ return new Property("vaccinationProtocol", "", "Contains information about the protocol(s) under which the vaccine was administered.", 0, java.lang.Integer.MAX_VALUE, vaccinationProtocol); 2703 default: return super.getNamedProperty(_hash, _name, _checkValid); 2704 } 2705 2706 } 2707 2708 @Override 2709 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2710 switch (hash) { 2711 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2712 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ImmunizationStatus> 2713 case 1554065514: /*notGiven*/ return this.notGiven == null ? new Base[0] : new Base[] {this.notGiven}; // BooleanType 2714 case 664556354: /*vaccineCode*/ return this.vaccineCode == null ? new Base[0] : new Base[] {this.vaccineCode}; // CodeableConcept 2715 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2716 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2717 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2718 case -528721731: /*primarySource*/ return this.primarySource == null ? new Base[0] : new Base[] {this.primarySource}; // BooleanType 2719 case 486750586: /*reportOrigin*/ return this.reportOrigin == null ? new Base[0] : new Base[] {this.reportOrigin}; // CodeableConcept 2720 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2721 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // Reference 2722 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 2723 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateType 2724 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // CodeableConcept 2725 case 108704329: /*route*/ return this.route == null ? new Base[0] : new Base[] {this.route}; // CodeableConcept 2726 case -2083618872: /*doseQuantity*/ return this.doseQuantity == null ? new Base[0] : new Base[] {this.doseQuantity}; // SimpleQuantity 2727 case 574573338: /*practitioner*/ return this.practitioner == null ? new Base[0] : this.practitioner.toArray(new Base[this.practitioner.size()]); // ImmunizationPractitionerComponent 2728 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2729 case -1105867239: /*explanation*/ return this.explanation == null ? new Base[0] : new Base[] {this.explanation}; // ImmunizationExplanationComponent 2730 case -867509719: /*reaction*/ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // ImmunizationReactionComponent 2731 case -179633155: /*vaccinationProtocol*/ return this.vaccinationProtocol == null ? new Base[0] : this.vaccinationProtocol.toArray(new Base[this.vaccinationProtocol.size()]); // ImmunizationVaccinationProtocolComponent 2732 default: return super.getProperty(hash, name, checkValid); 2733 } 2734 2735 } 2736 2737 @Override 2738 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2739 switch (hash) { 2740 case -1618432855: // identifier 2741 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2742 return value; 2743 case -892481550: // status 2744 value = new ImmunizationStatusEnumFactory().fromType(castToCode(value)); 2745 this.status = (Enumeration) value; // Enumeration<ImmunizationStatus> 2746 return value; 2747 case 1554065514: // notGiven 2748 this.notGiven = castToBoolean(value); // BooleanType 2749 return value; 2750 case 664556354: // vaccineCode 2751 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 2752 return value; 2753 case -791418107: // patient 2754 this.patient = castToReference(value); // Reference 2755 return value; 2756 case 1524132147: // encounter 2757 this.encounter = castToReference(value); // Reference 2758 return value; 2759 case 3076014: // date 2760 this.date = castToDateTime(value); // DateTimeType 2761 return value; 2762 case -528721731: // primarySource 2763 this.primarySource = castToBoolean(value); // BooleanType 2764 return value; 2765 case 486750586: // reportOrigin 2766 this.reportOrigin = castToCodeableConcept(value); // CodeableConcept 2767 return value; 2768 case 1901043637: // location 2769 this.location = castToReference(value); // Reference 2770 return value; 2771 case -1969347631: // manufacturer 2772 this.manufacturer = castToReference(value); // Reference 2773 return value; 2774 case 462547450: // lotNumber 2775 this.lotNumber = castToString(value); // StringType 2776 return value; 2777 case -668811523: // expirationDate 2778 this.expirationDate = castToDate(value); // DateType 2779 return value; 2780 case 3530567: // site 2781 this.site = castToCodeableConcept(value); // CodeableConcept 2782 return value; 2783 case 108704329: // route 2784 this.route = castToCodeableConcept(value); // CodeableConcept 2785 return value; 2786 case -2083618872: // doseQuantity 2787 this.doseQuantity = castToSimpleQuantity(value); // SimpleQuantity 2788 return value; 2789 case 574573338: // practitioner 2790 this.getPractitioner().add((ImmunizationPractitionerComponent) value); // ImmunizationPractitionerComponent 2791 return value; 2792 case 3387378: // note 2793 this.getNote().add(castToAnnotation(value)); // Annotation 2794 return value; 2795 case -1105867239: // explanation 2796 this.explanation = (ImmunizationExplanationComponent) value; // ImmunizationExplanationComponent 2797 return value; 2798 case -867509719: // reaction 2799 this.getReaction().add((ImmunizationReactionComponent) value); // ImmunizationReactionComponent 2800 return value; 2801 case -179633155: // vaccinationProtocol 2802 this.getVaccinationProtocol().add((ImmunizationVaccinationProtocolComponent) value); // ImmunizationVaccinationProtocolComponent 2803 return value; 2804 default: return super.setProperty(hash, name, value); 2805 } 2806 2807 } 2808 2809 @Override 2810 public Base setProperty(String name, Base value) throws FHIRException { 2811 if (name.equals("identifier")) { 2812 this.getIdentifier().add(castToIdentifier(value)); 2813 } else if (name.equals("status")) { 2814 value = new ImmunizationStatusEnumFactory().fromType(castToCode(value)); 2815 this.status = (Enumeration) value; // Enumeration<ImmunizationStatus> 2816 } else if (name.equals("notGiven")) { 2817 this.notGiven = castToBoolean(value); // BooleanType 2818 } else if (name.equals("vaccineCode")) { 2819 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 2820 } else if (name.equals("patient")) { 2821 this.patient = castToReference(value); // Reference 2822 } else if (name.equals("encounter")) { 2823 this.encounter = castToReference(value); // Reference 2824 } else if (name.equals("date")) { 2825 this.date = castToDateTime(value); // DateTimeType 2826 } else if (name.equals("primarySource")) { 2827 this.primarySource = castToBoolean(value); // BooleanType 2828 } else if (name.equals("reportOrigin")) { 2829 this.reportOrigin = castToCodeableConcept(value); // CodeableConcept 2830 } else if (name.equals("location")) { 2831 this.location = castToReference(value); // Reference 2832 } else if (name.equals("manufacturer")) { 2833 this.manufacturer = castToReference(value); // Reference 2834 } else if (name.equals("lotNumber")) { 2835 this.lotNumber = castToString(value); // StringType 2836 } else if (name.equals("expirationDate")) { 2837 this.expirationDate = castToDate(value); // DateType 2838 } else if (name.equals("site")) { 2839 this.site = castToCodeableConcept(value); // CodeableConcept 2840 } else if (name.equals("route")) { 2841 this.route = castToCodeableConcept(value); // CodeableConcept 2842 } else if (name.equals("doseQuantity")) { 2843 this.doseQuantity = castToSimpleQuantity(value); // SimpleQuantity 2844 } else if (name.equals("practitioner")) { 2845 this.getPractitioner().add((ImmunizationPractitionerComponent) value); 2846 } else if (name.equals("note")) { 2847 this.getNote().add(castToAnnotation(value)); 2848 } else if (name.equals("explanation")) { 2849 this.explanation = (ImmunizationExplanationComponent) value; // ImmunizationExplanationComponent 2850 } else if (name.equals("reaction")) { 2851 this.getReaction().add((ImmunizationReactionComponent) value); 2852 } else if (name.equals("vaccinationProtocol")) { 2853 this.getVaccinationProtocol().add((ImmunizationVaccinationProtocolComponent) value); 2854 } else 2855 return super.setProperty(name, value); 2856 return value; 2857 } 2858 2859 @Override 2860 public Base makeProperty(int hash, String name) throws FHIRException { 2861 switch (hash) { 2862 case -1618432855: return addIdentifier(); 2863 case -892481550: return getStatusElement(); 2864 case 1554065514: return getNotGivenElement(); 2865 case 664556354: return getVaccineCode(); 2866 case -791418107: return getPatient(); 2867 case 1524132147: return getEncounter(); 2868 case 3076014: return getDateElement(); 2869 case -528721731: return getPrimarySourceElement(); 2870 case 486750586: return getReportOrigin(); 2871 case 1901043637: return getLocation(); 2872 case -1969347631: return getManufacturer(); 2873 case 462547450: return getLotNumberElement(); 2874 case -668811523: return getExpirationDateElement(); 2875 case 3530567: return getSite(); 2876 case 108704329: return getRoute(); 2877 case -2083618872: return getDoseQuantity(); 2878 case 574573338: return addPractitioner(); 2879 case 3387378: return addNote(); 2880 case -1105867239: return getExplanation(); 2881 case -867509719: return addReaction(); 2882 case -179633155: return addVaccinationProtocol(); 2883 default: return super.makeProperty(hash, name); 2884 } 2885 2886 } 2887 2888 @Override 2889 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2890 switch (hash) { 2891 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2892 case -892481550: /*status*/ return new String[] {"code"}; 2893 case 1554065514: /*notGiven*/ return new String[] {"boolean"}; 2894 case 664556354: /*vaccineCode*/ return new String[] {"CodeableConcept"}; 2895 case -791418107: /*patient*/ return new String[] {"Reference"}; 2896 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2897 case 3076014: /*date*/ return new String[] {"dateTime"}; 2898 case -528721731: /*primarySource*/ return new String[] {"boolean"}; 2899 case 486750586: /*reportOrigin*/ return new String[] {"CodeableConcept"}; 2900 case 1901043637: /*location*/ return new String[] {"Reference"}; 2901 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 2902 case 462547450: /*lotNumber*/ return new String[] {"string"}; 2903 case -668811523: /*expirationDate*/ return new String[] {"date"}; 2904 case 3530567: /*site*/ return new String[] {"CodeableConcept"}; 2905 case 108704329: /*route*/ return new String[] {"CodeableConcept"}; 2906 case -2083618872: /*doseQuantity*/ return new String[] {"SimpleQuantity"}; 2907 case 574573338: /*practitioner*/ return new String[] {}; 2908 case 3387378: /*note*/ return new String[] {"Annotation"}; 2909 case -1105867239: /*explanation*/ return new String[] {}; 2910 case -867509719: /*reaction*/ return new String[] {}; 2911 case -179633155: /*vaccinationProtocol*/ return new String[] {}; 2912 default: return super.getTypesForProperty(hash, name); 2913 } 2914 2915 } 2916 2917 @Override 2918 public Base addChild(String name) throws FHIRException { 2919 if (name.equals("identifier")) { 2920 return addIdentifier(); 2921 } 2922 else if (name.equals("status")) { 2923 throw new FHIRException("Cannot call addChild on a singleton property Immunization.status"); 2924 } 2925 else if (name.equals("notGiven")) { 2926 throw new FHIRException("Cannot call addChild on a singleton property Immunization.notGiven"); 2927 } 2928 else if (name.equals("vaccineCode")) { 2929 this.vaccineCode = new CodeableConcept(); 2930 return this.vaccineCode; 2931 } 2932 else if (name.equals("patient")) { 2933 this.patient = new Reference(); 2934 return this.patient; 2935 } 2936 else if (name.equals("encounter")) { 2937 this.encounter = new Reference(); 2938 return this.encounter; 2939 } 2940 else if (name.equals("date")) { 2941 throw new FHIRException("Cannot call addChild on a singleton property Immunization.date"); 2942 } 2943 else if (name.equals("primarySource")) { 2944 throw new FHIRException("Cannot call addChild on a singleton property Immunization.primarySource"); 2945 } 2946 else if (name.equals("reportOrigin")) { 2947 this.reportOrigin = new CodeableConcept(); 2948 return this.reportOrigin; 2949 } 2950 else if (name.equals("location")) { 2951 this.location = new Reference(); 2952 return this.location; 2953 } 2954 else if (name.equals("manufacturer")) { 2955 this.manufacturer = new Reference(); 2956 return this.manufacturer; 2957 } 2958 else if (name.equals("lotNumber")) { 2959 throw new FHIRException("Cannot call addChild on a singleton property Immunization.lotNumber"); 2960 } 2961 else if (name.equals("expirationDate")) { 2962 throw new FHIRException("Cannot call addChild on a singleton property Immunization.expirationDate"); 2963 } 2964 else if (name.equals("site")) { 2965 this.site = new CodeableConcept(); 2966 return this.site; 2967 } 2968 else if (name.equals("route")) { 2969 this.route = new CodeableConcept(); 2970 return this.route; 2971 } 2972 else if (name.equals("doseQuantity")) { 2973 this.doseQuantity = new SimpleQuantity(); 2974 return this.doseQuantity; 2975 } 2976 else if (name.equals("practitioner")) { 2977 return addPractitioner(); 2978 } 2979 else if (name.equals("note")) { 2980 return addNote(); 2981 } 2982 else if (name.equals("explanation")) { 2983 this.explanation = new ImmunizationExplanationComponent(); 2984 return this.explanation; 2985 } 2986 else if (name.equals("reaction")) { 2987 return addReaction(); 2988 } 2989 else if (name.equals("vaccinationProtocol")) { 2990 return addVaccinationProtocol(); 2991 } 2992 else 2993 return super.addChild(name); 2994 } 2995 2996 public String fhirType() { 2997 return "Immunization"; 2998 2999 } 3000 3001 public Immunization copy() { 3002 Immunization dst = new Immunization(); 3003 copyValues(dst); 3004 if (identifier != null) { 3005 dst.identifier = new ArrayList<Identifier>(); 3006 for (Identifier i : identifier) 3007 dst.identifier.add(i.copy()); 3008 }; 3009 dst.status = status == null ? null : status.copy(); 3010 dst.notGiven = notGiven == null ? null : notGiven.copy(); 3011 dst.vaccineCode = vaccineCode == null ? null : vaccineCode.copy(); 3012 dst.patient = patient == null ? null : patient.copy(); 3013 dst.encounter = encounter == null ? null : encounter.copy(); 3014 dst.date = date == null ? null : date.copy(); 3015 dst.primarySource = primarySource == null ? null : primarySource.copy(); 3016 dst.reportOrigin = reportOrigin == null ? null : reportOrigin.copy(); 3017 dst.location = location == null ? null : location.copy(); 3018 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 3019 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 3020 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 3021 dst.site = site == null ? null : site.copy(); 3022 dst.route = route == null ? null : route.copy(); 3023 dst.doseQuantity = doseQuantity == null ? null : doseQuantity.copy(); 3024 if (practitioner != null) { 3025 dst.practitioner = new ArrayList<ImmunizationPractitionerComponent>(); 3026 for (ImmunizationPractitionerComponent i : practitioner) 3027 dst.practitioner.add(i.copy()); 3028 }; 3029 if (note != null) { 3030 dst.note = new ArrayList<Annotation>(); 3031 for (Annotation i : note) 3032 dst.note.add(i.copy()); 3033 }; 3034 dst.explanation = explanation == null ? null : explanation.copy(); 3035 if (reaction != null) { 3036 dst.reaction = new ArrayList<ImmunizationReactionComponent>(); 3037 for (ImmunizationReactionComponent i : reaction) 3038 dst.reaction.add(i.copy()); 3039 }; 3040 if (vaccinationProtocol != null) { 3041 dst.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 3042 for (ImmunizationVaccinationProtocolComponent i : vaccinationProtocol) 3043 dst.vaccinationProtocol.add(i.copy()); 3044 }; 3045 return dst; 3046 } 3047 3048 protected Immunization typedCopy() { 3049 return copy(); 3050 } 3051 3052 @Override 3053 public boolean equalsDeep(Base other_) { 3054 if (!super.equalsDeep(other_)) 3055 return false; 3056 if (!(other_ instanceof Immunization)) 3057 return false; 3058 Immunization o = (Immunization) other_; 3059 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(notGiven, o.notGiven, true) 3060 && compareDeep(vaccineCode, o.vaccineCode, true) && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) 3061 && compareDeep(date, o.date, true) && compareDeep(primarySource, o.primarySource, true) && compareDeep(reportOrigin, o.reportOrigin, true) 3062 && compareDeep(location, o.location, true) && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(lotNumber, o.lotNumber, true) 3063 && compareDeep(expirationDate, o.expirationDate, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 3064 && compareDeep(doseQuantity, o.doseQuantity, true) && compareDeep(practitioner, o.practitioner, true) 3065 && compareDeep(note, o.note, true) && compareDeep(explanation, o.explanation, true) && compareDeep(reaction, o.reaction, true) 3066 && compareDeep(vaccinationProtocol, o.vaccinationProtocol, true); 3067 } 3068 3069 @Override 3070 public boolean equalsShallow(Base other_) { 3071 if (!super.equalsShallow(other_)) 3072 return false; 3073 if (!(other_ instanceof Immunization)) 3074 return false; 3075 Immunization o = (Immunization) other_; 3076 return compareValues(status, o.status, true) && compareValues(notGiven, o.notGiven, true) && compareValues(date, o.date, true) 3077 && compareValues(primarySource, o.primarySource, true) && compareValues(lotNumber, o.lotNumber, true) 3078 && compareValues(expirationDate, o.expirationDate, true); 3079 } 3080 3081 public boolean isEmpty() { 3082 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, notGiven 3083 , vaccineCode, patient, encounter, date, primarySource, reportOrigin, location 3084 , manufacturer, lotNumber, expirationDate, site, route, doseQuantity, practitioner 3085 , note, explanation, reaction, vaccinationProtocol); 3086 } 3087 3088 @Override 3089 public ResourceType getResourceType() { 3090 return ResourceType.Immunization; 3091 } 3092 3093 /** 3094 * Search parameter: <b>date</b> 3095 * <p> 3096 * Description: <b>Vaccination (non)-Administration Date</b><br> 3097 * Type: <b>date</b><br> 3098 * Path: <b>Immunization.date</b><br> 3099 * </p> 3100 */ 3101 @SearchParamDefinition(name="date", path="Immunization.date", description="Vaccination (non)-Administration Date", type="date" ) 3102 public static final String SP_DATE = "date"; 3103 /** 3104 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3105 * <p> 3106 * Description: <b>Vaccination (non)-Administration Date</b><br> 3107 * Type: <b>date</b><br> 3108 * Path: <b>Immunization.date</b><br> 3109 * </p> 3110 */ 3111 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3112 3113 /** 3114 * Search parameter: <b>identifier</b> 3115 * <p> 3116 * Description: <b>Business identifier</b><br> 3117 * Type: <b>token</b><br> 3118 * Path: <b>Immunization.identifier</b><br> 3119 * </p> 3120 */ 3121 @SearchParamDefinition(name="identifier", path="Immunization.identifier", description="Business identifier", type="token" ) 3122 public static final String SP_IDENTIFIER = "identifier"; 3123 /** 3124 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3125 * <p> 3126 * Description: <b>Business identifier</b><br> 3127 * Type: <b>token</b><br> 3128 * Path: <b>Immunization.identifier</b><br> 3129 * </p> 3130 */ 3131 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3132 3133 /** 3134 * Search parameter: <b>reason</b> 3135 * <p> 3136 * Description: <b>Why immunization occurred</b><br> 3137 * Type: <b>token</b><br> 3138 * Path: <b>Immunization.explanation.reason</b><br> 3139 * </p> 3140 */ 3141 @SearchParamDefinition(name="reason", path="Immunization.explanation.reason", description="Why immunization occurred", type="token" ) 3142 public static final String SP_REASON = "reason"; 3143 /** 3144 * <b>Fluent Client</b> search parameter constant for <b>reason</b> 3145 * <p> 3146 * Description: <b>Why immunization occurred</b><br> 3147 * Type: <b>token</b><br> 3148 * Path: <b>Immunization.explanation.reason</b><br> 3149 * </p> 3150 */ 3151 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON); 3152 3153 /** 3154 * Search parameter: <b>reaction</b> 3155 * <p> 3156 * Description: <b>Additional information on reaction</b><br> 3157 * Type: <b>reference</b><br> 3158 * Path: <b>Immunization.reaction.detail</b><br> 3159 * </p> 3160 */ 3161 @SearchParamDefinition(name="reaction", path="Immunization.reaction.detail", description="Additional information on reaction", type="reference", target={Observation.class } ) 3162 public static final String SP_REACTION = "reaction"; 3163 /** 3164 * <b>Fluent Client</b> search parameter constant for <b>reaction</b> 3165 * <p> 3166 * Description: <b>Additional information on reaction</b><br> 3167 * Type: <b>reference</b><br> 3168 * Path: <b>Immunization.reaction.detail</b><br> 3169 * </p> 3170 */ 3171 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REACTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REACTION); 3172 3173/** 3174 * Constant for fluent queries to be used to add include statements. Specifies 3175 * the path value of "<b>Immunization:reaction</b>". 3176 */ 3177 public static final ca.uhn.fhir.model.api.Include INCLUDE_REACTION = new ca.uhn.fhir.model.api.Include("Immunization:reaction").toLocked(); 3178 3179 /** 3180 * Search parameter: <b>lot-number</b> 3181 * <p> 3182 * Description: <b>Vaccine Lot Number</b><br> 3183 * Type: <b>string</b><br> 3184 * Path: <b>Immunization.lotNumber</b><br> 3185 * </p> 3186 */ 3187 @SearchParamDefinition(name="lot-number", path="Immunization.lotNumber", description="Vaccine Lot Number", type="string" ) 3188 public static final String SP_LOT_NUMBER = "lot-number"; 3189 /** 3190 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 3191 * <p> 3192 * Description: <b>Vaccine Lot Number</b><br> 3193 * Type: <b>string</b><br> 3194 * Path: <b>Immunization.lotNumber</b><br> 3195 * </p> 3196 */ 3197 public static final ca.uhn.fhir.rest.gclient.StringClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_LOT_NUMBER); 3198 3199 /** 3200 * Search parameter: <b>practitioner</b> 3201 * <p> 3202 * Description: <b>The practitioner who played a role in the vaccination</b><br> 3203 * Type: <b>reference</b><br> 3204 * Path: <b>Immunization.practitioner.actor</b><br> 3205 * </p> 3206 */ 3207 @SearchParamDefinition(name="practitioner", path="Immunization.practitioner.actor", description="The practitioner who played a role in the vaccination", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 3208 public static final String SP_PRACTITIONER = "practitioner"; 3209 /** 3210 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 3211 * <p> 3212 * Description: <b>The practitioner who played a role in the vaccination</b><br> 3213 * Type: <b>reference</b><br> 3214 * Path: <b>Immunization.practitioner.actor</b><br> 3215 * </p> 3216 */ 3217 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 3218 3219/** 3220 * Constant for fluent queries to be used to add include statements. Specifies 3221 * the path value of "<b>Immunization:practitioner</b>". 3222 */ 3223 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Immunization:practitioner").toLocked(); 3224 3225 /** 3226 * Search parameter: <b>notgiven</b> 3227 * <p> 3228 * Description: <b>Administrations which were not given</b><br> 3229 * Type: <b>token</b><br> 3230 * Path: <b>Immunization.notGiven</b><br> 3231 * </p> 3232 */ 3233 @SearchParamDefinition(name="notgiven", path="Immunization.notGiven", description="Administrations which were not given", type="token" ) 3234 public static final String SP_NOTGIVEN = "notgiven"; 3235 /** 3236 * <b>Fluent Client</b> search parameter constant for <b>notgiven</b> 3237 * <p> 3238 * Description: <b>Administrations which were not given</b><br> 3239 * Type: <b>token</b><br> 3240 * Path: <b>Immunization.notGiven</b><br> 3241 * </p> 3242 */ 3243 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NOTGIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_NOTGIVEN); 3244 3245 /** 3246 * Search parameter: <b>manufacturer</b> 3247 * <p> 3248 * Description: <b>Vaccine Manufacturer</b><br> 3249 * Type: <b>reference</b><br> 3250 * Path: <b>Immunization.manufacturer</b><br> 3251 * </p> 3252 */ 3253 @SearchParamDefinition(name="manufacturer", path="Immunization.manufacturer", description="Vaccine Manufacturer", type="reference", target={Organization.class } ) 3254 public static final String SP_MANUFACTURER = "manufacturer"; 3255 /** 3256 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 3257 * <p> 3258 * Description: <b>Vaccine Manufacturer</b><br> 3259 * Type: <b>reference</b><br> 3260 * Path: <b>Immunization.manufacturer</b><br> 3261 * </p> 3262 */ 3263 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANUFACTURER); 3264 3265/** 3266 * Constant for fluent queries to be used to add include statements. Specifies 3267 * the path value of "<b>Immunization:manufacturer</b>". 3268 */ 3269 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include("Immunization:manufacturer").toLocked(); 3270 3271 /** 3272 * Search parameter: <b>dose-sequence</b> 3273 * <p> 3274 * Description: <b>Dose number within series</b><br> 3275 * Type: <b>number</b><br> 3276 * Path: <b>Immunization.vaccinationProtocol.doseSequence</b><br> 3277 * </p> 3278 */ 3279 @SearchParamDefinition(name="dose-sequence", path="Immunization.vaccinationProtocol.doseSequence", description="Dose number within series", type="number" ) 3280 public static final String SP_DOSE_SEQUENCE = "dose-sequence"; 3281 /** 3282 * <b>Fluent Client</b> search parameter constant for <b>dose-sequence</b> 3283 * <p> 3284 * Description: <b>Dose number within series</b><br> 3285 * Type: <b>number</b><br> 3286 * Path: <b>Immunization.vaccinationProtocol.doseSequence</b><br> 3287 * </p> 3288 */ 3289 public static final ca.uhn.fhir.rest.gclient.NumberClientParam DOSE_SEQUENCE = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_DOSE_SEQUENCE); 3290 3291 /** 3292 * Search parameter: <b>patient</b> 3293 * <p> 3294 * Description: <b>The patient for the vaccination record</b><br> 3295 * Type: <b>reference</b><br> 3296 * Path: <b>Immunization.patient</b><br> 3297 * </p> 3298 */ 3299 @SearchParamDefinition(name="patient", path="Immunization.patient", description="The patient for the vaccination record", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 3300 public static final String SP_PATIENT = "patient"; 3301 /** 3302 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3303 * <p> 3304 * Description: <b>The patient for the vaccination record</b><br> 3305 * Type: <b>reference</b><br> 3306 * Path: <b>Immunization.patient</b><br> 3307 * </p> 3308 */ 3309 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3310 3311/** 3312 * Constant for fluent queries to be used to add include statements. Specifies 3313 * the path value of "<b>Immunization:patient</b>". 3314 */ 3315 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Immunization:patient").toLocked(); 3316 3317 /** 3318 * Search parameter: <b>vaccine-code</b> 3319 * <p> 3320 * Description: <b>Vaccine Product Administered</b><br> 3321 * Type: <b>token</b><br> 3322 * Path: <b>Immunization.vaccineCode</b><br> 3323 * </p> 3324 */ 3325 @SearchParamDefinition(name="vaccine-code", path="Immunization.vaccineCode", description="Vaccine Product Administered", type="token" ) 3326 public static final String SP_VACCINE_CODE = "vaccine-code"; 3327 /** 3328 * <b>Fluent Client</b> search parameter constant for <b>vaccine-code</b> 3329 * <p> 3330 * Description: <b>Vaccine Product Administered</b><br> 3331 * Type: <b>token</b><br> 3332 * Path: <b>Immunization.vaccineCode</b><br> 3333 * </p> 3334 */ 3335 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VACCINE_CODE); 3336 3337 /** 3338 * Search parameter: <b>reason-not-given</b> 3339 * <p> 3340 * Description: <b>Explanation of reason vaccination was not administered</b><br> 3341 * Type: <b>token</b><br> 3342 * Path: <b>Immunization.explanation.reasonNotGiven</b><br> 3343 * </p> 3344 */ 3345 @SearchParamDefinition(name="reason-not-given", path="Immunization.explanation.reasonNotGiven", description="Explanation of reason vaccination was not administered", type="token" ) 3346 public static final String SP_REASON_NOT_GIVEN = "reason-not-given"; 3347 /** 3348 * <b>Fluent Client</b> search parameter constant for <b>reason-not-given</b> 3349 * <p> 3350 * Description: <b>Explanation of reason vaccination was not administered</b><br> 3351 * Type: <b>token</b><br> 3352 * Path: <b>Immunization.explanation.reasonNotGiven</b><br> 3353 * </p> 3354 */ 3355 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_NOT_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_NOT_GIVEN); 3356 3357 /** 3358 * Search parameter: <b>location</b> 3359 * <p> 3360 * Description: <b>The service delivery location or facility in which the vaccine was / was to be administered</b><br> 3361 * Type: <b>reference</b><br> 3362 * Path: <b>Immunization.location</b><br> 3363 * </p> 3364 */ 3365 @SearchParamDefinition(name="location", path="Immunization.location", description="The service delivery location or facility in which the vaccine was / was to be administered", type="reference", target={Location.class } ) 3366 public static final String SP_LOCATION = "location"; 3367 /** 3368 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3369 * <p> 3370 * Description: <b>The service delivery location or facility in which the vaccine was / was to be administered</b><br> 3371 * Type: <b>reference</b><br> 3372 * Path: <b>Immunization.location</b><br> 3373 * </p> 3374 */ 3375 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 3376 3377/** 3378 * Constant for fluent queries to be used to add include statements. Specifies 3379 * the path value of "<b>Immunization:location</b>". 3380 */ 3381 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Immunization:location").toLocked(); 3382 3383 /** 3384 * Search parameter: <b>reaction-date</b> 3385 * <p> 3386 * Description: <b>When reaction started</b><br> 3387 * Type: <b>date</b><br> 3388 * Path: <b>Immunization.reaction.date</b><br> 3389 * </p> 3390 */ 3391 @SearchParamDefinition(name="reaction-date", path="Immunization.reaction.date", description="When reaction started", type="date" ) 3392 public static final String SP_REACTION_DATE = "reaction-date"; 3393 /** 3394 * <b>Fluent Client</b> search parameter constant for <b>reaction-date</b> 3395 * <p> 3396 * Description: <b>When reaction started</b><br> 3397 * Type: <b>date</b><br> 3398 * Path: <b>Immunization.reaction.date</b><br> 3399 * </p> 3400 */ 3401 public static final ca.uhn.fhir.rest.gclient.DateClientParam REACTION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_REACTION_DATE); 3402 3403 /** 3404 * Search parameter: <b>status</b> 3405 * <p> 3406 * Description: <b>Immunization event status</b><br> 3407 * Type: <b>token</b><br> 3408 * Path: <b>Immunization.status</b><br> 3409 * </p> 3410 */ 3411 @SearchParamDefinition(name="status", path="Immunization.status", description="Immunization event status", type="token" ) 3412 public static final String SP_STATUS = "status"; 3413 /** 3414 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3415 * <p> 3416 * Description: <b>Immunization event status</b><br> 3417 * Type: <b>token</b><br> 3418 * Path: <b>Immunization.status</b><br> 3419 * </p> 3420 */ 3421 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3422 3423 3424}