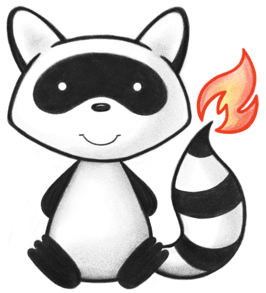
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 041import org.hl7.fhir.utilities.Utilities; 042 043import ca.uhn.fhir.model.api.annotation.Block; 044import ca.uhn.fhir.model.api.annotation.Child; 045import ca.uhn.fhir.model.api.annotation.Description; 046import ca.uhn.fhir.model.api.annotation.ResourceDef; 047import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 048/** 049 * A set of information summarized from a list of other resources. 050 */ 051@ResourceDef(name="List", profile="http://hl7.org/fhir/Profile/ListResource") 052public class ListResource extends DomainResource { 053 054 public enum ListStatus { 055 /** 056 * The list is considered to be an active part of the patient's record. 057 */ 058 CURRENT, 059 /** 060 * The list is "old" and should no longer be considered accurate or relevant. 061 */ 062 RETIRED, 063 /** 064 * The list was never accurate. It is retained for medico-legal purposes only. 065 */ 066 ENTEREDINERROR, 067 /** 068 * added to help the parsers with the generic types 069 */ 070 NULL; 071 public static ListStatus fromCode(String codeString) throws FHIRException { 072 if (codeString == null || "".equals(codeString)) 073 return null; 074 if ("current".equals(codeString)) 075 return CURRENT; 076 if ("retired".equals(codeString)) 077 return RETIRED; 078 if ("entered-in-error".equals(codeString)) 079 return ENTEREDINERROR; 080 if (Configuration.isAcceptInvalidEnums()) 081 return null; 082 else 083 throw new FHIRException("Unknown ListStatus code '"+codeString+"'"); 084 } 085 public String toCode() { 086 switch (this) { 087 case CURRENT: return "current"; 088 case RETIRED: return "retired"; 089 case ENTEREDINERROR: return "entered-in-error"; 090 case NULL: return null; 091 default: return "?"; 092 } 093 } 094 public String getSystem() { 095 switch (this) { 096 case CURRENT: return "http://hl7.org/fhir/list-status"; 097 case RETIRED: return "http://hl7.org/fhir/list-status"; 098 case ENTEREDINERROR: return "http://hl7.org/fhir/list-status"; 099 case NULL: return null; 100 default: return "?"; 101 } 102 } 103 public String getDefinition() { 104 switch (this) { 105 case CURRENT: return "The list is considered to be an active part of the patient's record."; 106 case RETIRED: return "The list is \"old\" and should no longer be considered accurate or relevant."; 107 case ENTEREDINERROR: return "The list was never accurate. It is retained for medico-legal purposes only."; 108 case NULL: return null; 109 default: return "?"; 110 } 111 } 112 public String getDisplay() { 113 switch (this) { 114 case CURRENT: return "Current"; 115 case RETIRED: return "Retired"; 116 case ENTEREDINERROR: return "Entered In Error"; 117 case NULL: return null; 118 default: return "?"; 119 } 120 } 121 } 122 123 public static class ListStatusEnumFactory implements EnumFactory<ListStatus> { 124 public ListStatus fromCode(String codeString) throws IllegalArgumentException { 125 if (codeString == null || "".equals(codeString)) 126 if (codeString == null || "".equals(codeString)) 127 return null; 128 if ("current".equals(codeString)) 129 return ListStatus.CURRENT; 130 if ("retired".equals(codeString)) 131 return ListStatus.RETIRED; 132 if ("entered-in-error".equals(codeString)) 133 return ListStatus.ENTEREDINERROR; 134 throw new IllegalArgumentException("Unknown ListStatus code '"+codeString+"'"); 135 } 136 public Enumeration<ListStatus> fromType(PrimitiveType<?> code) throws FHIRException { 137 if (code == null) 138 return null; 139 if (code.isEmpty()) 140 return new Enumeration<ListStatus>(this); 141 String codeString = code.asStringValue(); 142 if (codeString == null || "".equals(codeString)) 143 return null; 144 if ("current".equals(codeString)) 145 return new Enumeration<ListStatus>(this, ListStatus.CURRENT); 146 if ("retired".equals(codeString)) 147 return new Enumeration<ListStatus>(this, ListStatus.RETIRED); 148 if ("entered-in-error".equals(codeString)) 149 return new Enumeration<ListStatus>(this, ListStatus.ENTEREDINERROR); 150 throw new FHIRException("Unknown ListStatus code '"+codeString+"'"); 151 } 152 public String toCode(ListStatus code) { 153 if (code == ListStatus.NULL) 154 return null; 155 if (code == ListStatus.CURRENT) 156 return "current"; 157 if (code == ListStatus.RETIRED) 158 return "retired"; 159 if (code == ListStatus.ENTEREDINERROR) 160 return "entered-in-error"; 161 return "?"; 162 } 163 public String toSystem(ListStatus code) { 164 return code.getSystem(); 165 } 166 } 167 168 public enum ListMode { 169 /** 170 * This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes 171 */ 172 WORKING, 173 /** 174 * This list was prepared as a snapshot. It should not be assumed to be current 175 */ 176 SNAPSHOT, 177 /** 178 * A list that indicates where changes have been made or recommended 179 */ 180 CHANGES, 181 /** 182 * added to help the parsers with the generic types 183 */ 184 NULL; 185 public static ListMode fromCode(String codeString) throws FHIRException { 186 if (codeString == null || "".equals(codeString)) 187 return null; 188 if ("working".equals(codeString)) 189 return WORKING; 190 if ("snapshot".equals(codeString)) 191 return SNAPSHOT; 192 if ("changes".equals(codeString)) 193 return CHANGES; 194 if (Configuration.isAcceptInvalidEnums()) 195 return null; 196 else 197 throw new FHIRException("Unknown ListMode code '"+codeString+"'"); 198 } 199 public String toCode() { 200 switch (this) { 201 case WORKING: return "working"; 202 case SNAPSHOT: return "snapshot"; 203 case CHANGES: return "changes"; 204 case NULL: return null; 205 default: return "?"; 206 } 207 } 208 public String getSystem() { 209 switch (this) { 210 case WORKING: return "http://hl7.org/fhir/list-mode"; 211 case SNAPSHOT: return "http://hl7.org/fhir/list-mode"; 212 case CHANGES: return "http://hl7.org/fhir/list-mode"; 213 case NULL: return null; 214 default: return "?"; 215 } 216 } 217 public String getDefinition() { 218 switch (this) { 219 case WORKING: return "This list is the master list, maintained in an ongoing fashion with regular updates as the real world list it is tracking changes"; 220 case SNAPSHOT: return "This list was prepared as a snapshot. It should not be assumed to be current"; 221 case CHANGES: return "A list that indicates where changes have been made or recommended"; 222 case NULL: return null; 223 default: return "?"; 224 } 225 } 226 public String getDisplay() { 227 switch (this) { 228 case WORKING: return "Working List"; 229 case SNAPSHOT: return "Snapshot List"; 230 case CHANGES: return "Change List"; 231 case NULL: return null; 232 default: return "?"; 233 } 234 } 235 } 236 237 public static class ListModeEnumFactory implements EnumFactory<ListMode> { 238 public ListMode fromCode(String codeString) throws IllegalArgumentException { 239 if (codeString == null || "".equals(codeString)) 240 if (codeString == null || "".equals(codeString)) 241 return null; 242 if ("working".equals(codeString)) 243 return ListMode.WORKING; 244 if ("snapshot".equals(codeString)) 245 return ListMode.SNAPSHOT; 246 if ("changes".equals(codeString)) 247 return ListMode.CHANGES; 248 throw new IllegalArgumentException("Unknown ListMode code '"+codeString+"'"); 249 } 250 public Enumeration<ListMode> fromType(PrimitiveType<?> code) throws FHIRException { 251 if (code == null) 252 return null; 253 if (code.isEmpty()) 254 return new Enumeration<ListMode>(this); 255 String codeString = code.asStringValue(); 256 if (codeString == null || "".equals(codeString)) 257 return null; 258 if ("working".equals(codeString)) 259 return new Enumeration<ListMode>(this, ListMode.WORKING); 260 if ("snapshot".equals(codeString)) 261 return new Enumeration<ListMode>(this, ListMode.SNAPSHOT); 262 if ("changes".equals(codeString)) 263 return new Enumeration<ListMode>(this, ListMode.CHANGES); 264 throw new FHIRException("Unknown ListMode code '"+codeString+"'"); 265 } 266 public String toCode(ListMode code) { 267 if (code == ListMode.NULL) 268 return null; 269 if (code == ListMode.WORKING) 270 return "working"; 271 if (code == ListMode.SNAPSHOT) 272 return "snapshot"; 273 if (code == ListMode.CHANGES) 274 return "changes"; 275 return "?"; 276 } 277 public String toSystem(ListMode code) { 278 return code.getSystem(); 279 } 280 } 281 282 @Block() 283 public static class ListEntryComponent extends BackboneElement implements IBaseBackboneElement { 284 /** 285 * The flag allows the system constructing the list to indicate the role and significance of the item in the list. 286 */ 287 @Child(name = "flag", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 288 @Description(shortDefinition="Status/Workflow information about this item", formalDefinition="The flag allows the system constructing the list to indicate the role and significance of the item in the list." ) 289 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-item-flag") 290 protected CodeableConcept flag; 291 292 /** 293 * True if this item is marked as deleted in the list. 294 */ 295 @Child(name = "deleted", type = {BooleanType.class}, order=2, min=0, max=1, modifier=true, summary=false) 296 @Description(shortDefinition="If this item is actually marked as deleted", formalDefinition="True if this item is marked as deleted in the list." ) 297 protected BooleanType deleted; 298 299 /** 300 * When this item was added to the list. 301 */ 302 @Child(name = "date", type = {DateTimeType.class}, order=3, min=0, max=1, modifier=false, summary=false) 303 @Description(shortDefinition="When item added to list", formalDefinition="When this item was added to the list." ) 304 protected DateTimeType date; 305 306 /** 307 * A reference to the actual resource from which data was derived. 308 */ 309 @Child(name = "item", type = {Reference.class}, order=4, min=1, max=1, modifier=false, summary=false) 310 @Description(shortDefinition="Actual entry", formalDefinition="A reference to the actual resource from which data was derived." ) 311 protected Reference item; 312 313 /** 314 * The actual object that is the target of the reference (A reference to the actual resource from which data was derived.) 315 */ 316 protected Resource itemTarget; 317 318 private static final long serialVersionUID = -758164425L; 319 320 /** 321 * Constructor 322 */ 323 public ListEntryComponent() { 324 super(); 325 } 326 327 /** 328 * Constructor 329 */ 330 public ListEntryComponent(Reference item) { 331 super(); 332 this.item = item; 333 } 334 335 /** 336 * @return {@link #flag} (The flag allows the system constructing the list to indicate the role and significance of the item in the list.) 337 */ 338 public CodeableConcept getFlag() { 339 if (this.flag == null) 340 if (Configuration.errorOnAutoCreate()) 341 throw new Error("Attempt to auto-create ListEntryComponent.flag"); 342 else if (Configuration.doAutoCreate()) 343 this.flag = new CodeableConcept(); // cc 344 return this.flag; 345 } 346 347 public boolean hasFlag() { 348 return this.flag != null && !this.flag.isEmpty(); 349 } 350 351 /** 352 * @param value {@link #flag} (The flag allows the system constructing the list to indicate the role and significance of the item in the list.) 353 */ 354 public ListEntryComponent setFlag(CodeableConcept value) { 355 this.flag = value; 356 return this; 357 } 358 359 /** 360 * @return {@link #deleted} (True if this item is marked as deleted in the list.). This is the underlying object with id, value and extensions. The accessor "getDeleted" gives direct access to the value 361 */ 362 public BooleanType getDeletedElement() { 363 if (this.deleted == null) 364 if (Configuration.errorOnAutoCreate()) 365 throw new Error("Attempt to auto-create ListEntryComponent.deleted"); 366 else if (Configuration.doAutoCreate()) 367 this.deleted = new BooleanType(); // bb 368 return this.deleted; 369 } 370 371 public boolean hasDeletedElement() { 372 return this.deleted != null && !this.deleted.isEmpty(); 373 } 374 375 public boolean hasDeleted() { 376 return this.deleted != null && !this.deleted.isEmpty(); 377 } 378 379 /** 380 * @param value {@link #deleted} (True if this item is marked as deleted in the list.). This is the underlying object with id, value and extensions. The accessor "getDeleted" gives direct access to the value 381 */ 382 public ListEntryComponent setDeletedElement(BooleanType value) { 383 this.deleted = value; 384 return this; 385 } 386 387 /** 388 * @return True if this item is marked as deleted in the list. 389 */ 390 public boolean getDeleted() { 391 return this.deleted == null || this.deleted.isEmpty() ? false : this.deleted.getValue(); 392 } 393 394 /** 395 * @param value True if this item is marked as deleted in the list. 396 */ 397 public ListEntryComponent setDeleted(boolean value) { 398 if (this.deleted == null) 399 this.deleted = new BooleanType(); 400 this.deleted.setValue(value); 401 return this; 402 } 403 404 /** 405 * @return {@link #date} (When this item was added to the list.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 406 */ 407 public DateTimeType getDateElement() { 408 if (this.date == null) 409 if (Configuration.errorOnAutoCreate()) 410 throw new Error("Attempt to auto-create ListEntryComponent.date"); 411 else if (Configuration.doAutoCreate()) 412 this.date = new DateTimeType(); // bb 413 return this.date; 414 } 415 416 public boolean hasDateElement() { 417 return this.date != null && !this.date.isEmpty(); 418 } 419 420 public boolean hasDate() { 421 return this.date != null && !this.date.isEmpty(); 422 } 423 424 /** 425 * @param value {@link #date} (When this item was added to the list.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 426 */ 427 public ListEntryComponent setDateElement(DateTimeType value) { 428 this.date = value; 429 return this; 430 } 431 432 /** 433 * @return When this item was added to the list. 434 */ 435 public Date getDate() { 436 return this.date == null ? null : this.date.getValue(); 437 } 438 439 /** 440 * @param value When this item was added to the list. 441 */ 442 public ListEntryComponent setDate(Date value) { 443 if (value == null) 444 this.date = null; 445 else { 446 if (this.date == null) 447 this.date = new DateTimeType(); 448 this.date.setValue(value); 449 } 450 return this; 451 } 452 453 /** 454 * @return {@link #item} (A reference to the actual resource from which data was derived.) 455 */ 456 public Reference getItem() { 457 if (this.item == null) 458 if (Configuration.errorOnAutoCreate()) 459 throw new Error("Attempt to auto-create ListEntryComponent.item"); 460 else if (Configuration.doAutoCreate()) 461 this.item = new Reference(); // cc 462 return this.item; 463 } 464 465 public boolean hasItem() { 466 return this.item != null && !this.item.isEmpty(); 467 } 468 469 /** 470 * @param value {@link #item} (A reference to the actual resource from which data was derived.) 471 */ 472 public ListEntryComponent setItem(Reference value) { 473 this.item = value; 474 return this; 475 } 476 477 /** 478 * @return {@link #item} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the actual resource from which data was derived.) 479 */ 480 public Resource getItemTarget() { 481 return this.itemTarget; 482 } 483 484 /** 485 * @param value {@link #item} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the actual resource from which data was derived.) 486 */ 487 public ListEntryComponent setItemTarget(Resource value) { 488 this.itemTarget = value; 489 return this; 490 } 491 492 protected void listChildren(List<Property> children) { 493 super.listChildren(children); 494 children.add(new Property("flag", "CodeableConcept", "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 0, 1, flag)); 495 children.add(new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 0, 1, deleted)); 496 children.add(new Property("date", "dateTime", "When this item was added to the list.", 0, 1, date)); 497 children.add(new Property("item", "Reference(Any)", "A reference to the actual resource from which data was derived.", 0, 1, item)); 498 } 499 500 @Override 501 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 502 switch (_hash) { 503 case 3145580: /*flag*/ return new Property("flag", "CodeableConcept", "The flag allows the system constructing the list to indicate the role and significance of the item in the list.", 0, 1, flag); 504 case 1550463001: /*deleted*/ return new Property("deleted", "boolean", "True if this item is marked as deleted in the list.", 0, 1, deleted); 505 case 3076014: /*date*/ return new Property("date", "dateTime", "When this item was added to the list.", 0, 1, date); 506 case 3242771: /*item*/ return new Property("item", "Reference(Any)", "A reference to the actual resource from which data was derived.", 0, 1, item); 507 default: return super.getNamedProperty(_hash, _name, _checkValid); 508 } 509 510 } 511 512 @Override 513 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 514 switch (hash) { 515 case 3145580: /*flag*/ return this.flag == null ? new Base[0] : new Base[] {this.flag}; // CodeableConcept 516 case 1550463001: /*deleted*/ return this.deleted == null ? new Base[0] : new Base[] {this.deleted}; // BooleanType 517 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 518 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // Reference 519 default: return super.getProperty(hash, name, checkValid); 520 } 521 522 } 523 524 @Override 525 public Base setProperty(int hash, String name, Base value) throws FHIRException { 526 switch (hash) { 527 case 3145580: // flag 528 this.flag = castToCodeableConcept(value); // CodeableConcept 529 return value; 530 case 1550463001: // deleted 531 this.deleted = castToBoolean(value); // BooleanType 532 return value; 533 case 3076014: // date 534 this.date = castToDateTime(value); // DateTimeType 535 return value; 536 case 3242771: // item 537 this.item = castToReference(value); // Reference 538 return value; 539 default: return super.setProperty(hash, name, value); 540 } 541 542 } 543 544 @Override 545 public Base setProperty(String name, Base value) throws FHIRException { 546 if (name.equals("flag")) { 547 this.flag = castToCodeableConcept(value); // CodeableConcept 548 } else if (name.equals("deleted")) { 549 this.deleted = castToBoolean(value); // BooleanType 550 } else if (name.equals("date")) { 551 this.date = castToDateTime(value); // DateTimeType 552 } else if (name.equals("item")) { 553 this.item = castToReference(value); // Reference 554 } else 555 return super.setProperty(name, value); 556 return value; 557 } 558 559 @Override 560 public Base makeProperty(int hash, String name) throws FHIRException { 561 switch (hash) { 562 case 3145580: return getFlag(); 563 case 1550463001: return getDeletedElement(); 564 case 3076014: return getDateElement(); 565 case 3242771: return getItem(); 566 default: return super.makeProperty(hash, name); 567 } 568 569 } 570 571 @Override 572 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 573 switch (hash) { 574 case 3145580: /*flag*/ return new String[] {"CodeableConcept"}; 575 case 1550463001: /*deleted*/ return new String[] {"boolean"}; 576 case 3076014: /*date*/ return new String[] {"dateTime"}; 577 case 3242771: /*item*/ return new String[] {"Reference"}; 578 default: return super.getTypesForProperty(hash, name); 579 } 580 581 } 582 583 @Override 584 public Base addChild(String name) throws FHIRException { 585 if (name.equals("flag")) { 586 this.flag = new CodeableConcept(); 587 return this.flag; 588 } 589 else if (name.equals("deleted")) { 590 throw new FHIRException("Cannot call addChild on a singleton property List.deleted"); 591 } 592 else if (name.equals("date")) { 593 throw new FHIRException("Cannot call addChild on a singleton property List.date"); 594 } 595 else if (name.equals("item")) { 596 this.item = new Reference(); 597 return this.item; 598 } 599 else 600 return super.addChild(name); 601 } 602 603 public ListEntryComponent copy() { 604 ListEntryComponent dst = new ListEntryComponent(); 605 copyValues(dst); 606 dst.flag = flag == null ? null : flag.copy(); 607 dst.deleted = deleted == null ? null : deleted.copy(); 608 dst.date = date == null ? null : date.copy(); 609 dst.item = item == null ? null : item.copy(); 610 return dst; 611 } 612 613 @Override 614 public boolean equalsDeep(Base other_) { 615 if (!super.equalsDeep(other_)) 616 return false; 617 if (!(other_ instanceof ListEntryComponent)) 618 return false; 619 ListEntryComponent o = (ListEntryComponent) other_; 620 return compareDeep(flag, o.flag, true) && compareDeep(deleted, o.deleted, true) && compareDeep(date, o.date, true) 621 && compareDeep(item, o.item, true); 622 } 623 624 @Override 625 public boolean equalsShallow(Base other_) { 626 if (!super.equalsShallow(other_)) 627 return false; 628 if (!(other_ instanceof ListEntryComponent)) 629 return false; 630 ListEntryComponent o = (ListEntryComponent) other_; 631 return compareValues(deleted, o.deleted, true) && compareValues(date, o.date, true); 632 } 633 634 public boolean isEmpty() { 635 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(flag, deleted, date, item 636 ); 637 } 638 639 public String fhirType() { 640 return "List.entry"; 641 642 } 643 644 } 645 646 /** 647 * Identifier for the List assigned for business purposes outside the context of FHIR. 648 */ 649 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 650 @Description(shortDefinition="Business identifier", formalDefinition="Identifier for the List assigned for business purposes outside the context of FHIR." ) 651 protected List<Identifier> identifier; 652 653 /** 654 * Indicates the current state of this list. 655 */ 656 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 657 @Description(shortDefinition="current | retired | entered-in-error", formalDefinition="Indicates the current state of this list." ) 658 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-status") 659 protected Enumeration<ListStatus> status; 660 661 /** 662 * How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 663 */ 664 @Child(name = "mode", type = {CodeType.class}, order=2, min=1, max=1, modifier=true, summary=true) 665 @Description(shortDefinition="working | snapshot | changes", formalDefinition="How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted." ) 666 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-mode") 667 protected Enumeration<ListMode> mode; 668 669 /** 670 * A label for the list assigned by the author. 671 */ 672 @Child(name = "title", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 673 @Description(shortDefinition="Descriptive name for the list", formalDefinition="A label for the list assigned by the author." ) 674 protected StringType title; 675 676 /** 677 * This code defines the purpose of the list - why it was created. 678 */ 679 @Child(name = "code", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 680 @Description(shortDefinition="What the purpose of this list is", formalDefinition="This code defines the purpose of the list - why it was created." ) 681 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-example-codes") 682 protected CodeableConcept code; 683 684 /** 685 * The common subject (or patient) of the resources that are in the list, if there is one. 686 */ 687 @Child(name = "subject", type = {Patient.class, Group.class, Device.class, Location.class}, order=5, min=0, max=1, modifier=false, summary=true) 688 @Description(shortDefinition="If all resources have the same subject", formalDefinition="The common subject (or patient) of the resources that are in the list, if there is one." ) 689 protected Reference subject; 690 691 /** 692 * The actual object that is the target of the reference (The common subject (or patient) of the resources that are in the list, if there is one.) 693 */ 694 protected Resource subjectTarget; 695 696 /** 697 * The encounter that is the context in which this list was created. 698 */ 699 @Child(name = "encounter", type = {Encounter.class}, order=6, min=0, max=1, modifier=false, summary=false) 700 @Description(shortDefinition="Context in which list created", formalDefinition="The encounter that is the context in which this list was created." ) 701 protected Reference encounter; 702 703 /** 704 * The actual object that is the target of the reference (The encounter that is the context in which this list was created.) 705 */ 706 protected Encounter encounterTarget; 707 708 /** 709 * The date that the list was prepared. 710 */ 711 @Child(name = "date", type = {DateTimeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 712 @Description(shortDefinition="When the list was prepared", formalDefinition="The date that the list was prepared." ) 713 protected DateTimeType date; 714 715 /** 716 * The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list. 717 */ 718 @Child(name = "source", type = {Practitioner.class, Patient.class, Device.class}, order=8, min=0, max=1, modifier=false, summary=true) 719 @Description(shortDefinition="Who and/or what defined the list contents (aka Author)", formalDefinition="The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list." ) 720 protected Reference source; 721 722 /** 723 * The actual object that is the target of the reference (The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.) 724 */ 725 protected Resource sourceTarget; 726 727 /** 728 * What order applies to the items in the list. 729 */ 730 @Child(name = "orderedBy", type = {CodeableConcept.class}, order=9, min=0, max=1, modifier=false, summary=false) 731 @Description(shortDefinition="What order the list has", formalDefinition="What order applies to the items in the list." ) 732 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-order") 733 protected CodeableConcept orderedBy; 734 735 /** 736 * Comments that apply to the overall list. 737 */ 738 @Child(name = "note", type = {Annotation.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 739 @Description(shortDefinition="Comments about the list", formalDefinition="Comments that apply to the overall list." ) 740 protected List<Annotation> note; 741 742 /** 743 * Entries in this list. 744 */ 745 @Child(name = "entry", type = {}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 746 @Description(shortDefinition="Entries in the list", formalDefinition="Entries in this list." ) 747 protected List<ListEntryComponent> entry; 748 749 /** 750 * If the list is empty, why the list is empty. 751 */ 752 @Child(name = "emptyReason", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=false) 753 @Description(shortDefinition="Why list is empty", formalDefinition="If the list is empty, why the list is empty." ) 754 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/list-empty-reason") 755 protected CodeableConcept emptyReason; 756 757 private static final long serialVersionUID = 2071342704L; 758 759 /** 760 * Constructor 761 */ 762 public ListResource() { 763 super(); 764 } 765 766 /** 767 * Constructor 768 */ 769 public ListResource(Enumeration<ListStatus> status, Enumeration<ListMode> mode) { 770 super(); 771 this.status = status; 772 this.mode = mode; 773 } 774 775 /** 776 * @return {@link #identifier} (Identifier for the List assigned for business purposes outside the context of FHIR.) 777 */ 778 public List<Identifier> getIdentifier() { 779 if (this.identifier == null) 780 this.identifier = new ArrayList<Identifier>(); 781 return this.identifier; 782 } 783 784 /** 785 * @return Returns a reference to <code>this</code> for easy method chaining 786 */ 787 public ListResource setIdentifier(List<Identifier> theIdentifier) { 788 this.identifier = theIdentifier; 789 return this; 790 } 791 792 public boolean hasIdentifier() { 793 if (this.identifier == null) 794 return false; 795 for (Identifier item : this.identifier) 796 if (!item.isEmpty()) 797 return true; 798 return false; 799 } 800 801 public Identifier addIdentifier() { //3 802 Identifier t = new Identifier(); 803 if (this.identifier == null) 804 this.identifier = new ArrayList<Identifier>(); 805 this.identifier.add(t); 806 return t; 807 } 808 809 public ListResource addIdentifier(Identifier t) { //3 810 if (t == null) 811 return this; 812 if (this.identifier == null) 813 this.identifier = new ArrayList<Identifier>(); 814 this.identifier.add(t); 815 return this; 816 } 817 818 /** 819 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 820 */ 821 public Identifier getIdentifierFirstRep() { 822 if (getIdentifier().isEmpty()) { 823 addIdentifier(); 824 } 825 return getIdentifier().get(0); 826 } 827 828 /** 829 * @return {@link #status} (Indicates the current state of this list.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 830 */ 831 public Enumeration<ListStatus> getStatusElement() { 832 if (this.status == null) 833 if (Configuration.errorOnAutoCreate()) 834 throw new Error("Attempt to auto-create List.status"); 835 else if (Configuration.doAutoCreate()) 836 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); // bb 837 return this.status; 838 } 839 840 public boolean hasStatusElement() { 841 return this.status != null && !this.status.isEmpty(); 842 } 843 844 public boolean hasStatus() { 845 return this.status != null && !this.status.isEmpty(); 846 } 847 848 /** 849 * @param value {@link #status} (Indicates the current state of this list.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 850 */ 851 public ListResource setStatusElement(Enumeration<ListStatus> value) { 852 this.status = value; 853 return this; 854 } 855 856 /** 857 * @return Indicates the current state of this list. 858 */ 859 public ListStatus getStatus() { 860 return this.status == null ? null : this.status.getValue(); 861 } 862 863 /** 864 * @param value Indicates the current state of this list. 865 */ 866 public ListResource setStatus(ListStatus value) { 867 if (this.status == null) 868 this.status = new Enumeration<ListStatus>(new ListStatusEnumFactory()); 869 this.status.setValue(value); 870 return this; 871 } 872 873 /** 874 * @return {@link #mode} (How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 875 */ 876 public Enumeration<ListMode> getModeElement() { 877 if (this.mode == null) 878 if (Configuration.errorOnAutoCreate()) 879 throw new Error("Attempt to auto-create List.mode"); 880 else if (Configuration.doAutoCreate()) 881 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); // bb 882 return this.mode; 883 } 884 885 public boolean hasModeElement() { 886 return this.mode != null && !this.mode.isEmpty(); 887 } 888 889 public boolean hasMode() { 890 return this.mode != null && !this.mode.isEmpty(); 891 } 892 893 /** 894 * @param value {@link #mode} (How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 895 */ 896 public ListResource setModeElement(Enumeration<ListMode> value) { 897 this.mode = value; 898 return this; 899 } 900 901 /** 902 * @return How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 903 */ 904 public ListMode getMode() { 905 return this.mode == null ? null : this.mode.getValue(); 906 } 907 908 /** 909 * @param value How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted. 910 */ 911 public ListResource setMode(ListMode value) { 912 if (this.mode == null) 913 this.mode = new Enumeration<ListMode>(new ListModeEnumFactory()); 914 this.mode.setValue(value); 915 return this; 916 } 917 918 /** 919 * @return {@link #title} (A label for the list assigned by the author.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 920 */ 921 public StringType getTitleElement() { 922 if (this.title == null) 923 if (Configuration.errorOnAutoCreate()) 924 throw new Error("Attempt to auto-create List.title"); 925 else if (Configuration.doAutoCreate()) 926 this.title = new StringType(); // bb 927 return this.title; 928 } 929 930 public boolean hasTitleElement() { 931 return this.title != null && !this.title.isEmpty(); 932 } 933 934 public boolean hasTitle() { 935 return this.title != null && !this.title.isEmpty(); 936 } 937 938 /** 939 * @param value {@link #title} (A label for the list assigned by the author.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 940 */ 941 public ListResource setTitleElement(StringType value) { 942 this.title = value; 943 return this; 944 } 945 946 /** 947 * @return A label for the list assigned by the author. 948 */ 949 public String getTitle() { 950 return this.title == null ? null : this.title.getValue(); 951 } 952 953 /** 954 * @param value A label for the list assigned by the author. 955 */ 956 public ListResource setTitle(String value) { 957 if (Utilities.noString(value)) 958 this.title = null; 959 else { 960 if (this.title == null) 961 this.title = new StringType(); 962 this.title.setValue(value); 963 } 964 return this; 965 } 966 967 /** 968 * @return {@link #code} (This code defines the purpose of the list - why it was created.) 969 */ 970 public CodeableConcept getCode() { 971 if (this.code == null) 972 if (Configuration.errorOnAutoCreate()) 973 throw new Error("Attempt to auto-create List.code"); 974 else if (Configuration.doAutoCreate()) 975 this.code = new CodeableConcept(); // cc 976 return this.code; 977 } 978 979 public boolean hasCode() { 980 return this.code != null && !this.code.isEmpty(); 981 } 982 983 /** 984 * @param value {@link #code} (This code defines the purpose of the list - why it was created.) 985 */ 986 public ListResource setCode(CodeableConcept value) { 987 this.code = value; 988 return this; 989 } 990 991 /** 992 * @return {@link #subject} (The common subject (or patient) of the resources that are in the list, if there is one.) 993 */ 994 public Reference getSubject() { 995 if (this.subject == null) 996 if (Configuration.errorOnAutoCreate()) 997 throw new Error("Attempt to auto-create List.subject"); 998 else if (Configuration.doAutoCreate()) 999 this.subject = new Reference(); // cc 1000 return this.subject; 1001 } 1002 1003 public boolean hasSubject() { 1004 return this.subject != null && !this.subject.isEmpty(); 1005 } 1006 1007 /** 1008 * @param value {@link #subject} (The common subject (or patient) of the resources that are in the list, if there is one.) 1009 */ 1010 public ListResource setSubject(Reference value) { 1011 this.subject = value; 1012 return this; 1013 } 1014 1015 /** 1016 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The common subject (or patient) of the resources that are in the list, if there is one.) 1017 */ 1018 public Resource getSubjectTarget() { 1019 return this.subjectTarget; 1020 } 1021 1022 /** 1023 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The common subject (or patient) of the resources that are in the list, if there is one.) 1024 */ 1025 public ListResource setSubjectTarget(Resource value) { 1026 this.subjectTarget = value; 1027 return this; 1028 } 1029 1030 /** 1031 * @return {@link #encounter} (The encounter that is the context in which this list was created.) 1032 */ 1033 public Reference getEncounter() { 1034 if (this.encounter == null) 1035 if (Configuration.errorOnAutoCreate()) 1036 throw new Error("Attempt to auto-create List.encounter"); 1037 else if (Configuration.doAutoCreate()) 1038 this.encounter = new Reference(); // cc 1039 return this.encounter; 1040 } 1041 1042 public boolean hasEncounter() { 1043 return this.encounter != null && !this.encounter.isEmpty(); 1044 } 1045 1046 /** 1047 * @param value {@link #encounter} (The encounter that is the context in which this list was created.) 1048 */ 1049 public ListResource setEncounter(Reference value) { 1050 this.encounter = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return {@link #encounter} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter that is the context in which this list was created.) 1056 */ 1057 public Encounter getEncounterTarget() { 1058 if (this.encounterTarget == null) 1059 if (Configuration.errorOnAutoCreate()) 1060 throw new Error("Attempt to auto-create List.encounter"); 1061 else if (Configuration.doAutoCreate()) 1062 this.encounterTarget = new Encounter(); // aa 1063 return this.encounterTarget; 1064 } 1065 1066 /** 1067 * @param value {@link #encounter} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter that is the context in which this list was created.) 1068 */ 1069 public ListResource setEncounterTarget(Encounter value) { 1070 this.encounterTarget = value; 1071 return this; 1072 } 1073 1074 /** 1075 * @return {@link #date} (The date that the list was prepared.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1076 */ 1077 public DateTimeType getDateElement() { 1078 if (this.date == null) 1079 if (Configuration.errorOnAutoCreate()) 1080 throw new Error("Attempt to auto-create List.date"); 1081 else if (Configuration.doAutoCreate()) 1082 this.date = new DateTimeType(); // bb 1083 return this.date; 1084 } 1085 1086 public boolean hasDateElement() { 1087 return this.date != null && !this.date.isEmpty(); 1088 } 1089 1090 public boolean hasDate() { 1091 return this.date != null && !this.date.isEmpty(); 1092 } 1093 1094 /** 1095 * @param value {@link #date} (The date that the list was prepared.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1096 */ 1097 public ListResource setDateElement(DateTimeType value) { 1098 this.date = value; 1099 return this; 1100 } 1101 1102 /** 1103 * @return The date that the list was prepared. 1104 */ 1105 public Date getDate() { 1106 return this.date == null ? null : this.date.getValue(); 1107 } 1108 1109 /** 1110 * @param value The date that the list was prepared. 1111 */ 1112 public ListResource setDate(Date value) { 1113 if (value == null) 1114 this.date = null; 1115 else { 1116 if (this.date == null) 1117 this.date = new DateTimeType(); 1118 this.date.setValue(value); 1119 } 1120 return this; 1121 } 1122 1123 /** 1124 * @return {@link #source} (The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.) 1125 */ 1126 public Reference getSource() { 1127 if (this.source == null) 1128 if (Configuration.errorOnAutoCreate()) 1129 throw new Error("Attempt to auto-create List.source"); 1130 else if (Configuration.doAutoCreate()) 1131 this.source = new Reference(); // cc 1132 return this.source; 1133 } 1134 1135 public boolean hasSource() { 1136 return this.source != null && !this.source.isEmpty(); 1137 } 1138 1139 /** 1140 * @param value {@link #source} (The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.) 1141 */ 1142 public ListResource setSource(Reference value) { 1143 this.source = value; 1144 return this; 1145 } 1146 1147 /** 1148 * @return {@link #source} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.) 1149 */ 1150 public Resource getSourceTarget() { 1151 return this.sourceTarget; 1152 } 1153 1154 /** 1155 * @param value {@link #source} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.) 1156 */ 1157 public ListResource setSourceTarget(Resource value) { 1158 this.sourceTarget = value; 1159 return this; 1160 } 1161 1162 /** 1163 * @return {@link #orderedBy} (What order applies to the items in the list.) 1164 */ 1165 public CodeableConcept getOrderedBy() { 1166 if (this.orderedBy == null) 1167 if (Configuration.errorOnAutoCreate()) 1168 throw new Error("Attempt to auto-create List.orderedBy"); 1169 else if (Configuration.doAutoCreate()) 1170 this.orderedBy = new CodeableConcept(); // cc 1171 return this.orderedBy; 1172 } 1173 1174 public boolean hasOrderedBy() { 1175 return this.orderedBy != null && !this.orderedBy.isEmpty(); 1176 } 1177 1178 /** 1179 * @param value {@link #orderedBy} (What order applies to the items in the list.) 1180 */ 1181 public ListResource setOrderedBy(CodeableConcept value) { 1182 this.orderedBy = value; 1183 return this; 1184 } 1185 1186 /** 1187 * @return {@link #note} (Comments that apply to the overall list.) 1188 */ 1189 public List<Annotation> getNote() { 1190 if (this.note == null) 1191 this.note = new ArrayList<Annotation>(); 1192 return this.note; 1193 } 1194 1195 /** 1196 * @return Returns a reference to <code>this</code> for easy method chaining 1197 */ 1198 public ListResource setNote(List<Annotation> theNote) { 1199 this.note = theNote; 1200 return this; 1201 } 1202 1203 public boolean hasNote() { 1204 if (this.note == null) 1205 return false; 1206 for (Annotation item : this.note) 1207 if (!item.isEmpty()) 1208 return true; 1209 return false; 1210 } 1211 1212 public Annotation addNote() { //3 1213 Annotation t = new Annotation(); 1214 if (this.note == null) 1215 this.note = new ArrayList<Annotation>(); 1216 this.note.add(t); 1217 return t; 1218 } 1219 1220 public ListResource addNote(Annotation t) { //3 1221 if (t == null) 1222 return this; 1223 if (this.note == null) 1224 this.note = new ArrayList<Annotation>(); 1225 this.note.add(t); 1226 return this; 1227 } 1228 1229 /** 1230 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1231 */ 1232 public Annotation getNoteFirstRep() { 1233 if (getNote().isEmpty()) { 1234 addNote(); 1235 } 1236 return getNote().get(0); 1237 } 1238 1239 /** 1240 * @return {@link #entry} (Entries in this list.) 1241 */ 1242 public List<ListEntryComponent> getEntry() { 1243 if (this.entry == null) 1244 this.entry = new ArrayList<ListEntryComponent>(); 1245 return this.entry; 1246 } 1247 1248 /** 1249 * @return Returns a reference to <code>this</code> for easy method chaining 1250 */ 1251 public ListResource setEntry(List<ListEntryComponent> theEntry) { 1252 this.entry = theEntry; 1253 return this; 1254 } 1255 1256 public boolean hasEntry() { 1257 if (this.entry == null) 1258 return false; 1259 for (ListEntryComponent item : this.entry) 1260 if (!item.isEmpty()) 1261 return true; 1262 return false; 1263 } 1264 1265 public ListEntryComponent addEntry() { //3 1266 ListEntryComponent t = new ListEntryComponent(); 1267 if (this.entry == null) 1268 this.entry = new ArrayList<ListEntryComponent>(); 1269 this.entry.add(t); 1270 return t; 1271 } 1272 1273 public ListResource addEntry(ListEntryComponent t) { //3 1274 if (t == null) 1275 return this; 1276 if (this.entry == null) 1277 this.entry = new ArrayList<ListEntryComponent>(); 1278 this.entry.add(t); 1279 return this; 1280 } 1281 1282 /** 1283 * @return The first repetition of repeating field {@link #entry}, creating it if it does not already exist 1284 */ 1285 public ListEntryComponent getEntryFirstRep() { 1286 if (getEntry().isEmpty()) { 1287 addEntry(); 1288 } 1289 return getEntry().get(0); 1290 } 1291 1292 /** 1293 * @return {@link #emptyReason} (If the list is empty, why the list is empty.) 1294 */ 1295 public CodeableConcept getEmptyReason() { 1296 if (this.emptyReason == null) 1297 if (Configuration.errorOnAutoCreate()) 1298 throw new Error("Attempt to auto-create List.emptyReason"); 1299 else if (Configuration.doAutoCreate()) 1300 this.emptyReason = new CodeableConcept(); // cc 1301 return this.emptyReason; 1302 } 1303 1304 public boolean hasEmptyReason() { 1305 return this.emptyReason != null && !this.emptyReason.isEmpty(); 1306 } 1307 1308 /** 1309 * @param value {@link #emptyReason} (If the list is empty, why the list is empty.) 1310 */ 1311 public ListResource setEmptyReason(CodeableConcept value) { 1312 this.emptyReason = value; 1313 return this; 1314 } 1315 1316 protected void listChildren(List<Property> children) { 1317 super.listChildren(children); 1318 children.add(new Property("identifier", "Identifier", "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1319 children.add(new Property("status", "code", "Indicates the current state of this list.", 0, 1, status)); 1320 children.add(new Property("mode", "code", "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode)); 1321 children.add(new Property("title", "string", "A label for the list assigned by the author.", 0, 1, title)); 1322 children.add(new Property("code", "CodeableConcept", "This code defines the purpose of the list - why it was created.", 0, 1, code)); 1323 children.add(new Property("subject", "Reference(Patient|Group|Device|Location)", "The common subject (or patient) of the resources that are in the list, if there is one.", 0, 1, subject)); 1324 children.add(new Property("encounter", "Reference(Encounter)", "The encounter that is the context in which this list was created.", 0, 1, encounter)); 1325 children.add(new Property("date", "dateTime", "The date that the list was prepared.", 0, 1, date)); 1326 children.add(new Property("source", "Reference(Practitioner|Patient|Device)", "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 0, 1, source)); 1327 children.add(new Property("orderedBy", "CodeableConcept", "What order applies to the items in the list.", 0, 1, orderedBy)); 1328 children.add(new Property("note", "Annotation", "Comments that apply to the overall list.", 0, java.lang.Integer.MAX_VALUE, note)); 1329 children.add(new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry)); 1330 children.add(new Property("emptyReason", "CodeableConcept", "If the list is empty, why the list is empty.", 0, 1, emptyReason)); 1331 } 1332 1333 @Override 1334 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1335 switch (_hash) { 1336 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier for the List assigned for business purposes outside the context of FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier); 1337 case -892481550: /*status*/ return new Property("status", "code", "Indicates the current state of this list.", 0, 1, status); 1338 case 3357091: /*mode*/ return new Property("mode", "code", "How this list was prepared - whether it is a working list that is suitable for being maintained on an ongoing basis, or if it represents a snapshot of a list of items from another source, or whether it is a prepared list where items may be marked as added, modified or deleted.", 0, 1, mode); 1339 case 110371416: /*title*/ return new Property("title", "string", "A label for the list assigned by the author.", 0, 1, title); 1340 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "This code defines the purpose of the list - why it was created.", 0, 1, code); 1341 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group|Device|Location)", "The common subject (or patient) of the resources that are in the list, if there is one.", 0, 1, subject); 1342 case 1524132147: /*encounter*/ return new Property("encounter", "Reference(Encounter)", "The encounter that is the context in which this list was created.", 0, 1, encounter); 1343 case 3076014: /*date*/ return new Property("date", "dateTime", "The date that the list was prepared.", 0, 1, date); 1344 case -896505829: /*source*/ return new Property("source", "Reference(Practitioner|Patient|Device)", "The entity responsible for deciding what the contents of the list were. Where the list was created by a human, this is the same as the author of the list.", 0, 1, source); 1345 case -391079516: /*orderedBy*/ return new Property("orderedBy", "CodeableConcept", "What order applies to the items in the list.", 0, 1, orderedBy); 1346 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments that apply to the overall list.", 0, java.lang.Integer.MAX_VALUE, note); 1347 case 96667762: /*entry*/ return new Property("entry", "", "Entries in this list.", 0, java.lang.Integer.MAX_VALUE, entry); 1348 case 1140135409: /*emptyReason*/ return new Property("emptyReason", "CodeableConcept", "If the list is empty, why the list is empty.", 0, 1, emptyReason); 1349 default: return super.getNamedProperty(_hash, _name, _checkValid); 1350 } 1351 1352 } 1353 1354 @Override 1355 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1356 switch (hash) { 1357 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1358 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<ListStatus> 1359 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<ListMode> 1360 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 1361 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1362 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1363 case 1524132147: /*encounter*/ return this.encounter == null ? new Base[0] : new Base[] {this.encounter}; // Reference 1364 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 1365 case -896505829: /*source*/ return this.source == null ? new Base[0] : new Base[] {this.source}; // Reference 1366 case -391079516: /*orderedBy*/ return this.orderedBy == null ? new Base[0] : new Base[] {this.orderedBy}; // CodeableConcept 1367 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1368 case 96667762: /*entry*/ return this.entry == null ? new Base[0] : this.entry.toArray(new Base[this.entry.size()]); // ListEntryComponent 1369 case 1140135409: /*emptyReason*/ return this.emptyReason == null ? new Base[0] : new Base[] {this.emptyReason}; // CodeableConcept 1370 default: return super.getProperty(hash, name, checkValid); 1371 } 1372 1373 } 1374 1375 @Override 1376 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1377 switch (hash) { 1378 case -1618432855: // identifier 1379 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1380 return value; 1381 case -892481550: // status 1382 value = new ListStatusEnumFactory().fromType(castToCode(value)); 1383 this.status = (Enumeration) value; // Enumeration<ListStatus> 1384 return value; 1385 case 3357091: // mode 1386 value = new ListModeEnumFactory().fromType(castToCode(value)); 1387 this.mode = (Enumeration) value; // Enumeration<ListMode> 1388 return value; 1389 case 110371416: // title 1390 this.title = castToString(value); // StringType 1391 return value; 1392 case 3059181: // code 1393 this.code = castToCodeableConcept(value); // CodeableConcept 1394 return value; 1395 case -1867885268: // subject 1396 this.subject = castToReference(value); // Reference 1397 return value; 1398 case 1524132147: // encounter 1399 this.encounter = castToReference(value); // Reference 1400 return value; 1401 case 3076014: // date 1402 this.date = castToDateTime(value); // DateTimeType 1403 return value; 1404 case -896505829: // source 1405 this.source = castToReference(value); // Reference 1406 return value; 1407 case -391079516: // orderedBy 1408 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 1409 return value; 1410 case 3387378: // note 1411 this.getNote().add(castToAnnotation(value)); // Annotation 1412 return value; 1413 case 96667762: // entry 1414 this.getEntry().add((ListEntryComponent) value); // ListEntryComponent 1415 return value; 1416 case 1140135409: // emptyReason 1417 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 1418 return value; 1419 default: return super.setProperty(hash, name, value); 1420 } 1421 1422 } 1423 1424 @Override 1425 public Base setProperty(String name, Base value) throws FHIRException { 1426 if (name.equals("identifier")) { 1427 this.getIdentifier().add(castToIdentifier(value)); 1428 } else if (name.equals("status")) { 1429 value = new ListStatusEnumFactory().fromType(castToCode(value)); 1430 this.status = (Enumeration) value; // Enumeration<ListStatus> 1431 } else if (name.equals("mode")) { 1432 value = new ListModeEnumFactory().fromType(castToCode(value)); 1433 this.mode = (Enumeration) value; // Enumeration<ListMode> 1434 } else if (name.equals("title")) { 1435 this.title = castToString(value); // StringType 1436 } else if (name.equals("code")) { 1437 this.code = castToCodeableConcept(value); // CodeableConcept 1438 } else if (name.equals("subject")) { 1439 this.subject = castToReference(value); // Reference 1440 } else if (name.equals("encounter")) { 1441 this.encounter = castToReference(value); // Reference 1442 } else if (name.equals("date")) { 1443 this.date = castToDateTime(value); // DateTimeType 1444 } else if (name.equals("source")) { 1445 this.source = castToReference(value); // Reference 1446 } else if (name.equals("orderedBy")) { 1447 this.orderedBy = castToCodeableConcept(value); // CodeableConcept 1448 } else if (name.equals("note")) { 1449 this.getNote().add(castToAnnotation(value)); 1450 } else if (name.equals("entry")) { 1451 this.getEntry().add((ListEntryComponent) value); 1452 } else if (name.equals("emptyReason")) { 1453 this.emptyReason = castToCodeableConcept(value); // CodeableConcept 1454 } else 1455 return super.setProperty(name, value); 1456 return value; 1457 } 1458 1459 @Override 1460 public Base makeProperty(int hash, String name) throws FHIRException { 1461 switch (hash) { 1462 case -1618432855: return addIdentifier(); 1463 case -892481550: return getStatusElement(); 1464 case 3357091: return getModeElement(); 1465 case 110371416: return getTitleElement(); 1466 case 3059181: return getCode(); 1467 case -1867885268: return getSubject(); 1468 case 1524132147: return getEncounter(); 1469 case 3076014: return getDateElement(); 1470 case -896505829: return getSource(); 1471 case -391079516: return getOrderedBy(); 1472 case 3387378: return addNote(); 1473 case 96667762: return addEntry(); 1474 case 1140135409: return getEmptyReason(); 1475 default: return super.makeProperty(hash, name); 1476 } 1477 1478 } 1479 1480 @Override 1481 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1482 switch (hash) { 1483 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1484 case -892481550: /*status*/ return new String[] {"code"}; 1485 case 3357091: /*mode*/ return new String[] {"code"}; 1486 case 110371416: /*title*/ return new String[] {"string"}; 1487 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1488 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1489 case 1524132147: /*encounter*/ return new String[] {"Reference"}; 1490 case 3076014: /*date*/ return new String[] {"dateTime"}; 1491 case -896505829: /*source*/ return new String[] {"Reference"}; 1492 case -391079516: /*orderedBy*/ return new String[] {"CodeableConcept"}; 1493 case 3387378: /*note*/ return new String[] {"Annotation"}; 1494 case 96667762: /*entry*/ return new String[] {}; 1495 case 1140135409: /*emptyReason*/ return new String[] {"CodeableConcept"}; 1496 default: return super.getTypesForProperty(hash, name); 1497 } 1498 1499 } 1500 1501 @Override 1502 public Base addChild(String name) throws FHIRException { 1503 if (name.equals("identifier")) { 1504 return addIdentifier(); 1505 } 1506 else if (name.equals("status")) { 1507 throw new FHIRException("Cannot call addChild on a singleton property List.status"); 1508 } 1509 else if (name.equals("mode")) { 1510 throw new FHIRException("Cannot call addChild on a singleton property List.mode"); 1511 } 1512 else if (name.equals("title")) { 1513 throw new FHIRException("Cannot call addChild on a singleton property List.title"); 1514 } 1515 else if (name.equals("code")) { 1516 this.code = new CodeableConcept(); 1517 return this.code; 1518 } 1519 else if (name.equals("subject")) { 1520 this.subject = new Reference(); 1521 return this.subject; 1522 } 1523 else if (name.equals("encounter")) { 1524 this.encounter = new Reference(); 1525 return this.encounter; 1526 } 1527 else if (name.equals("date")) { 1528 throw new FHIRException("Cannot call addChild on a singleton property List.date"); 1529 } 1530 else if (name.equals("source")) { 1531 this.source = new Reference(); 1532 return this.source; 1533 } 1534 else if (name.equals("orderedBy")) { 1535 this.orderedBy = new CodeableConcept(); 1536 return this.orderedBy; 1537 } 1538 else if (name.equals("note")) { 1539 return addNote(); 1540 } 1541 else if (name.equals("entry")) { 1542 return addEntry(); 1543 } 1544 else if (name.equals("emptyReason")) { 1545 this.emptyReason = new CodeableConcept(); 1546 return this.emptyReason; 1547 } 1548 else 1549 return super.addChild(name); 1550 } 1551 1552 public String fhirType() { 1553 return "List"; 1554 1555 } 1556 1557 public ListResource copy() { 1558 ListResource dst = new ListResource(); 1559 copyValues(dst); 1560 if (identifier != null) { 1561 dst.identifier = new ArrayList<Identifier>(); 1562 for (Identifier i : identifier) 1563 dst.identifier.add(i.copy()); 1564 }; 1565 dst.status = status == null ? null : status.copy(); 1566 dst.mode = mode == null ? null : mode.copy(); 1567 dst.title = title == null ? null : title.copy(); 1568 dst.code = code == null ? null : code.copy(); 1569 dst.subject = subject == null ? null : subject.copy(); 1570 dst.encounter = encounter == null ? null : encounter.copy(); 1571 dst.date = date == null ? null : date.copy(); 1572 dst.source = source == null ? null : source.copy(); 1573 dst.orderedBy = orderedBy == null ? null : orderedBy.copy(); 1574 if (note != null) { 1575 dst.note = new ArrayList<Annotation>(); 1576 for (Annotation i : note) 1577 dst.note.add(i.copy()); 1578 }; 1579 if (entry != null) { 1580 dst.entry = new ArrayList<ListEntryComponent>(); 1581 for (ListEntryComponent i : entry) 1582 dst.entry.add(i.copy()); 1583 }; 1584 dst.emptyReason = emptyReason == null ? null : emptyReason.copy(); 1585 return dst; 1586 } 1587 1588 protected ListResource typedCopy() { 1589 return copy(); 1590 } 1591 1592 @Override 1593 public boolean equalsDeep(Base other_) { 1594 if (!super.equalsDeep(other_)) 1595 return false; 1596 if (!(other_ instanceof ListResource)) 1597 return false; 1598 ListResource o = (ListResource) other_; 1599 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(mode, o.mode, true) 1600 && compareDeep(title, o.title, true) && compareDeep(code, o.code, true) && compareDeep(subject, o.subject, true) 1601 && compareDeep(encounter, o.encounter, true) && compareDeep(date, o.date, true) && compareDeep(source, o.source, true) 1602 && compareDeep(orderedBy, o.orderedBy, true) && compareDeep(note, o.note, true) && compareDeep(entry, o.entry, true) 1603 && compareDeep(emptyReason, o.emptyReason, true); 1604 } 1605 1606 @Override 1607 public boolean equalsShallow(Base other_) { 1608 if (!super.equalsShallow(other_)) 1609 return false; 1610 if (!(other_ instanceof ListResource)) 1611 return false; 1612 ListResource o = (ListResource) other_; 1613 return compareValues(status, o.status, true) && compareValues(mode, o.mode, true) && compareValues(title, o.title, true) 1614 && compareValues(date, o.date, true); 1615 } 1616 1617 public boolean isEmpty() { 1618 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, mode 1619 , title, code, subject, encounter, date, source, orderedBy, note, entry, emptyReason 1620 ); 1621 } 1622 1623 @Override 1624 public ResourceType getResourceType() { 1625 return ResourceType.List; 1626 } 1627 1628 /** 1629 * Search parameter: <b>date</b> 1630 * <p> 1631 * Description: <b>When the list was prepared</b><br> 1632 * Type: <b>date</b><br> 1633 * Path: <b>List.date</b><br> 1634 * </p> 1635 */ 1636 @SearchParamDefinition(name="date", path="List.date", description="When the list was prepared", type="date" ) 1637 public static final String SP_DATE = "date"; 1638 /** 1639 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1640 * <p> 1641 * Description: <b>When the list was prepared</b><br> 1642 * Type: <b>date</b><br> 1643 * Path: <b>List.date</b><br> 1644 * </p> 1645 */ 1646 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1647 1648 /** 1649 * Search parameter: <b>identifier</b> 1650 * <p> 1651 * Description: <b>Business identifier</b><br> 1652 * Type: <b>token</b><br> 1653 * Path: <b>List.identifier</b><br> 1654 * </p> 1655 */ 1656 @SearchParamDefinition(name="identifier", path="List.identifier", description="Business identifier", type="token" ) 1657 public static final String SP_IDENTIFIER = "identifier"; 1658 /** 1659 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1660 * <p> 1661 * Description: <b>Business identifier</b><br> 1662 * Type: <b>token</b><br> 1663 * Path: <b>List.identifier</b><br> 1664 * </p> 1665 */ 1666 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1667 1668 /** 1669 * Search parameter: <b>item</b> 1670 * <p> 1671 * Description: <b>Actual entry</b><br> 1672 * Type: <b>reference</b><br> 1673 * Path: <b>List.entry.item</b><br> 1674 * </p> 1675 */ 1676 @SearchParamDefinition(name="item", path="List.entry.item", description="Actual entry", type="reference" ) 1677 public static final String SP_ITEM = "item"; 1678 /** 1679 * <b>Fluent Client</b> search parameter constant for <b>item</b> 1680 * <p> 1681 * Description: <b>Actual entry</b><br> 1682 * Type: <b>reference</b><br> 1683 * Path: <b>List.entry.item</b><br> 1684 * </p> 1685 */ 1686 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ITEM); 1687 1688/** 1689 * Constant for fluent queries to be used to add include statements. Specifies 1690 * the path value of "<b>List:item</b>". 1691 */ 1692 public static final ca.uhn.fhir.model.api.Include INCLUDE_ITEM = new ca.uhn.fhir.model.api.Include("List:item").toLocked(); 1693 1694 /** 1695 * Search parameter: <b>empty-reason</b> 1696 * <p> 1697 * Description: <b>Why list is empty</b><br> 1698 * Type: <b>token</b><br> 1699 * Path: <b>List.emptyReason</b><br> 1700 * </p> 1701 */ 1702 @SearchParamDefinition(name="empty-reason", path="List.emptyReason", description="Why list is empty", type="token" ) 1703 public static final String SP_EMPTY_REASON = "empty-reason"; 1704 /** 1705 * <b>Fluent Client</b> search parameter constant for <b>empty-reason</b> 1706 * <p> 1707 * Description: <b>Why list is empty</b><br> 1708 * Type: <b>token</b><br> 1709 * Path: <b>List.emptyReason</b><br> 1710 * </p> 1711 */ 1712 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EMPTY_REASON = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EMPTY_REASON); 1713 1714 /** 1715 * Search parameter: <b>code</b> 1716 * <p> 1717 * Description: <b>What the purpose of this list is</b><br> 1718 * Type: <b>token</b><br> 1719 * Path: <b>List.code</b><br> 1720 * </p> 1721 */ 1722 @SearchParamDefinition(name="code", path="List.code", description="What the purpose of this list is", type="token" ) 1723 public static final String SP_CODE = "code"; 1724 /** 1725 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1726 * <p> 1727 * Description: <b>What the purpose of this list is</b><br> 1728 * Type: <b>token</b><br> 1729 * Path: <b>List.code</b><br> 1730 * </p> 1731 */ 1732 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1733 1734 /** 1735 * Search parameter: <b>notes</b> 1736 * <p> 1737 * Description: <b>The annotation - text content</b><br> 1738 * Type: <b>string</b><br> 1739 * Path: <b>List.note.text</b><br> 1740 * </p> 1741 */ 1742 @SearchParamDefinition(name="notes", path="List.note.text", description="The annotation - text content", type="string" ) 1743 public static final String SP_NOTES = "notes"; 1744 /** 1745 * <b>Fluent Client</b> search parameter constant for <b>notes</b> 1746 * <p> 1747 * Description: <b>The annotation - text content</b><br> 1748 * Type: <b>string</b><br> 1749 * Path: <b>List.note.text</b><br> 1750 * </p> 1751 */ 1752 public static final ca.uhn.fhir.rest.gclient.StringClientParam NOTES = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NOTES); 1753 1754 /** 1755 * Search parameter: <b>subject</b> 1756 * <p> 1757 * Description: <b>If all resources have the same subject</b><br> 1758 * Type: <b>reference</b><br> 1759 * Path: <b>List.subject</b><br> 1760 * </p> 1761 */ 1762 @SearchParamDefinition(name="subject", path="List.subject", description="If all resources have the same subject", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient") }, target={Device.class, Group.class, Location.class, Patient.class } ) 1763 public static final String SP_SUBJECT = "subject"; 1764 /** 1765 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1766 * <p> 1767 * Description: <b>If all resources have the same subject</b><br> 1768 * Type: <b>reference</b><br> 1769 * Path: <b>List.subject</b><br> 1770 * </p> 1771 */ 1772 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1773 1774/** 1775 * Constant for fluent queries to be used to add include statements. Specifies 1776 * the path value of "<b>List:subject</b>". 1777 */ 1778 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("List:subject").toLocked(); 1779 1780 /** 1781 * Search parameter: <b>patient</b> 1782 * <p> 1783 * Description: <b>If all resources have the same subject</b><br> 1784 * Type: <b>reference</b><br> 1785 * Path: <b>List.subject</b><br> 1786 * </p> 1787 */ 1788 @SearchParamDefinition(name="patient", path="List.subject", description="If all resources have the same subject", type="reference", target={Patient.class } ) 1789 public static final String SP_PATIENT = "patient"; 1790 /** 1791 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1792 * <p> 1793 * Description: <b>If all resources have the same subject</b><br> 1794 * Type: <b>reference</b><br> 1795 * Path: <b>List.subject</b><br> 1796 * </p> 1797 */ 1798 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1799 1800/** 1801 * Constant for fluent queries to be used to add include statements. Specifies 1802 * the path value of "<b>List:patient</b>". 1803 */ 1804 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("List:patient").toLocked(); 1805 1806 /** 1807 * Search parameter: <b>source</b> 1808 * <p> 1809 * Description: <b>Who and/or what defined the list contents (aka Author)</b><br> 1810 * Type: <b>reference</b><br> 1811 * Path: <b>List.source</b><br> 1812 * </p> 1813 */ 1814 @SearchParamDefinition(name="source", path="List.source", description="Who and/or what defined the list contents (aka Author)", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Base FHIR compartment definition for Practitioner") }, target={Device.class, Patient.class, Practitioner.class } ) 1815 public static final String SP_SOURCE = "source"; 1816 /** 1817 * <b>Fluent Client</b> search parameter constant for <b>source</b> 1818 * <p> 1819 * Description: <b>Who and/or what defined the list contents (aka Author)</b><br> 1820 * Type: <b>reference</b><br> 1821 * Path: <b>List.source</b><br> 1822 * </p> 1823 */ 1824 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 1825 1826/** 1827 * Constant for fluent queries to be used to add include statements. Specifies 1828 * the path value of "<b>List:source</b>". 1829 */ 1830 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("List:source").toLocked(); 1831 1832 /** 1833 * Search parameter: <b>encounter</b> 1834 * <p> 1835 * Description: <b>Context in which list created</b><br> 1836 * Type: <b>reference</b><br> 1837 * Path: <b>List.encounter</b><br> 1838 * </p> 1839 */ 1840 @SearchParamDefinition(name="encounter", path="List.encounter", description="Context in which list created", type="reference", target={Encounter.class } ) 1841 public static final String SP_ENCOUNTER = "encounter"; 1842 /** 1843 * <b>Fluent Client</b> search parameter constant for <b>encounter</b> 1844 * <p> 1845 * Description: <b>Context in which list created</b><br> 1846 * Type: <b>reference</b><br> 1847 * Path: <b>List.encounter</b><br> 1848 * </p> 1849 */ 1850 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENCOUNTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENCOUNTER); 1851 1852/** 1853 * Constant for fluent queries to be used to add include statements. Specifies 1854 * the path value of "<b>List:encounter</b>". 1855 */ 1856 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENCOUNTER = new ca.uhn.fhir.model.api.Include("List:encounter").toLocked(); 1857 1858 /** 1859 * Search parameter: <b>title</b> 1860 * <p> 1861 * Description: <b>Descriptive name for the list</b><br> 1862 * Type: <b>string</b><br> 1863 * Path: <b>List.title</b><br> 1864 * </p> 1865 */ 1866 @SearchParamDefinition(name="title", path="List.title", description="Descriptive name for the list", type="string" ) 1867 public static final String SP_TITLE = "title"; 1868 /** 1869 * <b>Fluent Client</b> search parameter constant for <b>title</b> 1870 * <p> 1871 * Description: <b>Descriptive name for the list</b><br> 1872 * Type: <b>string</b><br> 1873 * Path: <b>List.title</b><br> 1874 * </p> 1875 */ 1876 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 1877 1878 /** 1879 * Search parameter: <b>status</b> 1880 * <p> 1881 * Description: <b>current | retired | entered-in-error</b><br> 1882 * Type: <b>token</b><br> 1883 * Path: <b>List.status</b><br> 1884 * </p> 1885 */ 1886 @SearchParamDefinition(name="status", path="List.status", description="current | retired | entered-in-error", type="token" ) 1887 public static final String SP_STATUS = "status"; 1888 /** 1889 * <b>Fluent Client</b> search parameter constant for <b>status</b> 1890 * <p> 1891 * Description: <b>current | retired | entered-in-error</b><br> 1892 * Type: <b>token</b><br> 1893 * Path: <b>List.status</b><br> 1894 * </p> 1895 */ 1896 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 1897 1898 1899}