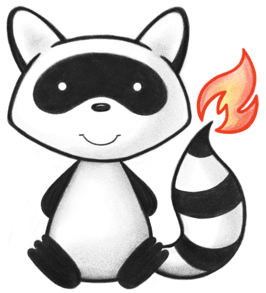
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006import java.math.BigDecimal; 007 008/* 009 Copyright (c) 2011+, HL7, Inc. 010 All rights reserved. 011 012 Redistribution and use in source and binary forms, with or without modification, 013 are permitted provided that the following conditions are met: 014 015 * Redistributions of source code must retain the above copyright notice, this 016 list of conditions and the following disclaimer. 017 * Redistributions in binary form must reproduce the above copyright notice, 018 this list of conditions and the following disclaimer in the documentation 019 and/or other materials provided with the distribution. 020 * Neither the name of HL7 nor the names of its contributors may be used to 021 endorse or promote products derived from this software without specific 022 prior written permission. 023 024 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 025 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 026 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 027 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 028 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 029 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 030 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 031 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 032 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 033 POSSIBILITY OF SUCH DAMAGE. 034 035*/ 036 037// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 038import java.util.ArrayList; 039import java.util.Date; 040import java.util.List; 041 042import org.hl7.fhir.exceptions.FHIRException; 043import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.Description; 048import ca.uhn.fhir.model.api.annotation.ResourceDef; 049import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 050/** 051 * The MeasureReport resource contains the results of evaluating a measure. 052 */ 053@ResourceDef(name="MeasureReport", profile="http://hl7.org/fhir/Profile/MeasureReport") 054public class MeasureReport extends DomainResource { 055 056 public enum MeasureReportStatus { 057 /** 058 * The report is complete and ready for use 059 */ 060 COMPLETE, 061 /** 062 * The report is currently being generated 063 */ 064 PENDING, 065 /** 066 * An error occurred attempting to generate the report 067 */ 068 ERROR, 069 /** 070 * added to help the parsers with the generic types 071 */ 072 NULL; 073 public static MeasureReportStatus fromCode(String codeString) throws FHIRException { 074 if (codeString == null || "".equals(codeString)) 075 return null; 076 if ("complete".equals(codeString)) 077 return COMPLETE; 078 if ("pending".equals(codeString)) 079 return PENDING; 080 if ("error".equals(codeString)) 081 return ERROR; 082 if (Configuration.isAcceptInvalidEnums()) 083 return null; 084 else 085 throw new FHIRException("Unknown MeasureReportStatus code '"+codeString+"'"); 086 } 087 public String toCode() { 088 switch (this) { 089 case COMPLETE: return "complete"; 090 case PENDING: return "pending"; 091 case ERROR: return "error"; 092 case NULL: return null; 093 default: return "?"; 094 } 095 } 096 public String getSystem() { 097 switch (this) { 098 case COMPLETE: return "http://hl7.org/fhir/measure-report-status"; 099 case PENDING: return "http://hl7.org/fhir/measure-report-status"; 100 case ERROR: return "http://hl7.org/fhir/measure-report-status"; 101 case NULL: return null; 102 default: return "?"; 103 } 104 } 105 public String getDefinition() { 106 switch (this) { 107 case COMPLETE: return "The report is complete and ready for use"; 108 case PENDING: return "The report is currently being generated"; 109 case ERROR: return "An error occurred attempting to generate the report"; 110 case NULL: return null; 111 default: return "?"; 112 } 113 } 114 public String getDisplay() { 115 switch (this) { 116 case COMPLETE: return "Complete"; 117 case PENDING: return "Pending"; 118 case ERROR: return "Error"; 119 case NULL: return null; 120 default: return "?"; 121 } 122 } 123 } 124 125 public static class MeasureReportStatusEnumFactory implements EnumFactory<MeasureReportStatus> { 126 public MeasureReportStatus fromCode(String codeString) throws IllegalArgumentException { 127 if (codeString == null || "".equals(codeString)) 128 if (codeString == null || "".equals(codeString)) 129 return null; 130 if ("complete".equals(codeString)) 131 return MeasureReportStatus.COMPLETE; 132 if ("pending".equals(codeString)) 133 return MeasureReportStatus.PENDING; 134 if ("error".equals(codeString)) 135 return MeasureReportStatus.ERROR; 136 throw new IllegalArgumentException("Unknown MeasureReportStatus code '"+codeString+"'"); 137 } 138 public Enumeration<MeasureReportStatus> fromType(PrimitiveType<?> code) throws FHIRException { 139 if (code == null) 140 return null; 141 if (code.isEmpty()) 142 return new Enumeration<MeasureReportStatus>(this); 143 String codeString = code.asStringValue(); 144 if (codeString == null || "".equals(codeString)) 145 return null; 146 if ("complete".equals(codeString)) 147 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.COMPLETE); 148 if ("pending".equals(codeString)) 149 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.PENDING); 150 if ("error".equals(codeString)) 151 return new Enumeration<MeasureReportStatus>(this, MeasureReportStatus.ERROR); 152 throw new FHIRException("Unknown MeasureReportStatus code '"+codeString+"'"); 153 } 154 public String toCode(MeasureReportStatus code) { 155 if (code == MeasureReportStatus.NULL) 156 return null; 157 if (code == MeasureReportStatus.COMPLETE) 158 return "complete"; 159 if (code == MeasureReportStatus.PENDING) 160 return "pending"; 161 if (code == MeasureReportStatus.ERROR) 162 return "error"; 163 return "?"; 164 } 165 public String toSystem(MeasureReportStatus code) { 166 return code.getSystem(); 167 } 168 } 169 170 public enum MeasureReportType { 171 /** 172 * An individual report that provides information on the performance for a given measure with respect to a single patient 173 */ 174 INDIVIDUAL, 175 /** 176 * A patient list report that includes a listing of patients that satisfied each population criteria in the measure 177 */ 178 PATIENTLIST, 179 /** 180 * A summary report that returns the number of patients in each population criteria for the measure 181 */ 182 SUMMARY, 183 /** 184 * added to help the parsers with the generic types 185 */ 186 NULL; 187 public static MeasureReportType fromCode(String codeString) throws FHIRException { 188 if (codeString == null || "".equals(codeString)) 189 return null; 190 if ("individual".equals(codeString)) 191 return INDIVIDUAL; 192 if ("patient-list".equals(codeString)) 193 return PATIENTLIST; 194 if ("summary".equals(codeString)) 195 return SUMMARY; 196 if (Configuration.isAcceptInvalidEnums()) 197 return null; 198 else 199 throw new FHIRException("Unknown MeasureReportType code '"+codeString+"'"); 200 } 201 public String toCode() { 202 switch (this) { 203 case INDIVIDUAL: return "individual"; 204 case PATIENTLIST: return "patient-list"; 205 case SUMMARY: return "summary"; 206 case NULL: return null; 207 default: return "?"; 208 } 209 } 210 public String getSystem() { 211 switch (this) { 212 case INDIVIDUAL: return "http://hl7.org/fhir/measure-report-type"; 213 case PATIENTLIST: return "http://hl7.org/fhir/measure-report-type"; 214 case SUMMARY: return "http://hl7.org/fhir/measure-report-type"; 215 case NULL: return null; 216 default: return "?"; 217 } 218 } 219 public String getDefinition() { 220 switch (this) { 221 case INDIVIDUAL: return "An individual report that provides information on the performance for a given measure with respect to a single patient"; 222 case PATIENTLIST: return "A patient list report that includes a listing of patients that satisfied each population criteria in the measure"; 223 case SUMMARY: return "A summary report that returns the number of patients in each population criteria for the measure"; 224 case NULL: return null; 225 default: return "?"; 226 } 227 } 228 public String getDisplay() { 229 switch (this) { 230 case INDIVIDUAL: return "Individual"; 231 case PATIENTLIST: return "Patient List"; 232 case SUMMARY: return "Summary"; 233 case NULL: return null; 234 default: return "?"; 235 } 236 } 237 } 238 239 public static class MeasureReportTypeEnumFactory implements EnumFactory<MeasureReportType> { 240 public MeasureReportType fromCode(String codeString) throws IllegalArgumentException { 241 if (codeString == null || "".equals(codeString)) 242 if (codeString == null || "".equals(codeString)) 243 return null; 244 if ("individual".equals(codeString)) 245 return MeasureReportType.INDIVIDUAL; 246 if ("patient-list".equals(codeString)) 247 return MeasureReportType.PATIENTLIST; 248 if ("summary".equals(codeString)) 249 return MeasureReportType.SUMMARY; 250 throw new IllegalArgumentException("Unknown MeasureReportType code '"+codeString+"'"); 251 } 252 public Enumeration<MeasureReportType> fromType(PrimitiveType<?> code) throws FHIRException { 253 if (code == null) 254 return null; 255 if (code.isEmpty()) 256 return new Enumeration<MeasureReportType>(this); 257 String codeString = code.asStringValue(); 258 if (codeString == null || "".equals(codeString)) 259 return null; 260 if ("individual".equals(codeString)) 261 return new Enumeration<MeasureReportType>(this, MeasureReportType.INDIVIDUAL); 262 if ("patient-list".equals(codeString)) 263 return new Enumeration<MeasureReportType>(this, MeasureReportType.PATIENTLIST); 264 if ("summary".equals(codeString)) 265 return new Enumeration<MeasureReportType>(this, MeasureReportType.SUMMARY); 266 throw new FHIRException("Unknown MeasureReportType code '"+codeString+"'"); 267 } 268 public String toCode(MeasureReportType code) { 269 if (code == MeasureReportType.NULL) 270 return null; 271 if (code == MeasureReportType.INDIVIDUAL) 272 return "individual"; 273 if (code == MeasureReportType.PATIENTLIST) 274 return "patient-list"; 275 if (code == MeasureReportType.SUMMARY) 276 return "summary"; 277 return "?"; 278 } 279 public String toSystem(MeasureReportType code) { 280 return code.getSystem(); 281 } 282 } 283 284 @Block() 285 public static class MeasureReportGroupComponent extends BackboneElement implements IBaseBackboneElement { 286 /** 287 * The identifier of the population group as defined in the measure definition. 288 */ 289 @Child(name = "identifier", type = {Identifier.class}, order=1, min=1, max=1, modifier=false, summary=false) 290 @Description(shortDefinition="What group of the measure", formalDefinition="The identifier of the population group as defined in the measure definition." ) 291 protected Identifier identifier; 292 293 /** 294 * The populations that make up the population group, one for each type of population appropriate for the measure. 295 */ 296 @Child(name = "population", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 297 @Description(shortDefinition="The populations in the group", formalDefinition="The populations that make up the population group, one for each type of population appropriate for the measure." ) 298 protected List<MeasureReportGroupPopulationComponent> population; 299 300 /** 301 * The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group. 302 */ 303 @Child(name = "measureScore", type = {DecimalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 304 @Description(shortDefinition="What score this group achieved", formalDefinition="The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group." ) 305 protected DecimalType measureScore; 306 307 /** 308 * When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure. 309 */ 310 @Child(name = "stratifier", type = {}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 311 @Description(shortDefinition="Stratification results", formalDefinition="When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure." ) 312 protected List<MeasureReportGroupStratifierComponent> stratifier; 313 314 private static final long serialVersionUID = 1520236061L; 315 316 /** 317 * Constructor 318 */ 319 public MeasureReportGroupComponent() { 320 super(); 321 } 322 323 /** 324 * Constructor 325 */ 326 public MeasureReportGroupComponent(Identifier identifier) { 327 super(); 328 this.identifier = identifier; 329 } 330 331 /** 332 * @return {@link #identifier} (The identifier of the population group as defined in the measure definition.) 333 */ 334 public Identifier getIdentifier() { 335 if (this.identifier == null) 336 if (Configuration.errorOnAutoCreate()) 337 throw new Error("Attempt to auto-create MeasureReportGroupComponent.identifier"); 338 else if (Configuration.doAutoCreate()) 339 this.identifier = new Identifier(); // cc 340 return this.identifier; 341 } 342 343 public boolean hasIdentifier() { 344 return this.identifier != null && !this.identifier.isEmpty(); 345 } 346 347 /** 348 * @param value {@link #identifier} (The identifier of the population group as defined in the measure definition.) 349 */ 350 public MeasureReportGroupComponent setIdentifier(Identifier value) { 351 this.identifier = value; 352 return this; 353 } 354 355 /** 356 * @return {@link #population} (The populations that make up the population group, one for each type of population appropriate for the measure.) 357 */ 358 public List<MeasureReportGroupPopulationComponent> getPopulation() { 359 if (this.population == null) 360 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 361 return this.population; 362 } 363 364 /** 365 * @return Returns a reference to <code>this</code> for easy method chaining 366 */ 367 public MeasureReportGroupComponent setPopulation(List<MeasureReportGroupPopulationComponent> thePopulation) { 368 this.population = thePopulation; 369 return this; 370 } 371 372 public boolean hasPopulation() { 373 if (this.population == null) 374 return false; 375 for (MeasureReportGroupPopulationComponent item : this.population) 376 if (!item.isEmpty()) 377 return true; 378 return false; 379 } 380 381 public MeasureReportGroupPopulationComponent addPopulation() { //3 382 MeasureReportGroupPopulationComponent t = new MeasureReportGroupPopulationComponent(); 383 if (this.population == null) 384 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 385 this.population.add(t); 386 return t; 387 } 388 389 public MeasureReportGroupComponent addPopulation(MeasureReportGroupPopulationComponent t) { //3 390 if (t == null) 391 return this; 392 if (this.population == null) 393 this.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 394 this.population.add(t); 395 return this; 396 } 397 398 /** 399 * @return The first repetition of repeating field {@link #population}, creating it if it does not already exist 400 */ 401 public MeasureReportGroupPopulationComponent getPopulationFirstRep() { 402 if (getPopulation().isEmpty()) { 403 addPopulation(); 404 } 405 return getPopulation().get(0); 406 } 407 408 /** 409 * @return {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.). This is the underlying object with id, value and extensions. The accessor "getMeasureScore" gives direct access to the value 410 */ 411 public DecimalType getMeasureScoreElement() { 412 if (this.measureScore == null) 413 if (Configuration.errorOnAutoCreate()) 414 throw new Error("Attempt to auto-create MeasureReportGroupComponent.measureScore"); 415 else if (Configuration.doAutoCreate()) 416 this.measureScore = new DecimalType(); // bb 417 return this.measureScore; 418 } 419 420 public boolean hasMeasureScoreElement() { 421 return this.measureScore != null && !this.measureScore.isEmpty(); 422 } 423 424 public boolean hasMeasureScore() { 425 return this.measureScore != null && !this.measureScore.isEmpty(); 426 } 427 428 /** 429 * @param value {@link #measureScore} (The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.). This is the underlying object with id, value and extensions. The accessor "getMeasureScore" gives direct access to the value 430 */ 431 public MeasureReportGroupComponent setMeasureScoreElement(DecimalType value) { 432 this.measureScore = value; 433 return this; 434 } 435 436 /** 437 * @return The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group. 438 */ 439 public BigDecimal getMeasureScore() { 440 return this.measureScore == null ? null : this.measureScore.getValue(); 441 } 442 443 /** 444 * @param value The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group. 445 */ 446 public MeasureReportGroupComponent setMeasureScore(BigDecimal value) { 447 if (value == null) 448 this.measureScore = null; 449 else { 450 if (this.measureScore == null) 451 this.measureScore = new DecimalType(); 452 this.measureScore.setValue(value); 453 } 454 return this; 455 } 456 457 /** 458 * @param value The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group. 459 */ 460 public MeasureReportGroupComponent setMeasureScore(long value) { 461 this.measureScore = new DecimalType(); 462 this.measureScore.setValue(value); 463 return this; 464 } 465 466 /** 467 * @param value The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group. 468 */ 469 public MeasureReportGroupComponent setMeasureScore(double value) { 470 this.measureScore = new DecimalType(); 471 this.measureScore.setValue(value); 472 return this; 473 } 474 475 /** 476 * @return {@link #stratifier} (When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.) 477 */ 478 public List<MeasureReportGroupStratifierComponent> getStratifier() { 479 if (this.stratifier == null) 480 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 481 return this.stratifier; 482 } 483 484 /** 485 * @return Returns a reference to <code>this</code> for easy method chaining 486 */ 487 public MeasureReportGroupComponent setStratifier(List<MeasureReportGroupStratifierComponent> theStratifier) { 488 this.stratifier = theStratifier; 489 return this; 490 } 491 492 public boolean hasStratifier() { 493 if (this.stratifier == null) 494 return false; 495 for (MeasureReportGroupStratifierComponent item : this.stratifier) 496 if (!item.isEmpty()) 497 return true; 498 return false; 499 } 500 501 public MeasureReportGroupStratifierComponent addStratifier() { //3 502 MeasureReportGroupStratifierComponent t = new MeasureReportGroupStratifierComponent(); 503 if (this.stratifier == null) 504 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 505 this.stratifier.add(t); 506 return t; 507 } 508 509 public MeasureReportGroupComponent addStratifier(MeasureReportGroupStratifierComponent t) { //3 510 if (t == null) 511 return this; 512 if (this.stratifier == null) 513 this.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 514 this.stratifier.add(t); 515 return this; 516 } 517 518 /** 519 * @return The first repetition of repeating field {@link #stratifier}, creating it if it does not already exist 520 */ 521 public MeasureReportGroupStratifierComponent getStratifierFirstRep() { 522 if (getStratifier().isEmpty()) { 523 addStratifier(); 524 } 525 return getStratifier().get(0); 526 } 527 528 protected void listChildren(List<Property> children) { 529 super.listChildren(children); 530 children.add(new Property("identifier", "Identifier", "The identifier of the population group as defined in the measure definition.", 0, 1, identifier)); 531 children.add(new Property("population", "", "The populations that make up the population group, one for each type of population appropriate for the measure.", 0, java.lang.Integer.MAX_VALUE, population)); 532 children.add(new Property("measureScore", "decimal", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore)); 533 children.add(new Property("stratifier", "", "When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.", 0, java.lang.Integer.MAX_VALUE, stratifier)); 534 } 535 536 @Override 537 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 538 switch (_hash) { 539 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier of the population group as defined in the measure definition.", 0, 1, identifier); 540 case -2023558323: /*population*/ return new Property("population", "", "The populations that make up the population group, one for each type of population appropriate for the measure.", 0, java.lang.Integer.MAX_VALUE, population); 541 case -386313260: /*measureScore*/ return new Property("measureScore", "decimal", "The measure score for this population group, calculated as appropriate for the measure type and scoring method, and based on the contents of the populations defined in the group.", 0, 1, measureScore); 542 case 90983669: /*stratifier*/ return new Property("stratifier", "", "When a measure includes multiple stratifiers, there will be a stratifier group for each stratifier defined by the measure.", 0, java.lang.Integer.MAX_VALUE, stratifier); 543 default: return super.getNamedProperty(_hash, _name, _checkValid); 544 } 545 546 } 547 548 @Override 549 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 550 switch (hash) { 551 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 552 case -2023558323: /*population*/ return this.population == null ? new Base[0] : this.population.toArray(new Base[this.population.size()]); // MeasureReportGroupPopulationComponent 553 case -386313260: /*measureScore*/ return this.measureScore == null ? new Base[0] : new Base[] {this.measureScore}; // DecimalType 554 case 90983669: /*stratifier*/ return this.stratifier == null ? new Base[0] : this.stratifier.toArray(new Base[this.stratifier.size()]); // MeasureReportGroupStratifierComponent 555 default: return super.getProperty(hash, name, checkValid); 556 } 557 558 } 559 560 @Override 561 public Base setProperty(int hash, String name, Base value) throws FHIRException { 562 switch (hash) { 563 case -1618432855: // identifier 564 this.identifier = castToIdentifier(value); // Identifier 565 return value; 566 case -2023558323: // population 567 this.getPopulation().add((MeasureReportGroupPopulationComponent) value); // MeasureReportGroupPopulationComponent 568 return value; 569 case -386313260: // measureScore 570 this.measureScore = castToDecimal(value); // DecimalType 571 return value; 572 case 90983669: // stratifier 573 this.getStratifier().add((MeasureReportGroupStratifierComponent) value); // MeasureReportGroupStratifierComponent 574 return value; 575 default: return super.setProperty(hash, name, value); 576 } 577 578 } 579 580 @Override 581 public Base setProperty(String name, Base value) throws FHIRException { 582 if (name.equals("identifier")) { 583 this.identifier = castToIdentifier(value); // Identifier 584 } else if (name.equals("population")) { 585 this.getPopulation().add((MeasureReportGroupPopulationComponent) value); 586 } else if (name.equals("measureScore")) { 587 this.measureScore = castToDecimal(value); // DecimalType 588 } else if (name.equals("stratifier")) { 589 this.getStratifier().add((MeasureReportGroupStratifierComponent) value); 590 } else 591 return super.setProperty(name, value); 592 return value; 593 } 594 595 @Override 596 public Base makeProperty(int hash, String name) throws FHIRException { 597 switch (hash) { 598 case -1618432855: return getIdentifier(); 599 case -2023558323: return addPopulation(); 600 case -386313260: return getMeasureScoreElement(); 601 case 90983669: return addStratifier(); 602 default: return super.makeProperty(hash, name); 603 } 604 605 } 606 607 @Override 608 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 609 switch (hash) { 610 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 611 case -2023558323: /*population*/ return new String[] {}; 612 case -386313260: /*measureScore*/ return new String[] {"decimal"}; 613 case 90983669: /*stratifier*/ return new String[] {}; 614 default: return super.getTypesForProperty(hash, name); 615 } 616 617 } 618 619 @Override 620 public Base addChild(String name) throws FHIRException { 621 if (name.equals("identifier")) { 622 this.identifier = new Identifier(); 623 return this.identifier; 624 } 625 else if (name.equals("population")) { 626 return addPopulation(); 627 } 628 else if (name.equals("measureScore")) { 629 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.measureScore"); 630 } 631 else if (name.equals("stratifier")) { 632 return addStratifier(); 633 } 634 else 635 return super.addChild(name); 636 } 637 638 public MeasureReportGroupComponent copy() { 639 MeasureReportGroupComponent dst = new MeasureReportGroupComponent(); 640 copyValues(dst); 641 dst.identifier = identifier == null ? null : identifier.copy(); 642 if (population != null) { 643 dst.population = new ArrayList<MeasureReportGroupPopulationComponent>(); 644 for (MeasureReportGroupPopulationComponent i : population) 645 dst.population.add(i.copy()); 646 }; 647 dst.measureScore = measureScore == null ? null : measureScore.copy(); 648 if (stratifier != null) { 649 dst.stratifier = new ArrayList<MeasureReportGroupStratifierComponent>(); 650 for (MeasureReportGroupStratifierComponent i : stratifier) 651 dst.stratifier.add(i.copy()); 652 }; 653 return dst; 654 } 655 656 @Override 657 public boolean equalsDeep(Base other_) { 658 if (!super.equalsDeep(other_)) 659 return false; 660 if (!(other_ instanceof MeasureReportGroupComponent)) 661 return false; 662 MeasureReportGroupComponent o = (MeasureReportGroupComponent) other_; 663 return compareDeep(identifier, o.identifier, true) && compareDeep(population, o.population, true) 664 && compareDeep(measureScore, o.measureScore, true) && compareDeep(stratifier, o.stratifier, true) 665 ; 666 } 667 668 @Override 669 public boolean equalsShallow(Base other_) { 670 if (!super.equalsShallow(other_)) 671 return false; 672 if (!(other_ instanceof MeasureReportGroupComponent)) 673 return false; 674 MeasureReportGroupComponent o = (MeasureReportGroupComponent) other_; 675 return compareValues(measureScore, o.measureScore, true); 676 } 677 678 public boolean isEmpty() { 679 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, population, measureScore 680 , stratifier); 681 } 682 683 public String fhirType() { 684 return "MeasureReport.group"; 685 686 } 687 688 } 689 690 @Block() 691 public static class MeasureReportGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 692 /** 693 * The identifier of the population being reported, as defined by the population element of the measure. 694 */ 695 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 696 @Description(shortDefinition="Population identifier as defined in the measure", formalDefinition="The identifier of the population being reported, as defined by the population element of the measure." ) 697 protected Identifier identifier; 698 699 /** 700 * The type of the population. 701 */ 702 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 703 @Description(shortDefinition="initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-score", formalDefinition="The type of the population." ) 704 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-population") 705 protected CodeableConcept code; 706 707 /** 708 * The number of members of the population. 709 */ 710 @Child(name = "count", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=false) 711 @Description(shortDefinition="Size of the population", formalDefinition="The number of members of the population." ) 712 protected IntegerType count; 713 714 /** 715 * This element refers to a List of patient level MeasureReport resources, one for each patient in this population. 716 */ 717 @Child(name = "patients", type = {ListResource.class}, order=4, min=0, max=1, modifier=false, summary=false) 718 @Description(shortDefinition="For patient-list reports, the patients in this population", formalDefinition="This element refers to a List of patient level MeasureReport resources, one for each patient in this population." ) 719 protected Reference patients; 720 721 /** 722 * The actual object that is the target of the reference (This element refers to a List of patient level MeasureReport resources, one for each patient in this population.) 723 */ 724 protected ListResource patientsTarget; 725 726 private static final long serialVersionUID = -1122075225L; 727 728 /** 729 * Constructor 730 */ 731 public MeasureReportGroupPopulationComponent() { 732 super(); 733 } 734 735 /** 736 * @return {@link #identifier} (The identifier of the population being reported, as defined by the population element of the measure.) 737 */ 738 public Identifier getIdentifier() { 739 if (this.identifier == null) 740 if (Configuration.errorOnAutoCreate()) 741 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.identifier"); 742 else if (Configuration.doAutoCreate()) 743 this.identifier = new Identifier(); // cc 744 return this.identifier; 745 } 746 747 public boolean hasIdentifier() { 748 return this.identifier != null && !this.identifier.isEmpty(); 749 } 750 751 /** 752 * @param value {@link #identifier} (The identifier of the population being reported, as defined by the population element of the measure.) 753 */ 754 public MeasureReportGroupPopulationComponent setIdentifier(Identifier value) { 755 this.identifier = value; 756 return this; 757 } 758 759 /** 760 * @return {@link #code} (The type of the population.) 761 */ 762 public CodeableConcept getCode() { 763 if (this.code == null) 764 if (Configuration.errorOnAutoCreate()) 765 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.code"); 766 else if (Configuration.doAutoCreate()) 767 this.code = new CodeableConcept(); // cc 768 return this.code; 769 } 770 771 public boolean hasCode() { 772 return this.code != null && !this.code.isEmpty(); 773 } 774 775 /** 776 * @param value {@link #code} (The type of the population.) 777 */ 778 public MeasureReportGroupPopulationComponent setCode(CodeableConcept value) { 779 this.code = value; 780 return this; 781 } 782 783 /** 784 * @return {@link #count} (The number of members of the population.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 785 */ 786 public IntegerType getCountElement() { 787 if (this.count == null) 788 if (Configuration.errorOnAutoCreate()) 789 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.count"); 790 else if (Configuration.doAutoCreate()) 791 this.count = new IntegerType(); // bb 792 return this.count; 793 } 794 795 public boolean hasCountElement() { 796 return this.count != null && !this.count.isEmpty(); 797 } 798 799 public boolean hasCount() { 800 return this.count != null && !this.count.isEmpty(); 801 } 802 803 /** 804 * @param value {@link #count} (The number of members of the population.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 805 */ 806 public MeasureReportGroupPopulationComponent setCountElement(IntegerType value) { 807 this.count = value; 808 return this; 809 } 810 811 /** 812 * @return The number of members of the population. 813 */ 814 public int getCount() { 815 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 816 } 817 818 /** 819 * @param value The number of members of the population. 820 */ 821 public MeasureReportGroupPopulationComponent setCount(int value) { 822 if (this.count == null) 823 this.count = new IntegerType(); 824 this.count.setValue(value); 825 return this; 826 } 827 828 /** 829 * @return {@link #patients} (This element refers to a List of patient level MeasureReport resources, one for each patient in this population.) 830 */ 831 public Reference getPatients() { 832 if (this.patients == null) 833 if (Configuration.errorOnAutoCreate()) 834 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.patients"); 835 else if (Configuration.doAutoCreate()) 836 this.patients = new Reference(); // cc 837 return this.patients; 838 } 839 840 public boolean hasPatients() { 841 return this.patients != null && !this.patients.isEmpty(); 842 } 843 844 /** 845 * @param value {@link #patients} (This element refers to a List of patient level MeasureReport resources, one for each patient in this population.) 846 */ 847 public MeasureReportGroupPopulationComponent setPatients(Reference value) { 848 this.patients = value; 849 return this; 850 } 851 852 /** 853 * @return {@link #patients} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (This element refers to a List of patient level MeasureReport resources, one for each patient in this population.) 854 */ 855 public ListResource getPatientsTarget() { 856 if (this.patientsTarget == null) 857 if (Configuration.errorOnAutoCreate()) 858 throw new Error("Attempt to auto-create MeasureReportGroupPopulationComponent.patients"); 859 else if (Configuration.doAutoCreate()) 860 this.patientsTarget = new ListResource(); // aa 861 return this.patientsTarget; 862 } 863 864 /** 865 * @param value {@link #patients} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (This element refers to a List of patient level MeasureReport resources, one for each patient in this population.) 866 */ 867 public MeasureReportGroupPopulationComponent setPatientsTarget(ListResource value) { 868 this.patientsTarget = value; 869 return this; 870 } 871 872 protected void listChildren(List<Property> children) { 873 super.listChildren(children); 874 children.add(new Property("identifier", "Identifier", "The identifier of the population being reported, as defined by the population element of the measure.", 0, 1, identifier)); 875 children.add(new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code)); 876 children.add(new Property("count", "integer", "The number of members of the population.", 0, 1, count)); 877 children.add(new Property("patients", "Reference(List)", "This element refers to a List of patient level MeasureReport resources, one for each patient in this population.", 0, 1, patients)); 878 } 879 880 @Override 881 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 882 switch (_hash) { 883 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier of the population being reported, as defined by the population element of the measure.", 0, 1, identifier); 884 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code); 885 case 94851343: /*count*/ return new Property("count", "integer", "The number of members of the population.", 0, 1, count); 886 case 1235842574: /*patients*/ return new Property("patients", "Reference(List)", "This element refers to a List of patient level MeasureReport resources, one for each patient in this population.", 0, 1, patients); 887 default: return super.getNamedProperty(_hash, _name, _checkValid); 888 } 889 890 } 891 892 @Override 893 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 894 switch (hash) { 895 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 896 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 897 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // IntegerType 898 case 1235842574: /*patients*/ return this.patients == null ? new Base[0] : new Base[] {this.patients}; // Reference 899 default: return super.getProperty(hash, name, checkValid); 900 } 901 902 } 903 904 @Override 905 public Base setProperty(int hash, String name, Base value) throws FHIRException { 906 switch (hash) { 907 case -1618432855: // identifier 908 this.identifier = castToIdentifier(value); // Identifier 909 return value; 910 case 3059181: // code 911 this.code = castToCodeableConcept(value); // CodeableConcept 912 return value; 913 case 94851343: // count 914 this.count = castToInteger(value); // IntegerType 915 return value; 916 case 1235842574: // patients 917 this.patients = castToReference(value); // Reference 918 return value; 919 default: return super.setProperty(hash, name, value); 920 } 921 922 } 923 924 @Override 925 public Base setProperty(String name, Base value) throws FHIRException { 926 if (name.equals("identifier")) { 927 this.identifier = castToIdentifier(value); // Identifier 928 } else if (name.equals("code")) { 929 this.code = castToCodeableConcept(value); // CodeableConcept 930 } else if (name.equals("count")) { 931 this.count = castToInteger(value); // IntegerType 932 } else if (name.equals("patients")) { 933 this.patients = castToReference(value); // Reference 934 } else 935 return super.setProperty(name, value); 936 return value; 937 } 938 939 @Override 940 public Base makeProperty(int hash, String name) throws FHIRException { 941 switch (hash) { 942 case -1618432855: return getIdentifier(); 943 case 3059181: return getCode(); 944 case 94851343: return getCountElement(); 945 case 1235842574: return getPatients(); 946 default: return super.makeProperty(hash, name); 947 } 948 949 } 950 951 @Override 952 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 953 switch (hash) { 954 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 955 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 956 case 94851343: /*count*/ return new String[] {"integer"}; 957 case 1235842574: /*patients*/ return new String[] {"Reference"}; 958 default: return super.getTypesForProperty(hash, name); 959 } 960 961 } 962 963 @Override 964 public Base addChild(String name) throws FHIRException { 965 if (name.equals("identifier")) { 966 this.identifier = new Identifier(); 967 return this.identifier; 968 } 969 else if (name.equals("code")) { 970 this.code = new CodeableConcept(); 971 return this.code; 972 } 973 else if (name.equals("count")) { 974 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.count"); 975 } 976 else if (name.equals("patients")) { 977 this.patients = new Reference(); 978 return this.patients; 979 } 980 else 981 return super.addChild(name); 982 } 983 984 public MeasureReportGroupPopulationComponent copy() { 985 MeasureReportGroupPopulationComponent dst = new MeasureReportGroupPopulationComponent(); 986 copyValues(dst); 987 dst.identifier = identifier == null ? null : identifier.copy(); 988 dst.code = code == null ? null : code.copy(); 989 dst.count = count == null ? null : count.copy(); 990 dst.patients = patients == null ? null : patients.copy(); 991 return dst; 992 } 993 994 @Override 995 public boolean equalsDeep(Base other_) { 996 if (!super.equalsDeep(other_)) 997 return false; 998 if (!(other_ instanceof MeasureReportGroupPopulationComponent)) 999 return false; 1000 MeasureReportGroupPopulationComponent o = (MeasureReportGroupPopulationComponent) other_; 1001 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(count, o.count, true) 1002 && compareDeep(patients, o.patients, true); 1003 } 1004 1005 @Override 1006 public boolean equalsShallow(Base other_) { 1007 if (!super.equalsShallow(other_)) 1008 return false; 1009 if (!(other_ instanceof MeasureReportGroupPopulationComponent)) 1010 return false; 1011 MeasureReportGroupPopulationComponent o = (MeasureReportGroupPopulationComponent) other_; 1012 return compareValues(count, o.count, true); 1013 } 1014 1015 public boolean isEmpty() { 1016 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, count 1017 , patients); 1018 } 1019 1020 public String fhirType() { 1021 return "MeasureReport.group.population"; 1022 1023 } 1024 1025 } 1026 1027 @Block() 1028 public static class MeasureReportGroupStratifierComponent extends BackboneElement implements IBaseBackboneElement { 1029 /** 1030 * The identifier of this stratifier, as defined in the measure definition. 1031 */ 1032 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=false) 1033 @Description(shortDefinition="What stratifier of the group", formalDefinition="The identifier of this stratifier, as defined in the measure definition." ) 1034 protected Identifier identifier; 1035 1036 /** 1037 * This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value. 1038 */ 1039 @Child(name = "stratum", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1040 @Description(shortDefinition="Stratum results, one for each unique value in the stratifier", formalDefinition="This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value." ) 1041 protected List<StratifierGroupComponent> stratum; 1042 1043 private static final long serialVersionUID = -1013521069L; 1044 1045 /** 1046 * Constructor 1047 */ 1048 public MeasureReportGroupStratifierComponent() { 1049 super(); 1050 } 1051 1052 /** 1053 * @return {@link #identifier} (The identifier of this stratifier, as defined in the measure definition.) 1054 */ 1055 public Identifier getIdentifier() { 1056 if (this.identifier == null) 1057 if (Configuration.errorOnAutoCreate()) 1058 throw new Error("Attempt to auto-create MeasureReportGroupStratifierComponent.identifier"); 1059 else if (Configuration.doAutoCreate()) 1060 this.identifier = new Identifier(); // cc 1061 return this.identifier; 1062 } 1063 1064 public boolean hasIdentifier() { 1065 return this.identifier != null && !this.identifier.isEmpty(); 1066 } 1067 1068 /** 1069 * @param value {@link #identifier} (The identifier of this stratifier, as defined in the measure definition.) 1070 */ 1071 public MeasureReportGroupStratifierComponent setIdentifier(Identifier value) { 1072 this.identifier = value; 1073 return this; 1074 } 1075 1076 /** 1077 * @return {@link #stratum} (This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.) 1078 */ 1079 public List<StratifierGroupComponent> getStratum() { 1080 if (this.stratum == null) 1081 this.stratum = new ArrayList<StratifierGroupComponent>(); 1082 return this.stratum; 1083 } 1084 1085 /** 1086 * @return Returns a reference to <code>this</code> for easy method chaining 1087 */ 1088 public MeasureReportGroupStratifierComponent setStratum(List<StratifierGroupComponent> theStratum) { 1089 this.stratum = theStratum; 1090 return this; 1091 } 1092 1093 public boolean hasStratum() { 1094 if (this.stratum == null) 1095 return false; 1096 for (StratifierGroupComponent item : this.stratum) 1097 if (!item.isEmpty()) 1098 return true; 1099 return false; 1100 } 1101 1102 public StratifierGroupComponent addStratum() { //3 1103 StratifierGroupComponent t = new StratifierGroupComponent(); 1104 if (this.stratum == null) 1105 this.stratum = new ArrayList<StratifierGroupComponent>(); 1106 this.stratum.add(t); 1107 return t; 1108 } 1109 1110 public MeasureReportGroupStratifierComponent addStratum(StratifierGroupComponent t) { //3 1111 if (t == null) 1112 return this; 1113 if (this.stratum == null) 1114 this.stratum = new ArrayList<StratifierGroupComponent>(); 1115 this.stratum.add(t); 1116 return this; 1117 } 1118 1119 /** 1120 * @return The first repetition of repeating field {@link #stratum}, creating it if it does not already exist 1121 */ 1122 public StratifierGroupComponent getStratumFirstRep() { 1123 if (getStratum().isEmpty()) { 1124 addStratum(); 1125 } 1126 return getStratum().get(0); 1127 } 1128 1129 protected void listChildren(List<Property> children) { 1130 super.listChildren(children); 1131 children.add(new Property("identifier", "Identifier", "The identifier of this stratifier, as defined in the measure definition.", 0, 1, identifier)); 1132 children.add(new Property("stratum", "", "This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.", 0, java.lang.Integer.MAX_VALUE, stratum)); 1133 } 1134 1135 @Override 1136 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1137 switch (_hash) { 1138 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier of this stratifier, as defined in the measure definition.", 0, 1, identifier); 1139 case -1881991236: /*stratum*/ return new Property("stratum", "", "This element contains the results for a single stratum within the stratifier. For example, when stratifying on administrative gender, there will be four strata, one for each possible gender value.", 0, java.lang.Integer.MAX_VALUE, stratum); 1140 default: return super.getNamedProperty(_hash, _name, _checkValid); 1141 } 1142 1143 } 1144 1145 @Override 1146 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1147 switch (hash) { 1148 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1149 case -1881991236: /*stratum*/ return this.stratum == null ? new Base[0] : this.stratum.toArray(new Base[this.stratum.size()]); // StratifierGroupComponent 1150 default: return super.getProperty(hash, name, checkValid); 1151 } 1152 1153 } 1154 1155 @Override 1156 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1157 switch (hash) { 1158 case -1618432855: // identifier 1159 this.identifier = castToIdentifier(value); // Identifier 1160 return value; 1161 case -1881991236: // stratum 1162 this.getStratum().add((StratifierGroupComponent) value); // StratifierGroupComponent 1163 return value; 1164 default: return super.setProperty(hash, name, value); 1165 } 1166 1167 } 1168 1169 @Override 1170 public Base setProperty(String name, Base value) throws FHIRException { 1171 if (name.equals("identifier")) { 1172 this.identifier = castToIdentifier(value); // Identifier 1173 } else if (name.equals("stratum")) { 1174 this.getStratum().add((StratifierGroupComponent) value); 1175 } else 1176 return super.setProperty(name, value); 1177 return value; 1178 } 1179 1180 @Override 1181 public Base makeProperty(int hash, String name) throws FHIRException { 1182 switch (hash) { 1183 case -1618432855: return getIdentifier(); 1184 case -1881991236: return addStratum(); 1185 default: return super.makeProperty(hash, name); 1186 } 1187 1188 } 1189 1190 @Override 1191 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1192 switch (hash) { 1193 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1194 case -1881991236: /*stratum*/ return new String[] {}; 1195 default: return super.getTypesForProperty(hash, name); 1196 } 1197 1198 } 1199 1200 @Override 1201 public Base addChild(String name) throws FHIRException { 1202 if (name.equals("identifier")) { 1203 this.identifier = new Identifier(); 1204 return this.identifier; 1205 } 1206 else if (name.equals("stratum")) { 1207 return addStratum(); 1208 } 1209 else 1210 return super.addChild(name); 1211 } 1212 1213 public MeasureReportGroupStratifierComponent copy() { 1214 MeasureReportGroupStratifierComponent dst = new MeasureReportGroupStratifierComponent(); 1215 copyValues(dst); 1216 dst.identifier = identifier == null ? null : identifier.copy(); 1217 if (stratum != null) { 1218 dst.stratum = new ArrayList<StratifierGroupComponent>(); 1219 for (StratifierGroupComponent i : stratum) 1220 dst.stratum.add(i.copy()); 1221 }; 1222 return dst; 1223 } 1224 1225 @Override 1226 public boolean equalsDeep(Base other_) { 1227 if (!super.equalsDeep(other_)) 1228 return false; 1229 if (!(other_ instanceof MeasureReportGroupStratifierComponent)) 1230 return false; 1231 MeasureReportGroupStratifierComponent o = (MeasureReportGroupStratifierComponent) other_; 1232 return compareDeep(identifier, o.identifier, true) && compareDeep(stratum, o.stratum, true); 1233 } 1234 1235 @Override 1236 public boolean equalsShallow(Base other_) { 1237 if (!super.equalsShallow(other_)) 1238 return false; 1239 if (!(other_ instanceof MeasureReportGroupStratifierComponent)) 1240 return false; 1241 MeasureReportGroupStratifierComponent o = (MeasureReportGroupStratifierComponent) other_; 1242 return true; 1243 } 1244 1245 public boolean isEmpty() { 1246 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, stratum); 1247 } 1248 1249 public String fhirType() { 1250 return "MeasureReport.group.stratifier"; 1251 1252 } 1253 1254 } 1255 1256 @Block() 1257 public static class StratifierGroupComponent extends BackboneElement implements IBaseBackboneElement { 1258 /** 1259 * The value for this stratum, expressed as a string. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique. 1260 */ 1261 @Child(name = "value", type = {StringType.class}, order=1, min=1, max=1, modifier=false, summary=false) 1262 @Description(shortDefinition="The stratum value, e.g. male", formalDefinition="The value for this stratum, expressed as a string. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique." ) 1263 protected StringType value; 1264 1265 /** 1266 * The populations that make up the stratum, one for each type of population appropriate to the measure. 1267 */ 1268 @Child(name = "population", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1269 @Description(shortDefinition="Population results in this stratum", formalDefinition="The populations that make up the stratum, one for each type of population appropriate to the measure." ) 1270 protected List<StratifierGroupPopulationComponent> population; 1271 1272 /** 1273 * The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum. 1274 */ 1275 @Child(name = "measureScore", type = {DecimalType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1276 @Description(shortDefinition="What score this stratum achieved", formalDefinition="The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum." ) 1277 protected DecimalType measureScore; 1278 1279 private static final long serialVersionUID = -772356228L; 1280 1281 /** 1282 * Constructor 1283 */ 1284 public StratifierGroupComponent() { 1285 super(); 1286 } 1287 1288 /** 1289 * Constructor 1290 */ 1291 public StratifierGroupComponent(StringType value) { 1292 super(); 1293 this.value = value; 1294 } 1295 1296 /** 1297 * @return {@link #value} (The value for this stratum, expressed as a string. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1298 */ 1299 public StringType getValueElement() { 1300 if (this.value == null) 1301 if (Configuration.errorOnAutoCreate()) 1302 throw new Error("Attempt to auto-create StratifierGroupComponent.value"); 1303 else if (Configuration.doAutoCreate()) 1304 this.value = new StringType(); // bb 1305 return this.value; 1306 } 1307 1308 public boolean hasValueElement() { 1309 return this.value != null && !this.value.isEmpty(); 1310 } 1311 1312 public boolean hasValue() { 1313 return this.value != null && !this.value.isEmpty(); 1314 } 1315 1316 /** 1317 * @param value {@link #value} (The value for this stratum, expressed as a string. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.). This is the underlying object with id, value and extensions. The accessor "getValue" gives direct access to the value 1318 */ 1319 public StratifierGroupComponent setValueElement(StringType value) { 1320 this.value = value; 1321 return this; 1322 } 1323 1324 /** 1325 * @return The value for this stratum, expressed as a string. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique. 1326 */ 1327 public String getValue() { 1328 return this.value == null ? null : this.value.getValue(); 1329 } 1330 1331 /** 1332 * @param value The value for this stratum, expressed as a string. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique. 1333 */ 1334 public StratifierGroupComponent setValue(String value) { 1335 if (this.value == null) 1336 this.value = new StringType(); 1337 this.value.setValue(value); 1338 return this; 1339 } 1340 1341 /** 1342 * @return {@link #population} (The populations that make up the stratum, one for each type of population appropriate to the measure.) 1343 */ 1344 public List<StratifierGroupPopulationComponent> getPopulation() { 1345 if (this.population == null) 1346 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 1347 return this.population; 1348 } 1349 1350 /** 1351 * @return Returns a reference to <code>this</code> for easy method chaining 1352 */ 1353 public StratifierGroupComponent setPopulation(List<StratifierGroupPopulationComponent> thePopulation) { 1354 this.population = thePopulation; 1355 return this; 1356 } 1357 1358 public boolean hasPopulation() { 1359 if (this.population == null) 1360 return false; 1361 for (StratifierGroupPopulationComponent item : this.population) 1362 if (!item.isEmpty()) 1363 return true; 1364 return false; 1365 } 1366 1367 public StratifierGroupPopulationComponent addPopulation() { //3 1368 StratifierGroupPopulationComponent t = new StratifierGroupPopulationComponent(); 1369 if (this.population == null) 1370 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 1371 this.population.add(t); 1372 return t; 1373 } 1374 1375 public StratifierGroupComponent addPopulation(StratifierGroupPopulationComponent t) { //3 1376 if (t == null) 1377 return this; 1378 if (this.population == null) 1379 this.population = new ArrayList<StratifierGroupPopulationComponent>(); 1380 this.population.add(t); 1381 return this; 1382 } 1383 1384 /** 1385 * @return The first repetition of repeating field {@link #population}, creating it if it does not already exist 1386 */ 1387 public StratifierGroupPopulationComponent getPopulationFirstRep() { 1388 if (getPopulation().isEmpty()) { 1389 addPopulation(); 1390 } 1391 return getPopulation().get(0); 1392 } 1393 1394 /** 1395 * @return {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.). This is the underlying object with id, value and extensions. The accessor "getMeasureScore" gives direct access to the value 1396 */ 1397 public DecimalType getMeasureScoreElement() { 1398 if (this.measureScore == null) 1399 if (Configuration.errorOnAutoCreate()) 1400 throw new Error("Attempt to auto-create StratifierGroupComponent.measureScore"); 1401 else if (Configuration.doAutoCreate()) 1402 this.measureScore = new DecimalType(); // bb 1403 return this.measureScore; 1404 } 1405 1406 public boolean hasMeasureScoreElement() { 1407 return this.measureScore != null && !this.measureScore.isEmpty(); 1408 } 1409 1410 public boolean hasMeasureScore() { 1411 return this.measureScore != null && !this.measureScore.isEmpty(); 1412 } 1413 1414 /** 1415 * @param value {@link #measureScore} (The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.). This is the underlying object with id, value and extensions. The accessor "getMeasureScore" gives direct access to the value 1416 */ 1417 public StratifierGroupComponent setMeasureScoreElement(DecimalType value) { 1418 this.measureScore = value; 1419 return this; 1420 } 1421 1422 /** 1423 * @return The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum. 1424 */ 1425 public BigDecimal getMeasureScore() { 1426 return this.measureScore == null ? null : this.measureScore.getValue(); 1427 } 1428 1429 /** 1430 * @param value The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum. 1431 */ 1432 public StratifierGroupComponent setMeasureScore(BigDecimal value) { 1433 if (value == null) 1434 this.measureScore = null; 1435 else { 1436 if (this.measureScore == null) 1437 this.measureScore = new DecimalType(); 1438 this.measureScore.setValue(value); 1439 } 1440 return this; 1441 } 1442 1443 /** 1444 * @param value The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum. 1445 */ 1446 public StratifierGroupComponent setMeasureScore(long value) { 1447 this.measureScore = new DecimalType(); 1448 this.measureScore.setValue(value); 1449 return this; 1450 } 1451 1452 /** 1453 * @param value The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum. 1454 */ 1455 public StratifierGroupComponent setMeasureScore(double value) { 1456 this.measureScore = new DecimalType(); 1457 this.measureScore.setValue(value); 1458 return this; 1459 } 1460 1461 protected void listChildren(List<Property> children) { 1462 super.listChildren(children); 1463 children.add(new Property("value", "string", "The value for this stratum, expressed as a string. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value)); 1464 children.add(new Property("population", "", "The populations that make up the stratum, one for each type of population appropriate to the measure.", 0, java.lang.Integer.MAX_VALUE, population)); 1465 children.add(new Property("measureScore", "decimal", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore)); 1466 } 1467 1468 @Override 1469 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1470 switch (_hash) { 1471 case 111972721: /*value*/ return new Property("value", "string", "The value for this stratum, expressed as a string. When defining stratifiers on complex values, the value must be rendered such that the value for each stratum within the stratifier is unique.", 0, 1, value); 1472 case -2023558323: /*population*/ return new Property("population", "", "The populations that make up the stratum, one for each type of population appropriate to the measure.", 0, java.lang.Integer.MAX_VALUE, population); 1473 case -386313260: /*measureScore*/ return new Property("measureScore", "decimal", "The measure score for this stratum, calculated as appropriate for the measure type and scoring method, and based on only the members of this stratum.", 0, 1, measureScore); 1474 default: return super.getNamedProperty(_hash, _name, _checkValid); 1475 } 1476 1477 } 1478 1479 @Override 1480 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1481 switch (hash) { 1482 case 111972721: /*value*/ return this.value == null ? new Base[0] : new Base[] {this.value}; // StringType 1483 case -2023558323: /*population*/ return this.population == null ? new Base[0] : this.population.toArray(new Base[this.population.size()]); // StratifierGroupPopulationComponent 1484 case -386313260: /*measureScore*/ return this.measureScore == null ? new Base[0] : new Base[] {this.measureScore}; // DecimalType 1485 default: return super.getProperty(hash, name, checkValid); 1486 } 1487 1488 } 1489 1490 @Override 1491 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1492 switch (hash) { 1493 case 111972721: // value 1494 this.value = castToString(value); // StringType 1495 return value; 1496 case -2023558323: // population 1497 this.getPopulation().add((StratifierGroupPopulationComponent) value); // StratifierGroupPopulationComponent 1498 return value; 1499 case -386313260: // measureScore 1500 this.measureScore = castToDecimal(value); // DecimalType 1501 return value; 1502 default: return super.setProperty(hash, name, value); 1503 } 1504 1505 } 1506 1507 @Override 1508 public Base setProperty(String name, Base value) throws FHIRException { 1509 if (name.equals("value")) { 1510 this.value = castToString(value); // StringType 1511 } else if (name.equals("population")) { 1512 this.getPopulation().add((StratifierGroupPopulationComponent) value); 1513 } else if (name.equals("measureScore")) { 1514 this.measureScore = castToDecimal(value); // DecimalType 1515 } else 1516 return super.setProperty(name, value); 1517 return value; 1518 } 1519 1520 @Override 1521 public Base makeProperty(int hash, String name) throws FHIRException { 1522 switch (hash) { 1523 case 111972721: return getValueElement(); 1524 case -2023558323: return addPopulation(); 1525 case -386313260: return getMeasureScoreElement(); 1526 default: return super.makeProperty(hash, name); 1527 } 1528 1529 } 1530 1531 @Override 1532 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1533 switch (hash) { 1534 case 111972721: /*value*/ return new String[] {"string"}; 1535 case -2023558323: /*population*/ return new String[] {}; 1536 case -386313260: /*measureScore*/ return new String[] {"decimal"}; 1537 default: return super.getTypesForProperty(hash, name); 1538 } 1539 1540 } 1541 1542 @Override 1543 public Base addChild(String name) throws FHIRException { 1544 if (name.equals("value")) { 1545 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.value"); 1546 } 1547 else if (name.equals("population")) { 1548 return addPopulation(); 1549 } 1550 else if (name.equals("measureScore")) { 1551 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.measureScore"); 1552 } 1553 else 1554 return super.addChild(name); 1555 } 1556 1557 public StratifierGroupComponent copy() { 1558 StratifierGroupComponent dst = new StratifierGroupComponent(); 1559 copyValues(dst); 1560 dst.value = value == null ? null : value.copy(); 1561 if (population != null) { 1562 dst.population = new ArrayList<StratifierGroupPopulationComponent>(); 1563 for (StratifierGroupPopulationComponent i : population) 1564 dst.population.add(i.copy()); 1565 }; 1566 dst.measureScore = measureScore == null ? null : measureScore.copy(); 1567 return dst; 1568 } 1569 1570 @Override 1571 public boolean equalsDeep(Base other_) { 1572 if (!super.equalsDeep(other_)) 1573 return false; 1574 if (!(other_ instanceof StratifierGroupComponent)) 1575 return false; 1576 StratifierGroupComponent o = (StratifierGroupComponent) other_; 1577 return compareDeep(value, o.value, true) && compareDeep(population, o.population, true) && compareDeep(measureScore, o.measureScore, true) 1578 ; 1579 } 1580 1581 @Override 1582 public boolean equalsShallow(Base other_) { 1583 if (!super.equalsShallow(other_)) 1584 return false; 1585 if (!(other_ instanceof StratifierGroupComponent)) 1586 return false; 1587 StratifierGroupComponent o = (StratifierGroupComponent) other_; 1588 return compareValues(value, o.value, true) && compareValues(measureScore, o.measureScore, true); 1589 } 1590 1591 public boolean isEmpty() { 1592 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(value, population, measureScore 1593 ); 1594 } 1595 1596 public String fhirType() { 1597 return "MeasureReport.group.stratifier.stratum"; 1598 1599 } 1600 1601 } 1602 1603 @Block() 1604 public static class StratifierGroupPopulationComponent extends BackboneElement implements IBaseBackboneElement { 1605 /** 1606 * The identifier of the population being reported, as defined by the population element of the measure. 1607 */ 1608 @Child(name = "identifier", type = {Identifier.class}, order=1, min=0, max=1, modifier=false, summary=true) 1609 @Description(shortDefinition="Population identifier as defined in the measure", formalDefinition="The identifier of the population being reported, as defined by the population element of the measure." ) 1610 protected Identifier identifier; 1611 1612 /** 1613 * The type of the population. 1614 */ 1615 @Child(name = "code", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=true) 1616 @Description(shortDefinition="initial-population | numerator | numerator-exclusion | denominator | denominator-exclusion | denominator-exception | measure-population | measure-population-exclusion | measure-score", formalDefinition="The type of the population." ) 1617 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-population") 1618 protected CodeableConcept code; 1619 1620 /** 1621 * The number of members of the population in this stratum. 1622 */ 1623 @Child(name = "count", type = {IntegerType.class}, order=3, min=0, max=1, modifier=false, summary=false) 1624 @Description(shortDefinition="Size of the population", formalDefinition="The number of members of the population in this stratum." ) 1625 protected IntegerType count; 1626 1627 /** 1628 * This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum. 1629 */ 1630 @Child(name = "patients", type = {ListResource.class}, order=4, min=0, max=1, modifier=false, summary=false) 1631 @Description(shortDefinition="For patient-list reports, the patients in this population", formalDefinition="This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum." ) 1632 protected Reference patients; 1633 1634 /** 1635 * The actual object that is the target of the reference (This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum.) 1636 */ 1637 protected ListResource patientsTarget; 1638 1639 private static final long serialVersionUID = -1122075225L; 1640 1641 /** 1642 * Constructor 1643 */ 1644 public StratifierGroupPopulationComponent() { 1645 super(); 1646 } 1647 1648 /** 1649 * @return {@link #identifier} (The identifier of the population being reported, as defined by the population element of the measure.) 1650 */ 1651 public Identifier getIdentifier() { 1652 if (this.identifier == null) 1653 if (Configuration.errorOnAutoCreate()) 1654 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.identifier"); 1655 else if (Configuration.doAutoCreate()) 1656 this.identifier = new Identifier(); // cc 1657 return this.identifier; 1658 } 1659 1660 public boolean hasIdentifier() { 1661 return this.identifier != null && !this.identifier.isEmpty(); 1662 } 1663 1664 /** 1665 * @param value {@link #identifier} (The identifier of the population being reported, as defined by the population element of the measure.) 1666 */ 1667 public StratifierGroupPopulationComponent setIdentifier(Identifier value) { 1668 this.identifier = value; 1669 return this; 1670 } 1671 1672 /** 1673 * @return {@link #code} (The type of the population.) 1674 */ 1675 public CodeableConcept getCode() { 1676 if (this.code == null) 1677 if (Configuration.errorOnAutoCreate()) 1678 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.code"); 1679 else if (Configuration.doAutoCreate()) 1680 this.code = new CodeableConcept(); // cc 1681 return this.code; 1682 } 1683 1684 public boolean hasCode() { 1685 return this.code != null && !this.code.isEmpty(); 1686 } 1687 1688 /** 1689 * @param value {@link #code} (The type of the population.) 1690 */ 1691 public StratifierGroupPopulationComponent setCode(CodeableConcept value) { 1692 this.code = value; 1693 return this; 1694 } 1695 1696 /** 1697 * @return {@link #count} (The number of members of the population in this stratum.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 1698 */ 1699 public IntegerType getCountElement() { 1700 if (this.count == null) 1701 if (Configuration.errorOnAutoCreate()) 1702 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.count"); 1703 else if (Configuration.doAutoCreate()) 1704 this.count = new IntegerType(); // bb 1705 return this.count; 1706 } 1707 1708 public boolean hasCountElement() { 1709 return this.count != null && !this.count.isEmpty(); 1710 } 1711 1712 public boolean hasCount() { 1713 return this.count != null && !this.count.isEmpty(); 1714 } 1715 1716 /** 1717 * @param value {@link #count} (The number of members of the population in this stratum.). This is the underlying object with id, value and extensions. The accessor "getCount" gives direct access to the value 1718 */ 1719 public StratifierGroupPopulationComponent setCountElement(IntegerType value) { 1720 this.count = value; 1721 return this; 1722 } 1723 1724 /** 1725 * @return The number of members of the population in this stratum. 1726 */ 1727 public int getCount() { 1728 return this.count == null || this.count.isEmpty() ? 0 : this.count.getValue(); 1729 } 1730 1731 /** 1732 * @param value The number of members of the population in this stratum. 1733 */ 1734 public StratifierGroupPopulationComponent setCount(int value) { 1735 if (this.count == null) 1736 this.count = new IntegerType(); 1737 this.count.setValue(value); 1738 return this; 1739 } 1740 1741 /** 1742 * @return {@link #patients} (This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum.) 1743 */ 1744 public Reference getPatients() { 1745 if (this.patients == null) 1746 if (Configuration.errorOnAutoCreate()) 1747 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.patients"); 1748 else if (Configuration.doAutoCreate()) 1749 this.patients = new Reference(); // cc 1750 return this.patients; 1751 } 1752 1753 public boolean hasPatients() { 1754 return this.patients != null && !this.patients.isEmpty(); 1755 } 1756 1757 /** 1758 * @param value {@link #patients} (This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum.) 1759 */ 1760 public StratifierGroupPopulationComponent setPatients(Reference value) { 1761 this.patients = value; 1762 return this; 1763 } 1764 1765 /** 1766 * @return {@link #patients} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum.) 1767 */ 1768 public ListResource getPatientsTarget() { 1769 if (this.patientsTarget == null) 1770 if (Configuration.errorOnAutoCreate()) 1771 throw new Error("Attempt to auto-create StratifierGroupPopulationComponent.patients"); 1772 else if (Configuration.doAutoCreate()) 1773 this.patientsTarget = new ListResource(); // aa 1774 return this.patientsTarget; 1775 } 1776 1777 /** 1778 * @param value {@link #patients} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum.) 1779 */ 1780 public StratifierGroupPopulationComponent setPatientsTarget(ListResource value) { 1781 this.patientsTarget = value; 1782 return this; 1783 } 1784 1785 protected void listChildren(List<Property> children) { 1786 super.listChildren(children); 1787 children.add(new Property("identifier", "Identifier", "The identifier of the population being reported, as defined by the population element of the measure.", 0, 1, identifier)); 1788 children.add(new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code)); 1789 children.add(new Property("count", "integer", "The number of members of the population in this stratum.", 0, 1, count)); 1790 children.add(new Property("patients", "Reference(List)", "This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum.", 0, 1, patients)); 1791 } 1792 1793 @Override 1794 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1795 switch (_hash) { 1796 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "The identifier of the population being reported, as defined by the population element of the measure.", 0, 1, identifier); 1797 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "The type of the population.", 0, 1, code); 1798 case 94851343: /*count*/ return new Property("count", "integer", "The number of members of the population in this stratum.", 0, 1, count); 1799 case 1235842574: /*patients*/ return new Property("patients", "Reference(List)", "This element refers to a List of patient level MeasureReport resources, one for each patient in this population in this stratum.", 0, 1, patients); 1800 default: return super.getNamedProperty(_hash, _name, _checkValid); 1801 } 1802 1803 } 1804 1805 @Override 1806 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1807 switch (hash) { 1808 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 1809 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1810 case 94851343: /*count*/ return this.count == null ? new Base[0] : new Base[] {this.count}; // IntegerType 1811 case 1235842574: /*patients*/ return this.patients == null ? new Base[0] : new Base[] {this.patients}; // Reference 1812 default: return super.getProperty(hash, name, checkValid); 1813 } 1814 1815 } 1816 1817 @Override 1818 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1819 switch (hash) { 1820 case -1618432855: // identifier 1821 this.identifier = castToIdentifier(value); // Identifier 1822 return value; 1823 case 3059181: // code 1824 this.code = castToCodeableConcept(value); // CodeableConcept 1825 return value; 1826 case 94851343: // count 1827 this.count = castToInteger(value); // IntegerType 1828 return value; 1829 case 1235842574: // patients 1830 this.patients = castToReference(value); // Reference 1831 return value; 1832 default: return super.setProperty(hash, name, value); 1833 } 1834 1835 } 1836 1837 @Override 1838 public Base setProperty(String name, Base value) throws FHIRException { 1839 if (name.equals("identifier")) { 1840 this.identifier = castToIdentifier(value); // Identifier 1841 } else if (name.equals("code")) { 1842 this.code = castToCodeableConcept(value); // CodeableConcept 1843 } else if (name.equals("count")) { 1844 this.count = castToInteger(value); // IntegerType 1845 } else if (name.equals("patients")) { 1846 this.patients = castToReference(value); // Reference 1847 } else 1848 return super.setProperty(name, value); 1849 return value; 1850 } 1851 1852 @Override 1853 public Base makeProperty(int hash, String name) throws FHIRException { 1854 switch (hash) { 1855 case -1618432855: return getIdentifier(); 1856 case 3059181: return getCode(); 1857 case 94851343: return getCountElement(); 1858 case 1235842574: return getPatients(); 1859 default: return super.makeProperty(hash, name); 1860 } 1861 1862 } 1863 1864 @Override 1865 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1866 switch (hash) { 1867 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1868 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1869 case 94851343: /*count*/ return new String[] {"integer"}; 1870 case 1235842574: /*patients*/ return new String[] {"Reference"}; 1871 default: return super.getTypesForProperty(hash, name); 1872 } 1873 1874 } 1875 1876 @Override 1877 public Base addChild(String name) throws FHIRException { 1878 if (name.equals("identifier")) { 1879 this.identifier = new Identifier(); 1880 return this.identifier; 1881 } 1882 else if (name.equals("code")) { 1883 this.code = new CodeableConcept(); 1884 return this.code; 1885 } 1886 else if (name.equals("count")) { 1887 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.count"); 1888 } 1889 else if (name.equals("patients")) { 1890 this.patients = new Reference(); 1891 return this.patients; 1892 } 1893 else 1894 return super.addChild(name); 1895 } 1896 1897 public StratifierGroupPopulationComponent copy() { 1898 StratifierGroupPopulationComponent dst = new StratifierGroupPopulationComponent(); 1899 copyValues(dst); 1900 dst.identifier = identifier == null ? null : identifier.copy(); 1901 dst.code = code == null ? null : code.copy(); 1902 dst.count = count == null ? null : count.copy(); 1903 dst.patients = patients == null ? null : patients.copy(); 1904 return dst; 1905 } 1906 1907 @Override 1908 public boolean equalsDeep(Base other_) { 1909 if (!super.equalsDeep(other_)) 1910 return false; 1911 if (!(other_ instanceof StratifierGroupPopulationComponent)) 1912 return false; 1913 StratifierGroupPopulationComponent o = (StratifierGroupPopulationComponent) other_; 1914 return compareDeep(identifier, o.identifier, true) && compareDeep(code, o.code, true) && compareDeep(count, o.count, true) 1915 && compareDeep(patients, o.patients, true); 1916 } 1917 1918 @Override 1919 public boolean equalsShallow(Base other_) { 1920 if (!super.equalsShallow(other_)) 1921 return false; 1922 if (!(other_ instanceof StratifierGroupPopulationComponent)) 1923 return false; 1924 StratifierGroupPopulationComponent o = (StratifierGroupPopulationComponent) other_; 1925 return compareValues(count, o.count, true); 1926 } 1927 1928 public boolean isEmpty() { 1929 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, code, count 1930 , patients); 1931 } 1932 1933 public String fhirType() { 1934 return "MeasureReport.group.stratifier.stratum.population"; 1935 1936 } 1937 1938 } 1939 1940 /** 1941 * A formal identifier that is used to identify this report when it is represented in other formats, or referenced in a specification, model, design or an instance. 1942 */ 1943 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 1944 @Description(shortDefinition="Additional identifier for the Report", formalDefinition="A formal identifier that is used to identify this report when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 1945 protected Identifier identifier; 1946 1947 /** 1948 * The report status. No data will be available until the report status is complete. 1949 */ 1950 @Child(name = "status", type = {CodeType.class}, order=1, min=1, max=1, modifier=true, summary=true) 1951 @Description(shortDefinition="complete | pending | error", formalDefinition="The report status. No data will be available until the report status is complete." ) 1952 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-report-status") 1953 protected Enumeration<MeasureReportStatus> status; 1954 1955 /** 1956 * The type of measure report. This may be an individual report, which provides a single patient's score for the measure; a patient listing, which returns the list of patients that meet the various criteria in the measure; or a summary report, which returns a population count for each of the criteria in the measure. 1957 */ 1958 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 1959 @Description(shortDefinition="individual | patient-list | summary", formalDefinition="The type of measure report. This may be an individual report, which provides a single patient's score for the measure; a patient listing, which returns the list of patients that meet the various criteria in the measure; or a summary report, which returns a population count for each of the criteria in the measure." ) 1960 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/measure-report-type") 1961 protected Enumeration<MeasureReportType> type; 1962 1963 /** 1964 * A reference to the Measure that was evaluated to produce this report. 1965 */ 1966 @Child(name = "measure", type = {Measure.class}, order=3, min=1, max=1, modifier=false, summary=true) 1967 @Description(shortDefinition="What measure was evaluated", formalDefinition="A reference to the Measure that was evaluated to produce this report." ) 1968 protected Reference measure; 1969 1970 /** 1971 * The actual object that is the target of the reference (A reference to the Measure that was evaluated to produce this report.) 1972 */ 1973 protected Measure measureTarget; 1974 1975 /** 1976 * Optional Patient if the report was requested for a single patient. 1977 */ 1978 @Child(name = "patient", type = {Patient.class}, order=4, min=0, max=1, modifier=false, summary=true) 1979 @Description(shortDefinition="What patient the report is for", formalDefinition="Optional Patient if the report was requested for a single patient." ) 1980 protected Reference patient; 1981 1982 /** 1983 * The actual object that is the target of the reference (Optional Patient if the report was requested for a single patient.) 1984 */ 1985 protected Patient patientTarget; 1986 1987 /** 1988 * The date this measure report was generated. 1989 */ 1990 @Child(name = "date", type = {DateTimeType.class}, order=5, min=0, max=1, modifier=false, summary=true) 1991 @Description(shortDefinition="When the report was generated", formalDefinition="The date this measure report was generated." ) 1992 protected DateTimeType date; 1993 1994 /** 1995 * Reporting Organization. 1996 */ 1997 @Child(name = "reportingOrganization", type = {Organization.class}, order=6, min=0, max=1, modifier=false, summary=true) 1998 @Description(shortDefinition="Who is reporting the data", formalDefinition="Reporting Organization." ) 1999 protected Reference reportingOrganization; 2000 2001 /** 2002 * The actual object that is the target of the reference (Reporting Organization.) 2003 */ 2004 protected Organization reportingOrganizationTarget; 2005 2006 /** 2007 * The reporting period for which the report was calculated. 2008 */ 2009 @Child(name = "period", type = {Period.class}, order=7, min=1, max=1, modifier=false, summary=true) 2010 @Description(shortDefinition="What period the report covers", formalDefinition="The reporting period for which the report was calculated." ) 2011 protected Period period; 2012 2013 /** 2014 * The results of the calculation, one for each population group in the measure. 2015 */ 2016 @Child(name = "group", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 2017 @Description(shortDefinition="Measure results for each group", formalDefinition="The results of the calculation, one for each population group in the measure." ) 2018 protected List<MeasureReportGroupComponent> group; 2019 2020 /** 2021 * A reference to a Bundle containing the Resources that were used in the evaluation of this report. 2022 */ 2023 @Child(name = "evaluatedResources", type = {Bundle.class}, order=9, min=0, max=1, modifier=false, summary=false) 2024 @Description(shortDefinition="What data was evaluated to produce the measure score", formalDefinition="A reference to a Bundle containing the Resources that were used in the evaluation of this report." ) 2025 protected Reference evaluatedResources; 2026 2027 /** 2028 * The actual object that is the target of the reference (A reference to a Bundle containing the Resources that were used in the evaluation of this report.) 2029 */ 2030 protected Bundle evaluatedResourcesTarget; 2031 2032 private static final long serialVersionUID = -1591529268L; 2033 2034 /** 2035 * Constructor 2036 */ 2037 public MeasureReport() { 2038 super(); 2039 } 2040 2041 /** 2042 * Constructor 2043 */ 2044 public MeasureReport(Enumeration<MeasureReportStatus> status, Enumeration<MeasureReportType> type, Reference measure, Period period) { 2045 super(); 2046 this.status = status; 2047 this.type = type; 2048 this.measure = measure; 2049 this.period = period; 2050 } 2051 2052 /** 2053 * @return {@link #identifier} (A formal identifier that is used to identify this report when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2054 */ 2055 public Identifier getIdentifier() { 2056 if (this.identifier == null) 2057 if (Configuration.errorOnAutoCreate()) 2058 throw new Error("Attempt to auto-create MeasureReport.identifier"); 2059 else if (Configuration.doAutoCreate()) 2060 this.identifier = new Identifier(); // cc 2061 return this.identifier; 2062 } 2063 2064 public boolean hasIdentifier() { 2065 return this.identifier != null && !this.identifier.isEmpty(); 2066 } 2067 2068 /** 2069 * @param value {@link #identifier} (A formal identifier that is used to identify this report when it is represented in other formats, or referenced in a specification, model, design or an instance.) 2070 */ 2071 public MeasureReport setIdentifier(Identifier value) { 2072 this.identifier = value; 2073 return this; 2074 } 2075 2076 /** 2077 * @return {@link #status} (The report status. No data will be available until the report status is complete.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2078 */ 2079 public Enumeration<MeasureReportStatus> getStatusElement() { 2080 if (this.status == null) 2081 if (Configuration.errorOnAutoCreate()) 2082 throw new Error("Attempt to auto-create MeasureReport.status"); 2083 else if (Configuration.doAutoCreate()) 2084 this.status = new Enumeration<MeasureReportStatus>(new MeasureReportStatusEnumFactory()); // bb 2085 return this.status; 2086 } 2087 2088 public boolean hasStatusElement() { 2089 return this.status != null && !this.status.isEmpty(); 2090 } 2091 2092 public boolean hasStatus() { 2093 return this.status != null && !this.status.isEmpty(); 2094 } 2095 2096 /** 2097 * @param value {@link #status} (The report status. No data will be available until the report status is complete.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 2098 */ 2099 public MeasureReport setStatusElement(Enumeration<MeasureReportStatus> value) { 2100 this.status = value; 2101 return this; 2102 } 2103 2104 /** 2105 * @return The report status. No data will be available until the report status is complete. 2106 */ 2107 public MeasureReportStatus getStatus() { 2108 return this.status == null ? null : this.status.getValue(); 2109 } 2110 2111 /** 2112 * @param value The report status. No data will be available until the report status is complete. 2113 */ 2114 public MeasureReport setStatus(MeasureReportStatus value) { 2115 if (this.status == null) 2116 this.status = new Enumeration<MeasureReportStatus>(new MeasureReportStatusEnumFactory()); 2117 this.status.setValue(value); 2118 return this; 2119 } 2120 2121 /** 2122 * @return {@link #type} (The type of measure report. This may be an individual report, which provides a single patient's score for the measure; a patient listing, which returns the list of patients that meet the various criteria in the measure; or a summary report, which returns a population count for each of the criteria in the measure.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2123 */ 2124 public Enumeration<MeasureReportType> getTypeElement() { 2125 if (this.type == null) 2126 if (Configuration.errorOnAutoCreate()) 2127 throw new Error("Attempt to auto-create MeasureReport.type"); 2128 else if (Configuration.doAutoCreate()) 2129 this.type = new Enumeration<MeasureReportType>(new MeasureReportTypeEnumFactory()); // bb 2130 return this.type; 2131 } 2132 2133 public boolean hasTypeElement() { 2134 return this.type != null && !this.type.isEmpty(); 2135 } 2136 2137 public boolean hasType() { 2138 return this.type != null && !this.type.isEmpty(); 2139 } 2140 2141 /** 2142 * @param value {@link #type} (The type of measure report. This may be an individual report, which provides a single patient's score for the measure; a patient listing, which returns the list of patients that meet the various criteria in the measure; or a summary report, which returns a population count for each of the criteria in the measure.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 2143 */ 2144 public MeasureReport setTypeElement(Enumeration<MeasureReportType> value) { 2145 this.type = value; 2146 return this; 2147 } 2148 2149 /** 2150 * @return The type of measure report. This may be an individual report, which provides a single patient's score for the measure; a patient listing, which returns the list of patients that meet the various criteria in the measure; or a summary report, which returns a population count for each of the criteria in the measure. 2151 */ 2152 public MeasureReportType getType() { 2153 return this.type == null ? null : this.type.getValue(); 2154 } 2155 2156 /** 2157 * @param value The type of measure report. This may be an individual report, which provides a single patient's score for the measure; a patient listing, which returns the list of patients that meet the various criteria in the measure; or a summary report, which returns a population count for each of the criteria in the measure. 2158 */ 2159 public MeasureReport setType(MeasureReportType value) { 2160 if (this.type == null) 2161 this.type = new Enumeration<MeasureReportType>(new MeasureReportTypeEnumFactory()); 2162 this.type.setValue(value); 2163 return this; 2164 } 2165 2166 /** 2167 * @return {@link #measure} (A reference to the Measure that was evaluated to produce this report.) 2168 */ 2169 public Reference getMeasure() { 2170 if (this.measure == null) 2171 if (Configuration.errorOnAutoCreate()) 2172 throw new Error("Attempt to auto-create MeasureReport.measure"); 2173 else if (Configuration.doAutoCreate()) 2174 this.measure = new Reference(); // cc 2175 return this.measure; 2176 } 2177 2178 public boolean hasMeasure() { 2179 return this.measure != null && !this.measure.isEmpty(); 2180 } 2181 2182 /** 2183 * @param value {@link #measure} (A reference to the Measure that was evaluated to produce this report.) 2184 */ 2185 public MeasureReport setMeasure(Reference value) { 2186 this.measure = value; 2187 return this; 2188 } 2189 2190 /** 2191 * @return {@link #measure} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the Measure that was evaluated to produce this report.) 2192 */ 2193 public Measure getMeasureTarget() { 2194 if (this.measureTarget == null) 2195 if (Configuration.errorOnAutoCreate()) 2196 throw new Error("Attempt to auto-create MeasureReport.measure"); 2197 else if (Configuration.doAutoCreate()) 2198 this.measureTarget = new Measure(); // aa 2199 return this.measureTarget; 2200 } 2201 2202 /** 2203 * @param value {@link #measure} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the Measure that was evaluated to produce this report.) 2204 */ 2205 public MeasureReport setMeasureTarget(Measure value) { 2206 this.measureTarget = value; 2207 return this; 2208 } 2209 2210 /** 2211 * @return {@link #patient} (Optional Patient if the report was requested for a single patient.) 2212 */ 2213 public Reference getPatient() { 2214 if (this.patient == null) 2215 if (Configuration.errorOnAutoCreate()) 2216 throw new Error("Attempt to auto-create MeasureReport.patient"); 2217 else if (Configuration.doAutoCreate()) 2218 this.patient = new Reference(); // cc 2219 return this.patient; 2220 } 2221 2222 public boolean hasPatient() { 2223 return this.patient != null && !this.patient.isEmpty(); 2224 } 2225 2226 /** 2227 * @param value {@link #patient} (Optional Patient if the report was requested for a single patient.) 2228 */ 2229 public MeasureReport setPatient(Reference value) { 2230 this.patient = value; 2231 return this; 2232 } 2233 2234 /** 2235 * @return {@link #patient} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Optional Patient if the report was requested for a single patient.) 2236 */ 2237 public Patient getPatientTarget() { 2238 if (this.patientTarget == null) 2239 if (Configuration.errorOnAutoCreate()) 2240 throw new Error("Attempt to auto-create MeasureReport.patient"); 2241 else if (Configuration.doAutoCreate()) 2242 this.patientTarget = new Patient(); // aa 2243 return this.patientTarget; 2244 } 2245 2246 /** 2247 * @param value {@link #patient} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Optional Patient if the report was requested for a single patient.) 2248 */ 2249 public MeasureReport setPatientTarget(Patient value) { 2250 this.patientTarget = value; 2251 return this; 2252 } 2253 2254 /** 2255 * @return {@link #date} (The date this measure report was generated.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2256 */ 2257 public DateTimeType getDateElement() { 2258 if (this.date == null) 2259 if (Configuration.errorOnAutoCreate()) 2260 throw new Error("Attempt to auto-create MeasureReport.date"); 2261 else if (Configuration.doAutoCreate()) 2262 this.date = new DateTimeType(); // bb 2263 return this.date; 2264 } 2265 2266 public boolean hasDateElement() { 2267 return this.date != null && !this.date.isEmpty(); 2268 } 2269 2270 public boolean hasDate() { 2271 return this.date != null && !this.date.isEmpty(); 2272 } 2273 2274 /** 2275 * @param value {@link #date} (The date this measure report was generated.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 2276 */ 2277 public MeasureReport setDateElement(DateTimeType value) { 2278 this.date = value; 2279 return this; 2280 } 2281 2282 /** 2283 * @return The date this measure report was generated. 2284 */ 2285 public Date getDate() { 2286 return this.date == null ? null : this.date.getValue(); 2287 } 2288 2289 /** 2290 * @param value The date this measure report was generated. 2291 */ 2292 public MeasureReport setDate(Date value) { 2293 if (value == null) 2294 this.date = null; 2295 else { 2296 if (this.date == null) 2297 this.date = new DateTimeType(); 2298 this.date.setValue(value); 2299 } 2300 return this; 2301 } 2302 2303 /** 2304 * @return {@link #reportingOrganization} (Reporting Organization.) 2305 */ 2306 public Reference getReportingOrganization() { 2307 if (this.reportingOrganization == null) 2308 if (Configuration.errorOnAutoCreate()) 2309 throw new Error("Attempt to auto-create MeasureReport.reportingOrganization"); 2310 else if (Configuration.doAutoCreate()) 2311 this.reportingOrganization = new Reference(); // cc 2312 return this.reportingOrganization; 2313 } 2314 2315 public boolean hasReportingOrganization() { 2316 return this.reportingOrganization != null && !this.reportingOrganization.isEmpty(); 2317 } 2318 2319 /** 2320 * @param value {@link #reportingOrganization} (Reporting Organization.) 2321 */ 2322 public MeasureReport setReportingOrganization(Reference value) { 2323 this.reportingOrganization = value; 2324 return this; 2325 } 2326 2327 /** 2328 * @return {@link #reportingOrganization} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Reporting Organization.) 2329 */ 2330 public Organization getReportingOrganizationTarget() { 2331 if (this.reportingOrganizationTarget == null) 2332 if (Configuration.errorOnAutoCreate()) 2333 throw new Error("Attempt to auto-create MeasureReport.reportingOrganization"); 2334 else if (Configuration.doAutoCreate()) 2335 this.reportingOrganizationTarget = new Organization(); // aa 2336 return this.reportingOrganizationTarget; 2337 } 2338 2339 /** 2340 * @param value {@link #reportingOrganization} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Reporting Organization.) 2341 */ 2342 public MeasureReport setReportingOrganizationTarget(Organization value) { 2343 this.reportingOrganizationTarget = value; 2344 return this; 2345 } 2346 2347 /** 2348 * @return {@link #period} (The reporting period for which the report was calculated.) 2349 */ 2350 public Period getPeriod() { 2351 if (this.period == null) 2352 if (Configuration.errorOnAutoCreate()) 2353 throw new Error("Attempt to auto-create MeasureReport.period"); 2354 else if (Configuration.doAutoCreate()) 2355 this.period = new Period(); // cc 2356 return this.period; 2357 } 2358 2359 public boolean hasPeriod() { 2360 return this.period != null && !this.period.isEmpty(); 2361 } 2362 2363 /** 2364 * @param value {@link #period} (The reporting period for which the report was calculated.) 2365 */ 2366 public MeasureReport setPeriod(Period value) { 2367 this.period = value; 2368 return this; 2369 } 2370 2371 /** 2372 * @return {@link #group} (The results of the calculation, one for each population group in the measure.) 2373 */ 2374 public List<MeasureReportGroupComponent> getGroup() { 2375 if (this.group == null) 2376 this.group = new ArrayList<MeasureReportGroupComponent>(); 2377 return this.group; 2378 } 2379 2380 /** 2381 * @return Returns a reference to <code>this</code> for easy method chaining 2382 */ 2383 public MeasureReport setGroup(List<MeasureReportGroupComponent> theGroup) { 2384 this.group = theGroup; 2385 return this; 2386 } 2387 2388 public boolean hasGroup() { 2389 if (this.group == null) 2390 return false; 2391 for (MeasureReportGroupComponent item : this.group) 2392 if (!item.isEmpty()) 2393 return true; 2394 return false; 2395 } 2396 2397 public MeasureReportGroupComponent addGroup() { //3 2398 MeasureReportGroupComponent t = new MeasureReportGroupComponent(); 2399 if (this.group == null) 2400 this.group = new ArrayList<MeasureReportGroupComponent>(); 2401 this.group.add(t); 2402 return t; 2403 } 2404 2405 public MeasureReport addGroup(MeasureReportGroupComponent t) { //3 2406 if (t == null) 2407 return this; 2408 if (this.group == null) 2409 this.group = new ArrayList<MeasureReportGroupComponent>(); 2410 this.group.add(t); 2411 return this; 2412 } 2413 2414 /** 2415 * @return The first repetition of repeating field {@link #group}, creating it if it does not already exist 2416 */ 2417 public MeasureReportGroupComponent getGroupFirstRep() { 2418 if (getGroup().isEmpty()) { 2419 addGroup(); 2420 } 2421 return getGroup().get(0); 2422 } 2423 2424 /** 2425 * @return {@link #evaluatedResources} (A reference to a Bundle containing the Resources that were used in the evaluation of this report.) 2426 */ 2427 public Reference getEvaluatedResources() { 2428 if (this.evaluatedResources == null) 2429 if (Configuration.errorOnAutoCreate()) 2430 throw new Error("Attempt to auto-create MeasureReport.evaluatedResources"); 2431 else if (Configuration.doAutoCreate()) 2432 this.evaluatedResources = new Reference(); // cc 2433 return this.evaluatedResources; 2434 } 2435 2436 public boolean hasEvaluatedResources() { 2437 return this.evaluatedResources != null && !this.evaluatedResources.isEmpty(); 2438 } 2439 2440 /** 2441 * @param value {@link #evaluatedResources} (A reference to a Bundle containing the Resources that were used in the evaluation of this report.) 2442 */ 2443 public MeasureReport setEvaluatedResources(Reference value) { 2444 this.evaluatedResources = value; 2445 return this; 2446 } 2447 2448 /** 2449 * @return {@link #evaluatedResources} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to a Bundle containing the Resources that were used in the evaluation of this report.) 2450 */ 2451 public Bundle getEvaluatedResourcesTarget() { 2452 if (this.evaluatedResourcesTarget == null) 2453 if (Configuration.errorOnAutoCreate()) 2454 throw new Error("Attempt to auto-create MeasureReport.evaluatedResources"); 2455 else if (Configuration.doAutoCreate()) 2456 this.evaluatedResourcesTarget = new Bundle(); // aa 2457 return this.evaluatedResourcesTarget; 2458 } 2459 2460 /** 2461 * @param value {@link #evaluatedResources} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to a Bundle containing the Resources that were used in the evaluation of this report.) 2462 */ 2463 public MeasureReport setEvaluatedResourcesTarget(Bundle value) { 2464 this.evaluatedResourcesTarget = value; 2465 return this; 2466 } 2467 2468 protected void listChildren(List<Property> children) { 2469 super.listChildren(children); 2470 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this report when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier)); 2471 children.add(new Property("status", "code", "The report status. No data will be available until the report status is complete.", 0, 1, status)); 2472 children.add(new Property("type", "code", "The type of measure report. This may be an individual report, which provides a single patient's score for the measure; a patient listing, which returns the list of patients that meet the various criteria in the measure; or a summary report, which returns a population count for each of the criteria in the measure.", 0, 1, type)); 2473 children.add(new Property("measure", "Reference(Measure)", "A reference to the Measure that was evaluated to produce this report.", 0, 1, measure)); 2474 children.add(new Property("patient", "Reference(Patient)", "Optional Patient if the report was requested for a single patient.", 0, 1, patient)); 2475 children.add(new Property("date", "dateTime", "The date this measure report was generated.", 0, 1, date)); 2476 children.add(new Property("reportingOrganization", "Reference(Organization)", "Reporting Organization.", 0, 1, reportingOrganization)); 2477 children.add(new Property("period", "Period", "The reporting period for which the report was calculated.", 0, 1, period)); 2478 children.add(new Property("group", "", "The results of the calculation, one for each population group in the measure.", 0, java.lang.Integer.MAX_VALUE, group)); 2479 children.add(new Property("evaluatedResources", "Reference(Bundle)", "A reference to a Bundle containing the Resources that were used in the evaluation of this report.", 0, 1, evaluatedResources)); 2480 } 2481 2482 @Override 2483 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2484 switch (_hash) { 2485 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this report when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier); 2486 case -892481550: /*status*/ return new Property("status", "code", "The report status. No data will be available until the report status is complete.", 0, 1, status); 2487 case 3575610: /*type*/ return new Property("type", "code", "The type of measure report. This may be an individual report, which provides a single patient's score for the measure; a patient listing, which returns the list of patients that meet the various criteria in the measure; or a summary report, which returns a population count for each of the criteria in the measure.", 0, 1, type); 2488 case 938321246: /*measure*/ return new Property("measure", "Reference(Measure)", "A reference to the Measure that was evaluated to produce this report.", 0, 1, measure); 2489 case -791418107: /*patient*/ return new Property("patient", "Reference(Patient)", "Optional Patient if the report was requested for a single patient.", 0, 1, patient); 2490 case 3076014: /*date*/ return new Property("date", "dateTime", "The date this measure report was generated.", 0, 1, date); 2491 case -2053950847: /*reportingOrganization*/ return new Property("reportingOrganization", "Reference(Organization)", "Reporting Organization.", 0, 1, reportingOrganization); 2492 case -991726143: /*period*/ return new Property("period", "Period", "The reporting period for which the report was calculated.", 0, 1, period); 2493 case 98629247: /*group*/ return new Property("group", "", "The results of the calculation, one for each population group in the measure.", 0, java.lang.Integer.MAX_VALUE, group); 2494 case 1599836026: /*evaluatedResources*/ return new Property("evaluatedResources", "Reference(Bundle)", "A reference to a Bundle containing the Resources that were used in the evaluation of this report.", 0, 1, evaluatedResources); 2495 default: return super.getNamedProperty(_hash, _name, _checkValid); 2496 } 2497 2498 } 2499 2500 @Override 2501 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2502 switch (hash) { 2503 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2504 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MeasureReportStatus> 2505 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<MeasureReportType> 2506 case 938321246: /*measure*/ return this.measure == null ? new Base[0] : new Base[] {this.measure}; // Reference 2507 case -791418107: /*patient*/ return this.patient == null ? new Base[0] : new Base[] {this.patient}; // Reference 2508 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2509 case -2053950847: /*reportingOrganization*/ return this.reportingOrganization == null ? new Base[0] : new Base[] {this.reportingOrganization}; // Reference 2510 case -991726143: /*period*/ return this.period == null ? new Base[0] : new Base[] {this.period}; // Period 2511 case 98629247: /*group*/ return this.group == null ? new Base[0] : this.group.toArray(new Base[this.group.size()]); // MeasureReportGroupComponent 2512 case 1599836026: /*evaluatedResources*/ return this.evaluatedResources == null ? new Base[0] : new Base[] {this.evaluatedResources}; // Reference 2513 default: return super.getProperty(hash, name, checkValid); 2514 } 2515 2516 } 2517 2518 @Override 2519 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2520 switch (hash) { 2521 case -1618432855: // identifier 2522 this.identifier = castToIdentifier(value); // Identifier 2523 return value; 2524 case -892481550: // status 2525 value = new MeasureReportStatusEnumFactory().fromType(castToCode(value)); 2526 this.status = (Enumeration) value; // Enumeration<MeasureReportStatus> 2527 return value; 2528 case 3575610: // type 2529 value = new MeasureReportTypeEnumFactory().fromType(castToCode(value)); 2530 this.type = (Enumeration) value; // Enumeration<MeasureReportType> 2531 return value; 2532 case 938321246: // measure 2533 this.measure = castToReference(value); // Reference 2534 return value; 2535 case -791418107: // patient 2536 this.patient = castToReference(value); // Reference 2537 return value; 2538 case 3076014: // date 2539 this.date = castToDateTime(value); // DateTimeType 2540 return value; 2541 case -2053950847: // reportingOrganization 2542 this.reportingOrganization = castToReference(value); // Reference 2543 return value; 2544 case -991726143: // period 2545 this.period = castToPeriod(value); // Period 2546 return value; 2547 case 98629247: // group 2548 this.getGroup().add((MeasureReportGroupComponent) value); // MeasureReportGroupComponent 2549 return value; 2550 case 1599836026: // evaluatedResources 2551 this.evaluatedResources = castToReference(value); // Reference 2552 return value; 2553 default: return super.setProperty(hash, name, value); 2554 } 2555 2556 } 2557 2558 @Override 2559 public Base setProperty(String name, Base value) throws FHIRException { 2560 if (name.equals("identifier")) { 2561 this.identifier = castToIdentifier(value); // Identifier 2562 } else if (name.equals("status")) { 2563 value = new MeasureReportStatusEnumFactory().fromType(castToCode(value)); 2564 this.status = (Enumeration) value; // Enumeration<MeasureReportStatus> 2565 } else if (name.equals("type")) { 2566 value = new MeasureReportTypeEnumFactory().fromType(castToCode(value)); 2567 this.type = (Enumeration) value; // Enumeration<MeasureReportType> 2568 } else if (name.equals("measure")) { 2569 this.measure = castToReference(value); // Reference 2570 } else if (name.equals("patient")) { 2571 this.patient = castToReference(value); // Reference 2572 } else if (name.equals("date")) { 2573 this.date = castToDateTime(value); // DateTimeType 2574 } else if (name.equals("reportingOrganization")) { 2575 this.reportingOrganization = castToReference(value); // Reference 2576 } else if (name.equals("period")) { 2577 this.period = castToPeriod(value); // Period 2578 } else if (name.equals("group")) { 2579 this.getGroup().add((MeasureReportGroupComponent) value); 2580 } else if (name.equals("evaluatedResources")) { 2581 this.evaluatedResources = castToReference(value); // Reference 2582 } else 2583 return super.setProperty(name, value); 2584 return value; 2585 } 2586 2587 @Override 2588 public Base makeProperty(int hash, String name) throws FHIRException { 2589 switch (hash) { 2590 case -1618432855: return getIdentifier(); 2591 case -892481550: return getStatusElement(); 2592 case 3575610: return getTypeElement(); 2593 case 938321246: return getMeasure(); 2594 case -791418107: return getPatient(); 2595 case 3076014: return getDateElement(); 2596 case -2053950847: return getReportingOrganization(); 2597 case -991726143: return getPeriod(); 2598 case 98629247: return addGroup(); 2599 case 1599836026: return getEvaluatedResources(); 2600 default: return super.makeProperty(hash, name); 2601 } 2602 2603 } 2604 2605 @Override 2606 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2607 switch (hash) { 2608 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2609 case -892481550: /*status*/ return new String[] {"code"}; 2610 case 3575610: /*type*/ return new String[] {"code"}; 2611 case 938321246: /*measure*/ return new String[] {"Reference"}; 2612 case -791418107: /*patient*/ return new String[] {"Reference"}; 2613 case 3076014: /*date*/ return new String[] {"dateTime"}; 2614 case -2053950847: /*reportingOrganization*/ return new String[] {"Reference"}; 2615 case -991726143: /*period*/ return new String[] {"Period"}; 2616 case 98629247: /*group*/ return new String[] {}; 2617 case 1599836026: /*evaluatedResources*/ return new String[] {"Reference"}; 2618 default: return super.getTypesForProperty(hash, name); 2619 } 2620 2621 } 2622 2623 @Override 2624 public Base addChild(String name) throws FHIRException { 2625 if (name.equals("identifier")) { 2626 this.identifier = new Identifier(); 2627 return this.identifier; 2628 } 2629 else if (name.equals("status")) { 2630 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.status"); 2631 } 2632 else if (name.equals("type")) { 2633 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.type"); 2634 } 2635 else if (name.equals("measure")) { 2636 this.measure = new Reference(); 2637 return this.measure; 2638 } 2639 else if (name.equals("patient")) { 2640 this.patient = new Reference(); 2641 return this.patient; 2642 } 2643 else if (name.equals("date")) { 2644 throw new FHIRException("Cannot call addChild on a singleton property MeasureReport.date"); 2645 } 2646 else if (name.equals("reportingOrganization")) { 2647 this.reportingOrganization = new Reference(); 2648 return this.reportingOrganization; 2649 } 2650 else if (name.equals("period")) { 2651 this.period = new Period(); 2652 return this.period; 2653 } 2654 else if (name.equals("group")) { 2655 return addGroup(); 2656 } 2657 else if (name.equals("evaluatedResources")) { 2658 this.evaluatedResources = new Reference(); 2659 return this.evaluatedResources; 2660 } 2661 else 2662 return super.addChild(name); 2663 } 2664 2665 public String fhirType() { 2666 return "MeasureReport"; 2667 2668 } 2669 2670 public MeasureReport copy() { 2671 MeasureReport dst = new MeasureReport(); 2672 copyValues(dst); 2673 dst.identifier = identifier == null ? null : identifier.copy(); 2674 dst.status = status == null ? null : status.copy(); 2675 dst.type = type == null ? null : type.copy(); 2676 dst.measure = measure == null ? null : measure.copy(); 2677 dst.patient = patient == null ? null : patient.copy(); 2678 dst.date = date == null ? null : date.copy(); 2679 dst.reportingOrganization = reportingOrganization == null ? null : reportingOrganization.copy(); 2680 dst.period = period == null ? null : period.copy(); 2681 if (group != null) { 2682 dst.group = new ArrayList<MeasureReportGroupComponent>(); 2683 for (MeasureReportGroupComponent i : group) 2684 dst.group.add(i.copy()); 2685 }; 2686 dst.evaluatedResources = evaluatedResources == null ? null : evaluatedResources.copy(); 2687 return dst; 2688 } 2689 2690 protected MeasureReport typedCopy() { 2691 return copy(); 2692 } 2693 2694 @Override 2695 public boolean equalsDeep(Base other_) { 2696 if (!super.equalsDeep(other_)) 2697 return false; 2698 if (!(other_ instanceof MeasureReport)) 2699 return false; 2700 MeasureReport o = (MeasureReport) other_; 2701 return compareDeep(identifier, o.identifier, true) && compareDeep(status, o.status, true) && compareDeep(type, o.type, true) 2702 && compareDeep(measure, o.measure, true) && compareDeep(patient, o.patient, true) && compareDeep(date, o.date, true) 2703 && compareDeep(reportingOrganization, o.reportingOrganization, true) && compareDeep(period, o.period, true) 2704 && compareDeep(group, o.group, true) && compareDeep(evaluatedResources, o.evaluatedResources, true) 2705 ; 2706 } 2707 2708 @Override 2709 public boolean equalsShallow(Base other_) { 2710 if (!super.equalsShallow(other_)) 2711 return false; 2712 if (!(other_ instanceof MeasureReport)) 2713 return false; 2714 MeasureReport o = (MeasureReport) other_; 2715 return compareValues(status, o.status, true) && compareValues(type, o.type, true) && compareValues(date, o.date, true) 2716 ; 2717 } 2718 2719 public boolean isEmpty() { 2720 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, status, type 2721 , measure, patient, date, reportingOrganization, period, group, evaluatedResources 2722 ); 2723 } 2724 2725 @Override 2726 public ResourceType getResourceType() { 2727 return ResourceType.MeasureReport; 2728 } 2729 2730 /** 2731 * Search parameter: <b>identifier</b> 2732 * <p> 2733 * Description: <b>External identifier of the measure report to be returned</b><br> 2734 * Type: <b>token</b><br> 2735 * Path: <b>MeasureReport.identifier</b><br> 2736 * </p> 2737 */ 2738 @SearchParamDefinition(name="identifier", path="MeasureReport.identifier", description="External identifier of the measure report to be returned", type="token" ) 2739 public static final String SP_IDENTIFIER = "identifier"; 2740 /** 2741 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2742 * <p> 2743 * Description: <b>External identifier of the measure report to be returned</b><br> 2744 * Type: <b>token</b><br> 2745 * Path: <b>MeasureReport.identifier</b><br> 2746 * </p> 2747 */ 2748 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2749 2750 /** 2751 * Search parameter: <b>patient</b> 2752 * <p> 2753 * Description: <b>The identity of a patient to search for individual measure report results for</b><br> 2754 * Type: <b>reference</b><br> 2755 * Path: <b>MeasureReport.patient</b><br> 2756 * </p> 2757 */ 2758 @SearchParamDefinition(name="patient", path="MeasureReport.patient", description="The identity of a patient to search for individual measure report results for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2759 public static final String SP_PATIENT = "patient"; 2760 /** 2761 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2762 * <p> 2763 * Description: <b>The identity of a patient to search for individual measure report results for</b><br> 2764 * Type: <b>reference</b><br> 2765 * Path: <b>MeasureReport.patient</b><br> 2766 * </p> 2767 */ 2768 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2769 2770/** 2771 * Constant for fluent queries to be used to add include statements. Specifies 2772 * the path value of "<b>MeasureReport:patient</b>". 2773 */ 2774 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MeasureReport:patient").toLocked(); 2775 2776 /** 2777 * Search parameter: <b>status</b> 2778 * <p> 2779 * Description: <b>The status of the measure report</b><br> 2780 * Type: <b>token</b><br> 2781 * Path: <b>MeasureReport.status</b><br> 2782 * </p> 2783 */ 2784 @SearchParamDefinition(name="status", path="MeasureReport.status", description="The status of the measure report", type="token" ) 2785 public static final String SP_STATUS = "status"; 2786 /** 2787 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2788 * <p> 2789 * Description: <b>The status of the measure report</b><br> 2790 * Type: <b>token</b><br> 2791 * Path: <b>MeasureReport.status</b><br> 2792 * </p> 2793 */ 2794 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2795 2796 2797}