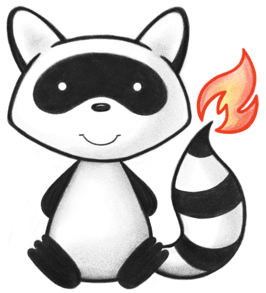
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006import java.math.BigDecimal; 007 008/* 009 Copyright (c) 2011+, HL7, Inc. 010 All rights reserved. 011 012 Redistribution and use in source and binary forms, with or without modification, 013 are permitted provided that the following conditions are met: 014 015 * Redistributions of source code must retain the above copyright notice, this 016 list of conditions and the following disclaimer. 017 * Redistributions in binary form must reproduce the above copyright notice, 018 this list of conditions and the following disclaimer in the documentation 019 and/or other materials provided with the distribution. 020 * Neither the name of HL7 nor the names of its contributors may be used to 021 endorse or promote products derived from this software without specific 022 prior written permission. 023 024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 025 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 027 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 028 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 029 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 030 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 031 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 032 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 033 POSSIBILITY OF SUCH DAMAGE. 034 035*/ 036 037// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 038import java.util.ArrayList; 039import java.util.List; 040 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * Details and position information for a physical place where services are provided and resources and participants may be stored, found, contained or accommodated. 052 */ 053@ResourceDef(name="Location", profile="http://hl7.org/fhir/Profile/Location") 054public class Location extends DomainResource { 055 056 public enum LocationStatus { 057 /** 058 * The location is operational. 059 */ 060 ACTIVE, 061 /** 062 * The location is temporarily closed. 063 */ 064 SUSPENDED, 065 /** 066 * The location is no longer used. 067 */ 068 INACTIVE, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static LocationStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("active".equals(codeString)) 077 return ACTIVE; 078 if ("suspended".equals(codeString)) 079 return SUSPENDED; 080 if ("inactive".equals(codeString)) 081 return INACTIVE; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown LocationStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case ACTIVE: return "active"; 090 case SUSPENDED: return "suspended"; 091 case INACTIVE: return "inactive"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case ACTIVE: return "http://hl7.org/fhir/location-status"; 099 case SUSPENDED: return "http://hl7.org/fhir/location-status"; 100 case INACTIVE: return "http://hl7.org/fhir/location-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case ACTIVE: return "The location is operational."; 108 case SUSPENDED: return "The location is temporarily closed."; 109 case INACTIVE: return "The location is no longer used."; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case ACTIVE: return "Active"; 117 case SUSPENDED: return "Suspended"; 118 case INACTIVE: return "Inactive"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class LocationStatusEnumFactory implements EnumFactory<LocationStatus> { 126 public LocationStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("active".equals(codeString)) 131 return LocationStatus.ACTIVE; 132 if ("suspended".equals(codeString)) 133 return LocationStatus.SUSPENDED; 134 if ("inactive".equals(codeString)) 135 return LocationStatus.INACTIVE; 136 throw new IllegalArgumentException("Unknown LocationStatus code '"+codeString+"'"); 137 } 138 public Enumeration<LocationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<LocationStatus>(this); 143 String codeString = code.asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("active".equals(codeString)) 147 return new Enumeration<LocationStatus>(this, LocationStatus.ACTIVE); 148 if ("suspended".equals(codeString)) 149 return new Enumeration<LocationStatus>(this, LocationStatus.SUSPENDED); 150 if ("inactive".equals(codeString)) 151 return new Enumeration<LocationStatus>(this, LocationStatus.INACTIVE); 152 throw new FHIRException("Unknown LocationStatus code '"+codeString+"'"); 153 } 154 public String toCode(LocationStatus code) { 155 if (code == LocationStatus.NULL) 156 return null; 157 if (code == LocationStatus.ACTIVE) 158 return "active"; 159 if (code == LocationStatus.SUSPENDED) 160 return "suspended"; 161 if (code == LocationStatus.INACTIVE) 162 return "inactive"; 163 return "?"; 164 } 165 public String toSystem(LocationStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum LocationMode { 171 /** 172 * The Location resource represents a specific instance of a location (e.g. Operating Theatre 1A). 173 */ 174 INSTANCE, 175 /** 176 * The Location represents a class of locations (e.g. Any Operating Theatre) although this class of locations could be constrained within a specific boundary (such as organization, or parent location, address etc.). 177 */ 178 KIND, 179 /** 180 * added to help the parsers with the generic types 181 */ 182 NULL; 183 public static LocationMode fromCode(String codeString) throws FHIRException { 184 if (codeString == null || "".equals(codeString)) 185 return null; 186 if ("instance".equals(codeString)) 187 return INSTANCE; 188 if ("kind".equals(codeString)) 189 return KIND; 190 if (Configuration.isAcceptInvalidEnums()) 191 return null; 192 else 193 throw new FHIRException("Unknown LocationMode code '"+codeString+"'"); 194 } 195 public String toCode() { 196 switch (this) { 197 case INSTANCE: return "instance"; 198 case KIND: return "kind"; 199 case NULL: return null; 200 default: return "?"; 201 } 202 } 203 public String getSystem() { 204 switch (this) { 205 case INSTANCE: return "http://hl7.org/fhir/location-mode"; 206 case KIND: return "http://hl7.org/fhir/location-mode"; 207 case NULL: return null; 208 default: return "?"; 209 } 210 } 211 public String getDefinition() { 212 switch (this) { 213 case INSTANCE: return "The Location resource represents a specific instance of a location (e.g. Operating Theatre 1A)."; 214 case KIND: return "The Location represents a class of locations (e.g. Any Operating Theatre) although this class of locations could be constrained within a specific boundary (such as organization, or parent location, address etc.)."; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 public String getDisplay() { 220 switch (this) { 221 case INSTANCE: return "Instance"; 222 case KIND: return "Kind"; 223 case NULL: return null; 224 default: return "?"; 225 } 226 } 227 } 228 229 public static class LocationModeEnumFactory implements EnumFactory<LocationMode> { 230 public LocationMode fromCode(String codeString) throws IllegalArgumentException { 231 if (codeString == null || "".equals(codeString)) 232 if (codeString == null || "".equals(codeString)) 233 return null; 234 if ("instance".equals(codeString)) 235 return LocationMode.INSTANCE; 236 if ("kind".equals(codeString)) 237 return LocationMode.KIND; 238 throw new IllegalArgumentException("Unknown LocationMode code '"+codeString+"'"); 239 } 240 public Enumeration<LocationMode> fromType(PrimitiveType<?> code) throws FHIRException { 241 if (code == null) 242 return null; 243 if (code.isEmpty()) 244 return new Enumeration<LocationMode>(this); 245 String codeString = code.asStringValue(); 246 if (codeString == null || "".equals(codeString)) 247 return null; 248 if ("instance".equals(codeString)) 249 return new Enumeration<LocationMode>(this, LocationMode.INSTANCE); 250 if ("kind".equals(codeString)) 251 return new Enumeration<LocationMode>(this, LocationMode.KIND); 252 throw new FHIRException("Unknown LocationMode code '"+codeString+"'"); 253 } 254 public String toCode(LocationMode code) { 255 if (code == LocationMode.NULL) 256 return null; 257 if (code == LocationMode.INSTANCE) 258 return "instance"; 259 if (code == LocationMode.KIND) 260 return "kind"; 261 return "?"; 262 } 263 public String toSystem(LocationMode code) { 264 return code.getSystem(); 265 } 266 } 267 268 @Block() 269 public static class LocationPositionComponent extends BackboneElement implements IBaseBackboneElement { 270 /** 271 * Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below). 272 */ 273 @Child(name = "longitude", type = {DecimalType.class}, order=1, min=1, max=1, modifier=false, summary=false) 274 @Description(shortDefinition="Longitude with WGS84 datum", formalDefinition="Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below)." ) 275 protected DecimalType longitude; 276 277 /** 278 * Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below). 279 */ 280 @Child(name = "latitude", type = {DecimalType.class}, order=2, min=1, max=1, modifier=false, summary=false) 281 @Description(shortDefinition="Latitude with WGS84 datum", formalDefinition="Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below)." ) 282 protected DecimalType latitude; 283 284 /** 285 * Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below). 286 */ 287 @Child(name = "altitude", type = {DecimalType.class}, order=3, min=0, max=1, modifier=false, summary=false) 288 @Description(shortDefinition="Altitude with WGS84 datum", formalDefinition="Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below)." ) 289 protected DecimalType altitude; 290 291 private static final long serialVersionUID = -74276134L; 292 293 /** 294 * Constructor 295 */ 296 public LocationPositionComponent() { 297 super(); 298 } 299 300 /** 301 * Constructor 302 */ 303 public LocationPositionComponent(DecimalType longitude, DecimalType latitude) { 304 super(); 305 this.longitude = longitude; 306 this.latitude = latitude; 307 } 308 309 /** 310 * @return {@link #longitude} (Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).). This is the underlying object with id, value and extensions. The accessor "getLongitude" gives direct access to the value 311 */ 312 public DecimalType getLongitudeElement() { 313 if (this.longitude == null) 314 if (Configuration.errorOnAutoCreate()) 315 throw new Error("Attempt to auto-create LocationPositionComponent.longitude"); 316 else if (Configuration.doAutoCreate()) 317 this.longitude = new DecimalType(); // bb 318 return this.longitude; 319 } 320 321 public boolean hasLongitudeElement() { 322 return this.longitude != null && !this.longitude.isEmpty(); 323 } 324 325 public boolean hasLongitude() { 326 return this.longitude != null && !this.longitude.isEmpty(); 327 } 328 329 /** 330 * @param value {@link #longitude} (Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).). This is the underlying object with id, value and extensions. The accessor "getLongitude" gives direct access to the value 331 */ 332 public LocationPositionComponent setLongitudeElement(DecimalType value) { 333 this.longitude = value; 334 return this; 335 } 336 337 /** 338 * @return Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below). 339 */ 340 public BigDecimal getLongitude() { 341 return this.longitude == null ? null : this.longitude.getValue(); 342 } 343 344 /** 345 * @param value Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below). 346 */ 347 public LocationPositionComponent setLongitude(BigDecimal value) { 348 if (this.longitude == null) 349 this.longitude = new DecimalType(); 350 this.longitude.setValue(value); 351 return this; 352 } 353 354 /** 355 * @param value Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below). 356 */ 357 public LocationPositionComponent setLongitude(long value) { 358 this.longitude = new DecimalType(); 359 this.longitude.setValue(value); 360 return this; 361 } 362 363 /** 364 * @param value Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below). 365 */ 366 public LocationPositionComponent setLongitude(double value) { 367 this.longitude = new DecimalType(); 368 this.longitude.setValue(value); 369 return this; 370 } 371 372 /** 373 * @return {@link #latitude} (Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).). This is the underlying object with id, value and extensions. The accessor "getLatitude" gives direct access to the value 374 */ 375 public DecimalType getLatitudeElement() { 376 if (this.latitude == null) 377 if (Configuration.errorOnAutoCreate()) 378 throw new Error("Attempt to auto-create LocationPositionComponent.latitude"); 379 else if (Configuration.doAutoCreate()) 380 this.latitude = new DecimalType(); // bb 381 return this.latitude; 382 } 383 384 public boolean hasLatitudeElement() { 385 return this.latitude != null && !this.latitude.isEmpty(); 386 } 387 388 public boolean hasLatitude() { 389 return this.latitude != null && !this.latitude.isEmpty(); 390 } 391 392 /** 393 * @param value {@link #latitude} (Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).). This is the underlying object with id, value and extensions. The accessor "getLatitude" gives direct access to the value 394 */ 395 public LocationPositionComponent setLatitudeElement(DecimalType value) { 396 this.latitude = value; 397 return this; 398 } 399 400 /** 401 * @return Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below). 402 */ 403 public BigDecimal getLatitude() { 404 return this.latitude == null ? null : this.latitude.getValue(); 405 } 406 407 /** 408 * @param value Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below). 409 */ 410 public LocationPositionComponent setLatitude(BigDecimal value) { 411 if (this.latitude == null) 412 this.latitude = new DecimalType(); 413 this.latitude.setValue(value); 414 return this; 415 } 416 417 /** 418 * @param value Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below). 419 */ 420 public LocationPositionComponent setLatitude(long value) { 421 this.latitude = new DecimalType(); 422 this.latitude.setValue(value); 423 return this; 424 } 425 426 /** 427 * @param value Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below). 428 */ 429 public LocationPositionComponent setLatitude(double value) { 430 this.latitude = new DecimalType(); 431 this.latitude.setValue(value); 432 return this; 433 } 434 435 /** 436 * @return {@link #altitude} (Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).). This is the underlying object with id, value and extensions. The accessor "getAltitude" gives direct access to the value 437 */ 438 public DecimalType getAltitudeElement() { 439 if (this.altitude == null) 440 if (Configuration.errorOnAutoCreate()) 441 throw new Error("Attempt to auto-create LocationPositionComponent.altitude"); 442 else if (Configuration.doAutoCreate()) 443 this.altitude = new DecimalType(); // bb 444 return this.altitude; 445 } 446 447 public boolean hasAltitudeElement() { 448 return this.altitude != null && !this.altitude.isEmpty(); 449 } 450 451 public boolean hasAltitude() { 452 return this.altitude != null && !this.altitude.isEmpty(); 453 } 454 455 /** 456 * @param value {@link #altitude} (Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).). This is the underlying object with id, value and extensions. The accessor "getAltitude" gives direct access to the value 457 */ 458 public LocationPositionComponent setAltitudeElement(DecimalType value) { 459 this.altitude = value; 460 return this; 461 } 462 463 /** 464 * @return Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below). 465 */ 466 public BigDecimal getAltitude() { 467 return this.altitude == null ? null : this.altitude.getValue(); 468 } 469 470 /** 471 * @param value Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below). 472 */ 473 public LocationPositionComponent setAltitude(BigDecimal value) { 474 if (value == null) 475 this.altitude = null; 476 else { 477 if (this.altitude == null) 478 this.altitude = new DecimalType(); 479 this.altitude.setValue(value); 480 } 481 return this; 482 } 483 484 /** 485 * @param value Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below). 486 */ 487 public LocationPositionComponent setAltitude(long value) { 488 this.altitude = new DecimalType(); 489 this.altitude.setValue(value); 490 return this; 491 } 492 493 /** 494 * @param value Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below). 495 */ 496 public LocationPositionComponent setAltitude(double value) { 497 this.altitude = new DecimalType(); 498 this.altitude.setValue(value); 499 return this; 500 } 501 502 protected void listChildren(List<Property> children) { 503 super.listChildren(children); 504 children.add(new Property("longitude", "decimal", "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).", 0, 1, longitude)); 505 children.add(new Property("latitude", "decimal", "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).", 0, 1, latitude)); 506 children.add(new Property("altitude", "decimal", "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).", 0, 1, altitude)); 507 } 508 509 @Override 510 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 511 switch (_hash) { 512 case 137365935: /*longitude*/ return new Property("longitude", "decimal", "Longitude. The value domain and the interpretation are the same as for the text of the longitude element in KML (see notes below).", 0, 1, longitude); 513 case -1439978388: /*latitude*/ return new Property("latitude", "decimal", "Latitude. The value domain and the interpretation are the same as for the text of the latitude element in KML (see notes below).", 0, 1, latitude); 514 case 2036550306: /*altitude*/ return new Property("altitude", "decimal", "Altitude. The value domain and the interpretation are the same as for the text of the altitude element in KML (see notes below).", 0, 1, altitude); 515 default: return super.getNamedProperty(_hash, _name, _checkValid); 516 } 517 518 } 519 520 @Override 521 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 522 switch (hash) { 523 case 137365935: /*longitude*/ return this.longitude == null ? new Base[0] : new Base[] {this.longitude}; // DecimalType 524 case -1439978388: /*latitude*/ return this.latitude == null ? new Base[0] : new Base[] {this.latitude}; // DecimalType 525 case 2036550306: /*altitude*/ return this.altitude == null ? new Base[0] : new Base[] {this.altitude}; // DecimalType 526 default: return super.getProperty(hash, name, checkValid); 527 } 528 529 } 530 531 @Override 532 public Base setProperty(int hash, String name, Base value) throws FHIRException { 533 switch (hash) { 534 case 137365935: // longitude 535 this.longitude = castToDecimal(value); // DecimalType 536 return value; 537 case -1439978388: // latitude 538 this.latitude = castToDecimal(value); // DecimalType 539 return value; 540 case 2036550306: // altitude 541 this.altitude = castToDecimal(value); // DecimalType 542 return value; 543 default: return super.setProperty(hash, name, value); 544 } 545 546 } 547 548 @Override 549 public Base setProperty(String name, Base value) throws FHIRException { 550 if (name.equals("longitude")) { 551 this.longitude = castToDecimal(value); // DecimalType 552 } else if (name.equals("latitude")) { 553 this.latitude = castToDecimal(value); // DecimalType 554 } else if (name.equals("altitude")) { 555 this.altitude = castToDecimal(value); // DecimalType 556 } else 557 return super.setProperty(name, value); 558 return value; 559 } 560 561 @Override 562 public Base makeProperty(int hash, String name) throws FHIRException { 563 switch (hash) { 564 case 137365935: return getLongitudeElement(); 565 case -1439978388: return getLatitudeElement(); 566 case 2036550306: return getAltitudeElement(); 567 default: return super.makeProperty(hash, name); 568 } 569 570 } 571 572 @Override 573 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 574 switch (hash) { 575 case 137365935: /*longitude*/ return new String[] {"decimal"}; 576 case -1439978388: /*latitude*/ return new String[] {"decimal"}; 577 case 2036550306: /*altitude*/ return new String[] {"decimal"}; 578 default: return super.getTypesForProperty(hash, name); 579 } 580 581 } 582 583 @Override 584 public Base addChild(String name) throws FHIRException { 585 if (name.equals("longitude")) { 586 throw new FHIRException("Cannot call addChild on a singleton property Location.longitude"); 587 } 588 else if (name.equals("latitude")) { 589 throw new FHIRException("Cannot call addChild on a singleton property Location.latitude"); 590 } 591 else if (name.equals("altitude")) { 592 throw new FHIRException("Cannot call addChild on a singleton property Location.altitude"); 593 } 594 else 595 return super.addChild(name); 596 } 597 598 public LocationPositionComponent copy() { 599 LocationPositionComponent dst = new LocationPositionComponent(); 600 copyValues(dst); 601 dst.longitude = longitude == null ? null : longitude.copy(); 602 dst.latitude = latitude == null ? null : latitude.copy(); 603 dst.altitude = altitude == null ? null : altitude.copy(); 604 return dst; 605 } 606 607 @Override 608 public boolean equalsDeep(Base other_) { 609 if (!super.equalsDeep(other_)) 610 return false; 611 if (!(other_ instanceof LocationPositionComponent)) 612 return false; 613 LocationPositionComponent o = (LocationPositionComponent) other_; 614 return compareDeep(longitude, o.longitude, true) && compareDeep(latitude, o.latitude, true) && compareDeep(altitude, o.altitude, true) 615 ; 616 } 617 618 @Override 619 public boolean equalsShallow(Base other_) { 620 if (!super.equalsShallow(other_)) 621 return false; 622 if (!(other_ instanceof LocationPositionComponent)) 623 return false; 624 LocationPositionComponent o = (LocationPositionComponent) other_; 625 return compareValues(longitude, o.longitude, true) && compareValues(latitude, o.latitude, true) && compareValues(altitude, o.altitude, true) 626 ; 627 } 628 629 public boolean isEmpty() { 630 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(longitude, latitude, altitude 631 ); 632 } 633 634 public String fhirType() { 635 return "Location.position"; 636 637 } 638 639 } 640 641 /** 642 * Unique code or number identifying the location to its users. 643 */ 644 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 645 @Description(shortDefinition="Unique code or number identifying the location to its users", formalDefinition="Unique code or number identifying the location to its users." ) 646 protected List<Identifier> identifier; 647 648 /** 649 * The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location. 650 */ 651 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=true, summary=true) 652 @Description(shortDefinition="active | suspended | inactive", formalDefinition="The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location." ) 653 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-status") 654 protected Enumeration<LocationStatus> status; 655 656 /** 657 * The Operational status covers operation values most relevant to beds (but can also apply to rooms/units/chair/etc such as an isolation unit/dialisys chair). This typically covers concepts such as contamination, housekeeping and other activities like maintenance. 658 */ 659 @Child(name = "operationalStatus", type = {Coding.class}, order=2, min=0, max=1, modifier=false, summary=true) 660 @Description(shortDefinition="The Operational status of the location (typically only for a bed/room)", formalDefinition="The Operational status covers operation values most relevant to beds (but can also apply to rooms/units/chair/etc such as an isolation unit/dialisys chair). This typically covers concepts such as contamination, housekeeping and other activities like maintenance." ) 661 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v2-0116") 662 protected Coding operationalStatus; 663 664 /** 665 * Name of the location as used by humans. Does not need to be unique. 666 */ 667 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=true) 668 @Description(shortDefinition="Name of the location as used by humans", formalDefinition="Name of the location as used by humans. Does not need to be unique." ) 669 protected StringType name; 670 671 /** 672 * A list of alternate names that the location is known as, or was known as in the past. 673 */ 674 @Child(name = "alias", type = {StringType.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 675 @Description(shortDefinition="A list of alternate names that the location is known as, or was known as in the past", formalDefinition="A list of alternate names that the location is known as, or was known as in the past." ) 676 protected List<StringType> alias; 677 678 /** 679 * Description of the Location, which helps in finding or referencing the place. 680 */ 681 @Child(name = "description", type = {StringType.class}, order=5, min=0, max=1, modifier=false, summary=true) 682 @Description(shortDefinition="Additional details about the location that could be displayed as further information to identify the location beyond its name", formalDefinition="Description of the Location, which helps in finding or referencing the place." ) 683 protected StringType description; 684 685 /** 686 * Indicates whether a resource instance represents a specific location or a class of locations. 687 */ 688 @Child(name = "mode", type = {CodeType.class}, order=6, min=0, max=1, modifier=true, summary=true) 689 @Description(shortDefinition="instance | kind", formalDefinition="Indicates whether a resource instance represents a specific location or a class of locations." ) 690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-mode") 691 protected Enumeration<LocationMode> mode; 692 693 /** 694 * Indicates the type of function performed at the location. 695 */ 696 @Child(name = "type", type = {CodeableConcept.class}, order=7, min=0, max=1, modifier=false, summary=true) 697 @Description(shortDefinition="Type of function performed", formalDefinition="Indicates the type of function performed at the location." ) 698 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ServiceDeliveryLocationRoleType") 699 protected CodeableConcept type; 700 701 /** 702 * The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites. 703 */ 704 @Child(name = "telecom", type = {ContactPoint.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 705 @Description(shortDefinition="Contact details of the location", formalDefinition="The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites." ) 706 protected List<ContactPoint> telecom; 707 708 /** 709 * Physical location. 710 */ 711 @Child(name = "address", type = {Address.class}, order=9, min=0, max=1, modifier=false, summary=false) 712 @Description(shortDefinition="Physical location", formalDefinition="Physical location." ) 713 protected Address address; 714 715 /** 716 * Physical form of the location, e.g. building, room, vehicle, road. 717 */ 718 @Child(name = "physicalType", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 719 @Description(shortDefinition="Physical form of the location", formalDefinition="Physical form of the location, e.g. building, room, vehicle, road." ) 720 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/location-physical-type") 721 protected CodeableConcept physicalType; 722 723 /** 724 * The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML). 725 */ 726 @Child(name = "position", type = {}, order=11, min=0, max=1, modifier=false, summary=false) 727 @Description(shortDefinition="The absolute geographic location", formalDefinition="The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML)." ) 728 protected LocationPositionComponent position; 729 730 /** 731 * The organization responsible for the provisioning and upkeep of the location. 732 */ 733 @Child(name = "managingOrganization", type = {Organization.class}, order=12, min=0, max=1, modifier=false, summary=true) 734 @Description(shortDefinition="Organization responsible for provisioning and upkeep", formalDefinition="The organization responsible for the provisioning and upkeep of the location." ) 735 protected Reference managingOrganization; 736 737 /** 738 * The actual object that is the target of the reference (The organization responsible for the provisioning and upkeep of the location.) 739 */ 740 protected Organization managingOrganizationTarget; 741 742 /** 743 * Another Location which this Location is physically part of. 744 */ 745 @Child(name = "partOf", type = {Location.class}, order=13, min=0, max=1, modifier=false, summary=false) 746 @Description(shortDefinition="Another Location this one is physically part of", formalDefinition="Another Location which this Location is physically part of." ) 747 protected Reference partOf; 748 749 /** 750 * The actual object that is the target of the reference (Another Location which this Location is physically part of.) 751 */ 752 protected Location partOfTarget; 753 754 /** 755 * Technical endpoints providing access to services operated for the location. 756 */ 757 @Child(name = "endpoint", type = {Endpoint.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 758 @Description(shortDefinition="Technical endpoints providing access to services operated for the location", formalDefinition="Technical endpoints providing access to services operated for the location." ) 759 protected List<Reference> endpoint; 760 /** 761 * The actual objects that are the target of the reference (Technical endpoints providing access to services operated for the location.) 762 */ 763 protected List<Endpoint> endpointTarget; 764 765 766 private static final long serialVersionUID = -1603579027L; 767 768 /** 769 * Constructor 770 */ 771 public Location() { 772 super(); 773 } 774 775 /** 776 * @return {@link #identifier} (Unique code or number identifying the location to its users.) 777 */ 778 public List<Identifier> getIdentifier() { 779 if (this.identifier == null) 780 this.identifier = new ArrayList<Identifier>(); 781 return this.identifier; 782 } 783 784 /** 785 * @return Returns a reference to <code>this</code> for easy method chaining 786 */ 787 public Location setIdentifier(List<Identifier> theIdentifier) { 788 this.identifier = theIdentifier; 789 return this; 790 } 791 792 public boolean hasIdentifier() { 793 if (this.identifier == null) 794 return false; 795 for (Identifier item : this.identifier) 796 if (!item.isEmpty()) 797 return true; 798 return false; 799 } 800 801 public Identifier addIdentifier() { //3 802 Identifier t = new Identifier(); 803 if (this.identifier == null) 804 this.identifier = new ArrayList<Identifier>(); 805 this.identifier.add(t); 806 return t; 807 } 808 809 public Location addIdentifier(Identifier t) { //3 810 if (t == null) 811 return this; 812 if (this.identifier == null) 813 this.identifier = new ArrayList<Identifier>(); 814 this.identifier.add(t); 815 return this; 816 } 817 818 /** 819 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 820 */ 821 public Identifier getIdentifierFirstRep() { 822 if (getIdentifier().isEmpty()) { 823 addIdentifier(); 824 } 825 return getIdentifier().get(0); 826 } 827 828 /** 829 * @return {@link #status} (The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 830 */ 831 public Enumeration<LocationStatus> getStatusElement() { 832 if (this.status == null) 833 if (Configuration.errorOnAutoCreate()) 834 throw new Error("Attempt to auto-create Location.status"); 835 else if (Configuration.doAutoCreate()) 836 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); // bb 837 return this.status; 838 } 839 840 public boolean hasStatusElement() { 841 return this.status != null && !this.status.isEmpty(); 842 } 843 844 public boolean hasStatus() { 845 return this.status != null && !this.status.isEmpty(); 846 } 847 848 /** 849 * @param value {@link #status} (The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 850 */ 851 public Location setStatusElement(Enumeration<LocationStatus> value) { 852 this.status = value; 853 return this; 854 } 855 856 /** 857 * @return The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location. 858 */ 859 public LocationStatus getStatus() { 860 return this.status == null ? null : this.status.getValue(); 861 } 862 863 /** 864 * @param value The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location. 865 */ 866 public Location setStatus(LocationStatus value) { 867 if (value == null) 868 this.status = null; 869 else { 870 if (this.status == null) 871 this.status = new Enumeration<LocationStatus>(new LocationStatusEnumFactory()); 872 this.status.setValue(value); 873 } 874 return this; 875 } 876 877 /** 878 * @return {@link #operationalStatus} (The Operational status covers operation values most relevant to beds (but can also apply to rooms/units/chair/etc such as an isolation unit/dialisys chair). This typically covers concepts such as contamination, housekeeping and other activities like maintenance.) 879 */ 880 public Coding getOperationalStatus() { 881 if (this.operationalStatus == null) 882 if (Configuration.errorOnAutoCreate()) 883 throw new Error("Attempt to auto-create Location.operationalStatus"); 884 else if (Configuration.doAutoCreate()) 885 this.operationalStatus = new Coding(); // cc 886 return this.operationalStatus; 887 } 888 889 public boolean hasOperationalStatus() { 890 return this.operationalStatus != null && !this.operationalStatus.isEmpty(); 891 } 892 893 /** 894 * @param value {@link #operationalStatus} (The Operational status covers operation values most relevant to beds (but can also apply to rooms/units/chair/etc such as an isolation unit/dialisys chair). This typically covers concepts such as contamination, housekeeping and other activities like maintenance.) 895 */ 896 public Location setOperationalStatus(Coding value) { 897 this.operationalStatus = value; 898 return this; 899 } 900 901 /** 902 * @return {@link #name} (Name of the location as used by humans. Does not need to be unique.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 903 */ 904 public StringType getNameElement() { 905 if (this.name == null) 906 if (Configuration.errorOnAutoCreate()) 907 throw new Error("Attempt to auto-create Location.name"); 908 else if (Configuration.doAutoCreate()) 909 this.name = new StringType(); // bb 910 return this.name; 911 } 912 913 public boolean hasNameElement() { 914 return this.name != null && !this.name.isEmpty(); 915 } 916 917 public boolean hasName() { 918 return this.name != null && !this.name.isEmpty(); 919 } 920 921 /** 922 * @param value {@link #name} (Name of the location as used by humans. Does not need to be unique.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 923 */ 924 public Location setNameElement(StringType value) { 925 this.name = value; 926 return this; 927 } 928 929 /** 930 * @return Name of the location as used by humans. Does not need to be unique. 931 */ 932 public String getName() { 933 return this.name == null ? null : this.name.getValue(); 934 } 935 936 /** 937 * @param value Name of the location as used by humans. Does not need to be unique. 938 */ 939 public Location setName(String value) { 940 if (Utilities.noString(value)) 941 this.name = null; 942 else { 943 if (this.name == null) 944 this.name = new StringType(); 945 this.name.setValue(value); 946 } 947 return this; 948 } 949 950 /** 951 * @return {@link #alias} (A list of alternate names that the location is known as, or was known as in the past.) 952 */ 953 public List<StringType> getAlias() { 954 if (this.alias == null) 955 this.alias = new ArrayList<StringType>(); 956 return this.alias; 957 } 958 959 /** 960 * @return Returns a reference to <code>this</code> for easy method chaining 961 */ 962 public Location setAlias(List<StringType> theAlias) { 963 this.alias = theAlias; 964 return this; 965 } 966 967 public boolean hasAlias() { 968 if (this.alias == null) 969 return false; 970 for (StringType item : this.alias) 971 if (!item.isEmpty()) 972 return true; 973 return false; 974 } 975 976 /** 977 * @return {@link #alias} (A list of alternate names that the location is known as, or was known as in the past.) 978 */ 979 public StringType addAliasElement() {//2 980 StringType t = new StringType(); 981 if (this.alias == null) 982 this.alias = new ArrayList<StringType>(); 983 this.alias.add(t); 984 return t; 985 } 986 987 /** 988 * @param value {@link #alias} (A list of alternate names that the location is known as, or was known as in the past.) 989 */ 990 public Location addAlias(String value) { //1 991 StringType t = new StringType(); 992 t.setValue(value); 993 if (this.alias == null) 994 this.alias = new ArrayList<StringType>(); 995 this.alias.add(t); 996 return this; 997 } 998 999 /** 1000 * @param value {@link #alias} (A list of alternate names that the location is known as, or was known as in the past.) 1001 */ 1002 public boolean hasAlias(String value) { 1003 if (this.alias == null) 1004 return false; 1005 for (StringType v : this.alias) 1006 if (v.getValue().equals(value)) // string 1007 return true; 1008 return false; 1009 } 1010 1011 /** 1012 * @return {@link #description} (Description of the Location, which helps in finding or referencing the place.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1013 */ 1014 public StringType getDescriptionElement() { 1015 if (this.description == null) 1016 if (Configuration.errorOnAutoCreate()) 1017 throw new Error("Attempt to auto-create Location.description"); 1018 else if (Configuration.doAutoCreate()) 1019 this.description = new StringType(); // bb 1020 return this.description; 1021 } 1022 1023 public boolean hasDescriptionElement() { 1024 return this.description != null && !this.description.isEmpty(); 1025 } 1026 1027 public boolean hasDescription() { 1028 return this.description != null && !this.description.isEmpty(); 1029 } 1030 1031 /** 1032 * @param value {@link #description} (Description of the Location, which helps in finding or referencing the place.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1033 */ 1034 public Location setDescriptionElement(StringType value) { 1035 this.description = value; 1036 return this; 1037 } 1038 1039 /** 1040 * @return Description of the Location, which helps in finding or referencing the place. 1041 */ 1042 public String getDescription() { 1043 return this.description == null ? null : this.description.getValue(); 1044 } 1045 1046 /** 1047 * @param value Description of the Location, which helps in finding or referencing the place. 1048 */ 1049 public Location setDescription(String value) { 1050 if (Utilities.noString(value)) 1051 this.description = null; 1052 else { 1053 if (this.description == null) 1054 this.description = new StringType(); 1055 this.description.setValue(value); 1056 } 1057 return this; 1058 } 1059 1060 /** 1061 * @return {@link #mode} (Indicates whether a resource instance represents a specific location or a class of locations.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1062 */ 1063 public Enumeration<LocationMode> getModeElement() { 1064 if (this.mode == null) 1065 if (Configuration.errorOnAutoCreate()) 1066 throw new Error("Attempt to auto-create Location.mode"); 1067 else if (Configuration.doAutoCreate()) 1068 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); // bb 1069 return this.mode; 1070 } 1071 1072 public boolean hasModeElement() { 1073 return this.mode != null && !this.mode.isEmpty(); 1074 } 1075 1076 public boolean hasMode() { 1077 return this.mode != null && !this.mode.isEmpty(); 1078 } 1079 1080 /** 1081 * @param value {@link #mode} (Indicates whether a resource instance represents a specific location or a class of locations.). This is the underlying object with id, value and extensions. The accessor "getMode" gives direct access to the value 1082 */ 1083 public Location setModeElement(Enumeration<LocationMode> value) { 1084 this.mode = value; 1085 return this; 1086 } 1087 1088 /** 1089 * @return Indicates whether a resource instance represents a specific location or a class of locations. 1090 */ 1091 public LocationMode getMode() { 1092 return this.mode == null ? null : this.mode.getValue(); 1093 } 1094 1095 /** 1096 * @param value Indicates whether a resource instance represents a specific location or a class of locations. 1097 */ 1098 public Location setMode(LocationMode value) { 1099 if (value == null) 1100 this.mode = null; 1101 else { 1102 if (this.mode == null) 1103 this.mode = new Enumeration<LocationMode>(new LocationModeEnumFactory()); 1104 this.mode.setValue(value); 1105 } 1106 return this; 1107 } 1108 1109 /** 1110 * @return {@link #type} (Indicates the type of function performed at the location.) 1111 */ 1112 public CodeableConcept getType() { 1113 if (this.type == null) 1114 if (Configuration.errorOnAutoCreate()) 1115 throw new Error("Attempt to auto-create Location.type"); 1116 else if (Configuration.doAutoCreate()) 1117 this.type = new CodeableConcept(); // cc 1118 return this.type; 1119 } 1120 1121 public boolean hasType() { 1122 return this.type != null && !this.type.isEmpty(); 1123 } 1124 1125 /** 1126 * @param value {@link #type} (Indicates the type of function performed at the location.) 1127 */ 1128 public Location setType(CodeableConcept value) { 1129 this.type = value; 1130 return this; 1131 } 1132 1133 /** 1134 * @return {@link #telecom} (The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.) 1135 */ 1136 public List<ContactPoint> getTelecom() { 1137 if (this.telecom == null) 1138 this.telecom = new ArrayList<ContactPoint>(); 1139 return this.telecom; 1140 } 1141 1142 /** 1143 * @return Returns a reference to <code>this</code> for easy method chaining 1144 */ 1145 public Location setTelecom(List<ContactPoint> theTelecom) { 1146 this.telecom = theTelecom; 1147 return this; 1148 } 1149 1150 public boolean hasTelecom() { 1151 if (this.telecom == null) 1152 return false; 1153 for (ContactPoint item : this.telecom) 1154 if (!item.isEmpty()) 1155 return true; 1156 return false; 1157 } 1158 1159 public ContactPoint addTelecom() { //3 1160 ContactPoint t = new ContactPoint(); 1161 if (this.telecom == null) 1162 this.telecom = new ArrayList<ContactPoint>(); 1163 this.telecom.add(t); 1164 return t; 1165 } 1166 1167 public Location addTelecom(ContactPoint t) { //3 1168 if (t == null) 1169 return this; 1170 if (this.telecom == null) 1171 this.telecom = new ArrayList<ContactPoint>(); 1172 this.telecom.add(t); 1173 return this; 1174 } 1175 1176 /** 1177 * @return The first repetition of repeating field {@link #telecom}, creating it if it does not already exist 1178 */ 1179 public ContactPoint getTelecomFirstRep() { 1180 if (getTelecom().isEmpty()) { 1181 addTelecom(); 1182 } 1183 return getTelecom().get(0); 1184 } 1185 1186 /** 1187 * @return {@link #address} (Physical location.) 1188 */ 1189 public Address getAddress() { 1190 if (this.address == null) 1191 if (Configuration.errorOnAutoCreate()) 1192 throw new Error("Attempt to auto-create Location.address"); 1193 else if (Configuration.doAutoCreate()) 1194 this.address = new Address(); // cc 1195 return this.address; 1196 } 1197 1198 public boolean hasAddress() { 1199 return this.address != null && !this.address.isEmpty(); 1200 } 1201 1202 /** 1203 * @param value {@link #address} (Physical location.) 1204 */ 1205 public Location setAddress(Address value) { 1206 this.address = value; 1207 return this; 1208 } 1209 1210 /** 1211 * @return {@link #physicalType} (Physical form of the location, e.g. building, room, vehicle, road.) 1212 */ 1213 public CodeableConcept getPhysicalType() { 1214 if (this.physicalType == null) 1215 if (Configuration.errorOnAutoCreate()) 1216 throw new Error("Attempt to auto-create Location.physicalType"); 1217 else if (Configuration.doAutoCreate()) 1218 this.physicalType = new CodeableConcept(); // cc 1219 return this.physicalType; 1220 } 1221 1222 public boolean hasPhysicalType() { 1223 return this.physicalType != null && !this.physicalType.isEmpty(); 1224 } 1225 1226 /** 1227 * @param value {@link #physicalType} (Physical form of the location, e.g. building, room, vehicle, road.) 1228 */ 1229 public Location setPhysicalType(CodeableConcept value) { 1230 this.physicalType = value; 1231 return this; 1232 } 1233 1234 /** 1235 * @return {@link #position} (The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).) 1236 */ 1237 public LocationPositionComponent getPosition() { 1238 if (this.position == null) 1239 if (Configuration.errorOnAutoCreate()) 1240 throw new Error("Attempt to auto-create Location.position"); 1241 else if (Configuration.doAutoCreate()) 1242 this.position = new LocationPositionComponent(); // cc 1243 return this.position; 1244 } 1245 1246 public boolean hasPosition() { 1247 return this.position != null && !this.position.isEmpty(); 1248 } 1249 1250 /** 1251 * @param value {@link #position} (The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).) 1252 */ 1253 public Location setPosition(LocationPositionComponent value) { 1254 this.position = value; 1255 return this; 1256 } 1257 1258 /** 1259 * @return {@link #managingOrganization} (The organization responsible for the provisioning and upkeep of the location.) 1260 */ 1261 public Reference getManagingOrganization() { 1262 if (this.managingOrganization == null) 1263 if (Configuration.errorOnAutoCreate()) 1264 throw new Error("Attempt to auto-create Location.managingOrganization"); 1265 else if (Configuration.doAutoCreate()) 1266 this.managingOrganization = new Reference(); // cc 1267 return this.managingOrganization; 1268 } 1269 1270 public boolean hasManagingOrganization() { 1271 return this.managingOrganization != null && !this.managingOrganization.isEmpty(); 1272 } 1273 1274 /** 1275 * @param value {@link #managingOrganization} (The organization responsible for the provisioning and upkeep of the location.) 1276 */ 1277 public Location setManagingOrganization(Reference value) { 1278 this.managingOrganization = value; 1279 return this; 1280 } 1281 1282 /** 1283 * @return {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization responsible for the provisioning and upkeep of the location.) 1284 */ 1285 public Organization getManagingOrganizationTarget() { 1286 if (this.managingOrganizationTarget == null) 1287 if (Configuration.errorOnAutoCreate()) 1288 throw new Error("Attempt to auto-create Location.managingOrganization"); 1289 else if (Configuration.doAutoCreate()) 1290 this.managingOrganizationTarget = new Organization(); // aa 1291 return this.managingOrganizationTarget; 1292 } 1293 1294 /** 1295 * @param value {@link #managingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization responsible for the provisioning and upkeep of the location.) 1296 */ 1297 public Location setManagingOrganizationTarget(Organization value) { 1298 this.managingOrganizationTarget = value; 1299 return this; 1300 } 1301 1302 /** 1303 * @return {@link #partOf} (Another Location which this Location is physically part of.) 1304 */ 1305 public Reference getPartOf() { 1306 if (this.partOf == null) 1307 if (Configuration.errorOnAutoCreate()) 1308 throw new Error("Attempt to auto-create Location.partOf"); 1309 else if (Configuration.doAutoCreate()) 1310 this.partOf = new Reference(); // cc 1311 return this.partOf; 1312 } 1313 1314 public boolean hasPartOf() { 1315 return this.partOf != null && !this.partOf.isEmpty(); 1316 } 1317 1318 /** 1319 * @param value {@link #partOf} (Another Location which this Location is physically part of.) 1320 */ 1321 public Location setPartOf(Reference value) { 1322 this.partOf = value; 1323 return this; 1324 } 1325 1326 /** 1327 * @return {@link #partOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Another Location which this Location is physically part of.) 1328 */ 1329 public Location getPartOfTarget() { 1330 if (this.partOfTarget == null) 1331 if (Configuration.errorOnAutoCreate()) 1332 throw new Error("Attempt to auto-create Location.partOf"); 1333 else if (Configuration.doAutoCreate()) 1334 this.partOfTarget = new Location(); // aa 1335 return this.partOfTarget; 1336 } 1337 1338 /** 1339 * @param value {@link #partOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Another Location which this Location is physically part of.) 1340 */ 1341 public Location setPartOfTarget(Location value) { 1342 this.partOfTarget = value; 1343 return this; 1344 } 1345 1346 /** 1347 * @return {@link #endpoint} (Technical endpoints providing access to services operated for the location.) 1348 */ 1349 public List<Reference> getEndpoint() { 1350 if (this.endpoint == null) 1351 this.endpoint = new ArrayList<Reference>(); 1352 return this.endpoint; 1353 } 1354 1355 /** 1356 * @return Returns a reference to <code>this</code> for easy method chaining 1357 */ 1358 public Location setEndpoint(List<Reference> theEndpoint) { 1359 this.endpoint = theEndpoint; 1360 return this; 1361 } 1362 1363 public boolean hasEndpoint() { 1364 if (this.endpoint == null) 1365 return false; 1366 for (Reference item : this.endpoint) 1367 if (!item.isEmpty()) 1368 return true; 1369 return false; 1370 } 1371 1372 public Reference addEndpoint() { //3 1373 Reference t = new Reference(); 1374 if (this.endpoint == null) 1375 this.endpoint = new ArrayList<Reference>(); 1376 this.endpoint.add(t); 1377 return t; 1378 } 1379 1380 public Location addEndpoint(Reference t) { //3 1381 if (t == null) 1382 return this; 1383 if (this.endpoint == null) 1384 this.endpoint = new ArrayList<Reference>(); 1385 this.endpoint.add(t); 1386 return this; 1387 } 1388 1389 /** 1390 * @return The first repetition of repeating field {@link #endpoint}, creating it if it does not already exist 1391 */ 1392 public Reference getEndpointFirstRep() { 1393 if (getEndpoint().isEmpty()) { 1394 addEndpoint(); 1395 } 1396 return getEndpoint().get(0); 1397 } 1398 1399 /** 1400 * @deprecated Use Reference#setResource(IBaseResource) instead 1401 */ 1402 @Deprecated 1403 public List<Endpoint> getEndpointTarget() { 1404 if (this.endpointTarget == null) 1405 this.endpointTarget = new ArrayList<Endpoint>(); 1406 return this.endpointTarget; 1407 } 1408 1409 /** 1410 * @deprecated Use Reference#setResource(IBaseResource) instead 1411 */ 1412 @Deprecated 1413 public Endpoint addEndpointTarget() { 1414 Endpoint r = new Endpoint(); 1415 if (this.endpointTarget == null) 1416 this.endpointTarget = new ArrayList<Endpoint>(); 1417 this.endpointTarget.add(r); 1418 return r; 1419 } 1420 1421 protected void listChildren(List<Property> children) { 1422 super.listChildren(children); 1423 children.add(new Property("identifier", "Identifier", "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1424 children.add(new Property("status", "code", "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.", 0, 1, status)); 1425 children.add(new Property("operationalStatus", "Coding", "The Operational status covers operation values most relevant to beds (but can also apply to rooms/units/chair/etc such as an isolation unit/dialisys chair). This typically covers concepts such as contamination, housekeeping and other activities like maintenance.", 0, 1, operationalStatus)); 1426 children.add(new Property("name", "string", "Name of the location as used by humans. Does not need to be unique.", 0, 1, name)); 1427 children.add(new Property("alias", "string", "A list of alternate names that the location is known as, or was known as in the past.", 0, java.lang.Integer.MAX_VALUE, alias)); 1428 children.add(new Property("description", "string", "Description of the Location, which helps in finding or referencing the place.", 0, 1, description)); 1429 children.add(new Property("mode", "code", "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1, mode)); 1430 children.add(new Property("type", "CodeableConcept", "Indicates the type of function performed at the location.", 0, 1, type)); 1431 children.add(new Property("telecom", "ContactPoint", "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, telecom)); 1432 children.add(new Property("address", "Address", "Physical location.", 0, 1, address)); 1433 children.add(new Property("physicalType", "CodeableConcept", "Physical form of the location, e.g. building, room, vehicle, road.", 0, 1, physicalType)); 1434 children.add(new Property("position", "", "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 0, 1, position)); 1435 children.add(new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the provisioning and upkeep of the location.", 0, 1, managingOrganization)); 1436 children.add(new Property("partOf", "Reference(Location)", "Another Location which this Location is physically part of.", 0, 1, partOf)); 1437 children.add(new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the location.", 0, java.lang.Integer.MAX_VALUE, endpoint)); 1438 } 1439 1440 @Override 1441 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1442 switch (_hash) { 1443 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Unique code or number identifying the location to its users.", 0, java.lang.Integer.MAX_VALUE, identifier); 1444 case -892481550: /*status*/ return new Property("status", "code", "The status property covers the general availability of the resource, not the current value which may be covered by the operationStatus, or by a schedule/slots if they are configured for the location.", 0, 1, status); 1445 case -2103166364: /*operationalStatus*/ return new Property("operationalStatus", "Coding", "The Operational status covers operation values most relevant to beds (but can also apply to rooms/units/chair/etc such as an isolation unit/dialisys chair). This typically covers concepts such as contamination, housekeeping and other activities like maintenance.", 0, 1, operationalStatus); 1446 case 3373707: /*name*/ return new Property("name", "string", "Name of the location as used by humans. Does not need to be unique.", 0, 1, name); 1447 case 92902992: /*alias*/ return new Property("alias", "string", "A list of alternate names that the location is known as, or was known as in the past.", 0, java.lang.Integer.MAX_VALUE, alias); 1448 case -1724546052: /*description*/ return new Property("description", "string", "Description of the Location, which helps in finding or referencing the place.", 0, 1, description); 1449 case 3357091: /*mode*/ return new Property("mode", "code", "Indicates whether a resource instance represents a specific location or a class of locations.", 0, 1, mode); 1450 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates the type of function performed at the location.", 0, 1, type); 1451 case -1429363305: /*telecom*/ return new Property("telecom", "ContactPoint", "The contact details of communication devices available at the location. This can include phone numbers, fax numbers, mobile numbers, email addresses and web sites.", 0, java.lang.Integer.MAX_VALUE, telecom); 1452 case -1147692044: /*address*/ return new Property("address", "Address", "Physical location.", 0, 1, address); 1453 case -1474715471: /*physicalType*/ return new Property("physicalType", "CodeableConcept", "Physical form of the location, e.g. building, room, vehicle, road.", 0, 1, physicalType); 1454 case 747804969: /*position*/ return new Property("position", "", "The absolute geographic location of the Location, expressed using the WGS84 datum (This is the same co-ordinate system used in KML).", 0, 1, position); 1455 case -2058947787: /*managingOrganization*/ return new Property("managingOrganization", "Reference(Organization)", "The organization responsible for the provisioning and upkeep of the location.", 0, 1, managingOrganization); 1456 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Location)", "Another Location which this Location is physically part of.", 0, 1, partOf); 1457 case 1741102485: /*endpoint*/ return new Property("endpoint", "Reference(Endpoint)", "Technical endpoints providing access to services operated for the location.", 0, java.lang.Integer.MAX_VALUE, endpoint); 1458 default: return super.getNamedProperty(_hash, _name, _checkValid); 1459 } 1460 1461 } 1462 1463 @Override 1464 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1465 switch (hash) { 1466 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1467 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<LocationStatus> 1468 case -2103166364: /*operationalStatus*/ return this.operationalStatus == null ? new Base[0] : new Base[] {this.operationalStatus}; // Coding 1469 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 1470 case 92902992: /*alias*/ return this.alias == null ? new Base[0] : this.alias.toArray(new Base[this.alias.size()]); // StringType 1471 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 1472 case 3357091: /*mode*/ return this.mode == null ? new Base[0] : new Base[] {this.mode}; // Enumeration<LocationMode> 1473 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 1474 case -1429363305: /*telecom*/ return this.telecom == null ? new Base[0] : this.telecom.toArray(new Base[this.telecom.size()]); // ContactPoint 1475 case -1147692044: /*address*/ return this.address == null ? new Base[0] : new Base[] {this.address}; // Address 1476 case -1474715471: /*physicalType*/ return this.physicalType == null ? new Base[0] : new Base[] {this.physicalType}; // CodeableConcept 1477 case 747804969: /*position*/ return this.position == null ? new Base[0] : new Base[] {this.position}; // LocationPositionComponent 1478 case -2058947787: /*managingOrganization*/ return this.managingOrganization == null ? new Base[0] : new Base[] {this.managingOrganization}; // Reference 1479 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : new Base[] {this.partOf}; // Reference 1480 case 1741102485: /*endpoint*/ return this.endpoint == null ? new Base[0] : this.endpoint.toArray(new Base[this.endpoint.size()]); // Reference 1481 default: return super.getProperty(hash, name, checkValid); 1482 } 1483 1484 } 1485 1486 @Override 1487 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1488 switch (hash) { 1489 case -1618432855: // identifier 1490 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1491 return value; 1492 case -892481550: // status 1493 value = new LocationStatusEnumFactory().fromType(castToCode(value)); 1494 this.status = (Enumeration) value; // Enumeration<LocationStatus> 1495 return value; 1496 case -2103166364: // operationalStatus 1497 this.operationalStatus = castToCoding(value); // Coding 1498 return value; 1499 case 3373707: // name 1500 this.name = castToString(value); // StringType 1501 return value; 1502 case 92902992: // alias 1503 this.getAlias().add(castToString(value)); // StringType 1504 return value; 1505 case -1724546052: // description 1506 this.description = castToString(value); // StringType 1507 return value; 1508 case 3357091: // mode 1509 value = new LocationModeEnumFactory().fromType(castToCode(value)); 1510 this.mode = (Enumeration) value; // Enumeration<LocationMode> 1511 return value; 1512 case 3575610: // type 1513 this.type = castToCodeableConcept(value); // CodeableConcept 1514 return value; 1515 case -1429363305: // telecom 1516 this.getTelecom().add(castToContactPoint(value)); // ContactPoint 1517 return value; 1518 case -1147692044: // address 1519 this.address = castToAddress(value); // Address 1520 return value; 1521 case -1474715471: // physicalType 1522 this.physicalType = castToCodeableConcept(value); // CodeableConcept 1523 return value; 1524 case 747804969: // position 1525 this.position = (LocationPositionComponent) value; // LocationPositionComponent 1526 return value; 1527 case -2058947787: // managingOrganization 1528 this.managingOrganization = castToReference(value); // Reference 1529 return value; 1530 case -995410646: // partOf 1531 this.partOf = castToReference(value); // Reference 1532 return value; 1533 case 1741102485: // endpoint 1534 this.getEndpoint().add(castToReference(value)); // Reference 1535 return value; 1536 default: return super.setProperty(hash, name, value); 1537 } 1538 1539 } 1540 1541 @Override 1542 public Base setProperty(String name, Base value) throws FHIRException { 1543 if (name.equals("identifier")) { 1544 this.getIdentifier().add(castToIdentifier(value)); 1545 } else if (name.equals("status")) { 1546 value = new LocationStatusEnumFactory().fromType(castToCode(value)); 1547 this.status = (Enumeration) value; // Enumeration<LocationStatus> 1548 } else if (name.equals("operationalStatus")) { 1549 this.operationalStatus = castToCoding(value); // Coding 1550 } else if (name.equals("name")) { 1551 this.name = castToString(value); // StringType 1552 } else if (name.equals("alias")) { 1553 this.getAlias().add(castToString(value)); 1554 } else if (name.equals("description")) { 1555 this.description = castToString(value); // StringType 1556 } else if (name.equals("mode")) { 1557 value = new LocationModeEnumFactory().fromType(castToCode(value)); 1558 this.mode = (Enumeration) value; // Enumeration<LocationMode> 1559 } else if (name.equals("type")) { 1560 this.type = castToCodeableConcept(value); // CodeableConcept 1561 } else if (name.equals("telecom")) { 1562 this.getTelecom().add(castToContactPoint(value)); 1563 } else if (name.equals("address")) { 1564 this.address = castToAddress(value); // Address 1565 } else if (name.equals("physicalType")) { 1566 this.physicalType = castToCodeableConcept(value); // CodeableConcept 1567 } else if (name.equals("position")) { 1568 this.position = (LocationPositionComponent) value; // LocationPositionComponent 1569 } else if (name.equals("managingOrganization")) { 1570 this.managingOrganization = castToReference(value); // Reference 1571 } else if (name.equals("partOf")) { 1572 this.partOf = castToReference(value); // Reference 1573 } else if (name.equals("endpoint")) { 1574 this.getEndpoint().add(castToReference(value)); 1575 } else 1576 return super.setProperty(name, value); 1577 return value; 1578 } 1579 1580 @Override 1581 public Base makeProperty(int hash, String name) throws FHIRException { 1582 switch (hash) { 1583 case -1618432855: return addIdentifier(); 1584 case -892481550: return getStatusElement(); 1585 case -2103166364: return getOperationalStatus(); 1586 case 3373707: return getNameElement(); 1587 case 92902992: return addAliasElement(); 1588 case -1724546052: return getDescriptionElement(); 1589 case 3357091: return getModeElement(); 1590 case 3575610: return getType(); 1591 case -1429363305: return addTelecom(); 1592 case -1147692044: return getAddress(); 1593 case -1474715471: return getPhysicalType(); 1594 case 747804969: return getPosition(); 1595 case -2058947787: return getManagingOrganization(); 1596 case -995410646: return getPartOf(); 1597 case 1741102485: return addEndpoint(); 1598 default: return super.makeProperty(hash, name); 1599 } 1600 1601 } 1602 1603 @Override 1604 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1605 switch (hash) { 1606 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1607 case -892481550: /*status*/ return new String[] {"code"}; 1608 case -2103166364: /*operationalStatus*/ return new String[] {"Coding"}; 1609 case 3373707: /*name*/ return new String[] {"string"}; 1610 case 92902992: /*alias*/ return new String[] {"string"}; 1611 case -1724546052: /*description*/ return new String[] {"string"}; 1612 case 3357091: /*mode*/ return new String[] {"code"}; 1613 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 1614 case -1429363305: /*telecom*/ return new String[] {"ContactPoint"}; 1615 case -1147692044: /*address*/ return new String[] {"Address"}; 1616 case -1474715471: /*physicalType*/ return new String[] {"CodeableConcept"}; 1617 case 747804969: /*position*/ return new String[] {}; 1618 case -2058947787: /*managingOrganization*/ return new String[] {"Reference"}; 1619 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1620 case 1741102485: /*endpoint*/ return new String[] {"Reference"}; 1621 default: return super.getTypesForProperty(hash, name); 1622 } 1623 1624 } 1625 1626 @Override 1627 public Base addChild(String name) throws FHIRException { 1628 if (name.equals("identifier")) { 1629 return addIdentifier(); 1630 } 1631 else if (name.equals("status")) { 1632 throw new FHIRException("Cannot call addChild on a singleton property Location.status"); 1633 } 1634 else if (name.equals("operationalStatus")) { 1635 this.operationalStatus = new Coding(); 1636 return this.operationalStatus; 1637 } 1638 else if (name.equals("name")) { 1639 throw new FHIRException("Cannot call addChild on a singleton property Location.name"); 1640 } 1641 else if (name.equals("alias")) { 1642 throw new FHIRException("Cannot call addChild on a singleton property Location.alias"); 1643 } 1644 else if (name.equals("description")) { 1645 throw new FHIRException("Cannot call addChild on a singleton property Location.description"); 1646 } 1647 else if (name.equals("mode")) { 1648 throw new FHIRException("Cannot call addChild on a singleton property Location.mode"); 1649 } 1650 else if (name.equals("type")) { 1651 this.type = new CodeableConcept(); 1652 return this.type; 1653 } 1654 else if (name.equals("telecom")) { 1655 return addTelecom(); 1656 } 1657 else if (name.equals("address")) { 1658 this.address = new Address(); 1659 return this.address; 1660 } 1661 else if (name.equals("physicalType")) { 1662 this.physicalType = new CodeableConcept(); 1663 return this.physicalType; 1664 } 1665 else if (name.equals("position")) { 1666 this.position = new LocationPositionComponent(); 1667 return this.position; 1668 } 1669 else if (name.equals("managingOrganization")) { 1670 this.managingOrganization = new Reference(); 1671 return this.managingOrganization; 1672 } 1673 else if (name.equals("partOf")) { 1674 this.partOf = new Reference(); 1675 return this.partOf; 1676 } 1677 else if (name.equals("endpoint")) { 1678 return addEndpoint(); 1679 } 1680 else 1681 return super.addChild(name); 1682 } 1683 1684 public String fhirType() { 1685 return "Location"; 1686 1687 } 1688 1689 public Location copy() { 1690 Location dst = new Location(); 1691 copyValues(dst); 1692 if (identifier != null) { 1693 dst.identifier = new ArrayList<Identifier>(); 1694 for (Identifier i : identifier) 1695 dst.identifier.add(i.copy()); 1696 }; 1697 dst.status = status == null ? null : status.copy(); 1698 dst.operationalStatus = operationalStatus == null ? null : operationalStatus.copy(); 1699 dst.name = name == null ? null : name.copy(); 1700 if (alias != null) { 1701 dst.alias = new ArrayList<StringType>(); 1702 for (StringType i : alias) 1703 dst.alias.add(i.copy()); 1704 }; 1705 dst.description = description == null ? null : description.copy(); 1706 dst.mode = mode == null ? null : mode.copy(); 1707 dst.type = type == null ? null : type.copy(); 1708 if (telecom != null) { 1709 dst.telecom = new ArrayList<ContactPoint>(); 1710 for (ContactPoint i : telecom) 1711 dst.telecom.add(i.copy()); 1712 }; 1713 dst.address = address == null ? null : address.copy(); 1714 dst.physicalType = physicalType == null ? null : physicalType.copy(); 1715 dst.position = position == null ? null : position.copy(); 1716 dst.managingOrganization = managingOrganization == null ? null : managingOrganization.copy(); 1717 dst.partOf = partOf == null ? null : partOf.copy(); 1718 if (endpoint != null) { 1719 dst.endpoint = new ArrayList<Reference>(); 1720 for (Reference i : endpoint) 1721 dst.endpoint.add(i.copy()); 1722 }; 1723 return dst; 1724 } 1725 1726 protected Location typedCopy() { 1727 return copy(); 1728 } 1729 1730 @Override 1731 public boolean equalsDeep(Base other_) { 1732 if (!super.equalsDeep(other_)) 1733 return false; 1734 if (!(other_ instanceof Location)) 1735 return false; 1736 Location o = (Location) other_; 1737 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(operationalStatus, o.operationalStatus, true) 1738 && compareDeep(name, o.name, true) && compareDeep(alias, o.alias, true) && compareDeep(description, o.description, true) 1739 && compareDeep(mode, o.mode, true) && compareDeep(type, o.type, true) && compareDeep(telecom, o.telecom, true) 1740 && compareDeep(address, o.address, true) && compareDeep(physicalType, o.physicalType, true) && compareDeep(position, o.position, true) 1741 && compareDeep(managingOrganization, o.managingOrganization, true) && compareDeep(partOf, o.partOf, true) 1742 && compareDeep(endpoint, o.endpoint, true); 1743 } 1744 1745 @Override 1746 public boolean equalsShallow(Base other_) { 1747 if (!super.equalsShallow(other_)) 1748 return false; 1749 if (!(other_ instanceof Location)) 1750 return false; 1751 Location o = (Location) other_; 1752 return compareValues(status, o.status, true) && compareValues(name, o.name, true) && compareValues(alias, o.alias, true) 1753 && compareValues(description, o.description, true) && compareValues(mode, o.mode, true); 1754 } 1755 1756 public boolean isEmpty() { 1757 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, operationalStatus 1758 , name, alias, description, mode, type, telecom, address, physicalType, position 1759 , managingOrganization, partOf, endpoint); 1760 } 1761 1762 @Override 1763 public ResourceType getResourceType() { 1764 return ResourceType.Location; 1765 } 1766 1767 /** 1768 * Search parameter: <b>identifier</b> 1769 * <p> 1770 * Description: <b>An identifier for the location</b><br> 1771 * Type: <b>token</b><br> 1772 * Path: <b>Location.identifier</b><br> 1773 * </p> 1774 */ 1775 @SearchParamDefinition(name="identifier", path="Location.identifier", description="An identifier for the location", type="token" ) 1776 public static final String SP_IDENTIFIER = "identifier"; 1777 /** 1778 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1779 * <p> 1780 * Description: <b>An identifier for the location</b><br> 1781 * Type: <b>token</b><br> 1782 * Path: <b>Location.identifier</b><br> 1783 * </p> 1784 */ 1785 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1786 1787 /** 1788 * Search parameter: <b>partof</b> 1789 * <p> 1790 * Description: <b>A location of which this location is a part</b><br> 1791 * Type: <b>reference</b><br> 1792 * Path: <b>Location.partOf</b><br> 1793 * </p> 1794 */ 1795 @SearchParamDefinition(name="partof", path="Location.partOf", description="A location of which this location is a part", type="reference", target={Location.class } ) 1796 public static final String SP_PARTOF = "partof"; 1797 /** 1798 * <b>Fluent Client</b> search parameter constant for <b>partof</b> 1799 * <p> 1800 * Description: <b>A location of which this location is a part</b><br> 1801 * Type: <b>reference</b><br> 1802 * Path: <b>Location.partOf</b><br> 1803 * </p> 1804 */ 1805 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PARTOF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PARTOF); 1806 1807/** 1808 * Constant for fluent queries to be used to add include statements. Specifies 1809 * the path value of "<b>Location:partof</b>". 1810 */ 1811 public static final ca.uhn.fhir.model.api.Include INCLUDE_PARTOF = new ca.uhn.fhir.model.api.Include("Location:partof").toLocked(); 1812 1813 /** 1814 * Search parameter: <b>near-distance</b> 1815 * <p> 1816 * Description: <b>A distance quantity to limit the near search to locations within a specific distance 1817 1818Requires the near parameter to also be included</b><br> 1819 * Type: <b>quantity</b><br> 1820 * Path: <b>Location.position</b><br> 1821 * </p> 1822 */ 1823 @SearchParamDefinition(name="near-distance", path="Location.position", description="A distance quantity to limit the near search to locations within a specific distance\n\nRequires the near parameter to also be included", type="quantity" ) 1824 public static final String SP_NEAR_DISTANCE = "near-distance"; 1825 /** 1826 * <b>Fluent Client</b> search parameter constant for <b>near-distance</b> 1827 * <p> 1828 * Description: <b>A distance quantity to limit the near search to locations within a specific distance 1829 1830Requires the near parameter to also be included</b><br> 1831 * Type: <b>quantity</b><br> 1832 * Path: <b>Location.position</b><br> 1833 * </p> 1834 */ 1835 public static final ca.uhn.fhir.rest.gclient.QuantityClientParam NEAR_DISTANCE = new ca.uhn.fhir.rest.gclient.QuantityClientParam(SP_NEAR_DISTANCE); 1836 1837 /** 1838 * Search parameter: <b>address</b> 1839 * <p> 1840 * Description: <b>A (part of the) address of the location</b><br> 1841 * Type: <b>string</b><br> 1842 * Path: <b>Location.address</b><br> 1843 * </p> 1844 */ 1845 @SearchParamDefinition(name="address", path="Location.address", description="A (part of the) address of the location", type="string" ) 1846 public static final String SP_ADDRESS = "address"; 1847 /** 1848 * <b>Fluent Client</b> search parameter constant for <b>address</b> 1849 * <p> 1850 * Description: <b>A (part of the) address of the location</b><br> 1851 * Type: <b>string</b><br> 1852 * Path: <b>Location.address</b><br> 1853 * </p> 1854 */ 1855 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS); 1856 1857 /** 1858 * Search parameter: <b>address-state</b> 1859 * <p> 1860 * Description: <b>A state specified in an address</b><br> 1861 * Type: <b>string</b><br> 1862 * Path: <b>Location.address.state</b><br> 1863 * </p> 1864 */ 1865 @SearchParamDefinition(name="address-state", path="Location.address.state", description="A state specified in an address", type="string" ) 1866 public static final String SP_ADDRESS_STATE = "address-state"; 1867 /** 1868 * <b>Fluent Client</b> search parameter constant for <b>address-state</b> 1869 * <p> 1870 * Description: <b>A state specified in an address</b><br> 1871 * Type: <b>string</b><br> 1872 * Path: <b>Location.address.state</b><br> 1873 * </p> 1874 */ 1875 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_STATE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_STATE); 1876 1877 /** 1878 * Search parameter: <b>operational-status</b> 1879 * <p> 1880 * Description: <b>Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)</b><br> 1881 * Type: <b>token</b><br> 1882 * Path: <b>Location.operationalStatus</b><br> 1883 * </p> 1884 */ 1885 @SearchParamDefinition(name="operational-status", path="Location.operationalStatus", description="Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)", type="token" ) 1886 public static final String SP_OPERATIONAL_STATUS = "operational-status"; 1887 /** 1888 * <b>Fluent Client</b> search parameter constant for <b>operational-status</b> 1889 * <p> 1890 * Description: <b>Searches for locations (typically bed/room) that have an operational status (e.g. contaminated, housekeeping)</b><br> 1891 * Type: <b>token</b><br> 1892 * Path: <b>Location.operationalStatus</b><br> 1893 * </p> 1894 */ 1895 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OPERATIONAL_STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OPERATIONAL_STATUS); 1896 1897 /** 1898 * Search parameter: <b>type</b> 1899 * <p> 1900 * Description: <b>A code for the type of location</b><br> 1901 * Type: <b>token</b><br> 1902 * Path: <b>Location.type</b><br> 1903 * </p> 1904 */ 1905 @SearchParamDefinition(name="type", path="Location.type", description="A code for the type of location", type="token" ) 1906 public static final String SP_TYPE = "type"; 1907 /** 1908 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1909 * <p> 1910 * Description: <b>A code for the type of location</b><br> 1911 * Type: <b>token</b><br> 1912 * Path: <b>Location.type</b><br> 1913 * </p> 1914 */ 1915 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1916 1917 /** 1918 * Search parameter: <b>address-postalcode</b> 1919 * <p> 1920 * Description: <b>A postal code specified in an address</b><br> 1921 * Type: <b>string</b><br> 1922 * Path: <b>Location.address.postalCode</b><br> 1923 * </p> 1924 */ 1925 @SearchParamDefinition(name="address-postalcode", path="Location.address.postalCode", description="A postal code specified in an address", type="string" ) 1926 public static final String SP_ADDRESS_POSTALCODE = "address-postalcode"; 1927 /** 1928 * <b>Fluent Client</b> search parameter constant for <b>address-postalcode</b> 1929 * <p> 1930 * Description: <b>A postal code specified in an address</b><br> 1931 * Type: <b>string</b><br> 1932 * Path: <b>Location.address.postalCode</b><br> 1933 * </p> 1934 */ 1935 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_POSTALCODE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_POSTALCODE); 1936 1937 /** 1938 * Search parameter: <b>address-country</b> 1939 * <p> 1940 * Description: <b>A country specified in an address</b><br> 1941 * Type: <b>string</b><br> 1942 * Path: <b>Location.address.country</b><br> 1943 * </p> 1944 */ 1945 @SearchParamDefinition(name="address-country", path="Location.address.country", description="A country specified in an address", type="string" ) 1946 public static final String SP_ADDRESS_COUNTRY = "address-country"; 1947 /** 1948 * <b>Fluent Client</b> search parameter constant for <b>address-country</b> 1949 * <p> 1950 * Description: <b>A country specified in an address</b><br> 1951 * Type: <b>string</b><br> 1952 * Path: <b>Location.address.country</b><br> 1953 * </p> 1954 */ 1955 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_COUNTRY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_COUNTRY); 1956 1957 /** 1958 * Search parameter: <b>endpoint</b> 1959 * <p> 1960 * Description: <b>Technical endpoints providing access to services operated for the location</b><br> 1961 * Type: <b>reference</b><br> 1962 * Path: <b>Location.endpoint</b><br> 1963 * </p> 1964 */ 1965 @SearchParamDefinition(name="endpoint", path="Location.endpoint", description="Technical endpoints providing access to services operated for the location", type="reference", target={Endpoint.class } ) 1966 public static final String SP_ENDPOINT = "endpoint"; 1967 /** 1968 * <b>Fluent Client</b> search parameter constant for <b>endpoint</b> 1969 * <p> 1970 * Description: <b>Technical endpoints providing access to services operated for the location</b><br> 1971 * Type: <b>reference</b><br> 1972 * Path: <b>Location.endpoint</b><br> 1973 * </p> 1974 */ 1975 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ENDPOINT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ENDPOINT); 1976 1977/** 1978 * Constant for fluent queries to be used to add include statements. Specifies 1979 * the path value of "<b>Location:endpoint</b>". 1980 */ 1981 public static final ca.uhn.fhir.model.api.Include INCLUDE_ENDPOINT = new ca.uhn.fhir.model.api.Include("Location:endpoint").toLocked(); 1982 1983 /** 1984 * Search parameter: <b>organization</b> 1985 * <p> 1986 * Description: <b>Searches for locations that are managed by the provided organization</b><br> 1987 * Type: <b>reference</b><br> 1988 * Path: <b>Location.managingOrganization</b><br> 1989 * </p> 1990 */ 1991 @SearchParamDefinition(name="organization", path="Location.managingOrganization", description="Searches for locations that are managed by the provided organization", type="reference", target={Organization.class } ) 1992 public static final String SP_ORGANIZATION = "organization"; 1993 /** 1994 * <b>Fluent Client</b> search parameter constant for <b>organization</b> 1995 * <p> 1996 * Description: <b>Searches for locations that are managed by the provided organization</b><br> 1997 * Type: <b>reference</b><br> 1998 * Path: <b>Location.managingOrganization</b><br> 1999 * </p> 2000 */ 2001 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam ORGANIZATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_ORGANIZATION); 2002 2003/** 2004 * Constant for fluent queries to be used to add include statements. Specifies 2005 * the path value of "<b>Location:organization</b>". 2006 */ 2007 public static final ca.uhn.fhir.model.api.Include INCLUDE_ORGANIZATION = new ca.uhn.fhir.model.api.Include("Location:organization").toLocked(); 2008 2009 /** 2010 * Search parameter: <b>name</b> 2011 * <p> 2012 * Description: <b>A portion of the location's name or alias</b><br> 2013 * Type: <b>string</b><br> 2014 * Path: <b>Location.name, Location.alias</b><br> 2015 * </p> 2016 */ 2017 @SearchParamDefinition(name="name", path="Location.name | Location.alias", description="A portion of the location's name or alias", type="string" ) 2018 public static final String SP_NAME = "name"; 2019 /** 2020 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2021 * <p> 2022 * Description: <b>A portion of the location's name or alias</b><br> 2023 * Type: <b>string</b><br> 2024 * Path: <b>Location.name, Location.alias</b><br> 2025 * </p> 2026 */ 2027 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2028 2029 /** 2030 * Search parameter: <b>address-use</b> 2031 * <p> 2032 * Description: <b>A use code specified in an address</b><br> 2033 * Type: <b>token</b><br> 2034 * Path: <b>Location.address.use</b><br> 2035 * </p> 2036 */ 2037 @SearchParamDefinition(name="address-use", path="Location.address.use", description="A use code specified in an address", type="token" ) 2038 public static final String SP_ADDRESS_USE = "address-use"; 2039 /** 2040 * <b>Fluent Client</b> search parameter constant for <b>address-use</b> 2041 * <p> 2042 * Description: <b>A use code specified in an address</b><br> 2043 * Type: <b>token</b><br> 2044 * Path: <b>Location.address.use</b><br> 2045 * </p> 2046 */ 2047 public static final ca.uhn.fhir.rest.gclient.TokenClientParam ADDRESS_USE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_ADDRESS_USE); 2048 2049 /** 2050 * Search parameter: <b>near</b> 2051 * <p> 2052 * Description: <b>The coordinates expressed as [latitude]:[longitude] (using the WGS84 datum, see notes) to find locations near to (servers may search using a square rather than a circle for efficiency) 2053 2054Requires the near-distance parameter to be provided also</b><br> 2055 * Type: <b>token</b><br> 2056 * Path: <b>Location.position</b><br> 2057 * </p> 2058 */ 2059 @SearchParamDefinition(name="near", path="Location.position", description="The coordinates expressed as [latitude]:[longitude] (using the WGS84 datum, see notes) to find locations near to (servers may search using a square rather than a circle for efficiency)\n\nRequires the near-distance parameter to be provided also", type="token" ) 2060 public static final String SP_NEAR = "near"; 2061 /** 2062 * <b>Fluent Client</b> search parameter constant for <b>near</b> 2063 * <p> 2064 * Description: <b>The coordinates expressed as [latitude]:[longitude] (using the WGS84 datum, see notes) to find locations near to (servers may search using a square rather than a circle for efficiency) 2065 2066Requires the near-distance parameter to be provided also</b><br> 2067 * Type: <b>token</b><br> 2068 * Path: <b>Location.position</b><br> 2069 * </p> 2070 */ 2071 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NEAR = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_NEAR); 2072 2073 /** 2074 * Search parameter: <b>address-city</b> 2075 * <p> 2076 * Description: <b>A city specified in an address</b><br> 2077 * Type: <b>string</b><br> 2078 * Path: <b>Location.address.city</b><br> 2079 * </p> 2080 */ 2081 @SearchParamDefinition(name="address-city", path="Location.address.city", description="A city specified in an address", type="string" ) 2082 public static final String SP_ADDRESS_CITY = "address-city"; 2083 /** 2084 * <b>Fluent Client</b> search parameter constant for <b>address-city</b> 2085 * <p> 2086 * Description: <b>A city specified in an address</b><br> 2087 * Type: <b>string</b><br> 2088 * Path: <b>Location.address.city</b><br> 2089 * </p> 2090 */ 2091 public static final ca.uhn.fhir.rest.gclient.StringClientParam ADDRESS_CITY = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_ADDRESS_CITY); 2092 2093 /** 2094 * Search parameter: <b>status</b> 2095 * <p> 2096 * Description: <b>Searches for locations with a specific kind of status</b><br> 2097 * Type: <b>token</b><br> 2098 * Path: <b>Location.status</b><br> 2099 * </p> 2100 */ 2101 @SearchParamDefinition(name="status", path="Location.status", description="Searches for locations with a specific kind of status", type="token" ) 2102 public static final String SP_STATUS = "status"; 2103 /** 2104 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2105 * <p> 2106 * Description: <b>Searches for locations with a specific kind of status</b><br> 2107 * Type: <b>token</b><br> 2108 * Path: <b>Location.status</b><br> 2109 * </p> 2110 */ 2111 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2112 2113 2114}