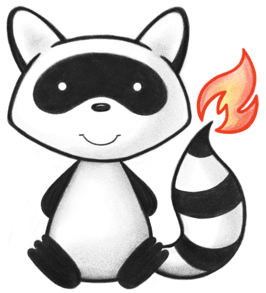
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * The Measure resource provides the definition of a quality measure. 053 */ 054@ResourceDef(name="Measure", profile="http://hl7.org/fhir/Profile/Measure") 055@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "description", "purpose", "usage", "approvalDate", "lastReviewDate", "effectivePeriod", "useContext", "jurisdiction", "topic", "contributor", "contact", "copyright", "relatedArtifact", "library", "disclaimer", "scoring", "compositeScoring", "type", "riskAdjustment", "rateAggregation", "rationale", "clinicalRecommendationStatement", "improvementNotation", "definition", "guidance", "set", "group", "supplementalData"}) 056public class Measure extends MetadataResource { 057 058 @Block() 059 public static class MeasureGroupComponent extends BackboneElement implements IBaseBackboneElement { 060 /** 061 * A unique identifier for the group. This identifier will used to report data for the group in the measure report. 062 */ 063 @Child(name = "identifier", type = {Identifier.class}, order=1, min=1, max=1, modifier=false, summary=false) 064 @Description(shortDefinition="Unique identifier", formalDefinition="A unique identifier for the group. This identifier will used to report data for the group in the measure report." ) 065 protected Identifier identifier; 066 067 /** 068 * Optional name or short description of this group. 069 */ 070 @Child(name = "name", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 071 @Description(shortDefinition="Short name", formalDefinition="Optional name or short description of this group." ) 072 protected StringType name; 073 074 /** 075 * The human readable description of this population group. 076 */ 077 @Child(name = "description", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 078 @Description(shortDefinition="Summary description", formalDefinition="The human readable description of this population group." ) 079 protected StringType description; 080 081 /** 082 * A population criteria for the measure. 083 */ 084 @Child(name = "population", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 085 @Description(shortDefinition="Population criteria", formalDefinition="A population criteria for the measure." ) 086 protected List<MeasureGroupPopulationComponent> population; 087 088 /** 089 * The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library, or a valid FHIR Resource Path. 090 */ 091 @Child(name = "stratifier", type = {}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 092 @Description(shortDefinition="Stratifier criteria for the measure", formalDefinition="The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library, or a valid FHIR Resource Path." ) 093 protected List<MeasureGroupStratifierComponent> stratifier; 094 095 private static final long serialVersionUID = 1287622059L; 096 097 /** 098 * Constructor 099 */ 100 public MeasureGroupComponent() { 101 super(); 102 } 103 104 /** 105 * Constructor 106 */ 107 public MeasureGroupComponent(Identifier identifier) { 108 super(); 109 this.identifier = identifier; 110 } 111 112 /** 113 * @return {@link #identifier} (A unique identifier for the group. This identifier will used to report data for the group in the measure report.) 114 */ 115 public Identifier getIdentifier() { 116 if (this.identifier == null) 117 if (Configuration.errorOnAutoCreate()) 118 throw new Error("Attempt to auto-create MeasureGroupComponent.identifier"); 119 else if (Configuration.doAutoCreate()) 120 this.identifier = new Identifier(); // cc 121 return this.identifier; 122 } 123 124 public boolean hasIdentifier() { 125 return this.identifier != null && !this.identifier.isEmpty(); 126 } 127 128 /** 129 * @param value {@link #identifier} (A unique identifier for the group. This identifier will used to report data for the group in the measure report.) 130 */ 131 public MeasureGroupComponent setIdentifier(Identifier value) { 132 this.identifier = value; 133 return this; 134 } 135 136 /** 137 * @return {@link #name} (Optional name or short description of this group.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 138 */ 139 public StringType getNameElement() { 140 if (this.name == null) 141 if (Configuration.errorOnAutoCreate()) 142 throw new Error("Attempt to auto-create MeasureGroupComponent.name"); 143 else if (Configuration.doAutoCreate()) 144 this.name = new StringType(); // bb 145 return this.name; 146 } 147 148 public boolean hasNameElement() { 149 return this.name != null && !this.name.isEmpty(); 150 } 151 152 public boolean hasName() { 153 return this.name != null && !this.name.isEmpty(); 154 } 155 156 /** 157 * @param value {@link #name} (Optional name or short description of this group.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 158 */ 159 public MeasureGroupComponent setNameElement(StringType value) { 160 this.name = value; 161 return this; 162 } 163 164 /** 165 * @return Optional name or short description of this group. 166 */ 167 public String getName() { 168 return this.name == null ? null : this.name.getValue(); 169 } 170 171 /** 172 * @param value Optional name or short description of this group. 173 */ 174 public MeasureGroupComponent setName(String value) { 175 if (Utilities.noString(value)) 176 this.name = null; 177 else { 178 if (this.name == null) 179 this.name = new StringType(); 180 this.name.setValue(value); 181 } 182 return this; 183 } 184 185 /** 186 * @return {@link #description} (The human readable description of this population group.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 187 */ 188 public StringType getDescriptionElement() { 189 if (this.description == null) 190 if (Configuration.errorOnAutoCreate()) 191 throw new Error("Attempt to auto-create MeasureGroupComponent.description"); 192 else if (Configuration.doAutoCreate()) 193 this.description = new StringType(); // bb 194 return this.description; 195 } 196 197 public boolean hasDescriptionElement() { 198 return this.description != null && !this.description.isEmpty(); 199 } 200 201 public boolean hasDescription() { 202 return this.description != null && !this.description.isEmpty(); 203 } 204 205 /** 206 * @param value {@link #description} (The human readable description of this population group.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 207 */ 208 public MeasureGroupComponent setDescriptionElement(StringType value) { 209 this.description = value; 210 return this; 211 } 212 213 /** 214 * @return The human readable description of this population group. 215 */ 216 public String getDescription() { 217 return this.description == null ? null : this.description.getValue(); 218 } 219 220 /** 221 * @param value The human readable description of this population group. 222 */ 223 public MeasureGroupComponent setDescription(String value) { 224 if (Utilities.noString(value)) 225 this.description = null; 226 else { 227 if (this.description == null) 228 this.description = new StringType(); 229 this.description.setValue(value); 230 } 231 return this; 232 } 233 234 /** 235 * @return {@link #population} (A population criteria for the measure.) 236 */ 237 public List<MeasureGroupPopulationComponent> getPopulation() { 238 if (this.population == null) 239 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 240 return this.population; 241 } 242 243 /** 244 * @return Returns a reference to <code>this</code> for easy method chaining 245 */ 246 public MeasureGroupComponent setPopulation(List<MeasureGroupPopulationComponent> thePopulation) { 247 this.population = thePopulation; 248 return this; 249 } 250 251 public boolean hasPopulation() { 252 if (this.population == null) 253 return false; 254 for (MeasureGroupPopulationComponent item : this.population) 255 if (!item.isEmpty()) 256 return true; 257 return false; 258 } 259 260 public MeasureGroupPopulationComponent addPopulation() { //3 261 MeasureGroupPopulationComponent t = new MeasureGroupPopulationComponent(); 262 if (this.population == null) 263 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 264 this.population.add(t); 265 return t; 266 } 267 268 public MeasureGroupComponent addPopulation(MeasureGroupPopulationComponent t) { //3 269 if (t == null) 270 return this; 271 if (this.population == null) 272 this.population = new ArrayList<MeasureGroupPopulationComponent>(); 273 this.population.add(t); 274 return this; 275 } 276 277 /** 278 * @return The first repetition of repeating field {@link #population}, creating it if it does not already exist 279 */ 280 public MeasureGroupPopulationComponent getPopulationFirstRep() { 281 if (getPopulation().isEmpty()) { 282 addPopulation(); 283 } 284 return getPopulation().get(0); 285 } 286 287 /** 288 * @return {@link #stratifier} (The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library, or a valid FHIR Resource Path.) 289 */ 290 public List<MeasureGroupStratifierComponent> getStratifier() { 291 if (this.stratifier == null) 292 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 293 return this.stratifier; 294 } 295 296 /** 297 * @return Returns a reference to <code>this</code> for easy method chaining 298 */ 299 public MeasureGroupComponent setStratifier(List<MeasureGroupStratifierComponent> theStratifier) { 300 this.stratifier = theStratifier; 301 return this; 302 } 303 304 public boolean hasStratifier() { 305 if (this.stratifier == null) 306 return false; 307 for (MeasureGroupStratifierComponent item : this.stratifier) 308 if (!item.isEmpty()) 309 return true; 310 return false; 311 } 312 313 public MeasureGroupStratifierComponent addStratifier() { //3 314 MeasureGroupStratifierComponent t = new MeasureGroupStratifierComponent(); 315 if (this.stratifier == null) 316 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 317 this.stratifier.add(t); 318 return t; 319 } 320 321 public MeasureGroupComponent addStratifier(MeasureGroupStratifierComponent t) { //3 322 if (t == null) 323 return this; 324 if (this.stratifier == null) 325 this.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 326 this.stratifier.add(t); 327 return this; 328 } 329 330 /** 331 * @return The first repetition of repeating field {@link #stratifier}, creating it if it does not already exist 332 */ 333 public MeasureGroupStratifierComponent getStratifierFirstRep() { 334 if (getStratifier().isEmpty()) { 335 addStratifier(); 336 } 337 return getStratifier().get(0); 338 } 339 340 protected void listChildren(List<Property> children) { 341 super.listChildren(children); 342 children.add(new Property("identifier", "Identifier", "A unique identifier for the group. This identifier will used to report data for the group in the measure report.", 0, 1, identifier)); 343 children.add(new Property("name", "string", "Optional name or short description of this group.", 0, 1, name)); 344 children.add(new Property("description", "string", "The human readable description of this population group.", 0, 1, description)); 345 children.add(new Property("population", "", "A population criteria for the measure.", 0, java.lang.Integer.MAX_VALUE, population)); 346 children.add(new Property("stratifier", "", "The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library, or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, stratifier)); 347 } 348 349 @Override 350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 351 switch (_hash) { 352 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier for the group. This identifier will used to report data for the group in the measure report.", 0, 1, identifier); 353 case 3373707: /*name*/ return new Property("name", "string", "Optional name or short description of this group.", 0, 1, name); 354 case -1724546052: /*description*/ return new Property("description", "string", "The human readable description of this population group.", 0, 1, description); 355 case -2023558323: /*population*/ return new Property("population", "", "A population criteria for the measure.", 0, java.lang.Integer.MAX_VALUE, population); 356 case 90983669: /*stratifier*/ return new Property("stratifier", "", "The stratifier criteria for the measure report, specified as either the name of a valid CQL expression defined within a referenced library, or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, stratifier); 357 default: return super.getNamedProperty(_hash, _name, _checkValid); 358 } 359 360 } 361 362 @Override 363 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 364 switch (hash) { 365 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 366 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 367 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 368 case -2023558323: /*population*/ return this.population == null ? new Base[0] : this.population.toArray(new Base[this.population.size()]); // MeasureGroupPopulationComponent 369 case 90983669: /*stratifier*/ return this.stratifier == null ? new Base[0] : this.stratifier.toArray(new Base[this.stratifier.size()]); // MeasureGroupStratifierComponent 370 default: return super.getProperty(hash, name, checkValid); 371 } 372 373 } 374 375 @Override 376 public Base setProperty(int hash, String name, Base value) throws FHIRException { 377 switch (hash) { 378 case -1618432855: // identifier 379 this.identifier = castToIdentifier(value); // Identifier 380 return value; 381 case 3373707: // name 382 this.name = castToString(value); // StringType 383 return value; 384 case -1724546052: // description 385 this.description = castToString(value); // StringType 386 return value; 387 case -2023558323: // population 388 this.getPopulation().add((MeasureGroupPopulationComponent) value); // MeasureGroupPopulationComponent 389 return value; 390 case 90983669: // stratifier 391 this.getStratifier().add((MeasureGroupStratifierComponent) value); // MeasureGroupStratifierComponent 392 return value; 393 default: return super.setProperty(hash, name, value); 394 } 395 396 } 397 398 @Override 399 public Base setProperty(String name, Base value) throws FHIRException { 400 if (name.equals("identifier")) { 401 this.identifier = castToIdentifier(value); // Identifier 402 } else if (name.equals("name")) { 403 this.name = castToString(value); // StringType 404 } else if (name.equals("description")) { 405 this.description = castToString(value); // StringType 406 } else if (name.equals("population")) { 407 this.getPopulation().add((MeasureGroupPopulationComponent) value); 408 } else if (name.equals("stratifier")) { 409 this.getStratifier().add((MeasureGroupStratifierComponent) value); 410 } else 411 return super.setProperty(name, value); 412 return value; 413 } 414 415 @Override 416 public Base makeProperty(int hash, String name) throws FHIRException { 417 switch (hash) { 418 case -1618432855: return getIdentifier(); 419 case 3373707: return getNameElement(); 420 case -1724546052: return getDescriptionElement(); 421 case -2023558323: return addPopulation(); 422 case 90983669: return addStratifier(); 423 default: return super.makeProperty(hash, name); 424 } 425 426 } 427 428 @Override 429 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 430 switch (hash) { 431 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 432 case 3373707: /*name*/ return new String[] {"string"}; 433 case -1724546052: /*description*/ return new String[] {"string"}; 434 case -2023558323: /*population*/ return new String[] {}; 435 case 90983669: /*stratifier*/ return new String[] {}; 436 default: return super.getTypesForProperty(hash, name); 437 } 438 439 } 440 441 @Override 442 public Base addChild(String name) throws FHIRException { 443 if (name.equals("identifier")) { 444 this.identifier = new Identifier(); 445 return this.identifier; 446 } 447 else if (name.equals("name")) { 448 throw new FHIRException("Cannot call addChild on a singleton property Measure.name"); 449 } 450 else if (name.equals("description")) { 451 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 452 } 453 else if (name.equals("population")) { 454 return addPopulation(); 455 } 456 else if (name.equals("stratifier")) { 457 return addStratifier(); 458 } 459 else 460 return super.addChild(name); 461 } 462 463 public MeasureGroupComponent copy() { 464 MeasureGroupComponent dst = new MeasureGroupComponent(); 465 copyValues(dst); 466 dst.identifier = identifier == null ? null : identifier.copy(); 467 dst.name = name == null ? null : name.copy(); 468 dst.description = description == null ? null : description.copy(); 469 if (population != null) { 470 dst.population = new ArrayList<MeasureGroupPopulationComponent>(); 471 for (MeasureGroupPopulationComponent i : population) 472 dst.population.add(i.copy()); 473 }; 474 if (stratifier != null) { 475 dst.stratifier = new ArrayList<MeasureGroupStratifierComponent>(); 476 for (MeasureGroupStratifierComponent i : stratifier) 477 dst.stratifier.add(i.copy()); 478 }; 479 return dst; 480 } 481 482 @Override 483 public boolean equalsDeep(Base other_) { 484 if (!super.equalsDeep(other_)) 485 return false; 486 if (!(other_ instanceof MeasureGroupComponent)) 487 return false; 488 MeasureGroupComponent o = (MeasureGroupComponent) other_; 489 return compareDeep(identifier, o.identifier, true) && compareDeep(name, o.name, true) && compareDeep(description, o.description, true) 490 && compareDeep(population, o.population, true) && compareDeep(stratifier, o.stratifier, true); 491 } 492 493 @Override 494 public boolean equalsShallow(Base other_) { 495 if (!super.equalsShallow(other_)) 496 return false; 497 if (!(other_ instanceof MeasureGroupComponent)) 498 return false; 499 MeasureGroupComponent o = (MeasureGroupComponent) other_; 500 return compareValues(name, o.name, true) && compareValues(description, o.description, true); 501 } 502 503 public boolean isEmpty() { 504 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, name, description 505 , population, stratifier); 506 } 507 508 public String fhirType() { 509 return "Measure.group"; 510 511 } 512 513 } 514 515 @Block() 516 public static class MeasureGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 517 /** 518 * A unique identifier for the population criteria. This identifier is used to report data against this criteria within the measure report. 519 */ 520 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=false) 521 @Description(shortDefinition="Unique identifier", formalDefinition="A unique identifier for the population criteria. This identifier is used to report data against this criteria within the measure report." ) 522 protected Identifier identifier; 523 524 /** 525 * The type of population criteria. 526 */ 527 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 528 @Description(shortDefinition="initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-observation", formalDefinition="The type of population criteria." ) 529 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-population") 530 protected CodeableConcept code; 531 532 /** 533 * Optional name or short description of this population. 534 */ 535 @Child(name = "name", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 536 @Description(shortDefinition="Short name", formalDefinition="Optional name or short description of this population." ) 537 protected StringType name; 538 539 /** 540 * The human readable description of this population criteria. 541 */ 542 @Child(name = "description", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 543 @Description(shortDefinition="The human readable description of this population criteria", formalDefinition="The human readable description of this population criteria." ) 544 protected StringType description; 545 546 /** 547 * The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria. 548 */ 549 @Child(name = "criteria", type = {StringType.class}, order=5, min=1, max=1, modifier=false, summary=false) 550 @Description(shortDefinition="The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria", formalDefinition="The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria." ) 551 protected StringType criteria; 552 553 private static final long serialVersionUID = -561575429L; 554 555 /** 556 * Constructor 557 */ 558 public MeasureGroupPopulationComponent() { 559 super(); 560 } 561 562 /** 563 * Constructor 564 */ 565 public MeasureGroupPopulationComponent(StringType criteria) { 566 super(); 567 this.criteria = criteria; 568 } 569 570 /** 571 * @return {@link #identifier} (A unique identifier for the population criteria. This identifier is used to report data against this criteria within the measure report.) 572 */ 573 public Identifier getIdentifier() { 574 if (this.identifier == null) 575 if (Configuration.errorOnAutoCreate()) 576 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.identifier"); 577 else if (Configuration.doAutoCreate()) 578 this.identifier = new Identifier(); // cc 579 return this.identifier; 580 } 581 582 public boolean hasIdentifier() { 583 return this.identifier != null && !this.identifier.isEmpty(); 584 } 585 586 /** 587 * @param value {@link #identifier} (A unique identifier for the population criteria. This identifier is used to report data against this criteria within the measure report.) 588 */ 589 public MeasureGroupPopulationComponent setIdentifier(Identifier value) { 590 this.identifier = value; 591 return this; 592 } 593 594 /** 595 * @return {@link #code} (The type of population criteria.) 596 */ 597 public CodeableConcept getCode() { 598 if (this.code == null) 599 if (Configuration.errorOnAutoCreate()) 600 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.code"); 601 else if (Configuration.doAutoCreate()) 602 this.code = new CodeableConcept(); // cc 603 return this.code; 604 } 605 606 public boolean hasCode() { 607 return this.code != null && !this.code.isEmpty(); 608 } 609 610 /** 611 * @param value {@link #code} (The type of population criteria.) 612 */ 613 public MeasureGroupPopulationComponent setCode(CodeableConcept value) { 614 this.code = value; 615 return this; 616 } 617 618 /** 619 * @return {@link #name} (Optional name or short description of this population.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 620 */ 621 public StringType getNameElement() { 622 if (this.name == null) 623 if (Configuration.errorOnAutoCreate()) 624 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.name"); 625 else if (Configuration.doAutoCreate()) 626 this.name = new StringType(); // bb 627 return this.name; 628 } 629 630 public boolean hasNameElement() { 631 return this.name != null && !this.name.isEmpty(); 632 } 633 634 public boolean hasName() { 635 return this.name != null && !this.name.isEmpty(); 636 } 637 638 /** 639 * @param value {@link #name} (Optional name or short description of this population.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 640 */ 641 public MeasureGroupPopulationComponent setNameElement(StringType value) { 642 this.name = value; 643 return this; 644 } 645 646 /** 647 * @return Optional name or short description of this population. 648 */ 649 public String getName() { 650 return this.name == null ? null : this.name.getValue(); 651 } 652 653 /** 654 * @param value Optional name or short description of this population. 655 */ 656 public MeasureGroupPopulationComponent setName(String value) { 657 if (Utilities.noString(value)) 658 this.name = null; 659 else { 660 if (this.name == null) 661 this.name = new StringType(); 662 this.name.setValue(value); 663 } 664 return this; 665 } 666 667 /** 668 * @return {@link #description} (The human readable description of this population criteria.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 669 */ 670 public StringType getDescriptionElement() { 671 if (this.description == null) 672 if (Configuration.errorOnAutoCreate()) 673 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.description"); 674 else if (Configuration.doAutoCreate()) 675 this.description = new StringType(); // bb 676 return this.description; 677 } 678 679 public boolean hasDescriptionElement() { 680 return this.description != null && !this.description.isEmpty(); 681 } 682 683 public boolean hasDescription() { 684 return this.description != null && !this.description.isEmpty(); 685 } 686 687 /** 688 * @param value {@link #description} (The human readable description of this population criteria.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 689 */ 690 public MeasureGroupPopulationComponent setDescriptionElement(StringType value) { 691 this.description = value; 692 return this; 693 } 694 695 /** 696 * @return The human readable description of this population criteria. 697 */ 698 public String getDescription() { 699 return this.description == null ? null : this.description.getValue(); 700 } 701 702 /** 703 * @param value The human readable description of this population criteria. 704 */ 705 public MeasureGroupPopulationComponent setDescription(String value) { 706 if (Utilities.noString(value)) 707 this.description = null; 708 else { 709 if (this.description == null) 710 this.description = new StringType(); 711 this.description.setValue(value); 712 } 713 return this; 714 } 715 716 /** 717 * @return {@link #criteria} (The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria.). This is the underlying object with id, value and extensions. The accessor "getCriteria" gives direct access to the value 718 */ 719 public StringType getCriteriaElement() { 720 if (this.criteria == null) 721 if (Configuration.errorOnAutoCreate()) 722 throw new Error("Attempt to auto-create MeasureGroupPopulationComponent.criteria"); 723 else if (Configuration.doAutoCreate()) 724 this.criteria = new StringType(); // bb 725 return this.criteria; 726 } 727 728 public boolean hasCriteriaElement() { 729 return this.criteria != null && !this.criteria.isEmpty(); 730 } 731 732 public boolean hasCriteria() { 733 return this.criteria != null && !this.criteria.isEmpty(); 734 } 735 736 /** 737 * @param value {@link #criteria} (The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria.). This is the underlying object with id, value and extensions. The accessor "getCriteria" gives direct access to the value 738 */ 739 public MeasureGroupPopulationComponent setCriteriaElement(StringType value) { 740 this.criteria = value; 741 return this; 742 } 743 744 /** 745 * @return The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria. 746 */ 747 public String getCriteria() { 748 return this.criteria == null ? null : this.criteria.getValue(); 749 } 750 751 /** 752 * @param value The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria. 753 */ 754 public MeasureGroupPopulationComponent setCriteria(String value) { 755 if (this.criteria == null) 756 this.criteria = new StringType(); 757 this.criteria.setValue(value); 758 return this; 759 } 760 761 protected void listChildren(List<Property> children) { 762 super.listChildren(children); 763 children.add(new Property("identifier", "Identifier", "A unique identifier for the population criteria. This identifier is used to report data against this criteria within the measure report.", 0, 1, identifier)); 764 children.add(new Property("code", "CodeableConcept", "The type of population criteria.", 0, 1, code)); 765 children.add(new Property("name", "string", "Optional name or short description of this population.", 0, 1, name)); 766 children.add(new Property("description", "string", "The human readable description of this population criteria.", 0, 1, description)); 767 children.add(new Property("criteria", "string", "The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria.", 0, 1, criteria)); 768 } 769 770 @Override 771 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 772 switch (_hash) { 773 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A unique identifier for the population criteria. This identifier is used to report data against this criteria within the measure report.", 0, 1, identifier); 774 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of population criteria.", 0, 1, code); 775 case 3373707: /*name*/ return new Property("name", "string", "Optional name or short description of this population.", 0, 1, name); 776 case -1724546052: /*description*/ return new Property("description", "string", "The human readable description of this population criteria.", 0, 1, description); 777 case 1952046943: /*criteria*/ return new Property("criteria", "string", "The name of a valid referenced CQL expression (may be namespaced) that defines this population criteria.", 0, 1, criteria); 778 default: return super.getNamedProperty(_hash, _name, _checkValid); 779 } 780 781 } 782 783 @Override 784 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 785 switch (hash) { 786 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 787 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 788 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 789 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // StringType 790 case 1952046943: /*criteria*/ return this.criteria == null ? new Base[0] : new Base[] {this.criteria}; // StringType 791 default: return super.getProperty(hash, name, checkValid); 792 } 793 794 } 795 796 @Override 797 public Base setProperty(int hash, String name, Base value) throws FHIRException { 798 switch (hash) { 799 case -1618432855: // identifier 800 this.identifier = castToIdentifier(value); // Identifier 801 return value; 802 case 3059181: // code 803 this.code = castToCodeableConcept(value); // CodeableConcept 804 return value; 805 case 3373707: // name 806 this.name = castToString(value); // StringType 807 return value; 808 case -1724546052: // description 809 this.description = castToString(value); // StringType 810 return value; 811 case 1952046943: // criteria 812 this.criteria = castToString(value); // StringType 813 return value; 814 default: return super.setProperty(hash, name, value); 815 } 816 817 } 818 819 @Override 820 public Base setProperty(String name, Base value) throws FHIRException { 821 if (name.equals("identifier")) { 822 this.identifier = castToIdentifier(value); // Identifier 823 } else if (name.equals("code")) { 824 this.code = castToCodeableConcept(value); // CodeableConcept 825 } else if (name.equals("name")) { 826 this.name = castToString(value); // StringType 827 } else if (name.equals("description")) { 828 this.description = castToString(value); // StringType 829 } else if (name.equals("criteria")) { 830 this.criteria = castToString(value); // StringType 831 } else 832 return super.setProperty(name, value); 833 return value; 834 } 835 836 @Override 837 public Base makeProperty(int hash, String name) throws FHIRException { 838 switch (hash) { 839 case -1618432855: return getIdentifier(); 840 case 3059181: return getCode(); 841 case 3373707: return getNameElement(); 842 case -1724546052: return getDescriptionElement(); 843 case 1952046943: return getCriteriaElement(); 844 default: return super.makeProperty(hash, name); 845 } 846 847 } 848 849 @Override 850 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 851 switch (hash) { 852 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 853 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 854 case 3373707: /*name*/ return new String[] {"string"}; 855 case -1724546052: /*description*/ return new String[] {"string"}; 856 case 1952046943: /*criteria*/ return new String[] {"string"}; 857 default: return super.getTypesForProperty(hash, name); 858 } 859 860 } 861 862 @Override 863 public Base addChild(String name) throws FHIRException { 864 if (name.equals("identifier")) { 865 this.identifier = new Identifier(); 866 return this.identifier; 867 } 868 else if (name.equals("code")) { 869 this.code = new CodeableConcept(); 870 return this.code; 871 } 872 else if (name.equals("name")) { 873 throw new FHIRException("Cannot call addChild on a singleton property Measure.name"); 874 } 875 else if (name.equals("description")) { 876 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 877 } 878 else if (name.equals("criteria")) { 879 throw new FHIRException("Cannot call addChild on a singleton property Measure.criteria"); 880 } 881 else 882 return super.addChild(name); 883 } 884 885 public MeasureGroupPopulationComponent copy() { 886 MeasureGroupPopulationComponent dst = new MeasureGroupPopulationComponent(); 887 copyValues(dst); 888 dst.identifier = identifier == null ? null : identifier.copy(); 889 dst.code = code == null ? null : code.copy(); 890 dst.name = name == null ? null : name.copy(); 891 dst.description = description == null ? null : description.copy(); 892 dst.criteria = criteria == null ? null : criteria.copy(); 893 return dst; 894 } 895 896 @Override 897 public boolean equalsDeep(Base other_) { 898 if (!super.equalsDeep(other_)) 899 return false; 900 if (!(other_ instanceof MeasureGroupPopulationComponent)) 901 return false; 902 MeasureGroupPopulationComponent o = (MeasureGroupPopulationComponent) other_; 903 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(name, o.name, true) 904 && compareDeep(description, o.description, true) && compareDeep(criteria, o.criteria, true); 905 } 906 907 @Override 908 public boolean equalsShallow(Base other_) { 909 if (!super.equalsShallow(other_)) 910 return false; 911 if (!(other_ instanceof MeasureGroupPopulationComponent)) 912 return false; 913 MeasureGroupPopulationComponent o = (MeasureGroupPopulationComponent) other_; 914 return compareValues(name, o.name, true) && compareValues(description, o.description, true) && compareValues(criteria, o.criteria, true) 915 ; 916 } 917 918 public boolean isEmpty() { 919 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, name, description 920 , criteria); 921 } 922 923 public String fhirType() { 924 return "Measure.group.population"; 925 926 } 927 928 } 929 930 @Block() 931 public static class MeasureGroupStratifierComponent extends BackboneElement implements IBaseBackboneElement { 932 /** 933 * The identifier for the stratifier used to coordinate the reported data back to this stratifier. 934 */ 935 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=false) 936 @Description(shortDefinition="The identifier for the stratifier used to coordinate the reported data back to this stratifier", formalDefinition="The identifier for the stratifier used to coordinate the reported data back to this stratifier." ) 937 protected Identifier identifier; 938 939 /** 940 * The criteria for the stratifier. This must be the name of an expression defined within a referenced library. 941 */ 942 @Child(name = "criteria", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 943 @Description(shortDefinition="How the measure should be stratified", formalDefinition="The criteria for the stratifier. This must be the name of an expression defined within a referenced library." ) 944 protected StringType criteria; 945 946 /** 947 * The path to an element that defines the stratifier, specified as a valid FHIR resource path. 948 */ 949 @Child(name = "path", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 950 @Description(shortDefinition="Path to the stratifier", formalDefinition="The path to an element that defines the stratifier, specified as a valid FHIR resource path." ) 951 protected StringType path; 952 953 private static final long serialVersionUID = -196134448L; 954 955 /** 956 * Constructor 957 */ 958 public MeasureGroupStratifierComponent() { 959 super(); 960 } 961 962 /** 963 * @return {@link #identifier} (The identifier for the stratifier used to coordinate the reported data back to this stratifier.) 964 */ 965 public Identifier getIdentifier() { 966 if (this.identifier == null) 967 if (Configuration.errorOnAutoCreate()) 968 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.identifier"); 969 else if (Configuration.doAutoCreate()) 970 this.identifier = new Identifier(); // cc 971 return this.identifier; 972 } 973 974 public boolean hasIdentifier() { 975 return this.identifier != null && !this.identifier.isEmpty(); 976 } 977 978 /** 979 * @param value {@link #identifier} (The identifier for the stratifier used to coordinate the reported data back to this stratifier.) 980 */ 981 public MeasureGroupStratifierComponent setIdentifier(Identifier value) { 982 this.identifier = value; 983 return this; 984 } 985 986 /** 987 * @return {@link #criteria} (The criteria for the stratifier. This must be the name of an expression defined within a referenced library.). This is the underlying object with id, value and extensions. The accessor "getCriteria" gives direct access to the value 988 */ 989 public StringType getCriteriaElement() { 990 if (this.criteria == null) 991 if (Configuration.errorOnAutoCreate()) 992 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.criteria"); 993 else if (Configuration.doAutoCreate()) 994 this.criteria = new StringType(); // bb 995 return this.criteria; 996 } 997 998 public boolean hasCriteriaElement() { 999 return this.criteria != null && !this.criteria.isEmpty(); 1000 } 1001 1002 public boolean hasCriteria() { 1003 return this.criteria != null && !this.criteria.isEmpty(); 1004 } 1005 1006 /** 1007 * @param value {@link #criteria} (The criteria for the stratifier. This must be the name of an expression defined within a referenced library.). This is the underlying object with id, value and extensions. The accessor "getCriteria" gives direct access to the value 1008 */ 1009 public MeasureGroupStratifierComponent setCriteriaElement(StringType value) { 1010 this.criteria = value; 1011 return this; 1012 } 1013 1014 /** 1015 * @return The criteria for the stratifier. This must be the name of an expression defined within a referenced library. 1016 */ 1017 public String getCriteria() { 1018 return this.criteria == null ? null : this.criteria.getValue(); 1019 } 1020 1021 /** 1022 * @param value The criteria for the stratifier. This must be the name of an expression defined within a referenced library. 1023 */ 1024 public MeasureGroupStratifierComponent setCriteria(String value) { 1025 if (Utilities.noString(value)) 1026 this.criteria = null; 1027 else { 1028 if (this.criteria == null) 1029 this.criteria = new StringType(); 1030 this.criteria.setValue(value); 1031 } 1032 return this; 1033 } 1034 1035 /** 1036 * @return {@link #path} (The path to an element that defines the stratifier, specified as a valid FHIR resource path.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1037 */ 1038 public StringType getPathElement() { 1039 if (this.path == null) 1040 if (Configuration.errorOnAutoCreate()) 1041 throw new Error("Attempt to auto-create MeasureGroupStratifierComponent.path"); 1042 else if (Configuration.doAutoCreate()) 1043 this.path = new StringType(); // bb 1044 return this.path; 1045 } 1046 1047 public boolean hasPathElement() { 1048 return this.path != null && !this.path.isEmpty(); 1049 } 1050 1051 public boolean hasPath() { 1052 return this.path != null && !this.path.isEmpty(); 1053 } 1054 1055 /** 1056 * @param value {@link #path} (The path to an element that defines the stratifier, specified as a valid FHIR resource path.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1057 */ 1058 public MeasureGroupStratifierComponent setPathElement(StringType value) { 1059 this.path = value; 1060 return this; 1061 } 1062 1063 /** 1064 * @return The path to an element that defines the stratifier, specified as a valid FHIR resource path. 1065 */ 1066 public String getPath() { 1067 return this.path == null ? null : this.path.getValue(); 1068 } 1069 1070 /** 1071 * @param value The path to an element that defines the stratifier, specified as a valid FHIR resource path. 1072 */ 1073 public MeasureGroupStratifierComponent setPath(String value) { 1074 if (Utilities.noString(value)) 1075 this.path = null; 1076 else { 1077 if (this.path == null) 1078 this.path = new StringType(); 1079 this.path.setValue(value); 1080 } 1081 return this; 1082 } 1083 1084 protected void listChildren(List<Property> children) { 1085 super.listChildren(children); 1086 children.add(new Property("identifier", "Identifier", "The identifier for the stratifier used to coordinate the reported data back to this stratifier.", 0, 1, identifier)); 1087 children.add(new Property("criteria", "string", "The criteria for the stratifier. This must be the name of an expression defined within a referenced library.", 0, 1, criteria)); 1088 children.add(new Property("path", "string", "The path to an element that defines the stratifier, specified as a valid FHIR resource path.", 0, 1, path)); 1089 } 1090 1091 @Override 1092 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1093 switch (_hash) { 1094 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier for the stratifier used to coordinate the reported data back to this stratifier.", 0, 1, identifier); 1095 case 1952046943: /*criteria*/ return new Property("criteria", "string", "The criteria for the stratifier. This must be the name of an expression defined within a referenced library.", 0, 1, criteria); 1096 case 3433509: /*path*/ return new Property("path", "string", "The path to an element that defines the stratifier, specified as a valid FHIR resource path.", 0, 1, path); 1097 default: return super.getNamedProperty(_hash, _name, _checkValid); 1098 } 1099 1100 } 1101 1102 @Override 1103 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1104 switch (hash) { 1105 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1106 case 1952046943: /*criteria*/ return this.criteria == null ? new Base[0] : new Base[] {this.criteria}; // StringType 1107 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1108 default: return super.getProperty(hash, name, checkValid); 1109 } 1110 1111 } 1112 1113 @Override 1114 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1115 switch (hash) { 1116 case -1618432855: // identifier 1117 this.identifier = castToIdentifier(value); // Identifier 1118 return value; 1119 case 1952046943: // criteria 1120 this.criteria = castToString(value); // StringType 1121 return value; 1122 case 3433509: // path 1123 this.path = castToString(value); // StringType 1124 return value; 1125 default: return super.setProperty(hash, name, value); 1126 } 1127 1128 } 1129 1130 @Override 1131 public Base setProperty(String name, Base value) throws FHIRException { 1132 if (name.equals("identifier")) { 1133 this.identifier = castToIdentifier(value); // Identifier 1134 } else if (name.equals("criteria")) { 1135 this.criteria = castToString(value); // StringType 1136 } else if (name.equals("path")) { 1137 this.path = castToString(value); // StringType 1138 } else 1139 return super.setProperty(name, value); 1140 return value; 1141 } 1142 1143 @Override 1144 public Base makeProperty(int hash, String name) throws FHIRException { 1145 switch (hash) { 1146 case -1618432855: return getIdentifier(); 1147 case 1952046943: return getCriteriaElement(); 1148 case 3433509: return getPathElement(); 1149 default: return super.makeProperty(hash, name); 1150 } 1151 1152 } 1153 1154 @Override 1155 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1156 switch (hash) { 1157 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1158 case 1952046943: /*criteria*/ return new String[] {"string"}; 1159 case 3433509: /*path*/ return new String[] {"string"}; 1160 default: return super.getTypesForProperty(hash, name); 1161 } 1162 1163 } 1164 1165 @Override 1166 public Base addChild(String name) throws FHIRException { 1167 if (name.equals("identifier")) { 1168 this.identifier = new Identifier(); 1169 return this.identifier; 1170 } 1171 else if (name.equals("criteria")) { 1172 throw new FHIRException("Cannot call addChild on a singleton property Measure.criteria"); 1173 } 1174 else if (name.equals("path")) { 1175 throw new FHIRException("Cannot call addChild on a singleton property Measure.path"); 1176 } 1177 else 1178 return super.addChild(name); 1179 } 1180 1181 public MeasureGroupStratifierComponent copy() { 1182 MeasureGroupStratifierComponent dst = new MeasureGroupStratifierComponent(); 1183 copyValues(dst); 1184 dst.identifier = identifier == null ? null : identifier.copy(); 1185 dst.criteria = criteria == null ? null : criteria.copy(); 1186 dst.path = path == null ? null : path.copy(); 1187 return dst; 1188 } 1189 1190 @Override 1191 public boolean equalsDeep(Base other_) { 1192 if (!super.equalsDeep(other_)) 1193 return false; 1194 if (!(other_ instanceof MeasureGroupStratifierComponent)) 1195 return false; 1196 MeasureGroupStratifierComponent o = (MeasureGroupStratifierComponent) other_; 1197 return compareDeep(identifier, o.identifier, true) && compareDeep(criteria, o.criteria, true) && compareDeep(path, o.path, true) 1198 ; 1199 } 1200 1201 @Override 1202 public boolean equalsShallow(Base other_) { 1203 if (!super.equalsShallow(other_)) 1204 return false; 1205 if (!(other_ instanceof MeasureGroupStratifierComponent)) 1206 return false; 1207 MeasureGroupStratifierComponent o = (MeasureGroupStratifierComponent) other_; 1208 return compareValues(criteria, o.criteria, true) && compareValues(path, o.path, true); 1209 } 1210 1211 public boolean isEmpty() { 1212 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, criteria, path 1213 ); 1214 } 1215 1216 public String fhirType() { 1217 return "Measure.group.stratifier"; 1218 1219 } 1220 1221 } 1222 1223 @Block() 1224 public static class MeasureSupplementalDataComponent extends BackboneElement implements IBaseBackboneElement { 1225 /** 1226 * An identifier for the supplemental data. 1227 */ 1228 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=false) 1229 @Description(shortDefinition="Identifier, unique within the measure", formalDefinition="An identifier for the supplemental data." ) 1230 protected Identifier identifier; 1231 1232 /** 1233 * An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation. 1234 */ 1235 @Child(name = "usage", type = {CodeableConcept.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1236 @Description(shortDefinition="supplemental-data | risk-adjustment-factor", formalDefinition="An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation." ) 1237 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-data-usage") 1238 protected List<CodeableConcept> usage; 1239 1240 /** 1241 * The criteria for the supplemental data. This must be the name of a valid expression defined within a referenced library, and defines the data to be returned for this element. 1242 */ 1243 @Child(name = "criteria", type = {StringType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1244 @Description(shortDefinition="Expression describing additional data to be reported", formalDefinition="The criteria for the supplemental data. This must be the name of a valid expression defined within a referenced library, and defines the data to be returned for this element." ) 1245 protected StringType criteria; 1246 1247 /** 1248 * The supplemental data to be supplied as part of the measure response, specified as a valid FHIR Resource Path. 1249 */ 1250 @Child(name = "path", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1251 @Description(shortDefinition="Path to the supplemental data element", formalDefinition="The supplemental data to be supplied as part of the measure response, specified as a valid FHIR Resource Path." ) 1252 protected StringType path; 1253 1254 private static final long serialVersionUID = -101576770L; 1255 1256 /** 1257 * Constructor 1258 */ 1259 public MeasureSupplementalDataComponent() { 1260 super(); 1261 } 1262 1263 /** 1264 * @return {@link #identifier} (An identifier for the supplemental data.) 1265 */ 1266 public Identifier getIdentifier() { 1267 if (this.identifier == null) 1268 if (Configuration.errorOnAutoCreate()) 1269 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.identifier"); 1270 else if (Configuration.doAutoCreate()) 1271 this.identifier = new Identifier(); // cc 1272 return this.identifier; 1273 } 1274 1275 public boolean hasIdentifier() { 1276 return this.identifier != null && !this.identifier.isEmpty(); 1277 } 1278 1279 /** 1280 * @param value {@link #identifier} (An identifier for the supplemental data.) 1281 */ 1282 public MeasureSupplementalDataComponent setIdentifier(Identifier value) { 1283 this.identifier = value; 1284 return this; 1285 } 1286 1287 /** 1288 * @return {@link #usage} (An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.) 1289 */ 1290 public List<CodeableConcept> getUsage() { 1291 if (this.usage == null) 1292 this.usage = new ArrayList<CodeableConcept>(); 1293 return this.usage; 1294 } 1295 1296 /** 1297 * @return Returns a reference to <code>this</code> for easy method chaining 1298 */ 1299 public MeasureSupplementalDataComponent setUsage(List<CodeableConcept> theUsage) { 1300 this.usage = theUsage; 1301 return this; 1302 } 1303 1304 public boolean hasUsage() { 1305 if (this.usage == null) 1306 return false; 1307 for (CodeableConcept item : this.usage) 1308 if (!item.isEmpty()) 1309 return true; 1310 return false; 1311 } 1312 1313 public CodeableConcept addUsage() { //3 1314 CodeableConcept t = new CodeableConcept(); 1315 if (this.usage == null) 1316 this.usage = new ArrayList<CodeableConcept>(); 1317 this.usage.add(t); 1318 return t; 1319 } 1320 1321 public MeasureSupplementalDataComponent addUsage(CodeableConcept t) { //3 1322 if (t == null) 1323 return this; 1324 if (this.usage == null) 1325 this.usage = new ArrayList<CodeableConcept>(); 1326 this.usage.add(t); 1327 return this; 1328 } 1329 1330 /** 1331 * @return The first repetition of repeating field {@link #usage}, creating it if it does not already exist 1332 */ 1333 public CodeableConcept getUsageFirstRep() { 1334 if (getUsage().isEmpty()) { 1335 addUsage(); 1336 } 1337 return getUsage().get(0); 1338 } 1339 1340 /** 1341 * @return {@link #criteria} (The criteria for the supplemental data. This must be the name of a valid expression defined within a referenced library, and defines the data to be returned for this element.). This is the underlying object with id, value and extensions. The accessor "getCriteria" gives direct access to the value 1342 */ 1343 public StringType getCriteriaElement() { 1344 if (this.criteria == null) 1345 if (Configuration.errorOnAutoCreate()) 1346 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.criteria"); 1347 else if (Configuration.doAutoCreate()) 1348 this.criteria = new StringType(); // bb 1349 return this.criteria; 1350 } 1351 1352 public boolean hasCriteriaElement() { 1353 return this.criteria != null && !this.criteria.isEmpty(); 1354 } 1355 1356 public boolean hasCriteria() { 1357 return this.criteria != null && !this.criteria.isEmpty(); 1358 } 1359 1360 /** 1361 * @param value {@link #criteria} (The criteria for the supplemental data. This must be the name of a valid expression defined within a referenced library, and defines the data to be returned for this element.). This is the underlying object with id, value and extensions. The accessor "getCriteria" gives direct access to the value 1362 */ 1363 public MeasureSupplementalDataComponent setCriteriaElement(StringType value) { 1364 this.criteria = value; 1365 return this; 1366 } 1367 1368 /** 1369 * @return The criteria for the supplemental data. This must be the name of a valid expression defined within a referenced library, and defines the data to be returned for this element. 1370 */ 1371 public String getCriteria() { 1372 return this.criteria == null ? null : this.criteria.getValue(); 1373 } 1374 1375 /** 1376 * @param value The criteria for the supplemental data. This must be the name of a valid expression defined within a referenced library, and defines the data to be returned for this element. 1377 */ 1378 public MeasureSupplementalDataComponent setCriteria(String value) { 1379 if (Utilities.noString(value)) 1380 this.criteria = null; 1381 else { 1382 if (this.criteria == null) 1383 this.criteria = new StringType(); 1384 this.criteria.setValue(value); 1385 } 1386 return this; 1387 } 1388 1389 /** 1390 * @return {@link #path} (The supplemental data to be supplied as part of the measure response, specified as a valid FHIR Resource Path.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1391 */ 1392 public StringType getPathElement() { 1393 if (this.path == null) 1394 if (Configuration.errorOnAutoCreate()) 1395 throw new Error("Attempt to auto-create MeasureSupplementalDataComponent.path"); 1396 else if (Configuration.doAutoCreate()) 1397 this.path = new StringType(); // bb 1398 return this.path; 1399 } 1400 1401 public boolean hasPathElement() { 1402 return this.path != null && !this.path.isEmpty(); 1403 } 1404 1405 public boolean hasPath() { 1406 return this.path != null && !this.path.isEmpty(); 1407 } 1408 1409 /** 1410 * @param value {@link #path} (The supplemental data to be supplied as part of the measure response, specified as a valid FHIR Resource Path.). This is the underlying object with id, value and extensions. The accessor "getPath" gives direct access to the value 1411 */ 1412 public MeasureSupplementalDataComponent setPathElement(StringType value) { 1413 this.path = value; 1414 return this; 1415 } 1416 1417 /** 1418 * @return The supplemental data to be supplied as part of the measure response, specified as a valid FHIR Resource Path. 1419 */ 1420 public String getPath() { 1421 return this.path == null ? null : this.path.getValue(); 1422 } 1423 1424 /** 1425 * @param value The supplemental data to be supplied as part of the measure response, specified as a valid FHIR Resource Path. 1426 */ 1427 public MeasureSupplementalDataComponent setPath(String value) { 1428 if (Utilities.noString(value)) 1429 this.path = null; 1430 else { 1431 if (this.path == null) 1432 this.path = new StringType(); 1433 this.path.setValue(value); 1434 } 1435 return this; 1436 } 1437 1438 protected void listChildren(List<Property> children) { 1439 super.listChildren(children); 1440 children.add(new Property("identifier", "Identifier", "An identifier for the supplemental data.", 0, 1, identifier)); 1441 children.add(new Property("usage", "CodeableConcept", "An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.", 0, java.lang.Integer.MAX_VALUE, usage)); 1442 children.add(new Property("criteria", "string", "The criteria for the supplemental data. This must be the name of a valid expression defined within a referenced library, and defines the data to be returned for this element.", 0, 1, criteria)); 1443 children.add(new Property("path", "string", "The supplemental data to be supplied as part of the measure response, specified as a valid FHIR Resource Path.", 0, 1, path)); 1444 } 1445 1446 @Override 1447 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1448 switch (_hash) { 1449 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "An identifier for the supplemental data.", 0, 1, identifier); 1450 case 111574433: /*usage*/ return new Property("usage", "CodeableConcept", "An indicator of the intended usage for the supplemental data element. Supplemental data indicates the data is additional information requested to augment the measure information. Risk adjustment factor indicates the data is additional information used to calculate risk adjustment factors when applying a risk model to the measure calculation.", 0, java.lang.Integer.MAX_VALUE, usage); 1451 case 1952046943: /*criteria*/ return new Property("criteria", "string", "The criteria for the supplemental data. This must be the name of a valid expression defined within a referenced library, and defines the data to be returned for this element.", 0, 1, criteria); 1452 case 3433509: /*path*/ return new Property("path", "string", "The supplemental data to be supplied as part of the measure response, specified as a valid FHIR Resource Path.", 0, 1, path); 1453 default: return super.getNamedProperty(_hash, _name, _checkValid); 1454 } 1455 1456 } 1457 1458 @Override 1459 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1460 switch (hash) { 1461 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1462 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : this.usage.toArray(new Base[this.usage.size()]); // CodeableConcept 1463 case 1952046943: /*criteria*/ return this.criteria == null ? new Base[0] : new Base[] {this.criteria}; // StringType 1464 case 3433509: /*path*/ return this.path == null ? new Base[0] : new Base[] {this.path}; // StringType 1465 default: return super.getProperty(hash, name, checkValid); 1466 } 1467 1468 } 1469 1470 @Override 1471 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1472 switch (hash) { 1473 case -1618432855: // identifier 1474 this.identifier = castToIdentifier(value); // Identifier 1475 return value; 1476 case 111574433: // usage 1477 this.getUsage().add(castToCodeableConcept(value)); // CodeableConcept 1478 return value; 1479 case 1952046943: // criteria 1480 this.criteria = castToString(value); // StringType 1481 return value; 1482 case 3433509: // path 1483 this.path = castToString(value); // StringType 1484 return value; 1485 default: return super.setProperty(hash, name, value); 1486 } 1487 1488 } 1489 1490 @Override 1491 public Base setProperty(String name, Base value) throws FHIRException { 1492 if (name.equals("identifier")) { 1493 this.identifier = castToIdentifier(value); // Identifier 1494 } else if (name.equals("usage")) { 1495 this.getUsage().add(castToCodeableConcept(value)); 1496 } else if (name.equals("criteria")) { 1497 this.criteria = castToString(value); // StringType 1498 } else if (name.equals("path")) { 1499 this.path = castToString(value); // StringType 1500 } else 1501 return super.setProperty(name, value); 1502 return value; 1503 } 1504 1505 @Override 1506 public Base makeProperty(int hash, String name) throws FHIRException { 1507 switch (hash) { 1508 case -1618432855: return getIdentifier(); 1509 case 111574433: return addUsage(); 1510 case 1952046943: return getCriteriaElement(); 1511 case 3433509: return getPathElement(); 1512 default: return super.makeProperty(hash, name); 1513 } 1514 1515 } 1516 1517 @Override 1518 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1519 switch (hash) { 1520 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1521 case 111574433: /*usage*/ return new String[] {"CodeableConcept"}; 1522 case 1952046943: /*criteria*/ return new String[] {"string"}; 1523 case 3433509: /*path*/ return new String[] {"string"}; 1524 default: return super.getTypesForProperty(hash, name); 1525 } 1526 1527 } 1528 1529 @Override 1530 public Base addChild(String name) throws FHIRException { 1531 if (name.equals("identifier")) { 1532 this.identifier = new Identifier(); 1533 return this.identifier; 1534 } 1535 else if (name.equals("usage")) { 1536 return addUsage(); 1537 } 1538 else if (name.equals("criteria")) { 1539 throw new FHIRException("Cannot call addChild on a singleton property Measure.criteria"); 1540 } 1541 else if (name.equals("path")) { 1542 throw new FHIRException("Cannot call addChild on a singleton property Measure.path"); 1543 } 1544 else 1545 return super.addChild(name); 1546 } 1547 1548 public MeasureSupplementalDataComponent copy() { 1549 MeasureSupplementalDataComponent dst = new MeasureSupplementalDataComponent(); 1550 copyValues(dst); 1551 dst.identifier = identifier == null ? null : identifier.copy(); 1552 if (usage != null) { 1553 dst.usage = new ArrayList<CodeableConcept>(); 1554 for (CodeableConcept i : usage) 1555 dst.usage.add(i.copy()); 1556 }; 1557 dst.criteria = criteria == null ? null : criteria.copy(); 1558 dst.path = path == null ? null : path.copy(); 1559 return dst; 1560 } 1561 1562 @Override 1563 public boolean equalsDeep(Base other_) { 1564 if (!super.equalsDeep(other_)) 1565 return false; 1566 if (!(other_ instanceof MeasureSupplementalDataComponent)) 1567 return false; 1568 MeasureSupplementalDataComponent o = (MeasureSupplementalDataComponent) other_; 1569 return compareDeep(identifier, o.identifier, true) && compareDeep(usage, o.usage, true) && compareDeep(criteria, o.criteria, true) 1570 && compareDeep(path, o.path, true); 1571 } 1572 1573 @Override 1574 public boolean equalsShallow(Base other_) { 1575 if (!super.equalsShallow(other_)) 1576 return false; 1577 if (!(other_ instanceof MeasureSupplementalDataComponent)) 1578 return false; 1579 MeasureSupplementalDataComponent o = (MeasureSupplementalDataComponent) other_; 1580 return compareValues(criteria, o.criteria, true) && compareValues(path, o.path, true); 1581 } 1582 1583 public boolean isEmpty() { 1584 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, usage, criteria 1585 , path); 1586 } 1587 1588 public String fhirType() { 1589 return "Measure.supplementalData"; 1590 1591 } 1592 1593 } 1594 1595 /** 1596 * A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance. 1597 */ 1598 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1599 @Description(shortDefinition="Additional identifier for the measure", formalDefinition="A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1600 protected List<Identifier> identifier; 1601 1602 /** 1603 * Explaination of why this measure is needed and why it has been designed as it has. 1604 */ 1605 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1606 @Description(shortDefinition="Why this measure is defined", formalDefinition="Explaination of why this measure is needed and why it has been designed as it has." ) 1607 protected MarkdownType purpose; 1608 1609 /** 1610 * A detailed description of how the measure is used from a clinical perspective. 1611 */ 1612 @Child(name = "usage", type = {StringType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1613 @Description(shortDefinition="Describes the clinical usage of the measure", formalDefinition="A detailed description of how the measure is used from a clinical perspective." ) 1614 protected StringType usage; 1615 1616 /** 1617 * The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 1618 */ 1619 @Child(name = "approvalDate", type = {DateType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1620 @Description(shortDefinition="When the measure was approved by publisher", formalDefinition="The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage." ) 1621 protected DateType approvalDate; 1622 1623 /** 1624 * The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 1625 */ 1626 @Child(name = "lastReviewDate", type = {DateType.class}, order=4, min=0, max=1, modifier=false, summary=false) 1627 @Description(shortDefinition="When the measure was last reviewed", formalDefinition="The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date." ) 1628 protected DateType lastReviewDate; 1629 1630 /** 1631 * The period during which the measure content was or is planned to be in active use. 1632 */ 1633 @Child(name = "effectivePeriod", type = {Period.class}, order=5, min=0, max=1, modifier=false, summary=true) 1634 @Description(shortDefinition="When the measure is expected to be used", formalDefinition="The period during which the measure content was or is planned to be in active use." ) 1635 protected Period effectivePeriod; 1636 1637 /** 1638 * Descriptive topics related to the content of the measure. Topics provide a high-level categorization of the type of the measure that can be useful for filtering and searching. 1639 */ 1640 @Child(name = "topic", type = {CodeableConcept.class}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1641 @Description(shortDefinition="E.g. Education, Treatment, Assessment, etc", formalDefinition="Descriptive topics related to the content of the measure. Topics provide a high-level categorization of the type of the measure that can be useful for filtering and searching." ) 1642 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/definition-topic") 1643 protected List<CodeableConcept> topic; 1644 1645 /** 1646 * A contributor to the content of the measure, including authors, editors, reviewers, and endorsers. 1647 */ 1648 @Child(name = "contributor", type = {Contributor.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1649 @Description(shortDefinition="A content contributor", formalDefinition="A contributor to the content of the measure, including authors, editors, reviewers, and endorsers." ) 1650 protected List<Contributor> contributor; 1651 1652 /** 1653 * A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure. 1654 */ 1655 @Child(name = "copyright", type = {MarkdownType.class}, order=8, min=0, max=1, modifier=false, summary=false) 1656 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure." ) 1657 protected MarkdownType copyright; 1658 1659 /** 1660 * Related artifacts such as additional documentation, justification, or bibliographic references. 1661 */ 1662 @Child(name = "relatedArtifact", type = {RelatedArtifact.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1663 @Description(shortDefinition="Additional documentation, citations, etc", formalDefinition="Related artifacts such as additional documentation, justification, or bibliographic references." ) 1664 protected List<RelatedArtifact> relatedArtifact; 1665 1666 /** 1667 * A reference to a Library resource containing the formal logic used by the measure. 1668 */ 1669 @Child(name = "library", type = {Library.class}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1670 @Description(shortDefinition="Logic used by the measure", formalDefinition="A reference to a Library resource containing the formal logic used by the measure." ) 1671 protected List<Reference> library; 1672 /** 1673 * The actual objects that are the target of the reference (A reference to a Library resource containing the formal logic used by the measure.) 1674 */ 1675 protected List<Library> libraryTarget; 1676 1677 1678 /** 1679 * Notices and disclaimers regarding the use of the measure, or related to intellectual property (such as code systems) referenced by the measure. 1680 */ 1681 @Child(name = "disclaimer", type = {MarkdownType.class}, order=11, min=0, max=1, modifier=false, summary=true) 1682 @Description(shortDefinition="Disclaimer for use of the measure or its referenced content", formalDefinition="Notices and disclaimers regarding the use of the measure, or related to intellectual property (such as code systems) referenced by the measure." ) 1683 protected MarkdownType disclaimer; 1684 1685 /** 1686 * Indicates how the calculation is performed for the measure, including proportion, ratio, continuous variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented. 1687 */ 1688 @Child(name = "scoring", type = {CodeableConcept.class}, order=12, min=0, max=1, modifier=false, summary=true) 1689 @Description(shortDefinition="proportion | ratio | continuous-variable | cohort", formalDefinition="Indicates how the calculation is performed for the measure, including proportion, ratio, continuous variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented." ) 1690 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-scoring") 1691 protected CodeableConcept scoring; 1692 1693 /** 1694 * If this is a composite measure, the scoring method used to combine the component measures to determine the composite score. 1695 */ 1696 @Child(name = "compositeScoring", type = {CodeableConcept.class}, order=13, min=0, max=1, modifier=false, summary=true) 1697 @Description(shortDefinition="opportunity | all-or-nothing | linear | weighted", formalDefinition="If this is a composite measure, the scoring method used to combine the component measures to determine the composite score." ) 1698 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/composite-measure-scoring") 1699 protected CodeableConcept compositeScoring; 1700 1701 /** 1702 * Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization. 1703 */ 1704 @Child(name = "type", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1705 @Description(shortDefinition="process | outcome | structure | patient-reported-outcome | composite", formalDefinition="Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization." ) 1706 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-type") 1707 protected List<CodeableConcept> type; 1708 1709 /** 1710 * A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results. 1711 */ 1712 @Child(name = "riskAdjustment", type = {StringType.class}, order=15, min=0, max=1, modifier=false, summary=true) 1713 @Description(shortDefinition="How is risk adjustment applied for this measure", formalDefinition="A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results." ) 1714 protected StringType riskAdjustment; 1715 1716 /** 1717 * Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 1718 */ 1719 @Child(name = "rateAggregation", type = {StringType.class}, order=16, min=0, max=1, modifier=false, summary=true) 1720 @Description(shortDefinition="How is rate aggregation performed for this measure", formalDefinition="Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result." ) 1721 protected StringType rateAggregation; 1722 1723 /** 1724 * Provides a succint statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence. 1725 */ 1726 @Child(name = "rationale", type = {MarkdownType.class}, order=17, min=0, max=1, modifier=false, summary=true) 1727 @Description(shortDefinition="Why does this measure exist", formalDefinition="Provides a succint statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence." ) 1728 protected MarkdownType rationale; 1729 1730 /** 1731 * Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure. 1732 */ 1733 @Child(name = "clinicalRecommendationStatement", type = {MarkdownType.class}, order=18, min=0, max=1, modifier=false, summary=true) 1734 @Description(shortDefinition="Summary of clinical guidelines", formalDefinition="Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure." ) 1735 protected MarkdownType clinicalRecommendationStatement; 1736 1737 /** 1738 * Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is whthin a range). 1739 */ 1740 @Child(name = "improvementNotation", type = {StringType.class}, order=19, min=0, max=1, modifier=false, summary=true) 1741 @Description(shortDefinition="Improvement notation for the measure, e.g. higher score indicates better quality", formalDefinition="Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is whthin a range)." ) 1742 protected StringType improvementNotation; 1743 1744 /** 1745 * Provides a description of an individual term used within the measure. 1746 */ 1747 @Child(name = "definition", type = {MarkdownType.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1748 @Description(shortDefinition="Defined terms used in the measure documentation", formalDefinition="Provides a description of an individual term used within the measure." ) 1749 protected List<MarkdownType> definition; 1750 1751 /** 1752 * Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure. 1753 */ 1754 @Child(name = "guidance", type = {MarkdownType.class}, order=21, min=0, max=1, modifier=false, summary=true) 1755 @Description(shortDefinition="Additional guidance for implementers", formalDefinition="Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure." ) 1756 protected MarkdownType guidance; 1757 1758 /** 1759 * The measure set, e.g. Preventive Care and Screening. 1760 */ 1761 @Child(name = "set", type = {StringType.class}, order=22, min=0, max=1, modifier=false, summary=true) 1762 @Description(shortDefinition="The measure set, e.g. Preventive Care and Screening", formalDefinition="The measure set, e.g. Preventive Care and Screening." ) 1763 protected StringType set; 1764 1765 /** 1766 * A group of population criteria for the measure. 1767 */ 1768 @Child(name = "group", type = {}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1769 @Description(shortDefinition="Population criteria group", formalDefinition="A group of population criteria for the measure." ) 1770 protected List<MeasureGroupComponent> group; 1771 1772 /** 1773 * The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path. 1774 */ 1775 @Child(name = "supplementalData", type = {}, order=24, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1776 @Description(shortDefinition="What other data should be reported with the measure", formalDefinition="The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path." ) 1777 protected List<MeasureSupplementalDataComponent> supplementalData; 1778 1779 private static final long serialVersionUID = -875918689L; 1780 1781 /** 1782 * Constructor 1783 */ 1784 public Measure() { 1785 super(); 1786 } 1787 1788 /** 1789 * Constructor 1790 */ 1791 public Measure(Enumeration<PublicationStatus> status) { 1792 super(); 1793 this.status = status; 1794 } 1795 1796 /** 1797 * @return {@link #url} (An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this measure is (or will be) published. The URL SHOULD include the major version of the measure. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1798 */ 1799 public UriType getUrlElement() { 1800 if (this.url == null) 1801 if (Configuration.errorOnAutoCreate()) 1802 throw new Error("Attempt to auto-create Measure.url"); 1803 else if (Configuration.doAutoCreate()) 1804 this.url = new UriType(); // bb 1805 return this.url; 1806 } 1807 1808 public boolean hasUrlElement() { 1809 return this.url != null && !this.url.isEmpty(); 1810 } 1811 1812 public boolean hasUrl() { 1813 return this.url != null && !this.url.isEmpty(); 1814 } 1815 1816 /** 1817 * @param value {@link #url} (An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this measure is (or will be) published. The URL SHOULD include the major version of the measure. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 1818 */ 1819 public Measure setUrlElement(UriType value) { 1820 this.url = value; 1821 return this; 1822 } 1823 1824 /** 1825 * @return An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this measure is (or will be) published. The URL SHOULD include the major version of the measure. For more information see [Technical and Business Versions](resource.html#versions). 1826 */ 1827 public String getUrl() { 1828 return this.url == null ? null : this.url.getValue(); 1829 } 1830 1831 /** 1832 * @param value An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this measure is (or will be) published. The URL SHOULD include the major version of the measure. For more information see [Technical and Business Versions](resource.html#versions). 1833 */ 1834 public Measure setUrl(String value) { 1835 if (Utilities.noString(value)) 1836 this.url = null; 1837 else { 1838 if (this.url == null) 1839 this.url = new UriType(); 1840 this.url.setValue(value); 1841 } 1842 return this; 1843 } 1844 1845 /** 1846 * @return {@link #identifier} (A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.) 1847 */ 1848 public List<Identifier> getIdentifier() { 1849 if (this.identifier == null) 1850 this.identifier = new ArrayList<Identifier>(); 1851 return this.identifier; 1852 } 1853 1854 /** 1855 * @return Returns a reference to <code>this</code> for easy method chaining 1856 */ 1857 public Measure setIdentifier(List<Identifier> theIdentifier) { 1858 this.identifier = theIdentifier; 1859 return this; 1860 } 1861 1862 public boolean hasIdentifier() { 1863 if (this.identifier == null) 1864 return false; 1865 for (Identifier item : this.identifier) 1866 if (!item.isEmpty()) 1867 return true; 1868 return false; 1869 } 1870 1871 public Identifier addIdentifier() { //3 1872 Identifier t = new Identifier(); 1873 if (this.identifier == null) 1874 this.identifier = new ArrayList<Identifier>(); 1875 this.identifier.add(t); 1876 return t; 1877 } 1878 1879 public Measure addIdentifier(Identifier t) { //3 1880 if (t == null) 1881 return this; 1882 if (this.identifier == null) 1883 this.identifier = new ArrayList<Identifier>(); 1884 this.identifier.add(t); 1885 return this; 1886 } 1887 1888 /** 1889 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1890 */ 1891 public Identifier getIdentifierFirstRep() { 1892 if (getIdentifier().isEmpty()) { 1893 addIdentifier(); 1894 } 1895 return getIdentifier().get(0); 1896 } 1897 1898 /** 1899 * @return {@link #version} (The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1900 */ 1901 public StringType getVersionElement() { 1902 if (this.version == null) 1903 if (Configuration.errorOnAutoCreate()) 1904 throw new Error("Attempt to auto-create Measure.version"); 1905 else if (Configuration.doAutoCreate()) 1906 this.version = new StringType(); // bb 1907 return this.version; 1908 } 1909 1910 public boolean hasVersionElement() { 1911 return this.version != null && !this.version.isEmpty(); 1912 } 1913 1914 public boolean hasVersion() { 1915 return this.version != null && !this.version.isEmpty(); 1916 } 1917 1918 /** 1919 * @param value {@link #version} (The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1920 */ 1921 public Measure setVersionElement(StringType value) { 1922 this.version = value; 1923 return this; 1924 } 1925 1926 /** 1927 * @return The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 1928 */ 1929 public String getVersion() { 1930 return this.version == null ? null : this.version.getValue(); 1931 } 1932 1933 /** 1934 * @param value The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts. 1935 */ 1936 public Measure setVersion(String value) { 1937 if (Utilities.noString(value)) 1938 this.version = null; 1939 else { 1940 if (this.version == null) 1941 this.version = new StringType(); 1942 this.version.setValue(value); 1943 } 1944 return this; 1945 } 1946 1947 /** 1948 * @return {@link #name} (A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1949 */ 1950 public StringType getNameElement() { 1951 if (this.name == null) 1952 if (Configuration.errorOnAutoCreate()) 1953 throw new Error("Attempt to auto-create Measure.name"); 1954 else if (Configuration.doAutoCreate()) 1955 this.name = new StringType(); // bb 1956 return this.name; 1957 } 1958 1959 public boolean hasNameElement() { 1960 return this.name != null && !this.name.isEmpty(); 1961 } 1962 1963 public boolean hasName() { 1964 return this.name != null && !this.name.isEmpty(); 1965 } 1966 1967 /** 1968 * @param value {@link #name} (A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1969 */ 1970 public Measure setNameElement(StringType value) { 1971 this.name = value; 1972 return this; 1973 } 1974 1975 /** 1976 * @return A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1977 */ 1978 public String getName() { 1979 return this.name == null ? null : this.name.getValue(); 1980 } 1981 1982 /** 1983 * @param value A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1984 */ 1985 public Measure setName(String value) { 1986 if (Utilities.noString(value)) 1987 this.name = null; 1988 else { 1989 if (this.name == null) 1990 this.name = new StringType(); 1991 this.name.setValue(value); 1992 } 1993 return this; 1994 } 1995 1996 /** 1997 * @return {@link #title} (A short, descriptive, user-friendly title for the measure.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1998 */ 1999 public StringType getTitleElement() { 2000 if (this.title == null) 2001 if (Configuration.errorOnAutoCreate()) 2002 throw new Error("Attempt to auto-create Measure.title"); 2003 else if (Configuration.doAutoCreate()) 2004 this.title = new StringType(); // bb 2005 return this.title; 2006 } 2007 2008 public boolean hasTitleElement() { 2009 return this.title != null && !this.title.isEmpty(); 2010 } 2011 2012 public boolean hasTitle() { 2013 return this.title != null && !this.title.isEmpty(); 2014 } 2015 2016 /** 2017 * @param value {@link #title} (A short, descriptive, user-friendly title for the measure.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 2018 */ 2019 public Measure setTitleElement(StringType value) { 2020 this.title = value; 2021 return this; 2022 } 2023 2024 /** 2025 * @return A short, descriptive, user-friendly title for the measure. 2026 */ 2027 public String getTitle() { 2028 return this.title == null ? null : this.title.getValue(); 2029 } 2030 2031 /** 2032 * @param value A short, descriptive, user-friendly title for the measure. 2033 */ 2034 public Measure setTitle(String value) { 2035 if (Utilities.noString(value)) 2036 this.title = null; 2037 else { 2038 if (this.title == null) 2039 this.title = new StringType(); 2040 this.title.setValue(value); 2041 } 2042 return this; 2043 } 2044 2045 /** 2046 * @return {@link #status} (The status of this measure. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2047 */ 2048 public Enumeration<PublicationStatus> getStatusElement() { 2049 if (this.status == null) 2050 if (Configuration.errorOnAutoCreate()) 2051 throw new Error("Attempt to auto-create Measure.status"); 2052 else if (Configuration.doAutoCreate()) 2053 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 2054 return this.status; 2055 } 2056 2057 public boolean hasStatusElement() { 2058 return this.status != null && !this.status.isEmpty(); 2059 } 2060 2061 public boolean hasStatus() { 2062 return this.status != null && !this.status.isEmpty(); 2063 } 2064 2065 /** 2066 * @param value {@link #status} (The status of this measure. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2067 */ 2068 public Measure setStatusElement(Enumeration<PublicationStatus> value) { 2069 this.status = value; 2070 return this; 2071 } 2072 2073 /** 2074 * @return The status of this measure. Enables tracking the life-cycle of the content. 2075 */ 2076 public PublicationStatus getStatus() { 2077 return this.status == null ? null : this.status.getValue(); 2078 } 2079 2080 /** 2081 * @param value The status of this measure. Enables tracking the life-cycle of the content. 2082 */ 2083 public Measure setStatus(PublicationStatus value) { 2084 if (this.status == null) 2085 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 2086 this.status.setValue(value); 2087 return this; 2088 } 2089 2090 /** 2091 * @return {@link #experimental} (A boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2092 */ 2093 public BooleanType getExperimentalElement() { 2094 if (this.experimental == null) 2095 if (Configuration.errorOnAutoCreate()) 2096 throw new Error("Attempt to auto-create Measure.experimental"); 2097 else if (Configuration.doAutoCreate()) 2098 this.experimental = new BooleanType(); // bb 2099 return this.experimental; 2100 } 2101 2102 public boolean hasExperimentalElement() { 2103 return this.experimental != null && !this.experimental.isEmpty(); 2104 } 2105 2106 public boolean hasExperimental() { 2107 return this.experimental != null && !this.experimental.isEmpty(); 2108 } 2109 2110 /** 2111 * @param value {@link #experimental} (A boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 2112 */ 2113 public Measure setExperimentalElement(BooleanType value) { 2114 this.experimental = value; 2115 return this; 2116 } 2117 2118 /** 2119 * @return A boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2120 */ 2121 public boolean getExperimental() { 2122 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 2123 } 2124 2125 /** 2126 * @param value A boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 2127 */ 2128 public Measure setExperimental(boolean value) { 2129 if (this.experimental == null) 2130 this.experimental = new BooleanType(); 2131 this.experimental.setValue(value); 2132 return this; 2133 } 2134 2135 /** 2136 * @return {@link #date} (The date (and optionally time) when the measure was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2137 */ 2138 public DateTimeType getDateElement() { 2139 if (this.date == null) 2140 if (Configuration.errorOnAutoCreate()) 2141 throw new Error("Attempt to auto-create Measure.date"); 2142 else if (Configuration.doAutoCreate()) 2143 this.date = new DateTimeType(); // bb 2144 return this.date; 2145 } 2146 2147 public boolean hasDateElement() { 2148 return this.date != null && !this.date.isEmpty(); 2149 } 2150 2151 public boolean hasDate() { 2152 return this.date != null && !this.date.isEmpty(); 2153 } 2154 2155 /** 2156 * @param value {@link #date} (The date (and optionally time) when the measure was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2157 */ 2158 public Measure setDateElement(DateTimeType value) { 2159 this.date = value; 2160 return this; 2161 } 2162 2163 /** 2164 * @return The date (and optionally time) when the measure was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes. 2165 */ 2166 public Date getDate() { 2167 return this.date == null ? null : this.date.getValue(); 2168 } 2169 2170 /** 2171 * @param value The date (and optionally time) when the measure was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes. 2172 */ 2173 public Measure setDate(Date value) { 2174 if (value == null) 2175 this.date = null; 2176 else { 2177 if (this.date == null) 2178 this.date = new DateTimeType(); 2179 this.date.setValue(value); 2180 } 2181 return this; 2182 } 2183 2184 /** 2185 * @return {@link #publisher} (The name of the individual or organization that published the measure.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2186 */ 2187 public StringType getPublisherElement() { 2188 if (this.publisher == null) 2189 if (Configuration.errorOnAutoCreate()) 2190 throw new Error("Attempt to auto-create Measure.publisher"); 2191 else if (Configuration.doAutoCreate()) 2192 this.publisher = new StringType(); // bb 2193 return this.publisher; 2194 } 2195 2196 public boolean hasPublisherElement() { 2197 return this.publisher != null && !this.publisher.isEmpty(); 2198 } 2199 2200 public boolean hasPublisher() { 2201 return this.publisher != null && !this.publisher.isEmpty(); 2202 } 2203 2204 /** 2205 * @param value {@link #publisher} (The name of the individual or organization that published the measure.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 2206 */ 2207 public Measure setPublisherElement(StringType value) { 2208 this.publisher = value; 2209 return this; 2210 } 2211 2212 /** 2213 * @return The name of the individual or organization that published the measure. 2214 */ 2215 public String getPublisher() { 2216 return this.publisher == null ? null : this.publisher.getValue(); 2217 } 2218 2219 /** 2220 * @param value The name of the individual or organization that published the measure. 2221 */ 2222 public Measure setPublisher(String value) { 2223 if (Utilities.noString(value)) 2224 this.publisher = null; 2225 else { 2226 if (this.publisher == null) 2227 this.publisher = new StringType(); 2228 this.publisher.setValue(value); 2229 } 2230 return this; 2231 } 2232 2233 /** 2234 * @return {@link #description} (A free text natural language description of the measure from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2235 */ 2236 public MarkdownType getDescriptionElement() { 2237 if (this.description == null) 2238 if (Configuration.errorOnAutoCreate()) 2239 throw new Error("Attempt to auto-create Measure.description"); 2240 else if (Configuration.doAutoCreate()) 2241 this.description = new MarkdownType(); // bb 2242 return this.description; 2243 } 2244 2245 public boolean hasDescriptionElement() { 2246 return this.description != null && !this.description.isEmpty(); 2247 } 2248 2249 public boolean hasDescription() { 2250 return this.description != null && !this.description.isEmpty(); 2251 } 2252 2253 /** 2254 * @param value {@link #description} (A free text natural language description of the measure from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 2255 */ 2256 public Measure setDescriptionElement(MarkdownType value) { 2257 this.description = value; 2258 return this; 2259 } 2260 2261 /** 2262 * @return A free text natural language description of the measure from a consumer's perspective. 2263 */ 2264 public String getDescription() { 2265 return this.description == null ? null : this.description.getValue(); 2266 } 2267 2268 /** 2269 * @param value A free text natural language description of the measure from a consumer's perspective. 2270 */ 2271 public Measure setDescription(String value) { 2272 if (value == null) 2273 this.description = null; 2274 else { 2275 if (this.description == null) 2276 this.description = new MarkdownType(); 2277 this.description.setValue(value); 2278 } 2279 return this; 2280 } 2281 2282 /** 2283 * @return {@link #purpose} (Explaination of why this measure is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2284 */ 2285 public MarkdownType getPurposeElement() { 2286 if (this.purpose == null) 2287 if (Configuration.errorOnAutoCreate()) 2288 throw new Error("Attempt to auto-create Measure.purpose"); 2289 else if (Configuration.doAutoCreate()) 2290 this.purpose = new MarkdownType(); // bb 2291 return this.purpose; 2292 } 2293 2294 public boolean hasPurposeElement() { 2295 return this.purpose != null && !this.purpose.isEmpty(); 2296 } 2297 2298 public boolean hasPurpose() { 2299 return this.purpose != null && !this.purpose.isEmpty(); 2300 } 2301 2302 /** 2303 * @param value {@link #purpose} (Explaination of why this measure is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 2304 */ 2305 public Measure setPurposeElement(MarkdownType value) { 2306 this.purpose = value; 2307 return this; 2308 } 2309 2310 /** 2311 * @return Explaination of why this measure is needed and why it has been designed as it has. 2312 */ 2313 public String getPurpose() { 2314 return this.purpose == null ? null : this.purpose.getValue(); 2315 } 2316 2317 /** 2318 * @param value Explaination of why this measure is needed and why it has been designed as it has. 2319 */ 2320 public Measure setPurpose(String value) { 2321 if (value == null) 2322 this.purpose = null; 2323 else { 2324 if (this.purpose == null) 2325 this.purpose = new MarkdownType(); 2326 this.purpose.setValue(value); 2327 } 2328 return this; 2329 } 2330 2331 /** 2332 * @return {@link #usage} (A detailed description of how the measure is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 2333 */ 2334 public StringType getUsageElement() { 2335 if (this.usage == null) 2336 if (Configuration.errorOnAutoCreate()) 2337 throw new Error("Attempt to auto-create Measure.usage"); 2338 else if (Configuration.doAutoCreate()) 2339 this.usage = new StringType(); // bb 2340 return this.usage; 2341 } 2342 2343 public boolean hasUsageElement() { 2344 return this.usage != null && !this.usage.isEmpty(); 2345 } 2346 2347 public boolean hasUsage() { 2348 return this.usage != null && !this.usage.isEmpty(); 2349 } 2350 2351 /** 2352 * @param value {@link #usage} (A detailed description of how the measure is used from a clinical perspective.). This is the underlying object with id, value and extensions. The accessor "getUsage" gives direct access to the value 2353 */ 2354 public Measure setUsageElement(StringType value) { 2355 this.usage = value; 2356 return this; 2357 } 2358 2359 /** 2360 * @return A detailed description of how the measure is used from a clinical perspective. 2361 */ 2362 public String getUsage() { 2363 return this.usage == null ? null : this.usage.getValue(); 2364 } 2365 2366 /** 2367 * @param value A detailed description of how the measure is used from a clinical perspective. 2368 */ 2369 public Measure setUsage(String value) { 2370 if (Utilities.noString(value)) 2371 this.usage = null; 2372 else { 2373 if (this.usage == null) 2374 this.usage = new StringType(); 2375 this.usage.setValue(value); 2376 } 2377 return this; 2378 } 2379 2380 /** 2381 * @return {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2382 */ 2383 public DateType getApprovalDateElement() { 2384 if (this.approvalDate == null) 2385 if (Configuration.errorOnAutoCreate()) 2386 throw new Error("Attempt to auto-create Measure.approvalDate"); 2387 else if (Configuration.doAutoCreate()) 2388 this.approvalDate = new DateType(); // bb 2389 return this.approvalDate; 2390 } 2391 2392 public boolean hasApprovalDateElement() { 2393 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2394 } 2395 2396 public boolean hasApprovalDate() { 2397 return this.approvalDate != null && !this.approvalDate.isEmpty(); 2398 } 2399 2400 /** 2401 * @param value {@link #approvalDate} (The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.). This is the underlying object with id, value and extensions. The accessor "getApprovalDate" gives direct access to the value 2402 */ 2403 public Measure setApprovalDateElement(DateType value) { 2404 this.approvalDate = value; 2405 return this; 2406 } 2407 2408 /** 2409 * @return The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2410 */ 2411 public Date getApprovalDate() { 2412 return this.approvalDate == null ? null : this.approvalDate.getValue(); 2413 } 2414 2415 /** 2416 * @param value The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage. 2417 */ 2418 public Measure setApprovalDate(Date value) { 2419 if (value == null) 2420 this.approvalDate = null; 2421 else { 2422 if (this.approvalDate == null) 2423 this.approvalDate = new DateType(); 2424 this.approvalDate.setValue(value); 2425 } 2426 return this; 2427 } 2428 2429 /** 2430 * @return {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2431 */ 2432 public DateType getLastReviewDateElement() { 2433 if (this.lastReviewDate == null) 2434 if (Configuration.errorOnAutoCreate()) 2435 throw new Error("Attempt to auto-create Measure.lastReviewDate"); 2436 else if (Configuration.doAutoCreate()) 2437 this.lastReviewDate = new DateType(); // bb 2438 return this.lastReviewDate; 2439 } 2440 2441 public boolean hasLastReviewDateElement() { 2442 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2443 } 2444 2445 public boolean hasLastReviewDate() { 2446 return this.lastReviewDate != null && !this.lastReviewDate.isEmpty(); 2447 } 2448 2449 /** 2450 * @param value {@link #lastReviewDate} (The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.). This is the underlying object with id, value and extensions. The accessor "getLastReviewDate" gives direct access to the value 2451 */ 2452 public Measure setLastReviewDateElement(DateType value) { 2453 this.lastReviewDate = value; 2454 return this; 2455 } 2456 2457 /** 2458 * @return The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 2459 */ 2460 public Date getLastReviewDate() { 2461 return this.lastReviewDate == null ? null : this.lastReviewDate.getValue(); 2462 } 2463 2464 /** 2465 * @param value The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date. 2466 */ 2467 public Measure setLastReviewDate(Date value) { 2468 if (value == null) 2469 this.lastReviewDate = null; 2470 else { 2471 if (this.lastReviewDate == null) 2472 this.lastReviewDate = new DateType(); 2473 this.lastReviewDate.setValue(value); 2474 } 2475 return this; 2476 } 2477 2478 /** 2479 * @return {@link #effectivePeriod} (The period during which the measure content was or is planned to be in active use.) 2480 */ 2481 public Period getEffectivePeriod() { 2482 if (this.effectivePeriod == null) 2483 if (Configuration.errorOnAutoCreate()) 2484 throw new Error("Attempt to auto-create Measure.effectivePeriod"); 2485 else if (Configuration.doAutoCreate()) 2486 this.effectivePeriod = new Period(); // cc 2487 return this.effectivePeriod; 2488 } 2489 2490 public boolean hasEffectivePeriod() { 2491 return this.effectivePeriod != null && !this.effectivePeriod.isEmpty(); 2492 } 2493 2494 /** 2495 * @param value {@link #effectivePeriod} (The period during which the measure content was or is planned to be in active use.) 2496 */ 2497 public Measure setEffectivePeriod(Period value) { 2498 this.effectivePeriod = value; 2499 return this; 2500 } 2501 2502 /** 2503 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate measure instances.) 2504 */ 2505 public List<UsageContext> getUseContext() { 2506 if (this.useContext == null) 2507 this.useContext = new ArrayList<UsageContext>(); 2508 return this.useContext; 2509 } 2510 2511 /** 2512 * @return Returns a reference to <code>this</code> for easy method chaining 2513 */ 2514 public Measure setUseContext(List<UsageContext> theUseContext) { 2515 this.useContext = theUseContext; 2516 return this; 2517 } 2518 2519 public boolean hasUseContext() { 2520 if (this.useContext == null) 2521 return false; 2522 for (UsageContext item : this.useContext) 2523 if (!item.isEmpty()) 2524 return true; 2525 return false; 2526 } 2527 2528 public UsageContext addUseContext() { //3 2529 UsageContext t = new UsageContext(); 2530 if (this.useContext == null) 2531 this.useContext = new ArrayList<UsageContext>(); 2532 this.useContext.add(t); 2533 return t; 2534 } 2535 2536 public Measure addUseContext(UsageContext t) { //3 2537 if (t == null) 2538 return this; 2539 if (this.useContext == null) 2540 this.useContext = new ArrayList<UsageContext>(); 2541 this.useContext.add(t); 2542 return this; 2543 } 2544 2545 /** 2546 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 2547 */ 2548 public UsageContext getUseContextFirstRep() { 2549 if (getUseContext().isEmpty()) { 2550 addUseContext(); 2551 } 2552 return getUseContext().get(0); 2553 } 2554 2555 /** 2556 * @return {@link #jurisdiction} (A legal or geographic region in which the measure is intended to be used.) 2557 */ 2558 public List<CodeableConcept> getJurisdiction() { 2559 if (this.jurisdiction == null) 2560 this.jurisdiction = new ArrayList<CodeableConcept>(); 2561 return this.jurisdiction; 2562 } 2563 2564 /** 2565 * @return Returns a reference to <code>this</code> for easy method chaining 2566 */ 2567 public Measure setJurisdiction(List<CodeableConcept> theJurisdiction) { 2568 this.jurisdiction = theJurisdiction; 2569 return this; 2570 } 2571 2572 public boolean hasJurisdiction() { 2573 if (this.jurisdiction == null) 2574 return false; 2575 for (CodeableConcept item : this.jurisdiction) 2576 if (!item.isEmpty()) 2577 return true; 2578 return false; 2579 } 2580 2581 public CodeableConcept addJurisdiction() { //3 2582 CodeableConcept t = new CodeableConcept(); 2583 if (this.jurisdiction == null) 2584 this.jurisdiction = new ArrayList<CodeableConcept>(); 2585 this.jurisdiction.add(t); 2586 return t; 2587 } 2588 2589 public Measure addJurisdiction(CodeableConcept t) { //3 2590 if (t == null) 2591 return this; 2592 if (this.jurisdiction == null) 2593 this.jurisdiction = new ArrayList<CodeableConcept>(); 2594 this.jurisdiction.add(t); 2595 return this; 2596 } 2597 2598 /** 2599 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 2600 */ 2601 public CodeableConcept getJurisdictionFirstRep() { 2602 if (getJurisdiction().isEmpty()) { 2603 addJurisdiction(); 2604 } 2605 return getJurisdiction().get(0); 2606 } 2607 2608 /** 2609 * @return {@link #topic} (Descriptive topics related to the content of the measure. Topics provide a high-level categorization of the type of the measure that can be useful for filtering and searching.) 2610 */ 2611 public List<CodeableConcept> getTopic() { 2612 if (this.topic == null) 2613 this.topic = new ArrayList<CodeableConcept>(); 2614 return this.topic; 2615 } 2616 2617 /** 2618 * @return Returns a reference to <code>this</code> for easy method chaining 2619 */ 2620 public Measure setTopic(List<CodeableConcept> theTopic) { 2621 this.topic = theTopic; 2622 return this; 2623 } 2624 2625 public boolean hasTopic() { 2626 if (this.topic == null) 2627 return false; 2628 for (CodeableConcept item : this.topic) 2629 if (!item.isEmpty()) 2630 return true; 2631 return false; 2632 } 2633 2634 public CodeableConcept addTopic() { //3 2635 CodeableConcept t = new CodeableConcept(); 2636 if (this.topic == null) 2637 this.topic = new ArrayList<CodeableConcept>(); 2638 this.topic.add(t); 2639 return t; 2640 } 2641 2642 public Measure addTopic(CodeableConcept t) { //3 2643 if (t == null) 2644 return this; 2645 if (this.topic == null) 2646 this.topic = new ArrayList<CodeableConcept>(); 2647 this.topic.add(t); 2648 return this; 2649 } 2650 2651 /** 2652 * @return The first repetition of repeating field {@link #topic}, creating it if it does not already exist 2653 */ 2654 public CodeableConcept getTopicFirstRep() { 2655 if (getTopic().isEmpty()) { 2656 addTopic(); 2657 } 2658 return getTopic().get(0); 2659 } 2660 2661 /** 2662 * @return {@link #contributor} (A contributor to the content of the measure, including authors, editors, reviewers, and endorsers.) 2663 */ 2664 public List<Contributor> getContributor() { 2665 if (this.contributor == null) 2666 this.contributor = new ArrayList<Contributor>(); 2667 return this.contributor; 2668 } 2669 2670 /** 2671 * @return Returns a reference to <code>this</code> for easy method chaining 2672 */ 2673 public Measure setContributor(List<Contributor> theContributor) { 2674 this.contributor = theContributor; 2675 return this; 2676 } 2677 2678 public boolean hasContributor() { 2679 if (this.contributor == null) 2680 return false; 2681 for (Contributor item : this.contributor) 2682 if (!item.isEmpty()) 2683 return true; 2684 return false; 2685 } 2686 2687 public Contributor addContributor() { //3 2688 Contributor t = new Contributor(); 2689 if (this.contributor == null) 2690 this.contributor = new ArrayList<Contributor>(); 2691 this.contributor.add(t); 2692 return t; 2693 } 2694 2695 public Measure addContributor(Contributor t) { //3 2696 if (t == null) 2697 return this; 2698 if (this.contributor == null) 2699 this.contributor = new ArrayList<Contributor>(); 2700 this.contributor.add(t); 2701 return this; 2702 } 2703 2704 /** 2705 * @return The first repetition of repeating field {@link #contributor}, creating it if it does not already exist 2706 */ 2707 public Contributor getContributorFirstRep() { 2708 if (getContributor().isEmpty()) { 2709 addContributor(); 2710 } 2711 return getContributor().get(0); 2712 } 2713 2714 /** 2715 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 2716 */ 2717 public List<ContactDetail> getContact() { 2718 if (this.contact == null) 2719 this.contact = new ArrayList<ContactDetail>(); 2720 return this.contact; 2721 } 2722 2723 /** 2724 * @return Returns a reference to <code>this</code> for easy method chaining 2725 */ 2726 public Measure setContact(List<ContactDetail> theContact) { 2727 this.contact = theContact; 2728 return this; 2729 } 2730 2731 public boolean hasContact() { 2732 if (this.contact == null) 2733 return false; 2734 for (ContactDetail item : this.contact) 2735 if (!item.isEmpty()) 2736 return true; 2737 return false; 2738 } 2739 2740 public ContactDetail addContact() { //3 2741 ContactDetail t = new ContactDetail(); 2742 if (this.contact == null) 2743 this.contact = new ArrayList<ContactDetail>(); 2744 this.contact.add(t); 2745 return t; 2746 } 2747 2748 public Measure addContact(ContactDetail t) { //3 2749 if (t == null) 2750 return this; 2751 if (this.contact == null) 2752 this.contact = new ArrayList<ContactDetail>(); 2753 this.contact.add(t); 2754 return this; 2755 } 2756 2757 /** 2758 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 2759 */ 2760 public ContactDetail getContactFirstRep() { 2761 if (getContact().isEmpty()) { 2762 addContact(); 2763 } 2764 return getContact().get(0); 2765 } 2766 2767 /** 2768 * @return {@link #copyright} (A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2769 */ 2770 public MarkdownType getCopyrightElement() { 2771 if (this.copyright == null) 2772 if (Configuration.errorOnAutoCreate()) 2773 throw new Error("Attempt to auto-create Measure.copyright"); 2774 else if (Configuration.doAutoCreate()) 2775 this.copyright = new MarkdownType(); // bb 2776 return this.copyright; 2777 } 2778 2779 public boolean hasCopyrightElement() { 2780 return this.copyright != null && !this.copyright.isEmpty(); 2781 } 2782 2783 public boolean hasCopyright() { 2784 return this.copyright != null && !this.copyright.isEmpty(); 2785 } 2786 2787 /** 2788 * @param value {@link #copyright} (A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 2789 */ 2790 public Measure setCopyrightElement(MarkdownType value) { 2791 this.copyright = value; 2792 return this; 2793 } 2794 2795 /** 2796 * @return A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure. 2797 */ 2798 public String getCopyright() { 2799 return this.copyright == null ? null : this.copyright.getValue(); 2800 } 2801 2802 /** 2803 * @param value A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure. 2804 */ 2805 public Measure setCopyright(String value) { 2806 if (value == null) 2807 this.copyright = null; 2808 else { 2809 if (this.copyright == null) 2810 this.copyright = new MarkdownType(); 2811 this.copyright.setValue(value); 2812 } 2813 return this; 2814 } 2815 2816 /** 2817 * @return {@link #relatedArtifact} (Related artifacts such as additional documentation, justification, or bibliographic references.) 2818 */ 2819 public List<RelatedArtifact> getRelatedArtifact() { 2820 if (this.relatedArtifact == null) 2821 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2822 return this.relatedArtifact; 2823 } 2824 2825 /** 2826 * @return Returns a reference to <code>this</code> for easy method chaining 2827 */ 2828 public Measure setRelatedArtifact(List<RelatedArtifact> theRelatedArtifact) { 2829 this.relatedArtifact = theRelatedArtifact; 2830 return this; 2831 } 2832 2833 public boolean hasRelatedArtifact() { 2834 if (this.relatedArtifact == null) 2835 return false; 2836 for (RelatedArtifact item : this.relatedArtifact) 2837 if (!item.isEmpty()) 2838 return true; 2839 return false; 2840 } 2841 2842 public RelatedArtifact addRelatedArtifact() { //3 2843 RelatedArtifact t = new RelatedArtifact(); 2844 if (this.relatedArtifact == null) 2845 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2846 this.relatedArtifact.add(t); 2847 return t; 2848 } 2849 2850 public Measure addRelatedArtifact(RelatedArtifact t) { //3 2851 if (t == null) 2852 return this; 2853 if (this.relatedArtifact == null) 2854 this.relatedArtifact = new ArrayList<RelatedArtifact>(); 2855 this.relatedArtifact.add(t); 2856 return this; 2857 } 2858 2859 /** 2860 * @return The first repetition of repeating field {@link #relatedArtifact}, creating it if it does not already exist 2861 */ 2862 public RelatedArtifact getRelatedArtifactFirstRep() { 2863 if (getRelatedArtifact().isEmpty()) { 2864 addRelatedArtifact(); 2865 } 2866 return getRelatedArtifact().get(0); 2867 } 2868 2869 /** 2870 * @return {@link #library} (A reference to a Library resource containing the formal logic used by the measure.) 2871 */ 2872 public List<Reference> getLibrary() { 2873 if (this.library == null) 2874 this.library = new ArrayList<Reference>(); 2875 return this.library; 2876 } 2877 2878 /** 2879 * @return Returns a reference to <code>this</code> for easy method chaining 2880 */ 2881 public Measure setLibrary(List<Reference> theLibrary) { 2882 this.library = theLibrary; 2883 return this; 2884 } 2885 2886 public boolean hasLibrary() { 2887 if (this.library == null) 2888 return false; 2889 for (Reference item : this.library) 2890 if (!item.isEmpty()) 2891 return true; 2892 return false; 2893 } 2894 2895 public Reference addLibrary() { //3 2896 Reference t = new Reference(); 2897 if (this.library == null) 2898 this.library = new ArrayList<Reference>(); 2899 this.library.add(t); 2900 return t; 2901 } 2902 2903 public Measure addLibrary(Reference t) { //3 2904 if (t == null) 2905 return this; 2906 if (this.library == null) 2907 this.library = new ArrayList<Reference>(); 2908 this.library.add(t); 2909 return this; 2910 } 2911 2912 /** 2913 * @return The first repetition of repeating field {@link #library}, creating it if it does not already exist 2914 */ 2915 public Reference getLibraryFirstRep() { 2916 if (getLibrary().isEmpty()) { 2917 addLibrary(); 2918 } 2919 return getLibrary().get(0); 2920 } 2921 2922 /** 2923 * @deprecated Use Reference#setResource(IBaseResource) instead 2924 */ 2925 @Deprecated 2926 public List<Library> getLibraryTarget() { 2927 if (this.libraryTarget == null) 2928 this.libraryTarget = new ArrayList<Library>(); 2929 return this.libraryTarget; 2930 } 2931 2932 /** 2933 * @deprecated Use Reference#setResource(IBaseResource) instead 2934 */ 2935 @Deprecated 2936 public Library addLibraryTarget() { 2937 Library r = new Library(); 2938 if (this.libraryTarget == null) 2939 this.libraryTarget = new ArrayList<Library>(); 2940 this.libraryTarget.add(r); 2941 return r; 2942 } 2943 2944 /** 2945 * @return {@link #disclaimer} (Notices and disclaimers regarding the use of the measure, or related to intellectual property (such as code systems) referenced by the measure.). This is the underlying object with id, value and extensions. The accessor "getDisclaimer" gives direct access to the value 2946 */ 2947 public MarkdownType getDisclaimerElement() { 2948 if (this.disclaimer == null) 2949 if (Configuration.errorOnAutoCreate()) 2950 throw new Error("Attempt to auto-create Measure.disclaimer"); 2951 else if (Configuration.doAutoCreate()) 2952 this.disclaimer = new MarkdownType(); // bb 2953 return this.disclaimer; 2954 } 2955 2956 public boolean hasDisclaimerElement() { 2957 return this.disclaimer != null && !this.disclaimer.isEmpty(); 2958 } 2959 2960 public boolean hasDisclaimer() { 2961 return this.disclaimer != null && !this.disclaimer.isEmpty(); 2962 } 2963 2964 /** 2965 * @param value {@link #disclaimer} (Notices and disclaimers regarding the use of the measure, or related to intellectual property (such as code systems) referenced by the measure.). This is the underlying object with id, value and extensions. The accessor "getDisclaimer" gives direct access to the value 2966 */ 2967 public Measure setDisclaimerElement(MarkdownType value) { 2968 this.disclaimer = value; 2969 return this; 2970 } 2971 2972 /** 2973 * @return Notices and disclaimers regarding the use of the measure, or related to intellectual property (such as code systems) referenced by the measure. 2974 */ 2975 public String getDisclaimer() { 2976 return this.disclaimer == null ? null : this.disclaimer.getValue(); 2977 } 2978 2979 /** 2980 * @param value Notices and disclaimers regarding the use of the measure, or related to intellectual property (such as code systems) referenced by the measure. 2981 */ 2982 public Measure setDisclaimer(String value) { 2983 if (value == null) 2984 this.disclaimer = null; 2985 else { 2986 if (this.disclaimer == null) 2987 this.disclaimer = new MarkdownType(); 2988 this.disclaimer.setValue(value); 2989 } 2990 return this; 2991 } 2992 2993 /** 2994 * @return {@link #scoring} (Indicates how the calculation is performed for the measure, including proportion, ratio, continuous variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.) 2995 */ 2996 public CodeableConcept getScoring() { 2997 if (this.scoring == null) 2998 if (Configuration.errorOnAutoCreate()) 2999 throw new Error("Attempt to auto-create Measure.scoring"); 3000 else if (Configuration.doAutoCreate()) 3001 this.scoring = new CodeableConcept(); // cc 3002 return this.scoring; 3003 } 3004 3005 public boolean hasScoring() { 3006 return this.scoring != null && !this.scoring.isEmpty(); 3007 } 3008 3009 /** 3010 * @param value {@link #scoring} (Indicates how the calculation is performed for the measure, including proportion, ratio, continuous variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.) 3011 */ 3012 public Measure setScoring(CodeableConcept value) { 3013 this.scoring = value; 3014 return this; 3015 } 3016 3017 /** 3018 * @return {@link #compositeScoring} (If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.) 3019 */ 3020 public CodeableConcept getCompositeScoring() { 3021 if (this.compositeScoring == null) 3022 if (Configuration.errorOnAutoCreate()) 3023 throw new Error("Attempt to auto-create Measure.compositeScoring"); 3024 else if (Configuration.doAutoCreate()) 3025 this.compositeScoring = new CodeableConcept(); // cc 3026 return this.compositeScoring; 3027 } 3028 3029 public boolean hasCompositeScoring() { 3030 return this.compositeScoring != null && !this.compositeScoring.isEmpty(); 3031 } 3032 3033 /** 3034 * @param value {@link #compositeScoring} (If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.) 3035 */ 3036 public Measure setCompositeScoring(CodeableConcept value) { 3037 this.compositeScoring = value; 3038 return this; 3039 } 3040 3041 /** 3042 * @return {@link #type} (Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.) 3043 */ 3044 public List<CodeableConcept> getType() { 3045 if (this.type == null) 3046 this.type = new ArrayList<CodeableConcept>(); 3047 return this.type; 3048 } 3049 3050 /** 3051 * @return Returns a reference to <code>this</code> for easy method chaining 3052 */ 3053 public Measure setType(List<CodeableConcept> theType) { 3054 this.type = theType; 3055 return this; 3056 } 3057 3058 public boolean hasType() { 3059 if (this.type == null) 3060 return false; 3061 for (CodeableConcept item : this.type) 3062 if (!item.isEmpty()) 3063 return true; 3064 return false; 3065 } 3066 3067 public CodeableConcept addType() { //3 3068 CodeableConcept t = new CodeableConcept(); 3069 if (this.type == null) 3070 this.type = new ArrayList<CodeableConcept>(); 3071 this.type.add(t); 3072 return t; 3073 } 3074 3075 public Measure addType(CodeableConcept t) { //3 3076 if (t == null) 3077 return this; 3078 if (this.type == null) 3079 this.type = new ArrayList<CodeableConcept>(); 3080 this.type.add(t); 3081 return this; 3082 } 3083 3084 /** 3085 * @return The first repetition of repeating field {@link #type}, creating it if it does not already exist 3086 */ 3087 public CodeableConcept getTypeFirstRep() { 3088 if (getType().isEmpty()) { 3089 addType(); 3090 } 3091 return getType().get(0); 3092 } 3093 3094 /** 3095 * @return {@link #riskAdjustment} (A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.). This is the underlying object with id, value and extensions. The accessor "getRiskAdjustment" gives direct access to the value 3096 */ 3097 public StringType getRiskAdjustmentElement() { 3098 if (this.riskAdjustment == null) 3099 if (Configuration.errorOnAutoCreate()) 3100 throw new Error("Attempt to auto-create Measure.riskAdjustment"); 3101 else if (Configuration.doAutoCreate()) 3102 this.riskAdjustment = new StringType(); // bb 3103 return this.riskAdjustment; 3104 } 3105 3106 public boolean hasRiskAdjustmentElement() { 3107 return this.riskAdjustment != null && !this.riskAdjustment.isEmpty(); 3108 } 3109 3110 public boolean hasRiskAdjustment() { 3111 return this.riskAdjustment != null && !this.riskAdjustment.isEmpty(); 3112 } 3113 3114 /** 3115 * @param value {@link #riskAdjustment} (A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.). This is the underlying object with id, value and extensions. The accessor "getRiskAdjustment" gives direct access to the value 3116 */ 3117 public Measure setRiskAdjustmentElement(StringType value) { 3118 this.riskAdjustment = value; 3119 return this; 3120 } 3121 3122 /** 3123 * @return A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results. 3124 */ 3125 public String getRiskAdjustment() { 3126 return this.riskAdjustment == null ? null : this.riskAdjustment.getValue(); 3127 } 3128 3129 /** 3130 * @param value A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results. 3131 */ 3132 public Measure setRiskAdjustment(String value) { 3133 if (Utilities.noString(value)) 3134 this.riskAdjustment = null; 3135 else { 3136 if (this.riskAdjustment == null) 3137 this.riskAdjustment = new StringType(); 3138 this.riskAdjustment.setValue(value); 3139 } 3140 return this; 3141 } 3142 3143 /** 3144 * @return {@link #rateAggregation} (Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.). This is the underlying object with id, value and extensions. The accessor "getRateAggregation" gives direct access to the value 3145 */ 3146 public StringType getRateAggregationElement() { 3147 if (this.rateAggregation == null) 3148 if (Configuration.errorOnAutoCreate()) 3149 throw new Error("Attempt to auto-create Measure.rateAggregation"); 3150 else if (Configuration.doAutoCreate()) 3151 this.rateAggregation = new StringType(); // bb 3152 return this.rateAggregation; 3153 } 3154 3155 public boolean hasRateAggregationElement() { 3156 return this.rateAggregation != null && !this.rateAggregation.isEmpty(); 3157 } 3158 3159 public boolean hasRateAggregation() { 3160 return this.rateAggregation != null && !this.rateAggregation.isEmpty(); 3161 } 3162 3163 /** 3164 * @param value {@link #rateAggregation} (Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.). This is the underlying object with id, value and extensions. The accessor "getRateAggregation" gives direct access to the value 3165 */ 3166 public Measure setRateAggregationElement(StringType value) { 3167 this.rateAggregation = value; 3168 return this; 3169 } 3170 3171 /** 3172 * @return Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 3173 */ 3174 public String getRateAggregation() { 3175 return this.rateAggregation == null ? null : this.rateAggregation.getValue(); 3176 } 3177 3178 /** 3179 * @param value Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result. 3180 */ 3181 public Measure setRateAggregation(String value) { 3182 if (Utilities.noString(value)) 3183 this.rateAggregation = null; 3184 else { 3185 if (this.rateAggregation == null) 3186 this.rateAggregation = new StringType(); 3187 this.rateAggregation.setValue(value); 3188 } 3189 return this; 3190 } 3191 3192 /** 3193 * @return {@link #rationale} (Provides a succint statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.). This is the underlying object with id, value and extensions. The accessor "getRationale" gives direct access to the value 3194 */ 3195 public MarkdownType getRationaleElement() { 3196 if (this.rationale == null) 3197 if (Configuration.errorOnAutoCreate()) 3198 throw new Error("Attempt to auto-create Measure.rationale"); 3199 else if (Configuration.doAutoCreate()) 3200 this.rationale = new MarkdownType(); // bb 3201 return this.rationale; 3202 } 3203 3204 public boolean hasRationaleElement() { 3205 return this.rationale != null && !this.rationale.isEmpty(); 3206 } 3207 3208 public boolean hasRationale() { 3209 return this.rationale != null && !this.rationale.isEmpty(); 3210 } 3211 3212 /** 3213 * @param value {@link #rationale} (Provides a succint statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.). This is the underlying object with id, value and extensions. The accessor "getRationale" gives direct access to the value 3214 */ 3215 public Measure setRationaleElement(MarkdownType value) { 3216 this.rationale = value; 3217 return this; 3218 } 3219 3220 /** 3221 * @return Provides a succint statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence. 3222 */ 3223 public String getRationale() { 3224 return this.rationale == null ? null : this.rationale.getValue(); 3225 } 3226 3227 /** 3228 * @param value Provides a succint statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence. 3229 */ 3230 public Measure setRationale(String value) { 3231 if (value == null) 3232 this.rationale = null; 3233 else { 3234 if (this.rationale == null) 3235 this.rationale = new MarkdownType(); 3236 this.rationale.setValue(value); 3237 } 3238 return this; 3239 } 3240 3241 /** 3242 * @return {@link #clinicalRecommendationStatement} (Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.). This is the underlying object with id, value and extensions. The accessor "getClinicalRecommendationStatement" gives direct access to the value 3243 */ 3244 public MarkdownType getClinicalRecommendationStatementElement() { 3245 if (this.clinicalRecommendationStatement == null) 3246 if (Configuration.errorOnAutoCreate()) 3247 throw new Error("Attempt to auto-create Measure.clinicalRecommendationStatement"); 3248 else if (Configuration.doAutoCreate()) 3249 this.clinicalRecommendationStatement = new MarkdownType(); // bb 3250 return this.clinicalRecommendationStatement; 3251 } 3252 3253 public boolean hasClinicalRecommendationStatementElement() { 3254 return this.clinicalRecommendationStatement != null && !this.clinicalRecommendationStatement.isEmpty(); 3255 } 3256 3257 public boolean hasClinicalRecommendationStatement() { 3258 return this.clinicalRecommendationStatement != null && !this.clinicalRecommendationStatement.isEmpty(); 3259 } 3260 3261 /** 3262 * @param value {@link #clinicalRecommendationStatement} (Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.). This is the underlying object with id, value and extensions. The accessor "getClinicalRecommendationStatement" gives direct access to the value 3263 */ 3264 public Measure setClinicalRecommendationStatementElement(MarkdownType value) { 3265 this.clinicalRecommendationStatement = value; 3266 return this; 3267 } 3268 3269 /** 3270 * @return Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure. 3271 */ 3272 public String getClinicalRecommendationStatement() { 3273 return this.clinicalRecommendationStatement == null ? null : this.clinicalRecommendationStatement.getValue(); 3274 } 3275 3276 /** 3277 * @param value Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure. 3278 */ 3279 public Measure setClinicalRecommendationStatement(String value) { 3280 if (value == null) 3281 this.clinicalRecommendationStatement = null; 3282 else { 3283 if (this.clinicalRecommendationStatement == null) 3284 this.clinicalRecommendationStatement = new MarkdownType(); 3285 this.clinicalRecommendationStatement.setValue(value); 3286 } 3287 return this; 3288 } 3289 3290 /** 3291 * @return {@link #improvementNotation} (Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is whthin a range).). This is the underlying object with id, value and extensions. The accessor "getImprovementNotation" gives direct access to the value 3292 */ 3293 public StringType getImprovementNotationElement() { 3294 if (this.improvementNotation == null) 3295 if (Configuration.errorOnAutoCreate()) 3296 throw new Error("Attempt to auto-create Measure.improvementNotation"); 3297 else if (Configuration.doAutoCreate()) 3298 this.improvementNotation = new StringType(); // bb 3299 return this.improvementNotation; 3300 } 3301 3302 public boolean hasImprovementNotationElement() { 3303 return this.improvementNotation != null && !this.improvementNotation.isEmpty(); 3304 } 3305 3306 public boolean hasImprovementNotation() { 3307 return this.improvementNotation != null && !this.improvementNotation.isEmpty(); 3308 } 3309 3310 /** 3311 * @param value {@link #improvementNotation} (Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is whthin a range).). This is the underlying object with id, value and extensions. The accessor "getImprovementNotation" gives direct access to the value 3312 */ 3313 public Measure setImprovementNotationElement(StringType value) { 3314 this.improvementNotation = value; 3315 return this; 3316 } 3317 3318 /** 3319 * @return Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is whthin a range). 3320 */ 3321 public String getImprovementNotation() { 3322 return this.improvementNotation == null ? null : this.improvementNotation.getValue(); 3323 } 3324 3325 /** 3326 * @param value Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is whthin a range). 3327 */ 3328 public Measure setImprovementNotation(String value) { 3329 if (Utilities.noString(value)) 3330 this.improvementNotation = null; 3331 else { 3332 if (this.improvementNotation == null) 3333 this.improvementNotation = new StringType(); 3334 this.improvementNotation.setValue(value); 3335 } 3336 return this; 3337 } 3338 3339 /** 3340 * @return {@link #definition} (Provides a description of an individual term used within the measure.) 3341 */ 3342 public List<MarkdownType> getDefinition() { 3343 if (this.definition == null) 3344 this.definition = new ArrayList<MarkdownType>(); 3345 return this.definition; 3346 } 3347 3348 /** 3349 * @return Returns a reference to <code>this</code> for easy method chaining 3350 */ 3351 public Measure setDefinition(List<MarkdownType> theDefinition) { 3352 this.definition = theDefinition; 3353 return this; 3354 } 3355 3356 public boolean hasDefinition() { 3357 if (this.definition == null) 3358 return false; 3359 for (MarkdownType item : this.definition) 3360 if (!item.isEmpty()) 3361 return true; 3362 return false; 3363 } 3364 3365 /** 3366 * @return {@link #definition} (Provides a description of an individual term used within the measure.) 3367 */ 3368 public MarkdownType addDefinitionElement() {//2 3369 MarkdownType t = new MarkdownType(); 3370 if (this.definition == null) 3371 this.definition = new ArrayList<MarkdownType>(); 3372 this.definition.add(t); 3373 return t; 3374 } 3375 3376 /** 3377 * @param value {@link #definition} (Provides a description of an individual term used within the measure.) 3378 */ 3379 public Measure addDefinition(String value) { //1 3380 MarkdownType t = new MarkdownType(); 3381 t.setValue(value); 3382 if (this.definition == null) 3383 this.definition = new ArrayList<MarkdownType>(); 3384 this.definition.add(t); 3385 return this; 3386 } 3387 3388 /** 3389 * @param value {@link #definition} (Provides a description of an individual term used within the measure.) 3390 */ 3391 public boolean hasDefinition(String value) { 3392 if (this.definition == null) 3393 return false; 3394 for (MarkdownType v : this.definition) 3395 if (v.getValue().equals(value)) // markdown 3396 return true; 3397 return false; 3398 } 3399 3400 /** 3401 * @return {@link #guidance} (Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.). This is the underlying object with id, value and extensions. The accessor "getGuidance" gives direct access to the value 3402 */ 3403 public MarkdownType getGuidanceElement() { 3404 if (this.guidance == null) 3405 if (Configuration.errorOnAutoCreate()) 3406 throw new Error("Attempt to auto-create Measure.guidance"); 3407 else if (Configuration.doAutoCreate()) 3408 this.guidance = new MarkdownType(); // bb 3409 return this.guidance; 3410 } 3411 3412 public boolean hasGuidanceElement() { 3413 return this.guidance != null && !this.guidance.isEmpty(); 3414 } 3415 3416 public boolean hasGuidance() { 3417 return this.guidance != null && !this.guidance.isEmpty(); 3418 } 3419 3420 /** 3421 * @param value {@link #guidance} (Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.). This is the underlying object with id, value and extensions. The accessor "getGuidance" gives direct access to the value 3422 */ 3423 public Measure setGuidanceElement(MarkdownType value) { 3424 this.guidance = value; 3425 return this; 3426 } 3427 3428 /** 3429 * @return Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure. 3430 */ 3431 public String getGuidance() { 3432 return this.guidance == null ? null : this.guidance.getValue(); 3433 } 3434 3435 /** 3436 * @param value Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure. 3437 */ 3438 public Measure setGuidance(String value) { 3439 if (value == null) 3440 this.guidance = null; 3441 else { 3442 if (this.guidance == null) 3443 this.guidance = new MarkdownType(); 3444 this.guidance.setValue(value); 3445 } 3446 return this; 3447 } 3448 3449 /** 3450 * @return {@link #set} (The measure set, e.g. Preventive Care and Screening.). This is the underlying object with id, value and extensions. The accessor "getSet" gives direct access to the value 3451 */ 3452 public StringType getSetElement() { 3453 if (this.set == null) 3454 if (Configuration.errorOnAutoCreate()) 3455 throw new Error("Attempt to auto-create Measure.set"); 3456 else if (Configuration.doAutoCreate()) 3457 this.set = new StringType(); // bb 3458 return this.set; 3459 } 3460 3461 public boolean hasSetElement() { 3462 return this.set != null && !this.set.isEmpty(); 3463 } 3464 3465 public boolean hasSet() { 3466 return this.set != null && !this.set.isEmpty(); 3467 } 3468 3469 /** 3470 * @param value {@link #set} (The measure set, e.g. Preventive Care and Screening.). This is the underlying object with id, value and extensions. The accessor "getSet" gives direct access to the value 3471 */ 3472 public Measure setSetElement(StringType value) { 3473 this.set = value; 3474 return this; 3475 } 3476 3477 /** 3478 * @return The measure set, e.g. Preventive Care and Screening. 3479 */ 3480 public String getSet() { 3481 return this.set == null ? null : this.set.getValue(); 3482 } 3483 3484 /** 3485 * @param value The measure set, e.g. Preventive Care and Screening. 3486 */ 3487 public Measure setSet(String value) { 3488 if (Utilities.noString(value)) 3489 this.set = null; 3490 else { 3491 if (this.set == null) 3492 this.set = new StringType(); 3493 this.set.setValue(value); 3494 } 3495 return this; 3496 } 3497 3498 /** 3499 * @return {@link #group} (A group of population criteria for the measure.) 3500 */ 3501 public List<MeasureGroupComponent> getGroup() { 3502 if (this.group == null) 3503 this.group = new ArrayList<MeasureGroupComponent>(); 3504 return this.group; 3505 } 3506 3507 /** 3508 * @return Returns a reference to <code>this</code> for easy method chaining 3509 */ 3510 public Measure setGroup(List<MeasureGroupComponent> theGroup) { 3511 this.group = theGroup; 3512 return this; 3513 } 3514 3515 public boolean hasGroup() { 3516 if (this.group == null) 3517 return false; 3518 for (MeasureGroupComponent item : this.group) 3519 if (!item.isEmpty()) 3520 return true; 3521 return false; 3522 } 3523 3524 public MeasureGroupComponent addGroup() { //3 3525 MeasureGroupComponent t = new MeasureGroupComponent(); 3526 if (this.group == null) 3527 this.group = new ArrayList<MeasureGroupComponent>(); 3528 this.group.add(t); 3529 return t; 3530 } 3531 3532 public Measure addGroup(MeasureGroupComponent t) { //3 3533 if (t == null) 3534 return this; 3535 if (this.group == null) 3536 this.group = new ArrayList<MeasureGroupComponent>(); 3537 this.group.add(t); 3538 return this; 3539 } 3540 3541 /** 3542 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist 3543 */ 3544 public MeasureGroupComponent getGroupFirstRep() { 3545 if (getGroup().isEmpty()) { 3546 addGroup(); 3547 } 3548 return getGroup().get(0); 3549 } 3550 3551 /** 3552 * @return {@link #supplementalData} (The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.) 3553 */ 3554 public List<MeasureSupplementalDataComponent> getSupplementalData() { 3555 if (this.supplementalData == null) 3556 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 3557 return this.supplementalData; 3558 } 3559 3560 /** 3561 * @return Returns a reference to <code>this</code> for easy method chaining 3562 */ 3563 public Measure setSupplementalData(List<MeasureSupplementalDataComponent> theSupplementalData) { 3564 this.supplementalData = theSupplementalData; 3565 return this; 3566 } 3567 3568 public boolean hasSupplementalData() { 3569 if (this.supplementalData == null) 3570 return false; 3571 for (MeasureSupplementalDataComponent item : this.supplementalData) 3572 if (!item.isEmpty()) 3573 return true; 3574 return false; 3575 } 3576 3577 public MeasureSupplementalDataComponent addSupplementalData() { //3 3578 MeasureSupplementalDataComponent t = new MeasureSupplementalDataComponent(); 3579 if (this.supplementalData == null) 3580 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 3581 this.supplementalData.add(t); 3582 return t; 3583 } 3584 3585 public Measure addSupplementalData(MeasureSupplementalDataComponent t) { //3 3586 if (t == null) 3587 return this; 3588 if (this.supplementalData == null) 3589 this.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 3590 this.supplementalData.add(t); 3591 return this; 3592 } 3593 3594 /** 3595 * @return The first repetition of repeating field {@link #supplementalData}, creating it if it does not already exist 3596 */ 3597 public MeasureSupplementalDataComponent getSupplementalDataFirstRep() { 3598 if (getSupplementalData().isEmpty()) { 3599 addSupplementalData(); 3600 } 3601 return getSupplementalData().get(0); 3602 } 3603 3604 protected void listChildren(List<Property> children) { 3605 super.listChildren(children); 3606 children.add(new Property("url", "uri", "An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this measure is (or will be) published. The URL SHOULD include the major version of the measure. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 3607 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier)); 3608 children.add(new Property("version", "string", "The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version)); 3609 children.add(new Property("name", "string", "A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 3610 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the measure.", 0, 1, title)); 3611 children.add(new Property("status", "code", "The status of this measure. Enables tracking the life-cycle of the content.", 0, 1, status)); 3612 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 3613 children.add(new Property("date", "dateTime", "The date (and optionally time) when the measure was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.", 0, 1, date)); 3614 children.add(new Property("publisher", "string", "The name of the individual or organization that published the measure.", 0, 1, publisher)); 3615 children.add(new Property("description", "markdown", "A free text natural language description of the measure from a consumer's perspective.", 0, 1, description)); 3616 children.add(new Property("purpose", "markdown", "Explaination of why this measure is needed and why it has been designed as it has.", 0, 1, purpose)); 3617 children.add(new Property("usage", "string", "A detailed description of how the measure is used from a clinical perspective.", 0, 1, usage)); 3618 children.add(new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate)); 3619 children.add(new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate)); 3620 children.add(new Property("effectivePeriod", "Period", "The period during which the measure content was or is planned to be in active use.", 0, 1, effectivePeriod)); 3621 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate measure instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 3622 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the measure is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 3623 children.add(new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the measure. Topics provide a high-level categorization of the type of the measure that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic)); 3624 children.add(new Property("contributor", "Contributor", "A contributor to the content of the measure, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor)); 3625 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 3626 children.add(new Property("copyright", "markdown", "A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.", 0, 1, copyright)); 3627 children.add(new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact)); 3628 children.add(new Property("library", "Reference(Library)", "A reference to a Library resource containing the formal logic used by the measure.", 0, java.lang.Integer.MAX_VALUE, library)); 3629 children.add(new Property("disclaimer", "markdown", "Notices and disclaimers regarding the use of the measure, or related to intellectual property (such as code systems) referenced by the measure.", 0, 1, disclaimer)); 3630 children.add(new Property("scoring", "CodeableConcept", "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.", 0, 1, scoring)); 3631 children.add(new Property("compositeScoring", "CodeableConcept", "If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.", 0, 1, compositeScoring)); 3632 children.add(new Property("type", "CodeableConcept", "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.", 0, java.lang.Integer.MAX_VALUE, type)); 3633 children.add(new Property("riskAdjustment", "string", "A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.", 0, 1, riskAdjustment)); 3634 children.add(new Property("rateAggregation", "string", "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.", 0, 1, rateAggregation)); 3635 children.add(new Property("rationale", "markdown", "Provides a succint statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.", 0, 1, rationale)); 3636 children.add(new Property("clinicalRecommendationStatement", "markdown", "Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.", 0, 1, clinicalRecommendationStatement)); 3637 children.add(new Property("improvementNotation", "string", "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is whthin a range).", 0, 1, improvementNotation)); 3638 children.add(new Property("definition", "markdown", "Provides a description of an individual term used within the measure.", 0, java.lang.Integer.MAX_VALUE, definition)); 3639 children.add(new Property("guidance", "markdown", "Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.", 0, 1, guidance)); 3640 children.add(new Property("set", "string", "The measure set, e.g. Preventive Care and Screening.", 0, 1, set)); 3641 children.add(new Property("group", "", "A group of population criteria for the measure.", 0, java.lang.Integer.MAX_VALUE, group)); 3642 children.add(new Property("supplementalData", "", "The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, supplementalData)); 3643 } 3644 3645 @Override 3646 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 3647 switch (_hash) { 3648 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this measure when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this measure is (or will be) published. The URL SHOULD include the major version of the measure. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 3649 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this measure when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, java.lang.Integer.MAX_VALUE, identifier); 3650 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the measure when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the measure author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. To provide a version consistent with the Decision Support Service specification, use the format Major.Minor.Revision (e.g. 1.0.0). For more information on versioning knowledge assets, refer to the Decision Support Service specification. Note that a version is required for non-experimental active artifacts.", 0, 1, version); 3651 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the measure. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 3652 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the measure.", 0, 1, title); 3653 case -892481550: /*status*/ return new Property("status", "code", "The status of this measure. Enables tracking the life-cycle of the content.", 0, 1, status); 3654 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this measure is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 3655 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the measure was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the measure changes.", 0, 1, date); 3656 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the measure.", 0, 1, publisher); 3657 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the measure from a consumer's perspective.", 0, 1, description); 3658 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this measure is needed and why it has been designed as it has.", 0, 1, purpose); 3659 case 111574433: /*usage*/ return new Property("usage", "string", "A detailed description of how the measure is used from a clinical perspective.", 0, 1, usage); 3660 case 223539345: /*approvalDate*/ return new Property("approvalDate", "date", "The date on which the resource content was approved by the publisher. Approval happens once when the content is officially approved for usage.", 0, 1, approvalDate); 3661 case -1687512484: /*lastReviewDate*/ return new Property("lastReviewDate", "date", "The date on which the resource content was last reviewed. Review happens periodically after approval, but doesn't change the original approval date.", 0, 1, lastReviewDate); 3662 case -403934648: /*effectivePeriod*/ return new Property("effectivePeriod", "Period", "The period during which the measure content was or is planned to be in active use.", 0, 1, effectivePeriod); 3663 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate measure instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 3664 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the measure is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 3665 case 110546223: /*topic*/ return new Property("topic", "CodeableConcept", "Descriptive topics related to the content of the measure. Topics provide a high-level categorization of the type of the measure that can be useful for filtering and searching.", 0, java.lang.Integer.MAX_VALUE, topic); 3666 case -1895276325: /*contributor*/ return new Property("contributor", "Contributor", "A contributor to the content of the measure, including authors, editors, reviewers, and endorsers.", 0, java.lang.Integer.MAX_VALUE, contributor); 3667 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 3668 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the measure and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the measure.", 0, 1, copyright); 3669 case 666807069: /*relatedArtifact*/ return new Property("relatedArtifact", "RelatedArtifact", "Related artifacts such as additional documentation, justification, or bibliographic references.", 0, java.lang.Integer.MAX_VALUE, relatedArtifact); 3670 case 166208699: /*library*/ return new Property("library", "Reference(Library)", "A reference to a Library resource containing the formal logic used by the measure.", 0, java.lang.Integer.MAX_VALUE, library); 3671 case 432371099: /*disclaimer*/ return new Property("disclaimer", "markdown", "Notices and disclaimers regarding the use of the measure, or related to intellectual property (such as code systems) referenced by the measure.", 0, 1, disclaimer); 3672 case 1924005583: /*scoring*/ return new Property("scoring", "CodeableConcept", "Indicates how the calculation is performed for the measure, including proportion, ratio, continuous variable, and cohort. The value set is extensible, allowing additional measure scoring types to be represented.", 0, 1, scoring); 3673 case 569347656: /*compositeScoring*/ return new Property("compositeScoring", "CodeableConcept", "If this is a composite measure, the scoring method used to combine the component measures to determine the composite score.", 0, 1, compositeScoring); 3674 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates whether the measure is used to examine a process, an outcome over time, a patient-reported outcome, or a structure measure such as utilization.", 0, java.lang.Integer.MAX_VALUE, type); 3675 case 93273500: /*riskAdjustment*/ return new Property("riskAdjustment", "string", "A description of the risk adjustment factors that may impact the resulting score for the measure and how they may be accounted for when computing and reporting measure results.", 0, 1, riskAdjustment); 3676 case 1254503906: /*rateAggregation*/ return new Property("rateAggregation", "string", "Describes how to combine the information calculated, based on logic in each of several populations, into one summarized result.", 0, 1, rateAggregation); 3677 case 345689335: /*rationale*/ return new Property("rationale", "markdown", "Provides a succint statement of the need for the measure. Usually includes statements pertaining to importance criterion: impact, gap in care, and evidence.", 0, 1, rationale); 3678 case -18631389: /*clinicalRecommendationStatement*/ return new Property("clinicalRecommendationStatement", "markdown", "Provides a summary of relevant clinical guidelines or other clinical recommendations supporting the measure.", 0, 1, clinicalRecommendationStatement); 3679 case -2085456136: /*improvementNotation*/ return new Property("improvementNotation", "string", "Information on whether an increase or decrease in score is the preferred result (e.g., a higher score indicates better quality OR a lower score indicates better quality OR quality is whthin a range).", 0, 1, improvementNotation); 3680 case -1014418093: /*definition*/ return new Property("definition", "markdown", "Provides a description of an individual term used within the measure.", 0, java.lang.Integer.MAX_VALUE, definition); 3681 case -1314002088: /*guidance*/ return new Property("guidance", "markdown", "Additional guidance for the measure including how it can be used in a clinical context, and the intent of the measure.", 0, 1, guidance); 3682 case 113762: /*set*/ return new Property("set", "string", "The measure set, e.g. Preventive Care and Screening.", 0, 1, set); 3683 case 98629247: /*group*/ return new Property("group", "", "A group of population criteria for the measure.", 0, java.lang.Integer.MAX_VALUE, group); 3684 case 1447496814: /*supplementalData*/ return new Property("supplementalData", "", "The supplemental data criteria for the measure report, specified as either the name of a valid CQL expression within a referenced library, or a valid FHIR Resource Path.", 0, java.lang.Integer.MAX_VALUE, supplementalData); 3685 default: return super.getNamedProperty(_hash, _name, _checkValid); 3686 } 3687 3688 } 3689 3690 @Override 3691 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 3692 switch (hash) { 3693 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 3694 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 3695 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 3696 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 3697 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 3698 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 3699 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 3700 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 3701 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 3702 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 3703 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 3704 case 111574433: /*usage*/ return this.usage == null ? new Base[0] : new Base[] {this.usage}; // StringType 3705 case 223539345: /*approvalDate*/ return this.approvalDate == null ? new Base[0] : new Base[] {this.approvalDate}; // DateType 3706 case -1687512484: /*lastReviewDate*/ return this.lastReviewDate == null ? new Base[0] : new Base[] {this.lastReviewDate}; // DateType 3707 case -403934648: /*effectivePeriod*/ return this.effectivePeriod == null ? new Base[0] : new Base[] {this.effectivePeriod}; // Period 3708 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 3709 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 3710 case 110546223: /*topic*/ return this.topic == null ? new Base[0] : this.topic.toArray(new Base[this.topic.size()]); // CodeableConcept 3711 case -1895276325: /*contributor*/ return this.contributor == null ? new Base[0] : this.contributor.toArray(new Base[this.contributor.size()]); // Contributor 3712 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 3713 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 3714 case 666807069: /*relatedArtifact*/ return this.relatedArtifact == null ? new Base[0] : this.relatedArtifact.toArray(new Base[this.relatedArtifact.size()]); // RelatedArtifact 3715 case 166208699: /*library*/ return this.library == null ? new Base[0] : this.library.toArray(new Base[this.library.size()]); // Reference 3716 case 432371099: /*disclaimer*/ return this.disclaimer == null ? new Base[0] : new Base[] {this.disclaimer}; // MarkdownType 3717 case 1924005583: /*scoring*/ return this.scoring == null ? new Base[0] : new Base[] {this.scoring}; // CodeableConcept 3718 case 569347656: /*compositeScoring*/ return this.compositeScoring == null ? new Base[0] : new Base[] {this.compositeScoring}; // CodeableConcept 3719 case 3575610: /*type*/ return this.type == null ? new Base[0] : this.type.toArray(new Base[this.type.size()]); // CodeableConcept 3720 case 93273500: /*riskAdjustment*/ return this.riskAdjustment == null ? new Base[0] : new Base[] {this.riskAdjustment}; // StringType 3721 case 1254503906: /*rateAggregation*/ return this.rateAggregation == null ? new Base[0] : new Base[] {this.rateAggregation}; // StringType 3722 case 345689335: /*rationale*/ return this.rationale == null ? new Base[0] : new Base[] {this.rationale}; // MarkdownType 3723 case -18631389: /*clinicalRecommendationStatement*/ return this.clinicalRecommendationStatement == null ? new Base[0] : new Base[] {this.clinicalRecommendationStatement}; // MarkdownType 3724 case -2085456136: /*improvementNotation*/ return this.improvementNotation == null ? new Base[0] : new Base[] {this.improvementNotation}; // StringType 3725 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // MarkdownType 3726 case -1314002088: /*guidance*/ return this.guidance == null ? new Base[0] : new Base[] {this.guidance}; // MarkdownType 3727 case 113762: /*set*/ return this.set == null ? new Base[0] : new Base[] {this.set}; // StringType 3728 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // MeasureGroupComponent 3729 case 1447496814: /*supplementalData*/ return this.supplementalData == null ? new Base[0] : this.supplementalData.toArray(new Base[this.supplementalData.size()]); // MeasureSupplementalDataComponent 3730 default: return super.getProperty(hash, name, checkValid); 3731 } 3732 3733 } 3734 3735 @Override 3736 public Base setProperty(int hash, String name, Base value) throws FHIRException { 3737 switch (hash) { 3738 case 116079: // url 3739 this.url = castToUri(value); // UriType 3740 return value; 3741 case -1618432855: // identifier 3742 this.getIdentifier().add(castToIdentifier(value)); // Identifier 3743 return value; 3744 case 351608024: // version 3745 this.version = castToString(value); // StringType 3746 return value; 3747 case 3373707: // name 3748 this.name = castToString(value); // StringType 3749 return value; 3750 case 110371416: // title 3751 this.title = castToString(value); // StringType 3752 return value; 3753 case -892481550: // status 3754 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3755 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3756 return value; 3757 case -404562712: // experimental 3758 this.experimental = castToBoolean(value); // BooleanType 3759 return value; 3760 case 3076014: // date 3761 this.date = castToDateTime(value); // DateTimeType 3762 return value; 3763 case 1447404028: // publisher 3764 this.publisher = castToString(value); // StringType 3765 return value; 3766 case -1724546052: // description 3767 this.description = castToMarkdown(value); // MarkdownType 3768 return value; 3769 case -220463842: // purpose 3770 this.purpose = castToMarkdown(value); // MarkdownType 3771 return value; 3772 case 111574433: // usage 3773 this.usage = castToString(value); // StringType 3774 return value; 3775 case 223539345: // approvalDate 3776 this.approvalDate = castToDate(value); // DateType 3777 return value; 3778 case -1687512484: // lastReviewDate 3779 this.lastReviewDate = castToDate(value); // DateType 3780 return value; 3781 case -403934648: // effectivePeriod 3782 this.effectivePeriod = castToPeriod(value); // Period 3783 return value; 3784 case -669707736: // useContext 3785 this.getUseContext().add(castToUsageContext(value)); // UsageContext 3786 return value; 3787 case -507075711: // jurisdiction 3788 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 3789 return value; 3790 case 110546223: // topic 3791 this.getTopic().add(castToCodeableConcept(value)); // CodeableConcept 3792 return value; 3793 case -1895276325: // contributor 3794 this.getContributor().add(castToContributor(value)); // Contributor 3795 return value; 3796 case 951526432: // contact 3797 this.getContact().add(castToContactDetail(value)); // ContactDetail 3798 return value; 3799 case 1522889671: // copyright 3800 this.copyright = castToMarkdown(value); // MarkdownType 3801 return value; 3802 case 666807069: // relatedArtifact 3803 this.getRelatedArtifact().add(castToRelatedArtifact(value)); // RelatedArtifact 3804 return value; 3805 case 166208699: // library 3806 this.getLibrary().add(castToReference(value)); // Reference 3807 return value; 3808 case 432371099: // disclaimer 3809 this.disclaimer = castToMarkdown(value); // MarkdownType 3810 return value; 3811 case 1924005583: // scoring 3812 this.scoring = castToCodeableConcept(value); // CodeableConcept 3813 return value; 3814 case 569347656: // compositeScoring 3815 this.compositeScoring = castToCodeableConcept(value); // CodeableConcept 3816 return value; 3817 case 3575610: // type 3818 this.getType().add(castToCodeableConcept(value)); // CodeableConcept 3819 return value; 3820 case 93273500: // riskAdjustment 3821 this.riskAdjustment = castToString(value); // StringType 3822 return value; 3823 case 1254503906: // rateAggregation 3824 this.rateAggregation = castToString(value); // StringType 3825 return value; 3826 case 345689335: // rationale 3827 this.rationale = castToMarkdown(value); // MarkdownType 3828 return value; 3829 case -18631389: // clinicalRecommendationStatement 3830 this.clinicalRecommendationStatement = castToMarkdown(value); // MarkdownType 3831 return value; 3832 case -2085456136: // improvementNotation 3833 this.improvementNotation = castToString(value); // StringType 3834 return value; 3835 case -1014418093: // definition 3836 this.getDefinition().add(castToMarkdown(value)); // MarkdownType 3837 return value; 3838 case -1314002088: // guidance 3839 this.guidance = castToMarkdown(value); // MarkdownType 3840 return value; 3841 case 113762: // set 3842 this.set = castToString(value); // StringType 3843 return value; 3844 case 98629247: // group 3845 this.getGroup().add((MeasureGroupComponent) value); // MeasureGroupComponent 3846 return value; 3847 case 1447496814: // supplementalData 3848 this.getSupplementalData().add((MeasureSupplementalDataComponent) value); // MeasureSupplementalDataComponent 3849 return value; 3850 default: return super.setProperty(hash, name, value); 3851 } 3852 3853 } 3854 3855 @Override 3856 public Base setProperty(String name, Base value) throws FHIRException { 3857 if (name.equals("url")) { 3858 this.url = castToUri(value); // UriType 3859 } else if (name.equals("identifier")) { 3860 this.getIdentifier().add(castToIdentifier(value)); 3861 } else if (name.equals("version")) { 3862 this.version = castToString(value); // StringType 3863 } else if (name.equals("name")) { 3864 this.name = castToString(value); // StringType 3865 } else if (name.equals("title")) { 3866 this.title = castToString(value); // StringType 3867 } else if (name.equals("status")) { 3868 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 3869 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 3870 } else if (name.equals("experimental")) { 3871 this.experimental = castToBoolean(value); // BooleanType 3872 } else if (name.equals("date")) { 3873 this.date = castToDateTime(value); // DateTimeType 3874 } else if (name.equals("publisher")) { 3875 this.publisher = castToString(value); // StringType 3876 } else if (name.equals("description")) { 3877 this.description = castToMarkdown(value); // MarkdownType 3878 } else if (name.equals("purpose")) { 3879 this.purpose = castToMarkdown(value); // MarkdownType 3880 } else if (name.equals("usage")) { 3881 this.usage = castToString(value); // StringType 3882 } else if (name.equals("approvalDate")) { 3883 this.approvalDate = castToDate(value); // DateType 3884 } else if (name.equals("lastReviewDate")) { 3885 this.lastReviewDate = castToDate(value); // DateType 3886 } else if (name.equals("effectivePeriod")) { 3887 this.effectivePeriod = castToPeriod(value); // Period 3888 } else if (name.equals("useContext")) { 3889 this.getUseContext().add(castToUsageContext(value)); 3890 } else if (name.equals("jurisdiction")) { 3891 this.getJurisdiction().add(castToCodeableConcept(value)); 3892 } else if (name.equals("topic")) { 3893 this.getTopic().add(castToCodeableConcept(value)); 3894 } else if (name.equals("contributor")) { 3895 this.getContributor().add(castToContributor(value)); 3896 } else if (name.equals("contact")) { 3897 this.getContact().add(castToContactDetail(value)); 3898 } else if (name.equals("copyright")) { 3899 this.copyright = castToMarkdown(value); // MarkdownType 3900 } else if (name.equals("relatedArtifact")) { 3901 this.getRelatedArtifact().add(castToRelatedArtifact(value)); 3902 } else if (name.equals("library")) { 3903 this.getLibrary().add(castToReference(value)); 3904 } else if (name.equals("disclaimer")) { 3905 this.disclaimer = castToMarkdown(value); // MarkdownType 3906 } else if (name.equals("scoring")) { 3907 this.scoring = castToCodeableConcept(value); // CodeableConcept 3908 } else if (name.equals("compositeScoring")) { 3909 this.compositeScoring = castToCodeableConcept(value); // CodeableConcept 3910 } else if (name.equals("type")) { 3911 this.getType().add(castToCodeableConcept(value)); 3912 } else if (name.equals("riskAdjustment")) { 3913 this.riskAdjustment = castToString(value); // StringType 3914 } else if (name.equals("rateAggregation")) { 3915 this.rateAggregation = castToString(value); // StringType 3916 } else if (name.equals("rationale")) { 3917 this.rationale = castToMarkdown(value); // MarkdownType 3918 } else if (name.equals("clinicalRecommendationStatement")) { 3919 this.clinicalRecommendationStatement = castToMarkdown(value); // MarkdownType 3920 } else if (name.equals("improvementNotation")) { 3921 this.improvementNotation = castToString(value); // StringType 3922 } else if (name.equals("definition")) { 3923 this.getDefinition().add(castToMarkdown(value)); 3924 } else if (name.equals("guidance")) { 3925 this.guidance = castToMarkdown(value); // MarkdownType 3926 } else if (name.equals("set")) { 3927 this.set = castToString(value); // StringType 3928 } else if (name.equals("group")) { 3929 this.getGroup().add((MeasureGroupComponent) value); 3930 } else if (name.equals("supplementalData")) { 3931 this.getSupplementalData().add((MeasureSupplementalDataComponent) value); 3932 } else 3933 return super.setProperty(name, value); 3934 return value; 3935 } 3936 3937 @Override 3938 public Base makeProperty(int hash, String name) throws FHIRException { 3939 switch (hash) { 3940 case 116079: return getUrlElement(); 3941 case -1618432855: return addIdentifier(); 3942 case 351608024: return getVersionElement(); 3943 case 3373707: return getNameElement(); 3944 case 110371416: return getTitleElement(); 3945 case -892481550: return getStatusElement(); 3946 case -404562712: return getExperimentalElement(); 3947 case 3076014: return getDateElement(); 3948 case 1447404028: return getPublisherElement(); 3949 case -1724546052: return getDescriptionElement(); 3950 case -220463842: return getPurposeElement(); 3951 case 111574433: return getUsageElement(); 3952 case 223539345: return getApprovalDateElement(); 3953 case -1687512484: return getLastReviewDateElement(); 3954 case -403934648: return getEffectivePeriod(); 3955 case -669707736: return addUseContext(); 3956 case -507075711: return addJurisdiction(); 3957 case 110546223: return addTopic(); 3958 case -1895276325: return addContributor(); 3959 case 951526432: return addContact(); 3960 case 1522889671: return getCopyrightElement(); 3961 case 666807069: return addRelatedArtifact(); 3962 case 166208699: return addLibrary(); 3963 case 432371099: return getDisclaimerElement(); 3964 case 1924005583: return getScoring(); 3965 case 569347656: return getCompositeScoring(); 3966 case 3575610: return addType(); 3967 case 93273500: return getRiskAdjustmentElement(); 3968 case 1254503906: return getRateAggregationElement(); 3969 case 345689335: return getRationaleElement(); 3970 case -18631389: return getClinicalRecommendationStatementElement(); 3971 case -2085456136: return getImprovementNotationElement(); 3972 case -1014418093: return addDefinitionElement(); 3973 case -1314002088: return getGuidanceElement(); 3974 case 113762: return getSetElement(); 3975 case 98629247: return addGroup(); 3976 case 1447496814: return addSupplementalData(); 3977 default: return super.makeProperty(hash, name); 3978 } 3979 3980 } 3981 3982 @Override 3983 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3984 switch (hash) { 3985 case 116079: /*url*/ return new String[] {"uri"}; 3986 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3987 case 351608024: /*version*/ return new String[] {"string"}; 3988 case 3373707: /*name*/ return new String[] {"string"}; 3989 case 110371416: /*title*/ return new String[] {"string"}; 3990 case -892481550: /*status*/ return new String[] {"code"}; 3991 case -404562712: /*experimental*/ return new String[] {"boolean"}; 3992 case 3076014: /*date*/ return new String[] {"dateTime"}; 3993 case 1447404028: /*publisher*/ return new String[] {"string"}; 3994 case -1724546052: /*description*/ return new String[] {"markdown"}; 3995 case -220463842: /*purpose*/ return new String[] {"markdown"}; 3996 case 111574433: /*usage*/ return new String[] {"string"}; 3997 case 223539345: /*approvalDate*/ return new String[] {"date"}; 3998 case -1687512484: /*lastReviewDate*/ return new String[] {"date"}; 3999 case -403934648: /*effectivePeriod*/ return new String[] {"Period"}; 4000 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 4001 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 4002 case 110546223: /*topic*/ return new String[] {"CodeableConcept"}; 4003 case -1895276325: /*contributor*/ return new String[] {"Contributor"}; 4004 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 4005 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 4006 case 666807069: /*relatedArtifact*/ return new String[] {"RelatedArtifact"}; 4007 case 166208699: /*library*/ return new String[] {"Reference"}; 4008 case 432371099: /*disclaimer*/ return new String[] {"markdown"}; 4009 case 1924005583: /*scoring*/ return new String[] {"CodeableConcept"}; 4010 case 569347656: /*compositeScoring*/ return new String[] {"CodeableConcept"}; 4011 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 4012 case 93273500: /*riskAdjustment*/ return new String[] {"string"}; 4013 case 1254503906: /*rateAggregation*/ return new String[] {"string"}; 4014 case 345689335: /*rationale*/ return new String[] {"markdown"}; 4015 case -18631389: /*clinicalRecommendationStatement*/ return new String[] {"markdown"}; 4016 case -2085456136: /*improvementNotation*/ return new String[] {"string"}; 4017 case -1014418093: /*definition*/ return new String[] {"markdown"}; 4018 case -1314002088: /*guidance*/ return new String[] {"markdown"}; 4019 case 113762: /*set*/ return new String[] {"string"}; 4020 case 98629247: /*group*/ return new String[] {}; 4021 case 1447496814: /*supplementalData*/ return new String[] {}; 4022 default: return super.getTypesForProperty(hash, name); 4023 } 4024 4025 } 4026 4027 @Override 4028 public Base addChild(String name) throws FHIRException { 4029 if (name.equals("url")) { 4030 throw new FHIRException("Cannot call addChild on a singleton property Measure.url"); 4031 } 4032 else if (name.equals("identifier")) { 4033 return addIdentifier(); 4034 } 4035 else if (name.equals("version")) { 4036 throw new FHIRException("Cannot call addChild on a singleton property Measure.version"); 4037 } 4038 else if (name.equals("name")) { 4039 throw new FHIRException("Cannot call addChild on a singleton property Measure.name"); 4040 } 4041 else if (name.equals("title")) { 4042 throw new FHIRException("Cannot call addChild on a singleton property Measure.title"); 4043 } 4044 else if (name.equals("status")) { 4045 throw new FHIRException("Cannot call addChild on a singleton property Measure.status"); 4046 } 4047 else if (name.equals("experimental")) { 4048 throw new FHIRException("Cannot call addChild on a singleton property Measure.experimental"); 4049 } 4050 else if (name.equals("date")) { 4051 throw new FHIRException("Cannot call addChild on a singleton property Measure.date"); 4052 } 4053 else if (name.equals("publisher")) { 4054 throw new FHIRException("Cannot call addChild on a singleton property Measure.publisher"); 4055 } 4056 else if (name.equals("description")) { 4057 throw new FHIRException("Cannot call addChild on a singleton property Measure.description"); 4058 } 4059 else if (name.equals("purpose")) { 4060 throw new FHIRException("Cannot call addChild on a singleton property Measure.purpose"); 4061 } 4062 else if (name.equals("usage")) { 4063 throw new FHIRException("Cannot call addChild on a singleton property Measure.usage"); 4064 } 4065 else if (name.equals("approvalDate")) { 4066 throw new FHIRException("Cannot call addChild on a singleton property Measure.approvalDate"); 4067 } 4068 else if (name.equals("lastReviewDate")) { 4069 throw new FHIRException("Cannot call addChild on a singleton property Measure.lastReviewDate"); 4070 } 4071 else if (name.equals("effectivePeriod")) { 4072 this.effectivePeriod = new Period(); 4073 return this.effectivePeriod; 4074 } 4075 else if (name.equals("useContext")) { 4076 return addUseContext(); 4077 } 4078 else if (name.equals("jurisdiction")) { 4079 return addJurisdiction(); 4080 } 4081 else if (name.equals("topic")) { 4082 return addTopic(); 4083 } 4084 else if (name.equals("contributor")) { 4085 return addContributor(); 4086 } 4087 else if (name.equals("contact")) { 4088 return addContact(); 4089 } 4090 else if (name.equals("copyright")) { 4091 throw new FHIRException("Cannot call addChild on a singleton property Measure.copyright"); 4092 } 4093 else if (name.equals("relatedArtifact")) { 4094 return addRelatedArtifact(); 4095 } 4096 else if (name.equals("library")) { 4097 return addLibrary(); 4098 } 4099 else if (name.equals("disclaimer")) { 4100 throw new FHIRException("Cannot call addChild on a singleton property Measure.disclaimer"); 4101 } 4102 else if (name.equals("scoring")) { 4103 this.scoring = new CodeableConcept(); 4104 return this.scoring; 4105 } 4106 else if (name.equals("compositeScoring")) { 4107 this.compositeScoring = new CodeableConcept(); 4108 return this.compositeScoring; 4109 } 4110 else if (name.equals("type")) { 4111 return addType(); 4112 } 4113 else if (name.equals("riskAdjustment")) { 4114 throw new FHIRException("Cannot call addChild on a singleton property Measure.riskAdjustment"); 4115 } 4116 else if (name.equals("rateAggregation")) { 4117 throw new FHIRException("Cannot call addChild on a singleton property Measure.rateAggregation"); 4118 } 4119 else if (name.equals("rationale")) { 4120 throw new FHIRException("Cannot call addChild on a singleton property Measure.rationale"); 4121 } 4122 else if (name.equals("clinicalRecommendationStatement")) { 4123 throw new FHIRException("Cannot call addChild on a singleton property Measure.clinicalRecommendationStatement"); 4124 } 4125 else if (name.equals("improvementNotation")) { 4126 throw new FHIRException("Cannot call addChild on a singleton property Measure.improvementNotation"); 4127 } 4128 else if (name.equals("definition")) { 4129 throw new FHIRException("Cannot call addChild on a singleton property Measure.definition"); 4130 } 4131 else if (name.equals("guidance")) { 4132 throw new FHIRException("Cannot call addChild on a singleton property Measure.guidance"); 4133 } 4134 else if (name.equals("set")) { 4135 throw new FHIRException("Cannot call addChild on a singleton property Measure.set"); 4136 } 4137 else if (name.equals("group")) { 4138 return addGroup(); 4139 } 4140 else if (name.equals("supplementalData")) { 4141 return addSupplementalData(); 4142 } 4143 else 4144 return super.addChild(name); 4145 } 4146 4147 public String fhirType() { 4148 return "Measure"; 4149 4150 } 4151 4152 public Measure copy() { 4153 Measure dst = new Measure(); 4154 copyValues(dst); 4155 dst.url = url == null ? null : url.copy(); 4156 if (identifier != null) { 4157 dst.identifier = new ArrayList<Identifier>(); 4158 for (Identifier i : identifier) 4159 dst.identifier.add(i.copy()); 4160 }; 4161 dst.version = version == null ? null : version.copy(); 4162 dst.name = name == null ? null : name.copy(); 4163 dst.title = title == null ? null : title.copy(); 4164 dst.status = status == null ? null : status.copy(); 4165 dst.experimental = experimental == null ? null : experimental.copy(); 4166 dst.date = date == null ? null : date.copy(); 4167 dst.publisher = publisher == null ? null : publisher.copy(); 4168 dst.description = description == null ? null : description.copy(); 4169 dst.purpose = purpose == null ? null : purpose.copy(); 4170 dst.usage = usage == null ? null : usage.copy(); 4171 dst.approvalDate = approvalDate == null ? null : approvalDate.copy(); 4172 dst.lastReviewDate = lastReviewDate == null ? null : lastReviewDate.copy(); 4173 dst.effectivePeriod = effectivePeriod == null ? null : effectivePeriod.copy(); 4174 if (useContext != null) { 4175 dst.useContext = new ArrayList<UsageContext>(); 4176 for (UsageContext i : useContext) 4177 dst.useContext.add(i.copy()); 4178 }; 4179 if (jurisdiction != null) { 4180 dst.jurisdiction = new ArrayList<CodeableConcept>(); 4181 for (CodeableConcept i : jurisdiction) 4182 dst.jurisdiction.add(i.copy()); 4183 }; 4184 if (topic != null) { 4185 dst.topic = new ArrayList<CodeableConcept>(); 4186 for (CodeableConcept i : topic) 4187 dst.topic.add(i.copy()); 4188 }; 4189 if (contributor != null) { 4190 dst.contributor = new ArrayList<Contributor>(); 4191 for (Contributor i : contributor) 4192 dst.contributor.add(i.copy()); 4193 }; 4194 if (contact != null) { 4195 dst.contact = new ArrayList<ContactDetail>(); 4196 for (ContactDetail i : contact) 4197 dst.contact.add(i.copy()); 4198 }; 4199 dst.copyright = copyright == null ? null : copyright.copy(); 4200 if (relatedArtifact != null) { 4201 dst.relatedArtifact = new ArrayList<RelatedArtifact>(); 4202 for (RelatedArtifact i : relatedArtifact) 4203 dst.relatedArtifact.add(i.copy()); 4204 }; 4205 if (library != null) { 4206 dst.library = new ArrayList<Reference>(); 4207 for (Reference i : library) 4208 dst.library.add(i.copy()); 4209 }; 4210 dst.disclaimer = disclaimer == null ? null : disclaimer.copy(); 4211 dst.scoring = scoring == null ? null : scoring.copy(); 4212 dst.compositeScoring = compositeScoring == null ? null : compositeScoring.copy(); 4213 if (type != null) { 4214 dst.type = new ArrayList<CodeableConcept>(); 4215 for (CodeableConcept i : type) 4216 dst.type.add(i.copy()); 4217 }; 4218 dst.riskAdjustment = riskAdjustment == null ? null : riskAdjustment.copy(); 4219 dst.rateAggregation = rateAggregation == null ? null : rateAggregation.copy(); 4220 dst.rationale = rationale == null ? null : rationale.copy(); 4221 dst.clinicalRecommendationStatement = clinicalRecommendationStatement == null ? null : clinicalRecommendationStatement.copy(); 4222 dst.improvementNotation = improvementNotation == null ? null : improvementNotation.copy(); 4223 if (definition != null) { 4224 dst.definition = new ArrayList<MarkdownType>(); 4225 for (MarkdownType i : definition) 4226 dst.definition.add(i.copy()); 4227 }; 4228 dst.guidance = guidance == null ? null : guidance.copy(); 4229 dst.set = set == null ? null : set.copy(); 4230 if (group != null) { 4231 dst.group = new ArrayList<MeasureGroupComponent>(); 4232 for (MeasureGroupComponent i : group) 4233 dst.group.add(i.copy()); 4234 }; 4235 if (supplementalData != null) { 4236 dst.supplementalData = new ArrayList<MeasureSupplementalDataComponent>(); 4237 for (MeasureSupplementalDataComponent i : supplementalData) 4238 dst.supplementalData.add(i.copy()); 4239 }; 4240 return dst; 4241 } 4242 4243 protected Measure typedCopy() { 4244 return copy(); 4245 } 4246 4247 @Override 4248 public boolean equalsDeep(Base other_) { 4249 if (!super.equalsDeep(other_)) 4250 return false; 4251 if (!(other_ instanceof Measure)) 4252 return false; 4253 Measure o = (Measure) other_; 4254 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(usage, o.usage, true) 4255 && compareDeep(approvalDate, o.approvalDate, true) && compareDeep(lastReviewDate, o.lastReviewDate, true) 4256 && compareDeep(effectivePeriod, o.effectivePeriod, true) && compareDeep(topic, o.topic, true) && compareDeep(contributor, o.contributor, true) 4257 && compareDeep(copyright, o.copyright, true) && compareDeep(relatedArtifact, o.relatedArtifact, true) 4258 && compareDeep(library, o.library, true) && compareDeep(disclaimer, o.disclaimer, true) && compareDeep(scoring, o.scoring, true) 4259 && compareDeep(compositeScoring, o.compositeScoring, true) && compareDeep(type, o.type, true) && compareDeep(riskAdjustment, o.riskAdjustment, true) 4260 && compareDeep(rateAggregation, o.rateAggregation, true) && compareDeep(rationale, o.rationale, true) 4261 && compareDeep(clinicalRecommendationStatement, o.clinicalRecommendationStatement, true) && compareDeep(improvementNotation, o.improvementNotation, true) 4262 && compareDeep(definition, o.definition, true) && compareDeep(guidance, o.guidance, true) && compareDeep(set, o.set, true) 4263 && compareDeep(group, o.group, true) && compareDeep(supplementalData, o.supplementalData, true) 4264 ; 4265 } 4266 4267 @Override 4268 public boolean equalsShallow(Base other_) { 4269 if (!super.equalsShallow(other_)) 4270 return false; 4271 if (!(other_ instanceof Measure)) 4272 return false; 4273 Measure o = (Measure) other_; 4274 return compareValues(purpose, o.purpose, true) && compareValues(usage, o.usage, true) && compareValues(approvalDate, o.approvalDate, true) 4275 && compareValues(lastReviewDate, o.lastReviewDate, true) && compareValues(copyright, o.copyright, true) 4276 && compareValues(disclaimer, o.disclaimer, true) && compareValues(riskAdjustment, o.riskAdjustment, true) 4277 && compareValues(rateAggregation, o.rateAggregation, true) && compareValues(rationale, o.rationale, true) 4278 && compareValues(clinicalRecommendationStatement, o.clinicalRecommendationStatement, true) && compareValues(improvementNotation, o.improvementNotation, true) 4279 && compareValues(definition, o.definition, true) && compareValues(guidance, o.guidance, true) && compareValues(set, o.set, true) 4280 ; 4281 } 4282 4283 public boolean isEmpty() { 4284 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, usage 4285 , approvalDate, lastReviewDate, effectivePeriod, topic, contributor, copyright, relatedArtifact 4286 , library, disclaimer, scoring, compositeScoring, type, riskAdjustment, rateAggregation 4287 , rationale, clinicalRecommendationStatement, improvementNotation, definition, guidance 4288 , set, group, supplementalData); 4289 } 4290 4291 @Override 4292 public ResourceType getResourceType() { 4293 return ResourceType.Measure; 4294 } 4295 4296 /** 4297 * Search parameter: <b>date</b> 4298 * <p> 4299 * Description: <b>The measure publication date</b><br> 4300 * Type: <b>date</b><br> 4301 * Path: <b>Measure.date</b><br> 4302 * </p> 4303 */ 4304 @SearchParamDefinition(name="date", path="Measure.date", description="The measure publication date", type="date" ) 4305 public static final String SP_DATE = "date"; 4306 /** 4307 * <b>Fluent Client</b> search parameter constant for <b>date</b> 4308 * <p> 4309 * Description: <b>The measure publication date</b><br> 4310 * Type: <b>date</b><br> 4311 * Path: <b>Measure.date</b><br> 4312 * </p> 4313 */ 4314 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 4315 4316 /** 4317 * Search parameter: <b>identifier</b> 4318 * <p> 4319 * Description: <b>External identifier for the measure</b><br> 4320 * Type: <b>token</b><br> 4321 * Path: <b>Measure.identifier</b><br> 4322 * </p> 4323 */ 4324 @SearchParamDefinition(name="identifier", path="Measure.identifier", description="External identifier for the measure", type="token" ) 4325 public static final String SP_IDENTIFIER = "identifier"; 4326 /** 4327 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 4328 * <p> 4329 * Description: <b>External identifier for the measure</b><br> 4330 * Type: <b>token</b><br> 4331 * Path: <b>Measure.identifier</b><br> 4332 * </p> 4333 */ 4334 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 4335 4336 /** 4337 * Search parameter: <b>successor</b> 4338 * <p> 4339 * Description: <b>What resource is being referenced</b><br> 4340 * Type: <b>reference</b><br> 4341 * Path: <b>Measure.relatedArtifact.resource</b><br> 4342 * </p> 4343 */ 4344 @SearchParamDefinition(name="successor", path="Measure.relatedArtifact.where(type='successor').resource", description="What resource is being referenced", type="reference" ) 4345 public static final String SP_SUCCESSOR = "successor"; 4346 /** 4347 * <b>Fluent Client</b> search parameter constant for <b>successor</b> 4348 * <p> 4349 * Description: <b>What resource is being referenced</b><br> 4350 * Type: <b>reference</b><br> 4351 * Path: <b>Measure.relatedArtifact.resource</b><br> 4352 * </p> 4353 */ 4354 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUCCESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUCCESSOR); 4355 4356/** 4357 * Constant for fluent queries to be used to add include statements. Specifies 4358 * the path value of "<b>Measure:successor</b>". 4359 */ 4360 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUCCESSOR = new ca.uhn.fhir.model.api.Include("Measure:successor").toLocked(); 4361 4362 /** 4363 * Search parameter: <b>jurisdiction</b> 4364 * <p> 4365 * Description: <b>Intended jurisdiction for the measure</b><br> 4366 * Type: <b>token</b><br> 4367 * Path: <b>Measure.jurisdiction</b><br> 4368 * </p> 4369 */ 4370 @SearchParamDefinition(name="jurisdiction", path="Measure.jurisdiction", description="Intended jurisdiction for the measure", type="token" ) 4371 public static final String SP_JURISDICTION = "jurisdiction"; 4372 /** 4373 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 4374 * <p> 4375 * Description: <b>Intended jurisdiction for the measure</b><br> 4376 * Type: <b>token</b><br> 4377 * Path: <b>Measure.jurisdiction</b><br> 4378 * </p> 4379 */ 4380 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 4381 4382 /** 4383 * Search parameter: <b>description</b> 4384 * <p> 4385 * Description: <b>The description of the measure</b><br> 4386 * Type: <b>string</b><br> 4387 * Path: <b>Measure.description</b><br> 4388 * </p> 4389 */ 4390 @SearchParamDefinition(name="description", path="Measure.description", description="The description of the measure", type="string" ) 4391 public static final String SP_DESCRIPTION = "description"; 4392 /** 4393 * <b>Fluent Client</b> search parameter constant for <b>description</b> 4394 * <p> 4395 * Description: <b>The description of the measure</b><br> 4396 * Type: <b>string</b><br> 4397 * Path: <b>Measure.description</b><br> 4398 * </p> 4399 */ 4400 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 4401 4402 /** 4403 * Search parameter: <b>derived-from</b> 4404 * <p> 4405 * Description: <b>What resource is being referenced</b><br> 4406 * Type: <b>reference</b><br> 4407 * Path: <b>Measure.relatedArtifact.resource</b><br> 4408 * </p> 4409 */ 4410 @SearchParamDefinition(name="derived-from", path="Measure.relatedArtifact.where(type='derived-from').resource", description="What resource is being referenced", type="reference" ) 4411 public static final String SP_DERIVED_FROM = "derived-from"; 4412 /** 4413 * <b>Fluent Client</b> search parameter constant for <b>derived-from</b> 4414 * <p> 4415 * Description: <b>What resource is being referenced</b><br> 4416 * Type: <b>reference</b><br> 4417 * Path: <b>Measure.relatedArtifact.resource</b><br> 4418 * </p> 4419 */ 4420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DERIVED_FROM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DERIVED_FROM); 4421 4422/** 4423 * Constant for fluent queries to be used to add include statements. Specifies 4424 * the path value of "<b>Measure:derived-from</b>". 4425 */ 4426 public static final ca.uhn.fhir.model.api.Include INCLUDE_DERIVED_FROM = new ca.uhn.fhir.model.api.Include("Measure:derived-from").toLocked(); 4427 4428 /** 4429 * Search parameter: <b>predecessor</b> 4430 * <p> 4431 * Description: <b>What resource is being referenced</b><br> 4432 * Type: <b>reference</b><br> 4433 * Path: <b>Measure.relatedArtifact.resource</b><br> 4434 * </p> 4435 */ 4436 @SearchParamDefinition(name="predecessor", path="Measure.relatedArtifact.where(type='predecessor').resource", description="What resource is being referenced", type="reference" ) 4437 public static final String SP_PREDECESSOR = "predecessor"; 4438 /** 4439 * <b>Fluent Client</b> search parameter constant for <b>predecessor</b> 4440 * <p> 4441 * Description: <b>What resource is being referenced</b><br> 4442 * Type: <b>reference</b><br> 4443 * Path: <b>Measure.relatedArtifact.resource</b><br> 4444 * </p> 4445 */ 4446 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PREDECESSOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PREDECESSOR); 4447 4448/** 4449 * Constant for fluent queries to be used to add include statements. Specifies 4450 * the path value of "<b>Measure:predecessor</b>". 4451 */ 4452 public static final ca.uhn.fhir.model.api.Include INCLUDE_PREDECESSOR = new ca.uhn.fhir.model.api.Include("Measure:predecessor").toLocked(); 4453 4454 /** 4455 * Search parameter: <b>title</b> 4456 * <p> 4457 * Description: <b>The human-friendly name of the measure</b><br> 4458 * Type: <b>string</b><br> 4459 * Path: <b>Measure.title</b><br> 4460 * </p> 4461 */ 4462 @SearchParamDefinition(name="title", path="Measure.title", description="The human-friendly name of the measure", type="string" ) 4463 public static final String SP_TITLE = "title"; 4464 /** 4465 * <b>Fluent Client</b> search parameter constant for <b>title</b> 4466 * <p> 4467 * Description: <b>The human-friendly name of the measure</b><br> 4468 * Type: <b>string</b><br> 4469 * Path: <b>Measure.title</b><br> 4470 * </p> 4471 */ 4472 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 4473 4474 /** 4475 * Search parameter: <b>composed-of</b> 4476 * <p> 4477 * Description: <b>What resource is being referenced</b><br> 4478 * Type: <b>reference</b><br> 4479 * Path: <b>Measure.relatedArtifact.resource</b><br> 4480 * </p> 4481 */ 4482 @SearchParamDefinition(name="composed-of", path="Measure.relatedArtifact.where(type='composed-of').resource", description="What resource is being referenced", type="reference" ) 4483 public static final String SP_COMPOSED_OF = "composed-of"; 4484 /** 4485 * <b>Fluent Client</b> search parameter constant for <b>composed-of</b> 4486 * <p> 4487 * Description: <b>What resource is being referenced</b><br> 4488 * Type: <b>reference</b><br> 4489 * Path: <b>Measure.relatedArtifact.resource</b><br> 4490 * </p> 4491 */ 4492 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam COMPOSED_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_COMPOSED_OF); 4493 4494/** 4495 * Constant for fluent queries to be used to add include statements. Specifies 4496 * the path value of "<b>Measure:composed-of</b>". 4497 */ 4498 public static final ca.uhn.fhir.model.api.Include INCLUDE_COMPOSED_OF = new ca.uhn.fhir.model.api.Include("Measure:composed-of").toLocked(); 4499 4500 /** 4501 * Search parameter: <b>version</b> 4502 * <p> 4503 * Description: <b>The business version of the measure</b><br> 4504 * Type: <b>token</b><br> 4505 * Path: <b>Measure.version</b><br> 4506 * </p> 4507 */ 4508 @SearchParamDefinition(name="version", path="Measure.version", description="The business version of the measure", type="token" ) 4509 public static final String SP_VERSION = "version"; 4510 /** 4511 * <b>Fluent Client</b> search parameter constant for <b>version</b> 4512 * <p> 4513 * Description: <b>The business version of the measure</b><br> 4514 * Type: <b>token</b><br> 4515 * Path: <b>Measure.version</b><br> 4516 * </p> 4517 */ 4518 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 4519 4520 /** 4521 * Search parameter: <b>url</b> 4522 * <p> 4523 * Description: <b>The uri that identifies the measure</b><br> 4524 * Type: <b>uri</b><br> 4525 * Path: <b>Measure.url</b><br> 4526 * </p> 4527 */ 4528 @SearchParamDefinition(name="url", path="Measure.url", description="The uri that identifies the measure", type="uri" ) 4529 public static final String SP_URL = "url"; 4530 /** 4531 * <b>Fluent Client</b> search parameter constant for <b>url</b> 4532 * <p> 4533 * Description: <b>The uri that identifies the measure</b><br> 4534 * Type: <b>uri</b><br> 4535 * Path: <b>Measure.url</b><br> 4536 * </p> 4537 */ 4538 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 4539 4540 /** 4541 * Search parameter: <b>effective</b> 4542 * <p> 4543 * Description: <b>The time during which the measure is intended to be in use</b><br> 4544 * Type: <b>date</b><br> 4545 * Path: <b>Measure.effectivePeriod</b><br> 4546 * </p> 4547 */ 4548 @SearchParamDefinition(name="effective", path="Measure.effectivePeriod", description="The time during which the measure is intended to be in use", type="date" ) 4549 public static final String SP_EFFECTIVE = "effective"; 4550 /** 4551 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 4552 * <p> 4553 * Description: <b>The time during which the measure is intended to be in use</b><br> 4554 * Type: <b>date</b><br> 4555 * Path: <b>Measure.effectivePeriod</b><br> 4556 * </p> 4557 */ 4558 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 4559 4560 /** 4561 * Search parameter: <b>depends-on</b> 4562 * <p> 4563 * Description: <b>What resource is being referenced</b><br> 4564 * Type: <b>reference</b><br> 4565 * Path: <b>Measure.relatedArtifact.resource, Measure.library</b><br> 4566 * </p> 4567 */ 4568 @SearchParamDefinition(name="depends-on", path="Measure.relatedArtifact.where(type='depends-on').resource | Measure.library", description="What resource is being referenced", type="reference" ) 4569 public static final String SP_DEPENDS_ON = "depends-on"; 4570 /** 4571 * <b>Fluent Client</b> search parameter constant for <b>depends-on</b> 4572 * <p> 4573 * Description: <b>What resource is being referenced</b><br> 4574 * Type: <b>reference</b><br> 4575 * Path: <b>Measure.relatedArtifact.resource, Measure.library</b><br> 4576 * </p> 4577 */ 4578 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEPENDS_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEPENDS_ON); 4579 4580/** 4581 * Constant for fluent queries to be used to add include statements. Specifies 4582 * the path value of "<b>Measure:depends-on</b>". 4583 */ 4584 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEPENDS_ON = new ca.uhn.fhir.model.api.Include("Measure:depends-on").toLocked(); 4585 4586 /** 4587 * Search parameter: <b>name</b> 4588 * <p> 4589 * Description: <b>Computationally friendly name of the measure</b><br> 4590 * Type: <b>string</b><br> 4591 * Path: <b>Measure.name</b><br> 4592 * </p> 4593 */ 4594 @SearchParamDefinition(name="name", path="Measure.name", description="Computationally friendly name of the measure", type="string" ) 4595 public static final String SP_NAME = "name"; 4596 /** 4597 * <b>Fluent Client</b> search parameter constant for <b>name</b> 4598 * <p> 4599 * Description: <b>Computationally friendly name of the measure</b><br> 4600 * Type: <b>string</b><br> 4601 * Path: <b>Measure.name</b><br> 4602 * </p> 4603 */ 4604 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 4605 4606 /** 4607 * Search parameter: <b>publisher</b> 4608 * <p> 4609 * Description: <b>Name of the publisher of the measure</b><br> 4610 * Type: <b>string</b><br> 4611 * Path: <b>Measure.publisher</b><br> 4612 * </p> 4613 */ 4614 @SearchParamDefinition(name="publisher", path="Measure.publisher", description="Name of the publisher of the measure", type="string" ) 4615 public static final String SP_PUBLISHER = "publisher"; 4616 /** 4617 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 4618 * <p> 4619 * Description: <b>Name of the publisher of the measure</b><br> 4620 * Type: <b>string</b><br> 4621 * Path: <b>Measure.publisher</b><br> 4622 * </p> 4623 */ 4624 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 4625 4626 /** 4627 * Search parameter: <b>topic</b> 4628 * <p> 4629 * Description: <b>Topics associated with the module</b><br> 4630 * Type: <b>token</b><br> 4631 * Path: <b>Measure.topic</b><br> 4632 * </p> 4633 */ 4634 @SearchParamDefinition(name="topic", path="Measure.topic", description="Topics associated with the module", type="token" ) 4635 public static final String SP_TOPIC = "topic"; 4636 /** 4637 * <b>Fluent Client</b> search parameter constant for <b>topic</b> 4638 * <p> 4639 * Description: <b>Topics associated with the module</b><br> 4640 * Type: <b>token</b><br> 4641 * Path: <b>Measure.topic</b><br> 4642 * </p> 4643 */ 4644 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TOPIC = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TOPIC); 4645 4646 /** 4647 * Search parameter: <b>status</b> 4648 * <p> 4649 * Description: <b>The current status of the measure</b><br> 4650 * Type: <b>token</b><br> 4651 * Path: <b>Measure.status</b><br> 4652 * </p> 4653 */ 4654 @SearchParamDefinition(name="status", path="Measure.status", description="The current status of the measure", type="token" ) 4655 public static final String SP_STATUS = "status"; 4656 /** 4657 * <b>Fluent Client</b> search parameter constant for <b>status</b> 4658 * <p> 4659 * Description: <b>The current status of the measure</b><br> 4660 * Type: <b>token</b><br> 4661 * Path: <b>Measure.status</b><br> 4662 * </p> 4663 */ 4664 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 4665 4666 4667}