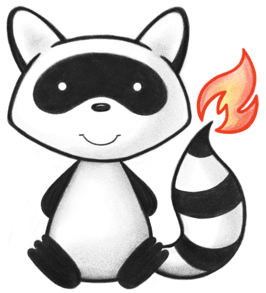
001package org.hl7.fhir.dstu3.model; 002 003/* 004 Copyright (c) 2011+, HL7, Inc. 005 All rights reserved. 006 007 Redistribution and use in source and binary forms, with or without modification, 008 are permitted provided that the following conditions are met: 009 010 * Redistributions of source code must retain the above copyright notice, this 011 list of conditions and the following disclaimer. 012 * Redistributions in binary form must reproduce the above copyright notice, 013 this list of conditions and the following disclaimer in the documentation 014 and/or other materials provided with the distribution. 015 * Neither the name of HL7 nor the names of its contributors may be used to 016 endorse or promote products derived from this software without specific 017 prior written permission. 018 019 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 020 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 021 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 022 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 023 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 024 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 025 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 026 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 027 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 028 POSSIBILITY OF SUCH DAMAGE. 029 030 */ 031 032 033 034import java.util.Calendar; 035import java.util.Date; 036import java.util.TimeZone; 037import java.util.zip.DataFormatException; 038 039import ca.uhn.fhir.model.api.TemporalPrecisionEnum; 040import ca.uhn.fhir.model.api.annotation.DatatypeDef; 041 042/** 043 * Represents a FHIR instant datatype. Valid precisions values for this type are: 044 * <ul> 045 * <li>{@link TemporalPrecisionEnum#SECOND} 046 * <li>{@link TemporalPrecisionEnum#MILLI} 047 * </ul> 048 */ 049@DatatypeDef(name="instant") 050public class InstantType extends BaseDateTimeType { 051 052 private static final long serialVersionUID = 3L; 053 054 /** 055 * The default precision for this type 056 */ 057 public static final TemporalPrecisionEnum DEFAULT_PRECISION = TemporalPrecisionEnum.MILLI; 058 059 /** 060 * Constructor which creates an InstantDt with <b>no timne value</b>. Note 061 * that unlike the default constructor for the Java {@link Date} or 062 * {@link Calendar} objects, this constructor does not initialize the object 063 * with the current time. 064 * 065 * @see #withCurrentTime() to create a new object that has been initialized 066 * with the current time. 067 */ 068 public InstantType() { 069 super(); 070 } 071 072 /** 073 * Create a new DateTimeDt 074 */ 075 public InstantType(Calendar theCalendar) { 076 super(theCalendar.getTime(), DEFAULT_PRECISION, theCalendar.getTimeZone()); 077 } 078 079 /** 080 * Create a new instance using the given date, precision level, and time zone 081 * 082 * @throws DataFormatException 083 * If the specified precision is not allowed for this type 084 */ 085 public InstantType(Date theDate, TemporalPrecisionEnum thePrecision, TimeZone theTimezone) { 086 super(theDate, thePrecision, theTimezone); 087 } 088 089 090 /** 091 * Create a new DateTimeDt using an existing value. <b>Use this constructor with caution</b>, 092 * as it may create more precision than warranted (since for example it is possible to pass in 093 * a DateTime with only a year, and this constructor will convert to an InstantDt with 094 * milliseconds precision). 095 */ 096 public InstantType(BaseDateTimeType theDateTime) { 097 // Do not call super(foo) here, we don't want to trigger a DataFormatException 098 setValue(theDateTime.getValue()); 099 setPrecision(DEFAULT_PRECISION); 100 setTimeZone(theDateTime.getTimeZone()); 101 } 102 103 /** 104 * Create a new DateTimeDt with the given date/time and {@link TemporalPrecisionEnum#MILLI} precision 105 */ 106 public InstantType(Date theDate) { 107 super(theDate, DEFAULT_PRECISION, TimeZone.getDefault()); 108 } 109 110 /** 111 * Constructor which accepts a date value and a precision value. Valid 112 * precisions values for this type are: 113 * <ul> 114 * <li>{@link TemporalPrecisionEnum#SECOND} 115 * <li>{@link TemporalPrecisionEnum#MILLI} 116 * </ul> 117 */ 118 public InstantType(Date theDate, TemporalPrecisionEnum thePrecision) { 119 setValue(theDate); 120 setPrecision(thePrecision); 121 setTimeZone(TimeZone.getDefault()); 122 } 123 124 /** 125 * Create a new InstantDt from a string value 126 * 127 * @param theString 128 * The string representation of the string. Must be in a valid 129 * format according to the FHIR specification 130 * @throws DataFormatException 131 */ 132 public InstantType(String theString) { 133 super(theString); 134 } 135 136 /** 137 * Invokes {@link Date#after(Date)} on the contained Date against the given 138 * date 139 * 140 * @throws NullPointerException 141 * If the {@link #getValue() contained Date} is null 142 */ 143 public boolean after(Date theDate) { 144 return getValue().after(theDate); 145 } 146 147 /** 148 * Invokes {@link Date#before(Date)} on the contained Date against the given 149 * date 150 * 151 * @throws NullPointerException 152 * If the {@link #getValue() contained Date} is null 153 */ 154 public boolean before(Date theDate) { 155 return getValue().before(theDate); 156 } 157 158 /** 159 * Sets the value of this instant to the current time (from the system 160 * clock) and the local/default timezone (as retrieved using 161 * {@link TimeZone#getDefault()}. This TimeZone is generally obtained from 162 * the underlying OS. 163 */ 164 public void setToCurrentTimeInLocalTimeZone() { 165 setValue(new Date()); 166 setTimeZone(TimeZone.getDefault()); 167 } 168 169 @Override 170 boolean isPrecisionAllowed(TemporalPrecisionEnum thePrecision) { 171 switch (thePrecision) { 172 case SECOND: 173 case MILLI: 174 return true; 175 default: 176 return false; 177 } 178 } 179 180 /** 181 * Factory method which creates a new InstantDt with millisecond precision and initializes it with the 182 * current time and the system local timezone. 183 */ 184 public static InstantType withCurrentTime() { 185 return new InstantType(new Date(), TemporalPrecisionEnum.MILLI, TimeZone.getDefault()); 186 } 187 188 /** 189 * Returns the default precision for this datatype 190 * 191 * @see #DEFAULT_PRECISION 192 */ 193 @Override 194 protected TemporalPrecisionEnum getDefaultPrecisionForDatatype() { 195 return DEFAULT_PRECISION; 196 } 197 198 199 @Override 200 public InstantType copy() { 201 InstantType ret = new InstantType(); 202 ret.setValueAsString(getValueAsString()); 203 return ret; 204 } 205 206 /** 207 * Returns a new instance of DateTimeType with the current system time and MILLI precision and the system local time 208 * zone 209 */ 210 public static InstantType now() { 211 return new InstantType(new Date(), TemporalPrecisionEnum.MILLI, TimeZone.getDefault()); 212 } 213 214 /** 215 * Creates a new instance by parsing an HL7 v3 format date time string 216 */ 217 public static InstantType parseV3(String theV3String) { 218 InstantType retVal = new InstantType(); 219 retVal.setValueAsV3String(theV3String); 220 return retVal; 221 } 222 223 public String fhirType() { 224 return "instant"; 225 } 226}