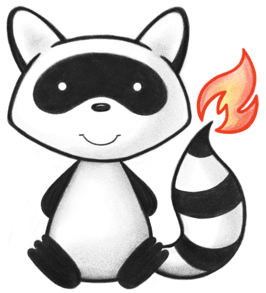
001package org.hl7.fhir.dstu3.model; 002 003 004 005 006/* 007 Copyright (c) 2011+, HL7, Inc. 008 All rights reserved. 009 010 Redistribution and use in source and binary forms, with or without modification, 011 are permitted provided that the following conditions are met: 012 013 * Redistributions of source code must retain the above copyright notice, this 014 list of conditions and the following disclaimer. 015 * Redistributions in binary form must reproduce the above copyright notice, 016 this list of conditions and the following disclaimer in the documentation 017 and/or other materials provided with the distribution. 018 * Neither the name of HL7 nor the names of its contributors may be used to 019 endorse or promote products derived from this software without specific 020 prior written permission. 021 022 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 023 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 024 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 025 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 026 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 027 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 028 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 029 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 030 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 031 POSSIBILITY OF SUCH DAMAGE. 032 033*/ 034 035// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 036import java.util.ArrayList; 037import java.util.Date; 038import java.util.List; 039 040import org.hl7.fhir.exceptions.FHIRException; 041import org.hl7.fhir.exceptions.FHIRFormatError; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043 044import ca.uhn.fhir.model.api.annotation.Block; 045import ca.uhn.fhir.model.api.annotation.Child; 046import ca.uhn.fhir.model.api.annotation.Description; 047import ca.uhn.fhir.model.api.annotation.ResourceDef; 048import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 049/** 050 * An order or request for both supply of the medication and the instructions for administration of the medication to a patient. The resource is called "MedicationRequest" rather than "MedicationPrescription" or "MedicationOrder" to generalize the use across inpatient and outpatient settings, including care plans, etc., and to harmonize with workflow patterns. 051 */ 052@ResourceDef(name="MedicationRequest", profile="http://hl7.org/fhir/Profile/MedicationRequest") 053public class MedicationRequest extends DomainResource { 054 055 public enum MedicationRequestStatus { 056 /** 057 * The prescription is 'actionable', but not all actions that are implied by it have occurred yet. 058 */ 059 ACTIVE, 060 /** 061 * Actions implied by the prescription are to be temporarily halted, but are expected to continue later. May also be called "suspended". 062 */ 063 ONHOLD, 064 /** 065 * The prescription has been withdrawn. 066 */ 067 CANCELLED, 068 /** 069 * All actions that are implied by the prescription have occurred. 070 */ 071 COMPLETED, 072 /** 073 * The prescription was entered in error. 074 */ 075 ENTEREDINERROR, 076 /** 077 * Actions implied by the prescription are to be permanently halted, before all of them occurred. 078 */ 079 STOPPED, 080 /** 081 * The prescription is not yet 'actionable', i.e. it is a work in progress, requires sign-off or verification, and needs to be run through decision support process. 082 */ 083 DRAFT, 084 /** 085 * The authoring system does not know which of the status values currently applies for this request 086 */ 087 UNKNOWN, 088 /** 089 * added to help the parsers with the generic types 090 */ 091 NULL; 092 public static MedicationRequestStatus fromCode(String codeString) throws FHIRException { 093 if (codeString == null || "".equals(codeString)) 094 return null; 095 if ("active".equals(codeString)) 096 return ACTIVE; 097 if ("on-hold".equals(codeString)) 098 return ONHOLD; 099 if ("cancelled".equals(codeString)) 100 return CANCELLED; 101 if ("completed".equals(codeString)) 102 return COMPLETED; 103 if ("entered-in-error".equals(codeString)) 104 return ENTEREDINERROR; 105 if ("stopped".equals(codeString)) 106 return STOPPED; 107 if ("draft".equals(codeString)) 108 return DRAFT; 109 if ("unknown".equals(codeString)) 110 return UNKNOWN; 111 if (Configuration.isAcceptInvalidEnums()) 112 return null; 113 else 114 throw new FHIRException("Unknown MedicationRequestStatus code '"+codeString+"'"); 115 } 116 public String toCode() { 117 switch (this) { 118 case ACTIVE: return "active"; 119 case ONHOLD: return "on-hold"; 120 case CANCELLED: return "cancelled"; 121 case COMPLETED: return "completed"; 122 case ENTEREDINERROR: return "entered-in-error"; 123 case STOPPED: return "stopped"; 124 case DRAFT: return "draft"; 125 case UNKNOWN: return "unknown"; 126 case NULL: return null; 127 default: return "?"; 128 } 129 } 130 public String getSystem() { 131 switch (this) { 132 case ACTIVE: return "http://hl7.org/fhir/medication-request-status"; 133 case ONHOLD: return "http://hl7.org/fhir/medication-request-status"; 134 case CANCELLED: return "http://hl7.org/fhir/medication-request-status"; 135 case COMPLETED: return "http://hl7.org/fhir/medication-request-status"; 136 case ENTEREDINERROR: return "http://hl7.org/fhir/medication-request-status"; 137 case STOPPED: return "http://hl7.org/fhir/medication-request-status"; 138 case DRAFT: return "http://hl7.org/fhir/medication-request-status"; 139 case UNKNOWN: return "http://hl7.org/fhir/medication-request-status"; 140 case NULL: return null; 141 default: return "?"; 142 } 143 } 144 public String getDefinition() { 145 switch (this) { 146 case ACTIVE: return "The prescription is 'actionable', but not all actions that are implied by it have occurred yet."; 147 case ONHOLD: return "Actions implied by the prescription are to be temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 148 case CANCELLED: return "The prescription has been withdrawn."; 149 case COMPLETED: return "All actions that are implied by the prescription have occurred."; 150 case ENTEREDINERROR: return "The prescription was entered in error."; 151 case STOPPED: return "Actions implied by the prescription are to be permanently halted, before all of them occurred."; 152 case DRAFT: return "The prescription is not yet 'actionable', i.e. it is a work in progress, requires sign-off or verification, and needs to be run through decision support process."; 153 case UNKNOWN: return "The authoring system does not know which of the status values currently applies for this request"; 154 case NULL: return null; 155 default: return "?"; 156 } 157 } 158 public String getDisplay() { 159 switch (this) { 160 case ACTIVE: return "Active"; 161 case ONHOLD: return "On Hold"; 162 case CANCELLED: return "Cancelled"; 163 case COMPLETED: return "Completed"; 164 case ENTEREDINERROR: return "Entered In Error"; 165 case STOPPED: return "Stopped"; 166 case DRAFT: return "Draft"; 167 case UNKNOWN: return "Unknown"; 168 case NULL: return null; 169 default: return "?"; 170 } 171 } 172 } 173 174 public static class MedicationRequestStatusEnumFactory implements EnumFactory<MedicationRequestStatus> { 175 public MedicationRequestStatus fromCode(String codeString) throws IllegalArgumentException { 176 if (codeString == null || "".equals(codeString)) 177 if (codeString == null || "".equals(codeString)) 178 return null; 179 if ("active".equals(codeString)) 180 return MedicationRequestStatus.ACTIVE; 181 if ("on-hold".equals(codeString)) 182 return MedicationRequestStatus.ONHOLD; 183 if ("cancelled".equals(codeString)) 184 return MedicationRequestStatus.CANCELLED; 185 if ("completed".equals(codeString)) 186 return MedicationRequestStatus.COMPLETED; 187 if ("entered-in-error".equals(codeString)) 188 return MedicationRequestStatus.ENTEREDINERROR; 189 if ("stopped".equals(codeString)) 190 return MedicationRequestStatus.STOPPED; 191 if ("draft".equals(codeString)) 192 return MedicationRequestStatus.DRAFT; 193 if ("unknown".equals(codeString)) 194 return MedicationRequestStatus.UNKNOWN; 195 throw new IllegalArgumentException("Unknown MedicationRequestStatus code '"+codeString+"'"); 196 } 197 public Enumeration<MedicationRequestStatus> fromType(PrimitiveType<?> code) throws FHIRException { 198 if (code == null) 199 return null; 200 if (code.isEmpty()) 201 return new Enumeration<MedicationRequestStatus>(this); 202 String codeString = code.asStringValue(); 203 if (codeString == null || "".equals(codeString)) 204 return null; 205 if ("active".equals(codeString)) 206 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.ACTIVE); 207 if ("on-hold".equals(codeString)) 208 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.ONHOLD); 209 if ("cancelled".equals(codeString)) 210 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.CANCELLED); 211 if ("completed".equals(codeString)) 212 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.COMPLETED); 213 if ("entered-in-error".equals(codeString)) 214 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.ENTEREDINERROR); 215 if ("stopped".equals(codeString)) 216 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.STOPPED); 217 if ("draft".equals(codeString)) 218 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.DRAFT); 219 if ("unknown".equals(codeString)) 220 return new Enumeration<MedicationRequestStatus>(this, MedicationRequestStatus.UNKNOWN); 221 throw new FHIRException("Unknown MedicationRequestStatus code '"+codeString+"'"); 222 } 223 public String toCode(MedicationRequestStatus code) { 224 if (code == MedicationRequestStatus.NULL) 225 return null; 226 if (code == MedicationRequestStatus.ACTIVE) 227 return "active"; 228 if (code == MedicationRequestStatus.ONHOLD) 229 return "on-hold"; 230 if (code == MedicationRequestStatus.CANCELLED) 231 return "cancelled"; 232 if (code == MedicationRequestStatus.COMPLETED) 233 return "completed"; 234 if (code == MedicationRequestStatus.ENTEREDINERROR) 235 return "entered-in-error"; 236 if (code == MedicationRequestStatus.STOPPED) 237 return "stopped"; 238 if (code == MedicationRequestStatus.DRAFT) 239 return "draft"; 240 if (code == MedicationRequestStatus.UNKNOWN) 241 return "unknown"; 242 return "?"; 243 } 244 public String toSystem(MedicationRequestStatus code) { 245 return code.getSystem(); 246 } 247 } 248 249 public enum MedicationRequestIntent { 250 /** 251 * The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act 252 */ 253 PROPOSAL, 254 /** 255 * The request represents an intension to ensure something occurs without providing an authorization for others to act 256 */ 257 PLAN, 258 /** 259 * The request represents a request/demand and authorization for action 260 */ 261 ORDER, 262 /** 263 * The request represents an instance for the particular order, for example a medication administration record. 264 */ 265 INSTANCEORDER, 266 /** 267 * added to help the parsers with the generic types 268 */ 269 NULL; 270 public static MedicationRequestIntent fromCode(String codeString) throws FHIRException { 271 if (codeString == null || "".equals(codeString)) 272 return null; 273 if ("proposal".equals(codeString)) 274 return PROPOSAL; 275 if ("plan".equals(codeString)) 276 return PLAN; 277 if ("order".equals(codeString)) 278 return ORDER; 279 if ("instance-order".equals(codeString)) 280 return INSTANCEORDER; 281 if (Configuration.isAcceptInvalidEnums()) 282 return null; 283 else 284 throw new FHIRException("Unknown MedicationRequestIntent code '"+codeString+"'"); 285 } 286 public String toCode() { 287 switch (this) { 288 case PROPOSAL: return "proposal"; 289 case PLAN: return "plan"; 290 case ORDER: return "order"; 291 case INSTANCEORDER: return "instance-order"; 292 case NULL: return null; 293 default: return "?"; 294 } 295 } 296 public String getSystem() { 297 switch (this) { 298 case PROPOSAL: return "http://hl7.org/fhir/medication-request-intent"; 299 case PLAN: return "http://hl7.org/fhir/medication-request-intent"; 300 case ORDER: return "http://hl7.org/fhir/medication-request-intent"; 301 case INSTANCEORDER: return "http://hl7.org/fhir/medication-request-intent"; 302 case NULL: return null; 303 default: return "?"; 304 } 305 } 306 public String getDefinition() { 307 switch (this) { 308 case PROPOSAL: return "The request is a suggestion made by someone/something that doesn't have an intention to ensure it occurs and without providing an authorization to act"; 309 case PLAN: return "The request represents an intension to ensure something occurs without providing an authorization for others to act"; 310 case ORDER: return "The request represents a request/demand and authorization for action"; 311 case INSTANCEORDER: return "The request represents an instance for the particular order, for example a medication administration record."; 312 case NULL: return null; 313 default: return "?"; 314 } 315 } 316 public String getDisplay() { 317 switch (this) { 318 case PROPOSAL: return "Proposal"; 319 case PLAN: return "Plan"; 320 case ORDER: return "Order"; 321 case INSTANCEORDER: return "Instance Order"; 322 case NULL: return null; 323 default: return "?"; 324 } 325 } 326 } 327 328 public static class MedicationRequestIntentEnumFactory implements EnumFactory<MedicationRequestIntent> { 329 public MedicationRequestIntent fromCode(String codeString) throws IllegalArgumentException { 330 if (codeString == null || "".equals(codeString)) 331 if (codeString == null || "".equals(codeString)) 332 return null; 333 if ("proposal".equals(codeString)) 334 return MedicationRequestIntent.PROPOSAL; 335 if ("plan".equals(codeString)) 336 return MedicationRequestIntent.PLAN; 337 if ("order".equals(codeString)) 338 return MedicationRequestIntent.ORDER; 339 if ("instance-order".equals(codeString)) 340 return MedicationRequestIntent.INSTANCEORDER; 341 throw new IllegalArgumentException("Unknown MedicationRequestIntent code '"+codeString+"'"); 342 } 343 public Enumeration<MedicationRequestIntent> fromType(PrimitiveType<?> code) throws FHIRException { 344 if (code == null) 345 return null; 346 if (code.isEmpty()) 347 return new Enumeration<MedicationRequestIntent>(this); 348 String codeString = code.asStringValue(); 349 if (codeString == null || "".equals(codeString)) 350 return null; 351 if ("proposal".equals(codeString)) 352 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.PROPOSAL); 353 if ("plan".equals(codeString)) 354 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.PLAN); 355 if ("order".equals(codeString)) 356 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.ORDER); 357 if ("instance-order".equals(codeString)) 358 return new Enumeration<MedicationRequestIntent>(this, MedicationRequestIntent.INSTANCEORDER); 359 throw new FHIRException("Unknown MedicationRequestIntent code '"+codeString+"'"); 360 } 361 public String toCode(MedicationRequestIntent code) { 362 if (code == MedicationRequestIntent.NULL) 363 return null; 364 if (code == MedicationRequestIntent.PROPOSAL) 365 return "proposal"; 366 if (code == MedicationRequestIntent.PLAN) 367 return "plan"; 368 if (code == MedicationRequestIntent.ORDER) 369 return "order"; 370 if (code == MedicationRequestIntent.INSTANCEORDER) 371 return "instance-order"; 372 return "?"; 373 } 374 public String toSystem(MedicationRequestIntent code) { 375 return code.getSystem(); 376 } 377 } 378 379 public enum MedicationRequestPriority { 380 /** 381 * The order has a normal priority . 382 */ 383 ROUTINE, 384 /** 385 * The order should be urgently. 386 */ 387 URGENT, 388 /** 389 * The order is time-critical. 390 */ 391 STAT, 392 /** 393 * The order should be acted on as soon as possible. 394 */ 395 ASAP, 396 /** 397 * added to help the parsers with the generic types 398 */ 399 NULL; 400 public static MedicationRequestPriority fromCode(String codeString) throws FHIRException { 401 if (codeString == null || "".equals(codeString)) 402 return null; 403 if ("routine".equals(codeString)) 404 return ROUTINE; 405 if ("urgent".equals(codeString)) 406 return URGENT; 407 if ("stat".equals(codeString)) 408 return STAT; 409 if ("asap".equals(codeString)) 410 return ASAP; 411 if (Configuration.isAcceptInvalidEnums()) 412 return null; 413 else 414 throw new FHIRException("Unknown MedicationRequestPriority code '"+codeString+"'"); 415 } 416 public String toCode() { 417 switch (this) { 418 case ROUTINE: return "routine"; 419 case URGENT: return "urgent"; 420 case STAT: return "stat"; 421 case ASAP: return "asap"; 422 case NULL: return null; 423 default: return "?"; 424 } 425 } 426 public String getSystem() { 427 switch (this) { 428 case ROUTINE: return "http://hl7.org/fhir/medication-request-priority"; 429 case URGENT: return "http://hl7.org/fhir/medication-request-priority"; 430 case STAT: return "http://hl7.org/fhir/medication-request-priority"; 431 case ASAP: return "http://hl7.org/fhir/medication-request-priority"; 432 case NULL: return null; 433 default: return "?"; 434 } 435 } 436 public String getDefinition() { 437 switch (this) { 438 case ROUTINE: return "The order has a normal priority ."; 439 case URGENT: return "The order should be urgently."; 440 case STAT: return "The order is time-critical."; 441 case ASAP: return "The order should be acted on as soon as possible."; 442 case NULL: return null; 443 default: return "?"; 444 } 445 } 446 public String getDisplay() { 447 switch (this) { 448 case ROUTINE: return "Routine"; 449 case URGENT: return "Urgent"; 450 case STAT: return "Stat"; 451 case ASAP: return "ASAP"; 452 case NULL: return null; 453 default: return "?"; 454 } 455 } 456 } 457 458 public static class MedicationRequestPriorityEnumFactory implements EnumFactory<MedicationRequestPriority> { 459 public MedicationRequestPriority fromCode(String codeString) throws IllegalArgumentException { 460 if (codeString == null || "".equals(codeString)) 461 if (codeString == null || "".equals(codeString)) 462 return null; 463 if ("routine".equals(codeString)) 464 return MedicationRequestPriority.ROUTINE; 465 if ("urgent".equals(codeString)) 466 return MedicationRequestPriority.URGENT; 467 if ("stat".equals(codeString)) 468 return MedicationRequestPriority.STAT; 469 if ("asap".equals(codeString)) 470 return MedicationRequestPriority.ASAP; 471 throw new IllegalArgumentException("Unknown MedicationRequestPriority code '"+codeString+"'"); 472 } 473 public Enumeration<MedicationRequestPriority> fromType(PrimitiveType<?> code) throws FHIRException { 474 if (code == null) 475 return null; 476 if (code.isEmpty()) 477 return new Enumeration<MedicationRequestPriority>(this); 478 String codeString = code.asStringValue(); 479 if (codeString == null || "".equals(codeString)) 480 return null; 481 if ("routine".equals(codeString)) 482 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.ROUTINE); 483 if ("urgent".equals(codeString)) 484 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.URGENT); 485 if ("stat".equals(codeString)) 486 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.STAT); 487 if ("asap".equals(codeString)) 488 return new Enumeration<MedicationRequestPriority>(this, MedicationRequestPriority.ASAP); 489 throw new FHIRException("Unknown MedicationRequestPriority code '"+codeString+"'"); 490 } 491 public String toCode(MedicationRequestPriority code) { 492 if (code == MedicationRequestPriority.NULL) 493 return null; 494 if (code == MedicationRequestPriority.ROUTINE) 495 return "routine"; 496 if (code == MedicationRequestPriority.URGENT) 497 return "urgent"; 498 if (code == MedicationRequestPriority.STAT) 499 return "stat"; 500 if (code == MedicationRequestPriority.ASAP) 501 return "asap"; 502 return "?"; 503 } 504 public String toSystem(MedicationRequestPriority code) { 505 return code.getSystem(); 506 } 507 } 508 509 @Block() 510 public static class MedicationRequestRequesterComponent extends BackboneElement implements IBaseBackboneElement { 511 /** 512 * The healthcare professional responsible for authorizing the initial prescription. 513 */ 514 @Child(name = "agent", type = {Practitioner.class, Organization.class, Patient.class, RelatedPerson.class, Device.class}, order=1, min=1, max=1, modifier=false, summary=true) 515 @Description(shortDefinition="Who ordered the initial medication(s)", formalDefinition="The healthcare professional responsible for authorizing the initial prescription." ) 516 protected Reference agent; 517 518 /** 519 * The actual object that is the target of the reference (The healthcare professional responsible for authorizing the initial prescription.) 520 */ 521 protected Resource agentTarget; 522 523 /** 524 * The organization the device or practitioner was acting on behalf of. 525 */ 526 @Child(name = "onBehalfOf", type = {Organization.class}, order=2, min=0, max=1, modifier=false, summary=true) 527 @Description(shortDefinition="Organization agent is acting for", formalDefinition="The organization the device or practitioner was acting on behalf of." ) 528 protected Reference onBehalfOf; 529 530 /** 531 * The actual object that is the target of the reference (The organization the device or practitioner was acting on behalf of.) 532 */ 533 protected Organization onBehalfOfTarget; 534 535 private static final long serialVersionUID = -71453027L; 536 537 /** 538 * Constructor 539 */ 540 public MedicationRequestRequesterComponent() { 541 super(); 542 } 543 544 /** 545 * Constructor 546 */ 547 public MedicationRequestRequesterComponent(Reference agent) { 548 super(); 549 this.agent = agent; 550 } 551 552 /** 553 * @return {@link #agent} (The healthcare professional responsible for authorizing the initial prescription.) 554 */ 555 public Reference getAgent() { 556 if (this.agent == null) 557 if (Configuration.errorOnAutoCreate()) 558 throw new Error("Attempt to auto-create MedicationRequestRequesterComponent.agent"); 559 else if (Configuration.doAutoCreate()) 560 this.agent = new Reference(); // cc 561 return this.agent; 562 } 563 564 public boolean hasAgent() { 565 return this.agent != null && !this.agent.isEmpty(); 566 } 567 568 /** 569 * @param value {@link #agent} (The healthcare professional responsible for authorizing the initial prescription.) 570 */ 571 public MedicationRequestRequesterComponent setAgent(Reference value) { 572 this.agent = value; 573 return this; 574 } 575 576 /** 577 * @return {@link #agent} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The healthcare professional responsible for authorizing the initial prescription.) 578 */ 579 public Resource getAgentTarget() { 580 return this.agentTarget; 581 } 582 583 /** 584 * @param value {@link #agent} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The healthcare professional responsible for authorizing the initial prescription.) 585 */ 586 public MedicationRequestRequesterComponent setAgentTarget(Resource value) { 587 this.agentTarget = value; 588 return this; 589 } 590 591 /** 592 * @return {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 593 */ 594 public Reference getOnBehalfOf() { 595 if (this.onBehalfOf == null) 596 if (Configuration.errorOnAutoCreate()) 597 throw new Error("Attempt to auto-create MedicationRequestRequesterComponent.onBehalfOf"); 598 else if (Configuration.doAutoCreate()) 599 this.onBehalfOf = new Reference(); // cc 600 return this.onBehalfOf; 601 } 602 603 public boolean hasOnBehalfOf() { 604 return this.onBehalfOf != null && !this.onBehalfOf.isEmpty(); 605 } 606 607 /** 608 * @param value {@link #onBehalfOf} (The organization the device or practitioner was acting on behalf of.) 609 */ 610 public MedicationRequestRequesterComponent setOnBehalfOf(Reference value) { 611 this.onBehalfOf = value; 612 return this; 613 } 614 615 /** 616 * @return {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 617 */ 618 public Organization getOnBehalfOfTarget() { 619 if (this.onBehalfOfTarget == null) 620 if (Configuration.errorOnAutoCreate()) 621 throw new Error("Attempt to auto-create MedicationRequestRequesterComponent.onBehalfOf"); 622 else if (Configuration.doAutoCreate()) 623 this.onBehalfOfTarget = new Organization(); // aa 624 return this.onBehalfOfTarget; 625 } 626 627 /** 628 * @param value {@link #onBehalfOf} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The organization the device or practitioner was acting on behalf of.) 629 */ 630 public MedicationRequestRequesterComponent setOnBehalfOfTarget(Organization value) { 631 this.onBehalfOfTarget = value; 632 return this; 633 } 634 635 protected void listChildren(List<Property> children) { 636 super.listChildren(children); 637 children.add(new Property("agent", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The healthcare professional responsible for authorizing the initial prescription.", 0, 1, agent)); 638 children.add(new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf)); 639 } 640 641 @Override 642 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 643 switch (_hash) { 644 case 92750597: /*agent*/ return new Property("agent", "Reference(Practitioner|Organization|Patient|RelatedPerson|Device)", "The healthcare professional responsible for authorizing the initial prescription.", 0, 1, agent); 645 case -14402964: /*onBehalfOf*/ return new Property("onBehalfOf", "Reference(Organization)", "The organization the device or practitioner was acting on behalf of.", 0, 1, onBehalfOf); 646 default: return super.getNamedProperty(_hash, _name, _checkValid); 647 } 648 649 } 650 651 @Override 652 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 653 switch (hash) { 654 case 92750597: /*agent*/ return this.agent == null ? new Base[0] : new Base[] {this.agent}; // Reference 655 case -14402964: /*onBehalfOf*/ return this.onBehalfOf == null ? new Base[0] : new Base[] {this.onBehalfOf}; // Reference 656 default: return super.getProperty(hash, name, checkValid); 657 } 658 659 } 660 661 @Override 662 public Base setProperty(int hash, String name, Base value) throws FHIRException { 663 switch (hash) { 664 case 92750597: // agent 665 this.agent = castToReference(value); // Reference 666 return value; 667 case -14402964: // onBehalfOf 668 this.onBehalfOf = castToReference(value); // Reference 669 return value; 670 default: return super.setProperty(hash, name, value); 671 } 672 673 } 674 675 @Override 676 public Base setProperty(String name, Base value) throws FHIRException { 677 if (name.equals("agent")) { 678 this.agent = castToReference(value); // Reference 679 } else if (name.equals("onBehalfOf")) { 680 this.onBehalfOf = castToReference(value); // Reference 681 } else 682 return super.setProperty(name, value); 683 return value; 684 } 685 686 @Override 687 public Base makeProperty(int hash, String name) throws FHIRException { 688 switch (hash) { 689 case 92750597: return getAgent(); 690 case -14402964: return getOnBehalfOf(); 691 default: return super.makeProperty(hash, name); 692 } 693 694 } 695 696 @Override 697 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 698 switch (hash) { 699 case 92750597: /*agent*/ return new String[] {"Reference"}; 700 case -14402964: /*onBehalfOf*/ return new String[] {"Reference"}; 701 default: return super.getTypesForProperty(hash, name); 702 } 703 704 } 705 706 @Override 707 public Base addChild(String name) throws FHIRException { 708 if (name.equals("agent")) { 709 this.agent = new Reference(); 710 return this.agent; 711 } 712 else if (name.equals("onBehalfOf")) { 713 this.onBehalfOf = new Reference(); 714 return this.onBehalfOf; 715 } 716 else 717 return super.addChild(name); 718 } 719 720 public MedicationRequestRequesterComponent copy() { 721 MedicationRequestRequesterComponent dst = new MedicationRequestRequesterComponent(); 722 copyValues(dst); 723 dst.agent = agent == null ? null : agent.copy(); 724 dst.onBehalfOf = onBehalfOf == null ? null : onBehalfOf.copy(); 725 return dst; 726 } 727 728 @Override 729 public boolean equalsDeep(Base other_) { 730 if (!super.equalsDeep(other_)) 731 return false; 732 if (!(other_ instanceof MedicationRequestRequesterComponent)) 733 return false; 734 MedicationRequestRequesterComponent o = (MedicationRequestRequesterComponent) other_; 735 return compareDeep(agent, o.agent, true) && compareDeep(onBehalfOf, o.onBehalfOf, true); 736 } 737 738 @Override 739 public boolean equalsShallow(Base other_) { 740 if (!super.equalsShallow(other_)) 741 return false; 742 if (!(other_ instanceof MedicationRequestRequesterComponent)) 743 return false; 744 MedicationRequestRequesterComponent o = (MedicationRequestRequesterComponent) other_; 745 return true; 746 } 747 748 public boolean isEmpty() { 749 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(agent, onBehalfOf); 750 } 751 752 public String fhirType() { 753 return "MedicationRequest.requester"; 754 755 } 756 757 } 758 759 @Block() 760 public static class MedicationRequestDispenseRequestComponent extends BackboneElement implements IBaseBackboneElement { 761 /** 762 * This indicates the validity period of a prescription (stale dating the Prescription). 763 */ 764 @Child(name = "validityPeriod", type = {Period.class}, order=1, min=0, max=1, modifier=false, summary=false) 765 @Description(shortDefinition="Time period supply is authorized for", formalDefinition="This indicates the validity period of a prescription (stale dating the Prescription)." ) 766 protected Period validityPeriod; 767 768 /** 769 * An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. 770 */ 771 @Child(name = "numberOfRepeatsAllowed", type = {PositiveIntType.class}, order=2, min=0, max=1, modifier=false, summary=false) 772 @Description(shortDefinition="Number of refills authorized", formalDefinition="An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets." ) 773 protected PositiveIntType numberOfRepeatsAllowed; 774 775 /** 776 * The amount that is to be dispensed for one fill. 777 */ 778 @Child(name = "quantity", type = {SimpleQuantity.class}, order=3, min=0, max=1, modifier=false, summary=false) 779 @Description(shortDefinition="Amount of medication to supply per dispense", formalDefinition="The amount that is to be dispensed for one fill." ) 780 protected SimpleQuantity quantity; 781 782 /** 783 * Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last. 784 */ 785 @Child(name = "expectedSupplyDuration", type = {Duration.class}, order=4, min=0, max=1, modifier=false, summary=false) 786 @Description(shortDefinition="Number of days supply per dispense", formalDefinition="Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last." ) 787 protected Duration expectedSupplyDuration; 788 789 /** 790 * Indicates the intended dispensing Organization specified by the prescriber. 791 */ 792 @Child(name = "performer", type = {Organization.class}, order=5, min=0, max=1, modifier=false, summary=false) 793 @Description(shortDefinition="Intended dispenser", formalDefinition="Indicates the intended dispensing Organization specified by the prescriber." ) 794 protected Reference performer; 795 796 /** 797 * The actual object that is the target of the reference (Indicates the intended dispensing Organization specified by the prescriber.) 798 */ 799 protected Organization performerTarget; 800 801 private static final long serialVersionUID = 280197622L; 802 803 /** 804 * Constructor 805 */ 806 public MedicationRequestDispenseRequestComponent() { 807 super(); 808 } 809 810 /** 811 * @return {@link #validityPeriod} (This indicates the validity period of a prescription (stale dating the Prescription).) 812 */ 813 public Period getValidityPeriod() { 814 if (this.validityPeriod == null) 815 if (Configuration.errorOnAutoCreate()) 816 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.validityPeriod"); 817 else if (Configuration.doAutoCreate()) 818 this.validityPeriod = new Period(); // cc 819 return this.validityPeriod; 820 } 821 822 public boolean hasValidityPeriod() { 823 return this.validityPeriod != null && !this.validityPeriod.isEmpty(); 824 } 825 826 /** 827 * @param value {@link #validityPeriod} (This indicates the validity period of a prescription (stale dating the Prescription).) 828 */ 829 public MedicationRequestDispenseRequestComponent setValidityPeriod(Period value) { 830 this.validityPeriod = value; 831 return this; 832 } 833 834 /** 835 * @return {@link #numberOfRepeatsAllowed} (An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets.). This is the underlying object with id, value and extensions. The accessor "getNumberOfRepeatsAllowed" gives direct access to the value 836 */ 837 public PositiveIntType getNumberOfRepeatsAllowedElement() { 838 if (this.numberOfRepeatsAllowed == null) 839 if (Configuration.errorOnAutoCreate()) 840 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.numberOfRepeatsAllowed"); 841 else if (Configuration.doAutoCreate()) 842 this.numberOfRepeatsAllowed = new PositiveIntType(); // bb 843 return this.numberOfRepeatsAllowed; 844 } 845 846 public boolean hasNumberOfRepeatsAllowedElement() { 847 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 848 } 849 850 public boolean hasNumberOfRepeatsAllowed() { 851 return this.numberOfRepeatsAllowed != null && !this.numberOfRepeatsAllowed.isEmpty(); 852 } 853 854 /** 855 * @param value {@link #numberOfRepeatsAllowed} (An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets.). This is the underlying object with id, value and extensions. The accessor "getNumberOfRepeatsAllowed" gives direct access to the value 856 */ 857 public MedicationRequestDispenseRequestComponent setNumberOfRepeatsAllowedElement(PositiveIntType value) { 858 this.numberOfRepeatsAllowed = value; 859 return this; 860 } 861 862 /** 863 * @return An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. 864 */ 865 public int getNumberOfRepeatsAllowed() { 866 return this.numberOfRepeatsAllowed == null || this.numberOfRepeatsAllowed.isEmpty() ? 0 : this.numberOfRepeatsAllowed.getValue(); 867 } 868 869 /** 870 * @param value An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus "3 repeats", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets. 871 */ 872 public MedicationRequestDispenseRequestComponent setNumberOfRepeatsAllowed(int value) { 873 if (this.numberOfRepeatsAllowed == null) 874 this.numberOfRepeatsAllowed = new PositiveIntType(); 875 this.numberOfRepeatsAllowed.setValue(value); 876 return this; 877 } 878 879 /** 880 * @return {@link #quantity} (The amount that is to be dispensed for one fill.) 881 */ 882 public SimpleQuantity getQuantity() { 883 if (this.quantity == null) 884 if (Configuration.errorOnAutoCreate()) 885 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.quantity"); 886 else if (Configuration.doAutoCreate()) 887 this.quantity = new SimpleQuantity(); // cc 888 return this.quantity; 889 } 890 891 public boolean hasQuantity() { 892 return this.quantity != null && !this.quantity.isEmpty(); 893 } 894 895 /** 896 * @param value {@link #quantity} (The amount that is to be dispensed for one fill.) 897 */ 898 public MedicationRequestDispenseRequestComponent setQuantity(SimpleQuantity value) { 899 this.quantity = value; 900 return this; 901 } 902 903 /** 904 * @return {@link #expectedSupplyDuration} (Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.) 905 */ 906 public Duration getExpectedSupplyDuration() { 907 if (this.expectedSupplyDuration == null) 908 if (Configuration.errorOnAutoCreate()) 909 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.expectedSupplyDuration"); 910 else if (Configuration.doAutoCreate()) 911 this.expectedSupplyDuration = new Duration(); // cc 912 return this.expectedSupplyDuration; 913 } 914 915 public boolean hasExpectedSupplyDuration() { 916 return this.expectedSupplyDuration != null && !this.expectedSupplyDuration.isEmpty(); 917 } 918 919 /** 920 * @param value {@link #expectedSupplyDuration} (Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.) 921 */ 922 public MedicationRequestDispenseRequestComponent setExpectedSupplyDuration(Duration value) { 923 this.expectedSupplyDuration = value; 924 return this; 925 } 926 927 /** 928 * @return {@link #performer} (Indicates the intended dispensing Organization specified by the prescriber.) 929 */ 930 public Reference getPerformer() { 931 if (this.performer == null) 932 if (Configuration.errorOnAutoCreate()) 933 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.performer"); 934 else if (Configuration.doAutoCreate()) 935 this.performer = new Reference(); // cc 936 return this.performer; 937 } 938 939 public boolean hasPerformer() { 940 return this.performer != null && !this.performer.isEmpty(); 941 } 942 943 /** 944 * @param value {@link #performer} (Indicates the intended dispensing Organization specified by the prescriber.) 945 */ 946 public MedicationRequestDispenseRequestComponent setPerformer(Reference value) { 947 this.performer = value; 948 return this; 949 } 950 951 /** 952 * @return {@link #performer} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (Indicates the intended dispensing Organization specified by the prescriber.) 953 */ 954 public Organization getPerformerTarget() { 955 if (this.performerTarget == null) 956 if (Configuration.errorOnAutoCreate()) 957 throw new Error("Attempt to auto-create MedicationRequestDispenseRequestComponent.performer"); 958 else if (Configuration.doAutoCreate()) 959 this.performerTarget = new Organization(); // aa 960 return this.performerTarget; 961 } 962 963 /** 964 * @param value {@link #performer} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (Indicates the intended dispensing Organization specified by the prescriber.) 965 */ 966 public MedicationRequestDispenseRequestComponent setPerformerTarget(Organization value) { 967 this.performerTarget = value; 968 return this; 969 } 970 971 protected void listChildren(List<Property> children) { 972 super.listChildren(children); 973 children.add(new Property("validityPeriod", "Period", "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1, validityPeriod)); 974 children.add(new Property("numberOfRepeatsAllowed", "positiveInt", "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets.", 0, 1, numberOfRepeatsAllowed)); 975 children.add(new Property("quantity", "SimpleQuantity", "The amount that is to be dispensed for one fill.", 0, 1, quantity)); 976 children.add(new Property("expectedSupplyDuration", "Duration", "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 0, 1, expectedSupplyDuration)); 977 children.add(new Property("performer", "Reference(Organization)", "Indicates the intended dispensing Organization specified by the prescriber.", 0, 1, performer)); 978 } 979 980 @Override 981 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 982 switch (_hash) { 983 case -1434195053: /*validityPeriod*/ return new Property("validityPeriod", "Period", "This indicates the validity period of a prescription (stale dating the Prescription).", 0, 1, validityPeriod); 984 case -239736976: /*numberOfRepeatsAllowed*/ return new Property("numberOfRepeatsAllowed", "positiveInt", "An integer indicating the number of times, in addition to the original dispense, (aka refills or repeats) that the patient can receive the prescribed medication. Usage Notes: This integer does not include the original order dispense. This means that if an order indicates dispense 30 tablets plus \"3 repeats\", then the order can be dispensed a total of 4 times and the patient can receive a total of 120 tablets.", 0, 1, numberOfRepeatsAllowed); 985 case -1285004149: /*quantity*/ return new Property("quantity", "SimpleQuantity", "The amount that is to be dispensed for one fill.", 0, 1, quantity); 986 case -1910182789: /*expectedSupplyDuration*/ return new Property("expectedSupplyDuration", "Duration", "Identifies the period time over which the supplied product is expected to be used, or the length of time the dispense is expected to last.", 0, 1, expectedSupplyDuration); 987 case 481140686: /*performer*/ return new Property("performer", "Reference(Organization)", "Indicates the intended dispensing Organization specified by the prescriber.", 0, 1, performer); 988 default: return super.getNamedProperty(_hash, _name, _checkValid); 989 } 990 991 } 992 993 @Override 994 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 995 switch (hash) { 996 case -1434195053: /*validityPeriod*/ return this.validityPeriod == null ? new Base[0] : new Base[] {this.validityPeriod}; // Period 997 case -239736976: /*numberOfRepeatsAllowed*/ return this.numberOfRepeatsAllowed == null ? new Base[0] : new Base[] {this.numberOfRepeatsAllowed}; // PositiveIntType 998 case -1285004149: /*quantity*/ return this.quantity == null ? new Base[0] : new Base[] {this.quantity}; // SimpleQuantity 999 case -1910182789: /*expectedSupplyDuration*/ return this.expectedSupplyDuration == null ? new Base[0] : new Base[] {this.expectedSupplyDuration}; // Duration 1000 case 481140686: /*performer*/ return this.performer == null ? new Base[0] : new Base[] {this.performer}; // Reference 1001 default: return super.getProperty(hash, name, checkValid); 1002 } 1003 1004 } 1005 1006 @Override 1007 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1008 switch (hash) { 1009 case -1434195053: // validityPeriod 1010 this.validityPeriod = castToPeriod(value); // Period 1011 return value; 1012 case -239736976: // numberOfRepeatsAllowed 1013 this.numberOfRepeatsAllowed = castToPositiveInt(value); // PositiveIntType 1014 return value; 1015 case -1285004149: // quantity 1016 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1017 return value; 1018 case -1910182789: // expectedSupplyDuration 1019 this.expectedSupplyDuration = castToDuration(value); // Duration 1020 return value; 1021 case 481140686: // performer 1022 this.performer = castToReference(value); // Reference 1023 return value; 1024 default: return super.setProperty(hash, name, value); 1025 } 1026 1027 } 1028 1029 @Override 1030 public Base setProperty(String name, Base value) throws FHIRException { 1031 if (name.equals("validityPeriod")) { 1032 this.validityPeriod = castToPeriod(value); // Period 1033 } else if (name.equals("numberOfRepeatsAllowed")) { 1034 this.numberOfRepeatsAllowed = castToPositiveInt(value); // PositiveIntType 1035 } else if (name.equals("quantity")) { 1036 this.quantity = castToSimpleQuantity(value); // SimpleQuantity 1037 } else if (name.equals("expectedSupplyDuration")) { 1038 this.expectedSupplyDuration = castToDuration(value); // Duration 1039 } else if (name.equals("performer")) { 1040 this.performer = castToReference(value); // Reference 1041 } else 1042 return super.setProperty(name, value); 1043 return value; 1044 } 1045 1046 @Override 1047 public Base makeProperty(int hash, String name) throws FHIRException { 1048 switch (hash) { 1049 case -1434195053: return getValidityPeriod(); 1050 case -239736976: return getNumberOfRepeatsAllowedElement(); 1051 case -1285004149: return getQuantity(); 1052 case -1910182789: return getExpectedSupplyDuration(); 1053 case 481140686: return getPerformer(); 1054 default: return super.makeProperty(hash, name); 1055 } 1056 1057 } 1058 1059 @Override 1060 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1061 switch (hash) { 1062 case -1434195053: /*validityPeriod*/ return new String[] {"Period"}; 1063 case -239736976: /*numberOfRepeatsAllowed*/ return new String[] {"positiveInt"}; 1064 case -1285004149: /*quantity*/ return new String[] {"SimpleQuantity"}; 1065 case -1910182789: /*expectedSupplyDuration*/ return new String[] {"Duration"}; 1066 case 481140686: /*performer*/ return new String[] {"Reference"}; 1067 default: return super.getTypesForProperty(hash, name); 1068 } 1069 1070 } 1071 1072 @Override 1073 public Base addChild(String name) throws FHIRException { 1074 if (name.equals("validityPeriod")) { 1075 this.validityPeriod = new Period(); 1076 return this.validityPeriod; 1077 } 1078 else if (name.equals("numberOfRepeatsAllowed")) { 1079 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.numberOfRepeatsAllowed"); 1080 } 1081 else if (name.equals("quantity")) { 1082 this.quantity = new SimpleQuantity(); 1083 return this.quantity; 1084 } 1085 else if (name.equals("expectedSupplyDuration")) { 1086 this.expectedSupplyDuration = new Duration(); 1087 return this.expectedSupplyDuration; 1088 } 1089 else if (name.equals("performer")) { 1090 this.performer = new Reference(); 1091 return this.performer; 1092 } 1093 else 1094 return super.addChild(name); 1095 } 1096 1097 public MedicationRequestDispenseRequestComponent copy() { 1098 MedicationRequestDispenseRequestComponent dst = new MedicationRequestDispenseRequestComponent(); 1099 copyValues(dst); 1100 dst.validityPeriod = validityPeriod == null ? null : validityPeriod.copy(); 1101 dst.numberOfRepeatsAllowed = numberOfRepeatsAllowed == null ? null : numberOfRepeatsAllowed.copy(); 1102 dst.quantity = quantity == null ? null : quantity.copy(); 1103 dst.expectedSupplyDuration = expectedSupplyDuration == null ? null : expectedSupplyDuration.copy(); 1104 dst.performer = performer == null ? null : performer.copy(); 1105 return dst; 1106 } 1107 1108 @Override 1109 public boolean equalsDeep(Base other_) { 1110 if (!super.equalsDeep(other_)) 1111 return false; 1112 if (!(other_ instanceof MedicationRequestDispenseRequestComponent)) 1113 return false; 1114 MedicationRequestDispenseRequestComponent o = (MedicationRequestDispenseRequestComponent) other_; 1115 return compareDeep(validityPeriod, o.validityPeriod, true) && compareDeep(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true) 1116 && compareDeep(quantity, o.quantity, true) && compareDeep(expectedSupplyDuration, o.expectedSupplyDuration, true) 1117 && compareDeep(performer, o.performer, true); 1118 } 1119 1120 @Override 1121 public boolean equalsShallow(Base other_) { 1122 if (!super.equalsShallow(other_)) 1123 return false; 1124 if (!(other_ instanceof MedicationRequestDispenseRequestComponent)) 1125 return false; 1126 MedicationRequestDispenseRequestComponent o = (MedicationRequestDispenseRequestComponent) other_; 1127 return compareValues(numberOfRepeatsAllowed, o.numberOfRepeatsAllowed, true); 1128 } 1129 1130 public boolean isEmpty() { 1131 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(validityPeriod, numberOfRepeatsAllowed 1132 , quantity, expectedSupplyDuration, performer); 1133 } 1134 1135 public String fhirType() { 1136 return "MedicationRequest.dispenseRequest"; 1137 1138 } 1139 1140 } 1141 1142 @Block() 1143 public static class MedicationRequestSubstitutionComponent extends BackboneElement implements IBaseBackboneElement { 1144 /** 1145 * True if the prescriber allows a different drug to be dispensed from what was prescribed. 1146 */ 1147 @Child(name = "allowed", type = {BooleanType.class}, order=1, min=1, max=1, modifier=true, summary=false) 1148 @Description(shortDefinition="Whether substitution is allowed or not", formalDefinition="True if the prescriber allows a different drug to be dispensed from what was prescribed." ) 1149 protected BooleanType allowed; 1150 1151 /** 1152 * Indicates the reason for the substitution, or why substitution must or must not be performed. 1153 */ 1154 @Child(name = "reason", type = {CodeableConcept.class}, order=2, min=0, max=1, modifier=false, summary=false) 1155 @Description(shortDefinition="Why should (not) substitution be made", formalDefinition="Indicates the reason for the substitution, or why substitution must or must not be performed." ) 1156 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/v3-SubstanceAdminSubstitutionReason") 1157 protected CodeableConcept reason; 1158 1159 private static final long serialVersionUID = -141547037L; 1160 1161 /** 1162 * Constructor 1163 */ 1164 public MedicationRequestSubstitutionComponent() { 1165 super(); 1166 } 1167 1168 /** 1169 * Constructor 1170 */ 1171 public MedicationRequestSubstitutionComponent(BooleanType allowed) { 1172 super(); 1173 this.allowed = allowed; 1174 } 1175 1176 /** 1177 * @return {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.). This is the underlying object with id, value and extensions. The accessor "getAllowed" gives direct access to the value 1178 */ 1179 public BooleanType getAllowedElement() { 1180 if (this.allowed == null) 1181 if (Configuration.errorOnAutoCreate()) 1182 throw new Error("Attempt to auto-create MedicationRequestSubstitutionComponent.allowed"); 1183 else if (Configuration.doAutoCreate()) 1184 this.allowed = new BooleanType(); // bb 1185 return this.allowed; 1186 } 1187 1188 public boolean hasAllowedElement() { 1189 return this.allowed != null && !this.allowed.isEmpty(); 1190 } 1191 1192 public boolean hasAllowed() { 1193 return this.allowed != null && !this.allowed.isEmpty(); 1194 } 1195 1196 /** 1197 * @param value {@link #allowed} (True if the prescriber allows a different drug to be dispensed from what was prescribed.). This is the underlying object with id, value and extensions. The accessor "getAllowed" gives direct access to the value 1198 */ 1199 public MedicationRequestSubstitutionComponent setAllowedElement(BooleanType value) { 1200 this.allowed = value; 1201 return this; 1202 } 1203 1204 /** 1205 * @return True if the prescriber allows a different drug to be dispensed from what was prescribed. 1206 */ 1207 public boolean getAllowed() { 1208 return this.allowed == null || this.allowed.isEmpty() ? false : this.allowed.getValue(); 1209 } 1210 1211 /** 1212 * @param value True if the prescriber allows a different drug to be dispensed from what was prescribed. 1213 */ 1214 public MedicationRequestSubstitutionComponent setAllowed(boolean value) { 1215 if (this.allowed == null) 1216 this.allowed = new BooleanType(); 1217 this.allowed.setValue(value); 1218 return this; 1219 } 1220 1221 /** 1222 * @return {@link #reason} (Indicates the reason for the substitution, or why substitution must or must not be performed.) 1223 */ 1224 public CodeableConcept getReason() { 1225 if (this.reason == null) 1226 if (Configuration.errorOnAutoCreate()) 1227 throw new Error("Attempt to auto-create MedicationRequestSubstitutionComponent.reason"); 1228 else if (Configuration.doAutoCreate()) 1229 this.reason = new CodeableConcept(); // cc 1230 return this.reason; 1231 } 1232 1233 public boolean hasReason() { 1234 return this.reason != null && !this.reason.isEmpty(); 1235 } 1236 1237 /** 1238 * @param value {@link #reason} (Indicates the reason for the substitution, or why substitution must or must not be performed.) 1239 */ 1240 public MedicationRequestSubstitutionComponent setReason(CodeableConcept value) { 1241 this.reason = value; 1242 return this; 1243 } 1244 1245 protected void listChildren(List<Property> children) { 1246 super.listChildren(children); 1247 children.add(new Property("allowed", "boolean", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed)); 1248 children.add(new Property("reason", "CodeableConcept", "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1, reason)); 1249 } 1250 1251 @Override 1252 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1253 switch (_hash) { 1254 case -911343192: /*allowed*/ return new Property("allowed", "boolean", "True if the prescriber allows a different drug to be dispensed from what was prescribed.", 0, 1, allowed); 1255 case -934964668: /*reason*/ return new Property("reason", "CodeableConcept", "Indicates the reason for the substitution, or why substitution must or must not be performed.", 0, 1, reason); 1256 default: return super.getNamedProperty(_hash, _name, _checkValid); 1257 } 1258 1259 } 1260 1261 @Override 1262 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1263 switch (hash) { 1264 case -911343192: /*allowed*/ return this.allowed == null ? new Base[0] : new Base[] {this.allowed}; // BooleanType 1265 case -934964668: /*reason*/ return this.reason == null ? new Base[0] : new Base[] {this.reason}; // CodeableConcept 1266 default: return super.getProperty(hash, name, checkValid); 1267 } 1268 1269 } 1270 1271 @Override 1272 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1273 switch (hash) { 1274 case -911343192: // allowed 1275 this.allowed = castToBoolean(value); // BooleanType 1276 return value; 1277 case -934964668: // reason 1278 this.reason = castToCodeableConcept(value); // CodeableConcept 1279 return value; 1280 default: return super.setProperty(hash, name, value); 1281 } 1282 1283 } 1284 1285 @Override 1286 public Base setProperty(String name, Base value) throws FHIRException { 1287 if (name.equals("allowed")) { 1288 this.allowed = castToBoolean(value); // BooleanType 1289 } else if (name.equals("reason")) { 1290 this.reason = castToCodeableConcept(value); // CodeableConcept 1291 } else 1292 return super.setProperty(name, value); 1293 return value; 1294 } 1295 1296 @Override 1297 public Base makeProperty(int hash, String name) throws FHIRException { 1298 switch (hash) { 1299 case -911343192: return getAllowedElement(); 1300 case -934964668: return getReason(); 1301 default: return super.makeProperty(hash, name); 1302 } 1303 1304 } 1305 1306 @Override 1307 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1308 switch (hash) { 1309 case -911343192: /*allowed*/ return new String[] {"boolean"}; 1310 case -934964668: /*reason*/ return new String[] {"CodeableConcept"}; 1311 default: return super.getTypesForProperty(hash, name); 1312 } 1313 1314 } 1315 1316 @Override 1317 public Base addChild(String name) throws FHIRException { 1318 if (name.equals("allowed")) { 1319 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.allowed"); 1320 } 1321 else if (name.equals("reason")) { 1322 this.reason = new CodeableConcept(); 1323 return this.reason; 1324 } 1325 else 1326 return super.addChild(name); 1327 } 1328 1329 public MedicationRequestSubstitutionComponent copy() { 1330 MedicationRequestSubstitutionComponent dst = new MedicationRequestSubstitutionComponent(); 1331 copyValues(dst); 1332 dst.allowed = allowed == null ? null : allowed.copy(); 1333 dst.reason = reason == null ? null : reason.copy(); 1334 return dst; 1335 } 1336 1337 @Override 1338 public boolean equalsDeep(Base other_) { 1339 if (!super.equalsDeep(other_)) 1340 return false; 1341 if (!(other_ instanceof MedicationRequestSubstitutionComponent)) 1342 return false; 1343 MedicationRequestSubstitutionComponent o = (MedicationRequestSubstitutionComponent) other_; 1344 return compareDeep(allowed, o.allowed, true) && compareDeep(reason, o.reason, true); 1345 } 1346 1347 @Override 1348 public boolean equalsShallow(Base other_) { 1349 if (!super.equalsShallow(other_)) 1350 return false; 1351 if (!(other_ instanceof MedicationRequestSubstitutionComponent)) 1352 return false; 1353 MedicationRequestSubstitutionComponent o = (MedicationRequestSubstitutionComponent) other_; 1354 return compareValues(allowed, o.allowed, true); 1355 } 1356 1357 public boolean isEmpty() { 1358 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(allowed, reason); 1359 } 1360 1361 public String fhirType() { 1362 return "MedicationRequest.substitution"; 1363 1364 } 1365 1366 } 1367 1368 /** 1369 * This records identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. For example a re-imbursement system might issue its own id for each prescription that is created. This is particularly important where FHIR only provides part of an entire workflow process where records must be tracked through an entire system. 1370 */ 1371 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1372 @Description(shortDefinition="External ids for this request", formalDefinition="This records identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. For example a re-imbursement system might issue its own id for each prescription that is created. This is particularly important where FHIR only provides part of an entire workflow process where records must be tracked through an entire system." ) 1373 protected List<Identifier> identifier; 1374 1375 /** 1376 * Protocol or definition followed by this request. 1377 */ 1378 @Child(name = "definition", type = {ActivityDefinition.class, PlanDefinition.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1379 @Description(shortDefinition="Protocol or definition", formalDefinition="Protocol or definition followed by this request." ) 1380 protected List<Reference> definition; 1381 /** 1382 * The actual objects that are the target of the reference (Protocol or definition followed by this request.) 1383 */ 1384 protected List<Resource> definitionTarget; 1385 1386 1387 /** 1388 * A plan or request that is fulfilled in whole or in part by this medication request. 1389 */ 1390 @Child(name = "basedOn", type = {CarePlan.class, MedicationRequest.class, ProcedureRequest.class, ReferralRequest.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 1391 @Description(shortDefinition="What request fulfills", formalDefinition="A plan or request that is fulfilled in whole or in part by this medication request." ) 1392 protected List<Reference> basedOn; 1393 /** 1394 * The actual objects that are the target of the reference (A plan or request that is fulfilled in whole or in part by this medication request.) 1395 */ 1396 protected List<Resource> basedOnTarget; 1397 1398 1399 /** 1400 * A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription. 1401 */ 1402 @Child(name = "groupIdentifier", type = {Identifier.class}, order=3, min=0, max=1, modifier=false, summary=true) 1403 @Description(shortDefinition="Composite request this is part of", formalDefinition="A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription." ) 1404 protected Identifier groupIdentifier; 1405 1406 /** 1407 * A code specifying the current state of the order. Generally this will be active or completed state. 1408 */ 1409 @Child(name = "status", type = {CodeType.class}, order=4, min=0, max=1, modifier=true, summary=true) 1410 @Description(shortDefinition="active | on-hold | cancelled | completed | entered-in-error | stopped | draft | unknown", formalDefinition="A code specifying the current state of the order. Generally this will be active or completed state." ) 1411 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-request-status") 1412 protected Enumeration<MedicationRequestStatus> status; 1413 1414 /** 1415 * Whether the request is a proposal, plan, or an original order. 1416 */ 1417 @Child(name = "intent", type = {CodeType.class}, order=5, min=1, max=1, modifier=true, summary=true) 1418 @Description(shortDefinition="proposal | plan | order | instance-order", formalDefinition="Whether the request is a proposal, plan, or an original order." ) 1419 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-request-intent") 1420 protected Enumeration<MedicationRequestIntent> intent; 1421 1422 /** 1423 * Indicates the type of medication order and where the medication is expected to be consumed or administered. 1424 */ 1425 @Child(name = "category", type = {CodeableConcept.class}, order=6, min=0, max=1, modifier=false, summary=false) 1426 @Description(shortDefinition="Type of medication usage", formalDefinition="Indicates the type of medication order and where the medication is expected to be consumed or administered." ) 1427 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-request-category") 1428 protected CodeableConcept category; 1429 1430 /** 1431 * Indicates how quickly the Medication Request should be addressed with respect to other requests. 1432 */ 1433 @Child(name = "priority", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 1434 @Description(shortDefinition="routine | urgent | stat | asap", formalDefinition="Indicates how quickly the Medication Request should be addressed with respect to other requests." ) 1435 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-request-priority") 1436 protected Enumeration<MedicationRequestPriority> priority; 1437 1438 /** 1439 * Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications. 1440 */ 1441 @Child(name = "medication", type = {CodeableConcept.class, Medication.class}, order=8, min=1, max=1, modifier=false, summary=true) 1442 @Description(shortDefinition="Medication to be taken", formalDefinition="Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications." ) 1443 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 1444 protected Type medication; 1445 1446 /** 1447 * A link to a resource representing the person or set of individuals to whom the medication will be given. 1448 */ 1449 @Child(name = "subject", type = {Patient.class, Group.class}, order=9, min=1, max=1, modifier=false, summary=true) 1450 @Description(shortDefinition="Who or group medication request is for", formalDefinition="A link to a resource representing the person or set of individuals to whom the medication will be given." ) 1451 protected Reference subject; 1452 1453 /** 1454 * The actual object that is the target of the reference (A link to a resource representing the person or set of individuals to whom the medication will be given.) 1455 */ 1456 protected Resource subjectTarget; 1457 1458 /** 1459 * A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider. 1460 */ 1461 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=10, min=0, max=1, modifier=false, summary=false) 1462 @Description(shortDefinition="Created during encounter/admission/stay", formalDefinition="A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider." ) 1463 protected Reference context; 1464 1465 /** 1466 * The actual object that is the target of the reference (A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider.) 1467 */ 1468 protected Resource contextTarget; 1469 1470 /** 1471 * Include additional information (for example, patient height and weight) that supports the ordering of the medication. 1472 */ 1473 @Child(name = "supportingInformation", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1474 @Description(shortDefinition="Information to support ordering of the medication", formalDefinition="Include additional information (for example, patient height and weight) that supports the ordering of the medication." ) 1475 protected List<Reference> supportingInformation; 1476 /** 1477 * The actual objects that are the target of the reference (Include additional information (for example, patient height and weight) that supports the ordering of the medication.) 1478 */ 1479 protected List<Resource> supportingInformationTarget; 1480 1481 1482 /** 1483 * The date (and perhaps time) when the prescription was initially written or authored on. 1484 */ 1485 @Child(name = "authoredOn", type = {DateTimeType.class}, order=12, min=0, max=1, modifier=false, summary=true) 1486 @Description(shortDefinition="When request was initially authored", formalDefinition="The date (and perhaps time) when the prescription was initially written or authored on." ) 1487 protected DateTimeType authoredOn; 1488 1489 /** 1490 * The individual, organization or device that initiated the request and has responsibility for its activation. 1491 */ 1492 @Child(name = "requester", type = {}, order=13, min=0, max=1, modifier=false, summary=true) 1493 @Description(shortDefinition="Who/What requested the Request", formalDefinition="The individual, organization or device that initiated the request and has responsibility for its activation." ) 1494 protected MedicationRequestRequesterComponent requester; 1495 1496 /** 1497 * The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order. 1498 */ 1499 @Child(name = "recorder", type = {Practitioner.class}, order=14, min=0, max=1, modifier=false, summary=false) 1500 @Description(shortDefinition="Person who entered the request", formalDefinition="The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order." ) 1501 protected Reference recorder; 1502 1503 /** 1504 * The actual object that is the target of the reference (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 1505 */ 1506 protected Practitioner recorderTarget; 1507 1508 /** 1509 * The reason or the indication for ordering the medication. 1510 */ 1511 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1512 @Description(shortDefinition="Reason or indication for writing the prescription", formalDefinition="The reason or the indication for ordering the medication." ) 1513 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 1514 protected List<CodeableConcept> reasonCode; 1515 1516 /** 1517 * Condition or observation that supports why the medication was ordered. 1518 */ 1519 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1520 @Description(shortDefinition="Condition or Observation that supports why the prescription is being written", formalDefinition="Condition or observation that supports why the medication was ordered." ) 1521 protected List<Reference> reasonReference; 1522 /** 1523 * The actual objects that are the target of the reference (Condition or observation that supports why the medication was ordered.) 1524 */ 1525 protected List<Resource> reasonReferenceTarget; 1526 1527 1528 /** 1529 * Extra information about the prescription that could not be conveyed by the other attributes. 1530 */ 1531 @Child(name = "note", type = {Annotation.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1532 @Description(shortDefinition="Information about the prescription", formalDefinition="Extra information about the prescription that could not be conveyed by the other attributes." ) 1533 protected List<Annotation> note; 1534 1535 /** 1536 * Indicates how the medication is to be used by the patient. 1537 */ 1538 @Child(name = "dosageInstruction", type = {Dosage.class}, order=18, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1539 @Description(shortDefinition="How the medication should be taken", formalDefinition="Indicates how the medication is to be used by the patient." ) 1540 protected List<Dosage> dosageInstruction; 1541 1542 /** 1543 * Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department. 1544 */ 1545 @Child(name = "dispenseRequest", type = {}, order=19, min=0, max=1, modifier=false, summary=false) 1546 @Description(shortDefinition="Medication supply authorization", formalDefinition="Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department." ) 1547 protected MedicationRequestDispenseRequestComponent dispenseRequest; 1548 1549 /** 1550 * Indicates whether or not substitution can or should be part of the dispense. In some cases substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done. 1551 */ 1552 @Child(name = "substitution", type = {}, order=20, min=0, max=1, modifier=false, summary=false) 1553 @Description(shortDefinition="Any restrictions on medication substitution", formalDefinition="Indicates whether or not substitution can or should be part of the dispense. In some cases substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done." ) 1554 protected MedicationRequestSubstitutionComponent substitution; 1555 1556 /** 1557 * A link to a resource representing an earlier order related order or prescription. 1558 */ 1559 @Child(name = "priorPrescription", type = {MedicationRequest.class}, order=21, min=0, max=1, modifier=false, summary=false) 1560 @Description(shortDefinition="An order/prescription that is being replaced", formalDefinition="A link to a resource representing an earlier order related order or prescription." ) 1561 protected Reference priorPrescription; 1562 1563 /** 1564 * The actual object that is the target of the reference (A link to a resource representing an earlier order related order or prescription.) 1565 */ 1566 protected MedicationRequest priorPrescriptionTarget; 1567 1568 /** 1569 * Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc. 1570 */ 1571 @Child(name = "detectedIssue", type = {DetectedIssue.class}, order=22, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1572 @Description(shortDefinition="Clinical Issue with action", formalDefinition="Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc." ) 1573 protected List<Reference> detectedIssue; 1574 /** 1575 * The actual objects that are the target of the reference (Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.) 1576 */ 1577 protected List<DetectedIssue> detectedIssueTarget; 1578 1579 1580 /** 1581 * Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource. 1582 */ 1583 @Child(name = "eventHistory", type = {Provenance.class}, order=23, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 1584 @Description(shortDefinition="A list of events of interest in the lifecycle", formalDefinition="Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource." ) 1585 protected List<Reference> eventHistory; 1586 /** 1587 * The actual objects that are the target of the reference (Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.) 1588 */ 1589 protected List<Provenance> eventHistoryTarget; 1590 1591 1592 private static final long serialVersionUID = 299392400L; 1593 1594 /** 1595 * Constructor 1596 */ 1597 public MedicationRequest() { 1598 super(); 1599 } 1600 1601 /** 1602 * Constructor 1603 */ 1604 public MedicationRequest(Enumeration<MedicationRequestIntent> intent, Type medication, Reference subject) { 1605 super(); 1606 this.intent = intent; 1607 this.medication = medication; 1608 this.subject = subject; 1609 } 1610 1611 /** 1612 * @return {@link #identifier} (This records identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. For example a re-imbursement system might issue its own id for each prescription that is created. This is particularly important where FHIR only provides part of an entire workflow process where records must be tracked through an entire system.) 1613 */ 1614 public List<Identifier> getIdentifier() { 1615 if (this.identifier == null) 1616 this.identifier = new ArrayList<Identifier>(); 1617 return this.identifier; 1618 } 1619 1620 /** 1621 * @return Returns a reference to <code>this</code> for easy method chaining 1622 */ 1623 public MedicationRequest setIdentifier(List<Identifier> theIdentifier) { 1624 this.identifier = theIdentifier; 1625 return this; 1626 } 1627 1628 public boolean hasIdentifier() { 1629 if (this.identifier == null) 1630 return false; 1631 for (Identifier item : this.identifier) 1632 if (!item.isEmpty()) 1633 return true; 1634 return false; 1635 } 1636 1637 public Identifier addIdentifier() { //3 1638 Identifier t = new Identifier(); 1639 if (this.identifier == null) 1640 this.identifier = new ArrayList<Identifier>(); 1641 this.identifier.add(t); 1642 return t; 1643 } 1644 1645 public MedicationRequest addIdentifier(Identifier t) { //3 1646 if (t == null) 1647 return this; 1648 if (this.identifier == null) 1649 this.identifier = new ArrayList<Identifier>(); 1650 this.identifier.add(t); 1651 return this; 1652 } 1653 1654 /** 1655 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 1656 */ 1657 public Identifier getIdentifierFirstRep() { 1658 if (getIdentifier().isEmpty()) { 1659 addIdentifier(); 1660 } 1661 return getIdentifier().get(0); 1662 } 1663 1664 /** 1665 * @return {@link #definition} (Protocol or definition followed by this request.) 1666 */ 1667 public List<Reference> getDefinition() { 1668 if (this.definition == null) 1669 this.definition = new ArrayList<Reference>(); 1670 return this.definition; 1671 } 1672 1673 /** 1674 * @return Returns a reference to <code>this</code> for easy method chaining 1675 */ 1676 public MedicationRequest setDefinition(List<Reference> theDefinition) { 1677 this.definition = theDefinition; 1678 return this; 1679 } 1680 1681 public boolean hasDefinition() { 1682 if (this.definition == null) 1683 return false; 1684 for (Reference item : this.definition) 1685 if (!item.isEmpty()) 1686 return true; 1687 return false; 1688 } 1689 1690 public Reference addDefinition() { //3 1691 Reference t = new Reference(); 1692 if (this.definition == null) 1693 this.definition = new ArrayList<Reference>(); 1694 this.definition.add(t); 1695 return t; 1696 } 1697 1698 public MedicationRequest addDefinition(Reference t) { //3 1699 if (t == null) 1700 return this; 1701 if (this.definition == null) 1702 this.definition = new ArrayList<Reference>(); 1703 this.definition.add(t); 1704 return this; 1705 } 1706 1707 /** 1708 * @return The first repetition of repeating field {@link #definition}, creating it if it does not already exist 1709 */ 1710 public Reference getDefinitionFirstRep() { 1711 if (getDefinition().isEmpty()) { 1712 addDefinition(); 1713 } 1714 return getDefinition().get(0); 1715 } 1716 1717 /** 1718 * @deprecated Use Reference#setResource(IBaseResource) instead 1719 */ 1720 @Deprecated 1721 public List<Resource> getDefinitionTarget() { 1722 if (this.definitionTarget == null) 1723 this.definitionTarget = new ArrayList<Resource>(); 1724 return this.definitionTarget; 1725 } 1726 1727 /** 1728 * @return {@link #basedOn} (A plan or request that is fulfilled in whole or in part by this medication request.) 1729 */ 1730 public List<Reference> getBasedOn() { 1731 if (this.basedOn == null) 1732 this.basedOn = new ArrayList<Reference>(); 1733 return this.basedOn; 1734 } 1735 1736 /** 1737 * @return Returns a reference to <code>this</code> for easy method chaining 1738 */ 1739 public MedicationRequest setBasedOn(List<Reference> theBasedOn) { 1740 this.basedOn = theBasedOn; 1741 return this; 1742 } 1743 1744 public boolean hasBasedOn() { 1745 if (this.basedOn == null) 1746 return false; 1747 for (Reference item : this.basedOn) 1748 if (!item.isEmpty()) 1749 return true; 1750 return false; 1751 } 1752 1753 public Reference addBasedOn() { //3 1754 Reference t = new Reference(); 1755 if (this.basedOn == null) 1756 this.basedOn = new ArrayList<Reference>(); 1757 this.basedOn.add(t); 1758 return t; 1759 } 1760 1761 public MedicationRequest addBasedOn(Reference t) { //3 1762 if (t == null) 1763 return this; 1764 if (this.basedOn == null) 1765 this.basedOn = new ArrayList<Reference>(); 1766 this.basedOn.add(t); 1767 return this; 1768 } 1769 1770 /** 1771 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 1772 */ 1773 public Reference getBasedOnFirstRep() { 1774 if (getBasedOn().isEmpty()) { 1775 addBasedOn(); 1776 } 1777 return getBasedOn().get(0); 1778 } 1779 1780 /** 1781 * @deprecated Use Reference#setResource(IBaseResource) instead 1782 */ 1783 @Deprecated 1784 public List<Resource> getBasedOnTarget() { 1785 if (this.basedOnTarget == null) 1786 this.basedOnTarget = new ArrayList<Resource>(); 1787 return this.basedOnTarget; 1788 } 1789 1790 /** 1791 * @return {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription.) 1792 */ 1793 public Identifier getGroupIdentifier() { 1794 if (this.groupIdentifier == null) 1795 if (Configuration.errorOnAutoCreate()) 1796 throw new Error("Attempt to auto-create MedicationRequest.groupIdentifier"); 1797 else if (Configuration.doAutoCreate()) 1798 this.groupIdentifier = new Identifier(); // cc 1799 return this.groupIdentifier; 1800 } 1801 1802 public boolean hasGroupIdentifier() { 1803 return this.groupIdentifier != null && !this.groupIdentifier.isEmpty(); 1804 } 1805 1806 /** 1807 * @param value {@link #groupIdentifier} (A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription.) 1808 */ 1809 public MedicationRequest setGroupIdentifier(Identifier value) { 1810 this.groupIdentifier = value; 1811 return this; 1812 } 1813 1814 /** 1815 * @return {@link #status} (A code specifying the current state of the order. Generally this will be active or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1816 */ 1817 public Enumeration<MedicationRequestStatus> getStatusElement() { 1818 if (this.status == null) 1819 if (Configuration.errorOnAutoCreate()) 1820 throw new Error("Attempt to auto-create MedicationRequest.status"); 1821 else if (Configuration.doAutoCreate()) 1822 this.status = new Enumeration<MedicationRequestStatus>(new MedicationRequestStatusEnumFactory()); // bb 1823 return this.status; 1824 } 1825 1826 public boolean hasStatusElement() { 1827 return this.status != null && !this.status.isEmpty(); 1828 } 1829 1830 public boolean hasStatus() { 1831 return this.status != null && !this.status.isEmpty(); 1832 } 1833 1834 /** 1835 * @param value {@link #status} (A code specifying the current state of the order. Generally this will be active or completed state.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1836 */ 1837 public MedicationRequest setStatusElement(Enumeration<MedicationRequestStatus> value) { 1838 this.status = value; 1839 return this; 1840 } 1841 1842 /** 1843 * @return A code specifying the current state of the order. Generally this will be active or completed state. 1844 */ 1845 public MedicationRequestStatus getStatus() { 1846 return this.status == null ? null : this.status.getValue(); 1847 } 1848 1849 /** 1850 * @param value A code specifying the current state of the order. Generally this will be active or completed state. 1851 */ 1852 public MedicationRequest setStatus(MedicationRequestStatus value) { 1853 if (value == null) 1854 this.status = null; 1855 else { 1856 if (this.status == null) 1857 this.status = new Enumeration<MedicationRequestStatus>(new MedicationRequestStatusEnumFactory()); 1858 this.status.setValue(value); 1859 } 1860 return this; 1861 } 1862 1863 /** 1864 * @return {@link #intent} (Whether the request is a proposal, plan, or an original order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1865 */ 1866 public Enumeration<MedicationRequestIntent> getIntentElement() { 1867 if (this.intent == null) 1868 if (Configuration.errorOnAutoCreate()) 1869 throw new Error("Attempt to auto-create MedicationRequest.intent"); 1870 else if (Configuration.doAutoCreate()) 1871 this.intent = new Enumeration<MedicationRequestIntent>(new MedicationRequestIntentEnumFactory()); // bb 1872 return this.intent; 1873 } 1874 1875 public boolean hasIntentElement() { 1876 return this.intent != null && !this.intent.isEmpty(); 1877 } 1878 1879 public boolean hasIntent() { 1880 return this.intent != null && !this.intent.isEmpty(); 1881 } 1882 1883 /** 1884 * @param value {@link #intent} (Whether the request is a proposal, plan, or an original order.). This is the underlying object with id, value and extensions. The accessor "getIntent" gives direct access to the value 1885 */ 1886 public MedicationRequest setIntentElement(Enumeration<MedicationRequestIntent> value) { 1887 this.intent = value; 1888 return this; 1889 } 1890 1891 /** 1892 * @return Whether the request is a proposal, plan, or an original order. 1893 */ 1894 public MedicationRequestIntent getIntent() { 1895 return this.intent == null ? null : this.intent.getValue(); 1896 } 1897 1898 /** 1899 * @param value Whether the request is a proposal, plan, or an original order. 1900 */ 1901 public MedicationRequest setIntent(MedicationRequestIntent value) { 1902 if (this.intent == null) 1903 this.intent = new Enumeration<MedicationRequestIntent>(new MedicationRequestIntentEnumFactory()); 1904 this.intent.setValue(value); 1905 return this; 1906 } 1907 1908 /** 1909 * @return {@link #category} (Indicates the type of medication order and where the medication is expected to be consumed or administered.) 1910 */ 1911 public CodeableConcept getCategory() { 1912 if (this.category == null) 1913 if (Configuration.errorOnAutoCreate()) 1914 throw new Error("Attempt to auto-create MedicationRequest.category"); 1915 else if (Configuration.doAutoCreate()) 1916 this.category = new CodeableConcept(); // cc 1917 return this.category; 1918 } 1919 1920 public boolean hasCategory() { 1921 return this.category != null && !this.category.isEmpty(); 1922 } 1923 1924 /** 1925 * @param value {@link #category} (Indicates the type of medication order and where the medication is expected to be consumed or administered.) 1926 */ 1927 public MedicationRequest setCategory(CodeableConcept value) { 1928 this.category = value; 1929 return this; 1930 } 1931 1932 /** 1933 * @return {@link #priority} (Indicates how quickly the Medication Request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1934 */ 1935 public Enumeration<MedicationRequestPriority> getPriorityElement() { 1936 if (this.priority == null) 1937 if (Configuration.errorOnAutoCreate()) 1938 throw new Error("Attempt to auto-create MedicationRequest.priority"); 1939 else if (Configuration.doAutoCreate()) 1940 this.priority = new Enumeration<MedicationRequestPriority>(new MedicationRequestPriorityEnumFactory()); // bb 1941 return this.priority; 1942 } 1943 1944 public boolean hasPriorityElement() { 1945 return this.priority != null && !this.priority.isEmpty(); 1946 } 1947 1948 public boolean hasPriority() { 1949 return this.priority != null && !this.priority.isEmpty(); 1950 } 1951 1952 /** 1953 * @param value {@link #priority} (Indicates how quickly the Medication Request should be addressed with respect to other requests.). This is the underlying object with id, value and extensions. The accessor "getPriority" gives direct access to the value 1954 */ 1955 public MedicationRequest setPriorityElement(Enumeration<MedicationRequestPriority> value) { 1956 this.priority = value; 1957 return this; 1958 } 1959 1960 /** 1961 * @return Indicates how quickly the Medication Request should be addressed with respect to other requests. 1962 */ 1963 public MedicationRequestPriority getPriority() { 1964 return this.priority == null ? null : this.priority.getValue(); 1965 } 1966 1967 /** 1968 * @param value Indicates how quickly the Medication Request should be addressed with respect to other requests. 1969 */ 1970 public MedicationRequest setPriority(MedicationRequestPriority value) { 1971 if (value == null) 1972 this.priority = null; 1973 else { 1974 if (this.priority == null) 1975 this.priority = new Enumeration<MedicationRequestPriority>(new MedicationRequestPriorityEnumFactory()); 1976 this.priority.setValue(value); 1977 } 1978 return this; 1979 } 1980 1981 /** 1982 * @return {@link #medication} (Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.) 1983 */ 1984 public Type getMedication() { 1985 return this.medication; 1986 } 1987 1988 /** 1989 * @return {@link #medication} (Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.) 1990 */ 1991 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 1992 if (this.medication == null) 1993 return null; 1994 if (!(this.medication instanceof CodeableConcept)) 1995 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.medication.getClass().getName()+" was encountered"); 1996 return (CodeableConcept) this.medication; 1997 } 1998 1999 public boolean hasMedicationCodeableConcept() { 2000 return this.medication instanceof CodeableConcept; 2001 } 2002 2003 /** 2004 * @return {@link #medication} (Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.) 2005 */ 2006 public Reference getMedicationReference() throws FHIRException { 2007 if (this.medication == null) 2008 return null; 2009 if (!(this.medication instanceof Reference)) 2010 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.medication.getClass().getName()+" was encountered"); 2011 return (Reference) this.medication; 2012 } 2013 2014 public boolean hasMedicationReference() { 2015 return this.medication instanceof Reference; 2016 } 2017 2018 public boolean hasMedication() { 2019 return this.medication != null && !this.medication.isEmpty(); 2020 } 2021 2022 /** 2023 * @param value {@link #medication} (Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.) 2024 */ 2025 public MedicationRequest setMedication(Type value) throws FHIRFormatError { 2026 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 2027 throw new FHIRFormatError("Not the right type for MedicationRequest.medication[x]: "+value.fhirType()); 2028 this.medication = value; 2029 return this; 2030 } 2031 2032 /** 2033 * @return {@link #subject} (A link to a resource representing the person or set of individuals to whom the medication will be given.) 2034 */ 2035 public Reference getSubject() { 2036 if (this.subject == null) 2037 if (Configuration.errorOnAutoCreate()) 2038 throw new Error("Attempt to auto-create MedicationRequest.subject"); 2039 else if (Configuration.doAutoCreate()) 2040 this.subject = new Reference(); // cc 2041 return this.subject; 2042 } 2043 2044 public boolean hasSubject() { 2045 return this.subject != null && !this.subject.isEmpty(); 2046 } 2047 2048 /** 2049 * @param value {@link #subject} (A link to a resource representing the person or set of individuals to whom the medication will be given.) 2050 */ 2051 public MedicationRequest setSubject(Reference value) { 2052 this.subject = value; 2053 return this; 2054 } 2055 2056 /** 2057 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A link to a resource representing the person or set of individuals to whom the medication will be given.) 2058 */ 2059 public Resource getSubjectTarget() { 2060 return this.subjectTarget; 2061 } 2062 2063 /** 2064 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A link to a resource representing the person or set of individuals to whom the medication will be given.) 2065 */ 2066 public MedicationRequest setSubjectTarget(Resource value) { 2067 this.subjectTarget = value; 2068 return this; 2069 } 2070 2071 /** 2072 * @return {@link #context} (A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider.) 2073 */ 2074 public Reference getContext() { 2075 if (this.context == null) 2076 if (Configuration.errorOnAutoCreate()) 2077 throw new Error("Attempt to auto-create MedicationRequest.context"); 2078 else if (Configuration.doAutoCreate()) 2079 this.context = new Reference(); // cc 2080 return this.context; 2081 } 2082 2083 public boolean hasContext() { 2084 return this.context != null && !this.context.isEmpty(); 2085 } 2086 2087 /** 2088 * @param value {@link #context} (A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider.) 2089 */ 2090 public MedicationRequest setContext(Reference value) { 2091 this.context = value; 2092 return this; 2093 } 2094 2095 /** 2096 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider.) 2097 */ 2098 public Resource getContextTarget() { 2099 return this.contextTarget; 2100 } 2101 2102 /** 2103 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider.) 2104 */ 2105 public MedicationRequest setContextTarget(Resource value) { 2106 this.contextTarget = value; 2107 return this; 2108 } 2109 2110 /** 2111 * @return {@link #supportingInformation} (Include additional information (for example, patient height and weight) that supports the ordering of the medication.) 2112 */ 2113 public List<Reference> getSupportingInformation() { 2114 if (this.supportingInformation == null) 2115 this.supportingInformation = new ArrayList<Reference>(); 2116 return this.supportingInformation; 2117 } 2118 2119 /** 2120 * @return Returns a reference to <code>this</code> for easy method chaining 2121 */ 2122 public MedicationRequest setSupportingInformation(List<Reference> theSupportingInformation) { 2123 this.supportingInformation = theSupportingInformation; 2124 return this; 2125 } 2126 2127 public boolean hasSupportingInformation() { 2128 if (this.supportingInformation == null) 2129 return false; 2130 for (Reference item : this.supportingInformation) 2131 if (!item.isEmpty()) 2132 return true; 2133 return false; 2134 } 2135 2136 public Reference addSupportingInformation() { //3 2137 Reference t = new Reference(); 2138 if (this.supportingInformation == null) 2139 this.supportingInformation = new ArrayList<Reference>(); 2140 this.supportingInformation.add(t); 2141 return t; 2142 } 2143 2144 public MedicationRequest addSupportingInformation(Reference t) { //3 2145 if (t == null) 2146 return this; 2147 if (this.supportingInformation == null) 2148 this.supportingInformation = new ArrayList<Reference>(); 2149 this.supportingInformation.add(t); 2150 return this; 2151 } 2152 2153 /** 2154 * @return The first repetition of repeating field {@link #supportingInformation}, creating it if it does not already exist 2155 */ 2156 public Reference getSupportingInformationFirstRep() { 2157 if (getSupportingInformation().isEmpty()) { 2158 addSupportingInformation(); 2159 } 2160 return getSupportingInformation().get(0); 2161 } 2162 2163 /** 2164 * @deprecated Use Reference#setResource(IBaseResource) instead 2165 */ 2166 @Deprecated 2167 public List<Resource> getSupportingInformationTarget() { 2168 if (this.supportingInformationTarget == null) 2169 this.supportingInformationTarget = new ArrayList<Resource>(); 2170 return this.supportingInformationTarget; 2171 } 2172 2173 /** 2174 * @return {@link #authoredOn} (The date (and perhaps time) when the prescription was initially written or authored on.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2175 */ 2176 public DateTimeType getAuthoredOnElement() { 2177 if (this.authoredOn == null) 2178 if (Configuration.errorOnAutoCreate()) 2179 throw new Error("Attempt to auto-create MedicationRequest.authoredOn"); 2180 else if (Configuration.doAutoCreate()) 2181 this.authoredOn = new DateTimeType(); // bb 2182 return this.authoredOn; 2183 } 2184 2185 public boolean hasAuthoredOnElement() { 2186 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2187 } 2188 2189 public boolean hasAuthoredOn() { 2190 return this.authoredOn != null && !this.authoredOn.isEmpty(); 2191 } 2192 2193 /** 2194 * @param value {@link #authoredOn} (The date (and perhaps time) when the prescription was initially written or authored on.). This is the underlying object with id, value and extensions. The accessor "getAuthoredOn" gives direct access to the value 2195 */ 2196 public MedicationRequest setAuthoredOnElement(DateTimeType value) { 2197 this.authoredOn = value; 2198 return this; 2199 } 2200 2201 /** 2202 * @return The date (and perhaps time) when the prescription was initially written or authored on. 2203 */ 2204 public Date getAuthoredOn() { 2205 return this.authoredOn == null ? null : this.authoredOn.getValue(); 2206 } 2207 2208 /** 2209 * @param value The date (and perhaps time) when the prescription was initially written or authored on. 2210 */ 2211 public MedicationRequest setAuthoredOn(Date value) { 2212 if (value == null) 2213 this.authoredOn = null; 2214 else { 2215 if (this.authoredOn == null) 2216 this.authoredOn = new DateTimeType(); 2217 this.authoredOn.setValue(value); 2218 } 2219 return this; 2220 } 2221 2222 /** 2223 * @return {@link #requester} (The individual, organization or device that initiated the request and has responsibility for its activation.) 2224 */ 2225 public MedicationRequestRequesterComponent getRequester() { 2226 if (this.requester == null) 2227 if (Configuration.errorOnAutoCreate()) 2228 throw new Error("Attempt to auto-create MedicationRequest.requester"); 2229 else if (Configuration.doAutoCreate()) 2230 this.requester = new MedicationRequestRequesterComponent(); // cc 2231 return this.requester; 2232 } 2233 2234 public boolean hasRequester() { 2235 return this.requester != null && !this.requester.isEmpty(); 2236 } 2237 2238 /** 2239 * @param value {@link #requester} (The individual, organization or device that initiated the request and has responsibility for its activation.) 2240 */ 2241 public MedicationRequest setRequester(MedicationRequestRequesterComponent value) { 2242 this.requester = value; 2243 return this; 2244 } 2245 2246 /** 2247 * @return {@link #recorder} (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 2248 */ 2249 public Reference getRecorder() { 2250 if (this.recorder == null) 2251 if (Configuration.errorOnAutoCreate()) 2252 throw new Error("Attempt to auto-create MedicationRequest.recorder"); 2253 else if (Configuration.doAutoCreate()) 2254 this.recorder = new Reference(); // cc 2255 return this.recorder; 2256 } 2257 2258 public boolean hasRecorder() { 2259 return this.recorder != null && !this.recorder.isEmpty(); 2260 } 2261 2262 /** 2263 * @param value {@link #recorder} (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 2264 */ 2265 public MedicationRequest setRecorder(Reference value) { 2266 this.recorder = value; 2267 return this; 2268 } 2269 2270 /** 2271 * @return {@link #recorder} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 2272 */ 2273 public Practitioner getRecorderTarget() { 2274 if (this.recorderTarget == null) 2275 if (Configuration.errorOnAutoCreate()) 2276 throw new Error("Attempt to auto-create MedicationRequest.recorder"); 2277 else if (Configuration.doAutoCreate()) 2278 this.recorderTarget = new Practitioner(); // aa 2279 return this.recorderTarget; 2280 } 2281 2282 /** 2283 * @param value {@link #recorder} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.) 2284 */ 2285 public MedicationRequest setRecorderTarget(Practitioner value) { 2286 this.recorderTarget = value; 2287 return this; 2288 } 2289 2290 /** 2291 * @return {@link #reasonCode} (The reason or the indication for ordering the medication.) 2292 */ 2293 public List<CodeableConcept> getReasonCode() { 2294 if (this.reasonCode == null) 2295 this.reasonCode = new ArrayList<CodeableConcept>(); 2296 return this.reasonCode; 2297 } 2298 2299 /** 2300 * @return Returns a reference to <code>this</code> for easy method chaining 2301 */ 2302 public MedicationRequest setReasonCode(List<CodeableConcept> theReasonCode) { 2303 this.reasonCode = theReasonCode; 2304 return this; 2305 } 2306 2307 public boolean hasReasonCode() { 2308 if (this.reasonCode == null) 2309 return false; 2310 for (CodeableConcept item : this.reasonCode) 2311 if (!item.isEmpty()) 2312 return true; 2313 return false; 2314 } 2315 2316 public CodeableConcept addReasonCode() { //3 2317 CodeableConcept t = new CodeableConcept(); 2318 if (this.reasonCode == null) 2319 this.reasonCode = new ArrayList<CodeableConcept>(); 2320 this.reasonCode.add(t); 2321 return t; 2322 } 2323 2324 public MedicationRequest addReasonCode(CodeableConcept t) { //3 2325 if (t == null) 2326 return this; 2327 if (this.reasonCode == null) 2328 this.reasonCode = new ArrayList<CodeableConcept>(); 2329 this.reasonCode.add(t); 2330 return this; 2331 } 2332 2333 /** 2334 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 2335 */ 2336 public CodeableConcept getReasonCodeFirstRep() { 2337 if (getReasonCode().isEmpty()) { 2338 addReasonCode(); 2339 } 2340 return getReasonCode().get(0); 2341 } 2342 2343 /** 2344 * @return {@link #reasonReference} (Condition or observation that supports why the medication was ordered.) 2345 */ 2346 public List<Reference> getReasonReference() { 2347 if (this.reasonReference == null) 2348 this.reasonReference = new ArrayList<Reference>(); 2349 return this.reasonReference; 2350 } 2351 2352 /** 2353 * @return Returns a reference to <code>this</code> for easy method chaining 2354 */ 2355 public MedicationRequest setReasonReference(List<Reference> theReasonReference) { 2356 this.reasonReference = theReasonReference; 2357 return this; 2358 } 2359 2360 public boolean hasReasonReference() { 2361 if (this.reasonReference == null) 2362 return false; 2363 for (Reference item : this.reasonReference) 2364 if (!item.isEmpty()) 2365 return true; 2366 return false; 2367 } 2368 2369 public Reference addReasonReference() { //3 2370 Reference t = new Reference(); 2371 if (this.reasonReference == null) 2372 this.reasonReference = new ArrayList<Reference>(); 2373 this.reasonReference.add(t); 2374 return t; 2375 } 2376 2377 public MedicationRequest addReasonReference(Reference t) { //3 2378 if (t == null) 2379 return this; 2380 if (this.reasonReference == null) 2381 this.reasonReference = new ArrayList<Reference>(); 2382 this.reasonReference.add(t); 2383 return this; 2384 } 2385 2386 /** 2387 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 2388 */ 2389 public Reference getReasonReferenceFirstRep() { 2390 if (getReasonReference().isEmpty()) { 2391 addReasonReference(); 2392 } 2393 return getReasonReference().get(0); 2394 } 2395 2396 /** 2397 * @deprecated Use Reference#setResource(IBaseResource) instead 2398 */ 2399 @Deprecated 2400 public List<Resource> getReasonReferenceTarget() { 2401 if (this.reasonReferenceTarget == null) 2402 this.reasonReferenceTarget = new ArrayList<Resource>(); 2403 return this.reasonReferenceTarget; 2404 } 2405 2406 /** 2407 * @return {@link #note} (Extra information about the prescription that could not be conveyed by the other attributes.) 2408 */ 2409 public List<Annotation> getNote() { 2410 if (this.note == null) 2411 this.note = new ArrayList<Annotation>(); 2412 return this.note; 2413 } 2414 2415 /** 2416 * @return Returns a reference to <code>this</code> for easy method chaining 2417 */ 2418 public MedicationRequest setNote(List<Annotation> theNote) { 2419 this.note = theNote; 2420 return this; 2421 } 2422 2423 public boolean hasNote() { 2424 if (this.note == null) 2425 return false; 2426 for (Annotation item : this.note) 2427 if (!item.isEmpty()) 2428 return true; 2429 return false; 2430 } 2431 2432 public Annotation addNote() { //3 2433 Annotation t = new Annotation(); 2434 if (this.note == null) 2435 this.note = new ArrayList<Annotation>(); 2436 this.note.add(t); 2437 return t; 2438 } 2439 2440 public MedicationRequest addNote(Annotation t) { //3 2441 if (t == null) 2442 return this; 2443 if (this.note == null) 2444 this.note = new ArrayList<Annotation>(); 2445 this.note.add(t); 2446 return this; 2447 } 2448 2449 /** 2450 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 2451 */ 2452 public Annotation getNoteFirstRep() { 2453 if (getNote().isEmpty()) { 2454 addNote(); 2455 } 2456 return getNote().get(0); 2457 } 2458 2459 /** 2460 * @return {@link #dosageInstruction} (Indicates how the medication is to be used by the patient.) 2461 */ 2462 public List<Dosage> getDosageInstruction() { 2463 if (this.dosageInstruction == null) 2464 this.dosageInstruction = new ArrayList<Dosage>(); 2465 return this.dosageInstruction; 2466 } 2467 2468 /** 2469 * @return Returns a reference to <code>this</code> for easy method chaining 2470 */ 2471 public MedicationRequest setDosageInstruction(List<Dosage> theDosageInstruction) { 2472 this.dosageInstruction = theDosageInstruction; 2473 return this; 2474 } 2475 2476 public boolean hasDosageInstruction() { 2477 if (this.dosageInstruction == null) 2478 return false; 2479 for (Dosage item : this.dosageInstruction) 2480 if (!item.isEmpty()) 2481 return true; 2482 return false; 2483 } 2484 2485 public Dosage addDosageInstruction() { //3 2486 Dosage t = new Dosage(); 2487 if (this.dosageInstruction == null) 2488 this.dosageInstruction = new ArrayList<Dosage>(); 2489 this.dosageInstruction.add(t); 2490 return t; 2491 } 2492 2493 public MedicationRequest addDosageInstruction(Dosage t) { //3 2494 if (t == null) 2495 return this; 2496 if (this.dosageInstruction == null) 2497 this.dosageInstruction = new ArrayList<Dosage>(); 2498 this.dosageInstruction.add(t); 2499 return this; 2500 } 2501 2502 /** 2503 * @return The first repetition of repeating field {@link #dosageInstruction}, creating it if it does not already exist 2504 */ 2505 public Dosage getDosageInstructionFirstRep() { 2506 if (getDosageInstruction().isEmpty()) { 2507 addDosageInstruction(); 2508 } 2509 return getDosageInstruction().get(0); 2510 } 2511 2512 /** 2513 * @return {@link #dispenseRequest} (Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.) 2514 */ 2515 public MedicationRequestDispenseRequestComponent getDispenseRequest() { 2516 if (this.dispenseRequest == null) 2517 if (Configuration.errorOnAutoCreate()) 2518 throw new Error("Attempt to auto-create MedicationRequest.dispenseRequest"); 2519 else if (Configuration.doAutoCreate()) 2520 this.dispenseRequest = new MedicationRequestDispenseRequestComponent(); // cc 2521 return this.dispenseRequest; 2522 } 2523 2524 public boolean hasDispenseRequest() { 2525 return this.dispenseRequest != null && !this.dispenseRequest.isEmpty(); 2526 } 2527 2528 /** 2529 * @param value {@link #dispenseRequest} (Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.) 2530 */ 2531 public MedicationRequest setDispenseRequest(MedicationRequestDispenseRequestComponent value) { 2532 this.dispenseRequest = value; 2533 return this; 2534 } 2535 2536 /** 2537 * @return {@link #substitution} (Indicates whether or not substitution can or should be part of the dispense. In some cases substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.) 2538 */ 2539 public MedicationRequestSubstitutionComponent getSubstitution() { 2540 if (this.substitution == null) 2541 if (Configuration.errorOnAutoCreate()) 2542 throw new Error("Attempt to auto-create MedicationRequest.substitution"); 2543 else if (Configuration.doAutoCreate()) 2544 this.substitution = new MedicationRequestSubstitutionComponent(); // cc 2545 return this.substitution; 2546 } 2547 2548 public boolean hasSubstitution() { 2549 return this.substitution != null && !this.substitution.isEmpty(); 2550 } 2551 2552 /** 2553 * @param value {@link #substitution} (Indicates whether or not substitution can or should be part of the dispense. In some cases substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.) 2554 */ 2555 public MedicationRequest setSubstitution(MedicationRequestSubstitutionComponent value) { 2556 this.substitution = value; 2557 return this; 2558 } 2559 2560 /** 2561 * @return {@link #priorPrescription} (A link to a resource representing an earlier order related order or prescription.) 2562 */ 2563 public Reference getPriorPrescription() { 2564 if (this.priorPrescription == null) 2565 if (Configuration.errorOnAutoCreate()) 2566 throw new Error("Attempt to auto-create MedicationRequest.priorPrescription"); 2567 else if (Configuration.doAutoCreate()) 2568 this.priorPrescription = new Reference(); // cc 2569 return this.priorPrescription; 2570 } 2571 2572 public boolean hasPriorPrescription() { 2573 return this.priorPrescription != null && !this.priorPrescription.isEmpty(); 2574 } 2575 2576 /** 2577 * @param value {@link #priorPrescription} (A link to a resource representing an earlier order related order or prescription.) 2578 */ 2579 public MedicationRequest setPriorPrescription(Reference value) { 2580 this.priorPrescription = value; 2581 return this; 2582 } 2583 2584 /** 2585 * @return {@link #priorPrescription} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A link to a resource representing an earlier order related order or prescription.) 2586 */ 2587 public MedicationRequest getPriorPrescriptionTarget() { 2588 if (this.priorPrescriptionTarget == null) 2589 if (Configuration.errorOnAutoCreate()) 2590 throw new Error("Attempt to auto-create MedicationRequest.priorPrescription"); 2591 else if (Configuration.doAutoCreate()) 2592 this.priorPrescriptionTarget = new MedicationRequest(); // aa 2593 return this.priorPrescriptionTarget; 2594 } 2595 2596 /** 2597 * @param value {@link #priorPrescription} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A link to a resource representing an earlier order related order or prescription.) 2598 */ 2599 public MedicationRequest setPriorPrescriptionTarget(MedicationRequest value) { 2600 this.priorPrescriptionTarget = value; 2601 return this; 2602 } 2603 2604 /** 2605 * @return {@link #detectedIssue} (Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.) 2606 */ 2607 public List<Reference> getDetectedIssue() { 2608 if (this.detectedIssue == null) 2609 this.detectedIssue = new ArrayList<Reference>(); 2610 return this.detectedIssue; 2611 } 2612 2613 /** 2614 * @return Returns a reference to <code>this</code> for easy method chaining 2615 */ 2616 public MedicationRequest setDetectedIssue(List<Reference> theDetectedIssue) { 2617 this.detectedIssue = theDetectedIssue; 2618 return this; 2619 } 2620 2621 public boolean hasDetectedIssue() { 2622 if (this.detectedIssue == null) 2623 return false; 2624 for (Reference item : this.detectedIssue) 2625 if (!item.isEmpty()) 2626 return true; 2627 return false; 2628 } 2629 2630 public Reference addDetectedIssue() { //3 2631 Reference t = new Reference(); 2632 if (this.detectedIssue == null) 2633 this.detectedIssue = new ArrayList<Reference>(); 2634 this.detectedIssue.add(t); 2635 return t; 2636 } 2637 2638 public MedicationRequest addDetectedIssue(Reference t) { //3 2639 if (t == null) 2640 return this; 2641 if (this.detectedIssue == null) 2642 this.detectedIssue = new ArrayList<Reference>(); 2643 this.detectedIssue.add(t); 2644 return this; 2645 } 2646 2647 /** 2648 * @return The first repetition of repeating field {@link #detectedIssue}, creating it if it does not already exist 2649 */ 2650 public Reference getDetectedIssueFirstRep() { 2651 if (getDetectedIssue().isEmpty()) { 2652 addDetectedIssue(); 2653 } 2654 return getDetectedIssue().get(0); 2655 } 2656 2657 /** 2658 * @deprecated Use Reference#setResource(IBaseResource) instead 2659 */ 2660 @Deprecated 2661 public List<DetectedIssue> getDetectedIssueTarget() { 2662 if (this.detectedIssueTarget == null) 2663 this.detectedIssueTarget = new ArrayList<DetectedIssue>(); 2664 return this.detectedIssueTarget; 2665 } 2666 2667 /** 2668 * @deprecated Use Reference#setResource(IBaseResource) instead 2669 */ 2670 @Deprecated 2671 public DetectedIssue addDetectedIssueTarget() { 2672 DetectedIssue r = new DetectedIssue(); 2673 if (this.detectedIssueTarget == null) 2674 this.detectedIssueTarget = new ArrayList<DetectedIssue>(); 2675 this.detectedIssueTarget.add(r); 2676 return r; 2677 } 2678 2679 /** 2680 * @return {@link #eventHistory} (Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.) 2681 */ 2682 public List<Reference> getEventHistory() { 2683 if (this.eventHistory == null) 2684 this.eventHistory = new ArrayList<Reference>(); 2685 return this.eventHistory; 2686 } 2687 2688 /** 2689 * @return Returns a reference to <code>this</code> for easy method chaining 2690 */ 2691 public MedicationRequest setEventHistory(List<Reference> theEventHistory) { 2692 this.eventHistory = theEventHistory; 2693 return this; 2694 } 2695 2696 public boolean hasEventHistory() { 2697 if (this.eventHistory == null) 2698 return false; 2699 for (Reference item : this.eventHistory) 2700 if (!item.isEmpty()) 2701 return true; 2702 return false; 2703 } 2704 2705 public Reference addEventHistory() { //3 2706 Reference t = new Reference(); 2707 if (this.eventHistory == null) 2708 this.eventHistory = new ArrayList<Reference>(); 2709 this.eventHistory.add(t); 2710 return t; 2711 } 2712 2713 public MedicationRequest addEventHistory(Reference t) { //3 2714 if (t == null) 2715 return this; 2716 if (this.eventHistory == null) 2717 this.eventHistory = new ArrayList<Reference>(); 2718 this.eventHistory.add(t); 2719 return this; 2720 } 2721 2722 /** 2723 * @return The first repetition of repeating field {@link #eventHistory}, creating it if it does not already exist 2724 */ 2725 public Reference getEventHistoryFirstRep() { 2726 if (getEventHistory().isEmpty()) { 2727 addEventHistory(); 2728 } 2729 return getEventHistory().get(0); 2730 } 2731 2732 /** 2733 * @deprecated Use Reference#setResource(IBaseResource) instead 2734 */ 2735 @Deprecated 2736 public List<Provenance> getEventHistoryTarget() { 2737 if (this.eventHistoryTarget == null) 2738 this.eventHistoryTarget = new ArrayList<Provenance>(); 2739 return this.eventHistoryTarget; 2740 } 2741 2742 /** 2743 * @deprecated Use Reference#setResource(IBaseResource) instead 2744 */ 2745 @Deprecated 2746 public Provenance addEventHistoryTarget() { 2747 Provenance r = new Provenance(); 2748 if (this.eventHistoryTarget == null) 2749 this.eventHistoryTarget = new ArrayList<Provenance>(); 2750 this.eventHistoryTarget.add(r); 2751 return r; 2752 } 2753 2754 protected void listChildren(List<Property> children) { 2755 super.listChildren(children); 2756 children.add(new Property("identifier", "Identifier", "This records identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. For example a re-imbursement system might issue its own id for each prescription that is created. This is particularly important where FHIR only provides part of an entire workflow process where records must be tracked through an entire system.", 0, java.lang.Integer.MAX_VALUE, identifier)); 2757 children.add(new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request.", 0, java.lang.Integer.MAX_VALUE, definition)); 2758 children.add(new Property("basedOn", "Reference(CarePlan|MedicationRequest|ProcedureRequest|ReferralRequest)", "A plan or request that is fulfilled in whole or in part by this medication request.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 2759 children.add(new Property("groupIdentifier", "Identifier", "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription.", 0, 1, groupIdentifier)); 2760 children.add(new Property("status", "code", "A code specifying the current state of the order. Generally this will be active or completed state.", 0, 1, status)); 2761 children.add(new Property("intent", "code", "Whether the request is a proposal, plan, or an original order.", 0, 1, intent)); 2762 children.add(new Property("category", "CodeableConcept", "Indicates the type of medication order and where the medication is expected to be consumed or administered.", 0, 1, category)); 2763 children.add(new Property("priority", "code", "Indicates how quickly the Medication Request should be addressed with respect to other requests.", 0, 1, priority)); 2764 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 2765 children.add(new Property("subject", "Reference(Patient|Group)", "A link to a resource representing the person or set of individuals to whom the medication will be given.", 0, 1, subject)); 2766 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider.", 0, 1, context)); 2767 children.add(new Property("supportingInformation", "Reference(Any)", "Include additional information (for example, patient height and weight) that supports the ordering of the medication.", 0, java.lang.Integer.MAX_VALUE, supportingInformation)); 2768 children.add(new Property("authoredOn", "dateTime", "The date (and perhaps time) when the prescription was initially written or authored on.", 0, 1, authoredOn)); 2769 children.add(new Property("requester", "", "The individual, organization or device that initiated the request and has responsibility for its activation.", 0, 1, requester)); 2770 children.add(new Property("recorder", "Reference(Practitioner)", "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.", 0, 1, recorder)); 2771 children.add(new Property("reasonCode", "CodeableConcept", "The reason or the indication for ordering the medication.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 2772 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Condition or observation that supports why the medication was ordered.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 2773 children.add(new Property("note", "Annotation", "Extra information about the prescription that could not be conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 2774 children.add(new Property("dosageInstruction", "Dosage", "Indicates how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction)); 2775 children.add(new Property("dispenseRequest", "", "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 0, 1, dispenseRequest)); 2776 children.add(new Property("substitution", "", "Indicates whether or not substitution can or should be part of the dispense. In some cases substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 0, 1, substitution)); 2777 children.add(new Property("priorPrescription", "Reference(MedicationRequest)", "A link to a resource representing an earlier order related order or prescription.", 0, 1, priorPrescription)); 2778 children.add(new Property("detectedIssue", "Reference(DetectedIssue)", "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.", 0, java.lang.Integer.MAX_VALUE, detectedIssue)); 2779 children.add(new Property("eventHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 0, java.lang.Integer.MAX_VALUE, eventHistory)); 2780 } 2781 2782 @Override 2783 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2784 switch (_hash) { 2785 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "This records identifiers associated with this medication request that are defined by business processes and/or used to refer to it when a direct URL reference to the resource itself is not appropriate. For example a re-imbursement system might issue its own id for each prescription that is created. This is particularly important where FHIR only provides part of an entire workflow process where records must be tracked through an entire system.", 0, java.lang.Integer.MAX_VALUE, identifier); 2786 case -1014418093: /*definition*/ return new Property("definition", "Reference(ActivityDefinition|PlanDefinition)", "Protocol or definition followed by this request.", 0, java.lang.Integer.MAX_VALUE, definition); 2787 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(CarePlan|MedicationRequest|ProcedureRequest|ReferralRequest)", "A plan or request that is fulfilled in whole or in part by this medication request.", 0, java.lang.Integer.MAX_VALUE, basedOn); 2788 case -445338488: /*groupIdentifier*/ return new Property("groupIdentifier", "Identifier", "A shared identifier common to all requests that were authorized more or less simultaneously by a single author, representing the identifier of the requisition or prescription.", 0, 1, groupIdentifier); 2789 case -892481550: /*status*/ return new Property("status", "code", "A code specifying the current state of the order. Generally this will be active or completed state.", 0, 1, status); 2790 case -1183762788: /*intent*/ return new Property("intent", "code", "Whether the request is a proposal, plan, or an original order.", 0, 1, intent); 2791 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates the type of medication order and where the medication is expected to be consumed or administered.", 0, 1, category); 2792 case -1165461084: /*priority*/ return new Property("priority", "code", "Indicates how quickly the Medication Request should be addressed with respect to other requests.", 0, 1, priority); 2793 case 1458402129: /*medication[x]*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2794 case 1998965455: /*medication*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2795 case -209845038: /*medicationCodeableConcept*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2796 case 2104315196: /*medicationReference*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being requested. This is a link to a resource that represents the medication which may be the details of the medication or simply an attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 2797 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "A link to a resource representing the person or set of individuals to whom the medication will be given.", 0, 1, subject); 2798 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "A link to an encounter, or episode of care, that identifies the particular occurrence or set occurrences of contact between patient and health care provider.", 0, 1, context); 2799 case -1248768647: /*supportingInformation*/ return new Property("supportingInformation", "Reference(Any)", "Include additional information (for example, patient height and weight) that supports the ordering of the medication.", 0, java.lang.Integer.MAX_VALUE, supportingInformation); 2800 case -1500852503: /*authoredOn*/ return new Property("authoredOn", "dateTime", "The date (and perhaps time) when the prescription was initially written or authored on.", 0, 1, authoredOn); 2801 case 693933948: /*requester*/ return new Property("requester", "", "The individual, organization or device that initiated the request and has responsibility for its activation.", 0, 1, requester); 2802 case -799233858: /*recorder*/ return new Property("recorder", "Reference(Practitioner)", "The person who entered the order on behalf of another individual for example in the case of a verbal or a telephone order.", 0, 1, recorder); 2803 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "The reason or the indication for ordering the medication.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 2804 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Condition or observation that supports why the medication was ordered.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 2805 case 3387378: /*note*/ return new Property("note", "Annotation", "Extra information about the prescription that could not be conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 2806 case -1201373865: /*dosageInstruction*/ return new Property("dosageInstruction", "Dosage", "Indicates how the medication is to be used by the patient.", 0, java.lang.Integer.MAX_VALUE, dosageInstruction); 2807 case 824620658: /*dispenseRequest*/ return new Property("dispenseRequest", "", "Indicates the specific details for the dispense or medication supply part of a medication request (also known as a Medication Prescription or Medication Order). Note that this information is not always sent with the order. There may be in some settings (e.g. hospitals) institutional or system support for completing the dispense details in the pharmacy department.", 0, 1, dispenseRequest); 2808 case 826147581: /*substitution*/ return new Property("substitution", "", "Indicates whether or not substitution can or should be part of the dispense. In some cases substitution must happen, in other cases substitution must not happen. This block explains the prescriber's intent. If nothing is specified substitution may be done.", 0, 1, substitution); 2809 case -486355964: /*priorPrescription*/ return new Property("priorPrescription", "Reference(MedicationRequest)", "A link to a resource representing an earlier order related order or prescription.", 0, 1, priorPrescription); 2810 case 51602295: /*detectedIssue*/ return new Property("detectedIssue", "Reference(DetectedIssue)", "Indicates an actual or potential clinical issue with or between one or more active or proposed clinical actions for a patient; e.g. Drug-drug interaction, duplicate therapy, dosage alert etc.", 0, java.lang.Integer.MAX_VALUE, detectedIssue); 2811 case 1835190426: /*eventHistory*/ return new Property("eventHistory", "Reference(Provenance)", "Links to Provenance records for past versions of this resource or fulfilling request or event resources that identify key state transitions or updates that are likely to be relevant to a user looking at the current version of the resource.", 0, java.lang.Integer.MAX_VALUE, eventHistory); 2812 default: return super.getNamedProperty(_hash, _name, _checkValid); 2813 } 2814 2815 } 2816 2817 @Override 2818 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2819 switch (hash) { 2820 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 2821 case -1014418093: /*definition*/ return this.definition == null ? new Base[0] : this.definition.toArray(new Base[this.definition.size()]); // Reference 2822 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 2823 case -445338488: /*groupIdentifier*/ return this.groupIdentifier == null ? new Base[0] : new Base[] {this.groupIdentifier}; // Identifier 2824 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationRequestStatus> 2825 case -1183762788: /*intent*/ return this.intent == null ? new Base[0] : new Base[] {this.intent}; // Enumeration<MedicationRequestIntent> 2826 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 2827 case -1165461084: /*priority*/ return this.priority == null ? new Base[0] : new Base[] {this.priority}; // Enumeration<MedicationRequestPriority> 2828 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // Type 2829 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 2830 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 2831 case -1248768647: /*supportingInformation*/ return this.supportingInformation == null ? new Base[0] : this.supportingInformation.toArray(new Base[this.supportingInformation.size()]); // Reference 2832 case -1500852503: /*authoredOn*/ return this.authoredOn == null ? new Base[0] : new Base[] {this.authoredOn}; // DateTimeType 2833 case 693933948: /*requester*/ return this.requester == null ? new Base[0] : new Base[] {this.requester}; // MedicationRequestRequesterComponent 2834 case -799233858: /*recorder*/ return this.recorder == null ? new Base[0] : new Base[] {this.recorder}; // Reference 2835 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 2836 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 2837 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 2838 case -1201373865: /*dosageInstruction*/ return this.dosageInstruction == null ? new Base[0] : this.dosageInstruction.toArray(new Base[this.dosageInstruction.size()]); // Dosage 2839 case 824620658: /*dispenseRequest*/ return this.dispenseRequest == null ? new Base[0] : new Base[] {this.dispenseRequest}; // MedicationRequestDispenseRequestComponent 2840 case 826147581: /*substitution*/ return this.substitution == null ? new Base[0] : new Base[] {this.substitution}; // MedicationRequestSubstitutionComponent 2841 case -486355964: /*priorPrescription*/ return this.priorPrescription == null ? new Base[0] : new Base[] {this.priorPrescription}; // Reference 2842 case 51602295: /*detectedIssue*/ return this.detectedIssue == null ? new Base[0] : this.detectedIssue.toArray(new Base[this.detectedIssue.size()]); // Reference 2843 case 1835190426: /*eventHistory*/ return this.eventHistory == null ? new Base[0] : this.eventHistory.toArray(new Base[this.eventHistory.size()]); // Reference 2844 default: return super.getProperty(hash, name, checkValid); 2845 } 2846 2847 } 2848 2849 @Override 2850 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2851 switch (hash) { 2852 case -1618432855: // identifier 2853 this.getIdentifier().add(castToIdentifier(value)); // Identifier 2854 return value; 2855 case -1014418093: // definition 2856 this.getDefinition().add(castToReference(value)); // Reference 2857 return value; 2858 case -332612366: // basedOn 2859 this.getBasedOn().add(castToReference(value)); // Reference 2860 return value; 2861 case -445338488: // groupIdentifier 2862 this.groupIdentifier = castToIdentifier(value); // Identifier 2863 return value; 2864 case -892481550: // status 2865 value = new MedicationRequestStatusEnumFactory().fromType(castToCode(value)); 2866 this.status = (Enumeration) value; // Enumeration<MedicationRequestStatus> 2867 return value; 2868 case -1183762788: // intent 2869 value = new MedicationRequestIntentEnumFactory().fromType(castToCode(value)); 2870 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 2871 return value; 2872 case 50511102: // category 2873 this.category = castToCodeableConcept(value); // CodeableConcept 2874 return value; 2875 case -1165461084: // priority 2876 value = new MedicationRequestPriorityEnumFactory().fromType(castToCode(value)); 2877 this.priority = (Enumeration) value; // Enumeration<MedicationRequestPriority> 2878 return value; 2879 case 1998965455: // medication 2880 this.medication = castToType(value); // Type 2881 return value; 2882 case -1867885268: // subject 2883 this.subject = castToReference(value); // Reference 2884 return value; 2885 case 951530927: // context 2886 this.context = castToReference(value); // Reference 2887 return value; 2888 case -1248768647: // supportingInformation 2889 this.getSupportingInformation().add(castToReference(value)); // Reference 2890 return value; 2891 case -1500852503: // authoredOn 2892 this.authoredOn = castToDateTime(value); // DateTimeType 2893 return value; 2894 case 693933948: // requester 2895 this.requester = (MedicationRequestRequesterComponent) value; // MedicationRequestRequesterComponent 2896 return value; 2897 case -799233858: // recorder 2898 this.recorder = castToReference(value); // Reference 2899 return value; 2900 case 722137681: // reasonCode 2901 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 2902 return value; 2903 case -1146218137: // reasonReference 2904 this.getReasonReference().add(castToReference(value)); // Reference 2905 return value; 2906 case 3387378: // note 2907 this.getNote().add(castToAnnotation(value)); // Annotation 2908 return value; 2909 case -1201373865: // dosageInstruction 2910 this.getDosageInstruction().add(castToDosage(value)); // Dosage 2911 return value; 2912 case 824620658: // dispenseRequest 2913 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 2914 return value; 2915 case 826147581: // substitution 2916 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 2917 return value; 2918 case -486355964: // priorPrescription 2919 this.priorPrescription = castToReference(value); // Reference 2920 return value; 2921 case 51602295: // detectedIssue 2922 this.getDetectedIssue().add(castToReference(value)); // Reference 2923 return value; 2924 case 1835190426: // eventHistory 2925 this.getEventHistory().add(castToReference(value)); // Reference 2926 return value; 2927 default: return super.setProperty(hash, name, value); 2928 } 2929 2930 } 2931 2932 @Override 2933 public Base setProperty(String name, Base value) throws FHIRException { 2934 if (name.equals("identifier")) { 2935 this.getIdentifier().add(castToIdentifier(value)); 2936 } else if (name.equals("definition")) { 2937 this.getDefinition().add(castToReference(value)); 2938 } else if (name.equals("basedOn")) { 2939 this.getBasedOn().add(castToReference(value)); 2940 } else if (name.equals("groupIdentifier")) { 2941 this.groupIdentifier = castToIdentifier(value); // Identifier 2942 } else if (name.equals("status")) { 2943 value = new MedicationRequestStatusEnumFactory().fromType(castToCode(value)); 2944 this.status = (Enumeration) value; // Enumeration<MedicationRequestStatus> 2945 } else if (name.equals("intent")) { 2946 value = new MedicationRequestIntentEnumFactory().fromType(castToCode(value)); 2947 this.intent = (Enumeration) value; // Enumeration<MedicationRequestIntent> 2948 } else if (name.equals("category")) { 2949 this.category = castToCodeableConcept(value); // CodeableConcept 2950 } else if (name.equals("priority")) { 2951 value = new MedicationRequestPriorityEnumFactory().fromType(castToCode(value)); 2952 this.priority = (Enumeration) value; // Enumeration<MedicationRequestPriority> 2953 } else if (name.equals("medication[x]")) { 2954 this.medication = castToType(value); // Type 2955 } else if (name.equals("subject")) { 2956 this.subject = castToReference(value); // Reference 2957 } else if (name.equals("context")) { 2958 this.context = castToReference(value); // Reference 2959 } else if (name.equals("supportingInformation")) { 2960 this.getSupportingInformation().add(castToReference(value)); 2961 } else if (name.equals("authoredOn")) { 2962 this.authoredOn = castToDateTime(value); // DateTimeType 2963 } else if (name.equals("requester")) { 2964 this.requester = (MedicationRequestRequesterComponent) value; // MedicationRequestRequesterComponent 2965 } else if (name.equals("recorder")) { 2966 this.recorder = castToReference(value); // Reference 2967 } else if (name.equals("reasonCode")) { 2968 this.getReasonCode().add(castToCodeableConcept(value)); 2969 } else if (name.equals("reasonReference")) { 2970 this.getReasonReference().add(castToReference(value)); 2971 } else if (name.equals("note")) { 2972 this.getNote().add(castToAnnotation(value)); 2973 } else if (name.equals("dosageInstruction")) { 2974 this.getDosageInstruction().add(castToDosage(value)); 2975 } else if (name.equals("dispenseRequest")) { 2976 this.dispenseRequest = (MedicationRequestDispenseRequestComponent) value; // MedicationRequestDispenseRequestComponent 2977 } else if (name.equals("substitution")) { 2978 this.substitution = (MedicationRequestSubstitutionComponent) value; // MedicationRequestSubstitutionComponent 2979 } else if (name.equals("priorPrescription")) { 2980 this.priorPrescription = castToReference(value); // Reference 2981 } else if (name.equals("detectedIssue")) { 2982 this.getDetectedIssue().add(castToReference(value)); 2983 } else if (name.equals("eventHistory")) { 2984 this.getEventHistory().add(castToReference(value)); 2985 } else 2986 return super.setProperty(name, value); 2987 return value; 2988 } 2989 2990 @Override 2991 public Base makeProperty(int hash, String name) throws FHIRException { 2992 switch (hash) { 2993 case -1618432855: return addIdentifier(); 2994 case -1014418093: return addDefinition(); 2995 case -332612366: return addBasedOn(); 2996 case -445338488: return getGroupIdentifier(); 2997 case -892481550: return getStatusElement(); 2998 case -1183762788: return getIntentElement(); 2999 case 50511102: return getCategory(); 3000 case -1165461084: return getPriorityElement(); 3001 case 1458402129: return getMedication(); 3002 case 1998965455: return getMedication(); 3003 case -1867885268: return getSubject(); 3004 case 951530927: return getContext(); 3005 case -1248768647: return addSupportingInformation(); 3006 case -1500852503: return getAuthoredOnElement(); 3007 case 693933948: return getRequester(); 3008 case -799233858: return getRecorder(); 3009 case 722137681: return addReasonCode(); 3010 case -1146218137: return addReasonReference(); 3011 case 3387378: return addNote(); 3012 case -1201373865: return addDosageInstruction(); 3013 case 824620658: return getDispenseRequest(); 3014 case 826147581: return getSubstitution(); 3015 case -486355964: return getPriorPrescription(); 3016 case 51602295: return addDetectedIssue(); 3017 case 1835190426: return addEventHistory(); 3018 default: return super.makeProperty(hash, name); 3019 } 3020 3021 } 3022 3023 @Override 3024 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 3025 switch (hash) { 3026 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 3027 case -1014418093: /*definition*/ return new String[] {"Reference"}; 3028 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 3029 case -445338488: /*groupIdentifier*/ return new String[] {"Identifier"}; 3030 case -892481550: /*status*/ return new String[] {"code"}; 3031 case -1183762788: /*intent*/ return new String[] {"code"}; 3032 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 3033 case -1165461084: /*priority*/ return new String[] {"code"}; 3034 case 1998965455: /*medication*/ return new String[] {"CodeableConcept", "Reference"}; 3035 case -1867885268: /*subject*/ return new String[] {"Reference"}; 3036 case 951530927: /*context*/ return new String[] {"Reference"}; 3037 case -1248768647: /*supportingInformation*/ return new String[] {"Reference"}; 3038 case -1500852503: /*authoredOn*/ return new String[] {"dateTime"}; 3039 case 693933948: /*requester*/ return new String[] {}; 3040 case -799233858: /*recorder*/ return new String[] {"Reference"}; 3041 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 3042 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 3043 case 3387378: /*note*/ return new String[] {"Annotation"}; 3044 case -1201373865: /*dosageInstruction*/ return new String[] {"Dosage"}; 3045 case 824620658: /*dispenseRequest*/ return new String[] {}; 3046 case 826147581: /*substitution*/ return new String[] {}; 3047 case -486355964: /*priorPrescription*/ return new String[] {"Reference"}; 3048 case 51602295: /*detectedIssue*/ return new String[] {"Reference"}; 3049 case 1835190426: /*eventHistory*/ return new String[] {"Reference"}; 3050 default: return super.getTypesForProperty(hash, name); 3051 } 3052 3053 } 3054 3055 @Override 3056 public Base addChild(String name) throws FHIRException { 3057 if (name.equals("identifier")) { 3058 return addIdentifier(); 3059 } 3060 else if (name.equals("definition")) { 3061 return addDefinition(); 3062 } 3063 else if (name.equals("basedOn")) { 3064 return addBasedOn(); 3065 } 3066 else if (name.equals("groupIdentifier")) { 3067 this.groupIdentifier = new Identifier(); 3068 return this.groupIdentifier; 3069 } 3070 else if (name.equals("status")) { 3071 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.status"); 3072 } 3073 else if (name.equals("intent")) { 3074 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.intent"); 3075 } 3076 else if (name.equals("category")) { 3077 this.category = new CodeableConcept(); 3078 return this.category; 3079 } 3080 else if (name.equals("priority")) { 3081 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.priority"); 3082 } 3083 else if (name.equals("medicationCodeableConcept")) { 3084 this.medication = new CodeableConcept(); 3085 return this.medication; 3086 } 3087 else if (name.equals("medicationReference")) { 3088 this.medication = new Reference(); 3089 return this.medication; 3090 } 3091 else if (name.equals("subject")) { 3092 this.subject = new Reference(); 3093 return this.subject; 3094 } 3095 else if (name.equals("context")) { 3096 this.context = new Reference(); 3097 return this.context; 3098 } 3099 else if (name.equals("supportingInformation")) { 3100 return addSupportingInformation(); 3101 } 3102 else if (name.equals("authoredOn")) { 3103 throw new FHIRException("Cannot call addChild on a singleton property MedicationRequest.authoredOn"); 3104 } 3105 else if (name.equals("requester")) { 3106 this.requester = new MedicationRequestRequesterComponent(); 3107 return this.requester; 3108 } 3109 else if (name.equals("recorder")) { 3110 this.recorder = new Reference(); 3111 return this.recorder; 3112 } 3113 else if (name.equals("reasonCode")) { 3114 return addReasonCode(); 3115 } 3116 else if (name.equals("reasonReference")) { 3117 return addReasonReference(); 3118 } 3119 else if (name.equals("note")) { 3120 return addNote(); 3121 } 3122 else if (name.equals("dosageInstruction")) { 3123 return addDosageInstruction(); 3124 } 3125 else if (name.equals("dispenseRequest")) { 3126 this.dispenseRequest = new MedicationRequestDispenseRequestComponent(); 3127 return this.dispenseRequest; 3128 } 3129 else if (name.equals("substitution")) { 3130 this.substitution = new MedicationRequestSubstitutionComponent(); 3131 return this.substitution; 3132 } 3133 else if (name.equals("priorPrescription")) { 3134 this.priorPrescription = new Reference(); 3135 return this.priorPrescription; 3136 } 3137 else if (name.equals("detectedIssue")) { 3138 return addDetectedIssue(); 3139 } 3140 else if (name.equals("eventHistory")) { 3141 return addEventHistory(); 3142 } 3143 else 3144 return super.addChild(name); 3145 } 3146 3147 public String fhirType() { 3148 return "MedicationRequest"; 3149 3150 } 3151 3152 public MedicationRequest copy() { 3153 MedicationRequest dst = new MedicationRequest(); 3154 copyValues(dst); 3155 if (identifier != null) { 3156 dst.identifier = new ArrayList<Identifier>(); 3157 for (Identifier i : identifier) 3158 dst.identifier.add(i.copy()); 3159 }; 3160 if (definition != null) { 3161 dst.definition = new ArrayList<Reference>(); 3162 for (Reference i : definition) 3163 dst.definition.add(i.copy()); 3164 }; 3165 if (basedOn != null) { 3166 dst.basedOn = new ArrayList<Reference>(); 3167 for (Reference i : basedOn) 3168 dst.basedOn.add(i.copy()); 3169 }; 3170 dst.groupIdentifier = groupIdentifier == null ? null : groupIdentifier.copy(); 3171 dst.status = status == null ? null : status.copy(); 3172 dst.intent = intent == null ? null : intent.copy(); 3173 dst.category = category == null ? null : category.copy(); 3174 dst.priority = priority == null ? null : priority.copy(); 3175 dst.medication = medication == null ? null : medication.copy(); 3176 dst.subject = subject == null ? null : subject.copy(); 3177 dst.context = context == null ? null : context.copy(); 3178 if (supportingInformation != null) { 3179 dst.supportingInformation = new ArrayList<Reference>(); 3180 for (Reference i : supportingInformation) 3181 dst.supportingInformation.add(i.copy()); 3182 }; 3183 dst.authoredOn = authoredOn == null ? null : authoredOn.copy(); 3184 dst.requester = requester == null ? null : requester.copy(); 3185 dst.recorder = recorder == null ? null : recorder.copy(); 3186 if (reasonCode != null) { 3187 dst.reasonCode = new ArrayList<CodeableConcept>(); 3188 for (CodeableConcept i : reasonCode) 3189 dst.reasonCode.add(i.copy()); 3190 }; 3191 if (reasonReference != null) { 3192 dst.reasonReference = new ArrayList<Reference>(); 3193 for (Reference i : reasonReference) 3194 dst.reasonReference.add(i.copy()); 3195 }; 3196 if (note != null) { 3197 dst.note = new ArrayList<Annotation>(); 3198 for (Annotation i : note) 3199 dst.note.add(i.copy()); 3200 }; 3201 if (dosageInstruction != null) { 3202 dst.dosageInstruction = new ArrayList<Dosage>(); 3203 for (Dosage i : dosageInstruction) 3204 dst.dosageInstruction.add(i.copy()); 3205 }; 3206 dst.dispenseRequest = dispenseRequest == null ? null : dispenseRequest.copy(); 3207 dst.substitution = substitution == null ? null : substitution.copy(); 3208 dst.priorPrescription = priorPrescription == null ? null : priorPrescription.copy(); 3209 if (detectedIssue != null) { 3210 dst.detectedIssue = new ArrayList<Reference>(); 3211 for (Reference i : detectedIssue) 3212 dst.detectedIssue.add(i.copy()); 3213 }; 3214 if (eventHistory != null) { 3215 dst.eventHistory = new ArrayList<Reference>(); 3216 for (Reference i : eventHistory) 3217 dst.eventHistory.add(i.copy()); 3218 }; 3219 return dst; 3220 } 3221 3222 protected MedicationRequest typedCopy() { 3223 return copy(); 3224 } 3225 3226 @Override 3227 public boolean equalsDeep(Base other_) { 3228 if (!super.equalsDeep(other_)) 3229 return false; 3230 if (!(other_ instanceof MedicationRequest)) 3231 return false; 3232 MedicationRequest o = (MedicationRequest) other_; 3233 return compareDeep(identifier, o.identifier, true) && compareDeep(definition, o.definition, true) 3234 && compareDeep(basedOn, o.basedOn, true) && compareDeep(groupIdentifier, o.groupIdentifier, true) 3235 && compareDeep(status, o.status, true) && compareDeep(intent, o.intent, true) && compareDeep(category, o.category, true) 3236 && compareDeep(priority, o.priority, true) && compareDeep(medication, o.medication, true) && compareDeep(subject, o.subject, true) 3237 && compareDeep(context, o.context, true) && compareDeep(supportingInformation, o.supportingInformation, true) 3238 && compareDeep(authoredOn, o.authoredOn, true) && compareDeep(requester, o.requester, true) && compareDeep(recorder, o.recorder, true) 3239 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 3240 && compareDeep(note, o.note, true) && compareDeep(dosageInstruction, o.dosageInstruction, true) 3241 && compareDeep(dispenseRequest, o.dispenseRequest, true) && compareDeep(substitution, o.substitution, true) 3242 && compareDeep(priorPrescription, o.priorPrescription, true) && compareDeep(detectedIssue, o.detectedIssue, true) 3243 && compareDeep(eventHistory, o.eventHistory, true); 3244 } 3245 3246 @Override 3247 public boolean equalsShallow(Base other_) { 3248 if (!super.equalsShallow(other_)) 3249 return false; 3250 if (!(other_ instanceof MedicationRequest)) 3251 return false; 3252 MedicationRequest o = (MedicationRequest) other_; 3253 return compareValues(status, o.status, true) && compareValues(intent, o.intent, true) && compareValues(priority, o.priority, true) 3254 && compareValues(authoredOn, o.authoredOn, true); 3255 } 3256 3257 public boolean isEmpty() { 3258 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, definition, basedOn 3259 , groupIdentifier, status, intent, category, priority, medication, subject, context 3260 , supportingInformation, authoredOn, requester, recorder, reasonCode, reasonReference 3261 , note, dosageInstruction, dispenseRequest, substitution, priorPrescription, detectedIssue 3262 , eventHistory); 3263 } 3264 3265 @Override 3266 public ResourceType getResourceType() { 3267 return ResourceType.MedicationRequest; 3268 } 3269 3270 /** 3271 * Search parameter: <b>requester</b> 3272 * <p> 3273 * Description: <b>Returns prescriptions prescribed by this prescriber</b><br> 3274 * Type: <b>reference</b><br> 3275 * Path: <b>MedicationRequest.requester.agent</b><br> 3276 * </p> 3277 */ 3278 @SearchParamDefinition(name="requester", path="MedicationRequest.requester.agent", description="Returns prescriptions prescribed by this prescriber", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner") }, target={Device.class, Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 3279 public static final String SP_REQUESTER = "requester"; 3280 /** 3281 * <b>Fluent Client</b> search parameter constant for <b>requester</b> 3282 * <p> 3283 * Description: <b>Returns prescriptions prescribed by this prescriber</b><br> 3284 * Type: <b>reference</b><br> 3285 * Path: <b>MedicationRequest.requester.agent</b><br> 3286 * </p> 3287 */ 3288 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam REQUESTER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_REQUESTER); 3289 3290/** 3291 * Constant for fluent queries to be used to add include statements. Specifies 3292 * the path value of "<b>MedicationRequest:requester</b>". 3293 */ 3294 public static final ca.uhn.fhir.model.api.Include INCLUDE_REQUESTER = new ca.uhn.fhir.model.api.Include("MedicationRequest:requester").toLocked(); 3295 3296 /** 3297 * Search parameter: <b>date</b> 3298 * <p> 3299 * Description: <b>Returns medication request to be administered on a specific date</b><br> 3300 * Type: <b>date</b><br> 3301 * Path: <b>MedicationRequest.dosageInstruction.timing.event</b><br> 3302 * </p> 3303 */ 3304 @SearchParamDefinition(name="date", path="MedicationRequest.dosageInstruction.timing.event", description="Returns medication request to be administered on a specific date", type="date" ) 3305 public static final String SP_DATE = "date"; 3306 /** 3307 * <b>Fluent Client</b> search parameter constant for <b>date</b> 3308 * <p> 3309 * Description: <b>Returns medication request to be administered on a specific date</b><br> 3310 * Type: <b>date</b><br> 3311 * Path: <b>MedicationRequest.dosageInstruction.timing.event</b><br> 3312 * </p> 3313 */ 3314 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 3315 3316 /** 3317 * Search parameter: <b>identifier</b> 3318 * <p> 3319 * Description: <b>Return prescriptions with this external identifier</b><br> 3320 * Type: <b>token</b><br> 3321 * Path: <b>MedicationRequest.identifier</b><br> 3322 * </p> 3323 */ 3324 @SearchParamDefinition(name="identifier", path="MedicationRequest.identifier", description="Return prescriptions with this external identifier", type="token" ) 3325 public static final String SP_IDENTIFIER = "identifier"; 3326 /** 3327 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 3328 * <p> 3329 * Description: <b>Return prescriptions with this external identifier</b><br> 3330 * Type: <b>token</b><br> 3331 * Path: <b>MedicationRequest.identifier</b><br> 3332 * </p> 3333 */ 3334 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 3335 3336 /** 3337 * Search parameter: <b>intended-dispenser</b> 3338 * <p> 3339 * Description: <b>Returns prescriptions intended to be dispensed by this Organization</b><br> 3340 * Type: <b>reference</b><br> 3341 * Path: <b>MedicationRequest.dispenseRequest.performer</b><br> 3342 * </p> 3343 */ 3344 @SearchParamDefinition(name="intended-dispenser", path="MedicationRequest.dispenseRequest.performer", description="Returns prescriptions intended to be dispensed by this Organization", type="reference", target={Organization.class } ) 3345 public static final String SP_INTENDED_DISPENSER = "intended-dispenser"; 3346 /** 3347 * <b>Fluent Client</b> search parameter constant for <b>intended-dispenser</b> 3348 * <p> 3349 * Description: <b>Returns prescriptions intended to be dispensed by this Organization</b><br> 3350 * Type: <b>reference</b><br> 3351 * Path: <b>MedicationRequest.dispenseRequest.performer</b><br> 3352 * </p> 3353 */ 3354 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam INTENDED_DISPENSER = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_INTENDED_DISPENSER); 3355 3356/** 3357 * Constant for fluent queries to be used to add include statements. Specifies 3358 * the path value of "<b>MedicationRequest:intended-dispenser</b>". 3359 */ 3360 public static final ca.uhn.fhir.model.api.Include INCLUDE_INTENDED_DISPENSER = new ca.uhn.fhir.model.api.Include("MedicationRequest:intended-dispenser").toLocked(); 3361 3362 /** 3363 * Search parameter: <b>authoredon</b> 3364 * <p> 3365 * Description: <b>Return prescriptions written on this date</b><br> 3366 * Type: <b>date</b><br> 3367 * Path: <b>MedicationRequest.authoredOn</b><br> 3368 * </p> 3369 */ 3370 @SearchParamDefinition(name="authoredon", path="MedicationRequest.authoredOn", description="Return prescriptions written on this date", type="date" ) 3371 public static final String SP_AUTHOREDON = "authoredon"; 3372 /** 3373 * <b>Fluent Client</b> search parameter constant for <b>authoredon</b> 3374 * <p> 3375 * Description: <b>Return prescriptions written on this date</b><br> 3376 * Type: <b>date</b><br> 3377 * Path: <b>MedicationRequest.authoredOn</b><br> 3378 * </p> 3379 */ 3380 public static final ca.uhn.fhir.rest.gclient.DateClientParam AUTHOREDON = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_AUTHOREDON); 3381 3382 /** 3383 * Search parameter: <b>code</b> 3384 * <p> 3385 * Description: <b>Return prescriptions of this medication code</b><br> 3386 * Type: <b>token</b><br> 3387 * Path: <b>MedicationRequest.medicationCodeableConcept</b><br> 3388 * </p> 3389 */ 3390 @SearchParamDefinition(name="code", path="MedicationRequest.medication.as(CodeableConcept)", description="Return prescriptions of this medication code", type="token" ) 3391 public static final String SP_CODE = "code"; 3392 /** 3393 * <b>Fluent Client</b> search parameter constant for <b>code</b> 3394 * <p> 3395 * Description: <b>Return prescriptions of this medication code</b><br> 3396 * Type: <b>token</b><br> 3397 * Path: <b>MedicationRequest.medicationCodeableConcept</b><br> 3398 * </p> 3399 */ 3400 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 3401 3402 /** 3403 * Search parameter: <b>subject</b> 3404 * <p> 3405 * Description: <b>The identity of a patient to list orders for</b><br> 3406 * Type: <b>reference</b><br> 3407 * Path: <b>MedicationRequest.subject</b><br> 3408 * </p> 3409 */ 3410 @SearchParamDefinition(name="subject", path="MedicationRequest.subject", description="The identity of a patient to list orders for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 3411 public static final String SP_SUBJECT = "subject"; 3412 /** 3413 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 3414 * <p> 3415 * Description: <b>The identity of a patient to list orders for</b><br> 3416 * Type: <b>reference</b><br> 3417 * Path: <b>MedicationRequest.subject</b><br> 3418 * </p> 3419 */ 3420 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 3421 3422/** 3423 * Constant for fluent queries to be used to add include statements. Specifies 3424 * the path value of "<b>MedicationRequest:subject</b>". 3425 */ 3426 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationRequest:subject").toLocked(); 3427 3428 /** 3429 * Search parameter: <b>medication</b> 3430 * <p> 3431 * Description: <b>Return prescriptions of this medication reference</b><br> 3432 * Type: <b>reference</b><br> 3433 * Path: <b>MedicationRequest.medicationReference</b><br> 3434 * </p> 3435 */ 3436 @SearchParamDefinition(name="medication", path="MedicationRequest.medication.as(Reference)", description="Return prescriptions of this medication reference", type="reference", target={Medication.class } ) 3437 public static final String SP_MEDICATION = "medication"; 3438 /** 3439 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 3440 * <p> 3441 * Description: <b>Return prescriptions of this medication reference</b><br> 3442 * Type: <b>reference</b><br> 3443 * Path: <b>MedicationRequest.medicationReference</b><br> 3444 * </p> 3445 */ 3446 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 3447 3448/** 3449 * Constant for fluent queries to be used to add include statements. Specifies 3450 * the path value of "<b>MedicationRequest:medication</b>". 3451 */ 3452 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationRequest:medication").toLocked(); 3453 3454 /** 3455 * Search parameter: <b>priority</b> 3456 * <p> 3457 * Description: <b>Returns prescriptions with different priorities</b><br> 3458 * Type: <b>token</b><br> 3459 * Path: <b>MedicationRequest.priority</b><br> 3460 * </p> 3461 */ 3462 @SearchParamDefinition(name="priority", path="MedicationRequest.priority", description="Returns prescriptions with different priorities", type="token" ) 3463 public static final String SP_PRIORITY = "priority"; 3464 /** 3465 * <b>Fluent Client</b> search parameter constant for <b>priority</b> 3466 * <p> 3467 * Description: <b>Returns prescriptions with different priorities</b><br> 3468 * Type: <b>token</b><br> 3469 * Path: <b>MedicationRequest.priority</b><br> 3470 * </p> 3471 */ 3472 public static final ca.uhn.fhir.rest.gclient.TokenClientParam PRIORITY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_PRIORITY); 3473 3474 /** 3475 * Search parameter: <b>intent</b> 3476 * <p> 3477 * Description: <b>Returns prescriptions with different intents</b><br> 3478 * Type: <b>token</b><br> 3479 * Path: <b>MedicationRequest.intent</b><br> 3480 * </p> 3481 */ 3482 @SearchParamDefinition(name="intent", path="MedicationRequest.intent", description="Returns prescriptions with different intents", type="token" ) 3483 public static final String SP_INTENT = "intent"; 3484 /** 3485 * <b>Fluent Client</b> search parameter constant for <b>intent</b> 3486 * <p> 3487 * Description: <b>Returns prescriptions with different intents</b><br> 3488 * Type: <b>token</b><br> 3489 * Path: <b>MedicationRequest.intent</b><br> 3490 * </p> 3491 */ 3492 public static final ca.uhn.fhir.rest.gclient.TokenClientParam INTENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_INTENT); 3493 3494 /** 3495 * Search parameter: <b>patient</b> 3496 * <p> 3497 * Description: <b>Returns prescriptions for a specific patient</b><br> 3498 * Type: <b>reference</b><br> 3499 * Path: <b>MedicationRequest.subject</b><br> 3500 * </p> 3501 */ 3502 @SearchParamDefinition(name="patient", path="MedicationRequest.subject", description="Returns prescriptions for a specific patient", type="reference", target={Patient.class } ) 3503 public static final String SP_PATIENT = "patient"; 3504 /** 3505 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 3506 * <p> 3507 * Description: <b>Returns prescriptions for a specific patient</b><br> 3508 * Type: <b>reference</b><br> 3509 * Path: <b>MedicationRequest.subject</b><br> 3510 * </p> 3511 */ 3512 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 3513 3514/** 3515 * Constant for fluent queries to be used to add include statements. Specifies 3516 * the path value of "<b>MedicationRequest:patient</b>". 3517 */ 3518 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationRequest:patient").toLocked(); 3519 3520 /** 3521 * Search parameter: <b>context</b> 3522 * <p> 3523 * Description: <b>Return prescriptions with this encounter or episode of care identifier</b><br> 3524 * Type: <b>reference</b><br> 3525 * Path: <b>MedicationRequest.context</b><br> 3526 * </p> 3527 */ 3528 @SearchParamDefinition(name="context", path="MedicationRequest.context", description="Return prescriptions with this encounter or episode of care identifier", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Encounter") }, target={Encounter.class, EpisodeOfCare.class } ) 3529 public static final String SP_CONTEXT = "context"; 3530 /** 3531 * <b>Fluent Client</b> search parameter constant for <b>context</b> 3532 * <p> 3533 * Description: <b>Return prescriptions with this encounter or episode of care identifier</b><br> 3534 * Type: <b>reference</b><br> 3535 * Path: <b>MedicationRequest.context</b><br> 3536 * </p> 3537 */ 3538 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 3539 3540/** 3541 * Constant for fluent queries to be used to add include statements. Specifies 3542 * the path value of "<b>MedicationRequest:context</b>". 3543 */ 3544 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("MedicationRequest:context").toLocked(); 3545 3546 /** 3547 * Search parameter: <b>category</b> 3548 * <p> 3549 * Description: <b>Returns prescriptions with different categories</b><br> 3550 * Type: <b>token</b><br> 3551 * Path: <b>MedicationRequest.category</b><br> 3552 * </p> 3553 */ 3554 @SearchParamDefinition(name="category", path="MedicationRequest.category", description="Returns prescriptions with different categories", type="token" ) 3555 public static final String SP_CATEGORY = "category"; 3556 /** 3557 * <b>Fluent Client</b> search parameter constant for <b>category</b> 3558 * <p> 3559 * Description: <b>Returns prescriptions with different categories</b><br> 3560 * Type: <b>token</b><br> 3561 * Path: <b>MedicationRequest.category</b><br> 3562 * </p> 3563 */ 3564 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 3565 3566 /** 3567 * Search parameter: <b>status</b> 3568 * <p> 3569 * Description: <b>Status of the prescription</b><br> 3570 * Type: <b>token</b><br> 3571 * Path: <b>MedicationRequest.status</b><br> 3572 * </p> 3573 */ 3574 @SearchParamDefinition(name="status", path="MedicationRequest.status", description="Status of the prescription", type="token" ) 3575 public static final String SP_STATUS = "status"; 3576 /** 3577 * <b>Fluent Client</b> search parameter constant for <b>status</b> 3578 * <p> 3579 * Description: <b>Status of the prescription</b><br> 3580 * Type: <b>token</b><br> 3581 * Path: <b>MedicationRequest.status</b><br> 3582 * </p> 3583 */ 3584 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 3585 3586 3587}