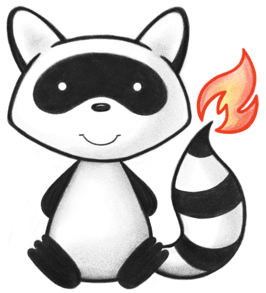
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * This resource is primarily used for the identification and definition of a medication. It covers the ingredients and the packaging for a medication. 051 */ 052@ResourceDef(name="Medication", profile="http://hl7.org/fhir/Profile/Medication") 053public class Medication extends DomainResource { 054 055 public enum MedicationStatus { 056 /** 057 * The medication is available for use 058 */ 059 ACTIVE, 060 /** 061 * The medication is not available for use 062 */ 063 INACTIVE, 064 /** 065 * The medication was entered in error 066 */ 067 ENTEREDINERROR, 068 /** 069 * added to help the parsers with the generic types 070 */ 071 NULL; 072 public static MedicationStatus fromCode(String codeString) throws FHIRException { 073 if (codeString == null || "".equals(codeString)) 074 return null; 075 if ("active".equals(codeString)) 076 return ACTIVE; 077 if ("inactive".equals(codeString)) 078 return INACTIVE; 079 if ("entered-in-error".equals(codeString)) 080 return ENTEREDINERROR; 081 if (Configuration.isAcceptInvalidEnums()) 082 return null; 083 else 084 throw new FHIRException("Unknown MedicationStatus code '"+codeString+"'"); 085 } 086 public String toCode() { 087 switch (this) { 088 case ACTIVE: return "active"; 089 case INACTIVE: return "inactive"; 090 case ENTEREDINERROR: return "entered-in-error"; 091 case NULL: return null; 092 default: return "?"; 093 } 094 } 095 public String getSystem() { 096 switch (this) { 097 case ACTIVE: return "http://hl7.org/fhir/medication-status"; 098 case INACTIVE: return "http://hl7.org/fhir/medication-status"; 099 case ENTEREDINERROR: return "http://hl7.org/fhir/medication-status"; 100 case NULL: return null; 101 default: return "?"; 102 } 103 } 104 public String getDefinition() { 105 switch (this) { 106 case ACTIVE: return "The medication is available for use"; 107 case INACTIVE: return "The medication is not available for use"; 108 case ENTEREDINERROR: return "The medication was entered in error"; 109 case NULL: return null; 110 default: return "?"; 111 } 112 } 113 public String getDisplay() { 114 switch (this) { 115 case ACTIVE: return "Active"; 116 case INACTIVE: return "Inactive"; 117 case ENTEREDINERROR: return "Entered in Error"; 118 case NULL: return null; 119 default: return "?"; 120 } 121 } 122 } 123 124 public static class MedicationStatusEnumFactory implements EnumFactory<MedicationStatus> { 125 public MedicationStatus fromCode(String codeString) throws IllegalArgumentException { 126 if (codeString == null || "".equals(codeString)) 127 if (codeString == null || "".equals(codeString)) 128 return null; 129 if ("active".equals(codeString)) 130 return MedicationStatus.ACTIVE; 131 if ("inactive".equals(codeString)) 132 return MedicationStatus.INACTIVE; 133 if ("entered-in-error".equals(codeString)) 134 return MedicationStatus.ENTEREDINERROR; 135 throw new IllegalArgumentException("Unknown MedicationStatus code '"+codeString+"'"); 136 } 137 public Enumeration<MedicationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 138 if (code == null) 139 return null; 140 if (code.isEmpty()) 141 return new Enumeration<MedicationStatus>(this); 142 String codeString = code.asStringValue(); 143 if (codeString == null || "".equals(codeString)) 144 return null; 145 if ("active".equals(codeString)) 146 return new Enumeration<MedicationStatus>(this, MedicationStatus.ACTIVE); 147 if ("inactive".equals(codeString)) 148 return new Enumeration<MedicationStatus>(this, MedicationStatus.INACTIVE); 149 if ("entered-in-error".equals(codeString)) 150 return new Enumeration<MedicationStatus>(this, MedicationStatus.ENTEREDINERROR); 151 throw new FHIRException("Unknown MedicationStatus code '"+codeString+"'"); 152 } 153 public String toCode(MedicationStatus code) { 154 if (code == MedicationStatus.NULL) 155 return null; 156 if (code == MedicationStatus.ACTIVE) 157 return "active"; 158 if (code == MedicationStatus.INACTIVE) 159 return "inactive"; 160 if (code == MedicationStatus.ENTEREDINERROR) 161 return "entered-in-error"; 162 return "?"; 163 } 164 public String toSystem(MedicationStatus code) { 165 return code.getSystem(); 166 } 167 } 168 169 @Block() 170 public static class MedicationIngredientComponent extends BackboneElement implements IBaseBackboneElement { 171 /** 172 * The actual ingredient - either a substance (simple ingredient) or another medication. 173 */ 174 @Child(name = "item", type = {CodeableConcept.class, Substance.class, Medication.class}, order=1, min=1, max=1, modifier=false, summary=false) 175 @Description(shortDefinition="The product contained", formalDefinition="The actual ingredient - either a substance (simple ingredient) or another medication." ) 176 protected Type item; 177 178 /** 179 * Indication of whether this ingredient affects the therapeutic action of the drug. 180 */ 181 @Child(name = "isActive", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=false) 182 @Description(shortDefinition="Active ingredient indicator", formalDefinition="Indication of whether this ingredient affects the therapeutic action of the drug." ) 183 protected BooleanType isActive; 184 185 /** 186 * Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet. 187 */ 188 @Child(name = "amount", type = {Ratio.class}, order=3, min=0, max=1, modifier=false, summary=false) 189 @Description(shortDefinition="Quantity of ingredient present", formalDefinition="Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet." ) 190 protected Ratio amount; 191 192 private static final long serialVersionUID = -1796655982L; 193 194 /** 195 * Constructor 196 */ 197 public MedicationIngredientComponent() { 198 super(); 199 } 200 201 /** 202 * Constructor 203 */ 204 public MedicationIngredientComponent(Type item) { 205 super(); 206 this.item = item; 207 } 208 209 /** 210 * @return {@link #item} (The actual ingredient - either a substance (simple ingredient) or another medication.) 211 */ 212 public Type getItem() { 213 return this.item; 214 } 215 216 /** 217 * @return {@link #item} (The actual ingredient - either a substance (simple ingredient) or another medication.) 218 */ 219 public CodeableConcept getItemCodeableConcept() throws FHIRException { 220 if (this.item == null) 221 return null; 222 if (!(this.item instanceof CodeableConcept)) 223 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 224 return (CodeableConcept) this.item; 225 } 226 227 public boolean hasItemCodeableConcept() { 228 return this != null && this.item instanceof CodeableConcept; 229 } 230 231 /** 232 * @return {@link #item} (The actual ingredient - either a substance (simple ingredient) or another medication.) 233 */ 234 public Reference getItemReference() throws FHIRException { 235 if (this.item == null) 236 return null; 237 if (!(this.item instanceof Reference)) 238 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 239 return (Reference) this.item; 240 } 241 242 public boolean hasItemReference() { 243 return this != null && this.item instanceof Reference; 244 } 245 246 public boolean hasItem() { 247 return this.item != null && !this.item.isEmpty(); 248 } 249 250 /** 251 * @param value {@link #item} (The actual ingredient - either a substance (simple ingredient) or another medication.) 252 */ 253 public MedicationIngredientComponent setItem(Type value) throws FHIRFormatError { 254 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 255 throw new FHIRFormatError("Not the right type for Medication.ingredient.item[x]: "+value.fhirType()); 256 this.item = value; 257 return this; 258 } 259 260 /** 261 * @return {@link #isActive} (Indication of whether this ingredient affects the therapeutic action of the drug.). This is the underlying object with id, value and extensions. The accessor "getIsActive" gives direct access to the value 262 */ 263 public BooleanType getIsActiveElement() { 264 if (this.isActive == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create MedicationIngredientComponent.isActive"); 267 else if (Configuration.doAutoCreate()) 268 this.isActive = new BooleanType(); // bb 269 return this.isActive; 270 } 271 272 public boolean hasIsActiveElement() { 273 return this.isActive != null && !this.isActive.isEmpty(); 274 } 275 276 public boolean hasIsActive() { 277 return this.isActive != null && !this.isActive.isEmpty(); 278 } 279 280 /** 281 * @param value {@link #isActive} (Indication of whether this ingredient affects the therapeutic action of the drug.). This is the underlying object with id, value and extensions. The accessor "getIsActive" gives direct access to the value 282 */ 283 public MedicationIngredientComponent setIsActiveElement(BooleanType value) { 284 this.isActive = value; 285 return this; 286 } 287 288 /** 289 * @return Indication of whether this ingredient affects the therapeutic action of the drug. 290 */ 291 public boolean getIsActive() { 292 return this.isActive == null || this.isActive.isEmpty() ? false : this.isActive.getValue(); 293 } 294 295 /** 296 * @param value Indication of whether this ingredient affects the therapeutic action of the drug. 297 */ 298 public MedicationIngredientComponent setIsActive(boolean value) { 299 if (this.isActive == null) 300 this.isActive = new BooleanType(); 301 this.isActive.setValue(value); 302 return this; 303 } 304 305 /** 306 * @return {@link #amount} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.) 307 */ 308 public Ratio getAmount() { 309 if (this.amount == null) 310 if (Configuration.errorOnAutoCreate()) 311 throw new Error("Attempt to auto-create MedicationIngredientComponent.amount"); 312 else if (Configuration.doAutoCreate()) 313 this.amount = new Ratio(); // cc 314 return this.amount; 315 } 316 317 public boolean hasAmount() { 318 return this.amount != null && !this.amount.isEmpty(); 319 } 320 321 /** 322 * @param value {@link #amount} (Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.) 323 */ 324 public MedicationIngredientComponent setAmount(Ratio value) { 325 this.amount = value; 326 return this; 327 } 328 329 protected void listChildren(List<Property> children) { 330 super.listChildren(children); 331 children.add(new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item)); 332 children.add(new Property("isActive", "boolean", "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive)); 333 children.add(new Property("amount", "Ratio", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.", 0, 1, amount)); 334 } 335 336 @Override 337 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 338 switch (_hash) { 339 case 2116201613: /*item[x]*/ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item); 340 case 3242771: /*item*/ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item); 341 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item); 342 case 1376364920: /*itemReference*/ return new Property("item[x]", "CodeableConcept|Reference(Substance|Medication)", "The actual ingredient - either a substance (simple ingredient) or another medication.", 0, 1, item); 343 case -748916528: /*isActive*/ return new Property("isActive", "boolean", "Indication of whether this ingredient affects the therapeutic action of the drug.", 0, 1, isActive); 344 case -1413853096: /*amount*/ return new Property("amount", "Ratio", "Specifies how many (or how much) of the items there are in this Medication. For example, 250 mg per tablet. This is expressed as a ratio where the numerator is 250mg and the denominator is 1 tablet.", 0, 1, amount); 345 default: return super.getNamedProperty(_hash, _name, _checkValid); 346 } 347 348 } 349 350 @Override 351 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 352 switch (hash) { 353 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // Type 354 case -748916528: /*isActive*/ return this.isActive == null ? new Base[0] : new Base[] {this.isActive}; // BooleanType 355 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // Ratio 356 default: return super.getProperty(hash, name, checkValid); 357 } 358 359 } 360 361 @Override 362 public Base setProperty(int hash, String name, Base value) throws FHIRException { 363 switch (hash) { 364 case 3242771: // item 365 this.item = castToType(value); // Type 366 return value; 367 case -748916528: // isActive 368 this.isActive = castToBoolean(value); // BooleanType 369 return value; 370 case -1413853096: // amount 371 this.amount = castToRatio(value); // Ratio 372 return value; 373 default: return super.setProperty(hash, name, value); 374 } 375 376 } 377 378 @Override 379 public Base setProperty(String name, Base value) throws FHIRException { 380 if (name.equals("item[x]")) { 381 this.item = castToType(value); // Type 382 } else if (name.equals("isActive")) { 383 this.isActive = castToBoolean(value); // BooleanType 384 } else if (name.equals("amount")) { 385 this.amount = castToRatio(value); // Ratio 386 } else 387 return super.setProperty(name, value); 388 return value; 389 } 390 391 @Override 392 public Base makeProperty(int hash, String name) throws FHIRException { 393 switch (hash) { 394 case 2116201613: return getItem(); 395 case 3242771: return getItem(); 396 case -748916528: return getIsActiveElement(); 397 case -1413853096: return getAmount(); 398 default: return super.makeProperty(hash, name); 399 } 400 401 } 402 403 @Override 404 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 405 switch (hash) { 406 case 3242771: /*item*/ return new String[] {"CodeableConcept", "Reference"}; 407 case -748916528: /*isActive*/ return new String[] {"boolean"}; 408 case -1413853096: /*amount*/ return new String[] {"Ratio"}; 409 default: return super.getTypesForProperty(hash, name); 410 } 411 412 } 413 414 @Override 415 public Base addChild(String name) throws FHIRException { 416 if (name.equals("itemCodeableConcept")) { 417 this.item = new CodeableConcept(); 418 return this.item; 419 } 420 else if (name.equals("itemReference")) { 421 this.item = new Reference(); 422 return this.item; 423 } 424 else if (name.equals("isActive")) { 425 throw new FHIRException("Cannot call addChild on a singleton property Medication.isActive"); 426 } 427 else if (name.equals("amount")) { 428 this.amount = new Ratio(); 429 return this.amount; 430 } 431 else 432 return super.addChild(name); 433 } 434 435 public MedicationIngredientComponent copy() { 436 MedicationIngredientComponent dst = new MedicationIngredientComponent(); 437 copyValues(dst); 438 dst.item = item == null ? null : item.copy(); 439 dst.isActive = isActive == null ? null : isActive.copy(); 440 dst.amount = amount == null ? null : amount.copy(); 441 return dst; 442 } 443 444 @Override 445 public boolean equalsDeep(Base other_) { 446 if (!super.equalsDeep(other_)) 447 return false; 448 if (!(other_ instanceof MedicationIngredientComponent)) 449 return false; 450 MedicationIngredientComponent o = (MedicationIngredientComponent) other_; 451 return compareDeep(item, o.item, true) && compareDeep(isActive, o.isActive, true) && compareDeep(amount, o.amount, true) 452 ; 453 } 454 455 @Override 456 public boolean equalsShallow(Base other_) { 457 if (!super.equalsShallow(other_)) 458 return false; 459 if (!(other_ instanceof MedicationIngredientComponent)) 460 return false; 461 MedicationIngredientComponent o = (MedicationIngredientComponent) other_; 462 return compareValues(isActive, o.isActive, true); 463 } 464 465 public boolean isEmpty() { 466 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, isActive, amount); 467 } 468 469 public String fhirType() { 470 return "Medication.ingredient"; 471 472 } 473 474 } 475 476 @Block() 477 public static class MedicationPackageComponent extends BackboneElement implements IBaseBackboneElement { 478 /** 479 * The kind of container that this package comes as. 480 */ 481 @Child(name = "container", type = {CodeableConcept.class}, order=1, min=0, max=1, modifier=false, summary=false) 482 @Description(shortDefinition="E.g. box, vial, blister-pack", formalDefinition="The kind of container that this package comes as." ) 483 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-package-form") 484 protected CodeableConcept container; 485 486 /** 487 * A set of components that go to make up the described item. 488 */ 489 @Child(name = "content", type = {}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 490 @Description(shortDefinition="What is in the package", formalDefinition="A set of components that go to make up the described item." ) 491 protected List<MedicationPackageContentComponent> content; 492 493 /** 494 * Information about a group of medication produced or packaged from one production run. 495 */ 496 @Child(name = "batch", type = {}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 497 @Description(shortDefinition="Identifies a single production run", formalDefinition="Information about a group of medication produced or packaged from one production run." ) 498 protected List<MedicationPackageBatchComponent> batch; 499 500 private static final long serialVersionUID = -255992250L; 501 502 /** 503 * Constructor 504 */ 505 public MedicationPackageComponent() { 506 super(); 507 } 508 509 /** 510 * @return {@link #container} (The kind of container that this package comes as.) 511 */ 512 public CodeableConcept getContainer() { 513 if (this.container == null) 514 if (Configuration.errorOnAutoCreate()) 515 throw new Error("Attempt to auto-create MedicationPackageComponent.container"); 516 else if (Configuration.doAutoCreate()) 517 this.container = new CodeableConcept(); // cc 518 return this.container; 519 } 520 521 public boolean hasContainer() { 522 return this.container != null && !this.container.isEmpty(); 523 } 524 525 /** 526 * @param value {@link #container} (The kind of container that this package comes as.) 527 */ 528 public MedicationPackageComponent setContainer(CodeableConcept value) { 529 this.container = value; 530 return this; 531 } 532 533 /** 534 * @return {@link #content} (A set of components that go to make up the described item.) 535 */ 536 public List<MedicationPackageContentComponent> getContent() { 537 if (this.content == null) 538 this.content = new ArrayList<MedicationPackageContentComponent>(); 539 return this.content; 540 } 541 542 /** 543 * @return Returns a reference to <code>this</code> for easy method chaining 544 */ 545 public MedicationPackageComponent setContent(List<MedicationPackageContentComponent> theContent) { 546 this.content = theContent; 547 return this; 548 } 549 550 public boolean hasContent() { 551 if (this.content == null) 552 return false; 553 for (MedicationPackageContentComponent item : this.content) 554 if (!item.isEmpty()) 555 return true; 556 return false; 557 } 558 559 public MedicationPackageContentComponent addContent() { //3 560 MedicationPackageContentComponent t = new MedicationPackageContentComponent(); 561 if (this.content == null) 562 this.content = new ArrayList<MedicationPackageContentComponent>(); 563 this.content.add(t); 564 return t; 565 } 566 567 public MedicationPackageComponent addContent(MedicationPackageContentComponent t) { //3 568 if (t == null) 569 return this; 570 if (this.content == null) 571 this.content = new ArrayList<MedicationPackageContentComponent>(); 572 this.content.add(t); 573 return this; 574 } 575 576 /** 577 * @return The first repetition of repeating field {@link #content}, creating it if it does not already exist 578 */ 579 public MedicationPackageContentComponent getContentFirstRep() { 580 if (getContent().isEmpty()) { 581 addContent(); 582 } 583 return getContent().get(0); 584 } 585 586 /** 587 * @return {@link #batch} (Information about a group of medication produced or packaged from one production run.) 588 */ 589 public List<MedicationPackageBatchComponent> getBatch() { 590 if (this.batch == null) 591 this.batch = new ArrayList<MedicationPackageBatchComponent>(); 592 return this.batch; 593 } 594 595 /** 596 * @return Returns a reference to <code>this</code> for easy method chaining 597 */ 598 public MedicationPackageComponent setBatch(List<MedicationPackageBatchComponent> theBatch) { 599 this.batch = theBatch; 600 return this; 601 } 602 603 public boolean hasBatch() { 604 if (this.batch == null) 605 return false; 606 for (MedicationPackageBatchComponent item : this.batch) 607 if (!item.isEmpty()) 608 return true; 609 return false; 610 } 611 612 public MedicationPackageBatchComponent addBatch() { //3 613 MedicationPackageBatchComponent t = new MedicationPackageBatchComponent(); 614 if (this.batch == null) 615 this.batch = new ArrayList<MedicationPackageBatchComponent>(); 616 this.batch.add(t); 617 return t; 618 } 619 620 public MedicationPackageComponent addBatch(MedicationPackageBatchComponent t) { //3 621 if (t == null) 622 return this; 623 if (this.batch == null) 624 this.batch = new ArrayList<MedicationPackageBatchComponent>(); 625 this.batch.add(t); 626 return this; 627 } 628 629 /** 630 * @return The first repetition of repeating field {@link #batch}, creating it if it does not already exist 631 */ 632 public MedicationPackageBatchComponent getBatchFirstRep() { 633 if (getBatch().isEmpty()) { 634 addBatch(); 635 } 636 return getBatch().get(0); 637 } 638 639 protected void listChildren(List<Property> children) { 640 super.listChildren(children); 641 children.add(new Property("container", "CodeableConcept", "The kind of container that this package comes as.", 0, 1, container)); 642 children.add(new Property("content", "", "A set of components that go to make up the described item.", 0, java.lang.Integer.MAX_VALUE, content)); 643 children.add(new Property("batch", "", "Information about a group of medication produced or packaged from one production run.", 0, java.lang.Integer.MAX_VALUE, batch)); 644 } 645 646 @Override 647 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 648 switch (_hash) { 649 case -410956671: /*container*/ return new Property("container", "CodeableConcept", "The kind of container that this package comes as.", 0, 1, container); 650 case 951530617: /*content*/ return new Property("content", "", "A set of components that go to make up the described item.", 0, java.lang.Integer.MAX_VALUE, content); 651 case 93509434: /*batch*/ return new Property("batch", "", "Information about a group of medication produced or packaged from one production run.", 0, java.lang.Integer.MAX_VALUE, batch); 652 default: return super.getNamedProperty(_hash, _name, _checkValid); 653 } 654 655 } 656 657 @Override 658 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 659 switch (hash) { 660 case -410956671: /*container*/ return this.container == null ? new Base[0] : new Base[] {this.container}; // CodeableConcept 661 case 951530617: /*content*/ return this.content == null ? new Base[0] : this.content.toArray(new Base[this.content.size()]); // MedicationPackageContentComponent 662 case 93509434: /*batch*/ return this.batch == null ? new Base[0] : this.batch.toArray(new Base[this.batch.size()]); // MedicationPackageBatchComponent 663 default: return super.getProperty(hash, name, checkValid); 664 } 665 666 } 667 668 @Override 669 public Base setProperty(int hash, String name, Base value) throws FHIRException { 670 switch (hash) { 671 case -410956671: // container 672 this.container = castToCodeableConcept(value); // CodeableConcept 673 return value; 674 case 951530617: // content 675 this.getContent().add((MedicationPackageContentComponent) value); // MedicationPackageContentComponent 676 return value; 677 case 93509434: // batch 678 this.getBatch().add((MedicationPackageBatchComponent) value); // MedicationPackageBatchComponent 679 return value; 680 default: return super.setProperty(hash, name, value); 681 } 682 683 } 684 685 @Override 686 public Base setProperty(String name, Base value) throws FHIRException { 687 if (name.equals("container")) { 688 this.container = castToCodeableConcept(value); // CodeableConcept 689 } else if (name.equals("content")) { 690 this.getContent().add((MedicationPackageContentComponent) value); 691 } else if (name.equals("batch")) { 692 this.getBatch().add((MedicationPackageBatchComponent) value); 693 } else 694 return super.setProperty(name, value); 695 return value; 696 } 697 698 @Override 699 public Base makeProperty(int hash, String name) throws FHIRException { 700 switch (hash) { 701 case -410956671: return getContainer(); 702 case 951530617: return addContent(); 703 case 93509434: return addBatch(); 704 default: return super.makeProperty(hash, name); 705 } 706 707 } 708 709 @Override 710 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 711 switch (hash) { 712 case -410956671: /*container*/ return new String[] {"CodeableConcept"}; 713 case 951530617: /*content*/ return new String[] {}; 714 case 93509434: /*batch*/ return new String[] {}; 715 default: return super.getTypesForProperty(hash, name); 716 } 717 718 } 719 720 @Override 721 public Base addChild(String name) throws FHIRException { 722 if (name.equals("container")) { 723 this.container = new CodeableConcept(); 724 return this.container; 725 } 726 else if (name.equals("content")) { 727 return addContent(); 728 } 729 else if (name.equals("batch")) { 730 return addBatch(); 731 } 732 else 733 return super.addChild(name); 734 } 735 736 public MedicationPackageComponent copy() { 737 MedicationPackageComponent dst = new MedicationPackageComponent(); 738 copyValues(dst); 739 dst.container = container == null ? null : container.copy(); 740 if (content != null) { 741 dst.content = new ArrayList<MedicationPackageContentComponent>(); 742 for (MedicationPackageContentComponent i : content) 743 dst.content.add(i.copy()); 744 }; 745 if (batch != null) { 746 dst.batch = new ArrayList<MedicationPackageBatchComponent>(); 747 for (MedicationPackageBatchComponent i : batch) 748 dst.batch.add(i.copy()); 749 }; 750 return dst; 751 } 752 753 @Override 754 public boolean equalsDeep(Base other_) { 755 if (!super.equalsDeep(other_)) 756 return false; 757 if (!(other_ instanceof MedicationPackageComponent)) 758 return false; 759 MedicationPackageComponent o = (MedicationPackageComponent) other_; 760 return compareDeep(container, o.container, true) && compareDeep(content, o.content, true) && compareDeep(batch, o.batch, true) 761 ; 762 } 763 764 @Override 765 public boolean equalsShallow(Base other_) { 766 if (!super.equalsShallow(other_)) 767 return false; 768 if (!(other_ instanceof MedicationPackageComponent)) 769 return false; 770 MedicationPackageComponent o = (MedicationPackageComponent) other_; 771 return true; 772 } 773 774 public boolean isEmpty() { 775 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(container, content, batch 776 ); 777 } 778 779 public String fhirType() { 780 return "Medication.package"; 781 782 } 783 784 } 785 786 @Block() 787 public static class MedicationPackageContentComponent extends BackboneElement implements IBaseBackboneElement { 788 /** 789 * Identifies one of the items in the package. 790 */ 791 @Child(name = "item", type = {CodeableConcept.class, Medication.class}, order=1, min=1, max=1, modifier=false, summary=false) 792 @Description(shortDefinition="The item in the package", formalDefinition="Identifies one of the items in the package." ) 793 protected Type item; 794 795 /** 796 * The amount of the product that is in the package. 797 */ 798 @Child(name = "amount", type = {SimpleQuantity.class}, order=2, min=0, max=1, modifier=false, summary=false) 799 @Description(shortDefinition="Quantity present in the package", formalDefinition="The amount of the product that is in the package." ) 800 protected SimpleQuantity amount; 801 802 private static final long serialVersionUID = 1669610080L; 803 804 /** 805 * Constructor 806 */ 807 public MedicationPackageContentComponent() { 808 super(); 809 } 810 811 /** 812 * Constructor 813 */ 814 public MedicationPackageContentComponent(Type item) { 815 super(); 816 this.item = item; 817 } 818 819 /** 820 * @return {@link #item} (Identifies one of the items in the package.) 821 */ 822 public Type getItem() { 823 return this.item; 824 } 825 826 /** 827 * @return {@link #item} (Identifies one of the items in the package.) 828 */ 829 public CodeableConcept getItemCodeableConcept() throws FHIRException { 830 if (this.item == null) 831 return null; 832 if (!(this.item instanceof CodeableConcept)) 833 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.item.getClass().getName()+" was encountered"); 834 return (CodeableConcept) this.item; 835 } 836 837 public boolean hasItemCodeableConcept() { 838 return this != null && this.item instanceof CodeableConcept; 839 } 840 841 /** 842 * @return {@link #item} (Identifies one of the items in the package.) 843 */ 844 public Reference getItemReference() throws FHIRException { 845 if (this.item == null) 846 return null; 847 if (!(this.item instanceof Reference)) 848 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.item.getClass().getName()+" was encountered"); 849 return (Reference) this.item; 850 } 851 852 public boolean hasItemReference() { 853 return this != null && this.item instanceof Reference; 854 } 855 856 public boolean hasItem() { 857 return this.item != null && !this.item.isEmpty(); 858 } 859 860 /** 861 * @param value {@link #item} (Identifies one of the items in the package.) 862 */ 863 public MedicationPackageContentComponent setItem(Type value) throws FHIRFormatError { 864 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 865 throw new FHIRFormatError("Not the right type for Medication.package.content.item[x]: "+value.fhirType()); 866 this.item = value; 867 return this; 868 } 869 870 /** 871 * @return {@link #amount} (The amount of the product that is in the package.) 872 */ 873 public SimpleQuantity getAmount() { 874 if (this.amount == null) 875 if (Configuration.errorOnAutoCreate()) 876 throw new Error("Attempt to auto-create MedicationPackageContentComponent.amount"); 877 else if (Configuration.doAutoCreate()) 878 this.amount = new SimpleQuantity(); // cc 879 return this.amount; 880 } 881 882 public boolean hasAmount() { 883 return this.amount != null && !this.amount.isEmpty(); 884 } 885 886 /** 887 * @param value {@link #amount} (The amount of the product that is in the package.) 888 */ 889 public MedicationPackageContentComponent setAmount(SimpleQuantity value) { 890 this.amount = value; 891 return this; 892 } 893 894 protected void listChildren(List<Property> children) { 895 super.listChildren(children); 896 children.add(new Property("item[x]", "CodeableConcept|Reference(Medication)", "Identifies one of the items in the package.", 0, 1, item)); 897 children.add(new Property("amount", "SimpleQuantity", "The amount of the product that is in the package.", 0, 1, amount)); 898 } 899 900 @Override 901 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 902 switch (_hash) { 903 case 2116201613: /*item[x]*/ return new Property("item[x]", "CodeableConcept|Reference(Medication)", "Identifies one of the items in the package.", 0, 1, item); 904 case 3242771: /*item*/ return new Property("item[x]", "CodeableConcept|Reference(Medication)", "Identifies one of the items in the package.", 0, 1, item); 905 case 106644494: /*itemCodeableConcept*/ return new Property("item[x]", "CodeableConcept|Reference(Medication)", "Identifies one of the items in the package.", 0, 1, item); 906 case 1376364920: /*itemReference*/ return new Property("item[x]", "CodeableConcept|Reference(Medication)", "Identifies one of the items in the package.", 0, 1, item); 907 case -1413853096: /*amount*/ return new Property("amount", "SimpleQuantity", "The amount of the product that is in the package.", 0, 1, amount); 908 default: return super.getNamedProperty(_hash, _name, _checkValid); 909 } 910 911 } 912 913 @Override 914 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 915 switch (hash) { 916 case 3242771: /*item*/ return this.item == null ? new Base[0] : new Base[] {this.item}; // Type 917 case -1413853096: /*amount*/ return this.amount == null ? new Base[0] : new Base[] {this.amount}; // SimpleQuantity 918 default: return super.getProperty(hash, name, checkValid); 919 } 920 921 } 922 923 @Override 924 public Base setProperty(int hash, String name, Base value) throws FHIRException { 925 switch (hash) { 926 case 3242771: // item 927 this.item = castToType(value); // Type 928 return value; 929 case -1413853096: // amount 930 this.amount = castToSimpleQuantity(value); // SimpleQuantity 931 return value; 932 default: return super.setProperty(hash, name, value); 933 } 934 935 } 936 937 @Override 938 public Base setProperty(String name, Base value) throws FHIRException { 939 if (name.equals("item[x]")) { 940 this.item = castToType(value); // Type 941 } else if (name.equals("amount")) { 942 this.amount = castToSimpleQuantity(value); // SimpleQuantity 943 } else 944 return super.setProperty(name, value); 945 return value; 946 } 947 948 @Override 949 public Base makeProperty(int hash, String name) throws FHIRException { 950 switch (hash) { 951 case 2116201613: return getItem(); 952 case 3242771: return getItem(); 953 case -1413853096: return getAmount(); 954 default: return super.makeProperty(hash, name); 955 } 956 957 } 958 959 @Override 960 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 961 switch (hash) { 962 case 3242771: /*item*/ return new String[] {"CodeableConcept", "Reference"}; 963 case -1413853096: /*amount*/ return new String[] {"SimpleQuantity"}; 964 default: return super.getTypesForProperty(hash, name); 965 } 966 967 } 968 969 @Override 970 public Base addChild(String name) throws FHIRException { 971 if (name.equals("itemCodeableConcept")) { 972 this.item = new CodeableConcept(); 973 return this.item; 974 } 975 else if (name.equals("itemReference")) { 976 this.item = new Reference(); 977 return this.item; 978 } 979 else if (name.equals("amount")) { 980 this.amount = new SimpleQuantity(); 981 return this.amount; 982 } 983 else 984 return super.addChild(name); 985 } 986 987 public MedicationPackageContentComponent copy() { 988 MedicationPackageContentComponent dst = new MedicationPackageContentComponent(); 989 copyValues(dst); 990 dst.item = item == null ? null : item.copy(); 991 dst.amount = amount == null ? null : amount.copy(); 992 return dst; 993 } 994 995 @Override 996 public boolean equalsDeep(Base other_) { 997 if (!super.equalsDeep(other_)) 998 return false; 999 if (!(other_ instanceof MedicationPackageContentComponent)) 1000 return false; 1001 MedicationPackageContentComponent o = (MedicationPackageContentComponent) other_; 1002 return compareDeep(item, o.item, true) && compareDeep(amount, o.amount, true); 1003 } 1004 1005 @Override 1006 public boolean equalsShallow(Base other_) { 1007 if (!super.equalsShallow(other_)) 1008 return false; 1009 if (!(other_ instanceof MedicationPackageContentComponent)) 1010 return false; 1011 MedicationPackageContentComponent o = (MedicationPackageContentComponent) other_; 1012 return true; 1013 } 1014 1015 public boolean isEmpty() { 1016 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(item, amount); 1017 } 1018 1019 public String fhirType() { 1020 return "Medication.package.content"; 1021 1022 } 1023 1024 } 1025 1026 @Block() 1027 public static class MedicationPackageBatchComponent extends BackboneElement implements IBaseBackboneElement { 1028 /** 1029 * The assigned lot number of a batch of the specified product. 1030 */ 1031 @Child(name = "lotNumber", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 1032 @Description(shortDefinition="Identifier assigned to batch", formalDefinition="The assigned lot number of a batch of the specified product." ) 1033 protected StringType lotNumber; 1034 1035 /** 1036 * When this specific batch of product will expire. 1037 */ 1038 @Child(name = "expirationDate", type = {DateTimeType.class}, order=2, min=0, max=1, modifier=false, summary=false) 1039 @Description(shortDefinition="When batch will expire", formalDefinition="When this specific batch of product will expire." ) 1040 protected DateTimeType expirationDate; 1041 1042 private static final long serialVersionUID = 1982738755L; 1043 1044 /** 1045 * Constructor 1046 */ 1047 public MedicationPackageBatchComponent() { 1048 super(); 1049 } 1050 1051 /** 1052 * @return {@link #lotNumber} (The assigned lot number of a batch of the specified product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1053 */ 1054 public StringType getLotNumberElement() { 1055 if (this.lotNumber == null) 1056 if (Configuration.errorOnAutoCreate()) 1057 throw new Error("Attempt to auto-create MedicationPackageBatchComponent.lotNumber"); 1058 else if (Configuration.doAutoCreate()) 1059 this.lotNumber = new StringType(); // bb 1060 return this.lotNumber; 1061 } 1062 1063 public boolean hasLotNumberElement() { 1064 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1065 } 1066 1067 public boolean hasLotNumber() { 1068 return this.lotNumber != null && !this.lotNumber.isEmpty(); 1069 } 1070 1071 /** 1072 * @param value {@link #lotNumber} (The assigned lot number of a batch of the specified product.). This is the underlying object with id, value and extensions. The accessor "getLotNumber" gives direct access to the value 1073 */ 1074 public MedicationPackageBatchComponent setLotNumberElement(StringType value) { 1075 this.lotNumber = value; 1076 return this; 1077 } 1078 1079 /** 1080 * @return The assigned lot number of a batch of the specified product. 1081 */ 1082 public String getLotNumber() { 1083 return this.lotNumber == null ? null : this.lotNumber.getValue(); 1084 } 1085 1086 /** 1087 * @param value The assigned lot number of a batch of the specified product. 1088 */ 1089 public MedicationPackageBatchComponent setLotNumber(String value) { 1090 if (Utilities.noString(value)) 1091 this.lotNumber = null; 1092 else { 1093 if (this.lotNumber == null) 1094 this.lotNumber = new StringType(); 1095 this.lotNumber.setValue(value); 1096 } 1097 return this; 1098 } 1099 1100 /** 1101 * @return {@link #expirationDate} (When this specific batch of product will expire.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 1102 */ 1103 public DateTimeType getExpirationDateElement() { 1104 if (this.expirationDate == null) 1105 if (Configuration.errorOnAutoCreate()) 1106 throw new Error("Attempt to auto-create MedicationPackageBatchComponent.expirationDate"); 1107 else if (Configuration.doAutoCreate()) 1108 this.expirationDate = new DateTimeType(); // bb 1109 return this.expirationDate; 1110 } 1111 1112 public boolean hasExpirationDateElement() { 1113 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1114 } 1115 1116 public boolean hasExpirationDate() { 1117 return this.expirationDate != null && !this.expirationDate.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #expirationDate} (When this specific batch of product will expire.). This is the underlying object with id, value and extensions. The accessor "getExpirationDate" gives direct access to the value 1122 */ 1123 public MedicationPackageBatchComponent setExpirationDateElement(DateTimeType value) { 1124 this.expirationDate = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return When this specific batch of product will expire. 1130 */ 1131 public Date getExpirationDate() { 1132 return this.expirationDate == null ? null : this.expirationDate.getValue(); 1133 } 1134 1135 /** 1136 * @param value When this specific batch of product will expire. 1137 */ 1138 public MedicationPackageBatchComponent setExpirationDate(Date value) { 1139 if (value == null) 1140 this.expirationDate = null; 1141 else { 1142 if (this.expirationDate == null) 1143 this.expirationDate = new DateTimeType(); 1144 this.expirationDate.setValue(value); 1145 } 1146 return this; 1147 } 1148 1149 protected void listChildren(List<Property> children) { 1150 super.listChildren(children); 1151 children.add(new Property("lotNumber", "string", "The assigned lot number of a batch of the specified product.", 0, 1, lotNumber)); 1152 children.add(new Property("expirationDate", "dateTime", "When this specific batch of product will expire.", 0, 1, expirationDate)); 1153 } 1154 1155 @Override 1156 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1157 switch (_hash) { 1158 case 462547450: /*lotNumber*/ return new Property("lotNumber", "string", "The assigned lot number of a batch of the specified product.", 0, 1, lotNumber); 1159 case -668811523: /*expirationDate*/ return new Property("expirationDate", "dateTime", "When this specific batch of product will expire.", 0, 1, expirationDate); 1160 default: return super.getNamedProperty(_hash, _name, _checkValid); 1161 } 1162 1163 } 1164 1165 @Override 1166 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1167 switch (hash) { 1168 case 462547450: /*lotNumber*/ return this.lotNumber == null ? new Base[0] : new Base[] {this.lotNumber}; // StringType 1169 case -668811523: /*expirationDate*/ return this.expirationDate == null ? new Base[0] : new Base[] {this.expirationDate}; // DateTimeType 1170 default: return super.getProperty(hash, name, checkValid); 1171 } 1172 1173 } 1174 1175 @Override 1176 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1177 switch (hash) { 1178 case 462547450: // lotNumber 1179 this.lotNumber = castToString(value); // StringType 1180 return value; 1181 case -668811523: // expirationDate 1182 this.expirationDate = castToDateTime(value); // DateTimeType 1183 return value; 1184 default: return super.setProperty(hash, name, value); 1185 } 1186 1187 } 1188 1189 @Override 1190 public Base setProperty(String name, Base value) throws FHIRException { 1191 if (name.equals("lotNumber")) { 1192 this.lotNumber = castToString(value); // StringType 1193 } else if (name.equals("expirationDate")) { 1194 this.expirationDate = castToDateTime(value); // DateTimeType 1195 } else 1196 return super.setProperty(name, value); 1197 return value; 1198 } 1199 1200 @Override 1201 public Base makeProperty(int hash, String name) throws FHIRException { 1202 switch (hash) { 1203 case 462547450: return getLotNumberElement(); 1204 case -668811523: return getExpirationDateElement(); 1205 default: return super.makeProperty(hash, name); 1206 } 1207 1208 } 1209 1210 @Override 1211 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1212 switch (hash) { 1213 case 462547450: /*lotNumber*/ return new String[] {"string"}; 1214 case -668811523: /*expirationDate*/ return new String[] {"dateTime"}; 1215 default: return super.getTypesForProperty(hash, name); 1216 } 1217 1218 } 1219 1220 @Override 1221 public Base addChild(String name) throws FHIRException { 1222 if (name.equals("lotNumber")) { 1223 throw new FHIRException("Cannot call addChild on a singleton property Medication.lotNumber"); 1224 } 1225 else if (name.equals("expirationDate")) { 1226 throw new FHIRException("Cannot call addChild on a singleton property Medication.expirationDate"); 1227 } 1228 else 1229 return super.addChild(name); 1230 } 1231 1232 public MedicationPackageBatchComponent copy() { 1233 MedicationPackageBatchComponent dst = new MedicationPackageBatchComponent(); 1234 copyValues(dst); 1235 dst.lotNumber = lotNumber == null ? null : lotNumber.copy(); 1236 dst.expirationDate = expirationDate == null ? null : expirationDate.copy(); 1237 return dst; 1238 } 1239 1240 @Override 1241 public boolean equalsDeep(Base other_) { 1242 if (!super.equalsDeep(other_)) 1243 return false; 1244 if (!(other_ instanceof MedicationPackageBatchComponent)) 1245 return false; 1246 MedicationPackageBatchComponent o = (MedicationPackageBatchComponent) other_; 1247 return compareDeep(lotNumber, o.lotNumber, true) && compareDeep(expirationDate, o.expirationDate, true) 1248 ; 1249 } 1250 1251 @Override 1252 public boolean equalsShallow(Base other_) { 1253 if (!super.equalsShallow(other_)) 1254 return false; 1255 if (!(other_ instanceof MedicationPackageBatchComponent)) 1256 return false; 1257 MedicationPackageBatchComponent o = (MedicationPackageBatchComponent) other_; 1258 return compareValues(lotNumber, o.lotNumber, true) && compareValues(expirationDate, o.expirationDate, true) 1259 ; 1260 } 1261 1262 public boolean isEmpty() { 1263 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(lotNumber, expirationDate 1264 ); 1265 } 1266 1267 public String fhirType() { 1268 return "Medication.package.batch"; 1269 1270 } 1271 1272 } 1273 1274 /** 1275 * A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems. 1276 */ 1277 @Child(name = "code", type = {CodeableConcept.class}, order=0, min=0, max=1, modifier=false, summary=true) 1278 @Description(shortDefinition="Codes that identify this medication", formalDefinition="A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems." ) 1279 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 1280 protected CodeableConcept code; 1281 1282 /** 1283 * A code to indicate if the medication is in active use. 1284 */ 1285 @Child(name = "status", type = {CodeType.class}, order=1, min=0, max=1, modifier=false, summary=true) 1286 @Description(shortDefinition="active | inactive | entered-in-error", formalDefinition="A code to indicate if the medication is in active use." ) 1287 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-status") 1288 protected Enumeration<MedicationStatus> status; 1289 1290 /** 1291 * Set to true if the item is attributable to a specific manufacturer. 1292 */ 1293 @Child(name = "isBrand", type = {BooleanType.class}, order=2, min=0, max=1, modifier=false, summary=true) 1294 @Description(shortDefinition="True if a brand", formalDefinition="Set to true if the item is attributable to a specific manufacturer." ) 1295 protected BooleanType isBrand; 1296 1297 /** 1298 * Set to true if the medication can be obtained without an order from a prescriber. 1299 */ 1300 @Child(name = "isOverTheCounter", type = {BooleanType.class}, order=3, min=0, max=1, modifier=false, summary=true) 1301 @Description(shortDefinition="True if medication does not require a prescription", formalDefinition="Set to true if the medication can be obtained without an order from a prescriber." ) 1302 protected BooleanType isOverTheCounter; 1303 1304 /** 1305 * Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product. 1306 */ 1307 @Child(name = "manufacturer", type = {Organization.class}, order=4, min=0, max=1, modifier=false, summary=true) 1308 @Description(shortDefinition="Manufacturer of the item", formalDefinition="Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product." ) 1309 protected Reference manufacturer; 1310 1311 /** 1312 * The actual object that is the target of the reference (Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.) 1313 */ 1314 protected Organization manufacturerTarget; 1315 1316 /** 1317 * Describes the form of the item. Powder; tablets; capsule. 1318 */ 1319 @Child(name = "form", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=false) 1320 @Description(shortDefinition="powder | tablets | capsule +", formalDefinition="Describes the form of the item. Powder; tablets; capsule." ) 1321 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-form-codes") 1322 protected CodeableConcept form; 1323 1324 /** 1325 * Identifies a particular constituent of interest in the product. 1326 */ 1327 @Child(name = "ingredient", type = {}, order=6, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1328 @Description(shortDefinition="Active or inactive ingredient", formalDefinition="Identifies a particular constituent of interest in the product." ) 1329 protected List<MedicationIngredientComponent> ingredient; 1330 1331 /** 1332 * Information that only applies to packages (not products). 1333 */ 1334 @Child(name = "package", type = {}, order=7, min=0, max=1, modifier=false, summary=false) 1335 @Description(shortDefinition="Details about packaged medications", formalDefinition="Information that only applies to packages (not products)." ) 1336 protected MedicationPackageComponent package_; 1337 1338 /** 1339 * Photo(s) or graphic representation(s) of the medication. 1340 */ 1341 @Child(name = "image", type = {Attachment.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1342 @Description(shortDefinition="Picture of the medication", formalDefinition="Photo(s) or graphic representation(s) of the medication." ) 1343 protected List<Attachment> image; 1344 1345 private static final long serialVersionUID = 860383645L; 1346 1347 /** 1348 * Constructor 1349 */ 1350 public Medication() { 1351 super(); 1352 } 1353 1354 /** 1355 * @return {@link #code} (A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.) 1356 */ 1357 public CodeableConcept getCode() { 1358 if (this.code == null) 1359 if (Configuration.errorOnAutoCreate()) 1360 throw new Error("Attempt to auto-create Medication.code"); 1361 else if (Configuration.doAutoCreate()) 1362 this.code = new CodeableConcept(); // cc 1363 return this.code; 1364 } 1365 1366 public boolean hasCode() { 1367 return this.code != null && !this.code.isEmpty(); 1368 } 1369 1370 /** 1371 * @param value {@link #code} (A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.) 1372 */ 1373 public Medication setCode(CodeableConcept value) { 1374 this.code = value; 1375 return this; 1376 } 1377 1378 /** 1379 * @return {@link #status} (A code to indicate if the medication is in active use.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1380 */ 1381 public Enumeration<MedicationStatus> getStatusElement() { 1382 if (this.status == null) 1383 if (Configuration.errorOnAutoCreate()) 1384 throw new Error("Attempt to auto-create Medication.status"); 1385 else if (Configuration.doAutoCreate()) 1386 this.status = new Enumeration<MedicationStatus>(new MedicationStatusEnumFactory()); // bb 1387 return this.status; 1388 } 1389 1390 public boolean hasStatusElement() { 1391 return this.status != null && !this.status.isEmpty(); 1392 } 1393 1394 public boolean hasStatus() { 1395 return this.status != null && !this.status.isEmpty(); 1396 } 1397 1398 /** 1399 * @param value {@link #status} (A code to indicate if the medication is in active use.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1400 */ 1401 public Medication setStatusElement(Enumeration<MedicationStatus> value) { 1402 this.status = value; 1403 return this; 1404 } 1405 1406 /** 1407 * @return A code to indicate if the medication is in active use. 1408 */ 1409 public MedicationStatus getStatus() { 1410 return this.status == null ? null : this.status.getValue(); 1411 } 1412 1413 /** 1414 * @param value A code to indicate if the medication is in active use. 1415 */ 1416 public Medication setStatus(MedicationStatus value) { 1417 if (value == null) 1418 this.status = null; 1419 else { 1420 if (this.status == null) 1421 this.status = new Enumeration<MedicationStatus>(new MedicationStatusEnumFactory()); 1422 this.status.setValue(value); 1423 } 1424 return this; 1425 } 1426 1427 /** 1428 * @return {@link #isBrand} (Set to true if the item is attributable to a specific manufacturer.). This is the underlying object with id, value and extensions. The accessor "getIsBrand" gives direct access to the value 1429 */ 1430 public BooleanType getIsBrandElement() { 1431 if (this.isBrand == null) 1432 if (Configuration.errorOnAutoCreate()) 1433 throw new Error("Attempt to auto-create Medication.isBrand"); 1434 else if (Configuration.doAutoCreate()) 1435 this.isBrand = new BooleanType(); // bb 1436 return this.isBrand; 1437 } 1438 1439 public boolean hasIsBrandElement() { 1440 return this.isBrand != null && !this.isBrand.isEmpty(); 1441 } 1442 1443 public boolean hasIsBrand() { 1444 return this.isBrand != null && !this.isBrand.isEmpty(); 1445 } 1446 1447 /** 1448 * @param value {@link #isBrand} (Set to true if the item is attributable to a specific manufacturer.). This is the underlying object with id, value and extensions. The accessor "getIsBrand" gives direct access to the value 1449 */ 1450 public Medication setIsBrandElement(BooleanType value) { 1451 this.isBrand = value; 1452 return this; 1453 } 1454 1455 /** 1456 * @return Set to true if the item is attributable to a specific manufacturer. 1457 */ 1458 public boolean getIsBrand() { 1459 return this.isBrand == null || this.isBrand.isEmpty() ? false : this.isBrand.getValue(); 1460 } 1461 1462 /** 1463 * @param value Set to true if the item is attributable to a specific manufacturer. 1464 */ 1465 public Medication setIsBrand(boolean value) { 1466 if (this.isBrand == null) 1467 this.isBrand = new BooleanType(); 1468 this.isBrand.setValue(value); 1469 return this; 1470 } 1471 1472 /** 1473 * @return {@link #isOverTheCounter} (Set to true if the medication can be obtained without an order from a prescriber.). This is the underlying object with id, value and extensions. The accessor "getIsOverTheCounter" gives direct access to the value 1474 */ 1475 public BooleanType getIsOverTheCounterElement() { 1476 if (this.isOverTheCounter == null) 1477 if (Configuration.errorOnAutoCreate()) 1478 throw new Error("Attempt to auto-create Medication.isOverTheCounter"); 1479 else if (Configuration.doAutoCreate()) 1480 this.isOverTheCounter = new BooleanType(); // bb 1481 return this.isOverTheCounter; 1482 } 1483 1484 public boolean hasIsOverTheCounterElement() { 1485 return this.isOverTheCounter != null && !this.isOverTheCounter.isEmpty(); 1486 } 1487 1488 public boolean hasIsOverTheCounter() { 1489 return this.isOverTheCounter != null && !this.isOverTheCounter.isEmpty(); 1490 } 1491 1492 /** 1493 * @param value {@link #isOverTheCounter} (Set to true if the medication can be obtained without an order from a prescriber.). This is the underlying object with id, value and extensions. The accessor "getIsOverTheCounter" gives direct access to the value 1494 */ 1495 public Medication setIsOverTheCounterElement(BooleanType value) { 1496 this.isOverTheCounter = value; 1497 return this; 1498 } 1499 1500 /** 1501 * @return Set to true if the medication can be obtained without an order from a prescriber. 1502 */ 1503 public boolean getIsOverTheCounter() { 1504 return this.isOverTheCounter == null || this.isOverTheCounter.isEmpty() ? false : this.isOverTheCounter.getValue(); 1505 } 1506 1507 /** 1508 * @param value Set to true if the medication can be obtained without an order from a prescriber. 1509 */ 1510 public Medication setIsOverTheCounter(boolean value) { 1511 if (this.isOverTheCounter == null) 1512 this.isOverTheCounter = new BooleanType(); 1513 this.isOverTheCounter.setValue(value); 1514 return this; 1515 } 1516 1517 /** 1518 * @return {@link #manufacturer} (Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.) 1519 */ 1520 public Reference getManufacturer() { 1521 if (this.manufacturer == null) 1522 if (Configuration.errorOnAutoCreate()) 1523 throw new Error("Attempt to auto-create Medication.manufacturer"); 1524 else if (Configuration.doAutoCreate()) 1525 this.manufacturer = new Reference(); // cc 1526 return this.manufacturer; 1527 } 1528 1529 public boolean hasManufacturer() { 1530 return this.manufacturer != null && !this.manufacturer.isEmpty(); 1531 } 1532 1533 /** 1534 * @param value {@link #manufacturer} (Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.) 1535 */ 1536 public Medication setManufacturer(Reference value) { 1537 this.manufacturer = value; 1538 return this; 1539 } 1540 1541 /** 1542 * @return {@link #manufacturer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.) 1543 */ 1544 public Organization getManufacturerTarget() { 1545 if (this.manufacturerTarget == null) 1546 if (Configuration.errorOnAutoCreate()) 1547 throw new Error("Attempt to auto-create Medication.manufacturer"); 1548 else if (Configuration.doAutoCreate()) 1549 this.manufacturerTarget = new Organization(); // aa 1550 return this.manufacturerTarget; 1551 } 1552 1553 /** 1554 * @param value {@link #manufacturer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.) 1555 */ 1556 public Medication setManufacturerTarget(Organization value) { 1557 this.manufacturerTarget = value; 1558 return this; 1559 } 1560 1561 /** 1562 * @return {@link #form} (Describes the form of the item. Powder; tablets; capsule.) 1563 */ 1564 public CodeableConcept getForm() { 1565 if (this.form == null) 1566 if (Configuration.errorOnAutoCreate()) 1567 throw new Error("Attempt to auto-create Medication.form"); 1568 else if (Configuration.doAutoCreate()) 1569 this.form = new CodeableConcept(); // cc 1570 return this.form; 1571 } 1572 1573 public boolean hasForm() { 1574 return this.form != null && !this.form.isEmpty(); 1575 } 1576 1577 /** 1578 * @param value {@link #form} (Describes the form of the item. Powder; tablets; capsule.) 1579 */ 1580 public Medication setForm(CodeableConcept value) { 1581 this.form = value; 1582 return this; 1583 } 1584 1585 /** 1586 * @return {@link #ingredient} (Identifies a particular constituent of interest in the product.) 1587 */ 1588 public List<MedicationIngredientComponent> getIngredient() { 1589 if (this.ingredient == null) 1590 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1591 return this.ingredient; 1592 } 1593 1594 /** 1595 * @return Returns a reference to <code>this</code> for easy method chaining 1596 */ 1597 public Medication setIngredient(List<MedicationIngredientComponent> theIngredient) { 1598 this.ingredient = theIngredient; 1599 return this; 1600 } 1601 1602 public boolean hasIngredient() { 1603 if (this.ingredient == null) 1604 return false; 1605 for (MedicationIngredientComponent item : this.ingredient) 1606 if (!item.isEmpty()) 1607 return true; 1608 return false; 1609 } 1610 1611 public MedicationIngredientComponent addIngredient() { //3 1612 MedicationIngredientComponent t = new MedicationIngredientComponent(); 1613 if (this.ingredient == null) 1614 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1615 this.ingredient.add(t); 1616 return t; 1617 } 1618 1619 public Medication addIngredient(MedicationIngredientComponent t) { //3 1620 if (t == null) 1621 return this; 1622 if (this.ingredient == null) 1623 this.ingredient = new ArrayList<MedicationIngredientComponent>(); 1624 this.ingredient.add(t); 1625 return this; 1626 } 1627 1628 /** 1629 * @return The first repetition of repeating field {@link #ingredient}, creating it if it does not already exist 1630 */ 1631 public MedicationIngredientComponent getIngredientFirstRep() { 1632 if (getIngredient().isEmpty()) { 1633 addIngredient(); 1634 } 1635 return getIngredient().get(0); 1636 } 1637 1638 /** 1639 * @return {@link #package_} (Information that only applies to packages (not products).) 1640 */ 1641 public MedicationPackageComponent getPackage() { 1642 if (this.package_ == null) 1643 if (Configuration.errorOnAutoCreate()) 1644 throw new Error("Attempt to auto-create Medication.package_"); 1645 else if (Configuration.doAutoCreate()) 1646 this.package_ = new MedicationPackageComponent(); // cc 1647 return this.package_; 1648 } 1649 1650 public boolean hasPackage() { 1651 return this.package_ != null && !this.package_.isEmpty(); 1652 } 1653 1654 /** 1655 * @param value {@link #package_} (Information that only applies to packages (not products).) 1656 */ 1657 public Medication setPackage(MedicationPackageComponent value) { 1658 this.package_ = value; 1659 return this; 1660 } 1661 1662 /** 1663 * @return {@link #image} (Photo(s) or graphic representation(s) of the medication.) 1664 */ 1665 public List<Attachment> getImage() { 1666 if (this.image == null) 1667 this.image = new ArrayList<Attachment>(); 1668 return this.image; 1669 } 1670 1671 /** 1672 * @return Returns a reference to <code>this</code> for easy method chaining 1673 */ 1674 public Medication setImage(List<Attachment> theImage) { 1675 this.image = theImage; 1676 return this; 1677 } 1678 1679 public boolean hasImage() { 1680 if (this.image == null) 1681 return false; 1682 for (Attachment item : this.image) 1683 if (!item.isEmpty()) 1684 return true; 1685 return false; 1686 } 1687 1688 public Attachment addImage() { //3 1689 Attachment t = new Attachment(); 1690 if (this.image == null) 1691 this.image = new ArrayList<Attachment>(); 1692 this.image.add(t); 1693 return t; 1694 } 1695 1696 public Medication addImage(Attachment t) { //3 1697 if (t == null) 1698 return this; 1699 if (this.image == null) 1700 this.image = new ArrayList<Attachment>(); 1701 this.image.add(t); 1702 return this; 1703 } 1704 1705 /** 1706 * @return The first repetition of repeating field {@link #image}, creating it if it does not already exist 1707 */ 1708 public Attachment getImageFirstRep() { 1709 if (getImage().isEmpty()) { 1710 addImage(); 1711 } 1712 return getImage().get(0); 1713 } 1714 1715 protected void listChildren(List<Property> children) { 1716 super.listChildren(children); 1717 children.add(new Property("code", "CodeableConcept", "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 0, 1, code)); 1718 children.add(new Property("status", "code", "A code to indicate if the medication is in active use.", 0, 1, status)); 1719 children.add(new Property("isBrand", "boolean", "Set to true if the item is attributable to a specific manufacturer.", 0, 1, isBrand)); 1720 children.add(new Property("isOverTheCounter", "boolean", "Set to true if the medication can be obtained without an order from a prescriber.", 0, 1, isOverTheCounter)); 1721 children.add(new Property("manufacturer", "Reference(Organization)", "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.", 0, 1, manufacturer)); 1722 children.add(new Property("form", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 0, 1, form)); 1723 children.add(new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, ingredient)); 1724 children.add(new Property("package", "", "Information that only applies to packages (not products).", 0, 1, package_)); 1725 children.add(new Property("image", "Attachment", "Photo(s) or graphic representation(s) of the medication.", 0, java.lang.Integer.MAX_VALUE, image)); 1726 } 1727 1728 @Override 1729 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1730 switch (_hash) { 1731 case 3059181: /*code*/ return new Property("code", "CodeableConcept", "A code (or set of codes) that specify this medication, or a textual description if no code is available. Usage note: This could be a standard medication code such as a code from RxNorm, SNOMED CT, IDMP etc. It could also be a national or local formulary code, optionally with translations to other code systems.", 0, 1, code); 1732 case -892481550: /*status*/ return new Property("status", "code", "A code to indicate if the medication is in active use.", 0, 1, status); 1733 case 2055403645: /*isBrand*/ return new Property("isBrand", "boolean", "Set to true if the item is attributable to a specific manufacturer.", 0, 1, isBrand); 1734 case -650796023: /*isOverTheCounter*/ return new Property("isOverTheCounter", "boolean", "Set to true if the medication can be obtained without an order from a prescriber.", 0, 1, isOverTheCounter); 1735 case -1969347631: /*manufacturer*/ return new Property("manufacturer", "Reference(Organization)", "Describes the details of the manufacturer of the medication product. This is not intended to represent the distributor of a medication product.", 0, 1, manufacturer); 1736 case 3148996: /*form*/ return new Property("form", "CodeableConcept", "Describes the form of the item. Powder; tablets; capsule.", 0, 1, form); 1737 case -206409263: /*ingredient*/ return new Property("ingredient", "", "Identifies a particular constituent of interest in the product.", 0, java.lang.Integer.MAX_VALUE, ingredient); 1738 case -807062458: /*package*/ return new Property("package", "", "Information that only applies to packages (not products).", 0, 1, package_); 1739 case 100313435: /*image*/ return new Property("image", "Attachment", "Photo(s) or graphic representation(s) of the medication.", 0, java.lang.Integer.MAX_VALUE, image); 1740 default: return super.getNamedProperty(_hash, _name, _checkValid); 1741 } 1742 1743 } 1744 1745 @Override 1746 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1747 switch (hash) { 1748 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeableConcept 1749 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationStatus> 1750 case 2055403645: /*isBrand*/ return this.isBrand == null ? new Base[0] : new Base[] {this.isBrand}; // BooleanType 1751 case -650796023: /*isOverTheCounter*/ return this.isOverTheCounter == null ? new Base[0] : new Base[] {this.isOverTheCounter}; // BooleanType 1752 case -1969347631: /*manufacturer*/ return this.manufacturer == null ? new Base[0] : new Base[] {this.manufacturer}; // Reference 1753 case 3148996: /*form*/ return this.form == null ? new Base[0] : new Base[] {this.form}; // CodeableConcept 1754 case -206409263: /*ingredient*/ return this.ingredient == null ? new Base[0] : this.ingredient.toArray(new Base[this.ingredient.size()]); // MedicationIngredientComponent 1755 case -807062458: /*package*/ return this.package_ == null ? new Base[0] : new Base[] {this.package_}; // MedicationPackageComponent 1756 case 100313435: /*image*/ return this.image == null ? new Base[0] : this.image.toArray(new Base[this.image.size()]); // Attachment 1757 default: return super.getProperty(hash, name, checkValid); 1758 } 1759 1760 } 1761 1762 @Override 1763 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1764 switch (hash) { 1765 case 3059181: // code 1766 this.code = castToCodeableConcept(value); // CodeableConcept 1767 return value; 1768 case -892481550: // status 1769 value = new MedicationStatusEnumFactory().fromType(castToCode(value)); 1770 this.status = (Enumeration) value; // Enumeration<MedicationStatus> 1771 return value; 1772 case 2055403645: // isBrand 1773 this.isBrand = castToBoolean(value); // BooleanType 1774 return value; 1775 case -650796023: // isOverTheCounter 1776 this.isOverTheCounter = castToBoolean(value); // BooleanType 1777 return value; 1778 case -1969347631: // manufacturer 1779 this.manufacturer = castToReference(value); // Reference 1780 return value; 1781 case 3148996: // form 1782 this.form = castToCodeableConcept(value); // CodeableConcept 1783 return value; 1784 case -206409263: // ingredient 1785 this.getIngredient().add((MedicationIngredientComponent) value); // MedicationIngredientComponent 1786 return value; 1787 case -807062458: // package 1788 this.package_ = (MedicationPackageComponent) value; // MedicationPackageComponent 1789 return value; 1790 case 100313435: // image 1791 this.getImage().add(castToAttachment(value)); // Attachment 1792 return value; 1793 default: return super.setProperty(hash, name, value); 1794 } 1795 1796 } 1797 1798 @Override 1799 public Base setProperty(String name, Base value) throws FHIRException { 1800 if (name.equals("code")) { 1801 this.code = castToCodeableConcept(value); // CodeableConcept 1802 } else if (name.equals("status")) { 1803 value = new MedicationStatusEnumFactory().fromType(castToCode(value)); 1804 this.status = (Enumeration) value; // Enumeration<MedicationStatus> 1805 } else if (name.equals("isBrand")) { 1806 this.isBrand = castToBoolean(value); // BooleanType 1807 } else if (name.equals("isOverTheCounter")) { 1808 this.isOverTheCounter = castToBoolean(value); // BooleanType 1809 } else if (name.equals("manufacturer")) { 1810 this.manufacturer = castToReference(value); // Reference 1811 } else if (name.equals("form")) { 1812 this.form = castToCodeableConcept(value); // CodeableConcept 1813 } else if (name.equals("ingredient")) { 1814 this.getIngredient().add((MedicationIngredientComponent) value); 1815 } else if (name.equals("package")) { 1816 this.package_ = (MedicationPackageComponent) value; // MedicationPackageComponent 1817 } else if (name.equals("image")) { 1818 this.getImage().add(castToAttachment(value)); 1819 } else 1820 return super.setProperty(name, value); 1821 return value; 1822 } 1823 1824 @Override 1825 public Base makeProperty(int hash, String name) throws FHIRException { 1826 switch (hash) { 1827 case 3059181: return getCode(); 1828 case -892481550: return getStatusElement(); 1829 case 2055403645: return getIsBrandElement(); 1830 case -650796023: return getIsOverTheCounterElement(); 1831 case -1969347631: return getManufacturer(); 1832 case 3148996: return getForm(); 1833 case -206409263: return addIngredient(); 1834 case -807062458: return getPackage(); 1835 case 100313435: return addImage(); 1836 default: return super.makeProperty(hash, name); 1837 } 1838 1839 } 1840 1841 @Override 1842 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1843 switch (hash) { 1844 case 3059181: /*code*/ return new String[] {"CodeableConcept"}; 1845 case -892481550: /*status*/ return new String[] {"code"}; 1846 case 2055403645: /*isBrand*/ return new String[] {"boolean"}; 1847 case -650796023: /*isOverTheCounter*/ return new String[] {"boolean"}; 1848 case -1969347631: /*manufacturer*/ return new String[] {"Reference"}; 1849 case 3148996: /*form*/ return new String[] {"CodeableConcept"}; 1850 case -206409263: /*ingredient*/ return new String[] {}; 1851 case -807062458: /*package*/ return new String[] {}; 1852 case 100313435: /*image*/ return new String[] {"Attachment"}; 1853 default: return super.getTypesForProperty(hash, name); 1854 } 1855 1856 } 1857 1858 @Override 1859 public Base addChild(String name) throws FHIRException { 1860 if (name.equals("code")) { 1861 this.code = new CodeableConcept(); 1862 return this.code; 1863 } 1864 else if (name.equals("status")) { 1865 throw new FHIRException("Cannot call addChild on a singleton property Medication.status"); 1866 } 1867 else if (name.equals("isBrand")) { 1868 throw new FHIRException("Cannot call addChild on a singleton property Medication.isBrand"); 1869 } 1870 else if (name.equals("isOverTheCounter")) { 1871 throw new FHIRException("Cannot call addChild on a singleton property Medication.isOverTheCounter"); 1872 } 1873 else if (name.equals("manufacturer")) { 1874 this.manufacturer = new Reference(); 1875 return this.manufacturer; 1876 } 1877 else if (name.equals("form")) { 1878 this.form = new CodeableConcept(); 1879 return this.form; 1880 } 1881 else if (name.equals("ingredient")) { 1882 return addIngredient(); 1883 } 1884 else if (name.equals("package")) { 1885 this.package_ = new MedicationPackageComponent(); 1886 return this.package_; 1887 } 1888 else if (name.equals("image")) { 1889 return addImage(); 1890 } 1891 else 1892 return super.addChild(name); 1893 } 1894 1895 public String fhirType() { 1896 return "Medication"; 1897 1898 } 1899 1900 public Medication copy() { 1901 Medication dst = new Medication(); 1902 copyValues(dst); 1903 dst.code = code == null ? null : code.copy(); 1904 dst.status = status == null ? null : status.copy(); 1905 dst.isBrand = isBrand == null ? null : isBrand.copy(); 1906 dst.isOverTheCounter = isOverTheCounter == null ? null : isOverTheCounter.copy(); 1907 dst.manufacturer = manufacturer == null ? null : manufacturer.copy(); 1908 dst.form = form == null ? null : form.copy(); 1909 if (ingredient != null) { 1910 dst.ingredient = new ArrayList<MedicationIngredientComponent>(); 1911 for (MedicationIngredientComponent i : ingredient) 1912 dst.ingredient.add(i.copy()); 1913 }; 1914 dst.package_ = package_ == null ? null : package_.copy(); 1915 if (image != null) { 1916 dst.image = new ArrayList<Attachment>(); 1917 for (Attachment i : image) 1918 dst.image.add(i.copy()); 1919 }; 1920 return dst; 1921 } 1922 1923 protected Medication typedCopy() { 1924 return copy(); 1925 } 1926 1927 @Override 1928 public boolean equalsDeep(Base other_) { 1929 if (!super.equalsDeep(other_)) 1930 return false; 1931 if (!(other_ instanceof Medication)) 1932 return false; 1933 Medication o = (Medication) other_; 1934 return compareDeep(code, o.code, true) && compareDeep(status, o.status, true) && compareDeep(isBrand, o.isBrand, true) 1935 && compareDeep(isOverTheCounter, o.isOverTheCounter, true) && compareDeep(manufacturer, o.manufacturer, true) 1936 && compareDeep(form, o.form, true) && compareDeep(ingredient, o.ingredient, true) && compareDeep(package_, o.package_, true) 1937 && compareDeep(image, o.image, true); 1938 } 1939 1940 @Override 1941 public boolean equalsShallow(Base other_) { 1942 if (!super.equalsShallow(other_)) 1943 return false; 1944 if (!(other_ instanceof Medication)) 1945 return false; 1946 Medication o = (Medication) other_; 1947 return compareValues(status, o.status, true) && compareValues(isBrand, o.isBrand, true) && compareValues(isOverTheCounter, o.isOverTheCounter, true) 1948 ; 1949 } 1950 1951 public boolean isEmpty() { 1952 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, status, isBrand, isOverTheCounter 1953 , manufacturer, form, ingredient, package_, image); 1954 } 1955 1956 @Override 1957 public ResourceType getResourceType() { 1958 return ResourceType.Medication; 1959 } 1960 1961 /** 1962 * Search parameter: <b>ingredient-code</b> 1963 * <p> 1964 * Description: <b>The product contained</b><br> 1965 * Type: <b>token</b><br> 1966 * Path: <b>Medication.ingredient.itemCodeableConcept</b><br> 1967 * </p> 1968 */ 1969 @SearchParamDefinition(name="ingredient-code", path="Medication.ingredient.item.as(CodeableConcept)", description="The product contained", type="token" ) 1970 public static final String SP_INGREDIENT_CODE = "ingredient-code"; 1971 /** 1972 * <b>Fluent Client</b> search parameter constant for <b>ingredient-code</b> 1973 * <p> 1974 * Description: <b>The product contained</b><br> 1975 * Type: <b>token</b><br> 1976 * Path: <b>Medication.ingredient.itemCodeableConcept</b><br> 1977 * </p> 1978 */ 1979 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INGREDIENT_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INGREDIENT_CODE); 1980 1981 /** 1982 * Search parameter: <b>container</b> 1983 * <p> 1984 * Description: <b>E.g. box, vial, blister-pack</b><br> 1985 * Type: <b>token</b><br> 1986 * Path: <b>Medication.package.container</b><br> 1987 * </p> 1988 */ 1989 @SearchParamDefinition(name="container", path="Medication.package.container", description="E.g. box, vial, blister-pack", type="token" ) 1990 public static final String SP_CONTAINER = "container"; 1991 /** 1992 * <b>Fluent Client</b> search parameter constant for <b>container</b> 1993 * <p> 1994 * Description: <b>E.g. box, vial, blister-pack</b><br> 1995 * Type: <b>token</b><br> 1996 * Path: <b>Medication.package.container</b><br> 1997 * </p> 1998 */ 1999 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CONTAINER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CONTAINER); 2000 2001 /** 2002 * Search parameter: <b>package-item</b> 2003 * <p> 2004 * Description: <b>The item in the package</b><br> 2005 * Type: <b>reference</b><br> 2006 * Path: <b>Medication.package.content.itemReference</b><br> 2007 * </p> 2008 */ 2009 @SearchParamDefinition(name="package-item", path="Medication.package.content.item.as(Reference)", description="The item in the package", type="reference", target={Medication.class } ) 2010 public static final String SP_PACKAGE_ITEM = "package-item"; 2011 /** 2012 * <b>Fluent Client</b> search parameter constant for <b>package-item</b> 2013 * <p> 2014 * Description: <b>The item in the package</b><br> 2015 * Type: <b>reference</b><br> 2016 * Path: <b>Medication.package.content.itemReference</b><br> 2017 * </p> 2018 */ 2019 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PACKAGE_ITEM = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PACKAGE_ITEM); 2020 2021/** 2022 * Constant for fluent queries to be used to add include statements. Specifies 2023 * the path value of "<b>Medication:package-item</b>". 2024 */ 2025 public static final ca.uhn.fhir.model.api.Include INCLUDE_PACKAGE_ITEM = new ca.uhn.fhir.model.api.Include("Medication:package-item").toLocked(); 2026 2027 /** 2028 * Search parameter: <b>code</b> 2029 * <p> 2030 * Description: <b>Codes that identify this medication</b><br> 2031 * Type: <b>token</b><br> 2032 * Path: <b>Medication.code</b><br> 2033 * </p> 2034 */ 2035 @SearchParamDefinition(name="code", path="Medication.code", description="Codes that identify this medication", type="token" ) 2036 public static final String SP_CODE = "code"; 2037 /** 2038 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2039 * <p> 2040 * Description: <b>Codes that identify this medication</b><br> 2041 * Type: <b>token</b><br> 2042 * Path: <b>Medication.code</b><br> 2043 * </p> 2044 */ 2045 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2046 2047 /** 2048 * Search parameter: <b>ingredient</b> 2049 * <p> 2050 * Description: <b>The product contained</b><br> 2051 * Type: <b>reference</b><br> 2052 * Path: <b>Medication.ingredient.itemReference</b><br> 2053 * </p> 2054 */ 2055 @SearchParamDefinition(name="ingredient", path="Medication.ingredient.item.as(Reference)", description="The product contained", type="reference", target={Medication.class, Substance.class } ) 2056 public static final String SP_INGREDIENT = "ingredient"; 2057 /** 2058 * <b>Fluent Client</b> search parameter constant for <b>ingredient</b> 2059 * <p> 2060 * Description: <b>The product contained</b><br> 2061 * Type: <b>reference</b><br> 2062 * Path: <b>Medication.ingredient.itemReference</b><br> 2063 * </p> 2064 */ 2065 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INGREDIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INGREDIENT); 2066 2067/** 2068 * Constant for fluent queries to be used to add include statements. Specifies 2069 * the path value of "<b>Medication:ingredient</b>". 2070 */ 2071 public static final ca.uhn.fhir.model.api.Include INCLUDE_INGREDIENT = new ca.uhn.fhir.model.api.Include("Medication:ingredient").toLocked(); 2072 2073 /** 2074 * Search parameter: <b>form</b> 2075 * <p> 2076 * Description: <b>powder | tablets | capsule +</b><br> 2077 * Type: <b>token</b><br> 2078 * Path: <b>Medication.form</b><br> 2079 * </p> 2080 */ 2081 @SearchParamDefinition(name="form", path="Medication.form", description="powder | tablets | capsule +", type="token" ) 2082 public static final String SP_FORM = "form"; 2083 /** 2084 * <b>Fluent Client</b> search parameter constant for <b>form</b> 2085 * <p> 2086 * Description: <b>powder | tablets | capsule +</b><br> 2087 * Type: <b>token</b><br> 2088 * Path: <b>Medication.form</b><br> 2089 * </p> 2090 */ 2091 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FORM = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FORM); 2092 2093 /** 2094 * Search parameter: <b>package-item-code</b> 2095 * <p> 2096 * Description: <b>The item in the package</b><br> 2097 * Type: <b>token</b><br> 2098 * Path: <b>Medication.package.content.itemCodeableConcept</b><br> 2099 * </p> 2100 */ 2101 @SearchParamDefinition(name="package-item-code", path="Medication.package.content.item.as(CodeableConcept)", description="The item in the package", type="token" ) 2102 public static final String SP_PACKAGE_ITEM_CODE = "package-item-code"; 2103 /** 2104 * <b>Fluent Client</b> search parameter constant for <b>package-item-code</b> 2105 * <p> 2106 * Description: <b>The item in the package</b><br> 2107 * Type: <b>token</b><br> 2108 * Path: <b>Medication.package.content.itemCodeableConcept</b><br> 2109 * </p> 2110 */ 2111 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PACKAGE_ITEM_CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PACKAGE_ITEM_CODE); 2112 2113 /** 2114 * Search parameter: <b>manufacturer</b> 2115 * <p> 2116 * Description: <b>Manufacturer of the item</b><br> 2117 * Type: <b>reference</b><br> 2118 * Path: <b>Medication.manufacturer</b><br> 2119 * </p> 2120 */ 2121 @SearchParamDefinition(name="manufacturer", path="Medication.manufacturer", description="Manufacturer of the item", type="reference", target={Organization.class } ) 2122 public static final String SP_MANUFACTURER = "manufacturer"; 2123 /** 2124 * <b>Fluent Client</b> search parameter constant for <b>manufacturer</b> 2125 * <p> 2126 * Description: <b>Manufacturer of the item</b><br> 2127 * Type: <b>reference</b><br> 2128 * Path: <b>Medication.manufacturer</b><br> 2129 * </p> 2130 */ 2131 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MANUFACTURER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MANUFACTURER); 2132 2133/** 2134 * Constant for fluent queries to be used to add include statements. Specifies 2135 * the path value of "<b>Medication:manufacturer</b>". 2136 */ 2137 public static final ca.uhn.fhir.model.api.Include INCLUDE_MANUFACTURER = new ca.uhn.fhir.model.api.Include("Medication:manufacturer").toLocked(); 2138 2139 /** 2140 * Search parameter: <b>over-the-counter</b> 2141 * <p> 2142 * Description: <b>True if medication does not require a prescription</b><br> 2143 * Type: <b>token</b><br> 2144 * Path: <b>Medication.isOverTheCounter</b><br> 2145 * </p> 2146 */ 2147 @SearchParamDefinition(name="over-the-counter", path="Medication.isOverTheCounter", description="True if medication does not require a prescription", type="token" ) 2148 public static final String SP_OVER_THE_COUNTER = "over-the-counter"; 2149 /** 2150 * <b>Fluent Client</b> search parameter constant for <b>over-the-counter</b> 2151 * <p> 2152 * Description: <b>True if medication does not require a prescription</b><br> 2153 * Type: <b>token</b><br> 2154 * Path: <b>Medication.isOverTheCounter</b><br> 2155 * </p> 2156 */ 2157 public static final ca.uhn.fhir.rest.gclient.TokenClientParam OVER_THE_COUNTER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_OVER_THE_COUNTER); 2158 2159 /** 2160 * Search parameter: <b>status</b> 2161 * <p> 2162 * Description: <b>active | inactive | entered-in-error</b><br> 2163 * Type: <b>token</b><br> 2164 * Path: <b>Medication.status</b><br> 2165 * </p> 2166 */ 2167 @SearchParamDefinition(name="status", path="Medication.status", description="active | inactive | entered-in-error", type="token" ) 2168 public static final String SP_STATUS = "status"; 2169 /** 2170 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2171 * <p> 2172 * Description: <b>active | inactive | entered-in-error</b><br> 2173 * Type: <b>token</b><br> 2174 * Path: <b>Medication.status</b><br> 2175 * </p> 2176 */ 2177 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2178 2179 2180}