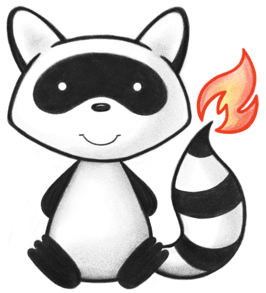
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.exceptions.FHIRException; 040import org.hl7.fhir.exceptions.FHIRFormatError; 041 042import ca.uhn.fhir.model.api.annotation.Child; 043import ca.uhn.fhir.model.api.annotation.Description; 044import ca.uhn.fhir.model.api.annotation.ResourceDef; 045import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 046/** 047 * A record of a medication that is being consumed by a patient. A MedicationStatement may indicate that the patient may be taking the medication now, or has taken the medication in the past or will be taking the medication in the future. The source of this information can be the patient, significant other (such as a family member or spouse), or a clinician. A common scenario where this information is captured is during the history taking process during a patient visit or stay. The medication information may come from sources such as the patient's memory, from a prescription bottle, or from a list of medications the patient, clinician or other party maintains 048 049The primary difference between a medication statement and a medication administration is that the medication administration has complete administration information and is based on actual administration information from the person who administered the medication. A medication statement is often, if not always, less specific. There is no required date/time when the medication was administered, in fact we only know that a source has reported the patient is taking this medication, where details such as time, quantity, or rate or even medication product may be incomplete or missing or less precise. As stated earlier, the medication statement information may come from the patient's memory, from a prescription bottle or from a list of medications the patient, clinician or other party maintains. Medication administration is more formal and is not missing detailed information. 050 */ 051@ResourceDef(name="MedicationStatement", profile="http://hl7.org/fhir/Profile/MedicationStatement") 052public class MedicationStatement extends DomainResource { 053 054 public enum MedicationStatementStatus { 055 /** 056 * The medication is still being taken. 057 */ 058 ACTIVE, 059 /** 060 * The medication is no longer being taken. 061 */ 062 COMPLETED, 063 /** 064 * The statement was recorded incorrectly. 065 */ 066 ENTEREDINERROR, 067 /** 068 * The medication may be taken at some time in the future. 069 */ 070 INTENDED, 071 /** 072 * Actions implied by the statement have been permanently halted, before all of them occurred. 073 */ 074 STOPPED, 075 /** 076 * Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called "suspended". 077 */ 078 ONHOLD, 079 /** 080 * added to help the parsers with the generic types 081 */ 082 NULL; 083 public static MedicationStatementStatus fromCode(String codeString) throws FHIRException { 084 if (codeString == null || "".equals(codeString)) 085 return null; 086 if ("active".equals(codeString)) 087 return ACTIVE; 088 if ("completed".equals(codeString)) 089 return COMPLETED; 090 if ("entered-in-error".equals(codeString)) 091 return ENTEREDINERROR; 092 if ("intended".equals(codeString)) 093 return INTENDED; 094 if ("stopped".equals(codeString)) 095 return STOPPED; 096 if ("on-hold".equals(codeString)) 097 return ONHOLD; 098 if (Configuration.isAcceptInvalidEnums()) 099 return null; 100 else 101 throw new FHIRException("Unknown MedicationStatementStatus code '"+codeString+"'"); 102 } 103 public String toCode() { 104 switch (this) { 105 case ACTIVE: return "active"; 106 case COMPLETED: return "completed"; 107 case ENTEREDINERROR: return "entered-in-error"; 108 case INTENDED: return "intended"; 109 case STOPPED: return "stopped"; 110 case ONHOLD: return "on-hold"; 111 case NULL: return null; 112 default: return "?"; 113 } 114 } 115 public String getSystem() { 116 switch (this) { 117 case ACTIVE: return "http://hl7.org/fhir/medication-statement-status"; 118 case COMPLETED: return "http://hl7.org/fhir/medication-statement-status"; 119 case ENTEREDINERROR: return "http://hl7.org/fhir/medication-statement-status"; 120 case INTENDED: return "http://hl7.org/fhir/medication-statement-status"; 121 case STOPPED: return "http://hl7.org/fhir/medication-statement-status"; 122 case ONHOLD: return "http://hl7.org/fhir/medication-statement-status"; 123 case NULL: return null; 124 default: return "?"; 125 } 126 } 127 public String getDefinition() { 128 switch (this) { 129 case ACTIVE: return "The medication is still being taken."; 130 case COMPLETED: return "The medication is no longer being taken."; 131 case ENTEREDINERROR: return "The statement was recorded incorrectly."; 132 case INTENDED: return "The medication may be taken at some time in the future."; 133 case STOPPED: return "Actions implied by the statement have been permanently halted, before all of them occurred."; 134 case ONHOLD: return "Actions implied by the statement have been temporarily halted, but are expected to continue later. May also be called \"suspended\"."; 135 case NULL: return null; 136 default: return "?"; 137 } 138 } 139 public String getDisplay() { 140 switch (this) { 141 case ACTIVE: return "Active"; 142 case COMPLETED: return "Completed"; 143 case ENTEREDINERROR: return "Entered in Error"; 144 case INTENDED: return "Intended"; 145 case STOPPED: return "Stopped"; 146 case ONHOLD: return "On Hold"; 147 case NULL: return null; 148 default: return "?"; 149 } 150 } 151 } 152 153 public static class MedicationStatementStatusEnumFactory implements EnumFactory<MedicationStatementStatus> { 154 public MedicationStatementStatus fromCode(String codeString) throws IllegalArgumentException { 155 if (codeString == null || "".equals(codeString)) 156 if (codeString == null || "".equals(codeString)) 157 return null; 158 if ("active".equals(codeString)) 159 return MedicationStatementStatus.ACTIVE; 160 if ("completed".equals(codeString)) 161 return MedicationStatementStatus.COMPLETED; 162 if ("entered-in-error".equals(codeString)) 163 return MedicationStatementStatus.ENTEREDINERROR; 164 if ("intended".equals(codeString)) 165 return MedicationStatementStatus.INTENDED; 166 if ("stopped".equals(codeString)) 167 return MedicationStatementStatus.STOPPED; 168 if ("on-hold".equals(codeString)) 169 return MedicationStatementStatus.ONHOLD; 170 throw new IllegalArgumentException("Unknown MedicationStatementStatus code '"+codeString+"'"); 171 } 172 public Enumeration<MedicationStatementStatus> fromType(PrimitiveType<?> code) throws FHIRException { 173 if (code == null) 174 return null; 175 if (code.isEmpty()) 176 return new Enumeration<MedicationStatementStatus>(this); 177 String codeString = code.asStringValue(); 178 if (codeString == null || "".equals(codeString)) 179 return null; 180 if ("active".equals(codeString)) 181 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ACTIVE); 182 if ("completed".equals(codeString)) 183 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.COMPLETED); 184 if ("entered-in-error".equals(codeString)) 185 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ENTEREDINERROR); 186 if ("intended".equals(codeString)) 187 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.INTENDED); 188 if ("stopped".equals(codeString)) 189 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.STOPPED); 190 if ("on-hold".equals(codeString)) 191 return new Enumeration<MedicationStatementStatus>(this, MedicationStatementStatus.ONHOLD); 192 throw new FHIRException("Unknown MedicationStatementStatus code '"+codeString+"'"); 193 } 194 public String toCode(MedicationStatementStatus code) { 195 if (code == MedicationStatementStatus.ACTIVE) 196 return "active"; 197 if (code == MedicationStatementStatus.COMPLETED) 198 return "completed"; 199 if (code == MedicationStatementStatus.ENTEREDINERROR) 200 return "entered-in-error"; 201 if (code == MedicationStatementStatus.INTENDED) 202 return "intended"; 203 if (code == MedicationStatementStatus.STOPPED) 204 return "stopped"; 205 if (code == MedicationStatementStatus.ONHOLD) 206 return "on-hold"; 207 return "?"; 208 } 209 public String toSystem(MedicationStatementStatus code) { 210 return code.getSystem(); 211 } 212 } 213 214 public enum MedicationStatementTaken { 215 /** 216 * Positive assertion that patient has taken medication 217 */ 218 Y, 219 /** 220 * Negative assertion that patient has not taken medication 221 */ 222 N, 223 /** 224 * Unknown assertion if patient has taken medication 225 */ 226 UNK, 227 /** 228 * Patient reporting does not apply 229 */ 230 NA, 231 /** 232 * added to help the parsers with the generic types 233 */ 234 NULL; 235 public static MedicationStatementTaken fromCode(String codeString) throws FHIRException { 236 if (codeString == null || "".equals(codeString)) 237 return null; 238 if ("y".equals(codeString)) 239 return Y; 240 if ("n".equals(codeString)) 241 return N; 242 if ("unk".equals(codeString)) 243 return UNK; 244 if ("na".equals(codeString)) 245 return NA; 246 if (Configuration.isAcceptInvalidEnums()) 247 return null; 248 else 249 throw new FHIRException("Unknown MedicationStatementTaken code '"+codeString+"'"); 250 } 251 public String toCode() { 252 switch (this) { 253 case Y: return "y"; 254 case N: return "n"; 255 case UNK: return "unk"; 256 case NA: return "na"; 257 case NULL: return null; 258 default: return "?"; 259 } 260 } 261 public String getSystem() { 262 switch (this) { 263 case Y: return "http://hl7.org/fhir/medication-statement-taken"; 264 case N: return "http://hl7.org/fhir/medication-statement-taken"; 265 case UNK: return "http://hl7.org/fhir/medication-statement-taken"; 266 case NA: return "http://hl7.org/fhir/medication-statement-taken"; 267 case NULL: return null; 268 default: return "?"; 269 } 270 } 271 public String getDefinition() { 272 switch (this) { 273 case Y: return "Positive assertion that patient has taken medication"; 274 case N: return "Negative assertion that patient has not taken medication"; 275 case UNK: return "Unknown assertion if patient has taken medication"; 276 case NA: return "Patient reporting does not apply"; 277 case NULL: return null; 278 default: return "?"; 279 } 280 } 281 public String getDisplay() { 282 switch (this) { 283 case Y: return "Yes"; 284 case N: return "No"; 285 case UNK: return "Unknown"; 286 case NA: return "Not Applicable"; 287 case NULL: return null; 288 default: return "?"; 289 } 290 } 291 } 292 293 public static class MedicationStatementTakenEnumFactory implements EnumFactory<MedicationStatementTaken> { 294 public MedicationStatementTaken fromCode(String codeString) throws IllegalArgumentException { 295 if (codeString == null || "".equals(codeString)) 296 if (codeString == null || "".equals(codeString)) 297 return null; 298 if ("y".equals(codeString)) 299 return MedicationStatementTaken.Y; 300 if ("n".equals(codeString)) 301 return MedicationStatementTaken.N; 302 if ("unk".equals(codeString)) 303 return MedicationStatementTaken.UNK; 304 if ("na".equals(codeString)) 305 return MedicationStatementTaken.NA; 306 throw new IllegalArgumentException("Unknown MedicationStatementTaken code '"+codeString+"'"); 307 } 308 public Enumeration<MedicationStatementTaken> fromType(PrimitiveType<?> code) throws FHIRException { 309 if (code == null) 310 return null; 311 if (code.isEmpty()) 312 return new Enumeration<MedicationStatementTaken>(this); 313 String codeString = code.asStringValue(); 314 if (codeString == null || "".equals(codeString)) 315 return null; 316 if ("y".equals(codeString)) 317 return new Enumeration<MedicationStatementTaken>(this, MedicationStatementTaken.Y); 318 if ("n".equals(codeString)) 319 return new Enumeration<MedicationStatementTaken>(this, MedicationStatementTaken.N); 320 if ("unk".equals(codeString)) 321 return new Enumeration<MedicationStatementTaken>(this, MedicationStatementTaken.UNK); 322 if ("na".equals(codeString)) 323 return new Enumeration<MedicationStatementTaken>(this, MedicationStatementTaken.NA); 324 throw new FHIRException("Unknown MedicationStatementTaken code '"+codeString+"'"); 325 } 326 public String toCode(MedicationStatementTaken code) { 327 if (code == MedicationStatementTaken.Y) 328 return "y"; 329 if (code == MedicationStatementTaken.N) 330 return "n"; 331 if (code == MedicationStatementTaken.UNK) 332 return "unk"; 333 if (code == MedicationStatementTaken.NA) 334 return "na"; 335 return "?"; 336 } 337 public String toSystem(MedicationStatementTaken code) { 338 return code.getSystem(); 339 } 340 } 341 342 /** 343 * External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated. 344 */ 345 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 346 @Description(shortDefinition="External identifier", formalDefinition="External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated." ) 347 protected List<Identifier> identifier; 348 349 /** 350 * A plan, proposal or order that is fulfilled in whole or in part by this event. 351 */ 352 @Child(name = "basedOn", type = {MedicationRequest.class, CarePlan.class, ProcedureRequest.class, ReferralRequest.class}, order=1, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 353 @Description(shortDefinition="Fulfils plan, proposal or order", formalDefinition="A plan, proposal or order that is fulfilled in whole or in part by this event." ) 354 protected List<Reference> basedOn; 355 /** 356 * The actual objects that are the target of the reference (A plan, proposal or order that is fulfilled in whole or in part by this event.) 357 */ 358 protected List<Resource> basedOnTarget; 359 360 361 /** 362 * A larger event of which this particular event is a component or step. 363 */ 364 @Child(name = "partOf", type = {MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Procedure.class, Observation.class}, order=2, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 365 @Description(shortDefinition="Part of referenced event", formalDefinition="A larger event of which this particular event is a component or step." ) 366 protected List<Reference> partOf; 367 /** 368 * The actual objects that are the target of the reference (A larger event of which this particular event is a component or step.) 369 */ 370 protected List<Resource> partOfTarget; 371 372 373 /** 374 * The encounter or episode of care that establishes the context for this MedicationStatement. 375 */ 376 @Child(name = "context", type = {Encounter.class, EpisodeOfCare.class}, order=3, min=0, max=1, modifier=false, summary=true) 377 @Description(shortDefinition="Encounter / Episode associated with MedicationStatement", formalDefinition="The encounter or episode of care that establishes the context for this MedicationStatement." ) 378 protected Reference context; 379 380 /** 381 * The actual object that is the target of the reference (The encounter or episode of care that establishes the context for this MedicationStatement.) 382 */ 383 protected Resource contextTarget; 384 385 /** 386 * A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed. 387 */ 388 @Child(name = "status", type = {CodeType.class}, order=4, min=1, max=1, modifier=true, summary=true) 389 @Description(shortDefinition="active | completed | entered-in-error | intended | stopped | on-hold", formalDefinition="A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed." ) 390 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-status") 391 protected Enumeration<MedicationStatementStatus> status; 392 393 /** 394 * Indicates where type of medication statement and where the medication is expected to be consumed or administered. 395 */ 396 @Child(name = "category", type = {CodeableConcept.class}, order=5, min=0, max=1, modifier=false, summary=true) 397 @Description(shortDefinition="Type of medication usage", formalDefinition="Indicates where type of medication statement and where the medication is expected to be consumed or administered." ) 398 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-category") 399 protected CodeableConcept category; 400 401 /** 402 * Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications. 403 */ 404 @Child(name = "medication", type = {CodeableConcept.class, Medication.class}, order=6, min=1, max=1, modifier=false, summary=true) 405 @Description(shortDefinition="What medication was taken", formalDefinition="Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications." ) 406 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-codes") 407 protected Type medication; 408 409 /** 410 * The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true). 411 */ 412 @Child(name = "effective", type = {DateTimeType.class, Period.class}, order=7, min=0, max=1, modifier=false, summary=true) 413 @Description(shortDefinition="The date/time or interval when the medication was taken", formalDefinition="The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true)." ) 414 protected Type effective; 415 416 /** 417 * The date when the medication statement was asserted by the information source. 418 */ 419 @Child(name = "dateAsserted", type = {DateTimeType.class}, order=8, min=0, max=1, modifier=false, summary=true) 420 @Description(shortDefinition="When the statement was asserted?", formalDefinition="The date when the medication statement was asserted by the information source." ) 421 protected DateTimeType dateAsserted; 422 423 /** 424 * The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest. 425 */ 426 @Child(name = "informationSource", type = {Patient.class, Practitioner.class, RelatedPerson.class, Organization.class}, order=9, min=0, max=1, modifier=false, summary=false) 427 @Description(shortDefinition="Person or organization that provided the information about the taking of this medication", formalDefinition="The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest." ) 428 protected Reference informationSource; 429 430 /** 431 * The actual object that is the target of the reference (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 432 */ 433 protected Resource informationSourceTarget; 434 435 /** 436 * The person, animal or group who is/was taking the medication. 437 */ 438 @Child(name = "subject", type = {Patient.class, Group.class}, order=10, min=1, max=1, modifier=false, summary=true) 439 @Description(shortDefinition="Who is/was taking the medication", formalDefinition="The person, animal or group who is/was taking the medication." ) 440 protected Reference subject; 441 442 /** 443 * The actual object that is the target of the reference (The person, animal or group who is/was taking the medication.) 444 */ 445 protected Resource subjectTarget; 446 447 /** 448 * Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement. 449 */ 450 @Child(name = "derivedFrom", type = {Reference.class}, order=11, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 451 @Description(shortDefinition="Additional supporting information", formalDefinition="Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement." ) 452 protected List<Reference> derivedFrom; 453 /** 454 * The actual objects that are the target of the reference (Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.) 455 */ 456 protected List<Resource> derivedFromTarget; 457 458 459 /** 460 * Indicator of the certainty of whether the medication was taken by the patient. 461 */ 462 @Child(name = "taken", type = {CodeType.class}, order=12, min=1, max=1, modifier=true, summary=true) 463 @Description(shortDefinition="y | n | unk | na", formalDefinition="Indicator of the certainty of whether the medication was taken by the patient." ) 464 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/medication-statement-taken") 465 protected Enumeration<MedicationStatementTaken> taken; 466 467 /** 468 * A code indicating why the medication was not taken. 469 */ 470 @Child(name = "reasonNotTaken", type = {CodeableConcept.class}, order=13, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 471 @Description(shortDefinition="True if asserting medication was not given", formalDefinition="A code indicating why the medication was not taken." ) 472 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/reason-medication-not-taken-codes") 473 protected List<CodeableConcept> reasonNotTaken; 474 475 /** 476 * A reason for why the medication is being/was taken. 477 */ 478 @Child(name = "reasonCode", type = {CodeableConcept.class}, order=14, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 479 @Description(shortDefinition="Reason for why the medication is being/was taken", formalDefinition="A reason for why the medication is being/was taken." ) 480 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/condition-code") 481 protected List<CodeableConcept> reasonCode; 482 483 /** 484 * Condition or observation that supports why the medication is being/was taken. 485 */ 486 @Child(name = "reasonReference", type = {Condition.class, Observation.class}, order=15, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 487 @Description(shortDefinition="Condition or observation that supports why the medication is being/was taken", formalDefinition="Condition or observation that supports why the medication is being/was taken." ) 488 protected List<Reference> reasonReference; 489 /** 490 * The actual objects that are the target of the reference (Condition or observation that supports why the medication is being/was taken.) 491 */ 492 protected List<Resource> reasonReferenceTarget; 493 494 495 /** 496 * Provides extra information about the medication statement that is not conveyed by the other attributes. 497 */ 498 @Child(name = "note", type = {Annotation.class}, order=16, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 499 @Description(shortDefinition="Further information about the statement", formalDefinition="Provides extra information about the medication statement that is not conveyed by the other attributes." ) 500 protected List<Annotation> note; 501 502 /** 503 * Indicates how the medication is/was or should be taken by the patient. 504 */ 505 @Child(name = "dosage", type = {Dosage.class}, order=17, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 506 @Description(shortDefinition="Details of how medication is/was taken or should be taken", formalDefinition="Indicates how the medication is/was or should be taken by the patient." ) 507 protected List<Dosage> dosage; 508 509 private static final long serialVersionUID = -1529480075L; 510 511 /** 512 * Constructor 513 */ 514 public MedicationStatement() { 515 super(); 516 } 517 518 /** 519 * Constructor 520 */ 521 public MedicationStatement(Enumeration<MedicationStatementStatus> status, Type medication, Reference subject, Enumeration<MedicationStatementTaken> taken) { 522 super(); 523 this.status = status; 524 this.medication = medication; 525 this.subject = subject; 526 this.taken = taken; 527 } 528 529 /** 530 * @return {@link #identifier} (External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.) 531 */ 532 public List<Identifier> getIdentifier() { 533 if (this.identifier == null) 534 this.identifier = new ArrayList<Identifier>(); 535 return this.identifier; 536 } 537 538 /** 539 * @return Returns a reference to <code>this</code> for easy method chaining 540 */ 541 public MedicationStatement setIdentifier(List<Identifier> theIdentifier) { 542 this.identifier = theIdentifier; 543 return this; 544 } 545 546 public boolean hasIdentifier() { 547 if (this.identifier == null) 548 return false; 549 for (Identifier item : this.identifier) 550 if (!item.isEmpty()) 551 return true; 552 return false; 553 } 554 555 public Identifier addIdentifier() { //3 556 Identifier t = new Identifier(); 557 if (this.identifier == null) 558 this.identifier = new ArrayList<Identifier>(); 559 this.identifier.add(t); 560 return t; 561 } 562 563 public MedicationStatement addIdentifier(Identifier t) { //3 564 if (t == null) 565 return this; 566 if (this.identifier == null) 567 this.identifier = new ArrayList<Identifier>(); 568 this.identifier.add(t); 569 return this; 570 } 571 572 /** 573 * @return The first repetition of repeating field {@link #identifier}, creating it if it does not already exist 574 */ 575 public Identifier getIdentifierFirstRep() { 576 if (getIdentifier().isEmpty()) { 577 addIdentifier(); 578 } 579 return getIdentifier().get(0); 580 } 581 582 /** 583 * @return {@link #basedOn} (A plan, proposal or order that is fulfilled in whole or in part by this event.) 584 */ 585 public List<Reference> getBasedOn() { 586 if (this.basedOn == null) 587 this.basedOn = new ArrayList<Reference>(); 588 return this.basedOn; 589 } 590 591 /** 592 * @return Returns a reference to <code>this</code> for easy method chaining 593 */ 594 public MedicationStatement setBasedOn(List<Reference> theBasedOn) { 595 this.basedOn = theBasedOn; 596 return this; 597 } 598 599 public boolean hasBasedOn() { 600 if (this.basedOn == null) 601 return false; 602 for (Reference item : this.basedOn) 603 if (!item.isEmpty()) 604 return true; 605 return false; 606 } 607 608 public Reference addBasedOn() { //3 609 Reference t = new Reference(); 610 if (this.basedOn == null) 611 this.basedOn = new ArrayList<Reference>(); 612 this.basedOn.add(t); 613 return t; 614 } 615 616 public MedicationStatement addBasedOn(Reference t) { //3 617 if (t == null) 618 return this; 619 if (this.basedOn == null) 620 this.basedOn = new ArrayList<Reference>(); 621 this.basedOn.add(t); 622 return this; 623 } 624 625 /** 626 * @return The first repetition of repeating field {@link #basedOn}, creating it if it does not already exist 627 */ 628 public Reference getBasedOnFirstRep() { 629 if (getBasedOn().isEmpty()) { 630 addBasedOn(); 631 } 632 return getBasedOn().get(0); 633 } 634 635 /** 636 * @deprecated Use Reference#setResource(IBaseResource) instead 637 */ 638 @Deprecated 639 public List<Resource> getBasedOnTarget() { 640 if (this.basedOnTarget == null) 641 this.basedOnTarget = new ArrayList<Resource>(); 642 return this.basedOnTarget; 643 } 644 645 /** 646 * @return {@link #partOf} (A larger event of which this particular event is a component or step.) 647 */ 648 public List<Reference> getPartOf() { 649 if (this.partOf == null) 650 this.partOf = new ArrayList<Reference>(); 651 return this.partOf; 652 } 653 654 /** 655 * @return Returns a reference to <code>this</code> for easy method chaining 656 */ 657 public MedicationStatement setPartOf(List<Reference> thePartOf) { 658 this.partOf = thePartOf; 659 return this; 660 } 661 662 public boolean hasPartOf() { 663 if (this.partOf == null) 664 return false; 665 for (Reference item : this.partOf) 666 if (!item.isEmpty()) 667 return true; 668 return false; 669 } 670 671 public Reference addPartOf() { //3 672 Reference t = new Reference(); 673 if (this.partOf == null) 674 this.partOf = new ArrayList<Reference>(); 675 this.partOf.add(t); 676 return t; 677 } 678 679 public MedicationStatement addPartOf(Reference t) { //3 680 if (t == null) 681 return this; 682 if (this.partOf == null) 683 this.partOf = new ArrayList<Reference>(); 684 this.partOf.add(t); 685 return this; 686 } 687 688 /** 689 * @return The first repetition of repeating field {@link #partOf}, creating it if it does not already exist 690 */ 691 public Reference getPartOfFirstRep() { 692 if (getPartOf().isEmpty()) { 693 addPartOf(); 694 } 695 return getPartOf().get(0); 696 } 697 698 /** 699 * @deprecated Use Reference#setResource(IBaseResource) instead 700 */ 701 @Deprecated 702 public List<Resource> getPartOfTarget() { 703 if (this.partOfTarget == null) 704 this.partOfTarget = new ArrayList<Resource>(); 705 return this.partOfTarget; 706 } 707 708 /** 709 * @return {@link #context} (The encounter or episode of care that establishes the context for this MedicationStatement.) 710 */ 711 public Reference getContext() { 712 if (this.context == null) 713 if (Configuration.errorOnAutoCreate()) 714 throw new Error("Attempt to auto-create MedicationStatement.context"); 715 else if (Configuration.doAutoCreate()) 716 this.context = new Reference(); // cc 717 return this.context; 718 } 719 720 public boolean hasContext() { 721 return this.context != null && !this.context.isEmpty(); 722 } 723 724 /** 725 * @param value {@link #context} (The encounter or episode of care that establishes the context for this MedicationStatement.) 726 */ 727 public MedicationStatement setContext(Reference value) { 728 this.context = value; 729 return this; 730 } 731 732 /** 733 * @return {@link #context} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this MedicationStatement.) 734 */ 735 public Resource getContextTarget() { 736 return this.contextTarget; 737 } 738 739 /** 740 * @param value {@link #context} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The encounter or episode of care that establishes the context for this MedicationStatement.) 741 */ 742 public MedicationStatement setContextTarget(Resource value) { 743 this.contextTarget = value; 744 return this; 745 } 746 747 /** 748 * @return {@link #status} (A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 749 */ 750 public Enumeration<MedicationStatementStatus> getStatusElement() { 751 if (this.status == null) 752 if (Configuration.errorOnAutoCreate()) 753 throw new Error("Attempt to auto-create MedicationStatement.status"); 754 else if (Configuration.doAutoCreate()) 755 this.status = new Enumeration<MedicationStatementStatus>(new MedicationStatementStatusEnumFactory()); // bb 756 return this.status; 757 } 758 759 public boolean hasStatusElement() { 760 return this.status != null && !this.status.isEmpty(); 761 } 762 763 public boolean hasStatus() { 764 return this.status != null && !this.status.isEmpty(); 765 } 766 767 /** 768 * @param value {@link #status} (A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 769 */ 770 public MedicationStatement setStatusElement(Enumeration<MedicationStatementStatus> value) { 771 this.status = value; 772 return this; 773 } 774 775 /** 776 * @return A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed. 777 */ 778 public MedicationStatementStatus getStatus() { 779 return this.status == null ? null : this.status.getValue(); 780 } 781 782 /** 783 * @param value A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed. 784 */ 785 public MedicationStatement setStatus(MedicationStatementStatus value) { 786 if (this.status == null) 787 this.status = new Enumeration<MedicationStatementStatus>(new MedicationStatementStatusEnumFactory()); 788 this.status.setValue(value); 789 return this; 790 } 791 792 /** 793 * @return {@link #category} (Indicates where type of medication statement and where the medication is expected to be consumed or administered.) 794 */ 795 public CodeableConcept getCategory() { 796 if (this.category == null) 797 if (Configuration.errorOnAutoCreate()) 798 throw new Error("Attempt to auto-create MedicationStatement.category"); 799 else if (Configuration.doAutoCreate()) 800 this.category = new CodeableConcept(); // cc 801 return this.category; 802 } 803 804 public boolean hasCategory() { 805 return this.category != null && !this.category.isEmpty(); 806 } 807 808 /** 809 * @param value {@link #category} (Indicates where type of medication statement and where the medication is expected to be consumed or administered.) 810 */ 811 public MedicationStatement setCategory(CodeableConcept value) { 812 this.category = value; 813 return this; 814 } 815 816 /** 817 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 818 */ 819 public Type getMedication() { 820 return this.medication; 821 } 822 823 /** 824 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 825 */ 826 public CodeableConcept getMedicationCodeableConcept() throws FHIRException { 827 if (this.medication == null) 828 return null; 829 if (!(this.medication instanceof CodeableConcept)) 830 throw new FHIRException("Type mismatch: the type CodeableConcept was expected, but "+this.medication.getClass().getName()+" was encountered"); 831 return (CodeableConcept) this.medication; 832 } 833 834 public boolean hasMedicationCodeableConcept() { 835 return this != null && this.medication instanceof CodeableConcept; 836 } 837 838 /** 839 * @return {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 840 */ 841 public Reference getMedicationReference() throws FHIRException { 842 if (this.medication == null) 843 return null; 844 if (!(this.medication instanceof Reference)) 845 throw new FHIRException("Type mismatch: the type Reference was expected, but "+this.medication.getClass().getName()+" was encountered"); 846 return (Reference) this.medication; 847 } 848 849 public boolean hasMedicationReference() { 850 return this != null && this.medication instanceof Reference; 851 } 852 853 public boolean hasMedication() { 854 return this.medication != null && !this.medication.isEmpty(); 855 } 856 857 /** 858 * @param value {@link #medication} (Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.) 859 */ 860 public MedicationStatement setMedication(Type value) throws FHIRFormatError { 861 if (value != null && !(value instanceof CodeableConcept || value instanceof Reference)) 862 throw new FHIRFormatError("Not the right type for MedicationStatement.medication[x]: "+value.fhirType()); 863 this.medication = value; 864 return this; 865 } 866 867 /** 868 * @return {@link #effective} (The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).) 869 */ 870 public Type getEffective() { 871 return this.effective; 872 } 873 874 /** 875 * @return {@link #effective} (The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).) 876 */ 877 public DateTimeType getEffectiveDateTimeType() throws FHIRException { 878 if (this.effective == null) 879 return null; 880 if (!(this.effective instanceof DateTimeType)) 881 throw new FHIRException("Type mismatch: the type DateTimeType was expected, but "+this.effective.getClass().getName()+" was encountered"); 882 return (DateTimeType) this.effective; 883 } 884 885 public boolean hasEffectiveDateTimeType() { 886 return this != null && this.effective instanceof DateTimeType; 887 } 888 889 /** 890 * @return {@link #effective} (The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).) 891 */ 892 public Period getEffectivePeriod() throws FHIRException { 893 if (this.effective == null) 894 return null; 895 if (!(this.effective instanceof Period)) 896 throw new FHIRException("Type mismatch: the type Period was expected, but "+this.effective.getClass().getName()+" was encountered"); 897 return (Period) this.effective; 898 } 899 900 public boolean hasEffectivePeriod() { 901 return this != null && this.effective instanceof Period; 902 } 903 904 public boolean hasEffective() { 905 return this.effective != null && !this.effective.isEmpty(); 906 } 907 908 /** 909 * @param value {@link #effective} (The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).) 910 */ 911 public MedicationStatement setEffective(Type value) throws FHIRFormatError { 912 if (value != null && !(value instanceof DateTimeType || value instanceof Period)) 913 throw new FHIRFormatError("Not the right type for MedicationStatement.effective[x]: "+value.fhirType()); 914 this.effective = value; 915 return this; 916 } 917 918 /** 919 * @return {@link #dateAsserted} (The date when the medication statement was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 920 */ 921 public DateTimeType getDateAssertedElement() { 922 if (this.dateAsserted == null) 923 if (Configuration.errorOnAutoCreate()) 924 throw new Error("Attempt to auto-create MedicationStatement.dateAsserted"); 925 else if (Configuration.doAutoCreate()) 926 this.dateAsserted = new DateTimeType(); // bb 927 return this.dateAsserted; 928 } 929 930 public boolean hasDateAssertedElement() { 931 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 932 } 933 934 public boolean hasDateAsserted() { 935 return this.dateAsserted != null && !this.dateAsserted.isEmpty(); 936 } 937 938 /** 939 * @param value {@link #dateAsserted} (The date when the medication statement was asserted by the information source.). This is the underlying object with id, value and extensions. The accessor "getDateAsserted" gives direct access to the value 940 */ 941 public MedicationStatement setDateAssertedElement(DateTimeType value) { 942 this.dateAsserted = value; 943 return this; 944 } 945 946 /** 947 * @return The date when the medication statement was asserted by the information source. 948 */ 949 public Date getDateAsserted() { 950 return this.dateAsserted == null ? null : this.dateAsserted.getValue(); 951 } 952 953 /** 954 * @param value The date when the medication statement was asserted by the information source. 955 */ 956 public MedicationStatement setDateAsserted(Date value) { 957 if (value == null) 958 this.dateAsserted = null; 959 else { 960 if (this.dateAsserted == null) 961 this.dateAsserted = new DateTimeType(); 962 this.dateAsserted.setValue(value); 963 } 964 return this; 965 } 966 967 /** 968 * @return {@link #informationSource} (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 969 */ 970 public Reference getInformationSource() { 971 if (this.informationSource == null) 972 if (Configuration.errorOnAutoCreate()) 973 throw new Error("Attempt to auto-create MedicationStatement.informationSource"); 974 else if (Configuration.doAutoCreate()) 975 this.informationSource = new Reference(); // cc 976 return this.informationSource; 977 } 978 979 public boolean hasInformationSource() { 980 return this.informationSource != null && !this.informationSource.isEmpty(); 981 } 982 983 /** 984 * @param value {@link #informationSource} (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 985 */ 986 public MedicationStatement setInformationSource(Reference value) { 987 this.informationSource = value; 988 return this; 989 } 990 991 /** 992 * @return {@link #informationSource} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 993 */ 994 public Resource getInformationSourceTarget() { 995 return this.informationSourceTarget; 996 } 997 998 /** 999 * @param value {@link #informationSource} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.) 1000 */ 1001 public MedicationStatement setInformationSourceTarget(Resource value) { 1002 this.informationSourceTarget = value; 1003 return this; 1004 } 1005 1006 /** 1007 * @return {@link #subject} (The person, animal or group who is/was taking the medication.) 1008 */ 1009 public Reference getSubject() { 1010 if (this.subject == null) 1011 if (Configuration.errorOnAutoCreate()) 1012 throw new Error("Attempt to auto-create MedicationStatement.subject"); 1013 else if (Configuration.doAutoCreate()) 1014 this.subject = new Reference(); // cc 1015 return this.subject; 1016 } 1017 1018 public boolean hasSubject() { 1019 return this.subject != null && !this.subject.isEmpty(); 1020 } 1021 1022 /** 1023 * @param value {@link #subject} (The person, animal or group who is/was taking the medication.) 1024 */ 1025 public MedicationStatement setSubject(Reference value) { 1026 this.subject = value; 1027 return this; 1028 } 1029 1030 /** 1031 * @return {@link #subject} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The person, animal or group who is/was taking the medication.) 1032 */ 1033 public Resource getSubjectTarget() { 1034 return this.subjectTarget; 1035 } 1036 1037 /** 1038 * @param value {@link #subject} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The person, animal or group who is/was taking the medication.) 1039 */ 1040 public MedicationStatement setSubjectTarget(Resource value) { 1041 this.subjectTarget = value; 1042 return this; 1043 } 1044 1045 /** 1046 * @return {@link #derivedFrom} (Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.) 1047 */ 1048 public List<Reference> getDerivedFrom() { 1049 if (this.derivedFrom == null) 1050 this.derivedFrom = new ArrayList<Reference>(); 1051 return this.derivedFrom; 1052 } 1053 1054 /** 1055 * @return Returns a reference to <code>this</code> for easy method chaining 1056 */ 1057 public MedicationStatement setDerivedFrom(List<Reference> theDerivedFrom) { 1058 this.derivedFrom = theDerivedFrom; 1059 return this; 1060 } 1061 1062 public boolean hasDerivedFrom() { 1063 if (this.derivedFrom == null) 1064 return false; 1065 for (Reference item : this.derivedFrom) 1066 if (!item.isEmpty()) 1067 return true; 1068 return false; 1069 } 1070 1071 public Reference addDerivedFrom() { //3 1072 Reference t = new Reference(); 1073 if (this.derivedFrom == null) 1074 this.derivedFrom = new ArrayList<Reference>(); 1075 this.derivedFrom.add(t); 1076 return t; 1077 } 1078 1079 public MedicationStatement addDerivedFrom(Reference t) { //3 1080 if (t == null) 1081 return this; 1082 if (this.derivedFrom == null) 1083 this.derivedFrom = new ArrayList<Reference>(); 1084 this.derivedFrom.add(t); 1085 return this; 1086 } 1087 1088 /** 1089 * @return The first repetition of repeating field {@link #derivedFrom}, creating it if it does not already exist 1090 */ 1091 public Reference getDerivedFromFirstRep() { 1092 if (getDerivedFrom().isEmpty()) { 1093 addDerivedFrom(); 1094 } 1095 return getDerivedFrom().get(0); 1096 } 1097 1098 /** 1099 * @deprecated Use Reference#setResource(IBaseResource) instead 1100 */ 1101 @Deprecated 1102 public List<Resource> getDerivedFromTarget() { 1103 if (this.derivedFromTarget == null) 1104 this.derivedFromTarget = new ArrayList<Resource>(); 1105 return this.derivedFromTarget; 1106 } 1107 1108 /** 1109 * @return {@link #taken} (Indicator of the certainty of whether the medication was taken by the patient.). This is the underlying object with id, value and extensions. The accessor "getTaken" gives direct access to the value 1110 */ 1111 public Enumeration<MedicationStatementTaken> getTakenElement() { 1112 if (this.taken == null) 1113 if (Configuration.errorOnAutoCreate()) 1114 throw new Error("Attempt to auto-create MedicationStatement.taken"); 1115 else if (Configuration.doAutoCreate()) 1116 this.taken = new Enumeration<MedicationStatementTaken>(new MedicationStatementTakenEnumFactory()); // bb 1117 return this.taken; 1118 } 1119 1120 public boolean hasTakenElement() { 1121 return this.taken != null && !this.taken.isEmpty(); 1122 } 1123 1124 public boolean hasTaken() { 1125 return this.taken != null && !this.taken.isEmpty(); 1126 } 1127 1128 /** 1129 * @param value {@link #taken} (Indicator of the certainty of whether the medication was taken by the patient.). This is the underlying object with id, value and extensions. The accessor "getTaken" gives direct access to the value 1130 */ 1131 public MedicationStatement setTakenElement(Enumeration<MedicationStatementTaken> value) { 1132 this.taken = value; 1133 return this; 1134 } 1135 1136 /** 1137 * @return Indicator of the certainty of whether the medication was taken by the patient. 1138 */ 1139 public MedicationStatementTaken getTaken() { 1140 return this.taken == null ? null : this.taken.getValue(); 1141 } 1142 1143 /** 1144 * @param value Indicator of the certainty of whether the medication was taken by the patient. 1145 */ 1146 public MedicationStatement setTaken(MedicationStatementTaken value) { 1147 if (this.taken == null) 1148 this.taken = new Enumeration<MedicationStatementTaken>(new MedicationStatementTakenEnumFactory()); 1149 this.taken.setValue(value); 1150 return this; 1151 } 1152 1153 /** 1154 * @return {@link #reasonNotTaken} (A code indicating why the medication was not taken.) 1155 */ 1156 public List<CodeableConcept> getReasonNotTaken() { 1157 if (this.reasonNotTaken == null) 1158 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1159 return this.reasonNotTaken; 1160 } 1161 1162 /** 1163 * @return Returns a reference to <code>this</code> for easy method chaining 1164 */ 1165 public MedicationStatement setReasonNotTaken(List<CodeableConcept> theReasonNotTaken) { 1166 this.reasonNotTaken = theReasonNotTaken; 1167 return this; 1168 } 1169 1170 public boolean hasReasonNotTaken() { 1171 if (this.reasonNotTaken == null) 1172 return false; 1173 for (CodeableConcept item : this.reasonNotTaken) 1174 if (!item.isEmpty()) 1175 return true; 1176 return false; 1177 } 1178 1179 public CodeableConcept addReasonNotTaken() { //3 1180 CodeableConcept t = new CodeableConcept(); 1181 if (this.reasonNotTaken == null) 1182 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1183 this.reasonNotTaken.add(t); 1184 return t; 1185 } 1186 1187 public MedicationStatement addReasonNotTaken(CodeableConcept t) { //3 1188 if (t == null) 1189 return this; 1190 if (this.reasonNotTaken == null) 1191 this.reasonNotTaken = new ArrayList<CodeableConcept>(); 1192 this.reasonNotTaken.add(t); 1193 return this; 1194 } 1195 1196 /** 1197 * @return The first repetition of repeating field {@link #reasonNotTaken}, creating it if it does not already exist 1198 */ 1199 public CodeableConcept getReasonNotTakenFirstRep() { 1200 if (getReasonNotTaken().isEmpty()) { 1201 addReasonNotTaken(); 1202 } 1203 return getReasonNotTaken().get(0); 1204 } 1205 1206 /** 1207 * @return {@link #reasonCode} (A reason for why the medication is being/was taken.) 1208 */ 1209 public List<CodeableConcept> getReasonCode() { 1210 if (this.reasonCode == null) 1211 this.reasonCode = new ArrayList<CodeableConcept>(); 1212 return this.reasonCode; 1213 } 1214 1215 /** 1216 * @return Returns a reference to <code>this</code> for easy method chaining 1217 */ 1218 public MedicationStatement setReasonCode(List<CodeableConcept> theReasonCode) { 1219 this.reasonCode = theReasonCode; 1220 return this; 1221 } 1222 1223 public boolean hasReasonCode() { 1224 if (this.reasonCode == null) 1225 return false; 1226 for (CodeableConcept item : this.reasonCode) 1227 if (!item.isEmpty()) 1228 return true; 1229 return false; 1230 } 1231 1232 public CodeableConcept addReasonCode() { //3 1233 CodeableConcept t = new CodeableConcept(); 1234 if (this.reasonCode == null) 1235 this.reasonCode = new ArrayList<CodeableConcept>(); 1236 this.reasonCode.add(t); 1237 return t; 1238 } 1239 1240 public MedicationStatement addReasonCode(CodeableConcept t) { //3 1241 if (t == null) 1242 return this; 1243 if (this.reasonCode == null) 1244 this.reasonCode = new ArrayList<CodeableConcept>(); 1245 this.reasonCode.add(t); 1246 return this; 1247 } 1248 1249 /** 1250 * @return The first repetition of repeating field {@link #reasonCode}, creating it if it does not already exist 1251 */ 1252 public CodeableConcept getReasonCodeFirstRep() { 1253 if (getReasonCode().isEmpty()) { 1254 addReasonCode(); 1255 } 1256 return getReasonCode().get(0); 1257 } 1258 1259 /** 1260 * @return {@link #reasonReference} (Condition or observation that supports why the medication is being/was taken.) 1261 */ 1262 public List<Reference> getReasonReference() { 1263 if (this.reasonReference == null) 1264 this.reasonReference = new ArrayList<Reference>(); 1265 return this.reasonReference; 1266 } 1267 1268 /** 1269 * @return Returns a reference to <code>this</code> for easy method chaining 1270 */ 1271 public MedicationStatement setReasonReference(List<Reference> theReasonReference) { 1272 this.reasonReference = theReasonReference; 1273 return this; 1274 } 1275 1276 public boolean hasReasonReference() { 1277 if (this.reasonReference == null) 1278 return false; 1279 for (Reference item : this.reasonReference) 1280 if (!item.isEmpty()) 1281 return true; 1282 return false; 1283 } 1284 1285 public Reference addReasonReference() { //3 1286 Reference t = new Reference(); 1287 if (this.reasonReference == null) 1288 this.reasonReference = new ArrayList<Reference>(); 1289 this.reasonReference.add(t); 1290 return t; 1291 } 1292 1293 public MedicationStatement addReasonReference(Reference t) { //3 1294 if (t == null) 1295 return this; 1296 if (this.reasonReference == null) 1297 this.reasonReference = new ArrayList<Reference>(); 1298 this.reasonReference.add(t); 1299 return this; 1300 } 1301 1302 /** 1303 * @return The first repetition of repeating field {@link #reasonReference}, creating it if it does not already exist 1304 */ 1305 public Reference getReasonReferenceFirstRep() { 1306 if (getReasonReference().isEmpty()) { 1307 addReasonReference(); 1308 } 1309 return getReasonReference().get(0); 1310 } 1311 1312 /** 1313 * @deprecated Use Reference#setResource(IBaseResource) instead 1314 */ 1315 @Deprecated 1316 public List<Resource> getReasonReferenceTarget() { 1317 if (this.reasonReferenceTarget == null) 1318 this.reasonReferenceTarget = new ArrayList<Resource>(); 1319 return this.reasonReferenceTarget; 1320 } 1321 1322 /** 1323 * @return {@link #note} (Provides extra information about the medication statement that is not conveyed by the other attributes.) 1324 */ 1325 public List<Annotation> getNote() { 1326 if (this.note == null) 1327 this.note = new ArrayList<Annotation>(); 1328 return this.note; 1329 } 1330 1331 /** 1332 * @return Returns a reference to <code>this</code> for easy method chaining 1333 */ 1334 public MedicationStatement setNote(List<Annotation> theNote) { 1335 this.note = theNote; 1336 return this; 1337 } 1338 1339 public boolean hasNote() { 1340 if (this.note == null) 1341 return false; 1342 for (Annotation item : this.note) 1343 if (!item.isEmpty()) 1344 return true; 1345 return false; 1346 } 1347 1348 public Annotation addNote() { //3 1349 Annotation t = new Annotation(); 1350 if (this.note == null) 1351 this.note = new ArrayList<Annotation>(); 1352 this.note.add(t); 1353 return t; 1354 } 1355 1356 public MedicationStatement addNote(Annotation t) { //3 1357 if (t == null) 1358 return this; 1359 if (this.note == null) 1360 this.note = new ArrayList<Annotation>(); 1361 this.note.add(t); 1362 return this; 1363 } 1364 1365 /** 1366 * @return The first repetition of repeating field {@link #note}, creating it if it does not already exist 1367 */ 1368 public Annotation getNoteFirstRep() { 1369 if (getNote().isEmpty()) { 1370 addNote(); 1371 } 1372 return getNote().get(0); 1373 } 1374 1375 /** 1376 * @return {@link #dosage} (Indicates how the medication is/was or should be taken by the patient.) 1377 */ 1378 public List<Dosage> getDosage() { 1379 if (this.dosage == null) 1380 this.dosage = new ArrayList<Dosage>(); 1381 return this.dosage; 1382 } 1383 1384 /** 1385 * @return Returns a reference to <code>this</code> for easy method chaining 1386 */ 1387 public MedicationStatement setDosage(List<Dosage> theDosage) { 1388 this.dosage = theDosage; 1389 return this; 1390 } 1391 1392 public boolean hasDosage() { 1393 if (this.dosage == null) 1394 return false; 1395 for (Dosage item : this.dosage) 1396 if (!item.isEmpty()) 1397 return true; 1398 return false; 1399 } 1400 1401 public Dosage addDosage() { //3 1402 Dosage t = new Dosage(); 1403 if (this.dosage == null) 1404 this.dosage = new ArrayList<Dosage>(); 1405 this.dosage.add(t); 1406 return t; 1407 } 1408 1409 public MedicationStatement addDosage(Dosage t) { //3 1410 if (t == null) 1411 return this; 1412 if (this.dosage == null) 1413 this.dosage = new ArrayList<Dosage>(); 1414 this.dosage.add(t); 1415 return this; 1416 } 1417 1418 /** 1419 * @return The first repetition of repeating field {@link #dosage}, creating it if it does not already exist 1420 */ 1421 public Dosage getDosageFirstRep() { 1422 if (getDosage().isEmpty()) { 1423 addDosage(); 1424 } 1425 return getDosage().get(0); 1426 } 1427 1428 protected void listChildren(List<Property> children) { 1429 super.listChildren(children); 1430 children.add(new Property("identifier", "Identifier", "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 0, java.lang.Integer.MAX_VALUE, identifier)); 1431 children.add(new Property("basedOn", "Reference(MedicationRequest|CarePlan|ProcedureRequest|ReferralRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn)); 1432 children.add(new Property("partOf", "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Observation)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf)); 1433 children.add(new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this MedicationStatement.", 0, 1, context)); 1434 children.add(new Property("status", "code", "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.", 0, 1, status)); 1435 children.add(new Property("category", "CodeableConcept", "Indicates where type of medication statement and where the medication is expected to be consumed or administered.", 0, 1, category)); 1436 children.add(new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication)); 1437 children.add(new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective)); 1438 children.add(new Property("dateAsserted", "dateTime", "The date when the medication statement was asserted by the information source.", 0, 1, dateAsserted)); 1439 children.add(new Property("informationSource", "Reference(Patient|Practitioner|RelatedPerson|Organization)", "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.", 0, 1, informationSource)); 1440 children.add(new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was taking the medication.", 0, 1, subject)); 1441 children.add(new Property("derivedFrom", "Reference(Any)", "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, derivedFrom)); 1442 children.add(new Property("taken", "code", "Indicator of the certainty of whether the medication was taken by the patient.", 0, 1, taken)); 1443 children.add(new Property("reasonNotTaken", "CodeableConcept", "A code indicating why the medication was not taken.", 0, java.lang.Integer.MAX_VALUE, reasonNotTaken)); 1444 children.add(new Property("reasonCode", "CodeableConcept", "A reason for why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonCode)); 1445 children.add(new Property("reasonReference", "Reference(Condition|Observation)", "Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonReference)); 1446 children.add(new Property("note", "Annotation", "Provides extra information about the medication statement that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note)); 1447 children.add(new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, dosage)); 1448 } 1449 1450 @Override 1451 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 1452 switch (_hash) { 1453 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "External identifier - FHIR will generate its own internal identifiers (probably URLs) which do not need to be explicitly managed by the resource. The identifier here is one that would be used by another non-FHIR system - for example an automated medication pump would provide a record each time it operated; an administration while the patient was off the ward might be made with a different system and entered after the event. Particularly important if these records have to be updated.", 0, java.lang.Integer.MAX_VALUE, identifier); 1454 case -332612366: /*basedOn*/ return new Property("basedOn", "Reference(MedicationRequest|CarePlan|ProcedureRequest|ReferralRequest)", "A plan, proposal or order that is fulfilled in whole or in part by this event.", 0, java.lang.Integer.MAX_VALUE, basedOn); 1455 case -995410646: /*partOf*/ return new Property("partOf", "Reference(MedicationAdministration|MedicationDispense|MedicationStatement|Procedure|Observation)", "A larger event of which this particular event is a component or step.", 0, java.lang.Integer.MAX_VALUE, partOf); 1456 case 951530927: /*context*/ return new Property("context", "Reference(Encounter|EpisodeOfCare)", "The encounter or episode of care that establishes the context for this MedicationStatement.", 0, 1, context); 1457 case -892481550: /*status*/ return new Property("status", "code", "A code representing the patient or other source's judgment about the state of the medication used that this statement is about. Generally this will be active or completed.", 0, 1, status); 1458 case 50511102: /*category*/ return new Property("category", "CodeableConcept", "Indicates where type of medication statement and where the medication is expected to be consumed or administered.", 0, 1, category); 1459 case 1458402129: /*medication[x]*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1460 case 1998965455: /*medication*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1461 case -209845038: /*medicationCodeableConcept*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1462 case 2104315196: /*medicationReference*/ return new Property("medication[x]", "CodeableConcept|Reference(Medication)", "Identifies the medication being administered. This is either a link to a resource representing the details of the medication or a simple attribute carrying a code that identifies the medication from a known list of medications.", 0, 1, medication); 1463 case 247104889: /*effective[x]*/ return new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective); 1464 case -1468651097: /*effective*/ return new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective); 1465 case -275306910: /*effectiveDateTime*/ return new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective); 1466 case -403934648: /*effectivePeriod*/ return new Property("effective[x]", "dateTime|Period", "The interval of time during which it is being asserted that the patient was taking the medication (or was not taking, when the wasNotGiven element is true).", 0, 1, effective); 1467 case -1980855245: /*dateAsserted*/ return new Property("dateAsserted", "dateTime", "The date when the medication statement was asserted by the information source.", 0, 1, dateAsserted); 1468 case -2123220889: /*informationSource*/ return new Property("informationSource", "Reference(Patient|Practitioner|RelatedPerson|Organization)", "The person or organization that provided the information about the taking of this medication. Note: Use derivedFrom when a MedicationStatement is derived from other resources, e.g Claim or MedicationRequest.", 0, 1, informationSource); 1469 case -1867885268: /*subject*/ return new Property("subject", "Reference(Patient|Group)", "The person, animal or group who is/was taking the medication.", 0, 1, subject); 1470 case 1077922663: /*derivedFrom*/ return new Property("derivedFrom", "Reference(Any)", "Allows linking the MedicationStatement to the underlying MedicationRequest, or to other information that supports or is used to derive the MedicationStatement.", 0, java.lang.Integer.MAX_VALUE, derivedFrom); 1471 case 110124231: /*taken*/ return new Property("taken", "code", "Indicator of the certainty of whether the medication was taken by the patient.", 0, 1, taken); 1472 case 2112880664: /*reasonNotTaken*/ return new Property("reasonNotTaken", "CodeableConcept", "A code indicating why the medication was not taken.", 0, java.lang.Integer.MAX_VALUE, reasonNotTaken); 1473 case 722137681: /*reasonCode*/ return new Property("reasonCode", "CodeableConcept", "A reason for why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonCode); 1474 case -1146218137: /*reasonReference*/ return new Property("reasonReference", "Reference(Condition|Observation)", "Condition or observation that supports why the medication is being/was taken.", 0, java.lang.Integer.MAX_VALUE, reasonReference); 1475 case 3387378: /*note*/ return new Property("note", "Annotation", "Provides extra information about the medication statement that is not conveyed by the other attributes.", 0, java.lang.Integer.MAX_VALUE, note); 1476 case -1326018889: /*dosage*/ return new Property("dosage", "Dosage", "Indicates how the medication is/was or should be taken by the patient.", 0, java.lang.Integer.MAX_VALUE, dosage); 1477 default: return super.getNamedProperty(_hash, _name, _checkValid); 1478 } 1479 1480 } 1481 1482 @Override 1483 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 1484 switch (hash) { 1485 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : this.identifier.toArray(new Base[this.identifier.size()]); // Identifier 1486 case -332612366: /*basedOn*/ return this.basedOn == null ? new Base[0] : this.basedOn.toArray(new Base[this.basedOn.size()]); // Reference 1487 case -995410646: /*partOf*/ return this.partOf == null ? new Base[0] : this.partOf.toArray(new Base[this.partOf.size()]); // Reference 1488 case 951530927: /*context*/ return this.context == null ? new Base[0] : new Base[] {this.context}; // Reference 1489 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<MedicationStatementStatus> 1490 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // CodeableConcept 1491 case 1998965455: /*medication*/ return this.medication == null ? new Base[0] : new Base[] {this.medication}; // Type 1492 case -1468651097: /*effective*/ return this.effective == null ? new Base[0] : new Base[] {this.effective}; // Type 1493 case -1980855245: /*dateAsserted*/ return this.dateAsserted == null ? new Base[0] : new Base[] {this.dateAsserted}; // DateTimeType 1494 case -2123220889: /*informationSource*/ return this.informationSource == null ? new Base[0] : new Base[] {this.informationSource}; // Reference 1495 case -1867885268: /*subject*/ return this.subject == null ? new Base[0] : new Base[] {this.subject}; // Reference 1496 case 1077922663: /*derivedFrom*/ return this.derivedFrom == null ? new Base[0] : this.derivedFrom.toArray(new Base[this.derivedFrom.size()]); // Reference 1497 case 110124231: /*taken*/ return this.taken == null ? new Base[0] : new Base[] {this.taken}; // Enumeration<MedicationStatementTaken> 1498 case 2112880664: /*reasonNotTaken*/ return this.reasonNotTaken == null ? new Base[0] : this.reasonNotTaken.toArray(new Base[this.reasonNotTaken.size()]); // CodeableConcept 1499 case 722137681: /*reasonCode*/ return this.reasonCode == null ? new Base[0] : this.reasonCode.toArray(new Base[this.reasonCode.size()]); // CodeableConcept 1500 case -1146218137: /*reasonReference*/ return this.reasonReference == null ? new Base[0] : this.reasonReference.toArray(new Base[this.reasonReference.size()]); // Reference 1501 case 3387378: /*note*/ return this.note == null ? new Base[0] : this.note.toArray(new Base[this.note.size()]); // Annotation 1502 case -1326018889: /*dosage*/ return this.dosage == null ? new Base[0] : this.dosage.toArray(new Base[this.dosage.size()]); // Dosage 1503 default: return super.getProperty(hash, name, checkValid); 1504 } 1505 1506 } 1507 1508 @Override 1509 public Base setProperty(int hash, String name, Base value) throws FHIRException { 1510 switch (hash) { 1511 case -1618432855: // identifier 1512 this.getIdentifier().add(castToIdentifier(value)); // Identifier 1513 return value; 1514 case -332612366: // basedOn 1515 this.getBasedOn().add(castToReference(value)); // Reference 1516 return value; 1517 case -995410646: // partOf 1518 this.getPartOf().add(castToReference(value)); // Reference 1519 return value; 1520 case 951530927: // context 1521 this.context = castToReference(value); // Reference 1522 return value; 1523 case -892481550: // status 1524 value = new MedicationStatementStatusEnumFactory().fromType(castToCode(value)); 1525 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatus> 1526 return value; 1527 case 50511102: // category 1528 this.category = castToCodeableConcept(value); // CodeableConcept 1529 return value; 1530 case 1998965455: // medication 1531 this.medication = castToType(value); // Type 1532 return value; 1533 case -1468651097: // effective 1534 this.effective = castToType(value); // Type 1535 return value; 1536 case -1980855245: // dateAsserted 1537 this.dateAsserted = castToDateTime(value); // DateTimeType 1538 return value; 1539 case -2123220889: // informationSource 1540 this.informationSource = castToReference(value); // Reference 1541 return value; 1542 case -1867885268: // subject 1543 this.subject = castToReference(value); // Reference 1544 return value; 1545 case 1077922663: // derivedFrom 1546 this.getDerivedFrom().add(castToReference(value)); // Reference 1547 return value; 1548 case 110124231: // taken 1549 value = new MedicationStatementTakenEnumFactory().fromType(castToCode(value)); 1550 this.taken = (Enumeration) value; // Enumeration<MedicationStatementTaken> 1551 return value; 1552 case 2112880664: // reasonNotTaken 1553 this.getReasonNotTaken().add(castToCodeableConcept(value)); // CodeableConcept 1554 return value; 1555 case 722137681: // reasonCode 1556 this.getReasonCode().add(castToCodeableConcept(value)); // CodeableConcept 1557 return value; 1558 case -1146218137: // reasonReference 1559 this.getReasonReference().add(castToReference(value)); // Reference 1560 return value; 1561 case 3387378: // note 1562 this.getNote().add(castToAnnotation(value)); // Annotation 1563 return value; 1564 case -1326018889: // dosage 1565 this.getDosage().add(castToDosage(value)); // Dosage 1566 return value; 1567 default: return super.setProperty(hash, name, value); 1568 } 1569 1570 } 1571 1572 @Override 1573 public Base setProperty(String name, Base value) throws FHIRException { 1574 if (name.equals("identifier")) { 1575 this.getIdentifier().add(castToIdentifier(value)); 1576 } else if (name.equals("basedOn")) { 1577 this.getBasedOn().add(castToReference(value)); 1578 } else if (name.equals("partOf")) { 1579 this.getPartOf().add(castToReference(value)); 1580 } else if (name.equals("context")) { 1581 this.context = castToReference(value); // Reference 1582 } else if (name.equals("status")) { 1583 value = new MedicationStatementStatusEnumFactory().fromType(castToCode(value)); 1584 this.status = (Enumeration) value; // Enumeration<MedicationStatementStatus> 1585 } else if (name.equals("category")) { 1586 this.category = castToCodeableConcept(value); // CodeableConcept 1587 } else if (name.equals("medication[x]")) { 1588 this.medication = castToType(value); // Type 1589 } else if (name.equals("effective[x]")) { 1590 this.effective = castToType(value); // Type 1591 } else if (name.equals("dateAsserted")) { 1592 this.dateAsserted = castToDateTime(value); // DateTimeType 1593 } else if (name.equals("informationSource")) { 1594 this.informationSource = castToReference(value); // Reference 1595 } else if (name.equals("subject")) { 1596 this.subject = castToReference(value); // Reference 1597 } else if (name.equals("derivedFrom")) { 1598 this.getDerivedFrom().add(castToReference(value)); 1599 } else if (name.equals("taken")) { 1600 value = new MedicationStatementTakenEnumFactory().fromType(castToCode(value)); 1601 this.taken = (Enumeration) value; // Enumeration<MedicationStatementTaken> 1602 } else if (name.equals("reasonNotTaken")) { 1603 this.getReasonNotTaken().add(castToCodeableConcept(value)); 1604 } else if (name.equals("reasonCode")) { 1605 this.getReasonCode().add(castToCodeableConcept(value)); 1606 } else if (name.equals("reasonReference")) { 1607 this.getReasonReference().add(castToReference(value)); 1608 } else if (name.equals("note")) { 1609 this.getNote().add(castToAnnotation(value)); 1610 } else if (name.equals("dosage")) { 1611 this.getDosage().add(castToDosage(value)); 1612 } else 1613 return super.setProperty(name, value); 1614 return value; 1615 } 1616 1617 @Override 1618 public Base makeProperty(int hash, String name) throws FHIRException { 1619 switch (hash) { 1620 case -1618432855: return addIdentifier(); 1621 case -332612366: return addBasedOn(); 1622 case -995410646: return addPartOf(); 1623 case 951530927: return getContext(); 1624 case -892481550: return getStatusElement(); 1625 case 50511102: return getCategory(); 1626 case 1458402129: return getMedication(); 1627 case 1998965455: return getMedication(); 1628 case 247104889: return getEffective(); 1629 case -1468651097: return getEffective(); 1630 case -1980855245: return getDateAssertedElement(); 1631 case -2123220889: return getInformationSource(); 1632 case -1867885268: return getSubject(); 1633 case 1077922663: return addDerivedFrom(); 1634 case 110124231: return getTakenElement(); 1635 case 2112880664: return addReasonNotTaken(); 1636 case 722137681: return addReasonCode(); 1637 case -1146218137: return addReasonReference(); 1638 case 3387378: return addNote(); 1639 case -1326018889: return addDosage(); 1640 default: return super.makeProperty(hash, name); 1641 } 1642 1643 } 1644 1645 @Override 1646 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 1647 switch (hash) { 1648 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 1649 case -332612366: /*basedOn*/ return new String[] {"Reference"}; 1650 case -995410646: /*partOf*/ return new String[] {"Reference"}; 1651 case 951530927: /*context*/ return new String[] {"Reference"}; 1652 case -892481550: /*status*/ return new String[] {"code"}; 1653 case 50511102: /*category*/ return new String[] {"CodeableConcept"}; 1654 case 1998965455: /*medication*/ return new String[] {"CodeableConcept", "Reference"}; 1655 case -1468651097: /*effective*/ return new String[] {"dateTime", "Period"}; 1656 case -1980855245: /*dateAsserted*/ return new String[] {"dateTime"}; 1657 case -2123220889: /*informationSource*/ return new String[] {"Reference"}; 1658 case -1867885268: /*subject*/ return new String[] {"Reference"}; 1659 case 1077922663: /*derivedFrom*/ return new String[] {"Reference"}; 1660 case 110124231: /*taken*/ return new String[] {"code"}; 1661 case 2112880664: /*reasonNotTaken*/ return new String[] {"CodeableConcept"}; 1662 case 722137681: /*reasonCode*/ return new String[] {"CodeableConcept"}; 1663 case -1146218137: /*reasonReference*/ return new String[] {"Reference"}; 1664 case 3387378: /*note*/ return new String[] {"Annotation"}; 1665 case -1326018889: /*dosage*/ return new String[] {"Dosage"}; 1666 default: return super.getTypesForProperty(hash, name); 1667 } 1668 1669 } 1670 1671 @Override 1672 public Base addChild(String name) throws FHIRException { 1673 if (name.equals("identifier")) { 1674 return addIdentifier(); 1675 } 1676 else if (name.equals("basedOn")) { 1677 return addBasedOn(); 1678 } 1679 else if (name.equals("partOf")) { 1680 return addPartOf(); 1681 } 1682 else if (name.equals("context")) { 1683 this.context = new Reference(); 1684 return this.context; 1685 } 1686 else if (name.equals("status")) { 1687 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.status"); 1688 } 1689 else if (name.equals("category")) { 1690 this.category = new CodeableConcept(); 1691 return this.category; 1692 } 1693 else if (name.equals("medicationCodeableConcept")) { 1694 this.medication = new CodeableConcept(); 1695 return this.medication; 1696 } 1697 else if (name.equals("medicationReference")) { 1698 this.medication = new Reference(); 1699 return this.medication; 1700 } 1701 else if (name.equals("effectiveDateTime")) { 1702 this.effective = new DateTimeType(); 1703 return this.effective; 1704 } 1705 else if (name.equals("effectivePeriod")) { 1706 this.effective = new Period(); 1707 return this.effective; 1708 } 1709 else if (name.equals("dateAsserted")) { 1710 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.dateAsserted"); 1711 } 1712 else if (name.equals("informationSource")) { 1713 this.informationSource = new Reference(); 1714 return this.informationSource; 1715 } 1716 else if (name.equals("subject")) { 1717 this.subject = new Reference(); 1718 return this.subject; 1719 } 1720 else if (name.equals("derivedFrom")) { 1721 return addDerivedFrom(); 1722 } 1723 else if (name.equals("taken")) { 1724 throw new FHIRException("Cannot call addChild on a singleton property MedicationStatement.taken"); 1725 } 1726 else if (name.equals("reasonNotTaken")) { 1727 return addReasonNotTaken(); 1728 } 1729 else if (name.equals("reasonCode")) { 1730 return addReasonCode(); 1731 } 1732 else if (name.equals("reasonReference")) { 1733 return addReasonReference(); 1734 } 1735 else if (name.equals("note")) { 1736 return addNote(); 1737 } 1738 else if (name.equals("dosage")) { 1739 return addDosage(); 1740 } 1741 else 1742 return super.addChild(name); 1743 } 1744 1745 public String fhirType() { 1746 return "MedicationStatement"; 1747 1748 } 1749 1750 public MedicationStatement copy() { 1751 MedicationStatement dst = new MedicationStatement(); 1752 copyValues(dst); 1753 if (identifier != null) { 1754 dst.identifier = new ArrayList<Identifier>(); 1755 for (Identifier i : identifier) 1756 dst.identifier.add(i.copy()); 1757 }; 1758 if (basedOn != null) { 1759 dst.basedOn = new ArrayList<Reference>(); 1760 for (Reference i : basedOn) 1761 dst.basedOn.add(i.copy()); 1762 }; 1763 if (partOf != null) { 1764 dst.partOf = new ArrayList<Reference>(); 1765 for (Reference i : partOf) 1766 dst.partOf.add(i.copy()); 1767 }; 1768 dst.context = context == null ? null : context.copy(); 1769 dst.status = status == null ? null : status.copy(); 1770 dst.category = category == null ? null : category.copy(); 1771 dst.medication = medication == null ? null : medication.copy(); 1772 dst.effective = effective == null ? null : effective.copy(); 1773 dst.dateAsserted = dateAsserted == null ? null : dateAsserted.copy(); 1774 dst.informationSource = informationSource == null ? null : informationSource.copy(); 1775 dst.subject = subject == null ? null : subject.copy(); 1776 if (derivedFrom != null) { 1777 dst.derivedFrom = new ArrayList<Reference>(); 1778 for (Reference i : derivedFrom) 1779 dst.derivedFrom.add(i.copy()); 1780 }; 1781 dst.taken = taken == null ? null : taken.copy(); 1782 if (reasonNotTaken != null) { 1783 dst.reasonNotTaken = new ArrayList<CodeableConcept>(); 1784 for (CodeableConcept i : reasonNotTaken) 1785 dst.reasonNotTaken.add(i.copy()); 1786 }; 1787 if (reasonCode != null) { 1788 dst.reasonCode = new ArrayList<CodeableConcept>(); 1789 for (CodeableConcept i : reasonCode) 1790 dst.reasonCode.add(i.copy()); 1791 }; 1792 if (reasonReference != null) { 1793 dst.reasonReference = new ArrayList<Reference>(); 1794 for (Reference i : reasonReference) 1795 dst.reasonReference.add(i.copy()); 1796 }; 1797 if (note != null) { 1798 dst.note = new ArrayList<Annotation>(); 1799 for (Annotation i : note) 1800 dst.note.add(i.copy()); 1801 }; 1802 if (dosage != null) { 1803 dst.dosage = new ArrayList<Dosage>(); 1804 for (Dosage i : dosage) 1805 dst.dosage.add(i.copy()); 1806 }; 1807 return dst; 1808 } 1809 1810 protected MedicationStatement typedCopy() { 1811 return copy(); 1812 } 1813 1814 @Override 1815 public boolean equalsDeep(Base other_) { 1816 if (!super.equalsDeep(other_)) 1817 return false; 1818 if (!(other_ instanceof MedicationStatement)) 1819 return false; 1820 MedicationStatement o = (MedicationStatement) other_; 1821 return compareDeep(identifier, o.identifier, true) && compareDeep(basedOn, o.basedOn, true) && compareDeep(partOf, o.partOf, true) 1822 && compareDeep(context, o.context, true) && compareDeep(status, o.status, true) && compareDeep(category, o.category, true) 1823 && compareDeep(medication, o.medication, true) && compareDeep(effective, o.effective, true) && compareDeep(dateAsserted, o.dateAsserted, true) 1824 && compareDeep(informationSource, o.informationSource, true) && compareDeep(subject, o.subject, true) 1825 && compareDeep(derivedFrom, o.derivedFrom, true) && compareDeep(taken, o.taken, true) && compareDeep(reasonNotTaken, o.reasonNotTaken, true) 1826 && compareDeep(reasonCode, o.reasonCode, true) && compareDeep(reasonReference, o.reasonReference, true) 1827 && compareDeep(note, o.note, true) && compareDeep(dosage, o.dosage, true); 1828 } 1829 1830 @Override 1831 public boolean equalsShallow(Base other_) { 1832 if (!super.equalsShallow(other_)) 1833 return false; 1834 if (!(other_ instanceof MedicationStatement)) 1835 return false; 1836 MedicationStatement o = (MedicationStatement) other_; 1837 return compareValues(status, o.status, true) && compareValues(dateAsserted, o.dateAsserted, true) && compareValues(taken, o.taken, true) 1838 ; 1839 } 1840 1841 public boolean isEmpty() { 1842 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, basedOn, partOf 1843 , context, status, category, medication, effective, dateAsserted, informationSource 1844 , subject, derivedFrom, taken, reasonNotTaken, reasonCode, reasonReference, note 1845 , dosage); 1846 } 1847 1848 @Override 1849 public ResourceType getResourceType() { 1850 return ResourceType.MedicationStatement; 1851 } 1852 1853 /** 1854 * Search parameter: <b>identifier</b> 1855 * <p> 1856 * Description: <b>Return statements with this external identifier</b><br> 1857 * Type: <b>token</b><br> 1858 * Path: <b>MedicationStatement.identifier</b><br> 1859 * </p> 1860 */ 1861 @SearchParamDefinition(name="identifier", path="MedicationStatement.identifier", description="Return statements with this external identifier", type="token" ) 1862 public static final String SP_IDENTIFIER = "identifier"; 1863 /** 1864 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 1865 * <p> 1866 * Description: <b>Return statements with this external identifier</b><br> 1867 * Type: <b>token</b><br> 1868 * Path: <b>MedicationStatement.identifier</b><br> 1869 * </p> 1870 */ 1871 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 1872 1873 /** 1874 * Search parameter: <b>effective</b> 1875 * <p> 1876 * Description: <b>Date when patient was taking (or not taking) the medication</b><br> 1877 * Type: <b>date</b><br> 1878 * Path: <b>MedicationStatement.effective[x]</b><br> 1879 * </p> 1880 */ 1881 @SearchParamDefinition(name="effective", path="MedicationStatement.effective", description="Date when patient was taking (or not taking) the medication", type="date" ) 1882 public static final String SP_EFFECTIVE = "effective"; 1883 /** 1884 * <b>Fluent Client</b> search parameter constant for <b>effective</b> 1885 * <p> 1886 * Description: <b>Date when patient was taking (or not taking) the medication</b><br> 1887 * Type: <b>date</b><br> 1888 * Path: <b>MedicationStatement.effective[x]</b><br> 1889 * </p> 1890 */ 1891 public static final ca.uhn.fhir.rest.gclient.DateClientParam EFFECTIVE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_EFFECTIVE); 1892 1893 /** 1894 * Search parameter: <b>code</b> 1895 * <p> 1896 * Description: <b>Return statements of this medication code</b><br> 1897 * Type: <b>token</b><br> 1898 * Path: <b>MedicationStatement.medicationCodeableConcept</b><br> 1899 * </p> 1900 */ 1901 @SearchParamDefinition(name="code", path="MedicationStatement.medication.as(CodeableConcept)", description="Return statements of this medication code", type="token" ) 1902 public static final String SP_CODE = "code"; 1903 /** 1904 * <b>Fluent Client</b> search parameter constant for <b>code</b> 1905 * <p> 1906 * Description: <b>Return statements of this medication code</b><br> 1907 * Type: <b>token</b><br> 1908 * Path: <b>MedicationStatement.medicationCodeableConcept</b><br> 1909 * </p> 1910 */ 1911 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CODE = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CODE); 1912 1913 /** 1914 * Search parameter: <b>subject</b> 1915 * <p> 1916 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 1917 * Type: <b>reference</b><br> 1918 * Path: <b>MedicationStatement.subject</b><br> 1919 * </p> 1920 */ 1921 @SearchParamDefinition(name="subject", path="MedicationStatement.subject", description="The identity of a patient, animal or group to list statements for", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Patient") }, target={Group.class, Patient.class } ) 1922 public static final String SP_SUBJECT = "subject"; 1923 /** 1924 * <b>Fluent Client</b> search parameter constant for <b>subject</b> 1925 * <p> 1926 * Description: <b>The identity of a patient, animal or group to list statements for</b><br> 1927 * Type: <b>reference</b><br> 1928 * Path: <b>MedicationStatement.subject</b><br> 1929 * </p> 1930 */ 1931 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SUBJECT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SUBJECT); 1932 1933/** 1934 * Constant for fluent queries to be used to add include statements. Specifies 1935 * the path value of "<b>MedicationStatement:subject</b>". 1936 */ 1937 public static final ca.uhn.fhir.model.api.Include INCLUDE_SUBJECT = new ca.uhn.fhir.model.api.Include("MedicationStatement:subject").toLocked(); 1938 1939 /** 1940 * Search parameter: <b>patient</b> 1941 * <p> 1942 * Description: <b>Returns statements for a specific patient.</b><br> 1943 * Type: <b>reference</b><br> 1944 * Path: <b>MedicationStatement.subject</b><br> 1945 * </p> 1946 */ 1947 @SearchParamDefinition(name="patient", path="MedicationStatement.subject", description="Returns statements for a specific patient.", type="reference", target={Patient.class } ) 1948 public static final String SP_PATIENT = "patient"; 1949 /** 1950 * <b>Fluent Client</b> search parameter constant for <b>patient</b> 1951 * <p> 1952 * Description: <b>Returns statements for a specific patient.</b><br> 1953 * Type: <b>reference</b><br> 1954 * Path: <b>MedicationStatement.subject</b><br> 1955 * </p> 1956 */ 1957 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PATIENT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PATIENT); 1958 1959/** 1960 * Constant for fluent queries to be used to add include statements. Specifies 1961 * the path value of "<b>MedicationStatement:patient</b>". 1962 */ 1963 public static final ca.uhn.fhir.model.api.Include INCLUDE_PATIENT = new ca.uhn.fhir.model.api.Include("MedicationStatement:patient").toLocked(); 1964 1965 /** 1966 * Search parameter: <b>context</b> 1967 * <p> 1968 * Description: <b>Returns statements for a specific context (episode or episode of Care).</b><br> 1969 * Type: <b>reference</b><br> 1970 * Path: <b>MedicationStatement.context</b><br> 1971 * </p> 1972 */ 1973 @SearchParamDefinition(name="context", path="MedicationStatement.context", description="Returns statements for a specific context (episode or episode of Care).", type="reference", target={Encounter.class, EpisodeOfCare.class } ) 1974 public static final String SP_CONTEXT = "context"; 1975 /** 1976 * <b>Fluent Client</b> search parameter constant for <b>context</b> 1977 * <p> 1978 * Description: <b>Returns statements for a specific context (episode or episode of Care).</b><br> 1979 * Type: <b>reference</b><br> 1980 * Path: <b>MedicationStatement.context</b><br> 1981 * </p> 1982 */ 1983 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam CONTEXT = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_CONTEXT); 1984 1985/** 1986 * Constant for fluent queries to be used to add include statements. Specifies 1987 * the path value of "<b>MedicationStatement:context</b>". 1988 */ 1989 public static final ca.uhn.fhir.model.api.Include INCLUDE_CONTEXT = new ca.uhn.fhir.model.api.Include("MedicationStatement:context").toLocked(); 1990 1991 /** 1992 * Search parameter: <b>medication</b> 1993 * <p> 1994 * Description: <b>Return statements of this medication reference</b><br> 1995 * Type: <b>reference</b><br> 1996 * Path: <b>MedicationStatement.medicationReference</b><br> 1997 * </p> 1998 */ 1999 @SearchParamDefinition(name="medication", path="MedicationStatement.medication.as(Reference)", description="Return statements of this medication reference", type="reference", target={Medication.class } ) 2000 public static final String SP_MEDICATION = "medication"; 2001 /** 2002 * <b>Fluent Client</b> search parameter constant for <b>medication</b> 2003 * <p> 2004 * Description: <b>Return statements of this medication reference</b><br> 2005 * Type: <b>reference</b><br> 2006 * Path: <b>MedicationStatement.medicationReference</b><br> 2007 * </p> 2008 */ 2009 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam MEDICATION = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_MEDICATION); 2010 2011/** 2012 * Constant for fluent queries to be used to add include statements. Specifies 2013 * the path value of "<b>MedicationStatement:medication</b>". 2014 */ 2015 public static final ca.uhn.fhir.model.api.Include INCLUDE_MEDICATION = new ca.uhn.fhir.model.api.Include("MedicationStatement:medication").toLocked(); 2016 2017 /** 2018 * Search parameter: <b>part-of</b> 2019 * <p> 2020 * Description: <b>Returns statements that are part of another event.</b><br> 2021 * Type: <b>reference</b><br> 2022 * Path: <b>MedicationStatement.partOf</b><br> 2023 * </p> 2024 */ 2025 @SearchParamDefinition(name="part-of", path="MedicationStatement.partOf", description="Returns statements that are part of another event.", type="reference", target={MedicationAdministration.class, MedicationDispense.class, MedicationStatement.class, Observation.class, Procedure.class } ) 2026 public static final String SP_PART_OF = "part-of"; 2027 /** 2028 * <b>Fluent Client</b> search parameter constant for <b>part-of</b> 2029 * <p> 2030 * Description: <b>Returns statements that are part of another event.</b><br> 2031 * Type: <b>reference</b><br> 2032 * Path: <b>MedicationStatement.partOf</b><br> 2033 * </p> 2034 */ 2035 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam PART_OF = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_PART_OF); 2036 2037/** 2038 * Constant for fluent queries to be used to add include statements. Specifies 2039 * the path value of "<b>MedicationStatement:part-of</b>". 2040 */ 2041 public static final ca.uhn.fhir.model.api.Include INCLUDE_PART_OF = new ca.uhn.fhir.model.api.Include("MedicationStatement:part-of").toLocked(); 2042 2043 /** 2044 * Search parameter: <b>source</b> 2045 * <p> 2046 * Description: <b>Who or where the information in the statement came from</b><br> 2047 * Type: <b>reference</b><br> 2048 * Path: <b>MedicationStatement.informationSource</b><br> 2049 * </p> 2050 */ 2051 @SearchParamDefinition(name="source", path="MedicationStatement.informationSource", description="Who or where the information in the statement came from", type="reference", providesMembershipIn={ @ca.uhn.fhir.model.api.annotation.Compartment(name="Practitioner"), @ca.uhn.fhir.model.api.annotation.Compartment(name="RelatedPerson") }, target={Organization.class, Patient.class, Practitioner.class, RelatedPerson.class } ) 2052 public static final String SP_SOURCE = "source"; 2053 /** 2054 * <b>Fluent Client</b> search parameter constant for <b>source</b> 2055 * <p> 2056 * Description: <b>Who or where the information in the statement came from</b><br> 2057 * Type: <b>reference</b><br> 2058 * Path: <b>MedicationStatement.informationSource</b><br> 2059 * </p> 2060 */ 2061 public static final ca.uhn.fhir.rest.gclient.ReferenceClientParam SOURCE = new ca.uhn.fhir.rest.gclient.ReferenceClientParam(SP_SOURCE); 2062 2063/** 2064 * Constant for fluent queries to be used to add include statements. Specifies 2065 * the path value of "<b>MedicationStatement:source</b>". 2066 */ 2067 public static final ca.uhn.fhir.model.api.Include INCLUDE_SOURCE = new ca.uhn.fhir.model.api.Include("MedicationStatement:source").toLocked(); 2068 2069 /** 2070 * Search parameter: <b>category</b> 2071 * <p> 2072 * Description: <b>Returns statements of this category of medicationstatement</b><br> 2073 * Type: <b>token</b><br> 2074 * Path: <b>MedicationStatement.category</b><br> 2075 * </p> 2076 */ 2077 @SearchParamDefinition(name="category", path="MedicationStatement.category", description="Returns statements of this category of medicationstatement", type="token" ) 2078 public static final String SP_CATEGORY = "category"; 2079 /** 2080 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2081 * <p> 2082 * Description: <b>Returns statements of this category of medicationstatement</b><br> 2083 * Type: <b>token</b><br> 2084 * Path: <b>MedicationStatement.category</b><br> 2085 * </p> 2086 */ 2087 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2088 2089 /** 2090 * Search parameter: <b>status</b> 2091 * <p> 2092 * Description: <b>Return statements that match the given status</b><br> 2093 * Type: <b>token</b><br> 2094 * Path: <b>MedicationStatement.status</b><br> 2095 * </p> 2096 */ 2097 @SearchParamDefinition(name="status", path="MedicationStatement.status", description="Return statements that match the given status", type="token" ) 2098 public static final String SP_STATUS = "status"; 2099 /** 2100 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2101 * <p> 2102 * Description: <b>Return statements that match the given status</b><br> 2103 * Type: <b>token</b><br> 2104 * Path: <b>MedicationStatement.status</b><br> 2105 * </p> 2106 */ 2107 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2108 2109 2110}