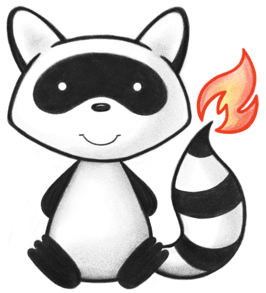
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A patient's point-in-time immunization and recommendation (i.e. forecasting a patient's immunization eligibility according to a published schedule) with optional supporting justification. 050 */ 051@ResourceDef(name="ImmunizationRecommendation", profile="http://hl7.org/fhir/Profile/ImmunizationRecommendation") 052public class ImmunizationRecommendation extends DomainResource { 053 054 @Block() 055 public static class ImmunizationRecommendationRecommendationComponent extends BackboneElement implements IBaseBackboneElement { 056 /** 057 * The date the immunization recommendation was created. 058 */ 059 @Child(name = "date", type = {DateTimeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 060 @Description(shortDefinition="Date recommendation created", formalDefinition="The date the immunization recommendation was created." ) 061 protected DateTimeType date; 062 063 /** 064 * Vaccine that pertains to the recommendation. 065 */ 066 @Child(name = "vaccineCode", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 067 @Description(shortDefinition="Vaccine recommendation applies to", formalDefinition="Vaccine that pertains to the recommendation." ) 068 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/vaccine-code") 069 protected CodeableConcept vaccineCode; 070 071 /** 072 * The targeted disease for the recommendation. 073 */ 074 @Child(name = "targetDisease", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 075 @Description(shortDefinition="Disease to be immunized against", formalDefinition="The targeted disease for the recommendation." ) 076 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-recommendation-target-disease") 077 protected CodeableConcept targetDisease; 078 079 /** 080 * The next recommended dose number (e.g. dose 2 is the next recommended dose). 081 */ 082 @Child(name = "doseNumber", type = {PositiveIntType.class}, order=4, min=0, max=1, modifier=false, summary=true) 083 @Description(shortDefinition="Recommended dose number", formalDefinition="The next recommended dose number (e.g. dose 2 is the next recommended dose)." ) 084 protected PositiveIntType doseNumber; 085 086 /** 087 * Vaccine administration status. 088 */ 089 @Child(name = "forecastStatus", type = {CodeableConcept.class}, order=5, min=1, max=1, modifier=false, summary=true) 090 @Description(shortDefinition="Vaccine administration status", formalDefinition="Vaccine administration status." ) 091 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-recommendation-status") 092 protected CodeableConcept forecastStatus; 093 094 /** 095 * Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc. 096 */ 097 @Child(name = "dateCriterion", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 098 @Description(shortDefinition="Dates governing proposed immunization", formalDefinition="Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc." ) 099 protected List<ImmunizationRecommendationRecommendationDateCriterionComponent> dateCriterion; 100 101 /** 102 * Contains information about the protocol under which the vaccine was administered. 103 */ 104 @Child(name = "protocol", type = {}, order=7, min=0, max=1, modifier=false, summary=false) 105 @Description(shortDefinition="Protocol used by recommendation", formalDefinition="Contains information about the protocol under which the vaccine was administered." ) 106 protected ImmunizationRecommendationRecommendationProtocolComponent protocol; 107 108 /** 109 * Immunization event history that supports the status and recommendation. 110 */ 111 @Child(name = "supportingImmunization", type = {Immunization.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 112 @Description(shortDefinition="Past immunizations supporting recommendation", formalDefinition="Immunization event history that supports the status and recommendation." ) 113 protected List<Reference> supportingImmunization; 114 /** 115 * The actual objects that are the target of the reference (Immunization event history that supports the status and recommendation.) 116 */ 117 protected List<Immunization> supportingImmunizationTarget; 118 119 120 /** 121 * Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information. 122 */ 123 @Child(name = "supportingPatientInformation", type = {Observation.class, AllergyIntolerance.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 124 @Description(shortDefinition="Patient observations supporting recommendation", formalDefinition="Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information." ) 125 protected List<Reference> supportingPatientInformation; 126 /** 127 * The actual objects that are the target of the reference (Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.) 128 */ 129 protected List<Resource> supportingPatientInformationTarget; 130 131 132 private static final long serialVersionUID = 1279700888L; 133 134 /** 135 * Constructor 136 */ 137 public ImmunizationRecommendationRecommendationComponent() { 138 super(); 139 } 140 141 /** 142 * Constructor 143 */ 144 public ImmunizationRecommendationRecommendationComponent(DateTimeType date, CodeableConcept forecastStatus) { 145 super(); 146 this.date = date; 147 this.forecastStatus = forecastStatus; 148 } 149 150 /** 151 * @return {@link #date} (The date the immunization recommendation was created.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 152 */ 153 public DateTimeType getDateElement() { 154 if (this.date == null) 155 if (Configuration.errorOnAutoCreate()) 156 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.date"); 157 else if (Configuration.doAutoCreate()) 158 this.date = new DateTimeType(); // bb 159 return this.date; 160 } 161 162 public boolean hasDateElement() { 163 return this.date != null && !this.date.isEmpty(); 164 } 165 166 public boolean hasDate() { 167 return this.date != null && !this.date.isEmpty(); 168 } 169 170 /** 171 * @param value {@link #date} (The date the immunization recommendation was created.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 172 */ 173 public ImmunizationRecommendationRecommendationComponent setDateElement(DateTimeType value) { 174 this.date = value; 175 return this; 176 } 177 178 /** 179 * @return The date the immunization recommendation was created. 180 */ 181 public Date getDate() { 182 return this.date == null ? null : this.date.getValue(); 183 } 184 185 /** 186 * @param value The date the immunization recommendation was created. 187 */ 188 public ImmunizationRecommendationRecommendationComponent setDate(Date value) { 189 if (this.date == null) 190 this.date = new DateTimeType(); 191 this.date.setValue(value); 192 return this; 193 } 194 195 /** 196 * @return {@link #vaccineCode} (Vaccine that pertains to the recommendation.) 197 */ 198 public CodeableConcept getVaccineCode() { 199 if (this.vaccineCode == null) 200 if (Configuration.errorOnAutoCreate()) 201 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.vaccineCode"); 202 else if (Configuration.doAutoCreate()) 203 this.vaccineCode = new CodeableConcept(); // cc 204 return this.vaccineCode; 205 } 206 207 public boolean hasVaccineCode() { 208 return this.vaccineCode != null && !this.vaccineCode.isEmpty(); 209 } 210 211 /** 212 * @param value {@link #vaccineCode} (Vaccine that pertains to the recommendation.) 213 */ 214 public ImmunizationRecommendationRecommendationComponent setVaccineCode(CodeableConcept value) { 215 this.vaccineCode = value; 216 return this; 217 } 218 219 /** 220 * @return {@link #targetDisease} (The targeted disease for the recommendation.) 221 */ 222 public CodeableConcept getTargetDisease() { 223 if (this.targetDisease == null) 224 if (Configuration.errorOnAutoCreate()) 225 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.targetDisease"); 226 else if (Configuration.doAutoCreate()) 227 this.targetDisease = new CodeableConcept(); // cc 228 return this.targetDisease; 229 } 230 231 public boolean hasTargetDisease() { 232 return this.targetDisease != null && !this.targetDisease.isEmpty(); 233 } 234 235 /** 236 * @param value {@link #targetDisease} (The targeted disease for the recommendation.) 237 */ 238 public ImmunizationRecommendationRecommendationComponent setTargetDisease(CodeableConcept value) { 239 this.targetDisease = value; 240 return this; 241 } 242 243 /** 244 * @return {@link #doseNumber} (The next recommended dose number (e.g. dose 2 is the next recommended dose).). This is the underlying object with id, value and extensions. The accessor "getDoseNumber" gives direct access to the value 245 */ 246 public PositiveIntType getDoseNumberElement() { 247 if (this.doseNumber == null) 248 if (Configuration.errorOnAutoCreate()) 249 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.doseNumber"); 250 else if (Configuration.doAutoCreate()) 251 this.doseNumber = new PositiveIntType(); // bb 252 return this.doseNumber; 253 } 254 255 public boolean hasDoseNumberElement() { 256 return this.doseNumber != null && !this.doseNumber.isEmpty(); 257 } 258 259 public boolean hasDoseNumber() { 260 return this.doseNumber != null && !this.doseNumber.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #doseNumber} (The next recommended dose number (e.g. dose 2 is the next recommended dose).). This is the underlying object with id, value and extensions. The accessor "getDoseNumber" gives direct access to the value 265 */ 266 public ImmunizationRecommendationRecommendationComponent setDoseNumberElement(PositiveIntType value) { 267 this.doseNumber = value; 268 return this; 269 } 270 271 /** 272 * @return The next recommended dose number (e.g. dose 2 is the next recommended dose). 273 */ 274 public int getDoseNumber() { 275 return this.doseNumber == null || this.doseNumber.isEmpty() ? 0 : this.doseNumber.getValue(); 276 } 277 278 /** 279 * @param value The next recommended dose number (e.g. dose 2 is the next recommended dose). 280 */ 281 public ImmunizationRecommendationRecommendationComponent setDoseNumber(int value) { 282 if (this.doseNumber == null) 283 this.doseNumber = new PositiveIntType(); 284 this.doseNumber.setValue(value); 285 return this; 286 } 287 288 /** 289 * @return {@link #forecastStatus} (Vaccine administration status.) 290 */ 291 public CodeableConcept getForecastStatus() { 292 if (this.forecastStatus == null) 293 if (Configuration.errorOnAutoCreate()) 294 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.forecastStatus"); 295 else if (Configuration.doAutoCreate()) 296 this.forecastStatus = new CodeableConcept(); // cc 297 return this.forecastStatus; 298 } 299 300 public boolean hasForecastStatus() { 301 return this.forecastStatus != null && !this.forecastStatus.isEmpty(); 302 } 303 304 /** 305 * @param value {@link #forecastStatus} (Vaccine administration status.) 306 */ 307 public ImmunizationRecommendationRecommendationComponent setForecastStatus(CodeableConcept value) { 308 this.forecastStatus = value; 309 return this; 310 } 311 312 /** 313 * @return {@link #dateCriterion} (Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.) 314 */ 315 public List<ImmunizationRecommendationRecommendationDateCriterionComponent> getDateCriterion() { 316 if (this.dateCriterion == null) 317 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 318 return this.dateCriterion; 319 } 320 321 /** 322 * @return Returns a reference to <code>this</code> for easy method chaining 323 */ 324 public ImmunizationRecommendationRecommendationComponent setDateCriterion(List<ImmunizationRecommendationRecommendationDateCriterionComponent> theDateCriterion) { 325 this.dateCriterion = theDateCriterion; 326 return this; 327 } 328 329 public boolean hasDateCriterion() { 330 if (this.dateCriterion == null) 331 return false; 332 for (ImmunizationRecommendationRecommendationDateCriterionComponent item : this.dateCriterion) 333 if (!item.isEmpty()) 334 return true; 335 return false; 336 } 337 338 public ImmunizationRecommendationRecommendationDateCriterionComponent addDateCriterion() { //3 339 ImmunizationRecommendationRecommendationDateCriterionComponent t = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 340 if (this.dateCriterion == null) 341 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 342 this.dateCriterion.add(t); 343 return t; 344 } 345 346 public ImmunizationRecommendationRecommendationComponent addDateCriterion(ImmunizationRecommendationRecommendationDateCriterionComponent t) { //3 347 if (t == null) 348 return this; 349 if (this.dateCriterion == null) 350 this.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 351 this.dateCriterion.add(t); 352 return this; 353 } 354 355 /** 356 * @return The first repetition of repeating field {@link #dateCriterion}, creating it if it does not already exist 357 */ 358 public ImmunizationRecommendationRecommendationDateCriterionComponent getDateCriterionFirstRep() { 359 if (getDateCriterion().isEmpty()) { 360 addDateCriterion(); 361 } 362 return getDateCriterion().get(0); 363 } 364 365 /** 366 * @return {@link #protocol} (Contains information about the protocol under which the vaccine was administered.) 367 */ 368 public ImmunizationRecommendationRecommendationProtocolComponent getProtocol() { 369 if (this.protocol == null) 370 if (Configuration.errorOnAutoCreate()) 371 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationComponent.protocol"); 372 else if (Configuration.doAutoCreate()) 373 this.protocol = new ImmunizationRecommendationRecommendationProtocolComponent(); // cc 374 return this.protocol; 375 } 376 377 public boolean hasProtocol() { 378 return this.protocol != null && !this.protocol.isEmpty(); 379 } 380 381 /** 382 * @param value {@link #protocol} (Contains information about the protocol under which the vaccine was administered.) 383 */ 384 public ImmunizationRecommendationRecommendationComponent setProtocol(ImmunizationRecommendationRecommendationProtocolComponent value) { 385 this.protocol = value; 386 return this; 387 } 388 389 /** 390 * @return {@link #supportingImmunization} (Immunization event history that supports the status and recommendation.) 391 */ 392 public List<Reference> getSupportingImmunization() { 393 if (this.supportingImmunization == null) 394 this.supportingImmunization = new ArrayList<Reference>(); 395 return this.supportingImmunization; 396 } 397 398 /** 399 * @return Returns a reference to <code>this</code> for easy method chaining 400 */ 401 public ImmunizationRecommendationRecommendationComponent setSupportingImmunization(List<Reference> theSupportingImmunization) { 402 this.supportingImmunization = theSupportingImmunization; 403 return this; 404 } 405 406 public boolean hasSupportingImmunization() { 407 if (this.supportingImmunization == null) 408 return false; 409 for (Reference item : this.supportingImmunization) 410 if (!item.isEmpty()) 411 return true; 412 return false; 413 } 414 415 public Reference addSupportingImmunization() { //3 416 Reference t = new Reference(); 417 if (this.supportingImmunization == null) 418 this.supportingImmunization = new ArrayList<Reference>(); 419 this.supportingImmunization.add(t); 420 return t; 421 } 422 423 public ImmunizationRecommendationRecommendationComponent addSupportingImmunization(Reference t) { //3 424 if (t == null) 425 return this; 426 if (this.supportingImmunization == null) 427 this.supportingImmunization = new ArrayList<Reference>(); 428 this.supportingImmunization.add(t); 429 return this; 430 } 431 432 /** 433 * @return The first repetition of repeating field {@link #supportingImmunization}, creating it if it does not already exist 434 */ 435 public Reference getSupportingImmunizationFirstRep() { 436 if (getSupportingImmunization().isEmpty()) { 437 addSupportingImmunization(); 438 } 439 return getSupportingImmunization().get(0); 440 } 441 442 /** 443 * @return {@link #supportingPatientInformation} (Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.) 444 */ 445 public List<Reference> getSupportingPatientInformation() { 446 if (this.supportingPatientInformation == null) 447 this.supportingPatientInformation = new ArrayList<Reference>(); 448 return this.supportingPatientInformation; 449 } 450 451 /** 452 * @return Returns a reference to <code>this</code> for easy method chaining 453 */ 454 public ImmunizationRecommendationRecommendationComponent setSupportingPatientInformation(List<Reference> theSupportingPatientInformation) { 455 this.supportingPatientInformation = theSupportingPatientInformation; 456 return this; 457 } 458 459 public boolean hasSupportingPatientInformation() { 460 if (this.supportingPatientInformation == null) 461 return false; 462 for (Reference item : this.supportingPatientInformation) 463 if (!item.isEmpty()) 464 return true; 465 return false; 466 } 467 468 public Reference addSupportingPatientInformation() { //3 469 Reference t = new Reference(); 470 if (this.supportingPatientInformation == null) 471 this.supportingPatientInformation = new ArrayList<Reference>(); 472 this.supportingPatientInformation.add(t); 473 return t; 474 } 475 476 public ImmunizationRecommendationRecommendationComponent addSupportingPatientInformation(Reference t) { //3 477 if (t == null) 478 return this; 479 if (this.supportingPatientInformation == null) 480 this.supportingPatientInformation = new ArrayList<Reference>(); 481 this.supportingPatientInformation.add(t); 482 return this; 483 } 484 485 /** 486 * @return The first repetition of repeating field {@link #supportingPatientInformation}, creating it if it does not already exist 487 */ 488 public Reference getSupportingPatientInformationFirstRep() { 489 if (getSupportingPatientInformation().isEmpty()) { 490 addSupportingPatientInformation(); 491 } 492 return getSupportingPatientInformation().get(0); 493 } 494 495 protected void listChildren(List<Property> children) { 496 super.listChildren(children); 497 children.add(new Property("date", "dateTime", "The date the immunization recommendation was created.", 0, 1, date)); 498 children.add(new Property("vaccineCode", "CodeableConcept", "Vaccine that pertains to the recommendation.", 0, 1, vaccineCode)); 499 children.add(new Property("targetDisease", "CodeableConcept", "The targeted disease for the recommendation.", 0, 1, targetDisease)); 500 children.add(new Property("doseNumber", "positiveInt", "The next recommended dose number (e.g. dose 2 is the next recommended dose).", 0, 1, doseNumber)); 501 children.add(new Property("forecastStatus", "CodeableConcept", "Vaccine administration status.", 0, 1, forecastStatus)); 502 children.add(new Property("dateCriterion", "", "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 0, java.lang.Integer.MAX_VALUE, dateCriterion)); 503 children.add(new Property("protocol", "", "Contains information about the protocol under which the vaccine was administered.", 0, 1, protocol)); 504 children.add(new Property("supportingImmunization", "Reference(Immunization)", "Immunization event history that supports the status and recommendation.", 0, java.lang.Integer.MAX_VALUE, supportingImmunization)); 505 children.add(new Property("supportingPatientInformation", "Reference(Observation|AllergyIntolerance)", "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation)); 506 } 507 508 @Override 509 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 510 switch (_hash) { 511 case 3076014: /*date*/ return new Property("date", "dateTime", "The date the immunization recommendation was created.", 0, 1, date); 512 case 664556354: /*vaccineCode*/ return new Property("vaccineCode", "CodeableConcept", "Vaccine that pertains to the recommendation.", 0, 1, vaccineCode); 513 case -319593813: /*targetDisease*/ return new Property("targetDisease", "CodeableConcept", "The targeted disease for the recommendation.", 0, 1, targetDisease); 514 case -887709242: /*doseNumber*/ return new Property("doseNumber", "positiveInt", "The next recommended dose number (e.g. dose 2 is the next recommended dose).", 0, 1, doseNumber); 515 case 1904598477: /*forecastStatus*/ return new Property("forecastStatus", "CodeableConcept", "Vaccine administration status.", 0, 1, forecastStatus); 516 case 2087518867: /*dateCriterion*/ return new Property("dateCriterion", "", "Vaccine date recommendations. For example, earliest date to administer, latest date to administer, etc.", 0, java.lang.Integer.MAX_VALUE, dateCriterion); 517 case -989163880: /*protocol*/ return new Property("protocol", "", "Contains information about the protocol under which the vaccine was administered.", 0, 1, protocol); 518 case 1171592021: /*supportingImmunization*/ return new Property("supportingImmunization", "Reference(Immunization)", "Immunization event history that supports the status and recommendation.", 0, java.lang.Integer.MAX_VALUE, supportingImmunization); 519 case -1234160646: /*supportingPatientInformation*/ return new Property("supportingPatientInformation", "Reference(Observation|AllergyIntolerance)", "Patient Information that supports the status and recommendation. This includes patient observations, adverse reactions and allergy/intolerance information.", 0, java.lang.Integer.MAX_VALUE, supportingPatientInformation); 520 default: return super.getNamedProperty(_hash, _name, _checkValid); 521 } 522 523 } 524 525 @Override 526 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 527 switch (hash) { 528 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 529 case 664556354: /*vaccineCode*/ return this.vaccineCode == null ? new Base[0] : new Base[] {this.vaccineCode}; // CodeableConcept 530 case -319593813: /*targetDisease*/ return this.targetDisease == null ? new Base[0] : new Base[] {this.targetDisease}; // CodeableConcept 531 case -887709242: /*doseNumber*/ return this.doseNumber == null ? new Base[0] : new Base[] {this.doseNumber}; // PositiveIntType 532 case 1904598477: /*forecastStatus*/ return this.forecastStatus == null ? new Base[0] : new Base[] {this.forecastStatus}; // CodeableConcept 533 case 2087518867: /*dateCriterion*/ return this.dateCriterion == null ? new Base[0] : this.dateCriterion.toArray(new Base[this.dateCriterion.size()]); // ImmunizationRecommendationRecommendationDateCriterionComponent 534 case -989163880: /*protocol*/ return this.protocol == null ? new Base[0] : new Base[] {this.protocol}; // ImmunizationRecommendationRecommendationProtocolComponent 535 case 1171592021: /*supportingImmunization*/ return this.supportingImmunization == null ? new Base[0] : this.supportingImmunization.toArray(new Base[this.supportingImmunization.size()]); // Reference 536 case -1234160646: /*supportingPatientInformation*/ return this.supportingPatientInformation == null ? new Base[0] : this.supportingPatientInformation.toArray(new Base[this.supportingPatientInformation.size()]); // Reference 537 default: return super.getProperty(hash, name, checkValid); 538 } 539 540 } 541 542 @Override 543 public Base setProperty(int hash, String name, Base value) throws FHIRException { 544 switch (hash) { 545 case 3076014: // date 546 this.date = castToDateTime(value); // DateTimeType 547 return value; 548 case 664556354: // vaccineCode 549 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 550 return value; 551 case -319593813: // targetDisease 552 this.targetDisease = castToCodeableConcept(value); // CodeableConcept 553 return value; 554 case -887709242: // doseNumber 555 this.doseNumber = castToPositiveInt(value); // PositiveIntType 556 return value; 557 case 1904598477: // forecastStatus 558 this.forecastStatus = castToCodeableConcept(value); // CodeableConcept 559 return value; 560 case 2087518867: // dateCriterion 561 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); // ImmunizationRecommendationRecommendationDateCriterionComponent 562 return value; 563 case -989163880: // protocol 564 this.protocol = (ImmunizationRecommendationRecommendationProtocolComponent) value; // ImmunizationRecommendationRecommendationProtocolComponent 565 return value; 566 case 1171592021: // supportingImmunization 567 this.getSupportingImmunization().add(castToReference(value)); // Reference 568 return value; 569 case -1234160646: // supportingPatientInformation 570 this.getSupportingPatientInformation().add(castToReference(value)); // Reference 571 return value; 572 default: return super.setProperty(hash, name, value); 573 } 574 575 } 576 577 @Override 578 public Base setProperty(String name, Base value) throws FHIRException { 579 if (name.equals("date")) { 580 this.date = castToDateTime(value); // DateTimeType 581 } else if (name.equals("vaccineCode")) { 582 this.vaccineCode = castToCodeableConcept(value); // CodeableConcept 583 } else if (name.equals("targetDisease")) { 584 this.targetDisease = castToCodeableConcept(value); // CodeableConcept 585 } else if (name.equals("doseNumber")) { 586 this.doseNumber = castToPositiveInt(value); // PositiveIntType 587 } else if (name.equals("forecastStatus")) { 588 this.forecastStatus = castToCodeableConcept(value); // CodeableConcept 589 } else if (name.equals("dateCriterion")) { 590 this.getDateCriterion().add((ImmunizationRecommendationRecommendationDateCriterionComponent) value); 591 } else if (name.equals("protocol")) { 592 this.protocol = (ImmunizationRecommendationRecommendationProtocolComponent) value; // ImmunizationRecommendationRecommendationProtocolComponent 593 } else if (name.equals("supportingImmunization")) { 594 this.getSupportingImmunization().add(castToReference(value)); 595 } else if (name.equals("supportingPatientInformation")) { 596 this.getSupportingPatientInformation().add(castToReference(value)); 597 } else 598 return super.setProperty(name, value); 599 return value; 600 } 601 602 @Override 603 public Base makeProperty(int hash, String name) throws FHIRException { 604 switch (hash) { 605 case 3076014: return getDateElement(); 606 case 664556354: return getVaccineCode(); 607 case -319593813: return getTargetDisease(); 608 case -887709242: return getDoseNumberElement(); 609 case 1904598477: return getForecastStatus(); 610 case 2087518867: return addDateCriterion(); 611 case -989163880: return getProtocol(); 612 case 1171592021: return addSupportingImmunization(); 613 case -1234160646: return addSupportingPatientInformation(); 614 default: return super.makeProperty(hash, name); 615 } 616 617 } 618 619 @Override 620 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 621 switch (hash) { 622 case 3076014: /*date*/ return new String[] {"dateTime"}; 623 case 664556354: /*vaccineCode*/ return new String[] {"CodeableConcept"}; 624 case -319593813: /*targetDisease*/ return new String[] {"CodeableConcept"}; 625 case -887709242: /*doseNumber*/ return new String[] {"positiveInt"}; 626 case 1904598477: /*forecastStatus*/ return new String[] {"CodeableConcept"}; 627 case 2087518867: /*dateCriterion*/ return new String[] {}; 628 case -989163880: /*protocol*/ return new String[] {}; 629 case 1171592021: /*supportingImmunization*/ return new String[] {"Reference"}; 630 case -1234160646: /*supportingPatientInformation*/ return new String[] {"Reference"}; 631 default: return super.getTypesForProperty(hash, name); 632 } 633 634 } 635 636 @Override 637 public Base addChild(String name) throws FHIRException { 638 if (name.equals("date")) { 639 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.date"); 640 } 641 else if (name.equals("vaccineCode")) { 642 this.vaccineCode = new CodeableConcept(); 643 return this.vaccineCode; 644 } 645 else if (name.equals("targetDisease")) { 646 this.targetDisease = new CodeableConcept(); 647 return this.targetDisease; 648 } 649 else if (name.equals("doseNumber")) { 650 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.doseNumber"); 651 } 652 else if (name.equals("forecastStatus")) { 653 this.forecastStatus = new CodeableConcept(); 654 return this.forecastStatus; 655 } 656 else if (name.equals("dateCriterion")) { 657 return addDateCriterion(); 658 } 659 else if (name.equals("protocol")) { 660 this.protocol = new ImmunizationRecommendationRecommendationProtocolComponent(); 661 return this.protocol; 662 } 663 else if (name.equals("supportingImmunization")) { 664 return addSupportingImmunization(); 665 } 666 else if (name.equals("supportingPatientInformation")) { 667 return addSupportingPatientInformation(); 668 } 669 else 670 return super.addChild(name); 671 } 672 673 public ImmunizationRecommendationRecommendationComponent copy() { 674 ImmunizationRecommendationRecommendationComponent dst = new ImmunizationRecommendationRecommendationComponent(); 675 copyValues(dst); 676 dst.date = date == null ? null : date.copy(); 677 dst.vaccineCode = vaccineCode == null ? null : vaccineCode.copy(); 678 dst.targetDisease = targetDisease == null ? null : targetDisease.copy(); 679 dst.doseNumber = doseNumber == null ? null : doseNumber.copy(); 680 dst.forecastStatus = forecastStatus == null ? null : forecastStatus.copy(); 681 if (dateCriterion != null) { 682 dst.dateCriterion = new ArrayList<ImmunizationRecommendationRecommendationDateCriterionComponent>(); 683 for (ImmunizationRecommendationRecommendationDateCriterionComponent i : dateCriterion) 684 dst.dateCriterion.add(i.copy()); 685 }; 686 dst.protocol = protocol == null ? null : protocol.copy(); 687 if (supportingImmunization != null) { 688 dst.supportingImmunization = new ArrayList<Reference>(); 689 for (Reference i : supportingImmunization) 690 dst.supportingImmunization.add(i.copy()); 691 }; 692 if (supportingPatientInformation != null) { 693 dst.supportingPatientInformation = new ArrayList<Reference>(); 694 for (Reference i : supportingPatientInformation) 695 dst.supportingPatientInformation.add(i.copy()); 696 }; 697 return dst; 698 } 699 700 @Override 701 public boolean equalsDeep(Base other_) { 702 if (!super.equalsDeep(other_)) 703 return false; 704 if (!(other_ instanceof ImmunizationRecommendationRecommendationComponent)) 705 return false; 706 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other_; 707 return compareDeep(date, o.date, true) && compareDeep(vaccineCode, o.vaccineCode, true) && compareDeep(targetDisease, o.targetDisease, true) 708 && compareDeep(doseNumber, o.doseNumber, true) && compareDeep(forecastStatus, o.forecastStatus, true) 709 && compareDeep(dateCriterion, o.dateCriterion, true) && compareDeep(protocol, o.protocol, true) 710 && compareDeep(supportingImmunization, o.supportingImmunization, true) && compareDeep(supportingPatientInformation, o.supportingPatientInformation, true) 711 ; 712 } 713 714 @Override 715 public boolean equalsShallow(Base other_) { 716 if (!super.equalsShallow(other_)) 717 return false; 718 if (!(other_ instanceof ImmunizationRecommendationRecommendationComponent)) 719 return false; 720 ImmunizationRecommendationRecommendationComponent o = (ImmunizationRecommendationRecommendationComponent) other_; 721 return compareValues(date, o.date, true) && compareValues(doseNumber, o.doseNumber, true); 722 } 723 724 public boolean isEmpty() { 725 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(date, vaccineCode, targetDisease 726 , doseNumber, forecastStatus, dateCriterion, protocol, supportingImmunization, supportingPatientInformation 727 ); 728 } 729 730 public String fhirType() { 731 return "ImmunizationRecommendation.recommendation"; 732 733 } 734 735 } 736 737 @Block() 738 public static class ImmunizationRecommendationRecommendationDateCriterionComponent extends BackboneElement implements IBaseBackboneElement { 739 /** 740 * Date classification of recommendation. For example, earliest date to give, latest date to give, etc. 741 */ 742 @Child(name = "code", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=false) 743 @Description(shortDefinition="Type of date", formalDefinition="Date classification of recommendation. For example, earliest date to give, latest date to give, etc." ) 744 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/immunization-recommendation-date-criterion") 745 protected CodeableConcept code; 746 747 /** 748 * The date whose meaning is specified by dateCriterion.code. 749 */ 750 @Child(name = "value", type = {DateTimeType.class}, order=2, min=1, max=1, modifier=false, summary=false) 751 @Description(shortDefinition="Recommended date", formalDefinition="The date whose meaning is specified by dateCriterion.code." ) 752 protected DateTimeType value; 753 754 private static final long serialVersionUID = 1036994566L; 755 756 /** 757 * Constructor 758 */ 759 public ImmunizationRecommendationRecommendationDateCriterionComponent() { 760 super(); 761 } 762 763 /** 764 * Constructor 765 */ 766 public ImmunizationRecommendationRecommendationDateCriterionComponent(CodeableConcept code, DateTimeType value) { 767 super(); 768 this.code = code; 769 this.value = value; 770 } 771 772 /** 773 * @return {@link #code} (Date classification of recommendation. For example, earliest date to give, latest date to give, etc.) 774 */ 775 public CodeableConcept getCode() { 776 if (this.code == null) 777 if (Configuration.errorOnAutoCreate()) 778 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.code"); 779 else if (Configuration.doAutoCreate()) 780 this.code = new CodeableConcept(); // cc 781 return this.code; 782 } 783 784 public boolean hasCode() { 785 return this.code != null && !this.code.isEmpty(); 786 } 787 788 /** 789 * @param value {@link #code} (Date classification of recommendation. For example, earliest date to give, latest date to give, etc.) 790 */ 791 public ImmunizationRecommendationRecommendationDateCriterionComponent setCode(CodeableConcept value) { 792 this.code = value; 793 return this; 794 } 795 796 /** 797 * @return {@link #value} (The date whose meaning is specified by dateCriterion.code.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 798 */ 799 public DateTimeType getValueElement() { 800 if (this.value == null) 801 if (Configuration.errorOnAutoCreate()) 802 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationDateCriterionComponent.value"); 803 else if (Configuration.doAutoCreate()) 804 this.value = new DateTimeType(); // bb 805 return this.value; 806 } 807 808 public boolean hasValueElement() { 809 return this.value != null && !this.value.isEmpty(); 810 } 811 812 public boolean hasValue() { 813 return this.value != null && !this.value.isEmpty(); 814 } 815 816 /** 817 * @param value {@link #value} (The date whose meaning is specified by dateCriterion.code.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 818 */ 819 public ImmunizationRecommendationRecommendationDateCriterionComponent setValueElement(DateTimeType value) { 820 this.value = value; 821 return this; 822 } 823 824 /** 825 * @return The date whose meaning is specified by dateCriterion.code. 826 */ 827 public Date getValue() { 828 return this.value == null ? null : this.value.getValue(); 829 } 830 831 /** 832 * @param value The date whose meaning is specified by dateCriterion.code. 833 */ 834 public ImmunizationRecommendationRecommendationDateCriterionComponent setValue(Date value) { 835 if (this.value == null) 836 this.value = new DateTimeType(); 837 this.value.setValue(value); 838 return this; 839 } 840 841 protected void listChildren(List<Property> children) { 842 super.listChildren(children); 843 children.add(new Property("code", "CodeableConcept", "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 1, code)); 844 children.add(new Property("value", "dateTime", "The date whose meaning is specified by dateCriterion.code.", 0, 1, value)); 845 } 846 847 @Override 848 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 849 switch (_hash) { 850 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "Date classification of recommendation. For example, earliest date to give, latest date to give, etc.", 0, 1, code); 851 case 111972721: /*value*/ return new Property("value", "dateTime", "The date whose meaning is specified by dateCriterion.code.", 0, 1, value); 852 default: return super.getNamedProperty(_hash, _name, _checkValid); 853 } 854 855 } 856 857 @Override 858 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 859 switch (hash) { 860 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 861 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // DateTimeType 862 default: return super.getProperty(hash, name, checkValid); 863 } 864 865 } 866 867 @Override 868 public Base setProperty(int hash, String name, Base value) throws FHIRException { 869 switch (hash) { 870 case 3059181: // code 871 this.code = castToCodeableConcept(value); // CodeableConcept 872 return value; 873 case 111972721: // value 874 this.value = castToDateTime(value); // DateTimeType 875 return value; 876 default: return super.setProperty(hash, name, value); 877 } 878 879 } 880 881 @Override 882 public Base setProperty(String name, Base value) throws FHIRException { 883 if (name.equals("code")) { 884 this.code = castToCodeableConcept(value); // CodeableConcept 885 } else if (name.equals("value")) { 886 this.value = castToDateTime(value); // DateTimeType 887 } else 888 return super.setProperty(name, value); 889 return value; 890 } 891 892 @Override 893 public Base makeProperty(int hash, String name) throws FHIRException { 894 switch (hash) { 895 case 3059181: return getCode(); 896 case 111972721: return getValueElement(); 897 default: return super.makeProperty(hash, name); 898 } 899 900 } 901 902 @Override 903 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 904 switch (hash) { 905 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 906 case 111972721: /*value*/ return new String[] {"dateTime"}; 907 default: return super.getTypesForProperty(hash, name); 908 } 909 910 } 911 912 @Override 913 public Base addChild(String name) throws FHIRException { 914 if (name.equals("code")) { 915 this.code = new CodeableConcept(); 916 return this.code; 917 } 918 else if (name.equals("value")) { 919 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.value"); 920 } 921 else 922 return super.addChild(name); 923 } 924 925 public ImmunizationRecommendationRecommendationDateCriterionComponent copy() { 926 ImmunizationRecommendationRecommendationDateCriterionComponent dst = new ImmunizationRecommendationRecommendationDateCriterionComponent(); 927 copyValues(dst); 928 dst.code = code == null ? null : code.copy(); 929 dst.value = value == null ? null : value.copy(); 930 return dst; 931 } 932 933 @Override 934 public boolean equalsDeep(Base other_) { 935 if (!super.equalsDeep(other_)) 936 return false; 937 if (!(other_ instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 938 return false; 939 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other_; 940 return compareDeep(code, o.code, true) && compareDeep(value, o.value, true); 941 } 942 943 @Override 944 public boolean equalsShallow(Base other_) { 945 if (!super.equalsShallow(other_)) 946 return false; 947 if (!(other_ instanceof ImmunizationRecommendationRecommendationDateCriterionComponent)) 948 return false; 949 ImmunizationRecommendationRecommendationDateCriterionComponent o = (ImmunizationRecommendationRecommendationDateCriterionComponent) other_; 950 return compareValues(value, o.value, true); 951 } 952 953 public boolean isEmpty() { 954 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, value); 955 } 956 957 public String fhirType() { 958 return "ImmunizationRecommendation.recommendation.dateCriterion"; 959 960 } 961 962 } 963 964 @Block() 965 public static class ImmunizationRecommendationRecommendationProtocolComponent extends BackboneElement implements IBaseBackboneElement { 966 /** 967 * Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol. 968 */ 969 @Child(name = "doseSequence", type = {PositiveIntType.class}, order=1, min=0, max=1, modifier=false, summary=false) 970 @Description(shortDefinition="Dose number within sequence", formalDefinition="Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol." ) 971 protected PositiveIntType doseSequence; 972 973 /** 974 * Contains the description about the protocol under which the vaccine was administered. 975 */ 976 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 977 @Description(shortDefinition="Protocol details", formalDefinition="Contains the description about the protocol under which the vaccine was administered." ) 978 protected StringType description; 979 980 /** 981 * Indicates the authority who published the protocol. For example, ACIP. 982 */ 983 @Child(name = "authority", type = {Organization.class}, order=3, min=0, max=1, modifier=false, summary=false) 984 @Description(shortDefinition="Who is responsible for protocol", formalDefinition="Indicates the authority who published the protocol. For example, ACIP." ) 985 protected Reference authority; 986 987 /** 988 * The actual object that is the target of the reference (Indicates the authority who published the protocol. For example, ACIP.) 989 */ 990 protected Organization authorityTarget; 991 992 /** 993 * One possible path to achieve presumed immunity against a disease - within the context of an authority. 994 */ 995 @Child(name = "series", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 996 @Description(shortDefinition="Name of vaccination series", formalDefinition="One possible path to achieve presumed immunity against a disease - within the context of an authority." ) 997 protected StringType series; 998 999 private static final long serialVersionUID = 215094970L; 1000 1001 /** 1002 * Constructor 1003 */ 1004 public ImmunizationRecommendationRecommendationProtocolComponent() { 1005 super(); 1006 } 1007 1008 /** 1009 * @return {@link #doseSequence} (Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol.). This is the underlying object with id, value and extensions. The accessor "getDoseSequence" gives direct access to the value 1010 */ 1011 public PositiveIntType getDoseSequenceElement() { 1012 if (this.doseSequence == null) 1013 if (Configuration.errorOnAutoCreate()) 1014 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.doseSequence"); 1015 else if (Configuration.doAutoCreate()) 1016 this.doseSequence = new PositiveIntType(); // bb 1017 return this.doseSequence; 1018 } 1019 1020 public boolean hasDoseSequenceElement() { 1021 return this.doseSequence != null && !this.doseSequence.isEmpty(); 1022 } 1023 1024 public boolean hasDoseSequence() { 1025 return this.doseSequence != null && !this.doseSequence.isEmpty(); 1026 } 1027 1028 /** 1029 * @param value {@link #doseSequence} (Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol.). This is the underlying object with id, value and extensions. The accessor "getDoseSequence" gives direct access to the value 1030 */ 1031 public ImmunizationRecommendationRecommendationProtocolComponent setDoseSequenceElement(PositiveIntType value) { 1032 this.doseSequence = value; 1033 return this; 1034 } 1035 1036 /** 1037 * @return Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol. 1038 */ 1039 public int getDoseSequence() { 1040 return this.doseSequence == null || this.doseSequence.isEmpty() ? 0 : this.doseSequence.getValue(); 1041 } 1042 1043 /** 1044 * @param value Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol. 1045 */ 1046 public ImmunizationRecommendationRecommendationProtocolComponent setDoseSequence(int value) { 1047 if (this.doseSequence == null) 1048 this.doseSequence = new PositiveIntType(); 1049 this.doseSequence.setValue(value); 1050 return this; 1051 } 1052 1053 /** 1054 * @return {@link #description} (Contains the description about the protocol under which the vaccine was administered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1055 */ 1056 public StringType getDescriptionElement() { 1057 if (this.description == null) 1058 if (Configuration.errorOnAutoCreate()) 1059 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.description"); 1060 else if (Configuration.doAutoCreate()) 1061 this.description = new StringType(); // bb 1062 return this.description; 1063 } 1064 1065 public boolean hasDescriptionElement() { 1066 return this.description != null && !this.description.isEmpty(); 1067 } 1068 1069 public boolean hasDescription() { 1070 return this.description != null && !this.description.isEmpty(); 1071 } 1072 1073 /** 1074 * @param value {@link #description} (Contains the description about the protocol under which the vaccine was administered.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1075 */ 1076 public ImmunizationRecommendationRecommendationProtocolComponent setDescriptionElement(StringType value) { 1077 this.description = value; 1078 return this; 1079 } 1080 1081 /** 1082 * @return Contains the description about the protocol under which the vaccine was administered. 1083 */ 1084 public String getDescription() { 1085 return this.description == null ? null : this.description.getValue(); 1086 } 1087 1088 /** 1089 * @param value Contains the description about the protocol under which the vaccine was administered. 1090 */ 1091 public ImmunizationRecommendationRecommendationProtocolComponent setDescription(String value) { 1092 if (Utilities.noString(value)) 1093 this.description = null; 1094 else { 1095 if (this.description == null) 1096 this.description = new StringType(); 1097 this.description.setValue(value); 1098 } 1099 return this; 1100 } 1101 1102 /** 1103 * @return {@link #authority} (Indicates the authority who published the protocol. For example, ACIP.) 1104 */ 1105 public Reference getAuthority() { 1106 if (this.authority == null) 1107 if (Configuration.errorOnAutoCreate()) 1108 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.authority"); 1109 else if (Configuration.doAutoCreate()) 1110 this.authority = new Reference(); // cc 1111 return this.authority; 1112 } 1113 1114 public boolean hasAuthority() { 1115 return this.authority != null && !this.authority.isEmpty(); 1116 } 1117 1118 /** 1119 * @param value {@link #authority} (Indicates the authority who published the protocol. For example, ACIP.) 1120 */ 1121 public ImmunizationRecommendationRecommendationProtocolComponent setAuthority(Reference value) { 1122 this.authority = value; 1123 return this; 1124 } 1125 1126 /** 1127 * @return {@link #authority} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the authority who published the protocol. For example, ACIP.) 1128 */ 1129 public Organization getAuthorityTarget() { 1130 if (this.authorityTarget == null) 1131 if (Configuration.errorOnAutoCreate()) 1132 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.authority"); 1133 else if (Configuration.doAutoCreate()) 1134 this.authorityTarget = new Organization(); // aa 1135 return this.authorityTarget; 1136 } 1137 1138 /** 1139 * @param value {@link #authority} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the authority who published the protocol. For example, ACIP.) 1140 */ 1141 public ImmunizationRecommendationRecommendationProtocolComponent setAuthorityTarget(Organization value) { 1142 this.authorityTarget = value; 1143 return this; 1144 } 1145 1146 /** 1147 * @return {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 1148 */ 1149 public StringType getSeriesElement() { 1150 if (this.series == null) 1151 if (Configuration.errorOnAutoCreate()) 1152 throw new Error("Attempt to auto-create ImmunizationRecommendationRecommendationProtocolComponent.series"); 1153 else if (Configuration.doAutoCreate()) 1154 this.series = new StringType(); // bb 1155 return this.series; 1156 } 1157 1158 public boolean hasSeriesElement() { 1159 return this.series != null && !this.series.isEmpty(); 1160 } 1161 1162 public boolean hasSeries() { 1163 return this.series != null && !this.series.isEmpty(); 1164 } 1165 1166 /** 1167 * @param value {@link #series} (One possible path to achieve presumed immunity against a disease - within the context of an authority.). This is the underlying object with id, value and extensions. The accessor "getSeries" gives direct access to the value 1168 */ 1169 public ImmunizationRecommendationRecommendationProtocolComponent setSeriesElement(StringType value) { 1170 this.series = value; 1171 return this; 1172 } 1173 1174 /** 1175 * @return One possible path to achieve presumed immunity against a disease - within the context of an authority. 1176 */ 1177 public String getSeries() { 1178 return this.series == null ? null : this.series.getValue(); 1179 } 1180 1181 /** 1182 * @param value One possible path to achieve presumed immunity against a disease - within the context of an authority. 1183 */ 1184 public ImmunizationRecommendationRecommendationProtocolComponent setSeries(String value) { 1185 if (Utilities.noString(value)) 1186 this.series = null; 1187 else { 1188 if (this.series == null) 1189 this.series = new StringType(); 1190 this.series.setValue(value); 1191 } 1192 return this; 1193 } 1194 1195 protected void listChildren(List<Property> children) { 1196 super.listChildren(children); 1197 children.add(new Property("doseSequence", "positiveInt", "Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol.", 0, 1, doseSequence)); 1198 children.add(new Property("description", "string", "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description)); 1199 children.add(new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol. For example, ACIP.", 0, 1, authority)); 1200 children.add(new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series)); 1201 } 1202 1203 @Override 1204 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1205 switch (_hash) { 1206 case 550933246: /*doseSequence*/ return new Property("doseSequence", "positiveInt", "Indicates the nominal position in a series of the next dose. This is the recommended dose number as per a specified protocol.", 0, 1, doseSequence); 1207 case -1724546052: /*description*/ return new Property("description", "string", "Contains the description about the protocol under which the vaccine was administered.", 0, 1, description); 1208 case 1475610435: /*authority*/ return new Property("authority", "Reference(Organization)", "Indicates the authority who published the protocol. For example, ACIP.", 0, 1, authority); 1209 case -905838985: /*series*/ return new Property("series", "string", "One possible path to achieve presumed immunity against a disease - within the context of an authority.", 0, 1, series); 1210 default: return super.getNamedProperty(_hash, _name, _checkValid); 1211 } 1212 1213 } 1214 1215 @Override 1216 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1217 switch (hash) { 1218 case 550933246: /*doseSequence*/ return this.doseSequence == null ? new Base[0] : new Base[] {this.doseSequence}; // PositiveIntType 1219 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1220 case 1475610435: /*authority*/ return this.authority == null ? new Base[0] : new Base[] {this.authority}; // Reference 1221 case -905838985: /*series*/ return this.series == null ? new Base[0] : new Base[] {this.series}; // StringType 1222 default: return super.getProperty(hash, name, checkValid); 1223 } 1224 1225 } 1226 1227 @Override 1228 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1229 switch (hash) { 1230 case 550933246: // doseSequence 1231 this.doseSequence = castToPositiveInt(value); // PositiveIntType 1232 return value; 1233 case -1724546052: // description 1234 this.description = castToString(value); // StringType 1235 return value; 1236 case 1475610435: // authority 1237 this.authority = castToReference(value); // Reference 1238 return value; 1239 case -905838985: // series 1240 this.series = castToString(value); // StringType 1241 return value; 1242 default: return super.setProperty(hash, name, value); 1243 } 1244 1245 } 1246 1247 @Override 1248 public Base setProperty(String name, Base value) throws FHIRException { 1249 if (name.equals("doseSequence")) { 1250 this.doseSequence = castToPositiveInt(value); // PositiveIntType 1251 } else if (name.equals("description")) { 1252 this.description = castToString(value); // StringType 1253 } else if (name.equals("authority")) { 1254 this.authority = castToReference(value); // Reference 1255 } else if (name.equals("series")) { 1256 this.series = castToString(value); // StringType 1257 } else 1258 return super.setProperty(name, value); 1259 return value; 1260 } 1261 1262 @Override 1263 public Base makeProperty(int hash, String name) throws FHIRException { 1264 switch (hash) { 1265 case 550933246: return getDoseSequenceElement(); 1266 case -1724546052: return getDescriptionElement(); 1267 case 1475610435: return getAuthority(); 1268 case -905838985: return getSeriesElement(); 1269 default: return super.makeProperty(hash, name); 1270 } 1271 1272 } 1273 1274 @Override 1275 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1276 switch (hash) { 1277 case 550933246: /*doseSequence*/ return new String[] {"positiveInt"}; 1278 case -1724546052: /*description*/ return new String[] {"string"}; 1279 case 1475610435: /*authority*/ return new String[] {"Reference"}; 1280 case -905838985: /*series*/ return new String[] {"string"}; 1281 default: return super.getTypesForProperty(hash, name); 1282 } 1283 1284 } 1285 1286 @Override 1287 public Base addChild(String name) throws FHIRException { 1288 if (name.equals("doseSequence")) { 1289 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.doseSequence"); 1290 } 1291 else if (name.equals("description")) { 1292 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.description"); 1293 } 1294 else if (name.equals("authority")) { 1295 this.authority = new Reference(); 1296 return this.authority; 1297 } 1298 else if (name.equals("series")) { 1299 throw new FHIRException("Cannot call addChild on a singleton property ImmunizationRecommendation.series"); 1300 } 1301 else 1302 return super.addChild(name); 1303 } 1304 1305 public ImmunizationRecommendationRecommendationProtocolComponent copy() { 1306 ImmunizationRecommendationRecommendationProtocolComponent dst = new ImmunizationRecommendationRecommendationProtocolComponent(); 1307 copyValues(dst); 1308 dst.doseSequence = doseSequence == null ? null : doseSequence.copy(); 1309 dst.description = description == null ? null : description.copy(); 1310 dst.authority = authority == null ? null : authority.copy(); 1311 dst.series = series == null ? null : series.copy(); 1312 return dst; 1313 } 1314 1315 @Override 1316 public boolean equalsDeep(Base other_) { 1317 if (!super.equalsDeep(other_)) 1318 return false; 1319 if (!(other_ instanceof ImmunizationRecommendationRecommendationProtocolComponent)) 1320 return false; 1321 ImmunizationRecommendationRecommendationProtocolComponent o = (ImmunizationRecommendationRecommendationProtocolComponent) other_; 1322 return compareDeep(doseSequence, o.doseSequence, true) && compareDeep(description, o.description, true) 1323 && compareDeep(authority, o.authority, true) && compareDeep(series, o.series, true); 1324 } 1325 1326 @Override 1327 public boolean equalsShallow(Base other_) { 1328 if (!super.equalsShallow(other_)) 1329 return false; 1330 if (!(other_ instanceof ImmunizationRecommendationRecommendationProtocolComponent)) 1331 return false; 1332 ImmunizationRecommendationRecommendationProtocolComponent o = (ImmunizationRecommendationRecommendationProtocolComponent) other_; 1333 return compareValues(doseSequence, o.doseSequence, true) && compareValues(description, o.description, true) 1334 && compareValues(series, o.series, true); 1335 } 1336 1337 public boolean isEmpty() { 1338 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(doseSequence, description 1339 , authority, series); 1340 } 1341 1342 public String fhirType() { 1343 return "ImmunizationRecommendation.recommendation.protocol"; 1344 1345 } 1346 1347 } 1348 1349 /** 1350 * A unique identifier assigned to this particular recommendation record. 1351 */ 1352 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1353 @Description(shortDefinition="Business identifier", formalDefinition="A unique identifier assigned to this particular recommendation record." ) 1354 protected List<Identifier> identifier; 1355 1356 /** 1357 * The patient the recommendations are for. 1358 */ 1359 @Child(name = "patient", type = {Patient.class}, order=1, min=1, max=1, modifier=false, summary=true) 1360 @Description(shortDefinition="Who this profile is for", formalDefinition="The patient the recommendations are for." ) 1361 protected Reference patient; 1362 1363 /** 1364 * The actual object that is the target of the reference (The patient the recommendations are for.) 1365 */ 1366 protected Patient patientTarget; 1367 1368 /** 1369 * Vaccine administration recommendations. 1370 */ 1371 @Child(name = "recommendation", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1372 @Description(shortDefinition="Vaccine administration recommendations", formalDefinition="Vaccine administration recommendations." ) 1373 protected List<ImmunizationRecommendationRecommendationComponent> recommendation; 1374 1375 private static final long serialVersionUID = 641058495L; 1376 1377 /** 1378 * Constructor 1379 */ 1380 public ImmunizationRecommendation() { 1381 super(); 1382 } 1383 1384 /** 1385 * Constructor 1386 */ 1387 public ImmunizationRecommendation(Reference patient) { 1388 super(); 1389 this.patient = patient; 1390 } 1391 1392 /** 1393 * @return {@link #identifier} (A unique identifier assigned to this particular recommendation record.) 1394 */ 1395 public List<Identifier> getIdentifier() { 1396 if (this.identifier == null) 1397 this.identifier = new ArrayList<Identifier>(); 1398 return this.identifier; 1399 } 1400 1401 /** 1402 * @return Returns a reference to <code>this</code> for easy method chaining 1403 */ 1404 public ImmunizationRecommendation setIdentifier(List<Identifier> theIdentifier) { 1405 this.identifier = theIdentifier; 1406 return this; 1407 } 1408 1409 public boolean hasIdentifier() { 1410 if (this.identifier == null) 1411 return false; 1412 for (Identifier item : this.identifier) 1413 if (!item.isEmpty()) 1414 return true; 1415 return false; 1416 } 1417 1418 public Identifier addIdentifier() { //3 1419 Identifier t = new Identifier(); 1420 if (this.identifier == null) 1421 this.identifier = new ArrayList<Identifier>(); 1422 this.identifier.add(t); 1423 return t; 1424 } 1425 1426 public ImmunizationRecommendation addIdentifier(Identifier t) { //3 1427 if (t == null) 1428 return this; 1429 if (this.identifier == null) 1430 this.identifier = new ArrayList<Identifier>(); 1431 this.identifier.add(t); 1432 return this; 1433 } 1434 1435 /** 1436 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1437 */ 1438 public Identifier getIdentifierFirstRep() { 1439 if (getIdentifier().isEmpty()) { 1440 addIdentifier(); 1441 } 1442 return getIdentifier().get(0); 1443 } 1444 1445 /** 1446 * @return {@link #patient} (The patient the recommendations are for.) 1447 */ 1448 public Reference getPatient() { 1449 if (this.patient == null) 1450 if (Configuration.errorOnAutoCreate()) 1451 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1452 else if (Configuration.doAutoCreate()) 1453 this.patient = new Reference(); // cc 1454 return this.patient; 1455 } 1456 1457 public boolean hasPatient() { 1458 return this.patient != null && !this.patient.isEmpty(); 1459 } 1460 1461 /** 1462 * @param value {@link #patient} (The patient the recommendations are for.) 1463 */ 1464 public ImmunizationRecommendation setPatient(Reference value) { 1465 this.patient = value; 1466 return this; 1467 } 1468 1469 /** 1470 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The patient the recommendations are for.) 1471 */ 1472 public Patient getPatientTarget() { 1473 if (this.patientTarget == null) 1474 if (Configuration.errorOnAutoCreate()) 1475 throw new Error("Attempt to auto-create ImmunizationRecommendation.patient"); 1476 else if (Configuration.doAutoCreate()) 1477 this.patientTarget = new Patient(); // aa 1478 return this.patientTarget; 1479 } 1480 1481 /** 1482 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The patient the recommendations are for.) 1483 */ 1484 public ImmunizationRecommendation setPatientTarget(Patient value) { 1485 this.patientTarget = value; 1486 return this; 1487 } 1488 1489 /** 1490 * @return {@link #recommendation} (Vaccine administration recommendations.) 1491 */ 1492 public List<ImmunizationRecommendationRecommendationComponent> getRecommendation() { 1493 if (this.recommendation == null) 1494 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1495 return this.recommendation; 1496 } 1497 1498 /** 1499 * @return Returns a reference to <code>this</code> for easy method chaining 1500 */ 1501 public ImmunizationRecommendation setRecommendation(List<ImmunizationRecommendationRecommendationComponent> theRecommendation) { 1502 this.recommendation = theRecommendation; 1503 return this; 1504 } 1505 1506 public boolean hasRecommendation() { 1507 if (this.recommendation == null) 1508 return false; 1509 for (ImmunizationRecommendationRecommendationComponent item : this.recommendation) 1510 if (!item.isEmpty()) 1511 return true; 1512 return false; 1513 } 1514 1515 public ImmunizationRecommendationRecommendationComponent addRecommendation() { //3 1516 ImmunizationRecommendationRecommendationComponent t = new ImmunizationRecommendationRecommendationComponent(); 1517 if (this.recommendation == null) 1518 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1519 this.recommendation.add(t); 1520 return t; 1521 } 1522 1523 public ImmunizationRecommendation addRecommendation(ImmunizationRecommendationRecommendationComponent t) { //3 1524 if (t == null) 1525 return this; 1526 if (this.recommendation == null) 1527 this.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1528 this.recommendation.add(t); 1529 return this; 1530 } 1531 1532 /** 1533 * @return The first repetition of repeating field {@link #recommendation}, creating it if it does not already exist 1534 */ 1535 public ImmunizationRecommendationRecommendationComponent getRecommendationFirstRep() { 1536 if (getRecommendation().isEmpty()) { 1537 addRecommendation(); 1538 } 1539 return getRecommendation().get(0); 1540 } 1541 1542 protected void listChildren(List<Property> children) { 1543 super.listChildren(children); 1544 children.add(new Property("identifier", "Identifier", "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1545 children.add(new Property("patient", "Reference(Patient)", "The patient the recommendations are for.", 0, 1, patient)); 1546 children.add(new Property("recommendation", "", "Vaccine administration recommendations.", 0, java.lang.Integer.MAX_VALUE, recommendation)); 1547 } 1548 1549 @Override 1550 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1551 switch (_hash) { 1552 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier assigned to this particular recommendation record.", 0, java.lang.Integer.MAX_VALUE, identifier); 1553 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "The patient the recommendations are for.", 0, 1, patient); 1554 case -1028636743: /*recommendation*/ return new Property("recommendation", "", "Vaccine administration recommendations.", 0, java.lang.Integer.MAX_VALUE, recommendation); 1555 default: return super.getNamedProperty(_hash, _name, _checkValid); 1556 } 1557 1558 } 1559 1560 @Override 1561 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1562 switch (hash) { 1563 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1564 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 1565 case -1028636743: /*recommendation*/ return this.recommendation == null ? new Base[0] : this.recommendation.toArray(new Base[this.recommendation.size()]); // ImmunizationRecommendationRecommendationComponent 1566 default: return super.getProperty(hash, name, checkValid); 1567 } 1568 1569 } 1570 1571 @Override 1572 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1573 switch (hash) { 1574 case -1618432855: // identifier 1575 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1576 return value; 1577 case -791418107: // patient 1578 this.patient = castToReference(value); // Reference 1579 return value; 1580 case -1028636743: // recommendation 1581 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); // ImmunizationRecommendationRecommendationComponent 1582 return value; 1583 default: return super.setProperty(hash, name, value); 1584 } 1585 1586 } 1587 1588 @Override 1589 public Base setProperty(String name, Base value) throws FHIRException { 1590 if (name.equals("identifier")) { 1591 this.getIdentifier().add(castToIdentifier(value)); 1592 } else if (name.equals("patient")) { 1593 this.patient = castToReference(value); // Reference 1594 } else if (name.equals("recommendation")) { 1595 this.getRecommendation().add((ImmunizationRecommendationRecommendationComponent) value); 1596 } else 1597 return super.setProperty(name, value); 1598 return value; 1599 } 1600 1601 @Override 1602 public Base makeProperty(int hash, String name) throws FHIRException { 1603 switch (hash) { 1604 case -1618432855: return addIdentifier(); 1605 case -791418107: return getPatient(); 1606 case -1028636743: return addRecommendation(); 1607 default: return super.makeProperty(hash, name); 1608 } 1609 1610 } 1611 1612 @Override 1613 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1614 switch (hash) { 1615 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1616 case -791418107: /*patient*/ return new String[] {"Reference"}; 1617 case -1028636743: /*recommendation*/ return new String[] {}; 1618 default: return super.getTypesForProperty(hash, name); 1619 } 1620 1621 } 1622 1623 @Override 1624 public Base addChild(String name) throws FHIRException { 1625 if (name.equals("identifier")) { 1626 return addIdentifier(); 1627 } 1628 else if (name.equals("patient")) { 1629 this.patient = new Reference(); 1630 return this.patient; 1631 } 1632 else if (name.equals("recommendation")) { 1633 return addRecommendation(); 1634 } 1635 else 1636 return super.addChild(name); 1637 } 1638 1639 public String fhirType() { 1640 return "ImmunizationRecommendation"; 1641 1642 } 1643 1644 public ImmunizationRecommendation copy() { 1645 ImmunizationRecommendation dst = new ImmunizationRecommendation(); 1646 copyValues(dst); 1647 if (identifier != null) { 1648 dst.identifier = new ArrayList<Identifier>(); 1649 for (Identifier i : identifier) 1650 dst.identifier.add(i.copy()); 1651 }; 1652 dst.patient = patient == null ? null : patient.copy(); 1653 if (recommendation != null) { 1654 dst.recommendation = new ArrayList<ImmunizationRecommendationRecommendationComponent>(); 1655 for (ImmunizationRecommendationRecommendationComponent i : recommendation) 1656 dst.recommendation.add(i.copy()); 1657 }; 1658 return dst; 1659 } 1660 1661 protected ImmunizationRecommendation typedCopy() { 1662 return copy(); 1663 } 1664 1665 @Override 1666 public boolean equalsDeep(Base other_) { 1667 if (!super.equalsDeep(other_)) 1668 return false; 1669 if (!(other_ instanceof ImmunizationRecommendation)) 1670 return false; 1671 ImmunizationRecommendation o = (ImmunizationRecommendation) other_; 1672 return compareDeep(identifier, o.identifier, true) && compareDeep(patient, o.patient, true) && compareDeep(recommendation, o.recommendation, true) 1673 ; 1674 } 1675 1676 @Override 1677 public boolean equalsShallow(Base other_) { 1678 if (!super.equalsShallow(other_)) 1679 return false; 1680 if (!(other_ instanceof ImmunizationRecommendation)) 1681 return false; 1682 ImmunizationRecommendation o = (ImmunizationRecommendation) other_; 1683 return true; 1684 } 1685 1686 public boolean isEmpty() { 1687 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, patient, recommendation 1688 ); 1689 } 1690 1691 @Override 1692 public ResourceType getResourceType() { 1693 return ResourceType.ImmunizationRecommendation; 1694 } 1695 1696 /** 1697 * Search parameter: <b>date</b> 1698 * <p> 1699 * Description: <b>Date recommendation created</b><br> 1700 * Type: <b>date</b><br> 1701 * Path: <b>ImmunizationRecommendation.recommendation.date</b><br> 1702 * </p> 1703 */ 1704 @SearchParamDefinition(name="date", path="ImmunizationRecommendation.recommendation.date", description="Date recommendation created", type="date" ) 1705 public static final String SP_DATE = "date"; 1706 /** 1707 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1708 * <p> 1709 * Description: <b>Date recommendation created</b><br> 1710 * Type: <b>date</b><br> 1711 * Path: <b>ImmunizationRecommendation.recommendation.date</b><br> 1712 * </p> 1713 */ 1714 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1715 1716 /** 1717 * Search parameter: <b>identifier</b> 1718 * <p> 1719 * Description: <b>Business identifier</b><br> 1720 * Type: <b>token</b><br> 1721 * Path: <b>ImmunizationRecommendation.identifier</b><br> 1722 * </p> 1723 */ 1724 @SearchParamDefinition(name="identifier", path="ImmunizationRecommendation.identifier", description="Business identifier", type="token" ) 1725 public static final String SP_IDENTIFIER = "identifier"; 1726 /** 1727 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1728 * <p> 1729 * Description: <b>Business identifier</b><br> 1730 * Type: <b>token</b><br> 1731 * Path: <b>ImmunizationRecommendation.identifier</b><br> 1732 * </p> 1733 */ 1734 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1735 1736 /** 1737 * Search parameter: <b>dose-sequence</b> 1738 * <p> 1739 * Description: <b>Dose number within sequence</b><br> 1740 * Type: <b>number</b><br> 1741 * Path: <b>ImmunizationRecommendation.recommendation.protocol.doseSequence</b><br> 1742 * </p> 1743 */ 1744 @SearchParamDefinition(name="dose-sequence", path="ImmunizationRecommendation.recommendation.protocol.doseSequence", description="Dose number within sequence", type="number" ) 1745 public static final String SP_DOSE_SEQUENCE = "dose-sequence"; 1746 /** 1747 * <b>Fluent Client</b> search parameter constant for <b>dose-sequence</b> 1748 * <p> 1749 * Description: <b>Dose number within sequence</b><br> 1750 * Type: <b>number</b><br> 1751 * Path: <b>ImmunizationRecommendation.recommendation.protocol.doseSequence</b><br> 1752 * </p> 1753 */ 1754 public static final ca.uhn.fhir.rest.gclient.NumberClientParam DOSE_SEQUENCE = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_DOSE_SEQUENCE); 1755 1756 /** 1757 * Search parameter: <b>target-disease</b> 1758 * <p> 1759 * Description: <b>Disease to be immunized against</b><br> 1760 * Type: <b>token</b><br> 1761 * Path: <b>ImmunizationRecommendation.recommendation.targetDisease</b><br> 1762 * </p> 1763 */ 1764 @SearchParamDefinition(name="target-disease", path="ImmunizationRecommendation.recommendation.targetDisease", description="Disease to be immunized against", type="token" ) 1765 public static final String SP_TARGET_DISEASE = "target-disease"; 1766 /** 1767 * <b>Fluent Client</b> search parameter constant for <b>target-disease</b> 1768 * <p> 1769 * Description: <b>Disease to be immunized against</b><br> 1770 * Type: <b>token</b><br> 1771 * Path: <b>ImmunizationRecommendation.recommendation.targetDisease</b><br> 1772 * </p> 1773 */ 1774 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TARGET_DISEASE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TARGET_DISEASE); 1775 1776 /** 1777 * Search parameter: <b>patient</b> 1778 * <p> 1779 * Description: <b>Who this profile is for</b><br> 1780 * Type: <b>reference</b><br> 1781 * Path: <b>ImmunizationRecommendation.patient</b><br> 1782 * </p> 1783 */ 1784 @SearchParamDefinition(name="patient", path="ImmunizationRecommendation.patient", description="Who this profile is for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 1785 public static final String SP_PATIENT = "patient"; 1786 /** 1787 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1788 * <p> 1789 * Description: <b>Who this profile is for</b><br> 1790 * Type: <b>reference</b><br> 1791 * Path: <b>ImmunizationRecommendation.patient</b><br> 1792 * </p> 1793 */ 1794 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1795 1796/** 1797 * Constant for fluent queries to be used to add include statements. Specifies 1798 * the path value of "<b>ImmunizationRecommendation:patient</b>". 1799 */ 1800 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("ImmunizationRecommendation:patient").toLocked(); 1801 1802 /** 1803 * Search parameter: <b>vaccine-type</b> 1804 * <p> 1805 * Description: <b>Vaccine recommendation applies to</b><br> 1806 * Type: <b>token</b><br> 1807 * Path: <b>ImmunizationRecommendation.recommendation.vaccineCode</b><br> 1808 * </p> 1809 */ 1810 @SearchParamDefinition(name="vaccine-type", path="ImmunizationRecommendation.recommendation.vaccineCode", description="Vaccine recommendation applies to", type="token" ) 1811 public static final String SP_VACCINE_TYPE = "vaccine-type"; 1812 /** 1813 * <b>Fluent Client</b> search parameter constant for <b>vaccine-type</b> 1814 * <p> 1815 * Description: <b>Vaccine recommendation applies to</b><br> 1816 * Type: <b>token</b><br> 1817 * Path: <b>ImmunizationRecommendation.recommendation.vaccineCode</b><br> 1818 * </p> 1819 */ 1820 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VACCINE_TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VACCINE_TYPE); 1821 1822 /** 1823 * Search parameter: <b>dose-number</b> 1824 * <p> 1825 * Description: <b>Recommended dose number</b><br> 1826 * Type: <b>number</b><br> 1827 * Path: <b>ImmunizationRecommendation.recommendation.doseNumber</b><br> 1828 * </p> 1829 */ 1830 @SearchParamDefinition(name="dose-number", path="ImmunizationRecommendation.recommendation.doseNumber", description="Recommended dose number", type="number" ) 1831 public static final String SP_DOSE_NUMBER = "dose-number"; 1832 /** 1833 * <b>Fluent Client</b> search parameter constant for <b>dose-number</b> 1834 * <p> 1835 * Description: <b>Recommended dose number</b><br> 1836 * Type: <b>number</b><br> 1837 * Path: <b>ImmunizationRecommendation.recommendation.doseNumber</b><br> 1838 * </p> 1839 */ 1840 public static final ca.uhn.fhir.rest.gclient.NumberClientParam DOSE_NUMBER = new ca.uhn.fhir.rest.gclient.NumberClientParam(SP_DOSE_NUMBER); 1841 1842 /** 1843 * Search parameter: <b>information</b> 1844 * <p> 1845 * Description: <b>Patient observations supporting recommendation</b><br> 1846 * Type: <b>reference</b><br> 1847 * Path: <b>ImmunizationRecommendation.recommendation.supportingPatientInformation</b><br> 1848 * </p> 1849 */ 1850 @SearchParamDefinition(name="information", path="ImmunizationRecommendation.recommendation.supportingPatientInformation", description="Patient observations supporting recommendation", type="reference", target={AllergyIntolerance.class, Observation.class } ) 1851 public static final String SP_INFORMATION = "information"; 1852 /** 1853 * <b>Fluent Client</b> search parameter constant for <b>information</b> 1854 * <p> 1855 * Description: <b>Patient observations supporting recommendation</b><br> 1856 * Type: <b>reference</b><br> 1857 * Path: <b>ImmunizationRecommendation.recommendation.supportingPatientInformation</b><br> 1858 * </p> 1859 */ 1860 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INFORMATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INFORMATION); 1861 1862/** 1863 * Constant for fluent queries to be used to add include statements. Specifies 1864 * the path value of "<b>ImmunizationRecommendation:information</b>". 1865 */ 1866 public static final ca.uhn.fhir.model.api.Include INCLUDE_INFORMATION = new ca.uhn.fhir.model.api.Include("ImmunizationRecommendation:information").toLocked(); 1867 1868 /** 1869 * Search parameter: <b>support</b> 1870 * <p> 1871 * Description: <b>Past immunizations supporting recommendation</b><br> 1872 * Type: <b>reference</b><br> 1873 * Path: <b>ImmunizationRecommendation.recommendation.supportingImmunization</b><br> 1874 * </p> 1875 */ 1876 @SearchParamDefinition(name="support", path="ImmunizationRecommendation.recommendation.supportingImmunization", description="Past immunizations supporting recommendation", type="reference", target={Immunization.class } ) 1877 public static final String SP_SUPPORT = "support"; 1878 /** 1879 * <b>Fluent Client</b> search parameter constant for <b>support</b> 1880 * <p> 1881 * Description: <b>Past immunizations supporting recommendation</b><br> 1882 * Type: <b>reference</b><br> 1883 * Path: <b>ImmunizationRecommendation.recommendation.supportingImmunization</b><br> 1884 * </p> 1885 */ 1886 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUPPORT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUPPORT); 1887 1888/** 1889 * Constant for fluent queries to be used to add include statements. Specifies 1890 * the path value of "<b>ImmunizationRecommendation:support</b>". 1891 */ 1892 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUPPORT = new ca.uhn.fhir.model.api.Include("ImmunizationRecommendation:support").toLocked(); 1893 1894 /** 1895 * Search parameter: <b>status</b> 1896 * <p> 1897 * Description: <b>Vaccine administration status</b><br> 1898 * Type: <b>token</b><br> 1899 * Path: <b>ImmunizationRecommendation.recommendation.forecastStatus</b><br> 1900 * </p> 1901 */ 1902 @SearchParamDefinition(name="status", path="ImmunizationRecommendation.recommendation.forecastStatus", description="Vaccine administration status", type="token" ) 1903 public static final String SP_STATUS = "status"; 1904 /** 1905 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1906 * <p> 1907 * Description: <b>Vaccine administration status</b><br> 1908 * Type: <b>token</b><br> 1909 * Path: <b>ImmunizationRecommendation.recommendation.forecastStatus</b><br> 1910 * </p> 1911 */ 1912 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1913 1914 1915}