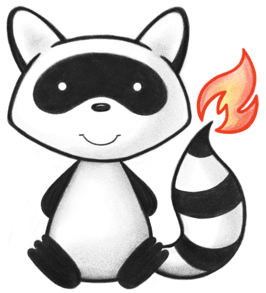
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.exceptions.FHIRFormatError; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044import org.hl7.fhir.utilities.Utilities; 045 046import ca.uhn.fhir.model.api.annotation.Block; 047import ca.uhn.fhir.model.api.annotation.Child; 048import ca.uhn.fhir.model.api.annotation.ChildOrder; 049import ca.uhn.fhir.model.api.annotation.Description; 050import ca.uhn.fhir.model.api.annotation.ResourceDef; 051import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 052/** 053 * A set of rules of how FHIR is used to solve a particular problem. This resource is used to gather all the parts of an implementation guide into a logical whole and to publish a computable definition of all the parts. 054 */ 055@ResourceDef(name="ImplementationGuide", profile="http://hl7.org/fhir/Profile/ImplementationGuide") 056@ChildOrder(names={"url", "version", "name", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "copyright", "fhirVersion", "dependency", "package", "global", "binary", "page"}) 057public class ImplementationGuide extends MetadataResource { 058 059 public enum GuideDependencyType { 060 /** 061 * The guide is referred to by URL. 062 */ 063 REFERENCE, 064 /** 065 * The guide is embedded in this guide when published. 066 */ 067 INCLUSION, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 public static GuideDependencyType fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("reference".equals(codeString)) 076 return REFERENCE; 077 if ("inclusion".equals(codeString)) 078 return INCLUSION; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown GuideDependencyType code '"+codeString+"'"); 083 } 084 public String toCode() { 085 switch (this) { 086 case REFERENCE: return "reference"; 087 case INCLUSION: return "inclusion"; 088 case NULL: return null; 089 default: return "?"; 090 } 091 } 092 public String getSystem() { 093 switch (this) { 094 case REFERENCE: return "http://hl7.org/fhir/guide-dependency-type"; 095 case INCLUSION: return "http://hl7.org/fhir/guide-dependency-type"; 096 case NULL: return null; 097 default: return "?"; 098 } 099 } 100 public String getDefinition() { 101 switch (this) { 102 case REFERENCE: return "The guide is referred to by URL."; 103 case INCLUSION: return "The guide is embedded in this guide when published."; 104 case NULL: return null; 105 default: return "?"; 106 } 107 } 108 public String getDisplay() { 109 switch (this) { 110 case REFERENCE: return "Reference"; 111 case INCLUSION: return "Inclusion"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 } 117 118 public static class GuideDependencyTypeEnumFactory implements EnumFactory<GuideDependencyType> { 119 public GuideDependencyType fromCode(String codeString) throws IllegalArgumentException { 120 if (codeString == null || "".equals(codeString)) 121 if (codeString == null || "".equals(codeString)) 122 return null; 123 if ("reference".equals(codeString)) 124 return GuideDependencyType.REFERENCE; 125 if ("inclusion".equals(codeString)) 126 return GuideDependencyType.INCLUSION; 127 throw new IllegalArgumentException("Unknown GuideDependencyType code '"+codeString+"'"); 128 } 129 public Enumeration<GuideDependencyType> fromType(PrimitiveType<?> code) throws FHIRException { 130 if (code == null) 131 return null; 132 if (code.isEmpty()) 133 return new Enumeration<GuideDependencyType>(this); 134 String codeString = code.asStringValue(); 135 if (codeString == null || "".equals(codeString)) 136 return null; 137 if ("reference".equals(codeString)) 138 return new Enumeration<GuideDependencyType>(this, GuideDependencyType.REFERENCE); 139 if ("inclusion".equals(codeString)) 140 return new Enumeration<GuideDependencyType>(this, GuideDependencyType.INCLUSION); 141 throw new FHIRException("Unknown GuideDependencyType code '"+codeString+"'"); 142 } 143 public String toCode(GuideDependencyType code) { 144 if (code == GuideDependencyType.NULL) 145 return null; 146 if (code == GuideDependencyType.REFERENCE) 147 return "reference"; 148 if (code == GuideDependencyType.INCLUSION) 149 return "inclusion"; 150 return "?"; 151 } 152 public String toSystem(GuideDependencyType code) { 153 return code.getSystem(); 154 } 155 } 156 157 public enum GuidePageKind { 158 /** 159 * This is a page of content that is included in the implementation guide. It has no particular function. 160 */ 161 PAGE, 162 /** 163 * This is a page that represents a human readable rendering of an example. 164 */ 165 EXAMPLE, 166 /** 167 * This is a page that represents a list of resources of one or more types. 168 */ 169 LIST, 170 /** 171 * This is a page showing where an included guide is injected. 172 */ 173 INCLUDE, 174 /** 175 * This is a page that lists the resources of a given type, and also creates pages for all the listed types as other pages in the section. 176 */ 177 DIRECTORY, 178 /** 179 * This is a page that creates the listed resources as a dictionary. 180 */ 181 DICTIONARY, 182 /** 183 * This is a generated page that contains the table of contents. 184 */ 185 TOC, 186 /** 187 * This is a page that represents a presented resource. This is typically used for generated conformance resource presentations. 188 */ 189 RESOURCE, 190 /** 191 * added to help the parsers with the generic types 192 */ 193 NULL; 194 public static GuidePageKind fromCode(String codeString) throws FHIRException { 195 if (codeString == null || "".equals(codeString)) 196 return null; 197 if ("page".equals(codeString)) 198 return PAGE; 199 if ("example".equals(codeString)) 200 return EXAMPLE; 201 if ("list".equals(codeString)) 202 return LIST; 203 if ("include".equals(codeString)) 204 return INCLUDE; 205 if ("directory".equals(codeString)) 206 return DIRECTORY; 207 if ("dictionary".equals(codeString)) 208 return DICTIONARY; 209 if ("toc".equals(codeString)) 210 return TOC; 211 if ("resource".equals(codeString)) 212 return RESOURCE; 213 if (Configuration.isAcceptInvalidEnums()) 214 return null; 215 else 216 throw new FHIRException("Unknown GuidePageKind code '"+codeString+"'"); 217 } 218 public String toCode() { 219 switch (this) { 220 case PAGE: return "page"; 221 case EXAMPLE: return "example"; 222 case LIST: return "list"; 223 case INCLUDE: return "include"; 224 case DIRECTORY: return "directory"; 225 case DICTIONARY: return "dictionary"; 226 case TOC: return "toc"; 227 case RESOURCE: return "resource"; 228 case NULL: return null; 229 default: return "?"; 230 } 231 } 232 public String getSystem() { 233 switch (this) { 234 case PAGE: return "http://hl7.org/fhir/guide-page-kind"; 235 case EXAMPLE: return "http://hl7.org/fhir/guide-page-kind"; 236 case LIST: return "http://hl7.org/fhir/guide-page-kind"; 237 case INCLUDE: return "http://hl7.org/fhir/guide-page-kind"; 238 case DIRECTORY: return "http://hl7.org/fhir/guide-page-kind"; 239 case DICTIONARY: return "http://hl7.org/fhir/guide-page-kind"; 240 case TOC: return "http://hl7.org/fhir/guide-page-kind"; 241 case RESOURCE: return "http://hl7.org/fhir/guide-page-kind"; 242 case NULL: return null; 243 default: return "?"; 244 } 245 } 246 public String getDefinition() { 247 switch (this) { 248 case PAGE: return "This is a page of content that is included in the implementation guide. It has no particular function."; 249 case EXAMPLE: return "This is a page that represents a human readable rendering of an example."; 250 case LIST: return "This is a page that represents a list of resources of one or more types."; 251 case INCLUDE: return "This is a page showing where an included guide is injected."; 252 case DIRECTORY: return "This is a page that lists the resources of a given type, and also creates pages for all the listed types as other pages in the section."; 253 case DICTIONARY: return "This is a page that creates the listed resources as a dictionary."; 254 case TOC: return "This is a generated page that contains the table of contents."; 255 case RESOURCE: return "This is a page that represents a presented resource. This is typically used for generated conformance resource presentations."; 256 case NULL: return null; 257 default: return "?"; 258 } 259 } 260 public String getDisplay() { 261 switch (this) { 262 case PAGE: return "Page"; 263 case EXAMPLE: return "Example"; 264 case LIST: return "List"; 265 case INCLUDE: return "Include"; 266 case DIRECTORY: return "Directory"; 267 case DICTIONARY: return "Dictionary"; 268 case TOC: return "Table Of Contents"; 269 case RESOURCE: return "Resource"; 270 case NULL: return null; 271 default: return "?"; 272 } 273 } 274 } 275 276 public static class GuidePageKindEnumFactory implements EnumFactory<GuidePageKind> { 277 public GuidePageKind fromCode(String codeString) throws IllegalArgumentException { 278 if (codeString == null || "".equals(codeString)) 279 if (codeString == null || "".equals(codeString)) 280 return null; 281 if ("page".equals(codeString)) 282 return GuidePageKind.PAGE; 283 if ("example".equals(codeString)) 284 return GuidePageKind.EXAMPLE; 285 if ("list".equals(codeString)) 286 return GuidePageKind.LIST; 287 if ("include".equals(codeString)) 288 return GuidePageKind.INCLUDE; 289 if ("directory".equals(codeString)) 290 return GuidePageKind.DIRECTORY; 291 if ("dictionary".equals(codeString)) 292 return GuidePageKind.DICTIONARY; 293 if ("toc".equals(codeString)) 294 return GuidePageKind.TOC; 295 if ("resource".equals(codeString)) 296 return GuidePageKind.RESOURCE; 297 throw new IllegalArgumentException("Unknown GuidePageKind code '"+codeString+"'"); 298 } 299 public Enumeration<GuidePageKind> fromType(PrimitiveType<?> code) throws FHIRException { 300 if (code == null) 301 return null; 302 if (code.isEmpty()) 303 return new Enumeration<GuidePageKind>(this); 304 String codeString = code.asStringValue(); 305 if (codeString == null || "".equals(codeString)) 306 return null; 307 if ("page".equals(codeString)) 308 return new Enumeration<GuidePageKind>(this, GuidePageKind.PAGE); 309 if ("example".equals(codeString)) 310 return new Enumeration<GuidePageKind>(this, GuidePageKind.EXAMPLE); 311 if ("list".equals(codeString)) 312 return new Enumeration<GuidePageKind>(this, GuidePageKind.LIST); 313 if ("include".equals(codeString)) 314 return new Enumeration<GuidePageKind>(this, GuidePageKind.INCLUDE); 315 if ("directory".equals(codeString)) 316 return new Enumeration<GuidePageKind>(this, GuidePageKind.DIRECTORY); 317 if ("dictionary".equals(codeString)) 318 return new Enumeration<GuidePageKind>(this, GuidePageKind.DICTIONARY); 319 if ("toc".equals(codeString)) 320 return new Enumeration<GuidePageKind>(this, GuidePageKind.TOC); 321 if ("resource".equals(codeString)) 322 return new Enumeration<GuidePageKind>(this, GuidePageKind.RESOURCE); 323 throw new FHIRException("Unknown GuidePageKind code '"+codeString+"'"); 324 } 325 public String toCode(GuidePageKind code) { 326 if (code == GuidePageKind.NULL) 327 return null; 328 if (code == GuidePageKind.PAGE) 329 return "page"; 330 if (code == GuidePageKind.EXAMPLE) 331 return "example"; 332 if (code == GuidePageKind.LIST) 333 return "list"; 334 if (code == GuidePageKind.INCLUDE) 335 return "include"; 336 if (code == GuidePageKind.DIRECTORY) 337 return "directory"; 338 if (code == GuidePageKind.DICTIONARY) 339 return "dictionary"; 340 if (code == GuidePageKind.TOC) 341 return "toc"; 342 if (code == GuidePageKind.RESOURCE) 343 return "resource"; 344 return "?"; 345 } 346 public String toSystem(GuidePageKind code) { 347 return code.getSystem(); 348 } 349 } 350 351 @Block() 352 public static class ImplementationGuideDependencyComponent extends BackboneElement implements IBaseBackboneElement { 353 /** 354 * How the dependency is represented when the guide is published. 355 */ 356 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 357 @Description(shortDefinition="reference | inclusion", formalDefinition="How the dependency is represented when the guide is published." ) 358 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/guide-dependency-type") 359 protected Enumeration<GuideDependencyType> type; 360 361 /** 362 * Where the dependency is located. 363 */ 364 @Child(name = "uri", type = {UriType.class}, order=2, min=1, max=1, modifier=false, summary=true) 365 @Description(shortDefinition="Where to find dependency", formalDefinition="Where the dependency is located." ) 366 protected UriType uri; 367 368 private static final long serialVersionUID = 162447098L; 369 370 /** 371 * Constructor 372 */ 373 public ImplementationGuideDependencyComponent() { 374 super(); 375 } 376 377 /** 378 * Constructor 379 */ 380 public ImplementationGuideDependencyComponent(Enumeration<GuideDependencyType> type, UriType uri) { 381 super(); 382 this.type = type; 383 this.uri = uri; 384 } 385 386 /** 387 * @return {@link #type} (How the dependency is represented when the guide is published.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 388 */ 389 public Enumeration<GuideDependencyType> getTypeElement() { 390 if (this.type == null) 391 if (Configuration.errorOnAutoCreate()) 392 throw new Error("Attempt to auto-create ImplementationGuideDependencyComponent.type"); 393 else if (Configuration.doAutoCreate()) 394 this.type = new Enumeration<GuideDependencyType>(new GuideDependencyTypeEnumFactory()); // bb 395 return this.type; 396 } 397 398 public boolean hasTypeElement() { 399 return this.type != null && !this.type.isEmpty(); 400 } 401 402 public boolean hasType() { 403 return this.type != null && !this.type.isEmpty(); 404 } 405 406 /** 407 * @param value {@link #type} (How the dependency is represented when the guide is published.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 408 */ 409 public ImplementationGuideDependencyComponent setTypeElement(Enumeration<GuideDependencyType> value) { 410 this.type = value; 411 return this; 412 } 413 414 /** 415 * @return How the dependency is represented when the guide is published. 416 */ 417 public GuideDependencyType getType() { 418 return this.type == null ? null : this.type.getValue(); 419 } 420 421 /** 422 * @param value How the dependency is represented when the guide is published. 423 */ 424 public ImplementationGuideDependencyComponent setType(GuideDependencyType value) { 425 if (this.type == null) 426 this.type = new Enumeration<GuideDependencyType>(new GuideDependencyTypeEnumFactory()); 427 this.type.setValue(value); 428 return this; 429 } 430 431 /** 432 * @return {@link #uri} (Where the dependency is located.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 433 */ 434 public UriType getUriElement() { 435 if (this.uri == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create ImplementationGuideDependencyComponent.uri"); 438 else if (Configuration.doAutoCreate()) 439 this.uri = new UriType(); // bb 440 return this.uri; 441 } 442 443 public boolean hasUriElement() { 444 return this.uri != null && !this.uri.isEmpty(); 445 } 446 447 public boolean hasUri() { 448 return this.uri != null && !this.uri.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #uri} (Where the dependency is located.). This is the underlying object with id, value and extensions. The accessor "getUri" gives direct access to the value 453 */ 454 public ImplementationGuideDependencyComponent setUriElement(UriType value) { 455 this.uri = value; 456 return this; 457 } 458 459 /** 460 * @return Where the dependency is located. 461 */ 462 public String getUri() { 463 return this.uri == null ? null : this.uri.getValue(); 464 } 465 466 /** 467 * @param value Where the dependency is located. 468 */ 469 public ImplementationGuideDependencyComponent setUri(String value) { 470 if (this.uri == null) 471 this.uri = new UriType(); 472 this.uri.setValue(value); 473 return this; 474 } 475 476 protected void listChildren(List<Property> children) { 477 super.listChildren(children); 478 children.add(new Property("type", "code", "How the dependency is represented when the guide is published.", 0, 1, type)); 479 children.add(new Property("uri", "uri", "Where the dependency is located.", 0, 1, uri)); 480 } 481 482 @Override 483 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 484 switch (_hash) { 485 case 3575610: /*type*/ return new Property("type", "code", "How the dependency is represented when the guide is published.", 0, 1, type); 486 case 116076: /*uri*/ return new Property("uri", "uri", "Where the dependency is located.", 0, 1, uri); 487 default: return super.getNamedProperty(_hash, _name, _checkValid); 488 } 489 490 } 491 492 @Override 493 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 494 switch (hash) { 495 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<GuideDependencyType> 496 case 116076: /*uri*/ return this.uri == null ? new Base[0] : new Base[] {this.uri}; // UriType 497 default: return super.getProperty(hash, name, checkValid); 498 } 499 500 } 501 502 @Override 503 public Base setProperty(int hash, String name, Base value) throws FHIRException { 504 switch (hash) { 505 case 3575610: // type 506 value = new GuideDependencyTypeEnumFactory().fromType(castToCode(value)); 507 this.type = (Enumeration) value; // Enumeration<GuideDependencyType> 508 return value; 509 case 116076: // uri 510 this.uri = castToUri(value); // UriType 511 return value; 512 default: return super.setProperty(hash, name, value); 513 } 514 515 } 516 517 @Override 518 public Base setProperty(String name, Base value) throws FHIRException { 519 if (name.equals("type")) { 520 value = new GuideDependencyTypeEnumFactory().fromType(castToCode(value)); 521 this.type = (Enumeration) value; // Enumeration<GuideDependencyType> 522 } else if (name.equals("uri")) { 523 this.uri = castToUri(value); // UriType 524 } else 525 return super.setProperty(name, value); 526 return value; 527 } 528 529 @Override 530 public Base makeProperty(int hash, String name) throws FHIRException { 531 switch (hash) { 532 case 3575610: return getTypeElement(); 533 case 116076: return getUriElement(); 534 default: return super.makeProperty(hash, name); 535 } 536 537 } 538 539 @Override 540 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 541 switch (hash) { 542 case 3575610: /*type*/ return new String[] {"code"}; 543 case 116076: /*uri*/ return new String[] {"uri"}; 544 default: return super.getTypesForProperty(hash, name); 545 } 546 547 } 548 549 @Override 550 public Base addChild(String name) throws FHIRException { 551 if (name.equals("type")) { 552 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.type"); 553 } 554 else if (name.equals("uri")) { 555 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.uri"); 556 } 557 else 558 return super.addChild(name); 559 } 560 561 public ImplementationGuideDependencyComponent copy() { 562 ImplementationGuideDependencyComponent dst = new ImplementationGuideDependencyComponent(); 563 copyValues(dst); 564 dst.type = type == null ? null : type.copy(); 565 dst.uri = uri == null ? null : uri.copy(); 566 return dst; 567 } 568 569 @Override 570 public boolean equalsDeep(Base other_) { 571 if (!super.equalsDeep(other_)) 572 return false; 573 if (!(other_ instanceof ImplementationGuideDependencyComponent)) 574 return false; 575 ImplementationGuideDependencyComponent o = (ImplementationGuideDependencyComponent) other_; 576 return compareDeep(type, o.type, true) && compareDeep(uri, o.uri, true); 577 } 578 579 @Override 580 public boolean equalsShallow(Base other_) { 581 if (!super.equalsShallow(other_)) 582 return false; 583 if (!(other_ instanceof ImplementationGuideDependencyComponent)) 584 return false; 585 ImplementationGuideDependencyComponent o = (ImplementationGuideDependencyComponent) other_; 586 return compareValues(type, o.type, true) && compareValues(uri, o.uri, true); 587 } 588 589 public boolean isEmpty() { 590 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, uri); 591 } 592 593 public String fhirType() { 594 return "ImplementationGuide.dependency"; 595 596 } 597 598 } 599 600 @Block() 601 public static class ImplementationGuidePackageComponent extends BackboneElement implements IBaseBackboneElement { 602 /** 603 * The name for the group, as used in page.package. 604 */ 605 @Child(name = "name", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=true) 606 @Description(shortDefinition="Name used .page.package", formalDefinition="The name for the group, as used in page.package." ) 607 protected StringType name; 608 609 /** 610 * Human readable text describing the package. 611 */ 612 @Child(name = "description", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 613 @Description(shortDefinition="Human readable text describing the package", formalDefinition="Human readable text describing the package." ) 614 protected StringType description; 615 616 /** 617 * A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource. 618 */ 619 @Child(name = "resource", type = {}, order=3, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 620 @Description(shortDefinition="Resource in the implementation guide", formalDefinition="A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource." ) 621 protected List<ImplementationGuidePackageResourceComponent> resource; 622 623 private static final long serialVersionUID = -701846580L; 624 625 /** 626 * Constructor 627 */ 628 public ImplementationGuidePackageComponent() { 629 super(); 630 } 631 632 /** 633 * Constructor 634 */ 635 public ImplementationGuidePackageComponent(StringType name) { 636 super(); 637 this.name = name; 638 } 639 640 /** 641 * @return {@link #name} (The name for the group, as used in page.package.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 642 */ 643 public StringType getNameElement() { 644 if (this.name == null) 645 if (Configuration.errorOnAutoCreate()) 646 throw new Error("Attempt to auto-create ImplementationGuidePackageComponent.name"); 647 else if (Configuration.doAutoCreate()) 648 this.name = new StringType(); // bb 649 return this.name; 650 } 651 652 public boolean hasNameElement() { 653 return this.name != null && !this.name.isEmpty(); 654 } 655 656 public boolean hasName() { 657 return this.name != null && !this.name.isEmpty(); 658 } 659 660 /** 661 * @param value {@link #name} (The name for the group, as used in page.package.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 662 */ 663 public ImplementationGuidePackageComponent setNameElement(StringType value) { 664 this.name = value; 665 return this; 666 } 667 668 /** 669 * @return The name for the group, as used in page.package. 670 */ 671 public String getName() { 672 return this.name == null ? null : this.name.getValue(); 673 } 674 675 /** 676 * @param value The name for the group, as used in page.package. 677 */ 678 public ImplementationGuidePackageComponent setName(String value) { 679 if (this.name == null) 680 this.name = new StringType(); 681 this.name.setValue(value); 682 return this; 683 } 684 685 /** 686 * @return {@link #description} (Human readable text describing the package.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 687 */ 688 public StringType getDescriptionElement() { 689 if (this.description == null) 690 if (Configuration.errorOnAutoCreate()) 691 throw new Error("Attempt to auto-create ImplementationGuidePackageComponent.description"); 692 else if (Configuration.doAutoCreate()) 693 this.description = new StringType(); // bb 694 return this.description; 695 } 696 697 public boolean hasDescriptionElement() { 698 return this.description != null && !this.description.isEmpty(); 699 } 700 701 public boolean hasDescription() { 702 return this.description != null && !this.description.isEmpty(); 703 } 704 705 /** 706 * @param value {@link #description} (Human readable text describing the package.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 707 */ 708 public ImplementationGuidePackageComponent setDescriptionElement(StringType value) { 709 this.description = value; 710 return this; 711 } 712 713 /** 714 * @return Human readable text describing the package. 715 */ 716 public String getDescription() { 717 return this.description == null ? null : this.description.getValue(); 718 } 719 720 /** 721 * @param value Human readable text describing the package. 722 */ 723 public ImplementationGuidePackageComponent setDescription(String value) { 724 if (Utilities.noString(value)) 725 this.description = null; 726 else { 727 if (this.description == null) 728 this.description = new StringType(); 729 this.description.setValue(value); 730 } 731 return this; 732 } 733 734 /** 735 * @return {@link #resource} (A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.) 736 */ 737 public List<ImplementationGuidePackageResourceComponent> getResource() { 738 if (this.resource == null) 739 this.resource = new ArrayList<ImplementationGuidePackageResourceComponent>(); 740 return this.resource; 741 } 742 743 /** 744 * @return Returns a reference to <code>this</code> for easy method chaining 745 */ 746 public ImplementationGuidePackageComponent setResource(List<ImplementationGuidePackageResourceComponent> theResource) { 747 this.resource = theResource; 748 return this; 749 } 750 751 public boolean hasResource() { 752 if (this.resource == null) 753 return false; 754 for (ImplementationGuidePackageResourceComponent item : this.resource) 755 if (!item.isEmpty()) 756 return true; 757 return false; 758 } 759 760 public ImplementationGuidePackageResourceComponent addResource() { //3 761 ImplementationGuidePackageResourceComponent t = new ImplementationGuidePackageResourceComponent(); 762 if (this.resource == null) 763 this.resource = new ArrayList<ImplementationGuidePackageResourceComponent>(); 764 this.resource.add(t); 765 return t; 766 } 767 768 public ImplementationGuidePackageComponent addResource(ImplementationGuidePackageResourceComponent t) { //3 769 if (t == null) 770 return this; 771 if (this.resource == null) 772 this.resource = new ArrayList<ImplementationGuidePackageResourceComponent>(); 773 this.resource.add(t); 774 return this; 775 } 776 777 /** 778 * @return The first repetition of repeating field {@link #resource}, creating it if it does not already exist 779 */ 780 public ImplementationGuidePackageResourceComponent getResourceFirstRep() { 781 if (getResource().isEmpty()) { 782 addResource(); 783 } 784 return getResource().get(0); 785 } 786 787 protected void listChildren(List<Property> children) { 788 super.listChildren(children); 789 children.add(new Property("name", "string", "The name for the group, as used in page.package.", 0, 1, name)); 790 children.add(new Property("description", "string", "Human readable text describing the package.", 0, 1, description)); 791 children.add(new Property("resource", "", "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 0, java.lang.Integer.MAX_VALUE, resource)); 792 } 793 794 @Override 795 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 796 switch (_hash) { 797 case 3373707: /*name*/ return new Property("name", "string", "The name for the group, as used in page.package.", 0, 1, name); 798 case -1724546052: /*description*/ return new Property("description", "string", "Human readable text describing the package.", 0, 1, description); 799 case -341064690: /*resource*/ return new Property("resource", "", "A resource that is part of the implementation guide. Conformance resources (value set, structure definition, capability statements etc.) are obvious candidates for inclusion, but any kind of resource can be included as an example resource.", 0, java.lang.Integer.MAX_VALUE, resource); 800 default: return super.getNamedProperty(_hash, _name, _checkValid); 801 } 802 803 } 804 805 @Override 806 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 807 switch (hash) { 808 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 809 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 810 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : this.resource.toArray(new Base[this.resource.size()]); // ImplementationGuidePackageResourceComponent 811 default: return super.getProperty(hash, name, checkValid); 812 } 813 814 } 815 816 @Override 817 public Base setProperty(int hash, String name, Base value) throws FHIRException { 818 switch (hash) { 819 case 3373707: // name 820 this.name = castToString(value); // StringType 821 return value; 822 case -1724546052: // description 823 this.description = castToString(value); // StringType 824 return value; 825 case -341064690: // resource 826 this.getResource().add((ImplementationGuidePackageResourceComponent) value); // ImplementationGuidePackageResourceComponent 827 return value; 828 default: return super.setProperty(hash, name, value); 829 } 830 831 } 832 833 @Override 834 public Base setProperty(String name, Base value) throws FHIRException { 835 if (name.equals("name")) { 836 this.name = castToString(value); // StringType 837 } else if (name.equals("description")) { 838 this.description = castToString(value); // StringType 839 } else if (name.equals("resource")) { 840 this.getResource().add((ImplementationGuidePackageResourceComponent) value); 841 } else 842 return super.setProperty(name, value); 843 return value; 844 } 845 846 @Override 847 public Base makeProperty(int hash, String name) throws FHIRException { 848 switch (hash) { 849 case 3373707: return getNameElement(); 850 case -1724546052: return getDescriptionElement(); 851 case -341064690: return addResource(); 852 default: return super.makeProperty(hash, name); 853 } 854 855 } 856 857 @Override 858 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 859 switch (hash) { 860 case 3373707: /*name*/ return new String[] {"string"}; 861 case -1724546052: /*description*/ return new String[] {"string"}; 862 case -341064690: /*resource*/ return new String[] {}; 863 default: return super.getTypesForProperty(hash, name); 864 } 865 866 } 867 868 @Override 869 public Base addChild(String name) throws FHIRException { 870 if (name.equals("name")) { 871 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 872 } 873 else if (name.equals("description")) { 874 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 875 } 876 else if (name.equals("resource")) { 877 return addResource(); 878 } 879 else 880 return super.addChild(name); 881 } 882 883 public ImplementationGuidePackageComponent copy() { 884 ImplementationGuidePackageComponent dst = new ImplementationGuidePackageComponent(); 885 copyValues(dst); 886 dst.name = name == null ? null : name.copy(); 887 dst.description = description == null ? null : description.copy(); 888 if (resource != null) { 889 dst.resource = new ArrayList<ImplementationGuidePackageResourceComponent>(); 890 for (ImplementationGuidePackageResourceComponent i : resource) 891 dst.resource.add(i.copy()); 892 }; 893 return dst; 894 } 895 896 @Override 897 public boolean equalsDeep(Base other_) { 898 if (!super.equalsDeep(other_)) 899 return false; 900 if (!(other_ instanceof ImplementationGuidePackageComponent)) 901 return false; 902 ImplementationGuidePackageComponent o = (ImplementationGuidePackageComponent) other_; 903 return compareDeep(name, o.name, true) && compareDeep(description, o.description, true) && compareDeep(resource, o.resource, true) 904 ; 905 } 906 907 @Override 908 public boolean equalsShallow(Base other_) { 909 if (!super.equalsShallow(other_)) 910 return false; 911 if (!(other_ instanceof ImplementationGuidePackageComponent)) 912 return false; 913 ImplementationGuidePackageComponent o = (ImplementationGuidePackageComponent) other_; 914 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 915 } 916 917 public boolean isEmpty() { 918 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(name, description, resource 919 ); 920 } 921 922 public String fhirType() { 923 return "ImplementationGuide.package"; 924 925 } 926 927 } 928 929 @Block() 930 public static class ImplementationGuidePackageResourceComponent extends BackboneElement implements IBaseBackboneElement { 931 /** 932 * Whether a resource is included in the guide as part of the rules defined by the guide, or just as an example of a resource that conforms to the rules and/or help implementers understand the intent of the guide. 933 */ 934 @Child(name = "example", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=true) 935 @Description(shortDefinition="If not an example, has its normal meaning", formalDefinition="Whether a resource is included in the guide as part of the rules defined by the guide, or just as an example of a resource that conforms to the rules and/or help implementers understand the intent of the guide." ) 936 protected BooleanType example; 937 938 /** 939 * A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name). 940 */ 941 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=true) 942 @Description(shortDefinition="Human Name for the resource", formalDefinition="A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name)." ) 943 protected StringType name; 944 945 /** 946 * A description of the reason that a resource has been included in the implementation guide. 947 */ 948 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 949 @Description(shortDefinition="Reason why included in guide", formalDefinition="A description of the reason that a resource has been included in the implementation guide." ) 950 protected StringType description; 951 952 /** 953 * A short code that may be used to identify the resource throughout the implementation guide. 954 */ 955 @Child(name = "acronym", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 956 @Description(shortDefinition="Short code to identify the resource", formalDefinition="A short code that may be used to identify the resource throughout the implementation guide." ) 957 protected StringType acronym; 958 959 /** 960 * Where this resource is found. 961 */ 962 @Child(name = "source", type = {UriType.class, Reference.class}, order=5, min=1, max=1, modifier=false, summary=true) 963 @Description(shortDefinition="Location of the resource", formalDefinition="Where this resource is found." ) 964 protected Type source; 965 966 /** 967 * Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions. 968 */ 969 @Child(name = "exampleFor", type = {StructureDefinition.class}, order=6, min=0, max=1, modifier=false, summary=false) 970 @Description(shortDefinition="Resource this is an example of (if applicable)", formalDefinition="Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions." ) 971 protected Reference exampleFor; 972 973 /** 974 * The actual object that is the target of the reference (Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.) 975 */ 976 protected StructureDefinition exampleForTarget; 977 978 private static final long serialVersionUID = 2085404852L; 979 980 /** 981 * Constructor 982 */ 983 public ImplementationGuidePackageResourceComponent() { 984 super(); 985 } 986 987 /** 988 * Constructor 989 */ 990 public ImplementationGuidePackageResourceComponent(BooleanType example, Type source) { 991 super(); 992 this.example = example; 993 this.source = source; 994 } 995 996 /** 997 * @return {@link #example} (Whether a resource is included in the guide as part of the rules defined by the guide, or just as an example of a resource that conforms to the rules and/or help implementers understand the intent of the guide.). This is the underlying object with id, value and extensions. The accessor "getExample" gives direct access to the value 998 */ 999 public BooleanType getExampleElement() { 1000 if (this.example == null) 1001 if (Configuration.errorOnAutoCreate()) 1002 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.example"); 1003 else if (Configuration.doAutoCreate()) 1004 this.example = new BooleanType(); // bb 1005 return this.example; 1006 } 1007 1008 public boolean hasExampleElement() { 1009 return this.example != null && !this.example.isEmpty(); 1010 } 1011 1012 public boolean hasExample() { 1013 return this.example != null && !this.example.isEmpty(); 1014 } 1015 1016 /** 1017 * @param value {@link #example} (Whether a resource is included in the guide as part of the rules defined by the guide, or just as an example of a resource that conforms to the rules and/or help implementers understand the intent of the guide.). This is the underlying object with id, value and extensions. The accessor "getExample" gives direct access to the value 1018 */ 1019 public ImplementationGuidePackageResourceComponent setExampleElement(BooleanType value) { 1020 this.example = value; 1021 return this; 1022 } 1023 1024 /** 1025 * @return Whether a resource is included in the guide as part of the rules defined by the guide, or just as an example of a resource that conforms to the rules and/or help implementers understand the intent of the guide. 1026 */ 1027 public boolean getExample() { 1028 return this.example == null || this.example.isEmpty() ? false : this.example.getValue(); 1029 } 1030 1031 /** 1032 * @param value Whether a resource is included in the guide as part of the rules defined by the guide, or just as an example of a resource that conforms to the rules and/or help implementers understand the intent of the guide. 1033 */ 1034 public ImplementationGuidePackageResourceComponent setExample(boolean value) { 1035 if (this.example == null) 1036 this.example = new BooleanType(); 1037 this.example.setValue(value); 1038 return this; 1039 } 1040 1041 /** 1042 * @return {@link #name} (A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1043 */ 1044 public StringType getNameElement() { 1045 if (this.name == null) 1046 if (Configuration.errorOnAutoCreate()) 1047 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.name"); 1048 else if (Configuration.doAutoCreate()) 1049 this.name = new StringType(); // bb 1050 return this.name; 1051 } 1052 1053 public boolean hasNameElement() { 1054 return this.name != null && !this.name.isEmpty(); 1055 } 1056 1057 public boolean hasName() { 1058 return this.name != null && !this.name.isEmpty(); 1059 } 1060 1061 /** 1062 * @param value {@link #name} (A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1063 */ 1064 public ImplementationGuidePackageResourceComponent setNameElement(StringType value) { 1065 this.name = value; 1066 return this; 1067 } 1068 1069 /** 1070 * @return A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name). 1071 */ 1072 public String getName() { 1073 return this.name == null ? null : this.name.getValue(); 1074 } 1075 1076 /** 1077 * @param value A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name). 1078 */ 1079 public ImplementationGuidePackageResourceComponent setName(String value) { 1080 if (Utilities.noString(value)) 1081 this.name = null; 1082 else { 1083 if (this.name == null) 1084 this.name = new StringType(); 1085 this.name.setValue(value); 1086 } 1087 return this; 1088 } 1089 1090 /** 1091 * @return {@link #description} (A description of the reason that a resource has been included in the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1092 */ 1093 public StringType getDescriptionElement() { 1094 if (this.description == null) 1095 if (Configuration.errorOnAutoCreate()) 1096 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.description"); 1097 else if (Configuration.doAutoCreate()) 1098 this.description = new StringType(); // bb 1099 return this.description; 1100 } 1101 1102 public boolean hasDescriptionElement() { 1103 return this.description != null && !this.description.isEmpty(); 1104 } 1105 1106 public boolean hasDescription() { 1107 return this.description != null && !this.description.isEmpty(); 1108 } 1109 1110 /** 1111 * @param value {@link #description} (A description of the reason that a resource has been included in the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1112 */ 1113 public ImplementationGuidePackageResourceComponent setDescriptionElement(StringType value) { 1114 this.description = value; 1115 return this; 1116 } 1117 1118 /** 1119 * @return A description of the reason that a resource has been included in the implementation guide. 1120 */ 1121 public String getDescription() { 1122 return this.description == null ? null : this.description.getValue(); 1123 } 1124 1125 /** 1126 * @param value A description of the reason that a resource has been included in the implementation guide. 1127 */ 1128 public ImplementationGuidePackageResourceComponent setDescription(String value) { 1129 if (Utilities.noString(value)) 1130 this.description = null; 1131 else { 1132 if (this.description == null) 1133 this.description = new StringType(); 1134 this.description.setValue(value); 1135 } 1136 return this; 1137 } 1138 1139 /** 1140 * @return {@link #acronym} (A short code that may be used to identify the resource throughout the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getAcronym" gives direct access to the value 1141 */ 1142 public StringType getAcronymElement() { 1143 if (this.acronym == null) 1144 if (Configuration.errorOnAutoCreate()) 1145 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.acronym"); 1146 else if (Configuration.doAutoCreate()) 1147 this.acronym = new StringType(); // bb 1148 return this.acronym; 1149 } 1150 1151 public boolean hasAcronymElement() { 1152 return this.acronym != null && !this.acronym.isEmpty(); 1153 } 1154 1155 public boolean hasAcronym() { 1156 return this.acronym != null && !this.acronym.isEmpty(); 1157 } 1158 1159 /** 1160 * @param value {@link #acronym} (A short code that may be used to identify the resource throughout the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getAcronym" gives direct access to the value 1161 */ 1162 public ImplementationGuidePackageResourceComponent setAcronymElement(StringType value) { 1163 this.acronym = value; 1164 return this; 1165 } 1166 1167 /** 1168 * @return A short code that may be used to identify the resource throughout the implementation guide. 1169 */ 1170 public String getAcronym() { 1171 return this.acronym == null ? null : this.acronym.getValue(); 1172 } 1173 1174 /** 1175 * @param value A short code that may be used to identify the resource throughout the implementation guide. 1176 */ 1177 public ImplementationGuidePackageResourceComponent setAcronym(String value) { 1178 if (Utilities.noString(value)) 1179 this.acronym = null; 1180 else { 1181 if (this.acronym == null) 1182 this.acronym = new StringType(); 1183 this.acronym.setValue(value); 1184 } 1185 return this; 1186 } 1187 1188 /** 1189 * @return {@link #source} (Where this resource is found.) 1190 */ 1191 public Type getSource() { 1192 return this.source; 1193 } 1194 1195 /** 1196 * @return {@link #source} (Where this resource is found.) 1197 */ 1198 public UriType getSourceUriType() throws FHIRException { 1199 if (this.source == null) 1200 return null; 1201 if (!(this.source instanceof UriType)) 1202 throw new FHIRException("Type mismatch: the type UriType was expected, but "+this.source.getClass().getName()+" was encountered"); 1203 return (UriType) this.source; 1204 } 1205 1206 public boolean hasSourceUriType() { 1207 return this != null && this.source instanceof UriType; 1208 } 1209 1210 /** 1211 * @return {@link #source} (Where this resource is found.) 1212 */ 1213 public Reference getSourceReference() throws FHIRException { 1214 if (this.source == null) 1215 return null; 1216 if (!(this.source instanceof Reference)) 1217 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.source.getClass().getName()+" was encountered"); 1218 return (Reference) this.source; 1219 } 1220 1221 public boolean hasSourceReference() { 1222 return this != null && this.source instanceof Reference; 1223 } 1224 1225 public boolean hasSource() { 1226 return this.source != null && !this.source.isEmpty(); 1227 } 1228 1229 /** 1230 * @param value {@link #source} (Where this resource is found.) 1231 */ 1232 public ImplementationGuidePackageResourceComponent setSource(Type value) throws FHIRFormatError { 1233 if (value != null && !(value instanceof UriType || value instanceof Reference)) 1234 throw new FHIRFormatError("Not the right type for ImplementationGuide.package.resource.source[x]: "+value.fhirType()); 1235 this.source = value; 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #exampleFor} (Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.) 1241 */ 1242 public Reference getExampleFor() { 1243 if (this.exampleFor == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.exampleFor"); 1246 else if (Configuration.doAutoCreate()) 1247 this.exampleFor = new Reference(); // cc 1248 return this.exampleFor; 1249 } 1250 1251 public boolean hasExampleFor() { 1252 return this.exampleFor != null && !this.exampleFor.isEmpty(); 1253 } 1254 1255 /** 1256 * @param value {@link #exampleFor} (Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.) 1257 */ 1258 public ImplementationGuidePackageResourceComponent setExampleFor(Reference value) { 1259 this.exampleFor = value; 1260 return this; 1261 } 1262 1263 /** 1264 * @return {@link #exampleFor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.) 1265 */ 1266 public StructureDefinition getExampleForTarget() { 1267 if (this.exampleForTarget == null) 1268 if (Configuration.errorOnAutoCreate()) 1269 throw new Error("Attempt to auto-create ImplementationGuidePackageResourceComponent.exampleFor"); 1270 else if (Configuration.doAutoCreate()) 1271 this.exampleForTarget = new StructureDefinition(); // aa 1272 return this.exampleForTarget; 1273 } 1274 1275 /** 1276 * @param value {@link #exampleFor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.) 1277 */ 1278 public ImplementationGuidePackageResourceComponent setExampleForTarget(StructureDefinition value) { 1279 this.exampleForTarget = value; 1280 return this; 1281 } 1282 1283 protected void listChildren(List<Property> children) { 1284 super.listChildren(children); 1285 children.add(new Property("example", "boolean", "Whether a resource is included in the guide as part of the rules defined by the guide, or just as an example of a resource that conforms to the rules and/or help implementers understand the intent of the guide.", 0, 1, example)); 1286 children.add(new Property("name", "string", "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).", 0, 1, name)); 1287 children.add(new Property("description", "string", "A description of the reason that a resource has been included in the implementation guide.", 0, 1, description)); 1288 children.add(new Property("acronym", "string", "A short code that may be used to identify the resource throughout the implementation guide.", 0, 1, acronym)); 1289 children.add(new Property("source[x]", "uri|Reference(Any)", "Where this resource is found.", 0, 1, source)); 1290 children.add(new Property("exampleFor", "Reference(StructureDefinition)", "Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.", 0, 1, exampleFor)); 1291 } 1292 1293 @Override 1294 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1295 switch (_hash) { 1296 case -1322970774: /*example*/ return new Property("example", "boolean", "Whether a resource is included in the guide as part of the rules defined by the guide, or just as an example of a resource that conforms to the rules and/or help implementers understand the intent of the guide.", 0, 1, example); 1297 case 3373707: /*name*/ return new Property("name", "string", "A human assigned name for the resource. All resources SHOULD have a name, but the name may be extracted from the resource (e.g. ValueSet.name).", 0, 1, name); 1298 case -1724546052: /*description*/ return new Property("description", "string", "A description of the reason that a resource has been included in the implementation guide.", 0, 1, description); 1299 case -1163472445: /*acronym*/ return new Property("acronym", "string", "A short code that may be used to identify the resource throughout the implementation guide.", 0, 1, acronym); 1300 case -1698413947: /*source[x]*/ return new Property("source[x]", "uri|Reference(Any)", "Where this resource is found.", 0, 1, source); 1301 case -896505829: /*source*/ return new Property("source[x]", "uri|Reference(Any)", "Where this resource is found.", 0, 1, source); 1302 case -1698419887: /*sourceUri*/ return new Property("source[x]", "uri|Reference(Any)", "Where this resource is found.", 0, 1, source); 1303 case -244259472: /*sourceReference*/ return new Property("source[x]", "uri|Reference(Any)", "Where this resource is found.", 0, 1, source); 1304 case -2002349313: /*exampleFor*/ return new Property("exampleFor", "Reference(StructureDefinition)", "Another resource that this resource is an example for. This is mostly used for resources that are included as examples of StructureDefinitions.", 0, 1, exampleFor); 1305 default: return super.getNamedProperty(_hash, _name, _checkValid); 1306 } 1307 1308 } 1309 1310 @Override 1311 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1312 switch (hash) { 1313 case -1322970774: /*example*/ return this.example == null ? new Base[0] : new Base[] {this.example}; // BooleanType 1314 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1315 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1316 case -1163472445: /*acronym*/ return this.acronym == null ? new Base[0] : new Base[] {this.acronym}; // StringType 1317 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Type 1318 case -2002349313: /*exampleFor*/ return this.exampleFor == null ? new Base[0] : new Base[] {this.exampleFor}; // Reference 1319 default: return super.getProperty(hash, name, checkValid); 1320 } 1321 1322 } 1323 1324 @Override 1325 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1326 switch (hash) { 1327 case -1322970774: // example 1328 this.example = castToBoolean(value); // BooleanType 1329 return value; 1330 case 3373707: // name 1331 this.name = castToString(value); // StringType 1332 return value; 1333 case -1724546052: // description 1334 this.description = castToString(value); // StringType 1335 return value; 1336 case -1163472445: // acronym 1337 this.acronym = castToString(value); // StringType 1338 return value; 1339 case -896505829: // source 1340 this.source = castToType(value); // Type 1341 return value; 1342 case -2002349313: // exampleFor 1343 this.exampleFor = castToReference(value); // Reference 1344 return value; 1345 default: return super.setProperty(hash, name, value); 1346 } 1347 1348 } 1349 1350 @Override 1351 public Base setProperty(String name, Base value) throws FHIRException { 1352 if (name.equals("example")) { 1353 this.example = castToBoolean(value); // BooleanType 1354 } else if (name.equals("name")) { 1355 this.name = castToString(value); // StringType 1356 } else if (name.equals("description")) { 1357 this.description = castToString(value); // StringType 1358 } else if (name.equals("acronym")) { 1359 this.acronym = castToString(value); // StringType 1360 } else if (name.equals("source[x]")) { 1361 this.source = castToType(value); // Type 1362 } else if (name.equals("exampleFor")) { 1363 this.exampleFor = castToReference(value); // Reference 1364 } else 1365 return super.setProperty(name, value); 1366 return value; 1367 } 1368 1369 @Override 1370 public Base makeProperty(int hash, String name) throws FHIRException { 1371 switch (hash) { 1372 case -1322970774: return getExampleElement(); 1373 case 3373707: return getNameElement(); 1374 case -1724546052: return getDescriptionElement(); 1375 case -1163472445: return getAcronymElement(); 1376 case -1698413947: return getSource(); 1377 case -896505829: return getSource(); 1378 case -2002349313: return getExampleFor(); 1379 default: return super.makeProperty(hash, name); 1380 } 1381 1382 } 1383 1384 @Override 1385 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1386 switch (hash) { 1387 case -1322970774: /*example*/ return new String[] {"boolean"}; 1388 case 3373707: /*name*/ return new String[] {"string"}; 1389 case -1724546052: /*description*/ return new String[] {"string"}; 1390 case -1163472445: /*acronym*/ return new String[] {"string"}; 1391 case -896505829: /*source*/ return new String[] {"uri", "Reference"}; 1392 case -2002349313: /*exampleFor*/ return new String[] {"Reference"}; 1393 default: return super.getTypesForProperty(hash, name); 1394 } 1395 1396 } 1397 1398 @Override 1399 public Base addChild(String name) throws FHIRException { 1400 if (name.equals("example")) { 1401 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.example"); 1402 } 1403 else if (name.equals("name")) { 1404 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 1405 } 1406 else if (name.equals("description")) { 1407 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 1408 } 1409 else if (name.equals("acronym")) { 1410 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.acronym"); 1411 } 1412 else if (name.equals("sourceUri")) { 1413 this.source = new UriType(); 1414 return this.source; 1415 } 1416 else if (name.equals("sourceReference")) { 1417 this.source = new Reference(); 1418 return this.source; 1419 } 1420 else if (name.equals("exampleFor")) { 1421 this.exampleFor = new Reference(); 1422 return this.exampleFor; 1423 } 1424 else 1425 return super.addChild(name); 1426 } 1427 1428 public ImplementationGuidePackageResourceComponent copy() { 1429 ImplementationGuidePackageResourceComponent dst = new ImplementationGuidePackageResourceComponent(); 1430 copyValues(dst); 1431 dst.example = example == null ? null : example.copy(); 1432 dst.name = name == null ? null : name.copy(); 1433 dst.description = description == null ? null : description.copy(); 1434 dst.acronym = acronym == null ? null : acronym.copy(); 1435 dst.source = source == null ? null : source.copy(); 1436 dst.exampleFor = exampleFor == null ? null : exampleFor.copy(); 1437 return dst; 1438 } 1439 1440 @Override 1441 public boolean equalsDeep(Base other_) { 1442 if (!super.equalsDeep(other_)) 1443 return false; 1444 if (!(other_ instanceof ImplementationGuidePackageResourceComponent)) 1445 return false; 1446 ImplementationGuidePackageResourceComponent o = (ImplementationGuidePackageResourceComponent) other_; 1447 return compareDeep(example, o.example, true) && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 1448 && compareDeep(acronym, o.acronym, true) && compareDeep(source, o.source, true) && compareDeep(exampleFor, o.exampleFor, true) 1449 ; 1450 } 1451 1452 @Override 1453 public boolean equalsShallow(Base other_) { 1454 if (!super.equalsShallow(other_)) 1455 return false; 1456 if (!(other_ instanceof ImplementationGuidePackageResourceComponent)) 1457 return false; 1458 ImplementationGuidePackageResourceComponent o = (ImplementationGuidePackageResourceComponent) other_; 1459 return compareValues(example, o.example, true) && compareValues(name, o.name, true) && compareValues(description, o.description, true) 1460 && compareValues(acronym, o.acronym, true); 1461 } 1462 1463 public boolean isEmpty() { 1464 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(example, name, description 1465 , acronym, source, exampleFor); 1466 } 1467 1468 public String fhirType() { 1469 return "ImplementationGuide.package.resource"; 1470 1471 } 1472 1473 } 1474 1475 @Block() 1476 public static class ImplementationGuideGlobalComponent extends BackboneElement implements IBaseBackboneElement { 1477 /** 1478 * The type of resource that all instances must conform to. 1479 */ 1480 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1481 @Description(shortDefinition="Type this profiles applies to", formalDefinition="The type of resource that all instances must conform to." ) 1482 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 1483 protected CodeType type; 1484 1485 /** 1486 * A reference to the profile that all instances must conform to. 1487 */ 1488 @Child(name = "profile", type = {StructureDefinition.class}, order=2, min=1, max=1, modifier=false, summary=true) 1489 @Description(shortDefinition="Profile that all resources must conform to", formalDefinition="A reference to the profile that all instances must conform to." ) 1490 protected Reference profile; 1491 1492 /** 1493 * The actual object that is the target of the reference (A reference to the profile that all instances must conform to.) 1494 */ 1495 protected StructureDefinition profileTarget; 1496 1497 private static final long serialVersionUID = 2011731959L; 1498 1499 /** 1500 * Constructor 1501 */ 1502 public ImplementationGuideGlobalComponent() { 1503 super(); 1504 } 1505 1506 /** 1507 * Constructor 1508 */ 1509 public ImplementationGuideGlobalComponent(CodeType type, Reference profile) { 1510 super(); 1511 this.type = type; 1512 this.profile = profile; 1513 } 1514 1515 /** 1516 * @return {@link #type} (The type of resource that all instances must conform to.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1517 */ 1518 public CodeType getTypeElement() { 1519 if (this.type == null) 1520 if (Configuration.errorOnAutoCreate()) 1521 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.type"); 1522 else if (Configuration.doAutoCreate()) 1523 this.type = new CodeType(); // bb 1524 return this.type; 1525 } 1526 1527 public boolean hasTypeElement() { 1528 return this.type != null && !this.type.isEmpty(); 1529 } 1530 1531 public boolean hasType() { 1532 return this.type != null && !this.type.isEmpty(); 1533 } 1534 1535 /** 1536 * @param value {@link #type} (The type of resource that all instances must conform to.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 1537 */ 1538 public ImplementationGuideGlobalComponent setTypeElement(CodeType value) { 1539 this.type = value; 1540 return this; 1541 } 1542 1543 /** 1544 * @return The type of resource that all instances must conform to. 1545 */ 1546 public String getType() { 1547 return this.type == null ? null : this.type.getValue(); 1548 } 1549 1550 /** 1551 * @param value The type of resource that all instances must conform to. 1552 */ 1553 public ImplementationGuideGlobalComponent setType(String value) { 1554 if (this.type == null) 1555 this.type = new CodeType(); 1556 this.type.setValue(value); 1557 return this; 1558 } 1559 1560 /** 1561 * @return {@link #profile} (A reference to the profile that all instances must conform to.) 1562 */ 1563 public Reference getProfile() { 1564 if (this.profile == null) 1565 if (Configuration.errorOnAutoCreate()) 1566 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.profile"); 1567 else if (Configuration.doAutoCreate()) 1568 this.profile = new Reference(); // cc 1569 return this.profile; 1570 } 1571 1572 public boolean hasProfile() { 1573 return this.profile != null && !this.profile.isEmpty(); 1574 } 1575 1576 /** 1577 * @param value {@link #profile} (A reference to the profile that all instances must conform to.) 1578 */ 1579 public ImplementationGuideGlobalComponent setProfile(Reference value) { 1580 this.profile = value; 1581 return this; 1582 } 1583 1584 /** 1585 * @return {@link #profile} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the profile that all instances must conform to.) 1586 */ 1587 public StructureDefinition getProfileTarget() { 1588 if (this.profileTarget == null) 1589 if (Configuration.errorOnAutoCreate()) 1590 throw new Error("Attempt to auto-create ImplementationGuideGlobalComponent.profile"); 1591 else if (Configuration.doAutoCreate()) 1592 this.profileTarget = new StructureDefinition(); // aa 1593 return this.profileTarget; 1594 } 1595 1596 /** 1597 * @param value {@link #profile} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the profile that all instances must conform to.) 1598 */ 1599 public ImplementationGuideGlobalComponent setProfileTarget(StructureDefinition value) { 1600 this.profileTarget = value; 1601 return this; 1602 } 1603 1604 protected void listChildren(List<Property> children) { 1605 super.listChildren(children); 1606 children.add(new Property("type", "code", "The type of resource that all instances must conform to.", 0, 1, type)); 1607 children.add(new Property("profile", "Reference(StructureDefinition)", "A reference to the profile that all instances must conform to.", 0, 1, profile)); 1608 } 1609 1610 @Override 1611 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1612 switch (_hash) { 1613 case 3575610: /*type*/ return new Property("type", "code", "The type of resource that all instances must conform to.", 0, 1, type); 1614 case -309425751: /*profile*/ return new Property("profile", "Reference(StructureDefinition)", "A reference to the profile that all instances must conform to.", 0, 1, profile); 1615 default: return super.getNamedProperty(_hash, _name, _checkValid); 1616 } 1617 1618 } 1619 1620 @Override 1621 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1622 switch (hash) { 1623 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeType 1624 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Reference 1625 default: return super.getProperty(hash, name, checkValid); 1626 } 1627 1628 } 1629 1630 @Override 1631 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1632 switch (hash) { 1633 case 3575610: // type 1634 this.type = castToCode(value); // CodeType 1635 return value; 1636 case -309425751: // profile 1637 this.profile = castToReference(value); // Reference 1638 return value; 1639 default: return super.setProperty(hash, name, value); 1640 } 1641 1642 } 1643 1644 @Override 1645 public Base setProperty(String name, Base value) throws FHIRException { 1646 if (name.equals("type")) { 1647 this.type = castToCode(value); // CodeType 1648 } else if (name.equals("profile")) { 1649 this.profile = castToReference(value); // Reference 1650 } else 1651 return super.setProperty(name, value); 1652 return value; 1653 } 1654 1655 @Override 1656 public Base makeProperty(int hash, String name) throws FHIRException { 1657 switch (hash) { 1658 case 3575610: return getTypeElement(); 1659 case -309425751: return getProfile(); 1660 default: return super.makeProperty(hash, name); 1661 } 1662 1663 } 1664 1665 @Override 1666 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1667 switch (hash) { 1668 case 3575610: /*type*/ return new String[] {"code"}; 1669 case -309425751: /*profile*/ return new String[] {"Reference"}; 1670 default: return super.getTypesForProperty(hash, name); 1671 } 1672 1673 } 1674 1675 @Override 1676 public Base addChild(String name) throws FHIRException { 1677 if (name.equals("type")) { 1678 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.type"); 1679 } 1680 else if (name.equals("profile")) { 1681 this.profile = new Reference(); 1682 return this.profile; 1683 } 1684 else 1685 return super.addChild(name); 1686 } 1687 1688 public ImplementationGuideGlobalComponent copy() { 1689 ImplementationGuideGlobalComponent dst = new ImplementationGuideGlobalComponent(); 1690 copyValues(dst); 1691 dst.type = type == null ? null : type.copy(); 1692 dst.profile = profile == null ? null : profile.copy(); 1693 return dst; 1694 } 1695 1696 @Override 1697 public boolean equalsDeep(Base other_) { 1698 if (!super.equalsDeep(other_)) 1699 return false; 1700 if (!(other_ instanceof ImplementationGuideGlobalComponent)) 1701 return false; 1702 ImplementationGuideGlobalComponent o = (ImplementationGuideGlobalComponent) other_; 1703 return compareDeep(type, o.type, true) && compareDeep(profile, o.profile, true); 1704 } 1705 1706 @Override 1707 public boolean equalsShallow(Base other_) { 1708 if (!super.equalsShallow(other_)) 1709 return false; 1710 if (!(other_ instanceof ImplementationGuideGlobalComponent)) 1711 return false; 1712 ImplementationGuideGlobalComponent o = (ImplementationGuideGlobalComponent) other_; 1713 return compareValues(type, o.type, true); 1714 } 1715 1716 public boolean isEmpty() { 1717 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, profile); 1718 } 1719 1720 public String fhirType() { 1721 return "ImplementationGuide.global"; 1722 1723 } 1724 1725 } 1726 1727 @Block() 1728 public static class ImplementationGuidePageComponent extends BackboneElement implements IBaseBackboneElement { 1729 /** 1730 * The source address for the page. 1731 */ 1732 @Child(name = "source", type = {UriType.class}, order=1, min=1, max=1, modifier=false, summary=true) 1733 @Description(shortDefinition="Where to find that page", formalDefinition="The source address for the page." ) 1734 protected UriType source; 1735 1736 /** 1737 * A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc. 1738 */ 1739 @Child(name = "title", type = {StringType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1740 @Description(shortDefinition="Short title shown for navigational assistance", formalDefinition="A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc." ) 1741 protected StringType title; 1742 1743 /** 1744 * The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest. 1745 */ 1746 @Child(name = "kind", type = {CodeType.class}, order=3, min=1, max=1, modifier=false, summary=true) 1747 @Description(shortDefinition="page | example | list | include | directory | dictionary | toc | resource", formalDefinition="The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest." ) 1748 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/guide-page-kind") 1749 protected Enumeration<GuidePageKind> kind; 1750 1751 /** 1752 * For constructed pages, what kind of resources to include in the list. 1753 */ 1754 @Child(name = "type", type = {CodeType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1755 @Description(shortDefinition="Kind of resource to include in the list", formalDefinition="For constructed pages, what kind of resources to include in the list." ) 1756 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 1757 protected List<CodeType> type; 1758 1759 /** 1760 * For constructed pages, a list of packages to include in the page (or else empty for everything). 1761 */ 1762 @Child(name = "package", type = {StringType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1763 @Description(shortDefinition="Name of package to include", formalDefinition="For constructed pages, a list of packages to include in the page (or else empty for everything)." ) 1764 protected List<StringType> package_; 1765 1766 /** 1767 * The format of the page. 1768 */ 1769 @Child(name = "format", type = {CodeType.class}, order=6, min=0, max=1, modifier=false, summary=false) 1770 @Description(shortDefinition="Format of the page (e.g. html, markdown, etc.)", formalDefinition="The format of the page." ) 1771 protected CodeType format; 1772 1773 /** 1774 * Nested Pages/Sections under this page. 1775 */ 1776 @Child(name = "page", type = {ImplementationGuidePageComponent.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1777 @Description(shortDefinition="Nested Pages / Sections", formalDefinition="Nested Pages/Sections under this page." ) 1778 protected List<ImplementationGuidePageComponent> page; 1779 1780 private static final long serialVersionUID = -687763908L; 1781 1782 /** 1783 * Constructor 1784 */ 1785 public ImplementationGuidePageComponent() { 1786 super(); 1787 } 1788 1789 /** 1790 * Constructor 1791 */ 1792 public ImplementationGuidePageComponent(UriType source, StringType title, Enumeration<GuidePageKind> kind) { 1793 super(); 1794 this.source = source; 1795 this.title = title; 1796 this.kind = kind; 1797 } 1798 1799 /** 1800 * @return {@link #source} (The source address for the page.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 1801 */ 1802 public UriType getSourceElement() { 1803 if (this.source == null) 1804 if (Configuration.errorOnAutoCreate()) 1805 throw new Error("Attempt to auto-create ImplementationGuidePageComponent.source"); 1806 else if (Configuration.doAutoCreate()) 1807 this.source = new UriType(); // bb 1808 return this.source; 1809 } 1810 1811 public boolean hasSourceElement() { 1812 return this.source != null && !this.source.isEmpty(); 1813 } 1814 1815 public boolean hasSource() { 1816 return this.source != null && !this.source.isEmpty(); 1817 } 1818 1819 /** 1820 * @param value {@link #source} (The source address for the page.). This is the underlying object with id, value and extensions. The accessor "getSource" gives direct access to the value 1821 */ 1822 public ImplementationGuidePageComponent setSourceElement(UriType value) { 1823 this.source = value; 1824 return this; 1825 } 1826 1827 /** 1828 * @return The source address for the page. 1829 */ 1830 public String getSource() { 1831 return this.source == null ? null : this.source.getValue(); 1832 } 1833 1834 /** 1835 * @param value The source address for the page. 1836 */ 1837 public ImplementationGuidePageComponent setSource(String value) { 1838 if (this.source == null) 1839 this.source = new UriType(); 1840 this.source.setValue(value); 1841 return this; 1842 } 1843 1844 /** 1845 * @return {@link #title} (A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1846 */ 1847 public StringType getTitleElement() { 1848 if (this.title == null) 1849 if (Configuration.errorOnAutoCreate()) 1850 throw new Error("Attempt to auto-create ImplementationGuidePageComponent.title"); 1851 else if (Configuration.doAutoCreate()) 1852 this.title = new StringType(); // bb 1853 return this.title; 1854 } 1855 1856 public boolean hasTitleElement() { 1857 return this.title != null && !this.title.isEmpty(); 1858 } 1859 1860 public boolean hasTitle() { 1861 return this.title != null && !this.title.isEmpty(); 1862 } 1863 1864 /** 1865 * @param value {@link #title} (A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1866 */ 1867 public ImplementationGuidePageComponent setTitleElement(StringType value) { 1868 this.title = value; 1869 return this; 1870 } 1871 1872 /** 1873 * @return A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc. 1874 */ 1875 public String getTitle() { 1876 return this.title == null ? null : this.title.getValue(); 1877 } 1878 1879 /** 1880 * @param value A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc. 1881 */ 1882 public ImplementationGuidePageComponent setTitle(String value) { 1883 if (this.title == null) 1884 this.title = new StringType(); 1885 this.title.setValue(value); 1886 return this; 1887 } 1888 1889 /** 1890 * @return {@link #kind} (The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1891 */ 1892 public Enumeration<GuidePageKind> getKindElement() { 1893 if (this.kind == null) 1894 if (Configuration.errorOnAutoCreate()) 1895 throw new Error("Attempt to auto-create ImplementationGuidePageComponent.kind"); 1896 else if (Configuration.doAutoCreate()) 1897 this.kind = new Enumeration<GuidePageKind>(new GuidePageKindEnumFactory()); // bb 1898 return this.kind; 1899 } 1900 1901 public boolean hasKindElement() { 1902 return this.kind != null && !this.kind.isEmpty(); 1903 } 1904 1905 public boolean hasKind() { 1906 return this.kind != null && !this.kind.isEmpty(); 1907 } 1908 1909 /** 1910 * @param value {@link #kind} (The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest.). This is the underlying object with id, value and extensions. The accessor "getKind" gives direct access to the value 1911 */ 1912 public ImplementationGuidePageComponent setKindElement(Enumeration<GuidePageKind> value) { 1913 this.kind = value; 1914 return this; 1915 } 1916 1917 /** 1918 * @return The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest. 1919 */ 1920 public GuidePageKind getKind() { 1921 return this.kind == null ? null : this.kind.getValue(); 1922 } 1923 1924 /** 1925 * @param value The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest. 1926 */ 1927 public ImplementationGuidePageComponent setKind(GuidePageKind value) { 1928 if (this.kind == null) 1929 this.kind = new Enumeration<GuidePageKind>(new GuidePageKindEnumFactory()); 1930 this.kind.setValue(value); 1931 return this; 1932 } 1933 1934 /** 1935 * @return {@link #type} (For constructed pages, what kind of resources to include in the list.) 1936 */ 1937 public List<CodeType> getType() { 1938 if (this.type == null) 1939 this.type = new ArrayList<CodeType>(); 1940 return this.type; 1941 } 1942 1943 /** 1944 * @return Returns a reference to <code>this</code> for easy method chaining 1945 */ 1946 public ImplementationGuidePageComponent setType(List<CodeType> theType) { 1947 this.type = theType; 1948 return this; 1949 } 1950 1951 public boolean hasType() { 1952 if (this.type == null) 1953 return false; 1954 for (CodeType item : this.type) 1955 if (!item.isEmpty()) 1956 return true; 1957 return false; 1958 } 1959 1960 /** 1961 * @return {@link #type} (For constructed pages, what kind of resources to include in the list.) 1962 */ 1963 public CodeType addTypeElement() {//2 1964 CodeType t = new CodeType(); 1965 if (this.type == null) 1966 this.type = new ArrayList<CodeType>(); 1967 this.type.add(t); 1968 return t; 1969 } 1970 1971 /** 1972 * @param value {@link #type} (For constructed pages, what kind of resources to include in the list.) 1973 */ 1974 public ImplementationGuidePageComponent addType(String value) { //1 1975 CodeType t = new CodeType(); 1976 t.setValue(value); 1977 if (this.type == null) 1978 this.type = new ArrayList<CodeType>(); 1979 this.type.add(t); 1980 return this; 1981 } 1982 1983 /** 1984 * @param value {@link #type} (For constructed pages, what kind of resources to include in the list.) 1985 */ 1986 public boolean hasType(String value) { 1987 if (this.type == null) 1988 return false; 1989 for (CodeType v : this.type) 1990 if (v.getValue().equals(value)) // code 1991 return true; 1992 return false; 1993 } 1994 1995 /** 1996 * @return {@link #package_} (For constructed pages, a list of packages to include in the page (or else empty for everything).) 1997 */ 1998 public List<StringType> getPackage() { 1999 if (this.package_ == null) 2000 this.package_ = new ArrayList<StringType>(); 2001 return this.package_; 2002 } 2003 2004 /** 2005 * @return Returns a reference to <code>this</code> for easy method chaining 2006 */ 2007 public ImplementationGuidePageComponent setPackage(List<StringType> thePackage) { 2008 this.package_ = thePackage; 2009 return this; 2010 } 2011 2012 public boolean hasPackage() { 2013 if (this.package_ == null) 2014 return false; 2015 for (StringType item : this.package_) 2016 if (!item.isEmpty()) 2017 return true; 2018 return false; 2019 } 2020 2021 /** 2022 * @return {@link #package_} (For constructed pages, a list of packages to include in the page (or else empty for everything).) 2023 */ 2024 public StringType addPackageElement() {//2 2025 StringType t = new StringType(); 2026 if (this.package_ == null) 2027 this.package_ = new ArrayList<StringType>(); 2028 this.package_.add(t); 2029 return t; 2030 } 2031 2032 /** 2033 * @param value {@link #package_} (For constructed pages, a list of packages to include in the page (or else empty for everything).) 2034 */ 2035 public ImplementationGuidePageComponent addPackage(String value) { //1 2036 StringType t = new StringType(); 2037 t.setValue(value); 2038 if (this.package_ == null) 2039 this.package_ = new ArrayList<StringType>(); 2040 this.package_.add(t); 2041 return this; 2042 } 2043 2044 /** 2045 * @param value {@link #package_} (For constructed pages, a list of packages to include in the page (or else empty for everything).) 2046 */ 2047 public boolean hasPackage(String value) { 2048 if (this.package_ == null) 2049 return false; 2050 for (StringType v : this.package_) 2051 if (v.getValue().equals(value)) // string 2052 return true; 2053 return false; 2054 } 2055 2056 /** 2057 * @return {@link #format} (The format of the page.). This is the underlying object with id, value and extensions. The accessor "getFormat" gives direct access to the value 2058 */ 2059 public CodeType getFormatElement() { 2060 if (this.format == null) 2061 if (Configuration.errorOnAutoCreate()) 2062 throw new Error("Attempt to auto-create ImplementationGuidePageComponent.format"); 2063 else if (Configuration.doAutoCreate()) 2064 this.format = new CodeType(); // bb 2065 return this.format; 2066 } 2067 2068 public boolean hasFormatElement() { 2069 return this.format != null && !this.format.isEmpty(); 2070 } 2071 2072 public boolean hasFormat() { 2073 return this.format != null && !this.format.isEmpty(); 2074 } 2075 2076 /** 2077 * @param value {@link #format} (The format of the page.). This is the underlying object with id, value and extensions. The accessor "getFormat" gives direct access to the value 2078 */ 2079 public ImplementationGuidePageComponent setFormatElement(CodeType value) { 2080 this.format = value; 2081 return this; 2082 } 2083 2084 /** 2085 * @return The format of the page. 2086 */ 2087 public String getFormat() { 2088 return this.format == null ? null : this.format.getValue(); 2089 } 2090 2091 /** 2092 * @param value The format of the page. 2093 */ 2094 public ImplementationGuidePageComponent setFormat(String value) { 2095 if (Utilities.noString(value)) 2096 this.format = null; 2097 else { 2098 if (this.format == null) 2099 this.format = new CodeType(); 2100 this.format.setValue(value); 2101 } 2102 return this; 2103 } 2104 2105 /** 2106 * @return {@link #page} (Nested Pages/Sections under this page.) 2107 */ 2108 public List<ImplementationGuidePageComponent> getPage() { 2109 if (this.page == null) 2110 this.page = new ArrayList<ImplementationGuidePageComponent>(); 2111 return this.page; 2112 } 2113 2114 /** 2115 * @return Returns a reference to <code>this</code> for easy method chaining 2116 */ 2117 public ImplementationGuidePageComponent setPage(List<ImplementationGuidePageComponent> thePage) { 2118 this.page = thePage; 2119 return this; 2120 } 2121 2122 public boolean hasPage() { 2123 if (this.page == null) 2124 return false; 2125 for (ImplementationGuidePageComponent item : this.page) 2126 if (!item.isEmpty()) 2127 return true; 2128 return false; 2129 } 2130 2131 public ImplementationGuidePageComponent addPage() { //3 2132 ImplementationGuidePageComponent t = new ImplementationGuidePageComponent(); 2133 if (this.page == null) 2134 this.page = new ArrayList<ImplementationGuidePageComponent>(); 2135 this.page.add(t); 2136 return t; 2137 } 2138 2139 public ImplementationGuidePageComponent addPage(ImplementationGuidePageComponent t) { //3 2140 if (t == null) 2141 return this; 2142 if (this.page == null) 2143 this.page = new ArrayList<ImplementationGuidePageComponent>(); 2144 this.page.add(t); 2145 return this; 2146 } 2147 2148 /** 2149 * @return The first repetition of repeating field {@link #page}, creating it if it does not already exist 2150 */ 2151 public ImplementationGuidePageComponent getPageFirstRep() { 2152 if (getPage().isEmpty()) { 2153 addPage(); 2154 } 2155 return getPage().get(0); 2156 } 2157 2158 protected void listChildren(List<Property> children) { 2159 super.listChildren(children); 2160 children.add(new Property("source", "uri", "The source address for the page.", 0, 1, source)); 2161 children.add(new Property("title", "string", "A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.", 0, 1, title)); 2162 children.add(new Property("kind", "code", "The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest.", 0, 1, kind)); 2163 children.add(new Property("type", "code", "For constructed pages, what kind of resources to include in the list.", 0, java.lang.Integer.MAX_VALUE, type)); 2164 children.add(new Property("package", "string", "For constructed pages, a list of packages to include in the page (or else empty for everything).", 0, java.lang.Integer.MAX_VALUE, package_)); 2165 children.add(new Property("format", "code", "The format of the page.", 0, 1, format)); 2166 children.add(new Property("page", "@ImplementationGuide.page", "Nested Pages/Sections under this page.", 0, java.lang.Integer.MAX_VALUE, page)); 2167 } 2168 2169 @Override 2170 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2171 switch (_hash) { 2172 case -896505829: /*source*/ return new Property("source", "uri", "The source address for the page.", 0, 1, source); 2173 case 110371416: /*title*/ return new Property("title", "string", "A short title used to represent this page in navigational structures such as table of contents, bread crumbs, etc.", 0, 1, title); 2174 case 3292052: /*kind*/ return new Property("kind", "code", "The kind of page that this is. Some pages are autogenerated (list, example), and other kinds are of interest so that tools can navigate the user to the page of interest.", 0, 1, kind); 2175 case 3575610: /*type*/ return new Property("type", "code", "For constructed pages, what kind of resources to include in the list.", 0, java.lang.Integer.MAX_VALUE, type); 2176 case -807062458: /*package*/ return new Property("package", "string", "For constructed pages, a list of packages to include in the page (or else empty for everything).", 0, java.lang.Integer.MAX_VALUE, package_); 2177 case -1268779017: /*format*/ return new Property("format", "code", "The format of the page.", 0, 1, format); 2178 case 3433103: /*page*/ return new Property("page", "@ImplementationGuide.page", "Nested Pages/Sections under this page.", 0, java.lang.Integer.MAX_VALUE, page); 2179 default: return super.getNamedProperty(_hash, _name, _checkValid); 2180 } 2181 2182 } 2183 2184 @Override 2185 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2186 switch (hash) { 2187 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // UriType 2188 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2189 case 3292052: /*kind*/ return this.kind == null ? new Base[0] : new Base[] {this.kind}; // Enumeration<GuidePageKind> 2190 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeType 2191 case -807062458: /*package*/ return this.package_ == null ? new Base[0] : this.package_.toArray(new Base[this.package_.size()]); // StringType 2192 case -1268779017: /*format*/ return this.format == null ? new Base[0] : new Base[] {this.format}; // CodeType 2193 case 3433103: /*page*/ return this.page == null ? new Base[0] : this.page.toArray(new Base[this.page.size()]); // ImplementationGuidePageComponent 2194 default: return super.getProperty(hash, name, checkValid); 2195 } 2196 2197 } 2198 2199 @Override 2200 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2201 switch (hash) { 2202 case -896505829: // source 2203 this.source = castToUri(value); // UriType 2204 return value; 2205 case 110371416: // title 2206 this.title = castToString(value); // StringType 2207 return value; 2208 case 3292052: // kind 2209 value = new GuidePageKindEnumFactory().fromType(castToCode(value)); 2210 this.kind = (Enumeration) value; // Enumeration<GuidePageKind> 2211 return value; 2212 case 3575610: // type 2213 this.getType().add(castToCode(value)); // CodeType 2214 return value; 2215 case -807062458: // package 2216 this.getPackage().add(castToString(value)); // StringType 2217 return value; 2218 case -1268779017: // format 2219 this.format = castToCode(value); // CodeType 2220 return value; 2221 case 3433103: // page 2222 this.getPage().add((ImplementationGuidePageComponent) value); // ImplementationGuidePageComponent 2223 return value; 2224 default: return super.setProperty(hash, name, value); 2225 } 2226 2227 } 2228 2229 @Override 2230 public Base setProperty(String name, Base value) throws FHIRException { 2231 if (name.equals("source")) { 2232 this.source = castToUri(value); // UriType 2233 } else if (name.equals("title")) { 2234 this.title = castToString(value); // StringType 2235 } else if (name.equals("kind")) { 2236 value = new GuidePageKindEnumFactory().fromType(castToCode(value)); 2237 this.kind = (Enumeration) value; // Enumeration<GuidePageKind> 2238 } else if (name.equals("type")) { 2239 this.getType().add(castToCode(value)); 2240 } else if (name.equals("package")) { 2241 this.getPackage().add(castToString(value)); 2242 } else if (name.equals("format")) { 2243 this.format = castToCode(value); // CodeType 2244 } else if (name.equals("page")) { 2245 this.getPage().add((ImplementationGuidePageComponent) value); 2246 } else 2247 return super.setProperty(name, value); 2248 return value; 2249 } 2250 2251 @Override 2252 public Base makeProperty(int hash, String name) throws FHIRException { 2253 switch (hash) { 2254 case -896505829: return getSourceElement(); 2255 case 110371416: return getTitleElement(); 2256 case 3292052: return getKindElement(); 2257 case 3575610: return addTypeElement(); 2258 case -807062458: return addPackageElement(); 2259 case -1268779017: return getFormatElement(); 2260 case 3433103: return addPage(); 2261 default: return super.makeProperty(hash, name); 2262 } 2263 2264 } 2265 2266 @Override 2267 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2268 switch (hash) { 2269 case -896505829: /*source*/ return new String[] {"uri"}; 2270 case 110371416: /*title*/ return new String[] {"string"}; 2271 case 3292052: /*kind*/ return new String[] {"code"}; 2272 case 3575610: /*type*/ return new String[] {"code"}; 2273 case -807062458: /*package*/ return new String[] {"string"}; 2274 case -1268779017: /*format*/ return new String[] {"code"}; 2275 case 3433103: /*page*/ return new String[] {"@ImplementationGuide.page"}; 2276 default: return super.getTypesForProperty(hash, name); 2277 } 2278 2279 } 2280 2281 @Override 2282 public Base addChild(String name) throws FHIRException { 2283 if (name.equals("source")) { 2284 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.source"); 2285 } 2286 else if (name.equals("title")) { 2287 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.title"); 2288 } 2289 else if (name.equals("kind")) { 2290 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.kind"); 2291 } 2292 else if (name.equals("type")) { 2293 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.type"); 2294 } 2295 else if (name.equals("package")) { 2296 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.package"); 2297 } 2298 else if (name.equals("format")) { 2299 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.format"); 2300 } 2301 else if (name.equals("page")) { 2302 return addPage(); 2303 } 2304 else 2305 return super.addChild(name); 2306 } 2307 2308 public ImplementationGuidePageComponent copy() { 2309 ImplementationGuidePageComponent dst = new ImplementationGuidePageComponent(); 2310 copyValues(dst); 2311 dst.source = source == null ? null : source.copy(); 2312 dst.title = title == null ? null : title.copy(); 2313 dst.kind = kind == null ? null : kind.copy(); 2314 if (type != null) { 2315 dst.type = new ArrayList<CodeType>(); 2316 for (CodeType i : type) 2317 dst.type.add(i.copy()); 2318 }; 2319 if (package_ != null) { 2320 dst.package_ = new ArrayList<StringType>(); 2321 for (StringType i : package_) 2322 dst.package_.add(i.copy()); 2323 }; 2324 dst.format = format == null ? null : format.copy(); 2325 if (page != null) { 2326 dst.page = new ArrayList<ImplementationGuidePageComponent>(); 2327 for (ImplementationGuidePageComponent i : page) 2328 dst.page.add(i.copy()); 2329 }; 2330 return dst; 2331 } 2332 2333 @Override 2334 public boolean equalsDeep(Base other_) { 2335 if (!super.equalsDeep(other_)) 2336 return false; 2337 if (!(other_ instanceof ImplementationGuidePageComponent)) 2338 return false; 2339 ImplementationGuidePageComponent o = (ImplementationGuidePageComponent) other_; 2340 return compareDeep(source, o.source, true) && compareDeep(title, o.title, true) && compareDeep(kind, o.kind, true) 2341 && compareDeep(type, o.type, true) && compareDeep(package_, o.package_, true) && compareDeep(format, o.format, true) 2342 && compareDeep(page, o.page, true); 2343 } 2344 2345 @Override 2346 public boolean equalsShallow(Base other_) { 2347 if (!super.equalsShallow(other_)) 2348 return false; 2349 if (!(other_ instanceof ImplementationGuidePageComponent)) 2350 return false; 2351 ImplementationGuidePageComponent o = (ImplementationGuidePageComponent) other_; 2352 return compareValues(source, o.source, true) && compareValues(title, o.title, true) && compareValues(kind, o.kind, true) 2353 && compareValues(type, o.type, true) && compareValues(package_, o.package_, true) && compareValues(format, o.format, true) 2354 ; 2355 } 2356 2357 public boolean isEmpty() { 2358 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(source, title, kind, type 2359 , package_, format, page); 2360 } 2361 2362 public String fhirType() { 2363 return "ImplementationGuide.page"; 2364 2365 } 2366 2367 } 2368 2369 /** 2370 * A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide. 2371 */ 2372 @Child(name = "copyright", type = {MarkdownType.class}, order=0, min=0, max=1, modifier=false, summary=false) 2373 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide." ) 2374 protected MarkdownType copyright; 2375 2376 /** 2377 * The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version. 2378 */ 2379 @Child(name = "fhirVersion", type = {IdType.class}, order=1, min=0, max=1, modifier=false, summary=true) 2380 @Description(shortDefinition="FHIR Version this Implementation Guide targets", formalDefinition="The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version." ) 2381 protected IdType fhirVersion; 2382 2383 /** 2384 * Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides. 2385 */ 2386 @Child(name = "dependency", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2387 @Description(shortDefinition="Another Implementation guide this depends on", formalDefinition="Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides." ) 2388 protected List<ImplementationGuideDependencyComponent> dependency; 2389 2390 /** 2391 * A logical group of resources. Logical groups can be used when building pages. 2392 */ 2393 @Child(name = "package", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2394 @Description(shortDefinition="Group of resources as used in .page.package", formalDefinition="A logical group of resources. Logical groups can be used when building pages." ) 2395 protected List<ImplementationGuidePackageComponent> package_; 2396 2397 /** 2398 * A set of profiles that all resources covered by this implementation guide must conform to. 2399 */ 2400 @Child(name = "global", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 2401 @Description(shortDefinition="Profiles that apply globally", formalDefinition="A set of profiles that all resources covered by this implementation guide must conform to." ) 2402 protected List<ImplementationGuideGlobalComponent> global; 2403 2404 /** 2405 * A binary file that is included in the implementation guide when it is published. 2406 */ 2407 @Child(name = "binary", type = {UriType.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2408 @Description(shortDefinition="Image, css, script, etc.", formalDefinition="A binary file that is included in the implementation guide when it is published." ) 2409 protected List<UriType> binary; 2410 2411 /** 2412 * A page / section in the implementation guide. The root page is the implementation guide home page. 2413 */ 2414 @Child(name = "page", type = {}, order=6, min=0, max=1, modifier=false, summary=true) 2415 @Description(shortDefinition="Page/Section in the Guide", formalDefinition="A page / section in the implementation guide. The root page is the implementation guide home page." ) 2416 protected ImplementationGuidePageComponent page; 2417 2418 private static final long serialVersionUID = -1252164384L; 2419 2420 /** 2421 * Constructor 2422 */ 2423 public ImplementationGuide() { 2424 super(); 2425 } 2426 2427 /** 2428 * Constructor 2429 */ 2430 public ImplementationGuide(UriType url, StringType name, Enumeration<PublicationStatus> status) { 2431 super(); 2432 this.url = url; 2433 this.name = name; 2434 this.status = status; 2435 } 2436 2437 /** 2438 * @return {@link #url} (An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this implementation guide is (or will be) published. The URL SHOULD include the major version of the implementation guide. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2439 */ 2440 public UriType getUrlElement() { 2441 if (this.url == null) 2442 if (Configuration.errorOnAutoCreate()) 2443 throw new Error("Attempt to auto-create ImplementationGuide.url"); 2444 else if (Configuration.doAutoCreate()) 2445 this.url = new UriType(); // bb 2446 return this.url; 2447 } 2448 2449 public boolean hasUrlElement() { 2450 return this.url != null && !this.url.isEmpty(); 2451 } 2452 2453 public boolean hasUrl() { 2454 return this.url != null && !this.url.isEmpty(); 2455 } 2456 2457 /** 2458 * @param value {@link #url} (An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this implementation guide is (or will be) published. The URL SHOULD include the major version of the implementation guide. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 2459 */ 2460 public ImplementationGuide setUrlElement(UriType value) { 2461 this.url = value; 2462 return this; 2463 } 2464 2465 /** 2466 * @return An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this implementation guide is (or will be) published. The URL SHOULD include the major version of the implementation guide. For more information see [Technical and Business Versions](resource.html#versions). 2467 */ 2468 public String getUrl() { 2469 return this.url == null ? null : this.url.getValue(); 2470 } 2471 2472 /** 2473 * @param value An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this implementation guide is (or will be) published. The URL SHOULD include the major version of the implementation guide. For more information see [Technical and Business Versions](resource.html#versions). 2474 */ 2475 public ImplementationGuide setUrl(String value) { 2476 if (this.url == null) 2477 this.url = new UriType(); 2478 this.url.setValue(value); 2479 return this; 2480 } 2481 2482 /** 2483 * @return {@link #version} (The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2484 */ 2485 public StringType getVersionElement() { 2486 if (this.version == null) 2487 if (Configuration.errorOnAutoCreate()) 2488 throw new Error("Attempt to auto-create ImplementationGuide.version"); 2489 else if (Configuration.doAutoCreate()) 2490 this.version = new StringType(); // bb 2491 return this.version; 2492 } 2493 2494 public boolean hasVersionElement() { 2495 return this.version != null && !this.version.isEmpty(); 2496 } 2497 2498 public boolean hasVersion() { 2499 return this.version != null && !this.version.isEmpty(); 2500 } 2501 2502 /** 2503 * @param value {@link #version} (The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 2504 */ 2505 public ImplementationGuide setVersionElement(StringType value) { 2506 this.version = value; 2507 return this; 2508 } 2509 2510 /** 2511 * @return The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2512 */ 2513 public String getVersion() { 2514 return this.version == null ? null : this.version.getValue(); 2515 } 2516 2517 /** 2518 * @param value The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 2519 */ 2520 public ImplementationGuide setVersion(String value) { 2521 if (Utilities.noString(value)) 2522 this.version = null; 2523 else { 2524 if (this.version == null) 2525 this.version = new StringType(); 2526 this.version.setValue(value); 2527 } 2528 return this; 2529 } 2530 2531 /** 2532 * @return {@link #name} (A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2533 */ 2534 public StringType getNameElement() { 2535 if (this.name == null) 2536 if (Configuration.errorOnAutoCreate()) 2537 throw new Error("Attempt to auto-create ImplementationGuide.name"); 2538 else if (Configuration.doAutoCreate()) 2539 this.name = new StringType(); // bb 2540 return this.name; 2541 } 2542 2543 public boolean hasNameElement() { 2544 return this.name != null && !this.name.isEmpty(); 2545 } 2546 2547 public boolean hasName() { 2548 return this.name != null && !this.name.isEmpty(); 2549 } 2550 2551 /** 2552 * @param value {@link #name} (A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 2553 */ 2554 public ImplementationGuide setNameElement(StringType value) { 2555 this.name = value; 2556 return this; 2557 } 2558 2559 /** 2560 * @return A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2561 */ 2562 public String getName() { 2563 return this.name == null ? null : this.name.getValue(); 2564 } 2565 2566 /** 2567 * @param value A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation. 2568 */ 2569 public ImplementationGuide setName(String value) { 2570 if (this.name == null) 2571 this.name = new StringType(); 2572 this.name.setValue(value); 2573 return this; 2574 } 2575 2576 /** 2577 * @return {@link #status} (The status of this implementation guide. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2578 */ 2579 public Enumeration<PublicationStatus> getStatusElement() { 2580 if (this.status == null) 2581 if (Configuration.errorOnAutoCreate()) 2582 throw new Error("Attempt to auto-create ImplementationGuide.status"); 2583 else if (Configuration.doAutoCreate()) 2584 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2585 return this.status; 2586 } 2587 2588 public boolean hasStatusElement() { 2589 return this.status != null && !this.status.isEmpty(); 2590 } 2591 2592 public boolean hasStatus() { 2593 return this.status != null && !this.status.isEmpty(); 2594 } 2595 2596 /** 2597 * @param value {@link #status} (The status of this implementation guide. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2598 */ 2599 public ImplementationGuide setStatusElement(Enumeration<PublicationStatus> value) { 2600 this.status = value; 2601 return this; 2602 } 2603 2604 /** 2605 * @return The status of this implementation guide. Enables tracking the life-cycle of the content. 2606 */ 2607 public PublicationStatus getStatus() { 2608 return this.status == null ? null : this.status.getValue(); 2609 } 2610 2611 /** 2612 * @param value The status of this implementation guide. Enables tracking the life-cycle of the content. 2613 */ 2614 public ImplementationGuide setStatus(PublicationStatus value) { 2615 if (this.status == null) 2616 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2617 this.status.setValue(value); 2618 return this; 2619 } 2620 2621 /** 2622 * @return {@link #experimental} (A boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2623 */ 2624 public BooleanType getExperimentalElement() { 2625 if (this.experimental == null) 2626 if (Configuration.errorOnAutoCreate()) 2627 throw new Error("Attempt to auto-create ImplementationGuide.experimental"); 2628 else if (Configuration.doAutoCreate()) 2629 this.experimental = new BooleanType(); // bb 2630 return this.experimental; 2631 } 2632 2633 public boolean hasExperimentalElement() { 2634 return this.experimental != null && !this.experimental.isEmpty(); 2635 } 2636 2637 public boolean hasExperimental() { 2638 return this.experimental != null && !this.experimental.isEmpty(); 2639 } 2640 2641 /** 2642 * @param value {@link #experimental} (A boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2643 */ 2644 public ImplementationGuide setExperimentalElement(BooleanType value) { 2645 this.experimental = value; 2646 return this; 2647 } 2648 2649 /** 2650 * @return A boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2651 */ 2652 public boolean getExperimental() { 2653 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2654 } 2655 2656 /** 2657 * @param value A boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2658 */ 2659 public ImplementationGuide setExperimental(boolean value) { 2660 if (this.experimental == null) 2661 this.experimental = new BooleanType(); 2662 this.experimental.setValue(value); 2663 return this; 2664 } 2665 2666 /** 2667 * @return {@link #date} (The date (and optionally time) when the implementation guide was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2668 */ 2669 public DateTimeType getDateElement() { 2670 if (this.date == null) 2671 if (Configuration.errorOnAutoCreate()) 2672 throw new Error("Attempt to auto-create ImplementationGuide.date"); 2673 else if (Configuration.doAutoCreate()) 2674 this.date = new DateTimeType(); // bb 2675 return this.date; 2676 } 2677 2678 public boolean hasDateElement() { 2679 return this.date != null && !this.date.isEmpty(); 2680 } 2681 2682 public boolean hasDate() { 2683 return this.date != null && !this.date.isEmpty(); 2684 } 2685 2686 /** 2687 * @param value {@link #date} (The date (and optionally time) when the implementation guide was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2688 */ 2689 public ImplementationGuide setDateElement(DateTimeType value) { 2690 this.date = value; 2691 return this; 2692 } 2693 2694 /** 2695 * @return The date (and optionally time) when the implementation guide was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes. 2696 */ 2697 public Date getDate() { 2698 return this.date == null ? null : this.date.getValue(); 2699 } 2700 2701 /** 2702 * @param value The date (and optionally time) when the implementation guide was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes. 2703 */ 2704 public ImplementationGuide setDate(Date value) { 2705 if (value == null) 2706 this.date = null; 2707 else { 2708 if (this.date == null) 2709 this.date = new DateTimeType(); 2710 this.date.setValue(value); 2711 } 2712 return this; 2713 } 2714 2715 /** 2716 * @return {@link #publisher} (The name of the individual or organization that published the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2717 */ 2718 public StringType getPublisherElement() { 2719 if (this.publisher == null) 2720 if (Configuration.errorOnAutoCreate()) 2721 throw new Error("Attempt to auto-create ImplementationGuide.publisher"); 2722 else if (Configuration.doAutoCreate()) 2723 this.publisher = new StringType(); // bb 2724 return this.publisher; 2725 } 2726 2727 public boolean hasPublisherElement() { 2728 return this.publisher != null && !this.publisher.isEmpty(); 2729 } 2730 2731 public boolean hasPublisher() { 2732 return this.publisher != null && !this.publisher.isEmpty(); 2733 } 2734 2735 /** 2736 * @param value {@link #publisher} (The name of the individual or organization that published the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2737 */ 2738 public ImplementationGuide setPublisherElement(StringType value) { 2739 this.publisher = value; 2740 return this; 2741 } 2742 2743 /** 2744 * @return The name of the individual or organization that published the implementation guide. 2745 */ 2746 public String getPublisher() { 2747 return this.publisher == null ? null : this.publisher.getValue(); 2748 } 2749 2750 /** 2751 * @param value The name of the individual or organization that published the implementation guide. 2752 */ 2753 public ImplementationGuide setPublisher(String value) { 2754 if (Utilities.noString(value)) 2755 this.publisher = null; 2756 else { 2757 if (this.publisher == null) 2758 this.publisher = new StringType(); 2759 this.publisher.setValue(value); 2760 } 2761 return this; 2762 } 2763 2764 /** 2765 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2766 */ 2767 public List<ContactDetail> getContact() { 2768 if (this.contact == null) 2769 this.contact = new ArrayList<ContactDetail>(); 2770 return this.contact; 2771 } 2772 2773 /** 2774 * @return Returns a reference to <code>this</code> for easy method chaining 2775 */ 2776 public ImplementationGuide setContact(List<ContactDetail> theContact) { 2777 this.contact = theContact; 2778 return this; 2779 } 2780 2781 public boolean hasContact() { 2782 if (this.contact == null) 2783 return false; 2784 for (ContactDetail item : this.contact) 2785 if (!item.isEmpty()) 2786 return true; 2787 return false; 2788 } 2789 2790 public ContactDetail addContact() { //3 2791 ContactDetail t = new ContactDetail(); 2792 if (this.contact == null) 2793 this.contact = new ArrayList<ContactDetail>(); 2794 this.contact.add(t); 2795 return t; 2796 } 2797 2798 public ImplementationGuide addContact(ContactDetail t) { //3 2799 if (t == null) 2800 return this; 2801 if (this.contact == null) 2802 this.contact = new ArrayList<ContactDetail>(); 2803 this.contact.add(t); 2804 return this; 2805 } 2806 2807 /** 2808 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 2809 */ 2810 public ContactDetail getContactFirstRep() { 2811 if (getContact().isEmpty()) { 2812 addContact(); 2813 } 2814 return getContact().get(0); 2815 } 2816 2817 /** 2818 * @return {@link #description} (A free text natural language description of the implementation guide from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2819 */ 2820 public MarkdownType getDescriptionElement() { 2821 if (this.description == null) 2822 if (Configuration.errorOnAutoCreate()) 2823 throw new Error("Attempt to auto-create ImplementationGuide.description"); 2824 else if (Configuration.doAutoCreate()) 2825 this.description = new MarkdownType(); // bb 2826 return this.description; 2827 } 2828 2829 public boolean hasDescriptionElement() { 2830 return this.description != null && !this.description.isEmpty(); 2831 } 2832 2833 public boolean hasDescription() { 2834 return this.description != null && !this.description.isEmpty(); 2835 } 2836 2837 /** 2838 * @param value {@link #description} (A free text natural language description of the implementation guide from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2839 */ 2840 public ImplementationGuide setDescriptionElement(MarkdownType value) { 2841 this.description = value; 2842 return this; 2843 } 2844 2845 /** 2846 * @return A free text natural language description of the implementation guide from a consumer's perspective. 2847 */ 2848 public String getDescription() { 2849 return this.description == null ? null : this.description.getValue(); 2850 } 2851 2852 /** 2853 * @param value A free text natural language description of the implementation guide from a consumer's perspective. 2854 */ 2855 public ImplementationGuide setDescription(String value) { 2856 if (value == null) 2857 this.description = null; 2858 else { 2859 if (this.description == null) 2860 this.description = new MarkdownType(); 2861 this.description.setValue(value); 2862 } 2863 return this; 2864 } 2865 2866 /** 2867 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate implementation guide instances.) 2868 */ 2869 public List<UsageContext> getUseContext() { 2870 if (this.useContext == null) 2871 this.useContext = new ArrayList<UsageContext>(); 2872 return this.useContext; 2873 } 2874 2875 /** 2876 * @return Returns a reference to <code>this</code> for easy method chaining 2877 */ 2878 public ImplementationGuide setUseContext(List<UsageContext> theUseContext) { 2879 this.useContext = theUseContext; 2880 return this; 2881 } 2882 2883 public boolean hasUseContext() { 2884 if (this.useContext == null) 2885 return false; 2886 for (UsageContext item : this.useContext) 2887 if (!item.isEmpty()) 2888 return true; 2889 return false; 2890 } 2891 2892 public UsageContext addUseContext() { //3 2893 UsageContext t = new UsageContext(); 2894 if (this.useContext == null) 2895 this.useContext = new ArrayList<UsageContext>(); 2896 this.useContext.add(t); 2897 return t; 2898 } 2899 2900 public ImplementationGuide addUseContext(UsageContext t) { //3 2901 if (t == null) 2902 return this; 2903 if (this.useContext == null) 2904 this.useContext = new ArrayList<UsageContext>(); 2905 this.useContext.add(t); 2906 return this; 2907 } 2908 2909 /** 2910 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 2911 */ 2912 public UsageContext getUseContextFirstRep() { 2913 if (getUseContext().isEmpty()) { 2914 addUseContext(); 2915 } 2916 return getUseContext().get(0); 2917 } 2918 2919 /** 2920 * @return {@link #jurisdiction} (A legal or geographic region in which the implementation guide is intended to be used.) 2921 */ 2922 public List<CodeableConcept> getJurisdiction() { 2923 if (this.jurisdiction == null) 2924 this.jurisdiction = new ArrayList<CodeableConcept>(); 2925 return this.jurisdiction; 2926 } 2927 2928 /** 2929 * @return Returns a reference to <code>this</code> for easy method chaining 2930 */ 2931 public ImplementationGuide setJurisdiction(List<CodeableConcept> theJurisdiction) { 2932 this.jurisdiction = theJurisdiction; 2933 return this; 2934 } 2935 2936 public boolean hasJurisdiction() { 2937 if (this.jurisdiction == null) 2938 return false; 2939 for (CodeableConcept item : this.jurisdiction) 2940 if (!item.isEmpty()) 2941 return true; 2942 return false; 2943 } 2944 2945 public CodeableConcept addJurisdiction() { //3 2946 CodeableConcept t = new CodeableConcept(); 2947 if (this.jurisdiction == null) 2948 this.jurisdiction = new ArrayList<CodeableConcept>(); 2949 this.jurisdiction.add(t); 2950 return t; 2951 } 2952 2953 public ImplementationGuide addJurisdiction(CodeableConcept t) { //3 2954 if (t == null) 2955 return this; 2956 if (this.jurisdiction == null) 2957 this.jurisdiction = new ArrayList<CodeableConcept>(); 2958 this.jurisdiction.add(t); 2959 return this; 2960 } 2961 2962 /** 2963 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 2964 */ 2965 public CodeableConcept getJurisdictionFirstRep() { 2966 if (getJurisdiction().isEmpty()) { 2967 addJurisdiction(); 2968 } 2969 return getJurisdiction().get(0); 2970 } 2971 2972 /** 2973 * @return {@link #copyright} (A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2974 */ 2975 public MarkdownType getCopyrightElement() { 2976 if (this.copyright == null) 2977 if (Configuration.errorOnAutoCreate()) 2978 throw new Error("Attempt to auto-create ImplementationGuide.copyright"); 2979 else if (Configuration.doAutoCreate()) 2980 this.copyright = new MarkdownType(); // bb 2981 return this.copyright; 2982 } 2983 2984 public boolean hasCopyrightElement() { 2985 return this.copyright != null && !this.copyright.isEmpty(); 2986 } 2987 2988 public boolean hasCopyright() { 2989 return this.copyright != null && !this.copyright.isEmpty(); 2990 } 2991 2992 /** 2993 * @param value {@link #copyright} (A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2994 */ 2995 public ImplementationGuide setCopyrightElement(MarkdownType value) { 2996 this.copyright = value; 2997 return this; 2998 } 2999 3000 /** 3001 * @return A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide. 3002 */ 3003 public String getCopyright() { 3004 return this.copyright == null ? null : this.copyright.getValue(); 3005 } 3006 3007 /** 3008 * @param value A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide. 3009 */ 3010 public ImplementationGuide setCopyright(String value) { 3011 if (value == null) 3012 this.copyright = null; 3013 else { 3014 if (this.copyright == null) 3015 this.copyright = new MarkdownType(); 3016 this.copyright.setValue(value); 3017 } 3018 return this; 3019 } 3020 3021 /** 3022 * @return {@link #fhirVersion} (The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 3023 */ 3024 public IdType getFhirVersionElement() { 3025 if (this.fhirVersion == null) 3026 if (Configuration.errorOnAutoCreate()) 3027 throw new Error("Attempt to auto-create ImplementationGuide.fhirVersion"); 3028 else if (Configuration.doAutoCreate()) 3029 this.fhirVersion = new IdType(); // bb 3030 return this.fhirVersion; 3031 } 3032 3033 public boolean hasFhirVersionElement() { 3034 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 3035 } 3036 3037 public boolean hasFhirVersion() { 3038 return this.fhirVersion != null && !this.fhirVersion.isEmpty(); 3039 } 3040 3041 /** 3042 * @param value {@link #fhirVersion} (The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version.). This is the underlying object with id, value and extensions. The accessor "getFhirVersion" gives direct access to the value 3043 */ 3044 public ImplementationGuide setFhirVersionElement(IdType value) { 3045 this.fhirVersion = value; 3046 return this; 3047 } 3048 3049 /** 3050 * @return The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version. 3051 */ 3052 public String getFhirVersion() { 3053 return this.fhirVersion == null ? null : this.fhirVersion.getValue(); 3054 } 3055 3056 /** 3057 * @param value The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version. 3058 */ 3059 public ImplementationGuide setFhirVersion(String value) { 3060 if (Utilities.noString(value)) 3061 this.fhirVersion = null; 3062 else { 3063 if (this.fhirVersion == null) 3064 this.fhirVersion = new IdType(); 3065 this.fhirVersion.setValue(value); 3066 } 3067 return this; 3068 } 3069 3070 /** 3071 * @return {@link #dependency} (Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.) 3072 */ 3073 public List<ImplementationGuideDependencyComponent> getDependency() { 3074 if (this.dependency == null) 3075 this.dependency = new ArrayList<ImplementationGuideDependencyComponent>(); 3076 return this.dependency; 3077 } 3078 3079 /** 3080 * @return Returns a reference to <code>this</code> for easy method chaining 3081 */ 3082 public ImplementationGuide setDependency(List<ImplementationGuideDependencyComponent> theDependency) { 3083 this.dependency = theDependency; 3084 return this; 3085 } 3086 3087 public boolean hasDependency() { 3088 if (this.dependency == null) 3089 return false; 3090 for (ImplementationGuideDependencyComponent item : this.dependency) 3091 if (!item.isEmpty()) 3092 return true; 3093 return false; 3094 } 3095 3096 public ImplementationGuideDependencyComponent addDependency() { //3 3097 ImplementationGuideDependencyComponent t = new ImplementationGuideDependencyComponent(); 3098 if (this.dependency == null) 3099 this.dependency = new ArrayList<ImplementationGuideDependencyComponent>(); 3100 this.dependency.add(t); 3101 return t; 3102 } 3103 3104 public ImplementationGuide addDependency(ImplementationGuideDependencyComponent t) { //3 3105 if (t == null) 3106 return this; 3107 if (this.dependency == null) 3108 this.dependency = new ArrayList<ImplementationGuideDependencyComponent>(); 3109 this.dependency.add(t); 3110 return this; 3111 } 3112 3113 /** 3114 * @return The first repetition of repeating field {@link #dependency}, creating it if it does not already exist 3115 */ 3116 public ImplementationGuideDependencyComponent getDependencyFirstRep() { 3117 if (getDependency().isEmpty()) { 3118 addDependency(); 3119 } 3120 return getDependency().get(0); 3121 } 3122 3123 /** 3124 * @return {@link #package_} (A logical group of resources. Logical groups can be used when building pages.) 3125 */ 3126 public List<ImplementationGuidePackageComponent> getPackage() { 3127 if (this.package_ == null) 3128 this.package_ = new ArrayList<ImplementationGuidePackageComponent>(); 3129 return this.package_; 3130 } 3131 3132 /** 3133 * @return Returns a reference to <code>this</code> for easy method chaining 3134 */ 3135 public ImplementationGuide setPackage(List<ImplementationGuidePackageComponent> thePackage) { 3136 this.package_ = thePackage; 3137 return this; 3138 } 3139 3140 public boolean hasPackage() { 3141 if (this.package_ == null) 3142 return false; 3143 for (ImplementationGuidePackageComponent item : this.package_) 3144 if (!item.isEmpty()) 3145 return true; 3146 return false; 3147 } 3148 3149 public ImplementationGuidePackageComponent addPackage() { //3 3150 ImplementationGuidePackageComponent t = new ImplementationGuidePackageComponent(); 3151 if (this.package_ == null) 3152 this.package_ = new ArrayList<ImplementationGuidePackageComponent>(); 3153 this.package_.add(t); 3154 return t; 3155 } 3156 3157 public ImplementationGuide addPackage(ImplementationGuidePackageComponent t) { //3 3158 if (t == null) 3159 return this; 3160 if (this.package_ == null) 3161 this.package_ = new ArrayList<ImplementationGuidePackageComponent>(); 3162 this.package_.add(t); 3163 return this; 3164 } 3165 3166 /** 3167 * @return The first repetition of repeating field {@link #package_}, creating it if it does not already exist 3168 */ 3169 public ImplementationGuidePackageComponent getPackageFirstRep() { 3170 if (getPackage().isEmpty()) { 3171 addPackage(); 3172 } 3173 return getPackage().get(0); 3174 } 3175 3176 /** 3177 * @return {@link #global} (A set of profiles that all resources covered by this implementation guide must conform to.) 3178 */ 3179 public List<ImplementationGuideGlobalComponent> getGlobal() { 3180 if (this.global == null) 3181 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 3182 return this.global; 3183 } 3184 3185 /** 3186 * @return Returns a reference to <code>this</code> for easy method chaining 3187 */ 3188 public ImplementationGuide setGlobal(List<ImplementationGuideGlobalComponent> theGlobal) { 3189 this.global = theGlobal; 3190 return this; 3191 } 3192 3193 public boolean hasGlobal() { 3194 if (this.global == null) 3195 return false; 3196 for (ImplementationGuideGlobalComponent item : this.global) 3197 if (!item.isEmpty()) 3198 return true; 3199 return false; 3200 } 3201 3202 public ImplementationGuideGlobalComponent addGlobal() { //3 3203 ImplementationGuideGlobalComponent t = new ImplementationGuideGlobalComponent(); 3204 if (this.global == null) 3205 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 3206 this.global.add(t); 3207 return t; 3208 } 3209 3210 public ImplementationGuide addGlobal(ImplementationGuideGlobalComponent t) { //3 3211 if (t == null) 3212 return this; 3213 if (this.global == null) 3214 this.global = new ArrayList<ImplementationGuideGlobalComponent>(); 3215 this.global.add(t); 3216 return this; 3217 } 3218 3219 /** 3220 * @return The first repetition of repeating field {@link #global}, creating it if it does not already exist 3221 */ 3222 public ImplementationGuideGlobalComponent getGlobalFirstRep() { 3223 if (getGlobal().isEmpty()) { 3224 addGlobal(); 3225 } 3226 return getGlobal().get(0); 3227 } 3228 3229 /** 3230 * @return {@link #binary} (A binary file that is included in the implementation guide when it is published.) 3231 */ 3232 public List<UriType> getBinary() { 3233 if (this.binary == null) 3234 this.binary = new ArrayList<UriType>(); 3235 return this.binary; 3236 } 3237 3238 /** 3239 * @return Returns a reference to <code>this</code> for easy method chaining 3240 */ 3241 public ImplementationGuide setBinary(List<UriType> theBinary) { 3242 this.binary = theBinary; 3243 return this; 3244 } 3245 3246 public boolean hasBinary() { 3247 if (this.binary == null) 3248 return false; 3249 for (UriType item : this.binary) 3250 if (!item.isEmpty()) 3251 return true; 3252 return false; 3253 } 3254 3255 /** 3256 * @return {@link #binary} (A binary file that is included in the implementation guide when it is published.) 3257 */ 3258 public UriType addBinaryElement() {//2 3259 UriType t = new UriType(); 3260 if (this.binary == null) 3261 this.binary = new ArrayList<UriType>(); 3262 this.binary.add(t); 3263 return t; 3264 } 3265 3266 /** 3267 * @param value {@link #binary} (A binary file that is included in the implementation guide when it is published.) 3268 */ 3269 public ImplementationGuide addBinary(String value) { //1 3270 UriType t = new UriType(); 3271 t.setValue(value); 3272 if (this.binary == null) 3273 this.binary = new ArrayList<UriType>(); 3274 this.binary.add(t); 3275 return this; 3276 } 3277 3278 /** 3279 * @param value {@link #binary} (A binary file that is included in the implementation guide when it is published.) 3280 */ 3281 public boolean hasBinary(String value) { 3282 if (this.binary == null) 3283 return false; 3284 for (UriType v : this.binary) 3285 if (v.getValue().equals(value)) // uri 3286 return true; 3287 return false; 3288 } 3289 3290 /** 3291 * @return {@link #page} (A page / section in the implementation guide. The root page is the implementation guide home page.) 3292 */ 3293 public ImplementationGuidePageComponent getPage() { 3294 if (this.page == null) 3295 if (Configuration.errorOnAutoCreate()) 3296 throw new Error("Attempt to auto-create ImplementationGuide.page"); 3297 else if (Configuration.doAutoCreate()) 3298 this.page = new ImplementationGuidePageComponent(); // cc 3299 return this.page; 3300 } 3301 3302 public boolean hasPage() { 3303 return this.page != null && !this.page.isEmpty(); 3304 } 3305 3306 /** 3307 * @param value {@link #page} (A page / section in the implementation guide. The root page is the implementation guide home page.) 3308 */ 3309 public ImplementationGuide setPage(ImplementationGuidePageComponent value) { 3310 this.page = value; 3311 return this; 3312 } 3313 3314 protected void listChildren(List<Property> children) { 3315 super.listChildren(children); 3316 children.add(new Property("url", "uri", "An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this implementation guide is (or will be) published. The URL SHOULD include the major version of the implementation guide. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 3317 children.add(new Property("version", "string", "The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 3318 children.add(new Property("name", "string", "A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3319 children.add(new Property("status", "code", "The status of this implementation guide. Enables tracking the life-cycle of the content.", 0, 1, status)); 3320 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 3321 children.add(new Property("date", "dateTime", "The date (and optionally time) when the implementation guide was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.", 0, 1, date)); 3322 children.add(new Property("publisher", "string", "The name of the individual or organization that published the implementation guide.", 0, 1, publisher)); 3323 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3324 children.add(new Property("description", "markdown", "A free text natural language description of the implementation guide from a consumer's perspective.", 0, 1, description)); 3325 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate implementation guide instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3326 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the implementation guide is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3327 children.add(new Property("copyright", "markdown", "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.", 0, 1, copyright)); 3328 children.add(new Property("fhirVersion", "id", "The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version.", 0, 1, fhirVersion)); 3329 children.add(new Property("dependency", "", "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.", 0, java.lang.Integer.MAX_VALUE, dependency)); 3330 children.add(new Property("package", "", "A logical group of resources. Logical groups can be used when building pages.", 0, java.lang.Integer.MAX_VALUE, package_)); 3331 children.add(new Property("global", "", "A set of profiles that all resources covered by this implementation guide must conform to.", 0, java.lang.Integer.MAX_VALUE, global)); 3332 children.add(new Property("binary", "uri", "A binary file that is included in the implementation guide when it is published.", 0, java.lang.Integer.MAX_VALUE, binary)); 3333 children.add(new Property("page", "", "A page / section in the implementation guide. The root page is the implementation guide home page.", 0, 1, page)); 3334 } 3335 3336 @Override 3337 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3338 switch (_hash) { 3339 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this implementation guide when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this implementation guide is (or will be) published. The URL SHOULD include the major version of the implementation guide. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 3340 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the implementation guide when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the implementation guide author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 3341 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the implementation guide. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3342 case -892481550: /*status*/ return new Property("status", "code", "The status of this implementation guide. Enables tracking the life-cycle of the content.", 0, 1, status); 3343 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this implementation guide is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 3344 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the implementation guide was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the implementation guide changes.", 0, 1, date); 3345 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the implementation guide.", 0, 1, publisher); 3346 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3347 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the implementation guide from a consumer's perspective.", 0, 1, description); 3348 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate implementation guide instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3349 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the implementation guide is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3350 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the implementation guide and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the implementation guide.", 0, 1, copyright); 3351 case 461006061: /*fhirVersion*/ return new Property("fhirVersion", "id", "The version of the FHIR specification on which this ImplementationGuide is based - this is the formal version of the specification, without the revision number, e.g. [publication].[major].[minor], which is 3.0.2 for this version.", 0, 1, fhirVersion); 3352 case -26291381: /*dependency*/ return new Property("dependency", "", "Another implementation guide that this implementation depends on. Typically, an implementation guide uses value sets, profiles etc.defined in other implementation guides.", 0, java.lang.Integer.MAX_VALUE, dependency); 3353 case -807062458: /*package*/ return new Property("package", "", "A logical group of resources. Logical groups can be used when building pages.", 0, java.lang.Integer.MAX_VALUE, package_); 3354 case -1243020381: /*global*/ return new Property("global", "", "A set of profiles that all resources covered by this implementation guide must conform to.", 0, java.lang.Integer.MAX_VALUE, global); 3355 case -1388966911: /*binary*/ return new Property("binary", "uri", "A binary file that is included in the implementation guide when it is published.", 0, java.lang.Integer.MAX_VALUE, binary); 3356 case 3433103: /*page*/ return new Property("page", "", "A page / section in the implementation guide. The root page is the implementation guide home page.", 0, 1, page); 3357 default: return super.getNamedProperty(_hash, _name, _checkValid); 3358 } 3359 3360 } 3361 3362 @Override 3363 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3364 switch (hash) { 3365 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3366 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3367 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3368 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3369 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3370 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3371 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3372 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3373 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3374 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3375 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3376 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3377 case 461006061: /*fhirVersion*/ return this.fhirVersion == null ? new Base[0] : new Base[] {this.fhirVersion}; // IdType 3378 case -26291381: /*dependency*/ return this.dependency == null ? new Base[0] : this.dependency.toArray(new Base[this.dependency.size()]); // ImplementationGuideDependencyComponent 3379 case -807062458: /*package*/ return this.package_ == null ? new Base[0] : this.package_.toArray(new Base[this.package_.size()]); // ImplementationGuidePackageComponent 3380 case -1243020381: /*global*/ return this.global == null ? new Base[0] : this.global.toArray(new Base[this.global.size()]); // ImplementationGuideGlobalComponent 3381 case -1388966911: /*binary*/ return this.binary == null ? new Base[0] : this.binary.toArray(new Base[this.binary.size()]); // UriType 3382 case 3433103: /*page*/ return this.page == null ? new Base[0] : new Base[] {this.page}; // ImplementationGuidePageComponent 3383 default: return super.getProperty(hash, name, checkValid); 3384 } 3385 3386 } 3387 3388 @Override 3389 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3390 switch (hash) { 3391 case 116079: // url 3392 this.url = castToUri(value); // UriType 3393 return value; 3394 case 351608024: // version 3395 this.version = castToString(value); // StringType 3396 return value; 3397 case 3373707: // name 3398 this.name = castToString(value); // StringType 3399 return value; 3400 case -892481550: // status 3401 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3402 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3403 return value; 3404 case -404562712: // experimental 3405 this.experimental = castToBoolean(value); // BooleanType 3406 return value; 3407 case 3076014: // date 3408 this.date = castToDateTime(value); // DateTimeType 3409 return value; 3410 case 1447404028: // publisher 3411 this.publisher = castToString(value); // StringType 3412 return value; 3413 case 951526432: // contact 3414 this.getContact().add(castToContactDetail(value)); // ContactDetail 3415 return value; 3416 case -1724546052: // description 3417 this.description = castToMarkdown(value); // MarkdownType 3418 return value; 3419 case -669707736: // useContext 3420 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3421 return value; 3422 case -507075711: // jurisdiction 3423 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3424 return value; 3425 case 1522889671: // copyright 3426 this.copyright = castToMarkdown(value); // MarkdownType 3427 return value; 3428 case 461006061: // fhirVersion 3429 this.fhirVersion = castToId(value); // IdType 3430 return value; 3431 case -26291381: // dependency 3432 this.getDependency().add((ImplementationGuideDependencyComponent) value); // ImplementationGuideDependencyComponent 3433 return value; 3434 case -807062458: // package 3435 this.getPackage().add((ImplementationGuidePackageComponent) value); // ImplementationGuidePackageComponent 3436 return value; 3437 case -1243020381: // global 3438 this.getGlobal().add((ImplementationGuideGlobalComponent) value); // ImplementationGuideGlobalComponent 3439 return value; 3440 case -1388966911: // binary 3441 this.getBinary().add(castToUri(value)); // UriType 3442 return value; 3443 case 3433103: // page 3444 this.page = (ImplementationGuidePageComponent) value; // ImplementationGuidePageComponent 3445 return value; 3446 default: return super.setProperty(hash, name, value); 3447 } 3448 3449 } 3450 3451 @Override 3452 public Base setProperty(String name, Base value) throws FHIRException { 3453 if (name.equals("url")) { 3454 this.url = castToUri(value); // UriType 3455 } else if (name.equals("version")) { 3456 this.version = castToString(value); // StringType 3457 } else if (name.equals("name")) { 3458 this.name = castToString(value); // StringType 3459 } else if (name.equals("status")) { 3460 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3461 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3462 } else if (name.equals("experimental")) { 3463 this.experimental = castToBoolean(value); // BooleanType 3464 } else if (name.equals("date")) { 3465 this.date = castToDateTime(value); // DateTimeType 3466 } else if (name.equals("publisher")) { 3467 this.publisher = castToString(value); // StringType 3468 } else if (name.equals("contact")) { 3469 this.getContact().add(castToContactDetail(value)); 3470 } else if (name.equals("description")) { 3471 this.description = castToMarkdown(value); // MarkdownType 3472 } else if (name.equals("useContext")) { 3473 this.getUseContext().add(castToUsageContext(value)); 3474 } else if (name.equals("jurisdiction")) { 3475 this.getJurisdiction().add(castToCodeableConcept(value)); 3476 } else if (name.equals("copyright")) { 3477 this.copyright = castToMarkdown(value); // MarkdownType 3478 } else if (name.equals("fhirVersion")) { 3479 this.fhirVersion = castToId(value); // IdType 3480 } else if (name.equals("dependency")) { 3481 this.getDependency().add((ImplementationGuideDependencyComponent) value); 3482 } else if (name.equals("package")) { 3483 this.getPackage().add((ImplementationGuidePackageComponent) value); 3484 } else if (name.equals("global")) { 3485 this.getGlobal().add((ImplementationGuideGlobalComponent) value); 3486 } else if (name.equals("binary")) { 3487 this.getBinary().add(castToUri(value)); 3488 } else if (name.equals("page")) { 3489 this.page = (ImplementationGuidePageComponent) value; // ImplementationGuidePageComponent 3490 } else 3491 return super.setProperty(name, value); 3492 return value; 3493 } 3494 3495 @Override 3496 public Base makeProperty(int hash, String name) throws FHIRException { 3497 switch (hash) { 3498 case 116079: return getUrlElement(); 3499 case 351608024: return getVersionElement(); 3500 case 3373707: return getNameElement(); 3501 case -892481550: return getStatusElement(); 3502 case -404562712: return getExperimentalElement(); 3503 case 3076014: return getDateElement(); 3504 case 1447404028: return getPublisherElement(); 3505 case 951526432: return addContact(); 3506 case -1724546052: return getDescriptionElement(); 3507 case -669707736: return addUseContext(); 3508 case -507075711: return addJurisdiction(); 3509 case 1522889671: return getCopyrightElement(); 3510 case 461006061: return getFhirVersionElement(); 3511 case -26291381: return addDependency(); 3512 case -807062458: return addPackage(); 3513 case -1243020381: return addGlobal(); 3514 case -1388966911: return addBinaryElement(); 3515 case 3433103: return getPage(); 3516 default: return super.makeProperty(hash, name); 3517 } 3518 3519 } 3520 3521 @Override 3522 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3523 switch (hash) { 3524 case 116079: /*url*/ return new String[] {"uri"}; 3525 case 351608024: /*version*/ return new String[] {"string"}; 3526 case 3373707: /*name*/ return new String[] {"string"}; 3527 case -892481550: /*status*/ return new String[] {"code"}; 3528 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3529 case 3076014: /*date*/ return new String[] {"dateTime"}; 3530 case 1447404028: /*publisher*/ return new String[] {"string"}; 3531 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 3532 case -1724546052: /*description*/ return new String[] {"markdown"}; 3533 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 3534 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 3535 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 3536 case 461006061: /*fhirVersion*/ return new String[] {"id"}; 3537 case -26291381: /*dependency*/ return new String[] {}; 3538 case -807062458: /*package*/ return new String[] {}; 3539 case -1243020381: /*global*/ return new String[] {}; 3540 case -1388966911: /*binary*/ return new String[] {"uri"}; 3541 case 3433103: /*page*/ return new String[] {}; 3542 default: return super.getTypesForProperty(hash, name); 3543 } 3544 3545 } 3546 3547 @Override 3548 public Base addChild(String name) throws FHIRException { 3549 if (name.equals("url")) { 3550 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.url"); 3551 } 3552 else if (name.equals("version")) { 3553 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.version"); 3554 } 3555 else if (name.equals("name")) { 3556 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.name"); 3557 } 3558 else if (name.equals("status")) { 3559 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.status"); 3560 } 3561 else if (name.equals("experimental")) { 3562 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.experimental"); 3563 } 3564 else if (name.equals("date")) { 3565 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.date"); 3566 } 3567 else if (name.equals("publisher")) { 3568 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.publisher"); 3569 } 3570 else if (name.equals("contact")) { 3571 return addContact(); 3572 } 3573 else if (name.equals("description")) { 3574 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.description"); 3575 } 3576 else if (name.equals("useContext")) { 3577 return addUseContext(); 3578 } 3579 else if (name.equals("jurisdiction")) { 3580 return addJurisdiction(); 3581 } 3582 else if (name.equals("copyright")) { 3583 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.copyright"); 3584 } 3585 else if (name.equals("fhirVersion")) { 3586 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.fhirVersion"); 3587 } 3588 else if (name.equals("dependency")) { 3589 return addDependency(); 3590 } 3591 else if (name.equals("package")) { 3592 return addPackage(); 3593 } 3594 else if (name.equals("global")) { 3595 return addGlobal(); 3596 } 3597 else if (name.equals("binary")) { 3598 throw new FHIRException("Cannot call addChild on a singleton property ImplementationGuide.binary"); 3599 } 3600 else if (name.equals("page")) { 3601 this.page = new ImplementationGuidePageComponent(); 3602 return this.page; 3603 } 3604 else 3605 return super.addChild(name); 3606 } 3607 3608 public String fhirType() { 3609 return "ImplementationGuide"; 3610 3611 } 3612 3613 public ImplementationGuide copy() { 3614 ImplementationGuide dst = new ImplementationGuide(); 3615 copyValues(dst); 3616 dst.url = url == null ? null : url.copy(); 3617 dst.version = version == null ? null : version.copy(); 3618 dst.name = name == null ? null : name.copy(); 3619 dst.status = status == null ? null : status.copy(); 3620 dst.experimental = experimental == null ? null : experimental.copy(); 3621 dst.date = date == null ? null : date.copy(); 3622 dst.publisher = publisher == null ? null : publisher.copy(); 3623 if (contact != null) { 3624 dst.contact = new ArrayList<ContactDetail>(); 3625 for (ContactDetail i : contact) 3626 dst.contact.add(i.copy()); 3627 }; 3628 dst.description = description == null ? null : description.copy(); 3629 if (useContext != null) { 3630 dst.useContext = new ArrayList<UsageContext>(); 3631 for (UsageContext i : useContext) 3632 dst.useContext.add(i.copy()); 3633 }; 3634 if (jurisdiction != null) { 3635 dst.jurisdiction = new ArrayList<CodeableConcept>(); 3636 for (CodeableConcept i : jurisdiction) 3637 dst.jurisdiction.add(i.copy()); 3638 }; 3639 dst.copyright = copyright == null ? null : copyright.copy(); 3640 dst.fhirVersion = fhirVersion == null ? null : fhirVersion.copy(); 3641 if (dependency != null) { 3642 dst.dependency = new ArrayList<ImplementationGuideDependencyComponent>(); 3643 for (ImplementationGuideDependencyComponent i : dependency) 3644 dst.dependency.add(i.copy()); 3645 }; 3646 if (package_ != null) { 3647 dst.package_ = new ArrayList<ImplementationGuidePackageComponent>(); 3648 for (ImplementationGuidePackageComponent i : package_) 3649 dst.package_.add(i.copy()); 3650 }; 3651 if (global != null) { 3652 dst.global = new ArrayList<ImplementationGuideGlobalComponent>(); 3653 for (ImplementationGuideGlobalComponent i : global) 3654 dst.global.add(i.copy()); 3655 }; 3656 if (binary != null) { 3657 dst.binary = new ArrayList<UriType>(); 3658 for (UriType i : binary) 3659 dst.binary.add(i.copy()); 3660 }; 3661 dst.page = page == null ? null : page.copy(); 3662 return dst; 3663 } 3664 3665 protected ImplementationGuide typedCopy() { 3666 return copy(); 3667 } 3668 3669 @Override 3670 public boolean equalsDeep(Base other_) { 3671 if (!super.equalsDeep(other_)) 3672 return false; 3673 if (!(other_ instanceof ImplementationGuide)) 3674 return false; 3675 ImplementationGuide o = (ImplementationGuide) other_; 3676 return compareDeep(copyright, o.copyright, true) && compareDeep(fhirVersion, o.fhirVersion, true) 3677 && compareDeep(dependency, o.dependency, true) && compareDeep(package_, o.package_, true) && compareDeep(global, o.global, true) 3678 && compareDeep(binary, o.binary, true) && compareDeep(page, o.page, true); 3679 } 3680 3681 @Override 3682 public boolean equalsShallow(Base other_) { 3683 if (!super.equalsShallow(other_)) 3684 return false; 3685 if (!(other_ instanceof ImplementationGuide)) 3686 return false; 3687 ImplementationGuide o = (ImplementationGuide) other_; 3688 return compareValues(copyright, o.copyright, true) && compareValues(fhirVersion, o.fhirVersion, true) 3689 && compareValues(binary, o.binary, true); 3690 } 3691 3692 public boolean isEmpty() { 3693 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(copyright, fhirVersion, dependency 3694 , package_, global, binary, page); 3695 } 3696 3697 @Override 3698 public ResourceType getResourceType() { 3699 return ResourceType.ImplementationGuide; 3700 } 3701 3702 /** 3703 * Search parameter: <b>date</b> 3704 * <p> 3705 * Description: <b>The implementation guide publication date</b><br> 3706 * Type: <b>date</b><br> 3707 * Path: <b>ImplementationGuide.date</b><br> 3708 * </p> 3709 */ 3710 @SearchParamDefinition(name="date", path="ImplementationGuide.date", description="The implementation guide publication date", type="date" ) 3711 public static final String SP_DATE = "date"; 3712 /** 3713 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3714 * <p> 3715 * Description: <b>The implementation guide publication date</b><br> 3716 * Type: <b>date</b><br> 3717 * Path: <b>ImplementationGuide.date</b><br> 3718 * </p> 3719 */ 3720 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3721 3722 /** 3723 * Search parameter: <b>dependency</b> 3724 * <p> 3725 * Description: <b>Where to find dependency</b><br> 3726 * Type: <b>uri</b><br> 3727 * Path: <b>ImplementationGuide.dependency.uri</b><br> 3728 * </p> 3729 */ 3730 @SearchParamDefinition(name="dependency", path="ImplementationGuide.dependency.uri", description="Where to find dependency", type="uri" ) 3731 public static final String SP_DEPENDENCY = "dependency"; 3732 /** 3733 * <b>Fluent Client</b> search parameter constant for <b>dependency</b> 3734 * <p> 3735 * Description: <b>Where to find dependency</b><br> 3736 * Type: <b>uri</b><br> 3737 * Path: <b>ImplementationGuide.dependency.uri</b><br> 3738 * </p> 3739 */ 3740 public static final ca.uhn.fhir.rest.gclient.UriClientParam DEPENDENCY = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_DEPENDENCY); 3741 3742 /** 3743 * Search parameter: <b>resource</b> 3744 * <p> 3745 * Description: <b>Location of the resource</b><br> 3746 * Type: <b>reference</b><br> 3747 * Path: <b>ImplementationGuide.package.resource.source[x]</b><br> 3748 * </p> 3749 */ 3750 @SearchParamDefinition(name="resource", path="ImplementationGuide.package.resource.source", description="Location of the resource", type="reference" ) 3751 public static final String SP_RESOURCE = "resource"; 3752 /** 3753 * <b>Fluent Client</b> search parameter constant for <b>resource</b> 3754 * <p> 3755 * Description: <b>Location of the resource</b><br> 3756 * Type: <b>reference</b><br> 3757 * Path: <b>ImplementationGuide.package.resource.source[x]</b><br> 3758 * </p> 3759 */ 3760 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESOURCE); 3761 3762/** 3763 * Constant for fluent queries to be used to add include statements. Specifies 3764 * the path value of "<b>ImplementationGuide:resource</b>". 3765 */ 3766 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESOURCE = new ca.uhn.fhir.model.api.Include("ImplementationGuide:resource").toLocked(); 3767 3768 /** 3769 * Search parameter: <b>jurisdiction</b> 3770 * <p> 3771 * Description: <b>Intended jurisdiction for the implementation guide</b><br> 3772 * Type: <b>token</b><br> 3773 * Path: <b>ImplementationGuide.jurisdiction</b><br> 3774 * </p> 3775 */ 3776 @SearchParamDefinition(name="jurisdiction", path="ImplementationGuide.jurisdiction", description="Intended jurisdiction for the implementation guide", type="token" ) 3777 public static final String SP_JURISDICTION = "jurisdiction"; 3778 /** 3779 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 3780 * <p> 3781 * Description: <b>Intended jurisdiction for the implementation guide</b><br> 3782 * Type: <b>token</b><br> 3783 * Path: <b>ImplementationGuide.jurisdiction</b><br> 3784 * </p> 3785 */ 3786 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 3787 3788 /** 3789 * Search parameter: <b>name</b> 3790 * <p> 3791 * Description: <b>Computationally friendly name of the implementation guide</b><br> 3792 * Type: <b>string</b><br> 3793 * Path: <b>ImplementationGuide.name</b><br> 3794 * </p> 3795 */ 3796 @SearchParamDefinition(name="name", path="ImplementationGuide.name", description="Computationally friendly name of the implementation guide", type="string" ) 3797 public static final String SP_NAME = "name"; 3798 /** 3799 * <b>Fluent Client</b> search parameter constant for <b>name</b> 3800 * <p> 3801 * Description: <b>Computationally friendly name of the implementation guide</b><br> 3802 * Type: <b>string</b><br> 3803 * Path: <b>ImplementationGuide.name</b><br> 3804 * </p> 3805 */ 3806 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 3807 3808 /** 3809 * Search parameter: <b>description</b> 3810 * <p> 3811 * Description: <b>The description of the implementation guide</b><br> 3812 * Type: <b>string</b><br> 3813 * Path: <b>ImplementationGuide.description</b><br> 3814 * </p> 3815 */ 3816 @SearchParamDefinition(name="description", path="ImplementationGuide.description", description="The description of the implementation guide", type="string" ) 3817 public static final String SP_DESCRIPTION = "description"; 3818 /** 3819 * <b>Fluent Client</b> search parameter constant for <b>description</b> 3820 * <p> 3821 * Description: <b>The description of the implementation guide</b><br> 3822 * Type: <b>string</b><br> 3823 * Path: <b>ImplementationGuide.description</b><br> 3824 * </p> 3825 */ 3826 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 3827 3828 /** 3829 * Search parameter: <b>publisher</b> 3830 * <p> 3831 * Description: <b>Name of the publisher of the implementation guide</b><br> 3832 * Type: <b>string</b><br> 3833 * Path: <b>ImplementationGuide.publisher</b><br> 3834 * </p> 3835 */ 3836 @SearchParamDefinition(name="publisher", path="ImplementationGuide.publisher", description="Name of the publisher of the implementation guide", type="string" ) 3837 public static final String SP_PUBLISHER = "publisher"; 3838 /** 3839 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 3840 * <p> 3841 * Description: <b>Name of the publisher of the implementation guide</b><br> 3842 * Type: <b>string</b><br> 3843 * Path: <b>ImplementationGuide.publisher</b><br> 3844 * </p> 3845 */ 3846 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 3847 3848 /** 3849 * Search parameter: <b>experimental</b> 3850 * <p> 3851 * Description: <b>For testing purposes, not real usage</b><br> 3852 * Type: <b>token</b><br> 3853 * Path: <b>ImplementationGuide.experimental</b><br> 3854 * </p> 3855 */ 3856 @SearchParamDefinition(name="experimental", path="ImplementationGuide.experimental", description="For testing purposes, not real usage", type="token" ) 3857 public static final String SP_EXPERIMENTAL = "experimental"; 3858 /** 3859 * <b>Fluent Client</b> search parameter constant for <b>experimental</b> 3860 * <p> 3861 * Description: <b>For testing purposes, not real usage</b><br> 3862 * Type: <b>token</b><br> 3863 * Path: <b>ImplementationGuide.experimental</b><br> 3864 * </p> 3865 */ 3866 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EXPERIMENTAL = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EXPERIMENTAL); 3867 3868 /** 3869 * Search parameter: <b>version</b> 3870 * <p> 3871 * Description: <b>The business version of the implementation guide</b><br> 3872 * Type: <b>token</b><br> 3873 * Path: <b>ImplementationGuide.version</b><br> 3874 * </p> 3875 */ 3876 @SearchParamDefinition(name="version", path="ImplementationGuide.version", description="The business version of the implementation guide", type="token" ) 3877 public static final String SP_VERSION = "version"; 3878 /** 3879 * <b>Fluent Client</b> search parameter constant for <b>version</b> 3880 * <p> 3881 * Description: <b>The business version of the implementation guide</b><br> 3882 * Type: <b>token</b><br> 3883 * Path: <b>ImplementationGuide.version</b><br> 3884 * </p> 3885 */ 3886 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 3887 3888 /** 3889 * Search parameter: <b>url</b> 3890 * <p> 3891 * Description: <b>The uri that identifies the implementation guide</b><br> 3892 * Type: <b>uri</b><br> 3893 * Path: <b>ImplementationGuide.url</b><br> 3894 * </p> 3895 */ 3896 @SearchParamDefinition(name="url", path="ImplementationGuide.url", description="The uri that identifies the implementation guide", type="uri" ) 3897 public static final String SP_URL = "url"; 3898 /** 3899 * <b>Fluent Client</b> search parameter constant for <b>url</b> 3900 * <p> 3901 * Description: <b>The uri that identifies the implementation guide</b><br> 3902 * Type: <b>uri</b><br> 3903 * Path: <b>ImplementationGuide.url</b><br> 3904 * </p> 3905 */ 3906 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 3907 3908 /** 3909 * Search parameter: <b>status</b> 3910 * <p> 3911 * Description: <b>The current status of the implementation guide</b><br> 3912 * Type: <b>token</b><br> 3913 * Path: <b>ImplementationGuide.status</b><br> 3914 * </p> 3915 */ 3916 @SearchParamDefinition(name="status", path="ImplementationGuide.status", description="The current status of the implementation guide", type="token" ) 3917 public static final String SP_STATUS = "status"; 3918 /** 3919 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3920 * <p> 3921 * Description: <b>The current status of the implementation guide</b><br> 3922 * Type: <b>token</b><br> 3923 * Path: <b>ImplementationGuide.status</b><br> 3924 * </p> 3925 */ 3926 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3927 3928 3929}