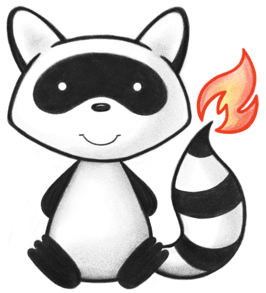
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * Describes the event of a patient being administered a vaccination or a record of a vaccination as reported by a patient, a clinician or another party and may include vaccine reaction information and what vaccination protocol was followed. 050 */ 051@ResourceDef(name="Immunization", profile="http://hl7.org/fhir/Profile/Immunization") 052public class Immunization extends DomainResource { 053 054 public enum ImmunizationStatus { 055 /** 056 * null 057 */ 058 COMPLETED, 059 /** 060 * null 061 */ 062 ENTEREDINERROR, 063 /** 064 * added to help the parsers with the generic types 065 */ 066 NULL; 067 public static ImmunizationStatus fromCode(String codeString) throws FHIRException { 068 if (codeString == null || "".equals(codeString)) 069 return null; 070 if ("completed".equals(codeString)) 071 return COMPLETED; 072 if ("entered-in-error".equals(codeString)) 073 return ENTEREDINERROR; 074 if (Configuration.isAcceptInvalidEnums()) 075 return null; 076 else 077 throw new FHIRException("Unknown ImmunizationStatus code '"+codeString+"'"); 078 } 079 public String toCode() { 080 switch (this) { 081 case COMPLETED: return "completed"; 082 case ENTEREDINERROR: return "entered-in-error"; 083 case NULL: return null; 084 default: return "?"; 085 } 086 } 087 public String getSystem() { 088 switch (this) { 089 case COMPLETED: return "http://hl7.org/fhir/medication-admin-status"; 090 case ENTEREDINERROR: return "http://hl7.org/fhir/medication-admin-status"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getDefinition() { 096 switch (this) { 097 case COMPLETED: return ""; 098 case ENTEREDINERROR: return ""; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDisplay() { 104 switch (this) { 105 case COMPLETED: return "completed"; 106 case ENTEREDINERROR: return "entered-in-error"; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 } 112 113 public static class ImmunizationStatusEnumFactory implements EnumFactory<ImmunizationStatus> { 114 public ImmunizationStatus fromCode(String codeString) throws IllegalArgumentException { 115 if (codeString == null || "".equals(codeString)) 116 if (codeString == null || "".equals(codeString)) 117 return null; 118 if ("completed".equals(codeString)) 119 return ImmunizationStatus.COMPLETED; 120 if ("entered-in-error".equals(codeString)) 121 return ImmunizationStatus.ENTEREDINERROR; 122 throw new IllegalArgumentException("Unknown ImmunizationStatus code '"+codeString+"'"); 123 } 124 public Enumeration<ImmunizationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 125 if (code == null) 126 return null; 127 if (code.isEmpty()) 128 return new Enumeration<ImmunizationStatus>(this); 129 String codeString = code.asStringValue(); 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("completed".equals(codeString)) 133 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.COMPLETED); 134 if ("entered-in-error".equals(codeString)) 135 return new Enumeration<ImmunizationStatus>(this, ImmunizationStatus.ENTEREDINERROR); 136 throw new FHIRException("Unknown ImmunizationStatus code '"+codeString+"'"); 137 } 138 public String toCode(ImmunizationStatus code) { 139 if (code == ImmunizationStatus.COMPLETED) 140 return "completed"; 141 if (code == ImmunizationStatus.ENTEREDINERROR) 142 return "entered-in-error"; 143 return "?"; 144 } 145 public String toSystem(ImmunizationStatus code) { 146 return code.getSystem(); 147 } 148 } 149 150 @Block() 151 public static class ImmunizationPractitionerComponent extends BackboneElement implements IBaseBackboneElement { 152 /** 153 * Describes the type of performance (e.g. ordering provider, administering provider, etc.). 154 */ 155 @Child(name = "role", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=true) 156 @Description(shortDefinition="What type of performance was done", formalDefinition="Describes the type of performance (e.g. ordering provider, administering provider, etc.)." ) 157 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-role") 158 protected CodeableConcept role; 159 160 /** 161 * The device, practitioner, etc. who performed the action. 162 */ 163 @Child(name = "actor", type = {Practitioner.class}, order=2, min=1, max=1, modifier=false, summary=true) 164 @Description(shortDefinition="Individual who was performing", formalDefinition="The device, practitioner, etc. who performed the action." ) 165 protected Reference actor; 166 167 /** 168 * The actual object that is the target of the reference (The device, practitioner, etc. who performed the action.) 169 */ 170 protected Practitioner actorTarget; 171 172 private static final long serialVersionUID = -922003669L; 173 174 /** 175 * Constructor 176 */ 177 public ImmunizationPractitionerComponent() { 178 super(); 179 } 180 181 /** 182 * Constructor 183 */ 184 public ImmunizationPractitionerComponent(Reference actor) { 185 super(); 186 this.actor = actor; 187 } 188 189 /** 190 * @return {@link #role} (Describes the type of performance (e.g. ordering provider, administering provider, etc.).) 191 */ 192 public CodeableConcept getRole() { 193 if (this.role == null) 194 if (Configuration.errorOnAutoCreate()) 195 throw new Error("Attempt to auto-create ImmunizationPractitionerComponent.role"); 196 else if (Configuration.doAutoCreate()) 197 this.role = new CodeableConcept(); // cc 198 return this.role; 199 } 200 201 public boolean hasRole() { 202 return this.role != null && !this.role.isEmpty(); 203 } 204 205 /** 206 * @param value {@link #role} (Describes the type of performance (e.g. ordering provider, administering provider, etc.).) 207 */ 208 public ImmunizationPractitionerComponent setRole(CodeableConcept value) { 209 this.role = value; 210 return this; 211 } 212 213 /** 214 * @return {@link #actor} (The device, practitioner, etc. who performed the action.) 215 */ 216 public Reference getActor() { 217 if (this.actor == null) 218 if (Configuration.errorOnAutoCreate()) 219 throw new Error("Attempt to auto-create ImmunizationPractitionerComponent.actor"); 220 else if (Configuration.doAutoCreate()) 221 this.actor = new Reference(); // cc 222 return this.actor; 223 } 224 225 public boolean hasActor() { 226 return this.actor != null && !this.actor.isEmpty(); 227 } 228 229 /** 230 * @param value {@link #actor} (The device, practitioner, etc. who performed the action.) 231 */ 232 public ImmunizationPractitionerComponent setActor(Reference value) { 233 this.actor = value; 234 return this; 235 } 236 237 /** 238 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed the action.) 239 */ 240 public Practitioner getActorTarget() { 241 if (this.actorTarget == null) 242 if (Configuration.errorOnAutoCreate()) 243 throw new Error("Attempt to auto-create ImmunizationPractitionerComponent.actor"); 244 else if (Configuration.doAutoCreate()) 245 this.actorTarget = new Practitioner(); // aa 246 return this.actorTarget; 247 } 248 249 /** 250 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed the action.) 251 */ 252 public ImmunizationPractitionerComponent setActorTarget(Practitioner value) { 253 this.actorTarget = value; 254 return this; 255 } 256 257 protected void listChildren(List<Property> children) { 258 super.listChildren(children); 259 children.add(new Property("role", "CodeableConcept", "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, role)); 260 children.add(new Property("actor", "Reference(Practitioner)", "The device, practitioner, etc. who performed the action.", 0, 1, actor)); 261 } 262 263 @Override 264 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 265 switch (_hash) { 266 case 3506294: /*role*/ return new Property("role", "CodeableConcept", "Describes the type of performance (e.g. ordering provider, administering provider, etc.).", 0, 1, role); 267 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner)", "The device, practitioner, etc. who performed the action.", 0, 1, actor); 268 default: return super.getNamedProperty(_hash, _name, _checkValid); 269 } 270 271 } 272 273 @Override 274 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 275 switch (hash) { 276 case 3506294: /*role*/ return this.role == null ? new Base[0] : new Base[] {this.role}; // CodeableConcept 277 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 278 default: return super.getProperty(hash, name, checkValid); 279 } 280 281 } 282 283 @Override 284 public Base setProperty(int hash, String name, Base value) throws FHIRException { 285 switch (hash) { 286 case 3506294: // role 287 this.role = castToCodeableConcept(value); // CodeableConcept 288 return value; 289 case 92645877: // actor 290 this.actor = castToReference(value); // Reference 291 return value; 292 default: return super.setProperty(hash, name, value); 293 } 294 295 } 296 297 @Override 298 public Base setProperty(String name, Base value) throws FHIRException { 299 if (name.equals("role")) { 300 this.role = castToCodeableConcept(value); // CodeableConcept 301 } else if (name.equals("actor")) { 302 this.actor = castToReference(value); // Reference 303 } else 304 return super.setProperty(name, value); 305 return value; 306 } 307 308 @Override 309 public Base makeProperty(int hash, String name) throws FHIRException { 310 switch (hash) { 311 case 3506294: return getRole(); 312 case 92645877: return getActor(); 313 default: return super.makeProperty(hash, name); 314 } 315 316 } 317 318 @Override 319 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 320 switch (hash) { 321 case 3506294: /*role*/ return new String[] {"CodeableConcept"}; 322 case 92645877: /*actor*/ return new String[] {"Reference"}; 323 default: return super.getTypesForProperty(hash, name); 324 } 325 326 } 327 328 @Override 329 public Base addChild(String name) throws FHIRException { 330 if (name.equals("role")) { 331 this.role = new CodeableConcept(); 332 return this.role; 333 } 334 else if (name.equals("actor")) { 335 this.actor = new Reference(); 336 return this.actor; 337 } 338 else 339 return super.addChild(name); 340 } 341 342 public ImmunizationPractitionerComponent copy() { 343 ImmunizationPractitionerComponent dst = new ImmunizationPractitionerComponent(); 344 copyValues(dst); 345 dst.role = role == null ? null : role.copy(); 346 dst.actor = actor == null ? null : actor.copy(); 347 return dst; 348 } 349 350 @Override 351 public boolean equalsDeep(Base other_) { 352 if (!super.equalsDeep(other_)) 353 return false; 354 if (!(other_ instanceof ImmunizationPractitionerComponent)) 355 return false; 356 ImmunizationPractitionerComponent o = (ImmunizationPractitionerComponent) other_; 357 return compareDeep(role, o.role, true) && compareDeep(actor, o.actor, true); 358 } 359 360 @Override 361 public boolean equalsShallow(Base other_) { 362 if (!super.equalsShallow(other_)) 363 return false; 364 if (!(other_ instanceof ImmunizationPractitionerComponent)) 365 return false; 366 ImmunizationPractitionerComponent o = (ImmunizationPractitionerComponent) other_; 367 return true; 368 } 369 370 public boolean isEmpty() { 371 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(role, actor); 372 } 373 374 public String fhirType() { 375 return "Immunization.practitioner"; 376 377 } 378 379 } 380 381 @Block() 382 public static class ImmunizationExplanationComponent extends BackboneElement implements IBaseBackboneElement { 383 /** 384 * Reasons why a vaccine was administered. 385 */ 386 @Child(name = "reason", type = {CodeableConcept.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 387 @Description(shortDefinition="Why immunization occurred", formalDefinition="Reasons why a vaccine was administered." ) 388 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-reason") 389 protected List<CodeableConcept> reason; 390 391 /** 392 * Reason why a vaccine was not administered. 393 */ 394 @Child(name = "reasonNotGiven", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 395 @Description(shortDefinition="Why immunization did not occur", formalDefinition="Reason why a vaccine was not administered." ) 396 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/no-immunization-reason") 397 protected List<CodeableConcept> reasonNotGiven; 398 399 private static final long serialVersionUID = -539821866L; 400 401 /** 402 * Constructor 403 */ 404 public ImmunizationExplanationComponent() { 405 super(); 406 } 407 408 /** 409 * @return {@link #reason} (Reasons why a vaccine was administered.) 410 */ 411 public List<CodeableConcept> getReason() { 412 if (this.reason == null) 413 this.reason = new ArrayList<CodeableConcept>(); 414 return this.reason; 415 } 416 417 /** 418 * @return Returns a reference to <code>this</code> for easy method chaining 419 */ 420 public ImmunizationExplanationComponent setReason(List<CodeableConcept> theReason) { 421 this.reason = theReason; 422 return this; 423 } 424 425 public boolean hasReason() { 426 if (this.reason == null) 427 return false; 428 for (CodeableConcept item : this.reason) 429 if (!item.isEmpty()) 430 return true; 431 return false; 432 } 433 434 public CodeableConcept addReason() { //3 435 CodeableConcept t = new CodeableConcept(); 436 if (this.reason == null) 437 this.reason = new ArrayList<CodeableConcept>(); 438 this.reason.add(t); 439 return t; 440 } 441 442 public ImmunizationExplanationComponent addReason(CodeableConcept t) { //3 443 if (t == null) 444 return this; 445 if (this.reason == null) 446 this.reason = new ArrayList<CodeableConcept>(); 447 this.reason.add(t); 448 return this; 449 } 450 451 /** 452 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist 453 */ 454 public CodeableConcept getReasonFirstRep() { 455 if (getReason().isEmpty()) { 456 addReason(); 457 } 458 return getReason().get(0); 459 } 460 461 /** 462 * @return {@link #reasonNotGiven} (Reason why a vaccine was not administered.) 463 */ 464 public List<CodeableConcept> getReasonNotGiven() { 465 if (this.reasonNotGiven == null) 466 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 467 return this.reasonNotGiven; 468 } 469 470 /** 471 * @return Returns a reference to <code>this</code> for easy method chaining 472 */ 473 public ImmunizationExplanationComponent setReasonNotGiven(List<CodeableConcept> theReasonNotGiven) { 474 this.reasonNotGiven = theReasonNotGiven; 475 return this; 476 } 477 478 public boolean hasReasonNotGiven() { 479 if (this.reasonNotGiven == null) 480 return false; 481 for (CodeableConcept item : this.reasonNotGiven) 482 if (!item.isEmpty()) 483 return true; 484 return false; 485 } 486 487 public CodeableConcept addReasonNotGiven() { //3 488 CodeableConcept t = new CodeableConcept(); 489 if (this.reasonNotGiven == null) 490 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 491 this.reasonNotGiven.add(t); 492 return t; 493 } 494 495 public ImmunizationExplanationComponent addReasonNotGiven(CodeableConcept t) { //3 496 if (t == null) 497 return this; 498 if (this.reasonNotGiven == null) 499 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 500 this.reasonNotGiven.add(t); 501 return this; 502 } 503 504 /** 505 * @return The first repetition of repeating field {@link #reasonNotGiven}, creating it if it does not already exist 506 */ 507 public CodeableConcept getReasonNotGivenFirstRep() { 508 if (getReasonNotGiven().isEmpty()) { 509 addReasonNotGiven(); 510 } 511 return getReasonNotGiven().get(0); 512 } 513 514 protected void listChildren(List<Property> children) { 515 super.listChildren(children); 516 children.add(new Property("reason", "CodeableConcept", "Reasons why a vaccine was administered.", 0, java.lang.Integer.MAX_VALUE, reason)); 517 children.add(new Property("reasonNotGiven", "CodeableConcept", "Reason why a vaccine was not administered.", 0, java.lang.Integer.MAX_VALUE, reasonNotGiven)); 518 } 519 520 @Override 521 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 522 switch (_hash) { 523 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Reasons why a vaccine was administered.", 0, java.lang.Integer.MAX_VALUE, reason); 524 case 2101123790: /*reasonNotGiven*/ return new Property("reasonNotGiven", "CodeableConcept", "Reason why a vaccine was not administered.", 0, java.lang.Integer.MAX_VALUE, reasonNotGiven); 525 default: return super.getNamedProperty(_hash, _name, _checkValid); 526 } 527 528 } 529 530 @Override 531 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 532 switch (hash) { 533 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 534 case 2101123790: /*reasonNotGiven*/ return this.reasonNotGiven == null ? new Base[0] : this.reasonNotGiven.toArray(new Base[this.reasonNotGiven.size()]); // CodeableConcept 535 default: return super.getProperty(hash, name, checkValid); 536 } 537 538 } 539 540 @Override 541 public Base setProperty(int hash, String name, Base value) throws FHIRException { 542 switch (hash) { 543 case -934964668: // reason 544 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 545 return value; 546 case 2101123790: // reasonNotGiven 547 this.getReasonNotGiven().add(castToCodeableConcept(value)); // CodeableConcept 548 return value; 549 default: return super.setProperty(hash, name, value); 550 } 551 552 } 553 554 @Override 555 public Base setProperty(String name, Base value) throws FHIRException { 556 if (name.equals("reason")) { 557 this.getReason().add(castToCodeableConcept(value)); 558 } else if (name.equals("reasonNotGiven")) { 559 this.getReasonNotGiven().add(castToCodeableConcept(value)); 560 } else 561 return super.setProperty(name, value); 562 return value; 563 } 564 565 @Override 566 public Base makeProperty(int hash, String name) throws FHIRException { 567 switch (hash) { 568 case -934964668: return addReason(); 569 case 2101123790: return addReasonNotGiven(); 570 default: return super.makeProperty(hash, name); 571 } 572 573 } 574 575 @Override 576 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 577 switch (hash) { 578 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 579 case 2101123790: /*reasonNotGiven*/ return new String[] {"CodeableConcept"}; 580 default: return super.getTypesForProperty(hash, name); 581 } 582 583 } 584 585 @Override 586 public Base addChild(String name) throws FHIRException { 587 if (name.equals("reason")) { 588 return addReason(); 589 } 590 else if (name.equals("reasonNotGiven")) { 591 return addReasonNotGiven(); 592 } 593 else 594 return super.addChild(name); 595 } 596 597 public ImmunizationExplanationComponent copy() { 598 ImmunizationExplanationComponent dst = new ImmunizationExplanationComponent(); 599 copyValues(dst); 600 if (reason != null) { 601 dst.reason = new ArrayList<CodeableConcept>(); 602 for (CodeableConcept i : reason) 603 dst.reason.add(i.copy()); 604 }; 605 if (reasonNotGiven != null) { 606 dst.reasonNotGiven = new ArrayList<CodeableConcept>(); 607 for (CodeableConcept i : reasonNotGiven) 608 dst.reasonNotGiven.add(i.copy()); 609 }; 610 return dst; 611 } 612 613 @Override 614 public boolean equalsDeep(Base other_) { 615 if (!super.equalsDeep(other_)) 616 return false; 617 if (!(other_ instanceof ImmunizationExplanationComponent)) 618 return false; 619 ImmunizationExplanationComponent o = (ImmunizationExplanationComponent) other_; 620 return compareDeep(reason, o.reason, true) && compareDeep(reasonNotGiven, o.reasonNotGiven, true) 621 ; 622 } 623 624 @Override 625 public boolean equalsShallow(Base other_) { 626 if (!super.equalsShallow(other_)) 627 return false; 628 if (!(other_ instanceof ImmunizationExplanationComponent)) 629 return false; 630 ImmunizationExplanationComponent o = (ImmunizationExplanationComponent) other_; 631 return true; 632 } 633 634 public boolean isEmpty() { 635 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(reason, reasonNotGiven); 636 } 637 638 public String fhirType() { 639 return "Immunization.explanation"; 640 641 } 642 643 } 644 645 @Block() 646 public static class ImmunizationReactionComponent extends BackboneElement implements IBaseBackboneElement { 647 /** 648 * Date of reaction to the immunization. 649 */ 650 @Child(name = "date", type = {DateTimeType.class}, order=1, min=0, max=1, modifier=false, summary=false) 651 @Description(shortDefinition="When reaction started", formalDefinition="Date of reaction to the immunization." ) 652 protected DateTimeType date; 653 654 /** 655 * Details of the reaction. 656 */ 657 @Child(name = "detail", type = {Observation.class}, order=2, min=0, max=1, modifier=false, summary=false) 658 @Description(shortDefinition="Additional information on reaction", formalDefinition="Details of the reaction." ) 659 protected Reference detail; 660 661 /** 662 * The actual object that is the target of the reference (Details of the reaction.) 663 */ 664 protected Observation detailTarget; 665 666 /** 667 * Self-reported indicator. 668 */ 669 @Child(name = "reported", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=false) 670 @Description(shortDefinition="Indicates self-reported reaction", formalDefinition="Self-reported indicator." ) 671 protected BooleanType reported; 672 673 private static final long serialVersionUID = -1297668556L; 674 675 /** 676 * Constructor 677 */ 678 public ImmunizationReactionComponent() { 679 super(); 680 } 681 682 /** 683 * @return {@link #date} (Date of reaction to the immunization.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 684 */ 685 public DateTimeType getDateElement() { 686 if (this.date == null) 687 if (Configuration.errorOnAutoCreate()) 688 throw new Error("Attempt to auto-create ImmunizationReactionComponent.date"); 689 else if (Configuration.doAutoCreate()) 690 this.date = new DateTimeType(); // bb 691 return this.date; 692 } 693 694 public boolean hasDateElement() { 695 return this.date != null && !this.date.isEmpty(); 696 } 697 698 public boolean hasDate() { 699 return this.date != null && !this.date.isEmpty(); 700 } 701 702 /** 703 * @param value {@link #date} (Date of reaction to the immunization.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 704 */ 705 public ImmunizationReactionComponent setDateElement(DateTimeType value) { 706 this.date = value; 707 return this; 708 } 709 710 /** 711 * @return Date of reaction to the immunization. 712 */ 713 public Date getDate() { 714 return this.date == null ? null : this.date.getValue(); 715 } 716 717 /** 718 * @param value Date of reaction to the immunization. 719 */ 720 public ImmunizationReactionComponent setDate(Date value) { 721 if (value == null) 722 this.date = null; 723 else { 724 if (this.date == null) 725 this.date = new DateTimeType(); 726 this.date.setValue(value); 727 } 728 return this; 729 } 730 731 /** 732 * @return {@link #detail} (Details of the reaction.) 733 */ 734 public Reference getDetail() { 735 if (this.detail == null) 736 if (Configuration.errorOnAutoCreate()) 737 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 738 else if (Configuration.doAutoCreate()) 739 this.detail = new Reference(); // cc 740 return this.detail; 741 } 742 743 public boolean hasDetail() { 744 return this.detail != null && !this.detail.isEmpty(); 745 } 746 747 /** 748 * @param value {@link #detail} (Details of the reaction.) 749 */ 750 public ImmunizationReactionComponent setDetail(Reference value) { 751 this.detail = value; 752 return this; 753 } 754 755 /** 756 * @return {@link #detail} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Details of the reaction.) 757 */ 758 public Observation getDetailTarget() { 759 if (this.detailTarget == null) 760 if (Configuration.errorOnAutoCreate()) 761 throw new Error("Attempt to auto-create ImmunizationReactionComponent.detail"); 762 else if (Configuration.doAutoCreate()) 763 this.detailTarget = new Observation(); // aa 764 return this.detailTarget; 765 } 766 767 /** 768 * @param value {@link #detail} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Details of the reaction.) 769 */ 770 public ImmunizationReactionComponent setDetailTarget(Observation value) { 771 this.detailTarget = value; 772 return this; 773 } 774 775 /** 776 * @return {@link #reported} (Self-reported indicator.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 777 */ 778 public BooleanType getReportedElement() { 779 if (this.reported == null) 780 if (Configuration.errorOnAutoCreate()) 781 throw new Error("Attempt to auto-create ImmunizationReactionComponent.reported"); 782 else if (Configuration.doAutoCreate()) 783 this.reported = new BooleanType(); // bb 784 return this.reported; 785 } 786 787 public boolean hasReportedElement() { 788 return this.reported != null && !this.reported.isEmpty(); 789 } 790 791 public boolean hasReported() { 792 return this.reported != null && !this.reported.isEmpty(); 793 } 794 795 /** 796 * @param value {@link #reported} (Self-reported indicator.). This is the underlying object with id, value and extensions. The accessor "getReported" gives direct access to the value 797 */ 798 public ImmunizationReactionComponent setReportedElement(BooleanType value) { 799 this.reported = value; 800 return this; 801 } 802 803 /** 804 * @return Self-reported indicator. 805 */ 806 public boolean getReported() { 807 return this.reported == null || this.reported.isEmpty() ? false : this.reported.getValue(); 808 } 809 810 /** 811 * @param value Self-reported indicator. 812 */ 813 public ImmunizationReactionComponent setReported(boolean value) { 814 if (this.reported == null) 815 this.reported = new BooleanType(); 816 this.reported.setValue(value); 817 return this; 818 } 819 820 protected void listChildren(List<Property> children) { 821 super.listChildren(children); 822 children.add(new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date)); 823 children.add(new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 1, detail)); 824 children.add(new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported)); 825 } 826 827 @Override 828 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 829 switch (_hash) { 830 case 3076014: /*date*/ return new Property("date", "dateTime", "Date of reaction to the immunization.", 0, 1, date); 831 case -1335224239: /*detail*/ return new Property("detail", "Reference(Observation)", "Details of the reaction.", 0, 1, detail); 832 case -427039533: /*reported*/ return new Property("reported", "boolean", "Self-reported indicator.", 0, 1, reported); 833 default: return super.getNamedProperty(_hash, _name, _checkValid); 834 } 835 836 } 837 838 @Override 839 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 840 switch (hash) { 841 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 842 case -1335224239: /*detail*/ return this.detail == null ? new Base[0] : new Base[] {this.detail}; // Reference 843 case -427039533: /*reported*/ return this.reported == null ? new Base[0] : new Base[] {this.reported}; // BooleanType 844 default: return super.getProperty(hash, name, checkValid); 845 } 846 847 } 848 849 @Override 850 public Base setProperty(int hash, String name, Base value) throws FHIRException { 851 switch (hash) { 852 case 3076014: // date 853 this.date = castToDateTime(value); // DateTimeType 854 return value; 855 case -1335224239: // detail 856 this.detail = castToReference(value); // Reference 857 return value; 858 case -427039533: // reported 859 this.reported = castToBoolean(value); // BooleanType 860 return value; 861 default: return super.setProperty(hash, name, value); 862 } 863 864 } 865 866 @Override 867 public Base setProperty(String name, Base value) throws FHIRException { 868 if (name.equals("date")) { 869 this.date = castToDateTime(value); // DateTimeType 870 } else if (name.equals("detail")) { 871 this.detail = castToReference(value); // Reference 872 } else if (name.equals("reported")) { 873 this.reported = castToBoolean(value); // BooleanType 874 } else 875 return super.setProperty(name, value); 876 return value; 877 } 878 879 @Override 880 public Base makeProperty(int hash, String name) throws FHIRException { 881 switch (hash) { 882 case 3076014: return getDateElement(); 883 case -1335224239: return getDetail(); 884 case -427039533: return getReportedElement(); 885 default: return super.makeProperty(hash, name); 886 } 887 888 } 889 890 @Override 891 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 892 switch (hash) { 893 case 3076014: /*date*/ return new String[] {"dateTime"}; 894 case -1335224239: /*detail*/ return new String[] {"Reference"}; 895 case -427039533: /*reported*/ return new String[] {"boolean"}; 896 default: return super.getTypesForProperty(hash, name); 897 } 898 899 } 900 901 @Override 902 public Base addChild(String name) throws FHIRException { 903 if (name.equals("date")) { 904 throw new FHIRException("Cannot call addChild on a singleton property Immunization.date"); 905 } 906 else if (name.equals("detail")) { 907 this.detail = new Reference(); 908 return this.detail; 909 } 910 else if (name.equals("reported")) { 911 throw new FHIRException("Cannot call addChild on a singleton property Immunization.reported"); 912 } 913 else 914 return super.addChild(name); 915 } 916 917 public ImmunizationReactionComponent copy() { 918 ImmunizationReactionComponent dst = new ImmunizationReactionComponent(); 919 copyValues(dst); 920 dst.date = date == null ? null : date.copy(); 921 dst.detail = detail == null ? null : detail.copy(); 922 dst.reported = reported == null ? null : reported.copy(); 923 return dst; 924 } 925 926 @Override 927 public boolean equalsDeep(Base other_) { 928 if (!super.equalsDeep(other_)) 929 return false; 930 if (!(other_ instanceof ImmunizationReactionComponent)) 931 return false; 932 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 933 return compareDeep(date, o.date, true) && compareDeep(detail, o.detail, true) && compareDeep(reported, o.reported, true) 934 ; 935 } 936 937 @Override 938 public boolean equalsShallow(Base other_) { 939 if (!super.equalsShallow(other_)) 940 return false; 941 if (!(other_ instanceof ImmunizationReactionComponent)) 942 return false; 943 ImmunizationReactionComponent o = (ImmunizationReactionComponent) other_; 944 return compareValues(date, o.date, true) && compareValues(reported, o.reported, true); 945 } 946 947 public boolean isEmpty() { 948 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, detail, reported); 949 } 950 951 public String fhirType() { 952 return "Immunization.reaction"; 953 954 } 955 956 } 957 958 @Block() 959 public static class ImmunizationVaccinationProtocolComponent extends BackboneElement implements IBaseBackboneElement { 960 /** 961 * Nominal position in a series. 962 */ 963 @Child(name = "doseSequence", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 964 @Description(shortDefinition="Dose number within series", formalDefinition="Nominal position in a series." ) 965 protected PositiveIntType doseSequence; 966 967 /** 968 * Contains the description about the protocol under which the vaccine was administered. 969 */ 970 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 971 @Description(shortDefinition="Details of vaccine protocol", formalDefinition="Contains the description about the protocol under which the vaccine was administered." ) 972 protected StringType description; 973 974 /** 975 * Indicates the authority who published the protocol. E.g. ACIP. 976 */ 977 @Child(name = "authority", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 978 @Description(shortDefinition="Who is responsible for protocol", formalDefinition="Indicates the authority who published the protocol. E.g. ACIP." ) 979 protected Reference authority; 980 981 /** 982 * The actual object that is the target of the reference (Indicates the authority who published the protocol. E.g. ACIP.) 983 */ 984 protected Organization authorityTarget; 985 986 /** 987 * One possible path to achieve presumed immunity against a disease - within the context of an authority. 988 */ 989 @Child(name = "series", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 990 @Description(shortDefinition="Name of vaccine series", formalDefinition="One possible path to achieve presumed immunity against a disease - within the context of an authority." ) 991 protected StringType series; 992 993 /** 994 * The recommended number of doses to achieve immunity. 995 */ 996 @Child(name = "seriesDoses", type = {PositiveIntType.class}, order=5, min=0, max=1, modifier=false, summary=false) 997 @Description(shortDefinition="Recommended number of doses for immunity", formalDefinition="The recommended number of doses to achieve immunity." ) 998 protected PositiveIntType seriesDoses; 999 1000 /** 1001 * The targeted disease. 1002 */ 1003 @Child(name = "targetDisease", type = {CodeableConcept.class}, order=6, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1004 @Description(shortDefinition="Disease immunized against", formalDefinition="The targeted disease." ) 1005 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccination-protocol-dose-target") 1006 protected List<CodeableConcept> targetDisease; 1007 1008 /** 1009 * Indicates if the immunization event should "count" against the protocol. 1010 */ 1011 @Child(name = "doseStatus", type = {CodeableConcept.class}, order=7, min=1, max=1, modifier=false, summary=false) 1012 @Description(shortDefinition="Indicates if dose counts towards immunity", formalDefinition="Indicates if the immunization event should \"count\" against the protocol." ) 1013 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccination-protocol-dose-status") 1014 protected CodeableConcept doseStatus; 1015 1016 /** 1017 * Provides an explanation as to why an immunization event should or should not count against the protocol. 1018 */ 1019 @Child(name = "doseStatusReason", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 1020 @Description(shortDefinition="Why dose does (not) count", formalDefinition="Provides an explanation as to why an immunization event should or should not count against the protocol." ) 1021 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccination-protocol-dose-status-reason") 1022 protected CodeableConcept doseStatusReason; 1023 1024 private static final long serialVersionUID = 386814037L; 1025 1026 /** 1027 * Constructor 1028 */ 1029 public ImmunizationVaccinationProtocolComponent() { 1030 super(); 1031 } 1032 1033 /** 1034 * Constructor 1035 */ 1036 public ImmunizationVaccinationProtocolComponent(CodeableConcept doseStatus) { 1037 super(); 1038 this.doseStatus = doseStatus; 1039 } 1040 1041 /** 1042 * @return {@link #doseSequence} (Nominal position in a series.). This is the underlying object with id, value and extensions. The accessor "getDoseSequence" gives direct access to the value 1043 */ 1044 public PositiveIntType getDoseSequenceElement() { 1045 if (this.doseSequence == null) 1046 if (Configuration.errorOnAutoCreate()) 1047 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseSequence"); 1048 else if (Configuration.doAutoCreate()) 1049 this.doseSequence = new PositiveIntType(); // bb 1050 return this.doseSequence; 1051 } 1052 1053 public boolean hasDoseSequenceElement() { 1054 return this.doseSequence != null && !this.doseSequence.isEmpty(); 1055 } 1056 1057 public boolean hasDoseSequence() { 1058 return this.doseSequence != null && !this.doseSequence.isEmpty(); 1059 } 1060 1061 /** 1062 * @param value {@link #doseSequence} (Nominal position in a series.). This is the underlying object with id, value and extensions. The accessor "getDoseSequence" gives direct access to the value 1063 */ 1064 public ImmunizationVaccinationProtocolComponent setDoseSequenceElement(PositiveIntType value) { 1065 this.doseSequence = value; 1066 return this; 1067 } 1068 1069 /** 1070 * @return Nominal position in a series. 1071 */ 1072 public int getDoseSequence() { 1073 return this.doseSequence == null || this.doseSequence.isEmpty() ? 0 : this.doseSequence.getValue(); 1074 } 1075 1076 /** 1077 * @param value Nominal position in a series. 1078 */ 1079 public ImmunizationVaccinationProtocolComponent setDoseSequence(int value) { 1080 if (this.doseSequence == null) 1081 this.doseSequence = new PositiveIntType(); 1082 this.doseSequence.setValue(value); 1083 return this; 1084 } 1085 1086 /** 1087 * @return {@link #description} (Contains the description about the protocol under which the vaccine was administered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1088 */ 1089 public StringType getDescriptionElement() { 1090 if (this.description == null) 1091 if (Configuration.errorOnAutoCreate()) 1092 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.description"); 1093 else if (Configuration.doAutoCreate()) 1094 this.description = new StringType(); // bb 1095 return this.description; 1096 } 1097 1098 public boolean hasDescriptionElement() { 1099 return this.description != null && !this.description.isEmpty(); 1100 } 1101 1102 public boolean hasDescription() { 1103 return this.description != null && !this.description.isEmpty(); 1104 } 1105 1106 /** 1107 * @param value {@link #description} (Contains the description about the protocol under which the vaccine was administered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1108 */ 1109 public ImmunizationVaccinationProtocolComponent setDescriptionElement(StringType value) { 1110 this.description = value; 1111 return this; 1112 } 1113 1114 /** 1115 * @return Contains the description about the protocol under which the vaccine was administered. 1116 */ 1117 public String getDescription() { 1118 return this.description == null ? null : this.description.getValue(); 1119 } 1120 1121 /** 1122 * @param value Contains the description about the protocol under which the vaccine was administered. 1123 */ 1124 public ImmunizationVaccinationProtocolComponent setDescription(String value) { 1125 if (Utilities.noString(value)) 1126 this.description = null; 1127 else { 1128 if (this.description == null) 1129 this.description = new StringType(); 1130 this.description.setValue(value); 1131 } 1132 return this; 1133 } 1134 1135 /** 1136 * @return {@link #authority} (Indicates the authority who published the protocol. E.g. ACIP.) 1137 */ 1138 public Reference getAuthority() { 1139 if (this.authority == null) 1140 if (Configuration.errorOnAutoCreate()) 1141 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.authority"); 1142 else if (Configuration.doAutoCreate()) 1143 this.authority = new Reference(); // cc 1144 return this.authority; 1145 } 1146 1147 public boolean hasAuthority() { 1148 return this.authority != null && !this.authority.isEmpty(); 1149 } 1150 1151 /** 1152 * @param value {@link #authority} (Indicates the authority who published the protocol. E.g. ACIP.) 1153 */ 1154 public ImmunizationVaccinationProtocolComponent setAuthority(Reference value) { 1155 this.authority = value; 1156 return this; 1157 } 1158 1159 /** 1160 * @return {@link #authority} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the authority who published the protocol. E.g. ACIP.) 1161 */ 1162 public Organization getAuthorityTarget() { 1163 if (this.authorityTarget == null) 1164 if (Configuration.errorOnAutoCreate()) 1165 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.authority"); 1166 else if (Configuration.doAutoCreate()) 1167 this.authorityTarget = new Organization(); // aa 1168 return this.authorityTarget; 1169 } 1170 1171 /** 1172 * @param value {@link #authority} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the authority who published the protocol. E.g. ACIP.) 1173 */ 1174 public ImmunizationVaccinationProtocolComponent setAuthorityTarget(Organization value) { 1175 this.authorityTarget = value; 1176 return this; 1177 } 1178 1179 /** 1180 * @return {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 1181 */ 1182 public StringType getSeriesElement() { 1183 if (this.series == null) 1184 if (Configuration.errorOnAutoCreate()) 1185 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.series"); 1186 else if (Configuration.doAutoCreate()) 1187 this.series = new StringType(); // bb 1188 return this.series; 1189 } 1190 1191 public boolean hasSeriesElement() { 1192 return this.series != null && !this.series.isEmpty(); 1193 } 1194 1195 public boolean hasSeries() { 1196 return this.series != null && !this.series.isEmpty(); 1197 } 1198 1199 /** 1200 * @param value {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 1201 */ 1202 public ImmunizationVaccinationProtocolComponent setSeriesElement(StringType value) { 1203 this.series = value; 1204 return this; 1205 } 1206 1207 /** 1208 * @return One possible path to achieve presumed immunity against a disease - within the context of an authority. 1209 */ 1210 public String getSeries() { 1211 return this.series == null ? null : this.series.getValue(); 1212 } 1213 1214 /** 1215 * @param value One possible path to achieve presumed immunity against a disease - within the context of an authority. 1216 */ 1217 public ImmunizationVaccinationProtocolComponent setSeries(String value) { 1218 if (Utilities.noString(value)) 1219 this.series = null; 1220 else { 1221 if (this.series == null) 1222 this.series = new StringType(); 1223 this.series.setValue(value); 1224 } 1225 return this; 1226 } 1227 1228 /** 1229 * @return {@link #seriesDoses} (The recommended number of doses to achieve immunity.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 1230 */ 1231 public PositiveIntType getSeriesDosesElement() { 1232 if (this.seriesDoses == null) 1233 if (Configuration.errorOnAutoCreate()) 1234 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.seriesDoses"); 1235 else if (Configuration.doAutoCreate()) 1236 this.seriesDoses = new PositiveIntType(); // bb 1237 return this.seriesDoses; 1238 } 1239 1240 public boolean hasSeriesDosesElement() { 1241 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 1242 } 1243 1244 public boolean hasSeriesDoses() { 1245 return this.seriesDoses != null && !this.seriesDoses.isEmpty(); 1246 } 1247 1248 /** 1249 * @param value {@link #seriesDoses} (The recommended number of doses to achieve immunity.). This is the underlying object with id, value and extensions. The accessor "getSeriesDoses" gives direct access to the value 1250 */ 1251 public ImmunizationVaccinationProtocolComponent setSeriesDosesElement(PositiveIntType value) { 1252 this.seriesDoses = value; 1253 return this; 1254 } 1255 1256 /** 1257 * @return The recommended number of doses to achieve immunity. 1258 */ 1259 public int getSeriesDoses() { 1260 return this.seriesDoses == null || this.seriesDoses.isEmpty() ? 0 : this.seriesDoses.getValue(); 1261 } 1262 1263 /** 1264 * @param value The recommended number of doses to achieve immunity. 1265 */ 1266 public ImmunizationVaccinationProtocolComponent setSeriesDoses(int value) { 1267 if (this.seriesDoses == null) 1268 this.seriesDoses = new PositiveIntType(); 1269 this.seriesDoses.setValue(value); 1270 return this; 1271 } 1272 1273 /** 1274 * @return {@link #targetDisease} (The targeted disease.) 1275 */ 1276 public List<CodeableConcept> getTargetDisease() { 1277 if (this.targetDisease == null) 1278 this.targetDisease = new ArrayList<CodeableConcept>(); 1279 return this.targetDisease; 1280 } 1281 1282 /** 1283 * @return Returns a reference to <code>this</code> for easy method chaining 1284 */ 1285 public ImmunizationVaccinationProtocolComponent setTargetDisease(List<CodeableConcept> theTargetDisease) { 1286 this.targetDisease = theTargetDisease; 1287 return this; 1288 } 1289 1290 public boolean hasTargetDisease() { 1291 if (this.targetDisease == null) 1292 return false; 1293 for (CodeableConcept item : this.targetDisease) 1294 if (!item.isEmpty()) 1295 return true; 1296 return false; 1297 } 1298 1299 public CodeableConcept addTargetDisease() { //3 1300 CodeableConcept t = new CodeableConcept(); 1301 if (this.targetDisease == null) 1302 this.targetDisease = new ArrayList<CodeableConcept>(); 1303 this.targetDisease.add(t); 1304 return t; 1305 } 1306 1307 public ImmunizationVaccinationProtocolComponent addTargetDisease(CodeableConcept t) { //3 1308 if (t == null) 1309 return this; 1310 if (this.targetDisease == null) 1311 this.targetDisease = new ArrayList<CodeableConcept>(); 1312 this.targetDisease.add(t); 1313 return this; 1314 } 1315 1316 /** 1317 * @return The first repetition of repeating field {@link #targetDisease}, creating it if it does not already exist 1318 */ 1319 public CodeableConcept getTargetDiseaseFirstRep() { 1320 if (getTargetDisease().isEmpty()) { 1321 addTargetDisease(); 1322 } 1323 return getTargetDisease().get(0); 1324 } 1325 1326 /** 1327 * @return {@link #doseStatus} (Indicates if the immunization event should "count" against the protocol.) 1328 */ 1329 public CodeableConcept getDoseStatus() { 1330 if (this.doseStatus == null) 1331 if (Configuration.errorOnAutoCreate()) 1332 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseStatus"); 1333 else if (Configuration.doAutoCreate()) 1334 this.doseStatus = new CodeableConcept(); // cc 1335 return this.doseStatus; 1336 } 1337 1338 public boolean hasDoseStatus() { 1339 return this.doseStatus != null && !this.doseStatus.isEmpty(); 1340 } 1341 1342 /** 1343 * @param value {@link #doseStatus} (Indicates if the immunization event should "count" against the protocol.) 1344 */ 1345 public ImmunizationVaccinationProtocolComponent setDoseStatus(CodeableConcept value) { 1346 this.doseStatus = value; 1347 return this; 1348 } 1349 1350 /** 1351 * @return {@link #doseStatusReason} (Provides an explanation as to why an immunization event should or should not count against the protocol.) 1352 */ 1353 public CodeableConcept getDoseStatusReason() { 1354 if (this.doseStatusReason == null) 1355 if (Configuration.errorOnAutoCreate()) 1356 throw new Error("Attempt to auto-create ImmunizationVaccinationProtocolComponent.doseStatusReason"); 1357 else if (Configuration.doAutoCreate()) 1358 this.doseStatusReason = new CodeableConcept(); // cc 1359 return this.doseStatusReason; 1360 } 1361 1362 public boolean hasDoseStatusReason() { 1363 return this.doseStatusReason != null && !this.doseStatusReason.isEmpty(); 1364 } 1365 1366 /** 1367 * @param value {@link #doseStatusReason} (Provides an explanation as to why an immunization event should or should not count against the protocol.) 1368 */ 1369 public ImmunizationVaccinationProtocolComponent setDoseStatusReason(CodeableConcept value) { 1370 this.doseStatusReason = value; 1371 return this; 1372 } 1373 1374 protected void listChildren(List<Property> children) { 1375 super.listChildren(children); 1376 children.add(new Property("doseSequence", "positiveInt", "Nominal position in a series.", 0, 1, doseSequence)); 1377 children.add(new Property("description", "string", "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description)); 1378 children.add(new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol. E.g. ACIP.", 0, 1, authority)); 1379 children.add(new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series)); 1380 children.add(new Property("seriesDoses", "positiveInt", "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses)); 1381 children.add(new Property("targetDisease", "CodeableConcept", "The targeted disease.", 0, java.lang.Integer.MAX_VALUE, targetDisease)); 1382 children.add(new Property("doseStatus", "CodeableConcept", "Indicates if the immunization event should \"count\" against the protocol.", 0, 1, doseStatus)); 1383 children.add(new Property("doseStatusReason", "CodeableConcept", "Provides an explanation as to why an immunization event should or should not count against the protocol.", 0, 1, doseStatusReason)); 1384 } 1385 1386 @Override 1387 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1388 switch (_hash) { 1389 case 550933246: /*doseSequence*/ return new Property("doseSequence", "positiveInt", "Nominal position in a series.", 0, 1, doseSequence); 1390 case -1724546052: /*description*/ return new Property("description", "string", "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description); 1391 case 1475610435: /*authority*/ return new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol. E.g. ACIP.", 0, 1, authority); 1392 case -905838985: /*series*/ return new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series); 1393 case -1936727105: /*seriesDoses*/ return new Property("seriesDoses", "positiveInt", "The recommended number of doses to achieve immunity.", 0, 1, seriesDoses); 1394 case -319593813: /*targetDisease*/ return new Property("targetDisease", "CodeableConcept", "The targeted disease.", 0, java.lang.Integer.MAX_VALUE, targetDisease); 1395 case -745826705: /*doseStatus*/ return new Property("doseStatus", "CodeableConcept", "Indicates if the immunization event should \"count\" against the protocol.", 0, 1, doseStatus); 1396 case 662783379: /*doseStatusReason*/ return new Property("doseStatusReason", "CodeableConcept", "Provides an explanation as to why an immunization event should or should not count against the protocol.", 0, 1, doseStatusReason); 1397 default: return super.getNamedProperty(_hash, _name, _checkValid); 1398 } 1399 1400 } 1401 1402 @Override 1403 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1404 switch (hash) { 1405 case 550933246: /*doseSequence*/ return this.doseSequence == null ? new Base[0] : new Base[] {this.doseSequence}; // PositiveIntType 1406 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1407 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // Reference 1408 case -905838985: /*series*/ return this.series == null ? new Base[0] : new Base[] {this.series}; // StringType 1409 case -1936727105: /*seriesDoses*/ return this.seriesDoses == null ? new Base[0] : new Base[] {this.seriesDoses}; // PositiveIntType 1410 case -319593813: /*targetDisease*/ return this.targetDisease == null ? new Base[0] : this.targetDisease.toArray(new Base[this.targetDisease.size()]); // CodeableConcept 1411 case -745826705: /*doseStatus*/ return this.doseStatus == null ? new Base[0] : new Base[] {this.doseStatus}; // CodeableConcept 1412 case 662783379: /*doseStatusReason*/ return this.doseStatusReason == null ? new Base[0] : new Base[] {this.doseStatusReason}; // CodeableConcept 1413 default: return super.getProperty(hash, name, checkValid); 1414 } 1415 1416 } 1417 1418 @Override 1419 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1420 switch (hash) { 1421 case 550933246: // doseSequence 1422 this.doseSequence = castToPositiveInt(value); // PositiveIntType 1423 return value; 1424 case -1724546052: // description 1425 this.description = castToString(value); // StringType 1426 return value; 1427 case 1475610435: // authority 1428 this.authority = castToReference(value); // Reference 1429 return value; 1430 case -905838985: // series 1431 this.series = castToString(value); // StringType 1432 return value; 1433 case -1936727105: // seriesDoses 1434 this.seriesDoses = castToPositiveInt(value); // PositiveIntType 1435 return value; 1436 case -319593813: // targetDisease 1437 this.getTargetDisease().add(castToCodeableConcept(value)); // CodeableConcept 1438 return value; 1439 case -745826705: // doseStatus 1440 this.doseStatus = castToCodeableConcept(value); // CodeableConcept 1441 return value; 1442 case 662783379: // doseStatusReason 1443 this.doseStatusReason = castToCodeableConcept(value); // CodeableConcept 1444 return value; 1445 default: return super.setProperty(hash, name, value); 1446 } 1447 1448 } 1449 1450 @Override 1451 public Base setProperty(String name, Base value) throws FHIRException { 1452 if (name.equals("doseSequence")) { 1453 this.doseSequence = castToPositiveInt(value); // PositiveIntType 1454 } else if (name.equals("description")) { 1455 this.description = castToString(value); // StringType 1456 } else if (name.equals("authority")) { 1457 this.authority = castToReference(value); // Reference 1458 } else if (name.equals("series")) { 1459 this.series = castToString(value); // StringType 1460 } else if (name.equals("seriesDoses")) { 1461 this.seriesDoses = castToPositiveInt(value); // PositiveIntType 1462 } else if (name.equals("targetDisease")) { 1463 this.getTargetDisease().add(castToCodeableConcept(value)); 1464 } else if (name.equals("doseStatus")) { 1465 this.doseStatus = castToCodeableConcept(value); // CodeableConcept 1466 } else if (name.equals("doseStatusReason")) { 1467 this.doseStatusReason = castToCodeableConcept(value); // CodeableConcept 1468 } else 1469 return super.setProperty(name, value); 1470 return value; 1471 } 1472 1473 @Override 1474 public Base makeProperty(int hash, String name) throws FHIRException { 1475 switch (hash) { 1476 case 550933246: return getDoseSequenceElement(); 1477 case -1724546052: return getDescriptionElement(); 1478 case 1475610435: return getAuthority(); 1479 case -905838985: return getSeriesElement(); 1480 case -1936727105: return getSeriesDosesElement(); 1481 case -319593813: return addTargetDisease(); 1482 case -745826705: return getDoseStatus(); 1483 case 662783379: return getDoseStatusReason(); 1484 default: return super.makeProperty(hash, name); 1485 } 1486 1487 } 1488 1489 @Override 1490 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1491 switch (hash) { 1492 case 550933246: /*doseSequence*/ return new String[] {"positiveInt"}; 1493 case -1724546052: /*description*/ return new String[] {"string"}; 1494 case 1475610435: /*authority*/ return new String[] {"Reference"}; 1495 case -905838985: /*series*/ return new String[] {"string"}; 1496 case -1936727105: /*seriesDoses*/ return new String[] {"positiveInt"}; 1497 case -319593813: /*targetDisease*/ return new String[] {"CodeableConcept"}; 1498 case -745826705: /*doseStatus*/ return new String[] {"CodeableConcept"}; 1499 case 662783379: /*doseStatusReason*/ return new String[] {"CodeableConcept"}; 1500 default: return super.getTypesForProperty(hash, name); 1501 } 1502 1503 } 1504 1505 @Override 1506 public Base addChild(String name) throws FHIRException { 1507 if (name.equals("doseSequence")) { 1508 throw new FHIRException("Cannot call addChild on a singleton property Immunization.doseSequence"); 1509 } 1510 else if (name.equals("description")) { 1511 throw new FHIRException("Cannot call addChild on a singleton property Immunization.description"); 1512 } 1513 else if (name.equals("authority")) { 1514 this.authority = new Reference(); 1515 return this.authority; 1516 } 1517 else if (name.equals("series")) { 1518 throw new FHIRException("Cannot call addChild on a singleton property Immunization.series"); 1519 } 1520 else if (name.equals("seriesDoses")) { 1521 throw new FHIRException("Cannot call addChild on a singleton property Immunization.seriesDoses"); 1522 } 1523 else if (name.equals("targetDisease")) { 1524 return addTargetDisease(); 1525 } 1526 else if (name.equals("doseStatus")) { 1527 this.doseStatus = new CodeableConcept(); 1528 return this.doseStatus; 1529 } 1530 else if (name.equals("doseStatusReason")) { 1531 this.doseStatusReason = new CodeableConcept(); 1532 return this.doseStatusReason; 1533 } 1534 else 1535 return super.addChild(name); 1536 } 1537 1538 public ImmunizationVaccinationProtocolComponent copy() { 1539 ImmunizationVaccinationProtocolComponent dst = new ImmunizationVaccinationProtocolComponent(); 1540 copyValues(dst); 1541 dst.doseSequence = doseSequence == null ? null : doseSequence.copy(); 1542 dst.description = description == null ? null : description.copy(); 1543 dst.authority = authority == null ? null : authority.copy(); 1544 dst.series = series == null ? null : series.copy(); 1545 dst.seriesDoses = seriesDoses == null ? null : seriesDoses.copy(); 1546 if (targetDisease != null) { 1547 dst.targetDisease = new ArrayList<CodeableConcept>(); 1548 for (CodeableConcept i : targetDisease) 1549 dst.targetDisease.add(i.copy()); 1550 }; 1551 dst.doseStatus = doseStatus == null ? null : doseStatus.copy(); 1552 dst.doseStatusReason = doseStatusReason == null ? null : doseStatusReason.copy(); 1553 return dst; 1554 } 1555 1556 @Override 1557 public boolean equalsDeep(Base other_) { 1558 if (!super.equalsDeep(other_)) 1559 return false; 1560 if (!(other_ instanceof ImmunizationVaccinationProtocolComponent)) 1561 return false; 1562 ImmunizationVaccinationProtocolComponent o = (ImmunizationVaccinationProtocolComponent) other_; 1563 return compareDeep(doseSequence, o.doseSequence, true) && compareDeep(description, o.description, true) 1564 && compareDeep(authority, o.authority, true) && compareDeep(series, o.series, true) && compareDeep(seriesDoses, o.seriesDoses, true) 1565 && compareDeep(targetDisease, o.targetDisease, true) && compareDeep(doseStatus, o.doseStatus, true) 1566 && compareDeep(doseStatusReason, o.doseStatusReason, true); 1567 } 1568 1569 @Override 1570 public boolean equalsShallow(Base other_) { 1571 if (!super.equalsShallow(other_)) 1572 return false; 1573 if (!(other_ instanceof ImmunizationVaccinationProtocolComponent)) 1574 return false; 1575 ImmunizationVaccinationProtocolComponent o = (ImmunizationVaccinationProtocolComponent) other_; 1576 return compareValues(doseSequence, o.doseSequence, true) && compareValues(description, o.description, true) 1577 && compareValues(series, o.series, true) && compareValues(seriesDoses, o.seriesDoses, true); 1578 } 1579 1580 public boolean isEmpty() { 1581 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(doseSequence, description 1582 , authority, series, seriesDoses, targetDisease, doseStatus, doseStatusReason); 1583 } 1584 1585 public String fhirType() { 1586 return "Immunization.vaccinationProtocol"; 1587 1588 } 1589 1590 } 1591 1592 /** 1593 * A unique identifier assigned to this immunization record. 1594 */ 1595 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1596 @Description(shortDefinition="Business identifier", formalDefinition="A unique identifier assigned to this immunization record." ) 1597 protected List<Identifier> identifier; 1598 1599 /** 1600 * Indicates the current status of the vaccination event. 1601 */ 1602 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1603 @Description(shortDefinition="completed | entered-in-error", formalDefinition="Indicates the current status of the vaccination event." ) 1604 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-status") 1605 protected Enumeration<ImmunizationStatus> status; 1606 1607 /** 1608 * Indicates if the vaccination was or was not given. 1609 */ 1610 @Child(name = "notGiven", type = {BooleanType.class}, order=2, min=1, max=1, modifier=true, summary=true) 1611 @Description(shortDefinition="Flag for whether immunization was given", formalDefinition="Indicates if the vaccination was or was not given." ) 1612 protected BooleanType notGiven; 1613 1614 /** 1615 * Vaccine that was administered or was to be administered. 1616 */ 1617 @Child(name = "vaccineCode", type = {CodeableConcept.class}, order=3, min=1, max=1, modifier=false, summary=false) 1618 @Description(shortDefinition="Vaccine product administered", formalDefinition="Vaccine that was administered or was to be administered." ) 1619 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccine-code") 1620 protected CodeableConcept vaccineCode; 1621 1622 /** 1623 * The patient who either received or did not receive the immunization. 1624 */ 1625 @Child(name = "patient", type = {Patient.class}, order=4, min=1, max=1, modifier=false, summary=false) 1626 @Description(shortDefinition="Who was immunized", formalDefinition="The patient who either received or did not receive the immunization." ) 1627 protected Reference patient; 1628 1629 /** 1630 * The actual object that is the target of the reference (The patient who either received or did not receive the immunization.) 1631 */ 1632 protected Patient patientTarget; 1633 1634 /** 1635 * The visit or admission or other contact between patient and health care provider the immunization was performed as part of. 1636 */ 1637 @Child(name = "encounter", type = {Encounter.class}, order=5, min=0, max=1, modifier=false, summary=false) 1638 @Description(shortDefinition="Encounter administered as part of", formalDefinition="The visit or admission or other contact between patient and health care provider the immunization was performed as part of." ) 1639 protected Reference encounter; 1640 1641 /** 1642 * The actual object that is the target of the reference (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 1643 */ 1644 protected Encounter encounterTarget; 1645 1646 /** 1647 * Date vaccine administered or was to be administered. 1648 */ 1649 @Child(name = "date", type = {DateTimeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1650 @Description(shortDefinition="Vaccination administration date", formalDefinition="Date vaccine administered or was to be administered." ) 1651 protected DateTimeType date; 1652 1653 /** 1654 * An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded. 1655 */ 1656 @Child(name = "primarySource", type = {BooleanType.class}, order=7, min=1, max=1, modifier=false, summary=false) 1657 @Description(shortDefinition="Indicates context the data was recorded in", formalDefinition="An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded." ) 1658 protected BooleanType primarySource; 1659 1660 /** 1661 * The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine. 1662 */ 1663 @Child(name = "reportOrigin", type = {CodeableConcept.class}, order=8, min=0, max=1, modifier=false, summary=false) 1664 @Description(shortDefinition="Indicates the source of a secondarily reported record", formalDefinition="The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine." ) 1665 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-origin") 1666 protected CodeableConcept reportOrigin; 1667 1668 /** 1669 * The service delivery location where the vaccine administration occurred. 1670 */ 1671 @Child(name = "location", type = {Location.class}, order=9, min=0, max=1, modifier=false, summary=false) 1672 @Description(shortDefinition="Where vaccination occurred", formalDefinition="The service delivery location where the vaccine administration occurred." ) 1673 protected Reference location; 1674 1675 /** 1676 * The actual object that is the target of the reference (The service delivery location where the vaccine administration occurred.) 1677 */ 1678 protected Location locationTarget; 1679 1680 /** 1681 * Name of vaccine manufacturer. 1682 */ 1683 @Child(name = "manufacturer", type = {Organization.class}, order=10, min=0, max=1, modifier=false, summary=false) 1684 @Description(shortDefinition="Vaccine manufacturer", formalDefinition="Name of vaccine manufacturer." ) 1685 protected Reference manufacturer; 1686 1687 /** 1688 * The actual object that is the target of the reference (Name of vaccine manufacturer.) 1689 */ 1690 protected Organization manufacturerTarget; 1691 1692 /** 1693 * Lot number of the vaccine product. 1694 */ 1695 @Child(name = "lotNumber", type = {StringType.class}, order=11, min=0, max=1, modifier=false, summary=false) 1696 @Description(shortDefinition="Vaccine lot number", formalDefinition="Lot number of the vaccine product." ) 1697 protected StringType lotNumber; 1698 1699 /** 1700 * Date vaccine batch expires. 1701 */ 1702 @Child(name = "expirationDate", type = {DateType.class}, order=12, min=0, max=1, modifier=false, summary=false) 1703 @Description(shortDefinition="Vaccine expiration date", formalDefinition="Date vaccine batch expires." ) 1704 protected DateType expirationDate; 1705 1706 /** 1707 * Body site where vaccine was administered. 1708 */ 1709 @Child(name = "site", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=false) 1710 @Description(shortDefinition="Body site vaccine was administered", formalDefinition="Body site where vaccine was administered." ) 1711 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-site") 1712 protected CodeableConcept site; 1713 1714 /** 1715 * The path by which the vaccine product is taken into the body. 1716 */ 1717 @Child(name = "route", type = {CodeableConcept.class}, order=14, min=0, max=1, modifier=false, summary=false) 1718 @Description(shortDefinition="How vaccine entered body", formalDefinition="The path by which the vaccine product is taken into the body." ) 1719 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-route") 1720 protected CodeableConcept route; 1721 1722 /** 1723 * The quantity of vaccine product that was administered. 1724 */ 1725 @Child(name = "doseQuantity", type = {SimpleQuantity.class}, order=15, min=0, max=1, modifier=false, summary=false) 1726 @Description(shortDefinition="Amount of vaccine administered", formalDefinition="The quantity of vaccine product that was administered." ) 1727 protected SimpleQuantity doseQuantity; 1728 1729 /** 1730 * Indicates who or what performed the event. 1731 */ 1732 @Child(name = "practitioner", type = {}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1733 @Description(shortDefinition="Who performed event", formalDefinition="Indicates who or what performed the event." ) 1734 protected List<ImmunizationPractitionerComponent> practitioner; 1735 1736 /** 1737 * Extra information about the immunization that is not conveyed by the other attributes. 1738 */ 1739 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1740 @Description(shortDefinition="Vaccination notes", formalDefinition="Extra information about the immunization that is not conveyed by the other attributes." ) 1741 protected List<Annotation> note; 1742 1743 /** 1744 * Reasons why a vaccine was or was not administered. 1745 */ 1746 @Child(name = "explanation", type = {}, order=18, min=0, max=1, modifier=false, summary=false) 1747 @Description(shortDefinition="Administration/non-administration reasons", formalDefinition="Reasons why a vaccine was or was not administered." ) 1748 protected ImmunizationExplanationComponent explanation; 1749 1750 /** 1751 * Categorical data indicating that an adverse event is associated in time to an immunization. 1752 */ 1753 @Child(name = "reaction", type = {}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1754 @Description(shortDefinition="Details of a reaction that follows immunization", formalDefinition="Categorical data indicating that an adverse event is associated in time to an immunization." ) 1755 protected List<ImmunizationReactionComponent> reaction; 1756 1757 /** 1758 * Contains information about the protocol(s) under which the vaccine was administered. 1759 */ 1760 @Child(name = "vaccinationProtocol", type = {}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1761 @Description(shortDefinition="What protocol was followed", formalDefinition="Contains information about the protocol(s) under which the vaccine was administered." ) 1762 protected List<ImmunizationVaccinationProtocolComponent> vaccinationProtocol; 1763 1764 private static final long serialVersionUID = 658058655L; 1765 1766 /** 1767 * Constructor 1768 */ 1769 public Immunization() { 1770 super(); 1771 } 1772 1773 /** 1774 * Constructor 1775 */ 1776 public Immunization(Enumeration<ImmunizationStatus> status, BooleanType notGiven, CodeableConcept vaccineCode, Reference patient, BooleanType primarySource) { 1777 super(); 1778 this.status = status; 1779 this.notGiven = notGiven; 1780 this.vaccineCode = vaccineCode; 1781 this.patient = patient; 1782 this.primarySource = primarySource; 1783 } 1784 1785 /** 1786 * @return {@link #identifier} (A unique identifier assigned to this immunization record.) 1787 */ 1788 public List<Identifier> getIdentifier() { 1789 if (this.identifier == null) 1790 this.identifier = new ArrayList<Identifier>(); 1791 return this.identifier; 1792 } 1793 1794 /** 1795 * @return Returns a reference to <code>this</code> for easy method chaining 1796 */ 1797 public Immunization setIdentifier(List<Identifier> theIdentifier) { 1798 this.identifier = theIdentifier; 1799 return this; 1800 } 1801 1802 public boolean hasIdentifier() { 1803 if (this.identifier == null) 1804 return false; 1805 for (Identifier item : this.identifier) 1806 if (!item.isEmpty()) 1807 return true; 1808 return false; 1809 } 1810 1811 public Identifier addIdentifier() { //3 1812 Identifier t = new Identifier(); 1813 if (this.identifier == null) 1814 this.identifier = new ArrayList<Identifier>(); 1815 this.identifier.add(t); 1816 return t; 1817 } 1818 1819 public Immunization addIdentifier(Identifier t) { //3 1820 if (t == null) 1821 return this; 1822 if (this.identifier == null) 1823 this.identifier = new ArrayList<Identifier>(); 1824 this.identifier.add(t); 1825 return this; 1826 } 1827 1828 /** 1829 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1830 */ 1831 public Identifier getIdentifierFirstRep() { 1832 if (getIdentifier().isEmpty()) { 1833 addIdentifier(); 1834 } 1835 return getIdentifier().get(0); 1836 } 1837 1838 /** 1839 * @return {@link #status} (Indicates the current status of the vaccination event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1840 */ 1841 public Enumeration<ImmunizationStatus> getStatusElement() { 1842 if (this.status == null) 1843 if (Configuration.errorOnAutoCreate()) 1844 throw new Error("Attempt to auto-create Immunization.status"); 1845 else if (Configuration.doAutoCreate()) 1846 this.status = new Enumeration<ImmunizationStatus>(new ImmunizationStatusEnumFactory()); // bb 1847 return this.status; 1848 } 1849 1850 public boolean hasStatusElement() { 1851 return this.status != null && !this.status.isEmpty(); 1852 } 1853 1854 public boolean hasStatus() { 1855 return this.status != null && !this.status.isEmpty(); 1856 } 1857 1858 /** 1859 * @param value {@link #status} (Indicates the current status of the vaccination event.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1860 */ 1861 public Immunization setStatusElement(Enumeration<ImmunizationStatus> value) { 1862 this.status = value; 1863 return this; 1864 } 1865 1866 /** 1867 * @return Indicates the current status of the vaccination event. 1868 */ 1869 public ImmunizationStatus getStatus() { 1870 return this.status == null ? null : this.status.getValue(); 1871 } 1872 1873 /** 1874 * @param value Indicates the current status of the vaccination event. 1875 */ 1876 public Immunization setStatus(ImmunizationStatus value) { 1877 if (this.status == null) 1878 this.status = new Enumeration<ImmunizationStatus>(new ImmunizationStatusEnumFactory()); 1879 this.status.setValue(value); 1880 return this; 1881 } 1882 1883 /** 1884 * @return {@link #notGiven} (Indicates if the vaccination was or was not given.). This is the underlying object with id, value and extensions. The accessor "getNotGiven" gives direct access to the value 1885 */ 1886 public BooleanType getNotGivenElement() { 1887 if (this.notGiven == null) 1888 if (Configuration.errorOnAutoCreate()) 1889 throw new Error("Attempt to auto-create Immunization.notGiven"); 1890 else if (Configuration.doAutoCreate()) 1891 this.notGiven = new BooleanType(); // bb 1892 return this.notGiven; 1893 } 1894 1895 public boolean hasNotGivenElement() { 1896 return this.notGiven != null && !this.notGiven.isEmpty(); 1897 } 1898 1899 public boolean hasNotGiven() { 1900 return this.notGiven != null && !this.notGiven.isEmpty(); 1901 } 1902 1903 /** 1904 * @param value {@link #notGiven} (Indicates if the vaccination was or was not given.). This is the underlying object with id, value and extensions. The accessor "getNotGiven" gives direct access to the value 1905 */ 1906 public Immunization setNotGivenElement(BooleanType value) { 1907 this.notGiven = value; 1908 return this; 1909 } 1910 1911 /** 1912 * @return Indicates if the vaccination was or was not given. 1913 */ 1914 public boolean getNotGiven() { 1915 return this.notGiven == null || this.notGiven.isEmpty() ? false : this.notGiven.getValue(); 1916 } 1917 1918 /** 1919 * @param value Indicates if the vaccination was or was not given. 1920 */ 1921 public Immunization setNotGiven(boolean value) { 1922 if (this.notGiven == null) 1923 this.notGiven = new BooleanType(); 1924 this.notGiven.setValue(value); 1925 return this; 1926 } 1927 1928 /** 1929 * @return {@link #vaccineCode} (Vaccine that was administered or was to be administered.) 1930 */ 1931 public CodeableConcept getVaccineCode() { 1932 if (this.vaccineCode == null) 1933 if (Configuration.errorOnAutoCreate()) 1934 throw new Error("Attempt to auto-create Immunization.vaccineCode"); 1935 else if (Configuration.doAutoCreate()) 1936 this.vaccineCode = new CodeableConcept(); // cc 1937 return this.vaccineCode; 1938 } 1939 1940 public boolean hasVaccineCode() { 1941 return this.vaccineCode != null && !this.vaccineCode.isEmpty(); 1942 } 1943 1944 /** 1945 * @param value {@link #vaccineCode} (Vaccine that was administered or was to be administered.) 1946 */ 1947 public Immunization setVaccineCode(CodeableConcept value) { 1948 this.vaccineCode = value; 1949 return this; 1950 } 1951 1952 /** 1953 * @return {@link #patient} (The patient who either received or did not receive the immunization.) 1954 */ 1955 public Reference getPatient() { 1956 if (this.patient == null) 1957 if (Configuration.errorOnAutoCreate()) 1958 throw new Error("Attempt to auto-create Immunization.patient"); 1959 else if (Configuration.doAutoCreate()) 1960 this.patient = new Reference(); // cc 1961 return this.patient; 1962 } 1963 1964 public boolean hasPatient() { 1965 return this.patient != null && !this.patient.isEmpty(); 1966 } 1967 1968 /** 1969 * @param value {@link #patient} (The patient who either received or did not receive the immunization.) 1970 */ 1971 public Immunization setPatient(Reference value) { 1972 this.patient = value; 1973 return this; 1974 } 1975 1976 /** 1977 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient who either received or did not receive the immunization.) 1978 */ 1979 public Patient getPatientTarget() { 1980 if (this.patientTarget == null) 1981 if (Configuration.errorOnAutoCreate()) 1982 throw new Error("Attempt to auto-create Immunization.patient"); 1983 else if (Configuration.doAutoCreate()) 1984 this.patientTarget = new Patient(); // aa 1985 return this.patientTarget; 1986 } 1987 1988 /** 1989 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient who either received or did not receive the immunization.) 1990 */ 1991 public Immunization setPatientTarget(Patient value) { 1992 this.patientTarget = value; 1993 return this; 1994 } 1995 1996 /** 1997 * @return {@link #encounter} (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 1998 */ 1999 public Reference getEncounter() { 2000 if (this.encounter == null) 2001 if (Configuration.errorOnAutoCreate()) 2002 throw new Error("Attempt to auto-create Immunization.encounter"); 2003 else if (Configuration.doAutoCreate()) 2004 this.encounter = new Reference(); // cc 2005 return this.encounter; 2006 } 2007 2008 public boolean hasEncounter() { 2009 return this.encounter != null && !this.encounter.isEmpty(); 2010 } 2011 2012 /** 2013 * @param value {@link #encounter} (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 2014 */ 2015 public Immunization setEncounter(Reference value) { 2016 this.encounter = value; 2017 return this; 2018 } 2019 2020 /** 2021 * @return {@link #encounter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 2022 */ 2023 public Encounter getEncounterTarget() { 2024 if (this.encounterTarget == null) 2025 if (Configuration.errorOnAutoCreate()) 2026 throw new Error("Attempt to auto-create Immunization.encounter"); 2027 else if (Configuration.doAutoCreate()) 2028 this.encounterTarget = new Encounter(); // aa 2029 return this.encounterTarget; 2030 } 2031 2032 /** 2033 * @param value {@link #encounter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The visit or admission or other contact between patient and health care provider the immunization was performed as part of.) 2034 */ 2035 public Immunization setEncounterTarget(Encounter value) { 2036 this.encounterTarget = value; 2037 return this; 2038 } 2039 2040 /** 2041 * @return {@link #date} (Date vaccine administered or was to be administered.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2042 */ 2043 public DateTimeType getDateElement() { 2044 if (this.date == null) 2045 if (Configuration.errorOnAutoCreate()) 2046 throw new Error("Attempt to auto-create Immunization.date"); 2047 else if (Configuration.doAutoCreate()) 2048 this.date = new DateTimeType(); // bb 2049 return this.date; 2050 } 2051 2052 public boolean hasDateElement() { 2053 return this.date != null && !this.date.isEmpty(); 2054 } 2055 2056 public boolean hasDate() { 2057 return this.date != null && !this.date.isEmpty(); 2058 } 2059 2060 /** 2061 * @param value {@link #date} (Date vaccine administered or was to be administered.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2062 */ 2063 public Immunization setDateElement(DateTimeType value) { 2064 this.date = value; 2065 return this; 2066 } 2067 2068 /** 2069 * @return Date vaccine administered or was to be administered. 2070 */ 2071 public Date getDate() { 2072 return this.date == null ? null : this.date.getValue(); 2073 } 2074 2075 /** 2076 * @param value Date vaccine administered or was to be administered. 2077 */ 2078 public Immunization setDate(Date value) { 2079 if (value == null) 2080 this.date = null; 2081 else { 2082 if (this.date == null) 2083 this.date = new DateTimeType(); 2084 this.date.setValue(value); 2085 } 2086 return this; 2087 } 2088 2089 /** 2090 * @return {@link #primarySource} (An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.). This is the underlying object with id, value and extensions. The accessor "getPrimarySource" gives direct access to the value 2091 */ 2092 public BooleanType getPrimarySourceElement() { 2093 if (this.primarySource == null) 2094 if (Configuration.errorOnAutoCreate()) 2095 throw new Error("Attempt to auto-create Immunization.primarySource"); 2096 else if (Configuration.doAutoCreate()) 2097 this.primarySource = new BooleanType(); // bb 2098 return this.primarySource; 2099 } 2100 2101 public boolean hasPrimarySourceElement() { 2102 return this.primarySource != null && !this.primarySource.isEmpty(); 2103 } 2104 2105 public boolean hasPrimarySource() { 2106 return this.primarySource != null && !this.primarySource.isEmpty(); 2107 } 2108 2109 /** 2110 * @param value {@link #primarySource} (An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.). This is the underlying object with id, value and extensions. The accessor "getPrimarySource" gives direct access to the value 2111 */ 2112 public Immunization setPrimarySourceElement(BooleanType value) { 2113 this.primarySource = value; 2114 return this; 2115 } 2116 2117 /** 2118 * @return An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded. 2119 */ 2120 public boolean getPrimarySource() { 2121 return this.primarySource == null || this.primarySource.isEmpty() ? false : this.primarySource.getValue(); 2122 } 2123 2124 /** 2125 * @param value An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded. 2126 */ 2127 public Immunization setPrimarySource(boolean value) { 2128 if (this.primarySource == null) 2129 this.primarySource = new BooleanType(); 2130 this.primarySource.setValue(value); 2131 return this; 2132 } 2133 2134 /** 2135 * @return {@link #reportOrigin} (The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.) 2136 */ 2137 public CodeableConcept getReportOrigin() { 2138 if (this.reportOrigin == null) 2139 if (Configuration.errorOnAutoCreate()) 2140 throw new Error("Attempt to auto-create Immunization.reportOrigin"); 2141 else if (Configuration.doAutoCreate()) 2142 this.reportOrigin = new CodeableConcept(); // cc 2143 return this.reportOrigin; 2144 } 2145 2146 public boolean hasReportOrigin() { 2147 return this.reportOrigin != null && !this.reportOrigin.isEmpty(); 2148 } 2149 2150 /** 2151 * @param value {@link #reportOrigin} (The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.) 2152 */ 2153 public Immunization setReportOrigin(CodeableConcept value) { 2154 this.reportOrigin = value; 2155 return this; 2156 } 2157 2158 /** 2159 * @return {@link #location} (The service delivery location where the vaccine administration occurred.) 2160 */ 2161 public Reference getLocation() { 2162 if (this.location == null) 2163 if (Configuration.errorOnAutoCreate()) 2164 throw new Error("Attempt to auto-create Immunization.location"); 2165 else if (Configuration.doAutoCreate()) 2166 this.location = new Reference(); // cc 2167 return this.location; 2168 } 2169 2170 public boolean hasLocation() { 2171 return this.location != null && !this.location.isEmpty(); 2172 } 2173 2174 /** 2175 * @param value {@link #location} (The service delivery location where the vaccine administration occurred.) 2176 */ 2177 public Immunization setLocation(Reference value) { 2178 this.location = value; 2179 return this; 2180 } 2181 2182 /** 2183 * @return {@link #location} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The service delivery location where the vaccine administration occurred.) 2184 */ 2185 public Location getLocationTarget() { 2186 if (this.locationTarget == null) 2187 if (Configuration.errorOnAutoCreate()) 2188 throw new Error("Attempt to auto-create Immunization.location"); 2189 else if (Configuration.doAutoCreate()) 2190 this.locationTarget = new Location(); // aa 2191 return this.locationTarget; 2192 } 2193 2194 /** 2195 * @param value {@link #location} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The service delivery location where the vaccine administration occurred.) 2196 */ 2197 public Immunization setLocationTarget(Location value) { 2198 this.locationTarget = value; 2199 return this; 2200 } 2201 2202 /** 2203 * @return {@link #manufacturer} (Name of vaccine manufacturer.) 2204 */ 2205 public Reference getManufacturer() { 2206 if (this.manufacturer == null) 2207 if (Configuration.errorOnAutoCreate()) 2208 throw new Error("Attempt to auto-create Immunization.manufacturer"); 2209 else if (Configuration.doAutoCreate()) 2210 this.manufacturer = new Reference(); // cc 2211 return this.manufacturer; 2212 } 2213 2214 public boolean hasManufacturer() { 2215 return this.manufacturer != null && !this.manufacturer.isEmpty(); 2216 } 2217 2218 /** 2219 * @param value {@link #manufacturer} (Name of vaccine manufacturer.) 2220 */ 2221 public Immunization setManufacturer(Reference value) { 2222 this.manufacturer = value; 2223 return this; 2224 } 2225 2226 /** 2227 * @return {@link #manufacturer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Name of vaccine manufacturer.) 2228 */ 2229 public Organization getManufacturerTarget() { 2230 if (this.manufacturerTarget == null) 2231 if (Configuration.errorOnAutoCreate()) 2232 throw new Error("Attempt to auto-create Immunization.manufacturer"); 2233 else if (Configuration.doAutoCreate()) 2234 this.manufacturerTarget = new Organization(); // aa 2235 return this.manufacturerTarget; 2236 } 2237 2238 /** 2239 * @param value {@link #manufacturer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Name of vaccine manufacturer.) 2240 */ 2241 public Immunization setManufacturerTarget(Organization value) { 2242 this.manufacturerTarget = value; 2243 return this; 2244 } 2245 2246 /** 2247 * @return {@link #lotNumber} (Lot number of the vaccine product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 2248 */ 2249 public StringType getLotNumberElement() { 2250 if (this.lotNumber == null) 2251 if (Configuration.errorOnAutoCreate()) 2252 throw new Error("Attempt to auto-create Immunization.lotNumber"); 2253 else if (Configuration.doAutoCreate()) 2254 this.lotNumber = new StringType(); // bb 2255 return this.lotNumber; 2256 } 2257 2258 public boolean hasLotNumberElement() { 2259 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2260 } 2261 2262 public boolean hasLotNumber() { 2263 return this.lotNumber != null && !this.lotNumber.isEmpty(); 2264 } 2265 2266 /** 2267 * @param value {@link #lotNumber} (Lot number of the vaccine product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 2268 */ 2269 public Immunization setLotNumberElement(StringType value) { 2270 this.lotNumber = value; 2271 return this; 2272 } 2273 2274 /** 2275 * @return Lot number of the vaccine product. 2276 */ 2277 public String getLotNumber() { 2278 return this.lotNumber == null ? null : this.lotNumber.getValue(); 2279 } 2280 2281 /** 2282 * @param value Lot number of the vaccine product. 2283 */ 2284 public Immunization setLotNumber(String value) { 2285 if (Utilities.noString(value)) 2286 this.lotNumber = null; 2287 else { 2288 if (this.lotNumber == null) 2289 this.lotNumber = new StringType(); 2290 this.lotNumber.setValue(value); 2291 } 2292 return this; 2293 } 2294 2295 /** 2296 * @return {@link #expirationDate} (Date vaccine batch expires.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2297 */ 2298 public DateType getExpirationDateElement() { 2299 if (this.expirationDate == null) 2300 if (Configuration.errorOnAutoCreate()) 2301 throw new Error("Attempt to auto-create Immunization.expirationDate"); 2302 else if (Configuration.doAutoCreate()) 2303 this.expirationDate = new DateType(); // bb 2304 return this.expirationDate; 2305 } 2306 2307 public boolean hasExpirationDateElement() { 2308 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2309 } 2310 2311 public boolean hasExpirationDate() { 2312 return this.expirationDate != null && !this.expirationDate.isEmpty(); 2313 } 2314 2315 /** 2316 * @param value {@link #expirationDate} (Date vaccine batch expires.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 2317 */ 2318 public Immunization setExpirationDateElement(DateType value) { 2319 this.expirationDate = value; 2320 return this; 2321 } 2322 2323 /** 2324 * @return Date vaccine batch expires. 2325 */ 2326 public Date getExpirationDate() { 2327 return this.expirationDate == null ? null : this.expirationDate.getValue(); 2328 } 2329 2330 /** 2331 * @param value Date vaccine batch expires. 2332 */ 2333 public Immunization setExpirationDate(Date value) { 2334 if (value == null) 2335 this.expirationDate = null; 2336 else { 2337 if (this.expirationDate == null) 2338 this.expirationDate = new DateType(); 2339 this.expirationDate.setValue(value); 2340 } 2341 return this; 2342 } 2343 2344 /** 2345 * @return {@link #site} (Body site where vaccine was administered.) 2346 */ 2347 public CodeableConcept getSite() { 2348 if (this.site == null) 2349 if (Configuration.errorOnAutoCreate()) 2350 throw new Error("Attempt to auto-create Immunization.site"); 2351 else if (Configuration.doAutoCreate()) 2352 this.site = new CodeableConcept(); // cc 2353 return this.site; 2354 } 2355 2356 public boolean hasSite() { 2357 return this.site != null && !this.site.isEmpty(); 2358 } 2359 2360 /** 2361 * @param value {@link #site} (Body site where vaccine was administered.) 2362 */ 2363 public Immunization setSite(CodeableConcept value) { 2364 this.site = value; 2365 return this; 2366 } 2367 2368 /** 2369 * @return {@link #route} (The path by which the vaccine product is taken into the body.) 2370 */ 2371 public CodeableConcept getRoute() { 2372 if (this.route == null) 2373 if (Configuration.errorOnAutoCreate()) 2374 throw new Error("Attempt to auto-create Immunization.route"); 2375 else if (Configuration.doAutoCreate()) 2376 this.route = new CodeableConcept(); // cc 2377 return this.route; 2378 } 2379 2380 public boolean hasRoute() { 2381 return this.route != null && !this.route.isEmpty(); 2382 } 2383 2384 /** 2385 * @param value {@link #route} (The path by which the vaccine product is taken into the body.) 2386 */ 2387 public Immunization setRoute(CodeableConcept value) { 2388 this.route = value; 2389 return this; 2390 } 2391 2392 /** 2393 * @return {@link #doseQuantity} (The quantity of vaccine product that was administered.) 2394 */ 2395 public SimpleQuantity getDoseQuantity() { 2396 if (this.doseQuantity == null) 2397 if (Configuration.errorOnAutoCreate()) 2398 throw new Error("Attempt to auto-create Immunization.doseQuantity"); 2399 else if (Configuration.doAutoCreate()) 2400 this.doseQuantity = new SimpleQuantity(); // cc 2401 return this.doseQuantity; 2402 } 2403 2404 public boolean hasDoseQuantity() { 2405 return this.doseQuantity != null && !this.doseQuantity.isEmpty(); 2406 } 2407 2408 /** 2409 * @param value {@link #doseQuantity} (The quantity of vaccine product that was administered.) 2410 */ 2411 public Immunization setDoseQuantity(SimpleQuantity value) { 2412 this.doseQuantity = value; 2413 return this; 2414 } 2415 2416 /** 2417 * @return {@link #practitioner} (Indicates who or what performed the event.) 2418 */ 2419 public List<ImmunizationPractitionerComponent> getPractitioner() { 2420 if (this.practitioner == null) 2421 this.practitioner = new ArrayList<ImmunizationPractitionerComponent>(); 2422 return this.practitioner; 2423 } 2424 2425 /** 2426 * @return Returns a reference to <code>this</code> for easy method chaining 2427 */ 2428 public Immunization setPractitioner(List<ImmunizationPractitionerComponent> thePractitioner) { 2429 this.practitioner = thePractitioner; 2430 return this; 2431 } 2432 2433 public boolean hasPractitioner() { 2434 if (this.practitioner == null) 2435 return false; 2436 for (ImmunizationPractitionerComponent item : this.practitioner) 2437 if (!item.isEmpty()) 2438 return true; 2439 return false; 2440 } 2441 2442 public ImmunizationPractitionerComponent addPractitioner() { //3 2443 ImmunizationPractitionerComponent t = new ImmunizationPractitionerComponent(); 2444 if (this.practitioner == null) 2445 this.practitioner = new ArrayList<ImmunizationPractitionerComponent>(); 2446 this.practitioner.add(t); 2447 return t; 2448 } 2449 2450 public Immunization addPractitioner(ImmunizationPractitionerComponent t) { //3 2451 if (t == null) 2452 return this; 2453 if (this.practitioner == null) 2454 this.practitioner = new ArrayList<ImmunizationPractitionerComponent>(); 2455 this.practitioner.add(t); 2456 return this; 2457 } 2458 2459 /** 2460 * @return The first repetition of repeating field {@link #practitioner}, creating it if it does not already exist 2461 */ 2462 public ImmunizationPractitionerComponent getPractitionerFirstRep() { 2463 if (getPractitioner().isEmpty()) { 2464 addPractitioner(); 2465 } 2466 return getPractitioner().get(0); 2467 } 2468 2469 /** 2470 * @return {@link #note} (Extra information about the immunization that is not conveyed by the other attributes.) 2471 */ 2472 public List<Annotation> getNote() { 2473 if (this.note == null) 2474 this.note = new ArrayList<Annotation>(); 2475 return this.note; 2476 } 2477 2478 /** 2479 * @return Returns a reference to <code>this</code> for easy method chaining 2480 */ 2481 public Immunization setNote(List<Annotation> theNote) { 2482 this.note = theNote; 2483 return this; 2484 } 2485 2486 public boolean hasNote() { 2487 if (this.note == null) 2488 return false; 2489 for (Annotation item : this.note) 2490 if (!item.isEmpty()) 2491 return true; 2492 return false; 2493 } 2494 2495 public Annotation addNote() { //3 2496 Annotation t = new Annotation(); 2497 if (this.note == null) 2498 this.note = new ArrayList<Annotation>(); 2499 this.note.add(t); 2500 return t; 2501 } 2502 2503 public Immunization addNote(Annotation t) { //3 2504 if (t == null) 2505 return this; 2506 if (this.note == null) 2507 this.note = new ArrayList<Annotation>(); 2508 this.note.add(t); 2509 return this; 2510 } 2511 2512 /** 2513 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2514 */ 2515 public Annotation getNoteFirstRep() { 2516 if (getNote().isEmpty()) { 2517 addNote(); 2518 } 2519 return getNote().get(0); 2520 } 2521 2522 /** 2523 * @return {@link #explanation} (Reasons why a vaccine was or was not administered.) 2524 */ 2525 public ImmunizationExplanationComponent getExplanation() { 2526 if (this.explanation == null) 2527 if (Configuration.errorOnAutoCreate()) 2528 throw new Error("Attempt to auto-create Immunization.explanation"); 2529 else if (Configuration.doAutoCreate()) 2530 this.explanation = new ImmunizationExplanationComponent(); // cc 2531 return this.explanation; 2532 } 2533 2534 public boolean hasExplanation() { 2535 return this.explanation != null && !this.explanation.isEmpty(); 2536 } 2537 2538 /** 2539 * @param value {@link #explanation} (Reasons why a vaccine was or was not administered.) 2540 */ 2541 public Immunization setExplanation(ImmunizationExplanationComponent value) { 2542 this.explanation = value; 2543 return this; 2544 } 2545 2546 /** 2547 * @return {@link #reaction} (Categorical data indicating that an adverse event is associated in time to an immunization.) 2548 */ 2549 public List<ImmunizationReactionComponent> getReaction() { 2550 if (this.reaction == null) 2551 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2552 return this.reaction; 2553 } 2554 2555 /** 2556 * @return Returns a reference to <code>this</code> for easy method chaining 2557 */ 2558 public Immunization setReaction(List<ImmunizationReactionComponent> theReaction) { 2559 this.reaction = theReaction; 2560 return this; 2561 } 2562 2563 public boolean hasReaction() { 2564 if (this.reaction == null) 2565 return false; 2566 for (ImmunizationReactionComponent item : this.reaction) 2567 if (!item.isEmpty()) 2568 return true; 2569 return false; 2570 } 2571 2572 public ImmunizationReactionComponent addReaction() { //3 2573 ImmunizationReactionComponent t = new ImmunizationReactionComponent(); 2574 if (this.reaction == null) 2575 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2576 this.reaction.add(t); 2577 return t; 2578 } 2579 2580 public Immunization addReaction(ImmunizationReactionComponent t) { //3 2581 if (t == null) 2582 return this; 2583 if (this.reaction == null) 2584 this.reaction = new ArrayList<ImmunizationReactionComponent>(); 2585 this.reaction.add(t); 2586 return this; 2587 } 2588 2589 /** 2590 * @return The first repetition of repeating field {@link #reaction}, creating it if it does not already exist 2591 */ 2592 public ImmunizationReactionComponent getReactionFirstRep() { 2593 if (getReaction().isEmpty()) { 2594 addReaction(); 2595 } 2596 return getReaction().get(0); 2597 } 2598 2599 /** 2600 * @return {@link #vaccinationProtocol} (Contains information about the protocol(s) under which the vaccine was administered.) 2601 */ 2602 public List<ImmunizationVaccinationProtocolComponent> getVaccinationProtocol() { 2603 if (this.vaccinationProtocol == null) 2604 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2605 return this.vaccinationProtocol; 2606 } 2607 2608 /** 2609 * @return Returns a reference to <code>this</code> for easy method chaining 2610 */ 2611 public Immunization setVaccinationProtocol(List<ImmunizationVaccinationProtocolComponent> theVaccinationProtocol) { 2612 this.vaccinationProtocol = theVaccinationProtocol; 2613 return this; 2614 } 2615 2616 public boolean hasVaccinationProtocol() { 2617 if (this.vaccinationProtocol == null) 2618 return false; 2619 for (ImmunizationVaccinationProtocolComponent item : this.vaccinationProtocol) 2620 if (!item.isEmpty()) 2621 return true; 2622 return false; 2623 } 2624 2625 public ImmunizationVaccinationProtocolComponent addVaccinationProtocol() { //3 2626 ImmunizationVaccinationProtocolComponent t = new ImmunizationVaccinationProtocolComponent(); 2627 if (this.vaccinationProtocol == null) 2628 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2629 this.vaccinationProtocol.add(t); 2630 return t; 2631 } 2632 2633 public Immunization addVaccinationProtocol(ImmunizationVaccinationProtocolComponent t) { //3 2634 if (t == null) 2635 return this; 2636 if (this.vaccinationProtocol == null) 2637 this.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 2638 this.vaccinationProtocol.add(t); 2639 return this; 2640 } 2641 2642 /** 2643 * @return The first repetition of repeating field {@link #vaccinationProtocol}, creating it if it does not already exist 2644 */ 2645 public ImmunizationVaccinationProtocolComponent getVaccinationProtocolFirstRep() { 2646 if (getVaccinationProtocol().isEmpty()) { 2647 addVaccinationProtocol(); 2648 } 2649 return getVaccinationProtocol().get(0); 2650 } 2651 2652 protected void listChildren(List<Property> children) { 2653 super.listChildren(children); 2654 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2655 children.add(new Property("status", "code", "Indicates the current status of the vaccination event.", 0, 1, status)); 2656 children.add(new Property("notGiven", "boolean", "Indicates if the vaccination was or was not given.", 0, 1, notGiven)); 2657 children.add(new Property("vaccineCode", "CodeableConcept", "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode)); 2658 children.add(new Property("patient", "Reference(Patient)", "The patient who either received or did not receive the immunization.", 0, 1, patient)); 2659 children.add(new Property("encounter", "Reference(Encounter)", "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 0, 1, encounter)); 2660 children.add(new Property("date", "dateTime", "Date vaccine administered or was to be administered.", 0, 1, date)); 2661 children.add(new Property("primarySource", "boolean", "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.", 0, 1, primarySource)); 2662 children.add(new Property("reportOrigin", "CodeableConcept", "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 0, 1, reportOrigin)); 2663 children.add(new Property("location", "Reference(Location)", "The service delivery location where the vaccine administration occurred.", 0, 1, location)); 2664 children.add(new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 0, 1, manufacturer)); 2665 children.add(new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, lotNumber)); 2666 children.add(new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, expirationDate)); 2667 children.add(new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, site)); 2668 children.add(new Property("route", "CodeableConcept", "The path by which the vaccine product is taken into the body.", 0, 1, route)); 2669 children.add(new Property("doseQuantity", "SimpleQuantity", "The quantity of vaccine product that was administered.", 0, 1, doseQuantity)); 2670 children.add(new Property("practitioner", "", "Indicates who or what performed the event.", 0, java.lang.Integer.MAX_VALUE, practitioner)); 2671 children.add(new Property("note", "Annotation", "Extra information about the immunization that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2672 children.add(new Property("explanation", "", "Reasons why a vaccine was or was not administered.", 0, 1, explanation)); 2673 children.add(new Property("reaction", "", "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, java.lang.Integer.MAX_VALUE, reaction)); 2674 children.add(new Property("vaccinationProtocol", "", "Contains information about the protocol(s) under which the vaccine was administered.", 0, java.lang.Integer.MAX_VALUE, vaccinationProtocol)); 2675 } 2676 2677 @Override 2678 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2679 switch (_hash) { 2680 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this immunization record.", 0, java.lang.Integer.MAX_VALUE, identifier); 2681 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current status of the vaccination event.", 0, 1, status); 2682 case 1554065514: /*notGiven*/ return new Property("notGiven", "boolean", "Indicates if the vaccination was or was not given.", 0, 1, notGiven); 2683 case 664556354: /*vaccineCode*/ return new Property("vaccineCode", "CodeableConcept", "Vaccine that was administered or was to be administered.", 0, 1, vaccineCode); 2684 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient who either received or did not receive the immunization.", 0, 1, patient); 2685 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The visit or admission or other contact between patient and health care provider the immunization was performed as part of.", 0, 1, encounter); 2686 case 3076014: /*date*/ return new Property("date", "dateTime", "Date vaccine administered or was to be administered.", 0, 1, date); 2687 case -528721731: /*primarySource*/ return new Property("primarySource", "boolean", "An indication that the content of the record is based on information from the person who administered the vaccine. This reflects the context under which the data was originally recorded.", 0, 1, primarySource); 2688 case 486750586: /*reportOrigin*/ return new Property("reportOrigin", "CodeableConcept", "The source of the data when the report of the immunization event is not based on information from the person who administered the vaccine.", 0, 1, reportOrigin); 2689 case 1901043637: /*location*/ return new Property("location", "Reference(Location)", "The service delivery location where the vaccine administration occurred.", 0, 1, location); 2690 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "Name of vaccine manufacturer.", 0, 1, manufacturer); 2691 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "Lot number of the vaccine product.", 0, 1, lotNumber); 2692 case -668811523: /*expirationDate*/ return new Property("expirationDate", "date", "Date vaccine batch expires.", 0, 1, expirationDate); 2693 case 3530567: /*site*/ return new Property("site", "CodeableConcept", "Body site where vaccine was administered.", 0, 1, site); 2694 case 108704329: /*route*/ return new Property("route", "CodeableConcept", "The path by which the vaccine product is taken into the body.", 0, 1, route); 2695 case -2083618872: /*doseQuantity*/ return new Property("doseQuantity", "SimpleQuantity", "The quantity of vaccine product that was administered.", 0, 1, doseQuantity); 2696 case 574573338: /*practitioner*/ return new Property("practitioner", "", "Indicates who or what performed the event.", 0, java.lang.Integer.MAX_VALUE, practitioner); 2697 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the immunization that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2698 case -1105867239: /*explanation*/ return new Property("explanation", "", "Reasons why a vaccine was or was not administered.", 0, 1, explanation); 2699 case -867509719: /*reaction*/ return new Property("reaction", "", "Categorical data indicating that an adverse event is associated in time to an immunization.", 0, java.lang.Integer.MAX_VALUE, reaction); 2700 case -179633155: /*vaccinationProtocol*/ return new Property("vaccinationProtocol", "", "Contains information about the protocol(s) under which the vaccine was administered.", 0, java.lang.Integer.MAX_VALUE, vaccinationProtocol); 2701 default: return super.getNamedProperty(_hash, _name, _checkValid); 2702 } 2703 2704 } 2705 2706 @Override 2707 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2708 switch (hash) { 2709 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2710 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ImmunizationStatus> 2711 case 1554065514: /*notGiven*/ return this.notGiven == null ? new Base[0] : new Base[] {this.notGiven}; // BooleanType 2712 case 664556354: /*vaccineCode*/ return this.vaccineCode == null ? new Base[0] : new Base[] {this.vaccineCode}; // CodeableConcept 2713 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2714 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 2715 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2716 case -528721731: /*primarySource*/ return this.primarySource == null ? new Base[0] : new Base[] {this.primarySource}; // BooleanType 2717 case 486750586: /*reportOrigin*/ return this.reportOrigin == null ? new Base[0] : new Base[] {this.reportOrigin}; // CodeableConcept 2718 case 1901043637: /*location*/ return this.location == null ? new Base[0] : new Base[] {this.location}; // Reference 2719 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // Reference 2720 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 2721 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateType 2722 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // CodeableConcept 2723 case 108704329: /*route*/ return this.route == null ? new Base[0] : new Base[] {this.route}; // CodeableConcept 2724 case -2083618872: /*doseQuantity*/ return this.doseQuantity == null ? new Base[0] : new Base[] {this.doseQuantity}; // SimpleQuantity 2725 case 574573338: /*practitioner*/ return this.practitioner == null ? new Base[0] : this.practitioner.toArray(new Base[this.practitioner.size()]); // ImmunizationPractitionerComponent 2726 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2727 case -1105867239: /*explanation*/ return this.explanation == null ? new Base[0] : new Base[] {this.explanation}; // ImmunizationExplanationComponent 2728 case -867509719: /*reaction*/ return this.reaction == null ? new Base[0] : this.reaction.toArray(new Base[this.reaction.size()]); // ImmunizationReactionComponent 2729 case -179633155: /*vaccinationProtocol*/ return this.vaccinationProtocol == null ? new Base[0] : this.vaccinationProtocol.toArray(new Base[this.vaccinationProtocol.size()]); // ImmunizationVaccinationProtocolComponent 2730 default: return super.getProperty(hash, name, checkValid); 2731 } 2732 2733 } 2734 2735 @Override 2736 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2737 switch (hash) { 2738 case -1618432855: // identifier 2739 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2740 return value; 2741 case -892481550: // status 2742 value = new ImmunizationStatusEnumFactory().fromType(castToCode(value)); 2743 this.status = (Enumeration) value; // Enumeration<ImmunizationStatus> 2744 return value; 2745 case 1554065514: // notGiven 2746 this.notGiven = castToBoolean(value); // BooleanType 2747 return value; 2748 case 664556354: // vaccineCode 2749 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 2750 return value; 2751 case -791418107: // patient 2752 this.patient = castToReference(value); // Reference 2753 return value; 2754 case 1524132147: // encounter 2755 this.encounter = castToReference(value); // Reference 2756 return value; 2757 case 3076014: // date 2758 this.date = castToDateTime(value); // DateTimeType 2759 return value; 2760 case -528721731: // primarySource 2761 this.primarySource = castToBoolean(value); // BooleanType 2762 return value; 2763 case 486750586: // reportOrigin 2764 this.reportOrigin = castToCodeableConcept(value); // CodeableConcept 2765 return value; 2766 case 1901043637: // location 2767 this.location = castToReference(value); // Reference 2768 return value; 2769 case -1969347631: // manufacturer 2770 this.manufacturer = castToReference(value); // Reference 2771 return value; 2772 case 462547450: // lotNumber 2773 this.lotNumber = castToString(value); // StringType 2774 return value; 2775 case -668811523: // expirationDate 2776 this.expirationDate = castToDate(value); // DateType 2777 return value; 2778 case 3530567: // site 2779 this.site = castToCodeableConcept(value); // CodeableConcept 2780 return value; 2781 case 108704329: // route 2782 this.route = castToCodeableConcept(value); // CodeableConcept 2783 return value; 2784 case -2083618872: // doseQuantity 2785 this.doseQuantity = castToSimpleQuantity(value); // SimpleQuantity 2786 return value; 2787 case 574573338: // practitioner 2788 this.getPractitioner().add((ImmunizationPractitionerComponent) value); // ImmunizationPractitionerComponent 2789 return value; 2790 case 3387378: // note 2791 this.getNote().add(castToAnnotation(value)); // Annotation 2792 return value; 2793 case -1105867239: // explanation 2794 this.explanation = (ImmunizationExplanationComponent) value; // ImmunizationExplanationComponent 2795 return value; 2796 case -867509719: // reaction 2797 this.getReaction().add((ImmunizationReactionComponent) value); // ImmunizationReactionComponent 2798 return value; 2799 case -179633155: // vaccinationProtocol 2800 this.getVaccinationProtocol().add((ImmunizationVaccinationProtocolComponent) value); // ImmunizationVaccinationProtocolComponent 2801 return value; 2802 default: return super.setProperty(hash, name, value); 2803 } 2804 2805 } 2806 2807 @Override 2808 public Base setProperty(String name, Base value) throws FHIRException { 2809 if (name.equals("identifier")) { 2810 this.getIdentifier().add(castToIdentifier(value)); 2811 } else if (name.equals("status")) { 2812 value = new ImmunizationStatusEnumFactory().fromType(castToCode(value)); 2813 this.status = (Enumeration) value; // Enumeration<ImmunizationStatus> 2814 } else if (name.equals("notGiven")) { 2815 this.notGiven = castToBoolean(value); // BooleanType 2816 } else if (name.equals("vaccineCode")) { 2817 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 2818 } else if (name.equals("patient")) { 2819 this.patient = castToReference(value); // Reference 2820 } else if (name.equals("encounter")) { 2821 this.encounter = castToReference(value); // Reference 2822 } else if (name.equals("date")) { 2823 this.date = castToDateTime(value); // DateTimeType 2824 } else if (name.equals("primarySource")) { 2825 this.primarySource = castToBoolean(value); // BooleanType 2826 } else if (name.equals("reportOrigin")) { 2827 this.reportOrigin = castToCodeableConcept(value); // CodeableConcept 2828 } else if (name.equals("location")) { 2829 this.location = castToReference(value); // Reference 2830 } else if (name.equals("manufacturer")) { 2831 this.manufacturer = castToReference(value); // Reference 2832 } else if (name.equals("lotNumber")) { 2833 this.lotNumber = castToString(value); // StringType 2834 } else if (name.equals("expirationDate")) { 2835 this.expirationDate = castToDate(value); // DateType 2836 } else if (name.equals("site")) { 2837 this.site = castToCodeableConcept(value); // CodeableConcept 2838 } else if (name.equals("route")) { 2839 this.route = castToCodeableConcept(value); // CodeableConcept 2840 } else if (name.equals("doseQuantity")) { 2841 this.doseQuantity = castToSimpleQuantity(value); // SimpleQuantity 2842 } else if (name.equals("practitioner")) { 2843 this.getPractitioner().add((ImmunizationPractitionerComponent) value); 2844 } else if (name.equals("note")) { 2845 this.getNote().add(castToAnnotation(value)); 2846 } else if (name.equals("explanation")) { 2847 this.explanation = (ImmunizationExplanationComponent) value; // ImmunizationExplanationComponent 2848 } else if (name.equals("reaction")) { 2849 this.getReaction().add((ImmunizationReactionComponent) value); 2850 } else if (name.equals("vaccinationProtocol")) { 2851 this.getVaccinationProtocol().add((ImmunizationVaccinationProtocolComponent) value); 2852 } else 2853 return super.setProperty(name, value); 2854 return value; 2855 } 2856 2857 @Override 2858 public Base makeProperty(int hash, String name) throws FHIRException { 2859 switch (hash) { 2860 case -1618432855: return addIdentifier(); 2861 case -892481550: return getStatusElement(); 2862 case 1554065514: return getNotGivenElement(); 2863 case 664556354: return getVaccineCode(); 2864 case -791418107: return getPatient(); 2865 case 1524132147: return getEncounter(); 2866 case 3076014: return getDateElement(); 2867 case -528721731: return getPrimarySourceElement(); 2868 case 486750586: return getReportOrigin(); 2869 case 1901043637: return getLocation(); 2870 case -1969347631: return getManufacturer(); 2871 case 462547450: return getLotNumberElement(); 2872 case -668811523: return getExpirationDateElement(); 2873 case 3530567: return getSite(); 2874 case 108704329: return getRoute(); 2875 case -2083618872: return getDoseQuantity(); 2876 case 574573338: return addPractitioner(); 2877 case 3387378: return addNote(); 2878 case -1105867239: return getExplanation(); 2879 case -867509719: return addReaction(); 2880 case -179633155: return addVaccinationProtocol(); 2881 default: return super.makeProperty(hash, name); 2882 } 2883 2884 } 2885 2886 @Override 2887 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2888 switch (hash) { 2889 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2890 case -892481550: /*status*/ return new String[] {"code"}; 2891 case 1554065514: /*notGiven*/ return new String[] {"boolean"}; 2892 case 664556354: /*vaccineCode*/ return new String[] {"CodeableConcept"}; 2893 case -791418107: /*patient*/ return new String[] {"Reference"}; 2894 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 2895 case 3076014: /*date*/ return new String[] {"dateTime"}; 2896 case -528721731: /*primarySource*/ return new String[] {"boolean"}; 2897 case 486750586: /*reportOrigin*/ return new String[] {"CodeableConcept"}; 2898 case 1901043637: /*location*/ return new String[] {"Reference"}; 2899 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 2900 case 462547450: /*lotNumber*/ return new String[] {"string"}; 2901 case -668811523: /*expirationDate*/ return new String[] {"date"}; 2902 case 3530567: /*site*/ return new String[] {"CodeableConcept"}; 2903 case 108704329: /*route*/ return new String[] {"CodeableConcept"}; 2904 case -2083618872: /*doseQuantity*/ return new String[] {"SimpleQuantity"}; 2905 case 574573338: /*practitioner*/ return new String[] {}; 2906 case 3387378: /*note*/ return new String[] {"Annotation"}; 2907 case -1105867239: /*explanation*/ return new String[] {}; 2908 case -867509719: /*reaction*/ return new String[] {}; 2909 case -179633155: /*vaccinationProtocol*/ return new String[] {}; 2910 default: return super.getTypesForProperty(hash, name); 2911 } 2912 2913 } 2914 2915 @Override 2916 public Base addChild(String name) throws FHIRException { 2917 if (name.equals("identifier")) { 2918 return addIdentifier(); 2919 } 2920 else if (name.equals("status")) { 2921 throw new FHIRException("Cannot call addChild on a singleton property Immunization.status"); 2922 } 2923 else if (name.equals("notGiven")) { 2924 throw new FHIRException("Cannot call addChild on a singleton property Immunization.notGiven"); 2925 } 2926 else if (name.equals("vaccineCode")) { 2927 this.vaccineCode = new CodeableConcept(); 2928 return this.vaccineCode; 2929 } 2930 else if (name.equals("patient")) { 2931 this.patient = new Reference(); 2932 return this.patient; 2933 } 2934 else if (name.equals("encounter")) { 2935 this.encounter = new Reference(); 2936 return this.encounter; 2937 } 2938 else if (name.equals("date")) { 2939 throw new FHIRException("Cannot call addChild on a singleton property Immunization.date"); 2940 } 2941 else if (name.equals("primarySource")) { 2942 throw new FHIRException("Cannot call addChild on a singleton property Immunization.primarySource"); 2943 } 2944 else if (name.equals("reportOrigin")) { 2945 this.reportOrigin = new CodeableConcept(); 2946 return this.reportOrigin; 2947 } 2948 else if (name.equals("location")) { 2949 this.location = new Reference(); 2950 return this.location; 2951 } 2952 else if (name.equals("manufacturer")) { 2953 this.manufacturer = new Reference(); 2954 return this.manufacturer; 2955 } 2956 else if (name.equals("lotNumber")) { 2957 throw new FHIRException("Cannot call addChild on a singleton property Immunization.lotNumber"); 2958 } 2959 else if (name.equals("expirationDate")) { 2960 throw new FHIRException("Cannot call addChild on a singleton property Immunization.expirationDate"); 2961 } 2962 else if (name.equals("site")) { 2963 this.site = new CodeableConcept(); 2964 return this.site; 2965 } 2966 else if (name.equals("route")) { 2967 this.route = new CodeableConcept(); 2968 return this.route; 2969 } 2970 else if (name.equals("doseQuantity")) { 2971 this.doseQuantity = new SimpleQuantity(); 2972 return this.doseQuantity; 2973 } 2974 else if (name.equals("practitioner")) { 2975 return addPractitioner(); 2976 } 2977 else if (name.equals("note")) { 2978 return addNote(); 2979 } 2980 else if (name.equals("explanation")) { 2981 this.explanation = new ImmunizationExplanationComponent(); 2982 return this.explanation; 2983 } 2984 else if (name.equals("reaction")) { 2985 return addReaction(); 2986 } 2987 else if (name.equals("vaccinationProtocol")) { 2988 return addVaccinationProtocol(); 2989 } 2990 else 2991 return super.addChild(name); 2992 } 2993 2994 public String fhirType() { 2995 return "Immunization"; 2996 2997 } 2998 2999 public Immunization copy() { 3000 Immunization dst = new Immunization(); 3001 copyValues(dst); 3002 if (identifier != null) { 3003 dst.identifier = new ArrayList<Identifier>(); 3004 for (Identifier i : identifier) 3005 dst.identifier.add(i.copy()); 3006 }; 3007 dst.status = status == null ? null : status.copy(); 3008 dst.notGiven = notGiven == null ? null : notGiven.copy(); 3009 dst.vaccineCode = vaccineCode == null ? null : vaccineCode.copy(); 3010 dst.patient = patient == null ? null : patient.copy(); 3011 dst.encounter = encounter == null ? null : encounter.copy(); 3012 dst.date = date == null ? null : date.copy(); 3013 dst.primarySource = primarySource == null ? null : primarySource.copy(); 3014 dst.reportOrigin = reportOrigin == null ? null : reportOrigin.copy(); 3015 dst.location = location == null ? null : location.copy(); 3016 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 3017 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 3018 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 3019 dst.site = site == null ? null : site.copy(); 3020 dst.route = route == null ? null : route.copy(); 3021 dst.doseQuantity = doseQuantity == null ? null : doseQuantity.copy(); 3022 if (practitioner != null) { 3023 dst.practitioner = new ArrayList<ImmunizationPractitionerComponent>(); 3024 for (ImmunizationPractitionerComponent i : practitioner) 3025 dst.practitioner.add(i.copy()); 3026 }; 3027 if (note != null) { 3028 dst.note = new ArrayList<Annotation>(); 3029 for (Annotation i : note) 3030 dst.note.add(i.copy()); 3031 }; 3032 dst.explanation = explanation == null ? null : explanation.copy(); 3033 if (reaction != null) { 3034 dst.reaction = new ArrayList<ImmunizationReactionComponent>(); 3035 for (ImmunizationReactionComponent i : reaction) 3036 dst.reaction.add(i.copy()); 3037 }; 3038 if (vaccinationProtocol != null) { 3039 dst.vaccinationProtocol = new ArrayList<ImmunizationVaccinationProtocolComponent>(); 3040 for (ImmunizationVaccinationProtocolComponent i : vaccinationProtocol) 3041 dst.vaccinationProtocol.add(i.copy()); 3042 }; 3043 return dst; 3044 } 3045 3046 protected Immunization typedCopy() { 3047 return copy(); 3048 } 3049 3050 @Override 3051 public boolean equalsDeep(Base other_) { 3052 if (!super.equalsDeep(other_)) 3053 return false; 3054 if (!(other_ instanceof Immunization)) 3055 return false; 3056 Immunization o = (Immunization) other_; 3057 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(notGiven, o.notGiven, true) 3058 && compareDeep(vaccineCode, o.vaccineCode, true) && compareDeep(patient, o.patient, true) && compareDeep(encounter, o.encounter, true) 3059 && compareDeep(date, o.date, true) && compareDeep(primarySource, o.primarySource, true) && compareDeep(reportOrigin, o.reportOrigin, true) 3060 && compareDeep(location, o.location, true) && compareDeep(manufacturer, o.manufacturer, true) && compareDeep(lotNumber, o.lotNumber, true) 3061 && compareDeep(expirationDate, o.expirationDate, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 3062 && compareDeep(doseQuantity, o.doseQuantity, true) && compareDeep(practitioner, o.practitioner, true) 3063 && compareDeep(note, o.note, true) && compareDeep(explanation, o.explanation, true) && compareDeep(reaction, o.reaction, true) 3064 && compareDeep(vaccinationProtocol, o.vaccinationProtocol, true); 3065 } 3066 3067 @Override 3068 public boolean equalsShallow(Base other_) { 3069 if (!super.equalsShallow(other_)) 3070 return false; 3071 if (!(other_ instanceof Immunization)) 3072 return false; 3073 Immunization o = (Immunization) other_; 3074 return compareValues(status, o.status, true) && compareValues(notGiven, o.notGiven, true) && compareValues(date, o.date, true) 3075 && compareValues(primarySource, o.primarySource, true) && compareValues(lotNumber, o.lotNumber, true) 3076 && compareValues(expirationDate, o.expirationDate, true); 3077 } 3078 3079 public boolean isEmpty() { 3080 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, notGiven 3081 , vaccineCode, patient, encounter, date, primarySource, reportOrigin, location 3082 , manufacturer, lotNumber, expirationDate, site, route, doseQuantity, practitioner 3083 , note, explanation, reaction, vaccinationProtocol); 3084 } 3085 3086 @Override 3087 public ResourceType getResourceType() { 3088 return ResourceType.Immunization; 3089 } 3090 3091 /** 3092 * Search parameter: <b>date</b> 3093 * <p> 3094 * Description: <b>Vaccination (non)-Administration Date</b><br> 3095 * Type: <b>date</b><br> 3096 * Path: <b>Immunization.date</b><br> 3097 * </p> 3098 */ 3099 @SearchParamDefinition(name="date", path="Immunization.date", description="Vaccination (non)-Administration Date", type="date" ) 3100 public static final String SP_DATE = "date"; 3101 /** 3102 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3103 * <p> 3104 * Description: <b>Vaccination (non)-Administration Date</b><br> 3105 * Type: <b>date</b><br> 3106 * Path: <b>Immunization.date</b><br> 3107 * </p> 3108 */ 3109 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3110 3111 /** 3112 * Search parameter: <b>identifier</b> 3113 * <p> 3114 * Description: <b>Business identifier</b><br> 3115 * Type: <b>token</b><br> 3116 * Path: <b>Immunization.identifier</b><br> 3117 * </p> 3118 */ 3119 @SearchParamDefinition(name="identifier", path="Immunization.identifier", description="Business identifier", type="token" ) 3120 public static final String SP_IDENTIFIER = "identifier"; 3121 /** 3122 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3123 * <p> 3124 * Description: <b>Business identifier</b><br> 3125 * Type: <b>token</b><br> 3126 * Path: <b>Immunization.identifier</b><br> 3127 * </p> 3128 */ 3129 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3130 3131 /** 3132 * Search parameter: <b>reason</b> 3133 * <p> 3134 * Description: <b>Why immunization occurred</b><br> 3135 * Type: <b>token</b><br> 3136 * Path: <b>Immunization.explanation.reason</b><br> 3137 * </p> 3138 */ 3139 @SearchParamDefinition(name="reason", path="Immunization.explanation.reason", description="Why immunization occurred", type="token" ) 3140 public static final String SP_REASON = "reason"; 3141 /** 3142 * <b>Fluent Client</b> search parameter constant for <b>reason</b> 3143 * <p> 3144 * Description: <b>Why immunization occurred</b><br> 3145 * Type: <b>token</b><br> 3146 * Path: <b>Immunization.explanation.reason</b><br> 3147 * </p> 3148 */ 3149 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON); 3150 3151 /** 3152 * Search parameter: <b>reaction</b> 3153 * <p> 3154 * Description: <b>Additional information on reaction</b><br> 3155 * Type: <b>reference</b><br> 3156 * Path: <b>Immunization.reaction.detail</b><br> 3157 * </p> 3158 */ 3159 @SearchParamDefinition(name="reaction", path="Immunization.reaction.detail", description="Additional information on reaction", type="reference", target={Observation.class } ) 3160 public static final String SP_REACTION = "reaction"; 3161 /** 3162 * <b>Fluent Client</b> search parameter constant for <b>reaction</b> 3163 * <p> 3164 * Description: <b>Additional information on reaction</b><br> 3165 * Type: <b>reference</b><br> 3166 * Path: <b>Immunization.reaction.detail</b><br> 3167 * </p> 3168 */ 3169 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REACTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REACTION); 3170 3171/** 3172 * Constant for fluent queries to be used to add include statements. Specifies 3173 * the path value of "<b>Immunization:reaction</b>". 3174 */ 3175 public static final ca.uhn.fhir.model.api.Include INCLUDE_REACTION = new ca.uhn.fhir.model.api.Include("Immunization:reaction").toLocked(); 3176 3177 /** 3178 * Search parameter: <b>lot-number</b> 3179 * <p> 3180 * Description: <b>Vaccine Lot Number</b><br> 3181 * Type: <b>string</b><br> 3182 * Path: <b>Immunization.lotNumber</b><br> 3183 * </p> 3184 */ 3185 @SearchParamDefinition(name="lot-number", path="Immunization.lotNumber", description="Vaccine Lot Number", type="string" ) 3186 public static final String SP_LOT_NUMBER = "lot-number"; 3187 /** 3188 * <b>Fluent Client</b> search parameter constant for <b>lot-number</b> 3189 * <p> 3190 * Description: <b>Vaccine Lot Number</b><br> 3191 * Type: <b>string</b><br> 3192 * Path: <b>Immunization.lotNumber</b><br> 3193 * </p> 3194 */ 3195 public static final ca.uhn.fhir.rest.gclient.StringClientParam LOT_NUMBER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_LOT_NUMBER); 3196 3197 /** 3198 * Search parameter: <b>practitioner</b> 3199 * <p> 3200 * Description: <b>The practitioner who played a role in the vaccination</b><br> 3201 * Type: <b>reference</b><br> 3202 * Path: <b>Immunization.practitioner.actor</b><br> 3203 * </p> 3204 */ 3205 @SearchParamDefinition(name="practitioner", path="Immunization.practitioner.actor", description="The practitioner who played a role in the vaccination", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 3206 public static final String SP_PRACTITIONER = "practitioner"; 3207 /** 3208 * <b>Fluent Client</b> search parameter constant for <b>practitioner</b> 3209 * <p> 3210 * Description: <b>The practitioner who played a role in the vaccination</b><br> 3211 * Type: <b>reference</b><br> 3212 * Path: <b>Immunization.practitioner.actor</b><br> 3213 * </p> 3214 */ 3215 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRACTITIONER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRACTITIONER); 3216 3217/** 3218 * Constant for fluent queries to be used to add include statements. Specifies 3219 * the path value of "<b>Immunization:practitioner</b>". 3220 */ 3221 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRACTITIONER = new ca.uhn.fhir.model.api.Include("Immunization:practitioner").toLocked(); 3222 3223 /** 3224 * Search parameter: <b>notgiven</b> 3225 * <p> 3226 * Description: <b>Administrations which were not given</b><br> 3227 * Type: <b>token</b><br> 3228 * Path: <b>Immunization.notGiven</b><br> 3229 * </p> 3230 */ 3231 @SearchParamDefinition(name="notgiven", path="Immunization.notGiven", description="Administrations which were not given", type="token" ) 3232 public static final String SP_NOTGIVEN = "notgiven"; 3233 /** 3234 * <b>Fluent Client</b> search parameter constant for <b>notgiven</b> 3235 * <p> 3236 * Description: <b>Administrations which were not given</b><br> 3237 * Type: <b>token</b><br> 3238 * Path: <b>Immunization.notGiven</b><br> 3239 * </p> 3240 */ 3241 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NOTGIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_NOTGIVEN); 3242 3243 /** 3244 * Search parameter: <b>manufacturer</b> 3245 * <p> 3246 * Description: <b>Vaccine Manufacturer</b><br> 3247 * Type: <b>reference</b><br> 3248 * Path: <b>Immunization.manufacturer</b><br> 3249 * </p> 3250 */ 3251 @SearchParamDefinition(name="manufacturer", path="Immunization.manufacturer", description="Vaccine Manufacturer", type="reference", target={Organization.class } ) 3252 public static final String SP_MANUFACTURER = "manufacturer"; 3253 /** 3254 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 3255 * <p> 3256 * Description: <b>Vaccine Manufacturer</b><br> 3257 * Type: <b>reference</b><br> 3258 * Path: <b>Immunization.manufacturer</b><br> 3259 * </p> 3260 */ 3261 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANUFACTURER); 3262 3263/** 3264 * Constant for fluent queries to be used to add include statements. Specifies 3265 * the path value of "<b>Immunization:manufacturer</b>". 3266 */ 3267 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include("Immunization:manufacturer").toLocked(); 3268 3269 /** 3270 * Search parameter: <b>dose-sequence</b> 3271 * <p> 3272 * Description: <b>Dose number within series</b><br> 3273 * Type: <b>number</b><br> 3274 * Path: <b>Immunization.vaccinationProtocol.doseSequence</b><br> 3275 * </p> 3276 */ 3277 @SearchParamDefinition(name="dose-sequence", path="Immunization.vaccinationProtocol.doseSequence", description="Dose number within series", type="number" ) 3278 public static final String SP_DOSE_SEQUENCE = "dose-sequence"; 3279 /** 3280 * <b>Fluent Client</b> search parameter constant for <b>dose-sequence</b> 3281 * <p> 3282 * Description: <b>Dose number within series</b><br> 3283 * Type: <b>number</b><br> 3284 * Path: <b>Immunization.vaccinationProtocol.doseSequence</b><br> 3285 * </p> 3286 */ 3287 public static final ca.uhn.fhir.rest.gclient.NumberClientParam DOSE_SEQUENCE = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_DOSE_SEQUENCE); 3288 3289 /** 3290 * Search parameter: <b>patient</b> 3291 * <p> 3292 * Description: <b>The patient for the vaccination record</b><br> 3293 * Type: <b>reference</b><br> 3294 * Path: <b>Immunization.patient</b><br> 3295 * </p> 3296 */ 3297 @SearchParamDefinition(name="patient", path="Immunization.patient", description="The patient for the vaccination record", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 3298 public static final String SP_PATIENT = "patient"; 3299 /** 3300 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3301 * <p> 3302 * Description: <b>The patient for the vaccination record</b><br> 3303 * Type: <b>reference</b><br> 3304 * Path: <b>Immunization.patient</b><br> 3305 * </p> 3306 */ 3307 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3308 3309/** 3310 * Constant for fluent queries to be used to add include statements. Specifies 3311 * the path value of "<b>Immunization:patient</b>". 3312 */ 3313 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Immunization:patient").toLocked(); 3314 3315 /** 3316 * Search parameter: <b>vaccine-code</b> 3317 * <p> 3318 * Description: <b>Vaccine Product Administered</b><br> 3319 * Type: <b>token</b><br> 3320 * Path: <b>Immunization.vaccineCode</b><br> 3321 * </p> 3322 */ 3323 @SearchParamDefinition(name="vaccine-code", path="Immunization.vaccineCode", description="Vaccine Product Administered", type="token" ) 3324 public static final String SP_VACCINE_CODE = "vaccine-code"; 3325 /** 3326 * <b>Fluent Client</b> search parameter constant for <b>vaccine-code</b> 3327 * <p> 3328 * Description: <b>Vaccine Product Administered</b><br> 3329 * Type: <b>token</b><br> 3330 * Path: <b>Immunization.vaccineCode</b><br> 3331 * </p> 3332 */ 3333 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VACCINE_CODE); 3334 3335 /** 3336 * Search parameter: <b>reason-not-given</b> 3337 * <p> 3338 * Description: <b>Explanation of reason vaccination was not administered</b><br> 3339 * Type: <b>token</b><br> 3340 * Path: <b>Immunization.explanation.reasonNotGiven</b><br> 3341 * </p> 3342 */ 3343 @SearchParamDefinition(name="reason-not-given", path="Immunization.explanation.reasonNotGiven", description="Explanation of reason vaccination was not administered", type="token" ) 3344 public static final String SP_REASON_NOT_GIVEN = "reason-not-given"; 3345 /** 3346 * <b>Fluent Client</b> search parameter constant for <b>reason-not-given</b> 3347 * <p> 3348 * Description: <b>Explanation of reason vaccination was not administered</b><br> 3349 * Type: <b>token</b><br> 3350 * Path: <b>Immunization.explanation.reasonNotGiven</b><br> 3351 * </p> 3352 */ 3353 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_NOT_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_NOT_GIVEN); 3354 3355 /** 3356 * Search parameter: <b>location</b> 3357 * <p> 3358 * Description: <b>The service delivery location or facility in which the vaccine was / was to be administered</b><br> 3359 * Type: <b>reference</b><br> 3360 * Path: <b>Immunization.location</b><br> 3361 * </p> 3362 */ 3363 @SearchParamDefinition(name="location", path="Immunization.location", description="The service delivery location or facility in which the vaccine was / was to be administered", type="reference", target={Location.class } ) 3364 public static final String SP_LOCATION = "location"; 3365 /** 3366 * <b>Fluent Client</b> search parameter constant for <b>location</b> 3367 * <p> 3368 * Description: <b>The service delivery location or facility in which the vaccine was / was to be administered</b><br> 3369 * Type: <b>reference</b><br> 3370 * Path: <b>Immunization.location</b><br> 3371 * </p> 3372 */ 3373 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam LOCATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_LOCATION); 3374 3375/** 3376 * Constant for fluent queries to be used to add include statements. Specifies 3377 * the path value of "<b>Immunization:location</b>". 3378 */ 3379 public static final ca.uhn.fhir.model.api.Include INCLUDE_LOCATION = new ca.uhn.fhir.model.api.Include("Immunization:location").toLocked(); 3380 3381 /** 3382 * Search parameter: <b>reaction-date</b> 3383 * <p> 3384 * Description: <b>When reaction started</b><br> 3385 * Type: <b>date</b><br> 3386 * Path: <b>Immunization.reaction.date</b><br> 3387 * </p> 3388 */ 3389 @SearchParamDefinition(name="reaction-date", path="Immunization.reaction.date", description="When reaction started", type="date" ) 3390 public static final String SP_REACTION_DATE = "reaction-date"; 3391 /** 3392 * <b>Fluent Client</b> search parameter constant for <b>reaction-date</b> 3393 * <p> 3394 * Description: <b>When reaction started</b><br> 3395 * Type: <b>date</b><br> 3396 * Path: <b>Immunization.reaction.date</b><br> 3397 * </p> 3398 */ 3399 public static final ca.uhn.fhir.rest.gclient.DateClientParam REACTION_DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_REACTION_DATE); 3400 3401 /** 3402 * Search parameter: <b>status</b> 3403 * <p> 3404 * Description: <b>Immunization event status</b><br> 3405 * Type: <b>token</b><br> 3406 * Path: <b>Immunization.status</b><br> 3407 * </p> 3408 */ 3409 @SearchParamDefinition(name="status", path="Immunization.status", description="Immunization event status", type="token" ) 3410 public static final String SP_STATUS = "status"; 3411 /** 3412 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3413 * <p> 3414 * Description: <b>Immunization event status</b><br> 3415 * Type: <b>token</b><br> 3416 * Path: <b>Immunization.status</b><br> 3417 * </p> 3418 */ 3419 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3420 3421 3422}