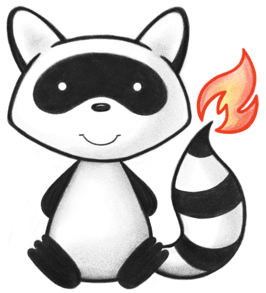
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * Indicates that a medication product is to be or has been dispensed for a named person/patient. This includes a description of the medication product (supply) provided and the instructions for administering the medication. The medication dispense is the result of a pharmacy system responding to a medication order. 051 */ 052@ResourceDef(name="MedicationDispense", profile="http://hl7.org/fhir/Profile/MedicationDispense") 053public class MedicationDispense extends DomainResource { 054 055 public enum MedicationDispenseStatus { 056 /** 057 * The core event has not started yet, but some staging activities have begun (e.g. initial compounding or packaging of medication). Preparation stages may be tracked for billing purposes. 058 */ 059 PREPARATION, 060 /** 061 * The dispense has started but has not yet completed. 062 */ 063 INPROGRESS, 064 /** 065 * Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called "suspended" 066 */ 067 ONHOLD, 068 /** 069 * All actions that are implied by the dispense have occurred. 070 */ 071 COMPLETED, 072 /** 073 * The dispense was entered in error and therefore nullified. 074 */ 075 ENTEREDINERROR, 076 /** 077 * Actions implied by the dispense have been permanently halted, before all of them occurred. 078 */ 079 STOPPED, 080 /** 081 * added to help the parsers with the generic types 082 */ 083 NULL; 084 public static MedicationDispenseStatus fromCode(String codeString) throws FHIRException { 085 if (codeString == null || "".equals(codeString)) 086 return null; 087 if ("preparation".equals(codeString)) 088 return PREPARATION; 089 if ("in-progress".equals(codeString)) 090 return INPROGRESS; 091 if ("on-hold".equals(codeString)) 092 return ONHOLD; 093 if ("completed".equals(codeString)) 094 return COMPLETED; 095 if ("entered-in-error".equals(codeString)) 096 return ENTEREDINERROR; 097 if ("stopped".equals(codeString)) 098 return STOPPED; 099 if (Configuration.isAcceptInvalidEnums()) 100 return null; 101 else 102 throw new FHIRException("Unknown MedicationDispenseStatus code '"+codeString+"'"); 103 } 104 public String toCode() { 105 switch (this) { 106 case PREPARATION: return "preparation"; 107 case INPROGRESS: return "in-progress"; 108 case ONHOLD: return "on-hold"; 109 case COMPLETED: return "completed"; 110 case ENTEREDINERROR: return "entered-in-error"; 111 case STOPPED: return "stopped"; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getSystem() { 117 switch (this) { 118 case PREPARATION: return "http://hl7.org/fhir/medication-dispense-status"; 119 case INPROGRESS: return "http://hl7.org/fhir/medication-dispense-status"; 120 case ONHOLD: return "http://hl7.org/fhir/medication-dispense-status"; 121 case COMPLETED: return "http://hl7.org/fhir/medication-dispense-status"; 122 case ENTEREDINERROR: return "http://hl7.org/fhir/medication-dispense-status"; 123 case STOPPED: return "http://hl7.org/fhir/medication-dispense-status"; 124 case NULL: return null; 125 default: return "?"; 126 } 127 } 128 public String getDefinition() { 129 switch (this) { 130 case PREPARATION: return "The core event has not started yet, but some staging activities have begun (e.g. initial compounding or packaging of medication). Preparation stages may be tracked for billing purposes."; 131 case INPROGRESS: return "The dispense has started but has not yet completed."; 132 case ONHOLD: return "Actions implied by the administration have been temporarily halted, but are expected to continue later. May also be called \"suspended\""; 133 case COMPLETED: return "All actions that are implied by the dispense have occurred."; 134 case ENTEREDINERROR: return "The dispense was entered in error and therefore nullified."; 135 case STOPPED: return "Actions implied by the dispense have been permanently halted, before all of them occurred."; 136 case NULL: return null; 137 default: return "?"; 138 } 139 } 140 public String getDisplay() { 141 switch (this) { 142 case PREPARATION: return "Preparation"; 143 case INPROGRESS: return "In Progress"; 144 case ONHOLD: return "On Hold"; 145 case COMPLETED: return "Completed"; 146 case ENTEREDINERROR: return "Entered in-Error"; 147 case STOPPED: return "Stopped"; 148 case NULL: return null; 149 default: return "?"; 150 } 151 } 152 } 153 154 public static class MedicationDispenseStatusEnumFactory implements EnumFactory<MedicationDispenseStatus> { 155 public MedicationDispenseStatus fromCode(String codeString) throws IllegalArgumentException { 156 if (codeString == null || "".equals(codeString)) 157 if (codeString == null || "".equals(codeString)) 158 return null; 159 if ("preparation".equals(codeString)) 160 return MedicationDispenseStatus.PREPARATION; 161 if ("in-progress".equals(codeString)) 162 return MedicationDispenseStatus.INPROGRESS; 163 if ("on-hold".equals(codeString)) 164 return MedicationDispenseStatus.ONHOLD; 165 if ("completed".equals(codeString)) 166 return MedicationDispenseStatus.COMPLETED; 167 if ("entered-in-error".equals(codeString)) 168 return MedicationDispenseStatus.ENTEREDINERROR; 169 if ("stopped".equals(codeString)) 170 return MedicationDispenseStatus.STOPPED; 171 throw new IllegalArgumentException("Unknown MedicationDispenseStatus code '"+codeString+"'"); 172 } 173 public Enumeration<MedicationDispenseStatus> fromType(PrimitiveType<?> code) throws FHIRException { 174 if (code == null) 175 return null; 176 if (code.isEmpty()) 177 return new Enumeration<MedicationDispenseStatus>(this); 178 String codeString = code.asStringValue(); 179 if (codeString == null || "".equals(codeString)) 180 return null; 181 if ("preparation".equals(codeString)) 182 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.PREPARATION); 183 if ("in-progress".equals(codeString)) 184 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.INPROGRESS); 185 if ("on-hold".equals(codeString)) 186 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.ONHOLD); 187 if ("completed".equals(codeString)) 188 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.COMPLETED); 189 if ("entered-in-error".equals(codeString)) 190 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.ENTEREDINERROR); 191 if ("stopped".equals(codeString)) 192 return new Enumeration<MedicationDispenseStatus>(this, MedicationDispenseStatus.STOPPED); 193 throw new FHIRException("Unknown MedicationDispenseStatus code '"+codeString+"'"); 194 } 195 public String toCode(MedicationDispenseStatus code) { 196 if (code == MedicationDispenseStatus.NULL) 197 return null; 198 if (code == MedicationDispenseStatus.PREPARATION) 199 return "preparation"; 200 if (code == MedicationDispenseStatus.INPROGRESS) 201 return "in-progress"; 202 if (code == MedicationDispenseStatus.ONHOLD) 203 return "on-hold"; 204 if (code == MedicationDispenseStatus.COMPLETED) 205 return "completed"; 206 if (code == MedicationDispenseStatus.ENTEREDINERROR) 207 return "entered-in-error"; 208 if (code == MedicationDispenseStatus.STOPPED) 209 return "stopped"; 210 return "?"; 211 } 212 public String toSystem(MedicationDispenseStatus code) { 213 return code.getSystem(); 214 } 215 } 216 217 @Block() 218 public static class MedicationDispensePerformerComponent extends BackboneElement implements IBaseBackboneElement { 219 /** 220 * The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication. 221 */ 222 @Child(name = "actor", type = {Practitioner.class, Organization.class, Patient.class, Device.class, RelatedPerson.class}, order=1, min=1, max=1, modifier=false, summary=false) 223 @Description(shortDefinition="Individual who was performing", formalDefinition="The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication." ) 224 protected Reference actor; 225 226 /** 227 * The actual object that is the target of the reference (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.) 228 */ 229 protected Resource actorTarget; 230 231 /** 232 * The organization the device or practitioner was acting on behalf of. 233 */ 234 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=false) 235 @Description(shortDefinition="Organization organization was acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 236 protected Reference onBehalfOf; 237 238 /** 239 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 240 */ 241 protected Organization onBehalfOfTarget; 242 243 private static final long serialVersionUID = -488386403L; 244 245 /** 246 * Constructor 247 */ 248 public MedicationDispensePerformerComponent() { 249 super(); 250 } 251 252 /** 253 * Constructor 254 */ 255 public MedicationDispensePerformerComponent(Reference actor) { 256 super(); 257 this.actor = actor; 258 } 259 260 /** 261 * @return {@link #actor} (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.) 262 */ 263 public Reference getActor() { 264 if (this.actor == null) 265 if (Configuration.errorOnAutoCreate()) 266 throw new Error("Attempt to auto-create MedicationDispensePerformerComponent.actor"); 267 else if (Configuration.doAutoCreate()) 268 this.actor = new Reference(); // cc 269 return this.actor; 270 } 271 272 public boolean hasActor() { 273 return this.actor != null && !this.actor.isEmpty(); 274 } 275 276 /** 277 * @param value {@link #actor} (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.) 278 */ 279 public MedicationDispensePerformerComponent setActor(Reference value) { 280 this.actor = value; 281 return this; 282 } 283 284 /** 285 * @return {@link #actor} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.) 286 */ 287 public Resource getActorTarget() { 288 return this.actorTarget; 289 } 290 291 /** 292 * @param value {@link #actor} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.) 293 */ 294 public MedicationDispensePerformerComponent setActorTarget(Resource value) { 295 this.actorTarget = value; 296 return this; 297 } 298 299 /** 300 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 301 */ 302 public Reference getOnBehalfOf() { 303 if (this.onBehalfOf == null) 304 if (Configuration.errorOnAutoCreate()) 305 throw new Error("Attempt to auto-create MedicationDispensePerformerComponent.onBehalfOf"); 306 else if (Configuration.doAutoCreate()) 307 this.onBehalfOf = new Reference(); // cc 308 return this.onBehalfOf; 309 } 310 311 public boolean hasOnBehalfOf() { 312 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 313 } 314 315 /** 316 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 317 */ 318 public MedicationDispensePerformerComponent setOnBehalfOf(Reference value) { 319 this.onBehalfOf = value; 320 return this; 321 } 322 323 /** 324 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 325 */ 326 public Organization getOnBehalfOfTarget() { 327 if (this.onBehalfOfTarget == null) 328 if (Configuration.errorOnAutoCreate()) 329 throw new Error("Attempt to auto-create MedicationDispensePerformerComponent.onBehalfOf"); 330 else if (Configuration.doAutoCreate()) 331 this.onBehalfOfTarget = new Organization(); // aa 332 return this.onBehalfOfTarget; 333 } 334 335 /** 336 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 337 */ 338 public MedicationDispensePerformerComponent setOnBehalfOfTarget(Organization value) { 339 this.onBehalfOfTarget = value; 340 return this; 341 } 342 343 protected void listChildren(List<Property> children) { 344 super.listChildren(children); 345 children.add(new Property("actor", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.", 0, 1, actor)); 346 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 347 } 348 349 @Override 350 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 351 switch (_hash) { 352 case 92645877: /*actor*/ return new Property("actor", "Reference(Practitioner|Organization|Patient|Device|RelatedPerson)", "The device, practitioner, etc. who performed the action. It should be assumed that the actor is the dispenser of the medication.", 0, 1, actor); 353 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 354 default: return super.getNamedProperty(_hash, _name, _checkValid); 355 } 356 357 } 358 359 @Override 360 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 361 switch (hash) { 362 case 92645877: /*actor*/ return this.actor == null ? new Base[0] : new Base[] {this.actor}; // Reference 363 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 364 default: return super.getProperty(hash, name, checkValid); 365 } 366 367 } 368 369 @Override 370 public Base setProperty(int hash, String name, Base value) throws FHIRException { 371 switch (hash) { 372 case 92645877: // actor 373 this.actor = castToReference(value); // Reference 374 return value; 375 case -14402964: // onBehalfOf 376 this.onBehalfOf = castToReference(value); // Reference 377 return value; 378 default: return super.setProperty(hash, name, value); 379 } 380 381 } 382 383 @Override 384 public Base setProperty(String name, Base value) throws FHIRException { 385 if (name.equals("actor")) { 386 this.actor = castToReference(value); // Reference 387 } else if (name.equals("onBehalfOf")) { 388 this.onBehalfOf = castToReference(value); // Reference 389 } else 390 return super.setProperty(name, value); 391 return value; 392 } 393 394 @Override 395 public Base makeProperty(int hash, String name) throws FHIRException { 396 switch (hash) { 397 case 92645877: return getActor(); 398 case -14402964: return getOnBehalfOf(); 399 default: return super.makeProperty(hash, name); 400 } 401 402 } 403 404 @Override 405 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 406 switch (hash) { 407 case 92645877: /*actor*/ return new String[] {"Reference"}; 408 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 409 default: return super.getTypesForProperty(hash, name); 410 } 411 412 } 413 414 @Override 415 public Base addChild(String name) throws FHIRException { 416 if (name.equals("actor")) { 417 this.actor = new Reference(); 418 return this.actor; 419 } 420 else if (name.equals("onBehalfOf")) { 421 this.onBehalfOf = new Reference(); 422 return this.onBehalfOf; 423 } 424 else 425 return super.addChild(name); 426 } 427 428 public MedicationDispensePerformerComponent copy() { 429 MedicationDispensePerformerComponent dst = new MedicationDispensePerformerComponent(); 430 copyValues(dst); 431 dst.actor = actor == null ? null : actor.copy(); 432 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 433 return dst; 434 } 435 436 @Override 437 public boolean equalsDeep(Base other_) { 438 if (!super.equalsDeep(other_)) 439 return false; 440 if (!(other_ instanceof MedicationDispensePerformerComponent)) 441 return false; 442 MedicationDispensePerformerComponent o = (MedicationDispensePerformerComponent) other_; 443 return compareDeep(actor, o.actor, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 444 } 445 446 @Override 447 public boolean equalsShallow(Base other_) { 448 if (!super.equalsShallow(other_)) 449 return false; 450 if (!(other_ instanceof MedicationDispensePerformerComponent)) 451 return false; 452 MedicationDispensePerformerComponent o = (MedicationDispensePerformerComponent) other_; 453 return true; 454 } 455 456 public boolean isEmpty() { 457 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(actor, onBehalfOf); 458 } 459 460 public String fhirType() { 461 return "MedicationDispense.performer"; 462 463 } 464 465 } 466 467 @Block() 468 public static class MedicationDispenseSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 469 /** 470 * True if the dispenser dispensed a different drug or product from what was prescribed. 471 */ 472 @Child(name = "wasSubstituted", type = {BooleanType.class}, order=1, min=1, max=1, modifier=false, summary=false) 473 @Description(shortDefinition="Whether a substitution was or was not performed on the dispense", formalDefinition="True if the dispenser dispensed a different drug or product from what was prescribed." ) 474 protected BooleanType wasSubstituted; 475 476 /** 477 * A code signifying whether a different drug was dispensed from what was prescribed. 478 */ 479 @Child(name = "type", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 480 @Description(shortDefinition="Code signifying whether a different drug was dispensed from what was prescribed", formalDefinition="A code signifying whether a different drug was dispensed from what was prescribed." ) 481 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActSubstanceAdminSubstitutionCode") 482 protected CodeableConcept type; 483 484 /** 485 * Indicates the reason for the substitution of (or lack of substitution) from what was prescribed. 486 */ 487 @Child(name = "reason", type = {CodeableConcept.class}, order=3, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 488 @Description(shortDefinition="Why was substitution made", formalDefinition="Indicates the reason for the substitution of (or lack of substitution) from what was prescribed." ) 489 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-SubstanceAdminSubstitutionReason") 490 protected List<CodeableConcept> reason; 491 492 /** 493 * The person or organization that has primary responsibility for the substitution. 494 */ 495 @Child(name = "responsibleParty", type = {Practitioner.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 496 @Description(shortDefinition="Who is responsible for the substitution", formalDefinition="The person or organization that has primary responsibility for the substitution." ) 497 protected List<Reference> responsibleParty; 498 /** 499 * The actual objects that are the target of the reference (The person or organization that has primary responsibility for the substitution.) 500 */ 501 protected List<Practitioner> responsiblePartyTarget; 502 503 504 private static final long serialVersionUID = -728152257L; 505 506 /** 507 * Constructor 508 */ 509 public MedicationDispenseSubstitutionComponent() { 510 super(); 511 } 512 513 /** 514 * Constructor 515 */ 516 public MedicationDispenseSubstitutionComponent(BooleanType wasSubstituted) { 517 super(); 518 this.wasSubstituted = wasSubstituted; 519 } 520 521 /** 522 * @return {@link #wasSubstituted} (True if the dispenser dispensed a different drug or product from what was prescribed.). This is the underlying object with id, value and extensions. The accessor "getWasSubstituted" gives direct access to the value 523 */ 524 public BooleanType getWasSubstitutedElement() { 525 if (this.wasSubstituted == null) 526 if (Configuration.errorOnAutoCreate()) 527 throw new Error("Attempt to auto-create MedicationDispenseSubstitutionComponent.wasSubstituted"); 528 else if (Configuration.doAutoCreate()) 529 this.wasSubstituted = new BooleanType(); // bb 530 return this.wasSubstituted; 531 } 532 533 public boolean hasWasSubstitutedElement() { 534 return this.wasSubstituted != null && !this.wasSubstituted.isEmpty(); 535 } 536 537 public boolean hasWasSubstituted() { 538 return this.wasSubstituted != null && !this.wasSubstituted.isEmpty(); 539 } 540 541 /** 542 * @param value {@link #wasSubstituted} (True if the dispenser dispensed a different drug or product from what was prescribed.). This is the underlying object with id, value and extensions. The accessor "getWasSubstituted" gives direct access to the value 543 */ 544 public MedicationDispenseSubstitutionComponent setWasSubstitutedElement(BooleanType value) { 545 this.wasSubstituted = value; 546 return this; 547 } 548 549 /** 550 * @return True if the dispenser dispensed a different drug or product from what was prescribed. 551 */ 552 public boolean getWasSubstituted() { 553 return this.wasSubstituted == null || this.wasSubstituted.isEmpty() ? false : this.wasSubstituted.getValue(); 554 } 555 556 /** 557 * @param value True if the dispenser dispensed a different drug or product from what was prescribed. 558 */ 559 public MedicationDispenseSubstitutionComponent setWasSubstituted(boolean value) { 560 if (this.wasSubstituted == null) 561 this.wasSubstituted = new BooleanType(); 562 this.wasSubstituted.setValue(value); 563 return this; 564 } 565 566 /** 567 * @return {@link #type} (A code signifying whether a different drug was dispensed from what was prescribed.) 568 */ 569 public CodeableConcept getType() { 570 if (this.type == null) 571 if (Configuration.errorOnAutoCreate()) 572 throw new Error("Attempt to auto-create MedicationDispenseSubstitutionComponent.type"); 573 else if (Configuration.doAutoCreate()) 574 this.type = new CodeableConcept(); // cc 575 return this.type; 576 } 577 578 public boolean hasType() { 579 return this.type != null && !this.type.isEmpty(); 580 } 581 582 /** 583 * @param value {@link #type} (A code signifying whether a different drug was dispensed from what was prescribed.) 584 */ 585 public MedicationDispenseSubstitutionComponent setType(CodeableConcept value) { 586 this.type = value; 587 return this; 588 } 589 590 /** 591 * @return {@link #reason} (Indicates the reason for the substitution of (or lack of substitution) from what was prescribed.) 592 */ 593 public List<CodeableConcept> getReason() { 594 if (this.reason == null) 595 this.reason = new ArrayList<CodeableConcept>(); 596 return this.reason; 597 } 598 599 /** 600 * @return Returns a reference to <code>this</code> for easy method chaining 601 */ 602 public MedicationDispenseSubstitutionComponent setReason(List<CodeableConcept> theReason) { 603 this.reason = theReason; 604 return this; 605 } 606 607 public boolean hasReason() { 608 if (this.reason == null) 609 return false; 610 for (CodeableConcept item : this.reason) 611 if (!item.isEmpty()) 612 return true; 613 return false; 614 } 615 616 public CodeableConcept addReason() { //3 617 CodeableConcept t = new CodeableConcept(); 618 if (this.reason == null) 619 this.reason = new ArrayList<CodeableConcept>(); 620 this.reason.add(t); 621 return t; 622 } 623 624 public MedicationDispenseSubstitutionComponent addReason(CodeableConcept t) { //3 625 if (t == null) 626 return this; 627 if (this.reason == null) 628 this.reason = new ArrayList<CodeableConcept>(); 629 this.reason.add(t); 630 return this; 631 } 632 633 /** 634 * @return The first repetition of repeating field {@link #reason}, creating it if it does not already exist 635 */ 636 public CodeableConcept getReasonFirstRep() { 637 if (getReason().isEmpty()) { 638 addReason(); 639 } 640 return getReason().get(0); 641 } 642 643 /** 644 * @return {@link #responsibleParty} (The person or organization that has primary responsibility for the substitution.) 645 */ 646 public List<Reference> getResponsibleParty() { 647 if (this.responsibleParty == null) 648 this.responsibleParty = new ArrayList<Reference>(); 649 return this.responsibleParty; 650 } 651 652 /** 653 * @return Returns a reference to <code>this</code> for easy method chaining 654 */ 655 public MedicationDispenseSubstitutionComponent setResponsibleParty(List<Reference> theResponsibleParty) { 656 this.responsibleParty = theResponsibleParty; 657 return this; 658 } 659 660 public boolean hasResponsibleParty() { 661 if (this.responsibleParty == null) 662 return false; 663 for (Reference item : this.responsibleParty) 664 if (!item.isEmpty()) 665 return true; 666 return false; 667 } 668 669 public Reference addResponsibleParty() { //3 670 Reference t = new Reference(); 671 if (this.responsibleParty == null) 672 this.responsibleParty = new ArrayList<Reference>(); 673 this.responsibleParty.add(t); 674 return t; 675 } 676 677 public MedicationDispenseSubstitutionComponent addResponsibleParty(Reference t) { //3 678 if (t == null) 679 return this; 680 if (this.responsibleParty == null) 681 this.responsibleParty = new ArrayList<Reference>(); 682 this.responsibleParty.add(t); 683 return this; 684 } 685 686 /** 687 * @return The first repetition of repeating field {@link #responsibleParty}, creating it if it does not already exist 688 */ 689 public Reference getResponsiblePartyFirstRep() { 690 if (getResponsibleParty().isEmpty()) { 691 addResponsibleParty(); 692 } 693 return getResponsibleParty().get(0); 694 } 695 696 /** 697 * @deprecated Use Reference#setResource(IBaseResource) instead 698 */ 699 @Deprecated 700 public List<Practitioner> getResponsiblePartyTarget() { 701 if (this.responsiblePartyTarget == null) 702 this.responsiblePartyTarget = new ArrayList<Practitioner>(); 703 return this.responsiblePartyTarget; 704 } 705 706 /** 707 * @deprecated Use Reference#setResource(IBaseResource) instead 708 */ 709 @Deprecated 710 public Practitioner addResponsiblePartyTarget() { 711 Practitioner r = new Practitioner(); 712 if (this.responsiblePartyTarget == null) 713 this.responsiblePartyTarget = new ArrayList<Practitioner>(); 714 this.responsiblePartyTarget.add(r); 715 return r; 716 } 717 718 protected void listChildren(List<Property> children) { 719 super.listChildren(children); 720 children.add(new Property("wasSubstituted", "boolean", "True if the dispenser dispensed a different drug or product from what was prescribed.", 0, 1, wasSubstituted)); 721 children.add(new Property("type", "CodeableConcept", "A code signifying whether a different drug was dispensed from what was prescribed.", 0, 1, type)); 722 children.add(new Property("reason", "CodeableConcept", "Indicates the reason for the substitution of (or lack of substitution) from what was prescribed.", 0, java.lang.Integer.MAX_VALUE, reason)); 723 children.add(new Property("responsibleParty", "Reference(Practitioner)", "The person or organization that has primary responsibility for the substitution.", 0, java.lang.Integer.MAX_VALUE, responsibleParty)); 724 } 725 726 @Override 727 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 728 switch (_hash) { 729 case -592113567: /*wasSubstituted*/ return new Property("wasSubstituted", "boolean", "True if the dispenser dispensed a different drug or product from what was prescribed.", 0, 1, wasSubstituted); 730 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "A code signifying whether a different drug was dispensed from what was prescribed.", 0, 1, type); 731 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Indicates the reason for the substitution of (or lack of substitution) from what was prescribed.", 0, java.lang.Integer.MAX_VALUE, reason); 732 case 1511509392: /*responsibleParty*/ return new Property("responsibleParty", "Reference(Practitioner)", "The person or organization that has primary responsibility for the substitution.", 0, java.lang.Integer.MAX_VALUE, responsibleParty); 733 default: return super.getNamedProperty(_hash, _name, _checkValid); 734 } 735 736 } 737 738 @Override 739 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 740 switch (hash) { 741 case -592113567: /*wasSubstituted*/ return this.wasSubstituted == null ? new Base[0] : new Base[] {this.wasSubstituted}; // BooleanType 742 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 743 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : this.reason.toArray(new Base[this.reason.size()]); // CodeableConcept 744 case 1511509392: /*responsibleParty*/ return this.responsibleParty == null ? new Base[0] : this.responsibleParty.toArray(new Base[this.responsibleParty.size()]); // Reference 745 default: return super.getProperty(hash, name, checkValid); 746 } 747 748 } 749 750 @Override 751 public Base setProperty(int hash, String name, Base value) throws FHIRException { 752 switch (hash) { 753 case -592113567: // wasSubstituted 754 this.wasSubstituted = castToBoolean(value); // BooleanType 755 return value; 756 case 3575610: // type 757 this.type = castToCodeableConcept(value); // CodeableConcept 758 return value; 759 case -934964668: // reason 760 this.getReason().add(castToCodeableConcept(value)); // CodeableConcept 761 return value; 762 case 1511509392: // responsibleParty 763 this.getResponsibleParty().add(castToReference(value)); // Reference 764 return value; 765 default: return super.setProperty(hash, name, value); 766 } 767 768 } 769 770 @Override 771 public Base setProperty(String name, Base value) throws FHIRException { 772 if (name.equals("wasSubstituted")) { 773 this.wasSubstituted = castToBoolean(value); // BooleanType 774 } else if (name.equals("type")) { 775 this.type = castToCodeableConcept(value); // CodeableConcept 776 } else if (name.equals("reason")) { 777 this.getReason().add(castToCodeableConcept(value)); 778 } else if (name.equals("responsibleParty")) { 779 this.getResponsibleParty().add(castToReference(value)); 780 } else 781 return super.setProperty(name, value); 782 return value; 783 } 784 785 @Override 786 public Base makeProperty(int hash, String name) throws FHIRException { 787 switch (hash) { 788 case -592113567: return getWasSubstitutedElement(); 789 case 3575610: return getType(); 790 case -934964668: return addReason(); 791 case 1511509392: return addResponsibleParty(); 792 default: return super.makeProperty(hash, name); 793 } 794 795 } 796 797 @Override 798 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 799 switch (hash) { 800 case -592113567: /*wasSubstituted*/ return new String[] {"boolean"}; 801 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 802 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 803 case 1511509392: /*responsibleParty*/ return new String[] {"Reference"}; 804 default: return super.getTypesForProperty(hash, name); 805 } 806 807 } 808 809 @Override 810 public Base addChild(String name) throws FHIRException { 811 if (name.equals("wasSubstituted")) { 812 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.wasSubstituted"); 813 } 814 else if (name.equals("type")) { 815 this.type = new CodeableConcept(); 816 return this.type; 817 } 818 else if (name.equals("reason")) { 819 return addReason(); 820 } 821 else if (name.equals("responsibleParty")) { 822 return addResponsibleParty(); 823 } 824 else 825 return super.addChild(name); 826 } 827 828 public MedicationDispenseSubstitutionComponent copy() { 829 MedicationDispenseSubstitutionComponent dst = new MedicationDispenseSubstitutionComponent(); 830 copyValues(dst); 831 dst.wasSubstituted = wasSubstituted == null ? null : wasSubstituted.copy(); 832 dst.type = type == null ? null : type.copy(); 833 if (reason != null) { 834 dst.reason = new ArrayList<CodeableConcept>(); 835 for (CodeableConcept i : reason) 836 dst.reason.add(i.copy()); 837 }; 838 if (responsibleParty != null) { 839 dst.responsibleParty = new ArrayList<Reference>(); 840 for (Reference i : responsibleParty) 841 dst.responsibleParty.add(i.copy()); 842 }; 843 return dst; 844 } 845 846 @Override 847 public boolean equalsDeep(Base other_) { 848 if (!super.equalsDeep(other_)) 849 return false; 850 if (!(other_ instanceof MedicationDispenseSubstitutionComponent)) 851 return false; 852 MedicationDispenseSubstitutionComponent o = (MedicationDispenseSubstitutionComponent) other_; 853 return compareDeep(wasSubstituted, o.wasSubstituted, true) && compareDeep(type, o.type, true) && compareDeep(reason, o.reason, true) 854 && compareDeep(responsibleParty, o.responsibleParty, true); 855 } 856 857 @Override 858 public boolean equalsShallow(Base other_) { 859 if (!super.equalsShallow(other_)) 860 return false; 861 if (!(other_ instanceof MedicationDispenseSubstitutionComponent)) 862 return false; 863 MedicationDispenseSubstitutionComponent o = (MedicationDispenseSubstitutionComponent) other_; 864 return compareValues(wasSubstituted, o.wasSubstituted, true); 865 } 866 867 public boolean isEmpty() { 868 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(wasSubstituted, type, reason 869 , responsibleParty); 870 } 871 872 public String fhirType() { 873 return "MedicationDispense.substitution"; 874 875 } 876 877 } 878 879 /** 880 * Identifier assigned by the dispensing facility - this is an identifier assigned outside FHIR. 881 */ 882 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 883 @Description(shortDefinition="External identifier", formalDefinition="Identifier assigned by the dispensing facility - this is an identifier assigned outside FHIR." ) 884 protected List<Identifier> identifier; 885 886 /** 887 * The procedure that the dispense is done because of. 888 */ 889 @Child(name = "partOf", type = {Procedure.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 890 @Description(shortDefinition="Event that dispense is part of", formalDefinition="The procedure that the dispense is done because of." ) 891 protected List<Reference> partOf; 892 /** 893 * The actual objects that are the target of the reference (The procedure that the dispense is done because of.) 894 */ 895 protected List<Procedure> partOfTarget; 896 897 898 /** 899 * A code specifying the state of the set of dispense events. 900 */ 901 @Child(name = "status", type = {CodeType.class}, order=2, min=0, max=1, modifier=true, summary=true) 902 @Description(shortDefinition="preparation | in-progress | on-hold | completed | entered-in-error | stopped", formalDefinition="A code specifying the state of the set of dispense events." ) 903 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-dispense-status") 904 protected Enumeration<MedicationDispenseStatus> status; 905 906 /** 907 * Indicates type of medication dispense and where the medication is expected to be consumed or administered. 908 */ 909 @Child(name = "category", type = {CodeableConcept.class}, order=3, min=0, max=1, modifier=false, summary=false) 910 @Description(shortDefinition="Type of medication dispense", formalDefinition="Indicates type of medication dispense and where the medication is expected to be consumed or administered." ) 911 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-dispense-category") 912 protected CodeableConcept category; 913 914 /** 915 * Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications. 916 */ 917 @Child(name = "medication", type = {CodeableConcept.class, Medication.class}, order=4, min=1, max=1, modifier=false, summary=true) 918 @Description(shortDefinition="What medication was supplied", formalDefinition="Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications." ) 919 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 920 protected Type medication; 921 922 /** 923 * A link to a resource representing the person or the group to whom the medication will be given. 924 */ 925 @Child(name = "subject", type = {Patient.class, Group.class}, order=5, min=0, max=1, modifier=false, summary=true) 926 @Description(shortDefinition="Who the dispense is for", formalDefinition="A link to a resource representing the person or the group to whom the medication will be given." ) 927 protected Reference subject; 928 929 /** 930 * The actual object that is the target of the reference (A link to a resource representing the person or the group to whom the medication will be given.) 931 */ 932 protected Resource subjectTarget; 933 934 /** 935 * The encounter or episode of care that establishes the context for this event. 936 */ 937 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=6, min=0, max=1, modifier=false, summary=false) 938 @Description(shortDefinition="Encounter / Episode associated with event", formalDefinition="The encounter or episode of care that establishes the context for this event." ) 939 protected Reference context; 940 941 /** 942 * The actual object that is the target of the reference (The encounter or episode of care that establishes the context for this event.) 943 */ 944 protected Resource contextTarget; 945 946 /** 947 * Additional information that supports the medication being dispensed. 948 */ 949 @Child(name = "supportingInformation", type = {Reference.class}, order=7, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 950 @Description(shortDefinition="Information that supports the dispensing of the medication", formalDefinition="Additional information that supports the medication being dispensed." ) 951 protected List<Reference> supportingInformation; 952 /** 953 * The actual objects that are the target of the reference (Additional information that supports the medication being dispensed.) 954 */ 955 protected List<Resource> supportingInformationTarget; 956 957 958 /** 959 * Indicates who or what performed the event. It should be assumed that the performer is the dispenser of the medication. 960 */ 961 @Child(name = "performer", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 962 @Description(shortDefinition="Who performed event", formalDefinition="Indicates who or what performed the event. It should be assumed that the performer is the dispenser of the medication." ) 963 protected List<MedicationDispensePerformerComponent> performer; 964 965 /** 966 * Indicates the medication order that is being dispensed against. 967 */ 968 @Child(name = "authorizingPrescription", type = {MedicationRequest.class}, order=9, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 969 @Description(shortDefinition="Medication order that authorizes the dispense", formalDefinition="Indicates the medication order that is being dispensed against." ) 970 protected List<Reference> authorizingPrescription; 971 /** 972 * The actual objects that are the target of the reference (Indicates the medication order that is being dispensed against.) 973 */ 974 protected List<MedicationRequest> authorizingPrescriptionTarget; 975 976 977 /** 978 * Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc. 979 */ 980 @Child(name = "type", type = {CodeableConcept.class}, order=10, min=0, max=1, modifier=false, summary=false) 981 @Description(shortDefinition="Trial fill, partial fill, emergency fill, etc.", formalDefinition="Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc." ) 982 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-ActPharmacySupplyType") 983 protected CodeableConcept type; 984 985 /** 986 * The amount of medication that has been dispensed. Includes unit of measure. 987 */ 988 @Child(name = "quantity", type = {SimpleQuantity.class}, order=11, min=0, max=1, modifier=false, summary=false) 989 @Description(shortDefinition="Amount dispensed", formalDefinition="The amount of medication that has been dispensed. Includes unit of measure." ) 990 protected SimpleQuantity quantity; 991 992 /** 993 * The amount of medication expressed as a timing amount. 994 */ 995 @Child(name = "daysSupply", type = {SimpleQuantity.class}, order=12, min=0, max=1, modifier=false, summary=false) 996 @Description(shortDefinition="Amount of medication expressed as a timing amount", formalDefinition="The amount of medication expressed as a timing amount." ) 997 protected SimpleQuantity daysSupply; 998 999 /** 1000 * The time when the dispensed product was packaged and reviewed. 1001 */ 1002 @Child(name = "whenPrepared", type = {DateTimeType.class}, order=13, min=0, max=1, modifier=false, summary=true) 1003 @Description(shortDefinition="When product was packaged and reviewed", formalDefinition="The time when the dispensed product was packaged and reviewed." ) 1004 protected DateTimeType whenPrepared; 1005 1006 /** 1007 * The time the dispensed product was provided to the patient or their representative. 1008 */ 1009 @Child(name = "whenHandedOver", type = {DateTimeType.class}, order=14, min=0, max=1, modifier=false, summary=false) 1010 @Description(shortDefinition="When product was given out", formalDefinition="The time the dispensed product was provided to the patient or their representative." ) 1011 protected DateTimeType whenHandedOver; 1012 1013 /** 1014 * Identification of the facility/location where the medication was shipped to, as part of the dispense event. 1015 */ 1016 @Child(name = "destination", type = {Location.class}, order=15, min=0, max=1, modifier=false, summary=false) 1017 @Description(shortDefinition="Where the medication was sent", formalDefinition="Identification of the facility/location where the medication was shipped to, as part of the dispense event." ) 1018 protected Reference destination; 1019 1020 /** 1021 * The actual object that is the target of the reference (Identification of the facility/location where the medication was shipped to, as part of the dispense event.) 1022 */ 1023 protected Location destinationTarget; 1024 1025 /** 1026 * Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional. 1027 */ 1028 @Child(name = "receiver", type = {Patient.class, Practitioner.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1029 @Description(shortDefinition="Who collected the medication", formalDefinition="Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional." ) 1030 protected List<Reference> receiver; 1031 /** 1032 * The actual objects that are the target of the reference (Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.) 1033 */ 1034 protected List<Resource> receiverTarget; 1035 1036 1037 /** 1038 * Extra information about the dispense that could not be conveyed in the other attributes. 1039 */ 1040 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1041 @Description(shortDefinition="Information about the dispense", formalDefinition="Extra information about the dispense that could not be conveyed in the other attributes." ) 1042 protected List<Annotation> note; 1043 1044 /** 1045 * Indicates how the medication is to be used by the patient. 1046 */ 1047 @Child(name = "dosageInstruction", type = {Dosage.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1048 @Description(shortDefinition="How the medication is to be used by the patient or administered by the caregiver", formalDefinition="Indicates how the medication is to be used by the patient." ) 1049 protected List<Dosage> dosageInstruction; 1050 1051 /** 1052 * Indicates whether or not substitution was made as part of the dispense. In some cases substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done. 1053 */ 1054 @Child(name = "substitution", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 1055 @Description(shortDefinition="Whether a substitution was performed on the dispense", formalDefinition="Indicates whether or not substitution was made as part of the dispense. In some cases substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done." ) 1056 protected MedicationDispenseSubstitutionComponent substitution; 1057 1058 /** 1059 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc. 1060 */ 1061 @Child(name = "detectedIssue", type = {DetectedIssue.class}, order=20, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1062 @Description(shortDefinition="Clinical issue with action", formalDefinition="Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc." ) 1063 protected List<Reference> detectedIssue; 1064 /** 1065 * The actual objects that are the target of the reference (Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.) 1066 */ 1067 protected List<DetectedIssue> detectedIssueTarget; 1068 1069 1070 /** 1071 * True if the dispense was not performed for some reason. 1072 */ 1073 @Child(name = "notDone", type = {BooleanType.class}, order=21, min=0, max=1, modifier=false, summary=false) 1074 @Description(shortDefinition="Whether the dispense was or was not performed", formalDefinition="True if the dispense was not performed for some reason." ) 1075 protected BooleanType notDone; 1076 1077 /** 1078 * Indicates the reason why a dispense was not performed. 1079 */ 1080 @Child(name = "notDoneReason", type = {CodeableConcept.class, DetectedIssue.class}, order=22, min=0, max=1, modifier=false, summary=false) 1081 @Description(shortDefinition="Why a dispense was not performed", formalDefinition="Indicates the reason why a dispense was not performed." ) 1082 protected Type notDoneReason; 1083 1084 /** 1085 * A summary of the events of interest that have occurred, such as when the dispense was verified. 1086 */ 1087 @Child(name = "eventHistory", type = {Provenance.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1088 @Description(shortDefinition="A list of releveant lifecycle events", formalDefinition="A summary of the events of interest that have occurred, such as when the dispense was verified." ) 1089 protected List<Reference> eventHistory; 1090 /** 1091 * The actual objects that are the target of the reference (A summary of the events of interest that have occurred, such as when the dispense was verified.) 1092 */ 1093 protected List<Provenance> eventHistoryTarget; 1094 1095 1096 private static final long serialVersionUID = 1951426869L; 1097 1098 /** 1099 * Constructor 1100 */ 1101 public MedicationDispense() { 1102 super(); 1103 } 1104 1105 /** 1106 * Constructor 1107 */ 1108 public MedicationDispense(Type medication) { 1109 super(); 1110 this.medication = medication; 1111 } 1112 1113 /** 1114 * @return {@link #identifier} (Identifier assigned by the dispensing facility - this is an identifier assigned outside FHIR.) 1115 */ 1116 public List<Identifier> getIdentifier() { 1117 if (this.identifier == null) 1118 this.identifier = new ArrayList<Identifier>(); 1119 return this.identifier; 1120 } 1121 1122 /** 1123 * @return Returns a reference to <code>this</code> for easy method chaining 1124 */ 1125 public MedicationDispense setIdentifier(List<Identifier> theIdentifier) { 1126 this.identifier = theIdentifier; 1127 return this; 1128 } 1129 1130 public boolean hasIdentifier() { 1131 if (this.identifier == null) 1132 return false; 1133 for (Identifier item : this.identifier) 1134 if (!item.isEmpty()) 1135 return true; 1136 return false; 1137 } 1138 1139 public Identifier addIdentifier() { //3 1140 Identifier t = new Identifier(); 1141 if (this.identifier == null) 1142 this.identifier = new ArrayList<Identifier>(); 1143 this.identifier.add(t); 1144 return t; 1145 } 1146 1147 public MedicationDispense addIdentifier(Identifier t) { //3 1148 if (t == null) 1149 return this; 1150 if (this.identifier == null) 1151 this.identifier = new ArrayList<Identifier>(); 1152 this.identifier.add(t); 1153 return this; 1154 } 1155 1156 /** 1157 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1158 */ 1159 public Identifier getIdentifierFirstRep() { 1160 if (getIdentifier().isEmpty()) { 1161 addIdentifier(); 1162 } 1163 return getIdentifier().get(0); 1164 } 1165 1166 /** 1167 * @return {@link #partOf} (The procedure that the dispense is done because of.) 1168 */ 1169 public List<Reference> getPartOf() { 1170 if (this.partOf == null) 1171 this.partOf = new ArrayList<Reference>(); 1172 return this.partOf; 1173 } 1174 1175 /** 1176 * @return Returns a reference to <code>this</code> for easy method chaining 1177 */ 1178 public MedicationDispense setPartOf(List<Reference> thePartOf) { 1179 this.partOf = thePartOf; 1180 return this; 1181 } 1182 1183 public boolean hasPartOf() { 1184 if (this.partOf == null) 1185 return false; 1186 for (Reference item : this.partOf) 1187 if (!item.isEmpty()) 1188 return true; 1189 return false; 1190 } 1191 1192 public Reference addPartOf() { //3 1193 Reference t = new Reference(); 1194 if (this.partOf == null) 1195 this.partOf = new ArrayList<Reference>(); 1196 this.partOf.add(t); 1197 return t; 1198 } 1199 1200 public MedicationDispense addPartOf(Reference t) { //3 1201 if (t == null) 1202 return this; 1203 if (this.partOf == null) 1204 this.partOf = new ArrayList<Reference>(); 1205 this.partOf.add(t); 1206 return this; 1207 } 1208 1209 /** 1210 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 1211 */ 1212 public Reference getPartOfFirstRep() { 1213 if (getPartOf().isEmpty()) { 1214 addPartOf(); 1215 } 1216 return getPartOf().get(0); 1217 } 1218 1219 /** 1220 * @deprecated Use Reference#setResource(IBaseResource) instead 1221 */ 1222 @Deprecated 1223 public List<Procedure> getPartOfTarget() { 1224 if (this.partOfTarget == null) 1225 this.partOfTarget = new ArrayList<Procedure>(); 1226 return this.partOfTarget; 1227 } 1228 1229 /** 1230 * @deprecated Use Reference#setResource(IBaseResource) instead 1231 */ 1232 @Deprecated 1233 public Procedure addPartOfTarget() { 1234 Procedure r = new Procedure(); 1235 if (this.partOfTarget == null) 1236 this.partOfTarget = new ArrayList<Procedure>(); 1237 this.partOfTarget.add(r); 1238 return r; 1239 } 1240 1241 /** 1242 * @return {@link #status} (A code specifying the state of the set of dispense events.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1243 */ 1244 public Enumeration<MedicationDispenseStatus> getStatusElement() { 1245 if (this.status == null) 1246 if (Configuration.errorOnAutoCreate()) 1247 throw new Error("Attempt to auto-create MedicationDispense.status"); 1248 else if (Configuration.doAutoCreate()) 1249 this.status = new Enumeration<MedicationDispenseStatus>(new MedicationDispenseStatusEnumFactory()); // bb 1250 return this.status; 1251 } 1252 1253 public boolean hasStatusElement() { 1254 return this.status != null && !this.status.isEmpty(); 1255 } 1256 1257 public boolean hasStatus() { 1258 return this.status != null && !this.status.isEmpty(); 1259 } 1260 1261 /** 1262 * @param value {@link #status} (A code specifying the state of the set of dispense events.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1263 */ 1264 public MedicationDispense setStatusElement(Enumeration<MedicationDispenseStatus> value) { 1265 this.status = value; 1266 return this; 1267 } 1268 1269 /** 1270 * @return A code specifying the state of the set of dispense events. 1271 */ 1272 public MedicationDispenseStatus getStatus() { 1273 return this.status == null ? null : this.status.getValue(); 1274 } 1275 1276 /** 1277 * @param value A code specifying the state of the set of dispense events. 1278 */ 1279 public MedicationDispense setStatus(MedicationDispenseStatus value) { 1280 if (value == null) 1281 this.status = null; 1282 else { 1283 if (this.status == null) 1284 this.status = new Enumeration<MedicationDispenseStatus>(new MedicationDispenseStatusEnumFactory()); 1285 this.status.setValue(value); 1286 } 1287 return this; 1288 } 1289 1290 /** 1291 * @return {@link #category} (Indicates type of medication dispense and where the medication is expected to be consumed or administered.) 1292 */ 1293 public CodeableConcept getCategory() { 1294 if (this.category == null) 1295 if (Configuration.errorOnAutoCreate()) 1296 throw new Error("Attempt to auto-create MedicationDispense.category"); 1297 else if (Configuration.doAutoCreate()) 1298 this.category = new CodeableConcept(); // cc 1299 return this.category; 1300 } 1301 1302 public boolean hasCategory() { 1303 return this.category != null && !this.category.isEmpty(); 1304 } 1305 1306 /** 1307 * @param value {@link #category} (Indicates type of medication dispense and where the medication is expected to be consumed or administered.) 1308 */ 1309 public MedicationDispense setCategory(CodeableConcept value) { 1310 this.category = value; 1311 return this; 1312 } 1313 1314 /** 1315 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1316 */ 1317 public Type getMedication() { 1318 return this.medication; 1319 } 1320 1321 /** 1322 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1323 */ 1324 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1325 if (this.medication == null) 1326 return null; 1327 if (!(this.medication instanceof CodeableConcept)) 1328 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.medication.getClass().getName()+" was encountered"); 1329 return (CodeableConcept) this.medication; 1330 } 1331 1332 public boolean hasMedicationCodeableConcept() { 1333 return this != null && this.medication instanceof CodeableConcept; 1334 } 1335 1336 /** 1337 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1338 */ 1339 public Reference getMedicationReference() throws FHIRException { 1340 if (this.medication == null) 1341 return null; 1342 if (!(this.medication instanceof Reference)) 1343 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.medication.getClass().getName()+" was encountered"); 1344 return (Reference) this.medication; 1345 } 1346 1347 public boolean hasMedicationReference() { 1348 return this != null && this.medication instanceof Reference; 1349 } 1350 1351 public boolean hasMedication() { 1352 return this.medication != null && !this.medication.isEmpty(); 1353 } 1354 1355 /** 1356 * @param value {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 1357 */ 1358 public MedicationDispense setMedication(Type value) throws FHIRFormatError { 1359 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 1360 throw new FHIRFormatError("Not the right type for MedicationDispense.medication[x]: "+value.fhirType()); 1361 this.medication = value; 1362 return this; 1363 } 1364 1365 /** 1366 * @return {@link #subject} (A link to a resource representing the person or the group to whom the medication will be given.) 1367 */ 1368 public Reference getSubject() { 1369 if (this.subject == null) 1370 if (Configuration.errorOnAutoCreate()) 1371 throw new Error("Attempt to auto-create MedicationDispense.subject"); 1372 else if (Configuration.doAutoCreate()) 1373 this.subject = new Reference(); // cc 1374 return this.subject; 1375 } 1376 1377 public boolean hasSubject() { 1378 return this.subject != null && !this.subject.isEmpty(); 1379 } 1380 1381 /** 1382 * @param value {@link #subject} (A link to a resource representing the person or the group to whom the medication will be given.) 1383 */ 1384 public MedicationDispense setSubject(Reference value) { 1385 this.subject = value; 1386 return this; 1387 } 1388 1389 /** 1390 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A link to a resource representing the person or the group to whom the medication will be given.) 1391 */ 1392 public Resource getSubjectTarget() { 1393 return this.subjectTarget; 1394 } 1395 1396 /** 1397 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A link to a resource representing the person or the group to whom the medication will be given.) 1398 */ 1399 public MedicationDispense setSubjectTarget(Resource value) { 1400 this.subjectTarget = value; 1401 return this; 1402 } 1403 1404 /** 1405 * @return {@link #context} (The encounter or episode of care that establishes the context for this event.) 1406 */ 1407 public Reference getContext() { 1408 if (this.context == null) 1409 if (Configuration.errorOnAutoCreate()) 1410 throw new Error("Attempt to auto-create MedicationDispense.context"); 1411 else if (Configuration.doAutoCreate()) 1412 this.context = new Reference(); // cc 1413 return this.context; 1414 } 1415 1416 public boolean hasContext() { 1417 return this.context != null && !this.context.isEmpty(); 1418 } 1419 1420 /** 1421 * @param value {@link #context} (The encounter or episode of care that establishes the context for this event.) 1422 */ 1423 public MedicationDispense setContext(Reference value) { 1424 this.context = value; 1425 return this; 1426 } 1427 1428 /** 1429 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this event.) 1430 */ 1431 public Resource getContextTarget() { 1432 return this.contextTarget; 1433 } 1434 1435 /** 1436 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this event.) 1437 */ 1438 public MedicationDispense setContextTarget(Resource value) { 1439 this.contextTarget = value; 1440 return this; 1441 } 1442 1443 /** 1444 * @return {@link #supportingInformation} (Additional information that supports the medication being dispensed.) 1445 */ 1446 public List<Reference> getSupportingInformation() { 1447 if (this.supportingInformation == null) 1448 this.supportingInformation = new ArrayList<Reference>(); 1449 return this.supportingInformation; 1450 } 1451 1452 /** 1453 * @return Returns a reference to <code>this</code> for easy method chaining 1454 */ 1455 public MedicationDispense setSupportingInformation(List<Reference> theSupportingInformation) { 1456 this.supportingInformation = theSupportingInformation; 1457 return this; 1458 } 1459 1460 public boolean hasSupportingInformation() { 1461 if (this.supportingInformation == null) 1462 return false; 1463 for (Reference item : this.supportingInformation) 1464 if (!item.isEmpty()) 1465 return true; 1466 return false; 1467 } 1468 1469 public Reference addSupportingInformation() { //3 1470 Reference t = new Reference(); 1471 if (this.supportingInformation == null) 1472 this.supportingInformation = new ArrayList<Reference>(); 1473 this.supportingInformation.add(t); 1474 return t; 1475 } 1476 1477 public MedicationDispense addSupportingInformation(Reference t) { //3 1478 if (t == null) 1479 return this; 1480 if (this.supportingInformation == null) 1481 this.supportingInformation = new ArrayList<Reference>(); 1482 this.supportingInformation.add(t); 1483 return this; 1484 } 1485 1486 /** 1487 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist 1488 */ 1489 public Reference getSupportingInformationFirstRep() { 1490 if (getSupportingInformation().isEmpty()) { 1491 addSupportingInformation(); 1492 } 1493 return getSupportingInformation().get(0); 1494 } 1495 1496 /** 1497 * @deprecated Use Reference#setResource(IBaseResource) instead 1498 */ 1499 @Deprecated 1500 public List<Resource> getSupportingInformationTarget() { 1501 if (this.supportingInformationTarget == null) 1502 this.supportingInformationTarget = new ArrayList<Resource>(); 1503 return this.supportingInformationTarget; 1504 } 1505 1506 /** 1507 * @return {@link #performer} (Indicates who or what performed the event. It should be assumed that the performer is the dispenser of the medication.) 1508 */ 1509 public List<MedicationDispensePerformerComponent> getPerformer() { 1510 if (this.performer == null) 1511 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1512 return this.performer; 1513 } 1514 1515 /** 1516 * @return Returns a reference to <code>this</code> for easy method chaining 1517 */ 1518 public MedicationDispense setPerformer(List<MedicationDispensePerformerComponent> thePerformer) { 1519 this.performer = thePerformer; 1520 return this; 1521 } 1522 1523 public boolean hasPerformer() { 1524 if (this.performer == null) 1525 return false; 1526 for (MedicationDispensePerformerComponent item : this.performer) 1527 if (!item.isEmpty()) 1528 return true; 1529 return false; 1530 } 1531 1532 public MedicationDispensePerformerComponent addPerformer() { //3 1533 MedicationDispensePerformerComponent t = new MedicationDispensePerformerComponent(); 1534 if (this.performer == null) 1535 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1536 this.performer.add(t); 1537 return t; 1538 } 1539 1540 public MedicationDispense addPerformer(MedicationDispensePerformerComponent t) { //3 1541 if (t == null) 1542 return this; 1543 if (this.performer == null) 1544 this.performer = new ArrayList<MedicationDispensePerformerComponent>(); 1545 this.performer.add(t); 1546 return this; 1547 } 1548 1549 /** 1550 * @return The first repetition of repeating field {@link #performer}, creating it if it does not already exist 1551 */ 1552 public MedicationDispensePerformerComponent getPerformerFirstRep() { 1553 if (getPerformer().isEmpty()) { 1554 addPerformer(); 1555 } 1556 return getPerformer().get(0); 1557 } 1558 1559 /** 1560 * @return {@link #authorizingPrescription} (Indicates the medication order that is being dispensed against.) 1561 */ 1562 public List<Reference> getAuthorizingPrescription() { 1563 if (this.authorizingPrescription == null) 1564 this.authorizingPrescription = new ArrayList<Reference>(); 1565 return this.authorizingPrescription; 1566 } 1567 1568 /** 1569 * @return Returns a reference to <code>this</code> for easy method chaining 1570 */ 1571 public MedicationDispense setAuthorizingPrescription(List<Reference> theAuthorizingPrescription) { 1572 this.authorizingPrescription = theAuthorizingPrescription; 1573 return this; 1574 } 1575 1576 public boolean hasAuthorizingPrescription() { 1577 if (this.authorizingPrescription == null) 1578 return false; 1579 for (Reference item : this.authorizingPrescription) 1580 if (!item.isEmpty()) 1581 return true; 1582 return false; 1583 } 1584 1585 public Reference addAuthorizingPrescription() { //3 1586 Reference t = new Reference(); 1587 if (this.authorizingPrescription == null) 1588 this.authorizingPrescription = new ArrayList<Reference>(); 1589 this.authorizingPrescription.add(t); 1590 return t; 1591 } 1592 1593 public MedicationDispense addAuthorizingPrescription(Reference t) { //3 1594 if (t == null) 1595 return this; 1596 if (this.authorizingPrescription == null) 1597 this.authorizingPrescription = new ArrayList<Reference>(); 1598 this.authorizingPrescription.add(t); 1599 return this; 1600 } 1601 1602 /** 1603 * @return The first repetition of repeating field {@link #authorizingPrescription}, creating it if it does not already exist 1604 */ 1605 public Reference getAuthorizingPrescriptionFirstRep() { 1606 if (getAuthorizingPrescription().isEmpty()) { 1607 addAuthorizingPrescription(); 1608 } 1609 return getAuthorizingPrescription().get(0); 1610 } 1611 1612 /** 1613 * @deprecated Use Reference#setResource(IBaseResource) instead 1614 */ 1615 @Deprecated 1616 public List<MedicationRequest> getAuthorizingPrescriptionTarget() { 1617 if (this.authorizingPrescriptionTarget == null) 1618 this.authorizingPrescriptionTarget = new ArrayList<MedicationRequest>(); 1619 return this.authorizingPrescriptionTarget; 1620 } 1621 1622 /** 1623 * @deprecated Use Reference#setResource(IBaseResource) instead 1624 */ 1625 @Deprecated 1626 public MedicationRequest addAuthorizingPrescriptionTarget() { 1627 MedicationRequest r = new MedicationRequest(); 1628 if (this.authorizingPrescriptionTarget == null) 1629 this.authorizingPrescriptionTarget = new ArrayList<MedicationRequest>(); 1630 this.authorizingPrescriptionTarget.add(r); 1631 return r; 1632 } 1633 1634 /** 1635 * @return {@link #type} (Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.) 1636 */ 1637 public CodeableConcept getType() { 1638 if (this.type == null) 1639 if (Configuration.errorOnAutoCreate()) 1640 throw new Error("Attempt to auto-create MedicationDispense.type"); 1641 else if (Configuration.doAutoCreate()) 1642 this.type = new CodeableConcept(); // cc 1643 return this.type; 1644 } 1645 1646 public boolean hasType() { 1647 return this.type != null && !this.type.isEmpty(); 1648 } 1649 1650 /** 1651 * @param value {@link #type} (Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.) 1652 */ 1653 public MedicationDispense setType(CodeableConcept value) { 1654 this.type = value; 1655 return this; 1656 } 1657 1658 /** 1659 * @return {@link #quantity} (The amount of medication that has been dispensed. Includes unit of measure.) 1660 */ 1661 public SimpleQuantity getQuantity() { 1662 if (this.quantity == null) 1663 if (Configuration.errorOnAutoCreate()) 1664 throw new Error("Attempt to auto-create MedicationDispense.quantity"); 1665 else if (Configuration.doAutoCreate()) 1666 this.quantity = new SimpleQuantity(); // cc 1667 return this.quantity; 1668 } 1669 1670 public boolean hasQuantity() { 1671 return this.quantity != null && !this.quantity.isEmpty(); 1672 } 1673 1674 /** 1675 * @param value {@link #quantity} (The amount of medication that has been dispensed. Includes unit of measure.) 1676 */ 1677 public MedicationDispense setQuantity(SimpleQuantity value) { 1678 this.quantity = value; 1679 return this; 1680 } 1681 1682 /** 1683 * @return {@link #daysSupply} (The amount of medication expressed as a timing amount.) 1684 */ 1685 public SimpleQuantity getDaysSupply() { 1686 if (this.daysSupply == null) 1687 if (Configuration.errorOnAutoCreate()) 1688 throw new Error("Attempt to auto-create MedicationDispense.daysSupply"); 1689 else if (Configuration.doAutoCreate()) 1690 this.daysSupply = new SimpleQuantity(); // cc 1691 return this.daysSupply; 1692 } 1693 1694 public boolean hasDaysSupply() { 1695 return this.daysSupply != null && !this.daysSupply.isEmpty(); 1696 } 1697 1698 /** 1699 * @param value {@link #daysSupply} (The amount of medication expressed as a timing amount.) 1700 */ 1701 public MedicationDispense setDaysSupply(SimpleQuantity value) { 1702 this.daysSupply = value; 1703 return this; 1704 } 1705 1706 /** 1707 * @return {@link #whenPrepared} (The time when the dispensed product was packaged and reviewed.). This is the underlying object with id, value and extensions. The accessor "getWhenPrepared" gives direct access to the value 1708 */ 1709 public DateTimeType getWhenPreparedElement() { 1710 if (this.whenPrepared == null) 1711 if (Configuration.errorOnAutoCreate()) 1712 throw new Error("Attempt to auto-create MedicationDispense.whenPrepared"); 1713 else if (Configuration.doAutoCreate()) 1714 this.whenPrepared = new DateTimeType(); // bb 1715 return this.whenPrepared; 1716 } 1717 1718 public boolean hasWhenPreparedElement() { 1719 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 1720 } 1721 1722 public boolean hasWhenPrepared() { 1723 return this.whenPrepared != null && !this.whenPrepared.isEmpty(); 1724 } 1725 1726 /** 1727 * @param value {@link #whenPrepared} (The time when the dispensed product was packaged and reviewed.). This is the underlying object with id, value and extensions. The accessor "getWhenPrepared" gives direct access to the value 1728 */ 1729 public MedicationDispense setWhenPreparedElement(DateTimeType value) { 1730 this.whenPrepared = value; 1731 return this; 1732 } 1733 1734 /** 1735 * @return The time when the dispensed product was packaged and reviewed. 1736 */ 1737 public Date getWhenPrepared() { 1738 return this.whenPrepared == null ? null : this.whenPrepared.getValue(); 1739 } 1740 1741 /** 1742 * @param value The time when the dispensed product was packaged and reviewed. 1743 */ 1744 public MedicationDispense setWhenPrepared(Date value) { 1745 if (value == null) 1746 this.whenPrepared = null; 1747 else { 1748 if (this.whenPrepared == null) 1749 this.whenPrepared = new DateTimeType(); 1750 this.whenPrepared.setValue(value); 1751 } 1752 return this; 1753 } 1754 1755 /** 1756 * @return {@link #whenHandedOver} (The time the dispensed product was provided to the patient or their representative.). This is the underlying object with id, value and extensions. The accessor "getWhenHandedOver" gives direct access to the value 1757 */ 1758 public DateTimeType getWhenHandedOverElement() { 1759 if (this.whenHandedOver == null) 1760 if (Configuration.errorOnAutoCreate()) 1761 throw new Error("Attempt to auto-create MedicationDispense.whenHandedOver"); 1762 else if (Configuration.doAutoCreate()) 1763 this.whenHandedOver = new DateTimeType(); // bb 1764 return this.whenHandedOver; 1765 } 1766 1767 public boolean hasWhenHandedOverElement() { 1768 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1769 } 1770 1771 public boolean hasWhenHandedOver() { 1772 return this.whenHandedOver != null && !this.whenHandedOver.isEmpty(); 1773 } 1774 1775 /** 1776 * @param value {@link #whenHandedOver} (The time the dispensed product was provided to the patient or their representative.). This is the underlying object with id, value and extensions. The accessor "getWhenHandedOver" gives direct access to the value 1777 */ 1778 public MedicationDispense setWhenHandedOverElement(DateTimeType value) { 1779 this.whenHandedOver = value; 1780 return this; 1781 } 1782 1783 /** 1784 * @return The time the dispensed product was provided to the patient or their representative. 1785 */ 1786 public Date getWhenHandedOver() { 1787 return this.whenHandedOver == null ? null : this.whenHandedOver.getValue(); 1788 } 1789 1790 /** 1791 * @param value The time the dispensed product was provided to the patient or their representative. 1792 */ 1793 public MedicationDispense setWhenHandedOver(Date value) { 1794 if (value == null) 1795 this.whenHandedOver = null; 1796 else { 1797 if (this.whenHandedOver == null) 1798 this.whenHandedOver = new DateTimeType(); 1799 this.whenHandedOver.setValue(value); 1800 } 1801 return this; 1802 } 1803 1804 /** 1805 * @return {@link #destination} (Identification of the facility/location where the medication was shipped to, as part of the dispense event.) 1806 */ 1807 public Reference getDestination() { 1808 if (this.destination == null) 1809 if (Configuration.errorOnAutoCreate()) 1810 throw new Error("Attempt to auto-create MedicationDispense.destination"); 1811 else if (Configuration.doAutoCreate()) 1812 this.destination = new Reference(); // cc 1813 return this.destination; 1814 } 1815 1816 public boolean hasDestination() { 1817 return this.destination != null && !this.destination.isEmpty(); 1818 } 1819 1820 /** 1821 * @param value {@link #destination} (Identification of the facility/location where the medication was shipped to, as part of the dispense event.) 1822 */ 1823 public MedicationDispense setDestination(Reference value) { 1824 this.destination = value; 1825 return this; 1826 } 1827 1828 /** 1829 * @return {@link #destination} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Identification of the facility/location where the medication was shipped to, as part of the dispense event.) 1830 */ 1831 public Location getDestinationTarget() { 1832 if (this.destinationTarget == null) 1833 if (Configuration.errorOnAutoCreate()) 1834 throw new Error("Attempt to auto-create MedicationDispense.destination"); 1835 else if (Configuration.doAutoCreate()) 1836 this.destinationTarget = new Location(); // aa 1837 return this.destinationTarget; 1838 } 1839 1840 /** 1841 * @param value {@link #destination} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Identification of the facility/location where the medication was shipped to, as part of the dispense event.) 1842 */ 1843 public MedicationDispense setDestinationTarget(Location value) { 1844 this.destinationTarget = value; 1845 return this; 1846 } 1847 1848 /** 1849 * @return {@link #receiver} (Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.) 1850 */ 1851 public List<Reference> getReceiver() { 1852 if (this.receiver == null) 1853 this.receiver = new ArrayList<Reference>(); 1854 return this.receiver; 1855 } 1856 1857 /** 1858 * @return Returns a reference to <code>this</code> for easy method chaining 1859 */ 1860 public MedicationDispense setReceiver(List<Reference> theReceiver) { 1861 this.receiver = theReceiver; 1862 return this; 1863 } 1864 1865 public boolean hasReceiver() { 1866 if (this.receiver == null) 1867 return false; 1868 for (Reference item : this.receiver) 1869 if (!item.isEmpty()) 1870 return true; 1871 return false; 1872 } 1873 1874 public Reference addReceiver() { //3 1875 Reference t = new Reference(); 1876 if (this.receiver == null) 1877 this.receiver = new ArrayList<Reference>(); 1878 this.receiver.add(t); 1879 return t; 1880 } 1881 1882 public MedicationDispense addReceiver(Reference t) { //3 1883 if (t == null) 1884 return this; 1885 if (this.receiver == null) 1886 this.receiver = new ArrayList<Reference>(); 1887 this.receiver.add(t); 1888 return this; 1889 } 1890 1891 /** 1892 * @return The first repetition of repeating field {@link #receiver}, creating it if it does not already exist 1893 */ 1894 public Reference getReceiverFirstRep() { 1895 if (getReceiver().isEmpty()) { 1896 addReceiver(); 1897 } 1898 return getReceiver().get(0); 1899 } 1900 1901 /** 1902 * @deprecated Use Reference#setResource(IBaseResource) instead 1903 */ 1904 @Deprecated 1905 public List<Resource> getReceiverTarget() { 1906 if (this.receiverTarget == null) 1907 this.receiverTarget = new ArrayList<Resource>(); 1908 return this.receiverTarget; 1909 } 1910 1911 /** 1912 * @return {@link #note} (Extra information about the dispense that could not be conveyed in the other attributes.) 1913 */ 1914 public List<Annotation> getNote() { 1915 if (this.note == null) 1916 this.note = new ArrayList<Annotation>(); 1917 return this.note; 1918 } 1919 1920 /** 1921 * @return Returns a reference to <code>this</code> for easy method chaining 1922 */ 1923 public MedicationDispense setNote(List<Annotation> theNote) { 1924 this.note = theNote; 1925 return this; 1926 } 1927 1928 public boolean hasNote() { 1929 if (this.note == null) 1930 return false; 1931 for (Annotation item : this.note) 1932 if (!item.isEmpty()) 1933 return true; 1934 return false; 1935 } 1936 1937 public Annotation addNote() { //3 1938 Annotation t = new Annotation(); 1939 if (this.note == null) 1940 this.note = new ArrayList<Annotation>(); 1941 this.note.add(t); 1942 return t; 1943 } 1944 1945 public MedicationDispense addNote(Annotation t) { //3 1946 if (t == null) 1947 return this; 1948 if (this.note == null) 1949 this.note = new ArrayList<Annotation>(); 1950 this.note.add(t); 1951 return this; 1952 } 1953 1954 /** 1955 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1956 */ 1957 public Annotation getNoteFirstRep() { 1958 if (getNote().isEmpty()) { 1959 addNote(); 1960 } 1961 return getNote().get(0); 1962 } 1963 1964 /** 1965 * @return {@link #dosageInstruction} (Indicates how the medication is to be used by the patient.) 1966 */ 1967 public List<Dosage> getDosageInstruction() { 1968 if (this.dosageInstruction == null) 1969 this.dosageInstruction = new ArrayList<Dosage>(); 1970 return this.dosageInstruction; 1971 } 1972 1973 /** 1974 * @return Returns a reference to <code>this</code> for easy method chaining 1975 */ 1976 public MedicationDispense setDosageInstruction(List<Dosage> theDosageInstruction) { 1977 this.dosageInstruction = theDosageInstruction; 1978 return this; 1979 } 1980 1981 public boolean hasDosageInstruction() { 1982 if (this.dosageInstruction == null) 1983 return false; 1984 for (Dosage item : this.dosageInstruction) 1985 if (!item.isEmpty()) 1986 return true; 1987 return false; 1988 } 1989 1990 public Dosage addDosageInstruction() { //3 1991 Dosage t = new Dosage(); 1992 if (this.dosageInstruction == null) 1993 this.dosageInstruction = new ArrayList<Dosage>(); 1994 this.dosageInstruction.add(t); 1995 return t; 1996 } 1997 1998 public MedicationDispense addDosageInstruction(Dosage t) { //3 1999 if (t == null) 2000 return this; 2001 if (this.dosageInstruction == null) 2002 this.dosageInstruction = new ArrayList<Dosage>(); 2003 this.dosageInstruction.add(t); 2004 return this; 2005 } 2006 2007 /** 2008 * @return The first repetition of repeating field {@link #dosageInstruction}, creating it if it does not already exist 2009 */ 2010 public Dosage getDosageInstructionFirstRep() { 2011 if (getDosageInstruction().isEmpty()) { 2012 addDosageInstruction(); 2013 } 2014 return getDosageInstruction().get(0); 2015 } 2016 2017 /** 2018 * @return {@link #substitution} (Indicates whether or not substitution was made as part of the dispense. In some cases substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.) 2019 */ 2020 public MedicationDispenseSubstitutionComponent getSubstitution() { 2021 if (this.substitution == null) 2022 if (Configuration.errorOnAutoCreate()) 2023 throw new Error("Attempt to auto-create MedicationDispense.substitution"); 2024 else if (Configuration.doAutoCreate()) 2025 this.substitution = new MedicationDispenseSubstitutionComponent(); // cc 2026 return this.substitution; 2027 } 2028 2029 public boolean hasSubstitution() { 2030 return this.substitution != null && !this.substitution.isEmpty(); 2031 } 2032 2033 /** 2034 * @param value {@link #substitution} (Indicates whether or not substitution was made as part of the dispense. In some cases substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.) 2035 */ 2036 public MedicationDispense setSubstitution(MedicationDispenseSubstitutionComponent value) { 2037 this.substitution = value; 2038 return this; 2039 } 2040 2041 /** 2042 * @return {@link #detectedIssue} (Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.) 2043 */ 2044 public List<Reference> getDetectedIssue() { 2045 if (this.detectedIssue == null) 2046 this.detectedIssue = new ArrayList<Reference>(); 2047 return this.detectedIssue; 2048 } 2049 2050 /** 2051 * @return Returns a reference to <code>this</code> for easy method chaining 2052 */ 2053 public MedicationDispense setDetectedIssue(List<Reference> theDetectedIssue) { 2054 this.detectedIssue = theDetectedIssue; 2055 return this; 2056 } 2057 2058 public boolean hasDetectedIssue() { 2059 if (this.detectedIssue == null) 2060 return false; 2061 for (Reference item : this.detectedIssue) 2062 if (!item.isEmpty()) 2063 return true; 2064 return false; 2065 } 2066 2067 public Reference addDetectedIssue() { //3 2068 Reference t = new Reference(); 2069 if (this.detectedIssue == null) 2070 this.detectedIssue = new ArrayList<Reference>(); 2071 this.detectedIssue.add(t); 2072 return t; 2073 } 2074 2075 public MedicationDispense addDetectedIssue(Reference t) { //3 2076 if (t == null) 2077 return this; 2078 if (this.detectedIssue == null) 2079 this.detectedIssue = new ArrayList<Reference>(); 2080 this.detectedIssue.add(t); 2081 return this; 2082 } 2083 2084 /** 2085 * @return The first repetition of repeating field {@link #detectedIssue}, creating it if it does not already exist 2086 */ 2087 public Reference getDetectedIssueFirstRep() { 2088 if (getDetectedIssue().isEmpty()) { 2089 addDetectedIssue(); 2090 } 2091 return getDetectedIssue().get(0); 2092 } 2093 2094 /** 2095 * @deprecated Use Reference#setResource(IBaseResource) instead 2096 */ 2097 @Deprecated 2098 public List<DetectedIssue> getDetectedIssueTarget() { 2099 if (this.detectedIssueTarget == null) 2100 this.detectedIssueTarget = new ArrayList<DetectedIssue>(); 2101 return this.detectedIssueTarget; 2102 } 2103 2104 /** 2105 * @deprecated Use Reference#setResource(IBaseResource) instead 2106 */ 2107 @Deprecated 2108 public DetectedIssue addDetectedIssueTarget() { 2109 DetectedIssue r = new DetectedIssue(); 2110 if (this.detectedIssueTarget == null) 2111 this.detectedIssueTarget = new ArrayList<DetectedIssue>(); 2112 this.detectedIssueTarget.add(r); 2113 return r; 2114 } 2115 2116 /** 2117 * @return {@link #notDone} (True if the dispense was not performed for some reason.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 2118 */ 2119 public BooleanType getNotDoneElement() { 2120 if (this.notDone == null) 2121 if (Configuration.errorOnAutoCreate()) 2122 throw new Error("Attempt to auto-create MedicationDispense.notDone"); 2123 else if (Configuration.doAutoCreate()) 2124 this.notDone = new BooleanType(); // bb 2125 return this.notDone; 2126 } 2127 2128 public boolean hasNotDoneElement() { 2129 return this.notDone != null && !this.notDone.isEmpty(); 2130 } 2131 2132 public boolean hasNotDone() { 2133 return this.notDone != null && !this.notDone.isEmpty(); 2134 } 2135 2136 /** 2137 * @param value {@link #notDone} (True if the dispense was not performed for some reason.). This is the underlying object with id, value and extensions. The accessor "getNotDone" gives direct access to the value 2138 */ 2139 public MedicationDispense setNotDoneElement(BooleanType value) { 2140 this.notDone = value; 2141 return this; 2142 } 2143 2144 /** 2145 * @return True if the dispense was not performed for some reason. 2146 */ 2147 public boolean getNotDone() { 2148 return this.notDone == null || this.notDone.isEmpty() ? false : this.notDone.getValue(); 2149 } 2150 2151 /** 2152 * @param value True if the dispense was not performed for some reason. 2153 */ 2154 public MedicationDispense setNotDone(boolean value) { 2155 if (this.notDone == null) 2156 this.notDone = new BooleanType(); 2157 this.notDone.setValue(value); 2158 return this; 2159 } 2160 2161 /** 2162 * @return {@link #notDoneReason} (Indicates the reason why a dispense was not performed.) 2163 */ 2164 public Type getNotDoneReason() { 2165 return this.notDoneReason; 2166 } 2167 2168 /** 2169 * @return {@link #notDoneReason} (Indicates the reason why a dispense was not performed.) 2170 */ 2171 public CodeableConcept getNotDoneReasonCodeableConcept() throws FHIRException { 2172 if (this.notDoneReason == null) 2173 return null; 2174 if (!(this.notDoneReason instanceof CodeableConcept)) 2175 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.notDoneReason.getClass().getName()+" was encountered"); 2176 return (CodeableConcept) this.notDoneReason; 2177 } 2178 2179 public boolean hasNotDoneReasonCodeableConcept() { 2180 return this != null && this.notDoneReason instanceof CodeableConcept; 2181 } 2182 2183 /** 2184 * @return {@link #notDoneReason} (Indicates the reason why a dispense was not performed.) 2185 */ 2186 public Reference getNotDoneReasonReference() throws FHIRException { 2187 if (this.notDoneReason == null) 2188 return null; 2189 if (!(this.notDoneReason instanceof Reference)) 2190 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.notDoneReason.getClass().getName()+" was encountered"); 2191 return (Reference) this.notDoneReason; 2192 } 2193 2194 public boolean hasNotDoneReasonReference() { 2195 return this != null && this.notDoneReason instanceof Reference; 2196 } 2197 2198 public boolean hasNotDoneReason() { 2199 return this.notDoneReason != null && !this.notDoneReason.isEmpty(); 2200 } 2201 2202 /** 2203 * @param value {@link #notDoneReason} (Indicates the reason why a dispense was not performed.) 2204 */ 2205 public MedicationDispense setNotDoneReason(Type value) throws FHIRFormatError { 2206 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2207 throw new FHIRFormatError("Not the right type for MedicationDispense.notDoneReason[x]: "+value.fhirType()); 2208 this.notDoneReason = value; 2209 return this; 2210 } 2211 2212 /** 2213 * @return {@link #eventHistory} (A summary of the events of interest that have occurred, such as when the dispense was verified.) 2214 */ 2215 public List<Reference> getEventHistory() { 2216 if (this.eventHistory == null) 2217 this.eventHistory = new ArrayList<Reference>(); 2218 return this.eventHistory; 2219 } 2220 2221 /** 2222 * @return Returns a reference to <code>this</code> for easy method chaining 2223 */ 2224 public MedicationDispense setEventHistory(List<Reference> theEventHistory) { 2225 this.eventHistory = theEventHistory; 2226 return this; 2227 } 2228 2229 public boolean hasEventHistory() { 2230 if (this.eventHistory == null) 2231 return false; 2232 for (Reference item : this.eventHistory) 2233 if (!item.isEmpty()) 2234 return true; 2235 return false; 2236 } 2237 2238 public Reference addEventHistory() { //3 2239 Reference t = new Reference(); 2240 if (this.eventHistory == null) 2241 this.eventHistory = new ArrayList<Reference>(); 2242 this.eventHistory.add(t); 2243 return t; 2244 } 2245 2246 public MedicationDispense addEventHistory(Reference t) { //3 2247 if (t == null) 2248 return this; 2249 if (this.eventHistory == null) 2250 this.eventHistory = new ArrayList<Reference>(); 2251 this.eventHistory.add(t); 2252 return this; 2253 } 2254 2255 /** 2256 * @return The first repetition of repeating field {@link #eventHistory}, creating it if it does not already exist 2257 */ 2258 public Reference getEventHistoryFirstRep() { 2259 if (getEventHistory().isEmpty()) { 2260 addEventHistory(); 2261 } 2262 return getEventHistory().get(0); 2263 } 2264 2265 /** 2266 * @deprecated Use Reference#setResource(IBaseResource) instead 2267 */ 2268 @Deprecated 2269 public List<Provenance> getEventHistoryTarget() { 2270 if (this.eventHistoryTarget == null) 2271 this.eventHistoryTarget = new ArrayList<Provenance>(); 2272 return this.eventHistoryTarget; 2273 } 2274 2275 /** 2276 * @deprecated Use Reference#setResource(IBaseResource) instead 2277 */ 2278 @Deprecated 2279 public Provenance addEventHistoryTarget() { 2280 Provenance r = new Provenance(); 2281 if (this.eventHistoryTarget == null) 2282 this.eventHistoryTarget = new ArrayList<Provenance>(); 2283 this.eventHistoryTarget.add(r); 2284 return r; 2285 } 2286 2287 protected void listChildren(List<Property> children) { 2288 super.listChildren(children); 2289 children.add(new Property("identifier", "Identifier", "Identifier assigned by the dispensing facility - this is an identifier assigned outside FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2290 children.add(new Property("partOf", "Reference(Procedure)", "The procedure that the dispense is done because of.", 0, java.lang.Integer.MAX_VALUE, partOf)); 2291 children.add(new Property("status", "code", "A code specifying the state of the set of dispense events.", 0, 1, status)); 2292 children.add(new Property("category", "CodeableConcept", "Indicates type of medication dispense and where the medication is expected to be consumed or administered.", 0, 1, category)); 2293 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 2294 children.add(new Property("subject", "Reference(Patient|Group)", "A link to a resource representing the person or the group to whom the medication will be given.", 0, 1, subject)); 2295 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this event.", 0, 1, context)); 2296 children.add(new Property("supportingInformation", "Reference(Any)", "Additional information that supports the medication being dispensed.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2297 children.add(new Property("performer", "", "Indicates who or what performed the event. It should be assumed that the performer is the dispenser of the medication.", 0, java.lang.Integer.MAX_VALUE, performer)); 2298 children.add(new Property("authorizingPrescription", "Reference(MedicationRequest)", "Indicates the medication order that is being dispensed against.", 0, java.lang.Integer.MAX_VALUE, authorizingPrescription)); 2299 children.add(new Property("type", "CodeableConcept", "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 0, 1, type)); 2300 children.add(new Property("quantity", "SimpleQuantity", "The amount of medication that has been dispensed. Includes unit of measure.", 0, 1, quantity)); 2301 children.add(new Property("daysSupply", "SimpleQuantity", "The amount of medication expressed as a timing amount.", 0, 1, daysSupply)); 2302 children.add(new Property("whenPrepared", "dateTime", "The time when the dispensed product was packaged and reviewed.", 0, 1, whenPrepared)); 2303 children.add(new Property("whenHandedOver", "dateTime", "The time the dispensed product was provided to the patient or their representative.", 0, 1, whenHandedOver)); 2304 children.add(new Property("destination", "Reference(Location)", "Identification of the facility/location where the medication was shipped to, as part of the dispense event.", 0, 1, destination)); 2305 children.add(new Property("receiver", "Reference(Patient|Practitioner)", "Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.", 0, java.lang.Integer.MAX_VALUE, receiver)); 2306 children.add(new Property("note", "Annotation", "Extra information about the dispense that could not be conveyed in the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2307 children.add(new Property("dosageInstruction", "Dosage", "Indicates how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 2308 children.add(new Property("substitution", "", "Indicates whether or not substitution was made as part of the dispense. In some cases substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.", 0, 1, substitution)); 2309 children.add(new Property("detectedIssue", "Reference(DetectedIssue)", "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.", 0, java.lang.Integer.MAX_VALUE, detectedIssue)); 2310 children.add(new Property("notDone", "boolean", "True if the dispense was not performed for some reason.", 0, 1, notDone)); 2311 children.add(new Property("notDoneReason[x]", "CodeableConcept|Reference(DetectedIssue)", "Indicates the reason why a dispense was not performed.", 0, 1, notDoneReason)); 2312 children.add(new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the dispense was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory)); 2313 } 2314 2315 @Override 2316 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2317 switch (_hash) { 2318 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "Identifier assigned by the dispensing facility - this is an identifier assigned outside FHIR.", 0, java.lang.Integer.MAX_VALUE, identifier); 2319 case -995410646: /*partOf*/ return new Property("partOf", "Reference(Procedure)", "The procedure that the dispense is done because of.", 0, java.lang.Integer.MAX_VALUE, partOf); 2320 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the state of the set of dispense events.", 0, 1, status); 2321 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates type of medication dispense and where the medication is expected to be consumed or administered.", 0, 1, category); 2322 case 1458402129: /*medication[x]*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2323 case 1998965455: /*medication*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2324 case -209845038: /*medicationCodeableConcept*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2325 case 2104315196: /*medicationReference*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2326 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "A link to a resource representing the person or the group to whom the medication will be given.", 0, 1, subject); 2327 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this event.", 0, 1, context); 2328 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Additional information that supports the medication being dispensed.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2329 case 481140686: /*performer*/ return new Property("performer", "", "Indicates who or what performed the event. It should be assumed that the performer is the dispenser of the medication.", 0, java.lang.Integer.MAX_VALUE, performer); 2330 case -1237557856: /*authorizingPrescription*/ return new Property("authorizingPrescription", "Reference(MedicationRequest)", "Indicates the medication order that is being dispensed against.", 0, java.lang.Integer.MAX_VALUE, authorizingPrescription); 2331 case 3575610: /*type*/ return new Property("type", "CodeableConcept", "Indicates the type of dispensing event that is performed. For example, Trial Fill, Completion of Trial, Partial Fill, Emergency Fill, Samples, etc.", 0, 1, type); 2332 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The amount of medication that has been dispensed. Includes unit of measure.", 0, 1, quantity); 2333 case 197175334: /*daysSupply*/ return new Property("daysSupply", "SimpleQuantity", "The amount of medication expressed as a timing amount.", 0, 1, daysSupply); 2334 case -562837097: /*whenPrepared*/ return new Property("whenPrepared", "dateTime", "The time when the dispensed product was packaged and reviewed.", 0, 1, whenPrepared); 2335 case -940241380: /*whenHandedOver*/ return new Property("whenHandedOver", "dateTime", "The time the dispensed product was provided to the patient or their representative.", 0, 1, whenHandedOver); 2336 case -1429847026: /*destination*/ return new Property("destination", "Reference(Location)", "Identification of the facility/location where the medication was shipped to, as part of the dispense event.", 0, 1, destination); 2337 case -808719889: /*receiver*/ return new Property("receiver", "Reference(Patient|Practitioner)", "Identifies the person who picked up the medication. This will usually be a patient or their caregiver, but some cases exist where it can be a healthcare professional.", 0, java.lang.Integer.MAX_VALUE, receiver); 2338 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the dispense that could not be conveyed in the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2339 case -1201373865: /*dosageInstruction*/ return new Property("dosageInstruction", "Dosage", "Indicates how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction); 2340 case 826147581: /*substitution*/ return new Property("substitution", "", "Indicates whether or not substitution was made as part of the dispense. In some cases substitution will be expected but does not happen, in other cases substitution is not expected but does happen. This block explains what substitution did or did not happen and why. If nothing is specified, substitution was not done.", 0, 1, substitution); 2341 case 51602295: /*detectedIssue*/ return new Property("detectedIssue", "Reference(DetectedIssue)", "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.", 0, java.lang.Integer.MAX_VALUE, detectedIssue); 2342 case 2128257269: /*notDone*/ return new Property("notDone", "boolean", "True if the dispense was not performed for some reason.", 0, 1, notDone); 2343 case -1762771385: /*notDoneReason[x]*/ return new Property("notDoneReason[x]", "CodeableConcept|Reference(DetectedIssue)", "Indicates the reason why a dispense was not performed.", 0, 1, notDoneReason); 2344 case -1973169255: /*notDoneReason*/ return new Property("notDoneReason[x]", "CodeableConcept|Reference(DetectedIssue)", "Indicates the reason why a dispense was not performed.", 0, 1, notDoneReason); 2345 case -930607416: /*notDoneReasonCodeableConcept*/ return new Property("notDoneReason[x]", "CodeableConcept|Reference(DetectedIssue)", "Indicates the reason why a dispense was not performed.", 0, 1, notDoneReason); 2346 case 1030457266: /*notDoneReasonReference*/ return new Property("notDoneReason[x]", "CodeableConcept|Reference(DetectedIssue)", "Indicates the reason why a dispense was not performed.", 0, 1, notDoneReason); 2347 case 1835190426: /*eventHistory*/ return new Property("eventHistory", "Reference(Provenance)", "A summary of the events of interest that have occurred, such as when the dispense was verified.", 0, java.lang.Integer.MAX_VALUE, eventHistory); 2348 default: return super.getNamedProperty(_hash, _name, _checkValid); 2349 } 2350 2351 } 2352 2353 @Override 2354 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2355 switch (hash) { 2356 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2357 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 2358 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationDispenseStatus> 2359 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2360 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // Type 2361 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2362 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2363 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2364 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : this.performer.toArray(new Base[this.performer.size()]); // MedicationDispensePerformerComponent 2365 case -1237557856: /*authorizingPrescription*/ return this.authorizingPrescription == null ? new Base[0] : this.authorizingPrescription.toArray(new Base[this.authorizingPrescription.size()]); // Reference 2366 case 3575610: /*type*/ return this.type == null ? new Base[0] : new Base[] {this.type}; // CodeableConcept 2367 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 2368 case 197175334: /*daysSupply*/ return this.daysSupply == null ? new Base[0] : new Base[] {this.daysSupply}; // SimpleQuantity 2369 case -562837097: /*whenPrepared*/ return this.whenPrepared == null ? new Base[0] : new Base[] {this.whenPrepared}; // DateTimeType 2370 case -940241380: /*whenHandedOver*/ return this.whenHandedOver == null ? new Base[0] : new Base[] {this.whenHandedOver}; // DateTimeType 2371 case -1429847026: /*destination*/ return this.destination == null ? new Base[0] : new Base[] {this.destination}; // Reference 2372 case -808719889: /*receiver*/ return this.receiver == null ? new Base[0] : this.receiver.toArray(new Base[this.receiver.size()]); // Reference 2373 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2374 case -1201373865: /*dosageInstruction*/ return this.dosageInstruction == null ? new Base[0] : this.dosageInstruction.toArray(new Base[this.dosageInstruction.size()]); // Dosage 2375 case 826147581: /*substitution*/ return this.substitution == null ? new Base[0] : new Base[] {this.substitution}; // MedicationDispenseSubstitutionComponent 2376 case 51602295: /*detectedIssue*/ return this.detectedIssue == null ? new Base[0] : this.detectedIssue.toArray(new Base[this.detectedIssue.size()]); // Reference 2377 case 2128257269: /*notDone*/ return this.notDone == null ? new Base[0] : new Base[] {this.notDone}; // BooleanType 2378 case -1973169255: /*notDoneReason*/ return this.notDoneReason == null ? new Base[0] : new Base[] {this.notDoneReason}; // Type 2379 case 1835190426: /*eventHistory*/ return this.eventHistory == null ? new Base[0] : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 2380 default: return super.getProperty(hash, name, checkValid); 2381 } 2382 2383 } 2384 2385 @Override 2386 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2387 switch (hash) { 2388 case -1618432855: // identifier 2389 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2390 return value; 2391 case -995410646: // partOf 2392 this.getPartOf().add(castToReference(value)); // Reference 2393 return value; 2394 case -892481550: // status 2395 value = new MedicationDispenseStatusEnumFactory().fromType(castToCode(value)); 2396 this.status = (Enumeration) value; // Enumeration<MedicationDispenseStatus> 2397 return value; 2398 case 50511102: // category 2399 this.category = castToCodeableConcept(value); // CodeableConcept 2400 return value; 2401 case 1998965455: // medication 2402 this.medication = castToType(value); // Type 2403 return value; 2404 case -1867885268: // subject 2405 this.subject = castToReference(value); // Reference 2406 return value; 2407 case 951530927: // context 2408 this.context = castToReference(value); // Reference 2409 return value; 2410 case -1248768647: // supportingInformation 2411 this.getSupportingInformation().add(castToReference(value)); // Reference 2412 return value; 2413 case 481140686: // performer 2414 this.getPerformer().add((MedicationDispensePerformerComponent) value); // MedicationDispensePerformerComponent 2415 return value; 2416 case -1237557856: // authorizingPrescription 2417 this.getAuthorizingPrescription().add(castToReference(value)); // Reference 2418 return value; 2419 case 3575610: // type 2420 this.type = castToCodeableConcept(value); // CodeableConcept 2421 return value; 2422 case -1285004149: // quantity 2423 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2424 return value; 2425 case 197175334: // daysSupply 2426 this.daysSupply = castToSimpleQuantity(value); // SimpleQuantity 2427 return value; 2428 case -562837097: // whenPrepared 2429 this.whenPrepared = castToDateTime(value); // DateTimeType 2430 return value; 2431 case -940241380: // whenHandedOver 2432 this.whenHandedOver = castToDateTime(value); // DateTimeType 2433 return value; 2434 case -1429847026: // destination 2435 this.destination = castToReference(value); // Reference 2436 return value; 2437 case -808719889: // receiver 2438 this.getReceiver().add(castToReference(value)); // Reference 2439 return value; 2440 case 3387378: // note 2441 this.getNote().add(castToAnnotation(value)); // Annotation 2442 return value; 2443 case -1201373865: // dosageInstruction 2444 this.getDosageInstruction().add(castToDosage(value)); // Dosage 2445 return value; 2446 case 826147581: // substitution 2447 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 2448 return value; 2449 case 51602295: // detectedIssue 2450 this.getDetectedIssue().add(castToReference(value)); // Reference 2451 return value; 2452 case 2128257269: // notDone 2453 this.notDone = castToBoolean(value); // BooleanType 2454 return value; 2455 case -1973169255: // notDoneReason 2456 this.notDoneReason = castToType(value); // Type 2457 return value; 2458 case 1835190426: // eventHistory 2459 this.getEventHistory().add(castToReference(value)); // Reference 2460 return value; 2461 default: return super.setProperty(hash, name, value); 2462 } 2463 2464 } 2465 2466 @Override 2467 public Base setProperty(String name, Base value) throws FHIRException { 2468 if (name.equals("identifier")) { 2469 this.getIdentifier().add(castToIdentifier(value)); 2470 } else if (name.equals("partOf")) { 2471 this.getPartOf().add(castToReference(value)); 2472 } else if (name.equals("status")) { 2473 value = new MedicationDispenseStatusEnumFactory().fromType(castToCode(value)); 2474 this.status = (Enumeration) value; // Enumeration<MedicationDispenseStatus> 2475 } else if (name.equals("category")) { 2476 this.category = castToCodeableConcept(value); // CodeableConcept 2477 } else if (name.equals("medication[x]")) { 2478 this.medication = castToType(value); // Type 2479 } else if (name.equals("subject")) { 2480 this.subject = castToReference(value); // Reference 2481 } else if (name.equals("context")) { 2482 this.context = castToReference(value); // Reference 2483 } else if (name.equals("supportingInformation")) { 2484 this.getSupportingInformation().add(castToReference(value)); 2485 } else if (name.equals("performer")) { 2486 this.getPerformer().add((MedicationDispensePerformerComponent) value); 2487 } else if (name.equals("authorizingPrescription")) { 2488 this.getAuthorizingPrescription().add(castToReference(value)); 2489 } else if (name.equals("type")) { 2490 this.type = castToCodeableConcept(value); // CodeableConcept 2491 } else if (name.equals("quantity")) { 2492 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 2493 } else if (name.equals("daysSupply")) { 2494 this.daysSupply = castToSimpleQuantity(value); // SimpleQuantity 2495 } else if (name.equals("whenPrepared")) { 2496 this.whenPrepared = castToDateTime(value); // DateTimeType 2497 } else if (name.equals("whenHandedOver")) { 2498 this.whenHandedOver = castToDateTime(value); // DateTimeType 2499 } else if (name.equals("destination")) { 2500 this.destination = castToReference(value); // Reference 2501 } else if (name.equals("receiver")) { 2502 this.getReceiver().add(castToReference(value)); 2503 } else if (name.equals("note")) { 2504 this.getNote().add(castToAnnotation(value)); 2505 } else if (name.equals("dosageInstruction")) { 2506 this.getDosageInstruction().add(castToDosage(value)); 2507 } else if (name.equals("substitution")) { 2508 this.substitution = (MedicationDispenseSubstitutionComponent) value; // MedicationDispenseSubstitutionComponent 2509 } else if (name.equals("detectedIssue")) { 2510 this.getDetectedIssue().add(castToReference(value)); 2511 } else if (name.equals("notDone")) { 2512 this.notDone = castToBoolean(value); // BooleanType 2513 } else if (name.equals("notDoneReason[x]")) { 2514 this.notDoneReason = castToType(value); // Type 2515 } else if (name.equals("eventHistory")) { 2516 this.getEventHistory().add(castToReference(value)); 2517 } else 2518 return super.setProperty(name, value); 2519 return value; 2520 } 2521 2522 @Override 2523 public Base makeProperty(int hash, String name) throws FHIRException { 2524 switch (hash) { 2525 case -1618432855: return addIdentifier(); 2526 case -995410646: return addPartOf(); 2527 case -892481550: return getStatusElement(); 2528 case 50511102: return getCategory(); 2529 case 1458402129: return getMedication(); 2530 case 1998965455: return getMedication(); 2531 case -1867885268: return getSubject(); 2532 case 951530927: return getContext(); 2533 case -1248768647: return addSupportingInformation(); 2534 case 481140686: return addPerformer(); 2535 case -1237557856: return addAuthorizingPrescription(); 2536 case 3575610: return getType(); 2537 case -1285004149: return getQuantity(); 2538 case 197175334: return getDaysSupply(); 2539 case -562837097: return getWhenPreparedElement(); 2540 case -940241380: return getWhenHandedOverElement(); 2541 case -1429847026: return getDestination(); 2542 case -808719889: return addReceiver(); 2543 case 3387378: return addNote(); 2544 case -1201373865: return addDosageInstruction(); 2545 case 826147581: return getSubstitution(); 2546 case 51602295: return addDetectedIssue(); 2547 case 2128257269: return getNotDoneElement(); 2548 case -1762771385: return getNotDoneReason(); 2549 case -1973169255: return getNotDoneReason(); 2550 case 1835190426: return addEventHistory(); 2551 default: return super.makeProperty(hash, name); 2552 } 2553 2554 } 2555 2556 @Override 2557 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2558 switch (hash) { 2559 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2560 case -995410646: /*partOf*/ return new String[] {"Reference"}; 2561 case -892481550: /*status*/ return new String[] {"code"}; 2562 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 2563 case 1998965455: /*medication*/ return new String[] {"CodeableConcept", "Reference"}; 2564 case -1867885268: /*subject*/ return new String[] {"Reference"}; 2565 case 951530927: /*context*/ return new String[] {"Reference"}; 2566 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 2567 case 481140686: /*performer*/ return new String[] {}; 2568 case -1237557856: /*authorizingPrescription*/ return new String[] {"Reference"}; 2569 case 3575610: /*type*/ return new String[] {"CodeableConcept"}; 2570 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 2571 case 197175334: /*daysSupply*/ return new String[] {"SimpleQuantity"}; 2572 case -562837097: /*whenPrepared*/ return new String[] {"dateTime"}; 2573 case -940241380: /*whenHandedOver*/ return new String[] {"dateTime"}; 2574 case -1429847026: /*destination*/ return new String[] {"Reference"}; 2575 case -808719889: /*receiver*/ return new String[] {"Reference"}; 2576 case 3387378: /*note*/ return new String[] {"Annotation"}; 2577 case -1201373865: /*dosageInstruction*/ return new String[] {"Dosage"}; 2578 case 826147581: /*substitution*/ return new String[] {}; 2579 case 51602295: /*detectedIssue*/ return new String[] {"Reference"}; 2580 case 2128257269: /*notDone*/ return new String[] {"boolean"}; 2581 case -1973169255: /*notDoneReason*/ return new String[] {"CodeableConcept", "Reference"}; 2582 case 1835190426: /*eventHistory*/ return new String[] {"Reference"}; 2583 default: return super.getTypesForProperty(hash, name); 2584 } 2585 2586 } 2587 2588 @Override 2589 public Base addChild(String name) throws FHIRException { 2590 if (name.equals("identifier")) { 2591 return addIdentifier(); 2592 } 2593 else if (name.equals("partOf")) { 2594 return addPartOf(); 2595 } 2596 else if (name.equals("status")) { 2597 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.status"); 2598 } 2599 else if (name.equals("category")) { 2600 this.category = new CodeableConcept(); 2601 return this.category; 2602 } 2603 else if (name.equals("medicationCodeableConcept")) { 2604 this.medication = new CodeableConcept(); 2605 return this.medication; 2606 } 2607 else if (name.equals("medicationReference")) { 2608 this.medication = new Reference(); 2609 return this.medication; 2610 } 2611 else if (name.equals("subject")) { 2612 this.subject = new Reference(); 2613 return this.subject; 2614 } 2615 else if (name.equals("context")) { 2616 this.context = new Reference(); 2617 return this.context; 2618 } 2619 else if (name.equals("supportingInformation")) { 2620 return addSupportingInformation(); 2621 } 2622 else if (name.equals("performer")) { 2623 return addPerformer(); 2624 } 2625 else if (name.equals("authorizingPrescription")) { 2626 return addAuthorizingPrescription(); 2627 } 2628 else if (name.equals("type")) { 2629 this.type = new CodeableConcept(); 2630 return this.type; 2631 } 2632 else if (name.equals("quantity")) { 2633 this.quantity = new SimpleQuantity(); 2634 return this.quantity; 2635 } 2636 else if (name.equals("daysSupply")) { 2637 this.daysSupply = new SimpleQuantity(); 2638 return this.daysSupply; 2639 } 2640 else if (name.equals("whenPrepared")) { 2641 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.whenPrepared"); 2642 } 2643 else if (name.equals("whenHandedOver")) { 2644 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.whenHandedOver"); 2645 } 2646 else if (name.equals("destination")) { 2647 this.destination = new Reference(); 2648 return this.destination; 2649 } 2650 else if (name.equals("receiver")) { 2651 return addReceiver(); 2652 } 2653 else if (name.equals("note")) { 2654 return addNote(); 2655 } 2656 else if (name.equals("dosageInstruction")) { 2657 return addDosageInstruction(); 2658 } 2659 else if (name.equals("substitution")) { 2660 this.substitution = new MedicationDispenseSubstitutionComponent(); 2661 return this.substitution; 2662 } 2663 else if (name.equals("detectedIssue")) { 2664 return addDetectedIssue(); 2665 } 2666 else if (name.equals("notDone")) { 2667 throw new FHIRException("Cannot call addChild on a singleton property MedicationDispense.notDone"); 2668 } 2669 else if (name.equals("notDoneReasonCodeableConcept")) { 2670 this.notDoneReason = new CodeableConcept(); 2671 return this.notDoneReason; 2672 } 2673 else if (name.equals("notDoneReasonReference")) { 2674 this.notDoneReason = new Reference(); 2675 return this.notDoneReason; 2676 } 2677 else if (name.equals("eventHistory")) { 2678 return addEventHistory(); 2679 } 2680 else 2681 return super.addChild(name); 2682 } 2683 2684 public String fhirType() { 2685 return "MedicationDispense"; 2686 2687 } 2688 2689 public MedicationDispense copy() { 2690 MedicationDispense dst = new MedicationDispense(); 2691 copyValues(dst); 2692 if (identifier != null) { 2693 dst.identifier = new ArrayList<Identifier>(); 2694 for (Identifier i : identifier) 2695 dst.identifier.add(i.copy()); 2696 }; 2697 if (partOf != null) { 2698 dst.partOf = new ArrayList<Reference>(); 2699 for (Reference i : partOf) 2700 dst.partOf.add(i.copy()); 2701 }; 2702 dst.status = status == null ? null : status.copy(); 2703 dst.category = category == null ? null : category.copy(); 2704 dst.medication = medication == null ? null : medication.copy(); 2705 dst.subject = subject == null ? null : subject.copy(); 2706 dst.context = context == null ? null : context.copy(); 2707 if (supportingInformation != null) { 2708 dst.supportingInformation = new ArrayList<Reference>(); 2709 for (Reference i : supportingInformation) 2710 dst.supportingInformation.add(i.copy()); 2711 }; 2712 if (performer != null) { 2713 dst.performer = new ArrayList<MedicationDispensePerformerComponent>(); 2714 for (MedicationDispensePerformerComponent i : performer) 2715 dst.performer.add(i.copy()); 2716 }; 2717 if (authorizingPrescription != null) { 2718 dst.authorizingPrescription = new ArrayList<Reference>(); 2719 for (Reference i : authorizingPrescription) 2720 dst.authorizingPrescription.add(i.copy()); 2721 }; 2722 dst.type = type == null ? null : type.copy(); 2723 dst.quantity = quantity == null ? null : quantity.copy(); 2724 dst.daysSupply = daysSupply == null ? null : daysSupply.copy(); 2725 dst.whenPrepared = whenPrepared == null ? null : whenPrepared.copy(); 2726 dst.whenHandedOver = whenHandedOver == null ? null : whenHandedOver.copy(); 2727 dst.destination = destination == null ? null : destination.copy(); 2728 if (receiver != null) { 2729 dst.receiver = new ArrayList<Reference>(); 2730 for (Reference i : receiver) 2731 dst.receiver.add(i.copy()); 2732 }; 2733 if (note != null) { 2734 dst.note = new ArrayList<Annotation>(); 2735 for (Annotation i : note) 2736 dst.note.add(i.copy()); 2737 }; 2738 if (dosageInstruction != null) { 2739 dst.dosageInstruction = new ArrayList<Dosage>(); 2740 for (Dosage i : dosageInstruction) 2741 dst.dosageInstruction.add(i.copy()); 2742 }; 2743 dst.substitution = substitution == null ? null : substitution.copy(); 2744 if (detectedIssue != null) { 2745 dst.detectedIssue = new ArrayList<Reference>(); 2746 for (Reference i : detectedIssue) 2747 dst.detectedIssue.add(i.copy()); 2748 }; 2749 dst.notDone = notDone == null ? null : notDone.copy(); 2750 dst.notDoneReason = notDoneReason == null ? null : notDoneReason.copy(); 2751 if (eventHistory != null) { 2752 dst.eventHistory = new ArrayList<Reference>(); 2753 for (Reference i : eventHistory) 2754 dst.eventHistory.add(i.copy()); 2755 }; 2756 return dst; 2757 } 2758 2759 protected MedicationDispense typedCopy() { 2760 return copy(); 2761 } 2762 2763 @Override 2764 public boolean equalsDeep(Base other_) { 2765 if (!super.equalsDeep(other_)) 2766 return false; 2767 if (!(other_ instanceof MedicationDispense)) 2768 return false; 2769 MedicationDispense o = (MedicationDispense) other_; 2770 return compareDeep(identifier, o.identifier, true) && compareDeep(partOf, o.partOf, true) && compareDeep(status, o.status, true) 2771 && compareDeep(category, o.category, true) && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) 2772 && compareDeep(context, o.context, true) && compareDeep(supportingInformation, o.supportingInformation, true) 2773 && compareDeep(performer, o.performer, true) && compareDeep(authorizingPrescription, o.authorizingPrescription, true) 2774 && compareDeep(type, o.type, true) && compareDeep(quantity, o.quantity, true) && compareDeep(daysSupply, o.daysSupply, true) 2775 && compareDeep(whenPrepared, o.whenPrepared, true) && compareDeep(whenHandedOver, o.whenHandedOver, true) 2776 && compareDeep(destination, o.destination, true) && compareDeep(receiver, o.receiver, true) && compareDeep(note, o.note, true) 2777 && compareDeep(dosageInstruction, o.dosageInstruction, true) && compareDeep(substitution, o.substitution, true) 2778 && compareDeep(detectedIssue, o.detectedIssue, true) && compareDeep(notDone, o.notDone, true) && compareDeep(notDoneReason, o.notDoneReason, true) 2779 && compareDeep(eventHistory, o.eventHistory, true); 2780 } 2781 2782 @Override 2783 public boolean equalsShallow(Base other_) { 2784 if (!super.equalsShallow(other_)) 2785 return false; 2786 if (!(other_ instanceof MedicationDispense)) 2787 return false; 2788 MedicationDispense o = (MedicationDispense) other_; 2789 return compareValues(status, o.status, true) && compareValues(whenPrepared, o.whenPrepared, true) && compareValues(whenHandedOver, o.whenHandedOver, true) 2790 && compareValues(notDone, o.notDone, true); 2791 } 2792 2793 public boolean isEmpty() { 2794 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, partOf, status 2795 , category, medication, subject, context, supportingInformation, performer, authorizingPrescription 2796 , type, quantity, daysSupply, whenPrepared, whenHandedOver, destination, receiver 2797 , note, dosageInstruction, substitution, detectedIssue, notDone, notDoneReason, eventHistory 2798 ); 2799 } 2800 2801 @Override 2802 public ResourceType getResourceType() { 2803 return ResourceType.MedicationDispense; 2804 } 2805 2806 /** 2807 * Search parameter: <b>identifier</b> 2808 * <p> 2809 * Description: <b>Return dispenses with this external identifier</b><br> 2810 * Type: <b>token</b><br> 2811 * Path: <b>MedicationDispense.identifier</b><br> 2812 * </p> 2813 */ 2814 @SearchParamDefinition(name="identifier", path="MedicationDispense.identifier", description="Return dispenses with this external identifier", type="token" ) 2815 public static final String SP_IDENTIFIER = "identifier"; 2816 /** 2817 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2818 * <p> 2819 * Description: <b>Return dispenses with this external identifier</b><br> 2820 * Type: <b>token</b><br> 2821 * Path: <b>MedicationDispense.identifier</b><br> 2822 * </p> 2823 */ 2824 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2825 2826 /** 2827 * Search parameter: <b>performer</b> 2828 * <p> 2829 * Description: <b>Return dispenses performed by a specific individual</b><br> 2830 * Type: <b>reference</b><br> 2831 * Path: <b>MedicationDispense.performer.actor</b><br> 2832 * </p> 2833 */ 2834 @SearchParamDefinition(name="performer", path="MedicationDispense.performer.actor", description="Return dispenses performed by a specific individual", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2835 public static final String SP_PERFORMER = "performer"; 2836 /** 2837 * <b>Fluent Client</b> search parameter constant for <b>performer</b> 2838 * <p> 2839 * Description: <b>Return dispenses performed by a specific individual</b><br> 2840 * Type: <b>reference</b><br> 2841 * Path: <b>MedicationDispense.performer.actor</b><br> 2842 * </p> 2843 */ 2844 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PERFORMER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PERFORMER); 2845 2846/** 2847 * Constant for fluent queries to be used to add include statements. Specifies 2848 * the path value of "<b>MedicationDispense:performer</b>". 2849 */ 2850 public static final ca.uhn.fhir.model.api.Include INCLUDE_PERFORMER = new ca.uhn.fhir.model.api.Include("MedicationDispense:performer").toLocked(); 2851 2852 /** 2853 * Search parameter: <b>code</b> 2854 * <p> 2855 * Description: <b>Return dispenses of this medicine code</b><br> 2856 * Type: <b>token</b><br> 2857 * Path: <b>MedicationDispense.medicationCodeableConcept</b><br> 2858 * </p> 2859 */ 2860 @SearchParamDefinition(name="code", path="MedicationDispense.medication.as(CodeableConcept)", description="Return dispenses of this medicine code", type="token" ) 2861 public static final String SP_CODE = "code"; 2862 /** 2863 * <b>Fluent Client</b> search parameter constant for <b>code</b> 2864 * <p> 2865 * Description: <b>Return dispenses of this medicine code</b><br> 2866 * Type: <b>token</b><br> 2867 * Path: <b>MedicationDispense.medicationCodeableConcept</b><br> 2868 * </p> 2869 */ 2870 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 2871 2872 /** 2873 * Search parameter: <b>receiver</b> 2874 * <p> 2875 * Description: <b>The identity of a receiver to list dispenses for</b><br> 2876 * Type: <b>reference</b><br> 2877 * Path: <b>MedicationDispense.receiver</b><br> 2878 * </p> 2879 */ 2880 @SearchParamDefinition(name="receiver", path="MedicationDispense.receiver", description="The identity of a receiver to list dispenses for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient"), @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Patient.class, Practitioner.class } ) 2881 public static final String SP_RECEIVER = "receiver"; 2882 /** 2883 * <b>Fluent Client</b> search parameter constant for <b>receiver</b> 2884 * <p> 2885 * Description: <b>The identity of a receiver to list dispenses for</b><br> 2886 * Type: <b>reference</b><br> 2887 * Path: <b>MedicationDispense.receiver</b><br> 2888 * </p> 2889 */ 2890 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RECEIVER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RECEIVER); 2891 2892/** 2893 * Constant for fluent queries to be used to add include statements. Specifies 2894 * the path value of "<b>MedicationDispense:receiver</b>". 2895 */ 2896 public static final ca.uhn.fhir.model.api.Include INCLUDE_RECEIVER = new ca.uhn.fhir.model.api.Include("MedicationDispense:receiver").toLocked(); 2897 2898 /** 2899 * Search parameter: <b>subject</b> 2900 * <p> 2901 * Description: <b>The identity of a patient to list dispenses for</b><br> 2902 * Type: <b>reference</b><br> 2903 * Path: <b>MedicationDispense.subject</b><br> 2904 * </p> 2905 */ 2906 @SearchParamDefinition(name="subject", path="MedicationDispense.subject", description="The identity of a patient to list dispenses for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 2907 public static final String SP_SUBJECT = "subject"; 2908 /** 2909 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 2910 * <p> 2911 * Description: <b>The identity of a patient to list dispenses for</b><br> 2912 * Type: <b>reference</b><br> 2913 * Path: <b>MedicationDispense.subject</b><br> 2914 * </p> 2915 */ 2916 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 2917 2918/** 2919 * Constant for fluent queries to be used to add include statements. Specifies 2920 * the path value of "<b>MedicationDispense:subject</b>". 2921 */ 2922 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationDispense:subject").toLocked(); 2923 2924 /** 2925 * Search parameter: <b>destination</b> 2926 * <p> 2927 * Description: <b>Return dispenses that should be sent to a specific destination</b><br> 2928 * Type: <b>reference</b><br> 2929 * Path: <b>MedicationDispense.destination</b><br> 2930 * </p> 2931 */ 2932 @SearchParamDefinition(name="destination", path="MedicationDispense.destination", description="Return dispenses that should be sent to a specific destination", type="reference", target={Location.class } ) 2933 public static final String SP_DESTINATION = "destination"; 2934 /** 2935 * <b>Fluent Client</b> search parameter constant for <b>destination</b> 2936 * <p> 2937 * Description: <b>Return dispenses that should be sent to a specific destination</b><br> 2938 * Type: <b>reference</b><br> 2939 * Path: <b>MedicationDispense.destination</b><br> 2940 * </p> 2941 */ 2942 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam DESTINATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_DESTINATION); 2943 2944/** 2945 * Constant for fluent queries to be used to add include statements. Specifies 2946 * the path value of "<b>MedicationDispense:destination</b>". 2947 */ 2948 public static final ca.uhn.fhir.model.api.Include INCLUDE_DESTINATION = new ca.uhn.fhir.model.api.Include("MedicationDispense:destination").toLocked(); 2949 2950 /** 2951 * Search parameter: <b>medication</b> 2952 * <p> 2953 * Description: <b>Return dispenses of this medicine resource</b><br> 2954 * Type: <b>reference</b><br> 2955 * Path: <b>MedicationDispense.medicationReference</b><br> 2956 * </p> 2957 */ 2958 @SearchParamDefinition(name="medication", path="MedicationDispense.medication.as(Reference)", description="Return dispenses of this medicine resource", type="reference", target={Medication.class } ) 2959 public static final String SP_MEDICATION = "medication"; 2960 /** 2961 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 2962 * <p> 2963 * Description: <b>Return dispenses of this medicine resource</b><br> 2964 * Type: <b>reference</b><br> 2965 * Path: <b>MedicationDispense.medicationReference</b><br> 2966 * </p> 2967 */ 2968 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 2969 2970/** 2971 * Constant for fluent queries to be used to add include statements. Specifies 2972 * the path value of "<b>MedicationDispense:medication</b>". 2973 */ 2974 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationDispense:medication").toLocked(); 2975 2976 /** 2977 * Search parameter: <b>responsibleparty</b> 2978 * <p> 2979 * Description: <b>Return dispenses with the specified responsible party</b><br> 2980 * Type: <b>reference</b><br> 2981 * Path: <b>MedicationDispense.substitution.responsibleParty</b><br> 2982 * </p> 2983 */ 2984 @SearchParamDefinition(name="responsibleparty", path="MedicationDispense.substitution.responsibleParty", description="Return dispenses with the specified responsible party", type="reference", target={Practitioner.class } ) 2985 public static final String SP_RESPONSIBLEPARTY = "responsibleparty"; 2986 /** 2987 * <b>Fluent Client</b> search parameter constant for <b>responsibleparty</b> 2988 * <p> 2989 * Description: <b>Return dispenses with the specified responsible party</b><br> 2990 * Type: <b>reference</b><br> 2991 * Path: <b>MedicationDispense.substitution.responsibleParty</b><br> 2992 * </p> 2993 */ 2994 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam RESPONSIBLEPARTY = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_RESPONSIBLEPARTY); 2995 2996/** 2997 * Constant for fluent queries to be used to add include statements. Specifies 2998 * the path value of "<b>MedicationDispense:responsibleparty</b>". 2999 */ 3000 public static final ca.uhn.fhir.model.api.Include INCLUDE_RESPONSIBLEPARTY = new ca.uhn.fhir.model.api.Include("MedicationDispense:responsibleparty").toLocked(); 3001 3002 /** 3003 * Search parameter: <b>type</b> 3004 * <p> 3005 * Description: <b>Return dispenses of a specific type</b><br> 3006 * Type: <b>token</b><br> 3007 * Path: <b>MedicationDispense.type</b><br> 3008 * </p> 3009 */ 3010 @SearchParamDefinition(name="type", path="MedicationDispense.type", description="Return dispenses of a specific type", type="token" ) 3011 public static final String SP_TYPE = "type"; 3012 /** 3013 * <b>Fluent Client</b> search parameter constant for <b>type</b> 3014 * <p> 3015 * Description: <b>Return dispenses of a specific type</b><br> 3016 * Type: <b>token</b><br> 3017 * Path: <b>MedicationDispense.type</b><br> 3018 * </p> 3019 */ 3020 public static final ca.uhn.fhir.rest.gclient.TokenClientParam TYPE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_TYPE); 3021 3022 /** 3023 * Search parameter: <b>whenhandedover</b> 3024 * <p> 3025 * Description: <b>Returns dispenses handed over on this date</b><br> 3026 * Type: <b>date</b><br> 3027 * Path: <b>MedicationDispense.whenHandedOver</b><br> 3028 * </p> 3029 */ 3030 @SearchParamDefinition(name="whenhandedover", path="MedicationDispense.whenHandedOver", description="Returns dispenses handed over on this date", type="date" ) 3031 public static final String SP_WHENHANDEDOVER = "whenhandedover"; 3032 /** 3033 * <b>Fluent Client</b> search parameter constant for <b>whenhandedover</b> 3034 * <p> 3035 * Description: <b>Returns dispenses handed over on this date</b><br> 3036 * Type: <b>date</b><br> 3037 * Path: <b>MedicationDispense.whenHandedOver</b><br> 3038 * </p> 3039 */ 3040 public static final ca.uhn.fhir.rest.gclient.DateClientParam WHENHANDEDOVER = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_WHENHANDEDOVER); 3041 3042 /** 3043 * Search parameter: <b>whenprepared</b> 3044 * <p> 3045 * Description: <b>Returns dispenses prepared on this date</b><br> 3046 * Type: <b>date</b><br> 3047 * Path: <b>MedicationDispense.whenPrepared</b><br> 3048 * </p> 3049 */ 3050 @SearchParamDefinition(name="whenprepared", path="MedicationDispense.whenPrepared", description="Returns dispenses prepared on this date", type="date" ) 3051 public static final String SP_WHENPREPARED = "whenprepared"; 3052 /** 3053 * <b>Fluent Client</b> search parameter constant for <b>whenprepared</b> 3054 * <p> 3055 * Description: <b>Returns dispenses prepared on this date</b><br> 3056 * Type: <b>date</b><br> 3057 * Path: <b>MedicationDispense.whenPrepared</b><br> 3058 * </p> 3059 */ 3060 public static final ca.uhn.fhir.rest.gclient.DateClientParam WHENPREPARED = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_WHENPREPARED); 3061 3062 /** 3063 * Search parameter: <b>prescription</b> 3064 * <p> 3065 * Description: <b>The identity of a prescription to list dispenses from</b><br> 3066 * Type: <b>reference</b><br> 3067 * Path: <b>MedicationDispense.authorizingPrescription</b><br> 3068 * </p> 3069 */ 3070 @SearchParamDefinition(name="prescription", path="MedicationDispense.authorizingPrescription", description="The identity of a prescription to list dispenses from", type="reference", target={MedicationRequest.class } ) 3071 public static final String SP_PRESCRIPTION = "prescription"; 3072 /** 3073 * <b>Fluent Client</b> search parameter constant for <b>prescription</b> 3074 * <p> 3075 * Description: <b>The identity of a prescription to list dispenses from</b><br> 3076 * Type: <b>reference</b><br> 3077 * Path: <b>MedicationDispense.authorizingPrescription</b><br> 3078 * </p> 3079 */ 3080 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PRESCRIPTION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PRESCRIPTION); 3081 3082/** 3083 * Constant for fluent queries to be used to add include statements. Specifies 3084 * the path value of "<b>MedicationDispense:prescription</b>". 3085 */ 3086 public static final ca.uhn.fhir.model.api.Include INCLUDE_PRESCRIPTION = new ca.uhn.fhir.model.api.Include("MedicationDispense:prescription").toLocked(); 3087 3088 /** 3089 * Search parameter: <b>patient</b> 3090 * <p> 3091 * Description: <b>The identity of a patient to list dispenses for</b><br> 3092 * Type: <b>reference</b><br> 3093 * Path: <b>MedicationDispense.subject</b><br> 3094 * </p> 3095 */ 3096 @SearchParamDefinition(name="patient", path="MedicationDispense.subject", description="The identity of a patient to list dispenses for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Patient.class } ) 3097 public static final String SP_PATIENT = "patient"; 3098 /** 3099 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3100 * <p> 3101 * Description: <b>The identity of a patient to list dispenses for</b><br> 3102 * Type: <b>reference</b><br> 3103 * Path: <b>MedicationDispense.subject</b><br> 3104 * </p> 3105 */ 3106 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3107 3108/** 3109 * Constant for fluent queries to be used to add include statements. Specifies 3110 * the path value of "<b>MedicationDispense:patient</b>". 3111 */ 3112 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationDispense:patient").toLocked(); 3113 3114 /** 3115 * Search parameter: <b>context</b> 3116 * <p> 3117 * Description: <b>Returns dispenses with a specific context (episode or episode of care)</b><br> 3118 * Type: <b>reference</b><br> 3119 * Path: <b>MedicationDispense.context</b><br> 3120 * </p> 3121 */ 3122 @SearchParamDefinition(name="context", path="MedicationDispense.context", description="Returns dispenses with a specific context (episode or episode of care)", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 3123 public static final String SP_CONTEXT = "context"; 3124 /** 3125 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3126 * <p> 3127 * Description: <b>Returns dispenses with a specific context (episode or episode of care)</b><br> 3128 * Type: <b>reference</b><br> 3129 * Path: <b>MedicationDispense.context</b><br> 3130 * </p> 3131 */ 3132 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3133 3134/** 3135 * Constant for fluent queries to be used to add include statements. Specifies 3136 * the path value of "<b>MedicationDispense:context</b>". 3137 */ 3138 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("MedicationDispense:context").toLocked(); 3139 3140 /** 3141 * Search parameter: <b>status</b> 3142 * <p> 3143 * Description: <b>Return dispenses with a specified dispense status</b><br> 3144 * Type: <b>token</b><br> 3145 * Path: <b>MedicationDispense.status</b><br> 3146 * </p> 3147 */ 3148 @SearchParamDefinition(name="status", path="MedicationDispense.status", description="Return dispenses with a specified dispense status", type="token" ) 3149 public static final String SP_STATUS = "status"; 3150 /** 3151 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3152 * <p> 3153 * Description: <b>Return dispenses with a specified dispense status</b><br> 3154 * Type: <b>token</b><br> 3155 * Path: <b>MedicationDispense.status</b><br> 3156 * </p> 3157 */ 3158 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3159 3160 3161}