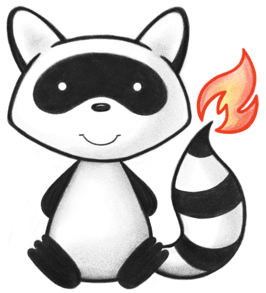
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * A photo, video, or audio recording acquired or used in healthcare. The actual content may be inline or provided by direct reference. 048 */ 049@ResourceDef(name="Media", profile="http://hl7.org/fhir/Profile/Media") 050public class Media extends DomainResource { 051 052 public enum DigitalMediaType { 053 /** 054 * The media consists of one or more unmoving images, including photographs, computer-generated graphs and charts, and scanned documents 055 */ 056 PHOTO, 057 /** 058 * The media consists of a series of frames that capture a moving image 059 */ 060 VIDEO, 061 /** 062 * The media consists of a sound recording 063 */ 064 AUDIO, 065 /** 066 * added to help the parsers with the generic types 067 */ 068 NULL; 069 public static DigitalMediaType fromCode(String codeString) throws FHIRException { 070 if (codeString == null || "".equals(codeString)) 071 return null; 072 if ("photo".equals(codeString)) 073 return PHOTO; 074 if ("video".equals(codeString)) 075 return VIDEO; 076 if ("audio".equals(codeString)) 077 return AUDIO; 078 if (Configuration.isAcceptInvalidEnums()) 079 return null; 080 else 081 throw new FHIRException("Unknown DigitalMediaType code '"+codeString+"'"); 082 } 083 public String toCode() { 084 switch (this) { 085 case PHOTO: return "photo"; 086 case VIDEO: return "video"; 087 case AUDIO: return "audio"; 088 case NULL: return null; 089 default: return "?"; 090 } 091 } 092 public String getSystem() { 093 switch (this) { 094 case PHOTO: return "http://hl7.org/fhir/digital-media-type"; 095 case VIDEO: return "http://hl7.org/fhir/digital-media-type"; 096 case AUDIO: return "http://hl7.org/fhir/digital-media-type"; 097 case NULL: return null; 098 default: return "?"; 099 } 100 } 101 public String getDefinition() { 102 switch (this) { 103 case PHOTO: return "The media consists of one or more unmoving images, including photographs, computer-generated graphs and charts, and scanned documents"; 104 case VIDEO: return "The media consists of a series of frames that capture a moving image"; 105 case AUDIO: return "The media consists of a sound recording"; 106 case NULL: return null; 107 default: return "?"; 108 } 109 } 110 public String getDisplay() { 111 switch (this) { 112 case PHOTO: return "Photo"; 113 case VIDEO: return "Video"; 114 case AUDIO: return "Audio"; 115 case NULL: return null; 116 default: return "?"; 117 } 118 } 119 } 120 121 public static class DigitalMediaTypeEnumFactory implements EnumFactory<DigitalMediaType> { 122 public DigitalMediaType fromCode(String codeString) throws IllegalArgumentException { 123 if (codeString == null || "".equals(codeString)) 124 if (codeString == null || "".equals(codeString)) 125 return null; 126 if ("photo".equals(codeString)) 127 return DigitalMediaType.PHOTO; 128 if ("video".equals(codeString)) 129 return DigitalMediaType.VIDEO; 130 if ("audio".equals(codeString)) 131 return DigitalMediaType.AUDIO; 132 throw new IllegalArgumentException("Unknown DigitalMediaType code '"+codeString+"'"); 133 } 134 public Enumeration<DigitalMediaType> fromType(PrimitiveType<?> code) throws FHIRException { 135 if (code == null) 136 return null; 137 if (code.isEmpty()) 138 return new Enumeration<DigitalMediaType>(this); 139 String codeString = code.asStringValue(); 140 if (codeString == null || "".equals(codeString)) 141 return null; 142 if ("photo".equals(codeString)) 143 return new Enumeration<DigitalMediaType>(this, DigitalMediaType.PHOTO); 144 if ("video".equals(codeString)) 145 return new Enumeration<DigitalMediaType>(this, DigitalMediaType.VIDEO); 146 if ("audio".equals(codeString)) 147 return new Enumeration<DigitalMediaType>(this, DigitalMediaType.AUDIO); 148 throw new FHIRException("Unknown DigitalMediaType code '"+codeString+"'"); 149 } 150 public String toCode(DigitalMediaType code) { 151 if (code == DigitalMediaType.NULL) 152 return null; 153 if (code == DigitalMediaType.PHOTO) 154 return "photo"; 155 if (code == DigitalMediaType.VIDEO) 156 return "video"; 157 if (code == DigitalMediaType.AUDIO) 158 return "audio"; 159 return "?"; 160 } 161 public String toSystem(DigitalMediaType code) { 162 return code.getSystem(); 163 } 164 } 165 166 /** 167 * Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers. 168 */ 169 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 170 @Description(shortDefinition="Identifier(s) for the image", formalDefinition="Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers." ) 171 protected List<Identifier> identifier; 172 173 /** 174 * A procedure that is fulfilled in whole or in part by the creation of this media. 175 */ 176 @Child(name = "basedOn", type = {ProcedureRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 177 @Description(shortDefinition="Procedure that caused this media to be created", formalDefinition="A procedure that is fulfilled in whole or in part by the creation of this media." ) 178 protected List<Reference> basedOn; 179 /** 180 * The actual objects that are the target of the reference (A procedure that is fulfilled in whole or in part by the creation of this media.) 181 */ 182 protected List<ProcedureRequest> basedOnTarget; 183 184 185 /** 186 * Whether the media is a photo (still image), an audio recording, or a video recording. 187 */ 188 @Child(name = "type", type = {CodeType.class}, order=2, min=1, max=1, modifier=false, summary=true) 189 @Description(shortDefinition="photo | video | audio", formalDefinition="Whether the media is a photo (still image), an audio recording, or a video recording." ) 190 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/digital-media-type") 191 protected Enumeration<DigitalMediaType> type; 192 193 /** 194 * Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality. 195 */ 196 @Child(name = "subtype", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=true) 197 @Description(shortDefinition="The type of acquisition equipment/process", formalDefinition="Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality." ) 198 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/digital-media-subtype") 199 protected CodeableConcept subtype; 200 201 /** 202 * The name of the imaging view e.g. Lateral or Antero-posterior (AP). 203 */ 204 @Child(name = "view", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=true) 205 @Description(shortDefinition="Imaging view, e.g. Lateral or Antero-posterior", formalDefinition="The name of the imaging view e.g. Lateral or Antero-posterior (AP)." ) 206 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/media-view") 207 protected CodeableConcept view; 208 209 /** 210 * Who/What this Media is a record of. 211 */ 212 @Child(name = "subject", type = {Patient.class, Practitioner.class, Group.class, Device.class, Specimen.class}, order=5, min=0, max=1, modifier=false, summary=true) 213 @Description(shortDefinition="Who/What this Media is a record of", formalDefinition="Who/What this Media is a record of." ) 214 protected Reference subject; 215 216 /** 217 * The actual object that is the target of the reference (Who/What this Media is a record of.) 218 */ 219 protected Resource subjectTarget; 220 221 /** 222 * The encounter or episode of care that establishes the context for this media. 223 */ 224 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=true) 225 @Description(shortDefinition="Encounter / Episode associated with media", formalDefinition="The encounter or episode of care that establishes the context for this media." ) 226 protected Reference context; 227 228 /** 229 * The actual object that is the target of the reference (The encounter or episode of care that establishes the context for this media.) 230 */ 231 protected Resource contextTarget; 232 233 /** 234 * The date and time(s) at which the media was collected. 235 */ 236 @Child(name = "occurrence", type = {DateTimeType.class, Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 237 @Description(shortDefinition="When Media was collected", formalDefinition="The date and time(s) at which the media was collected." ) 238 protected Type occurrence; 239 240 /** 241 * The person who administered the collection of the image. 242 */ 243 @Child(name = "operator", type = {Practitioner.class}, order=8, min=0, max=1, modifier=false, summary=true) 244 @Description(shortDefinition="The person who generated the image", formalDefinition="The person who administered the collection of the image." ) 245 protected Reference operator; 246 247 /** 248 * The actual object that is the target of the reference (The person who administered the collection of the image.) 249 */ 250 protected Practitioner operatorTarget; 251 252 /** 253 * Describes why the event occurred in coded or textual form. 254 */ 255 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 256 @Description(shortDefinition="Why was event performed?", formalDefinition="Describes why the event occurred in coded or textual form." ) 257 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/procedure-reason") 258 protected List<CodeableConcept> reasonCode; 259 260 /** 261 * Indicates the site on the subject's body where the media was collected (i.e. the target site). 262 */ 263 @Child(name = "bodySite", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=true) 264 @Description(shortDefinition="Body part in media", formalDefinition="Indicates the site on the subject's body where the media was collected (i.e. the target site)." ) 265 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/body-site") 266 protected CodeableConcept bodySite; 267 268 /** 269 * The device used to collect the media. 270 */ 271 @Child(name = "device", type = {Device.class, DeviceMetric.class}, order=11, min=0, max=1, modifier=false, summary=true) 272 @Description(shortDefinition="Observing Device", formalDefinition="The device used to collect the media." ) 273 protected Reference device; 274 275 /** 276 * The actual object that is the target of the reference (The device used to collect the media.) 277 */ 278 protected Resource deviceTarget; 279 280 /** 281 * Height of the image in pixels (photo/video). 282 */ 283 @Child(name = "height", type = {PositiveIntType.class}, order=12, min=0, max=1, modifier=false, summary=true) 284 @Description(shortDefinition="Height of the image in pixels (photo/video)", formalDefinition="Height of the image in pixels (photo/video)." ) 285 protected PositiveIntType height; 286 287 /** 288 * Width of the image in pixels (photo/video). 289 */ 290 @Child(name = "width", type = {PositiveIntType.class}, order=13, min=0, max=1, modifier=false, summary=true) 291 @Description(shortDefinition="Width of the image in pixels (photo/video)", formalDefinition="Width of the image in pixels (photo/video)." ) 292 protected PositiveIntType width; 293 294 /** 295 * The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required. 296 */ 297 @Child(name = "frames", type = {PositiveIntType.class}, order=14, min=0, max=1, modifier=false, summary=true) 298 @Description(shortDefinition="Number of frames if > 1 (photo)", formalDefinition="The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required." ) 299 protected PositiveIntType frames; 300 301 /** 302 * The duration of the recording in seconds - for audio and video. 303 */ 304 @Child(name = "duration", type = {UnsignedIntType.class}, order=15, min=0, max=1, modifier=false, summary=true) 305 @Description(shortDefinition="Length in seconds (audio / video)", formalDefinition="The duration of the recording in seconds - for audio and video." ) 306 protected UnsignedIntType duration; 307 308 /** 309 * The actual content of the media - inline or by direct reference to the media source file. 310 */ 311 @Child(name = "content", type = {Attachment.class}, order=16, min=1, max=1, modifier=false, summary=false) 312 @Description(shortDefinition="Actual Media - reference or data", formalDefinition="The actual content of the media - inline or by direct reference to the media source file." ) 313 protected Attachment content; 314 315 /** 316 * Comments made about the media by the performer, subject or other participants. 317 */ 318 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 319 @Description(shortDefinition="Comments made about the media", formalDefinition="Comments made about the media by the performer, subject or other participants." ) 320 protected List<Annotation> note; 321 322 private static final long serialVersionUID = -831317677L; 323 324 /** 325 * Constructor 326 */ 327 public Media() { 328 super(); 329 } 330 331 /** 332 * Constructor 333 */ 334 public Media(Enumeration<DigitalMediaType> type, Attachment content) { 335 super(); 336 this.type = type; 337 this.content = content; 338 } 339 340 /** 341 * @return {@link #identifier} (Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.) 342 */ 343 public List<Identifier> getIdentifier() { 344 if (this.identifier == null) 345 this.identifier = new ArrayList<Identifier>(); 346 return this.identifier; 347 } 348 349 /** 350 * @return Returns a reference to <code>this</code> for easy method chaining 351 */ 352 public Media setIdentifier(List<Identifier> theIdentifier) { 353 this.identifier = theIdentifier; 354 return this; 355 } 356 357 public boolean hasIdentifier() { 358 if (this.identifier == null) 359 return false; 360 for (Identifier item : this.identifier) 361 if (!item.isEmpty()) 362 return true; 363 return false; 364 } 365 366 public Identifier addIdentifier() { //3 367 Identifier t = new Identifier(); 368 if (this.identifier == null) 369 this.identifier = new ArrayList<Identifier>(); 370 this.identifier.add(t); 371 return t; 372 } 373 374 public Media addIdentifier(Identifier t) { //3 375 if (t == null) 376 return this; 377 if (this.identifier == null) 378 this.identifier = new ArrayList<Identifier>(); 379 this.identifier.add(t); 380 return this; 381 } 382 383 /** 384 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 385 */ 386 public Identifier getIdentifierFirstRep() { 387 if (getIdentifier().isEmpty()) { 388 addIdentifier(); 389 } 390 return getIdentifier().get(0); 391 } 392 393 /** 394 * @return {@link #basedOn} (A procedure that is fulfilled in whole or in part by the creation of this media.) 395 */ 396 public List<Reference> getBasedOn() { 397 if (this.basedOn == null) 398 this.basedOn = new ArrayList<Reference>(); 399 return this.basedOn; 400 } 401 402 /** 403 * @return Returns a reference to <code>this</code> for easy method chaining 404 */ 405 public Media setBasedOn(List<Reference> theBasedOn) { 406 this.basedOn = theBasedOn; 407 return this; 408 } 409 410 public boolean hasBasedOn() { 411 if (this.basedOn == null) 412 return false; 413 for (Reference item : this.basedOn) 414 if (!item.isEmpty()) 415 return true; 416 return false; 417 } 418 419 public Reference addBasedOn() { //3 420 Reference t = new Reference(); 421 if (this.basedOn == null) 422 this.basedOn = new ArrayList<Reference>(); 423 this.basedOn.add(t); 424 return t; 425 } 426 427 public Media addBasedOn(Reference t) { //3 428 if (t == null) 429 return this; 430 if (this.basedOn == null) 431 this.basedOn = new ArrayList<Reference>(); 432 this.basedOn.add(t); 433 return this; 434 } 435 436 /** 437 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 438 */ 439 public Reference getBasedOnFirstRep() { 440 if (getBasedOn().isEmpty()) { 441 addBasedOn(); 442 } 443 return getBasedOn().get(0); 444 } 445 446 /** 447 * @deprecated Use Reference#setResource(IBaseResource) instead 448 */ 449 @Deprecated 450 public List<ProcedureRequest> getBasedOnTarget() { 451 if (this.basedOnTarget == null) 452 this.basedOnTarget = new ArrayList<ProcedureRequest>(); 453 return this.basedOnTarget; 454 } 455 456 /** 457 * @deprecated Use Reference#setResource(IBaseResource) instead 458 */ 459 @Deprecated 460 public ProcedureRequest addBasedOnTarget() { 461 ProcedureRequest r = new ProcedureRequest(); 462 if (this.basedOnTarget == null) 463 this.basedOnTarget = new ArrayList<ProcedureRequest>(); 464 this.basedOnTarget.add(r); 465 return r; 466 } 467 468 /** 469 * @return {@link #type} (Whether the media is a photo (still image), an audio recording, or a video recording.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 470 */ 471 public Enumeration<DigitalMediaType> getTypeElement() { 472 if (this.type == null) 473 if (Configuration.errorOnAutoCreate()) 474 throw new Error("Attempt to auto-create Media.type"); 475 else if (Configuration.doAutoCreate()) 476 this.type = new Enumeration<DigitalMediaType>(new DigitalMediaTypeEnumFactory()); // bb 477 return this.type; 478 } 479 480 public boolean hasTypeElement() { 481 return this.type != null && !this.type.isEmpty(); 482 } 483 484 public boolean hasType() { 485 return this.type != null && !this.type.isEmpty(); 486 } 487 488 /** 489 * @param value {@link #type} (Whether the media is a photo (still image), an audio recording, or a video recording.). This is the underlying object with id, value and extensions. The accessor "getType" gives direct access to the value 490 */ 491 public Media setTypeElement(Enumeration<DigitalMediaType> value) { 492 this.type = value; 493 return this; 494 } 495 496 /** 497 * @return Whether the media is a photo (still image), an audio recording, or a video recording. 498 */ 499 public DigitalMediaType getType() { 500 return this.type == null ? null : this.type.getValue(); 501 } 502 503 /** 504 * @param value Whether the media is a photo (still image), an audio recording, or a video recording. 505 */ 506 public Media setType(DigitalMediaType value) { 507 if (this.type == null) 508 this.type = new Enumeration<DigitalMediaType>(new DigitalMediaTypeEnumFactory()); 509 this.type.setValue(value); 510 return this; 511 } 512 513 /** 514 * @return {@link #subtype} (Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.) 515 */ 516 public CodeableConcept getSubtype() { 517 if (this.subtype == null) 518 if (Configuration.errorOnAutoCreate()) 519 throw new Error("Attempt to auto-create Media.subtype"); 520 else if (Configuration.doAutoCreate()) 521 this.subtype = new CodeableConcept(); // cc 522 return this.subtype; 523 } 524 525 public boolean hasSubtype() { 526 return this.subtype != null && !this.subtype.isEmpty(); 527 } 528 529 /** 530 * @param value {@link #subtype} (Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.) 531 */ 532 public Media setSubtype(CodeableConcept value) { 533 this.subtype = value; 534 return this; 535 } 536 537 /** 538 * @return {@link #view} (The name of the imaging view e.g. Lateral or Antero-posterior (AP).) 539 */ 540 public CodeableConcept getView() { 541 if (this.view == null) 542 if (Configuration.errorOnAutoCreate()) 543 throw new Error("Attempt to auto-create Media.view"); 544 else if (Configuration.doAutoCreate()) 545 this.view = new CodeableConcept(); // cc 546 return this.view; 547 } 548 549 public boolean hasView() { 550 return this.view != null && !this.view.isEmpty(); 551 } 552 553 /** 554 * @param value {@link #view} (The name of the imaging view e.g. Lateral or Antero-posterior (AP).) 555 */ 556 public Media setView(CodeableConcept value) { 557 this.view = value; 558 return this; 559 } 560 561 /** 562 * @return {@link #subject} (Who/What this Media is a record of.) 563 */ 564 public Reference getSubject() { 565 if (this.subject == null) 566 if (Configuration.errorOnAutoCreate()) 567 throw new Error("Attempt to auto-create Media.subject"); 568 else if (Configuration.doAutoCreate()) 569 this.subject = new Reference(); // cc 570 return this.subject; 571 } 572 573 public boolean hasSubject() { 574 return this.subject != null && !this.subject.isEmpty(); 575 } 576 577 /** 578 * @param value {@link #subject} (Who/What this Media is a record of.) 579 */ 580 public Media setSubject(Reference value) { 581 this.subject = value; 582 return this; 583 } 584 585 /** 586 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Who/What this Media is a record of.) 587 */ 588 public Resource getSubjectTarget() { 589 return this.subjectTarget; 590 } 591 592 /** 593 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Who/What this Media is a record of.) 594 */ 595 public Media setSubjectTarget(Resource value) { 596 this.subjectTarget = value; 597 return this; 598 } 599 600 /** 601 * @return {@link #context} (The encounter or episode of care that establishes the context for this media.) 602 */ 603 public Reference getContext() { 604 if (this.context == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create Media.context"); 607 else if (Configuration.doAutoCreate()) 608 this.context = new Reference(); // cc 609 return this.context; 610 } 611 612 public boolean hasContext() { 613 return this.context != null && !this.context.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #context} (The encounter or episode of care that establishes the context for this media.) 618 */ 619 public Media setContext(Reference value) { 620 this.context = value; 621 return this; 622 } 623 624 /** 625 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this media.) 626 */ 627 public Resource getContextTarget() { 628 return this.contextTarget; 629 } 630 631 /** 632 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this media.) 633 */ 634 public Media setContextTarget(Resource value) { 635 this.contextTarget = value; 636 return this; 637 } 638 639 /** 640 * @return {@link #occurrence} (The date and time(s) at which the media was collected.) 641 */ 642 public Type getOccurrence() { 643 return this.occurrence; 644 } 645 646 /** 647 * @return {@link #occurrence} (The date and time(s) at which the media was collected.) 648 */ 649 public DateTimeType getOccurrenceDateTimeType() throws FHIRException { 650 if (this.occurrence == null) 651 return null; 652 if (!(this.occurrence instanceof DateTimeType)) 653 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 654 return (DateTimeType) this.occurrence; 655 } 656 657 public boolean hasOccurrenceDateTimeType() { 658 return this != null && this.occurrence instanceof DateTimeType; 659 } 660 661 /** 662 * @return {@link #occurrence} (The date and time(s) at which the media was collected.) 663 */ 664 public Period getOccurrencePeriod() throws FHIRException { 665 if (this.occurrence == null) 666 return null; 667 if (!(this.occurrence instanceof Period)) 668 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.occurrence.getClass().getName()+" was encountered"); 669 return (Period) this.occurrence; 670 } 671 672 public boolean hasOccurrencePeriod() { 673 return this != null && this.occurrence instanceof Period; 674 } 675 676 public boolean hasOccurrence() { 677 return this.occurrence != null && !this.occurrence.isEmpty(); 678 } 679 680 /** 681 * @param value {@link #occurrence} (The date and time(s) at which the media was collected.) 682 */ 683 public Media setOccurrence(Type value) throws FHIRFormatError { 684 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 685 throw new FHIRFormatError("Not the right type for Media.occurrence[x]: "+value.fhirType()); 686 this.occurrence = value; 687 return this; 688 } 689 690 /** 691 * @return {@link #operator} (The person who administered the collection of the image.) 692 */ 693 public Reference getOperator() { 694 if (this.operator == null) 695 if (Configuration.errorOnAutoCreate()) 696 throw new Error("Attempt to auto-create Media.operator"); 697 else if (Configuration.doAutoCreate()) 698 this.operator = new Reference(); // cc 699 return this.operator; 700 } 701 702 public boolean hasOperator() { 703 return this.operator != null && !this.operator.isEmpty(); 704 } 705 706 /** 707 * @param value {@link #operator} (The person who administered the collection of the image.) 708 */ 709 public Media setOperator(Reference value) { 710 this.operator = value; 711 return this; 712 } 713 714 /** 715 * @return {@link #operator} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person who administered the collection of the image.) 716 */ 717 public Practitioner getOperatorTarget() { 718 if (this.operatorTarget == null) 719 if (Configuration.errorOnAutoCreate()) 720 throw new Error("Attempt to auto-create Media.operator"); 721 else if (Configuration.doAutoCreate()) 722 this.operatorTarget = new Practitioner(); // aa 723 return this.operatorTarget; 724 } 725 726 /** 727 * @param value {@link #operator} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person who administered the collection of the image.) 728 */ 729 public Media setOperatorTarget(Practitioner value) { 730 this.operatorTarget = value; 731 return this; 732 } 733 734 /** 735 * @return {@link #reasonCode} (Describes why the event occurred in coded or textual form.) 736 */ 737 public List<CodeableConcept> getReasonCode() { 738 if (this.reasonCode == null) 739 this.reasonCode = new ArrayList<CodeableConcept>(); 740 return this.reasonCode; 741 } 742 743 /** 744 * @return Returns a reference to <code>this</code> for easy method chaining 745 */ 746 public Media setReasonCode(List<CodeableConcept> theReasonCode) { 747 this.reasonCode = theReasonCode; 748 return this; 749 } 750 751 public boolean hasReasonCode() { 752 if (this.reasonCode == null) 753 return false; 754 for (CodeableConcept item : this.reasonCode) 755 if (!item.isEmpty()) 756 return true; 757 return false; 758 } 759 760 public CodeableConcept addReasonCode() { //3 761 CodeableConcept t = new CodeableConcept(); 762 if (this.reasonCode == null) 763 this.reasonCode = new ArrayList<CodeableConcept>(); 764 this.reasonCode.add(t); 765 return t; 766 } 767 768 public Media addReasonCode(CodeableConcept t) { //3 769 if (t == null) 770 return this; 771 if (this.reasonCode == null) 772 this.reasonCode = new ArrayList<CodeableConcept>(); 773 this.reasonCode.add(t); 774 return this; 775 } 776 777 /** 778 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 779 */ 780 public CodeableConcept getReasonCodeFirstRep() { 781 if (getReasonCode().isEmpty()) { 782 addReasonCode(); 783 } 784 return getReasonCode().get(0); 785 } 786 787 /** 788 * @return {@link #bodySite} (Indicates the site on the subject's body where the media was collected (i.e. the target site).) 789 */ 790 public CodeableConcept getBodySite() { 791 if (this.bodySite == null) 792 if (Configuration.errorOnAutoCreate()) 793 throw new Error("Attempt to auto-create Media.bodySite"); 794 else if (Configuration.doAutoCreate()) 795 this.bodySite = new CodeableConcept(); // cc 796 return this.bodySite; 797 } 798 799 public boolean hasBodySite() { 800 return this.bodySite != null && !this.bodySite.isEmpty(); 801 } 802 803 /** 804 * @param value {@link #bodySite} (Indicates the site on the subject's body where the media was collected (i.e. the target site).) 805 */ 806 public Media setBodySite(CodeableConcept value) { 807 this.bodySite = value; 808 return this; 809 } 810 811 /** 812 * @return {@link #device} (The device used to collect the media.) 813 */ 814 public Reference getDevice() { 815 if (this.device == null) 816 if (Configuration.errorOnAutoCreate()) 817 throw new Error("Attempt to auto-create Media.device"); 818 else if (Configuration.doAutoCreate()) 819 this.device = new Reference(); // cc 820 return this.device; 821 } 822 823 public boolean hasDevice() { 824 return this.device != null && !this.device.isEmpty(); 825 } 826 827 /** 828 * @param value {@link #device} (The device used to collect the media.) 829 */ 830 public Media setDevice(Reference value) { 831 this.device = value; 832 return this; 833 } 834 835 /** 836 * @return {@link #device} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device used to collect the media.) 837 */ 838 public Resource getDeviceTarget() { 839 return this.deviceTarget; 840 } 841 842 /** 843 * @param value {@link #device} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device used to collect the media.) 844 */ 845 public Media setDeviceTarget(Resource value) { 846 this.deviceTarget = value; 847 return this; 848 } 849 850 /** 851 * @return {@link #height} (Height of the image in pixels (photo/video).). This is the underlying object with id, value and extensions. The accessor "getHeight" gives direct access to the value 852 */ 853 public PositiveIntType getHeightElement() { 854 if (this.height == null) 855 if (Configuration.errorOnAutoCreate()) 856 throw new Error("Attempt to auto-create Media.height"); 857 else if (Configuration.doAutoCreate()) 858 this.height = new PositiveIntType(); // bb 859 return this.height; 860 } 861 862 public boolean hasHeightElement() { 863 return this.height != null && !this.height.isEmpty(); 864 } 865 866 public boolean hasHeight() { 867 return this.height != null && !this.height.isEmpty(); 868 } 869 870 /** 871 * @param value {@link #height} (Height of the image in pixels (photo/video).). This is the underlying object with id, value and extensions. The accessor "getHeight" gives direct access to the value 872 */ 873 public Media setHeightElement(PositiveIntType value) { 874 this.height = value; 875 return this; 876 } 877 878 /** 879 * @return Height of the image in pixels (photo/video). 880 */ 881 public int getHeight() { 882 return this.height == null || this.height.isEmpty() ? 0 : this.height.getValue(); 883 } 884 885 /** 886 * @param value Height of the image in pixels (photo/video). 887 */ 888 public Media setHeight(int value) { 889 if (this.height == null) 890 this.height = new PositiveIntType(); 891 this.height.setValue(value); 892 return this; 893 } 894 895 /** 896 * @return {@link #width} (Width of the image in pixels (photo/video).). This is the underlying object with id, value and extensions. The accessor "getWidth" gives direct access to the value 897 */ 898 public PositiveIntType getWidthElement() { 899 if (this.width == null) 900 if (Configuration.errorOnAutoCreate()) 901 throw new Error("Attempt to auto-create Media.width"); 902 else if (Configuration.doAutoCreate()) 903 this.width = new PositiveIntType(); // bb 904 return this.width; 905 } 906 907 public boolean hasWidthElement() { 908 return this.width != null && !this.width.isEmpty(); 909 } 910 911 public boolean hasWidth() { 912 return this.width != null && !this.width.isEmpty(); 913 } 914 915 /** 916 * @param value {@link #width} (Width of the image in pixels (photo/video).). This is the underlying object with id, value and extensions. The accessor "getWidth" gives direct access to the value 917 */ 918 public Media setWidthElement(PositiveIntType value) { 919 this.width = value; 920 return this; 921 } 922 923 /** 924 * @return Width of the image in pixels (photo/video). 925 */ 926 public int getWidth() { 927 return this.width == null || this.width.isEmpty() ? 0 : this.width.getValue(); 928 } 929 930 /** 931 * @param value Width of the image in pixels (photo/video). 932 */ 933 public Media setWidth(int value) { 934 if (this.width == null) 935 this.width = new PositiveIntType(); 936 this.width.setValue(value); 937 return this; 938 } 939 940 /** 941 * @return {@link #frames} (The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.). This is the underlying object with id, value and extensions. The accessor "getFrames" gives direct access to the value 942 */ 943 public PositiveIntType getFramesElement() { 944 if (this.frames == null) 945 if (Configuration.errorOnAutoCreate()) 946 throw new Error("Attempt to auto-create Media.frames"); 947 else if (Configuration.doAutoCreate()) 948 this.frames = new PositiveIntType(); // bb 949 return this.frames; 950 } 951 952 public boolean hasFramesElement() { 953 return this.frames != null && !this.frames.isEmpty(); 954 } 955 956 public boolean hasFrames() { 957 return this.frames != null && !this.frames.isEmpty(); 958 } 959 960 /** 961 * @param value {@link #frames} (The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.). This is the underlying object with id, value and extensions. The accessor "getFrames" gives direct access to the value 962 */ 963 public Media setFramesElement(PositiveIntType value) { 964 this.frames = value; 965 return this; 966 } 967 968 /** 969 * @return The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required. 970 */ 971 public int getFrames() { 972 return this.frames == null || this.frames.isEmpty() ? 0 : this.frames.getValue(); 973 } 974 975 /** 976 * @param value The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required. 977 */ 978 public Media setFrames(int value) { 979 if (this.frames == null) 980 this.frames = new PositiveIntType(); 981 this.frames.setValue(value); 982 return this; 983 } 984 985 /** 986 * @return {@link #duration} (The duration of the recording in seconds - for audio and video.). This is the underlying object with id, value and extensions. The accessor "getDuration" gives direct access to the value 987 */ 988 public UnsignedIntType getDurationElement() { 989 if (this.duration == null) 990 if (Configuration.errorOnAutoCreate()) 991 throw new Error("Attempt to auto-create Media.duration"); 992 else if (Configuration.doAutoCreate()) 993 this.duration = new UnsignedIntType(); // bb 994 return this.duration; 995 } 996 997 public boolean hasDurationElement() { 998 return this.duration != null && !this.duration.isEmpty(); 999 } 1000 1001 public boolean hasDuration() { 1002 return this.duration != null && !this.duration.isEmpty(); 1003 } 1004 1005 /** 1006 * @param value {@link #duration} (The duration of the recording in seconds - for audio and video.). This is the underlying object with id, value and extensions. The accessor "getDuration" gives direct access to the value 1007 */ 1008 public Media setDurationElement(UnsignedIntType value) { 1009 this.duration = value; 1010 return this; 1011 } 1012 1013 /** 1014 * @return The duration of the recording in seconds - for audio and video. 1015 */ 1016 public int getDuration() { 1017 return this.duration == null || this.duration.isEmpty() ? 0 : this.duration.getValue(); 1018 } 1019 1020 /** 1021 * @param value The duration of the recording in seconds - for audio and video. 1022 */ 1023 public Media setDuration(int value) { 1024 if (this.duration == null) 1025 this.duration = new UnsignedIntType(); 1026 this.duration.setValue(value); 1027 return this; 1028 } 1029 1030 /** 1031 * @return {@link #content} (The actual content of the media - inline or by direct reference to the media source file.) 1032 */ 1033 public Attachment getContent() { 1034 if (this.content == null) 1035 if (Configuration.errorOnAutoCreate()) 1036 throw new Error("Attempt to auto-create Media.content"); 1037 else if (Configuration.doAutoCreate()) 1038 this.content = new Attachment(); // cc 1039 return this.content; 1040 } 1041 1042 public boolean hasContent() { 1043 return this.content != null && !this.content.isEmpty(); 1044 } 1045 1046 /** 1047 * @param value {@link #content} (The actual content of the media - inline or by direct reference to the media source file.) 1048 */ 1049 public Media setContent(Attachment value) { 1050 this.content = value; 1051 return this; 1052 } 1053 1054 /** 1055 * @return {@link #note} (Comments made about the media by the performer, subject or other participants.) 1056 */ 1057 public List<Annotation> getNote() { 1058 if (this.note == null) 1059 this.note = new ArrayList<Annotation>(); 1060 return this.note; 1061 } 1062 1063 /** 1064 * @return Returns a reference to <code>this</code> for easy method chaining 1065 */ 1066 public Media setNote(List<Annotation> theNote) { 1067 this.note = theNote; 1068 return this; 1069 } 1070 1071 public boolean hasNote() { 1072 if (this.note == null) 1073 return false; 1074 for (Annotation item : this.note) 1075 if (!item.isEmpty()) 1076 return true; 1077 return false; 1078 } 1079 1080 public Annotation addNote() { //3 1081 Annotation t = new Annotation(); 1082 if (this.note == null) 1083 this.note = new ArrayList<Annotation>(); 1084 this.note.add(t); 1085 return t; 1086 } 1087 1088 public Media addNote(Annotation t) { //3 1089 if (t == null) 1090 return this; 1091 if (this.note == null) 1092 this.note = new ArrayList<Annotation>(); 1093 this.note.add(t); 1094 return this; 1095 } 1096 1097 /** 1098 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1099 */ 1100 public Annotation getNoteFirstRep() { 1101 if (getNote().isEmpty()) { 1102 addNote(); 1103 } 1104 return getNote().get(0); 1105 } 1106 1107 protected void listChildren(List<Property> children) { 1108 super.listChildren(children); 1109 children.add(new Property("identifier", "Identifier", "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1110 children.add(new Property("basedOn", "Reference(ProcedureRequest)", "A procedure that is fulfilled in whole or in part by the creation of this media.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1111 children.add(new Property("type", "code", "Whether the media is a photo (still image), an audio recording, or a video recording.", 0, 1, type)); 1112 children.add(new Property("subtype", "CodeableConcept", "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.", 0, 1, subtype)); 1113 children.add(new Property("view", "CodeableConcept", "The name of the imaging view e.g. Lateral or Antero-posterior (AP).", 0, 1, view)); 1114 children.add(new Property("subject", "Reference(Patient|Practitioner|Group|Device|Specimen)", "Who/What this Media is a record of.", 0, 1, subject)); 1115 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this media.", 0, 1, context)); 1116 children.add(new Property("occurrence[x]", "dateTime|Period", "The date and time(s) at which the media was collected.", 0, 1, occurrence)); 1117 children.add(new Property("operator", "Reference(Practitioner)", "The person who administered the collection of the image.", 0, 1, operator)); 1118 children.add(new Property("reasonCode", "CodeableConcept", "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1119 children.add(new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the media was collected (i.e. the target site).", 0, 1, bodySite)); 1120 children.add(new Property("device", "Reference(Device|DeviceMetric)", "The device used to collect the media.", 0, 1, device)); 1121 children.add(new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 1, height)); 1122 children.add(new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 1, width)); 1123 children.add(new Property("frames", "positiveInt", "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 0, 1, frames)); 1124 children.add(new Property("duration", "unsignedInt", "The duration of the recording in seconds - for audio and video.", 0, 1, duration)); 1125 children.add(new Property("content", "Attachment", "The actual content of the media - inline or by direct reference to the media source file.", 0, 1, content)); 1126 children.add(new Property("note", "Annotation", "Comments made about the media by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note)); 1127 } 1128 1129 @Override 1130 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1131 switch (_hash) { 1132 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifiers associated with the image - these may include identifiers for the image itself, identifiers for the context of its collection (e.g. series ids) and context ids such as accession numbers or other workflow identifiers.", 0, java.lang.Integer.MAX_VALUE, identifier); 1133 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(ProcedureRequest)", "A procedure that is fulfilled in whole or in part by the creation of this media.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1134 case 3575610: /*type*/ return new Property("type", "code", "Whether the media is a photo (still image), an audio recording, or a video recording.", 0, 1, type); 1135 case -1867567750: /*subtype*/ return new Property("subtype", "CodeableConcept", "Details of the type of the media - usually, how it was acquired (what type of device). If images sourced from a DICOM system, are wrapped in a Media resource, then this is the modality.", 0, 1, subtype); 1136 case 3619493: /*view*/ return new Property("view", "CodeableConcept", "The name of the imaging view e.g. Lateral or Antero-posterior (AP).", 0, 1, view); 1137 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Practitioner|Group|Device|Specimen)", "Who/What this Media is a record of.", 0, 1, subject); 1138 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this media.", 0, 1, context); 1139 case -2022646513: /*occurrence[x]*/ return new Property("occurrence[x]", "dateTime|Period", "The date and time(s) at which the media was collected.", 0, 1, occurrence); 1140 case 1687874001: /*occurrence*/ return new Property("occurrence[x]", "dateTime|Period", "The date and time(s) at which the media was collected.", 0, 1, occurrence); 1141 case -298443636: /*occurrenceDateTime*/ return new Property("occurrence[x]", "dateTime|Period", "The date and time(s) at which the media was collected.", 0, 1, occurrence); 1142 case 1397156594: /*occurrencePeriod*/ return new Property("occurrence[x]", "dateTime|Period", "The date and time(s) at which the media was collected.", 0, 1, occurrence); 1143 case -500553564: /*operator*/ return new Property("operator", "Reference(Practitioner)", "The person who administered the collection of the image.", 0, 1, operator); 1144 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "Describes why the event occurred in coded or textual form.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1145 case 1702620169: /*bodySite*/ return new Property("bodySite", "CodeableConcept", "Indicates the site on the subject's body where the media was collected (i.e. the target site).", 0, 1, bodySite); 1146 case -1335157162: /*device*/ return new Property("device", "Reference(Device|DeviceMetric)", "The device used to collect the media.", 0, 1, device); 1147 case -1221029593: /*height*/ return new Property("height", "positiveInt", "Height of the image in pixels (photo/video).", 0, 1, height); 1148 case 113126854: /*width*/ return new Property("width", "positiveInt", "Width of the image in pixels (photo/video).", 0, 1, width); 1149 case -1266514778: /*frames*/ return new Property("frames", "positiveInt", "The number of frames in a photo. This is used with a multi-page fax, or an imaging acquisition context that takes multiple slices in a single image, or an animated gif. If there is more than one frame, this SHALL have a value in order to alert interface software that a multi-frame capable rendering widget is required.", 0, 1, frames); 1150 case -1992012396: /*duration*/ return new Property("duration", "unsignedInt", "The duration of the recording in seconds - for audio and video.", 0, 1, duration); 1151 case 951530617: /*content*/ return new Property("content", "Attachment", "The actual content of the media - inline or by direct reference to the media source file.", 0, 1, content); 1152 case 3387378: /*note*/ return new Property("note", "Annotation", "Comments made about the media by the performer, subject or other participants.", 0, java.lang.Integer.MAX_VALUE, note); 1153 default: return super.getNamedProperty(_hash, _name, _checkValid); 1154 } 1155 1156 } 1157 1158 @Override 1159 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1160 switch (hash) { 1161 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1162 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1163 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // Enumeration<DigitalMediaType> 1164 case -1867567750: /*subtype*/ return this.subtype == null ? new Base[0] : new Base[] {this.subtype}; // CodeableConcept 1165 case 3619493: /*view*/ return this.view == null ? new Base[0] : new Base[] {this.view}; // CodeableConcept 1166 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1167 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1168 case 1687874001: /*occurrence*/ return this.occurrence == null ? new Base[0] : new Base[] {this.occurrence}; // Type 1169 case -500553564: /*operator*/ return this.operator == null ? new Base[0] : new Base[] {this.operator}; // Reference 1170 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1171 case 1702620169: /*bodySite*/ return this.bodySite == null ? new Base[0] : new Base[] {this.bodySite}; // CodeableConcept 1172 case -1335157162: /*device*/ return this.device == null ? new Base[0] : new Base[] {this.device}; // Reference 1173 case -1221029593: /*height*/ return this.height == null ? new Base[0] : new Base[] {this.height}; // PositiveIntType 1174 case 113126854: /*width*/ return this.width == null ? new Base[0] : new Base[] {this.width}; // PositiveIntType 1175 case -1266514778: /*frames*/ return this.frames == null ? new Base[0] : new Base[] {this.frames}; // PositiveIntType 1176 case -1992012396: /*duration*/ return this.duration == null ? new Base[0] : new Base[] {this.duration}; // UnsignedIntType 1177 case 951530617: /*content*/ return this.content == null ? new Base[0] : new Base[] {this.content}; // Attachment 1178 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1179 default: return super.getProperty(hash, name, checkValid); 1180 } 1181 1182 } 1183 1184 @Override 1185 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1186 switch (hash) { 1187 case -1618432855: // identifier 1188 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1189 return value; 1190 case -332612366: // basedOn 1191 this.getBasedOn().add(castToReference(value)); // Reference 1192 return value; 1193 case 3575610: // type 1194 value = new DigitalMediaTypeEnumFactory().fromType(castToCode(value)); 1195 this.type = (Enumeration) value; // Enumeration<DigitalMediaType> 1196 return value; 1197 case -1867567750: // subtype 1198 this.subtype = castToCodeableConcept(value); // CodeableConcept 1199 return value; 1200 case 3619493: // view 1201 this.view = castToCodeableConcept(value); // CodeableConcept 1202 return value; 1203 case -1867885268: // subject 1204 this.subject = castToReference(value); // Reference 1205 return value; 1206 case 951530927: // context 1207 this.context = castToReference(value); // Reference 1208 return value; 1209 case 1687874001: // occurrence 1210 this.occurrence = castToType(value); // Type 1211 return value; 1212 case -500553564: // operator 1213 this.operator = castToReference(value); // Reference 1214 return value; 1215 case 722137681: // reasonCode 1216 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1217 return value; 1218 case 1702620169: // bodySite 1219 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1220 return value; 1221 case -1335157162: // device 1222 this.device = castToReference(value); // Reference 1223 return value; 1224 case -1221029593: // height 1225 this.height = castToPositiveInt(value); // PositiveIntType 1226 return value; 1227 case 113126854: // width 1228 this.width = castToPositiveInt(value); // PositiveIntType 1229 return value; 1230 case -1266514778: // frames 1231 this.frames = castToPositiveInt(value); // PositiveIntType 1232 return value; 1233 case -1992012396: // duration 1234 this.duration = castToUnsignedInt(value); // UnsignedIntType 1235 return value; 1236 case 951530617: // content 1237 this.content = castToAttachment(value); // Attachment 1238 return value; 1239 case 3387378: // note 1240 this.getNote().add(castToAnnotation(value)); // Annotation 1241 return value; 1242 default: return super.setProperty(hash, name, value); 1243 } 1244 1245 } 1246 1247 @Override 1248 public Base setProperty(String name, Base value) throws FHIRException { 1249 if (name.equals("identifier")) { 1250 this.getIdentifier().add(castToIdentifier(value)); 1251 } else if (name.equals("basedOn")) { 1252 this.getBasedOn().add(castToReference(value)); 1253 } else if (name.equals("type")) { 1254 value = new DigitalMediaTypeEnumFactory().fromType(castToCode(value)); 1255 this.type = (Enumeration) value; // Enumeration<DigitalMediaType> 1256 } else if (name.equals("subtype")) { 1257 this.subtype = castToCodeableConcept(value); // CodeableConcept 1258 } else if (name.equals("view")) { 1259 this.view = castToCodeableConcept(value); // CodeableConcept 1260 } else if (name.equals("subject")) { 1261 this.subject = castToReference(value); // Reference 1262 } else if (name.equals("context")) { 1263 this.context = castToReference(value); // Reference 1264 } else if (name.equals("occurrence[x]")) { 1265 this.occurrence = castToType(value); // Type 1266 } else if (name.equals("operator")) { 1267 this.operator = castToReference(value); // Reference 1268 } else if (name.equals("reasonCode")) { 1269 this.getReasonCode().add(castToCodeableConcept(value)); 1270 } else if (name.equals("bodySite")) { 1271 this.bodySite = castToCodeableConcept(value); // CodeableConcept 1272 } else if (name.equals("device")) { 1273 this.device = castToReference(value); // Reference 1274 } else if (name.equals("height")) { 1275 this.height = castToPositiveInt(value); // PositiveIntType 1276 } else if (name.equals("width")) { 1277 this.width = castToPositiveInt(value); // PositiveIntType 1278 } else if (name.equals("frames")) { 1279 this.frames = castToPositiveInt(value); // PositiveIntType 1280 } else if (name.equals("duration")) { 1281 this.duration = castToUnsignedInt(value); // UnsignedIntType 1282 } else if (name.equals("content")) { 1283 this.content = castToAttachment(value); // Attachment 1284 } else if (name.equals("note")) { 1285 this.getNote().add(castToAnnotation(value)); 1286 } else 1287 return super.setProperty(name, value); 1288 return value; 1289 } 1290 1291 @Override 1292 public Base makeProperty(int hash, String name) throws FHIRException { 1293 switch (hash) { 1294 case -1618432855: return addIdentifier(); 1295 case -332612366: return addBasedOn(); 1296 case 3575610: return getTypeElement(); 1297 case -1867567750: return getSubtype(); 1298 case 3619493: return getView(); 1299 case -1867885268: return getSubject(); 1300 case 951530927: return getContext(); 1301 case -2022646513: return getOccurrence(); 1302 case 1687874001: return getOccurrence(); 1303 case -500553564: return getOperator(); 1304 case 722137681: return addReasonCode(); 1305 case 1702620169: return getBodySite(); 1306 case -1335157162: return getDevice(); 1307 case -1221029593: return getHeightElement(); 1308 case 113126854: return getWidthElement(); 1309 case -1266514778: return getFramesElement(); 1310 case -1992012396: return getDurationElement(); 1311 case 951530617: return getContent(); 1312 case 3387378: return addNote(); 1313 default: return super.makeProperty(hash, name); 1314 } 1315 1316 } 1317 1318 @Override 1319 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1320 switch (hash) { 1321 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1322 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1323 case 3575610: /*type*/ return new String[] {"code"}; 1324 case -1867567750: /*subtype*/ return new String[] {"CodeableConcept"}; 1325 case 3619493: /*view*/ return new String[] {"CodeableConcept"}; 1326 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1327 case 951530927: /*context*/ return new String[] {"Reference"}; 1328 case 1687874001: /*occurrence*/ return new String[] {"dateTime", "Period"}; 1329 case -500553564: /*operator*/ return new String[] {"Reference"}; 1330 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 1331 case 1702620169: /*bodySite*/ return new String[] {"CodeableConcept"}; 1332 case -1335157162: /*device*/ return new String[] {"Reference"}; 1333 case -1221029593: /*height*/ return new String[] {"positiveInt"}; 1334 case 113126854: /*width*/ return new String[] {"positiveInt"}; 1335 case -1266514778: /*frames*/ return new String[] {"positiveInt"}; 1336 case -1992012396: /*duration*/ return new String[] {"unsignedInt"}; 1337 case 951530617: /*content*/ return new String[] {"Attachment"}; 1338 case 3387378: /*note*/ return new String[] {"Annotation"}; 1339 default: return super.getTypesForProperty(hash, name); 1340 } 1341 1342 } 1343 1344 @Override 1345 public Base addChild(String name) throws FHIRException { 1346 if (name.equals("identifier")) { 1347 return addIdentifier(); 1348 } 1349 else if (name.equals("basedOn")) { 1350 return addBasedOn(); 1351 } 1352 else if (name.equals("type")) { 1353 throw new FHIRException("Cannot call addChild on a singleton property Media.type"); 1354 } 1355 else if (name.equals("subtype")) { 1356 this.subtype = new CodeableConcept(); 1357 return this.subtype; 1358 } 1359 else if (name.equals("view")) { 1360 this.view = new CodeableConcept(); 1361 return this.view; 1362 } 1363 else if (name.equals("subject")) { 1364 this.subject = new Reference(); 1365 return this.subject; 1366 } 1367 else if (name.equals("context")) { 1368 this.context = new Reference(); 1369 return this.context; 1370 } 1371 else if (name.equals("occurrenceDateTime")) { 1372 this.occurrence = new DateTimeType(); 1373 return this.occurrence; 1374 } 1375 else if (name.equals("occurrencePeriod")) { 1376 this.occurrence = new Period(); 1377 return this.occurrence; 1378 } 1379 else if (name.equals("operator")) { 1380 this.operator = new Reference(); 1381 return this.operator; 1382 } 1383 else if (name.equals("reasonCode")) { 1384 return addReasonCode(); 1385 } 1386 else if (name.equals("bodySite")) { 1387 this.bodySite = new CodeableConcept(); 1388 return this.bodySite; 1389 } 1390 else if (name.equals("device")) { 1391 this.device = new Reference(); 1392 return this.device; 1393 } 1394 else if (name.equals("height")) { 1395 throw new FHIRException("Cannot call addChild on a singleton property Media.height"); 1396 } 1397 else if (name.equals("width")) { 1398 throw new FHIRException("Cannot call addChild on a singleton property Media.width"); 1399 } 1400 else if (name.equals("frames")) { 1401 throw new FHIRException("Cannot call addChild on a singleton property Media.frames"); 1402 } 1403 else if (name.equals("duration")) { 1404 throw new FHIRException("Cannot call addChild on a singleton property Media.duration"); 1405 } 1406 else if (name.equals("content")) { 1407 this.content = new Attachment(); 1408 return this.content; 1409 } 1410 else if (name.equals("note")) { 1411 return addNote(); 1412 } 1413 else 1414 return super.addChild(name); 1415 } 1416 1417 public String fhirType() { 1418 return "Media"; 1419 1420 } 1421 1422 public Media copy() { 1423 Media dst = new Media(); 1424 copyValues(dst); 1425 if (identifier != null) { 1426 dst.identifier = new ArrayList<Identifier>(); 1427 for (Identifier i : identifier) 1428 dst.identifier.add(i.copy()); 1429 }; 1430 if (basedOn != null) { 1431 dst.basedOn = new ArrayList<Reference>(); 1432 for (Reference i : basedOn) 1433 dst.basedOn.add(i.copy()); 1434 }; 1435 dst.type = type == null ? null : type.copy(); 1436 dst.subtype = subtype == null ? null : subtype.copy(); 1437 dst.view = view == null ? null : view.copy(); 1438 dst.subject = subject == null ? null : subject.copy(); 1439 dst.context = context == null ? null : context.copy(); 1440 dst.occurrence = occurrence == null ? null : occurrence.copy(); 1441 dst.operator = operator == null ? null : operator.copy(); 1442 if (reasonCode != null) { 1443 dst.reasonCode = new ArrayList<CodeableConcept>(); 1444 for (CodeableConcept i : reasonCode) 1445 dst.reasonCode.add(i.copy()); 1446 }; 1447 dst.bodySite = bodySite == null ? null : bodySite.copy(); 1448 dst.device = device == null ? null : device.copy(); 1449 dst.height = height == null ? null : height.copy(); 1450 dst.width = width == null ? null : width.copy(); 1451 dst.frames = frames == null ? null : frames.copy(); 1452 dst.duration = duration == null ? null : duration.copy(); 1453 dst.content = content == null ? null : content.copy(); 1454 if (note != null) { 1455 dst.note = new ArrayList<Annotation>(); 1456 for (Annotation i : note) 1457 dst.note.add(i.copy()); 1458 }; 1459 return dst; 1460 } 1461 1462 protected Media typedCopy() { 1463 return copy(); 1464 } 1465 1466 @Override 1467 public boolean equalsDeep(Base other_) { 1468 if (!super.equalsDeep(other_)) 1469 return false; 1470 if (!(other_ instanceof Media)) 1471 return false; 1472 Media o = (Media) other_; 1473 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(type, o.type, true) 1474 && compareDeep(subtype, o.subtype, true) && compareDeep(view, o.view, true) && compareDeep(subject, o.subject, true) 1475 && compareDeep(context, o.context, true) && compareDeep(occurrence, o.occurrence, true) && compareDeep(operator, o.operator, true) 1476 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(bodySite, o.bodySite, true) && compareDeep(device, o.device, true) 1477 && compareDeep(height, o.height, true) && compareDeep(width, o.width, true) && compareDeep(frames, o.frames, true) 1478 && compareDeep(duration, o.duration, true) && compareDeep(content, o.content, true) && compareDeep(note, o.note, true) 1479 ; 1480 } 1481 1482 @Override 1483 public boolean equalsShallow(Base other_) { 1484 if (!super.equalsShallow(other_)) 1485 return false; 1486 if (!(other_ instanceof Media)) 1487 return false; 1488 Media o = (Media) other_; 1489 return compareValues(type, o.type, true) && compareValues(height, o.height, true) && compareValues(width, o.width, true) 1490 && compareValues(frames, o.frames, true) && compareValues(duration, o.duration, true); 1491 } 1492 1493 public boolean isEmpty() { 1494 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, type 1495 , subtype, view, subject, context, occurrence, operator, reasonCode, bodySite 1496 , device, height, width, frames, duration, content, note); 1497 } 1498 1499 @Override 1500 public ResourceType getResourceType() { 1501 return ResourceType.Media; 1502 } 1503 1504 /** 1505 * Search parameter: <b>date</b> 1506 * <p> 1507 * Description: <b>When Media was collected</b><br> 1508 * Type: <b>date</b><br> 1509 * Path: <b>Media.occurrence[x]</b><br> 1510 * </p> 1511 */ 1512 @SearchParamDefinition(name="date", path="Media.occurrence", description="When Media was collected", type="date" ) 1513 public static final String SP_DATE = "date"; 1514 /** 1515 * <b>Fluent Client</b> search parameter constant for <b>date</b> 1516 * <p> 1517 * Description: <b>When Media was collected</b><br> 1518 * Type: <b>date</b><br> 1519 * Path: <b>Media.occurrence[x]</b><br> 1520 * </p> 1521 */ 1522 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 1523 1524 /** 1525 * Search parameter: <b>identifier</b> 1526 * <p> 1527 * Description: <b>Identifier(s) for the image</b><br> 1528 * Type: <b>token</b><br> 1529 * Path: <b>Media.identifier</b><br> 1530 * </p> 1531 */ 1532 @SearchParamDefinition(name="identifier", path="Media.identifier", description="Identifier(s) for the image", type="token" ) 1533 public static final String SP_IDENTIFIER = "identifier"; 1534 /** 1535 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1536 * <p> 1537 * Description: <b>Identifier(s) for the image</b><br> 1538 * Type: <b>token</b><br> 1539 * Path: <b>Media.identifier</b><br> 1540 * </p> 1541 */ 1542 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1543 1544 /** 1545 * Search parameter: <b>created</b> 1546 * <p> 1547 * Description: <b>Date attachment was first created</b><br> 1548 * Type: <b>date</b><br> 1549 * Path: <b>Media.content.creation</b><br> 1550 * </p> 1551 */ 1552 @SearchParamDefinition(name="created", path="Media.content.creation", description="Date attachment was first created", type="date" ) 1553 public static final String SP_CREATED = "created"; 1554 /** 1555 * <b>Fluent Client</b> search parameter constant for <b>created</b> 1556 * <p> 1557 * Description: <b>Date attachment was first created</b><br> 1558 * Type: <b>date</b><br> 1559 * Path: <b>Media.content.creation</b><br> 1560 * </p> 1561 */ 1562 public static final ca.uhn.fhir.rest.gclient.DateClientParam CREATED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_CREATED); 1563 1564 /** 1565 * Search parameter: <b>subject</b> 1566 * <p> 1567 * Description: <b>Who/What this Media is a record of</b><br> 1568 * Type: <b>reference</b><br> 1569 * Path: <b>Media.subject</b><br> 1570 * </p> 1571 */ 1572 @SearchParamDefinition(name="subject", path="Media.subject", description="Who/What this Media is a record of", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Group.class, Patient.class, Practitioner.class, Specimen.class } ) 1573 public static final String SP_SUBJECT = "subject"; 1574 /** 1575 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1576 * <p> 1577 * Description: <b>Who/What this Media is a record of</b><br> 1578 * Type: <b>reference</b><br> 1579 * Path: <b>Media.subject</b><br> 1580 * </p> 1581 */ 1582 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1583 1584/** 1585 * Constant for fluent queries to be used to add include statements. Specifies 1586 * the path value of "<b>Media:subject</b>". 1587 */ 1588 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("Media:subject").toLocked(); 1589 1590 /** 1591 * Search parameter: <b>type</b> 1592 * <p> 1593 * Description: <b>photo | video | audio</b><br> 1594 * Type: <b>token</b><br> 1595 * Path: <b>Media.type</b><br> 1596 * </p> 1597 */ 1598 @SearchParamDefinition(name="type", path="Media.type", description="photo | video | audio", type="token" ) 1599 public static final String SP_TYPE = "type"; 1600 /** 1601 * <b>Fluent Client</b> search parameter constant for <b>type</b> 1602 * <p> 1603 * Description: <b>photo | video | audio</b><br> 1604 * Type: <b>token</b><br> 1605 * Path: <b>Media.type</b><br> 1606 * </p> 1607 */ 1608 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 1609 1610 /** 1611 * Search parameter: <b>operator</b> 1612 * <p> 1613 * Description: <b>The person who generated the image</b><br> 1614 * Type: <b>reference</b><br> 1615 * Path: <b>Media.operator</b><br> 1616 * </p> 1617 */ 1618 @SearchParamDefinition(name="operator", path="Media.operator", description="The person who generated the image", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Practitioner.class } ) 1619 public static final String SP_OPERATOR = "operator"; 1620 /** 1621 * <b>Fluent Client</b> search parameter constant for <b>operator</b> 1622 * <p> 1623 * Description: <b>The person who generated the image</b><br> 1624 * Type: <b>reference</b><br> 1625 * Path: <b>Media.operator</b><br> 1626 * </p> 1627 */ 1628 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam OPERATOR = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_OPERATOR); 1629 1630/** 1631 * Constant for fluent queries to be used to add include statements. Specifies 1632 * the path value of "<b>Media:operator</b>". 1633 */ 1634 public static final ca.uhn.fhir.model.api.Include INCLUDE_OPERATOR = new ca.uhn.fhir.model.api.Include("Media:operator").toLocked(); 1635 1636 /** 1637 * Search parameter: <b>view</b> 1638 * <p> 1639 * Description: <b>Imaging view, e.g. Lateral or Antero-posterior</b><br> 1640 * Type: <b>token</b><br> 1641 * Path: <b>Media.view</b><br> 1642 * </p> 1643 */ 1644 @SearchParamDefinition(name="view", path="Media.view", description="Imaging view, e.g. Lateral or Antero-posterior", type="token" ) 1645 public static final String SP_VIEW = "view"; 1646 /** 1647 * <b>Fluent Client</b> search parameter constant for <b>view</b> 1648 * <p> 1649 * Description: <b>Imaging view, e.g. Lateral or Antero-posterior</b><br> 1650 * Type: <b>token</b><br> 1651 * Path: <b>Media.view</b><br> 1652 * </p> 1653 */ 1654 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VIEW = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VIEW); 1655 1656 /** 1657 * Search parameter: <b>site</b> 1658 * <p> 1659 * Description: <b>Body part in media</b><br> 1660 * Type: <b>token</b><br> 1661 * Path: <b>Media.bodySite</b><br> 1662 * </p> 1663 */ 1664 @SearchParamDefinition(name="site", path="Media.bodySite", description="Body part in media", type="token" ) 1665 public static final String SP_SITE = "site"; 1666 /** 1667 * <b>Fluent Client</b> search parameter constant for <b>site</b> 1668 * <p> 1669 * Description: <b>Body part in media</b><br> 1670 * Type: <b>token</b><br> 1671 * Path: <b>Media.bodySite</b><br> 1672 * </p> 1673 */ 1674 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SITE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SITE); 1675 1676 /** 1677 * Search parameter: <b>based-on</b> 1678 * <p> 1679 * Description: <b>Procedure that caused this media to be created</b><br> 1680 * Type: <b>reference</b><br> 1681 * Path: <b>Media.basedOn</b><br> 1682 * </p> 1683 */ 1684 @SearchParamDefinition(name="based-on", path="Media.basedOn", description="Procedure that caused this media to be created", type="reference", target={ProcedureRequest.class } ) 1685 public static final String SP_BASED_ON = "based-on"; 1686 /** 1687 * <b>Fluent Client</b> search parameter constant for <b>based-on</b> 1688 * <p> 1689 * Description: <b>Procedure that caused this media to be created</b><br> 1690 * Type: <b>reference</b><br> 1691 * Path: <b>Media.basedOn</b><br> 1692 * </p> 1693 */ 1694 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam BASED_ON = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_BASED_ON); 1695 1696/** 1697 * Constant for fluent queries to be used to add include statements. Specifies 1698 * the path value of "<b>Media:based-on</b>". 1699 */ 1700 public static final ca.uhn.fhir.model.api.Include INCLUDE_BASED_ON = new ca.uhn.fhir.model.api.Include("Media:based-on").toLocked(); 1701 1702 /** 1703 * Search parameter: <b>subtype</b> 1704 * <p> 1705 * Description: <b>The type of acquisition equipment/process</b><br> 1706 * Type: <b>token</b><br> 1707 * Path: <b>Media.subtype</b><br> 1708 * </p> 1709 */ 1710 @SearchParamDefinition(name="subtype", path="Media.subtype", description="The type of acquisition equipment/process", type="token" ) 1711 public static final String SP_SUBTYPE = "subtype"; 1712 /** 1713 * <b>Fluent Client</b> search parameter constant for <b>subtype</b> 1714 * <p> 1715 * Description: <b>The type of acquisition equipment/process</b><br> 1716 * Type: <b>token</b><br> 1717 * Path: <b>Media.subtype</b><br> 1718 * </p> 1719 */ 1720 public static final ca.uhn.fhir.rest.gclient.TokenClientParam SUBTYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_SUBTYPE); 1721 1722 /** 1723 * Search parameter: <b>patient</b> 1724 * <p> 1725 * Description: <b>Who/What this Media is a record of</b><br> 1726 * Type: <b>reference</b><br> 1727 * Path: <b>Media.subject</b><br> 1728 * </p> 1729 */ 1730 @SearchParamDefinition(name="patient", path="Media.subject", description="Who/What this Media is a record of", type="reference", target={Patient.class } ) 1731 public static final String SP_PATIENT = "patient"; 1732 /** 1733 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1734 * <p> 1735 * Description: <b>Who/What this Media is a record of</b><br> 1736 * Type: <b>reference</b><br> 1737 * Path: <b>Media.subject</b><br> 1738 * </p> 1739 */ 1740 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1741 1742/** 1743 * Constant for fluent queries to be used to add include statements. Specifies 1744 * the path value of "<b>Media:patient</b>". 1745 */ 1746 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("Media:patient").toLocked(); 1747 1748 /** 1749 * Search parameter: <b>context</b> 1750 * <p> 1751 * Description: <b>Encounter / Episode associated with media</b><br> 1752 * Type: <b>reference</b><br> 1753 * Path: <b>Media.context</b><br> 1754 * </p> 1755 */ 1756 @SearchParamDefinition(name="context", path="Media.context", description="Encounter / Episode associated with media", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 1757 public static final String SP_CONTEXT = "context"; 1758 /** 1759 * <b>Fluent Client</b> search parameter constant for <b>context</b> 1760 * <p> 1761 * Description: <b>Encounter / Episode associated with media</b><br> 1762 * Type: <b>reference</b><br> 1763 * Path: <b>Media.context</b><br> 1764 * </p> 1765 */ 1766 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 1767 1768/** 1769 * Constant for fluent queries to be used to add include statements. Specifies 1770 * the path value of "<b>Media:context</b>". 1771 */ 1772 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("Media:context").toLocked(); 1773 1774 /** 1775 * Search parameter: <b>device</b> 1776 * <p> 1777 * Description: <b>Observing Device</b><br> 1778 * Type: <b>reference</b><br> 1779 * Path: <b>Media.device</b><br> 1780 * </p> 1781 */ 1782 @SearchParamDefinition(name="device", path="Media.device", description="Observing Device", type="reference", target={Device.class, DeviceMetric.class } ) 1783 public static final String SP_DEVICE = "device"; 1784 /** 1785 * <b>Fluent Client</b> search parameter constant for <b>device</b> 1786 * <p> 1787 * Description: <b>Observing Device</b><br> 1788 * Type: <b>reference</b><br> 1789 * Path: <b>Media.device</b><br> 1790 * </p> 1791 */ 1792 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 1793 1794/** 1795 * Constant for fluent queries to be used to add include statements. Specifies 1796 * the path value of "<b>Media:device</b>". 1797 */ 1798 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("Media:device").toLocked(); 1799 1800 1801}