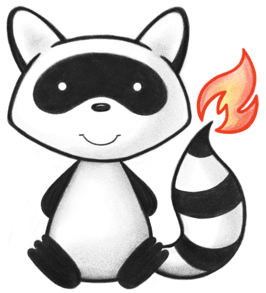
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.ChildOrder; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * The Library resource is a general-purpose container for knowledge asset definitions. It can be used to describe and expose existing knowledge assets such as logic libraries and information model descriptions, as well as to describe a collection of knowledge assets. 051 */ 052@ResourceDef(name="Library", profile="http://hl7.org/fhir/Profile/Library") 053@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "type", "date", "publisher", "description", "purpose", "usage", "approvalDate", "lastReviewDate", "effectivePeriod", "useContext", "jurisdiction", "topic", "contributor", "contact", "copyright", "relatedArtifact", "parameter", "dataRequirement", "content"}) 054public class Library extends MetadataResource { 055 056 /** 057 * A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts. 058 */ 059 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 060 @Description(shortDefinition="Additional identifier for the library", formalDefinition="A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts." ) 061 protected List<Identifier> identifier; 062 063 /** 064 * Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition. 065 */ 066 @Child(name = "type", type = {CodeableConcept.class}, order=1, min=1, max=1, modifier=false, summary=true) 067 @Description(shortDefinition="logic-library | model-definition | asset-collection | module-definition", formalDefinition="Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition." ) 068 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/library-type") 069 protected CodeableConcept type; 070 071 /** 072 * Explaination of why this library is needed and why it has been designed as it has. 073 */ 074 @Child(name = "purpose", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 075 @Description(shortDefinition="Why this library is defined", formalDefinition="Explaination of why this library is needed and why it has been designed as it has." ) 076 protected MarkdownType purpose; 077 078 /** 079 * A detailed description of how the library is used from a clinical perspective. 080 */ 081 @Child(name = "usage", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 082 @Description(shortDefinition="Describes the clinical usage of the library", formalDefinition="A detailed description of how the library is used from a clinical perspective." ) 083 protected StringType usage; 084 085 /** 086 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 087 */ 088 @Child(name = "approvalDate", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 089 @Description(shortDefinition="When the library was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 090 protected DateType approvalDate; 091 092 /** 093 * The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 094 */ 095 @Child(name = "lastReviewDate", type = {DateType.class}, order=5, min=0, max=1, modifier=false, summary=false) 096 @Description(shortDefinition="When the library was last reviewed", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date." ) 097 protected DateType lastReviewDate; 098 099 /** 100 * The period during which the library content was or is planned to be in active use. 101 */ 102 @Child(name = "effectivePeriod", type = {Period.class}, order=6, min=0, max=1, modifier=false, summary=true) 103 @Description(shortDefinition="When the library is expected to be used", formalDefinition="The period during which the library content was or is planned to be in active use." ) 104 protected Period effectivePeriod; 105 106 /** 107 * Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching. 108 */ 109 @Child(name = "topic", type = {CodeableConcept.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 110 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching." ) 111 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 112 protected List<CodeableConcept> topic; 113 114 /** 115 * A contributor to the content of the library, including authors, editors, reviewers, and endorsers. 116 */ 117 @Child(name = "contributor", type = {Contributor.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 118 @Description(shortDefinition="A content contributor", formalDefinition="A contributor to the content of the library, including authors, editors, reviewers, and endorsers." ) 119 protected List<Contributor> contributor; 120 121 /** 122 * A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library. 123 */ 124 @Child(name = "copyright", type = {MarkdownType.class}, order=9, min=0, max=1, modifier=false, summary=false) 125 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library." ) 126 protected MarkdownType copyright; 127 128 /** 129 * Related artifacts such as additional documentation, justification, or bibliographic references. 130 */ 131 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 132 @Description(shortDefinition="Additional documentation, citations, etc.", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 133 protected List<RelatedArtifact> relatedArtifact; 134 135 /** 136 * The parameter element defines parameters used by the library. 137 */ 138 @Child(name = "parameter", type = {ParameterDefinition.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 139 @Description(shortDefinition="Parameters defined by the library", formalDefinition="The parameter element defines parameters used by the library." ) 140 protected List<ParameterDefinition> parameter; 141 142 /** 143 * Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library. 144 */ 145 @Child(name = "dataRequirement", type = {DataRequirement.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 146 @Description(shortDefinition="What data is referenced by this library", formalDefinition="Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library." ) 147 protected List<DataRequirement> dataRequirement; 148 149 /** 150 * The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content. 151 */ 152 @Child(name = "content", type = {Attachment.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 153 @Description(shortDefinition="Contents of the library, either embedded or referenced", formalDefinition="The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content." ) 154 protected List<Attachment> content; 155 156 private static final long serialVersionUID = -39463327L; 157 158 /** 159 * Constructor 160 */ 161 public Library() { 162 super(); 163 } 164 165 /** 166 * Constructor 167 */ 168 public Library(Enumeration<PublicationStatus> status, CodeableConcept type) { 169 super(); 170 this.status = status; 171 this.type = type; 172 } 173 174 /** 175 * @return {@link #url} (An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this library is (or will be) published. The URL SHOULD include the major version of the library. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 176 */ 177 public UriType getUrlElement() { 178 if (this.url == null) 179 if (Configuration.errorOnAutoCreate()) 180 throw new Error("Attempt to auto-create Library.url"); 181 else if (Configuration.doAutoCreate()) 182 this.url = new UriType(); // bb 183 return this.url; 184 } 185 186 public boolean hasUrlElement() { 187 return this.url != null && !this.url.isEmpty(); 188 } 189 190 public boolean hasUrl() { 191 return this.url != null && !this.url.isEmpty(); 192 } 193 194 /** 195 * @param value {@link #url} (An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this library is (or will be) published. The URL SHOULD include the major version of the library. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 196 */ 197 public Library setUrlElement(UriType value) { 198 this.url = value; 199 return this; 200 } 201 202 /** 203 * @return An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this library is (or will be) published. The URL SHOULD include the major version of the library. For more information see [Technical and Business Versions](resource.html#versions). 204 */ 205 public String getUrl() { 206 return this.url == null ? null : this.url.getValue(); 207 } 208 209 /** 210 * @param value An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this library is (or will be) published. The URL SHOULD include the major version of the library. For more information see [Technical and Business Versions](resource.html#versions). 211 */ 212 public Library setUrl(String value) { 213 if (Utilities.noString(value)) 214 this.url = null; 215 else { 216 if (this.url == null) 217 this.url = new UriType(); 218 this.url.setValue(value); 219 } 220 return this; 221 } 222 223 /** 224 * @return {@link #identifier} (A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.) 225 */ 226 public List<Identifier> getIdentifier() { 227 if (this.identifier == null) 228 this.identifier = new ArrayList<Identifier>(); 229 return this.identifier; 230 } 231 232 /** 233 * @return Returns a reference to <code>this</code> for easy method chaining 234 */ 235 public Library setIdentifier(List<Identifier> theIdentifier) { 236 this.identifier = theIdentifier; 237 return this; 238 } 239 240 public boolean hasIdentifier() { 241 if (this.identifier == null) 242 return false; 243 for (Identifier item : this.identifier) 244 if (!item.isEmpty()) 245 return true; 246 return false; 247 } 248 249 public Identifier addIdentifier() { //3 250 Identifier t = new Identifier(); 251 if (this.identifier == null) 252 this.identifier = new ArrayList<Identifier>(); 253 this.identifier.add(t); 254 return t; 255 } 256 257 public Library addIdentifier(Identifier t) { //3 258 if (t == null) 259 return this; 260 if (this.identifier == null) 261 this.identifier = new ArrayList<Identifier>(); 262 this.identifier.add(t); 263 return this; 264 } 265 266 /** 267 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 268 */ 269 public Identifier getIdentifierFirstRep() { 270 if (getIdentifier().isEmpty()) { 271 addIdentifier(); 272 } 273 return getIdentifier().get(0); 274 } 275 276 /** 277 * @return {@link #version} (The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 278 */ 279 public StringType getVersionElement() { 280 if (this.version == null) 281 if (Configuration.errorOnAutoCreate()) 282 throw new Error("Attempt to auto-create Library.version"); 283 else if (Configuration.doAutoCreate()) 284 this.version = new StringType(); // bb 285 return this.version; 286 } 287 288 public boolean hasVersionElement() { 289 return this.version != null && !this.version.isEmpty(); 290 } 291 292 public boolean hasVersion() { 293 return this.version != null && !this.version.isEmpty(); 294 } 295 296 /** 297 * @param value {@link #version} (The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 298 */ 299 public Library setVersionElement(StringType value) { 300 this.version = value; 301 return this; 302 } 303 304 /** 305 * @return The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 306 */ 307 public String getVersion() { 308 return this.version == null ? null : this.version.getValue(); 309 } 310 311 /** 312 * @param value The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 313 */ 314 public Library setVersion(String value) { 315 if (Utilities.noString(value)) 316 this.version = null; 317 else { 318 if (this.version == null) 319 this.version = new StringType(); 320 this.version.setValue(value); 321 } 322 return this; 323 } 324 325 /** 326 * @return {@link #name} (A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 327 */ 328 public StringType getNameElement() { 329 if (this.name == null) 330 if (Configuration.errorOnAutoCreate()) 331 throw new Error("Attempt to auto-create Library.name"); 332 else if (Configuration.doAutoCreate()) 333 this.name = new StringType(); // bb 334 return this.name; 335 } 336 337 public boolean hasNameElement() { 338 return this.name != null && !this.name.isEmpty(); 339 } 340 341 public boolean hasName() { 342 return this.name != null && !this.name.isEmpty(); 343 } 344 345 /** 346 * @param value {@link #name} (A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 347 */ 348 public Library setNameElement(StringType value) { 349 this.name = value; 350 return this; 351 } 352 353 /** 354 * @return A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation. 355 */ 356 public String getName() { 357 return this.name == null ? null : this.name.getValue(); 358 } 359 360 /** 361 * @param value A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation. 362 */ 363 public Library setName(String value) { 364 if (Utilities.noString(value)) 365 this.name = null; 366 else { 367 if (this.name == null) 368 this.name = new StringType(); 369 this.name.setValue(value); 370 } 371 return this; 372 } 373 374 /** 375 * @return {@link #title} (A short, descriptive, user-friendly title for the library.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 376 */ 377 public StringType getTitleElement() { 378 if (this.title == null) 379 if (Configuration.errorOnAutoCreate()) 380 throw new Error("Attempt to auto-create Library.title"); 381 else if (Configuration.doAutoCreate()) 382 this.title = new StringType(); // bb 383 return this.title; 384 } 385 386 public boolean hasTitleElement() { 387 return this.title != null && !this.title.isEmpty(); 388 } 389 390 public boolean hasTitle() { 391 return this.title != null && !this.title.isEmpty(); 392 } 393 394 /** 395 * @param value {@link #title} (A short, descriptive, user-friendly title for the library.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 396 */ 397 public Library setTitleElement(StringType value) { 398 this.title = value; 399 return this; 400 } 401 402 /** 403 * @return A short, descriptive, user-friendly title for the library. 404 */ 405 public String getTitle() { 406 return this.title == null ? null : this.title.getValue(); 407 } 408 409 /** 410 * @param value A short, descriptive, user-friendly title for the library. 411 */ 412 public Library setTitle(String value) { 413 if (Utilities.noString(value)) 414 this.title = null; 415 else { 416 if (this.title == null) 417 this.title = new StringType(); 418 this.title.setValue(value); 419 } 420 return this; 421 } 422 423 /** 424 * @return {@link #status} (The status of this library. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 425 */ 426 public Enumeration<PublicationStatus> getStatusElement() { 427 if (this.status == null) 428 if (Configuration.errorOnAutoCreate()) 429 throw new Error("Attempt to auto-create Library.status"); 430 else if (Configuration.doAutoCreate()) 431 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 432 return this.status; 433 } 434 435 public boolean hasStatusElement() { 436 return this.status != null && !this.status.isEmpty(); 437 } 438 439 public boolean hasStatus() { 440 return this.status != null && !this.status.isEmpty(); 441 } 442 443 /** 444 * @param value {@link #status} (The status of this library. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 445 */ 446 public Library setStatusElement(Enumeration<PublicationStatus> value) { 447 this.status = value; 448 return this; 449 } 450 451 /** 452 * @return The status of this library. Enables tracking the life-cycle of the content. 453 */ 454 public PublicationStatus getStatus() { 455 return this.status == null ? null : this.status.getValue(); 456 } 457 458 /** 459 * @param value The status of this library. Enables tracking the life-cycle of the content. 460 */ 461 public Library setStatus(PublicationStatus value) { 462 if (this.status == null) 463 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 464 this.status.setValue(value); 465 return this; 466 } 467 468 /** 469 * @return {@link #experimental} (A boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 470 */ 471 public BooleanType getExperimentalElement() { 472 if (this.experimental == null) 473 if (Configuration.errorOnAutoCreate()) 474 throw new Error("Attempt to auto-create Library.experimental"); 475 else if (Configuration.doAutoCreate()) 476 this.experimental = new BooleanType(); // bb 477 return this.experimental; 478 } 479 480 public boolean hasExperimentalElement() { 481 return this.experimental != null && !this.experimental.isEmpty(); 482 } 483 484 public boolean hasExperimental() { 485 return this.experimental != null && !this.experimental.isEmpty(); 486 } 487 488 /** 489 * @param value {@link #experimental} (A boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 490 */ 491 public Library setExperimentalElement(BooleanType value) { 492 this.experimental = value; 493 return this; 494 } 495 496 /** 497 * @return A boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 498 */ 499 public boolean getExperimental() { 500 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 501 } 502 503 /** 504 * @param value A boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 505 */ 506 public Library setExperimental(boolean value) { 507 if (this.experimental == null) 508 this.experimental = new BooleanType(); 509 this.experimental.setValue(value); 510 return this; 511 } 512 513 /** 514 * @return {@link #type} (Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.) 515 */ 516 public CodeableConcept getType() { 517 if (this.type == null) 518 if (Configuration.errorOnAutoCreate()) 519 throw new Error("Attempt to auto-create Library.type"); 520 else if (Configuration.doAutoCreate()) 521 this.type = new CodeableConcept(); // cc 522 return this.type; 523 } 524 525 public boolean hasType() { 526 return this.type != null && !this.type.isEmpty(); 527 } 528 529 /** 530 * @param value {@link #type} (Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.) 531 */ 532 public Library setType(CodeableConcept value) { 533 this.type = value; 534 return this; 535 } 536 537 /** 538 * @return {@link #date} (The date (and optionally time) when the library was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 539 */ 540 public DateTimeType getDateElement() { 541 if (this.date == null) 542 if (Configuration.errorOnAutoCreate()) 543 throw new Error("Attempt to auto-create Library.date"); 544 else if (Configuration.doAutoCreate()) 545 this.date = new DateTimeType(); // bb 546 return this.date; 547 } 548 549 public boolean hasDateElement() { 550 return this.date != null && !this.date.isEmpty(); 551 } 552 553 public boolean hasDate() { 554 return this.date != null && !this.date.isEmpty(); 555 } 556 557 /** 558 * @param value {@link #date} (The date (and optionally time) when the library was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 559 */ 560 public Library setDateElement(DateTimeType value) { 561 this.date = value; 562 return this; 563 } 564 565 /** 566 * @return The date (and optionally time) when the library was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes. 567 */ 568 public Date getDate() { 569 return this.date == null ? null : this.date.getValue(); 570 } 571 572 /** 573 * @param value The date (and optionally time) when the library was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes. 574 */ 575 public Library setDate(Date value) { 576 if (value == null) 577 this.date = null; 578 else { 579 if (this.date == null) 580 this.date = new DateTimeType(); 581 this.date.setValue(value); 582 } 583 return this; 584 } 585 586 /** 587 * @return {@link #publisher} (The name of the individual or organization that published the library.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 588 */ 589 public StringType getPublisherElement() { 590 if (this.publisher == null) 591 if (Configuration.errorOnAutoCreate()) 592 throw new Error("Attempt to auto-create Library.publisher"); 593 else if (Configuration.doAutoCreate()) 594 this.publisher = new StringType(); // bb 595 return this.publisher; 596 } 597 598 public boolean hasPublisherElement() { 599 return this.publisher != null && !this.publisher.isEmpty(); 600 } 601 602 public boolean hasPublisher() { 603 return this.publisher != null && !this.publisher.isEmpty(); 604 } 605 606 /** 607 * @param value {@link #publisher} (The name of the individual or organization that published the library.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 608 */ 609 public Library setPublisherElement(StringType value) { 610 this.publisher = value; 611 return this; 612 } 613 614 /** 615 * @return The name of the individual or organization that published the library. 616 */ 617 public String getPublisher() { 618 return this.publisher == null ? null : this.publisher.getValue(); 619 } 620 621 /** 622 * @param value The name of the individual or organization that published the library. 623 */ 624 public Library setPublisher(String value) { 625 if (Utilities.noString(value)) 626 this.publisher = null; 627 else { 628 if (this.publisher == null) 629 this.publisher = new StringType(); 630 this.publisher.setValue(value); 631 } 632 return this; 633 } 634 635 /** 636 * @return {@link #description} (A free text natural language description of the library from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 637 */ 638 public MarkdownType getDescriptionElement() { 639 if (this.description == null) 640 if (Configuration.errorOnAutoCreate()) 641 throw new Error("Attempt to auto-create Library.description"); 642 else if (Configuration.doAutoCreate()) 643 this.description = new MarkdownType(); // bb 644 return this.description; 645 } 646 647 public boolean hasDescriptionElement() { 648 return this.description != null && !this.description.isEmpty(); 649 } 650 651 public boolean hasDescription() { 652 return this.description != null && !this.description.isEmpty(); 653 } 654 655 /** 656 * @param value {@link #description} (A free text natural language description of the library from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 657 */ 658 public Library setDescriptionElement(MarkdownType value) { 659 this.description = value; 660 return this; 661 } 662 663 /** 664 * @return A free text natural language description of the library from a consumer's perspective. 665 */ 666 public String getDescription() { 667 return this.description == null ? null : this.description.getValue(); 668 } 669 670 /** 671 * @param value A free text natural language description of the library from a consumer's perspective. 672 */ 673 public Library setDescription(String value) { 674 if (value == null) 675 this.description = null; 676 else { 677 if (this.description == null) 678 this.description = new MarkdownType(); 679 this.description.setValue(value); 680 } 681 return this; 682 } 683 684 /** 685 * @return {@link #purpose} (Explaination of why this library is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 686 */ 687 public MarkdownType getPurposeElement() { 688 if (this.purpose == null) 689 if (Configuration.errorOnAutoCreate()) 690 throw new Error("Attempt to auto-create Library.purpose"); 691 else if (Configuration.doAutoCreate()) 692 this.purpose = new MarkdownType(); // bb 693 return this.purpose; 694 } 695 696 public boolean hasPurposeElement() { 697 return this.purpose != null && !this.purpose.isEmpty(); 698 } 699 700 public boolean hasPurpose() { 701 return this.purpose != null && !this.purpose.isEmpty(); 702 } 703 704 /** 705 * @param value {@link #purpose} (Explaination of why this library is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 706 */ 707 public Library setPurposeElement(MarkdownType value) { 708 this.purpose = value; 709 return this; 710 } 711 712 /** 713 * @return Explaination of why this library is needed and why it has been designed as it has. 714 */ 715 public String getPurpose() { 716 return this.purpose == null ? null : this.purpose.getValue(); 717 } 718 719 /** 720 * @param value Explaination of why this library is needed and why it has been designed as it has. 721 */ 722 public Library setPurpose(String value) { 723 if (value == null) 724 this.purpose = null; 725 else { 726 if (this.purpose == null) 727 this.purpose = new MarkdownType(); 728 this.purpose.setValue(value); 729 } 730 return this; 731 } 732 733 /** 734 * @return {@link #usage} (A detailed description of how the library is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 735 */ 736 public StringType getUsageElement() { 737 if (this.usage == null) 738 if (Configuration.errorOnAutoCreate()) 739 throw new Error("Attempt to auto-create Library.usage"); 740 else if (Configuration.doAutoCreate()) 741 this.usage = new StringType(); // bb 742 return this.usage; 743 } 744 745 public boolean hasUsageElement() { 746 return this.usage != null && !this.usage.isEmpty(); 747 } 748 749 public boolean hasUsage() { 750 return this.usage != null && !this.usage.isEmpty(); 751 } 752 753 /** 754 * @param value {@link #usage} (A detailed description of how the library is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 755 */ 756 public Library setUsageElement(StringType value) { 757 this.usage = value; 758 return this; 759 } 760 761 /** 762 * @return A detailed description of how the library is used from a clinical perspective. 763 */ 764 public String getUsage() { 765 return this.usage == null ? null : this.usage.getValue(); 766 } 767 768 /** 769 * @param value A detailed description of how the library is used from a clinical perspective. 770 */ 771 public Library setUsage(String value) { 772 if (Utilities.noString(value)) 773 this.usage = null; 774 else { 775 if (this.usage == null) 776 this.usage = new StringType(); 777 this.usage.setValue(value); 778 } 779 return this; 780 } 781 782 /** 783 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 784 */ 785 public DateType getApprovalDateElement() { 786 if (this.approvalDate == null) 787 if (Configuration.errorOnAutoCreate()) 788 throw new Error("Attempt to auto-create Library.approvalDate"); 789 else if (Configuration.doAutoCreate()) 790 this.approvalDate = new DateType(); // bb 791 return this.approvalDate; 792 } 793 794 public boolean hasApprovalDateElement() { 795 return this.approvalDate != null && !this.approvalDate.isEmpty(); 796 } 797 798 public boolean hasApprovalDate() { 799 return this.approvalDate != null && !this.approvalDate.isEmpty(); 800 } 801 802 /** 803 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 804 */ 805 public Library setApprovalDateElement(DateType value) { 806 this.approvalDate = value; 807 return this; 808 } 809 810 /** 811 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 812 */ 813 public Date getApprovalDate() { 814 return this.approvalDate == null ? null : this.approvalDate.getValue(); 815 } 816 817 /** 818 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 819 */ 820 public Library setApprovalDate(Date value) { 821 if (value == null) 822 this.approvalDate = null; 823 else { 824 if (this.approvalDate == null) 825 this.approvalDate = new DateType(); 826 this.approvalDate.setValue(value); 827 } 828 return this; 829 } 830 831 /** 832 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 833 */ 834 public DateType getLastReviewDateElement() { 835 if (this.lastReviewDate == null) 836 if (Configuration.errorOnAutoCreate()) 837 throw new Error("Attempt to auto-create Library.lastReviewDate"); 838 else if (Configuration.doAutoCreate()) 839 this.lastReviewDate = new DateType(); // bb 840 return this.lastReviewDate; 841 } 842 843 public boolean hasLastReviewDateElement() { 844 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 845 } 846 847 public boolean hasLastReviewDate() { 848 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 849 } 850 851 /** 852 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 853 */ 854 public Library setLastReviewDateElement(DateType value) { 855 this.lastReviewDate = value; 856 return this; 857 } 858 859 /** 860 * @return The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 861 */ 862 public Date getLastReviewDate() { 863 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 864 } 865 866 /** 867 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 868 */ 869 public Library setLastReviewDate(Date value) { 870 if (value == null) 871 this.lastReviewDate = null; 872 else { 873 if (this.lastReviewDate == null) 874 this.lastReviewDate = new DateType(); 875 this.lastReviewDate.setValue(value); 876 } 877 return this; 878 } 879 880 /** 881 * @return {@link #effectivePeriod} (The period during which the library content was or is planned to be in active use.) 882 */ 883 public Period getEffectivePeriod() { 884 if (this.effectivePeriod == null) 885 if (Configuration.errorOnAutoCreate()) 886 throw new Error("Attempt to auto-create Library.effectivePeriod"); 887 else if (Configuration.doAutoCreate()) 888 this.effectivePeriod = new Period(); // cc 889 return this.effectivePeriod; 890 } 891 892 public boolean hasEffectivePeriod() { 893 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 894 } 895 896 /** 897 * @param value {@link #effectivePeriod} (The period during which the library content was or is planned to be in active use.) 898 */ 899 public Library setEffectivePeriod(Period value) { 900 this.effectivePeriod = value; 901 return this; 902 } 903 904 /** 905 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate library instances.) 906 */ 907 public List<UsageContext> getUseContext() { 908 if (this.useContext == null) 909 this.useContext = new ArrayList<UsageContext>(); 910 return this.useContext; 911 } 912 913 /** 914 * @return Returns a reference to <code>this</code> for easy method chaining 915 */ 916 public Library setUseContext(List<UsageContext> theUseContext) { 917 this.useContext = theUseContext; 918 return this; 919 } 920 921 public boolean hasUseContext() { 922 if (this.useContext == null) 923 return false; 924 for (UsageContext item : this.useContext) 925 if (!item.isEmpty()) 926 return true; 927 return false; 928 } 929 930 public UsageContext addUseContext() { //3 931 UsageContext t = new UsageContext(); 932 if (this.useContext == null) 933 this.useContext = new ArrayList<UsageContext>(); 934 this.useContext.add(t); 935 return t; 936 } 937 938 public Library addUseContext(UsageContext t) { //3 939 if (t == null) 940 return this; 941 if (this.useContext == null) 942 this.useContext = new ArrayList<UsageContext>(); 943 this.useContext.add(t); 944 return this; 945 } 946 947 /** 948 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 949 */ 950 public UsageContext getUseContextFirstRep() { 951 if (getUseContext().isEmpty()) { 952 addUseContext(); 953 } 954 return getUseContext().get(0); 955 } 956 957 /** 958 * @return {@link #jurisdiction} (A legal or geographic region in which the library is intended to be used.) 959 */ 960 public List<CodeableConcept> getJurisdiction() { 961 if (this.jurisdiction == null) 962 this.jurisdiction = new ArrayList<CodeableConcept>(); 963 return this.jurisdiction; 964 } 965 966 /** 967 * @return Returns a reference to <code>this</code> for easy method chaining 968 */ 969 public Library setJurisdiction(List<CodeableConcept> theJurisdiction) { 970 this.jurisdiction = theJurisdiction; 971 return this; 972 } 973 974 public boolean hasJurisdiction() { 975 if (this.jurisdiction == null) 976 return false; 977 for (CodeableConcept item : this.jurisdiction) 978 if (!item.isEmpty()) 979 return true; 980 return false; 981 } 982 983 public CodeableConcept addJurisdiction() { //3 984 CodeableConcept t = new CodeableConcept(); 985 if (this.jurisdiction == null) 986 this.jurisdiction = new ArrayList<CodeableConcept>(); 987 this.jurisdiction.add(t); 988 return t; 989 } 990 991 public Library addJurisdiction(CodeableConcept t) { //3 992 if (t == null) 993 return this; 994 if (this.jurisdiction == null) 995 this.jurisdiction = new ArrayList<CodeableConcept>(); 996 this.jurisdiction.add(t); 997 return this; 998 } 999 1000 /** 1001 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1002 */ 1003 public CodeableConcept getJurisdictionFirstRep() { 1004 if (getJurisdiction().isEmpty()) { 1005 addJurisdiction(); 1006 } 1007 return getJurisdiction().get(0); 1008 } 1009 1010 /** 1011 * @return {@link #topic} (Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.) 1012 */ 1013 public List<CodeableConcept> getTopic() { 1014 if (this.topic == null) 1015 this.topic = new ArrayList<CodeableConcept>(); 1016 return this.topic; 1017 } 1018 1019 /** 1020 * @return Returns a reference to <code>this</code> for easy method chaining 1021 */ 1022 public Library setTopic(List<CodeableConcept> theTopic) { 1023 this.topic = theTopic; 1024 return this; 1025 } 1026 1027 public boolean hasTopic() { 1028 if (this.topic == null) 1029 return false; 1030 for (CodeableConcept item : this.topic) 1031 if (!item.isEmpty()) 1032 return true; 1033 return false; 1034 } 1035 1036 public CodeableConcept addTopic() { //3 1037 CodeableConcept t = new CodeableConcept(); 1038 if (this.topic == null) 1039 this.topic = new ArrayList<CodeableConcept>(); 1040 this.topic.add(t); 1041 return t; 1042 } 1043 1044 public Library addTopic(CodeableConcept t) { //3 1045 if (t == null) 1046 return this; 1047 if (this.topic == null) 1048 this.topic = new ArrayList<CodeableConcept>(); 1049 this.topic.add(t); 1050 return this; 1051 } 1052 1053 /** 1054 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 1055 */ 1056 public CodeableConcept getTopicFirstRep() { 1057 if (getTopic().isEmpty()) { 1058 addTopic(); 1059 } 1060 return getTopic().get(0); 1061 } 1062 1063 /** 1064 * @return {@link #contributor} (A contributor to the content of the library, including authors, editors, reviewers, and endorsers.) 1065 */ 1066 public List<Contributor> getContributor() { 1067 if (this.contributor == null) 1068 this.contributor = new ArrayList<Contributor>(); 1069 return this.contributor; 1070 } 1071 1072 /** 1073 * @return Returns a reference to <code>this</code> for easy method chaining 1074 */ 1075 public Library setContributor(List<Contributor> theContributor) { 1076 this.contributor = theContributor; 1077 return this; 1078 } 1079 1080 public boolean hasContributor() { 1081 if (this.contributor == null) 1082 return false; 1083 for (Contributor item : this.contributor) 1084 if (!item.isEmpty()) 1085 return true; 1086 return false; 1087 } 1088 1089 public Contributor addContributor() { //3 1090 Contributor t = new Contributor(); 1091 if (this.contributor == null) 1092 this.contributor = new ArrayList<Contributor>(); 1093 this.contributor.add(t); 1094 return t; 1095 } 1096 1097 public Library addContributor(Contributor t) { //3 1098 if (t == null) 1099 return this; 1100 if (this.contributor == null) 1101 this.contributor = new ArrayList<Contributor>(); 1102 this.contributor.add(t); 1103 return this; 1104 } 1105 1106 /** 1107 * @return The first repetition of repeating field {@link #contributor}, creating it if it does not already exist 1108 */ 1109 public Contributor getContributorFirstRep() { 1110 if (getContributor().isEmpty()) { 1111 addContributor(); 1112 } 1113 return getContributor().get(0); 1114 } 1115 1116 /** 1117 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1118 */ 1119 public List<ContactDetail> getContact() { 1120 if (this.contact == null) 1121 this.contact = new ArrayList<ContactDetail>(); 1122 return this.contact; 1123 } 1124 1125 /** 1126 * @return Returns a reference to <code>this</code> for easy method chaining 1127 */ 1128 public Library setContact(List<ContactDetail> theContact) { 1129 this.contact = theContact; 1130 return this; 1131 } 1132 1133 public boolean hasContact() { 1134 if (this.contact == null) 1135 return false; 1136 for (ContactDetail item : this.contact) 1137 if (!item.isEmpty()) 1138 return true; 1139 return false; 1140 } 1141 1142 public ContactDetail addContact() { //3 1143 ContactDetail t = new ContactDetail(); 1144 if (this.contact == null) 1145 this.contact = new ArrayList<ContactDetail>(); 1146 this.contact.add(t); 1147 return t; 1148 } 1149 1150 public Library addContact(ContactDetail t) { //3 1151 if (t == null) 1152 return this; 1153 if (this.contact == null) 1154 this.contact = new ArrayList<ContactDetail>(); 1155 this.contact.add(t); 1156 return this; 1157 } 1158 1159 /** 1160 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1161 */ 1162 public ContactDetail getContactFirstRep() { 1163 if (getContact().isEmpty()) { 1164 addContact(); 1165 } 1166 return getContact().get(0); 1167 } 1168 1169 /** 1170 * @return {@link #copyright} (A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1171 */ 1172 public MarkdownType getCopyrightElement() { 1173 if (this.copyright == null) 1174 if (Configuration.errorOnAutoCreate()) 1175 throw new Error("Attempt to auto-create Library.copyright"); 1176 else if (Configuration.doAutoCreate()) 1177 this.copyright = new MarkdownType(); // bb 1178 return this.copyright; 1179 } 1180 1181 public boolean hasCopyrightElement() { 1182 return this.copyright != null && !this.copyright.isEmpty(); 1183 } 1184 1185 public boolean hasCopyright() { 1186 return this.copyright != null && !this.copyright.isEmpty(); 1187 } 1188 1189 /** 1190 * @param value {@link #copyright} (A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1191 */ 1192 public Library setCopyrightElement(MarkdownType value) { 1193 this.copyright = value; 1194 return this; 1195 } 1196 1197 /** 1198 * @return A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library. 1199 */ 1200 public String getCopyright() { 1201 return this.copyright == null ? null : this.copyright.getValue(); 1202 } 1203 1204 /** 1205 * @param value A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library. 1206 */ 1207 public Library setCopyright(String value) { 1208 if (value == null) 1209 this.copyright = null; 1210 else { 1211 if (this.copyright == null) 1212 this.copyright = new MarkdownType(); 1213 this.copyright.setValue(value); 1214 } 1215 return this; 1216 } 1217 1218 /** 1219 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 1220 */ 1221 public List<RelatedArtifact> getRelatedArtifact() { 1222 if (this.relatedArtifact == null) 1223 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1224 return this.relatedArtifact; 1225 } 1226 1227 /** 1228 * @return Returns a reference to <code>this</code> for easy method chaining 1229 */ 1230 public Library setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 1231 this.relatedArtifact = theRelatedArtifact; 1232 return this; 1233 } 1234 1235 public boolean hasRelatedArtifact() { 1236 if (this.relatedArtifact == null) 1237 return false; 1238 for (RelatedArtifact item : this.relatedArtifact) 1239 if (!item.isEmpty()) 1240 return true; 1241 return false; 1242 } 1243 1244 public RelatedArtifact addRelatedArtifact() { //3 1245 RelatedArtifact t = new RelatedArtifact(); 1246 if (this.relatedArtifact == null) 1247 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1248 this.relatedArtifact.add(t); 1249 return t; 1250 } 1251 1252 public Library addRelatedArtifact(RelatedArtifact t) { //3 1253 if (t == null) 1254 return this; 1255 if (this.relatedArtifact == null) 1256 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 1257 this.relatedArtifact.add(t); 1258 return this; 1259 } 1260 1261 /** 1262 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist 1263 */ 1264 public RelatedArtifact getRelatedArtifactFirstRep() { 1265 if (getRelatedArtifact().isEmpty()) { 1266 addRelatedArtifact(); 1267 } 1268 return getRelatedArtifact().get(0); 1269 } 1270 1271 /** 1272 * @return {@link #parameter} (The parameter element defines parameters used by the library.) 1273 */ 1274 public List<ParameterDefinition> getParameter() { 1275 if (this.parameter == null) 1276 this.parameter = new ArrayList<ParameterDefinition>(); 1277 return this.parameter; 1278 } 1279 1280 /** 1281 * @return Returns a reference to <code>this</code> for easy method chaining 1282 */ 1283 public Library setParameter(List<ParameterDefinition> theParameter) { 1284 this.parameter = theParameter; 1285 return this; 1286 } 1287 1288 public boolean hasParameter() { 1289 if (this.parameter == null) 1290 return false; 1291 for (ParameterDefinition item : this.parameter) 1292 if (!item.isEmpty()) 1293 return true; 1294 return false; 1295 } 1296 1297 public ParameterDefinition addParameter() { //3 1298 ParameterDefinition t = new ParameterDefinition(); 1299 if (this.parameter == null) 1300 this.parameter = new ArrayList<ParameterDefinition>(); 1301 this.parameter.add(t); 1302 return t; 1303 } 1304 1305 public Library addParameter(ParameterDefinition t) { //3 1306 if (t == null) 1307 return this; 1308 if (this.parameter == null) 1309 this.parameter = new ArrayList<ParameterDefinition>(); 1310 this.parameter.add(t); 1311 return this; 1312 } 1313 1314 /** 1315 * @return The first repetition of repeating field {@link #parameter}, creating it if it does not already exist 1316 */ 1317 public ParameterDefinition getParameterFirstRep() { 1318 if (getParameter().isEmpty()) { 1319 addParameter(); 1320 } 1321 return getParameter().get(0); 1322 } 1323 1324 /** 1325 * @return {@link #dataRequirement} (Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.) 1326 */ 1327 public List<DataRequirement> getDataRequirement() { 1328 if (this.dataRequirement == null) 1329 this.dataRequirement = new ArrayList<DataRequirement>(); 1330 return this.dataRequirement; 1331 } 1332 1333 /** 1334 * @return Returns a reference to <code>this</code> for easy method chaining 1335 */ 1336 public Library setDataRequirement(List<DataRequirement> theDataRequirement) { 1337 this.dataRequirement = theDataRequirement; 1338 return this; 1339 } 1340 1341 public boolean hasDataRequirement() { 1342 if (this.dataRequirement == null) 1343 return false; 1344 for (DataRequirement item : this.dataRequirement) 1345 if (!item.isEmpty()) 1346 return true; 1347 return false; 1348 } 1349 1350 public DataRequirement addDataRequirement() { //3 1351 DataRequirement t = new DataRequirement(); 1352 if (this.dataRequirement == null) 1353 this.dataRequirement = new ArrayList<DataRequirement>(); 1354 this.dataRequirement.add(t); 1355 return t; 1356 } 1357 1358 public Library addDataRequirement(DataRequirement t) { //3 1359 if (t == null) 1360 return this; 1361 if (this.dataRequirement == null) 1362 this.dataRequirement = new ArrayList<DataRequirement>(); 1363 this.dataRequirement.add(t); 1364 return this; 1365 } 1366 1367 /** 1368 * @return The first repetition of repeating field {@link #dataRequirement}, creating it if it does not already exist 1369 */ 1370 public DataRequirement getDataRequirementFirstRep() { 1371 if (getDataRequirement().isEmpty()) { 1372 addDataRequirement(); 1373 } 1374 return getDataRequirement().get(0); 1375 } 1376 1377 /** 1378 * @return {@link #content} (The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.) 1379 */ 1380 public List<Attachment> getContent() { 1381 if (this.content == null) 1382 this.content = new ArrayList<Attachment>(); 1383 return this.content; 1384 } 1385 1386 /** 1387 * @return Returns a reference to <code>this</code> for easy method chaining 1388 */ 1389 public Library setContent(List<Attachment> theContent) { 1390 this.content = theContent; 1391 return this; 1392 } 1393 1394 public boolean hasContent() { 1395 if (this.content == null) 1396 return false; 1397 for (Attachment item : this.content) 1398 if (!item.isEmpty()) 1399 return true; 1400 return false; 1401 } 1402 1403 public Attachment addContent() { //3 1404 Attachment t = new Attachment(); 1405 if (this.content == null) 1406 this.content = new ArrayList<Attachment>(); 1407 this.content.add(t); 1408 return t; 1409 } 1410 1411 public Library addContent(Attachment t) { //3 1412 if (t == null) 1413 return this; 1414 if (this.content == null) 1415 this.content = new ArrayList<Attachment>(); 1416 this.content.add(t); 1417 return this; 1418 } 1419 1420 /** 1421 * @return The first repetition of repeating field {@link #content}, creating it if it does not already exist 1422 */ 1423 public Attachment getContentFirstRep() { 1424 if (getContent().isEmpty()) { 1425 addContent(); 1426 } 1427 return getContent().get(0); 1428 } 1429 1430 protected void listChildren(List<Property> children) { 1431 super.listChildren(children); 1432 children.add(new Property("url", "uri", "An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this library is (or will be) published. The URL SHOULD include the major version of the library. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 1433 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1434 children.add(new Property("version", "string", "The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version)); 1435 children.add(new Property("name", "string", "A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 1436 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the library.", 0, 1, title)); 1437 children.add(new Property("status", "code", "The status of this library. Enables tracking the life-cycle of the content.", 0, 1, status)); 1438 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 1439 children.add(new Property("type", "CodeableConcept", "Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.", 0, 1, type)); 1440 children.add(new Property("date", "dateTime", "The date (and optionally time) when the library was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.", 0, 1, date)); 1441 children.add(new Property("publisher", "string", "The name of the individual or organization that published the library.", 0, 1, publisher)); 1442 children.add(new Property("description", "markdown", "A free text natural language description of the library from a consumer's perspective.", 0, 1, description)); 1443 children.add(new Property("purpose", "markdown", "Explaination of why this library is needed and why it has been designed as it has.", 0, 1, purpose)); 1444 children.add(new Property("usage", "string", "A detailed description of how the library is used from a clinical perspective.", 0, 1, usage)); 1445 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 1446 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 1447 children.add(new Property("effectivePeriod", "Period", "The period during which the library content was or is planned to be in active use.", 0, 1, effectivePeriod)); 1448 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate library instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 1449 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the library is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 1450 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 1451 children.add(new Property("contributor", "Contributor", "A contributor to the content of the library, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor)); 1452 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 1453 children.add(new Property("copyright", "markdown", "A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.", 0, 1, copyright)); 1454 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 1455 children.add(new Property("parameter", "ParameterDefinition", "The parameter element defines parameters used by the library.", 0, java.lang.Integer.MAX_VALUE, parameter)); 1456 children.add(new Property("dataRequirement", "DataRequirement", "Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.", 0, java.lang.Integer.MAX_VALUE, dataRequirement)); 1457 children.add(new Property("content", "Attachment", "The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.", 0, java.lang.Integer.MAX_VALUE, content)); 1458 } 1459 1460 @Override 1461 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1462 switch (_hash) { 1463 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this library when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this library is (or will be) published. The URL SHOULD include the major version of the library. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 1464 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this library when it is represented in other formats, or referenced in a specification, model, design or an instance. e.g. CMS or NQF identifiers for a measure artifact. Note that at least one identifier is required for non-experimental active artifacts.", 0, java.lang.Integer.MAX_VALUE, identifier); 1465 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the library when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the library author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version); 1466 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the library. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 1467 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the library.", 0, 1, title); 1468 case -892481550: /*status*/ return new Property("status", "code", "The status of this library. Enables tracking the life-cycle of the content.", 0, 1, status); 1469 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this library is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 1470 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Identifies the type of library such as a Logic Library, Model Definition, Asset Collection, or Module Definition.", 0, 1, type); 1471 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the library was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the library changes.", 0, 1, date); 1472 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the library.", 0, 1, publisher); 1473 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the library from a consumer's perspective.", 0, 1, description); 1474 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this library is needed and why it has been designed as it has.", 0, 1, purpose); 1475 case 111574433: /*usage*/ return new Property("usage", "string", "A detailed description of how the library is used from a clinical perspective.", 0, 1, usage); 1476 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 1477 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate); 1478 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the library content was or is planned to be in active use.", 0, 1, effectivePeriod); 1479 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate library instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 1480 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the library is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 1481 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the library. Topics provide a high-level categorization of the library that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 1482 case -1895276325: /*contributor*/ return new Property("contributor", "Contributor", "A contributor to the content of the library, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor); 1483 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 1484 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the library and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the library.", 0, 1, copyright); 1485 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 1486 case 1954460585: /*parameter*/ return new Property("parameter", "ParameterDefinition", "The parameter element defines parameters used by the library.", 0, java.lang.Integer.MAX_VALUE, parameter); 1487 case 629147193: /*dataRequirement*/ return new Property("dataRequirement", "DataRequirement", "Describes a set of data that must be provided in order to be able to successfully perform the computations defined by the library.", 0, java.lang.Integer.MAX_VALUE, dataRequirement); 1488 case 951530617: /*content*/ return new Property("content", "Attachment", "The content of the library as an Attachment. The content may be a reference to a url, or may be directly embedded as a base-64 string. Either way, the contentType of the attachment determines how to interpret the content.", 0, java.lang.Integer.MAX_VALUE, content); 1489 default: return super.getNamedProperty(_hash, _name, _checkValid); 1490 } 1491 1492 } 1493 1494 @Override 1495 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1496 switch (hash) { 1497 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 1498 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1499 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 1500 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1501 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1502 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 1503 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 1504 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1505 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1506 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 1507 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 1508 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 1509 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // StringType 1510 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 1511 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 1512 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 1513 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 1514 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 1515 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 1516 case -1895276325: /*contributor*/ return this.contributor == null ? new Base[0] : this.contributor.toArray(new Base[this.contributor.size()]); // Contributor 1517 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 1518 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 1519 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 1520 case 1954460585: /*parameter*/ return this.parameter == null ? new Base[0] : this.parameter.toArray(new Base[this.parameter.size()]); // ParameterDefinition 1521 case 629147193: /*dataRequirement*/ return this.dataRequirement == null ? new Base[0] : this.dataRequirement.toArray(new Base[this.dataRequirement.size()]); // DataRequirement 1522 case 951530617: /*content*/ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // Attachment 1523 default: return super.getProperty(hash, name, checkValid); 1524 } 1525 1526 } 1527 1528 @Override 1529 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1530 switch (hash) { 1531 case 116079: // url 1532 this.url = castToUri(value); // UriType 1533 return value; 1534 case -1618432855: // identifier 1535 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1536 return value; 1537 case 351608024: // version 1538 this.version = castToString(value); // StringType 1539 return value; 1540 case 3373707: // name 1541 this.name = castToString(value); // StringType 1542 return value; 1543 case 110371416: // title 1544 this.title = castToString(value); // StringType 1545 return value; 1546 case -892481550: // status 1547 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1548 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1549 return value; 1550 case -404562712: // experimental 1551 this.experimental = castToBoolean(value); // BooleanType 1552 return value; 1553 case 3575610: // type 1554 this.type = castToCodeableConcept(value); // CodeableConcept 1555 return value; 1556 case 3076014: // date 1557 this.date = castToDateTime(value); // DateTimeType 1558 return value; 1559 case 1447404028: // publisher 1560 this.publisher = castToString(value); // StringType 1561 return value; 1562 case -1724546052: // description 1563 this.description = castToMarkdown(value); // MarkdownType 1564 return value; 1565 case -220463842: // purpose 1566 this.purpose = castToMarkdown(value); // MarkdownType 1567 return value; 1568 case 111574433: // usage 1569 this.usage = castToString(value); // StringType 1570 return value; 1571 case 223539345: // approvalDate 1572 this.approvalDate = castToDate(value); // DateType 1573 return value; 1574 case -1687512484: // lastReviewDate 1575 this.lastReviewDate = castToDate(value); // DateType 1576 return value; 1577 case -403934648: // effectivePeriod 1578 this.effectivePeriod = castToPeriod(value); // Period 1579 return value; 1580 case -669707736: // useContext 1581 this.getUseContext().add(castToUsageContext(value)); // UsageContext 1582 return value; 1583 case -507075711: // jurisdiction 1584 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 1585 return value; 1586 case 110546223: // topic 1587 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 1588 return value; 1589 case -1895276325: // contributor 1590 this.getContributor().add(castToContributor(value)); // Contributor 1591 return value; 1592 case 951526432: // contact 1593 this.getContact().add(castToContactDetail(value)); // ContactDetail 1594 return value; 1595 case 1522889671: // copyright 1596 this.copyright = castToMarkdown(value); // MarkdownType 1597 return value; 1598 case 666807069: // relatedArtifact 1599 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 1600 return value; 1601 case 1954460585: // parameter 1602 this.getParameter().add(castToParameterDefinition(value)); // ParameterDefinition 1603 return value; 1604 case 629147193: // dataRequirement 1605 this.getDataRequirement().add(castToDataRequirement(value)); // DataRequirement 1606 return value; 1607 case 951530617: // content 1608 this.getContent().add(castToAttachment(value)); // Attachment 1609 return value; 1610 default: return super.setProperty(hash, name, value); 1611 } 1612 1613 } 1614 1615 @Override 1616 public Base setProperty(String name, Base value) throws FHIRException { 1617 if (name.equals("url")) { 1618 this.url = castToUri(value); // UriType 1619 } else if (name.equals("identifier")) { 1620 this.getIdentifier().add(castToIdentifier(value)); 1621 } else if (name.equals("version")) { 1622 this.version = castToString(value); // StringType 1623 } else if (name.equals("name")) { 1624 this.name = castToString(value); // StringType 1625 } else if (name.equals("title")) { 1626 this.title = castToString(value); // StringType 1627 } else if (name.equals("status")) { 1628 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 1629 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 1630 } else if (name.equals("experimental")) { 1631 this.experimental = castToBoolean(value); // BooleanType 1632 } else if (name.equals("type")) { 1633 this.type = castToCodeableConcept(value); // CodeableConcept 1634 } else if (name.equals("date")) { 1635 this.date = castToDateTime(value); // DateTimeType 1636 } else if (name.equals("publisher")) { 1637 this.publisher = castToString(value); // StringType 1638 } else if (name.equals("description")) { 1639 this.description = castToMarkdown(value); // MarkdownType 1640 } else if (name.equals("purpose")) { 1641 this.purpose = castToMarkdown(value); // MarkdownType 1642 } else if (name.equals("usage")) { 1643 this.usage = castToString(value); // StringType 1644 } else if (name.equals("approvalDate")) { 1645 this.approvalDate = castToDate(value); // DateType 1646 } else if (name.equals("lastReviewDate")) { 1647 this.lastReviewDate = castToDate(value); // DateType 1648 } else if (name.equals("effectivePeriod")) { 1649 this.effectivePeriod = castToPeriod(value); // Period 1650 } else if (name.equals("useContext")) { 1651 this.getUseContext().add(castToUsageContext(value)); 1652 } else if (name.equals("jurisdiction")) { 1653 this.getJurisdiction().add(castToCodeableConcept(value)); 1654 } else if (name.equals("topic")) { 1655 this.getTopic().add(castToCodeableConcept(value)); 1656 } else if (name.equals("contributor")) { 1657 this.getContributor().add(castToContributor(value)); 1658 } else if (name.equals("contact")) { 1659 this.getContact().add(castToContactDetail(value)); 1660 } else if (name.equals("copyright")) { 1661 this.copyright = castToMarkdown(value); // MarkdownType 1662 } else if (name.equals("relatedArtifact")) { 1663 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 1664 } else if (name.equals("parameter")) { 1665 this.getParameter().add(castToParameterDefinition(value)); 1666 } else if (name.equals("dataRequirement")) { 1667 this.getDataRequirement().add(castToDataRequirement(value)); 1668 } else if (name.equals("content")) { 1669 this.getContent().add(castToAttachment(value)); 1670 } else 1671 return super.setProperty(name, value); 1672 return value; 1673 } 1674 1675 @Override 1676 public Base makeProperty(int hash, String name) throws FHIRException { 1677 switch (hash) { 1678 case 116079: return getUrlElement(); 1679 case -1618432855: return addIdentifier(); 1680 case 351608024: return getVersionElement(); 1681 case 3373707: return getNameElement(); 1682 case 110371416: return getTitleElement(); 1683 case -892481550: return getStatusElement(); 1684 case -404562712: return getExperimentalElement(); 1685 case 3575610: return getType(); 1686 case 3076014: return getDateElement(); 1687 case 1447404028: return getPublisherElement(); 1688 case -1724546052: return getDescriptionElement(); 1689 case -220463842: return getPurposeElement(); 1690 case 111574433: return getUsageElement(); 1691 case 223539345: return getApprovalDateElement(); 1692 case -1687512484: return getLastReviewDateElement(); 1693 case -403934648: return getEffectivePeriod(); 1694 case -669707736: return addUseContext(); 1695 case -507075711: return addJurisdiction(); 1696 case 110546223: return addTopic(); 1697 case -1895276325: return addContributor(); 1698 case 951526432: return addContact(); 1699 case 1522889671: return getCopyrightElement(); 1700 case 666807069: return addRelatedArtifact(); 1701 case 1954460585: return addParameter(); 1702 case 629147193: return addDataRequirement(); 1703 case 951530617: return addContent(); 1704 default: return super.makeProperty(hash, name); 1705 } 1706 1707 } 1708 1709 @Override 1710 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1711 switch (hash) { 1712 case 116079: /*url*/ return new String[] {"uri"}; 1713 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1714 case 351608024: /*version*/ return new String[] {"string"}; 1715 case 3373707: /*name*/ return new String[] {"string"}; 1716 case 110371416: /*title*/ return new String[] {"string"}; 1717 case -892481550: /*status*/ return new String[] {"code"}; 1718 case -404562712: /*experimental*/ return new String[] {"boolean"}; 1719 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1720 case 3076014: /*date*/ return new String[] {"dateTime"}; 1721 case 1447404028: /*publisher*/ return new String[] {"string"}; 1722 case -1724546052: /*description*/ return new String[] {"markdown"}; 1723 case -220463842: /*purpose*/ return new String[] {"markdown"}; 1724 case 111574433: /*usage*/ return new String[] {"string"}; 1725 case 223539345: /*approvalDate*/ return new String[] {"date"}; 1726 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 1727 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 1728 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 1729 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 1730 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 1731 case -1895276325: /*contributor*/ return new String[] {"Contributor"}; 1732 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 1733 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 1734 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 1735 case 1954460585: /*parameter*/ return new String[] {"ParameterDefinition"}; 1736 case 629147193: /*dataRequirement*/ return new String[] {"DataRequirement"}; 1737 case 951530617: /*content*/ return new String[] {"Attachment"}; 1738 default: return super.getTypesForProperty(hash, name); 1739 } 1740 1741 } 1742 1743 @Override 1744 public Base addChild(String name) throws FHIRException { 1745 if (name.equals("url")) { 1746 throw new FHIRException("Cannot call addChild on a singleton property Library.url"); 1747 } 1748 else if (name.equals("identifier")) { 1749 return addIdentifier(); 1750 } 1751 else if (name.equals("version")) { 1752 throw new FHIRException("Cannot call addChild on a singleton property Library.version"); 1753 } 1754 else if (name.equals("name")) { 1755 throw new FHIRException("Cannot call addChild on a singleton property Library.name"); 1756 } 1757 else if (name.equals("title")) { 1758 throw new FHIRException("Cannot call addChild on a singleton property Library.title"); 1759 } 1760 else if (name.equals("status")) { 1761 throw new FHIRException("Cannot call addChild on a singleton property Library.status"); 1762 } 1763 else if (name.equals("experimental")) { 1764 throw new FHIRException("Cannot call addChild on a singleton property Library.experimental"); 1765 } 1766 else if (name.equals("type")) { 1767 this.type = new CodeableConcept(); 1768 return this.type; 1769 } 1770 else if (name.equals("date")) { 1771 throw new FHIRException("Cannot call addChild on a singleton property Library.date"); 1772 } 1773 else if (name.equals("publisher")) { 1774 throw new FHIRException("Cannot call addChild on a singleton property Library.publisher"); 1775 } 1776 else if (name.equals("description")) { 1777 throw new FHIRException("Cannot call addChild on a singleton property Library.description"); 1778 } 1779 else if (name.equals("purpose")) { 1780 throw new FHIRException("Cannot call addChild on a singleton property Library.purpose"); 1781 } 1782 else if (name.equals("usage")) { 1783 throw new FHIRException("Cannot call addChild on a singleton property Library.usage"); 1784 } 1785 else if (name.equals("approvalDate")) { 1786 throw new FHIRException("Cannot call addChild on a singleton property Library.approvalDate"); 1787 } 1788 else if (name.equals("lastReviewDate")) { 1789 throw new FHIRException("Cannot call addChild on a singleton property Library.lastReviewDate"); 1790 } 1791 else if (name.equals("effectivePeriod")) { 1792 this.effectivePeriod = new Period(); 1793 return this.effectivePeriod; 1794 } 1795 else if (name.equals("useContext")) { 1796 return addUseContext(); 1797 } 1798 else if (name.equals("jurisdiction")) { 1799 return addJurisdiction(); 1800 } 1801 else if (name.equals("topic")) { 1802 return addTopic(); 1803 } 1804 else if (name.equals("contributor")) { 1805 return addContributor(); 1806 } 1807 else if (name.equals("contact")) { 1808 return addContact(); 1809 } 1810 else if (name.equals("copyright")) { 1811 throw new FHIRException("Cannot call addChild on a singleton property Library.copyright"); 1812 } 1813 else if (name.equals("relatedArtifact")) { 1814 return addRelatedArtifact(); 1815 } 1816 else if (name.equals("parameter")) { 1817 return addParameter(); 1818 } 1819 else if (name.equals("dataRequirement")) { 1820 return addDataRequirement(); 1821 } 1822 else if (name.equals("content")) { 1823 return addContent(); 1824 } 1825 else 1826 return super.addChild(name); 1827 } 1828 1829 public String fhirType() { 1830 return "Library"; 1831 1832 } 1833 1834 public Library copy() { 1835 Library dst = new Library(); 1836 copyValues(dst); 1837 dst.url = url == null ? null : url.copy(); 1838 if (identifier != null) { 1839 dst.identifier = new ArrayList<Identifier>(); 1840 for (Identifier i : identifier) 1841 dst.identifier.add(i.copy()); 1842 }; 1843 dst.version = version == null ? null : version.copy(); 1844 dst.name = name == null ? null : name.copy(); 1845 dst.title = title == null ? null : title.copy(); 1846 dst.status = status == null ? null : status.copy(); 1847 dst.experimental = experimental == null ? null : experimental.copy(); 1848 dst.type = type == null ? null : type.copy(); 1849 dst.date = date == null ? null : date.copy(); 1850 dst.publisher = publisher == null ? null : publisher.copy(); 1851 dst.description = description == null ? null : description.copy(); 1852 dst.purpose = purpose == null ? null : purpose.copy(); 1853 dst.usage = usage == null ? null : usage.copy(); 1854 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 1855 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 1856 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 1857 if (useContext != null) { 1858 dst.useContext = new ArrayList<UsageContext>(); 1859 for (UsageContext i : useContext) 1860 dst.useContext.add(i.copy()); 1861 }; 1862 if (jurisdiction != null) { 1863 dst.jurisdiction = new ArrayList<CodeableConcept>(); 1864 for (CodeableConcept i : jurisdiction) 1865 dst.jurisdiction.add(i.copy()); 1866 }; 1867 if (topic != null) { 1868 dst.topic = new ArrayList<CodeableConcept>(); 1869 for (CodeableConcept i : topic) 1870 dst.topic.add(i.copy()); 1871 }; 1872 if (contributor != null) { 1873 dst.contributor = new ArrayList<Contributor>(); 1874 for (Contributor i : contributor) 1875 dst.contributor.add(i.copy()); 1876 }; 1877 if (contact != null) { 1878 dst.contact = new ArrayList<ContactDetail>(); 1879 for (ContactDetail i : contact) 1880 dst.contact.add(i.copy()); 1881 }; 1882 dst.copyright = copyright == null ? null : copyright.copy(); 1883 if (relatedArtifact != null) { 1884 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 1885 for (RelatedArtifact i : relatedArtifact) 1886 dst.relatedArtifact.add(i.copy()); 1887 }; 1888 if (parameter != null) { 1889 dst.parameter = new ArrayList<ParameterDefinition>(); 1890 for (ParameterDefinition i : parameter) 1891 dst.parameter.add(i.copy()); 1892 }; 1893 if (dataRequirement != null) { 1894 dst.dataRequirement = new ArrayList<DataRequirement>(); 1895 for (DataRequirement i : dataRequirement) 1896 dst.dataRequirement.add(i.copy()); 1897 }; 1898 if (content != null) { 1899 dst.content = new ArrayList<Attachment>(); 1900 for (Attachment i : content) 1901 dst.content.add(i.copy()); 1902 }; 1903 return dst; 1904 } 1905 1906 protected Library typedCopy() { 1907 return copy(); 1908 } 1909 1910 @Override 1911 public boolean equalsDeep(Base other_) { 1912 if (!super.equalsDeep(other_)) 1913 return false; 1914 if (!(other_ instanceof Library)) 1915 return false; 1916 Library o = (Library) other_; 1917 return compareDeep(identifier, o.identifier, true) && compareDeep(type, o.type, true) && compareDeep(purpose, o.purpose, true) 1918 && compareDeep(usage, o.usage, true) && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 1919 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(contributor, o.contributor, true) 1920 && compareDeep(copyright, o.copyright, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 1921 && compareDeep(parameter, o.parameter, true) && compareDeep(dataRequirement, o.dataRequirement, true) 1922 && compareDeep(content, o.content, true); 1923 } 1924 1925 @Override 1926 public boolean equalsShallow(Base other_) { 1927 if (!super.equalsShallow(other_)) 1928 return false; 1929 if (!(other_ instanceof Library)) 1930 return false; 1931 Library o = (Library) other_; 1932 return compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) && compareValues(approvalDate, o.approvalDate, true) 1933 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(copyright, o.copyright, true) 1934 ; 1935 } 1936 1937 public boolean isEmpty() { 1938 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, type, purpose 1939 , usage, approvalDate, lastReviewDate, effectivePeriod, topic, contributor, copyright 1940 , relatedArtifact, parameter, dataRequirement, content); 1941 } 1942 1943 @Override 1944 public ResourceType getResourceType() { 1945 return ResourceType.Library; 1946 } 1947 1948 /** 1949 * Search parameter: <b>date</b> 1950 * <p> 1951 * Description: <b>The library publication date</b><br> 1952 * Type: <b>date</b><br> 1953 * Path: <b>Library.date</b><br> 1954 * </p> 1955 */ 1956 @SearchParamDefinition(name="date", path="Library.date", description="The library publication date", type="date" ) 1957 public static final String SP_DATE = "date"; 1958 /** 1959 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1960 * <p> 1961 * Description: <b>The library publication date</b><br> 1962 * Type: <b>date</b><br> 1963 * Path: <b>Library.date</b><br> 1964 * </p> 1965 */ 1966 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1967 1968 /** 1969 * Search parameter: <b>identifier</b> 1970 * <p> 1971 * Description: <b>External identifier for the library</b><br> 1972 * Type: <b>token</b><br> 1973 * Path: <b>Library.identifier</b><br> 1974 * </p> 1975 */ 1976 @SearchParamDefinition(name="identifier", path="Library.identifier", description="External identifier for the library", type="token" ) 1977 public static final String SP_IDENTIFIER = "identifier"; 1978 /** 1979 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1980 * <p> 1981 * Description: <b>External identifier for the library</b><br> 1982 * Type: <b>token</b><br> 1983 * Path: <b>Library.identifier</b><br> 1984 * </p> 1985 */ 1986 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1987 1988 /** 1989 * Search parameter: <b>successor</b> 1990 * <p> 1991 * Description: <b>What resource is being referenced</b><br> 1992 * Type: <b>reference</b><br> 1993 * Path: <b>Library.relatedArtifact.resource</b><br> 1994 * </p> 1995 */ 1996 @SearchParamDefinition(name="successor", path="Library.relatedArtifact.where(type='successor').resource", description="What resource is being referenced", type="reference" ) 1997 public static final String SP_SUCCESSOR = "successor"; 1998 /** 1999 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 2000 * <p> 2001 * Description: <b>What resource is being referenced</b><br> 2002 * Type: <b>reference</b><br> 2003 * Path: <b>Library.relatedArtifact.resource</b><br> 2004 * </p> 2005 */ 2006 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 2007 2008/** 2009 * Constant for fluent queries to be used to add include statements. Specifies 2010 * the path value of "<b>Library:successor</b>". 2011 */ 2012 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("Library:successor").toLocked(); 2013 2014 /** 2015 * Search parameter: <b>jurisdiction</b> 2016 * <p> 2017 * Description: <b>Intended jurisdiction for the library</b><br> 2018 * Type: <b>token</b><br> 2019 * Path: <b>Library.jurisdiction</b><br> 2020 * </p> 2021 */ 2022 @SearchParamDefinition(name="jurisdiction", path="Library.jurisdiction", description="Intended jurisdiction for the library", type="token" ) 2023 public static final String SP_JURISDICTION = "jurisdiction"; 2024 /** 2025 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2026 * <p> 2027 * Description: <b>Intended jurisdiction for the library</b><br> 2028 * Type: <b>token</b><br> 2029 * Path: <b>Library.jurisdiction</b><br> 2030 * </p> 2031 */ 2032 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 2033 2034 /** 2035 * Search parameter: <b>description</b> 2036 * <p> 2037 * Description: <b>The description of the library</b><br> 2038 * Type: <b>string</b><br> 2039 * Path: <b>Library.description</b><br> 2040 * </p> 2041 */ 2042 @SearchParamDefinition(name="description", path="Library.description", description="The description of the library", type="string" ) 2043 public static final String SP_DESCRIPTION = "description"; 2044 /** 2045 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2046 * <p> 2047 * Description: <b>The description of the library</b><br> 2048 * Type: <b>string</b><br> 2049 * Path: <b>Library.description</b><br> 2050 * </p> 2051 */ 2052 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 2053 2054 /** 2055 * Search parameter: <b>derived-from</b> 2056 * <p> 2057 * Description: <b>What resource is being referenced</b><br> 2058 * Type: <b>reference</b><br> 2059 * Path: <b>Library.relatedArtifact.resource</b><br> 2060 * </p> 2061 */ 2062 @SearchParamDefinition(name="derived-from", path="Library.relatedArtifact.where(type='derived-from').resource", description="What resource is being referenced", type="reference" ) 2063 public static final String SP_DERIVED_FROM = "derived-from"; 2064 /** 2065 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 2066 * <p> 2067 * Description: <b>What resource is being referenced</b><br> 2068 * Type: <b>reference</b><br> 2069 * Path: <b>Library.relatedArtifact.resource</b><br> 2070 * </p> 2071 */ 2072 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 2073 2074/** 2075 * Constant for fluent queries to be used to add include statements. Specifies 2076 * the path value of "<b>Library:derived-from</b>". 2077 */ 2078 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("Library:derived-from").toLocked(); 2079 2080 /** 2081 * Search parameter: <b>predecessor</b> 2082 * <p> 2083 * Description: <b>What resource is being referenced</b><br> 2084 * Type: <b>reference</b><br> 2085 * Path: <b>Library.relatedArtifact.resource</b><br> 2086 * </p> 2087 */ 2088 @SearchParamDefinition(name="predecessor", path="Library.relatedArtifact.where(type='predecessor').resource", description="What resource is being referenced", type="reference" ) 2089 public static final String SP_PREDECESSOR = "predecessor"; 2090 /** 2091 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 2092 * <p> 2093 * Description: <b>What resource is being referenced</b><br> 2094 * Type: <b>reference</b><br> 2095 * Path: <b>Library.relatedArtifact.resource</b><br> 2096 * </p> 2097 */ 2098 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 2099 2100/** 2101 * Constant for fluent queries to be used to add include statements. Specifies 2102 * the path value of "<b>Library:predecessor</b>". 2103 */ 2104 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("Library:predecessor").toLocked(); 2105 2106 /** 2107 * Search parameter: <b>title</b> 2108 * <p> 2109 * Description: <b>The human-friendly name of the library</b><br> 2110 * Type: <b>string</b><br> 2111 * Path: <b>Library.title</b><br> 2112 * </p> 2113 */ 2114 @SearchParamDefinition(name="title", path="Library.title", description="The human-friendly name of the library", type="string" ) 2115 public static final String SP_TITLE = "title"; 2116 /** 2117 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2118 * <p> 2119 * Description: <b>The human-friendly name of the library</b><br> 2120 * Type: <b>string</b><br> 2121 * Path: <b>Library.title</b><br> 2122 * </p> 2123 */ 2124 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 2125 2126 /** 2127 * Search parameter: <b>composed-of</b> 2128 * <p> 2129 * Description: <b>What resource is being referenced</b><br> 2130 * Type: <b>reference</b><br> 2131 * Path: <b>Library.relatedArtifact.resource</b><br> 2132 * </p> 2133 */ 2134 @SearchParamDefinition(name="composed-of", path="Library.relatedArtifact.where(type='composed-of').resource", description="What resource is being referenced", type="reference" ) 2135 public static final String SP_COMPOSED_OF = "composed-of"; 2136 /** 2137 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 2138 * <p> 2139 * Description: <b>What resource is being referenced</b><br> 2140 * Type: <b>reference</b><br> 2141 * Path: <b>Library.relatedArtifact.resource</b><br> 2142 * </p> 2143 */ 2144 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 2145 2146/** 2147 * Constant for fluent queries to be used to add include statements. Specifies 2148 * the path value of "<b>Library:composed-of</b>". 2149 */ 2150 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("Library:composed-of").toLocked(); 2151 2152 /** 2153 * Search parameter: <b>version</b> 2154 * <p> 2155 * Description: <b>The business version of the library</b><br> 2156 * Type: <b>token</b><br> 2157 * Path: <b>Library.version</b><br> 2158 * </p> 2159 */ 2160 @SearchParamDefinition(name="version", path="Library.version", description="The business version of the library", type="token" ) 2161 public static final String SP_VERSION = "version"; 2162 /** 2163 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2164 * <p> 2165 * Description: <b>The business version of the library</b><br> 2166 * Type: <b>token</b><br> 2167 * Path: <b>Library.version</b><br> 2168 * </p> 2169 */ 2170 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 2171 2172 /** 2173 * Search parameter: <b>url</b> 2174 * <p> 2175 * Description: <b>The uri that identifies the library</b><br> 2176 * Type: <b>uri</b><br> 2177 * Path: <b>Library.url</b><br> 2178 * </p> 2179 */ 2180 @SearchParamDefinition(name="url", path="Library.url", description="The uri that identifies the library", type="uri" ) 2181 public static final String SP_URL = "url"; 2182 /** 2183 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2184 * <p> 2185 * Description: <b>The uri that identifies the library</b><br> 2186 * Type: <b>uri</b><br> 2187 * Path: <b>Library.url</b><br> 2188 * </p> 2189 */ 2190 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2191 2192 /** 2193 * Search parameter: <b>effective</b> 2194 * <p> 2195 * Description: <b>The time during which the library is intended to be in use</b><br> 2196 * Type: <b>date</b><br> 2197 * Path: <b>Library.effectivePeriod</b><br> 2198 * </p> 2199 */ 2200 @SearchParamDefinition(name="effective", path="Library.effectivePeriod", description="The time during which the library is intended to be in use", type="date" ) 2201 public static final String SP_EFFECTIVE = "effective"; 2202 /** 2203 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 2204 * <p> 2205 * Description: <b>The time during which the library is intended to be in use</b><br> 2206 * Type: <b>date</b><br> 2207 * Path: <b>Library.effectivePeriod</b><br> 2208 * </p> 2209 */ 2210 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 2211 2212 /** 2213 * Search parameter: <b>depends-on</b> 2214 * <p> 2215 * Description: <b>What resource is being referenced</b><br> 2216 * Type: <b>reference</b><br> 2217 * Path: <b>Library.relatedArtifact.resource</b><br> 2218 * </p> 2219 */ 2220 @SearchParamDefinition(name="depends-on", path="Library.relatedArtifact.where(type='depends-on').resource", description="What resource is being referenced", type="reference" ) 2221 public static final String SP_DEPENDS_ON = "depends-on"; 2222 /** 2223 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 2224 * <p> 2225 * Description: <b>What resource is being referenced</b><br> 2226 * Type: <b>reference</b><br> 2227 * Path: <b>Library.relatedArtifact.resource</b><br> 2228 * </p> 2229 */ 2230 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 2231 2232/** 2233 * Constant for fluent queries to be used to add include statements. Specifies 2234 * the path value of "<b>Library:depends-on</b>". 2235 */ 2236 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("Library:depends-on").toLocked(); 2237 2238 /** 2239 * Search parameter: <b>name</b> 2240 * <p> 2241 * Description: <b>Computationally friendly name of the library</b><br> 2242 * Type: <b>string</b><br> 2243 * Path: <b>Library.name</b><br> 2244 * </p> 2245 */ 2246 @SearchParamDefinition(name="name", path="Library.name", description="Computationally friendly name of the library", type="string" ) 2247 public static final String SP_NAME = "name"; 2248 /** 2249 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2250 * <p> 2251 * Description: <b>Computationally friendly name of the library</b><br> 2252 * Type: <b>string</b><br> 2253 * Path: <b>Library.name</b><br> 2254 * </p> 2255 */ 2256 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2257 2258 /** 2259 * Search parameter: <b>publisher</b> 2260 * <p> 2261 * Description: <b>Name of the publisher of the library</b><br> 2262 * Type: <b>string</b><br> 2263 * Path: <b>Library.publisher</b><br> 2264 * </p> 2265 */ 2266 @SearchParamDefinition(name="publisher", path="Library.publisher", description="Name of the publisher of the library", type="string" ) 2267 public static final String SP_PUBLISHER = "publisher"; 2268 /** 2269 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2270 * <p> 2271 * Description: <b>Name of the publisher of the library</b><br> 2272 * Type: <b>string</b><br> 2273 * Path: <b>Library.publisher</b><br> 2274 * </p> 2275 */ 2276 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2277 2278 /** 2279 * Search parameter: <b>topic</b> 2280 * <p> 2281 * Description: <b>Topics associated with the module</b><br> 2282 * Type: <b>token</b><br> 2283 * Path: <b>Library.topic</b><br> 2284 * </p> 2285 */ 2286 @SearchParamDefinition(name="topic", path="Library.topic", description="Topics associated with the module", type="token" ) 2287 public static final String SP_TOPIC = "topic"; 2288 /** 2289 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 2290 * <p> 2291 * Description: <b>Topics associated with the module</b><br> 2292 * Type: <b>token</b><br> 2293 * Path: <b>Library.topic</b><br> 2294 * </p> 2295 */ 2296 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 2297 2298 /** 2299 * Search parameter: <b>status</b> 2300 * <p> 2301 * Description: <b>The current status of the library</b><br> 2302 * Type: <b>token</b><br> 2303 * Path: <b>Library.status</b><br> 2304 * </p> 2305 */ 2306 @SearchParamDefinition(name="status", path="Library.status", description="The current status of the library", type="token" ) 2307 public static final String SP_STATUS = "status"; 2308 /** 2309 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2310 * <p> 2311 * Description: <b>The current status of the library</b><br> 2312 * Type: <b>token</b><br> 2313 * Path: <b>Library.status</b><br> 2314 * </p> 2315 */ 2316 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2317 2318 2319}