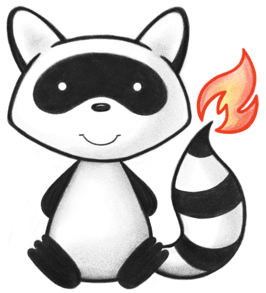
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 048 049The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 050 */ 051@ResourceDef(name="MedicationStatement", profile="http://hl7.org/fhir/Profile/MedicationStatement") 052public class MedicationStatement extends DomainResource { 053 054 public enum MedicationStatementStatus { 055 /** 056 * The medication is still being taken. 057 */ 058 ACTIVE, 059 /** 060 * The medication is no longer being taken. 061 */ 062 COMPLETED, 063 /** 064 * The statement was recorded incorrectly. 065 */ 066 ENTEREDINERROR, 067 /** 068 * The medication may be taken at some time in the future. 069 */ 070 INTENDED, 071 /** 072 * Actions implied by the statement have been permanently halted, before all of them occurred. 073 */ 074 STOPPED, 075 /** 076 * Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called "suspended". 077 */ 078 ONHOLD, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 public static MedicationStatementStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("active".equals(codeString)) 087 return ACTIVE; 088 if ("completed".equals(codeString)) 089 return COMPLETED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("intended".equals(codeString)) 093 return INTENDED; 094 if ("stopped".equals(codeString)) 095 return STOPPED; 096 if ("on-hold".equals(codeString)) 097 return ONHOLD; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown MedicationStatementStatus code '"+codeString+"'"); 102 } 103 public String toCode() { 104 switch (this) { 105 case ACTIVE: return "active"; 106 case COMPLETED: return "completed"; 107 case ENTEREDINERROR: return "entered-in-error"; 108 case INTENDED: return "intended"; 109 case STOPPED: return "stopped"; 110 case ONHOLD: return "on-hold"; 111 case NULL: return null; 112 default: return "?"; 113 } 114 } 115 public String getSystem() { 116 switch (this) { 117 case ACTIVE: return "http://hl7.org/fhir/medication-statement-status"; 118 case COMPLETED: return "http://hl7.org/fhir/medication-statement-status"; 119 case ENTEREDINERROR: return "http://hl7.org/fhir/medication-statement-status"; 120 case INTENDED: return "http://hl7.org/fhir/medication-statement-status"; 121 case STOPPED: return "http://hl7.org/fhir/medication-statement-status"; 122 case ONHOLD: return "http://hl7.org/fhir/medication-statement-status"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 public String getDefinition() { 128 switch (this) { 129 case ACTIVE: return "The medication is still being taken."; 130 case COMPLETED: return "The medication is no longer being taken."; 131 case ENTEREDINERROR: return "The statement was recorded incorrectly."; 132 case INTENDED: return "The medication may be taken at some time in the future."; 133 case STOPPED: return "Actions implied by the statement have been permanently halted, before all of them occurred."; 134 case ONHOLD: return "Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 135 case NULL: return null; 136 default: return "?"; 137 } 138 } 139 public String getDisplay() { 140 switch (this) { 141 case ACTIVE: return "Active"; 142 case COMPLETED: return "Completed"; 143 case ENTEREDINERROR: return "Entered in Error"; 144 case INTENDED: return "Intended"; 145 case STOPPED: return "Stopped"; 146 case ONHOLD: return "On Hold"; 147 case NULL: return null; 148 default: return "?"; 149 } 150 } 151 } 152 153 public static class MedicationStatementStatusEnumFactory implements EnumFactory<MedicationStatementStatus> { 154 public MedicationStatementStatus fromCode(String codeString) throws IllegalArgumentException { 155 if (codeString == null || "".equals(codeString)) 156 if (codeString == null || "".equals(codeString)) 157 return null; 158 if ("active".equals(codeString)) 159 return MedicationStatementStatus.ACTIVE; 160 if ("completed".equals(codeString)) 161 return MedicationStatementStatus.COMPLETED; 162 if ("entered-in-error".equals(codeString)) 163 return MedicationStatementStatus.ENTEREDINERROR; 164 if ("intended".equals(codeString)) 165 return MedicationStatementStatus.INTENDED; 166 if ("stopped".equals(codeString)) 167 return MedicationStatementStatus.STOPPED; 168 if ("on-hold".equals(codeString)) 169 return MedicationStatementStatus.ONHOLD; 170 throw new IllegalArgumentException("Unknown MedicationStatementStatus code '"+codeString+"'"); 171 } 172 public Enumeration<MedicationStatementStatus> fromType(PrimitiveType<?> code) throws FHIRException { 173 if (code == null) 174 return null; 175 if (code.isEmpty()) 176 return new Enumeration<MedicationStatementStatus>(this); 177 String codeString = code.asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("active".equals(codeString)) 181 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ACTIVE); 182 if ("completed".equals(codeString)) 183 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.COMPLETED); 184 if ("entered-in-error".equals(codeString)) 185 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ENTEREDINERROR); 186 if ("intended".equals(codeString)) 187 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.INTENDED); 188 if ("stopped".equals(codeString)) 189 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.STOPPED); 190 if ("on-hold".equals(codeString)) 191 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ONHOLD); 192 throw new FHIRException("Unknown MedicationStatementStatus code '"+codeString+"'"); 193 } 194 public String toCode(MedicationStatementStatus code) { 195 if (code == MedicationStatementStatus.NULL) 196 return null; 197 if (code == MedicationStatementStatus.ACTIVE) 198 return "active"; 199 if (code == MedicationStatementStatus.COMPLETED) 200 return "completed"; 201 if (code == MedicationStatementStatus.ENTEREDINERROR) 202 return "entered-in-error"; 203 if (code == MedicationStatementStatus.INTENDED) 204 return "intended"; 205 if (code == MedicationStatementStatus.STOPPED) 206 return "stopped"; 207 if (code == MedicationStatementStatus.ONHOLD) 208 return "on-hold"; 209 return "?"; 210 } 211 public String toSystem(MedicationStatementStatus code) { 212 return code.getSystem(); 213 } 214 } 215 216 public enum MedicationStatementTaken { 217 /** 218 * Positive assertion that patient has taken medication 219 */ 220 Y, 221 /** 222 * Negative assertion that patient has not taken medication 223 */ 224 N, 225 /** 226 * Unknown assertion if patient has taken medication 227 */ 228 UNK, 229 /** 230 * Patient reporting does not apply 231 */ 232 NA, 233 /** 234 * added to help the parsers with the generic types 235 */ 236 NULL; 237 public static MedicationStatementTaken fromCode(String codeString) throws FHIRException { 238 if (codeString == null || "".equals(codeString)) 239 return null; 240 if ("y".equals(codeString)) 241 return Y; 242 if ("n".equals(codeString)) 243 return N; 244 if ("unk".equals(codeString)) 245 return UNK; 246 if ("na".equals(codeString)) 247 return NA; 248 if (Configuration.isAcceptInvalidEnums()) 249 return null; 250 else 251 throw new FHIRException("Unknown MedicationStatementTaken code '"+codeString+"'"); 252 } 253 public String toCode() { 254 switch (this) { 255 case Y: return "y"; 256 case N: return "n"; 257 case UNK: return "unk"; 258 case NA: return "na"; 259 case NULL: return null; 260 default: return "?"; 261 } 262 } 263 public String getSystem() { 264 switch (this) { 265 case Y: return "http://hl7.org/fhir/medication-statement-taken"; 266 case N: return "http://hl7.org/fhir/medication-statement-taken"; 267 case UNK: return "http://hl7.org/fhir/medication-statement-taken"; 268 case NA: return "http://hl7.org/fhir/medication-statement-taken"; 269 case NULL: return null; 270 default: return "?"; 271 } 272 } 273 public String getDefinition() { 274 switch (this) { 275 case Y: return "Positive assertion that patient has taken medication"; 276 case N: return "Negative assertion that patient has not taken medication"; 277 case UNK: return "Unknown assertion if patient has taken medication"; 278 case NA: return "Patient reporting does not apply"; 279 case NULL: return null; 280 default: return "?"; 281 } 282 } 283 public String getDisplay() { 284 switch (this) { 285 case Y: return "Yes"; 286 case N: return "No"; 287 case UNK: return "Unknown"; 288 case NA: return "Not Applicable"; 289 case NULL: return null; 290 default: return "?"; 291 } 292 } 293 } 294 295 public static class MedicationStatementTakenEnumFactory implements EnumFactory<MedicationStatementTaken> { 296 public MedicationStatementTaken fromCode(String codeString) throws IllegalArgumentException { 297 if (codeString == null || "".equals(codeString)) 298 if (codeString == null || "".equals(codeString)) 299 return null; 300 if ("y".equals(codeString)) 301 return MedicationStatementTaken.Y; 302 if ("n".equals(codeString)) 303 return MedicationStatementTaken.N; 304 if ("unk".equals(codeString)) 305 return MedicationStatementTaken.UNK; 306 if ("na".equals(codeString)) 307 return MedicationStatementTaken.NA; 308 throw new IllegalArgumentException("Unknown MedicationStatementTaken code '"+codeString+"'"); 309 } 310 public Enumeration<MedicationStatementTaken> fromType(PrimitiveType<?> code) throws FHIRException { 311 if (code == null) 312 return null; 313 if (code.isEmpty()) 314 return new Enumeration<MedicationStatementTaken>(this); 315 String codeString = code.asStringValue(); 316 if (codeString == null || "".equals(codeString)) 317 return null; 318 if ("y".equals(codeString)) 319 return new Enumeration<MedicationStatementTaken>(this, MedicationStatementTaken.Y); 320 if ("n".equals(codeString)) 321 return new Enumeration<MedicationStatementTaken>(this, MedicationStatementTaken.N); 322 if ("unk".equals(codeString)) 323 return new Enumeration<MedicationStatementTaken>(this, MedicationStatementTaken.UNK); 324 if ("na".equals(codeString)) 325 return new Enumeration<MedicationStatementTaken>(this, MedicationStatementTaken.NA); 326 throw new FHIRException("Unknown MedicationStatementTaken code '"+codeString+"'"); 327 } 328 public String toCode(MedicationStatementTaken code) { 329 if (code == MedicationStatementTaken.NULL) 330 return null; 331 if (code == MedicationStatementTaken.Y) 332 return "y"; 333 if (code == MedicationStatementTaken.N) 334 return "n"; 335 if (code == MedicationStatementTaken.UNK) 336 return "unk"; 337 if (code == MedicationStatementTaken.NA) 338 return "na"; 339 return "?"; 340 } 341 public String toSystem(MedicationStatementTaken code) { 342 return code.getSystem(); 343 } 344 } 345 346 /** 347 * External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated. 348 */ 349 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 350 @Description(shortDefinition="External identifier", formalDefinition="External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated." ) 351 protected List<Identifier> identifier; 352 353 /** 354 * A plan, proposal or order that is fulfilled in whole or in part by this event. 355 */ 356 @Child(name = "basedOn", type = {MedicationRequest.class, CarePlan.class, ProcedureRequest.class, ReferralRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 357 @Description(shortDefinition="Fulfils plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event." ) 358 protected List<Reference> basedOn; 359 /** 360 * The actual objects that are the target of the reference (A plan, proposal or order that is fulfilled in whole or in part by this event.) 361 */ 362 protected List<Resource> basedOnTarget; 363 364 365 /** 366 * A larger event of which this particular event is a component or step. 367 */ 368 @Child(name = "partOf", type = {MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Procedure.class, Observation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 369 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 370 protected List<Reference> partOf; 371 /** 372 * The actual objects that are the target of the reference (A larger event of which this particular event is a component or step.) 373 */ 374 protected List<Resource> partOfTarget; 375 376 377 /** 378 * The encounter or episode of care that establishes the context for this MedicationStatement. 379 */ 380 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=3, min=0, max=1, modifier=false, summary=true) 381 @Description(shortDefinition="Encounter / Episode associated with MedicationStatement", formalDefinition="The encounter or episode of care that establishes the context for this MedicationStatement." ) 382 protected Reference context; 383 384 /** 385 * The actual object that is the target of the reference (The encounter or episode of care that establishes the context for this MedicationStatement.) 386 */ 387 protected Resource contextTarget; 388 389 /** 390 * A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed. 391 */ 392 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 393 @Description(shortDefinition="active | completed | entered-in-error | intended | stopped | on-hold", formalDefinition="A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed." ) 394 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-status") 395 protected Enumeration<MedicationStatementStatus> status; 396 397 /** 398 * Indicates where type of medication statement and where the medication is expected to be consumed or administered. 399 */ 400 @Child(name = "category", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 401 @Description(shortDefinition="Type of medication usage", formalDefinition="Indicates where type of medication statement and where the medication is expected to be consumed or administered." ) 402 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-category") 403 protected CodeableConcept category; 404 405 /** 406 * Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications. 407 */ 408 @Child(name = "medication", type = {CodeableConcept.class, Medication.class}, order=6, min=1, max=1, modifier=false, summary=true) 409 @Description(shortDefinition="What medication was taken", formalDefinition="Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications." ) 410 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 411 protected Type medication; 412 413 /** 414 * The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true). 415 */ 416 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 417 @Description(shortDefinition="The date/time or interval when the medication was taken", formalDefinition="The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true)." ) 418 protected Type effective; 419 420 /** 421 * The date when the medication statement was asserted by the information source. 422 */ 423 @Child(name = "dateAsserted", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 424 @Description(shortDefinition="When the statement was asserted?", formalDefinition="The date when the medication statement was asserted by the information source." ) 425 protected DateTimeType dateAsserted; 426 427 /** 428 * The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest. 429 */ 430 @Child(name = "informationSource", type = {Patient.class, Practitioner.class, RelatedPerson.class, Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 431 @Description(shortDefinition="Person or organization that provided the information about the taking of this medication", formalDefinition="The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest." ) 432 protected Reference informationSource; 433 434 /** 435 * The actual object that is the target of the reference (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 436 */ 437 protected Resource informationSourceTarget; 438 439 /** 440 * The person, animal or group who is/was taking the medication. 441 */ 442 @Child(name = "subject", type = {Patient.class, Group.class}, order=10, min=1, max=1, modifier=false, summary=true) 443 @Description(shortDefinition="Who is/was taking the medication", formalDefinition="The person, animal or group who is/was taking the medication." ) 444 protected Reference subject; 445 446 /** 447 * The actual object that is the target of the reference (The person, animal or group who is/was taking the medication.) 448 */ 449 protected Resource subjectTarget; 450 451 /** 452 * Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement. 453 */ 454 @Child(name = "derivedFrom", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 455 @Description(shortDefinition="Additional supporting information", formalDefinition="Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement." ) 456 protected List<Reference> derivedFrom; 457 /** 458 * The actual objects that are the target of the reference (Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.) 459 */ 460 protected List<Resource> derivedFromTarget; 461 462 463 /** 464 * Indicator of the certainty of whether the medication was taken by the patient. 465 */ 466 @Child(name = "taken", type = {CodeType.class}, order=12, min=1, max=1, modifier=true, summary=true) 467 @Description(shortDefinition="y | n | unk | na", formalDefinition="Indicator of the certainty of whether the medication was taken by the patient." ) 468 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-taken") 469 protected Enumeration<MedicationStatementTaken> taken; 470 471 /** 472 * A code indicating why the medication was not taken. 473 */ 474 @Child(name = "reasonNotTaken", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 475 @Description(shortDefinition="True if asserting medication was not given", formalDefinition="A code indicating why the medication was not taken." ) 476 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reason-medication-not-taken-codes") 477 protected List<CodeableConcept> reasonNotTaken; 478 479 /** 480 * A reason for why the medication is being/was taken. 481 */ 482 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 483 @Description(shortDefinition="Reason for why the medication is being/was taken", formalDefinition="A reason for why the medication is being/was taken." ) 484 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 485 protected List<CodeableConcept> reasonCode; 486 487 /** 488 * Condition or observation that supports why the medication is being/was taken. 489 */ 490 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 491 @Description(shortDefinition="Condition or observation that supports why the medication is being/was taken", formalDefinition="Condition or observation that supports why the medication is being/was taken." ) 492 protected List<Reference> reasonReference; 493 /** 494 * The actual objects that are the target of the reference (Condition or observation that supports why the medication is being/was taken.) 495 */ 496 protected List<Resource> reasonReferenceTarget; 497 498 499 /** 500 * Provides extra information about the medication statement that is not conveyed by the other attributes. 501 */ 502 @Child(name = "note", type = {Annotation.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 503 @Description(shortDefinition="Further information about the statement", formalDefinition="Provides extra information about the medication statement that is not conveyed by the other attributes." ) 504 protected List<Annotation> note; 505 506 /** 507 * Indicates how the medication is/was or should be taken by the patient. 508 */ 509 @Child(name = "dosage", type = {Dosage.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 510 @Description(shortDefinition="Details of how medication is/was taken or should be taken", formalDefinition="Indicates how the medication is/was or should be taken by the patient." ) 511 protected List<Dosage> dosage; 512 513 private static final long serialVersionUID = -1529480075L; 514 515 /** 516 * Constructor 517 */ 518 public MedicationStatement() { 519 super(); 520 } 521 522 /** 523 * Constructor 524 */ 525 public MedicationStatement(Enumeration<MedicationStatementStatus> status, Type medication, Reference subject, Enumeration<MedicationStatementTaken> taken) { 526 super(); 527 this.status = status; 528 this.medication = medication; 529 this.subject = subject; 530 this.taken = taken; 531 } 532 533 /** 534 * @return {@link #identifier} (External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.) 535 */ 536 public List<Identifier> getIdentifier() { 537 if (this.identifier == null) 538 this.identifier = new ArrayList<Identifier>(); 539 return this.identifier; 540 } 541 542 /** 543 * @return Returns a reference to <code>this</code> for easy method chaining 544 */ 545 public MedicationStatement setIdentifier(List<Identifier> theIdentifier) { 546 this.identifier = theIdentifier; 547 return this; 548 } 549 550 public boolean hasIdentifier() { 551 if (this.identifier == null) 552 return false; 553 for (Identifier item : this.identifier) 554 if (!item.isEmpty()) 555 return true; 556 return false; 557 } 558 559 public Identifier addIdentifier() { //3 560 Identifier t = new Identifier(); 561 if (this.identifier == null) 562 this.identifier = new ArrayList<Identifier>(); 563 this.identifier.add(t); 564 return t; 565 } 566 567 public MedicationStatement addIdentifier(Identifier t) { //3 568 if (t == null) 569 return this; 570 if (this.identifier == null) 571 this.identifier = new ArrayList<Identifier>(); 572 this.identifier.add(t); 573 return this; 574 } 575 576 /** 577 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 578 */ 579 public Identifier getIdentifierFirstRep() { 580 if (getIdentifier().isEmpty()) { 581 addIdentifier(); 582 } 583 return getIdentifier().get(0); 584 } 585 586 /** 587 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event.) 588 */ 589 public List<Reference> getBasedOn() { 590 if (this.basedOn == null) 591 this.basedOn = new ArrayList<Reference>(); 592 return this.basedOn; 593 } 594 595 /** 596 * @return Returns a reference to <code>this</code> for easy method chaining 597 */ 598 public MedicationStatement setBasedOn(List<Reference> theBasedOn) { 599 this.basedOn = theBasedOn; 600 return this; 601 } 602 603 public boolean hasBasedOn() { 604 if (this.basedOn == null) 605 return false; 606 for (Reference item : this.basedOn) 607 if (!item.isEmpty()) 608 return true; 609 return false; 610 } 611 612 public Reference addBasedOn() { //3 613 Reference t = new Reference(); 614 if (this.basedOn == null) 615 this.basedOn = new ArrayList<Reference>(); 616 this.basedOn.add(t); 617 return t; 618 } 619 620 public MedicationStatement addBasedOn(Reference t) { //3 621 if (t == null) 622 return this; 623 if (this.basedOn == null) 624 this.basedOn = new ArrayList<Reference>(); 625 this.basedOn.add(t); 626 return this; 627 } 628 629 /** 630 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 631 */ 632 public Reference getBasedOnFirstRep() { 633 if (getBasedOn().isEmpty()) { 634 addBasedOn(); 635 } 636 return getBasedOn().get(0); 637 } 638 639 /** 640 * @deprecated Use Reference#setResource(IBaseResource) instead 641 */ 642 @Deprecated 643 public List<Resource> getBasedOnTarget() { 644 if (this.basedOnTarget == null) 645 this.basedOnTarget = new ArrayList<Resource>(); 646 return this.basedOnTarget; 647 } 648 649 /** 650 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 651 */ 652 public List<Reference> getPartOf() { 653 if (this.partOf == null) 654 this.partOf = new ArrayList<Reference>(); 655 return this.partOf; 656 } 657 658 /** 659 * @return Returns a reference to <code>this</code> for easy method chaining 660 */ 661 public MedicationStatement setPartOf(List<Reference> thePartOf) { 662 this.partOf = thePartOf; 663 return this; 664 } 665 666 public boolean hasPartOf() { 667 if (this.partOf == null) 668 return false; 669 for (Reference item : this.partOf) 670 if (!item.isEmpty()) 671 return true; 672 return false; 673 } 674 675 public Reference addPartOf() { //3 676 Reference t = new Reference(); 677 if (this.partOf == null) 678 this.partOf = new ArrayList<Reference>(); 679 this.partOf.add(t); 680 return t; 681 } 682 683 public MedicationStatement addPartOf(Reference t) { //3 684 if (t == null) 685 return this; 686 if (this.partOf == null) 687 this.partOf = new ArrayList<Reference>(); 688 this.partOf.add(t); 689 return this; 690 } 691 692 /** 693 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 694 */ 695 public Reference getPartOfFirstRep() { 696 if (getPartOf().isEmpty()) { 697 addPartOf(); 698 } 699 return getPartOf().get(0); 700 } 701 702 /** 703 * @deprecated Use Reference#setResource(IBaseResource) instead 704 */ 705 @Deprecated 706 public List<Resource> getPartOfTarget() { 707 if (this.partOfTarget == null) 708 this.partOfTarget = new ArrayList<Resource>(); 709 return this.partOfTarget; 710 } 711 712 /** 713 * @return {@link #context} (The encounter or episode of care that establishes the context for this MedicationStatement.) 714 */ 715 public Reference getContext() { 716 if (this.context == null) 717 if (Configuration.errorOnAutoCreate()) 718 throw new Error("Attempt to auto-create MedicationStatement.context"); 719 else if (Configuration.doAutoCreate()) 720 this.context = new Reference(); // cc 721 return this.context; 722 } 723 724 public boolean hasContext() { 725 return this.context != null && !this.context.isEmpty(); 726 } 727 728 /** 729 * @param value {@link #context} (The encounter or episode of care that establishes the context for this MedicationStatement.) 730 */ 731 public MedicationStatement setContext(Reference value) { 732 this.context = value; 733 return this; 734 } 735 736 /** 737 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this MedicationStatement.) 738 */ 739 public Resource getContextTarget() { 740 return this.contextTarget; 741 } 742 743 /** 744 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this MedicationStatement.) 745 */ 746 public MedicationStatement setContextTarget(Resource value) { 747 this.contextTarget = value; 748 return this; 749 } 750 751 /** 752 * @return {@link #status} (A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 753 */ 754 public Enumeration<MedicationStatementStatus> getStatusElement() { 755 if (this.status == null) 756 if (Configuration.errorOnAutoCreate()) 757 throw new Error("Attempt to auto-create MedicationStatement.status"); 758 else if (Configuration.doAutoCreate()) 759 this.status = new Enumeration<MedicationStatementStatus>(new MedicationStatementStatusEnumFactory()); // bb 760 return this.status; 761 } 762 763 public boolean hasStatusElement() { 764 return this.status != null && !this.status.isEmpty(); 765 } 766 767 public boolean hasStatus() { 768 return this.status != null && !this.status.isEmpty(); 769 } 770 771 /** 772 * @param value {@link #status} (A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 773 */ 774 public MedicationStatement setStatusElement(Enumeration<MedicationStatementStatus> value) { 775 this.status = value; 776 return this; 777 } 778 779 /** 780 * @return A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed. 781 */ 782 public MedicationStatementStatus getStatus() { 783 return this.status == null ? null : this.status.getValue(); 784 } 785 786 /** 787 * @param value A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed. 788 */ 789 public MedicationStatement setStatus(MedicationStatementStatus value) { 790 if (this.status == null) 791 this.status = new Enumeration<MedicationStatementStatus>(new MedicationStatementStatusEnumFactory()); 792 this.status.setValue(value); 793 return this; 794 } 795 796 /** 797 * @return {@link #category} (Indicates where type of medication statement and where the medication is expected to be consumed or administered.) 798 */ 799 public CodeableConcept getCategory() { 800 if (this.category == null) 801 if (Configuration.errorOnAutoCreate()) 802 throw new Error("Attempt to auto-create MedicationStatement.category"); 803 else if (Configuration.doAutoCreate()) 804 this.category = new CodeableConcept(); // cc 805 return this.category; 806 } 807 808 public boolean hasCategory() { 809 return this.category != null && !this.category.isEmpty(); 810 } 811 812 /** 813 * @param value {@link #category} (Indicates where type of medication statement and where the medication is expected to be consumed or administered.) 814 */ 815 public MedicationStatement setCategory(CodeableConcept value) { 816 this.category = value; 817 return this; 818 } 819 820 /** 821 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 822 */ 823 public Type getMedication() { 824 return this.medication; 825 } 826 827 /** 828 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 829 */ 830 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 831 if (this.medication == null) 832 return null; 833 if (!(this.medication instanceof CodeableConcept)) 834 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.medication.getClass().getName()+" was encountered"); 835 return (CodeableConcept) this.medication; 836 } 837 838 public boolean hasMedicationCodeableConcept() { 839 return this != null && this.medication instanceof CodeableConcept; 840 } 841 842 /** 843 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 844 */ 845 public Reference getMedicationReference() throws FHIRException { 846 if (this.medication == null) 847 return null; 848 if (!(this.medication instanceof Reference)) 849 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.medication.getClass().getName()+" was encountered"); 850 return (Reference) this.medication; 851 } 852 853 public boolean hasMedicationReference() { 854 return this != null && this.medication instanceof Reference; 855 } 856 857 public boolean hasMedication() { 858 return this.medication != null && !this.medication.isEmpty(); 859 } 860 861 /** 862 * @param value {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 863 */ 864 public MedicationStatement setMedication(Type value) throws FHIRFormatError { 865 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 866 throw new FHIRFormatError("Not the right type for MedicationStatement.medication[x]: "+value.fhirType()); 867 this.medication = value; 868 return this; 869 } 870 871 /** 872 * @return {@link #effective} (The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).) 873 */ 874 public Type getEffective() { 875 return this.effective; 876 } 877 878 /** 879 * @return {@link #effective} (The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).) 880 */ 881 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 882 if (this.effective == null) 883 return null; 884 if (!(this.effective instanceof DateTimeType)) 885 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 886 return (DateTimeType) this.effective; 887 } 888 889 public boolean hasEffectiveDateTimeType() { 890 return this != null && this.effective instanceof DateTimeType; 891 } 892 893 /** 894 * @return {@link #effective} (The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).) 895 */ 896 public Period getEffectivePeriod() throws FHIRException { 897 if (this.effective == null) 898 return null; 899 if (!(this.effective instanceof Period)) 900 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 901 return (Period) this.effective; 902 } 903 904 public boolean hasEffectivePeriod() { 905 return this != null && this.effective instanceof Period; 906 } 907 908 public boolean hasEffective() { 909 return this.effective != null && !this.effective.isEmpty(); 910 } 911 912 /** 913 * @param value {@link #effective} (The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).) 914 */ 915 public MedicationStatement setEffective(Type value) throws FHIRFormatError { 916 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 917 throw new FHIRFormatError("Not the right type for MedicationStatement.effective[x]: "+value.fhirType()); 918 this.effective = value; 919 return this; 920 } 921 922 /** 923 * @return {@link #dateAsserted} (The date when the medication statement was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 924 */ 925 public DateTimeType getDateAssertedElement() { 926 if (this.dateAsserted == null) 927 if (Configuration.errorOnAutoCreate()) 928 throw new Error("Attempt to auto-create MedicationStatement.dateAsserted"); 929 else if (Configuration.doAutoCreate()) 930 this.dateAsserted = new DateTimeType(); // bb 931 return this.dateAsserted; 932 } 933 934 public boolean hasDateAssertedElement() { 935 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 936 } 937 938 public boolean hasDateAsserted() { 939 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 940 } 941 942 /** 943 * @param value {@link #dateAsserted} (The date when the medication statement was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 944 */ 945 public MedicationStatement setDateAssertedElement(DateTimeType value) { 946 this.dateAsserted = value; 947 return this; 948 } 949 950 /** 951 * @return The date when the medication statement was asserted by the information source. 952 */ 953 public Date getDateAsserted() { 954 return this.dateAsserted == null ? null : this.dateAsserted.getValue(); 955 } 956 957 /** 958 * @param value The date when the medication statement was asserted by the information source. 959 */ 960 public MedicationStatement setDateAsserted(Date value) { 961 if (value == null) 962 this.dateAsserted = null; 963 else { 964 if (this.dateAsserted == null) 965 this.dateAsserted = new DateTimeType(); 966 this.dateAsserted.setValue(value); 967 } 968 return this; 969 } 970 971 /** 972 * @return {@link #informationSource} (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 973 */ 974 public Reference getInformationSource() { 975 if (this.informationSource == null) 976 if (Configuration.errorOnAutoCreate()) 977 throw new Error("Attempt to auto-create MedicationStatement.informationSource"); 978 else if (Configuration.doAutoCreate()) 979 this.informationSource = new Reference(); // cc 980 return this.informationSource; 981 } 982 983 public boolean hasInformationSource() { 984 return this.informationSource != null && !this.informationSource.isEmpty(); 985 } 986 987 /** 988 * @param value {@link #informationSource} (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 989 */ 990 public MedicationStatement setInformationSource(Reference value) { 991 this.informationSource = value; 992 return this; 993 } 994 995 /** 996 * @return {@link #informationSource} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 997 */ 998 public Resource getInformationSourceTarget() { 999 return this.informationSourceTarget; 1000 } 1001 1002 /** 1003 * @param value {@link #informationSource} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 1004 */ 1005 public MedicationStatement setInformationSourceTarget(Resource value) { 1006 this.informationSourceTarget = value; 1007 return this; 1008 } 1009 1010 /** 1011 * @return {@link #subject} (The person, animal or group who is/was taking the medication.) 1012 */ 1013 public Reference getSubject() { 1014 if (this.subject == null) 1015 if (Configuration.errorOnAutoCreate()) 1016 throw new Error("Attempt to auto-create MedicationStatement.subject"); 1017 else if (Configuration.doAutoCreate()) 1018 this.subject = new Reference(); // cc 1019 return this.subject; 1020 } 1021 1022 public boolean hasSubject() { 1023 return this.subject != null && !this.subject.isEmpty(); 1024 } 1025 1026 /** 1027 * @param value {@link #subject} (The person, animal or group who is/was taking the medication.) 1028 */ 1029 public MedicationStatement setSubject(Reference value) { 1030 this.subject = value; 1031 return this; 1032 } 1033 1034 /** 1035 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person, animal or group who is/was taking the medication.) 1036 */ 1037 public Resource getSubjectTarget() { 1038 return this.subjectTarget; 1039 } 1040 1041 /** 1042 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person, animal or group who is/was taking the medication.) 1043 */ 1044 public MedicationStatement setSubjectTarget(Resource value) { 1045 this.subjectTarget = value; 1046 return this; 1047 } 1048 1049 /** 1050 * @return {@link #derivedFrom} (Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.) 1051 */ 1052 public List<Reference> getDerivedFrom() { 1053 if (this.derivedFrom == null) 1054 this.derivedFrom = new ArrayList<Reference>(); 1055 return this.derivedFrom; 1056 } 1057 1058 /** 1059 * @return Returns a reference to <code>this</code> for easy method chaining 1060 */ 1061 public MedicationStatement setDerivedFrom(List<Reference> theDerivedFrom) { 1062 this.derivedFrom = theDerivedFrom; 1063 return this; 1064 } 1065 1066 public boolean hasDerivedFrom() { 1067 if (this.derivedFrom == null) 1068 return false; 1069 for (Reference item : this.derivedFrom) 1070 if (!item.isEmpty()) 1071 return true; 1072 return false; 1073 } 1074 1075 public Reference addDerivedFrom() { //3 1076 Reference t = new Reference(); 1077 if (this.derivedFrom == null) 1078 this.derivedFrom = new ArrayList<Reference>(); 1079 this.derivedFrom.add(t); 1080 return t; 1081 } 1082 1083 public MedicationStatement addDerivedFrom(Reference t) { //3 1084 if (t == null) 1085 return this; 1086 if (this.derivedFrom == null) 1087 this.derivedFrom = new ArrayList<Reference>(); 1088 this.derivedFrom.add(t); 1089 return this; 1090 } 1091 1092 /** 1093 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist 1094 */ 1095 public Reference getDerivedFromFirstRep() { 1096 if (getDerivedFrom().isEmpty()) { 1097 addDerivedFrom(); 1098 } 1099 return getDerivedFrom().get(0); 1100 } 1101 1102 /** 1103 * @deprecated Use Reference#setResource(IBaseResource) instead 1104 */ 1105 @Deprecated 1106 public List<Resource> getDerivedFromTarget() { 1107 if (this.derivedFromTarget == null) 1108 this.derivedFromTarget = new ArrayList<Resource>(); 1109 return this.derivedFromTarget; 1110 } 1111 1112 /** 1113 * @return {@link #taken} (Indicator of the certainty of whether the medication was taken by the patient.). This is the underlying object with id, value and extensions. The accessor "getTaken" gives direct access to the value 1114 */ 1115 public Enumeration<MedicationStatementTaken> getTakenElement() { 1116 if (this.taken == null) 1117 if (Configuration.errorOnAutoCreate()) 1118 throw new Error("Attempt to auto-create MedicationStatement.taken"); 1119 else if (Configuration.doAutoCreate()) 1120 this.taken = new Enumeration<MedicationStatementTaken>(new MedicationStatementTakenEnumFactory()); // bb 1121 return this.taken; 1122 } 1123 1124 public boolean hasTakenElement() { 1125 return this.taken != null && !this.taken.isEmpty(); 1126 } 1127 1128 public boolean hasTaken() { 1129 return this.taken != null && !this.taken.isEmpty(); 1130 } 1131 1132 /** 1133 * @param value {@link #taken} (Indicator of the certainty of whether the medication was taken by the patient.). This is the underlying object with id, value and extensions. The accessor "getTaken" gives direct access to the value 1134 */ 1135 public MedicationStatement setTakenElement(Enumeration<MedicationStatementTaken> value) { 1136 this.taken = value; 1137 return this; 1138 } 1139 1140 /** 1141 * @return Indicator of the certainty of whether the medication was taken by the patient. 1142 */ 1143 public MedicationStatementTaken getTaken() { 1144 return this.taken == null ? null : this.taken.getValue(); 1145 } 1146 1147 /** 1148 * @param value Indicator of the certainty of whether the medication was taken by the patient. 1149 */ 1150 public MedicationStatement setTaken(MedicationStatementTaken value) { 1151 if (this.taken == null) 1152 this.taken = new Enumeration<MedicationStatementTaken>(new MedicationStatementTakenEnumFactory()); 1153 this.taken.setValue(value); 1154 return this; 1155 } 1156 1157 /** 1158 * @return {@link #reasonNotTaken} (A code indicating why the medication was not taken.) 1159 */ 1160 public List<CodeableConcept> getReasonNotTaken() { 1161 if (this.reasonNotTaken == null) 1162 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1163 return this.reasonNotTaken; 1164 } 1165 1166 /** 1167 * @return Returns a reference to <code>this</code> for easy method chaining 1168 */ 1169 public MedicationStatement setReasonNotTaken(List<CodeableConcept> theReasonNotTaken) { 1170 this.reasonNotTaken = theReasonNotTaken; 1171 return this; 1172 } 1173 1174 public boolean hasReasonNotTaken() { 1175 if (this.reasonNotTaken == null) 1176 return false; 1177 for (CodeableConcept item : this.reasonNotTaken) 1178 if (!item.isEmpty()) 1179 return true; 1180 return false; 1181 } 1182 1183 public CodeableConcept addReasonNotTaken() { //3 1184 CodeableConcept t = new CodeableConcept(); 1185 if (this.reasonNotTaken == null) 1186 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1187 this.reasonNotTaken.add(t); 1188 return t; 1189 } 1190 1191 public MedicationStatement addReasonNotTaken(CodeableConcept t) { //3 1192 if (t == null) 1193 return this; 1194 if (this.reasonNotTaken == null) 1195 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1196 this.reasonNotTaken.add(t); 1197 return this; 1198 } 1199 1200 /** 1201 * @return The first repetition of repeating field {@link #reasonNotTaken}, creating it if it does not already exist 1202 */ 1203 public CodeableConcept getReasonNotTakenFirstRep() { 1204 if (getReasonNotTaken().isEmpty()) { 1205 addReasonNotTaken(); 1206 } 1207 return getReasonNotTaken().get(0); 1208 } 1209 1210 /** 1211 * @return {@link #reasonCode} (A reason for why the medication is being/was taken.) 1212 */ 1213 public List<CodeableConcept> getReasonCode() { 1214 if (this.reasonCode == null) 1215 this.reasonCode = new ArrayList<CodeableConcept>(); 1216 return this.reasonCode; 1217 } 1218 1219 /** 1220 * @return Returns a reference to <code>this</code> for easy method chaining 1221 */ 1222 public MedicationStatement setReasonCode(List<CodeableConcept> theReasonCode) { 1223 this.reasonCode = theReasonCode; 1224 return this; 1225 } 1226 1227 public boolean hasReasonCode() { 1228 if (this.reasonCode == null) 1229 return false; 1230 for (CodeableConcept item : this.reasonCode) 1231 if (!item.isEmpty()) 1232 return true; 1233 return false; 1234 } 1235 1236 public CodeableConcept addReasonCode() { //3 1237 CodeableConcept t = new CodeableConcept(); 1238 if (this.reasonCode == null) 1239 this.reasonCode = new ArrayList<CodeableConcept>(); 1240 this.reasonCode.add(t); 1241 return t; 1242 } 1243 1244 public MedicationStatement addReasonCode(CodeableConcept t) { //3 1245 if (t == null) 1246 return this; 1247 if (this.reasonCode == null) 1248 this.reasonCode = new ArrayList<CodeableConcept>(); 1249 this.reasonCode.add(t); 1250 return this; 1251 } 1252 1253 /** 1254 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1255 */ 1256 public CodeableConcept getReasonCodeFirstRep() { 1257 if (getReasonCode().isEmpty()) { 1258 addReasonCode(); 1259 } 1260 return getReasonCode().get(0); 1261 } 1262 1263 /** 1264 * @return {@link #reasonReference} (Condition or observation that supports why the medication is being/was taken.) 1265 */ 1266 public List<Reference> getReasonReference() { 1267 if (this.reasonReference == null) 1268 this.reasonReference = new ArrayList<Reference>(); 1269 return this.reasonReference; 1270 } 1271 1272 /** 1273 * @return Returns a reference to <code>this</code> for easy method chaining 1274 */ 1275 public MedicationStatement setReasonReference(List<Reference> theReasonReference) { 1276 this.reasonReference = theReasonReference; 1277 return this; 1278 } 1279 1280 public boolean hasReasonReference() { 1281 if (this.reasonReference == null) 1282 return false; 1283 for (Reference item : this.reasonReference) 1284 if (!item.isEmpty()) 1285 return true; 1286 return false; 1287 } 1288 1289 public Reference addReasonReference() { //3 1290 Reference t = new Reference(); 1291 if (this.reasonReference == null) 1292 this.reasonReference = new ArrayList<Reference>(); 1293 this.reasonReference.add(t); 1294 return t; 1295 } 1296 1297 public MedicationStatement addReasonReference(Reference t) { //3 1298 if (t == null) 1299 return this; 1300 if (this.reasonReference == null) 1301 this.reasonReference = new ArrayList<Reference>(); 1302 this.reasonReference.add(t); 1303 return this; 1304 } 1305 1306 /** 1307 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1308 */ 1309 public Reference getReasonReferenceFirstRep() { 1310 if (getReasonReference().isEmpty()) { 1311 addReasonReference(); 1312 } 1313 return getReasonReference().get(0); 1314 } 1315 1316 /** 1317 * @deprecated Use Reference#setResource(IBaseResource) instead 1318 */ 1319 @Deprecated 1320 public List<Resource> getReasonReferenceTarget() { 1321 if (this.reasonReferenceTarget == null) 1322 this.reasonReferenceTarget = new ArrayList<Resource>(); 1323 return this.reasonReferenceTarget; 1324 } 1325 1326 /** 1327 * @return {@link #note} (Provides extra information about the medication statement that is not conveyed by the other attributes.) 1328 */ 1329 public List<Annotation> getNote() { 1330 if (this.note == null) 1331 this.note = new ArrayList<Annotation>(); 1332 return this.note; 1333 } 1334 1335 /** 1336 * @return Returns a reference to <code>this</code> for easy method chaining 1337 */ 1338 public MedicationStatement setNote(List<Annotation> theNote) { 1339 this.note = theNote; 1340 return this; 1341 } 1342 1343 public boolean hasNote() { 1344 if (this.note == null) 1345 return false; 1346 for (Annotation item : this.note) 1347 if (!item.isEmpty()) 1348 return true; 1349 return false; 1350 } 1351 1352 public Annotation addNote() { //3 1353 Annotation t = new Annotation(); 1354 if (this.note == null) 1355 this.note = new ArrayList<Annotation>(); 1356 this.note.add(t); 1357 return t; 1358 } 1359 1360 public MedicationStatement addNote(Annotation t) { //3 1361 if (t == null) 1362 return this; 1363 if (this.note == null) 1364 this.note = new ArrayList<Annotation>(); 1365 this.note.add(t); 1366 return this; 1367 } 1368 1369 /** 1370 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1371 */ 1372 public Annotation getNoteFirstRep() { 1373 if (getNote().isEmpty()) { 1374 addNote(); 1375 } 1376 return getNote().get(0); 1377 } 1378 1379 /** 1380 * @return {@link #dosage} (Indicates how the medication is/was or should be taken by the patient.) 1381 */ 1382 public List<Dosage> getDosage() { 1383 if (this.dosage == null) 1384 this.dosage = new ArrayList<Dosage>(); 1385 return this.dosage; 1386 } 1387 1388 /** 1389 * @return Returns a reference to <code>this</code> for easy method chaining 1390 */ 1391 public MedicationStatement setDosage(List<Dosage> theDosage) { 1392 this.dosage = theDosage; 1393 return this; 1394 } 1395 1396 public boolean hasDosage() { 1397 if (this.dosage == null) 1398 return false; 1399 for (Dosage item : this.dosage) 1400 if (!item.isEmpty()) 1401 return true; 1402 return false; 1403 } 1404 1405 public Dosage addDosage() { //3 1406 Dosage t = new Dosage(); 1407 if (this.dosage == null) 1408 this.dosage = new ArrayList<Dosage>(); 1409 this.dosage.add(t); 1410 return t; 1411 } 1412 1413 public MedicationStatement addDosage(Dosage t) { //3 1414 if (t == null) 1415 return this; 1416 if (this.dosage == null) 1417 this.dosage = new ArrayList<Dosage>(); 1418 this.dosage.add(t); 1419 return this; 1420 } 1421 1422 /** 1423 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist 1424 */ 1425 public Dosage getDosageFirstRep() { 1426 if (getDosage().isEmpty()) { 1427 addDosage(); 1428 } 1429 return getDosage().get(0); 1430 } 1431 1432 protected void listChildren(List<Property> children) { 1433 super.listChildren(children); 1434 children.add(new Property("identifier", "Identifier", "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1435 children.add(new Property("basedOn", "Reference(MedicationRequest|CarePlan|ProcedureRequest|ReferralRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1436 children.add(new Property("partOf", "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Observation)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1437 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this MedicationStatement.", 0, 1, context)); 1438 children.add(new Property("status", "code", "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.", 0, 1, status)); 1439 children.add(new Property("category", "CodeableConcept", "Indicates where type of medication statement and where the medication is expected to be consumed or administered.", 0, 1, category)); 1440 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 1441 children.add(new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective)); 1442 children.add(new Property("dateAsserted", "dateTime", "The date when the medication statement was asserted by the information source.", 0, 1, dateAsserted)); 1443 children.add(new Property("informationSource", "Reference(Patient|Practitioner|RelatedPerson|Organization)", "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.", 0, 1, informationSource)); 1444 children.add(new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was taking the medication.", 0, 1, subject)); 1445 children.add(new Property("derivedFrom", "Reference(Any)", "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1446 children.add(new Property("taken", "code", "Indicator of the certainty of whether the medication was taken by the patient.", 0, 1, taken)); 1447 children.add(new Property("reasonNotTaken", "CodeableConcept", "A code indicating why the medication was not taken.", 0, java.lang.Integer.MAX_VALUE, reasonNotTaken)); 1448 children.add(new Property("reasonCode", "CodeableConcept", "A reason for why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1449 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1450 children.add(new Property("note", "Annotation", "Provides extra information about the medication statement that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 1451 children.add(new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, dosage)); 1452 } 1453 1454 @Override 1455 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1456 switch (_hash) { 1457 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 0, java.lang.Integer.MAX_VALUE, identifier); 1458 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(MedicationRequest|CarePlan|ProcedureRequest|ReferralRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1459 case -995410646: /*partOf*/ return new Property("partOf", "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Observation)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1460 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this MedicationStatement.", 0, 1, context); 1461 case -892481550: /*status*/ return new Property("status", "code", "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.", 0, 1, status); 1462 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates where type of medication statement and where the medication is expected to be consumed or administered.", 0, 1, category); 1463 case 1458402129: /*medication[x]*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1464 case 1998965455: /*medication*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1465 case -209845038: /*medicationCodeableConcept*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1466 case 2104315196: /*medicationReference*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1467 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective); 1468 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective); 1469 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective); 1470 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective); 1471 case -1980855245: /*dateAsserted*/ return new Property("dateAsserted", "dateTime", "The date when the medication statement was asserted by the information source.", 0, 1, dateAsserted); 1472 case -2123220889: /*informationSource*/ return new Property("informationSource", "Reference(Patient|Practitioner|RelatedPerson|Organization)", "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.", 0, 1, informationSource); 1473 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was taking the medication.", 0, 1, subject); 1474 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(Any)", "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1475 case 110124231: /*taken*/ return new Property("taken", "code", "Indicator of the certainty of whether the medication was taken by the patient.", 0, 1, taken); 1476 case 2112880664: /*reasonNotTaken*/ return new Property("reasonNotTaken", "CodeableConcept", "A code indicating why the medication was not taken.", 0, java.lang.Integer.MAX_VALUE, reasonNotTaken); 1477 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "A reason for why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1478 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1479 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides extra information about the medication statement that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 1480 case -1326018889: /*dosage*/ return new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, dosage); 1481 default: return super.getNamedProperty(_hash, _name, _checkValid); 1482 } 1483 1484 } 1485 1486 @Override 1487 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1488 switch (hash) { 1489 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1490 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1491 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1492 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1493 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationStatementStatus> 1494 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1495 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // Type 1496 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // Type 1497 case -1980855245: /*dateAsserted*/ return this.dateAsserted == null ? new Base[0] : new Base[] {this.dateAsserted}; // DateTimeType 1498 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : new Base[] {this.informationSource}; // Reference 1499 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1500 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 1501 case 110124231: /*taken*/ return this.taken == null ? new Base[0] : new Base[] {this.taken}; // Enumeration<MedicationStatementTaken> 1502 case 2112880664: /*reasonNotTaken*/ return this.reasonNotTaken == null ? new Base[0] : this.reasonNotTaken.toArray(new Base[this.reasonNotTaken.size()]); // CodeableConcept 1503 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1504 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1505 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1506 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 1507 default: return super.getProperty(hash, name, checkValid); 1508 } 1509 1510 } 1511 1512 @Override 1513 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1514 switch (hash) { 1515 case -1618432855: // identifier 1516 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1517 return value; 1518 case -332612366: // basedOn 1519 this.getBasedOn().add(castToReference(value)); // Reference 1520 return value; 1521 case -995410646: // partOf 1522 this.getPartOf().add(castToReference(value)); // Reference 1523 return value; 1524 case 951530927: // context 1525 this.context = castToReference(value); // Reference 1526 return value; 1527 case -892481550: // status 1528 value = new MedicationStatementStatusEnumFactory().fromType(castToCode(value)); 1529 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatus> 1530 return value; 1531 case 50511102: // category 1532 this.category = castToCodeableConcept(value); // CodeableConcept 1533 return value; 1534 case 1998965455: // medication 1535 this.medication = castToType(value); // Type 1536 return value; 1537 case -1468651097: // effective 1538 this.effective = castToType(value); // Type 1539 return value; 1540 case -1980855245: // dateAsserted 1541 this.dateAsserted = castToDateTime(value); // DateTimeType 1542 return value; 1543 case -2123220889: // informationSource 1544 this.informationSource = castToReference(value); // Reference 1545 return value; 1546 case -1867885268: // subject 1547 this.subject = castToReference(value); // Reference 1548 return value; 1549 case 1077922663: // derivedFrom 1550 this.getDerivedFrom().add(castToReference(value)); // Reference 1551 return value; 1552 case 110124231: // taken 1553 value = new MedicationStatementTakenEnumFactory().fromType(castToCode(value)); 1554 this.taken = (Enumeration) value; // Enumeration<MedicationStatementTaken> 1555 return value; 1556 case 2112880664: // reasonNotTaken 1557 this.getReasonNotTaken().add(castToCodeableConcept(value)); // CodeableConcept 1558 return value; 1559 case 722137681: // reasonCode 1560 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1561 return value; 1562 case -1146218137: // reasonReference 1563 this.getReasonReference().add(castToReference(value)); // Reference 1564 return value; 1565 case 3387378: // note 1566 this.getNote().add(castToAnnotation(value)); // Annotation 1567 return value; 1568 case -1326018889: // dosage 1569 this.getDosage().add(castToDosage(value)); // Dosage 1570 return value; 1571 default: return super.setProperty(hash, name, value); 1572 } 1573 1574 } 1575 1576 @Override 1577 public Base setProperty(String name, Base value) throws FHIRException { 1578 if (name.equals("identifier")) { 1579 this.getIdentifier().add(castToIdentifier(value)); 1580 } else if (name.equals("basedOn")) { 1581 this.getBasedOn().add(castToReference(value)); 1582 } else if (name.equals("partOf")) { 1583 this.getPartOf().add(castToReference(value)); 1584 } else if (name.equals("context")) { 1585 this.context = castToReference(value); // Reference 1586 } else if (name.equals("status")) { 1587 value = new MedicationStatementStatusEnumFactory().fromType(castToCode(value)); 1588 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatus> 1589 } else if (name.equals("category")) { 1590 this.category = castToCodeableConcept(value); // CodeableConcept 1591 } else if (name.equals("medication[x]")) { 1592 this.medication = castToType(value); // Type 1593 } else if (name.equals("effective[x]")) { 1594 this.effective = castToType(value); // Type 1595 } else if (name.equals("dateAsserted")) { 1596 this.dateAsserted = castToDateTime(value); // DateTimeType 1597 } else if (name.equals("informationSource")) { 1598 this.informationSource = castToReference(value); // Reference 1599 } else if (name.equals("subject")) { 1600 this.subject = castToReference(value); // Reference 1601 } else if (name.equals("derivedFrom")) { 1602 this.getDerivedFrom().add(castToReference(value)); 1603 } else if (name.equals("taken")) { 1604 value = new MedicationStatementTakenEnumFactory().fromType(castToCode(value)); 1605 this.taken = (Enumeration) value; // Enumeration<MedicationStatementTaken> 1606 } else if (name.equals("reasonNotTaken")) { 1607 this.getReasonNotTaken().add(castToCodeableConcept(value)); 1608 } else if (name.equals("reasonCode")) { 1609 this.getReasonCode().add(castToCodeableConcept(value)); 1610 } else if (name.equals("reasonReference")) { 1611 this.getReasonReference().add(castToReference(value)); 1612 } else if (name.equals("note")) { 1613 this.getNote().add(castToAnnotation(value)); 1614 } else if (name.equals("dosage")) { 1615 this.getDosage().add(castToDosage(value)); 1616 } else 1617 return super.setProperty(name, value); 1618 return value; 1619 } 1620 1621 @Override 1622 public Base makeProperty(int hash, String name) throws FHIRException { 1623 switch (hash) { 1624 case -1618432855: return addIdentifier(); 1625 case -332612366: return addBasedOn(); 1626 case -995410646: return addPartOf(); 1627 case 951530927: return getContext(); 1628 case -892481550: return getStatusElement(); 1629 case 50511102: return getCategory(); 1630 case 1458402129: return getMedication(); 1631 case 1998965455: return getMedication(); 1632 case 247104889: return getEffective(); 1633 case -1468651097: return getEffective(); 1634 case -1980855245: return getDateAssertedElement(); 1635 case -2123220889: return getInformationSource(); 1636 case -1867885268: return getSubject(); 1637 case 1077922663: return addDerivedFrom(); 1638 case 110124231: return getTakenElement(); 1639 case 2112880664: return addReasonNotTaken(); 1640 case 722137681: return addReasonCode(); 1641 case -1146218137: return addReasonReference(); 1642 case 3387378: return addNote(); 1643 case -1326018889: return addDosage(); 1644 default: return super.makeProperty(hash, name); 1645 } 1646 1647 } 1648 1649 @Override 1650 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1651 switch (hash) { 1652 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1653 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1654 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1655 case 951530927: /*context*/ return new String[] {"Reference"}; 1656 case -892481550: /*status*/ return new String[] {"code"}; 1657 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1658 case 1998965455: /*medication*/ return new String[] {"CodeableConcept", "Reference"}; 1659 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 1660 case -1980855245: /*dateAsserted*/ return new String[] {"dateTime"}; 1661 case -2123220889: /*informationSource*/ return new String[] {"Reference"}; 1662 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1663 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 1664 case 110124231: /*taken*/ return new String[] {"code"}; 1665 case 2112880664: /*reasonNotTaken*/ return new String[] {"CodeableConcept"}; 1666 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 1667 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 1668 case 3387378: /*note*/ return new String[] {"Annotation"}; 1669 case -1326018889: /*dosage*/ return new String[] {"Dosage"}; 1670 default: return super.getTypesForProperty(hash, name); 1671 } 1672 1673 } 1674 1675 @Override 1676 public Base addChild(String name) throws FHIRException { 1677 if (name.equals("identifier")) { 1678 return addIdentifier(); 1679 } 1680 else if (name.equals("basedOn")) { 1681 return addBasedOn(); 1682 } 1683 else if (name.equals("partOf")) { 1684 return addPartOf(); 1685 } 1686 else if (name.equals("context")) { 1687 this.context = new Reference(); 1688 return this.context; 1689 } 1690 else if (name.equals("status")) { 1691 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.status"); 1692 } 1693 else if (name.equals("category")) { 1694 this.category = new CodeableConcept(); 1695 return this.category; 1696 } 1697 else if (name.equals("medicationCodeableConcept")) { 1698 this.medication = new CodeableConcept(); 1699 return this.medication; 1700 } 1701 else if (name.equals("medicationReference")) { 1702 this.medication = new Reference(); 1703 return this.medication; 1704 } 1705 else if (name.equals("effectiveDateTime")) { 1706 this.effective = new DateTimeType(); 1707 return this.effective; 1708 } 1709 else if (name.equals("effectivePeriod")) { 1710 this.effective = new Period(); 1711 return this.effective; 1712 } 1713 else if (name.equals("dateAsserted")) { 1714 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.dateAsserted"); 1715 } 1716 else if (name.equals("informationSource")) { 1717 this.informationSource = new Reference(); 1718 return this.informationSource; 1719 } 1720 else if (name.equals("subject")) { 1721 this.subject = new Reference(); 1722 return this.subject; 1723 } 1724 else if (name.equals("derivedFrom")) { 1725 return addDerivedFrom(); 1726 } 1727 else if (name.equals("taken")) { 1728 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.taken"); 1729 } 1730 else if (name.equals("reasonNotTaken")) { 1731 return addReasonNotTaken(); 1732 } 1733 else if (name.equals("reasonCode")) { 1734 return addReasonCode(); 1735 } 1736 else if (name.equals("reasonReference")) { 1737 return addReasonReference(); 1738 } 1739 else if (name.equals("note")) { 1740 return addNote(); 1741 } 1742 else if (name.equals("dosage")) { 1743 return addDosage(); 1744 } 1745 else 1746 return super.addChild(name); 1747 } 1748 1749 public String fhirType() { 1750 return "MedicationStatement"; 1751 1752 } 1753 1754 public MedicationStatement copy() { 1755 MedicationStatement dst = new MedicationStatement(); 1756 copyValues(dst); 1757 if (identifier != null) { 1758 dst.identifier = new ArrayList<Identifier>(); 1759 for (Identifier i : identifier) 1760 dst.identifier.add(i.copy()); 1761 }; 1762 if (basedOn != null) { 1763 dst.basedOn = new ArrayList<Reference>(); 1764 for (Reference i : basedOn) 1765 dst.basedOn.add(i.copy()); 1766 }; 1767 if (partOf != null) { 1768 dst.partOf = new ArrayList<Reference>(); 1769 for (Reference i : partOf) 1770 dst.partOf.add(i.copy()); 1771 }; 1772 dst.context = context == null ? null : context.copy(); 1773 dst.status = status == null ? null : status.copy(); 1774 dst.category = category == null ? null : category.copy(); 1775 dst.medication = medication == null ? null : medication.copy(); 1776 dst.effective = effective == null ? null : effective.copy(); 1777 dst.dateAsserted = dateAsserted == null ? null : dateAsserted.copy(); 1778 dst.informationSource = informationSource == null ? null : informationSource.copy(); 1779 dst.subject = subject == null ? null : subject.copy(); 1780 if (derivedFrom != null) { 1781 dst.derivedFrom = new ArrayList<Reference>(); 1782 for (Reference i : derivedFrom) 1783 dst.derivedFrom.add(i.copy()); 1784 }; 1785 dst.taken = taken == null ? null : taken.copy(); 1786 if (reasonNotTaken != null) { 1787 dst.reasonNotTaken = new ArrayList<CodeableConcept>(); 1788 for (CodeableConcept i : reasonNotTaken) 1789 dst.reasonNotTaken.add(i.copy()); 1790 }; 1791 if (reasonCode != null) { 1792 dst.reasonCode = new ArrayList<CodeableConcept>(); 1793 for (CodeableConcept i : reasonCode) 1794 dst.reasonCode.add(i.copy()); 1795 }; 1796 if (reasonReference != null) { 1797 dst.reasonReference = new ArrayList<Reference>(); 1798 for (Reference i : reasonReference) 1799 dst.reasonReference.add(i.copy()); 1800 }; 1801 if (note != null) { 1802 dst.note = new ArrayList<Annotation>(); 1803 for (Annotation i : note) 1804 dst.note.add(i.copy()); 1805 }; 1806 if (dosage != null) { 1807 dst.dosage = new ArrayList<Dosage>(); 1808 for (Dosage i : dosage) 1809 dst.dosage.add(i.copy()); 1810 }; 1811 return dst; 1812 } 1813 1814 protected MedicationStatement typedCopy() { 1815 return copy(); 1816 } 1817 1818 @Override 1819 public boolean equalsDeep(Base other_) { 1820 if (!super.equalsDeep(other_)) 1821 return false; 1822 if (!(other_ instanceof MedicationStatement)) 1823 return false; 1824 MedicationStatement o = (MedicationStatement) other_; 1825 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 1826 && compareDeep(context, o.context, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1827 && compareDeep(medication, o.medication, true) && compareDeep(effective, o.effective, true) && compareDeep(dateAsserted, o.dateAsserted, true) 1828 && compareDeep(informationSource, o.informationSource, true) && compareDeep(subject, o.subject, true) 1829 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(taken, o.taken, true) && compareDeep(reasonNotTaken, o.reasonNotTaken, true) 1830 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 1831 && compareDeep(note, o.note, true) && compareDeep(dosage, o.dosage, true); 1832 } 1833 1834 @Override 1835 public boolean equalsShallow(Base other_) { 1836 if (!super.equalsShallow(other_)) 1837 return false; 1838 if (!(other_ instanceof MedicationStatement)) 1839 return false; 1840 MedicationStatement o = (MedicationStatement) other_; 1841 return compareValues(status, o.status, true) && compareValues(dateAsserted, o.dateAsserted, true) && compareValues(taken, o.taken, true) 1842 ; 1843 } 1844 1845 public boolean isEmpty() { 1846 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 1847 , context, status, category, medication, effective, dateAsserted, informationSource 1848 , subject, derivedFrom, taken, reasonNotTaken, reasonCode, reasonReference, note 1849 , dosage); 1850 } 1851 1852 @Override 1853 public ResourceType getResourceType() { 1854 return ResourceType.MedicationStatement; 1855 } 1856 1857 /** 1858 * Search parameter: <b>identifier</b> 1859 * <p> 1860 * Description: <b>Return statements with this external identifier</b><br> 1861 * Type: <b>token</b><br> 1862 * Path: <b>MedicationStatement.identifier</b><br> 1863 * </p> 1864 */ 1865 @SearchParamDefinition(name="identifier", path="MedicationStatement.identifier", description="Return statements with this external identifier", type="token" ) 1866 public static final String SP_IDENTIFIER = "identifier"; 1867 /** 1868 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1869 * <p> 1870 * Description: <b>Return statements with this external identifier</b><br> 1871 * Type: <b>token</b><br> 1872 * Path: <b>MedicationStatement.identifier</b><br> 1873 * </p> 1874 */ 1875 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1876 1877 /** 1878 * Search parameter: <b>effective</b> 1879 * <p> 1880 * Description: <b>Date when patient was taking (or not taking) the medication</b><br> 1881 * Type: <b>date</b><br> 1882 * Path: <b>MedicationStatement.effective[x]</b><br> 1883 * </p> 1884 */ 1885 @SearchParamDefinition(name="effective", path="MedicationStatement.effective", description="Date when patient was taking (or not taking) the medication", type="date" ) 1886 public static final String SP_EFFECTIVE = "effective"; 1887 /** 1888 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 1889 * <p> 1890 * Description: <b>Date when patient was taking (or not taking) the medication</b><br> 1891 * Type: <b>date</b><br> 1892 * Path: <b>MedicationStatement.effective[x]</b><br> 1893 * </p> 1894 */ 1895 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 1896 1897 /** 1898 * Search parameter: <b>code</b> 1899 * <p> 1900 * Description: <b>Return statements of this medication code</b><br> 1901 * Type: <b>token</b><br> 1902 * Path: <b>MedicationStatement.medicationCodeableConcept</b><br> 1903 * </p> 1904 */ 1905 @SearchParamDefinition(name="code", path="MedicationStatement.medication.as(CodeableConcept)", description="Return statements of this medication code", type="token" ) 1906 public static final String SP_CODE = "code"; 1907 /** 1908 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1909 * <p> 1910 * Description: <b>Return statements of this medication code</b><br> 1911 * Type: <b>token</b><br> 1912 * Path: <b>MedicationStatement.medicationCodeableConcept</b><br> 1913 * </p> 1914 */ 1915 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1916 1917 /** 1918 * Search parameter: <b>subject</b> 1919 * <p> 1920 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 1921 * Type: <b>reference</b><br> 1922 * Path: <b>MedicationStatement.subject</b><br> 1923 * </p> 1924 */ 1925 @SearchParamDefinition(name="subject", path="MedicationStatement.subject", description="The identity of a patient, animal or group to list statements for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 1926 public static final String SP_SUBJECT = "subject"; 1927 /** 1928 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1929 * <p> 1930 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 1931 * Type: <b>reference</b><br> 1932 * Path: <b>MedicationStatement.subject</b><br> 1933 * </p> 1934 */ 1935 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1936 1937/** 1938 * Constant for fluent queries to be used to add include statements. Specifies 1939 * the path value of "<b>MedicationStatement:subject</b>". 1940 */ 1941 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationStatement:subject").toLocked(); 1942 1943 /** 1944 * Search parameter: <b>patient</b> 1945 * <p> 1946 * Description: <b>Returns statements for a specific patient.</b><br> 1947 * Type: <b>reference</b><br> 1948 * Path: <b>MedicationStatement.subject</b><br> 1949 * </p> 1950 */ 1951 @SearchParamDefinition(name="patient", path="MedicationStatement.subject", description="Returns statements for a specific patient.", type="reference", target={Patient.class } ) 1952 public static final String SP_PATIENT = "patient"; 1953 /** 1954 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1955 * <p> 1956 * Description: <b>Returns statements for a specific patient.</b><br> 1957 * Type: <b>reference</b><br> 1958 * Path: <b>MedicationStatement.subject</b><br> 1959 * </p> 1960 */ 1961 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1962 1963/** 1964 * Constant for fluent queries to be used to add include statements. Specifies 1965 * the path value of "<b>MedicationStatement:patient</b>". 1966 */ 1967 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationStatement:patient").toLocked(); 1968 1969 /** 1970 * Search parameter: <b>context</b> 1971 * <p> 1972 * Description: <b>Returns statements for a specific context (episode or episode of Care).</b><br> 1973 * Type: <b>reference</b><br> 1974 * Path: <b>MedicationStatement.context</b><br> 1975 * </p> 1976 */ 1977 @SearchParamDefinition(name="context", path="MedicationStatement.context", description="Returns statements for a specific context (episode or episode of Care).", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 1978 public static final String SP_CONTEXT = "context"; 1979 /** 1980 * <b>Fluent Client</b> search parameter constant for <b>context</b> 1981 * <p> 1982 * Description: <b>Returns statements for a specific context (episode or episode of Care).</b><br> 1983 * Type: <b>reference</b><br> 1984 * Path: <b>MedicationStatement.context</b><br> 1985 * </p> 1986 */ 1987 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 1988 1989/** 1990 * Constant for fluent queries to be used to add include statements. Specifies 1991 * the path value of "<b>MedicationStatement:context</b>". 1992 */ 1993 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("MedicationStatement:context").toLocked(); 1994 1995 /** 1996 * Search parameter: <b>medication</b> 1997 * <p> 1998 * Description: <b>Return statements of this medication reference</b><br> 1999 * Type: <b>reference</b><br> 2000 * Path: <b>MedicationStatement.medicationReference</b><br> 2001 * </p> 2002 */ 2003 @SearchParamDefinition(name="medication", path="MedicationStatement.medication.as(Reference)", description="Return statements of this medication reference", type="reference", target={Medication.class } ) 2004 public static final String SP_MEDICATION = "medication"; 2005 /** 2006 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 2007 * <p> 2008 * Description: <b>Return statements of this medication reference</b><br> 2009 * Type: <b>reference</b><br> 2010 * Path: <b>MedicationStatement.medicationReference</b><br> 2011 * </p> 2012 */ 2013 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 2014 2015/** 2016 * Constant for fluent queries to be used to add include statements. Specifies 2017 * the path value of "<b>MedicationStatement:medication</b>". 2018 */ 2019 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationStatement:medication").toLocked(); 2020 2021 /** 2022 * Search parameter: <b>part-of</b> 2023 * <p> 2024 * Description: <b>Returns statements that are part of another event.</b><br> 2025 * Type: <b>reference</b><br> 2026 * Path: <b>MedicationStatement.partOf</b><br> 2027 * </p> 2028 */ 2029 @SearchParamDefinition(name="part-of", path="MedicationStatement.partOf", description="Returns statements that are part of another event.", type="reference", target={MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Observation.class, Procedure.class } ) 2030 public static final String SP_PART_OF = "part-of"; 2031 /** 2032 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2033 * <p> 2034 * Description: <b>Returns statements that are part of another event.</b><br> 2035 * Type: <b>reference</b><br> 2036 * Path: <b>MedicationStatement.partOf</b><br> 2037 * </p> 2038 */ 2039 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2040 2041/** 2042 * Constant for fluent queries to be used to add include statements. Specifies 2043 * the path value of "<b>MedicationStatement:part-of</b>". 2044 */ 2045 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("MedicationStatement:part-of").toLocked(); 2046 2047 /** 2048 * Search parameter: <b>source</b> 2049 * <p> 2050 * Description: <b>Who or where the information in the statement came from</b><br> 2051 * Type: <b>reference</b><br> 2052 * Path: <b>MedicationStatement.informationSource</b><br> 2053 * </p> 2054 */ 2055 @SearchParamDefinition(name="source", path="MedicationStatement.informationSource", description="Who or where the information in the statement came from", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2056 public static final String SP_SOURCE = "source"; 2057 /** 2058 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2059 * <p> 2060 * Description: <b>Who or where the information in the statement came from</b><br> 2061 * Type: <b>reference</b><br> 2062 * Path: <b>MedicationStatement.informationSource</b><br> 2063 * </p> 2064 */ 2065 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 2066 2067/** 2068 * Constant for fluent queries to be used to add include statements. Specifies 2069 * the path value of "<b>MedicationStatement:source</b>". 2070 */ 2071 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("MedicationStatement:source").toLocked(); 2072 2073 /** 2074 * Search parameter: <b>category</b> 2075 * <p> 2076 * Description: <b>Returns statements of this category of medicationstatement</b><br> 2077 * Type: <b>token</b><br> 2078 * Path: <b>MedicationStatement.category</b><br> 2079 * </p> 2080 */ 2081 @SearchParamDefinition(name="category", path="MedicationStatement.category", description="Returns statements of this category of medicationstatement", type="token" ) 2082 public static final String SP_CATEGORY = "category"; 2083 /** 2084 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2085 * <p> 2086 * Description: <b>Returns statements of this category of medicationstatement</b><br> 2087 * Type: <b>token</b><br> 2088 * Path: <b>MedicationStatement.category</b><br> 2089 * </p> 2090 */ 2091 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2092 2093 /** 2094 * Search parameter: <b>status</b> 2095 * <p> 2096 * Description: <b>Return statements that match the given status</b><br> 2097 * Type: <b>token</b><br> 2098 * Path: <b>MedicationStatement.status</b><br> 2099 * </p> 2100 */ 2101 @SearchParamDefinition(name="status", path="MedicationStatement.status", description="Return statements that match the given status", type="token" ) 2102 public static final String SP_STATUS = "status"; 2103 /** 2104 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2105 * <p> 2106 * Description: <b>Return statements that match the given status</b><br> 2107 * Type: <b>token</b><br> 2108 * Path: <b>MedicationStatement.status</b><br> 2109 * </p> 2110 */ 2111 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2112 2113 2114}