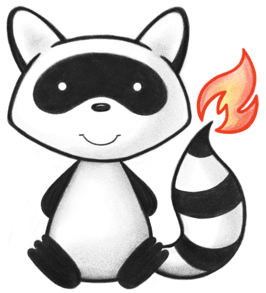
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 042import org.hl7.fhir.utilities.Utilities; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * Describes the event of a patient consuming or otherwise being administered a medication. This may be as simple as swallowing a tablet or it may be a long running infusion. Related resources tie this event to the authorizing prescription, and the specific encounter between patient and health care practitioner. 051 */ 052@ResourceDef(name="MedicationAdministration", profile="http://hl7.org/fhir/Profile/MedicationAdministration") 053public class MedicationAdministration extends DomainResource { 054 055 public enum MedicationAdministrationStatus { 056 /** 057 * The administration has started but has not yet completed. 058 */ 059 INPROGRESS, 060 /** 061 * Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called "suspended". 062 */ 063 ONHOLD, 064 /** 065 * All actions that are implied by the administration have occurred. 066 */ 067 COMPLETED, 068 /** 069 * The administration was entered in error and therefore nullified. 070 */ 071 ENTEREDINERROR, 072 /** 073 * Actions implied by the administration have been permanently halted, before all of them occurred. 074 */ 075 STOPPED, 076 /** 077 * The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for "other" - one of the listed statuses is presumed to apply, it's just not known which one. 078 */ 079 UNKNOWN, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 public static MedicationAdministrationStatus fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("in-progress".equals(codeString)) 088 return INPROGRESS; 089 if ("on-hold".equals(codeString)) 090 return ONHOLD; 091 if ("completed".equals(codeString)) 092 return COMPLETED; 093 if ("entered-in-error".equals(codeString)) 094 return ENTEREDINERROR; 095 if ("stopped".equals(codeString)) 096 return STOPPED; 097 if ("unknown".equals(codeString)) 098 return UNKNOWN; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown MedicationAdministrationStatus code '"+codeString+"'"); 103 } 104 public String toCode() { 105 switch (this) { 106 case INPROGRESS: return "in-progress"; 107 case ONHOLD: return "on-hold"; 108 case COMPLETED: return "completed"; 109 case ENTEREDINERROR: return "entered-in-error"; 110 case STOPPED: return "stopped"; 111 case UNKNOWN: return "unknown"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getSystem() { 117 switch (this) { 118 case INPROGRESS: return "http://hl7.org/fhir/medication-admin-status"; 119 case ONHOLD: return "http://hl7.org/fhir/medication-admin-status"; 120 case COMPLETED: return "http://hl7.org/fhir/medication-admin-status"; 121 case ENTEREDINERROR: return "http://hl7.org/fhir/medication-admin-status"; 122 case STOPPED: return "http://hl7.org/fhir/medication-admin-status"; 123 case UNKNOWN: return "http://hl7.org/fhir/medication-admin-status"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 public String getDefinition() { 129 switch (this) { 130 case INPROGRESS: return "The administration has started but has not yet completed."; 131 case ONHOLD: return "Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 132 case COMPLETED: return "All actions that are implied by the administration have occurred."; 133 case ENTEREDINERROR: return "The administration was entered in error and therefore nullified."; 134 case STOPPED: return "Actions implied by the administration have been permanently halted, before all of them occurred."; 135 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request. Note: This concept is not to be used for \"other\" - one of the listed statuses is presumed to apply, it's just not known which one."; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 public String getDisplay() { 141 switch (this) { 142 case INPROGRESS: return "In Progress"; 143 case ONHOLD: return "On Hold"; 144 case COMPLETED: return "Completed"; 145 case ENTEREDINERROR: return "Entered in Error"; 146 case STOPPED: return "Stopped"; 147 case UNKNOWN: return "Unknown"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 } 153 154 public static class MedicationAdministrationStatusEnumFactory implements EnumFactory<MedicationAdministrationStatus> { 155 public MedicationAdministrationStatus fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("in-progress".equals(codeString)) 160 return MedicationAdministrationStatus.INPROGRESS; 161 if ("on-hold".equals(codeString)) 162 return MedicationAdministrationStatus.ONHOLD; 163 if ("completed".equals(codeString)) 164 return MedicationAdministrationStatus.COMPLETED; 165 if ("entered-in-error".equals(codeString)) 166 return MedicationAdministrationStatus.ENTEREDINERROR; 167 if ("stopped".equals(codeString)) 168 return MedicationAdministrationStatus.STOPPED; 169 if ("unknown".equals(codeString)) 170 return MedicationAdministrationStatus.UNKNOWN; 171 throw new IllegalArgumentException("Unknown MedicationAdministrationStatus code '"+codeString+"'"); 172 } 173 public Enumeration<MedicationAdministrationStatus> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<MedicationAdministrationStatus>(this); 178 String codeString = code.asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("in-progress".equals(codeString)) 182 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.INPROGRESS); 183 if ("on-hold".equals(codeString)) 184 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ONHOLD); 185 if ("completed".equals(codeString)) 186 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.COMPLETED); 187 if ("entered-in-error".equals(codeString)) 188 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.ENTEREDINERROR); 189 if ("stopped".equals(codeString)) 190 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.STOPPED); 191 if ("unknown".equals(codeString)) 192 return new Enumeration<MedicationAdministrationStatus>(this, MedicationAdministrationStatus.UNKNOWN); 193 throw new FHIRException("Unknown MedicationAdministrationStatus code '"+codeString+"'"); 194 } 195 public String toCode(MedicationAdministrationStatus code) { 196 if (code == MedicationAdministrationStatus.NULL) 197 return null; 198 if (code == MedicationAdministrationStatus.INPROGRESS) 199 return "in-progress"; 200 if (code == MedicationAdministrationStatus.ONHOLD) 201 return "on-hold"; 202 if (code == MedicationAdministrationStatus.COMPLETED) 203 return "completed"; 204 if (code == MedicationAdministrationStatus.ENTEREDINERROR) 205 return "entered-in-error"; 206 if (code == MedicationAdministrationStatus.STOPPED) 207 return "stopped"; 208 if (code == MedicationAdministrationStatus.UNKNOWN) 209 return "unknown"; 210 return "?"; 211 } 212 public String toSystem(MedicationAdministrationStatus code) { 213 return code.getSystem(); 214 } 215 } 216 217 @Block() 218 public static class MedicationAdministrationPerformerComponent extends BackboneElement implements IBaseBackboneElement { 219 /** 220 * The device, practitioner, etc. who performed the action. 221 */ 222 @Child(name = "actor", type = {Practitioner.class, Patient.class, RelatedPerson.class, Device.class}, order=1, min=1, max=1, modifier=false, summary=true) 223 @Description(shortDefinition="Individual who was performing", formalDefinition="The device, practitioner, etc. who performed the action." ) 224 protected Reference actor; 225 226 /** 227 * The actual object that is the target of the reference (The device, practitioner, etc. who performed the action.) 228 */ 229 protected Resource actorTarget; 230 231 /** 232 * The organization the device or practitioner was acting on behalf of. 233 */ 234 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=false) 235 @Description(shortDefinition="Organization organization was acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 236 protected Reference onBehalfOf; 237 238 /** 239 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 240 */ 241 protected Organization onBehalfOfTarget; 242 243 private static final long serialVersionUID = -488386403L; 244 245 /** 246 * Constructor 247 */ 248 public MedicationAdministrationPerformerComponent() { 249 super(); 250 } 251 252 /** 253 * Constructor 254 */ 255 public MedicationAdministrationPerformerComponent(Reference actor) { 256 super(); 257 this.actor = actor; 258 } 259 260 /** 261 * @return {@link #actor} (The device, practitioner, etc. who performed the action.) 262 */ 263 public Reference getActor() { 264 if (this.actor == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.actor"); 267 else if (Configuration.doAutoCreate()) 268 this.actor = new Reference(); // cc 269 return this.actor; 270 } 271 272 public boolean hasActor() { 273 return this.actor != null && !this.actor.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #actor} (The device, practitioner, etc. who performed the action.) 278 */ 279 public MedicationAdministrationPerformerComponent setActor(Reference value) { 280 this.actor = value; 281 return this; 282 } 283 284 /** 285 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed the action.) 286 */ 287 public Resource getActorTarget() { 288 return this.actorTarget; 289 } 290 291 /** 292 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed the action.) 293 */ 294 public MedicationAdministrationPerformerComponent setActorTarget(Resource value) { 295 this.actorTarget = value; 296 return this; 297 } 298 299 /** 300 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 301 */ 302 public Reference getOnBehalfOf() { 303 if (this.onBehalfOf == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.onBehalfOf"); 306 else if (Configuration.doAutoCreate()) 307 this.onBehalfOf = new Reference(); // cc 308 return this.onBehalfOf; 309 } 310 311 public boolean hasOnBehalfOf() { 312 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 313 } 314 315 /** 316 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 317 */ 318 public MedicationAdministrationPerformerComponent setOnBehalfOf(Reference value) { 319 this.onBehalfOf = value; 320 return this; 321 } 322 323 /** 324 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 325 */ 326 public Organization getOnBehalfOfTarget() { 327 if (this.onBehalfOfTarget == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create MedicationAdministrationPerformerComponent.onBehalfOf"); 330 else if (Configuration.doAutoCreate()) 331 this.onBehalfOfTarget = new Organization(); // aa 332 return this.onBehalfOfTarget; 333 } 334 335 /** 336 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 337 */ 338 public MedicationAdministrationPerformerComponent setOnBehalfOfTarget(Organization value) { 339 this.onBehalfOfTarget = value; 340 return this; 341 } 342 343 protected void listChildren(List<Property> children) { 344 super.listChildren(children); 345 children.add(new Property("actor", "Reference(Practitioner|Patient|RelatedPerson|Device)", "The device, practitioner, etc. who performed the action.", 0, 1, actor)); 346 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 347 } 348 349 @Override 350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 351 switch (_hash) { 352 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|Patient|RelatedPerson|Device)", "The device, practitioner, etc. who performed the action.", 0, 1, actor); 353 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 354 default: return super.getNamedProperty(_hash, _name, _checkValid); 355 } 356 357 } 358 359 @Override 360 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 361 switch (hash) { 362 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 363 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 364 default: return super.getProperty(hash, name, checkValid); 365 } 366 367 } 368 369 @Override 370 public Base setProperty(int hash, String name, Base value) throws FHIRException { 371 switch (hash) { 372 case 92645877: // actor 373 this.actor = castToReference(value); // Reference 374 return value; 375 case -14402964: // onBehalfOf 376 this.onBehalfOf = castToReference(value); // Reference 377 return value; 378 default: return super.setProperty(hash, name, value); 379 } 380 381 } 382 383 @Override 384 public Base setProperty(String name, Base value) throws FHIRException { 385 if (name.equals("actor")) { 386 this.actor = castToReference(value); // Reference 387 } else if (name.equals("onBehalfOf")) { 388 this.onBehalfOf = castToReference(value); // Reference 389 } else 390 return super.setProperty(name, value); 391 return value; 392 } 393 394 @Override 395 public Base makeProperty(int hash, String name) throws FHIRException { 396 switch (hash) { 397 case 92645877: return getActor(); 398 case -14402964: return getOnBehalfOf(); 399 default: return super.makeProperty(hash, name); 400 } 401 402 } 403 404 @Override 405 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 406 switch (hash) { 407 case 92645877: /*actor*/ return new String[] {"Reference"}; 408 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 409 default: return super.getTypesForProperty(hash, name); 410 } 411 412 } 413 414 @Override 415 public Base addChild(String name) throws FHIRException { 416 if (name.equals("actor")) { 417 this.actor = new Reference(); 418 return this.actor; 419 } 420 else if (name.equals("onBehalfOf")) { 421 this.onBehalfOf = new Reference(); 422 return this.onBehalfOf; 423 } 424 else 425 return super.addChild(name); 426 } 427 428 public MedicationAdministrationPerformerComponent copy() { 429 MedicationAdministrationPerformerComponent dst = new MedicationAdministrationPerformerComponent(); 430 copyValues(dst); 431 dst.actor = actor == null ? null : actor.copy(); 432 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 433 return dst; 434 } 435 436 @Override 437 public boolean equalsDeep(Base other_) { 438 if (!super.equalsDeep(other_)) 439 return false; 440 if (!(other_ instanceof MedicationAdministrationPerformerComponent)) 441 return false; 442 MedicationAdministrationPerformerComponent o = (MedicationAdministrationPerformerComponent) other_; 443 return compareDeep(actor, o.actor, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 444 } 445 446 @Override 447 public boolean equalsShallow(Base other_) { 448 if (!super.equalsShallow(other_)) 449 return false; 450 if (!(other_ instanceof MedicationAdministrationPerformerComponent)) 451 return false; 452 MedicationAdministrationPerformerComponent o = (MedicationAdministrationPerformerComponent) other_; 453 return true; 454 } 455 456 public boolean isEmpty() { 457 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actor, onBehalfOf); 458 } 459 460 public String fhirType() { 461 return "MedicationAdministration.performer"; 462 463 } 464 465 } 466 467 @Block() 468 public static class MedicationAdministrationDosageComponent extends BackboneElement implements IBaseBackboneElement { 469 /** 470 * Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 471 472The dosage instructions should reflect the dosage of the medication that was administered. 473 */ 474 @Child(name = "text", type = {StringType.class}, order=1, min=0, max=1, modifier=false, summary=false) 475 @Description(shortDefinition="Free text dosage instructions e.g. SIG", formalDefinition="Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered." ) 476 protected StringType text; 477 478 /** 479 * A coded specification of the anatomic site where the medication first entered the body. For example, "left arm". 480 */ 481 @Child(name = "site", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 482 @Description(shortDefinition="Body site administered to", formalDefinition="A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\"." ) 483 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/approach-site-codes") 484 protected CodeableConcept site; 485 486 /** 487 * A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc. 488 */ 489 @Child(name = "route", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 490 @Description(shortDefinition="Path of substance into body", formalDefinition="A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc." ) 491 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/route-codes") 492 protected CodeableConcept route; 493 494 /** 495 * A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV. 496 */ 497 @Child(name = "method", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 498 @Description(shortDefinition="How drug was administered", formalDefinition="A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV." ) 499 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/administration-method-codes") 500 protected CodeableConcept method; 501 502 /** 503 * The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection. 504 */ 505 @Child(name = "dose", type = {SimpleQuantity.class}, order=5, min=0, max=1, modifier=false, summary=false) 506 @Description(shortDefinition="Amount of medication per dose", formalDefinition="The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection." ) 507 protected SimpleQuantity dose; 508 509 /** 510 * Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours. 511 */ 512 @Child(name = "rate", type = {Ratio.class, SimpleQuantity.class}, order=6, min=0, max=1, modifier=false, summary=false) 513 @Description(shortDefinition="Dose quantity per unit of time", formalDefinition="Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours." ) 514 protected Type rate; 515 516 private static final long serialVersionUID = 1316915516L; 517 518 /** 519 * Constructor 520 */ 521 public MedicationAdministrationDosageComponent() { 522 super(); 523 } 524 525 /** 526 * @return {@link #text} (Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 527 528The dosage instructions should reflect the dosage of the medication that was administered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 529 */ 530 public StringType getTextElement() { 531 if (this.text == null) 532 if (Configuration.errorOnAutoCreate()) 533 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.text"); 534 else if (Configuration.doAutoCreate()) 535 this.text = new StringType(); // bb 536 return this.text; 537 } 538 539 public boolean hasTextElement() { 540 return this.text != null && !this.text.isEmpty(); 541 } 542 543 public boolean hasText() { 544 return this.text != null && !this.text.isEmpty(); 545 } 546 547 /** 548 * @param value {@link #text} (Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 549 550The dosage instructions should reflect the dosage of the medication that was administered.). This is the underlying object with id, value and extensions. The accessor "getText" gives direct access to the value 551 */ 552 public MedicationAdministrationDosageComponent setTextElement(StringType value) { 553 this.text = value; 554 return this; 555 } 556 557 /** 558 * @return Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 559 560The dosage instructions should reflect the dosage of the medication that was administered. 561 */ 562 public String getText() { 563 return this.text == null ? null : this.text.getValue(); 564 } 565 566 /** 567 * @param value Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans. 568 569The dosage instructions should reflect the dosage of the medication that was administered. 570 */ 571 public MedicationAdministrationDosageComponent setText(String value) { 572 if (Utilities.noString(value)) 573 this.text = null; 574 else { 575 if (this.text == null) 576 this.text = new StringType(); 577 this.text.setValue(value); 578 } 579 return this; 580 } 581 582 /** 583 * @return {@link #site} (A coded specification of the anatomic site where the medication first entered the body. For example, "left arm".) 584 */ 585 public CodeableConcept getSite() { 586 if (this.site == null) 587 if (Configuration.errorOnAutoCreate()) 588 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.site"); 589 else if (Configuration.doAutoCreate()) 590 this.site = new CodeableConcept(); // cc 591 return this.site; 592 } 593 594 public boolean hasSite() { 595 return this.site != null && !this.site.isEmpty(); 596 } 597 598 /** 599 * @param value {@link #site} (A coded specification of the anatomic site where the medication first entered the body. For example, "left arm".) 600 */ 601 public MedicationAdministrationDosageComponent setSite(CodeableConcept value) { 602 this.site = value; 603 return this; 604 } 605 606 /** 607 * @return {@link #route} (A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.) 608 */ 609 public CodeableConcept getRoute() { 610 if (this.route == null) 611 if (Configuration.errorOnAutoCreate()) 612 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.route"); 613 else if (Configuration.doAutoCreate()) 614 this.route = new CodeableConcept(); // cc 615 return this.route; 616 } 617 618 public boolean hasRoute() { 619 return this.route != null && !this.route.isEmpty(); 620 } 621 622 /** 623 * @param value {@link #route} (A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.) 624 */ 625 public MedicationAdministrationDosageComponent setRoute(CodeableConcept value) { 626 this.route = value; 627 return this; 628 } 629 630 /** 631 * @return {@link #method} (A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.) 632 */ 633 public CodeableConcept getMethod() { 634 if (this.method == null) 635 if (Configuration.errorOnAutoCreate()) 636 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.method"); 637 else if (Configuration.doAutoCreate()) 638 this.method = new CodeableConcept(); // cc 639 return this.method; 640 } 641 642 public boolean hasMethod() { 643 return this.method != null && !this.method.isEmpty(); 644 } 645 646 /** 647 * @param value {@link #method} (A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.) 648 */ 649 public MedicationAdministrationDosageComponent setMethod(CodeableConcept value) { 650 this.method = value; 651 return this; 652 } 653 654 /** 655 * @return {@link #dose} (The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.) 656 */ 657 public SimpleQuantity getDose() { 658 if (this.dose == null) 659 if (Configuration.errorOnAutoCreate()) 660 throw new Error("Attempt to auto-create MedicationAdministrationDosageComponent.dose"); 661 else if (Configuration.doAutoCreate()) 662 this.dose = new SimpleQuantity(); // cc 663 return this.dose; 664 } 665 666 public boolean hasDose() { 667 return this.dose != null && !this.dose.isEmpty(); 668 } 669 670 /** 671 * @param value {@link #dose} (The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.) 672 */ 673 public MedicationAdministrationDosageComponent setDose(SimpleQuantity value) { 674 this.dose = value; 675 return this; 676 } 677 678 /** 679 * @return {@link #rate} (Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 680 */ 681 public Type getRate() { 682 return this.rate; 683 } 684 685 /** 686 * @return {@link #rate} (Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 687 */ 688 public Ratio getRateRatio() throws FHIRException { 689 if (this.rate == null) 690 return null; 691 if (!(this.rate instanceof Ratio)) 692 throw new FHIRException("Type mismatch: the type Ratio was expected, but "+this.rate.getClass().getName()+" was encountered"); 693 return (Ratio) this.rate; 694 } 695 696 public boolean hasRateRatio() { 697 return this != null && this.rate instanceof Ratio; 698 } 699 700 /** 701 * @return {@link #rate} (Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 702 */ 703 public SimpleQuantity getRateSimpleQuantity() throws FHIRException { 704 if (this.rate == null) 705 return null; 706 if (!(this.rate instanceof SimpleQuantity)) 707 throw new FHIRException("Type mismatch: the type SimpleQuantity was expected, but "+this.rate.getClass().getName()+" was encountered"); 708 return (SimpleQuantity) this.rate; 709 } 710 711 public boolean hasRateSimpleQuantity() { 712 return this != null && this.rate instanceof SimpleQuantity; 713 } 714 715 public boolean hasRate() { 716 return this.rate != null && !this.rate.isEmpty(); 717 } 718 719 /** 720 * @param value {@link #rate} (Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.) 721 */ 722 public MedicationAdministrationDosageComponent setRate(Type value) throws FHIRFormatError { 723 if (value != null && !(value instanceof Ratio || value instanceof Quantity)) 724 throw new FHIRFormatError("Not the right type for MedicationAdministration.dosage.rate[x]: "+value.fhirType()); 725 this.rate = value; 726 return this; 727 } 728 729 protected void listChildren(List<Property> children) { 730 super.listChildren(children); 731 children.add(new Property("text", "string", "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.", 0, 1, text)); 732 children.add(new Property("site", "CodeableConcept", "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 0, 1, site)); 733 children.add(new Property("route", "CodeableConcept", "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 0, 1, route)); 734 children.add(new Property("method", "CodeableConcept", "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 0, 1, method)); 735 children.add(new Property("dose", "SimpleQuantity", "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 0, 1, dose)); 736 children.add(new Property("rate[x]", "Ratio|SimpleQuantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate)); 737 } 738 739 @Override 740 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 741 switch (_hash) { 742 case 3556653: /*text*/ return new Property("text", "string", "Free text dosage can be used for cases where the dosage administered is too complex to code. When coded dosage is present, the free text dosage may still be present for display to humans.\r\rThe dosage instructions should reflect the dosage of the medication that was administered.", 0, 1, text); 743 case 3530567: /*site*/ return new Property("site", "CodeableConcept", "A coded specification of the anatomic site where the medication first entered the body. For example, \"left arm\".", 0, 1, site); 744 case 108704329: /*route*/ return new Property("route", "CodeableConcept", "A code specifying the route or physiological path of administration of a therapeutic agent into or onto the patient. For example, topical, intravenous, etc.", 0, 1, route); 745 case -1077554975: /*method*/ return new Property("method", "CodeableConcept", "A coded value indicating the method by which the medication is intended to be or was introduced into or on the body. This attribute will most often NOT be populated. It is most commonly used for injections. For example, Slow Push, Deep IV.", 0, 1, method); 746 case 3089437: /*dose*/ return new Property("dose", "SimpleQuantity", "The amount of the medication given at one administration event. Use this value when the administration is essentially an instantaneous event such as a swallowing a tablet or giving an injection.", 0, 1, dose); 747 case 983460768: /*rate[x]*/ return new Property("rate[x]", "Ratio|SimpleQuantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate); 748 case 3493088: /*rate*/ return new Property("rate[x]", "Ratio|SimpleQuantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate); 749 case 204021515: /*rateRatio*/ return new Property("rate[x]", "Ratio|SimpleQuantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate); 750 case -2121057955: /*rateSimpleQuantity*/ return new Property("rate[x]", "Ratio|SimpleQuantity", "Identifies the speed with which the medication was or will be introduced into the patient. Typically the rate for an infusion e.g. 100 ml per 1 hour or 100 ml/hr. May also be expressed as a rate per unit of time e.g. 500 ml per 2 hours. Other examples: 200 mcg/min or 200 mcg/1 minute; 1 liter/8 hours.", 0, 1, rate); 751 default: return super.getNamedProperty(_hash, _name, _checkValid); 752 } 753 754 } 755 756 @Override 757 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 758 switch (hash) { 759 case 3556653: /*text*/ return this.text == null ? new Base[0] : new Base[] {this.text}; // StringType 760 case 3530567: /*site*/ return this.site == null ? new Base[0] : new Base[] {this.site}; // CodeableConcept 761 case 108704329: /*route*/ return this.route == null ? new Base[0] : new Base[] {this.route}; // CodeableConcept 762 case -1077554975: /*method*/ return this.method == null ? new Base[0] : new Base[] {this.method}; // CodeableConcept 763 case 3089437: /*dose*/ return this.dose == null ? new Base[0] : new Base[] {this.dose}; // SimpleQuantity 764 case 3493088: /*rate*/ return this.rate == null ? new Base[0] : new Base[] {this.rate}; // Type 765 default: return super.getProperty(hash, name, checkValid); 766 } 767 768 } 769 770 @Override 771 public Base setProperty(int hash, String name, Base value) throws FHIRException { 772 switch (hash) { 773 case 3556653: // text 774 this.text = castToString(value); // StringType 775 return value; 776 case 3530567: // site 777 this.site = castToCodeableConcept(value); // CodeableConcept 778 return value; 779 case 108704329: // route 780 this.route = castToCodeableConcept(value); // CodeableConcept 781 return value; 782 case -1077554975: // method 783 this.method = castToCodeableConcept(value); // CodeableConcept 784 return value; 785 case 3089437: // dose 786 this.dose = castToSimpleQuantity(value); // SimpleQuantity 787 return value; 788 case 3493088: // rate 789 this.rate = castToType(value); // Type 790 return value; 791 default: return super.setProperty(hash, name, value); 792 } 793 794 } 795 796 @Override 797 public Base setProperty(String name, Base value) throws FHIRException { 798 if (name.equals("text")) { 799 this.text = castToString(value); // StringType 800 } else if (name.equals("site")) { 801 this.site = castToCodeableConcept(value); // CodeableConcept 802 } else if (name.equals("route")) { 803 this.route = castToCodeableConcept(value); // CodeableConcept 804 } else if (name.equals("method")) { 805 this.method = castToCodeableConcept(value); // CodeableConcept 806 } else if (name.equals("dose")) { 807 this.dose = castToSimpleQuantity(value); // SimpleQuantity 808 } else if (name.equals("rate[x]")) { 809 this.rate = castToType(value); // Type 810 } else 811 return super.setProperty(name, value); 812 return value; 813 } 814 815 @Override 816 public Base makeProperty(int hash, String name) throws FHIRException { 817 switch (hash) { 818 case 3556653: return getTextElement(); 819 case 3530567: return getSite(); 820 case 108704329: return getRoute(); 821 case -1077554975: return getMethod(); 822 case 3089437: return getDose(); 823 case 983460768: return getRate(); 824 case 3493088: return getRate(); 825 default: return super.makeProperty(hash, name); 826 } 827 828 } 829 830 @Override 831 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 832 switch (hash) { 833 case 3556653: /*text*/ return new String[] {"string"}; 834 case 3530567: /*site*/ return new String[] {"CodeableConcept"}; 835 case 108704329: /*route*/ return new String[] {"CodeableConcept"}; 836 case -1077554975: /*method*/ return new String[] {"CodeableConcept"}; 837 case 3089437: /*dose*/ return new String[] {"SimpleQuantity"}; 838 case 3493088: /*rate*/ return new String[] {"Ratio", "SimpleQuantity"}; 839 default: return super.getTypesForProperty(hash, name); 840 } 841 842 } 843 844 @Override 845 public Base addChild(String name) throws FHIRException { 846 if (name.equals("text")) { 847 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.text"); 848 } 849 else if (name.equals("site")) { 850 this.site = new CodeableConcept(); 851 return this.site; 852 } 853 else if (name.equals("route")) { 854 this.route = new CodeableConcept(); 855 return this.route; 856 } 857 else if (name.equals("method")) { 858 this.method = new CodeableConcept(); 859 return this.method; 860 } 861 else if (name.equals("dose")) { 862 this.dose = new SimpleQuantity(); 863 return this.dose; 864 } 865 else if (name.equals("rateRatio")) { 866 this.rate = new Ratio(); 867 return this.rate; 868 } 869 else if (name.equals("rateSimpleQuantity")) { 870 this.rate = new SimpleQuantity(); 871 return this.rate; 872 } 873 else 874 return super.addChild(name); 875 } 876 877 public MedicationAdministrationDosageComponent copy() { 878 MedicationAdministrationDosageComponent dst = new MedicationAdministrationDosageComponent(); 879 copyValues(dst); 880 dst.text = text == null ? null : text.copy(); 881 dst.site = site == null ? null : site.copy(); 882 dst.route = route == null ? null : route.copy(); 883 dst.method = method == null ? null : method.copy(); 884 dst.dose = dose == null ? null : dose.copy(); 885 dst.rate = rate == null ? null : rate.copy(); 886 return dst; 887 } 888 889 @Override 890 public boolean equalsDeep(Base other_) { 891 if (!super.equalsDeep(other_)) 892 return false; 893 if (!(other_ instanceof MedicationAdministrationDosageComponent)) 894 return false; 895 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other_; 896 return compareDeep(text, o.text, true) && compareDeep(site, o.site, true) && compareDeep(route, o.route, true) 897 && compareDeep(method, o.method, true) && compareDeep(dose, o.dose, true) && compareDeep(rate, o.rate, true) 898 ; 899 } 900 901 @Override 902 public boolean equalsShallow(Base other_) { 903 if (!super.equalsShallow(other_)) 904 return false; 905 if (!(other_ instanceof MedicationAdministrationDosageComponent)) 906 return false; 907 MedicationAdministrationDosageComponent o = (MedicationAdministrationDosageComponent) other_; 908 return compareValues(text, o.text, true); 909 } 910 911 public boolean isEmpty() { 912 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(text, site, route, method 913 , dose, rate); 914 } 915 916 public String fhirType() { 917 return "MedicationAdministration.dosage"; 918 919 } 920 921 } 922 923 /** 924 * External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated. 925 */ 926 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 927 @Description(shortDefinition="External identifier", formalDefinition="External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated." ) 928 protected List<Identifier> identifier; 929 930 /** 931 * A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this event. 932 */ 933 @Child(name = "definition", type = {PlanDefinition.class, ActivityDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 934 @Description(shortDefinition="Instantiates protocol or definition", formalDefinition="A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this event." ) 935 protected List<Reference> definition; 936 /** 937 * The actual objects that are the target of the reference (A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this event.) 938 */ 939 protected List<Resource> definitionTarget; 940 941 942 /** 943 * A larger event of which this particular event is a component or step. 944 */ 945 @Child(name = "partOf", type = {MedicationAdministration.class, Procedure.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 946 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 947 protected List<Reference> partOf; 948 /** 949 * The actual objects that are the target of the reference (A larger event of which this particular event is a component or step.) 950 */ 951 protected List<Resource> partOfTarget; 952 953 954 /** 955 * Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way. 956 */ 957 @Child(name = "status", type = {CodeType.class}, order=3, min=1, max=1, modifier=true, summary=true) 958 @Description(shortDefinition="in-progress | on-hold | completed | entered-in-error | stopped | unknown", formalDefinition="Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way." ) 959 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-admin-status") 960 protected Enumeration<MedicationAdministrationStatus> status; 961 962 /** 963 * Indicates the type of medication administration and where the medication is expected to be consumed or administered. 964 */ 965 @Child(name = "category", type = {CodeableConcept.class}, order=4, min=0, max=1, modifier=false, summary=false) 966 @Description(shortDefinition="Type of medication usage", formalDefinition="Indicates the type of medication administration and where the medication is expected to be consumed or administered." ) 967 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-admin-category") 968 protected CodeableConcept category; 969 970 /** 971 * Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications. 972 */ 973 @Child(name = "medication", type = {CodeableConcept.class, Medication.class}, order=5, min=1, max=1, modifier=false, summary=true) 974 @Description(shortDefinition="What was administered", formalDefinition="Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications." ) 975 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 976 protected Type medication; 977 978 /** 979 * The person or animal or group receiving the medication. 980 */ 981 @Child(name = "subject", type = {Patient.class, Group.class}, order=6, min=1, max=1, modifier=false, summary=true) 982 @Description(shortDefinition="Who received medication", formalDefinition="The person or animal or group receiving the medication." ) 983 protected Reference subject; 984 985 /** 986 * The actual object that is the target of the reference (The person or animal or group receiving the medication.) 987 */ 988 protected Resource subjectTarget; 989 990 /** 991 * The visit, admission or other contact between patient and health care provider the medication administration was performed as part of. 992 */ 993 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=7, min=0, max=1, modifier=false, summary=false) 994 @Description(shortDefinition="Encounter or Episode of Care administered as part of", formalDefinition="The visit, admission or other contact between patient and health care provider the medication administration was performed as part of." ) 995 protected Reference context; 996 997 /** 998 * The actual object that is the target of the reference (The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.) 999 */ 1000 protected Resource contextTarget; 1001 1002 /** 1003 * Additional information (for example, patient height and weight) that supports the administration of the medication. 1004 */ 1005 @Child(name = "supportingInformation", type = {Reference.class}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1006 @Description(shortDefinition="Additional information to support administration", formalDefinition="Additional information (for example, patient height and weight) that supports the administration of the medication." ) 1007 protected List<Reference> supportingInformation; 1008 /** 1009 * The actual objects that are the target of the reference (Additional information (for example, patient height and weight) that supports the administration of the medication.) 1010 */ 1011 protected List<Resource> supportingInformationTarget; 1012 1013 1014 /** 1015 * A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate. 1016 */ 1017 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=9, min=1, max=1, modifier=false, summary=true) 1018 @Description(shortDefinition="Start and end time of administration", formalDefinition="A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate." ) 1019 protected Type effective; 1020 1021 /** 1022 * The individual who was responsible for giving the medication to the patient. 1023 */ 1024 @Child(name = "performer", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1025 @Description(shortDefinition="Who administered substance", formalDefinition="The individual who was responsible for giving the medication to the patient." ) 1026 protected List<MedicationAdministrationPerformerComponent> performer; 1027 1028 /** 1029 * Set this to true if the record is saying that the medication was NOT administered. 1030 */ 1031 @Child(name = "notGiven", type = {BooleanType.class}, order=11, min=0, max=1, modifier=true, summary=true) 1032 @Description(shortDefinition="True if medication not administered", formalDefinition="Set this to true if the record is saying that the medication was NOT administered." ) 1033 protected BooleanType notGiven; 1034 1035 /** 1036 * A code indicating why the administration was not performed. 1037 */ 1038 @Child(name = "reasonNotGiven", type = {CodeableConcept.class}, order=12, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1039 @Description(shortDefinition="Reason administration not performed", formalDefinition="A code indicating why the administration was not performed." ) 1040 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reason-medication-not-given-codes") 1041 protected List<CodeableConcept> reasonNotGiven; 1042 1043 /** 1044 * A code indicating why the medication was given. 1045 */ 1046 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1047 @Description(shortDefinition="Reason administration performed", formalDefinition="A code indicating why the medication was given." ) 1048 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reason-medication-given-codes") 1049 protected List<CodeableConcept> reasonCode; 1050 1051 /** 1052 * Condition or observation that supports why the medication was administered. 1053 */ 1054 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1055 @Description(shortDefinition="Condition or Observation that supports why the medication was administered", formalDefinition="Condition or observation that supports why the medication was administered." ) 1056 protected List<Reference> reasonReference; 1057 /** 1058 * The actual objects that are the target of the reference (Condition or observation that supports why the medication was administered.) 1059 */ 1060 protected List<Resource> reasonReferenceTarget; 1061 1062 1063 /** 1064 * The original request, instruction or authority to perform the administration. 1065 */ 1066 @Child(name = "prescription", type = {MedicationRequest.class}, order=15, min=0, max=1, modifier=false, summary=false) 1067 @Description(shortDefinition="Request administration performed against", formalDefinition="The original request, instruction or authority to perform the administration." ) 1068 protected Reference prescription; 1069 1070 /** 1071 * The actual object that is the target of the reference (The original request, instruction or authority to perform the administration.) 1072 */ 1073 protected MedicationRequest prescriptionTarget; 1074 1075 /** 1076 * The device used in administering the medication to the patient. For example, a particular infusion pump. 1077 */ 1078 @Child(name = "device", type = {Device.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1079 @Description(shortDefinition="Device used to administer", formalDefinition="The device used in administering the medication to the patient. For example, a particular infusion pump." ) 1080 protected List<Reference> device; 1081 /** 1082 * The actual objects that are the target of the reference (The device used in administering the medication to the patient. For example, a particular infusion pump.) 1083 */ 1084 protected List<Device> deviceTarget; 1085 1086 1087 /** 1088 * Extra information about the medication administration that is not conveyed by the other attributes. 1089 */ 1090 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1091 @Description(shortDefinition="Information about the administration", formalDefinition="Extra information about the medication administration that is not conveyed by the other attributes." ) 1092 protected List<Annotation> note; 1093 1094 /** 1095 * Describes the medication dosage information details e.g. dose, rate, site, route, etc. 1096 */ 1097 @Child(name = "dosage", type = {}, order=18, min=0, max=1, modifier=false, summary=false) 1098 @Description(shortDefinition="Details of how medication was taken", formalDefinition="Describes the medication dosage information details e.g. dose, rate, site, route, etc." ) 1099 protected MedicationAdministrationDosageComponent dosage; 1100 1101 /** 1102 * A summary of the events of interest that have occurred, such as when the administration was verified. 1103 */ 1104 @Child(name = "eventHistory", type = {Provenance.class}, order=19, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1105 @Description(shortDefinition="A list of events of interest in the lifecycle", formalDefinition="A summary of the events of interest that have occurred, such as when the administration was verified." ) 1106 protected List<Reference> eventHistory; 1107 /** 1108 * The actual objects that are the target of the reference (A summary of the events of interest that have occurred, such as when the administration was verified.) 1109 */ 1110 protected List<Provenance> eventHistoryTarget; 1111 1112 1113 private static final long serialVersionUID = 673777544L; 1114 1115 /** 1116 * Constructor 1117 */ 1118 public MedicationAdministration() { 1119 super(); 1120 } 1121 1122 /** 1123 * Constructor 1124 */ 1125 public MedicationAdministration(Enumeration<MedicationAdministrationStatus> status, Type medication, Reference subject, Type effective) { 1126 super(); 1127 this.status = status; 1128 this.medication = medication; 1129 this.subject = subject; 1130 this.effective = effective; 1131 } 1132 1133 /** 1134 * @return {@link #identifier} (External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.) 1135 */ 1136 public List<Identifier> getIdentifier() { 1137 if (this.identifier == null) 1138 this.identifier = new ArrayList<Identifier>(); 1139 return this.identifier; 1140 } 1141 1142 /** 1143 * @return Returns a reference to <code>this</code> for easy method chaining 1144 */ 1145 public MedicationAdministration setIdentifier(List<Identifier> theIdentifier) { 1146 this.identifier = theIdentifier; 1147 return this; 1148 } 1149 1150 public boolean hasIdentifier() { 1151 if (this.identifier == null) 1152 return false; 1153 for (Identifier item : this.identifier) 1154 if (!item.isEmpty()) 1155 return true; 1156 return false; 1157 } 1158 1159 public Identifier addIdentifier() { //3 1160 Identifier t = new Identifier(); 1161 if (this.identifier == null) 1162 this.identifier = new ArrayList<Identifier>(); 1163 this.identifier.add(t); 1164 return t; 1165 } 1166 1167 public MedicationAdministration addIdentifier(Identifier t) { //3 1168 if (t == null) 1169 return this; 1170 if (this.identifier == null) 1171 this.identifier = new ArrayList<Identifier>(); 1172 this.identifier.add(t); 1173 return this; 1174 } 1175 1176 /** 1177 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1178 */ 1179 public Identifier getIdentifierFirstRep() { 1180 if (getIdentifier().isEmpty()) { 1181 addIdentifier(); 1182 } 1183 return getIdentifier().get(0); 1184 } 1185 1186 /** 1187 * @return {@link #definition} (A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this event.) 1188 */ 1189 public List<Reference> getDefinition() { 1190 if (this.definition == null) 1191 this.definition = new ArrayList<Reference>(); 1192 return this.definition; 1193 } 1194 1195 /** 1196 * @return Returns a reference to <code>this</code> for easy method chaining 1197 */ 1198 public MedicationAdministration setDefinition(List<Reference> theDefinition) { 1199 this.definition = theDefinition; 1200 return this; 1201 } 1202 1203 public boolean hasDefinition() { 1204 if (this.definition == null) 1205 return false; 1206 for (Reference item : this.definition) 1207 if (!item.isEmpty()) 1208 return true; 1209 return false; 1210 } 1211 1212 public Reference addDefinition() { //3 1213 Reference t = new Reference(); 1214 if (this.definition == null) 1215 this.definition = new ArrayList<Reference>(); 1216 this.definition.add(t); 1217 return t; 1218 } 1219 1220 public MedicationAdministration addDefinition(Reference t) { //3 1221 if (t == null) 1222 return this; 1223 if (this.definition == null) 1224 this.definition = new ArrayList<Reference>(); 1225 this.definition.add(t); 1226 return this; 1227 } 1228 1229 /** 1230 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 1231 */ 1232 public Reference getDefinitionFirstRep() { 1233 if (getDefinition().isEmpty()) { 1234 addDefinition(); 1235 } 1236 return getDefinition().get(0); 1237 } 1238 1239 /** 1240 * @deprecated Use Reference#setResource(IBaseResource) instead 1241 */ 1242 @Deprecated 1243 public List<Resource> getDefinitionTarget() { 1244 if (this.definitionTarget == null) 1245 this.definitionTarget = new ArrayList<Resource>(); 1246 return this.definitionTarget; 1247 } 1248 1249 /** 1250 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 1251 */ 1252 public List<Reference> getPartOf() { 1253 if (this.partOf == null) 1254 this.partOf = new ArrayList<Reference>(); 1255 return this.partOf; 1256 } 1257 1258 /** 1259 * @return Returns a reference to <code>this</code> for easy method chaining 1260 */ 1261 public MedicationAdministration setPartOf(List<Reference> thePartOf) { 1262 this.partOf = thePartOf; 1263 return this; 1264 } 1265 1266 public boolean hasPartOf() { 1267 if (this.partOf == null) 1268 return false; 1269 for (Reference item : this.partOf) 1270 if (!item.isEmpty()) 1271 return true; 1272 return false; 1273 } 1274 1275 public Reference addPartOf() { //3 1276 Reference t = new Reference(); 1277 if (this.partOf == null) 1278 this.partOf = new ArrayList<Reference>(); 1279 this.partOf.add(t); 1280 return t; 1281 } 1282 1283 public MedicationAdministration addPartOf(Reference t) { //3 1284 if (t == null) 1285 return this; 1286 if (this.partOf == null) 1287 this.partOf = new ArrayList<Reference>(); 1288 this.partOf.add(t); 1289 return this; 1290 } 1291 1292 /** 1293 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 1294 */ 1295 public Reference getPartOfFirstRep() { 1296 if (getPartOf().isEmpty()) { 1297 addPartOf(); 1298 } 1299 return getPartOf().get(0); 1300 } 1301 1302 /** 1303 * @deprecated Use Reference#setResource(IBaseResource) instead 1304 */ 1305 @Deprecated 1306 public List<Resource> getPartOfTarget() { 1307 if (this.partOfTarget == null) 1308 this.partOfTarget = new ArrayList<Resource>(); 1309 return this.partOfTarget; 1310 } 1311 1312 /** 1313 * @return {@link #status} (Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1314 */ 1315 public Enumeration<MedicationAdministrationStatus> getStatusElement() { 1316 if (this.status == null) 1317 if (Configuration.errorOnAutoCreate()) 1318 throw new Error("Attempt to auto-create MedicationAdministration.status"); 1319 else if (Configuration.doAutoCreate()) 1320 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); // bb 1321 return this.status; 1322 } 1323 1324 public boolean hasStatusElement() { 1325 return this.status != null && !this.status.isEmpty(); 1326 } 1327 1328 public boolean hasStatus() { 1329 return this.status != null && !this.status.isEmpty(); 1330 } 1331 1332 /** 1333 * @param value {@link #status} (Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1334 */ 1335 public MedicationAdministration setStatusElement(Enumeration<MedicationAdministrationStatus> value) { 1336 this.status = value; 1337 return this; 1338 } 1339 1340 /** 1341 * @return Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way. 1342 */ 1343 public MedicationAdministrationStatus getStatus() { 1344 return this.status == null ? null : this.status.getValue(); 1345 } 1346 1347 /** 1348 * @param value Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way. 1349 */ 1350 public MedicationAdministration setStatus(MedicationAdministrationStatus value) { 1351 if (this.status == null) 1352 this.status = new Enumeration<MedicationAdministrationStatus>(new MedicationAdministrationStatusEnumFactory()); 1353 this.status.setValue(value); 1354 return this; 1355 } 1356 1357 /** 1358 * @return {@link #category} (Indicates the type of medication administration and where the medication is expected to be consumed or administered.) 1359 */ 1360 public CodeableConcept getCategory() { 1361 if (this.category == null) 1362 if (Configuration.errorOnAutoCreate()) 1363 throw new Error("Attempt to auto-create MedicationAdministration.category"); 1364 else if (Configuration.doAutoCreate()) 1365 this.category = new CodeableConcept(); // cc 1366 return this.category; 1367 } 1368 1369 public boolean hasCategory() { 1370 return this.category != null && !this.category.isEmpty(); 1371 } 1372 1373 /** 1374 * @param value {@link #category} (Indicates the type of medication administration and where the medication is expected to be consumed or administered.) 1375 */ 1376 public MedicationAdministration setCategory(CodeableConcept value) { 1377 this.category = value; 1378 return this; 1379 } 1380 1381 /** 1382 * @return {@link #medication} (Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1383 */ 1384 public Type getMedication() { 1385 return this.medication; 1386 } 1387 1388 /** 1389 * @return {@link #medication} (Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1390 */ 1391 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1392 if (this.medication == null) 1393 return null; 1394 if (!(this.medication instanceof CodeableConcept)) 1395 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.medication.getClass().getName()+" was encountered"); 1396 return (CodeableConcept) this.medication; 1397 } 1398 1399 public boolean hasMedicationCodeableConcept() { 1400 return this != null && this.medication instanceof CodeableConcept; 1401 } 1402 1403 /** 1404 * @return {@link #medication} (Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1405 */ 1406 public Reference getMedicationReference() throws FHIRException { 1407 if (this.medication == null) 1408 return null; 1409 if (!(this.medication instanceof Reference)) 1410 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.medication.getClass().getName()+" was encountered"); 1411 return (Reference) this.medication; 1412 } 1413 1414 public boolean hasMedicationReference() { 1415 return this != null && this.medication instanceof Reference; 1416 } 1417 1418 public boolean hasMedication() { 1419 return this.medication != null && !this.medication.isEmpty(); 1420 } 1421 1422 /** 1423 * @param value {@link #medication} (Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1424 */ 1425 public MedicationAdministration setMedication(Type value) throws FHIRFormatError { 1426 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1427 throw new FHIRFormatError("Not the right type for MedicationAdministration.medication[x]: "+value.fhirType()); 1428 this.medication = value; 1429 return this; 1430 } 1431 1432 /** 1433 * @return {@link #subject} (The person or animal or group receiving the medication.) 1434 */ 1435 public Reference getSubject() { 1436 if (this.subject == null) 1437 if (Configuration.errorOnAutoCreate()) 1438 throw new Error("Attempt to auto-create MedicationAdministration.subject"); 1439 else if (Configuration.doAutoCreate()) 1440 this.subject = new Reference(); // cc 1441 return this.subject; 1442 } 1443 1444 public boolean hasSubject() { 1445 return this.subject != null && !this.subject.isEmpty(); 1446 } 1447 1448 /** 1449 * @param value {@link #subject} (The person or animal or group receiving the medication.) 1450 */ 1451 public MedicationAdministration setSubject(Reference value) { 1452 this.subject = value; 1453 return this; 1454 } 1455 1456 /** 1457 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person or animal or group receiving the medication.) 1458 */ 1459 public Resource getSubjectTarget() { 1460 return this.subjectTarget; 1461 } 1462 1463 /** 1464 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person or animal or group receiving the medication.) 1465 */ 1466 public MedicationAdministration setSubjectTarget(Resource value) { 1467 this.subjectTarget = value; 1468 return this; 1469 } 1470 1471 /** 1472 * @return {@link #context} (The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.) 1473 */ 1474 public Reference getContext() { 1475 if (this.context == null) 1476 if (Configuration.errorOnAutoCreate()) 1477 throw new Error("Attempt to auto-create MedicationAdministration.context"); 1478 else if (Configuration.doAutoCreate()) 1479 this.context = new Reference(); // cc 1480 return this.context; 1481 } 1482 1483 public boolean hasContext() { 1484 return this.context != null && !this.context.isEmpty(); 1485 } 1486 1487 /** 1488 * @param value {@link #context} (The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.) 1489 */ 1490 public MedicationAdministration setContext(Reference value) { 1491 this.context = value; 1492 return this; 1493 } 1494 1495 /** 1496 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.) 1497 */ 1498 public Resource getContextTarget() { 1499 return this.contextTarget; 1500 } 1501 1502 /** 1503 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.) 1504 */ 1505 public MedicationAdministration setContextTarget(Resource value) { 1506 this.contextTarget = value; 1507 return this; 1508 } 1509 1510 /** 1511 * @return {@link #supportingInformation} (Additional information (for example, patient height and weight) that supports the administration of the medication.) 1512 */ 1513 public List<Reference> getSupportingInformation() { 1514 if (this.supportingInformation == null) 1515 this.supportingInformation = new ArrayList<Reference>(); 1516 return this.supportingInformation; 1517 } 1518 1519 /** 1520 * @return Returns a reference to <code>this</code> for easy method chaining 1521 */ 1522 public MedicationAdministration setSupportingInformation(List<Reference> theSupportingInformation) { 1523 this.supportingInformation = theSupportingInformation; 1524 return this; 1525 } 1526 1527 public boolean hasSupportingInformation() { 1528 if (this.supportingInformation == null) 1529 return false; 1530 for (Reference item : this.supportingInformation) 1531 if (!item.isEmpty()) 1532 return true; 1533 return false; 1534 } 1535 1536 public Reference addSupportingInformation() { //3 1537 Reference t = new Reference(); 1538 if (this.supportingInformation == null) 1539 this.supportingInformation = new ArrayList<Reference>(); 1540 this.supportingInformation.add(t); 1541 return t; 1542 } 1543 1544 public MedicationAdministration addSupportingInformation(Reference t) { //3 1545 if (t == null) 1546 return this; 1547 if (this.supportingInformation == null) 1548 this.supportingInformation = new ArrayList<Reference>(); 1549 this.supportingInformation.add(t); 1550 return this; 1551 } 1552 1553 /** 1554 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist 1555 */ 1556 public Reference getSupportingInformationFirstRep() { 1557 if (getSupportingInformation().isEmpty()) { 1558 addSupportingInformation(); 1559 } 1560 return getSupportingInformation().get(0); 1561 } 1562 1563 /** 1564 * @deprecated Use Reference#setResource(IBaseResource) instead 1565 */ 1566 @Deprecated 1567 public List<Resource> getSupportingInformationTarget() { 1568 if (this.supportingInformationTarget == null) 1569 this.supportingInformationTarget = new ArrayList<Resource>(); 1570 return this.supportingInformationTarget; 1571 } 1572 1573 /** 1574 * @return {@link #effective} (A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1575 */ 1576 public Type getEffective() { 1577 return this.effective; 1578 } 1579 1580 /** 1581 * @return {@link #effective} (A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1582 */ 1583 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 1584 if (this.effective == null) 1585 return null; 1586 if (!(this.effective instanceof DateTimeType)) 1587 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 1588 return (DateTimeType) this.effective; 1589 } 1590 1591 public boolean hasEffectiveDateTimeType() { 1592 return this != null && this.effective instanceof DateTimeType; 1593 } 1594 1595 /** 1596 * @return {@link #effective} (A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1597 */ 1598 public Period getEffectivePeriod() throws FHIRException { 1599 if (this.effective == null) 1600 return null; 1601 if (!(this.effective instanceof Period)) 1602 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 1603 return (Period) this.effective; 1604 } 1605 1606 public boolean hasEffectivePeriod() { 1607 return this != null && this.effective instanceof Period; 1608 } 1609 1610 public boolean hasEffective() { 1611 return this.effective != null && !this.effective.isEmpty(); 1612 } 1613 1614 /** 1615 * @param value {@link #effective} (A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.) 1616 */ 1617 public MedicationAdministration setEffective(Type value) throws FHIRFormatError { 1618 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 1619 throw new FHIRFormatError("Not the right type for MedicationAdministration.effective[x]: "+value.fhirType()); 1620 this.effective = value; 1621 return this; 1622 } 1623 1624 /** 1625 * @return {@link #performer} (The individual who was responsible for giving the medication to the patient.) 1626 */ 1627 public List<MedicationAdministrationPerformerComponent> getPerformer() { 1628 if (this.performer == null) 1629 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 1630 return this.performer; 1631 } 1632 1633 /** 1634 * @return Returns a reference to <code>this</code> for easy method chaining 1635 */ 1636 public MedicationAdministration setPerformer(List<MedicationAdministrationPerformerComponent> thePerformer) { 1637 this.performer = thePerformer; 1638 return this; 1639 } 1640 1641 public boolean hasPerformer() { 1642 if (this.performer == null) 1643 return false; 1644 for (MedicationAdministrationPerformerComponent item : this.performer) 1645 if (!item.isEmpty()) 1646 return true; 1647 return false; 1648 } 1649 1650 public MedicationAdministrationPerformerComponent addPerformer() { //3 1651 MedicationAdministrationPerformerComponent t = new MedicationAdministrationPerformerComponent(); 1652 if (this.performer == null) 1653 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 1654 this.performer.add(t); 1655 return t; 1656 } 1657 1658 public MedicationAdministration addPerformer(MedicationAdministrationPerformerComponent t) { //3 1659 if (t == null) 1660 return this; 1661 if (this.performer == null) 1662 this.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 1663 this.performer.add(t); 1664 return this; 1665 } 1666 1667 /** 1668 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 1669 */ 1670 public MedicationAdministrationPerformerComponent getPerformerFirstRep() { 1671 if (getPerformer().isEmpty()) { 1672 addPerformer(); 1673 } 1674 return getPerformer().get(0); 1675 } 1676 1677 /** 1678 * @return {@link #notGiven} (Set this to true if the record is saying that the medication was NOT administered.). This is the underlying object with id, value and extensions. The accessor "getNotGiven" gives direct access to the value 1679 */ 1680 public BooleanType getNotGivenElement() { 1681 if (this.notGiven == null) 1682 if (Configuration.errorOnAutoCreate()) 1683 throw new Error("Attempt to auto-create MedicationAdministration.notGiven"); 1684 else if (Configuration.doAutoCreate()) 1685 this.notGiven = new BooleanType(); // bb 1686 return this.notGiven; 1687 } 1688 1689 public boolean hasNotGivenElement() { 1690 return this.notGiven != null && !this.notGiven.isEmpty(); 1691 } 1692 1693 public boolean hasNotGiven() { 1694 return this.notGiven != null && !this.notGiven.isEmpty(); 1695 } 1696 1697 /** 1698 * @param value {@link #notGiven} (Set this to true if the record is saying that the medication was NOT administered.). This is the underlying object with id, value and extensions. The accessor "getNotGiven" gives direct access to the value 1699 */ 1700 public MedicationAdministration setNotGivenElement(BooleanType value) { 1701 this.notGiven = value; 1702 return this; 1703 } 1704 1705 /** 1706 * @return Set this to true if the record is saying that the medication was NOT administered. 1707 */ 1708 public boolean getNotGiven() { 1709 return this.notGiven == null || this.notGiven.isEmpty() ? false : this.notGiven.getValue(); 1710 } 1711 1712 /** 1713 * @param value Set this to true if the record is saying that the medication was NOT administered. 1714 */ 1715 public MedicationAdministration setNotGiven(boolean value) { 1716 if (this.notGiven == null) 1717 this.notGiven = new BooleanType(); 1718 this.notGiven.setValue(value); 1719 return this; 1720 } 1721 1722 /** 1723 * @return {@link #reasonNotGiven} (A code indicating why the administration was not performed.) 1724 */ 1725 public List<CodeableConcept> getReasonNotGiven() { 1726 if (this.reasonNotGiven == null) 1727 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1728 return this.reasonNotGiven; 1729 } 1730 1731 /** 1732 * @return Returns a reference to <code>this</code> for easy method chaining 1733 */ 1734 public MedicationAdministration setReasonNotGiven(List<CodeableConcept> theReasonNotGiven) { 1735 this.reasonNotGiven = theReasonNotGiven; 1736 return this; 1737 } 1738 1739 public boolean hasReasonNotGiven() { 1740 if (this.reasonNotGiven == null) 1741 return false; 1742 for (CodeableConcept item : this.reasonNotGiven) 1743 if (!item.isEmpty()) 1744 return true; 1745 return false; 1746 } 1747 1748 public CodeableConcept addReasonNotGiven() { //3 1749 CodeableConcept t = new CodeableConcept(); 1750 if (this.reasonNotGiven == null) 1751 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1752 this.reasonNotGiven.add(t); 1753 return t; 1754 } 1755 1756 public MedicationAdministration addReasonNotGiven(CodeableConcept t) { //3 1757 if (t == null) 1758 return this; 1759 if (this.reasonNotGiven == null) 1760 this.reasonNotGiven = new ArrayList<CodeableConcept>(); 1761 this.reasonNotGiven.add(t); 1762 return this; 1763 } 1764 1765 /** 1766 * @return The first repetition of repeating field {@link #reasonNotGiven}, creating it if it does not already exist 1767 */ 1768 public CodeableConcept getReasonNotGivenFirstRep() { 1769 if (getReasonNotGiven().isEmpty()) { 1770 addReasonNotGiven(); 1771 } 1772 return getReasonNotGiven().get(0); 1773 } 1774 1775 /** 1776 * @return {@link #reasonCode} (A code indicating why the medication was given.) 1777 */ 1778 public List<CodeableConcept> getReasonCode() { 1779 if (this.reasonCode == null) 1780 this.reasonCode = new ArrayList<CodeableConcept>(); 1781 return this.reasonCode; 1782 } 1783 1784 /** 1785 * @return Returns a reference to <code>this</code> for easy method chaining 1786 */ 1787 public MedicationAdministration setReasonCode(List<CodeableConcept> theReasonCode) { 1788 this.reasonCode = theReasonCode; 1789 return this; 1790 } 1791 1792 public boolean hasReasonCode() { 1793 if (this.reasonCode == null) 1794 return false; 1795 for (CodeableConcept item : this.reasonCode) 1796 if (!item.isEmpty()) 1797 return true; 1798 return false; 1799 } 1800 1801 public CodeableConcept addReasonCode() { //3 1802 CodeableConcept t = new CodeableConcept(); 1803 if (this.reasonCode == null) 1804 this.reasonCode = new ArrayList<CodeableConcept>(); 1805 this.reasonCode.add(t); 1806 return t; 1807 } 1808 1809 public MedicationAdministration addReasonCode(CodeableConcept t) { //3 1810 if (t == null) 1811 return this; 1812 if (this.reasonCode == null) 1813 this.reasonCode = new ArrayList<CodeableConcept>(); 1814 this.reasonCode.add(t); 1815 return this; 1816 } 1817 1818 /** 1819 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1820 */ 1821 public CodeableConcept getReasonCodeFirstRep() { 1822 if (getReasonCode().isEmpty()) { 1823 addReasonCode(); 1824 } 1825 return getReasonCode().get(0); 1826 } 1827 1828 /** 1829 * @return {@link #reasonReference} (Condition or observation that supports why the medication was administered.) 1830 */ 1831 public List<Reference> getReasonReference() { 1832 if (this.reasonReference == null) 1833 this.reasonReference = new ArrayList<Reference>(); 1834 return this.reasonReference; 1835 } 1836 1837 /** 1838 * @return Returns a reference to <code>this</code> for easy method chaining 1839 */ 1840 public MedicationAdministration setReasonReference(List<Reference> theReasonReference) { 1841 this.reasonReference = theReasonReference; 1842 return this; 1843 } 1844 1845 public boolean hasReasonReference() { 1846 if (this.reasonReference == null) 1847 return false; 1848 for (Reference item : this.reasonReference) 1849 if (!item.isEmpty()) 1850 return true; 1851 return false; 1852 } 1853 1854 public Reference addReasonReference() { //3 1855 Reference t = new Reference(); 1856 if (this.reasonReference == null) 1857 this.reasonReference = new ArrayList<Reference>(); 1858 this.reasonReference.add(t); 1859 return t; 1860 } 1861 1862 public MedicationAdministration addReasonReference(Reference t) { //3 1863 if (t == null) 1864 return this; 1865 if (this.reasonReference == null) 1866 this.reasonReference = new ArrayList<Reference>(); 1867 this.reasonReference.add(t); 1868 return this; 1869 } 1870 1871 /** 1872 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1873 */ 1874 public Reference getReasonReferenceFirstRep() { 1875 if (getReasonReference().isEmpty()) { 1876 addReasonReference(); 1877 } 1878 return getReasonReference().get(0); 1879 } 1880 1881 /** 1882 * @deprecated Use Reference#setResource(IBaseResource) instead 1883 */ 1884 @Deprecated 1885 public List<Resource> getReasonReferenceTarget() { 1886 if (this.reasonReferenceTarget == null) 1887 this.reasonReferenceTarget = new ArrayList<Resource>(); 1888 return this.reasonReferenceTarget; 1889 } 1890 1891 /** 1892 * @return {@link #prescription} (The original request, instruction or authority to perform the administration.) 1893 */ 1894 public Reference getPrescription() { 1895 if (this.prescription == null) 1896 if (Configuration.errorOnAutoCreate()) 1897 throw new Error("Attempt to auto-create MedicationAdministration.prescription"); 1898 else if (Configuration.doAutoCreate()) 1899 this.prescription = new Reference(); // cc 1900 return this.prescription; 1901 } 1902 1903 public boolean hasPrescription() { 1904 return this.prescription != null && !this.prescription.isEmpty(); 1905 } 1906 1907 /** 1908 * @param value {@link #prescription} (The original request, instruction or authority to perform the administration.) 1909 */ 1910 public MedicationAdministration setPrescription(Reference value) { 1911 this.prescription = value; 1912 return this; 1913 } 1914 1915 /** 1916 * @return {@link #prescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The original request, instruction or authority to perform the administration.) 1917 */ 1918 public MedicationRequest getPrescriptionTarget() { 1919 if (this.prescriptionTarget == null) 1920 if (Configuration.errorOnAutoCreate()) 1921 throw new Error("Attempt to auto-create MedicationAdministration.prescription"); 1922 else if (Configuration.doAutoCreate()) 1923 this.prescriptionTarget = new MedicationRequest(); // aa 1924 return this.prescriptionTarget; 1925 } 1926 1927 /** 1928 * @param value {@link #prescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The original request, instruction or authority to perform the administration.) 1929 */ 1930 public MedicationAdministration setPrescriptionTarget(MedicationRequest value) { 1931 this.prescriptionTarget = value; 1932 return this; 1933 } 1934 1935 /** 1936 * @return {@link #device} (The device used in administering the medication to the patient. For example, a particular infusion pump.) 1937 */ 1938 public List<Reference> getDevice() { 1939 if (this.device == null) 1940 this.device = new ArrayList<Reference>(); 1941 return this.device; 1942 } 1943 1944 /** 1945 * @return Returns a reference to <code>this</code> for easy method chaining 1946 */ 1947 public MedicationAdministration setDevice(List<Reference> theDevice) { 1948 this.device = theDevice; 1949 return this; 1950 } 1951 1952 public boolean hasDevice() { 1953 if (this.device == null) 1954 return false; 1955 for (Reference item : this.device) 1956 if (!item.isEmpty()) 1957 return true; 1958 return false; 1959 } 1960 1961 public Reference addDevice() { //3 1962 Reference t = new Reference(); 1963 if (this.device == null) 1964 this.device = new ArrayList<Reference>(); 1965 this.device.add(t); 1966 return t; 1967 } 1968 1969 public MedicationAdministration addDevice(Reference t) { //3 1970 if (t == null) 1971 return this; 1972 if (this.device == null) 1973 this.device = new ArrayList<Reference>(); 1974 this.device.add(t); 1975 return this; 1976 } 1977 1978 /** 1979 * @return The first repetition of repeating field {@link #device}, creating it if it does not already exist 1980 */ 1981 public Reference getDeviceFirstRep() { 1982 if (getDevice().isEmpty()) { 1983 addDevice(); 1984 } 1985 return getDevice().get(0); 1986 } 1987 1988 /** 1989 * @deprecated Use Reference#setResource(IBaseResource) instead 1990 */ 1991 @Deprecated 1992 public List<Device> getDeviceTarget() { 1993 if (this.deviceTarget == null) 1994 this.deviceTarget = new ArrayList<Device>(); 1995 return this.deviceTarget; 1996 } 1997 1998 /** 1999 * @deprecated Use Reference#setResource(IBaseResource) instead 2000 */ 2001 @Deprecated 2002 public Device addDeviceTarget() { 2003 Device r = new Device(); 2004 if (this.deviceTarget == null) 2005 this.deviceTarget = new ArrayList<Device>(); 2006 this.deviceTarget.add(r); 2007 return r; 2008 } 2009 2010 /** 2011 * @return {@link #note} (Extra information about the medication administration that is not conveyed by the other attributes.) 2012 */ 2013 public List<Annotation> getNote() { 2014 if (this.note == null) 2015 this.note = new ArrayList<Annotation>(); 2016 return this.note; 2017 } 2018 2019 /** 2020 * @return Returns a reference to <code>this</code> for easy method chaining 2021 */ 2022 public MedicationAdministration setNote(List<Annotation> theNote) { 2023 this.note = theNote; 2024 return this; 2025 } 2026 2027 public boolean hasNote() { 2028 if (this.note == null) 2029 return false; 2030 for (Annotation item : this.note) 2031 if (!item.isEmpty()) 2032 return true; 2033 return false; 2034 } 2035 2036 public Annotation addNote() { //3 2037 Annotation t = new Annotation(); 2038 if (this.note == null) 2039 this.note = new ArrayList<Annotation>(); 2040 this.note.add(t); 2041 return t; 2042 } 2043 2044 public MedicationAdministration addNote(Annotation t) { //3 2045 if (t == null) 2046 return this; 2047 if (this.note == null) 2048 this.note = new ArrayList<Annotation>(); 2049 this.note.add(t); 2050 return this; 2051 } 2052 2053 /** 2054 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2055 */ 2056 public Annotation getNoteFirstRep() { 2057 if (getNote().isEmpty()) { 2058 addNote(); 2059 } 2060 return getNote().get(0); 2061 } 2062 2063 /** 2064 * @return {@link #dosage} (Describes the medication dosage information details e.g. dose, rate, site, route, etc.) 2065 */ 2066 public MedicationAdministrationDosageComponent getDosage() { 2067 if (this.dosage == null) 2068 if (Configuration.errorOnAutoCreate()) 2069 throw new Error("Attempt to auto-create MedicationAdministration.dosage"); 2070 else if (Configuration.doAutoCreate()) 2071 this.dosage = new MedicationAdministrationDosageComponent(); // cc 2072 return this.dosage; 2073 } 2074 2075 public boolean hasDosage() { 2076 return this.dosage != null && !this.dosage.isEmpty(); 2077 } 2078 2079 /** 2080 * @param value {@link #dosage} (Describes the medication dosage information details e.g. dose, rate, site, route, etc.) 2081 */ 2082 public MedicationAdministration setDosage(MedicationAdministrationDosageComponent value) { 2083 this.dosage = value; 2084 return this; 2085 } 2086 2087 /** 2088 * @return {@link #eventHistory} (A summary of the events of interest that have occurred, such as when the administration was verified.) 2089 */ 2090 public List<Reference> getEventHistory() { 2091 if (this.eventHistory == null) 2092 this.eventHistory = new ArrayList<Reference>(); 2093 return this.eventHistory; 2094 } 2095 2096 /** 2097 * @return Returns a reference to <code>this</code> for easy method chaining 2098 */ 2099 public MedicationAdministration setEventHistory(List<Reference> theEventHistory) { 2100 this.eventHistory = theEventHistory; 2101 return this; 2102 } 2103 2104 public boolean hasEventHistory() { 2105 if (this.eventHistory == null) 2106 return false; 2107 for (Reference item : this.eventHistory) 2108 if (!item.isEmpty()) 2109 return true; 2110 return false; 2111 } 2112 2113 public Reference addEventHistory() { //3 2114 Reference t = new Reference(); 2115 if (this.eventHistory == null) 2116 this.eventHistory = new ArrayList<Reference>(); 2117 this.eventHistory.add(t); 2118 return t; 2119 } 2120 2121 public MedicationAdministration addEventHistory(Reference t) { //3 2122 if (t == null) 2123 return this; 2124 if (this.eventHistory == null) 2125 this.eventHistory = new ArrayList<Reference>(); 2126 this.eventHistory.add(t); 2127 return this; 2128 } 2129 2130 /** 2131 * @return The first repetition of repeating field {@link #eventHistory}, creating it if it does not already exist 2132 */ 2133 public Reference getEventHistoryFirstRep() { 2134 if (getEventHistory().isEmpty()) { 2135 addEventHistory(); 2136 } 2137 return getEventHistory().get(0); 2138 } 2139 2140 /** 2141 * @deprecated Use Reference#setResource(IBaseResource) instead 2142 */ 2143 @Deprecated 2144 public List<Provenance> getEventHistoryTarget() { 2145 if (this.eventHistoryTarget == null) 2146 this.eventHistoryTarget = new ArrayList<Provenance>(); 2147 return this.eventHistoryTarget; 2148 } 2149 2150 /** 2151 * @deprecated Use Reference#setResource(IBaseResource) instead 2152 */ 2153 @Deprecated 2154 public Provenance addEventHistoryTarget() { 2155 Provenance r = new Provenance(); 2156 if (this.eventHistoryTarget == null) 2157 this.eventHistoryTarget = new ArrayList<Provenance>(); 2158 this.eventHistoryTarget.add(r); 2159 return r; 2160 } 2161 2162 protected void listChildren(List<Property> children) { 2163 super.listChildren(children); 2164 children.add(new Property("identifier", "Identifier", "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2165 children.add(new Property("definition", "Reference(PlanDefinition|ActivityDefinition)", "A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, definition)); 2166 children.add(new Property("partOf", "Reference(MedicationAdministration|Procedure)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2167 children.add(new Property("status", "code", "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 0, 1, status)); 2168 children.add(new Property("category", "CodeableConcept", "Indicates the type of medication administration and where the medication is expected to be consumed or administered.", 0, 1, category)); 2169 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 2170 children.add(new Property("subject", "Reference(Patient|Group)", "The person or animal or group receiving the medication.", 0, 1, subject)); 2171 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.", 0, 1, context)); 2172 children.add(new Property("supportingInformation", "Reference(Any)", "Additional information (for example, patient height and weight) that supports the administration of the medication.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2173 children.add(new Property("effective[x]", "dateTime|Period", "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, effective)); 2174 children.add(new Property("performer", "", "The individual who was responsible for giving the medication to the patient.", 0, java.lang.Integer.MAX_VALUE, performer)); 2175 children.add(new Property("notGiven", "boolean", "Set this to true if the record is saying that the medication was NOT administered.", 0, 1, notGiven)); 2176 children.add(new Property("reasonNotGiven", "CodeableConcept", "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, reasonNotGiven)); 2177 children.add(new Property("reasonCode", "CodeableConcept", "A code indicating why the medication was given.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2178 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Condition or observation that supports why the medication was administered.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2179 children.add(new Property("prescription", "Reference(MedicationRequest)", "The original request, instruction or authority to perform the administration.", 0, 1, prescription)); 2180 children.add(new Property("device", "Reference(Device)", "The device used in administering the medication to the patient. For example, a particular infusion pump.", 0, java.lang.Integer.MAX_VALUE, device)); 2181 children.add(new Property("note", "Annotation", "Extra information about the medication administration that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2182 children.add(new Property("dosage", "", "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1, dosage)); 2183 children.add(new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the administration was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory)); 2184 } 2185 2186 @Override 2187 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2188 switch (_hash) { 2189 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 0, java.lang.Integer.MAX_VALUE, identifier); 2190 case -1014418093: /*definition*/ return new Property("definition", "Reference(PlanDefinition|ActivityDefinition)", "A protocol, guideline, orderset or other definition that was adhered to in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, definition); 2191 case -995410646: /*partOf*/ return new Property("partOf", "Reference(MedicationAdministration|Procedure)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 2192 case -892481550: /*status*/ return new Property("status", "code", "Will generally be set to show that the administration has been completed. For some long running administrations such as infusions it is possible for an administration to be started but not completed or it may be paused while some other process is under way.", 0, 1, status); 2193 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates the type of medication administration and where the medication is expected to be consumed or administered.", 0, 1, category); 2194 case 1458402129: /*medication[x]*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2195 case 1998965455: /*medication*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2196 case -209845038: /*medicationCodeableConcept*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2197 case 2104315196: /*medicationReference*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication that was administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2198 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person or animal or group receiving the medication.", 0, 1, subject); 2199 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The visit, admission or other contact between patient and health care provider the medication administration was performed as part of.", 0, 1, context); 2200 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Additional information (for example, patient height and weight) that supports the administration of the medication.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2201 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, effective); 2202 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, effective); 2203 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime|Period", "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, effective); 2204 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "dateTime|Period", "A specific date/time or interval of time during which the administration took place (or did not take place, when the 'notGiven' attribute is true). For many administrations, such as swallowing a tablet the use of dateTime is more appropriate.", 0, 1, effective); 2205 case 481140686: /*performer*/ return new Property("performer", "", "The individual who was responsible for giving the medication to the patient.", 0, java.lang.Integer.MAX_VALUE, performer); 2206 case 1554065514: /*notGiven*/ return new Property("notGiven", "boolean", "Set this to true if the record is saying that the medication was NOT administered.", 0, 1, notGiven); 2207 case 2101123790: /*reasonNotGiven*/ return new Property("reasonNotGiven", "CodeableConcept", "A code indicating why the administration was not performed.", 0, java.lang.Integer.MAX_VALUE, reasonNotGiven); 2208 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "A code indicating why the medication was given.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2209 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Condition or observation that supports why the medication was administered.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 2210 case 460301338: /*prescription*/ return new Property("prescription", "Reference(MedicationRequest)", "The original request, instruction or authority to perform the administration.", 0, 1, prescription); 2211 case -1335157162: /*device*/ return new Property("device", "Reference(Device)", "The device used in administering the medication to the patient. For example, a particular infusion pump.", 0, java.lang.Integer.MAX_VALUE, device); 2212 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the medication administration that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2213 case -1326018889: /*dosage*/ return new Property("dosage", "", "Describes the medication dosage information details e.g. dose, rate, site, route, etc.", 0, 1, dosage); 2214 case 1835190426: /*eventHistory*/ return new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the administration was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory); 2215 default: return super.getNamedProperty(_hash, _name, _checkValid); 2216 } 2217 2218 } 2219 2220 @Override 2221 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2222 switch (hash) { 2223 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2224 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 2225 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2226 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationAdministrationStatus> 2227 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2228 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // Type 2229 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2230 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2231 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2232 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // Type 2233 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // MedicationAdministrationPerformerComponent 2234 case 1554065514: /*notGiven*/ return this.notGiven == null ? new Base[0] : new Base[] {this.notGiven}; // BooleanType 2235 case 2101123790: /*reasonNotGiven*/ return this.reasonNotGiven == null ? new Base[0] : this.reasonNotGiven.toArray(new Base[this.reasonNotGiven.size()]); // CodeableConcept 2236 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2237 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2238 case 460301338: /*prescription*/ return this.prescription == null ? new Base[0] : new Base[] {this.prescription}; // Reference 2239 case -1335157162: /*device*/ return this.device == null ? new Base[0] : this.device.toArray(new Base[this.device.size()]); // Reference 2240 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2241 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : new Base[] {this.dosage}; // MedicationAdministrationDosageComponent 2242 case 1835190426: /*eventHistory*/ return this.eventHistory == null ? new Base[0] : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 2243 default: return super.getProperty(hash, name, checkValid); 2244 } 2245 2246 } 2247 2248 @Override 2249 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2250 switch (hash) { 2251 case -1618432855: // identifier 2252 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2253 return value; 2254 case -1014418093: // definition 2255 this.getDefinition().add(castToReference(value)); // Reference 2256 return value; 2257 case -995410646: // partOf 2258 this.getPartOf().add(castToReference(value)); // Reference 2259 return value; 2260 case -892481550: // status 2261 value = new MedicationAdministrationStatusEnumFactory().fromType(castToCode(value)); 2262 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatus> 2263 return value; 2264 case 50511102: // category 2265 this.category = castToCodeableConcept(value); // CodeableConcept 2266 return value; 2267 case 1998965455: // medication 2268 this.medication = castToType(value); // Type 2269 return value; 2270 case -1867885268: // subject 2271 this.subject = castToReference(value); // Reference 2272 return value; 2273 case 951530927: // context 2274 this.context = castToReference(value); // Reference 2275 return value; 2276 case -1248768647: // supportingInformation 2277 this.getSupportingInformation().add(castToReference(value)); // Reference 2278 return value; 2279 case -1468651097: // effective 2280 this.effective = castToType(value); // Type 2281 return value; 2282 case 481140686: // performer 2283 this.getPerformer().add((MedicationAdministrationPerformerComponent) value); // MedicationAdministrationPerformerComponent 2284 return value; 2285 case 1554065514: // notGiven 2286 this.notGiven = castToBoolean(value); // BooleanType 2287 return value; 2288 case 2101123790: // reasonNotGiven 2289 this.getReasonNotGiven().add(castToCodeableConcept(value)); // CodeableConcept 2290 return value; 2291 case 722137681: // reasonCode 2292 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2293 return value; 2294 case -1146218137: // reasonReference 2295 this.getReasonReference().add(castToReference(value)); // Reference 2296 return value; 2297 case 460301338: // prescription 2298 this.prescription = castToReference(value); // Reference 2299 return value; 2300 case -1335157162: // device 2301 this.getDevice().add(castToReference(value)); // Reference 2302 return value; 2303 case 3387378: // note 2304 this.getNote().add(castToAnnotation(value)); // Annotation 2305 return value; 2306 case -1326018889: // dosage 2307 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2308 return value; 2309 case 1835190426: // eventHistory 2310 this.getEventHistory().add(castToReference(value)); // Reference 2311 return value; 2312 default: return super.setProperty(hash, name, value); 2313 } 2314 2315 } 2316 2317 @Override 2318 public Base setProperty(String name, Base value) throws FHIRException { 2319 if (name.equals("identifier")) { 2320 this.getIdentifier().add(castToIdentifier(value)); 2321 } else if (name.equals("definition")) { 2322 this.getDefinition().add(castToReference(value)); 2323 } else if (name.equals("partOf")) { 2324 this.getPartOf().add(castToReference(value)); 2325 } else if (name.equals("status")) { 2326 value = new MedicationAdministrationStatusEnumFactory().fromType(castToCode(value)); 2327 this.status = (Enumeration) value; // Enumeration<MedicationAdministrationStatus> 2328 } else if (name.equals("category")) { 2329 this.category = castToCodeableConcept(value); // CodeableConcept 2330 } else if (name.equals("medication[x]")) { 2331 this.medication = castToType(value); // Type 2332 } else if (name.equals("subject")) { 2333 this.subject = castToReference(value); // Reference 2334 } else if (name.equals("context")) { 2335 this.context = castToReference(value); // Reference 2336 } else if (name.equals("supportingInformation")) { 2337 this.getSupportingInformation().add(castToReference(value)); 2338 } else if (name.equals("effective[x]")) { 2339 this.effective = castToType(value); // Type 2340 } else if (name.equals("performer")) { 2341 this.getPerformer().add((MedicationAdministrationPerformerComponent) value); 2342 } else if (name.equals("notGiven")) { 2343 this.notGiven = castToBoolean(value); // BooleanType 2344 } else if (name.equals("reasonNotGiven")) { 2345 this.getReasonNotGiven().add(castToCodeableConcept(value)); 2346 } else if (name.equals("reasonCode")) { 2347 this.getReasonCode().add(castToCodeableConcept(value)); 2348 } else if (name.equals("reasonReference")) { 2349 this.getReasonReference().add(castToReference(value)); 2350 } else if (name.equals("prescription")) { 2351 this.prescription = castToReference(value); // Reference 2352 } else if (name.equals("device")) { 2353 this.getDevice().add(castToReference(value)); 2354 } else if (name.equals("note")) { 2355 this.getNote().add(castToAnnotation(value)); 2356 } else if (name.equals("dosage")) { 2357 this.dosage = (MedicationAdministrationDosageComponent) value; // MedicationAdministrationDosageComponent 2358 } else if (name.equals("eventHistory")) { 2359 this.getEventHistory().add(castToReference(value)); 2360 } else 2361 return super.setProperty(name, value); 2362 return value; 2363 } 2364 2365 @Override 2366 public Base makeProperty(int hash, String name) throws FHIRException { 2367 switch (hash) { 2368 case -1618432855: return addIdentifier(); 2369 case -1014418093: return addDefinition(); 2370 case -995410646: return addPartOf(); 2371 case -892481550: return getStatusElement(); 2372 case 50511102: return getCategory(); 2373 case 1458402129: return getMedication(); 2374 case 1998965455: return getMedication(); 2375 case -1867885268: return getSubject(); 2376 case 951530927: return getContext(); 2377 case -1248768647: return addSupportingInformation(); 2378 case 247104889: return getEffective(); 2379 case -1468651097: return getEffective(); 2380 case 481140686: return addPerformer(); 2381 case 1554065514: return getNotGivenElement(); 2382 case 2101123790: return addReasonNotGiven(); 2383 case 722137681: return addReasonCode(); 2384 case -1146218137: return addReasonReference(); 2385 case 460301338: return getPrescription(); 2386 case -1335157162: return addDevice(); 2387 case 3387378: return addNote(); 2388 case -1326018889: return getDosage(); 2389 case 1835190426: return addEventHistory(); 2390 default: return super.makeProperty(hash, name); 2391 } 2392 2393 } 2394 2395 @Override 2396 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2397 switch (hash) { 2398 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2399 case -1014418093: /*definition*/ return new String[] {"Reference"}; 2400 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2401 case -892481550: /*status*/ return new String[] {"code"}; 2402 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2403 case 1998965455: /*medication*/ return new String[] {"CodeableConcept", "Reference"}; 2404 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2405 case 951530927: /*context*/ return new String[] {"Reference"}; 2406 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 2407 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 2408 case 481140686: /*performer*/ return new String[] {}; 2409 case 1554065514: /*notGiven*/ return new String[] {"boolean"}; 2410 case 2101123790: /*reasonNotGiven*/ return new String[] {"CodeableConcept"}; 2411 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 2412 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 2413 case 460301338: /*prescription*/ return new String[] {"Reference"}; 2414 case -1335157162: /*device*/ return new String[] {"Reference"}; 2415 case 3387378: /*note*/ return new String[] {"Annotation"}; 2416 case -1326018889: /*dosage*/ return new String[] {}; 2417 case 1835190426: /*eventHistory*/ return new String[] {"Reference"}; 2418 default: return super.getTypesForProperty(hash, name); 2419 } 2420 2421 } 2422 2423 @Override 2424 public Base addChild(String name) throws FHIRException { 2425 if (name.equals("identifier")) { 2426 return addIdentifier(); 2427 } 2428 else if (name.equals("definition")) { 2429 return addDefinition(); 2430 } 2431 else if (name.equals("partOf")) { 2432 return addPartOf(); 2433 } 2434 else if (name.equals("status")) { 2435 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.status"); 2436 } 2437 else if (name.equals("category")) { 2438 this.category = new CodeableConcept(); 2439 return this.category; 2440 } 2441 else if (name.equals("medicationCodeableConcept")) { 2442 this.medication = new CodeableConcept(); 2443 return this.medication; 2444 } 2445 else if (name.equals("medicationReference")) { 2446 this.medication = new Reference(); 2447 return this.medication; 2448 } 2449 else if (name.equals("subject")) { 2450 this.subject = new Reference(); 2451 return this.subject; 2452 } 2453 else if (name.equals("context")) { 2454 this.context = new Reference(); 2455 return this.context; 2456 } 2457 else if (name.equals("supportingInformation")) { 2458 return addSupportingInformation(); 2459 } 2460 else if (name.equals("effectiveDateTime")) { 2461 this.effective = new DateTimeType(); 2462 return this.effective; 2463 } 2464 else if (name.equals("effectivePeriod")) { 2465 this.effective = new Period(); 2466 return this.effective; 2467 } 2468 else if (name.equals("performer")) { 2469 return addPerformer(); 2470 } 2471 else if (name.equals("notGiven")) { 2472 throw new FHIRException("Cannot call addChild on a singleton property MedicationAdministration.notGiven"); 2473 } 2474 else if (name.equals("reasonNotGiven")) { 2475 return addReasonNotGiven(); 2476 } 2477 else if (name.equals("reasonCode")) { 2478 return addReasonCode(); 2479 } 2480 else if (name.equals("reasonReference")) { 2481 return addReasonReference(); 2482 } 2483 else if (name.equals("prescription")) { 2484 this.prescription = new Reference(); 2485 return this.prescription; 2486 } 2487 else if (name.equals("device")) { 2488 return addDevice(); 2489 } 2490 else if (name.equals("note")) { 2491 return addNote(); 2492 } 2493 else if (name.equals("dosage")) { 2494 this.dosage = new MedicationAdministrationDosageComponent(); 2495 return this.dosage; 2496 } 2497 else if (name.equals("eventHistory")) { 2498 return addEventHistory(); 2499 } 2500 else 2501 return super.addChild(name); 2502 } 2503 2504 public String fhirType() { 2505 return "MedicationAdministration"; 2506 2507 } 2508 2509 public MedicationAdministration copy() { 2510 MedicationAdministration dst = new MedicationAdministration(); 2511 copyValues(dst); 2512 if (identifier != null) { 2513 dst.identifier = new ArrayList<Identifier>(); 2514 for (Identifier i : identifier) 2515 dst.identifier.add(i.copy()); 2516 }; 2517 if (definition != null) { 2518 dst.definition = new ArrayList<Reference>(); 2519 for (Reference i : definition) 2520 dst.definition.add(i.copy()); 2521 }; 2522 if (partOf != null) { 2523 dst.partOf = new ArrayList<Reference>(); 2524 for (Reference i : partOf) 2525 dst.partOf.add(i.copy()); 2526 }; 2527 dst.status = status == null ? null : status.copy(); 2528 dst.category = category == null ? null : category.copy(); 2529 dst.medication = medication == null ? null : medication.copy(); 2530 dst.subject = subject == null ? null : subject.copy(); 2531 dst.context = context == null ? null : context.copy(); 2532 if (supportingInformation != null) { 2533 dst.supportingInformation = new ArrayList<Reference>(); 2534 for (Reference i : supportingInformation) 2535 dst.supportingInformation.add(i.copy()); 2536 }; 2537 dst.effective = effective == null ? null : effective.copy(); 2538 if (performer != null) { 2539 dst.performer = new ArrayList<MedicationAdministrationPerformerComponent>(); 2540 for (MedicationAdministrationPerformerComponent i : performer) 2541 dst.performer.add(i.copy()); 2542 }; 2543 dst.notGiven = notGiven == null ? null : notGiven.copy(); 2544 if (reasonNotGiven != null) { 2545 dst.reasonNotGiven = new ArrayList<CodeableConcept>(); 2546 for (CodeableConcept i : reasonNotGiven) 2547 dst.reasonNotGiven.add(i.copy()); 2548 }; 2549 if (reasonCode != null) { 2550 dst.reasonCode = new ArrayList<CodeableConcept>(); 2551 for (CodeableConcept i : reasonCode) 2552 dst.reasonCode.add(i.copy()); 2553 }; 2554 if (reasonReference != null) { 2555 dst.reasonReference = new ArrayList<Reference>(); 2556 for (Reference i : reasonReference) 2557 dst.reasonReference.add(i.copy()); 2558 }; 2559 dst.prescription = prescription == null ? null : prescription.copy(); 2560 if (device != null) { 2561 dst.device = new ArrayList<Reference>(); 2562 for (Reference i : device) 2563 dst.device.add(i.copy()); 2564 }; 2565 if (note != null) { 2566 dst.note = new ArrayList<Annotation>(); 2567 for (Annotation i : note) 2568 dst.note.add(i.copy()); 2569 }; 2570 dst.dosage = dosage == null ? null : dosage.copy(); 2571 if (eventHistory != null) { 2572 dst.eventHistory = new ArrayList<Reference>(); 2573 for (Reference i : eventHistory) 2574 dst.eventHistory.add(i.copy()); 2575 }; 2576 return dst; 2577 } 2578 2579 protected MedicationAdministration typedCopy() { 2580 return copy(); 2581 } 2582 2583 @Override 2584 public boolean equalsDeep(Base other_) { 2585 if (!super.equalsDeep(other_)) 2586 return false; 2587 if (!(other_ instanceof MedicationAdministration)) 2588 return false; 2589 MedicationAdministration o = (MedicationAdministration) other_; 2590 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 2591 && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 2592 && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) && compareDeep(context, o.context, true) 2593 && compareDeep(supportingInformation, o.supportingInformation, true) && compareDeep(effective, o.effective, true) 2594 && compareDeep(performer, o.performer, true) && compareDeep(notGiven, o.notGiven, true) && compareDeep(reasonNotGiven, o.reasonNotGiven, true) 2595 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 2596 && compareDeep(prescription, o.prescription, true) && compareDeep(device, o.device, true) && compareDeep(note, o.note, true) 2597 && compareDeep(dosage, o.dosage, true) && compareDeep(eventHistory, o.eventHistory, true); 2598 } 2599 2600 @Override 2601 public boolean equalsShallow(Base other_) { 2602 if (!super.equalsShallow(other_)) 2603 return false; 2604 if (!(other_ instanceof MedicationAdministration)) 2605 return false; 2606 MedicationAdministration o = (MedicationAdministration) other_; 2607 return compareValues(status, o.status, true) && compareValues(notGiven, o.notGiven, true); 2608 } 2609 2610 public boolean isEmpty() { 2611 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, partOf 2612 , status, category, medication, subject, context, supportingInformation, effective 2613 , performer, notGiven, reasonNotGiven, reasonCode, reasonReference, prescription 2614 , device, note, dosage, eventHistory); 2615 } 2616 2617 @Override 2618 public ResourceType getResourceType() { 2619 return ResourceType.MedicationAdministration; 2620 } 2621 2622 /** 2623 * Search parameter: <b>identifier</b> 2624 * <p> 2625 * Description: <b>Return administrations with this external identifier</b><br> 2626 * Type: <b>token</b><br> 2627 * Path: <b>MedicationAdministration.identifier</b><br> 2628 * </p> 2629 */ 2630 @SearchParamDefinition(name="identifier", path="MedicationAdministration.identifier", description="Return administrations with this external identifier", type="token" ) 2631 public static final String SP_IDENTIFIER = "identifier"; 2632 /** 2633 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2634 * <p> 2635 * Description: <b>Return administrations with this external identifier</b><br> 2636 * Type: <b>token</b><br> 2637 * Path: <b>MedicationAdministration.identifier</b><br> 2638 * </p> 2639 */ 2640 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2641 2642 /** 2643 * Search parameter: <b>code</b> 2644 * <p> 2645 * Description: <b>Return administrations of this medication code</b><br> 2646 * Type: <b>token</b><br> 2647 * Path: <b>MedicationAdministration.medicationCodeableConcept</b><br> 2648 * </p> 2649 */ 2650 @SearchParamDefinition(name="code", path="MedicationAdministration.medication.as(CodeableConcept)", description="Return administrations of this medication code", type="token" ) 2651 public static final String SP_CODE = "code"; 2652 /** 2653 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2654 * <p> 2655 * Description: <b>Return administrations of this medication code</b><br> 2656 * Type: <b>token</b><br> 2657 * Path: <b>MedicationAdministration.medicationCodeableConcept</b><br> 2658 * </p> 2659 */ 2660 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2661 2662 /** 2663 * Search parameter: <b>performer</b> 2664 * <p> 2665 * Description: <b>The identify of the individual who administered the medication</b><br> 2666 * Type: <b>reference</b><br> 2667 * Path: <b>MedicationAdministration.performer.actor</b><br> 2668 * </p> 2669 */ 2670 @SearchParamDefinition(name="performer", path="MedicationAdministration.performer.actor", description="The identify of the individual who administered the medication", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Device.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2671 public static final String SP_PERFORMER = "performer"; 2672 /** 2673 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2674 * <p> 2675 * Description: <b>The identify of the individual who administered the medication</b><br> 2676 * Type: <b>reference</b><br> 2677 * Path: <b>MedicationAdministration.performer.actor</b><br> 2678 * </p> 2679 */ 2680 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2681 2682/** 2683 * Constant for fluent queries to be used to add include statements. Specifies 2684 * the path value of "<b>MedicationAdministration:performer</b>". 2685 */ 2686 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("MedicationAdministration:performer").toLocked(); 2687 2688 /** 2689 * Search parameter: <b>subject</b> 2690 * <p> 2691 * Description: <b>The identify of the individual or group to list administrations for</b><br> 2692 * Type: <b>reference</b><br> 2693 * Path: <b>MedicationAdministration.subject</b><br> 2694 * </p> 2695 */ 2696 @SearchParamDefinition(name="subject", path="MedicationAdministration.subject", description="The identify of the individual or group to list administrations for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 2697 public static final String SP_SUBJECT = "subject"; 2698 /** 2699 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2700 * <p> 2701 * Description: <b>The identify of the individual or group to list administrations for</b><br> 2702 * Type: <b>reference</b><br> 2703 * Path: <b>MedicationAdministration.subject</b><br> 2704 * </p> 2705 */ 2706 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2707 2708/** 2709 * Constant for fluent queries to be used to add include statements. Specifies 2710 * the path value of "<b>MedicationAdministration:subject</b>". 2711 */ 2712 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationAdministration:subject").toLocked(); 2713 2714 /** 2715 * Search parameter: <b>not-given</b> 2716 * <p> 2717 * Description: <b>Administrations that were not made</b><br> 2718 * Type: <b>token</b><br> 2719 * Path: <b>MedicationAdministration.notGiven</b><br> 2720 * </p> 2721 */ 2722 @SearchParamDefinition(name="not-given", path="MedicationAdministration.notGiven", description="Administrations that were not made", type="token" ) 2723 public static final String SP_NOT_GIVEN = "not-given"; 2724 /** 2725 * <b>Fluent Client</b> search parameter constant for <b>not-given</b> 2726 * <p> 2727 * Description: <b>Administrations that were not made</b><br> 2728 * Type: <b>token</b><br> 2729 * Path: <b>MedicationAdministration.notGiven</b><br> 2730 * </p> 2731 */ 2732 public static final ca.uhn.fhir.rest.gclient.TokenClientParam NOT_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_NOT_GIVEN); 2733 2734 /** 2735 * Search parameter: <b>medication</b> 2736 * <p> 2737 * Description: <b>Return administrations of this medication resource</b><br> 2738 * Type: <b>reference</b><br> 2739 * Path: <b>MedicationAdministration.medicationReference</b><br> 2740 * </p> 2741 */ 2742 @SearchParamDefinition(name="medication", path="MedicationAdministration.medication.as(Reference)", description="Return administrations of this medication resource", type="reference", target={Medication.class } ) 2743 public static final String SP_MEDICATION = "medication"; 2744 /** 2745 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 2746 * <p> 2747 * Description: <b>Return administrations of this medication resource</b><br> 2748 * Type: <b>reference</b><br> 2749 * Path: <b>MedicationAdministration.medicationReference</b><br> 2750 * </p> 2751 */ 2752 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 2753 2754/** 2755 * Constant for fluent queries to be used to add include statements. Specifies 2756 * the path value of "<b>MedicationAdministration:medication</b>". 2757 */ 2758 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationAdministration:medication").toLocked(); 2759 2760 /** 2761 * Search parameter: <b>reason-given</b> 2762 * <p> 2763 * Description: <b>Reasons for administering the medication</b><br> 2764 * Type: <b>token</b><br> 2765 * Path: <b>MedicationAdministration.reasonCode</b><br> 2766 * </p> 2767 */ 2768 @SearchParamDefinition(name="reason-given", path="MedicationAdministration.reasonCode", description="Reasons for administering the medication", type="token" ) 2769 public static final String SP_REASON_GIVEN = "reason-given"; 2770 /** 2771 * <b>Fluent Client</b> search parameter constant for <b>reason-given</b> 2772 * <p> 2773 * Description: <b>Reasons for administering the medication</b><br> 2774 * Type: <b>token</b><br> 2775 * Path: <b>MedicationAdministration.reasonCode</b><br> 2776 * </p> 2777 */ 2778 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_GIVEN); 2779 2780 /** 2781 * Search parameter: <b>prescription</b> 2782 * <p> 2783 * Description: <b>The identity of a prescription to list administrations from</b><br> 2784 * Type: <b>reference</b><br> 2785 * Path: <b>MedicationAdministration.prescription</b><br> 2786 * </p> 2787 */ 2788 @SearchParamDefinition(name="prescription", path="MedicationAdministration.prescription", description="The identity of a prescription to list administrations from", type="reference", target={MedicationRequest.class } ) 2789 public static final String SP_PRESCRIPTION = "prescription"; 2790 /** 2791 * <b>Fluent Client</b> search parameter constant for <b>prescription</b> 2792 * <p> 2793 * Description: <b>The identity of a prescription to list administrations from</b><br> 2794 * Type: <b>reference</b><br> 2795 * Path: <b>MedicationAdministration.prescription</b><br> 2796 * </p> 2797 */ 2798 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRESCRIPTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRESCRIPTION); 2799 2800/** 2801 * Constant for fluent queries to be used to add include statements. Specifies 2802 * the path value of "<b>MedicationAdministration:prescription</b>". 2803 */ 2804 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRESCRIPTION = new ca.uhn.fhir.model.api.Include("MedicationAdministration:prescription").toLocked(); 2805 2806 /** 2807 * Search parameter: <b>patient</b> 2808 * <p> 2809 * Description: <b>The identity of a patient to list administrations for</b><br> 2810 * Type: <b>reference</b><br> 2811 * Path: <b>MedicationAdministration.subject</b><br> 2812 * </p> 2813 */ 2814 @SearchParamDefinition(name="patient", path="MedicationAdministration.subject", description="The identity of a patient to list administrations for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 2815 public static final String SP_PATIENT = "patient"; 2816 /** 2817 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 2818 * <p> 2819 * Description: <b>The identity of a patient to list administrations for</b><br> 2820 * Type: <b>reference</b><br> 2821 * Path: <b>MedicationAdministration.subject</b><br> 2822 * </p> 2823 */ 2824 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 2825 2826/** 2827 * Constant for fluent queries to be used to add include statements. Specifies 2828 * the path value of "<b>MedicationAdministration:patient</b>". 2829 */ 2830 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationAdministration:patient").toLocked(); 2831 2832 /** 2833 * Search parameter: <b>effective-time</b> 2834 * <p> 2835 * Description: <b>Date administration happened (or did not happen)</b><br> 2836 * Type: <b>date</b><br> 2837 * Path: <b>MedicationAdministration.effective[x]</b><br> 2838 * </p> 2839 */ 2840 @SearchParamDefinition(name="effective-time", path="MedicationAdministration.effective", description="Date administration happened (or did not happen)", type="date" ) 2841 public static final String SP_EFFECTIVE_TIME = "effective-time"; 2842 /** 2843 * <b>Fluent Client</b> search parameter constant for <b>effective-time</b> 2844 * <p> 2845 * Description: <b>Date administration happened (or did not happen)</b><br> 2846 * Type: <b>date</b><br> 2847 * Path: <b>MedicationAdministration.effective[x]</b><br> 2848 * </p> 2849 */ 2850 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE_TIME = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE_TIME); 2851 2852 /** 2853 * Search parameter: <b>context</b> 2854 * <p> 2855 * Description: <b>Return administrations that share this encounter or episode of care</b><br> 2856 * Type: <b>reference</b><br> 2857 * Path: <b>MedicationAdministration.context</b><br> 2858 * </p> 2859 */ 2860 @SearchParamDefinition(name="context", path="MedicationAdministration.context", description="Return administrations that share this encounter or episode of care", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 2861 public static final String SP_CONTEXT = "context"; 2862 /** 2863 * <b>Fluent Client</b> search parameter constant for <b>context</b> 2864 * <p> 2865 * Description: <b>Return administrations that share this encounter or episode of care</b><br> 2866 * Type: <b>reference</b><br> 2867 * Path: <b>MedicationAdministration.context</b><br> 2868 * </p> 2869 */ 2870 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 2871 2872/** 2873 * Constant for fluent queries to be used to add include statements. Specifies 2874 * the path value of "<b>MedicationAdministration:context</b>". 2875 */ 2876 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("MedicationAdministration:context").toLocked(); 2877 2878 /** 2879 * Search parameter: <b>reason-not-given</b> 2880 * <p> 2881 * Description: <b>Reasons for not administering the medication</b><br> 2882 * Type: <b>token</b><br> 2883 * Path: <b>MedicationAdministration.reasonNotGiven</b><br> 2884 * </p> 2885 */ 2886 @SearchParamDefinition(name="reason-not-given", path="MedicationAdministration.reasonNotGiven", description="Reasons for not administering the medication", type="token" ) 2887 public static final String SP_REASON_NOT_GIVEN = "reason-not-given"; 2888 /** 2889 * <b>Fluent Client</b> search parameter constant for <b>reason-not-given</b> 2890 * <p> 2891 * Description: <b>Reasons for not administering the medication</b><br> 2892 * Type: <b>token</b><br> 2893 * Path: <b>MedicationAdministration.reasonNotGiven</b><br> 2894 * </p> 2895 */ 2896 public static final ca.uhn.fhir.rest.gclient.TokenClientParam REASON_NOT_GIVEN = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_REASON_NOT_GIVEN); 2897 2898 /** 2899 * Search parameter: <b>device</b> 2900 * <p> 2901 * Description: <b>Return administrations with this administration device identity</b><br> 2902 * Type: <b>reference</b><br> 2903 * Path: <b>MedicationAdministration.device</b><br> 2904 * </p> 2905 */ 2906 @SearchParamDefinition(name="device", path="MedicationAdministration.device", description="Return administrations with this administration device identity", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Device") }, target={Device.class } ) 2907 public static final String SP_DEVICE = "device"; 2908 /** 2909 * <b>Fluent Client</b> search parameter constant for <b>device</b> 2910 * <p> 2911 * Description: <b>Return administrations with this administration device identity</b><br> 2912 * Type: <b>reference</b><br> 2913 * Path: <b>MedicationAdministration.device</b><br> 2914 * </p> 2915 */ 2916 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DEVICE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DEVICE); 2917 2918/** 2919 * Constant for fluent queries to be used to add include statements. Specifies 2920 * the path value of "<b>MedicationAdministration:device</b>". 2921 */ 2922 public static final ca.uhn.fhir.model.api.Include INCLUDE_DEVICE = new ca.uhn.fhir.model.api.Include("MedicationAdministration:device").toLocked(); 2923 2924 /** 2925 * Search parameter: <b>status</b> 2926 * <p> 2927 * Description: <b>MedicationAdministration event status (for example one of active/paused/completed/nullified)</b><br> 2928 * Type: <b>token</b><br> 2929 * Path: <b>MedicationAdministration.status</b><br> 2930 * </p> 2931 */ 2932 @SearchParamDefinition(name="status", path="MedicationAdministration.status", description="MedicationAdministration event status (for example one of active/paused/completed/nullified)", type="token" ) 2933 public static final String SP_STATUS = "status"; 2934 /** 2935 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2936 * <p> 2937 * Description: <b>MedicationAdministration event status (for example one of active/paused/completed/nullified)</b><br> 2938 * Type: <b>token</b><br> 2939 * Path: <b>MedicationAdministration.status</b><br> 2940 * </p> 2941 */ 2942 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2943 2944 2945}