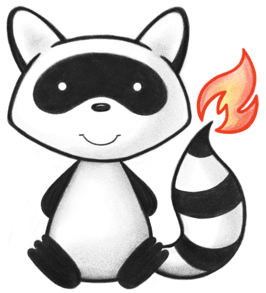
001package org.hl7.fhir.dstu3.model; 002 003 004 005/* 006 Copyright (c) 2011+, HL7, Inc. 007 All rights reserved. 008 009 Redistribution and use in source and binary forms, with or without modification, 010 are permitted provided that the following conditions are met: 011 012 * Redistributions of source code must retain the above copyright notice, this 013 list of conditions and the following disclaimer. 014 * Redistributions in binary form must reproduce the above copyright notice, 015 this list of conditions and the following disclaimer in the documentation 016 and/or other materials provided with the distribution. 017 * Neither the name of HL7 nor the names of its contributors may be used to 018 endorse or promote products derived from this software without specific 019 prior written permission. 020 021 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 022 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 023 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 024 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 025 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 026 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 027 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 028 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 029 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 030 POSSIBILITY OF SUCH DAMAGE. 031 032*/ 033 034// Generated on Fri, Mar 16, 2018 15:21+1100 for FHIR v3.0.x 035import java.util.ArrayList; 036import java.util.Date; 037import java.util.List; 038 039import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatus; 040import org.hl7.fhir.dstu3.model.Enumerations.PublicationStatusEnumFactory; 041import org.hl7.fhir.exceptions.FHIRException; 042import org.hl7.fhir.instance.model.api.IBaseBackboneElement; 043import org.hl7.fhir.utilities.Utilities; 044 045import ca.uhn.fhir.model.api.annotation.Block; 046import ca.uhn.fhir.model.api.annotation.Child; 047import ca.uhn.fhir.model.api.annotation.ChildOrder; 048import ca.uhn.fhir.model.api.annotation.Description; 049import ca.uhn.fhir.model.api.annotation.ResourceDef; 050import ca.uhn.fhir.model.api.annotation.SearchParamDefinition; 051/** 052 * Defines the characteristics of a message that can be shared between systems, including the type of event that initiates the message, the content to be transmitted and what response(s), if any, are permitted. 053 */ 054@ResourceDef(name="MessageDefinition", profile="http://hl7.org/fhir/Profile/MessageDefinition") 055@ChildOrder(names={"url", "identifier", "version", "name", "title", "status", "experimental", "date", "publisher", "contact", "description", "useContext", "jurisdiction", "purpose", "copyright", "base", "parent", "replaces", "event", "category", "focus", "responseRequired", "allowedResponse"}) 056public class MessageDefinition extends MetadataResource { 057 058 public enum MessageSignificanceCategory { 059 /** 060 * The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment. 061 */ 062 CONSEQUENCE, 063 /** 064 * The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful. 065 */ 066 CURRENCY, 067 /** 068 * The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications. 069 */ 070 NOTIFICATION, 071 /** 072 * added to help the parsers with the generic types 073 */ 074 NULL; 075 public static MessageSignificanceCategory fromCode(String codeString) throws FHIRException { 076 if (codeString == null || "".equals(codeString)) 077 return null; 078 if ("Consequence".equals(codeString)) 079 return CONSEQUENCE; 080 if ("Currency".equals(codeString)) 081 return CURRENCY; 082 if ("Notification".equals(codeString)) 083 return NOTIFICATION; 084 if (Configuration.isAcceptInvalidEnums()) 085 return null; 086 else 087 throw new FHIRException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 088 } 089 public String toCode() { 090 switch (this) { 091 case CONSEQUENCE: return "Consequence"; 092 case CURRENCY: return "Currency"; 093 case NOTIFICATION: return "Notification"; 094 case NULL: return null; 095 default: return "?"; 096 } 097 } 098 public String getSystem() { 099 switch (this) { 100 case CONSEQUENCE: return "http://hl7.org/fhir/message-significance-category"; 101 case CURRENCY: return "http://hl7.org/fhir/message-significance-category"; 102 case NOTIFICATION: return "http://hl7.org/fhir/message-significance-category"; 103 case NULL: return null; 104 default: return "?"; 105 } 106 } 107 public String getDefinition() { 108 switch (this) { 109 case CONSEQUENCE: return "The message represents/requests a change that should not be processed more than once; e.g., making a booking for an appointment."; 110 case CURRENCY: return "The message represents a response to query for current information. Retrospective processing is wrong and/or wasteful."; 111 case NOTIFICATION: return "The content is not necessarily intended to be current, and it can be reprocessed, though there may be version issues created by processing old notifications."; 112 case NULL: return null; 113 default: return "?"; 114 } 115 } 116 public String getDisplay() { 117 switch (this) { 118 case CONSEQUENCE: return "Consequence"; 119 case CURRENCY: return "Currency"; 120 case NOTIFICATION: return "Notification"; 121 case NULL: return null; 122 default: return "?"; 123 } 124 } 125 } 126 127 public static class MessageSignificanceCategoryEnumFactory implements EnumFactory<MessageSignificanceCategory> { 128 public MessageSignificanceCategory fromCode(String codeString) throws IllegalArgumentException { 129 if (codeString == null || "".equals(codeString)) 130 if (codeString == null || "".equals(codeString)) 131 return null; 132 if ("Consequence".equals(codeString)) 133 return MessageSignificanceCategory.CONSEQUENCE; 134 if ("Currency".equals(codeString)) 135 return MessageSignificanceCategory.CURRENCY; 136 if ("Notification".equals(codeString)) 137 return MessageSignificanceCategory.NOTIFICATION; 138 throw new IllegalArgumentException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 139 } 140 public Enumeration<MessageSignificanceCategory> fromType(PrimitiveType<?> code) throws FHIRException { 141 if (code == null) 142 return null; 143 if (code.isEmpty()) 144 return new Enumeration<MessageSignificanceCategory>(this); 145 String codeString = code.asStringValue(); 146 if (codeString == null || "".equals(codeString)) 147 return null; 148 if ("Consequence".equals(codeString)) 149 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CONSEQUENCE); 150 if ("Currency".equals(codeString)) 151 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.CURRENCY); 152 if ("Notification".equals(codeString)) 153 return new Enumeration<MessageSignificanceCategory>(this, MessageSignificanceCategory.NOTIFICATION); 154 throw new FHIRException("Unknown MessageSignificanceCategory code '"+codeString+"'"); 155 } 156 public String toCode(MessageSignificanceCategory code) { 157 if (code == MessageSignificanceCategory.NULL) 158 return null; 159 if (code == MessageSignificanceCategory.CONSEQUENCE) 160 return "Consequence"; 161 if (code == MessageSignificanceCategory.CURRENCY) 162 return "Currency"; 163 if (code == MessageSignificanceCategory.NOTIFICATION) 164 return "Notification"; 165 return "?"; 166 } 167 public String toSystem(MessageSignificanceCategory code) { 168 return code.getSystem(); 169 } 170 } 171 172 @Block() 173 public static class MessageDefinitionFocusComponent extends BackboneElement implements IBaseBackboneElement { 174 /** 175 * The kind of resource that must be the focus for this message. 176 */ 177 @Child(name = "code", type = {CodeType.class}, order=1, min=1, max=1, modifier=false, summary=true) 178 @Description(shortDefinition="Type of resource", formalDefinition="The kind of resource that must be the focus for this message." ) 179 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/resource-types") 180 protected CodeType code; 181 182 /** 183 * A profile that reflects constraints for the focal resource (and potentially for related resources). 184 */ 185 @Child(name = "profile", type = {StructureDefinition.class}, order=2, min=0, max=1, modifier=false, summary=false) 186 @Description(shortDefinition="Profile that must be adhered to by focus", formalDefinition="A profile that reflects constraints for the focal resource (and potentially for related resources)." ) 187 protected Reference profile; 188 189 /** 190 * The actual object that is the target of the reference (A profile that reflects constraints for the focal resource (and potentially for related resources).) 191 */ 192 protected StructureDefinition profileTarget; 193 194 /** 195 * Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 196 */ 197 @Child(name = "min", type = {UnsignedIntType.class}, order=3, min=0, max=1, modifier=false, summary=false) 198 @Description(shortDefinition="Minimum number of focuses of this type", formalDefinition="Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition." ) 199 protected UnsignedIntType min; 200 201 /** 202 * Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 203 */ 204 @Child(name = "max", type = {StringType.class}, order=4, min=0, max=1, modifier=false, summary=false) 205 @Description(shortDefinition="Maximum number of focuses of this type", formalDefinition="Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition." ) 206 protected StringType max; 207 208 private static final long serialVersionUID = 35658543L; 209 210 /** 211 * Constructor 212 */ 213 public MessageDefinitionFocusComponent() { 214 super(); 215 } 216 217 /** 218 * Constructor 219 */ 220 public MessageDefinitionFocusComponent(CodeType code) { 221 super(); 222 this.code = code; 223 } 224 225 /** 226 * @return {@link #code} (The kind of resource that must be the focus for this message.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 227 */ 228 public CodeType getCodeElement() { 229 if (this.code == null) 230 if (Configuration.errorOnAutoCreate()) 231 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.code"); 232 else if (Configuration.doAutoCreate()) 233 this.code = new CodeType(); // bb 234 return this.code; 235 } 236 237 public boolean hasCodeElement() { 238 return this.code != null && !this.code.isEmpty(); 239 } 240 241 public boolean hasCode() { 242 return this.code != null && !this.code.isEmpty(); 243 } 244 245 /** 246 * @param value {@link #code} (The kind of resource that must be the focus for this message.). This is the underlying object with id, value and extensions. The accessor "getCode" gives direct access to the value 247 */ 248 public MessageDefinitionFocusComponent setCodeElement(CodeType value) { 249 this.code = value; 250 return this; 251 } 252 253 /** 254 * @return The kind of resource that must be the focus for this message. 255 */ 256 public String getCode() { 257 return this.code == null ? null : this.code.getValue(); 258 } 259 260 /** 261 * @param value The kind of resource that must be the focus for this message. 262 */ 263 public MessageDefinitionFocusComponent setCode(String value) { 264 if (this.code == null) 265 this.code = new CodeType(); 266 this.code.setValue(value); 267 return this; 268 } 269 270 /** 271 * @return {@link #profile} (A profile that reflects constraints for the focal resource (and potentially for related resources).) 272 */ 273 public Reference getProfile() { 274 if (this.profile == null) 275 if (Configuration.errorOnAutoCreate()) 276 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.profile"); 277 else if (Configuration.doAutoCreate()) 278 this.profile = new Reference(); // cc 279 return this.profile; 280 } 281 282 public boolean hasProfile() { 283 return this.profile != null && !this.profile.isEmpty(); 284 } 285 286 /** 287 * @param value {@link #profile} (A profile that reflects constraints for the focal resource (and potentially for related resources).) 288 */ 289 public MessageDefinitionFocusComponent setProfile(Reference value) { 290 this.profile = value; 291 return this; 292 } 293 294 /** 295 * @return {@link #profile} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A profile that reflects constraints for the focal resource (and potentially for related resources).) 296 */ 297 public StructureDefinition getProfileTarget() { 298 if (this.profileTarget == null) 299 if (Configuration.errorOnAutoCreate()) 300 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.profile"); 301 else if (Configuration.doAutoCreate()) 302 this.profileTarget = new StructureDefinition(); // aa 303 return this.profileTarget; 304 } 305 306 /** 307 * @param value {@link #profile} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A profile that reflects constraints for the focal resource (and potentially for related resources).) 308 */ 309 public MessageDefinitionFocusComponent setProfileTarget(StructureDefinition value) { 310 this.profileTarget = value; 311 return this; 312 } 313 314 /** 315 * @return {@link #min} (Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 316 */ 317 public UnsignedIntType getMinElement() { 318 if (this.min == null) 319 if (Configuration.errorOnAutoCreate()) 320 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.min"); 321 else if (Configuration.doAutoCreate()) 322 this.min = new UnsignedIntType(); // bb 323 return this.min; 324 } 325 326 public boolean hasMinElement() { 327 return this.min != null && !this.min.isEmpty(); 328 } 329 330 public boolean hasMin() { 331 return this.min != null && !this.min.isEmpty(); 332 } 333 334 /** 335 * @param value {@link #min} (Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMin" gives direct access to the value 336 */ 337 public MessageDefinitionFocusComponent setMinElement(UnsignedIntType value) { 338 this.min = value; 339 return this; 340 } 341 342 /** 343 * @return Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 344 */ 345 public int getMin() { 346 return this.min == null || this.min.isEmpty() ? 0 : this.min.getValue(); 347 } 348 349 /** 350 * @param value Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 351 */ 352 public MessageDefinitionFocusComponent setMin(int value) { 353 if (this.min == null) 354 this.min = new UnsignedIntType(); 355 this.min.setValue(value); 356 return this; 357 } 358 359 /** 360 * @return {@link #max} (Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 361 */ 362 public StringType getMaxElement() { 363 if (this.max == null) 364 if (Configuration.errorOnAutoCreate()) 365 throw new Error("Attempt to auto-create MessageDefinitionFocusComponent.max"); 366 else if (Configuration.doAutoCreate()) 367 this.max = new StringType(); // bb 368 return this.max; 369 } 370 371 public boolean hasMaxElement() { 372 return this.max != null && !this.max.isEmpty(); 373 } 374 375 public boolean hasMax() { 376 return this.max != null && !this.max.isEmpty(); 377 } 378 379 /** 380 * @param value {@link #max} (Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.). This is the underlying object with id, value and extensions. The accessor "getMax" gives direct access to the value 381 */ 382 public MessageDefinitionFocusComponent setMaxElement(StringType value) { 383 this.max = value; 384 return this; 385 } 386 387 /** 388 * @return Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 389 */ 390 public String getMax() { 391 return this.max == null ? null : this.max.getValue(); 392 } 393 394 /** 395 * @param value Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition. 396 */ 397 public MessageDefinitionFocusComponent setMax(String value) { 398 if (Utilities.noString(value)) 399 this.max = null; 400 else { 401 if (this.max == null) 402 this.max = new StringType(); 403 this.max.setValue(value); 404 } 405 return this; 406 } 407 408 protected void listChildren(List<Property> children) { 409 super.listChildren(children); 410 children.add(new Property("code", "code", "The kind of resource that must be the focus for this message.", 0, 1, code)); 411 children.add(new Property("profile", "Reference(StructureDefinition)", "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, profile)); 412 children.add(new Property("min", "unsignedInt", "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, min)); 413 children.add(new Property("max", "string", "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, max)); 414 } 415 416 @Override 417 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 418 switch (_hash) { 419 case 3059181: /*code*/ return new Property("code", "code", "The kind of resource that must be the focus for this message.", 0, 1, code); 420 case -309425751: /*profile*/ return new Property("profile", "Reference(StructureDefinition)", "A profile that reflects constraints for the focal resource (and potentially for related resources).", 0, 1, profile); 421 case 108114: /*min*/ return new Property("min", "unsignedInt", "Identifies the minimum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, min); 422 case 107876: /*max*/ return new Property("max", "string", "Identifies the maximum number of resources of this type that must be pointed to by a message in order for it to be valid against this MessageDefinition.", 0, 1, max); 423 default: return super.getNamedProperty(_hash, _name, _checkValid); 424 } 425 426 } 427 428 @Override 429 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 430 switch (hash) { 431 case 3059181: /*code*/ return this.code == null ? new Base[0] : new Base[] {this.code}; // CodeType 432 case -309425751: /*profile*/ return this.profile == null ? new Base[0] : new Base[] {this.profile}; // Reference 433 case 108114: /*min*/ return this.min == null ? new Base[0] : new Base[] {this.min}; // UnsignedIntType 434 case 107876: /*max*/ return this.max == null ? new Base[0] : new Base[] {this.max}; // StringType 435 default: return super.getProperty(hash, name, checkValid); 436 } 437 438 } 439 440 @Override 441 public Base setProperty(int hash, String name, Base value) throws FHIRException { 442 switch (hash) { 443 case 3059181: // code 444 this.code = castToCode(value); // CodeType 445 return value; 446 case -309425751: // profile 447 this.profile = castToReference(value); // Reference 448 return value; 449 case 108114: // min 450 this.min = castToUnsignedInt(value); // UnsignedIntType 451 return value; 452 case 107876: // max 453 this.max = castToString(value); // StringType 454 return value; 455 default: return super.setProperty(hash, name, value); 456 } 457 458 } 459 460 @Override 461 public Base setProperty(String name, Base value) throws FHIRException { 462 if (name.equals("code")) { 463 this.code = castToCode(value); // CodeType 464 } else if (name.equals("profile")) { 465 this.profile = castToReference(value); // Reference 466 } else if (name.equals("min")) { 467 this.min = castToUnsignedInt(value); // UnsignedIntType 468 } else if (name.equals("max")) { 469 this.max = castToString(value); // StringType 470 } else 471 return super.setProperty(name, value); 472 return value; 473 } 474 475 @Override 476 public Base makeProperty(int hash, String name) throws FHIRException { 477 switch (hash) { 478 case 3059181: return getCodeElement(); 479 case -309425751: return getProfile(); 480 case 108114: return getMinElement(); 481 case 107876: return getMaxElement(); 482 default: return super.makeProperty(hash, name); 483 } 484 485 } 486 487 @Override 488 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 489 switch (hash) { 490 case 3059181: /*code*/ return new String[] {"code"}; 491 case -309425751: /*profile*/ return new String[] {"Reference"}; 492 case 108114: /*min*/ return new String[] {"unsignedInt"}; 493 case 107876: /*max*/ return new String[] {"string"}; 494 default: return super.getTypesForProperty(hash, name); 495 } 496 497 } 498 499 @Override 500 public Base addChild(String name) throws FHIRException { 501 if (name.equals("code")) { 502 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.code"); 503 } 504 else if (name.equals("profile")) { 505 this.profile = new Reference(); 506 return this.profile; 507 } 508 else if (name.equals("min")) { 509 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.min"); 510 } 511 else if (name.equals("max")) { 512 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.max"); 513 } 514 else 515 return super.addChild(name); 516 } 517 518 public MessageDefinitionFocusComponent copy() { 519 MessageDefinitionFocusComponent dst = new MessageDefinitionFocusComponent(); 520 copyValues(dst); 521 dst.code = code == null ? null : code.copy(); 522 dst.profile = profile == null ? null : profile.copy(); 523 dst.min = min == null ? null : min.copy(); 524 dst.max = max == null ? null : max.copy(); 525 return dst; 526 } 527 528 @Override 529 public boolean equalsDeep(Base other_) { 530 if (!super.equalsDeep(other_)) 531 return false; 532 if (!(other_ instanceof MessageDefinitionFocusComponent)) 533 return false; 534 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 535 return compareDeep(code, o.code, true) && compareDeep(profile, o.profile, true) && compareDeep(min, o.min, true) 536 && compareDeep(max, o.max, true); 537 } 538 539 @Override 540 public boolean equalsShallow(Base other_) { 541 if (!super.equalsShallow(other_)) 542 return false; 543 if (!(other_ instanceof MessageDefinitionFocusComponent)) 544 return false; 545 MessageDefinitionFocusComponent o = (MessageDefinitionFocusComponent) other_; 546 return compareValues(code, o.code, true) && compareValues(min, o.min, true) && compareValues(max, o.max, true) 547 ; 548 } 549 550 public boolean isEmpty() { 551 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(code, profile, min, max 552 ); 553 } 554 555 public String fhirType() { 556 return "MessageDefinition.focus"; 557 558 } 559 560 } 561 562 @Block() 563 public static class MessageDefinitionAllowedResponseComponent extends BackboneElement implements IBaseBackboneElement { 564 /** 565 * A reference to the message definition that must be adhered to by this supported response. 566 */ 567 @Child(name = "message", type = {MessageDefinition.class}, order=1, min=1, max=1, modifier=false, summary=false) 568 @Description(shortDefinition="Reference to allowed message definition response", formalDefinition="A reference to the message definition that must be adhered to by this supported response." ) 569 protected Reference message; 570 571 /** 572 * The actual object that is the target of the reference (A reference to the message definition that must be adhered to by this supported response.) 573 */ 574 protected MessageDefinition messageTarget; 575 576 /** 577 * Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 578 */ 579 @Child(name = "situation", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 580 @Description(shortDefinition="When should this response be used", formalDefinition="Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses)." ) 581 protected MarkdownType situation; 582 583 private static final long serialVersionUID = 825230127L; 584 585 /** 586 * Constructor 587 */ 588 public MessageDefinitionAllowedResponseComponent() { 589 super(); 590 } 591 592 /** 593 * Constructor 594 */ 595 public MessageDefinitionAllowedResponseComponent(Reference message) { 596 super(); 597 this.message = message; 598 } 599 600 /** 601 * @return {@link #message} (A reference to the message definition that must be adhered to by this supported response.) 602 */ 603 public Reference getMessage() { 604 if (this.message == null) 605 if (Configuration.errorOnAutoCreate()) 606 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.message"); 607 else if (Configuration.doAutoCreate()) 608 this.message = new Reference(); // cc 609 return this.message; 610 } 611 612 public boolean hasMessage() { 613 return this.message != null && !this.message.isEmpty(); 614 } 615 616 /** 617 * @param value {@link #message} (A reference to the message definition that must be adhered to by this supported response.) 618 */ 619 public MessageDefinitionAllowedResponseComponent setMessage(Reference value) { 620 this.message = value; 621 return this; 622 } 623 624 /** 625 * @return {@link #message} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (A reference to the message definition that must be adhered to by this supported response.) 626 */ 627 public MessageDefinition getMessageTarget() { 628 if (this.messageTarget == null) 629 if (Configuration.errorOnAutoCreate()) 630 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.message"); 631 else if (Configuration.doAutoCreate()) 632 this.messageTarget = new MessageDefinition(); // aa 633 return this.messageTarget; 634 } 635 636 /** 637 * @param value {@link #message} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (A reference to the message definition that must be adhered to by this supported response.) 638 */ 639 public MessageDefinitionAllowedResponseComponent setMessageTarget(MessageDefinition value) { 640 this.messageTarget = value; 641 return this; 642 } 643 644 /** 645 * @return {@link #situation} (Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).). This is the underlying object with id, value and extensions. The accessor "getSituation" gives direct access to the value 646 */ 647 public MarkdownType getSituationElement() { 648 if (this.situation == null) 649 if (Configuration.errorOnAutoCreate()) 650 throw new Error("Attempt to auto-create MessageDefinitionAllowedResponseComponent.situation"); 651 else if (Configuration.doAutoCreate()) 652 this.situation = new MarkdownType(); // bb 653 return this.situation; 654 } 655 656 public boolean hasSituationElement() { 657 return this.situation != null && !this.situation.isEmpty(); 658 } 659 660 public boolean hasSituation() { 661 return this.situation != null && !this.situation.isEmpty(); 662 } 663 664 /** 665 * @param value {@link #situation} (Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).). This is the underlying object with id, value and extensions. The accessor "getSituation" gives direct access to the value 666 */ 667 public MessageDefinitionAllowedResponseComponent setSituationElement(MarkdownType value) { 668 this.situation = value; 669 return this; 670 } 671 672 /** 673 * @return Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 674 */ 675 public String getSituation() { 676 return this.situation == null ? null : this.situation.getValue(); 677 } 678 679 /** 680 * @param value Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses). 681 */ 682 public MessageDefinitionAllowedResponseComponent setSituation(String value) { 683 if (value == null) 684 this.situation = null; 685 else { 686 if (this.situation == null) 687 this.situation = new MarkdownType(); 688 this.situation.setValue(value); 689 } 690 return this; 691 } 692 693 protected void listChildren(List<Property> children) { 694 super.listChildren(children); 695 children.add(new Property("message", "Reference(MessageDefinition)", "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message)); 696 children.add(new Property("situation", "markdown", "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 0, 1, situation)); 697 } 698 699 @Override 700 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 701 switch (_hash) { 702 case 954925063: /*message*/ return new Property("message", "Reference(MessageDefinition)", "A reference to the message definition that must be adhered to by this supported response.", 0, 1, message); 703 case -73377282: /*situation*/ return new Property("situation", "markdown", "Provides a description of the circumstances in which this response should be used (as opposed to one of the alternative responses).", 0, 1, situation); 704 default: return super.getNamedProperty(_hash, _name, _checkValid); 705 } 706 707 } 708 709 @Override 710 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 711 switch (hash) { 712 case 954925063: /*message*/ return this.message == null ? new Base[0] : new Base[] {this.message}; // Reference 713 case -73377282: /*situation*/ return this.situation == null ? new Base[0] : new Base[] {this.situation}; // MarkdownType 714 default: return super.getProperty(hash, name, checkValid); 715 } 716 717 } 718 719 @Override 720 public Base setProperty(int hash, String name, Base value) throws FHIRException { 721 switch (hash) { 722 case 954925063: // message 723 this.message = castToReference(value); // Reference 724 return value; 725 case -73377282: // situation 726 this.situation = castToMarkdown(value); // MarkdownType 727 return value; 728 default: return super.setProperty(hash, name, value); 729 } 730 731 } 732 733 @Override 734 public Base setProperty(String name, Base value) throws FHIRException { 735 if (name.equals("message")) { 736 this.message = castToReference(value); // Reference 737 } else if (name.equals("situation")) { 738 this.situation = castToMarkdown(value); // MarkdownType 739 } else 740 return super.setProperty(name, value); 741 return value; 742 } 743 744 @Override 745 public Base makeProperty(int hash, String name) throws FHIRException { 746 switch (hash) { 747 case 954925063: return getMessage(); 748 case -73377282: return getSituationElement(); 749 default: return super.makeProperty(hash, name); 750 } 751 752 } 753 754 @Override 755 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 756 switch (hash) { 757 case 954925063: /*message*/ return new String[] {"Reference"}; 758 case -73377282: /*situation*/ return new String[] {"markdown"}; 759 default: return super.getTypesForProperty(hash, name); 760 } 761 762 } 763 764 @Override 765 public Base addChild(String name) throws FHIRException { 766 if (name.equals("message")) { 767 this.message = new Reference(); 768 return this.message; 769 } 770 else if (name.equals("situation")) { 771 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.situation"); 772 } 773 else 774 return super.addChild(name); 775 } 776 777 public MessageDefinitionAllowedResponseComponent copy() { 778 MessageDefinitionAllowedResponseComponent dst = new MessageDefinitionAllowedResponseComponent(); 779 copyValues(dst); 780 dst.message = message == null ? null : message.copy(); 781 dst.situation = situation == null ? null : situation.copy(); 782 return dst; 783 } 784 785 @Override 786 public boolean equalsDeep(Base other_) { 787 if (!super.equalsDeep(other_)) 788 return false; 789 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 790 return false; 791 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 792 return compareDeep(message, o.message, true) && compareDeep(situation, o.situation, true); 793 } 794 795 @Override 796 public boolean equalsShallow(Base other_) { 797 if (!super.equalsShallow(other_)) 798 return false; 799 if (!(other_ instanceof MessageDefinitionAllowedResponseComponent)) 800 return false; 801 MessageDefinitionAllowedResponseComponent o = (MessageDefinitionAllowedResponseComponent) other_; 802 return compareValues(situation, o.situation, true); 803 } 804 805 public boolean isEmpty() { 806 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(message, situation); 807 } 808 809 public String fhirType() { 810 return "MessageDefinition.allowedResponse"; 811 812 } 813 814 } 815 816 /** 817 * A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance. 818 */ 819 @Child(name = "identifier", type = {Identifier.class}, order=0, min=0, max=1, modifier=false, summary=true) 820 @Description(shortDefinition="Additional identifier for the message definition", formalDefinition="A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance." ) 821 protected Identifier identifier; 822 823 /** 824 * Explaination of why this message definition is needed and why it has been designed as it has. 825 */ 826 @Child(name = "purpose", type = {MarkdownType.class}, order=1, min=0, max=1, modifier=false, summary=true) 827 @Description(shortDefinition="Why this message definition is defined", formalDefinition="Explaination of why this message definition is needed and why it has been designed as it has." ) 828 protected MarkdownType purpose; 829 830 /** 831 * A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 832 */ 833 @Child(name = "copyright", type = {MarkdownType.class}, order=2, min=0, max=1, modifier=false, summary=false) 834 @Description(shortDefinition="Use and/or publishing restrictions", formalDefinition="A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition." ) 835 protected MarkdownType copyright; 836 837 /** 838 * The MessageDefinition that is the basis for the contents of this resource. 839 */ 840 @Child(name = "base", type = {MessageDefinition.class}, order=3, min=0, max=1, modifier=false, summary=true) 841 @Description(shortDefinition="Definition this one is based on", formalDefinition="The MessageDefinition that is the basis for the contents of this resource." ) 842 protected Reference base; 843 844 /** 845 * The actual object that is the target of the reference (The MessageDefinition that is the basis for the contents of this resource.) 846 */ 847 protected MessageDefinition baseTarget; 848 849 /** 850 * Identifies a protocol or workflow that this MessageDefinition represents a step in. 851 */ 852 @Child(name = "parent", type = {ActivityDefinition.class, PlanDefinition.class}, order=4, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 853 @Description(shortDefinition="Protocol/workflow this is part of", formalDefinition="Identifies a protocol or workflow that this MessageDefinition represents a step in." ) 854 protected List<Reference> parent; 855 /** 856 * The actual objects that are the target of the reference (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 857 */ 858 protected List<Resource> parentTarget; 859 860 861 /** 862 * A MessageDefinition that is superseded by this definition. 863 */ 864 @Child(name = "replaces", type = {MessageDefinition.class}, order=5, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 865 @Description(shortDefinition="Takes the place of", formalDefinition="A MessageDefinition that is superseded by this definition." ) 866 protected List<Reference> replaces; 867 /** 868 * The actual objects that are the target of the reference (A MessageDefinition that is superseded by this definition.) 869 */ 870 protected List<MessageDefinition> replacesTarget; 871 872 873 /** 874 * A coded identifier of a supported messaging event. 875 */ 876 @Child(name = "event", type = {Coding.class}, order=6, min=1, max=1, modifier=false, summary=true) 877 @Description(shortDefinition="Event type", formalDefinition="A coded identifier of a supported messaging event." ) 878 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-events") 879 protected Coding event; 880 881 /** 882 * The impact of the content of the message. 883 */ 884 @Child(name = "category", type = {CodeType.class}, order=7, min=0, max=1, modifier=false, summary=true) 885 @Description(shortDefinition="Consequence | Currency | Notification", formalDefinition="The impact of the content of the message." ) 886 @ca.uhn.fhir.model.api.annotation.Binding(valueSet="http://hl7.org/fhir/ValueSet/message-significance-category") 887 protected Enumeration<MessageSignificanceCategory> category; 888 889 /** 890 * Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge. 891 */ 892 @Child(name = "focus", type = {}, order=8, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=true) 893 @Description(shortDefinition="Resource(s) that are the subject of the event", formalDefinition="Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge." ) 894 protected List<MessageDefinitionFocusComponent> focus; 895 896 /** 897 * Indicates whether a response is required for this message. 898 */ 899 @Child(name = "responseRequired", type = {BooleanType.class}, order=9, min=0, max=1, modifier=false, summary=false) 900 @Description(shortDefinition="Is a response required?", formalDefinition="Indicates whether a response is required for this message." ) 901 protected BooleanType responseRequired; 902 903 /** 904 * Indicates what types of messages may be sent as an application-level response to this message. 905 */ 906 @Child(name = "allowedResponse", type = {}, order=10, min=0, max=Child.MAX_UNLIMITED, modifier=false, summary=false) 907 @Description(shortDefinition="Responses to this message", formalDefinition="Indicates what types of messages may be sent as an application-level response to this message." ) 908 protected List<MessageDefinitionAllowedResponseComponent> allowedResponse; 909 910 private static final long serialVersionUID = -219916580L; 911 912 /** 913 * Constructor 914 */ 915 public MessageDefinition() { 916 super(); 917 } 918 919 /** 920 * Constructor 921 */ 922 public MessageDefinition(Enumeration<PublicationStatus> status, DateTimeType date, Coding event) { 923 super(); 924 this.status = status; 925 this.date = date; 926 this.event = event; 927 } 928 929 /** 930 * @return {@link #url} (An absolute URI that is used to identify this message definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this message definition is (or will be) published. The URL SHOULD include the major version of the message definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 931 */ 932 public UriType getUrlElement() { 933 if (this.url == null) 934 if (Configuration.errorOnAutoCreate()) 935 throw new Error("Attempt to auto-create MessageDefinition.url"); 936 else if (Configuration.doAutoCreate()) 937 this.url = new UriType(); // bb 938 return this.url; 939 } 940 941 public boolean hasUrlElement() { 942 return this.url != null && !this.url.isEmpty(); 943 } 944 945 public boolean hasUrl() { 946 return this.url != null && !this.url.isEmpty(); 947 } 948 949 /** 950 * @param value {@link #url} (An absolute URI that is used to identify this message definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this message definition is (or will be) published. The URL SHOULD include the major version of the message definition. For more information see [Technical and Business Versions](resource.html#versions).). This is the underlying object with id, value and extensions. The accessor "getUrl" gives direct access to the value 951 */ 952 public MessageDefinition setUrlElement(UriType value) { 953 this.url = value; 954 return this; 955 } 956 957 /** 958 * @return An absolute URI that is used to identify this message definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this message definition is (or will be) published. The URL SHOULD include the major version of the message definition. For more information see [Technical and Business Versions](resource.html#versions). 959 */ 960 public String getUrl() { 961 return this.url == null ? null : this.url.getValue(); 962 } 963 964 /** 965 * @param value An absolute URI that is used to identify this message definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this message definition is (or will be) published. The URL SHOULD include the major version of the message definition. For more information see [Technical and Business Versions](resource.html#versions). 966 */ 967 public MessageDefinition setUrl(String value) { 968 if (Utilities.noString(value)) 969 this.url = null; 970 else { 971 if (this.url == null) 972 this.url = new UriType(); 973 this.url.setValue(value); 974 } 975 return this; 976 } 977 978 /** 979 * @return {@link #identifier} (A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 980 */ 981 public Identifier getIdentifier() { 982 if (this.identifier == null) 983 if (Configuration.errorOnAutoCreate()) 984 throw new Error("Attempt to auto-create MessageDefinition.identifier"); 985 else if (Configuration.doAutoCreate()) 986 this.identifier = new Identifier(); // cc 987 return this.identifier; 988 } 989 990 public boolean hasIdentifier() { 991 return this.identifier != null && !this.identifier.isEmpty(); 992 } 993 994 /** 995 * @param value {@link #identifier} (A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.) 996 */ 997 public MessageDefinition setIdentifier(Identifier value) { 998 this.identifier = value; 999 return this; 1000 } 1001 1002 /** 1003 * @return {@link #version} (The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1004 */ 1005 public StringType getVersionElement() { 1006 if (this.version == null) 1007 if (Configuration.errorOnAutoCreate()) 1008 throw new Error("Attempt to auto-create MessageDefinition.version"); 1009 else if (Configuration.doAutoCreate()) 1010 this.version = new StringType(); // bb 1011 return this.version; 1012 } 1013 1014 public boolean hasVersionElement() { 1015 return this.version != null && !this.version.isEmpty(); 1016 } 1017 1018 public boolean hasVersion() { 1019 return this.version != null && !this.version.isEmpty(); 1020 } 1021 1022 /** 1023 * @param value {@link #version} (The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.). This is the underlying object with id, value and extensions. The accessor "getVersion" gives direct access to the value 1024 */ 1025 public MessageDefinition setVersionElement(StringType value) { 1026 this.version = value; 1027 return this; 1028 } 1029 1030 /** 1031 * @return The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1032 */ 1033 public String getVersion() { 1034 return this.version == null ? null : this.version.getValue(); 1035 } 1036 1037 /** 1038 * @param value The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence. 1039 */ 1040 public MessageDefinition setVersion(String value) { 1041 if (Utilities.noString(value)) 1042 this.version = null; 1043 else { 1044 if (this.version == null) 1045 this.version = new StringType(); 1046 this.version.setValue(value); 1047 } 1048 return this; 1049 } 1050 1051 /** 1052 * @return {@link #name} (A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1053 */ 1054 public StringType getNameElement() { 1055 if (this.name == null) 1056 if (Configuration.errorOnAutoCreate()) 1057 throw new Error("Attempt to auto-create MessageDefinition.name"); 1058 else if (Configuration.doAutoCreate()) 1059 this.name = new StringType(); // bb 1060 return this.name; 1061 } 1062 1063 public boolean hasNameElement() { 1064 return this.name != null && !this.name.isEmpty(); 1065 } 1066 1067 public boolean hasName() { 1068 return this.name != null && !this.name.isEmpty(); 1069 } 1070 1071 /** 1072 * @param value {@link #name} (A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.). This is the underlying object with id, value and extensions. The accessor "getName" gives direct access to the value 1073 */ 1074 public MessageDefinition setNameElement(StringType value) { 1075 this.name = value; 1076 return this; 1077 } 1078 1079 /** 1080 * @return A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1081 */ 1082 public String getName() { 1083 return this.name == null ? null : this.name.getValue(); 1084 } 1085 1086 /** 1087 * @param value A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation. 1088 */ 1089 public MessageDefinition setName(String value) { 1090 if (Utilities.noString(value)) 1091 this.name = null; 1092 else { 1093 if (this.name == null) 1094 this.name = new StringType(); 1095 this.name.setValue(value); 1096 } 1097 return this; 1098 } 1099 1100 /** 1101 * @return {@link #title} (A short, descriptive, user-friendly title for the message definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1102 */ 1103 public StringType getTitleElement() { 1104 if (this.title == null) 1105 if (Configuration.errorOnAutoCreate()) 1106 throw new Error("Attempt to auto-create MessageDefinition.title"); 1107 else if (Configuration.doAutoCreate()) 1108 this.title = new StringType(); // bb 1109 return this.title; 1110 } 1111 1112 public boolean hasTitleElement() { 1113 return this.title != null && !this.title.isEmpty(); 1114 } 1115 1116 public boolean hasTitle() { 1117 return this.title != null && !this.title.isEmpty(); 1118 } 1119 1120 /** 1121 * @param value {@link #title} (A short, descriptive, user-friendly title for the message definition.). This is the underlying object with id, value and extensions. The accessor "getTitle" gives direct access to the value 1122 */ 1123 public MessageDefinition setTitleElement(StringType value) { 1124 this.title = value; 1125 return this; 1126 } 1127 1128 /** 1129 * @return A short, descriptive, user-friendly title for the message definition. 1130 */ 1131 public String getTitle() { 1132 return this.title == null ? null : this.title.getValue(); 1133 } 1134 1135 /** 1136 * @param value A short, descriptive, user-friendly title for the message definition. 1137 */ 1138 public MessageDefinition setTitle(String value) { 1139 if (Utilities.noString(value)) 1140 this.title = null; 1141 else { 1142 if (this.title == null) 1143 this.title = new StringType(); 1144 this.title.setValue(value); 1145 } 1146 return this; 1147 } 1148 1149 /** 1150 * @return {@link #status} (The status of this message definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1151 */ 1152 public Enumeration<PublicationStatus> getStatusElement() { 1153 if (this.status == null) 1154 if (Configuration.errorOnAutoCreate()) 1155 throw new Error("Attempt to auto-create MessageDefinition.status"); 1156 else if (Configuration.doAutoCreate()) 1157 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); // bb 1158 return this.status; 1159 } 1160 1161 public boolean hasStatusElement() { 1162 return this.status != null && !this.status.isEmpty(); 1163 } 1164 1165 public boolean hasStatus() { 1166 return this.status != null && !this.status.isEmpty(); 1167 } 1168 1169 /** 1170 * @param value {@link #status} (The status of this message definition. Enables tracking the life-cycle of the content.). This is the underlying object with id, value and extensions. The accessor "getStatus" gives direct access to the value 1171 */ 1172 public MessageDefinition setStatusElement(Enumeration<PublicationStatus> value) { 1173 this.status = value; 1174 return this; 1175 } 1176 1177 /** 1178 * @return The status of this message definition. Enables tracking the life-cycle of the content. 1179 */ 1180 public PublicationStatus getStatus() { 1181 return this.status == null ? null : this.status.getValue(); 1182 } 1183 1184 /** 1185 * @param value The status of this message definition. Enables tracking the life-cycle of the content. 1186 */ 1187 public MessageDefinition setStatus(PublicationStatus value) { 1188 if (this.status == null) 1189 this.status = new Enumeration<PublicationStatus>(new PublicationStatusEnumFactory()); 1190 this.status.setValue(value); 1191 return this; 1192 } 1193 1194 /** 1195 * @return {@link #experimental} (A boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1196 */ 1197 public BooleanType getExperimentalElement() { 1198 if (this.experimental == null) 1199 if (Configuration.errorOnAutoCreate()) 1200 throw new Error("Attempt to auto-create MessageDefinition.experimental"); 1201 else if (Configuration.doAutoCreate()) 1202 this.experimental = new BooleanType(); // bb 1203 return this.experimental; 1204 } 1205 1206 public boolean hasExperimentalElement() { 1207 return this.experimental != null && !this.experimental.isEmpty(); 1208 } 1209 1210 public boolean hasExperimental() { 1211 return this.experimental != null && !this.experimental.isEmpty(); 1212 } 1213 1214 /** 1215 * @param value {@link #experimental} (A boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.). This is the underlying object with id, value and extensions. The accessor "getExperimental" gives direct access to the value 1216 */ 1217 public MessageDefinition setExperimentalElement(BooleanType value) { 1218 this.experimental = value; 1219 return this; 1220 } 1221 1222 /** 1223 * @return A boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1224 */ 1225 public boolean getExperimental() { 1226 return this.experimental == null || this.experimental.isEmpty() ? false : this.experimental.getValue(); 1227 } 1228 1229 /** 1230 * @param value A boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage. 1231 */ 1232 public MessageDefinition setExperimental(boolean value) { 1233 if (this.experimental == null) 1234 this.experimental = new BooleanType(); 1235 this.experimental.setValue(value); 1236 return this; 1237 } 1238 1239 /** 1240 * @return {@link #date} (The date (and optionally time) when the message definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1241 */ 1242 public DateTimeType getDateElement() { 1243 if (this.date == null) 1244 if (Configuration.errorOnAutoCreate()) 1245 throw new Error("Attempt to auto-create MessageDefinition.date"); 1246 else if (Configuration.doAutoCreate()) 1247 this.date = new DateTimeType(); // bb 1248 return this.date; 1249 } 1250 1251 public boolean hasDateElement() { 1252 return this.date != null && !this.date.isEmpty(); 1253 } 1254 1255 public boolean hasDate() { 1256 return this.date != null && !this.date.isEmpty(); 1257 } 1258 1259 /** 1260 * @param value {@link #date} (The date (and optionally time) when the message definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.). This is the underlying object with id, value and extensions. The accessor "getDate" gives direct access to the value 1261 */ 1262 public MessageDefinition setDateElement(DateTimeType value) { 1263 this.date = value; 1264 return this; 1265 } 1266 1267 /** 1268 * @return The date (and optionally time) when the message definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes. 1269 */ 1270 public Date getDate() { 1271 return this.date == null ? null : this.date.getValue(); 1272 } 1273 1274 /** 1275 * @param value The date (and optionally time) when the message definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes. 1276 */ 1277 public MessageDefinition setDate(Date value) { 1278 if (this.date == null) 1279 this.date = new DateTimeType(); 1280 this.date.setValue(value); 1281 return this; 1282 } 1283 1284 /** 1285 * @return {@link #publisher} (The name of the individual or organization that published the message definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1286 */ 1287 public StringType getPublisherElement() { 1288 if (this.publisher == null) 1289 if (Configuration.errorOnAutoCreate()) 1290 throw new Error("Attempt to auto-create MessageDefinition.publisher"); 1291 else if (Configuration.doAutoCreate()) 1292 this.publisher = new StringType(); // bb 1293 return this.publisher; 1294 } 1295 1296 public boolean hasPublisherElement() { 1297 return this.publisher != null && !this.publisher.isEmpty(); 1298 } 1299 1300 public boolean hasPublisher() { 1301 return this.publisher != null && !this.publisher.isEmpty(); 1302 } 1303 1304 /** 1305 * @param value {@link #publisher} (The name of the individual or organization that published the message definition.). This is the underlying object with id, value and extensions. The accessor "getPublisher" gives direct access to the value 1306 */ 1307 public MessageDefinition setPublisherElement(StringType value) { 1308 this.publisher = value; 1309 return this; 1310 } 1311 1312 /** 1313 * @return The name of the individual or organization that published the message definition. 1314 */ 1315 public String getPublisher() { 1316 return this.publisher == null ? null : this.publisher.getValue(); 1317 } 1318 1319 /** 1320 * @param value The name of the individual or organization that published the message definition. 1321 */ 1322 public MessageDefinition setPublisher(String value) { 1323 if (Utilities.noString(value)) 1324 this.publisher = null; 1325 else { 1326 if (this.publisher == null) 1327 this.publisher = new StringType(); 1328 this.publisher.setValue(value); 1329 } 1330 return this; 1331 } 1332 1333 /** 1334 * @return {@link #contact} (Contact details to assist a user in finding and communicating with the publisher.) 1335 */ 1336 public List<ContactDetail> getContact() { 1337 if (this.contact == null) 1338 this.contact = new ArrayList<ContactDetail>(); 1339 return this.contact; 1340 } 1341 1342 /** 1343 * @return Returns a reference to <code>this</code> for easy method chaining 1344 */ 1345 public MessageDefinition setContact(List<ContactDetail> theContact) { 1346 this.contact = theContact; 1347 return this; 1348 } 1349 1350 public boolean hasContact() { 1351 if (this.contact == null) 1352 return false; 1353 for (ContactDetail item : this.contact) 1354 if (!item.isEmpty()) 1355 return true; 1356 return false; 1357 } 1358 1359 public ContactDetail addContact() { //3 1360 ContactDetail t = new ContactDetail(); 1361 if (this.contact == null) 1362 this.contact = new ArrayList<ContactDetail>(); 1363 this.contact.add(t); 1364 return t; 1365 } 1366 1367 public MessageDefinition addContact(ContactDetail t) { //3 1368 if (t == null) 1369 return this; 1370 if (this.contact == null) 1371 this.contact = new ArrayList<ContactDetail>(); 1372 this.contact.add(t); 1373 return this; 1374 } 1375 1376 /** 1377 * @return The first repetition of repeating field {@link #contact}, creating it if it does not already exist 1378 */ 1379 public ContactDetail getContactFirstRep() { 1380 if (getContact().isEmpty()) { 1381 addContact(); 1382 } 1383 return getContact().get(0); 1384 } 1385 1386 /** 1387 * @return {@link #description} (A free text natural language description of the message definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1388 */ 1389 public MarkdownType getDescriptionElement() { 1390 if (this.description == null) 1391 if (Configuration.errorOnAutoCreate()) 1392 throw new Error("Attempt to auto-create MessageDefinition.description"); 1393 else if (Configuration.doAutoCreate()) 1394 this.description = new MarkdownType(); // bb 1395 return this.description; 1396 } 1397 1398 public boolean hasDescriptionElement() { 1399 return this.description != null && !this.description.isEmpty(); 1400 } 1401 1402 public boolean hasDescription() { 1403 return this.description != null && !this.description.isEmpty(); 1404 } 1405 1406 /** 1407 * @param value {@link #description} (A free text natural language description of the message definition from a consumer's perspective.). This is the underlying object with id, value and extensions. The accessor "getDescription" gives direct access to the value 1408 */ 1409 public MessageDefinition setDescriptionElement(MarkdownType value) { 1410 this.description = value; 1411 return this; 1412 } 1413 1414 /** 1415 * @return A free text natural language description of the message definition from a consumer's perspective. 1416 */ 1417 public String getDescription() { 1418 return this.description == null ? null : this.description.getValue(); 1419 } 1420 1421 /** 1422 * @param value A free text natural language description of the message definition from a consumer's perspective. 1423 */ 1424 public MessageDefinition setDescription(String value) { 1425 if (value == null) 1426 this.description = null; 1427 else { 1428 if (this.description == null) 1429 this.description = new MarkdownType(); 1430 this.description.setValue(value); 1431 } 1432 return this; 1433 } 1434 1435 /** 1436 * @return {@link #useContext} (The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate message definition instances.) 1437 */ 1438 public List<UsageContext> getUseContext() { 1439 if (this.useContext == null) 1440 this.useContext = new ArrayList<UsageContext>(); 1441 return this.useContext; 1442 } 1443 1444 /** 1445 * @return Returns a reference to <code>this</code> for easy method chaining 1446 */ 1447 public MessageDefinition setUseContext(List<UsageContext> theUseContext) { 1448 this.useContext = theUseContext; 1449 return this; 1450 } 1451 1452 public boolean hasUseContext() { 1453 if (this.useContext == null) 1454 return false; 1455 for (UsageContext item : this.useContext) 1456 if (!item.isEmpty()) 1457 return true; 1458 return false; 1459 } 1460 1461 public UsageContext addUseContext() { //3 1462 UsageContext t = new UsageContext(); 1463 if (this.useContext == null) 1464 this.useContext = new ArrayList<UsageContext>(); 1465 this.useContext.add(t); 1466 return t; 1467 } 1468 1469 public MessageDefinition addUseContext(UsageContext t) { //3 1470 if (t == null) 1471 return this; 1472 if (this.useContext == null) 1473 this.useContext = new ArrayList<UsageContext>(); 1474 this.useContext.add(t); 1475 return this; 1476 } 1477 1478 /** 1479 * @return The first repetition of repeating field {@link #useContext}, creating it if it does not already exist 1480 */ 1481 public UsageContext getUseContextFirstRep() { 1482 if (getUseContext().isEmpty()) { 1483 addUseContext(); 1484 } 1485 return getUseContext().get(0); 1486 } 1487 1488 /** 1489 * @return {@link #jurisdiction} (A legal or geographic region in which the message definition is intended to be used.) 1490 */ 1491 public List<CodeableConcept> getJurisdiction() { 1492 if (this.jurisdiction == null) 1493 this.jurisdiction = new ArrayList<CodeableConcept>(); 1494 return this.jurisdiction; 1495 } 1496 1497 /** 1498 * @return Returns a reference to <code>this</code> for easy method chaining 1499 */ 1500 public MessageDefinition setJurisdiction(List<CodeableConcept> theJurisdiction) { 1501 this.jurisdiction = theJurisdiction; 1502 return this; 1503 } 1504 1505 public boolean hasJurisdiction() { 1506 if (this.jurisdiction == null) 1507 return false; 1508 for (CodeableConcept item : this.jurisdiction) 1509 if (!item.isEmpty()) 1510 return true; 1511 return false; 1512 } 1513 1514 public CodeableConcept addJurisdiction() { //3 1515 CodeableConcept t = new CodeableConcept(); 1516 if (this.jurisdiction == null) 1517 this.jurisdiction = new ArrayList<CodeableConcept>(); 1518 this.jurisdiction.add(t); 1519 return t; 1520 } 1521 1522 public MessageDefinition addJurisdiction(CodeableConcept t) { //3 1523 if (t == null) 1524 return this; 1525 if (this.jurisdiction == null) 1526 this.jurisdiction = new ArrayList<CodeableConcept>(); 1527 this.jurisdiction.add(t); 1528 return this; 1529 } 1530 1531 /** 1532 * @return The first repetition of repeating field {@link #jurisdiction}, creating it if it does not already exist 1533 */ 1534 public CodeableConcept getJurisdictionFirstRep() { 1535 if (getJurisdiction().isEmpty()) { 1536 addJurisdiction(); 1537 } 1538 return getJurisdiction().get(0); 1539 } 1540 1541 /** 1542 * @return {@link #purpose} (Explaination of why this message definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1543 */ 1544 public MarkdownType getPurposeElement() { 1545 if (this.purpose == null) 1546 if (Configuration.errorOnAutoCreate()) 1547 throw new Error("Attempt to auto-create MessageDefinition.purpose"); 1548 else if (Configuration.doAutoCreate()) 1549 this.purpose = new MarkdownType(); // bb 1550 return this.purpose; 1551 } 1552 1553 public boolean hasPurposeElement() { 1554 return this.purpose != null && !this.purpose.isEmpty(); 1555 } 1556 1557 public boolean hasPurpose() { 1558 return this.purpose != null && !this.purpose.isEmpty(); 1559 } 1560 1561 /** 1562 * @param value {@link #purpose} (Explaination of why this message definition is needed and why it has been designed as it has.). This is the underlying object with id, value and extensions. The accessor "getPurpose" gives direct access to the value 1563 */ 1564 public MessageDefinition setPurposeElement(MarkdownType value) { 1565 this.purpose = value; 1566 return this; 1567 } 1568 1569 /** 1570 * @return Explaination of why this message definition is needed and why it has been designed as it has. 1571 */ 1572 public String getPurpose() { 1573 return this.purpose == null ? null : this.purpose.getValue(); 1574 } 1575 1576 /** 1577 * @param value Explaination of why this message definition is needed and why it has been designed as it has. 1578 */ 1579 public MessageDefinition setPurpose(String value) { 1580 if (value == null) 1581 this.purpose = null; 1582 else { 1583 if (this.purpose == null) 1584 this.purpose = new MarkdownType(); 1585 this.purpose.setValue(value); 1586 } 1587 return this; 1588 } 1589 1590 /** 1591 * @return {@link #copyright} (A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1592 */ 1593 public MarkdownType getCopyrightElement() { 1594 if (this.copyright == null) 1595 if (Configuration.errorOnAutoCreate()) 1596 throw new Error("Attempt to auto-create MessageDefinition.copyright"); 1597 else if (Configuration.doAutoCreate()) 1598 this.copyright = new MarkdownType(); // bb 1599 return this.copyright; 1600 } 1601 1602 public boolean hasCopyrightElement() { 1603 return this.copyright != null && !this.copyright.isEmpty(); 1604 } 1605 1606 public boolean hasCopyright() { 1607 return this.copyright != null && !this.copyright.isEmpty(); 1608 } 1609 1610 /** 1611 * @param value {@link #copyright} (A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.). This is the underlying object with id, value and extensions. The accessor "getCopyright" gives direct access to the value 1612 */ 1613 public MessageDefinition setCopyrightElement(MarkdownType value) { 1614 this.copyright = value; 1615 return this; 1616 } 1617 1618 /** 1619 * @return A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 1620 */ 1621 public String getCopyright() { 1622 return this.copyright == null ? null : this.copyright.getValue(); 1623 } 1624 1625 /** 1626 * @param value A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition. 1627 */ 1628 public MessageDefinition setCopyright(String value) { 1629 if (value == null) 1630 this.copyright = null; 1631 else { 1632 if (this.copyright == null) 1633 this.copyright = new MarkdownType(); 1634 this.copyright.setValue(value); 1635 } 1636 return this; 1637 } 1638 1639 /** 1640 * @return {@link #base} (The MessageDefinition that is the basis for the contents of this resource.) 1641 */ 1642 public Reference getBase() { 1643 if (this.base == null) 1644 if (Configuration.errorOnAutoCreate()) 1645 throw new Error("Attempt to auto-create MessageDefinition.base"); 1646 else if (Configuration.doAutoCreate()) 1647 this.base = new Reference(); // cc 1648 return this.base; 1649 } 1650 1651 public boolean hasBase() { 1652 return this.base != null && !this.base.isEmpty(); 1653 } 1654 1655 /** 1656 * @param value {@link #base} (The MessageDefinition that is the basis for the contents of this resource.) 1657 */ 1658 public MessageDefinition setBase(Reference value) { 1659 this.base = value; 1660 return this; 1661 } 1662 1663 /** 1664 * @return {@link #base} The actual object that is the target of the reference. The reference library doesn't populate this, but you can use it to hold the resource if you resolve it. (The MessageDefinition that is the basis for the contents of this resource.) 1665 */ 1666 public MessageDefinition getBaseTarget() { 1667 if (this.baseTarget == null) 1668 if (Configuration.errorOnAutoCreate()) 1669 throw new Error("Attempt to auto-create MessageDefinition.base"); 1670 else if (Configuration.doAutoCreate()) 1671 this.baseTarget = new MessageDefinition(); // aa 1672 return this.baseTarget; 1673 } 1674 1675 /** 1676 * @param value {@link #base} The actual object that is the target of the reference. The reference library doesn't use these, but you can use it to hold the resource if you resolve it. (The MessageDefinition that is the basis for the contents of this resource.) 1677 */ 1678 public MessageDefinition setBaseTarget(MessageDefinition value) { 1679 this.baseTarget = value; 1680 return this; 1681 } 1682 1683 /** 1684 * @return {@link #parent} (Identifies a protocol or workflow that this MessageDefinition represents a step in.) 1685 */ 1686 public List<Reference> getParent() { 1687 if (this.parent == null) 1688 this.parent = new ArrayList<Reference>(); 1689 return this.parent; 1690 } 1691 1692 /** 1693 * @return Returns a reference to <code>this</code> for easy method chaining 1694 */ 1695 public MessageDefinition setParent(List<Reference> theParent) { 1696 this.parent = theParent; 1697 return this; 1698 } 1699 1700 public boolean hasParent() { 1701 if (this.parent == null) 1702 return false; 1703 for (Reference item : this.parent) 1704 if (!item.isEmpty()) 1705 return true; 1706 return false; 1707 } 1708 1709 public Reference addParent() { //3 1710 Reference t = new Reference(); 1711 if (this.parent == null) 1712 this.parent = new ArrayList<Reference>(); 1713 this.parent.add(t); 1714 return t; 1715 } 1716 1717 public MessageDefinition addParent(Reference t) { //3 1718 if (t == null) 1719 return this; 1720 if (this.parent == null) 1721 this.parent = new ArrayList<Reference>(); 1722 this.parent.add(t); 1723 return this; 1724 } 1725 1726 /** 1727 * @return The first repetition of repeating field {@link #parent}, creating it if it does not already exist 1728 */ 1729 public Reference getParentFirstRep() { 1730 if (getParent().isEmpty()) { 1731 addParent(); 1732 } 1733 return getParent().get(0); 1734 } 1735 1736 /** 1737 * @deprecated Use Reference#setResource(IBaseResource) instead 1738 */ 1739 @Deprecated 1740 public List<Resource> getParentTarget() { 1741 if (this.parentTarget == null) 1742 this.parentTarget = new ArrayList<Resource>(); 1743 return this.parentTarget; 1744 } 1745 1746 /** 1747 * @return {@link #replaces} (A MessageDefinition that is superseded by this definition.) 1748 */ 1749 public List<Reference> getReplaces() { 1750 if (this.replaces == null) 1751 this.replaces = new ArrayList<Reference>(); 1752 return this.replaces; 1753 } 1754 1755 /** 1756 * @return Returns a reference to <code>this</code> for easy method chaining 1757 */ 1758 public MessageDefinition setReplaces(List<Reference> theReplaces) { 1759 this.replaces = theReplaces; 1760 return this; 1761 } 1762 1763 public boolean hasReplaces() { 1764 if (this.replaces == null) 1765 return false; 1766 for (Reference item : this.replaces) 1767 if (!item.isEmpty()) 1768 return true; 1769 return false; 1770 } 1771 1772 public Reference addReplaces() { //3 1773 Reference t = new Reference(); 1774 if (this.replaces == null) 1775 this.replaces = new ArrayList<Reference>(); 1776 this.replaces.add(t); 1777 return t; 1778 } 1779 1780 public MessageDefinition addReplaces(Reference t) { //3 1781 if (t == null) 1782 return this; 1783 if (this.replaces == null) 1784 this.replaces = new ArrayList<Reference>(); 1785 this.replaces.add(t); 1786 return this; 1787 } 1788 1789 /** 1790 * @return The first repetition of repeating field {@link #replaces}, creating it if it does not already exist 1791 */ 1792 public Reference getReplacesFirstRep() { 1793 if (getReplaces().isEmpty()) { 1794 addReplaces(); 1795 } 1796 return getReplaces().get(0); 1797 } 1798 1799 /** 1800 * @deprecated Use Reference#setResource(IBaseResource) instead 1801 */ 1802 @Deprecated 1803 public List<MessageDefinition> getReplacesTarget() { 1804 if (this.replacesTarget == null) 1805 this.replacesTarget = new ArrayList<MessageDefinition>(); 1806 return this.replacesTarget; 1807 } 1808 1809 /** 1810 * @deprecated Use Reference#setResource(IBaseResource) instead 1811 */ 1812 @Deprecated 1813 public MessageDefinition addReplacesTarget() { 1814 MessageDefinition r = new MessageDefinition(); 1815 if (this.replacesTarget == null) 1816 this.replacesTarget = new ArrayList<MessageDefinition>(); 1817 this.replacesTarget.add(r); 1818 return r; 1819 } 1820 1821 /** 1822 * @return {@link #event} (A coded identifier of a supported messaging event.) 1823 */ 1824 public Coding getEvent() { 1825 if (this.event == null) 1826 if (Configuration.errorOnAutoCreate()) 1827 throw new Error("Attempt to auto-create MessageDefinition.event"); 1828 else if (Configuration.doAutoCreate()) 1829 this.event = new Coding(); // cc 1830 return this.event; 1831 } 1832 1833 public boolean hasEvent() { 1834 return this.event != null && !this.event.isEmpty(); 1835 } 1836 1837 /** 1838 * @param value {@link #event} (A coded identifier of a supported messaging event.) 1839 */ 1840 public MessageDefinition setEvent(Coding value) { 1841 this.event = value; 1842 return this; 1843 } 1844 1845 /** 1846 * @return {@link #category} (The impact of the content of the message.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 1847 */ 1848 public Enumeration<MessageSignificanceCategory> getCategoryElement() { 1849 if (this.category == null) 1850 if (Configuration.errorOnAutoCreate()) 1851 throw new Error("Attempt to auto-create MessageDefinition.category"); 1852 else if (Configuration.doAutoCreate()) 1853 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); // bb 1854 return this.category; 1855 } 1856 1857 public boolean hasCategoryElement() { 1858 return this.category != null && !this.category.isEmpty(); 1859 } 1860 1861 public boolean hasCategory() { 1862 return this.category != null && !this.category.isEmpty(); 1863 } 1864 1865 /** 1866 * @param value {@link #category} (The impact of the content of the message.). This is the underlying object with id, value and extensions. The accessor "getCategory" gives direct access to the value 1867 */ 1868 public MessageDefinition setCategoryElement(Enumeration<MessageSignificanceCategory> value) { 1869 this.category = value; 1870 return this; 1871 } 1872 1873 /** 1874 * @return The impact of the content of the message. 1875 */ 1876 public MessageSignificanceCategory getCategory() { 1877 return this.category == null ? null : this.category.getValue(); 1878 } 1879 1880 /** 1881 * @param value The impact of the content of the message. 1882 */ 1883 public MessageDefinition setCategory(MessageSignificanceCategory value) { 1884 if (value == null) 1885 this.category = null; 1886 else { 1887 if (this.category == null) 1888 this.category = new Enumeration<MessageSignificanceCategory>(new MessageSignificanceCategoryEnumFactory()); 1889 this.category.setValue(value); 1890 } 1891 return this; 1892 } 1893 1894 /** 1895 * @return {@link #focus} (Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.) 1896 */ 1897 public List<MessageDefinitionFocusComponent> getFocus() { 1898 if (this.focus == null) 1899 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 1900 return this.focus; 1901 } 1902 1903 /** 1904 * @return Returns a reference to <code>this</code> for easy method chaining 1905 */ 1906 public MessageDefinition setFocus(List<MessageDefinitionFocusComponent> theFocus) { 1907 this.focus = theFocus; 1908 return this; 1909 } 1910 1911 public boolean hasFocus() { 1912 if (this.focus == null) 1913 return false; 1914 for (MessageDefinitionFocusComponent item : this.focus) 1915 if (!item.isEmpty()) 1916 return true; 1917 return false; 1918 } 1919 1920 public MessageDefinitionFocusComponent addFocus() { //3 1921 MessageDefinitionFocusComponent t = new MessageDefinitionFocusComponent(); 1922 if (this.focus == null) 1923 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 1924 this.focus.add(t); 1925 return t; 1926 } 1927 1928 public MessageDefinition addFocus(MessageDefinitionFocusComponent t) { //3 1929 if (t == null) 1930 return this; 1931 if (this.focus == null) 1932 this.focus = new ArrayList<MessageDefinitionFocusComponent>(); 1933 this.focus.add(t); 1934 return this; 1935 } 1936 1937 /** 1938 * @return The first repetition of repeating field {@link #focus}, creating it if it does not already exist 1939 */ 1940 public MessageDefinitionFocusComponent getFocusFirstRep() { 1941 if (getFocus().isEmpty()) { 1942 addFocus(); 1943 } 1944 return getFocus().get(0); 1945 } 1946 1947 /** 1948 * @return {@link #responseRequired} (Indicates whether a response is required for this message.). This is the underlying object with id, value and extensions. The accessor "getResponseRequired" gives direct access to the value 1949 */ 1950 public BooleanType getResponseRequiredElement() { 1951 if (this.responseRequired == null) 1952 if (Configuration.errorOnAutoCreate()) 1953 throw new Error("Attempt to auto-create MessageDefinition.responseRequired"); 1954 else if (Configuration.doAutoCreate()) 1955 this.responseRequired = new BooleanType(); // bb 1956 return this.responseRequired; 1957 } 1958 1959 public boolean hasResponseRequiredElement() { 1960 return this.responseRequired != null && !this.responseRequired.isEmpty(); 1961 } 1962 1963 public boolean hasResponseRequired() { 1964 return this.responseRequired != null && !this.responseRequired.isEmpty(); 1965 } 1966 1967 /** 1968 * @param value {@link #responseRequired} (Indicates whether a response is required for this message.). This is the underlying object with id, value and extensions. The accessor "getResponseRequired" gives direct access to the value 1969 */ 1970 public MessageDefinition setResponseRequiredElement(BooleanType value) { 1971 this.responseRequired = value; 1972 return this; 1973 } 1974 1975 /** 1976 * @return Indicates whether a response is required for this message. 1977 */ 1978 public boolean getResponseRequired() { 1979 return this.responseRequired == null || this.responseRequired.isEmpty() ? false : this.responseRequired.getValue(); 1980 } 1981 1982 /** 1983 * @param value Indicates whether a response is required for this message. 1984 */ 1985 public MessageDefinition setResponseRequired(boolean value) { 1986 if (this.responseRequired == null) 1987 this.responseRequired = new BooleanType(); 1988 this.responseRequired.setValue(value); 1989 return this; 1990 } 1991 1992 /** 1993 * @return {@link #allowedResponse} (Indicates what types of messages may be sent as an application-level response to this message.) 1994 */ 1995 public List<MessageDefinitionAllowedResponseComponent> getAllowedResponse() { 1996 if (this.allowedResponse == null) 1997 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 1998 return this.allowedResponse; 1999 } 2000 2001 /** 2002 * @return Returns a reference to <code>this</code> for easy method chaining 2003 */ 2004 public MessageDefinition setAllowedResponse(List<MessageDefinitionAllowedResponseComponent> theAllowedResponse) { 2005 this.allowedResponse = theAllowedResponse; 2006 return this; 2007 } 2008 2009 public boolean hasAllowedResponse() { 2010 if (this.allowedResponse == null) 2011 return false; 2012 for (MessageDefinitionAllowedResponseComponent item : this.allowedResponse) 2013 if (!item.isEmpty()) 2014 return true; 2015 return false; 2016 } 2017 2018 public MessageDefinitionAllowedResponseComponent addAllowedResponse() { //3 2019 MessageDefinitionAllowedResponseComponent t = new MessageDefinitionAllowedResponseComponent(); 2020 if (this.allowedResponse == null) 2021 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2022 this.allowedResponse.add(t); 2023 return t; 2024 } 2025 2026 public MessageDefinition addAllowedResponse(MessageDefinitionAllowedResponseComponent t) { //3 2027 if (t == null) 2028 return this; 2029 if (this.allowedResponse == null) 2030 this.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2031 this.allowedResponse.add(t); 2032 return this; 2033 } 2034 2035 /** 2036 * @return The first repetition of repeating field {@link #allowedResponse}, creating it if it does not already exist 2037 */ 2038 public MessageDefinitionAllowedResponseComponent getAllowedResponseFirstRep() { 2039 if (getAllowedResponse().isEmpty()) { 2040 addAllowedResponse(); 2041 } 2042 return getAllowedResponse().get(0); 2043 } 2044 2045 protected void listChildren(List<Property> children) { 2046 super.listChildren(children); 2047 children.add(new Property("url", "uri", "An absolute URI that is used to identify this message definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this message definition is (or will be) published. The URL SHOULD include the major version of the message definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url)); 2048 children.add(new Property("identifier", "Identifier", "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier)); 2049 children.add(new Property("version", "string", "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version)); 2050 children.add(new Property("name", "string", "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name)); 2051 children.add(new Property("title", "string", "A short, descriptive, user-friendly title for the message definition.", 0, 1, title)); 2052 children.add(new Property("status", "code", "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status)); 2053 children.add(new Property("experimental", "boolean", "A boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental)); 2054 children.add(new Property("date", "dateTime", "The date (and optionally time) when the message definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 0, 1, date)); 2055 children.add(new Property("publisher", "string", "The name of the individual or organization that published the message definition.", 0, 1, publisher)); 2056 children.add(new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact)); 2057 children.add(new Property("description", "markdown", "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, description)); 2058 children.add(new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate message definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext)); 2059 children.add(new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the message definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction)); 2060 children.add(new Property("purpose", "markdown", "Explaination of why this message definition is needed and why it has been designed as it has.", 0, 1, purpose)); 2061 children.add(new Property("copyright", "markdown", "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 0, 1, copyright)); 2062 children.add(new Property("base", "Reference(MessageDefinition)", "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base)); 2063 children.add(new Property("parent", "Reference(ActivityDefinition|PlanDefinition)", "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, java.lang.Integer.MAX_VALUE, parent)); 2064 children.add(new Property("replaces", "Reference(MessageDefinition)", "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces)); 2065 children.add(new Property("event", "Coding", "A coded identifier of a supported messaging event.", 0, 1, event)); 2066 children.add(new Property("category", "code", "The impact of the content of the message.", 0, 1, category)); 2067 children.add(new Property("focus", "", "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 0, java.lang.Integer.MAX_VALUE, focus)); 2068 children.add(new Property("responseRequired", "boolean", "Indicates whether a response is required for this message.", 0, 1, responseRequired)); 2069 children.add(new Property("allowedResponse", "", "Indicates what types of messages may be sent as an application-level response to this message.", 0, java.lang.Integer.MAX_VALUE, allowedResponse)); 2070 } 2071 2072 @Override 2073 public Property getNamedProperty(int _hash, String _name, boolean _checkValid) throws FHIRException { 2074 switch (_hash) { 2075 case 116079: /*url*/ return new Property("url", "uri", "An absolute URI that is used to identify this message definition when it is referenced in a specification, model, design or an instance. This SHALL be a URL, SHOULD be globally unique, and SHOULD be an address at which this message definition is (or will be) published. The URL SHOULD include the major version of the message definition. For more information see [Technical and Business Versions](resource.html#versions).", 0, 1, url); 2076 case -1618432855: /*identifier*/ return new Property("identifier", "Identifier", "A formal identifier that is used to identify this message definition when it is represented in other formats, or referenced in a specification, model, design or an instance.", 0, 1, identifier); 2077 case 351608024: /*version*/ return new Property("version", "string", "The identifier that is used to identify this version of the message definition when it is referenced in a specification, model, design or instance. This is an arbitrary value managed by the message definition author and is not expected to be globally unique. For example, it might be a timestamp (e.g. yyyymmdd) if a managed version is not available. There is also no expectation that versions can be placed in a lexicographical sequence.", 0, 1, version); 2078 case 3373707: /*name*/ return new Property("name", "string", "A natural language name identifying the message definition. This name should be usable as an identifier for the module by machine processing applications such as code generation.", 0, 1, name); 2079 case 110371416: /*title*/ return new Property("title", "string", "A short, descriptive, user-friendly title for the message definition.", 0, 1, title); 2080 case -892481550: /*status*/ return new Property("status", "code", "The status of this message definition. Enables tracking the life-cycle of the content.", 0, 1, status); 2081 case -404562712: /*experimental*/ return new Property("experimental", "boolean", "A boolean value to indicate that this message definition is authored for testing purposes (or education/evaluation/marketing), and is not intended to be used for genuine usage.", 0, 1, experimental); 2082 case 3076014: /*date*/ return new Property("date", "dateTime", "The date (and optionally time) when the message definition was published. The date must change if and when the business version changes and it must change if the status code changes. In addition, it should change when the substantive content of the message definition changes.", 0, 1, date); 2083 case 1447404028: /*publisher*/ return new Property("publisher", "string", "The name of the individual or organization that published the message definition.", 0, 1, publisher); 2084 case 951526432: /*contact*/ return new Property("contact", "ContactDetail", "Contact details to assist a user in finding and communicating with the publisher.", 0, java.lang.Integer.MAX_VALUE, contact); 2085 case -1724546052: /*description*/ return new Property("description", "markdown", "A free text natural language description of the message definition from a consumer's perspective.", 0, 1, description); 2086 case -669707736: /*useContext*/ return new Property("useContext", "UsageContext", "The content was developed with a focus and intent of supporting the contexts that are listed. These terms may be used to assist with indexing and searching for appropriate message definition instances.", 0, java.lang.Integer.MAX_VALUE, useContext); 2087 case -507075711: /*jurisdiction*/ return new Property("jurisdiction", "CodeableConcept", "A legal or geographic region in which the message definition is intended to be used.", 0, java.lang.Integer.MAX_VALUE, jurisdiction); 2088 case -220463842: /*purpose*/ return new Property("purpose", "markdown", "Explaination of why this message definition is needed and why it has been designed as it has.", 0, 1, purpose); 2089 case 1522889671: /*copyright*/ return new Property("copyright", "markdown", "A copyright statement relating to the message definition and/or its contents. Copyright statements are generally legal restrictions on the use and publishing of the message definition.", 0, 1, copyright); 2090 case 3016401: /*base*/ return new Property("base", "Reference(MessageDefinition)", "The MessageDefinition that is the basis for the contents of this resource.", 0, 1, base); 2091 case -995424086: /*parent*/ return new Property("parent", "Reference(ActivityDefinition|PlanDefinition)", "Identifies a protocol or workflow that this MessageDefinition represents a step in.", 0, java.lang.Integer.MAX_VALUE, parent); 2092 case -430332865: /*replaces*/ return new Property("replaces", "Reference(MessageDefinition)", "A MessageDefinition that is superseded by this definition.", 0, java.lang.Integer.MAX_VALUE, replaces); 2093 case 96891546: /*event*/ return new Property("event", "Coding", "A coded identifier of a supported messaging event.", 0, 1, event); 2094 case 50511102: /*category*/ return new Property("category", "code", "The impact of the content of the message.", 0, 1, category); 2095 case 97604824: /*focus*/ return new Property("focus", "", "Identifies the resource (or resources) that are being addressed by the event. For example, the Encounter for an admit message or two Account records for a merge.", 0, java.lang.Integer.MAX_VALUE, focus); 2096 case 791597824: /*responseRequired*/ return new Property("responseRequired", "boolean", "Indicates whether a response is required for this message.", 0, 1, responseRequired); 2097 case -1130933751: /*allowedResponse*/ return new Property("allowedResponse", "", "Indicates what types of messages may be sent as an application-level response to this message.", 0, java.lang.Integer.MAX_VALUE, allowedResponse); 2098 default: return super.getNamedProperty(_hash, _name, _checkValid); 2099 } 2100 2101 } 2102 2103 @Override 2104 public Base[] getProperty(int hash, String name, boolean checkValid) throws FHIRException { 2105 switch (hash) { 2106 case 116079: /*url*/ return this.url == null ? new Base[0] : new Base[] {this.url}; // UriType 2107 case -1618432855: /*identifier*/ return this.identifier == null ? new Base[0] : new Base[] {this.identifier}; // Identifier 2108 case 351608024: /*version*/ return this.version == null ? new Base[0] : new Base[] {this.version}; // StringType 2109 case 3373707: /*name*/ return this.name == null ? new Base[0] : new Base[] {this.name}; // StringType 2110 case 110371416: /*title*/ return this.title == null ? new Base[0] : new Base[] {this.title}; // StringType 2111 case -892481550: /*status*/ return this.status == null ? new Base[0] : new Base[] {this.status}; // Enumeration<PublicationStatus> 2112 case -404562712: /*experimental*/ return this.experimental == null ? new Base[0] : new Base[] {this.experimental}; // BooleanType 2113 case 3076014: /*date*/ return this.date == null ? new Base[0] : new Base[] {this.date}; // DateTimeType 2114 case 1447404028: /*publisher*/ return this.publisher == null ? new Base[0] : new Base[] {this.publisher}; // StringType 2115 case 951526432: /*contact*/ return this.contact == null ? new Base[0] : this.contact.toArray(new Base[this.contact.size()]); // ContactDetail 2116 case -1724546052: /*description*/ return this.description == null ? new Base[0] : new Base[] {this.description}; // MarkdownType 2117 case -669707736: /*useContext*/ return this.useContext == null ? new Base[0] : this.useContext.toArray(new Base[this.useContext.size()]); // UsageContext 2118 case -507075711: /*jurisdiction*/ return this.jurisdiction == null ? new Base[0] : this.jurisdiction.toArray(new Base[this.jurisdiction.size()]); // CodeableConcept 2119 case -220463842: /*purpose*/ return this.purpose == null ? new Base[0] : new Base[] {this.purpose}; // MarkdownType 2120 case 1522889671: /*copyright*/ return this.copyright == null ? new Base[0] : new Base[] {this.copyright}; // MarkdownType 2121 case 3016401: /*base*/ return this.base == null ? new Base[0] : new Base[] {this.base}; // Reference 2122 case -995424086: /*parent*/ return this.parent == null ? new Base[0] : this.parent.toArray(new Base[this.parent.size()]); // Reference 2123 case -430332865: /*replaces*/ return this.replaces == null ? new Base[0] : this.replaces.toArray(new Base[this.replaces.size()]); // Reference 2124 case 96891546: /*event*/ return this.event == null ? new Base[0] : new Base[] {this.event}; // Coding 2125 case 50511102: /*category*/ return this.category == null ? new Base[0] : new Base[] {this.category}; // Enumeration<MessageSignificanceCategory> 2126 case 97604824: /*focus*/ return this.focus == null ? new Base[0] : this.focus.toArray(new Base[this.focus.size()]); // MessageDefinitionFocusComponent 2127 case 791597824: /*responseRequired*/ return this.responseRequired == null ? new Base[0] : new Base[] {this.responseRequired}; // BooleanType 2128 case -1130933751: /*allowedResponse*/ return this.allowedResponse == null ? new Base[0] : this.allowedResponse.toArray(new Base[this.allowedResponse.size()]); // MessageDefinitionAllowedResponseComponent 2129 default: return super.getProperty(hash, name, checkValid); 2130 } 2131 2132 } 2133 2134 @Override 2135 public Base setProperty(int hash, String name, Base value) throws FHIRException { 2136 switch (hash) { 2137 case 116079: // url 2138 this.url = castToUri(value); // UriType 2139 return value; 2140 case -1618432855: // identifier 2141 this.identifier = castToIdentifier(value); // Identifier 2142 return value; 2143 case 351608024: // version 2144 this.version = castToString(value); // StringType 2145 return value; 2146 case 3373707: // name 2147 this.name = castToString(value); // StringType 2148 return value; 2149 case 110371416: // title 2150 this.title = castToString(value); // StringType 2151 return value; 2152 case -892481550: // status 2153 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2154 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2155 return value; 2156 case -404562712: // experimental 2157 this.experimental = castToBoolean(value); // BooleanType 2158 return value; 2159 case 3076014: // date 2160 this.date = castToDateTime(value); // DateTimeType 2161 return value; 2162 case 1447404028: // publisher 2163 this.publisher = castToString(value); // StringType 2164 return value; 2165 case 951526432: // contact 2166 this.getContact().add(castToContactDetail(value)); // ContactDetail 2167 return value; 2168 case -1724546052: // description 2169 this.description = castToMarkdown(value); // MarkdownType 2170 return value; 2171 case -669707736: // useContext 2172 this.getUseContext().add(castToUsageContext(value)); // UsageContext 2173 return value; 2174 case -507075711: // jurisdiction 2175 this.getJurisdiction().add(castToCodeableConcept(value)); // CodeableConcept 2176 return value; 2177 case -220463842: // purpose 2178 this.purpose = castToMarkdown(value); // MarkdownType 2179 return value; 2180 case 1522889671: // copyright 2181 this.copyright = castToMarkdown(value); // MarkdownType 2182 return value; 2183 case 3016401: // base 2184 this.base = castToReference(value); // Reference 2185 return value; 2186 case -995424086: // parent 2187 this.getParent().add(castToReference(value)); // Reference 2188 return value; 2189 case -430332865: // replaces 2190 this.getReplaces().add(castToReference(value)); // Reference 2191 return value; 2192 case 96891546: // event 2193 this.event = castToCoding(value); // Coding 2194 return value; 2195 case 50511102: // category 2196 value = new MessageSignificanceCategoryEnumFactory().fromType(castToCode(value)); 2197 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 2198 return value; 2199 case 97604824: // focus 2200 this.getFocus().add((MessageDefinitionFocusComponent) value); // MessageDefinitionFocusComponent 2201 return value; 2202 case 791597824: // responseRequired 2203 this.responseRequired = castToBoolean(value); // BooleanType 2204 return value; 2205 case -1130933751: // allowedResponse 2206 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); // MessageDefinitionAllowedResponseComponent 2207 return value; 2208 default: return super.setProperty(hash, name, value); 2209 } 2210 2211 } 2212 2213 @Override 2214 public Base setProperty(String name, Base value) throws FHIRException { 2215 if (name.equals("url")) { 2216 this.url = castToUri(value); // UriType 2217 } else if (name.equals("identifier")) { 2218 this.identifier = castToIdentifier(value); // Identifier 2219 } else if (name.equals("version")) { 2220 this.version = castToString(value); // StringType 2221 } else if (name.equals("name")) { 2222 this.name = castToString(value); // StringType 2223 } else if (name.equals("title")) { 2224 this.title = castToString(value); // StringType 2225 } else if (name.equals("status")) { 2226 value = new PublicationStatusEnumFactory().fromType(castToCode(value)); 2227 this.status = (Enumeration) value; // Enumeration<PublicationStatus> 2228 } else if (name.equals("experimental")) { 2229 this.experimental = castToBoolean(value); // BooleanType 2230 } else if (name.equals("date")) { 2231 this.date = castToDateTime(value); // DateTimeType 2232 } else if (name.equals("publisher")) { 2233 this.publisher = castToString(value); // StringType 2234 } else if (name.equals("contact")) { 2235 this.getContact().add(castToContactDetail(value)); 2236 } else if (name.equals("description")) { 2237 this.description = castToMarkdown(value); // MarkdownType 2238 } else if (name.equals("useContext")) { 2239 this.getUseContext().add(castToUsageContext(value)); 2240 } else if (name.equals("jurisdiction")) { 2241 this.getJurisdiction().add(castToCodeableConcept(value)); 2242 } else if (name.equals("purpose")) { 2243 this.purpose = castToMarkdown(value); // MarkdownType 2244 } else if (name.equals("copyright")) { 2245 this.copyright = castToMarkdown(value); // MarkdownType 2246 } else if (name.equals("base")) { 2247 this.base = castToReference(value); // Reference 2248 } else if (name.equals("parent")) { 2249 this.getParent().add(castToReference(value)); 2250 } else if (name.equals("replaces")) { 2251 this.getReplaces().add(castToReference(value)); 2252 } else if (name.equals("event")) { 2253 this.event = castToCoding(value); // Coding 2254 } else if (name.equals("category")) { 2255 value = new MessageSignificanceCategoryEnumFactory().fromType(castToCode(value)); 2256 this.category = (Enumeration) value; // Enumeration<MessageSignificanceCategory> 2257 } else if (name.equals("focus")) { 2258 this.getFocus().add((MessageDefinitionFocusComponent) value); 2259 } else if (name.equals("responseRequired")) { 2260 this.responseRequired = castToBoolean(value); // BooleanType 2261 } else if (name.equals("allowedResponse")) { 2262 this.getAllowedResponse().add((MessageDefinitionAllowedResponseComponent) value); 2263 } else 2264 return super.setProperty(name, value); 2265 return value; 2266 } 2267 2268 @Override 2269 public Base makeProperty(int hash, String name) throws FHIRException { 2270 switch (hash) { 2271 case 116079: return getUrlElement(); 2272 case -1618432855: return getIdentifier(); 2273 case 351608024: return getVersionElement(); 2274 case 3373707: return getNameElement(); 2275 case 110371416: return getTitleElement(); 2276 case -892481550: return getStatusElement(); 2277 case -404562712: return getExperimentalElement(); 2278 case 3076014: return getDateElement(); 2279 case 1447404028: return getPublisherElement(); 2280 case 951526432: return addContact(); 2281 case -1724546052: return getDescriptionElement(); 2282 case -669707736: return addUseContext(); 2283 case -507075711: return addJurisdiction(); 2284 case -220463842: return getPurposeElement(); 2285 case 1522889671: return getCopyrightElement(); 2286 case 3016401: return getBase(); 2287 case -995424086: return addParent(); 2288 case -430332865: return addReplaces(); 2289 case 96891546: return getEvent(); 2290 case 50511102: return getCategoryElement(); 2291 case 97604824: return addFocus(); 2292 case 791597824: return getResponseRequiredElement(); 2293 case -1130933751: return addAllowedResponse(); 2294 default: return super.makeProperty(hash, name); 2295 } 2296 2297 } 2298 2299 @Override 2300 public String[] getTypesForProperty(int hash, String name) throws FHIRException { 2301 switch (hash) { 2302 case 116079: /*url*/ return new String[] {"uri"}; 2303 case -1618432855: /*identifier*/ return new String[] {"Identifier"}; 2304 case 351608024: /*version*/ return new String[] {"string"}; 2305 case 3373707: /*name*/ return new String[] {"string"}; 2306 case 110371416: /*title*/ return new String[] {"string"}; 2307 case -892481550: /*status*/ return new String[] {"code"}; 2308 case -404562712: /*experimental*/ return new String[] {"boolean"}; 2309 case 3076014: /*date*/ return new String[] {"dateTime"}; 2310 case 1447404028: /*publisher*/ return new String[] {"string"}; 2311 case 951526432: /*contact*/ return new String[] {"ContactDetail"}; 2312 case -1724546052: /*description*/ return new String[] {"markdown"}; 2313 case -669707736: /*useContext*/ return new String[] {"UsageContext"}; 2314 case -507075711: /*jurisdiction*/ return new String[] {"CodeableConcept"}; 2315 case -220463842: /*purpose*/ return new String[] {"markdown"}; 2316 case 1522889671: /*copyright*/ return new String[] {"markdown"}; 2317 case 3016401: /*base*/ return new String[] {"Reference"}; 2318 case -995424086: /*parent*/ return new String[] {"Reference"}; 2319 case -430332865: /*replaces*/ return new String[] {"Reference"}; 2320 case 96891546: /*event*/ return new String[] {"Coding"}; 2321 case 50511102: /*category*/ return new String[] {"code"}; 2322 case 97604824: /*focus*/ return new String[] {}; 2323 case 791597824: /*responseRequired*/ return new String[] {"boolean"}; 2324 case -1130933751: /*allowedResponse*/ return new String[] {}; 2325 default: return super.getTypesForProperty(hash, name); 2326 } 2327 2328 } 2329 2330 @Override 2331 public Base addChild(String name) throws FHIRException { 2332 if (name.equals("url")) { 2333 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.url"); 2334 } 2335 else if (name.equals("identifier")) { 2336 this.identifier = new Identifier(); 2337 return this.identifier; 2338 } 2339 else if (name.equals("version")) { 2340 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.version"); 2341 } 2342 else if (name.equals("name")) { 2343 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.name"); 2344 } 2345 else if (name.equals("title")) { 2346 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.title"); 2347 } 2348 else if (name.equals("status")) { 2349 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.status"); 2350 } 2351 else if (name.equals("experimental")) { 2352 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.experimental"); 2353 } 2354 else if (name.equals("date")) { 2355 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.date"); 2356 } 2357 else if (name.equals("publisher")) { 2358 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.publisher"); 2359 } 2360 else if (name.equals("contact")) { 2361 return addContact(); 2362 } 2363 else if (name.equals("description")) { 2364 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.description"); 2365 } 2366 else if (name.equals("useContext")) { 2367 return addUseContext(); 2368 } 2369 else if (name.equals("jurisdiction")) { 2370 return addJurisdiction(); 2371 } 2372 else if (name.equals("purpose")) { 2373 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.purpose"); 2374 } 2375 else if (name.equals("copyright")) { 2376 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.copyright"); 2377 } 2378 else if (name.equals("base")) { 2379 this.base = new Reference(); 2380 return this.base; 2381 } 2382 else if (name.equals("parent")) { 2383 return addParent(); 2384 } 2385 else if (name.equals("replaces")) { 2386 return addReplaces(); 2387 } 2388 else if (name.equals("event")) { 2389 this.event = new Coding(); 2390 return this.event; 2391 } 2392 else if (name.equals("category")) { 2393 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.category"); 2394 } 2395 else if (name.equals("focus")) { 2396 return addFocus(); 2397 } 2398 else if (name.equals("responseRequired")) { 2399 throw new FHIRException("Cannot call addChild on a singleton property MessageDefinition.responseRequired"); 2400 } 2401 else if (name.equals("allowedResponse")) { 2402 return addAllowedResponse(); 2403 } 2404 else 2405 return super.addChild(name); 2406 } 2407 2408 public String fhirType() { 2409 return "MessageDefinition"; 2410 2411 } 2412 2413 public MessageDefinition copy() { 2414 MessageDefinition dst = new MessageDefinition(); 2415 copyValues(dst); 2416 dst.url = url == null ? null : url.copy(); 2417 dst.identifier = identifier == null ? null : identifier.copy(); 2418 dst.version = version == null ? null : version.copy(); 2419 dst.name = name == null ? null : name.copy(); 2420 dst.title = title == null ? null : title.copy(); 2421 dst.status = status == null ? null : status.copy(); 2422 dst.experimental = experimental == null ? null : experimental.copy(); 2423 dst.date = date == null ? null : date.copy(); 2424 dst.publisher = publisher == null ? null : publisher.copy(); 2425 if (contact != null) { 2426 dst.contact = new ArrayList<ContactDetail>(); 2427 for (ContactDetail i : contact) 2428 dst.contact.add(i.copy()); 2429 }; 2430 dst.description = description == null ? null : description.copy(); 2431 if (useContext != null) { 2432 dst.useContext = new ArrayList<UsageContext>(); 2433 for (UsageContext i : useContext) 2434 dst.useContext.add(i.copy()); 2435 }; 2436 if (jurisdiction != null) { 2437 dst.jurisdiction = new ArrayList<CodeableConcept>(); 2438 for (CodeableConcept i : jurisdiction) 2439 dst.jurisdiction.add(i.copy()); 2440 }; 2441 dst.purpose = purpose == null ? null : purpose.copy(); 2442 dst.copyright = copyright == null ? null : copyright.copy(); 2443 dst.base = base == null ? null : base.copy(); 2444 if (parent != null) { 2445 dst.parent = new ArrayList<Reference>(); 2446 for (Reference i : parent) 2447 dst.parent.add(i.copy()); 2448 }; 2449 if (replaces != null) { 2450 dst.replaces = new ArrayList<Reference>(); 2451 for (Reference i : replaces) 2452 dst.replaces.add(i.copy()); 2453 }; 2454 dst.event = event == null ? null : event.copy(); 2455 dst.category = category == null ? null : category.copy(); 2456 if (focus != null) { 2457 dst.focus = new ArrayList<MessageDefinitionFocusComponent>(); 2458 for (MessageDefinitionFocusComponent i : focus) 2459 dst.focus.add(i.copy()); 2460 }; 2461 dst.responseRequired = responseRequired == null ? null : responseRequired.copy(); 2462 if (allowedResponse != null) { 2463 dst.allowedResponse = new ArrayList<MessageDefinitionAllowedResponseComponent>(); 2464 for (MessageDefinitionAllowedResponseComponent i : allowedResponse) 2465 dst.allowedResponse.add(i.copy()); 2466 }; 2467 return dst; 2468 } 2469 2470 protected MessageDefinition typedCopy() { 2471 return copy(); 2472 } 2473 2474 @Override 2475 public boolean equalsDeep(Base other_) { 2476 if (!super.equalsDeep(other_)) 2477 return false; 2478 if (!(other_ instanceof MessageDefinition)) 2479 return false; 2480 MessageDefinition o = (MessageDefinition) other_; 2481 return compareDeep(identifier, o.identifier, true) && compareDeep(purpose, o.purpose, true) && compareDeep(copyright, o.copyright, true) 2482 && compareDeep(base, o.base, true) && compareDeep(parent, o.parent, true) && compareDeep(replaces, o.replaces, true) 2483 && compareDeep(event, o.event, true) && compareDeep(category, o.category, true) && compareDeep(focus, o.focus, true) 2484 && compareDeep(responseRequired, o.responseRequired, true) && compareDeep(allowedResponse, o.allowedResponse, true) 2485 ; 2486 } 2487 2488 @Override 2489 public boolean equalsShallow(Base other_) { 2490 if (!super.equalsShallow(other_)) 2491 return false; 2492 if (!(other_ instanceof MessageDefinition)) 2493 return false; 2494 MessageDefinition o = (MessageDefinition) other_; 2495 return compareValues(purpose, o.purpose, true) && compareValues(copyright, o.copyright, true) && compareValues(category, o.category, true) 2496 && compareValues(responseRequired, o.responseRequired, true); 2497 } 2498 2499 public boolean isEmpty() { 2500 return super.isEmpty() && ca.uhn.fhir.util.ElementUtil.isEmpty(identifier, purpose, copyright 2501 , base, parent, replaces, event, category, focus, responseRequired, allowedResponse 2502 ); 2503 } 2504 2505 @Override 2506 public ResourceType getResourceType() { 2507 return ResourceType.MessageDefinition; 2508 } 2509 2510 /** 2511 * Search parameter: <b>date</b> 2512 * <p> 2513 * Description: <b>The message definition publication date</b><br> 2514 * Type: <b>date</b><br> 2515 * Path: <b>MessageDefinition.date</b><br> 2516 * </p> 2517 */ 2518 @SearchParamDefinition(name="date", path="MessageDefinition.date", description="The message definition publication date", type="date" ) 2519 public static final String SP_DATE = "date"; 2520 /** 2521 * <b>Fluent Client</b> search parameter constant for <b>date</b> 2522 * <p> 2523 * Description: <b>The message definition publication date</b><br> 2524 * Type: <b>date</b><br> 2525 * Path: <b>MessageDefinition.date</b><br> 2526 * </p> 2527 */ 2528 public static final ca.uhn.fhir.rest.gclient.DateClientParam DATE = new ca.uhn.fhir.rest.gclient.DateClientParam(SP_DATE); 2529 2530 /** 2531 * Search parameter: <b>identifier</b> 2532 * <p> 2533 * Description: <b>External identifier for the message definition</b><br> 2534 * Type: <b>token</b><br> 2535 * Path: <b>MessageDefinition.identifier</b><br> 2536 * </p> 2537 */ 2538 @SearchParamDefinition(name="identifier", path="MessageDefinition.identifier", description="External identifier for the message definition", type="token" ) 2539 public static final String SP_IDENTIFIER = "identifier"; 2540 /** 2541 * <b>Fluent Client</b> search parameter constant for <b>identifier</b> 2542 * <p> 2543 * Description: <b>External identifier for the message definition</b><br> 2544 * Type: <b>token</b><br> 2545 * Path: <b>MessageDefinition.identifier</b><br> 2546 * </p> 2547 */ 2548 public static final ca.uhn.fhir.rest.gclient.TokenClientParam IDENTIFIER = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_IDENTIFIER); 2549 2550 /** 2551 * Search parameter: <b>jurisdiction</b> 2552 * <p> 2553 * Description: <b>Intended jurisdiction for the message definition</b><br> 2554 * Type: <b>token</b><br> 2555 * Path: <b>MessageDefinition.jurisdiction</b><br> 2556 * </p> 2557 */ 2558 @SearchParamDefinition(name="jurisdiction", path="MessageDefinition.jurisdiction", description="Intended jurisdiction for the message definition", type="token" ) 2559 public static final String SP_JURISDICTION = "jurisdiction"; 2560 /** 2561 * <b>Fluent Client</b> search parameter constant for <b>jurisdiction</b> 2562 * <p> 2563 * Description: <b>Intended jurisdiction for the message definition</b><br> 2564 * Type: <b>token</b><br> 2565 * Path: <b>MessageDefinition.jurisdiction</b><br> 2566 * </p> 2567 */ 2568 public static final ca.uhn.fhir.rest.gclient.TokenClientParam JURISDICTION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_JURISDICTION); 2569 2570 /** 2571 * Search parameter: <b>description</b> 2572 * <p> 2573 * Description: <b>The description of the message definition</b><br> 2574 * Type: <b>string</b><br> 2575 * Path: <b>MessageDefinition.description</b><br> 2576 * </p> 2577 */ 2578 @SearchParamDefinition(name="description", path="MessageDefinition.description", description="The description of the message definition", type="string" ) 2579 public static final String SP_DESCRIPTION = "description"; 2580 /** 2581 * <b>Fluent Client</b> search parameter constant for <b>description</b> 2582 * <p> 2583 * Description: <b>The description of the message definition</b><br> 2584 * Type: <b>string</b><br> 2585 * Path: <b>MessageDefinition.description</b><br> 2586 * </p> 2587 */ 2588 public static final ca.uhn.fhir.rest.gclient.StringClientParam DESCRIPTION = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_DESCRIPTION); 2589 2590 /** 2591 * Search parameter: <b>focus</b> 2592 * <p> 2593 * Description: <b>A resource that is a permitted focus of the message</b><br> 2594 * Type: <b>token</b><br> 2595 * Path: <b>MessageDefinition.focus.code</b><br> 2596 * </p> 2597 */ 2598 @SearchParamDefinition(name="focus", path="MessageDefinition.focus.code", description="A resource that is a permitted focus of the message", type="token" ) 2599 public static final String SP_FOCUS = "focus"; 2600 /** 2601 * <b>Fluent Client</b> search parameter constant for <b>focus</b> 2602 * <p> 2603 * Description: <b>A resource that is a permitted focus of the message</b><br> 2604 * Type: <b>token</b><br> 2605 * Path: <b>MessageDefinition.focus.code</b><br> 2606 * </p> 2607 */ 2608 public static final ca.uhn.fhir.rest.gclient.TokenClientParam FOCUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_FOCUS); 2609 2610 /** 2611 * Search parameter: <b>title</b> 2612 * <p> 2613 * Description: <b>The human-friendly name of the message definition</b><br> 2614 * Type: <b>string</b><br> 2615 * Path: <b>MessageDefinition.title</b><br> 2616 * </p> 2617 */ 2618 @SearchParamDefinition(name="title", path="MessageDefinition.title", description="The human-friendly name of the message definition", type="string" ) 2619 public static final String SP_TITLE = "title"; 2620 /** 2621 * <b>Fluent Client</b> search parameter constant for <b>title</b> 2622 * <p> 2623 * Description: <b>The human-friendly name of the message definition</b><br> 2624 * Type: <b>string</b><br> 2625 * Path: <b>MessageDefinition.title</b><br> 2626 * </p> 2627 */ 2628 public static final ca.uhn.fhir.rest.gclient.StringClientParam TITLE = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_TITLE); 2629 2630 /** 2631 * Search parameter: <b>version</b> 2632 * <p> 2633 * Description: <b>The business version of the message definition</b><br> 2634 * Type: <b>token</b><br> 2635 * Path: <b>MessageDefinition.version</b><br> 2636 * </p> 2637 */ 2638 @SearchParamDefinition(name="version", path="MessageDefinition.version", description="The business version of the message definition", type="token" ) 2639 public static final String SP_VERSION = "version"; 2640 /** 2641 * <b>Fluent Client</b> search parameter constant for <b>version</b> 2642 * <p> 2643 * Description: <b>The business version of the message definition</b><br> 2644 * Type: <b>token</b><br> 2645 * Path: <b>MessageDefinition.version</b><br> 2646 * </p> 2647 */ 2648 public static final ca.uhn.fhir.rest.gclient.TokenClientParam VERSION = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_VERSION); 2649 2650 /** 2651 * Search parameter: <b>url</b> 2652 * <p> 2653 * Description: <b>The uri that identifies the message definition</b><br> 2654 * Type: <b>uri</b><br> 2655 * Path: <b>MessageDefinition.url</b><br> 2656 * </p> 2657 */ 2658 @SearchParamDefinition(name="url", path="MessageDefinition.url", description="The uri that identifies the message definition", type="uri" ) 2659 public static final String SP_URL = "url"; 2660 /** 2661 * <b>Fluent Client</b> search parameter constant for <b>url</b> 2662 * <p> 2663 * Description: <b>The uri that identifies the message definition</b><br> 2664 * Type: <b>uri</b><br> 2665 * Path: <b>MessageDefinition.url</b><br> 2666 * </p> 2667 */ 2668 public static final ca.uhn.fhir.rest.gclient.UriClientParam URL = new ca.uhn.fhir.rest.gclient.UriClientParam(SP_URL); 2669 2670 /** 2671 * Search parameter: <b>name</b> 2672 * <p> 2673 * Description: <b>Computationally friendly name of the message definition</b><br> 2674 * Type: <b>string</b><br> 2675 * Path: <b>MessageDefinition.name</b><br> 2676 * </p> 2677 */ 2678 @SearchParamDefinition(name="name", path="MessageDefinition.name", description="Computationally friendly name of the message definition", type="string" ) 2679 public static final String SP_NAME = "name"; 2680 /** 2681 * <b>Fluent Client</b> search parameter constant for <b>name</b> 2682 * <p> 2683 * Description: <b>Computationally friendly name of the message definition</b><br> 2684 * Type: <b>string</b><br> 2685 * Path: <b>MessageDefinition.name</b><br> 2686 * </p> 2687 */ 2688 public static final ca.uhn.fhir.rest.gclient.StringClientParam NAME = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_NAME); 2689 2690 /** 2691 * Search parameter: <b>publisher</b> 2692 * <p> 2693 * Description: <b>Name of the publisher of the message definition</b><br> 2694 * Type: <b>string</b><br> 2695 * Path: <b>MessageDefinition.publisher</b><br> 2696 * </p> 2697 */ 2698 @SearchParamDefinition(name="publisher", path="MessageDefinition.publisher", description="Name of the publisher of the message definition", type="string" ) 2699 public static final String SP_PUBLISHER = "publisher"; 2700 /** 2701 * <b>Fluent Client</b> search parameter constant for <b>publisher</b> 2702 * <p> 2703 * Description: <b>Name of the publisher of the message definition</b><br> 2704 * Type: <b>string</b><br> 2705 * Path: <b>MessageDefinition.publisher</b><br> 2706 * </p> 2707 */ 2708 public static final ca.uhn.fhir.rest.gclient.StringClientParam PUBLISHER = new ca.uhn.fhir.rest.gclient.StringClientParam(SP_PUBLISHER); 2709 2710 /** 2711 * Search parameter: <b>event</b> 2712 * <p> 2713 * Description: <b>The event that triggers the message</b><br> 2714 * Type: <b>token</b><br> 2715 * Path: <b>MessageDefinition.event</b><br> 2716 * </p> 2717 */ 2718 @SearchParamDefinition(name="event", path="MessageDefinition.event", description="The event that triggers the message", type="token" ) 2719 public static final String SP_EVENT = "event"; 2720 /** 2721 * <b>Fluent Client</b> search parameter constant for <b>event</b> 2722 * <p> 2723 * Description: <b>The event that triggers the message</b><br> 2724 * Type: <b>token</b><br> 2725 * Path: <b>MessageDefinition.event</b><br> 2726 * </p> 2727 */ 2728 public static final ca.uhn.fhir.rest.gclient.TokenClientParam EVENT = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_EVENT); 2729 2730 /** 2731 * Search parameter: <b>category</b> 2732 * <p> 2733 * Description: <b>The behavior associated with the message</b><br> 2734 * Type: <b>token</b><br> 2735 * Path: <b>MessageDefinition.category</b><br> 2736 * </p> 2737 */ 2738 @SearchParamDefinition(name="category", path="MessageDefinition.category", description="The behavior associated with the message", type="token" ) 2739 public static final String SP_CATEGORY = "category"; 2740 /** 2741 * <b>Fluent Client</b> search parameter constant for <b>category</b> 2742 * <p> 2743 * Description: <b>The behavior associated with the message</b><br> 2744 * Type: <b>token</b><br> 2745 * Path: <b>MessageDefinition.category</b><br> 2746 * </p> 2747 */ 2748 public static final ca.uhn.fhir.rest.gclient.TokenClientParam CATEGORY = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_CATEGORY); 2749 2750 /** 2751 * Search parameter: <b>status</b> 2752 * <p> 2753 * Description: <b>The current status of the message definition</b><br> 2754 * Type: <b>token</b><br> 2755 * Path: <b>MessageDefinition.status</b><br> 2756 * </p> 2757 */ 2758 @SearchParamDefinition(name="status", path="MessageDefinition.status", description="The current status of the message definition", type="token" ) 2759 public static final String SP_STATUS = "status"; 2760 /** 2761 * <b>Fluent Client</b> search parameter constant for <b>status</b> 2762 * <p> 2763 * Description: <b>The current status of the message definition</b><br> 2764 * Type: <b>token</b><br> 2765 * Path: <b>MessageDefinition.status</b><br> 2766 * </p> 2767 */ 2768 public static final ca.uhn.fhir.rest.gclient.TokenClientParam STATUS = new ca.uhn.fhir.rest.gclient.TokenClientParam(SP_STATUS); 2769 2770 2771}