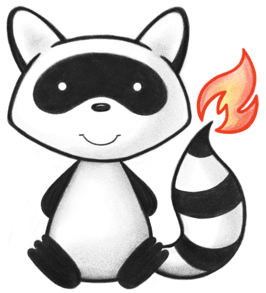
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041 042import ca.uhn.fhir.model.api.annotation.Block; 043import ca.uhn.fhir.model.api.annotation.Child; 044import ca.uhn.fhir.model.api.annotation.Description; 045import ca.uhn.fhir.model.api.annotation.ResourceDef; 046import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 047/** 048 * Identifies two or more records (resource instances) that are referring to the same real-world "occurrence". 049 */ 050@ResourceDef(name="Linkage", profile="http://hl7.org/fhir/Profile/Linkage") 051public class Linkage extends DomainResource { 052 053 public enum LinkageType { 054 /** 055 * The record represents the "source of truth" (from the perspective of this Linkage resource) for the underlying event/condition/etc. 056 */ 057 SOURCE, 058 /** 059 * The record represents the alternative view of the underlying event/condition/etc. The record may still be actively maintained, even though it is not considered to be the source of truth. 060 */ 061 ALTERNATE, 062 /** 063 * The record represents an obsolete record of the underlyng event/condition/etc. It is not expected to be actively maintained. 064 */ 065 HISTORICAL, 066 /** 067 * added to help the parsers with the generic types 068 */ 069 NULL; 070 public static LinkageType fromCode(String codeString) throws FHIRException { 071 if (codeString == null || "".equals(codeString)) 072 return null; 073 if ("source".equals(codeString)) 074 return SOURCE; 075 if ("alternate".equals(codeString)) 076 return ALTERNATE; 077 if ("historical".equals(codeString)) 078 return HISTORICAL; 079 if (Configuration.isAcceptInvalidEnums()) 080 return null; 081 else 082 throw new FHIRException("Unknown LinkageType code '"+codeString+"'"); 083 } 084 public String toCode() { 085 switch (this) { 086 case SOURCE: return "source"; 087 case ALTERNATE: return "alternate"; 088 case HISTORICAL: return "historical"; 089 case NULL: return null; 090 default: return "?"; 091 } 092 } 093 public String getSystem() { 094 switch (this) { 095 case SOURCE: return "http://hl7.org/fhir/linkage-type"; 096 case ALTERNATE: return "http://hl7.org/fhir/linkage-type"; 097 case HISTORICAL: return "http://hl7.org/fhir/linkage-type"; 098 case NULL: return null; 099 default: return "?"; 100 } 101 } 102 public String getDefinition() { 103 switch (this) { 104 case SOURCE: return "The record represents the \"source of truth\" (from the perspective of this Linkage resource) for the underlying event/condition/etc."; 105 case ALTERNATE: return "The record represents the alternative view of the underlying event/condition/etc. The record may still be actively maintained, even though it is not considered to be the source of truth."; 106 case HISTORICAL: return "The record represents an obsolete record of the underlyng event/condition/etc. It is not expected to be actively maintained."; 107 case NULL: return null; 108 default: return "?"; 109 } 110 } 111 public String getDisplay() { 112 switch (this) { 113 case SOURCE: return "Source of truth"; 114 case ALTERNATE: return "Alternate record"; 115 case HISTORICAL: return "Historical/obsolete record"; 116 case NULL: return null; 117 default: return "?"; 118 } 119 } 120 } 121 122 public static class LinkageTypeEnumFactory implements EnumFactory<LinkageType> { 123 public LinkageType fromCode(String codeString) throws IllegalArgumentException { 124 if (codeString == null || "".equals(codeString)) 125 if (codeString == null || "".equals(codeString)) 126 return null; 127 if ("source".equals(codeString)) 128 return LinkageType.SOURCE; 129 if ("alternate".equals(codeString)) 130 return LinkageType.ALTERNATE; 131 if ("historical".equals(codeString)) 132 return LinkageType.HISTORICAL; 133 throw new IllegalArgumentException("Unknown LinkageType code '"+codeString+"'"); 134 } 135 public Enumeration<LinkageType> fromType(PrimitiveType<?> code) throws FHIRException { 136 if (code == null) 137 return null; 138 if (code.isEmpty()) 139 return new Enumeration<LinkageType>(this); 140 String codeString = code.asStringValue(); 141 if (codeString == null || "".equals(codeString)) 142 return null; 143 if ("source".equals(codeString)) 144 return new Enumeration<LinkageType>(this, LinkageType.SOURCE); 145 if ("alternate".equals(codeString)) 146 return new Enumeration<LinkageType>(this, LinkageType.ALTERNATE); 147 if ("historical".equals(codeString)) 148 return new Enumeration<LinkageType>(this, LinkageType.HISTORICAL); 149 throw new FHIRException("Unknown LinkageType code '"+codeString+"'"); 150 } 151 public String toCode(LinkageType code) { 152 if (code == LinkageType.NULL) 153 return null; 154 if (code == LinkageType.SOURCE) 155 return "source"; 156 if (code == LinkageType.ALTERNATE) 157 return "alternate"; 158 if (code == LinkageType.HISTORICAL) 159 return "historical"; 160 return "?"; 161 } 162 public String toSystem(LinkageType code) { 163 return code.getSystem(); 164 } 165 } 166 167 @Block() 168 public static class LinkageItemComponent extends BackboneElement implements IBaseBackboneElement { 169 /** 170 * Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 171 */ 172 @Child(name = "type", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 173 @Description(shortDefinition="source | alternate | historical", formalDefinition="Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations." ) 174 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/linkage-type") 175 protected Enumeration<LinkageType> type; 176 177 /** 178 * The resource instance being linked as part of the group. 179 */ 180 @Child(name = "resource", type = {Reference.class}, order=2, min=1, max=1, modifier=false, summary=true) 181 @Description(shortDefinition="Resource being linked", formalDefinition="The resource instance being linked as part of the group." ) 182 protected Reference resource; 183 184 private static final long serialVersionUID = 527428511L; 185 186 /** 187 * Constructor 188 */ 189 public LinkageItemComponent() { 190 super(); 191 } 192 193 /** 194 * Constructor 195 */ 196 public LinkageItemComponent(Enumeration<LinkageType> type, Reference resource) { 197 super(); 198 this.type = type; 199 this.resource = resource; 200 } 201 202 /** 203 * @return {@link #type} (Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 204 */ 205 public Enumeration<LinkageType> getTypeElement() { 206 if (this.type == null) 207 if (Configuration.errorOnAutoCreate()) 208 throw new Error("Attempt to auto-create LinkageItemComponent.type"); 209 else if (Configuration.doAutoCreate()) 210 this.type = new Enumeration<LinkageType>(new LinkageTypeEnumFactory()); // bb 211 return this.type; 212 } 213 214 public boolean hasTypeElement() { 215 return this.type != null && !this.type.isEmpty(); 216 } 217 218 public boolean hasType() { 219 return this.type != null && !this.type.isEmpty(); 220 } 221 222 /** 223 * @param value {@link #type} (Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 224 */ 225 public LinkageItemComponent setTypeElement(Enumeration<LinkageType> value) { 226 this.type = value; 227 return this; 228 } 229 230 /** 231 * @return Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 232 */ 233 public LinkageType getType() { 234 return this.type == null ? null : this.type.getValue(); 235 } 236 237 /** 238 * @param value Distinguishes which item is "source of truth" (if any) and which items are no longer considered to be current representations. 239 */ 240 public LinkageItemComponent setType(LinkageType value) { 241 if (this.type == null) 242 this.type = new Enumeration<LinkageType>(new LinkageTypeEnumFactory()); 243 this.type.setValue(value); 244 return this; 245 } 246 247 /** 248 * @return {@link #resource} (The resource instance being linked as part of the group.) 249 */ 250 public Reference getResource() { 251 if (this.resource == null) 252 if (Configuration.errorOnAutoCreate()) 253 throw new Error("Attempt to auto-create LinkageItemComponent.resource"); 254 else if (Configuration.doAutoCreate()) 255 this.resource = new Reference(); // cc 256 return this.resource; 257 } 258 259 public boolean hasResource() { 260 return this.resource != null && !this.resource.isEmpty(); 261 } 262 263 /** 264 * @param value {@link #resource} (The resource instance being linked as part of the group.) 265 */ 266 public LinkageItemComponent setResource(Reference value) { 267 this.resource = value; 268 return this; 269 } 270 271 protected void listChildren(List<Property> children) { 272 super.listChildren(children); 273 children.add(new Property("type", "code", "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.", 0, 1, type)); 274 children.add(new Property("resource", "Reference", "The resource instance being linked as part of the group.", 0, 1, resource)); 275 } 276 277 @Override 278 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 279 switch (_hash) { 280 case 3575610: /*type*/ return new Property("type", "code", "Distinguishes which item is \"source of truth\" (if any) and which items are no longer considered to be current representations.", 0, 1, type); 281 case -341064690: /*resource*/ return new Property("resource", "Reference", "The resource instance being linked as part of the group.", 0, 1, resource); 282 default: return super.getNamedProperty(_hash, _name, _checkValid); 283 } 284 285 } 286 287 @Override 288 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 289 switch (hash) { 290 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<LinkageType> 291 case -341064690: /*resource*/ return this.resource == null ? new Base[0] : new Base[] {this.resource}; // Reference 292 default: return super.getProperty(hash, name, checkValid); 293 } 294 295 } 296 297 @Override 298 public Base setProperty(int hash, String name, Base value) throws FHIRException { 299 switch (hash) { 300 case 3575610: // type 301 value = new LinkageTypeEnumFactory().fromType(castToCode(value)); 302 this.type = (Enumeration) value; // Enumeration<LinkageType> 303 return value; 304 case -341064690: // resource 305 this.resource = castToReference(value); // Reference 306 return value; 307 default: return super.setProperty(hash, name, value); 308 } 309 310 } 311 312 @Override 313 public Base setProperty(String name, Base value) throws FHIRException { 314 if (name.equals("type")) { 315 value = new LinkageTypeEnumFactory().fromType(castToCode(value)); 316 this.type = (Enumeration) value; // Enumeration<LinkageType> 317 } else if (name.equals("resource")) { 318 this.resource = castToReference(value); // Reference 319 } else 320 return super.setProperty(name, value); 321 return value; 322 } 323 324 @Override 325 public Base makeProperty(int hash, String name) throws FHIRException { 326 switch (hash) { 327 case 3575610: return getTypeElement(); 328 case -341064690: return getResource(); 329 default: return super.makeProperty(hash, name); 330 } 331 332 } 333 334 @Override 335 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 336 switch (hash) { 337 case 3575610: /*type*/ return new String[] {"code"}; 338 case -341064690: /*resource*/ return new String[] {"Reference"}; 339 default: return super.getTypesForProperty(hash, name); 340 } 341 342 } 343 344 @Override 345 public Base addChild(String name) throws FHIRException { 346 if (name.equals("type")) { 347 throw new FHIRException("Cannot call addChild on a singleton property Linkage.type"); 348 } 349 else if (name.equals("resource")) { 350 this.resource = new Reference(); 351 return this.resource; 352 } 353 else 354 return super.addChild(name); 355 } 356 357 public LinkageItemComponent copy() { 358 LinkageItemComponent dst = new LinkageItemComponent(); 359 copyValues(dst); 360 dst.type = type == null ? null : type.copy(); 361 dst.resource = resource == null ? null : resource.copy(); 362 return dst; 363 } 364 365 @Override 366 public boolean equalsDeep(Base other_) { 367 if (!super.equalsDeep(other_)) 368 return false; 369 if (!(other_ instanceof LinkageItemComponent)) 370 return false; 371 LinkageItemComponent o = (LinkageItemComponent) other_; 372 return compareDeep(type, o.type, true) && compareDeep(resource, o.resource, true); 373 } 374 375 @Override 376 public boolean equalsShallow(Base other_) { 377 if (!super.equalsShallow(other_)) 378 return false; 379 if (!(other_ instanceof LinkageItemComponent)) 380 return false; 381 LinkageItemComponent o = (LinkageItemComponent) other_; 382 return compareValues(type, o.type, true); 383 } 384 385 public boolean isEmpty() { 386 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(type, resource); 387 } 388 389 public String fhirType() { 390 return "Linkage.item"; 391 392 } 393 394 } 395 396 /** 397 * Indicates whether the asserted set of linkages are considered to be "in effect". 398 */ 399 @Child(name = "active", type = {BooleanType.class}, order=0, min=0, max=1, modifier=false, summary=true) 400 @Description(shortDefinition="Whether this linkage assertion is active or not", formalDefinition="Indicates whether the asserted set of linkages are considered to be \"in effect\"." ) 401 protected BooleanType active; 402 403 /** 404 * Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage. 405 */ 406 @Child(name = "author", type = {Practitioner.class, Organization.class}, order=1, min=0, max=1, modifier=false, summary=true) 407 @Description(shortDefinition="Who is responsible for linkages", formalDefinition="Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage." ) 408 protected Reference author; 409 410 /** 411 * The actual object that is the target of the reference (Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage.) 412 */ 413 protected Resource authorTarget; 414 415 /** 416 * Identifies one of the records that is considered to refer to the same real-world occurrence as well as how the items hould be evaluated within the collection of linked items. 417 */ 418 @Child(name = "item", type = {}, order=2, min=1, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 419 @Description(shortDefinition="Item to be linked", formalDefinition="Identifies one of the records that is considered to refer to the same real-world occurrence as well as how the items hould be evaluated within the collection of linked items." ) 420 protected List<LinkageItemComponent> item; 421 422 private static final long serialVersionUID = 25900306L; 423 424 /** 425 * Constructor 426 */ 427 public Linkage() { 428 super(); 429 } 430 431 /** 432 * @return {@link #active} (Indicates whether the asserted set of linkages are considered to be "in effect".). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 433 */ 434 public BooleanType getActiveElement() { 435 if (this.active == null) 436 if (Configuration.errorOnAutoCreate()) 437 throw new Error("Attempt to auto-create Linkage.active"); 438 else if (Configuration.doAutoCreate()) 439 this.active = new BooleanType(); // bb 440 return this.active; 441 } 442 443 public boolean hasActiveElement() { 444 return this.active != null && !this.active.isEmpty(); 445 } 446 447 public boolean hasActive() { 448 return this.active != null && !this.active.isEmpty(); 449 } 450 451 /** 452 * @param value {@link #active} (Indicates whether the asserted set of linkages are considered to be "in effect".). This is the underlying object with id, value and extensions. The accessor "getActive" gives direct access to the value 453 */ 454 public Linkage setActiveElement(BooleanType value) { 455 this.active = value; 456 return this; 457 } 458 459 /** 460 * @return Indicates whether the asserted set of linkages are considered to be "in effect". 461 */ 462 public boolean getActive() { 463 return this.active == null || this.active.isEmpty() ? false : this.active.getValue(); 464 } 465 466 /** 467 * @param value Indicates whether the asserted set of linkages are considered to be "in effect". 468 */ 469 public Linkage setActive(boolean value) { 470 if (this.active == null) 471 this.active = new BooleanType(); 472 this.active.setValue(value); 473 return this; 474 } 475 476 /** 477 * @return {@link #author} (Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage.) 478 */ 479 public Reference getAuthor() { 480 if (this.author == null) 481 if (Configuration.errorOnAutoCreate()) 482 throw new Error("Attempt to auto-create Linkage.author"); 483 else if (Configuration.doAutoCreate()) 484 this.author = new Reference(); // cc 485 return this.author; 486 } 487 488 public boolean hasAuthor() { 489 return this.author != null && !this.author.isEmpty(); 490 } 491 492 /** 493 * @param value {@link #author} (Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage.) 494 */ 495 public Linkage setAuthor(Reference value) { 496 this.author = value; 497 return this; 498 } 499 500 /** 501 * @return {@link #author} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage.) 502 */ 503 public Resource getAuthorTarget() { 504 return this.authorTarget; 505 } 506 507 /** 508 * @param value {@link #author} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage.) 509 */ 510 public Linkage setAuthorTarget(Resource value) { 511 this.authorTarget = value; 512 return this; 513 } 514 515 /** 516 * @return {@link #item} (Identifies one of the records that is considered to refer to the same real-world occurrence as well as how the items hould be evaluated within the collection of linked items.) 517 */ 518 public List<LinkageItemComponent> getItem() { 519 if (this.item == null) 520 this.item = new ArrayList<LinkageItemComponent>(); 521 return this.item; 522 } 523 524 /** 525 * @return Returns a reference to <code>this</code> for easy method chaining 526 */ 527 public Linkage setItem(List<LinkageItemComponent> theItem) { 528 this.item = theItem; 529 return this; 530 } 531 532 public boolean hasItem() { 533 if (this.item == null) 534 return false; 535 for (LinkageItemComponent item : this.item) 536 if (!item.isEmpty()) 537 return true; 538 return false; 539 } 540 541 public LinkageItemComponent addItem() { //3 542 LinkageItemComponent t = new LinkageItemComponent(); 543 if (this.item == null) 544 this.item = new ArrayList<LinkageItemComponent>(); 545 this.item.add(t); 546 return t; 547 } 548 549 public Linkage addItem(LinkageItemComponent t) { //3 550 if (t == null) 551 return this; 552 if (this.item == null) 553 this.item = new ArrayList<LinkageItemComponent>(); 554 this.item.add(t); 555 return this; 556 } 557 558 /** 559 * @return The first repetition of repeating field {@link #item}, creating it if it does not already exist 560 */ 561 public LinkageItemComponent getItemFirstRep() { 562 if (getItem().isEmpty()) { 563 addItem(); 564 } 565 return getItem().get(0); 566 } 567 568 protected void listChildren(List<Property> children) { 569 super.listChildren(children); 570 children.add(new Property("active", "boolean", "Indicates whether the asserted set of linkages are considered to be \"in effect\".", 0, 1, active)); 571 children.add(new Property("author", "Reference(Practitioner|Organization)", "Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage.", 0, 1, author)); 572 children.add(new Property("item", "", "Identifies one of the records that is considered to refer to the same real-world occurrence as well as how the items hould be evaluated within the collection of linked items.", 0, java.lang.Integer.MAX_VALUE, item)); 573 } 574 575 @Override 576 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 577 switch (_hash) { 578 case -1422950650: /*active*/ return new Property("active", "boolean", "Indicates whether the asserted set of linkages are considered to be \"in effect\".", 0, 1, active); 579 case -1406328437: /*author*/ return new Property("author", "Reference(Practitioner|Organization)", "Identifies the user or organization responsible for asserting the linkages and who establishes the context for evaluating the nature of each linkage.", 0, 1, author); 580 case 3242771: /*item*/ return new Property("item", "", "Identifies one of the records that is considered to refer to the same real-world occurrence as well as how the items hould be evaluated within the collection of linked items.", 0, java.lang.Integer.MAX_VALUE, item); 581 default: return super.getNamedProperty(_hash, _name, _checkValid); 582 } 583 584 } 585 586 @Override 587 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 588 switch (hash) { 589 case -1422950650: /*active*/ return this.active == null ? new Base[0] : new Base[] {this.active}; // BooleanType 590 case -1406328437: /*author*/ return this.author == null ? new Base[0] : new Base[] {this.author}; // Reference 591 case 3242771: /*item*/ return this.item == null ? new Base[0] : this.item.toArray(new Base[this.item.size()]); // LinkageItemComponent 592 default: return super.getProperty(hash, name, checkValid); 593 } 594 595 } 596 597 @Override 598 public Base setProperty(int hash, String name, Base value) throws FHIRException { 599 switch (hash) { 600 case -1422950650: // active 601 this.active = castToBoolean(value); // BooleanType 602 return value; 603 case -1406328437: // author 604 this.author = castToReference(value); // Reference 605 return value; 606 case 3242771: // item 607 this.getItem().add((LinkageItemComponent) value); // LinkageItemComponent 608 return value; 609 default: return super.setProperty(hash, name, value); 610 } 611 612 } 613 614 @Override 615 public Base setProperty(String name, Base value) throws FHIRException { 616 if (name.equals("active")) { 617 this.active = castToBoolean(value); // BooleanType 618 } else if (name.equals("author")) { 619 this.author = castToReference(value); // Reference 620 } else if (name.equals("item")) { 621 this.getItem().add((LinkageItemComponent) value); 622 } else 623 return super.setProperty(name, value); 624 return value; 625 } 626 627 @Override 628 public Base makeProperty(int hash, String name) throws FHIRException { 629 switch (hash) { 630 case -1422950650: return getActiveElement(); 631 case -1406328437: return getAuthor(); 632 case 3242771: return addItem(); 633 default: return super.makeProperty(hash, name); 634 } 635 636 } 637 638 @Override 639 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 640 switch (hash) { 641 case -1422950650: /*active*/ return new String[] {"boolean"}; 642 case -1406328437: /*author*/ return new String[] {"Reference"}; 643 case 3242771: /*item*/ return new String[] {}; 644 default: return super.getTypesForProperty(hash, name); 645 } 646 647 } 648 649 @Override 650 public Base addChild(String name) throws FHIRException { 651 if (name.equals("active")) { 652 throw new FHIRException("Cannot call addChild on a singleton property Linkage.active"); 653 } 654 else if (name.equals("author")) { 655 this.author = new Reference(); 656 return this.author; 657 } 658 else if (name.equals("item")) { 659 return addItem(); 660 } 661 else 662 return super.addChild(name); 663 } 664 665 public String fhirType() { 666 return "Linkage"; 667 668 } 669 670 public Linkage copy() { 671 Linkage dst = new Linkage(); 672 copyValues(dst); 673 dst.active = active == null ? null : active.copy(); 674 dst.author = author == null ? null : author.copy(); 675 if (item != null) { 676 dst.item = new ArrayList<LinkageItemComponent>(); 677 for (LinkageItemComponent i : item) 678 dst.item.add(i.copy()); 679 }; 680 return dst; 681 } 682 683 protected Linkage typedCopy() { 684 return copy(); 685 } 686 687 @Override 688 public boolean equalsDeep(Base other_) { 689 if (!super.equalsDeep(other_)) 690 return false; 691 if (!(other_ instanceof Linkage)) 692 return false; 693 Linkage o = (Linkage) other_; 694 return compareDeep(active, o.active, true) && compareDeep(author, o.author, true) && compareDeep(item, o.item, true) 695 ; 696 } 697 698 @Override 699 public boolean equalsShallow(Base other_) { 700 if (!super.equalsShallow(other_)) 701 return false; 702 if (!(other_ instanceof Linkage)) 703 return false; 704 Linkage o = (Linkage) other_; 705 return compareValues(active, o.active, true); 706 } 707 708 public boolean isEmpty() { 709 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(active, author, item); 710 } 711 712 @Override 713 public ResourceType getResourceType() { 714 return ResourceType.Linkage; 715 } 716 717 /** 718 * Search parameter: <b>item</b> 719 * <p> 720 * Description: <b>Matches on any item in the Linkage</b><br> 721 * Type: <b>reference</b><br> 722 * Path: <b>Linkage.item.resource</b><br> 723 * </p> 724 */ 725 @SearchParamDefinition(name="item", path="Linkage.item.resource", description="Matches on any item in the Linkage", type="reference" ) 726 public static final String SP_ITEM = "item"; 727 /** 728 * <b>Fluent Client</b> search parameter constant for <b>item</b> 729 * <p> 730 * Description: <b>Matches on any item in the Linkage</b><br> 731 * Type: <b>reference</b><br> 732 * Path: <b>Linkage.item.resource</b><br> 733 * </p> 734 */ 735 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM); 736 737/** 738 * Constant for fluent queries to be used to add include statements. Specifies 739 * the path value of "<b>Linkage:item</b>". 740 */ 741 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include("Linkage:item").toLocked(); 742 743 /** 744 * Search parameter: <b>author</b> 745 * <p> 746 * Description: <b>Author of the Linkage</b><br> 747 * Type: <b>reference</b><br> 748 * Path: <b>Linkage.author</b><br> 749 * </p> 750 */ 751 @SearchParamDefinition(name="author", path="Linkage.author", description="Author of the Linkage", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Organization.class, Practitioner.class } ) 752 public static final String SP_AUTHOR = "author"; 753 /** 754 * <b>Fluent Client</b> search parameter constant for <b>author</b> 755 * <p> 756 * Description: <b>Author of the Linkage</b><br> 757 * Type: <b>reference</b><br> 758 * Path: <b>Linkage.author</b><br> 759 * </p> 760 */ 761 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam AUTHOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_AUTHOR); 762 763/** 764 * Constant for fluent queries to be used to add include statements. Specifies 765 * the path value of "<b>Linkage:author</b>". 766 */ 767 public static final ca.uhn.fhir.model.api.Include INCLUDE_AUTHOR = new ca.uhn.fhir.model.api.Include("Linkage:author").toLocked(); 768 769 /** 770 * Search parameter: <b>source</b> 771 * <p> 772 * Description: <b>Matches on any item in the Linkage with a type of 'source'</b><br> 773 * Type: <b>reference</b><br> 774 * Path: <b>Linkage.item.resource</b><br> 775 * </p> 776 */ 777 @SearchParamDefinition(name="source", path="Linkage.item.resource", description="Matches on any item in the Linkage with a type of 'source'", type="reference" ) 778 public static final String SP_SOURCE = "source"; 779 /** 780 * <b>Fluent Client</b> search parameter constant for <b>source</b> 781 * <p> 782 * Description: <b>Matches on any item in the Linkage with a type of 'source'</b><br> 783 * Type: <b>reference</b><br> 784 * Path: <b>Linkage.item.resource</b><br> 785 * </p> 786 */ 787 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 788 789/** 790 * Constant for fluent queries to be used to add include statements. Specifies 791 * the path value of "<b>Linkage:source</b>". 792 */ 793 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("Linkage:source").toLocked(); 794 795 796}